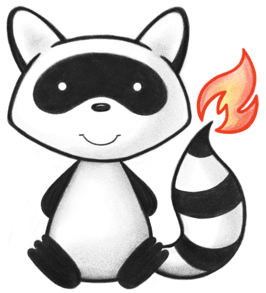
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A definition of a FHIR structure. This resource is used to describe the 050 * underlying resources, data types defined in FHIR, and also for describing 051 * extensions, and constraints on resources and data types. 052 */ 053@ResourceDef(name = "StructureDefinition", profile = "http://hl7.org/fhir/Profile/StructureDefinition") 054public class StructureDefinition extends DomainResource { 055 056 public enum StructureDefinitionKind { 057 /** 058 * A data type - either a primitive or complex structure that defines a set of 059 * data elements. These can be used throughout Resource and extension 060 * definitions. 061 */ 062 DATATYPE, 063 /** 064 * A resource defined by the FHIR specification. 065 */ 066 RESOURCE, 067 /** 068 * A logical model - a conceptual package of data that will be mapped to 069 * resources for implementation. 070 */ 071 LOGICAL, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static StructureDefinitionKind fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("datatype".equals(codeString)) 081 return DATATYPE; 082 if ("resource".equals(codeString)) 083 return RESOURCE; 084 if ("logical".equals(codeString)) 085 return LOGICAL; 086 throw new FHIRException("Unknown StructureDefinitionKind code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case DATATYPE: 092 return "datatype"; 093 case RESOURCE: 094 return "resource"; 095 case LOGICAL: 096 return "logical"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case DATATYPE: 107 return "http://hl7.org/fhir/structure-definition-kind"; 108 case RESOURCE: 109 return "http://hl7.org/fhir/structure-definition-kind"; 110 case LOGICAL: 111 return "http://hl7.org/fhir/structure-definition-kind"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case DATATYPE: 122 return "A data type - either a primitive or complex structure that defines a set of data elements. These can be used throughout Resource and extension definitions."; 123 case RESOURCE: 124 return "A resource defined by the FHIR specification."; 125 case LOGICAL: 126 return "A logical model - a conceptual package of data that will be mapped to resources for implementation."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case DATATYPE: 137 return "Data Type"; 138 case RESOURCE: 139 return "Resource"; 140 case LOGICAL: 141 return "Logical Model"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class StructureDefinitionKindEnumFactory implements EnumFactory<StructureDefinitionKind> { 151 public StructureDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("datatype".equals(codeString)) 156 return StructureDefinitionKind.DATATYPE; 157 if ("resource".equals(codeString)) 158 return StructureDefinitionKind.RESOURCE; 159 if ("logical".equals(codeString)) 160 return StructureDefinitionKind.LOGICAL; 161 throw new IllegalArgumentException("Unknown StructureDefinitionKind code '" + codeString + "'"); 162 } 163 164 public Enumeration<StructureDefinitionKind> fromType(Base code) throws FHIRException { 165 if (code == null || code.isEmpty()) 166 return null; 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("datatype".equals(codeString)) 171 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.DATATYPE); 172 if ("resource".equals(codeString)) 173 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.RESOURCE); 174 if ("logical".equals(codeString)) 175 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.LOGICAL); 176 throw new FHIRException("Unknown StructureDefinitionKind code '" + codeString + "'"); 177 } 178 179 public String toCode(StructureDefinitionKind code) 180 { 181 if (code == StructureDefinitionKind.NULL) 182 return null; 183 if (code == StructureDefinitionKind.DATATYPE) 184 return "datatype"; 185 if (code == StructureDefinitionKind.RESOURCE) 186 return "resource"; 187 if (code == StructureDefinitionKind.LOGICAL) 188 return "logical"; 189 return "?"; 190 } 191 } 192 193 public enum ExtensionContext { 194 /** 195 * The context is all elements matching a particular resource element path. 196 */ 197 RESOURCE, 198 /** 199 * The context is all nodes matching a particular data type element path (root 200 * or repeating element) or all elements referencing a particular primitive data 201 * type (expressed as the datatype name). 202 */ 203 DATATYPE, 204 /** 205 * The context is all nodes whose mapping to a specified reference model 206 * corresponds to a particular mapping structure. The context identifies the 207 * mapping target. The mapping should clearly identify where such an extension 208 * could be used. 209 */ 210 MAPPING, 211 /** 212 * The context is a particular extension from a particular profile, a uri that 213 * identifies the extension definition. 214 */ 215 EXTENSION, 216 /** 217 * added to help the parsers 218 */ 219 NULL; 220 221 public static ExtensionContext fromCode(String codeString) throws FHIRException { 222 if (codeString == null || "".equals(codeString)) 223 return null; 224 if ("resource".equals(codeString)) 225 return RESOURCE; 226 if ("datatype".equals(codeString)) 227 return DATATYPE; 228 if ("mapping".equals(codeString)) 229 return MAPPING; 230 if ("extension".equals(codeString)) 231 return EXTENSION; 232 throw new FHIRException("Unknown ExtensionContext code '" + codeString + "'"); 233 } 234 235 public String toCode() { 236 switch (this) { 237 case RESOURCE: 238 return "resource"; 239 case DATATYPE: 240 return "datatype"; 241 case MAPPING: 242 return "mapping"; 243 case EXTENSION: 244 return "extension"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getSystem() { 253 switch (this) { 254 case RESOURCE: 255 return "http://hl7.org/fhir/extension-context"; 256 case DATATYPE: 257 return "http://hl7.org/fhir/extension-context"; 258 case MAPPING: 259 return "http://hl7.org/fhir/extension-context"; 260 case EXTENSION: 261 return "http://hl7.org/fhir/extension-context"; 262 case NULL: 263 return null; 264 default: 265 return "?"; 266 } 267 } 268 269 public String getDefinition() { 270 switch (this) { 271 case RESOURCE: 272 return "The context is all elements matching a particular resource element path."; 273 case DATATYPE: 274 return "The context is all nodes matching a particular data type element path (root or repeating element) or all elements referencing a particular primitive data type (expressed as the datatype name)."; 275 case MAPPING: 276 return "The context is all nodes whose mapping to a specified reference model corresponds to a particular mapping structure. The context identifies the mapping target. The mapping should clearly identify where such an extension could be used."; 277 case EXTENSION: 278 return "The context is a particular extension from a particular profile, a uri that identifies the extension definition."; 279 case NULL: 280 return null; 281 default: 282 return "?"; 283 } 284 } 285 286 public String getDisplay() { 287 switch (this) { 288 case RESOURCE: 289 return "Resource"; 290 case DATATYPE: 291 return "Datatype"; 292 case MAPPING: 293 return "Mapping"; 294 case EXTENSION: 295 return "Extension"; 296 case NULL: 297 return null; 298 default: 299 return "?"; 300 } 301 } 302 } 303 304 public static class ExtensionContextEnumFactory implements EnumFactory<ExtensionContext> { 305 public ExtensionContext fromCode(String codeString) throws IllegalArgumentException { 306 if (codeString == null || "".equals(codeString)) 307 if (codeString == null || "".equals(codeString)) 308 return null; 309 if ("resource".equals(codeString)) 310 return ExtensionContext.RESOURCE; 311 if ("datatype".equals(codeString)) 312 return ExtensionContext.DATATYPE; 313 if ("mapping".equals(codeString)) 314 return ExtensionContext.MAPPING; 315 if ("extension".equals(codeString)) 316 return ExtensionContext.EXTENSION; 317 throw new IllegalArgumentException("Unknown ExtensionContext code '" + codeString + "'"); 318 } 319 320 public Enumeration<ExtensionContext> fromType(Base code) throws FHIRException { 321 if (code == null || code.isEmpty()) 322 return null; 323 String codeString = ((PrimitiveType) code).asStringValue(); 324 if (codeString == null || "".equals(codeString)) 325 return null; 326 if ("resource".equals(codeString)) 327 return new Enumeration<ExtensionContext>(this, ExtensionContext.RESOURCE); 328 if ("datatype".equals(codeString)) 329 return new Enumeration<ExtensionContext>(this, ExtensionContext.DATATYPE); 330 if ("mapping".equals(codeString)) 331 return new Enumeration<ExtensionContext>(this, ExtensionContext.MAPPING); 332 if ("extension".equals(codeString)) 333 return new Enumeration<ExtensionContext>(this, ExtensionContext.EXTENSION); 334 throw new FHIRException("Unknown ExtensionContext code '" + codeString + "'"); 335 } 336 337 public String toCode(ExtensionContext code) 338 { 339 if (code == ExtensionContext.NULL) 340 return null; 341 if (code == ExtensionContext.RESOURCE) 342 return "resource"; 343 if (code == ExtensionContext.DATATYPE) 344 return "datatype"; 345 if (code == ExtensionContext.MAPPING) 346 return "mapping"; 347 if (code == ExtensionContext.EXTENSION) 348 return "extension"; 349 return "?"; 350 } 351 } 352 353 @Block() 354 public static class StructureDefinitionContactComponent extends BackboneElement implements IBaseBackboneElement { 355 /** 356 * The name of an individual to contact regarding the structure definition. 357 */ 358 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 359 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the structure definition.") 360 protected StringType name; 361 362 /** 363 * Contact details for individual (if a name was provided) or the publisher. 364 */ 365 @Child(name = "telecom", type = { 366 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 367 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 368 protected List<ContactPoint> telecom; 369 370 private static final long serialVersionUID = -1179697803L; 371 372 /* 373 * Constructor 374 */ 375 public StructureDefinitionContactComponent() { 376 super(); 377 } 378 379 /** 380 * @return {@link #name} (The name of an individual to contact regarding the 381 * structure definition.). This is the underlying object with id, value 382 * and extensions. The accessor "getName" gives direct access to the 383 * value 384 */ 385 public StringType getNameElement() { 386 if (this.name == null) 387 if (Configuration.errorOnAutoCreate()) 388 throw new Error("Attempt to auto-create StructureDefinitionContactComponent.name"); 389 else if (Configuration.doAutoCreate()) 390 this.name = new StringType(); // bb 391 return this.name; 392 } 393 394 public boolean hasNameElement() { 395 return this.name != null && !this.name.isEmpty(); 396 } 397 398 public boolean hasName() { 399 return this.name != null && !this.name.isEmpty(); 400 } 401 402 /** 403 * @param value {@link #name} (The name of an individual to contact regarding 404 * the structure definition.). This is the underlying object with 405 * id, value and extensions. The accessor "getName" gives direct 406 * access to the value 407 */ 408 public StructureDefinitionContactComponent setNameElement(StringType value) { 409 this.name = value; 410 return this; 411 } 412 413 /** 414 * @return The name of an individual to contact regarding the structure 415 * definition. 416 */ 417 public String getName() { 418 return this.name == null ? null : this.name.getValue(); 419 } 420 421 /** 422 * @param value The name of an individual to contact regarding the structure 423 * definition. 424 */ 425 public StructureDefinitionContactComponent setName(String value) { 426 if (Utilities.noString(value)) 427 this.name = null; 428 else { 429 if (this.name == null) 430 this.name = new StringType(); 431 this.name.setValue(value); 432 } 433 return this; 434 } 435 436 /** 437 * @return {@link #telecom} (Contact details for individual (if a name was 438 * provided) or the publisher.) 439 */ 440 public List<ContactPoint> getTelecom() { 441 if (this.telecom == null) 442 this.telecom = new ArrayList<ContactPoint>(); 443 return this.telecom; 444 } 445 446 public boolean hasTelecom() { 447 if (this.telecom == null) 448 return false; 449 for (ContactPoint item : this.telecom) 450 if (!item.isEmpty()) 451 return true; 452 return false; 453 } 454 455 /** 456 * @return {@link #telecom} (Contact details for individual (if a name was 457 * provided) or the publisher.) 458 */ 459 // syntactic sugar 460 public ContactPoint addTelecom() { // 3 461 ContactPoint t = new ContactPoint(); 462 if (this.telecom == null) 463 this.telecom = new ArrayList<ContactPoint>(); 464 this.telecom.add(t); 465 return t; 466 } 467 468 // syntactic sugar 469 public StructureDefinitionContactComponent addTelecom(ContactPoint t) { // 3 470 if (t == null) 471 return this; 472 if (this.telecom == null) 473 this.telecom = new ArrayList<ContactPoint>(); 474 this.telecom.add(t); 475 return this; 476 } 477 478 protected void listChildren(List<Property> childrenList) { 479 super.listChildren(childrenList); 480 childrenList.add( 481 new Property("name", "string", "The name of an individual to contact regarding the structure definition.", 0, 482 java.lang.Integer.MAX_VALUE, name)); 483 childrenList.add(new Property("telecom", "ContactPoint", 484 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 485 telecom)); 486 } 487 488 @Override 489 public void setProperty(String name, Base value) throws FHIRException { 490 if (name.equals("name")) 491 this.name = castToString(value); // StringType 492 else if (name.equals("telecom")) 493 this.getTelecom().add(castToContactPoint(value)); 494 else 495 super.setProperty(name, value); 496 } 497 498 @Override 499 public Base addChild(String name) throws FHIRException { 500 if (name.equals("name")) { 501 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 502 } else if (name.equals("telecom")) { 503 return addTelecom(); 504 } else 505 return super.addChild(name); 506 } 507 508 public StructureDefinitionContactComponent copy() { 509 StructureDefinitionContactComponent dst = new StructureDefinitionContactComponent(); 510 copyValues(dst); 511 dst.name = name == null ? null : name.copy(); 512 if (telecom != null) { 513 dst.telecom = new ArrayList<ContactPoint>(); 514 for (ContactPoint i : telecom) 515 dst.telecom.add(i.copy()); 516 } 517 ; 518 return dst; 519 } 520 521 @Override 522 public boolean equalsDeep(Base other) { 523 if (!super.equalsDeep(other)) 524 return false; 525 if (!(other instanceof StructureDefinitionContactComponent)) 526 return false; 527 StructureDefinitionContactComponent o = (StructureDefinitionContactComponent) other; 528 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 529 } 530 531 @Override 532 public boolean equalsShallow(Base other) { 533 if (!super.equalsShallow(other)) 534 return false; 535 if (!