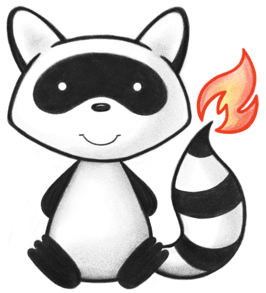
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A sample to be used for analysis. 048 */ 049@ResourceDef(name = "Specimen", profile = "http://hl7.org/fhir/Profile/Specimen") 050public class Specimen extends DomainResource { 051 052 public enum SpecimenStatus { 053 /** 054 * The physical specimen is present and in good condition. 055 */ 056 AVAILABLE, 057 /** 058 * There is no physical specimen because it is either lost, destroyed or 059 * consumed. 060 */ 061 UNAVAILABLE, 062 /** 063 * The specimen cannot be used because of a quality issue such as a broken 064 * container, contamination, or too old. 065 */ 066 UNSATISFACTORY, 067 /** 068 * The specimen was entered in error and therefore nullified. 069 */ 070 ENTEREDINERROR, 071 /** 072 * added to help the parsers 073 */ 074 NULL; 075 076 public static SpecimenStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("available".equals(codeString)) 080 return AVAILABLE; 081 if ("unavailable".equals(codeString)) 082 return UNAVAILABLE; 083 if ("unsatisfactory".equals(codeString)) 084 return UNSATISFACTORY; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 throw new FHIRException("Unknown SpecimenStatus code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case AVAILABLE: 093 return "available"; 094 case UNAVAILABLE: 095 return "unavailable"; 096 case UNSATISFACTORY: 097 return "unsatisfactory"; 098 case ENTEREDINERROR: 099 return "entered-in-error"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case AVAILABLE: 110 return "http://hl7.org/fhir/specimen-status"; 111 case UNAVAILABLE: 112 return "http://hl7.org/fhir/specimen-status"; 113 case UNSATISFACTORY: 114 return "http://hl7.org/fhir/specimen-status"; 115 case ENTEREDINERROR: 116 return "http://hl7.org/fhir/specimen-status"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case AVAILABLE: 127 return "The physical specimen is present and in good condition."; 128 case UNAVAILABLE: 129 return "There is no physical specimen because it is either lost, destroyed or consumed."; 130 case UNSATISFACTORY: 131 return "The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old."; 132 case ENTEREDINERROR: 133 return "The specimen was entered in error and therefore nullified."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case AVAILABLE: 144 return "Available"; 145 case UNAVAILABLE: 146 return "Unavailable"; 147 case UNSATISFACTORY: 148 return "Unsatisfactory"; 149 case ENTEREDINERROR: 150 return "Entered-in-error"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class SpecimenStatusEnumFactory implements EnumFactory<SpecimenStatus> { 160 public SpecimenStatus fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("available".equals(codeString)) 165 return SpecimenStatus.AVAILABLE; 166 if ("unavailable".equals(codeString)) 167 return SpecimenStatus.UNAVAILABLE; 168 if ("unsatisfactory".equals(codeString)) 169 return SpecimenStatus.UNSATISFACTORY; 170 if ("entered-in-error".equals(codeString)) 171 return SpecimenStatus.ENTEREDINERROR; 172 throw new IllegalArgumentException("Unknown SpecimenStatus code '" + codeString + "'"); 173 } 174 175 public Enumeration<SpecimenStatus> fromType(Base code) throws FHIRException { 176 if (code == null || code.isEmpty()) 177 return null; 178 String codeString = ((PrimitiveType) code).asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("available".equals(codeString)) 182 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.AVAILABLE); 183 if ("unavailable".equals(codeString)) 184 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNAVAILABLE); 185 if ("unsatisfactory".equals(codeString)) 186 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNSATISFACTORY); 187 if ("entered-in-error".equals(codeString)) 188 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.ENTEREDINERROR); 189 throw new FHIRException("Unknown SpecimenStatus code '" + codeString + "'"); 190 } 191 192 public String toCode(SpecimenStatus code) 193 { 194 if (code == SpecimenStatus.NULL) 195 return null; 196 if (code == SpecimenStatus.AVAILABLE) 197 return "available"; 198 if (code == SpecimenStatus.UNAVAILABLE) 199 return "unavailable"; 200 if (code == SpecimenStatus.UNSATISFACTORY) 201 return "unsatisfactory"; 202 if (code == SpecimenStatus.ENTEREDINERROR) 203 return "entered-in-error"; 204 return "?"; 205 } 206 } 207 208 @Block() 209 public static class SpecimenCollectionComponent extends BackboneElement implements IBaseBackboneElement { 210 /** 211 * Person who collected the specimen. 212 */ 213 @Child(name = "collector", type = { 214 Practitioner.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 215 @Description(shortDefinition = "Who collected the specimen", formalDefinition = "Person who collected the specimen.") 216 protected Reference collector; 217 218 /** 219 * The actual object that is the target of the reference (Person who collected 220 * the specimen.) 221 */ 222 protected Practitioner collectorTarget; 223 224 /** 225 * To communicate any details or issues encountered during the specimen 226 * collection procedure. 227 */ 228 @Child(name = "comment", type = { 229 StringType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 230 @Description(shortDefinition = "Collector comments", formalDefinition = "To communicate any details or issues encountered during the specimen collection procedure.") 231 protected List<StringType> comment; 232 233 /** 234 * Time when specimen was collected from subject - the physiologically relevant 235 * time. 236 */ 237 @Child(name = "collected", type = { DateTimeType.class, 238 Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 239 @Description(shortDefinition = "Collection time", formalDefinition = "Time when specimen was collected from subject - the physiologically relevant time.") 240 protected Type collected; 241 242 /** 243 * The quantity of specimen collected; for instance the volume of a blood 244 * sample, or the physical measurement of an anatomic pathology sample. 245 */ 246 @Child(name = "quantity", type = { 247 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 248 @Description(shortDefinition = "The quantity of specimen collected", formalDefinition = "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.") 249 protected SimpleQuantity quantity; 250 251 /** 252 * A coded value specifying the technique that is used to perform the procedure. 253 */ 254 @Child(name = "method", type = { 255 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 256 @Description(shortDefinition = "Technique used to perform collection", formalDefinition = "A coded value specifying the technique that is used to perform the procedure.") 257 protected CodeableConcept method; 258 259 /** 260 * Anatomical location from which the specimen was collected (if subject is a 261 * patient). This is the target site. This element is not used for environmental 262 * specimens. 263 */ 264 @Child(name = "bodySite", type = { 265 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 266 @Description(shortDefinition = "Anatomical collection site", formalDefinition = "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.") 267 protected CodeableConcept bodySite; 268 269 private static final long serialVersionUID = -1418734687L; 270 271 /* 272 * Constructor 273 */ 274 public SpecimenCollectionComponent() { 275 super(); 276 } 277 278 /** 279 * @return {@link #collector} (Person who collected the specimen.) 280 */ 281 public Reference getCollector() { 282 if (this.collector == null) 283 if (Configuration.errorOnAutoCreate()) 284 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 285 else if (Configuration.doAutoCreate()) 286 this.collector = new Reference(); // cc 287 return this.collector; 288 } 289 290 public boolean hasCollector() { 291 return this.collector != null && !this.collector.isEmpty(); 292 } 293 294 /** 295 * @param value {@link #collector} (Person who collected the specimen.) 296 */ 297 public SpecimenCollectionComponent setCollector(Reference value) { 298 this.collector = value; 299 return this; 300 } 301 302 /** 303 * @return {@link #collector} The actual object that is the target of the 304 * reference. The reference library doesn't populate this, but you can 305 * use it to hold the resource if you resolve it. (Person who collected 306 * the specimen.) 307 */ 308 public Practitioner getCollectorTarget() { 309 if (this.collectorTarget == null) 310 if (Configuration.errorOnAutoCreate()) 311 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 312 else if (Configuration.doAutoCreate()) 313 this.collectorTarget = new Practitioner(); // aa 314 return this.collectorTarget; 315 } 316 317 /** 318 * @param value {@link #collector} The actual object that is the target of the 319 * reference. The reference library doesn't use these, but you can 320 * use it to hold the resource if you resolve it. (Person who 321 * collected the specimen.) 322 */ 323 public SpecimenCollectionComponent setCollectorTarget(Practitioner value) { 324 this.collectorTarget = value; 325 return this; 326 } 327 328 /** 329 * @return {@link #comment} (To communicate any details or issues encountered 330 * during the specimen collection procedure.) 