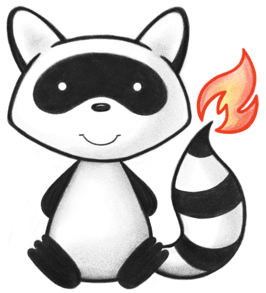
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * The subscription resource is used to define a push based subscription from a 048 * server to another system. Once a subscription is registered with the server, 049 * the server checks every resource that is created or updated, and if the 050 * resource matches the given criteria, it sends a message on the defined 051 * "channel" so that another system is able to take an appropriate action. 052 */ 053@ResourceDef(name = "Subscription", profile = "http://hl7.org/fhir/Profile/Subscription") 054public class Subscription extends DomainResource { 055 056 public enum SubscriptionStatus { 057 /** 058 * The client has requested the subscription, and the server has not yet set it 059 * up. 060 */ 061 REQUESTED, 062 /** 063 * The subscription is active. 064 */ 065 ACTIVE, 066 /** 067 * The server has an error executing the notification. 068 */ 069 ERROR, 070 /** 071 * Too many errors have occurred or the subscription has expired. 072 */ 073 OFF, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 079 public static SubscriptionStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("requested".equals(codeString)) 083 return REQUESTED; 084 if ("active".equals(codeString)) 085 return ACTIVE; 086 if ("error".equals(codeString)) 087 return ERROR; 088 if ("off".equals(codeString)) 089 return OFF; 090 throw new FHIRException("Unknown SubscriptionStatus code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case REQUESTED: 096 return "requested"; 097 case ACTIVE: 098 return "active"; 099 case ERROR: 100 return "error"; 101 case OFF: 102 return "off"; 103 case NULL: 104 return null; 105 default: 106 return "?"; 107 } 108 } 109 110 public String getSystem() { 111 switch (this) { 112 case REQUESTED: 113 return "http://hl7.org/fhir/subscription-status"; 114 case ACTIVE: 115 return "http://hl7.org/fhir/subscription-status"; 116 case ERROR: 117 return "http://hl7.org/fhir/subscription-status"; 118 case OFF: 119 return "http://hl7.org/fhir/subscription-status"; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDefinition() { 128 switch (this) { 129 case REQUESTED: 130 return "The client has requested the subscription, and the server has not yet set it up."; 131 case ACTIVE: 132 return "The subscription is active."; 133 case ERROR: 134 return "The server has an error executing the notification."; 135 case OFF: 136 return "Too many errors have occurred or the subscription has expired."; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getDisplay() { 145 switch (this) { 146 case REQUESTED: 147 return "Requested"; 148 case ACTIVE: 149 return "Active"; 150 case ERROR: 151 return "Error"; 152 case OFF: 153 return "Off"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 } 161 162 public static class SubscriptionStatusEnumFactory implements EnumFactory<SubscriptionStatus> { 163 public SubscriptionStatus fromCode(String codeString) throws IllegalArgumentException { 164 if (codeString == null || "".equals(codeString)) 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("requested".equals(codeString)) 168 return SubscriptionStatus.REQUESTED; 169 if ("active".equals(codeString)) 170 return SubscriptionStatus.ACTIVE; 171 if ("error".equals(codeString)) 172 return SubscriptionStatus.ERROR; 173 if ("off".equals(codeString)) 174 return SubscriptionStatus.OFF; 175 throw new IllegalArgumentException("Unknown SubscriptionStatus code '" + codeString + "'"); 176 } 177 178 public Enumeration<SubscriptionStatus> fromType(Base code) throws FHIRException { 179 if (code == null || code.isEmpty()) 180 return null; 181 String codeString = ((PrimitiveType) code).asStringValue(); 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("requested".equals(codeString)) 185 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.REQUESTED); 186 if ("active".equals(codeString)) 187 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.ACTIVE); 188 if ("error".equals(codeString)) 189 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.ERROR); 190 if ("off".equals(codeString)) 191 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.OFF); 192 throw new FHIRException("Unknown SubscriptionStatus code '" + codeString + "'"); 193 } 194 195 public String toCode(SubscriptionStatus