(other instanceof StructureDefinitionContactComponent)) 536 return false; 537 StructureDefinitionContactComponent o = (StructureDefinitionContactComponent) other; 538 return compareValues(name, o.name, true); 539 } 540 541 public boolean isEmpty() { 542 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 543 } 544 545 public String fhirType() { 546 return "StructureDefinition.contact"; 547 548 } 549 550 } 551 552 @Block() 553 public static class StructureDefinitionMappingComponent extends BackboneElement implements IBaseBackboneElement { 554 /** 555 * An Internal id that is used to identify this mapping set when specific 556 * mappings are made. 557 */ 558 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 559 @Description(shortDefinition = "Internal id when this mapping is used", formalDefinition = "An Internal id that is used to identify this mapping set when specific mappings are made.") 560 protected IdType identity; 561 562 /** 563 * An absolute URI that identifies the specification that this mapping is 564 * expressed to. 565 */ 566 @Child(name = "uri", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 567 @Description(shortDefinition = "Identifies what this mapping refers to", formalDefinition = "An absolute URI that identifies the specification that this mapping is expressed to.") 568 protected UriType uri; 569 570 /** 571 * A name for the specification that is being mapped to. 572 */ 573 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 574 @Description(shortDefinition = "Names what this mapping refers to", formalDefinition = "A name for the specification that is being mapped to.") 575 protected StringType name; 576 577 /** 578 * Comments about this mapping, including version notes, issues, scope 579 * limitations, and other important notes for usage. 580 */ 581 @Child(name = "comments", type = { 582 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 583 @Description(shortDefinition = "Versions, Issues, Scope limitations etc.", formalDefinition = "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.") 584 protected StringType comments; 585 586 private static final long serialVersionUID = 299630820L; 587 588 /* 589 * Constructor 590 */ 591 public StructureDefinitionMappingComponent() { 592 super(); 593 } 594 595 /* 596 * Constructor 597 */ 598 public StructureDefinitionMappingComponent(IdType identity) { 599 super(); 600 this.identity = identity; 601 } 602 603 /** 604 * @return {@link #identity} (An Internal id that is used to identify this 605 * mapping set when specific mappings are made.). This is the underlying 606 * object with id, value and extensions. The accessor "getIdentity" 607 * gives direct access to the value 608 */ 609 public IdType getIdentityElement() { 610 if (this.identity == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.identity"); 613 else if (Configuration.doAutoCreate()) 614 this.identity = new IdType(); // bb 615 return this.identity; 616 } 617 618 public boolean hasIdentityElement() { 619 return this.identity != null && !this.identity.isEmpty(); 620 } 621 622 public boolean hasIdentity() { 623 return this.identity != null && !this.identity.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #identity} (An Internal id that is used to identify this 628 * mapping set when specific mappings are made.). This is the 629 * underlying object with id, value and extensions. The accessor 630 * "getIdentity" gives direct access to the value 631 */ 632 public StructureDefinitionMappingComponent setIdentityElement(IdType value) { 633 this.identity = value; 634 return this; 635 } 636 637 /** 638 * @return An Internal id that is used to identify this mapping set when 639 * specific mappings are made. 640 */ 641 public String getIdentity() { 642 return this.identity == null ? null : this.identity.getValue(); 643 } 644 645 /** 646 * @param value An Internal id that is used to identify this mapping set when 647 * specific mappings are made. 648 */ 649 public StructureDefinitionMappingComponent setIdentity(String value) { 650 if (this.identity == null) 651 this.identity = new IdType(); 652 this.identity.setValue(value); 653 return this; 654 } 655 656 /** 657 * @return {@link #uri} (An absolute URI that identifies the specification that 658 * this mapping is expressed to.). This is the underlying object with 659 * id, value and extensions. The accessor "getUri" gives direct access 660 * to the value 661 */ 662 public UriType getUriElement() { 663 if (this.uri == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.uri"); 666 else if (Configuration.doAutoCreate()) 667 this.uri = new UriType(); // bb 668 return this.uri; 669 } 670 671 public boolean hasUriElement() { 672 return this.uri != null && !this.uri.isEmpty(); 673 } 674 675 public boolean hasUri() { 676 return this.uri != null && !this.uri.isEmpty(); 677 } 678 679 /** 680 * @param value {@link #uri} (An absolute URI that identifies the specification 681 * that this mapping is expressed to.). This is the underlying 682 * object with id, value and extensions. The accessor "getUri" 683 * gives direct access to the value 684 */ 685 public StructureDefinitionMappingComponent setUriElement(UriType value) { 686 this.uri = value; 687 return this; 688 } 689 690 /** 691 * @return An absolute URI that identifies the specification that this mapping 692 * is expressed to. 693 */ 694 public String getUri() { 695 return this.uri == null ? null : this.uri.getValue(); 696 } 697 698 /** 699 * @param value An absolute URI that identifies the specification that this 700 * mapping is expressed to. 701 */ 702 public StructureDefinitionMappingComponent setUri(String value) { 703 if (Utilities.noString(value)) 704 this.uri = null; 705 else { 706 if (this.uri == null) 707 this.uri = new UriType(); 708 this.uri.setValue(value); 709 } 710 return this; 711 } 712 713 /** 714 * @return {@link #name} (A name for the specification that is being mapped 715 * to.). This is the underlying object with id, value and extensions. 716 * The accessor "getName" gives direct access to the value 717 */ 718 public StringType getNameElement() { 719 if (this.name == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.name"); 722 else if (Configuration.doAutoCreate()) 723 this.name = new StringType(); // bb 724 return this.name; 725 } 726 727 public boolean hasNameElement() { 728 return this.name != null && !this.name.isEmpty(); 729 } 730 731 public boolean hasName() { 732 return this.name != null && !this.name.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #name} (A name for the specification that is being mapped 737 * to.). This is the underlying object with id, value and 738 * extensions. The accessor "getName" gives direct access to the 739 * value 740 */ 741 public StructureDefinitionMappingComponent setNameElement(StringType value) { 742 this.name = value; 743 return this; 744 } 745 746 /** 747 * @return A name for the specification that is being mapped to. 748 */ 749 public String getName() { 750 return this.name == null ? null : this.name.getValue(); 751 } 752 753 /** 754 * @param value A name for the specification that is being mapped to. 755 */ 756 public StructureDefinitionMappingComponent setName(String value) { 757 if (Utilities.noString(value)) 758 this.name = null; 759 else { 760 if (this.name == null) 761 this.name = new StringType(); 762 this.name.setValue(value); 763 } 764 return this; 765 } 766 767 /** 768 * @return {@link #comments} (Comments about this mapping, including version 769 * notes, issues, scope limitations, and other important notes for 770 * usage.). This is the underlying object with id, value and extensions. 771 * The accessor "getComments" gives direct access to the value 772 */ 773 public StringType getCommentsElement() { 774 if (this.comments == null) 775 if (Configuration.errorOnAutoCreate()) 776 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.comments"); 777 else if (Configuration.doAutoCreate()) 778 this.comments = new StringType(); // bb 779 return this.comments; 780 } 781 782 public boolean hasCommentsElement() { 783 return this.comments != null && !this.comments.isEmpty(); 784 } 785 786 public boolean hasComments() { 787 return this.comments != null && !this.comments.isEmpty(); 788 } 789 790 /** 791 * @param value {@link #comments} (Comments about this mapping, including 792 * version notes, issues, scope limitations, and other important 793 * notes for usage.). This is the underlying object with id, value 794 * and extensions. The accessor "getComments" gives direct access 795 * to the value 796 */ 797 public StructureDefinitionMappingComponent setCommentsElement(StringType value) { 798 this.comments = value; 799 return this; 800 } 801 802 /** 803 * @return Comments about this mapping, including version notes, issues, scope 804 * limitations, and other important notes for usage. 805 */ 806 public String getComments() { 807 return this.comments == null ? null : this.comments.getValue(); 808 } 809 810 /** 811 * @param value Comments about this mapping, including version notes, issues, 812 * scope limitations, and other important notes for usage. 813 */ 814 public StructureDefinitionMappingComponent setComments(String value) { 815 if (Utilities.noString(value)) 816 this.comments = null; 817 else { 818 if (this.comments == null) 819 this.comments = new StringType(); 820 this.comments.setValue(value); 821 } 822 return this; 823 } 824 825 protected void listChildren(List<Property> childrenList) { 826 super.listChildren(childrenList); 827 childrenList.add(new Property("identity", "id", 828 "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 829 java.lang.Integer.MAX_VALUE, identity)); 830 childrenList.add(new Property("uri", "uri", 831 "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 832 java.lang.Integer.MAX_VALUE, uri)); 833 childrenList.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 834 java.lang.Integer.MAX_VALUE, name)); 835 childrenList.add(new Property("comments", "string", 836 "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 837 0, java.lang.Integer.MAX_VALUE, comments)); 838 } 839 840 @Override 841 public void setProperty(String name, Base value) throws FHIRException { 842 if (name.equals("identity")) 843 this.identity = castToId(value); // IdType 844 else if (name.equals("uri")) 845 this.uri = castToUri(value); // UriType 846 else if (name.equals("name")) 847 this.name = castToString(value); // StringType 848 else if (name.equals("comments")) 849 this.comments = castToString(value); // StringType 850 else 851 super.setProperty(name, value); 852 } 853 854 @Override 855 public Base addChild(String name) throws FHIRException { 856 if (name.equals("identity")) { 857 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.identity"); 858 } else if (name.equals("uri")) { 859 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.uri"); 860 } else if (name.equals("name")) { 861 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 862 } else if (name.equals("comments")) { 863 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.comments"); 864 } else 865 return super.addChild(name); 866 } 867 868 public StructureDefinitionMappingComponent copy() { 869 StructureDefinitionMappingComponent dst = new StructureDefinitionMappingComponent(); 870 copyValues(dst); 871 dst.identity = identity == null ? null : identity.copy(); 872 dst.uri = uri == null ? null : uri.copy(); 873 dst.name = name == null ? null : name.copy(); 874 dst.comments = comments == null ? null : comments.copy(); 875 return dst; 876 } 877 878 @Override 879 public boolean equalsDeep(Base other) { 880 if (!super.equalsDeep(other)) 881 return false; 882 if (!(other instanceof StructureDefinitionMappingComponent)) 883 return false; 884 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other; 885 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 886 && compareDeep(comments, o.comments, true); 887 } 888 889 @Override 890 public boolean equalsShallow(Base other) { 891 if (!super.equalsShallow(other)) 892 return false; 893 if (!(other instanceof StructureDefinitionMappingComponent)) 894 return false; 895 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other; 896 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) 897 && compareValues(name, o.name, true) && compareValues(comments, o.comments, true); 898 } 899 900 public boolean isEmpty() { 901 return super.isEmpty() && (identity == null || identity.isEmpty()) && (uri == null || uri.isEmpty()) 902 && (name == null || name.isEmpty()) && (comments == null || comments.isEmpty()); 903 } 904 905 public String fhirType() { 906 return "StructureDefinition.mapping"; 907 908 } 909 910 } 911 912 @Block() 913 public static class StructureDefinitionSnapshotComponent extends BackboneElement implements IBaseBackboneElement { 914 /** 915 * Captures constraints on each element within the resource. 916 */ 917 @Child(name = "element", type = { 918 ElementDefinition.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 919 @Description(shortDefinition = "Definition of elements in the resource (if no StructureDefinition)", formalDefinition = "Captures constraints on each element within the resource.") 920 protected List<ElementDefinition> element; 921 922 private static final long serialVersionUID = 53896641L; 923 924 /* 925 * Constructor 926 */ 927 public StructureDefinitionSnapshotComponent() { 928 super(); 929 } 930 931 /** 932 * @return {@link #element} (Captures constraints on each element within the 933 * resource.) 934 */ 935 public List<ElementDefinition> getElement() { 936 if (this.element == null) 937 this.element = new ArrayList<ElementDefinition>(); 938 return this.element; 939 } 940 941 public boolean hasElement() { 942 if (this.element == null) 943 return false; 944 for (ElementDefinition item : this.element) 945 if (!item.isEmpty()) 946 return true; 947 return false; 948 } 949 950 /** 951 * @return {@link #element} (Captures constraints on each element within the 952 * resource.) 