331 */ 332 public List<StringType> getComment() { 333 if (this.comment == null) 334 this.comment = new ArrayList<StringType>(); 335 return this.comment; 336 } 337 338 public boolean hasComment() { 339 if (this.comment == null) 340 return false; 341 for (StringType item : this.comment) 342 if (!item.isEmpty()) 343 return true; 344 return false; 345 } 346 347 /** 348 * @return {@link #comment} (To communicate any details or issues encountered 349 * during the specimen collection procedure.) 350 */ 351 // syntactic sugar 352 public StringType addCommentElement() {// 2 353 StringType t = new StringType(); 354 if (this.comment == null) 355 this.comment = new ArrayList<StringType>(); 356 this.comment.add(t); 357 return t; 358 } 359 360 /** 361 * @param value {@link #comment} (To communicate any details or issues 362 * encountered during the specimen collection procedure.) 363 */ 364 public SpecimenCollectionComponent addComment(String value) { // 1 365 StringType t = new StringType(); 366 t.setValue(value); 367 if (this.comment == null) 368 this.comment = new ArrayList<StringType>(); 369 this.comment.add(t); 370 return this; 371 } 372 373 /** 374 * @param value {@link #comment} (To communicate any details or issues 375 * encountered during the specimen collection procedure.) 376 */ 377 public boolean hasComment(String value) { 378 if (this.comment == null) 379 return false; 380 for (StringType v : this.comment) 381 if (v.equals(value)) // string 382 return true; 383 return false; 384 } 385 386 /** 387 * @return {@link #collected} (Time when specimen was collected from subject - 388 * the physiologically relevant time.) 389 */ 390 public Type getCollected() { 391 return this.collected; 392 } 393 394 /** 395 * @return {@link #collected} (Time when specimen was collected from subject - 396 * the physiologically relevant time.) 397 */ 398 public DateTimeType getCollectedDateTimeType() throws FHIRException { 399 if (!(this.collected instanceof DateTimeType)) 400 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 401 + this.collected.getClass().getName() + " was encountered"); 402 return (DateTimeType) this.collected; 403 } 404 405 public boolean hasCollectedDateTimeType() { 406 return this.collected instanceof DateTimeType; 407 } 408 409 /** 410 * @return {@link #collected} (Time when specimen was collected from subject - 411 * the physiologically relevant time.) 412 */ 413 public Period getCollectedPeriod() throws FHIRException { 414 if (!(this.collected instanceof Period)) 415 throw new FHIRException("Type mismatch: the type Period was expected, but " 416 + this.collected.getClass().getName() + " was encountered"); 417 return (Period) this.collected; 418 } 419 420 public boolean hasCollectedPeriod() { 421 return this.collected instanceof Period; 422 } 423 424 public boolean hasCollected() { 425 return this.collected != null && !this.collected.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #collected} (Time when specimen was collected from 430 * subject - the physiologically relevant time.) 431 */ 432 public SpecimenCollectionComponent setCollected(Type value) { 433 this.collected = value; 434 return this; 435 } 436 437 /** 438 * @return {@link #quantity} (The quantity of specimen collected; for instance 439 * the volume of a blood sample, or the physical measurement of an 440 * anatomic pathology sample.) 441 */ 442 public SimpleQuantity getQuantity() { 443 if (this.quantity == null) 444 if (Configuration.errorOnAutoCreate()) 445 throw new Error("Attempt to auto-create SpecimenCollectionComponent.quantity"); 446 else if (Configuration.doAutoCreate()) 447 this.quantity = new SimpleQuantity(); // cc 448 return this.quantity; 449 } 450 451 public boolean hasQuantity() { 452 return this.quantity != null && !this.quantity.isEmpty(); 453 } 454 455 /** 456 * @param value {@link #quantity} (The quantity of specimen collected; for 457 * instance the volume of a blood sample, or the physical 458 * measurement of an anatomic pathology sample.) 459 */ 460 public SpecimenCollectionComponent setQuantity(SimpleQuantity value) { 461 this.quantity = value; 462 return this; 463 } 464 465 /** 466 * @return {@link #method} (A coded value specifying the technique that is used 467 * to perform the procedure.) 468 */ 469 public CodeableConcept getMethod() { 470 if (this.method == null) 471 if (Configuration.errorOnAutoCreate()) 472 throw new Error("Attempt to auto-create SpecimenCollectionComponent.method"); 473 else if (Configuration.doAutoCreate()) 474 this.method = new CodeableConcept(); // cc 475 return this.method; 476 } 477 478 public boolean hasMethod() { 479 return this.method != null && !this.method.isEmpty(); 480 } 481 482 /** 483 * @param value {@link #method} (A coded value specifying the technique that is 484 * used to perform the procedure.) 485 */ 486 public SpecimenCollectionComponent setMethod(CodeableConcept value) { 487 this.method = value; 488 return this; 489 } 490 491 /** 492 * @return {@link #bodySite} (Anatomical location from which the specimen was 493 * collected (if subject is a patient). This is the target site. This 494 * element is not used for environmental specimens.) 495 */ 496 public CodeableConcept getBodySite() { 497 if (this.bodySite == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create SpecimenCollectionComponent.bodySite"); 500 else if (Configuration.doAutoCreate()) 501 this.bodySite = new CodeableConcept(); // cc 502 return this.bodySite; 503 } 504 505 public boolean hasBodySite() { 506 return this.bodySite != null && !this.bodySite.isEmpty(); 507 } 508 509 /** 510 * @param value {@link #bodySite} (Anatomical location from which the specimen 511 * was collected (if subject is a patient). This is the target 512 * site. This element is not used for environmental specimens.) 513 */ 514 public SpecimenCollectionComponent setBodySite(CodeableConcept value) { 515 this.bodySite = value; 516 return this; 517 } 518 519 protected void listChildren(List<Property> childrenList) { 520 super.listChildren(childrenList); 521 childrenList.add(new Property("collector", "Reference(Practitioner)", "Person who collected the specimen.", 0, 522 java.lang.Integer.MAX_VALUE, collector)); 523 childrenList.add(new Property("comment", "string", 524 "To communicate any details or issues encountered during the specimen collection procedure.", 0, 525 java.lang.Integer.MAX_VALUE, comment)); 526 childrenList.add(new Property("collected[x]", "dateTime|Period", 527 "Time when specimen was collected from subject - the physiologically relevant time.", 0, 528 java.lang.Integer.MAX_VALUE, collected)); 529 childrenList.add(new Property("quantity", "SimpleQuantity", 530 "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 531 0, java.lang.Integer.MAX_VALUE, quantity)); 532 childrenList.add(new Property("method", "CodeableConcept", 533 "A coded value specifying the technique that is used to perform the procedure.", 0, 534 java.lang.Integer.MAX_VALUE, method)); 535 childrenList.add(new Property("bodySite", "CodeableConcept", 536 "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 537 0, java.lang.Integer.MAX_VALUE, bodySite)); 538 } 539 540 @Override 541 public void setProperty(String name, Base value) throws FHIRException { 542 if (name.equals("collector")) 543 this.collector = castToReference(value); // Reference 544 else if (name.equals("comment")) 545 this.getComment().add(castToString(value)); 546 else if (name.equals("collected[x]")) 547 this.collected = (Type) value; // Type 548 else if (name.equals("quantity")) 549 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 550 else if (name.equals("method")) 551 this.method = castToCodeableConcept(value); // CodeableConcept 552 else if (name.equals("bodySite")) 553 this.bodySite = castToCodeableConcept(value); // CodeableConcept 554 else 555 super.setProperty(name, value); 556 } 557 558 @Override 559 public Base addChild(String name) throws FHIRException { 560 if (name.equals("collector")) { 561 this.collector = new Reference(); 562 return this.collector; 563 } else if (name.equals("comment")) { 564 throw new FHIRException("Cannot call addChild on a singleton property Specimen.comment"); 565 } else if (name.equals("collectedDateTime")) { 566 this.collected = new DateTimeType(); 567 return this.collected; 568 } else if (name.equals("collectedPeriod")) { 569 this.collected = new Period(); 570 return this.collected; 571 } else if (name.equals("quantity")) { 572 this.quantity = new SimpleQuantity(); 573 return this.quantity; 574 } else if (name.equals("method")) { 575 this.method = new CodeableConcept(); 576 return this.method; 577 } else if (name.equals("bodySite")) { 578 this.bodySite = new CodeableConcept(); 579 return this.bodySite; 580 } else 581 return super.addChild(name); 582 } 583 584 public SpecimenCollectionComponent copy() { 585 SpecimenCollectionComponent dst = new SpecimenCollectionComponent(); 586 copyValues(dst); 587 dst.collector = collector == null ? null : collector.copy(); 588 if (comment != null) { 589 dst.comment = new ArrayList<StringType>(); 590 for (StringType i : comment) 591 dst.comment.add(i.copy()); 592 } 593 ; 594 dst.collected = collected == null ? null : collected.copy(); 595 dst.quantity = quantity == null ? null : quantity.copy(); 596 dst.method = method == null ? null : method.copy(); 597 dst.bodySite = bodySite == null ? null : bodySite.copy(); 598 return dst; 599 } 600 601 @Override 602 public boolean equalsDeep(Base other) { 603 if (!super.equalsDeep(other)) 604 return false; 605 if (!