code) { 196 if (code == SubscriptionStatus.REQUESTED) 197 return "requested"; 198 if (code == SubscriptionStatus.ACTIVE) 199 return "active"; 200 if (code == SubscriptionStatus.ERROR) 201 return "error"; 202 if (code == SubscriptionStatus.OFF) 203 return "off"; 204 return "?"; 205 } 206 } 207 208 public enum SubscriptionChannelType { 209 /** 210 * The channel is executed by making a post to the URI. If a payload is 211 * included, the URL is interpreted as the service base, and an update (PUT) is 212 * made. 213 */ 214 RESTHOOK, 215 /** 216 * The channel is executed by sending a packet across a web socket connection 217 * maintained by the client. The URL identifies the websocket, and the client 218 * binds to this URL. 219 */ 220 WEBSOCKET, 221 /** 222 * The channel is executed by sending an email to the email addressed in the URI 223 * (which must be a mailto:). 224 */ 225 EMAIL, 226 /** 227 * The channel is executed by sending an SMS message to the phone number 228 * identified in the URL (tel:). 229 */ 230 SMS, 231 /** 232 * The channel is executed by sending a message (e.g. a Bundle with a 233 * MessageHeader resource etc.) to the application identified in the URI. 234 */ 235 MESSAGE, 236 /** 237 * added to help the parsers 238 */ 239 NULL; 240 241 public static SubscriptionChannelType fromCode(String codeString) throws FHIRException { 242 if (codeString == null || "".equals(codeString)) 243 return null; 244 if ("rest-hook".equals(codeString)) 245 return RESTHOOK; 246 if ("websocket".equals(codeString)) 247 return WEBSOCKET; 248 if ("email".equals(codeString)) 249 return EMAIL; 250 if ("sms".equals(codeString)) 251 return SMS; 252 if ("message".equals(codeString)) 253 return MESSAGE; 254 throw new FHIRException("Unknown SubscriptionChannelType code '" + codeString + "'"); 255 } 256 257 public String toCode() { 258 switch (this) { 259 case RESTHOOK: 260 return "rest-hook"; 261 case WEBSOCKET: 262 return "websocket"; 263 case EMAIL: 264 return "email"; 265 case SMS: 266 return "sms"; 267 case MESSAGE: 268 return "message"; 269 case NULL: 270 return null; 271 default: 272 return "?"; 273 } 274 } 275 276 public String getSystem() { 277 switch (this) { 278 case RESTHOOK: 279 return "http://hl7.org/fhir/subscription-channel-type"; 280 case WEBSOCKET: 281 return "http://hl7.org/fhir/subscription-channel-type"; 282 case EMAIL: 283 return "http://hl7.org/fhir/subscription-channel-type"; 284 case SMS: 285 return "http://hl7.org/fhir/subscription-channel-type"; 286 case MESSAGE: 287 return "http://hl7.org/fhir/subscription-channel-type"; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 295 public String getDefinition() { 296 switch (this) { 297 case RESTHOOK: 298 return "The channel is executed by making a post to the URI. If a payload is included, the URL is interpreted as the service base, and an update (PUT) is made."; 299 case WEBSOCKET: 300 return "The channel is executed by sending a packet across a web socket connection maintained by the client. The URL identifies the websocket, and the client binds to this URL."; 301 case EMAIL: 302 return "The channel is executed by sending an email to the email addressed in the URI (which must be a mailto:)."; 303 case SMS: 304 return "The channel is executed by sending an SMS message to the phone number identified in the URL (tel:)."; 305 case MESSAGE: 306 return "The channel is executed by sending a message (e.g. a Bundle with a MessageHeader resource etc.) to the application identified in the URI."; 307 case NULL: 308 return null; 309 default: 310 return "?"; 311 } 312 } 313 314 public String getDisplay() { 315 switch (this) { 316 case RESTHOOK: 317 return "Rest Hook"; 318 case WEBSOCKET: 319 return "Websocket"; 320 case EMAIL: 321 return "Email"; 322 case SMS: 323 return "SMS"; 324 case MESSAGE: 325 return "Message"; 326 case NULL: 327 return null; 328 default: 329 return "?"; 330 } 331 } 332 } 333 334 public static class SubscriptionChannelTypeEnumFactory implements EnumFactory<SubscriptionChannelType> { 335 public SubscriptionChannelType fromCode(String codeString) throws IllegalArgumentException { 336 if (codeString == null || "".equals(codeString)) 337 if (codeString == null || "".equals(codeString)) 338 return null; 339 if ("rest-hook".equals(codeString)) 