953 */ 954 // syntactic sugar 955 public ElementDefinition addElement() { // 3 956 ElementDefinition t = new ElementDefinition(); 957 if (this.element == null) 958 this.element = new ArrayList<ElementDefinition>(); 959 this.element.add(t); 960 return t; 961 } 962 963 // syntactic sugar 964 public StructureDefinitionSnapshotComponent addElement(ElementDefinition t) { // 3 965 if (t == null) 966 return this; 967 if (this.element == null) 968 this.element = new ArrayList<ElementDefinition>(); 969 this.element.add(t); 970 return this; 971 } 972 973 protected void listChildren(List<Property> childrenList) { 974 super.listChildren(childrenList); 975 childrenList.add(new Property("element", "ElementDefinition", 976 "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 977 } 978 979 @Override 980 public void setProperty(String name, Base value) throws FHIRException { 981 if (name.equals("element")) 982 this.getElement().add(castToElementDefinition(value)); 983 else 984 super.setProperty(name, value); 985 } 986 987 @Override 988 public Base addChild(String name) throws FHIRException { 989 if (name.equals("element")) { 990 return addElement(); 991 } else 992 return super.addChild(name); 993 } 994 995 public StructureDefinitionSnapshotComponent copy() { 996 StructureDefinitionSnapshotComponent dst = new StructureDefinitionSnapshotComponent(); 997 copyValues(dst); 998 if (element != null) { 999 dst.element = new ArrayList<ElementDefinition>(); 1000 for (ElementDefinition i : element) 1001 dst.element.add(i.copy()); 1002 } 1003 ; 1004 return dst; 1005 } 1006 1007 @Override 1008 public boolean equalsDeep(Base other) { 1009 if (!super.equalsDeep(other)) 1010 return false; 1011 if (!(other instanceof StructureDefinitionSnapshotComponent)) 1012 return false; 1013 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other; 1014 return compareDeep(element, o.element, true); 1015 } 1016 1017 @Override 1018 public boolean equalsShallow(Base other) { 1019 if (!super.equalsShallow(other)) 1020 return false; 1021 if (!(other instanceof StructureDefinitionSnapshotComponent)) 1022 return false; 1023 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other; 1024 return true; 1025 } 1026 1027 public boolean isEmpty() { 1028 return super.isEmpty() && (element == null || element.isEmpty()); 1029 } 1030 1031 public String fhirType() { 1032 return "StructureDefinition.snapshot"; 1033 1034 } 1035 1036 } 1037 1038 @Block() 1039 public static class StructureDefinitionDifferentialComponent extends BackboneElement implements IBaseBackboneElement { 1040 /** 1041 * Captures constraints on each element within the resource. 1042 */ 1043 @Child(name = "element", type = { 1044 ElementDefinition.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1045 @Description(shortDefinition = "Definition of elements in the resource (if no StructureDefinition)", formalDefinition = "Captures constraints on each element within the resource.") 1046 protected List<ElementDefinition> element; 1047 1048 private static final long serialVersionUID = 53896641L; 1049 1050 /* 1051 * Constructor 1052 */ 1053 public StructureDefinitionDifferentialComponent() { 1054 super(); 1055 } 1056 1057 /** 1058 * @return {@link #element} (Captures constraints on each element within the 1059 * resource.) 1060 */ 1061 public List<ElementDefinition> getElement() { 1062 if (this.element == null) 1063 this.element = new ArrayList<ElementDefinition>(); 1064 return this.element; 1065 } 1066 1067 public boolean hasElement() { 1068 if (this.element == null) 1069 return false; 1070 for (ElementDefinition item : this.element) 1071 if (!item.isEmpty()) 1072 return true; 1073 return false; 1074 } 1075 1076 /** 1077 * @return {@link #element} (Captures constraints on each element within the 1078 * resource.) 1079 */ 1080 // syntactic sugar 1081 public ElementDefinition addElement() { // 3 1082 ElementDefinition t = new ElementDefinition(); 1083 if (this.element == null) 1084 this.element = new ArrayList<ElementDefinition>(); 1085 this.element.add(t); 1086 return t; 1087 } 1088 1089 // syntactic sugar 1090 public StructureDefinitionDifferentialComponent addElement(ElementDefinition t) { // 3 1091 if (t == null) 1092 return this; 1093 if (this.element == null) 1094 this.element = new ArrayList<ElementDefinition>(); 1095 this.element.add(t); 1096 return this; 1097 } 1098 1099 protected void listChildren(List<Property> childrenList) { 1100 super.listChildren(childrenList); 1101 childrenList.add(new Property("element", "ElementDefinition", 1102 "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1103 } 1104 1105 @Override 1106 public void setProperty(String name, Base value) throws FHIRException { 1107 if (name.equals("element")) 1108 this.getElement().add(castToElementDefinition(value)); 1109 else 1110 super.setProperty(name, value); 1111 } 1112 1113 @Override 1114 public Base addChild(String name) throws FHIRException { 1115 if (name.equals("element")) { 1116 return addElement(); 1117 } else 1118 return super.addChild(name); 1119 } 1120 1121 public StructureDefinitionDifferentialComponent copy() { 1122 StructureDefinitionDifferentialComponent dst = new StructureDefinitionDifferentialComponent(); 1123 copyValues(dst); 1124 if (element != null) { 1125 dst.element = new ArrayList<ElementDefinition>(); 1126 for (ElementDefinition i : element) 1127 dst.element.add(i.copy()); 1128 } 1129 ; 1130 return dst; 1131 } 1132 1133 @Override 1134 public boolean equalsDeep(Base other) { 1135 if (!super.equalsDeep(other)) 1136 return false; 1137 if (!(other instanceof StructureDefinitionDifferentialComponent)) 1138 return false; 1139 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other; 1140 return compareDeep(element, o.element, true); 1141 } 1142 1143 @Override 1144 public boolean equalsShallow(Base other) { 1145 if (!super.equalsShallow(other)) 1146 return false; 1147 if (!(other instanceof StructureDefinitionDifferentialComponent)) 1148 return false; 1149 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other; 1150 return true; 1151 } 1152 1153 public boolean isEmpty() { 1154 return super.isEmpty() && (element == null || element.isEmpty()); 1155 } 1156 1157 public String fhirType() { 1158 return "StructureDefinition.differential"; 1159 1160 } 1161 1162 } 1163 1164 /** 1165 * An absolute URL that is used to identify this structure definition when it is 1166 * referenced in a specification, model, design or an instance. This SHALL be a 1167 * URL, SHOULD be globally unique, and SHOULD be an address at which this 1168 * structure definition is (or will be) published. 1169 */ 1170 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1171 @Description(shortDefinition = "Absolute URL used to reference this StructureDefinition", formalDefinition = "An absolute URL that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published.") 1172 protected UriType url; 1173 1174 /** 1175 * Formal identifier that is used to identify this StructureDefinition when it 1176 * is represented in other formats, or referenced in a specification, model, 1177 * design or an instance (should be globally unique OID, UUID, or URI), (if it's 1178 * not possible to use the literal URI). 1179 */ 1180 @Child(name = "identifier", type = { 1181 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1182 @Description(shortDefinition = "Other identifiers for the StructureDefinition", formalDefinition = "Formal identifier that is used to identify this StructureDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance (should be globally unique OID, UUID, or URI), (if it's not possible to use the literal URI).") 1183 protected List<Identifier> identifier; 1184 1185 /** 1186 * The identifier that is used to identify this version of the 1187 * StructureDefinition when it is referenced in a specification, model, design 1188 * or instance. This is an arbitrary value managed by the StructureDefinition 1189 * author manually. 1190 */ 1191 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1192 @Description(shortDefinition = "Logical id for this version of the StructureDefinition", formalDefinition = "The identifier that is used to identify this version of the StructureDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the StructureDefinition author manually.") 1193 protected StringType version; 1194 1195 /** 1196 * A free text natural language name identifying the StructureDefinition. 1197 */ 1198 @Child(name = "name", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1199 @Description(shortDefinition = "Informal name for this StructureDefinition", formalDefinition = "A free text natural language name identifying the StructureDefinition.") 1200 protected StringType name; 1201 1202 /** 1203 * Defined so that applications can use this name when displaying the value of 1204 * the extension to the user. 1205 */ 1206 @Child(name = "display", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1207 @Description(shortDefinition = "Use this name when displaying the value", formalDefinition = "Defined so that applications can use this name when displaying the value of the extension to the user.") 1208 protected StringType display; 1209 1210 /** 1211 * The status of the StructureDefinition. 1212 */ 1213 @Child(name = "status", type = { CodeType.class }, order = 5, min = 1, max = 1, modifier = true, summary = true) 1214 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the StructureDefinition.") 1215 protected Enumeration<ConformanceResourceStatus> status; 1216 1217 /** 1218 * This StructureDefinition was authored for testing purposes (or 1219 * education/evaluation/marketing), and is not intended to be used for genuine 1220 * usage. 1221 */ 1222 @Child(name = "experimental", type = { 1223 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1224 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "This StructureDefinition was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 1225 protected BooleanType experimental; 1226 1227 /** 1228 * The name of the individual or organization that published the structure 1229 * definition. 1230 */ 1231 @Child(name = "publisher", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1232 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the structure definition.") 1233 protected StringType publisher; 1234 1235 /** 1236 * Contacts to assist a user in finding and communicating with the publisher. 1237 */ 1238 @Child(name = "contact", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1239 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 1240 protected List<StructureDefinitionContactComponent> contact; 1241 1242 /** 1243 * The date this version of the structure definition was published. The date 1244 * must change when the business version changes, if it does, and it must change 1245 * if the status code changes. In addition, it should change when the 1246 * substantive content of the structure definition changes. 1247 */ 1248 @Child(name = "date", type = { DateTimeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1249 @Description(shortDefinition = "Date for this version of the StructureDefinition", formalDefinition = "The date this version of the structure definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.") 1250 protected DateTimeType date; 1251 1252 /** 1253 * A free text natural language description of the StructureDefinition and its 1254 * use. 1255 */ 1256 @Child(name = "description", type = { 1257 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1258 @Description(shortDefinition = "Natural language description of the StructureDefinition", formalDefinition = "A free text natural language description of the StructureDefinition and its use.") 1259 protected StringType description; 1260 1261 /** 1262 * The content was developed with a focus and intent of supporting the contexts 1263 * that are listed. These terms may be used to assist with indexing and 1264 * searching of structure definitions. 1265 */ 1266 @Child(name = "useContext", type = { 1267 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1268 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of structure definitions.") 1269 protected List<CodeableConcept> useContext; 1270 1271 /** 1272 * Explains why this structure definition is needed and why it's been 1273 * constrained as it has. 1274 */ 1275 @Child(name = "requirements", type = { 1276 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1277 @Description(shortDefinition = "Scope and Usage this structure definition is for", formalDefinition = "Explains why this structure definition is needed and why it's been constrained as it has.") 1278 protected StringType requirements; 1279 1280 /** 1281 * A copyright statement relating to the structure definition and/or its 1282 * contents. Copyright statements are generally legal restrictions on the use 1283 * and publishing of the details of the constraints and mappings. 1284 */ 1285 @Child(name = "copyright", type = { 1286 StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1287 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the constraints and mappings.") 1288 protected StringType copyright; 1289 1290 /** 1291 * A set of terms from external terminologies that may be used to assist with 1292 * indexing and searching of templates. 1293 */ 1294 @Child(name = "code", type = { 1295 Coding.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1296 @Description(shortDefinition = "Assist with indexing and finding", formalDefinition = "A set of terms from external terminologies that may be used to assist with indexing and searching of templates.") 1297 protected List<Coding> code; 1298 1299 /** 1300 * The version of the FHIR specification on which this StructureDefinition is 1301 * based - this is the formal version of the specification, without the revision 1302 * number, e.g. [publication].[major].[minor], which is 1.0.2 for this version. 1303 */ 1304 @Child(name = "fhirVersion", type = { IdType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1305 @Description(shortDefinition = "FHIR Version this StructureDefinition targets", formalDefinition = "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 1.0.2 for this version.") 1306 protected IdType fhirVersion; 1307 1308 /** 1309 * An external specification that the content is mapped to. 1310 */ 1311 @Child(name = "mapping", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1312 @Description(shortDefinition = "External specification that the content is mapped to", formalDefinition = "An external specification that the content is mapped to.") 1313 protected List<StructureDefinitionMappingComponent> mapping; 1314 1315 /** 1316 * Defines the kind of structure that this definition is describing. 1317 */ 1318 @Child(name = "kind", type = { CodeType.class }, order = 17, min = 1, max = 1, modifier = false, summary = true) 1319 @Description(shortDefinition = "datatype | resource | logical", formalDefinition = "Defines the kind of structure that this definition is describing.") 