(other instanceof SpecimenCollectionComponent)) 606 return false; 607 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other; 608 return compareDeep(collector, o.collector, true) && compareDeep(comment, o.comment, true) 609 && compareDeep(collected, o.collected, true) && compareDeep(quantity, o.quantity, true) 610 && compareDeep(method, o.method, true) && compareDeep(bodySite, o.bodySite, true); 611 } 612 613 @Override 614 public boolean equalsShallow(Base other) { 615 if (!super.equalsShallow(other)) 616 return false; 617 if (!(other instanceof SpecimenCollectionComponent)) 618 return false; 619 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other; 620 return compareValues(comment, o.comment, true); 621 } 622 623 public boolean isEmpty() { 624 return super.isEmpty() && (collector == null || collector.isEmpty()) && (comment == null || comment.isEmpty()) 625 && (collected == null || collected.isEmpty()) && (quantity == null || quantity.isEmpty()) 626 && (method == null || method.isEmpty()) && (bodySite == null || bodySite.isEmpty()); 627 } 628 629 public String fhirType() { 630 return "Specimen.collection"; 631 632 } 633 634 } 635 636 @Block() 637 public static class SpecimenTreatmentComponent extends BackboneElement implements IBaseBackboneElement { 638 /** 639 * Textual description of procedure. 640 */ 641 @Child(name = "description", type = { 642 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 643 @Description(shortDefinition = "Textual description of procedure", formalDefinition = "Textual description of procedure.") 644 protected StringType description; 645 646 /** 647 * A coded value specifying the procedure used to process the specimen. 648 */ 649 @Child(name = "procedure", type = { 650 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 651 @Description(shortDefinition = "Indicates the treatment or processing step applied to the specimen", formalDefinition = "A coded value specifying the procedure used to process the specimen.") 652 protected CodeableConcept procedure; 653 654 /** 655 * Material used in the processing step. 656 */ 657 @Child(name = "additive", type = { 658 Substance.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 659 @Description(shortDefinition = "Material used in the processing step", formalDefinition = "Material used in the processing step.") 660 protected List<Reference> additive; 661 /** 662 * The actual objects that are the target of the reference (Material used in the 663 * processing step.) 664 */ 665 protected List<Substance> additiveTarget; 666 667 private static final long serialVersionUID = -373251521L; 668 669 /* 670 * Constructor 671 */ 672 public SpecimenTreatmentComponent() { 673 super(); 674 } 675 676 /** 677 * @return {@link #description} (Textual description of procedure.). This is the 678 * underlying object with id, value and extensions. The accessor 679 * "getDescription" gives direct access to the value 680 */ 681 public StringType getDescriptionElement() { 682 if (this.description == null) 683 if (Configuration.errorOnAutoCreate()) 684 throw new Error("Attempt to auto-create SpecimenTreatmentComponent.description"); 685 else if (Configuration.doAutoCreate()) 686 this.description = new StringType(); // bb 687 return this.description; 688 } 689 690 public boolean hasDescriptionElement() { 691 return this.description != null && !this.description.isEmpty(); 692 } 693 694 public boolean hasDescription() { 695 return this.description != null && !this.description.isEmpty(); 696 } 697 698 /** 699 * @param value {@link #description} (Textual description of procedure.). This 700 * is the underlying object with id, value and extensions. The 701 * accessor "getDescription" gives direct access to the value 702 */ 703 public SpecimenTreatmentComponent setDescriptionElement(StringType value) { 704 this.description = value; 705 return this; 706 } 707 708 /** 709 * @return Textual description of procedure. 710 */ 711 public String getDescription() { 712 return this.description == null ? null : this.description.getValue(); 713 } 714 715 /** 716 * @param value Textual description of procedure. 717 */ 718 public SpecimenTreatmentComponent setDescription(String value) { 719 if (Utilities.noString(value)) 720 this.description = null; 721 else { 722 if (this.description == null) 723 this.description = new StringType(); 724 this.description.setValue(value); 725 } 726 return this; 727 } 728 729 /** 730 * @return {@link #procedure} (A coded value specifying the procedure used to 731 * process the specimen.) 732 */ 733 public CodeableConcept getProcedure() { 734 if (this.procedure == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create SpecimenTreatmentComponent.procedure"); 737 else if (Configuration.doAutoCreate()) 738 this.procedure = new CodeableConcept(); // cc 739 return this.procedure; 740 } 741 742 public boolean hasProcedure() { 743 return this.procedure != null && !this.procedure.isEmpty(); 744 } 745 746 /** 747 * @param value {@link #procedure} (A coded value specifying the procedure used 748 * to process the specimen.) 749 */ 750 public SpecimenTreatmentComponent setProcedure(CodeableConcept value) { 751 this.procedure = value; 752 return this; 753 } 754 755 /** 756 * @return {@link #additive} (Material used in the processing step.) 757 */ 758 public List<Reference> getAdditive() { 759 if (this.additive == null) 760 this.additive = new ArrayList<Reference>(); 761 return this.additive; 762 } 763 764 public boolean hasAdditive() { 765 if (this.additive == null) 766 return false; 767 for (Reference item : this.additive) 768 if (!item.isEmpty()) 769 return true; 770 return false; 771 } 772 773 /** 774 * @return {@link #additive} (Material used in the processing step.) 775 */ 776 // syntactic sugar 777 public Reference addAdditive() { // 3 778 Reference t = new Reference(); 779 if (this.additive == null) 780 this.additive = new ArrayList<Reference>(); 781 this.additive.add(t); 782 return t; 783 } 784 785 // syntactic sugar 786 public SpecimenTreatmentComponent addAdditive(Reference t) { // 3 787 if (t == null) 788 return this; 789 if (this.additive == null) 790 this.additive = new ArrayList<Reference>(); 791 this.additive.add(t); 792 return this; 793 } 794 795 /** 796 * @return {@link #additive} (The actual objects that are the target of the 797 * reference. The reference library doesn't populate this, but you can 798 * use this to hold the resources if you resolvethemt. Material used in 799 * the processing step.) 800 */ 801 public List<Substance> getAdditiveTarget() { 802 if (this.additiveTarget == null) 803 this.additiveTarget = new ArrayList<Substance>(); 804 return this.additiveTarget; 805 } 806 807 // syntactic sugar 808 /** 809 * @return {@link #additive} (Add an actual object that is the target of the 810 * reference. The reference library doesn't use these, but you can use 811 * this to hold the resources if you resolvethemt. Material used in the 812 * processing step.) 813 */ 814 public Substance addAdditiveTarget() { 815 Substance r = new Substance(); 816 if (this.additiveTarget == null) 817 this.additiveTarget = new ArrayList<Substance>(); 818 this.additiveTarget.add(r); 819 return r; 820 } 821 822 protected void listChildren(List<Property> childrenList) { 823 super.listChildren(childrenList); 824 childrenList.add(new Property("description", "string", "Textual description of procedure.", 0, 825 java.lang.Integer.MAX_VALUE, description)); 826 childrenList.add(new Property("procedure", "CodeableConcept", 827 "A coded value specifying the procedure used to process the specimen.", 0, java.lang.Integer.MAX_VALUE, 828 procedure)); 829 childrenList.add(new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, 830 java.lang.Integer.MAX_VALUE, additive)); 831 } 832 833 @Override 834 public void setProperty(String name, Base value) throws FHIRException { 835 if (name.equals("description")) 836 this.description = castToString(value); // StringType 837 else if (name.equals("procedure")) 838 this.procedure = castToCodeableConcept(value); // CodeableConcept 839 else if (name.equals("additive")) 840 this.getAdditive().add(castToReference(value)); 841 else 842 super.setProperty(name, value); 843 } 844 845 @Override 846 public Base addChild(String name) throws FHIRException { 847 if (name.equals("description")) { 848 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 849 } else if (name.equals("procedure")) { 850 this.procedure = new CodeableConcept(); 851 return this.procedure; 852 } else if (name.equals("additive")) { 853 return addAdditive(); 854 } else 855 return super.addChild(name); 856 } 857 858 public SpecimenTreatmentComponent copy() { 859 SpecimenTreatmentComponent dst = new SpecimenTreatmentComponent(); 860 copyValues(dst); 861 dst.description = description == null ? null : description.copy(); 862 dst.procedure = procedure == null ? null : procedure.copy(); 863 if (additive != null) { 864 dst.additive = new ArrayList<Reference>(); 865 for (Reference i : additive) 866 dst.additive.add(i.copy()); 867 } 868 ; 869 return dst; 870 } 871 872 @Override 873 public boolean equalsDeep(Base other) { 874 if (!super.equalsDeep(other)) 875 return false; 876 if (!