340 return SubscriptionChannelType.RESTHOOK; 341 if ("websocket".equals(codeString)) 342 return SubscriptionChannelType.WEBSOCKET; 343 if ("email".equals(codeString)) 344 return SubscriptionChannelType.EMAIL; 345 if ("sms".equals(codeString)) 346 return SubscriptionChannelType.SMS; 347 if ("message".equals(codeString)) 348 return SubscriptionChannelType.MESSAGE; 349 throw new IllegalArgumentException("Unknown SubscriptionChannelType code '" + codeString + "'"); 350 } 351 352 public Enumeration<SubscriptionChannelType> fromType(Base code) throws FHIRException { 353 if (code == null || code.isEmpty()) 354 return null; 355 String codeString = ((PrimitiveType) code).asStringValue(); 356 if (codeString == null || "".equals(codeString)) 357 return null; 358 if ("rest-hook".equals(codeString)) 359 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.RESTHOOK); 360 if ("websocket".equals(codeString)) 361 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.WEBSOCKET); 362 if ("email".equals(codeString)) 363 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.EMAIL); 364 if ("sms".equals(codeString)) 365 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.SMS); 366 if ("message".equals(codeString)) 367 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.MESSAGE); 368 throw new FHIRException("Unknown SubscriptionChannelType code '" + codeString + "'"); 369 } 370 371 public String toCode(SubscriptionChannelType code) { 372 if (code == SubscriptionChannelType.RESTHOOK) 373 return "rest-hook"; 374 if (code == SubscriptionChannelType.WEBSOCKET) 375 return "websocket"; 376 if (code == SubscriptionChannelType.EMAIL) 377 return "email"; 378 if (code == SubscriptionChannelType.SMS) 379 return "sms"; 380 if (code == SubscriptionChannelType.MESSAGE) 381 return "message"; 382 return "?"; 383 } 384 } 385 386 @Block() 387 public static class SubscriptionChannelComponent extends BackboneElement implements IBaseBackboneElement { 388 /** 389 * The type of channel to send notifications on. 390 */ 391 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 392 @Description(shortDefinition = "rest-hook | websocket | email | sms | message", formalDefinition = "The type of channel to send notifications on.") 393 protected Enumeration<SubscriptionChannelType> type; 394 395 /** 396 * The uri that describes the actual end-point to send messages to. 397 */ 398 @Child(name = "endpoint", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 399 @Description(shortDefinition = "Where the channel points to", formalDefinition = "The uri that describes the actual end-point to send messages to.") 400 protected UriType endpoint; 401 402 /** 403 * The mime type to send the payload in - either application/xml+fhir, or 404 * application/json+fhir. If the mime type is blank, then there is no payload in 405 * the notification, just a notification. 406 */ 407 @Child(name = "payload", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 408 @Description(shortDefinition = "Mimetype to send, or blank for no payload", formalDefinition = "The mime type to send the payload in - either application/xml+fhir, or application/json+fhir. If the mime type is blank, then there is no payload in the notification, just a notification.") 409 protected StringType payload; 410 411 /** 412 * Additional headers / information to send as part of the notification. 413 */ 414 @Child(name = "header", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 415 @Description(shortDefinition = "Usage depends on the channel type", formalDefinition = "Additional headers / information to send as part of the notification.") 416 protected StringType header; 417 418 private static final long serialVersionUID = -279715391L; 419 420 /* 421 * Constructor 422 */ 423 public SubscriptionChannelComponent() { 424 super(); 425 } 426 427 /* 428 * Constructor 429 */ 430 public SubscriptionChannelComponent(Enumeration<SubscriptionChannelType> type, StringType payload) { 431 super(); 432 this.type = type; 433 this.payload = payload; 434 } 435 436 /** 437 * @return {@link #type} (The type of channel to send notifications on.). This 438 * is the underlying object with id, value and extensions. The accessor 439 * "getType" gives direct access to the value 440 */ 441 public Enumeration<SubscriptionChannelType> getTypeElement() { 442 if (this.type == null) 443 if (Configuration.errorOnAutoCreate()) 444 throw new Error("Attempt to auto-create SubscriptionChannelComponent.type"); 445 else if (Configuration.doAutoCreate()) 446 this.type = new Enumeration<SubscriptionChannelType>(new SubscriptionChannelTypeEnumFactory()); // bb 447 return this.type; 448 } 449 450 public boolean hasTypeElement() { 451 return this.type != null && !this.type.isEmpty(); 452 } 453 454 public boolean hasType() { 455 return this.type != null && !this.type.