1320 protected Enumeration<StructureDefinitionKind> kind; 1321 1322 /** 1323 * The type of type that is being constrained - a data type, an extension, a 1324 * resource, including abstract ones. If this field is present, it indicates 1325 * that the structure definition is a constraint. If it is not present, then the 1326 * structure definition is the definition of a base structure. 1327 */ 1328 @Child(name = "constrainedType", type = { 1329 CodeType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 1330 @Description(shortDefinition = "Any datatype or resource, including abstract ones", formalDefinition = "The type of type that is being constrained - a data type, an extension, a resource, including abstract ones. If this field is present, it indicates that the structure definition is a constraint. If it is not present, then the structure definition is the definition of a base structure.") 1331 protected CodeType constrainedType; 1332 1333 /** 1334 * Whether structure this definition describes is abstract or not - that is, 1335 * whether an actual exchanged item can ever be of this type. 1336 */ 1337 @Child(name = "abstract", type = { 1338 BooleanType.class }, order = 19, min = 1, max = 1, modifier = false, summary = true) 1339 @Description(shortDefinition = "Whether the structure is abstract", formalDefinition = "Whether structure this definition describes is abstract or not - that is, whether an actual exchanged item can ever be of this type.") 1340 protected BooleanType abstract_; 1341 1342 /** 1343 * If this is an extension, Identifies the context within FHIR resources where 1344 * the extension can be used. 1345 */ 1346 @Child(name = "contextType", type = { 1347 CodeType.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 1348 @Description(shortDefinition = "resource | datatype | mapping | extension", formalDefinition = "If this is an extension, Identifies the context within FHIR resources where the extension can be used.") 1349 protected Enumeration<ExtensionContext> contextType; 1350 1351 /** 1352 * Identifies the types of resource or data type elements to which the extension 1353 * can be applied. 1354 */ 1355 @Child(name = "context", type = { 1356 StringType.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1357 @Description(shortDefinition = "Where the extension can be used in instances", formalDefinition = "Identifies the types of resource or data type elements to which the extension can be applied.") 1358 protected List<StringType> context; 1359 1360 /** 1361 * An absolute URI that is the base structure from which this set of constraints 1362 * is derived. 1363 */ 1364 @Child(name = "base", type = { UriType.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 1365 @Description(shortDefinition = "Structure that this set of constraints applies to", formalDefinition = "An absolute URI that is the base structure from which this set of constraints is derived.") 1366 protected UriType base; 1367 1368 /** 1369 * A snapshot view is expressed in a stand alone form that can be used and 1370 * interpreted without considering the base StructureDefinition. 1371 */ 1372 @Child(name = "snapshot", type = {}, order = 23, min = 0, max = 1, modifier = false, summary = false) 1373 @Description(shortDefinition = "Snapshot view of the structure", formalDefinition = "A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition.") 1374 protected StructureDefinitionSnapshotComponent snapshot; 1375 1376 /** 1377 * A differential view is expressed relative to the base StructureDefinition - a 1378 * statement of differences that it applies. 1379 */ 1380 @Child(name = "differential", type = {}, order = 24, min = 0, max = 1, modifier = false, summary = false) 1381 @Description(shortDefinition = "Differential view of the structure", formalDefinition = "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.") 1382 protected StructureDefinitionDifferentialComponent differential; 1383 1384 private static final long serialVersionUID = -580779569L; 1385 1386 /* 1387 * Constructor 1388 */ 1389 public StructureDefinition() { 1390 super(); 1391 } 1392 1393 /* 1394 * Constructor 1395 */ 1396 public StructureDefinition(UriType url, StringType name, Enumeration<ConformanceResourceStatus> status, 1397 Enumeration<StructureDefinitionKind> kind, BooleanType abstract_) { 1398 super(); 1399 this.url = url; 1400 this.name = name; 1401 this.status = status; 1402 this.kind = kind; 1403 this.abstract_ = abstract_; 1404 } 1405 1406 /** 1407 * @return {@link #url} (An absolute URL that is used to identify this structure 1408 * definition when it is referenced in a specification, model, design or 1409 * an instance. This SHALL be a URL, SHOULD be globally unique, and 1410 * SHOULD be an address at which this structure definition is (or will 1411 * be) published.). This is the underlying object with id, value and 1412 * extensions. The accessor "getUrl" gives direct access to the value 1413 */ 1414 public UriType getUrlElement() { 1415 if (this.url == null) 1416 if (Configuration.errorOnAutoCreate()) 1417 throw new Error("Attempt to auto-create StructureDefinition.url"); 1418 else if (Configuration.doAutoCreate()) 1419 this.url = new UriType(); // bb 1420 return this.url; 1421 } 1422 1423 public boolean hasUrlElement() { 1424 return this.url != null && !this.url.isEmpty(); 1425 } 1426 1427 public boolean hasUrl() { 1428 return this.url != null && !this.url.isEmpty(); 1429 } 1430 1431 /** 1432 * @param value {@link #url} (An absolute URL that is used to identify this 1433 * structure definition when it is referenced in a specification, 1434 * model, design or an instance. This SHALL be a URL, SHOULD be 1435 * globally unique, and SHOULD be an address at which this 1436 * structure definition is (or will be) published.). This is the 1437 * underlying object with id, value and extensions. The accessor 1438 * "getUrl" gives direct access to the value 1439 */ 1440 public StructureDefinition setUrlElement(UriType value) { 1441 this.url = value; 1442 return this; 1443 } 1444 1445 /** 1446 * @return An absolute URL that is used to identify this structure definition 1447 * when it is referenced in a specification, model, design or an 1448 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 1449 * be an address at which this structure definition is (or will be) 1450 * published. 1451 */ 1452 public String getUrl() { 1453 return this.url == null ? null : this.url.getValue(); 1454 } 1455 1456 /** 1457 * @param value An absolute URL that is used to identify this structure 1458 * definition when it is referenced in a specification, model, 1459 * design or an instance. This SHALL be a URL, SHOULD be globally 1460 * unique, and SHOULD be an address at which this structure 1461 * definition is (or will be) published. 1462 */ 1463 public StructureDefinition setUrl(String value) { 1464 if (this.url == null) 1465 this.url = new UriType(); 1466 this.url.setValue(value); 1467 return this; 1468 } 1469 1470 /** 1471 * @return {@link #identifier} (Formal identifier that is used to identify this 1472 * StructureDefinition when it is represented in other formats, or 1473 * referenced in a specification, model, design or an instance (should 1474 * be globally unique OID, UUID, or URI), (if it's not possible to use 1475 * the literal URI).) 1476 */ 1477 public List<Identifier> getIdentifier() { 1478 if (this.identifier == null) 1479 this.identifier = new ArrayList<Identifier>(); 1480 return this.identifier; 1481 } 1482 1483 public boolean hasIdentifier() { 1484 if (this.identifier == null) 1485 return false; 1486 for (Identifier item : this.identifier) 1487 if (!item.isEmpty()) 1488 return true; 1489 return false; 1490 } 1491 1492 /** 1493 * @return {@link #identifier} (Formal identifier that is used to identify this 1494 * StructureDefinition when it is represented in other formats, or 1495 * referenced in a specification, model, design or an instance (should 1496 * be globally unique OID, UUID, or URI), (if it's not possible to use 1497 * the literal URI).) 1498 */ 1499 // syntactic sugar 1500 public Identifier addIdentifier() { // 3 1501 Identifier t = new Identifier(); 1502 if (this.identifier == null) 1503 this.identifier = new ArrayList<Identifier>(); 1504 this.identifier.add(t); 1505 return t; 1506 } 1507 1508 // syntactic sugar 1509 public StructureDefinition addIdentifier(Identifier t) { // 3 1510 if (t == null) 1511 return this; 1512 if (this.identifier == null) 1513 this.identifier = new ArrayList<Identifier>(); 1514 this.identifier.add(t); 1515 return this; 1516 } 1517 1518 /** 1519 * @return {@link #version} (The identifier that is used to identify this 1520 * version of the StructureDefinition when it is referenced in a 1521 * specification, model, design or instance. This is an arbitrary value 1522 * managed by the StructureDefinition author manually.). This is the 1523 * underlying object with id, value and extensions. The accessor 1524 * "getVersion" gives direct access to the value 1525 */ 1526 public StringType getVersionElement() { 1527 if (this.version == null) 1528 if (Configuration.errorOnAutoCreate()) 1529 throw new Error("Attempt to auto-create StructureDefinition.version"); 1530 else if (Configuration.doAutoCreate()) 1531 this.version = new StringType(); // bb 1532 return this.version; 1533 } 1534 1535 public boolean hasVersionElement() { 1536 return this.version != null && !this.version.isEmpty(); 1537 } 1538 1539 public boolean hasVersion() { 1540 return this.version != null && !this.version.isEmpty(); 1541 } 1542 1543 /** 1544 * @param value {@link #version} (The identifier that is used to identify this 1545 * version of the StructureDefinition when it is referenced in a 1546 * specification, model, design or instance. This is an arbitrary 1547 * value managed by the StructureDefinition author manually.). This 1548 * is the underlying object with id, value and extensions. The 1549 * accessor "getVersion" gives direct access to the value 1550 */ 1551 public StructureDefinition setVersionElement(StringType value) { 1552 this.version = value; 1553 return this; 1554 } 1555 1556 /** 1557 * @return The identifier that is used to identify this version of the 1558 * StructureDefinition when it is referenced in a specification, model, 1559 * design or instance. This is an arbitrary value managed by the 1560 * StructureDefinition author manually. 1561 */ 1562 public String getVersion() { 1563 return this.version == null ? null : this.version.getValue(); 1564 } 1565 1566 /** 1567 * @param value The identifier that is used to identify this version of the 1568 * StructureDefinition when it is referenced in a specification, 1569 * model, design or instance. This is an arbitrary value managed by 1570 * the StructureDefinition author manually. 1571 */ 1572 public StructureDefinition setVersion(String value) { 1573 if (Utilities.noString(value)) 1574 this.version = null; 1575 else { 1576 if (this.version == null) 1577 this.version = new StringType(); 1578 this.version.setValue(value); 1579 } 1580 return this; 1581 } 1582 1583 /** 1584 * @return {@link #name} (A free text natural language name identifying the 1585 * StructureDefinition.). This is the underlying object with id, value 1586 * and extensions. The accessor "getName" gives direct access to the 1587 * value 1588 */ 1589 public StringType getNameElement() { 1590 if (this.name == null) 1591 if (Configuration.errorOnAutoCreate()) 1592 throw new Error("Attempt to auto-create StructureDefinition.name"); 1593 else if (Configuration.doAutoCreate()) 1594 this.name = new StringType(); // bb 1595 return this.name; 1596 } 1597 1598 public boolean hasNameElement() { 1599 return this.name != null && !this.name.isEmpty(); 1600 } 1601 1602 public boolean hasName() { 1603 return this.name != null && !this.name.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #name} (A free text natural language name identifying the 1608 * StructureDefinition.). This is the underlying object with id, 1609 * value and extensions. The accessor "getName" gives direct access 1610 * to the value 1611 */ 1612 public StructureDefinition setNameElement(StringType value) { 1613 this.name = value; 1614 return this; 1615 } 1616 1617 /** 1618 * @return A free text natural language name identifying the 1619 * StructureDefinition. 1620 */ 1621 public String getName() { 1622 return this.name == null ? null : this.name.getValue(); 1623 } 1624 1625 /** 1626 * @param value A free text natural language name identifying the 1627 * StructureDefinition. 1628 */ 1629 public StructureDefinition setName(String value) { 1630 if (this.name == null) 1631 this.name = new StringType(); 1632 this.name.setValue(value); 1633 return this; 1634 } 1635 1636 /** 1637 * @return {@link #display} (Defined so that applications can use this name when 1638 * displaying the value of the extension to the user.). This is the 1639 * underlying object with id, value and extensions. The accessor 1640 * "getDisplay" gives direct access to the value 1641 */ 1642 public StringType getDisplayElement() { 1643 if (this.display == null) 1644 if (Configuration.errorOnAutoCreate()) 1645 throw new Error("Attempt to auto-create StructureDefinition.display"); 1646 else if (Configuration.doAutoCreate()) 1647 this.display = new StringType(); // bb 1648 return this.display; 1649 } 1650 1651 public boolean hasDisplayElement() { 1652 return this.display != null && !this.display.isEmpty(); 1653 } 1654 1655 public boolean hasDisplay() { 1656 return this.display != null && !this.display.isEmpty(); 1657 } 1658 1659 /** 1660 * @param value {@link #display} (Defined so that applications can use this name 1661 * when displaying the value of the extension to the user.). This 1662 * is the underlying object with id, value and extensions. The 1663 * accessor "getDisplay" gives direct access to the value 1664 */ 1665 public StructureDefinition setDisplayElement(StringType value) { 1666 this.display = value; 1667 return this; 1668 } 1669 1670 /** 1671 * @return Defined so that applications can use this name when displaying the 1672 * value of the extension to the user. 1673 */ 1674 public String getDisplay() { 1675 return this.display == null ? null : this.display.getValue(); 1676 } 1677 1678 /** 1679 * @param value Defined so that applications can use this name when displaying 1680 * the value of the extension to the user. 1681 */ 1682 public StructureDefinition setDisplay(String value) { 1683 if (Utilities.noString(value)) 1684 this.display = null; 1685 else { 1686 if (this.display == null) 1687 this.display = new StringType(); 1688 this.display.setValue(value); 1689 } 1690 return this; 1691 } 1692 1693 /** 1694 * @return {@link #status} (The status of the StructureDefinition.). This is the 1695 * underlying object with id, value and extensions. The accessor 1696 * "getStatus" gives direct access to the value 1697 */ 1698 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1699 if (this.status == null) 1700 if (Configuration.errorOnAutoCreate()) 1701 throw new Error("Attempt to auto-create StructureDefinition.status"); 1702 else if (Configuration.doAutoCreate()) 1703 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1704 return this.status; 1705 } 1706 1707 public boolean hasStatusElement() { 1708 return this.status != null && !this.status.isEmpty(); 1709 } 1710 1711 public boolean hasStatus() { 1712 return this.status != null && !this.status.isEmpty(); 1713 } 1714 1715 /** 1716 * @param value {@link #status} (The status of the StructureDefinition.). This 1717 * is the underlying object with id, value and extensions. The 1718 * accessor "getStatus" gives direct access to the value 1719 */ 1720 public StructureDefinition setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1721 this.status = value; 1722 return this; 1723 } 1724 1725 /** 1726 * @return The status of the StructureDefinition. 