(other instanceof SpecimenTreatmentComponent)) 877 return false; 878 SpecimenTreatmentComponent o = (SpecimenTreatmentComponent) other; 879 return compareDeep(description, o.description, true) && compareDeep(procedure, o.procedure, true) 880 && compareDeep(additive, o.additive, true); 881 } 882 883 @Override 884 public boolean equalsShallow(Base other) { 885 if (!super.equalsShallow(other)) 886 return false; 887 if (!(other instanceof SpecimenTreatmentComponent)) 888 return false; 889 SpecimenTreatmentComponent o = (SpecimenTreatmentComponent) other; 890 return compareValues(description, o.description, true); 891 } 892 893 public boolean isEmpty() { 894 return super.isEmpty() && (description == null || description.isEmpty()) 895 && (procedure == null || procedure.isEmpty()) && (additive == null || additive.isEmpty()); 896 } 897 898 public String fhirType() { 899 return "Specimen.treatment"; 900 901 } 902 903 } 904 905 @Block() 906 public static class SpecimenContainerComponent extends BackboneElement implements IBaseBackboneElement { 907 /** 908 * Id for container. There may be multiple; a manufacturer's bar code, lab 909 * assigned identifier, etc. The container ID may differ from the specimen id in 910 * some circumstances. 911 */ 912 @Child(name = "identifier", type = { 913 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 914 @Description(shortDefinition = "Id for the container", formalDefinition = "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.") 915 protected List<Identifier> identifier; 916 917 /** 918 * Textual description of the container. 919 */ 920 @Child(name = "description", type = { 921 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 922 @Description(shortDefinition = "Textual description of the container", formalDefinition = "Textual description of the container.") 923 protected StringType description; 924 925 /** 926 * The type of container associated with the specimen (e.g. slide, aliquot, 927 * etc.). 928 */ 929 @Child(name = "type", type = { 930 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 931 @Description(shortDefinition = "Kind of container directly associated with specimen", formalDefinition = "The type of container associated with the specimen (e.g. slide, aliquot, etc.).") 932 protected CodeableConcept type; 933 934 /** 935 * The capacity (volume or other measure) the container may contain. 936 */ 937 @Child(name = "capacity", type = { 938 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 939 @Description(shortDefinition = "Container volume or size", formalDefinition = "The capacity (volume or other measure) the container may contain.") 940 protected SimpleQuantity capacity; 941 942 /** 943 * The quantity of specimen in the container; may be volume, dimensions, or 944 * other appropriate measurements, depending on the specimen type. 945 */ 946 @Child(name = "specimenQuantity", type = { 947 SimpleQuantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 948 @Description(shortDefinition = "Quantity of specimen within container", formalDefinition = "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.") 949 protected SimpleQuantity specimenQuantity; 950 951 /** 952 * Introduced substance to preserve, maintain or enhance the specimen. Examples: 953 * Formalin, Citrate, EDTA. 954 */ 955 @Child(name = "additive", type = { CodeableConcept.class, 956 Substance.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 957 @Description(shortDefinition = "Additive associated with container", formalDefinition = "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.") 958 protected Type additive; 959 960 private static final long serialVersionUID = 187274879L; 961 962 /* 963 * Constructor 964 */ 965 public SpecimenContainerComponent() { 966 super(); 967 } 968 969 /** 970 * @return {@link #identifier} (Id for container. There may be multiple; a 971 * manufacturer's bar code, lab assigned identifier, etc. The container 972 * ID may differ from the specimen id in some circumstances.) 973 */ 974 public List<Identifier> getIdentifier() { 975 if (this.identifier == null) 976 this.identifier = new ArrayList<Identifier>(); 977 return this.identifier; 978 } 979 980 public boolean hasIdentifier() { 981 if (this.identifier == null) 982 return false; 983 for (Identifier item : this.identifier) 984 if (!item.isEmpty()) 985 return true; 986 return false; 987 } 988 989 /** 990 * @return {@link #identifier} (Id for container. There may be multiple; a 991 * manufacturer's bar code, lab assigned identifier, etc. The container 992 * ID may differ from the specimen id in some circumstances.) 993 */ 994 // syntactic sugar 995 public Identifier addIdentifier() { // 3 996 Identifier t = new Identifier(); 997 if (this.identifier == null) 998 this.identifier = new ArrayList<Identifier>(); 999 this.identifier.add(t); 1000 return t; 1001 } 1002 1003 // syntactic sugar 1004 public SpecimenContainerComponent addIdentifier(Identifier t) { // 3 1005 if (t == null) 1006 return this; 1007 if (this.identifier == null) 1008 this.identifier = new ArrayList<Identifier>(); 1009 this.identifier.add(t); 1010 return this; 1011 } 1012 1013 /** 1014 * @return {@link #description} (Textual description of the container.). This is 1015 * the underlying object with id, value and extensions. The accessor 1016 * "getDescription" gives direct access to the value 1017 */ 1018 public StringType getDescriptionElement() { 1019 if (this.description == null) 1020 if (Configuration.errorOnAutoCreate()) 1021 throw new Error("Attempt to auto-create SpecimenContainerComponent.description"); 1022 else if (Configuration.doAutoCreate()) 1023 this.description = new StringType(); // bb 1024 return this.description; 1025 } 1026 1027 public boolean hasDescriptionElement() { 1028 return this.description != null && !this.description.isEmpty(); 1029 } 1030 1031 public boolean hasDescription() { 1032 return this.description != null && !this.description.isEmpty(); 1033 } 1034 1035 /** 1036 * @param value {@link #description} (Textual description of the container.). 1037 * This is the underlying object with id, value and extensions. The 1038 * accessor "getDescription" gives direct access to the value 1039 */ 1040 public SpecimenContainerComponent setDescriptionElement(StringType value) { 1041 this.description = value; 1042 return this; 1043 } 1044 1045 /** 1046 * @return Textual description of the container. 1047 */ 1048 public String getDescription() { 1049 return this.description == null ? null : this.description.getValue(); 1050 } 1051 1052 /** 1053 * @param value Textual description of the container. 1054 */ 1055 public SpecimenContainerComponent setDescription(String value) { 1056 if (Utilities.noString(value)) 1057 this.description = null; 1058 else { 1059 if (this.description == null) 1060 this.description = new StringType(); 1061 this.description.setValue(value); 1062 } 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #type} (The type of container associated with the specimen 1068 * (e.g. slide, aliquot, etc.).) 1069 */ 1070 public CodeableConcept getType() { 1071 if (this.type == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create SpecimenContainerComponent.type"); 1074 else if (Configuration.doAutoCreate()) 1075 this.type = new CodeableConcept(); // cc 1076 return this.type; 1077 } 1078 1079 public boolean hasType() { 1080 return this.type != null && !this.type.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #type} (The type of container associated with the 1085 * specimen (e.g. slide, aliquot, etc.).) 1086 */ 1087 public SpecimenContainerComponent setType(CodeableConcept value) { 1088 this.type = value; 1089 return this; 1090 } 1091 1092 /** 1093 * @return {@link #capacity} (The capacity (volume or other measure) the 1094 * container may contain.) 1095 */ 1096 public SimpleQuantity getCapacity() { 1097 if (this.capacity == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create SpecimenContainerComponent.capacity"); 1100 else if (Configuration.doAutoCreate()) 1101 this.capacity = new SimpleQuantity(); // cc 1102 return this.capacity; 1103 } 1104 1105 public boolean hasCapacity() { 1106 return this.capacity != null && !this.capacity.isEmpty(); 1107 } 1108 1109 /** 1110 * @param value {@link #capacity} (The capacity (volume or other measure) the 1111 * container may contain.) 