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #type} (The type of channel to send notifications on.). 460 * This is the underlying object with id, value and extensions. The 461 * accessor "getType" gives direct access to the value 462 */ 463 public SubscriptionChannelComponent setTypeElement(Enumeration<SubscriptionChannelType> value) { 464 this.type = value; 465 return this; 466 } 467 468 /** 469 * @return The type of channel to send notifications on. 470 */ 471 public SubscriptionChannelType getType() { 472 return this.type == null ? null : this.type.getValue(); 473 } 474 475 /** 476 * @param value The type of channel to send notifications on. 477 */ 478 public SubscriptionChannelComponent setType(SubscriptionChannelType value) { 479 if (this.type == null) 480 this.type = new Enumeration<SubscriptionChannelType>(new SubscriptionChannelTypeEnumFactory()); 481 this.type.setValue(value); 482 return this; 483 } 484 485 /** 486 * @return {@link #endpoint} (The uri that describes the actual end-point to 487 * send messages to.). This is the underlying object with id, value and 488 * extensions. The accessor "getEndpoint" gives direct access to the 489 * value 490 */ 491 public UriType getEndpointElement() { 492 if (this.endpoint == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create SubscriptionChannelComponent.endpoint"); 495 else if (Configuration.doAutoCreate()) 496 this.endpoint = new UriType(); // bb 497 return this.endpoint; 498 } 499 500 public boolean hasEndpointElement() { 501 return this.endpoint != null && !this.endpoint.isEmpty(); 502 } 503 504 public boolean hasEndpoint() { 505 return this.endpoint != null && !this.endpoint.isEmpty(); 506 } 507 508 /** 509 * @param value {@link #endpoint} (The uri that describes the actual end-point 510 * to send messages to.). This is the underlying object with id, 511 * value and extensions. The accessor "getEndpoint" gives direct 512 * access to the value 513 */ 514 public SubscriptionChannelComponent setEndpointElement(UriType value) { 515 this.endpoint = value; 516 return this; 517 } 518 519 /** 520 * @return The uri that describes the actual end-point to send messages to. 521 */ 522 public String getEndpoint() { 523 return this.endpoint == null ? null : this.endpoint.getValue(); 524 } 525 526 /** 527 * @param value The uri that describes the actual end-point to send messages to. 528 */ 529 public SubscriptionChannelComponent setEndpoint(String value) { 530 if (Utilities.noString(value)) 531 this.endpoint = null; 532 else { 533 if (this.endpoint == null) 534 this.endpoint = new UriType(); 535 this.endpoint.setValue(value); 536 } 537 return this; 538 } 539 540 /** 541 * @return {@link #payload} (The mime type to send the payload in - either 542 * application/xml+fhir, or application/json+fhir. If the mime type is 543 * blank, then there is no payload in the notification, just a 544 * notification.). This is the underlying object with id, value and 545 * extensions. The accessor "getPayload" gives direct access to the 546 * value 547 */ 548 public StringType getPayloadElement() { 549 if (this.payload == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create SubscriptionChannelComponent.payload"); 552 else if (Configuration.doAutoCreate()) 553 this.payload = new StringType(); // bb 554 return this.payload; 555 } 556 557 public boolean hasPayloadElement() { 558 return this.payload != null && !this.payload.isEmpty(); 559 } 560 561 public boolean hasPayload() { 562 return this.payload != null && !this.payload.