1727 */ 1728 public ConformanceResourceStatus getStatus() { 1729 return this.status == null ? null : this.status.getValue(); 1730 } 1731 1732 /** 1733 * @param value The status of the StructureDefinition. 1734 */ 1735 public StructureDefinition setStatus(ConformanceResourceStatus value) { 1736 if (this.status == null) 1737 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1738 this.status.setValue(value); 1739 return this; 1740 } 1741 1742 /** 1743 * @return {@link #experimental} (This StructureDefinition was authored for 1744 * testing purposes (or education/evaluation/marketing), and is not 1745 * intended to be used for genuine usage.). This is the underlying 1746 * object with id, value and extensions. The accessor "getExperimental" 1747 * gives direct access to the value 1748 */ 1749 public BooleanType getExperimentalElement() { 1750 if (this.experimental == null) 1751 if (Configuration.errorOnAutoCreate()) 1752 throw new Error("Attempt to auto-create StructureDefinition.experimental"); 1753 else if (Configuration.doAutoCreate()) 1754 this.experimental = new BooleanType(); // bb 1755 return this.experimental; 1756 } 1757 1758 public boolean hasExperimentalElement() { 1759 return this.experimental != null && !this.experimental.isEmpty(); 1760 } 1761 1762 public boolean hasExperimental() { 1763 return this.experimental != null && !this.experimental.isEmpty(); 1764 } 1765 1766 /** 1767 * @param value {@link #experimental} (This StructureDefinition was authored for 1768 * testing purposes (or education/evaluation/marketing), and is not 1769 * intended to be used for genuine usage.). This is the underlying 1770 * object with id, value and extensions. The accessor 1771 * "getExperimental" gives direct access to the value 1772 */ 1773 public StructureDefinition setExperimentalElement(BooleanType value) { 1774 this.experimental = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return This StructureDefinition was authored for testing purposes (or 1780 * education/evaluation/marketing), and is not intended to be used for 1781 * genuine usage. 1782 */ 1783 public boolean getExperimental() { 1784 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1785 } 1786 1787 /** 1788 * @param value This StructureDefinition was authored for testing purposes (or 1789 * education/evaluation/marketing), and is not intended to be used 1790 * for genuine usage. 1791 */ 1792 public StructureDefinition setExperimental(boolean value) { 1793 if (this.experimental == null) 1794 this.experimental = new BooleanType(); 1795 this.experimental.setValue(value); 1796 return this; 1797 } 1798 1799 /** 1800 * @return {@link #publisher} (The name of the individual or organization that 1801 * published the structure definition.). This is the underlying object 1802 * with id, value and extensions. The accessor "getPublisher" gives 1803 * direct access to the value 1804 */ 1805 public StringType getPublisherElement() { 1806 if (this.publisher == null) 1807 if (Configuration.errorOnAutoCreate()) 1808 throw new Error("Attempt to auto-create StructureDefinition.publisher"); 1809 else if (Configuration.doAutoCreate()) 1810 this.publisher = new StringType(); // bb 1811 return this.publisher; 1812 } 1813 1814 public boolean hasPublisherElement() { 1815 return this.publisher != null && !this.publisher.isEmpty(); 1816 } 1817 1818 public boolean hasPublisher() { 1819 return this.publisher != null && !this.publisher.isEmpty(); 1820 } 1821 1822 /** 1823 * @param value {@link #publisher} (The name of the individual or organization 1824 * that published the structure definition.). This is the 1825 * underlying object with id, value and extensions. The accessor 1826 * "getPublisher" gives direct access to the value 1827 */ 1828 public StructureDefinition setPublisherElement(StringType value) { 1829 this.publisher = value; 1830 return this; 1831 } 1832 1833 /** 1834 * @return The name of the individual or organization that published the 1835 * structure definition. 1836 */ 1837 public String getPublisher() { 1838 return this.publisher == null ? null : this.publisher.getValue(); 1839 } 1840 1841 /** 1842 * @param value The name of the individual or organization that published the 1843 * structure definition. 1844 */ 1845 public StructureDefinition setPublisher(String value) { 1846 if (Utilities.noString(value)) 1847 this.publisher = null; 1848 else { 1849 if (this.publisher == null) 1850 this.publisher = new StringType(); 1851 this.publisher.setValue(value); 1852 } 1853 return this; 1854 } 1855 1856 /** 1857 * @return {@link #contact} (Contacts to assist a user in finding and 1858 * communicating with the publisher.) 1859 */ 1860 public List<StructureDefinitionContactComponent> getContact() { 1861 if (this.contact == null) 1862 this.contact = new ArrayList<StructureDefinitionContactComponent>(); 1863 return this.contact; 1864 } 1865 1866 public boolean hasContact() { 1867 if (this.contact == null) 1868 return false; 1869 for (StructureDefinitionContactComponent item : this.contact) 1870 if (!item.isEmpty()) 1871 return true; 1872 return false; 1873 } 1874 1875 /** 1876 * @return {@link #contact} (Contacts to assist a user in finding and 1877 * communicating with the publisher.) 1878 */ 1879 // syntactic sugar 1880 public StructureDefinitionContactComponent addContact() { // 3 1881 StructureDefinitionContactComponent t = new StructureDefinitionContactComponent(); 1882 if (this.contact == null) 1883 this.contact = new ArrayList<StructureDefinitionContactComponent>(); 1884 this.contact.add(t); 1885 return t; 1886 } 1887 1888 // syntactic sugar 1889 public StructureDefinition addContact(StructureDefinitionContactComponent t) { // 3 1890 if (t == null) 1891 return this; 1892 if (this.contact == null) 1893 this.contact = new ArrayList<StructureDefinitionContactComponent>(); 1894 this.contact.add(t); 1895 return this; 1896 } 1897 1898 /** 1899 * @return {@link #date} (The date this version of the structure definition was 1900 * published. The date must change when the business version changes, if 1901 * it does, and it must change if the status code changes. In addition, 1902 * it should change when the substantive content of the structure 1903 * definition changes.). This is the underlying object with id, value 1904 * and extensions. The accessor "getDate" gives direct access to the 1905 * value 1906 */ 1907 public DateTimeType getDateElement() { 1908 if (this.date == null) 1909 if (Configuration.errorOnAutoCreate()) 1910 throw new Error("Attempt to auto-create StructureDefinition.date"); 1911 else if (Configuration.doAutoCreate()) 1912 this.date = new DateTimeType(); // bb 1913 return this.date; 1914 } 1915 1916 public boolean hasDateElement() { 1917 return this.date != null && !this.date.isEmpty(); 1918 } 1919 1920 public boolean hasDate() { 1921 return this.date != null && !this.date.isEmpty(); 1922 } 1923 1924 /** 1925 * @param value {@link #date} (The date this version of the structure definition 1926 * was published. The date must change when the business version 1927 * changes, if it does, and it must change if the status code 1928 * changes. In addition, it should change when the substantive 1929 * content of the structure definition changes.). This is the 1930 * underlying object with id, value and extensions. The accessor 1931 * "getDate" gives direct access to the value 1932 */ 1933 public StructureDefinition setDateElement(DateTimeType value) { 1934 this.date = value; 1935 return this; 1936 } 1937 1938 /** 1939 * @return The date this version of the structure definition was published. The 1940 * date must change when the business version changes, if it does, and 1941 * it must change if the status code changes. In addition, it should 1942 * change when the substantive content of the structure definition 1943 * changes. 1944 */ 1945 public Date getDate() { 1946 return this.date == null ? null : this.date.getValue(); 1947 } 1948 1949 /** 1950 * @param value The date this version of the structure definition was published. 1951 * The date must change when the business version changes, if it 1952 * does, and it must change if the status code changes. In 1953 * addition, it should change when the substantive content of the 1954 * structure definition changes. 1955 */ 1956 public StructureDefinition setDate(Date value) { 1957 if (value == null) 1958 this.date = null; 1959 else { 1960 if (this.date == null) 1961 this.date = new DateTimeType(); 1962 this.date.setValue(value); 1963 } 1964 return this; 1965 } 1966 1967 /** 1968 * @return {@link #description} (A free text natural language description of the 1969 * StructureDefinition and its use.). This is the underlying object with 1970 * id, value and extensions. The accessor "getDescription" gives direct 1971 * access to the value 1972 */ 1973 public StringType getDescriptionElement() { 1974 if (this.description == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create StructureDefinition.description"); 1977 else if (Configuration.doAutoCreate()) 1978 this.description = new StringType(); // bb 1979 return this.description; 1980 } 1981 1982 public boolean hasDescriptionElement() { 1983 return this.description != null && !this.description.isEmpty(); 1984 } 1985 1986 public boolean hasDescription() { 1987 return this.description != null && !this.description.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #description} (A free text natural language description 1992 * of the StructureDefinition and its use.). This is the underlying 1993 * object with id, value and extensions. The accessor 1994 * "getDescription" gives direct access to the value 1995 */ 1996 public StructureDefinition setDescriptionElement(StringType value) { 1997 this.description = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return A free text natural language description of the StructureDefinition 2003 * and its use. 2004 */ 2005 public String getDescription() { 2006 return this.description == null ? null : this.description.getValue(); 2007 } 2008 2009 /** 2010 * @param value A free text natural language description of the 2011 * StructureDefinition and its use. 2012 */ 2013 public StructureDefinition setDescription(String value) { 2014 if (Utilities.noString(value)) 2015 this.description = null; 2016 else { 2017 if (this.description == null) 2018 this.description = new StringType(); 2019 this.description.setValue(value); 2020 } 2021 return this; 2022 } 2023 2024 /** 2025 * @return {@link #useContext} (The content was developed with a focus and 2026 * intent of supporting the contexts that are listed. These terms may be 2027 * used to assist with indexing and searching of structure definitions.) 2028 */ 2029 public List<CodeableConcept> getUseContext() { 2030 if (this.useContext == null) 2031 this.useContext = new ArrayList<CodeableConcept>(); 2032 return this.useContext; 2033 } 2034 2035 public boolean hasUseContext() { 2036 if (this.useContext == null) 2037 return false; 2038 for (CodeableConcept item : this.useContext) 2039 if (!item.isEmpty()) 2040 return true; 2041 return false; 2042 } 2043 2044 /** 2045 * @return {@link #useContext} (The content was developed with a focus and 2046 * intent of supporting the contexts that are listed. These terms may be 2047 * used to assist with indexing and searching of structure definitions.) 2048 */ 2049 // syntactic sugar 2050 public CodeableConcept addUseContext() { // 3 2051 CodeableConcept t = new CodeableConcept(); 2052 if (this.useContext == null) 2053 this.useContext = new ArrayList<CodeableConcept>(); 2054 this.useContext.add(t); 2055 return t; 2056 } 2057 2058 // syntactic sugar 2059 public StructureDefinition addUseContext(CodeableConcept t) { // 3 2060 if (t == null) 2061 return this; 2062 if (this.useContext == null) 2063 this.useContext = new ArrayList<CodeableConcept>(); 2064 this.useContext.add(t); 2065 return this; 2066 } 2067 2068 /** 2069 * @return {@link #requirements} (Explains why this structure definition is 2070 * needed and why it's been constrained as it has.). This is the 2071 * underlying object with id, value and extensions. The accessor 2072 * "getRequirements" gives direct access to the value 2073 */ 2074 public StringType getRequirementsElement() { 2075 if (this.requirements == null) 2076 if (Configuration.errorOnAutoCreate()) 2077 throw new Error("Attempt to auto-create StructureDefinition.requirements"); 2078 else if (Configuration.doAutoCreate()) 2079 this.requirements = new StringType(); // bb 2080 return this.requirements; 2081 } 2082 2083 public boolean hasRequirementsElement() { 2084 return this.requirements != null && !this.requirements.isEmpty(); 2085 } 2086 2087 public boolean hasRequirements() { 2088 return this.requirements != null && !this.requirements.isEmpty(); 2089 } 2090 2091 /** 2092 * @param value {@link #requirements} (Explains why this structure definition is 2093 * needed and why it's been constrained as it has.). This is the 2094 * underlying object with id, value and extensions. The accessor 2095 * "getRequirements" gives direct access to the value 2096 */ 2097 public StructureDefinition setRequirementsElement(StringType value) { 2098 this.requirements = value; 2099 return this; 2100 } 2101 2102 /** 2103 * @return Explains why this structure definition is needed and why it's been 2104 * constrained as it has. 2105 */ 2106 public String getRequirements() { 2107 return this.requirements == null ? null : this.requirements.getValue(); 2108 } 2109 2110 /** 2111 * @param value Explains why this structure definition is needed and why it's 2112 * been constrained as it has. 2113 */ 2114 public StructureDefinition setRequirements(String value) { 2115 if (Utilities.noString(value)) 2116 this.requirements = null; 2117 else { 2118 if (this.requirements == null) 2119 this.requirements = new StringType(); 2120 this.requirements.setValue(value); 2121 } 2122 return this; 2123 } 2124 2125 /** 2126 * @return {@link #copyright} (A copyright statement relating to the structure 2127 * definition and/or its contents. Copyright statements are generally 2128 * legal restrictions on the use and publishing of the details of the 2129 * constraints and mappings.). This is the underlying object with id, 2130 * value and extensions. The accessor "getCopyright" gives direct access 2131 * to the value 2132 */ 2133 public StringType getCopyrightElement() { 2134 if (this.copyright == null) 2135 if (Configuration.errorOnAutoCreate()) 2136 throw new Error("Attempt to auto-create StructureDefinition.copyright"); 2137 else if (Configuration.doAutoCreate()) 2138 this.copyright = new StringType(); // bb 2139 return this.copyright; 2140 } 2141 2142 public boolean hasCopyrightElement() { 2143 return this.copyright != null && !this.copyright.isEmpty(); 2144 } 2145 2146 public boolean hasCopyright() { 2147 return this.copyright != null && !this.copyright.isEmpty(); 2148 } 2149 2150 /** 2151 * @param value {@link #copyright} (A copyright statement relating to the 2152 * structure definition and/or its contents. Copyright statements 2153 * are generally legal restrictions on the use and publishing of 2154 * the details of the constraints and mappings.). This is the 2155 * underlying object with id, value and extensions. The accessor 2156 * "getCopyright" gives direct access to the value 2157 */ 2158 public StructureDefinition setCopyrightElement(StringType value) { 2159 this.copyright = value; 2160 return this; 2161 } 2162 2163 /** 2164 * @return A copyright statement relating to the structure definition and/or its 2165 * contents. Copyright statements are generally legal restrictions on 2166 * the use and publishing of the details of the constraints and 2167 * mappings. 