1112 */ 1113 public SpecimenContainerComponent setCapacity(SimpleQuantity value) { 1114 this.capacity = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return {@link #specimenQuantity} (The quantity of specimen in the container; 1120 * may be volume, dimensions, or other appropriate measurements, 1121 * depending on the specimen type.) 1122 */ 1123 public SimpleQuantity getSpecimenQuantity() { 1124 if (this.specimenQuantity == null) 1125 if (Configuration.errorOnAutoCreate()) 1126 throw new Error("Attempt to auto-create SpecimenContainerComponent.specimenQuantity"); 1127 else if (Configuration.doAutoCreate()) 1128 this.specimenQuantity = new SimpleQuantity(); // cc 1129 return this.specimenQuantity; 1130 } 1131 1132 public boolean hasSpecimenQuantity() { 1133 return this.specimenQuantity != null && !this.specimenQuantity.isEmpty(); 1134 } 1135 1136 /** 1137 * @param value {@link #specimenQuantity} (The quantity of specimen in the 1138 * container; may be volume, dimensions, or other appropriate 1139 * measurements, depending on the specimen type.) 1140 */ 1141 public SpecimenContainerComponent setSpecimenQuantity(SimpleQuantity value) { 1142 this.specimenQuantity = value; 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #additive} (Introduced substance to preserve, maintain or 1148 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1149 */ 1150 public Type getAdditive() { 1151 return this.additive; 1152 } 1153 1154 /** 1155 * @return {@link #additive} (Introduced substance to preserve, maintain or 1156 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1157 */ 1158 public CodeableConcept getAdditiveCodeableConcept() throws FHIRException { 1159 if (!(this.additive instanceof CodeableConcept)) 1160 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1161 + this.additive.getClass().getName() + " was encountered"); 1162 return (CodeableConcept) this.additive; 1163 } 1164 1165 public boolean hasAdditiveCodeableConcept() { 1166 return this.additive instanceof CodeableConcept; 1167 } 1168 1169 /** 1170 * @return {@link #additive} (Introduced substance to preserve, maintain or 1171 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1172 */ 1173 public Reference getAdditiveReference() throws FHIRException { 1174 if (!(this.additive instanceof Reference)) 1175 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1176 + this.additive.getClass().getName() + " was encountered"); 1177 return (Reference) this.additive; 1178 } 1179 1180 public boolean hasAdditiveReference() { 1181 return this.additive instanceof Reference; 1182 } 1183 1184 public boolean hasAdditive() { 1185 return this.additive != null && !this.additive.isEmpty(); 1186 } 1187 1188 /** 1189 * @param value {@link #additive} (Introduced substance to preserve, maintain or 1190 * enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1191 */ 1192 public SpecimenContainerComponent setAdditive(Type value) { 1193 this.additive = value; 1194 return this; 1195 } 1196 1197 protected void listChildren(List<Property> childrenList) { 1198 super.listChildren(childrenList); 1199 childrenList.add(new Property("identifier", "Identifier", 1200 "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 1201 0, java.lang.Integer.MAX_VALUE, identifier)); 1202 childrenList.add(new Property("description", "string", "Textual description of the container.", 0, 1203 java.lang.Integer.MAX_VALUE, description)); 1204 childrenList.add(new Property("type", "CodeableConcept", 1205 "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1206 java.lang.Integer.MAX_VALUE, type)); 1207 childrenList.add(new Property("capacity", "SimpleQuantity", 1208 "The capacity (volume or other measure) the container may contain.", 0, java.lang.Integer.MAX_VALUE, 1209 capacity)); 1210 childrenList.add(new Property("specimenQuantity", "SimpleQuantity", 1211 "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 1212 0, java.lang.Integer.MAX_VALUE, specimenQuantity)); 1213 childrenList.add(new Property("additive[x]", "CodeableConcept|Reference(Substance)", 1214 "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1215 java.lang.Integer.MAX_VALUE, additive)); 1216 } 1217 1218 @Override 1219 public void setProperty(String name, Base value) throws FHIRException { 1220 if (name.equals("identifier")) 1221 this.getIdentifier().add(castToIdentifier(value)); 1222 else if (name.equals("description")) 1223 this.description = castToString(value); // StringType 1224 else if (name.equals("type")) 1225 this.type = castToCodeableConcept(value); // CodeableConcept 1226 else if (name.equals("capacity")) 1227 this.capacity = castToSimpleQuantity(value); // SimpleQuantity 1228 else if (name.equals("specimenQuantity")) 1229 this.specimenQuantity = castToSimpleQuantity(value); // SimpleQuantity 1230 else if (name.equals("additive[x]")) 1231 this.additive = (Type) value; // Type 1232 else 1233 super.setProperty(name, value); 1234 } 1235 1236 @Override 1237 public Base addChild(String name) throws FHIRException { 1238 if (name.equals("identifier")) { 1239 return addIdentifier(); 1240 } else if (name.equals("description")) { 1241 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 1242 } else if (name.equals("type")) { 1243 this.type = new CodeableConcept(); 1244 return this.type; 1245 } else if (name.equals("capacity")) { 1246 this.capacity = new SimpleQuantity(); 1247 return this.capacity; 1248 } else if (name.equals("specimenQuantity")) { 1249 this.specimenQuantity = new SimpleQuantity(); 1250 return this.specimenQuantity; 1251 } else if (name.equals("additiveCodeableConcept")) { 1252 this.additive = new CodeableConcept(); 1253 return this.additive; 1254 } else if (name.equals("additiveReference")) { 1255 this.additive = new Reference(); 1256 return this.additive; 1257 } else 1258 return super.addChild(name); 1259 } 1260 1261 public SpecimenContainerComponent copy() { 1262 SpecimenContainerComponent dst = new SpecimenContainerComponent(); 1263 copyValues(dst); 1264 if (identifier != null) { 1265 dst.identifier = new ArrayList<Identifier>(); 1266 for (Identifier i : identifier) 1267 dst.identifier.add(i.copy()); 1268 } 1269 ; 1270 dst.description = description == null ? null : description.copy(); 1271 dst.type = type == null ? null : type.copy(); 1272 dst.capacity = capacity == null ? null : capacity.copy(); 1273 dst.specimenQuantity = specimenQuantity == null ? null : specimenQuantity.copy(); 1274 dst.additive = additive == null ? null : additive.copy(); 1275 return dst; 1276 } 1277 1278 @Override 1279 public boolean equalsDeep(Base other) { 1280 if (!super.equalsDeep(other)) 1281 return false; 1282 if (!(other instanceof SpecimenContainerComponent)) 1283 return false; 1284 SpecimenContainerComponent o = (SpecimenContainerComponent) other; 1285 return compareDeep(identifier, o.identifier, true) && compareDeep(description, o.description, true) 1286 && compareDeep(type, o.type, true) && compareDeep(capacity, o.capacity, true) 1287 && compareDeep(specimenQuantity, o.specimenQuantity, true) && compareDeep(additive, o.additive, true); 1288 } 1289 1290 @Override 1291 public boolean equalsShallow(Base other) { 1292 if (!super.equalsShallow(other)) 1293 return false; 1294 if (!(other instanceof SpecimenContainerComponent)) 1295 return false; 1296 SpecimenContainerComponent o = (SpecimenContainerComponent) other; 1297 return compareValues(description, o.description, true); 1298 } 1299 1300 public boolean isEmpty() { 1301 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 1302 && (description == null || description.isEmpty()) && (type == null || type.isEmpty()) 1303 && (capacity == null || capacity.isEmpty()) && (specimenQuantity == null || specimenQuantity.isEmpty()) 1304 && (additive == null || additive.isEmpty()); 1305 } 1306 1307 public String fhirType() { 1308 return "Specimen.container"; 1309 1310 } 1311 1312 } 1313 1314 /** 1315 * Id for specimen. 1316 */ 1317 @Child(name = "identifier", type = { 1318 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1319 @Description(shortDefinition = "External Identifier", formalDefinition = "Id for specimen.") 1320 protected List<Identifier> identifier; 1321 1322 /** 1323 * The availability of the specimen. 1324 */ 1325 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1326 @Description(shortDefinition = "available | unavailable | unsatisfactory | entered-in-error", formalDefinition = "The availability of the specimen.") 1327 protected Enumeration<SpecimenStatus> status; 1328 1329 /** 1330 * The kind of material that forms the specimen. 1331 */ 1332 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1333 @Description(shortDefinition = "Kind of material that forms the specimen", formalDefinition = "The kind of material that forms the specimen.") 1334 protected CodeableConcept type; 1335 1336 /** 1337 * Reference to the parent (source) specimen which is used when the specimen was 1338 * either derived from or a component of another specimen. 1339 */ 1340 @Child(name = "parent", type = { 1341 Specimen.