2168 */ 2169 public String getCopyright() { 2170 return this.copyright == null ? null : this.copyright.getValue(); 2171 } 2172 2173 /** 2174 * @param value A copyright statement relating to the structure definition 2175 * and/or its contents. Copyright statements are generally legal 2176 * restrictions on the use and publishing of the details of the 2177 * constraints and mappings. 2178 */ 2179 public StructureDefinition setCopyright(String value) { 2180 if (Utilities.noString(value)) 2181 this.copyright = null; 2182 else { 2183 if (this.copyright == null) 2184 this.copyright = new StringType(); 2185 this.copyright.setValue(value); 2186 } 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #code} (A set of terms from external terminologies that may be 2192 * used to assist with indexing and searching of templates.) 2193 */ 2194 public List<Coding> getCode() { 2195 if (this.code == null) 2196 this.code = new ArrayList<Coding>(); 2197 return this.code; 2198 } 2199 2200 public boolean hasCode() { 2201 if (this.code == null) 2202 return false; 2203 for (Coding item : this.code) 2204 if (!item.isEmpty()) 2205 return true; 2206 return false; 2207 } 2208 2209 /** 2210 * @return {@link #code} (A set of terms from external terminologies that may be 2211 * used to assist with indexing and searching of templates.) 2212 */ 2213 // syntactic sugar 2214 public Coding addCode() { // 3 2215 Coding t = new Coding(); 2216 if (this.code == null) 2217 this.code = new ArrayList<Coding>(); 2218 this.code.add(t); 2219 return t; 2220 } 2221 2222 // syntactic sugar 2223 public StructureDefinition addCode(Coding t) { // 3 2224 if (t == null) 2225 return this; 2226 if (this.code == null) 2227 this.code = new ArrayList<Coding>(); 2228 this.code.add(t); 2229 return this; 2230 } 2231 2232 /** 2233 * @return {@link #fhirVersion} (The version of the FHIR specification on which 2234 * this StructureDefinition is based - this is the formal version of the 2235 * specification, without the revision number, e.g. 2236 * [publication].[major].[minor], which is 1.0.2 for this version.). 2237 * This is the underlying object with id, value and extensions. The 2238 * accessor "getFhirVersion" gives direct access to the value 2239 */ 2240 public IdType getFhirVersionElement() { 2241 if (this.fhirVersion == null) 2242 if (Configuration.errorOnAutoCreate()) 2243 throw new Error("Attempt to auto-create StructureDefinition.fhirVersion"); 2244 else if (Configuration.doAutoCreate()) 2245 this.fhirVersion = new IdType(); // bb 2246 return this.fhirVersion; 2247 } 2248 2249 public boolean hasFhirVersionElement() { 2250 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2251 } 2252 2253 public boolean hasFhirVersion() { 2254 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2255 } 2256 2257 /** 2258 * @param value {@link #fhirVersion} (The version of the FHIR specification on 2259 * which this StructureDefinition is based - this is the formal 2260 * version of the specification, without the revision number, e.g. 2261 * [publication].[major].[minor], which is 1.0.2 for this 2262 * version.). This is the underlying object with id, value and 2263 * extensions. The accessor "getFhirVersion" gives direct access to 2264 * the value 2265 */ 2266 public StructureDefinition setFhirVersionElement(IdType value) { 2267 this.fhirVersion = value; 2268 return this; 2269 } 2270 2271 /** 2272 * @return The version of the FHIR specification on which this 2273 * StructureDefinition is based - this is the formal version of the 2274 * specification, without the revision number, e.g. 2275 * [publication].[major].[minor], which is 1.0.2 for this version. 2276 */ 2277 public String getFhirVersion() { 2278 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 2279 } 2280 2281 /** 2282 * @param value The version of the FHIR specification on which this 2283 * StructureDefinition is based - this is the formal version of the 2284 * specification, without the revision number, e.g. 2285 * [publication].[major].[minor], which is 1.0.2 for this version. 2286 */ 2287 public StructureDefinition setFhirVersion(String value) { 2288 if (Utilities.noString(value)) 2289 this.fhirVersion = null; 2290 else { 2291 if (this.fhirVersion == null) 2292 this.fhirVersion = new IdType(); 2293 this.fhirVersion.setValue(value); 2294 } 2295 return this; 2296 } 2297 2298 /** 2299 * @return {@link #mapping} (An external specification that the content is 2300 * mapped to.) 2301 */ 2302 public List<StructureDefinitionMappingComponent> getMapping() { 2303 if (this.mapping == null) 2304 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2305 return this.mapping; 2306 } 2307 2308 public boolean hasMapping() { 2309 if (this.mapping == null) 2310 return false; 2311 for (StructureDefinitionMappingComponent item : this.mapping) 2312 if (!item.isEmpty()) 2313 return true; 2314 return false; 2315 } 2316 2317 /** 2318 * @return {@link #mapping} (An external specification that the content is 2319 * mapped to.) 2320 */ 2321 // syntactic sugar 2322 public StructureDefinitionMappingComponent addMapping() { // 3 2323 StructureDefinitionMappingComponent t = new StructureDefinitionMappingComponent(); 2324 if (this.mapping == null) 2325 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2326 this.mapping.add(t); 2327 return t; 2328 } 2329 2330 // syntactic sugar 2331 public StructureDefinition addMapping(StructureDefinitionMappingComponent t) { // 3 2332 if (t == null) 2333 return this; 2334 if (this.mapping == null) 2335 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2336 this.mapping.add(t); 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #kind} (Defines the kind of structure that this definition is 2342 * describing.). This is the underlying object with id, value and 2343 * extensions. The accessor "getKind" gives direct access to the value 2344 */ 2345 public Enumeration<StructureDefinitionKind> getKindElement() { 2346 if (this.kind == null) 2347 if (Configuration.errorOnAutoCreate()) 2348 throw new Error("Attempt to auto-create StructureDefinition.kind"); 2349 else if (Configuration.doAutoCreate()) 2350 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); // bb 2351 return this.kind; 2352 } 2353 2354 public boolean hasKindElement() { 2355 return this.kind != null && !this.kind.isEmpty(); 2356 } 2357 2358 public boolean hasKind() { 2359 return this.kind != null && !this.kind.isEmpty(); 2360 } 2361 2362 /** 2363 * @param value {@link #kind} (Defines the kind of structure that this 2364 * definition is describing.). This is the underlying object with 2365 * id, value and extensions. The accessor "getKind" gives direct 2366 * access to the value 2367 */ 2368 public StructureDefinition setKindElement(Enumeration<StructureDefinitionKind> value) { 2369 this.kind = value; 2370 return this; 2371 } 2372 2373 /** 2374 * @return Defines the kind of structure that this definition is describing. 2375 */ 2376 public StructureDefinitionKind getKind() { 2377 return this.kind == null ? null : this.kind.getValue(); 2378 } 2379 2380 /** 2381 * @param value Defines the kind of structure that this definition is 2382 * describing. 2383 */ 2384 public StructureDefinition setKind(StructureDefinitionKind value) { 2385 if (this.kind == null) 2386 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); 2387 this.kind.setValue(value); 2388 return this; 2389 } 2390 2391 /** 2392 * @return {@link #constrainedType} (The type of type that is being constrained 2393 * - a data type, an extension, a resource, including abstract ones. If 2394 * this field is present, it indicates that the structure definition is 2395 * a constraint. If it is not present, then the structure definition is 2396 * the definition of a base structure.). This is the underlying object 2397 * with id, value and extensions. The accessor "getConstrainedType" 2398 * gives direct access to the value 2399 */ 2400 public CodeType getConstrainedTypeElement() { 2401 if (this.constrainedType == null) 2402 if (Configuration.errorOnAutoCreate()) 2403 throw new Error("Attempt to auto-create StructureDefinition.constrainedType"); 2404 else if (Configuration.doAutoCreate()) 2405 this.constrainedType = new CodeType(); // bb 2406 return this.constrainedType; 2407 } 2408 2409 public boolean hasConstrainedTypeElement() { 2410 return this.constrainedType != null && !this.constrainedType.isEmpty(); 2411 } 2412 2413 public boolean hasConstrainedType() { 2414 return this.constrainedType != null && !this.constrainedType.isEmpty(); 2415 } 2416 2417 /** 2418 * @param value {@link #constrainedType} (The type of type that is being 2419 * constrained - a data type, an extension, a resource, including 2420 * abstract ones. If this field is present, it indicates that the 2421 * structure definition is a constraint. If it is not present, then 2422 * the structure definition is the definition of a base 2423 * structure.). This is the underlying object with id, value and 2424 * extensions. The accessor "getConstrainedType" gives direct 2425 * access to the value 2426 */ 2427 public StructureDefinition setConstrainedTypeElement(CodeType value) { 2428 this.constrainedType = value; 2429 return this; 2430 } 2431 2432 /** 2433 * @return The type of type that is being constrained - a data type, an 2434 * extension, a resource, including abstract ones. If this field is 2435 * present, it indicates that the structure definition is a constraint. 2436 * If it is not present, then the structure definition is the definition 2437 * of a base structure. 2438 */ 2439 public String getConstrainedType() { 2440 return this.constrainedType == null ? null : this.constrainedType.getValue(); 2441 } 2442 2443 /** 2444 * @param value The type of type that is being constrained - a data type, an 2445 * extension, a resource, including abstract ones. If this field is 2446 * present, it indicates that the structure definition is a 2447 * constraint. If it is not present, then the structure definition 2448 * is the definition of a base structure. 2449 */ 2450 public StructureDefinition setConstrainedType(String value) { 2451 if (Utilities.noString(value)) 2452 this.constrainedType = null; 2453 else { 2454 if (this.constrainedType == null) 2455 this.constrainedType = new CodeType(); 2456 this.constrainedType.setValue(value); 2457 } 2458 return this; 2459 } 2460 2461 /** 2462 * @return {@link #abstract_} (Whether structure this definition describes is 2463 * abstract or not - that is, whether an actual exchanged item can ever 2464 * be of this type.). This is the underlying object with id, value and 2465 * extensions. The accessor "getAbstract" gives direct access to the 2466 * value 2467 */ 2468 public BooleanType getAbstractElement() { 2469 if (this.abstract_ == null) 2470 if (Configuration.errorOnAutoCreate()) 2471 throw new Error("Attempt to auto-create StructureDefinition.abstract_"); 2472 else if (Configuration.doAutoCreate()) 2473 this.abstract_ = new BooleanType(); // bb 2474 return this.abstract_; 2475 } 2476 2477 public boolean hasAbstractElement() { 2478 return this.abstract_ != null && !this.abstract_.isEmpty(); 2479 } 2480 2481 public boolean hasAbstract() { 2482 return this.abstract_ != null && !this.abstract_.isEmpty(); 2483 } 2484 2485 /** 2486 * @param value {@link #abstract_} (Whether structure this definition describes 2487 * is abstract or not - that is, whether an actual exchanged item 2488 * can ever be of this type.). This is the underlying object with 2489 * id, value and extensions. The accessor "getAbstract" gives 2490 * direct access to the value 2491 */ 2492 public StructureDefinition setAbstractElement(BooleanType value) { 2493 this.abstract_ = value; 2494 return this; 2495 } 2496 2497 /** 2498 * @return Whether structure this definition describes is abstract or not - that 2499 * is, whether an actual exchanged item can ever be of this type. 2500 */ 2501 public boolean getAbstract() { 2502 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 2503 } 2504 2505 /** 2506 * @param value Whether structure this definition describes is abstract or not - 2507 * that is, whether an actual exchanged item can ever be of this 2508 * type. 2509 */ 2510 public StructureDefinition setAbstract(boolean value) { 2511 if (this.abstract_ == null) 2512 this.abstract_ = new BooleanType(); 2513 this.abstract_.setValue(value); 2514 return this; 2515 } 2516 2517 /** 2518 * @return {@link #contextType} (If this is an extension, Identifies the context 2519 * within FHIR resources where the extension can be used.). This is the 2520 * underlying object with id, value and extensions. The accessor 2521 * "getContextType" gives direct access to the value 2522 */ 2523 public Enumeration<ExtensionContext> getContextTypeElement() { 2524 if (this.contextType == null) 2525 if (Configuration.errorOnAutoCreate()) 2526 throw new Error("Attempt to auto-create StructureDefinition.contextType"); 2527 else if (Configuration.doAutoCreate()) 2528 this.contextType = new Enumeration<ExtensionContext>(new ExtensionContextEnumFactory()); // bb 2529 return this.contextType; 2530 } 2531 2532 public boolean hasContextTypeElement() { 2533 return this.contextType != null && !this.contextType.isEmpty(); 2534 } 2535 2536 public boolean hasContextType() { 2537 return this.contextType != null && !this.contextType.isEmpty(); 2538 } 2539 2540 /** 2541 * @param value {@link #contextType} (If this is an extension, Identifies the 2542 * context within FHIR resources where the extension can be used.). 