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1342 @Description(shortDefinition = "Specimen from which this specimen originated", formalDefinition = "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.") 1343 protected List<Reference> parent; 1344 /** 1345 * The actual objects that are the target of the reference (Reference to the 1346 * parent (source) specimen which is used when the specimen was either derived 1347 * from or a component of another specimen.) 1348 */ 1349 protected List<Specimen> parentTarget; 1350 1351 /** 1352 * Where the specimen came from. This may be from the patient(s) or from the 1353 * environment or a device. 1354 */ 1355 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 1356 Substance.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1357 @Description(shortDefinition = "Where the specimen came from. This may be from the patient(s) or from the environment or a device", formalDefinition = "Where the specimen came from. This may be from the patient(s) or from the environment or a device.") 1358 protected Reference subject; 1359 1360 /** 1361 * The actual object that is the target of the reference (Where the specimen 1362 * came from. This may be from the patient(s) or from the environment or a 1363 * device.) 1364 */ 1365 protected Resource subjectTarget; 1366 1367 /** 1368 * The identifier assigned by the lab when accessioning specimen(s). This is not 1369 * necessarily the same as the specimen identifier, depending on local lab 1370 * procedures. 1371 */ 1372 @Child(name = "accessionIdentifier", type = { 1373 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1374 @Description(shortDefinition = "Identifier assigned by the lab", formalDefinition = "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.") 1375 protected Identifier accessionIdentifier; 1376 1377 /** 1378 * Time when specimen was received for processing or testing. 1379 */ 1380 @Child(name = "receivedTime", type = { 1381 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1382 @Description(shortDefinition = "The time when specimen was received for processing", formalDefinition = "Time when specimen was received for processing or testing.") 1383 protected DateTimeType receivedTime; 1384 1385 /** 1386 * Details concerning the specimen collection. 1387 */ 1388 @Child(name = "collection", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 1389 @Description(shortDefinition = "Collection details", formalDefinition = "Details concerning the specimen collection.") 1390 protected SpecimenCollectionComponent collection; 1391 1392 /** 1393 * Details concerning treatment and processing steps for the specimen. 1394 */ 1395 @Child(name = "treatment", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1396 @Description(shortDefinition = "Treatment and processing step details", formalDefinition = "Details concerning treatment and processing steps for the specimen.") 1397 protected List<SpecimenTreatmentComponent> treatment; 1398 1399 /** 1400 * The container holding the specimen. The recursive nature of containers; i.e. 1401 * blood in tube in tray in rack is not addressed here. 1402 */ 1403 @Child(name = "container", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1404 @Description(shortDefinition = "Direct container of specimen (tube/slide, etc.)", formalDefinition = "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.") 1405 protected List<SpecimenContainerComponent> container; 1406 1407 private static final long serialVersionUID = 1025605602L; 1408 1409 /* 1410 * Constructor 1411 */ 1412 public Specimen() { 1413 super(); 1414 } 1415 1416 /* 1417 * Constructor 1418 */ 1419 public Specimen(Reference subject) { 1420 super(); 1421 this.subject = subject; 1422 } 1423 1424 /** 1425 * @return {@link #identifier} (Id for specimen.) 1426 */ 1427 public List<Identifier> getIdentifier() { 1428 if (this.identifier == null) 1429 this.identifier = new ArrayList<Identifier>(); 1430 return this.identifier; 1431 } 1432 1433 public boolean hasIdentifier() { 1434 if (this.identifier == null) 1435 return false; 1436 for (Identifier item : this.identifier) 1437 if (!item.isEmpty()) 1438 return true; 1439 return false; 1440 } 1441 1442 /** 1443 * @return {@link #identifier} (Id for specimen.) 1444 */ 1445 // syntactic sugar 1446 public Identifier addIdentifier() { // 3 1447 Identifier t = new Identifier(); 1448 if (this.identifier == null) 1449 this.identifier = new ArrayList<Identifier>(); 1450 this.identifier.add(t); 1451 return t; 1452 } 1453 1454 // syntactic sugar 1455 public Specimen addIdentifier(Identifier t) { // 3 1456 if (t == null) 1457 return this; 1458 if (this.identifier == null) 1459 this.identifier = new ArrayList<Identifier>(); 1460 this.identifier.add(t); 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #status} (The availability of the specimen.). This is the 1466 * underlying object with id, value and extensions. The accessor 1467 * "getStatus" gives direct access to the value 1468 */ 1469 public Enumeration<SpecimenStatus> getStatusElement() { 1470 if (this.status == null) 1471 if (Configuration.errorOnAutoCreate()) 1472 throw new Error("Attempt to auto-create Specimen.status"); 1473 else if (Configuration.doAutoCreate()) 1474 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); // bb 1475 return this.status; 1476 } 1477 1478 public boolean hasStatusElement() { 1479 return this.status != null && !this.status.isEmpty(); 1480 } 1481 1482 public boolean hasStatus() { 1483 return this.status != null && !this.status.isEmpty(); 1484 } 1485 1486 /** 1487 * @param value {@link #status} (The availability of the specimen.). This is the 1488 * underlying object with id, value and extensions. The accessor 1489 * "getStatus" gives direct access to the value 1490 */ 1491 public Specimen setStatusElement(Enumeration<SpecimenStatus> value) { 1492 this.status = value; 1493 return this; 1494 } 1495 1496 /** 1497 * @return The availability of the specimen. 1498 */ 1499 public SpecimenStatus getStatus() { 1500 return this.status == null ? null : this.status.getValue(); 1501 } 1502 1503 /** 1504 * @param value The availability of the specimen. 1505 */ 1506 public Specimen setStatus(SpecimenStatus value) { 1507 if (value == null) 1508 this.status = null; 1509 else { 1510 if (this.status == null) 1511 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); 1512 this.status.setValue(value); 1513 } 1514 return this; 1515 } 1516 1517 /** 1518 * @return {@link #type} (The kind of material that forms the specimen.) 1519 */ 1520 public CodeableConcept getType() { 1521 if (this.type == null) 1522 if (Configuration.errorOnAutoCreate()) 1523 throw new Error("Attempt to auto-create Specimen.type"); 1524 else if (Configuration.doAutoCreate()) 1525 this.type = new CodeableConcept(); // cc 1526 return this.type; 1527 } 1528 1529 public boolean hasType() { 1530 return this.type != null && !this.type.isEmpty(); 1531 } 1532 1533 /** 1534 * @param value {@link #type} (The kind of material that forms the specimen.) 1535 */ 1536 public Specimen setType(CodeableConcept value) { 1537 this.type = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return {@link #parent} (Reference to the parent (source) specimen which is 1543 * used when the specimen was either derived from or a component of 1544 * another specimen.) 1545 */ 1546 public List<Reference> getParent() { 1547 if (this.parent == null) 1548 this.parent = new ArrayList<Reference>(); 1549 return this.parent; 1550 } 1551 1552 public boolean hasParent() { 1553 if (this.parent == null) 1554 return false; 1555 for (Reference item : this.parent) 1556 if (!item.isEmpty()) 1557 return true; 1558 return false; 1559 } 1560 1561 /** 1562 * @return {@link #parent} (Reference to the parent (source) specimen which is 1563 * used when the specimen was either derived from or a component of 1564 * another specimen.) 1565 */ 1566 // syntactic sugar 1567 public Reference addParent() { // 3 1568 Reference t = new Reference(); 1569 if (this.parent == null) 1570 this.parent = new ArrayList<Reference>(); 1571 this.parent.add(t); 1572 return t; 1573 } 1574 1575 // syntactic sugar 1576 public Specimen addParent(Reference t) { // 3 1577 if (t == null) 1578 return this; 1579 if (this.parent == null) 1580 this.parent = new ArrayList<Reference>(); 1581 this.parent.add(t); 1582 return this; 1583 } 1584 1585 /** 1586 * @return {@link #parent} (The actual objects that are the target of the 1587 * reference. The reference library doesn't populate this, but you can 1588 * use this to hold the resources if you resolvethemt. Reference to the 1589 * parent (source) specimen which is used when the specimen was either 1590 * derived from or a component of another specimen.) 1591 */ 1592 public List<Specimen> getParentTarget() { 1593 if (this.parentTarget == null) 1594 this.parentTarget = new ArrayList<Specimen>(); 1595 return this.parentTarget; 1596 } 1597 1598 // syntactic sugar 1599 /** 1600 * @return {@link #parent} (Add an actual object that is the target of the 1601 * reference. The reference library doesn't use these, but you can use 1602 * this to hold the resources if you resolvethemt. Reference to the 1603 * parent (source) specimen which is used when the specimen was either 1604 * derived from or a component of another specimen.) 1605 */ 1606 public Specimen addParentTarget() { 1607 Specimen r = new Specimen(); 1608 if (this.parentTarget == null) 1609 this.parentTarget = new ArrayList<Specimen>(); 1610 this.parentTarget.add(r); 1611 return r; 1612 } 1613 1614 /** 1615 * @return {@link #subject} (Where the specimen came from. This may be from the 1616 * patient(s) or from the environment or a device.) 1617 */ 1618 public Reference getSubject() { 1619 if (this.subject == null) 1620 if (Configuration.errorOnAutoCreate()) 1621 throw new Error("Attempt to auto-create Specimen.subject"); 1622 else if (Configuration.doAutoCreate()) 1623 this.subject = new Reference(); // cc 1624 return this.subject; 1625 } 1626 1627 public boolean hasSubject() { 1628 return this.subject != null && !this.subject.isEmpty(); 1629 } 1630 1631 /** 1632 * @param value {@link #subject} (Where the specimen came from. This may be from 1633 * the patient(s) or from the environment or a device.) 1634 */ 1635 public Specimen setSubject(Reference value) { 1636 this.subject = value; 1637 return this; 1638 } 1639 1640 /** 1641 * @return {@link #subject} The actual object that is the target of the 1642 * reference. The reference library doesn't populate this, but you can 1643 * use it to hold the resource if you resolve it. (Where the specimen 1644 * came from. This may be from the patient(s) or from the environment or 1645 * a device.) 1646 */ 1647 public Resource getSubjectTarget() { 1648 return this.subjectTarget; 1649 } 1650 1651 /** 1652 * @param value {@link #subject} The actual object that is the target of the 1653 * reference. The reference library doesn't use these, but you can 1654 * use it to hold the resource if you resolve it. (Where the 1655 * specimen came from. This may be from the patient(s) or from the 1656 * environment or a device.) 1657 */ 1658 public Specimen setSubjectTarget(Resource value) { 1659 this.subjectTarget = value; 1660 return this; 1661 } 1662 1663 /** 1664 * @return {@link #accessionIdentifier} (The identifier assigned by the lab when 1665 * accessioning specimen(s). This is not necessarily the same as the 1666 * specimen identifier, depending on local lab procedures.) 1667 */ 1668 public Identifier getAccessionIdentifier() { 1669 if (this.accessionIdentifier == null) 1670 if (Configuration.errorOnAutoCreate()) 1671 throw new Error("Attempt to auto-create Specimen.accessionIdentifier"); 1672 else if (Configuration.doAutoCreate()) 1673 this.accessionIdentifier = new Identifier(); // cc 1674 return this.accessionIdentifier; 1675 } 1676 1677 public boolean hasAccessionIdentifier() { 1678 return this.accessionIdentifier != null && !this.accessionIdentifier.isEmpty(); 1679 } 1680 1681 /** 1682 * @param value {@link #accessionIdentifier} (The identifier assigned by the lab 1683 * when accessioning specimen(s). This is not necessarily the same 1684 * as the specimen identifier, depending on local lab procedures.) 1685 */ 1686 public Specimen setAccessionIdentifier(Identifier value) { 1687 this.accessionIdentifier = value; 1688 return this; 1689 } 1690 1691 /** 1692 * @return {@link #receivedTime} (Time when specimen was received for processing 1693 * or testing.). This is the underlying object with id, value and 1694 * extensions. The accessor "getReceivedTime" gives direct access to the 1695 * value 1696 */ 1697 public DateTimeType getReceivedTimeElement() { 1698 if (this.receivedTime == null) 1699 if (Configuration.errorOnAutoCreate()) 1700 throw new Error("Attempt to auto-create Specimen.receivedTime"); 1701 else if (Configuration.doAutoCreate()) 1702 this.receivedTime = new DateTimeType(); // bb 1703 return this.receivedTime; 1704 } 1705 1706 public boolean hasReceivedTimeElement() { 1707 return this.receivedTime != null && !this.receivedTime.isEmpty(); 1708 } 1709 1710 public boolean hasReceivedTime() { 1711 return this.receivedTime != null && !this.receivedTime.isEmpty(); 1712 } 1713 1714 /** 1715 * @param value {@link #receivedTime} (Time when specimen was received for 1716 * processing or testing.). This is the underlying object with id, 1717 * value and extensions. The accessor "getReceivedTime" gives 1718 * direct access to the value 1719 */ 1720 public Specimen setReceivedTimeElement(DateTimeType value) { 1721 this.receivedTime = value; 1722 return this; 1723 } 1724 1725 /** 1726 * @return Time when specimen was received for processing or testing. 1727 */ 1728 public Date getReceivedTime() { 1729 return this.receivedTime == null ? null : this.receivedTime.getValue(); 1730 } 1731 1732 /** 1733 * @param value Time when specimen was received for processing or testing. 1734 */ 1735 public Specimen setReceivedTime(Date value) { 1736 if (value == null) 1737 this.receivedTime = null; 1738 else { 1739 if (this.receivedTime == null) 1740 this.receivedTime = new DateTimeType(); 1741 this.receivedTime.setValue(value); 1742 } 1743 return this; 1744 } 1745 1746 /** 1747 * @return {@link #collection} (Details concerning the specimen collection.) 1748 */ 1749 public SpecimenCollectionComponent getCollection() { 1750 if (this.collection == null) 1751 if (Configuration.errorOnAutoCreate()) 1752 throw new Error("Attempt to auto-create Specimen.collection"); 1753 else if (Configuration.doAutoCreate()) 1754 this.collection = new SpecimenCollectionComponent(); // cc 1755 return this.collection; 1756 } 1757 1758 public boolean hasCollection() { 1759 return this.collection != null && !this.collection.isEmpty(); 1760 } 1761 1762 /** 1763 * @param value {@link #collection} (Details concerning the specimen 1764 * collection.) 1765 */ 1766 public Specimen setCollection(SpecimenCollectionComponent value) { 1767 this.collection = value; 1768 return this; 1769 } 1770 1771 /** 1772 * @return {@link #treatment} (Details concerning treatment and processing steps 1773 * for the specimen.) 1774 */ 1775 public List<SpecimenTreatmentComponent> getTreatment() { 1776 if (this.treatment == null) 1777 this.treatment = new ArrayList<SpecimenTreatmentComponent>(); 1778 return this.treatment; 1779 } 1780 1781 public boolean hasTreatment() { 1782 if (this.treatment == null) 1783 return false; 1784 for (SpecimenTreatmentComponent item : this.treatment) 1785 if (!item.isEmpty()) 1786 return true; 1787 return false; 1788 } 1789 1790 /** 1791 * @return {@link #treatment} (Details concerning treatment and processing steps 1792 * for the specimen.) 1793 */ 1794 // syntactic sugar 1795 public SpecimenTreatmentComponent addTreatment() { // 3 1796 SpecimenTreatmentComponent t = new SpecimenTreatmentComponent(); 1797 if (this.treatment == null) 1798 this.treatment = new ArrayList<SpecimenTreatmentComponent>(); 1799 this.treatment.add(t); 1800 return t; 1801 } 1802 1803 // syntactic sugar 1804 public Specimen addTreatment(SpecimenTreatmentComponent t) { // 3 1805 if (t == null) 1806 return this; 1807 if (this.treatment == null) 1808 this.treatment = new ArrayList<SpecimenTreatmentComponent>(); 1809 this.treatment.add(t); 1810 return this; 1811 } 1812 1813 /** 1814 * @return {@link #container} (The container holding the specimen. The recursive 1815 * nature of containers; i.e. blood in tube in tray in rack is not 1816 * addressed here.) 1817 */ 1818 public List<SpecimenContainerComponent> getContainer() { 1819 if (this.container == null) 1820 this.container = new ArrayList<SpecimenContainerComponent>(); 1821 return this.container; 1822 } 1823 1824 public boolean hasContainer() { 1825 if (this.container == null) 1826 return false; 1827 for (SpecimenContainerComponent item : this.container) 1828 if (!item.isEmpty()) 1829 return true; 1830 return false; 1831 } 1832 1833 /** 1834 * @return {@link #container} (The container holding the specimen. The recursive 1835 * nature of containers; i.e. blood in tube in tray in rack is not 1836 * addressed here.) 1837 */ 1838 // syntactic sugar 1839 public SpecimenContainerComponent addContainer() { // 3 1840 SpecimenContainerComponent t = new SpecimenContainerComponent(); 1841 if (this.container == null) 1842 this.container = new ArrayList<SpecimenContainerComponent>(); 1843 this.container.add(t); 1844 return t; 1845 } 1846 1847 // syntactic sugar 1848 public Specimen addContainer(SpecimenContainerComponent t) { // 3 1849 if (t == null) 1850 return this; 1851 if (this.container == null) 1852 this.container = new ArrayList<SpecimenContainerComponent>(); 1853 this.container.add(t); 1854 return this; 1855 } 1856 1857 protected void listChildren(List<Property> childrenList) { 1858 super.listChildren(childrenList); 1859 childrenList 1860 .add(new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1861 childrenList.add( 1862 new Property("status", "code", "The availability of the specimen.", 0, java.lang.Integer.MAX_VALUE, status)); 1863 childrenList.add(new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1864 