2543 * This is the underlying object with id, value and extensions. The 2544 * accessor "getContextType" gives direct access to the value 2545 */ 2546 public StructureDefinition setContextTypeElement(Enumeration<ExtensionContext> value) { 2547 this.contextType = value; 2548 return this; 2549 } 2550 2551 /** 2552 * @return If this is an extension, Identifies the context within FHIR resources 2553 * where the extension can be used. 2554 */ 2555 public ExtensionContext getContextType() { 2556 return this.contextType == null ? null : this.contextType.getValue(); 2557 } 2558 2559 /** 2560 * @param value If this is an extension, Identifies the context within FHIR 2561 * resources where the extension can be used. 2562 */ 2563 public StructureDefinition setContextType(ExtensionContext value) { 2564 if (value == null) 2565 this.contextType = null; 2566 else { 2567 if (this.contextType == null) 2568 this.contextType = new Enumeration<ExtensionContext>(new ExtensionContextEnumFactory()); 2569 this.contextType.setValue(value); 2570 } 2571 return this; 2572 } 2573 2574 /** 2575 * @return {@link #context} (Identifies the types of resource or data type 2576 * elements to which the extension can be applied.) 2577 */ 2578 public List<StringType> getContext() { 2579 if (this.context == null) 2580 this.context = new ArrayList<StringType>(); 2581 return this.context; 2582 } 2583 2584 public boolean hasContext() { 2585 if (this.context == null) 2586 return false; 2587 for (StringType item : this.context) 2588 if (!item.isEmpty()) 2589 return true; 2590 return false; 2591 } 2592 2593 /** 2594 * @return {@link #context} (Identifies the types of resource or data type 2595 * elements to which the extension can be applied.) 2596 */ 2597 // syntactic sugar 2598 public StringType addContextElement() {// 2 2599 StringType t = new StringType(); 2600 if (this.context == null) 2601 this.context = new ArrayList<StringType>(); 2602 this.context.add(t); 2603 return t; 2604 } 2605 2606 /** 2607 * @param value {@link #context} (Identifies the types of resource or data type 2608 * elements to which the extension can be applied.) 2609 */ 2610 public StructureDefinition addContext(String value) { // 1 2611 StringType t = new StringType(); 2612 t.setValue(value); 2613 if (this.context == null) 2614 this.context = new ArrayList<StringType>(); 2615 this.context.add(t); 2616 return this; 2617 } 2618 2619 /** 2620 * @param value {@link #context} (Identifies the types of resource or data type 2621 * elements to which the extension can be applied.) 2622 */ 2623 public boolean hasContext(String value) { 2624 if (this.context == null) 2625 return false; 2626 for (StringType v : this.context) 2627 if (v.equals(value)) // string 2628 return true; 2629 return false; 2630 } 2631 2632 /** 2633 * @return {@link #base} (An absolute URI that is the base structure from which 2634 * this set of constraints is derived.). This is the underlying object 2635 * with id, value and extensions. The accessor "getBase" gives direct 2636 * access to the value 2637 */ 2638 public UriType getBaseElement() { 2639 if (this.base == null) 2640 if (Configuration.errorOnAutoCreate()) 2641 throw new Error("Attempt to auto-create StructureDefinition.base"); 2642 else if (Configuration.doAutoCreate()) 2643 this.base = new UriType(); // bb 2644 return this.base; 2645 } 2646 2647 public boolean hasBaseElement() { 2648 return this.base != null && !this.base.isEmpty(); 2649 } 2650 2651 public boolean hasBase() { 2652 return this.base != null && !this.base.isEmpty(); 2653 } 2654 2655 /** 2656 * @param value {@link #base} (An absolute URI that is the base structure from 2657 * which this set of constraints is derived.). This is the 2658 * underlying object with id, value and extensions. The accessor 2659 * "getBase" gives direct access to the value 2660 */ 2661 public StructureDefinition setBaseElement(UriType value) { 2662 this.base = value; 2663 return this; 2664 } 2665 2666 /** 2667 * @return An absolute URI that is the base structure from which this set of 2668 * constraints is derived. 2669 */ 2670 public String getBase() { 2671 return this.base == null ? null : this.base.getValue(); 2672 } 2673 2674 /** 2675 * @param value An absolute URI that is the base structure from which this set 2676 * of constraints is derived. 2677 */ 2678 public StructureDefinition setBase(String value) { 2679 if (Utilities.noString(value)) 2680 this.base = null; 2681 else { 2682 if (this.base == null) 2683 this.base = new UriType(); 2684 this.base.setValue(value); 2685 } 2686 return this; 2687 } 2688 2689 /** 2690 * @return {@link #snapshot} (A snapshot view is expressed in a stand alone form 2691 * that can be used and interpreted without considering the base 2692 * StructureDefinition.) 2693 */ 2694 public StructureDefinitionSnapshotComponent getSnapshot() { 2695 if (this.snapshot == null) 2696 if (Configuration.errorOnAutoCreate()) 2697 throw new Error("Attempt to auto-create StructureDefinition.snapshot"); 2698 else if (Configuration.doAutoCreate()) 2699 this.snapshot = new StructureDefinitionSnapshotComponent(); // cc 2700 return this.snapshot; 2701 } 2702 2703 public boolean hasSnapshot() { 2704 return this.snapshot != null && !this.snapshot.isEmpty(); 2705 } 2706 2707 /** 2708 * @param value {@link #snapshot} (A snapshot view is expressed in a stand alone 2709 * form that can be used and interpreted without considering the 2710 * base StructureDefinition.) 2711 */ 2712 public StructureDefinition setSnapshot(StructureDefinitionSnapshotComponent value) { 2713 this.snapshot = value; 2714 return this; 2715 } 2716 2717 /** 2718 * @return {@link #differential} (A differential view is expressed relative to 2719 * the base StructureDefinition - a statement of differences that it 2720 * applies.) 2721 */ 2722 public StructureDefinitionDifferentialComponent getDifferential() { 2723 if (this.differential == null) 2724 if (Configuration.errorOnAutoCreate()) 2725 throw new Error("Attempt to auto-create StructureDefinition.differential"); 2726 else if (Configuration.doAutoCreate()) 2727 this.differential = new StructureDefinitionDifferentialComponent(); // cc 2728 return this.differential; 2729 } 2730 2731 public boolean hasDifferential() { 2732 return this.differential != null && !this.differential.isEmpty(); 2733 } 2734 2735 /** 2736 * @param value {@link #differential} (A differential view is expressed relative 2737 * to the base StructureDefinition - a statement of differences 2738 * that it applies.) 2739 */ 2740 public StructureDefinition setDifferential(StructureDefinitionDifferentialComponent value) { 2741 this.differential = value; 2742 return this; 2743 } 2744 2745 protected void listChildren(List<Property> childrenList) { 2746 super.listChildren(childrenList); 2747 childrenList.add(new Property("url", "uri", 2748 "An absolute URL that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published.", 2749 0, java.lang.Integer.MAX_VALUE, url)); 2750 childrenList.add(new Property("identifier", "Identifier", 2751 "Formal identifier that is used to identify this StructureDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance (should be globally unique OID, UUID, or URI), (if it's not possible to use the literal URI).", 2752 0, java.lang.Integer.MAX_VALUE, identifier)); 2753 childrenList.add(new Property("version", "string", 2754 "The identifier that is used to identify this version of the StructureDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the StructureDefinition author manually.", 2755 0, java.lang.Integer.MAX_VALUE, version)); 2756 childrenList 2757 .add(new Property("name", "string", "A free text natural language name identifying the StructureDefinition.", 0, 2758 java.lang.Integer.MAX_VALUE, name)); 2759 childrenList.add(new Property("display", "string", 2760 "Defined so that applications can use this name when displaying the value of the extension to the user.", 0, 2761 java.lang.Integer.MAX_VALUE, display)); 2762 childrenList.add(new Property("status", "code", "The status of the StructureDefinition.", 0, 2763 java.lang.Integer.MAX_VALUE, status)); 2764 childrenList.add(new Property("experimental", "boolean", 2765 "This StructureDefinition was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 2766 0, java.lang.Integer.MAX_VALUE, experimental)); 2767 childrenList.add(new Property("publisher", "string", 2768 "The name of the individual or organization that published the structure definition.", 0, 2769 java.lang.Integer.MAX_VALUE, publisher)); 2770 childrenList 2771 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 2772 0, java.lang.Integer.MAX_VALUE, contact)); 2773 childrenList.add(new Property("date", "dateTime", 2774 "The date this version of the structure definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 2775 0, java.lang.Integer.MAX_VALUE, date)); 2776 childrenList.add(new Property("description", "string", 2777 "A free text natural language description of the StructureDefinition and its use.", 0, 2778 java.lang.Integer.MAX_VALUE, description)); 2779 childrenList.add(new Property("useContext", "CodeableConcept", 2780 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of structure definitions.", 2781 0, java.lang.Integer.MAX_VALUE, useContext)); 2782 childrenList.add(new Property("requirements", "string", 2783 "Explains why this structure definition is needed and why it's been constrained as it has.", 0, 2784 java.lang.Integer.MAX_VALUE, requirements)); 2785 childrenList.add(new Property("copyright", "string", 2786 "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the constraints and mappings.", 2787 0, java.lang.Integer.MAX_VALUE, copyright)); 2788 childrenList.add(new Property("code", "Coding", 2789 "A set of terms from external terminologies that may be used to assist with indexing and searching of templates.", 2790 0, java.lang.Integer.MAX_VALUE, code)); 2791 childrenList.add(new Property("fhirVersion", "id", 2792 "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 1.0.2 for this version.", 2793 0, java.lang.Integer.MAX_VALUE, fhirVersion)); 2794 childrenList.add(new Property("mapping", "", "An external specification that the content is mapped to.", 0, 2795 java.lang.Integer.MAX_VALUE, mapping)); 2796 childrenList.add(new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 2797 0, java.lang.Integer.MAX_VALUE, kind)); 2798 childrenList.add(new Property("constrainedType", "code", 2799 "The type of type that is being constrained - a data type, an extension, a resource, including abstract ones. If this field is present, it indicates that the structure definition is a constraint. If it is not present, then the structure definition is the definition of a base structure.", 2800 0, java.lang.Integer.MAX_VALUE, constrainedType)); 2801 childrenList.add(new Property("abstract", "boolean", 2802 "Whether structure this definition describes is abstract or not - that is, whether an actual exchanged item can ever be of this type.", 2803 0, java.lang.Integer.MAX_VALUE, abstract_)); 2804 childrenList.add(new Property("contextType", "code", 2805 "If this is an extension, Identifies the context within FHIR resources where the extension can be used.", 0, 2806 java.lang.Integer.MAX_VALUE, contextType)); 2807 childrenList.add(new Property("context", "string", 2808 "Identifies the types of resource or data type elements to which the extension can be applied.", 0, 2809 java.lang.Integer.MAX_VALUE, context)); 2810 childrenList.add(new Property("base", "uri", 2811 "An absolute URI that is the base structure from which this set of constraints is derived.", 0, 2812 java.lang.Integer.MAX_VALUE, base)); 2813 childrenList.add(new Property("snapshot", "", 2814 "A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition.", 2815 0, java.lang.Integer.MAX_VALUE, snapshot)); 2816 childrenList.add(new Property("differential", "", 2817 "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 2818 0, java.lang.Integer.MAX_VALUE, differential)); 2819 } 2820 2821 @Override 2822 public void setProperty(String name, Base value) throws FHIRException { 2823 if (name.equals("url")) 2824 this.url = castToUri(value); // UriType 2825 else if (name.equals("identifier")) 2826 this.getIdentifier().add(castToIdentifier(value)); 2827 else if (name.equals("version")) 2828 this.version = castToString(value); // StringType 2829 else if (name.equals("name")) 2830 this.name = castToString(value); // StringType 2831 else if (name.equals("display")) 2832 this.display = castToString(value); // StringType 2833 else if (name.equals("status")) 2834 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 2835 else if (name.equals("experimental")) 2836 this.experimental = castToBoolean(value); // BooleanType 2837 else if (name.equals("publisher")) 2838 this.publisher = castToString(value); // StringType 2839 else if (name.equals("contact")) 2840 this.getContact().add((StructureDefinitionContactComponent) value); 2841 else if (name.equals("date")) 2842 this.date = castToDateTime(value); // DateTimeType 2843 else if (name.equals("description")) 2844 this.description = castToString(value); // StringType 2845 else if (name.equals("useContext")) 2846 this.getUseContext().add(castToCodeableConcept(value)); 2847 else if (name.equals("requirements")) 2848 this.requirements = castToString(value); // StringType 2849 else if (name.equals("copyright")) 2850 this.copyright = castToString(value); // StringType 2851 else if (name.equals("code")) 2852 this.getCode().add(castToCoding(value)); 2853 else if (name.equals("fhirVersion")) 2854 this.fhirVersion = castToId(value); // IdType 2855 else if (name.equals("mapping")) 2856 this.getMapping().add((StructureDefinitionMappingComponent) value); 2857 else if (name.equals("kind")) 2858 this.kind = new StructureDefinitionKindEnumFactory().fromType(value); // Enumeration<StructureDefinitionKind> 2859 else if (name.equals("constrainedType")) 2860 this.constrainedType = castToCode(value); // CodeType 2861 else if (name.equals("abstract")) 2862 this.abstract_ = castToBoolean(value); // BooleanType 2863 else if (name.equals("contextType")) 2864 this.contextType = new ExtensionContextEnumFactory().fromType(value); // Enumeration<ExtensionContext> 2865 else if (name.equals("context")) 2866 this.getContext().add(castToString(value)); 2867 else if (name.equals("base")) 2868 this.base = castToUri(value); // UriType 2869 else if (name.equals("snapshot")) 2870 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 2871 else if (name.equals("differential")) 2872 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 2873 else 2874 super.setProperty(name, value); 2875 } 2876 2877 @Override 2878 public Base addChild(String name) throws FHIRException { 2879 if (name.equals("url")) { 2880 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.url"); 2881 } else if (name.equals("identifier")) { 2882 return addIdentifier(); 2883 } else if (name.equals("version")) { 2884 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.version"); 2885 } else if (name.equals("name")) { 2886 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 2887 } else if (name.equals("display")) { 2888 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.display"); 2889 } else if (name.equals("status")) { 2890 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.status"); 2891 } else if (name.equals("experimental")) { 2892 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.experimental"); 2893 } else if (name.equals("publisher")) { 2894 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.publisher"); 2895 } else if (name.equals("contact")) { 2896 return addContact(); 2897 } else if (name.equals("date")) { 2898 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.date"); 2899 } else if (name.equals("description")) { 2900 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.description"); 2901 } else if (name.equals("useContext")) { 2902 return addUseContext(); 2903 } else if (name.equals("requirements")) { 2904 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.requirements"); 2905 } else if (name.equals("copyright")) { 2906 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyright"); 2907 } else if (name.equals("code")) { 2908 return addCode(); 2909 } else if (name.equals("fhirVersion")) { 2910 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.fhirVersion"); 2911 } else if (name.equals("mapping")) { 2912 return addMapping(); 2913 } else if (name.equals("kind")) { 2914 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.kind"); 2915 } else if (name.equals("constrainedType")) { 2916 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.constrainedType"); 2917 } else if (name.equals("abstract")) { 2918 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.abstract"); 2919 } else if (name.equals("contextType")) { 2920 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.contextType"); 2921 } else if (name.equals("context")) { 2922 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.context"); 2923 } else if (name.equals("base")) { 2924 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.base"); 2925 } else if (name.equals("snapshot")) { 2926 this.snapshot = new StructureDefinitionSnapshotComponent(); 2927 return this.snapshot; 2928 } else if (name.equals("differential")) { 2929 this.differential = new StructureDefinitionDifferentialComponent(); 2930 return this.differential; 2931 } else 2932 return super.addChild(name); 2933 } 2934 2935 public String fhirType() { 2936 return "StructureDefinition"; 2937 2938 } 2939 2940 public StructureDefinition copy() { 2941 StructureDefinition dst = new StructureDefinition(); 2942 copyValues(dst); 2943 dst.url = url == null ? null : url.copy(); 2944 if (identifier != null) { 2945 dst.identifier = new ArrayList<Identifier>(); 2946 for (Identifier i : identifier) 2947 dst.identifier.add(i.copy()); 2948 } 2949 ; 2950 dst.version = version == null ? null : version.copy(); 2951 dst.name = name == null ? null : name.copy(); 2952 dst.display = display == null ? null : display.copy(); 2953 dst.status = status == null ? null : status.copy(); 2954 dst.experimental = experimental == null ? null : experimental.copy(); 2955 dst.publisher = publisher == null ? null : publisher.copy(); 2956 if (contact != null) { 2957 dst.contact = new ArrayList<StructureDefinitionContactComponent>(); 2958 for (StructureDefinitionContactComponent i : contact) 2959 dst.contact.add(i.copy()); 2960 } 2961 ; 2962 dst.date = date == null ? null : date.copy(); 2963 dst.description = description == null ? null : description.copy(); 2964 if (useContext != null) { 2965 dst.useContext = new ArrayList<CodeableConcept>(); 2966 for (CodeableConcept i : useContext) 2967 dst.useContext.add(i.copy()); 2968 } 2969 ; 2970 dst.requirements = requirements == null ? null : requirements.copy(); 2971 dst.copyright = copyright == null ? null : copyright.copy(); 2972 if (code != null) { 2973 dst.code = new ArrayList<Coding>(); 2974 for (Coding i : code) 2975 dst.code.add(i.copy()); 2976 } 2977 ; 2978 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 2979 if (mapping != null) { 2980 dst.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2981 for (StructureDefinitionMappingComponent i : mapping) 2982 dst.mapping.add(i.copy()); 2983 } 2984 ; 2985 dst.kind = kind == null ? null : kind.copy(); 2986 dst.constrainedType = constrainedType == null ? null : constrainedType.copy(); 2987 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 2988 dst.contextType = contextType == null ? null : contextType.copy(); 2989 if (context != null) { 2990 dst.context = new ArrayList<StringType>(); 2991 for (StringType i : context) 2992 dst.context.add(i.copy()); 2993 } 2994 ; 2995 dst.base = base == null ? null : base.copy(); 2996 dst.snapshot = snapshot == null ? null : snapshot.copy(); 2997 dst.differential = differential == null ? null : differential.copy(); 2998 return dst; 2999 } 3000 3001 protected StructureDefinition typedCopy() { 3002 return copy(); 3003 } 3004 3005 @Override 3006 public boolean equalsDeep(Base other) { 3007 if (!super.equalsDeep(other)) 3008 return false; 3009 if (!(other instanceof StructureDefinition)) 3010 return false; 3011 StructureDefinition o = (StructureDefinition) other; 3012 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) 3013 && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 3014 && compareDeep(display, o.display, true) && compareDeep(status, o.status, true) 3015 && compareDeep(experimental, o.experimental, true) && compareDeep(publisher, o.publisher, true) 3016 && compareDeep(contact, o.contact, true) && compareDeep(date, o.date, true) 3017 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 3018 && compareDeep(requirements, o.requirements, true) && compareDeep(copyright, o.copyright, true) 3019 && compareDeep(code, o.code, true) && compareDeep(fhirVersion, o.fhirVersion, true) 3020 && compareDeep(mapping, o.mapping, true) && compareDeep(kind, o.kind, true) 3021 && compareDeep(constrainedType, o.constrainedType, true) && compareDeep(abstract_, o.abstract_, true) 3022 && compareDeep(contextType, o.contextType, true) && compareDeep(context, o.context, true) 3023 && compareDeep(base, o.base, true) && compareDeep(snapshot, o.snapshot, true) 3024 && compareDeep(differential, o.differential, true); 3025 } 3026 3027 @Override 3028 public boolean equalsShallow(Base other) { 3029 if (!super.equalsShallow(other)) 3030 return false; 3031 if (!(other instanceof StructureDefinition)) 3032 return false; 3033 StructureDefinition o = (StructureDefinition) other; 3034 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 3035 && compareValues(name, o.name, true) && compareValues(display, o.display, true) 3036 && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 3037 && compareValues(publisher, o.publisher, true) && compareValues(date, o.date, true) 3038 && compareValues(description, o.description, true) && compareValues(requirements, o.requirements, true) 3039 && compareValues(copyright, o.copyright, true) && compareValues(fhirVersion, o.fhirVersion, true) 3040 && compareValues(kind, o.kind, true) && compareValues(constrainedType, o.constrainedType, true) 3041 && compareValues(abstract_, o.abstract_, true) && compareValues(contextType, o.contextType, true) 3042 && compareValues(context, o.context, true) && compareValues(base, o.base, true); 3043 } 3044 3045 public boolean isEmpty() { 3046 return super.isEmpty() && (url == null || url.isEmpty()) && (identifier == null || identifier.isEmpty()) 3047 && (version == null || version.isEmpty()) && (name == null || name.isEmpty()) 3048 && (display == null || display.isEmpty()) && (status == null || status.isEmpty()) 3049 && (experimental == null || experimental.isEmpty()) && (publisher == null || publisher.isEmpty()) 3050 && (contact == null || contact.isEmpty()) && (date == null || date.isEmpty()) 3051 && (description == null || description.isEmpty()) && (useContext == null || useContext.isEmpty()) 3052 && (requirements == null || requirements.isEmpty()) && (copyright == null || copyright.isEmpty()) 3053 && (code == null || code.isEmpty()) && (fhirVersion == null || fhirVersion.isEmpty()) 3054 && (mapping == null || mapping.isEmpty()) && (kind == null || kind.isEmpty()) 3055 && (constrainedType == null || constrainedType.isEmpty()) && (abstract_ == null || abstract_.isEmpty()) 3056 && (contextType == null || contextType.isEmpty()) && (context == null || context.isEmpty()) 3057 && (base == null || base.isEmpty()) && (snapshot == null || snapshot.isEmpty()) 3058 && (differential == null || differential.isEmpty()); 3059 } 3060 3061 @Override 3062 public ResourceType getResourceType() { 3063 return ResourceType.StructureDefinition; 3064 } 3065 3066 @SearchParamDefinition(name = "date", path = "StructureDefinition.date", description = "The profile publication date", type = "date") 3067 public static final String SP_DATE = "date"; 3068 @SearchParamDefinition(name = "identifier", path = "StructureDefinition.identifier", description = "The identifier of the profile", type = "token") 3069 public static final String SP_IDENTIFIER = "identifier"; 3070 @SearchParamDefinition(name = "code", path = "StructureDefinition.code", description = "A code for the profile", type = "token") 3071 public static final String SP_CODE = "code"; 3072 @SearchParamDefinition(name = "valueset", path = "StructureDefinition.snapshot.element.binding.valueSet[x]", description = "A vocabulary binding reference", type = "reference") 3073 public static final String SP_VALUESET = "valueset"; 3074 @SearchParamDefinition(name = "kind", path = "StructureDefinition.kind", description = "datatype | resource | logical", type = "token") 3075 public static final String SP_KIND = "kind"; 3076 @SearchParamDefinition(name = "display", path = "StructureDefinition.display", description = "Use this name when displaying the value", type = "string") 3077 public static final String SP_DISPLAY = "display"; 3078 @SearchParamDefinition(name = "description", path = "StructureDefinition.description", description = "Text search in the description of the profile", type = "string") 3079 public static final String SP_DESCRIPTION = "description"; 3080 @SearchParamDefinition(name = "experimental", path = "StructureDefinition.experimental", description = "If for testing purposes, not real usage", type = "token") 3081 public static final String SP_EXPERIMENTAL = "experimental"; 3082 @SearchParamDefinition(name = "context-type", path = "StructureDefinition.contextType", description = "resource | datatype | mapping | extension", type = "token") 3083 public static final String SP_CONTEXTTYPE = "context-type"; 3084 @SearchParamDefinition(name = "abstract", path = "StructureDefinition.abstract", description = "Whether the structure is abstract", type = "token") 3085 public static final String SP_ABSTRACT = "abstract"; 3086 @SearchParamDefinition(name = "type", path = "StructureDefinition.constrainedType", description = "Any datatype or resource, including abstract ones", type = "token") 3087 public static final String SP_TYPE = "type"; 3088 @SearchParamDefinition(name = "version", path = "StructureDefinition.version", description = "The version identifier of the profile", type = "token") 3089 public static final String SP_VERSION = "version"; 3090 @SearchParamDefinition(name = "url", path = "StructureDefinition.url", description = "Absolute URL used to reference this StructureDefinition", type = "uri") 3091 public static final String SP_URL = "url"; 3092 @SearchParamDefinition(name = "path", path = "StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path", description = "A path that is constrained in the profile", type = "token") 3093 public static final String SP_PATH = "path"; 3094 @SearchParamDefinition(name = "ext-context", path = "StructureDefinition.context", description = "Where the extension can be used in instances", type = "string") 3095 public static final String SP_EXTCONTEXT = "ext-context"; 3096 @SearchParamDefinition(name = "name", path = "StructureDefinition.name", description = "Name of the profile", type = "string") 3097 public static final String SP_NAME = "name"; 3098 @SearchParamDefinition(name = "context", path = "StructureDefinition.useContext", description = "A use context assigned to the structure", type = "token") 3099 public static final String SP_CONTEXT = "context"; 3100 @SearchParamDefinition(name = "base-path", path = "StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path", description = "Path that identifies the base element", type = "token") 3101 public static final String SP_BASEPATH = "base-path"; 3102 @SearchParamDefinition(name = "publisher", path = "StructureDefinition.publisher", description = "Name of the publisher of the profile", type = "string") 3103 public static final String SP_PUBLISHER = "publisher"; 3104 @SearchParamDefinition(name = "status", path = "StructureDefinition.status", description = "The current status of the profile", type = "token") 3105 public static final String SP_STATUS = "status"; 3106 @SearchParamDefinition(name = "base", path = "StructureDefinition.base", description = "Structure that this set of constraints applies to", type = "uri") 3107 public static final String SP_BASE = "base"; 3108 3109}