java.lang.Integer.MAX_VALUE, type)); 1865 childrenList.add(new Property("parent", "Reference(Specimen)", 1866 "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 1867 0, java.lang.Integer.MAX_VALUE, parent)); 1868 childrenList.add(new Property("subject", "Reference(Patient|Group|Device|Substance)", 1869 "Where the specimen came from. This may be from the patient(s) or from the environment or a device.", 0, 1870 java.lang.Integer.MAX_VALUE, subject)); 1871 childrenList.add(new Property("accessionIdentifier", "Identifier", 1872 "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 1873 0, java.lang.Integer.MAX_VALUE, accessionIdentifier)); 1874 childrenList.add(new Property("receivedTime", "dateTime", 1875 "Time when specimen was received for processing or testing.", 0, java.lang.Integer.MAX_VALUE, receivedTime)); 1876 childrenList.add(new Property("collection", "", "Details concerning the specimen collection.", 0, 1877 java.lang.Integer.MAX_VALUE, collection)); 1878 childrenList 1879 .add(new Property("treatment", "", "Details concerning treatment and processing steps for the specimen.", 0, 1880 java.lang.Integer.MAX_VALUE, treatment)); 1881 childrenList.add(new Property("container", "", 1882 "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 1883 0, java.lang.Integer.MAX_VALUE, container)); 1884 } 1885 1886 @Override 1887 public void setProperty(String name, Base value) throws FHIRException { 1888 if (name.equals("identifier")) 1889 this.getIdentifier().add(castToIdentifier(value)); 1890 else if (name.equals("status")) 1891 this.status = new SpecimenStatusEnumFactory().fromType(value); // Enumeration<SpecimenStatus> 1892 else if (name.equals("type")) 1893 this.type = castToCodeableConcept(value); // CodeableConcept 1894 else if (name.equals("parent")) 1895 this.getParent().add(castToReference(value)); 1896 else if (name.equals("subject")) 1897 this.subject = castToReference(value); // Reference 1898 else if (name.equals("accessionIdentifier")) 1899 this.accessionIdentifier = castToIdentifier(value); // Identifier 1900 else if (name.equals("receivedTime")) 1901 this.receivedTime = castToDateTime(value); // DateTimeType 1902 else if (name.equals("collection")) 1903 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 1904 else if (name.equals("treatment")) 1905 this.getTreatment().add((SpecimenTreatmentComponent) value); 1906 else if (name.equals("container")) 1907 this.getContainer().add((SpecimenContainerComponent) value); 1908 else 1909 super.setProperty(name, value); 1910 } 1911 1912 @Override 1913 public Base addChild(String name) throws FHIRException { 1914 if (name.equals("identifier")) { 1915 return addIdentifier(); 1916 } else if (name.equals("status")) { 1917 throw new FHIRException("Cannot call addChild on a singleton property Specimen.status"); 1918 } else if (name.equals("type")) { 1919 this.type = new CodeableConcept(); 1920 return this.type; 1921 } else if (name.equals("parent")) { 1922 return addParent(); 1923 } else if (name.equals("subject")) { 1924 this.subject = new Reference(); 1925 return this.subject; 1926 } else if (name.equals("accessionIdentifier")) { 1927 this.accessionIdentifier = new Identifier(); 1928 return this.accessionIdentifier; 1929 } else if (name.equals("receivedTime")) { 1930 throw new FHIRException("Cannot call addChild on a singleton property Specimen.receivedTime"); 1931 } else if (name.equals("collection")) { 1932 this.collection = new SpecimenCollectionComponent(); 1933 return this.collection; 1934 } else if (name.equals("treatment")) { 1935 return addTreatment(); 1936 } else if (name.equals("container")) { 1937 return addContainer(); 1938 } else 1939 return super.addChild(name); 1940 } 1941 1942 public String fhirType() { 1943 return "Specimen"; 1944 1945 } 1946 1947 public Specimen copy() { 1948 Specimen dst = new Specimen(); 1949 copyValues(dst); 1950 if (identifier != null) { 1951 dst.identifier = new ArrayList<Identifier>(); 1952 for (Identifier i : identifier) 1953 dst.identifier.add(i.copy()); 1954 } 1955 ; 1956 dst.status = status == null ? null : status.copy(); 1957 dst.type = type == null ? null : type.copy(); 1958 if (parent != null) { 1959 dst.parent = new ArrayList<Reference>(); 1960 for (Reference i : parent) 1961 dst.parent.add(i.copy()); 1962 } 1963 ; 1964 dst.subject = subject == null ? null : subject.copy(); 1965 dst.accessionIdentifier = accessionIdentifier == null ? null : accessionIdentifier.copy(); 1966 dst.receivedTime = receivedTime == null ? null : receivedTime.copy(); 1967 dst.collection = collection == null ? null : collection.copy(); 1968 if (treatment != null) { 1969 dst.treatment = new ArrayList<SpecimenTreatmentComponent>(); 1970 for (SpecimenTreatmentComponent i : treatment) 1971 dst.treatment.add(i.copy()); 1972 } 1973 ; 1974 if (container != null) { 1975 dst.container = new ArrayList<SpecimenContainerComponent>(); 1976 for (SpecimenContainerComponent i : container) 1977 dst.container.add(i.copy()); 1978 } 1979 ; 1980 return dst; 1981 } 1982 1983 protected Specimen typedCopy() { 1984 return copy(); 1985 } 1986 1987 @Override 1988 public boolean equalsDeep(Base other) { 1989 if (!super.equalsDeep(other)) 1990 return false; 1991 if (!(other instanceof Specimen)) 1992 return false; 1993 Specimen o = (Specimen) other; 1994 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1995 && compareDeep(type, o.type, true) && compareDeep(parent, o.parent, true) 1996 && compareDeep(subject, o.subject, true) && compareDeep(accessionIdentifier, o.accessionIdentifier, true) 1997 && compareDeep(receivedTime, o.receivedTime, true) && compareDeep(collection, o.collection, true) 1998 && compareDeep(treatment, o.treatment, true) && compareDeep(container, o.container, true); 1999 } 2000 2001 @Override 2002 public boolean equalsShallow(Base other) { 2003 if (!super.equalsShallow(other)) 2004 return false; 2005 if (!(other instanceof Specimen)) 2006 return false; 2007 Specimen o = (Specimen) other; 2008 return compareValues(status, o.status, true) && compareValues(receivedTime, o.receivedTime, true); 2009 } 2010 2011 public boolean isEmpty() { 2012 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 2013 && (type == null || type.isEmpty()) && (parent == null || parent.isEmpty()) 2014 && (subject == null || subject.isEmpty()) && (accessionIdentifier == null || accessionIdentifier.isEmpty()) 2015 && (receivedTime == null || receivedTime.isEmpty()) && (collection == null || collection.isEmpty()) 2016 && (treatment == null || treatment.isEmpty()) && (container == null || container.isEmpty()); 2017 } 2018 2019 @Override 2020 public ResourceType getResourceType() { 2021 return ResourceType.Specimen; 2022 } 2023 2024 @SearchParamDefinition(name = "container", path = "Specimen.container.type", description = "The kind of specimen container", type = "token") 2025 public static final String SP_CONTAINER = "container"; 2026 @SearchParamDefinition(name = "identifier", path = "Specimen.identifier", description = "The unique identifier associated with the specimen", type = "token") 2027 public static final String SP_IDENTIFIER = "identifier"; 2028 @SearchParamDefinition(name = "parent", path = "Specimen.parent", description = "The parent of the specimen", type = "reference") 2029 public static final String SP_PARENT = "parent"; 2030 @SearchParamDefinition(name = "container-id", path = "Specimen.container.identifier", description = "The unique identifier associated with the specimen container", type = "token") 2031 public static final String SP_CONTAINERID = "container-id"; 2032 @SearchParamDefinition(name = "bodysite", path = "Specimen.collection.bodySite", description = "The code for the body site from where the specimen originated", type = "token") 2033 public static final String SP_BODYSITE = "bodysite"; 2034 @SearchParamDefinition(name = "subject", path = "Specimen.subject", description = "The subject of the specimen", type = "reference") 2035 public static final String SP_SUBJECT = "subject"; 2036 @SearchParamDefinition(name = "patient", path = "Specimen.subject", description = "The patient the specimen comes from", type = "reference") 2037 public static final String SP_PATIENT = "patient"; 2038 @SearchParamDefinition(name = "collected", path = "Specimen.collection.collected[x]", description = "The date the specimen was collected", type = "date") 2039 public static final String SP_COLLECTED = "collected"; 2040 @SearchParamDefinition(name = "accession", path = "Specimen.accessionIdentifier", description = "The accession number associated with the specimen", type = "token") 2041 public static final String SP_ACCESSION = "accession"; 2042 @SearchParamDefinition(name = "type", path = "Specimen.type", description = "The specimen type", type = "token") 2043 public static final String SP_TYPE = "type"; 2044 @SearchParamDefinition(name = "collector", path = "Specimen.collection.collector", description = "Who collected the specimen", type = "reference") 2045 public static final String SP_COLLECTOR = "collector"; 2046 2047}