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #payload} (The mime type to send the payload in - either 567 * application/xml+fhir, or application/json+fhir. If the mime type 568 * is blank, then there is no payload in the notification, just a 569 * notification.). This is the underlying object with id, value and 570 * extensions. The accessor "getPayload" gives direct access to the 571 * value 572 */ 573 public SubscriptionChannelComponent setPayloadElement(StringType value) { 574 this.payload = value; 575 return this; 576 } 577 578 /** 579 * @return The mime type to send the payload in - either application/xml+fhir, 580 * or application/json+fhir. If the mime type is blank, then there is no 581 * payload in the notification, just a notification. 582 */ 583 public String getPayload() { 584 return this.payload == null ? null : this.payload.getValue(); 585 } 586 587 /** 588 * @param value The mime type to send the payload in - either 589 * application/xml+fhir, or application/json+fhir. If the mime type 590 * is blank, then there is no payload in the notification, just a 591 * notification. 592 */ 593 public SubscriptionChannelComponent setPayload(String value) { 594 if (this.payload == null) 595 this.payload = new StringType(); 596 this.payload.setValue(value); 597 return this; 598 } 599 600 /** 601 * @return {@link #header} (Additional headers / information to send as part of 602 * the notification.). This is the underlying object with id, value and 603 * extensions. The accessor "getHeader" gives direct access to the value 604 */ 605 public StringType getHeaderElement() { 606 if (this.header == null) 607 if (Configuration.errorOnAutoCreate()) 608 throw new Error("Attempt to auto-create SubscriptionChannelComponent.header"); 609 else if (Configuration.doAutoCreate()) 610 this.header = new StringType(); // bb 611 return this.header; 612 } 613 614 public boolean hasHeaderElement() { 615 return this.header != null && !this.header.isEmpty(); 616 } 617 618 public boolean hasHeader() { 619 return this.header != null && !this.header.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #header} (Additional headers / information to send as 624 * part of the notification.). This is the underlying object with 625 * id, value and extensions. The accessor "getHeader" gives direct 626 * access to the value 627 */ 628 public SubscriptionChannelComponent setHeaderElement(StringType value) { 629 this.header = value; 630 return this; 631 } 632 633 /** 634 * @return Additional headers / information to send as part of the notification. 635 */ 636 public String getHeader() { 637 return this.header == null ? null : this.header.getValue(); 638 } 639 640 /** 641 * @param value Additional headers / information to send as part of the 642 * notification. 643 */ 644 public SubscriptionChannelComponent setHeader(String value) { 645 if (Utilities.noString(value)) 646 this.header = null; 647 else { 648 if (this.header == null) 649 this.header = new StringType(); 650 this.header.setValue(value); 651 } 652 return this; 653 } 654 655 protected void listChildren(List<Property> childrenList) { 656 super.listChildren(childrenList); 657 childrenList.add(new Property("type", "code", "The type of channel to send notifications on.", 0, 658 java.lang.Integer.MAX_VALUE, type)); 659 childrenList 660 .add(new Property("endpoint", "uri", "The uri that describes the actual end-point to send messages to.", 0, 661 java.lang.Integer.MAX_VALUE, endpoint)); 662 childrenList.add(new Property("payload", "string", 663 "The mime type to send the payload in - either application/xml+fhir, or application/json+fhir. If the mime type is blank, then there is no payload in the notification, just a notification.", 664 0, java.lang.Integer.MAX_VALUE, payload)); 665 childrenList 666 .add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 667 0, java.lang.Integer.MAX_VALUE, header)); 668 } 669 670 @Override 671 public void setProperty(String name, Base value) throws FHIRException { 672 if (name.equals("type")) 673 this.type = new SubscriptionChannelTypeEnumFactory().fromType(value); // Enumeration<SubscriptionChannelType> 674 else if (name.equals("endpoint")) 675 this.endpoint = castToUri(value); // UriType 676 else if (name.equals("payload")) 677 this.payload = castToString(value); // StringType 678 else if (name.equals("header")) 679 this.header = castToString(value); // StringType 680 else 681 super.setProperty(name, value); 682 } 683 684 @Override 685 public Base addChild(String name) throws FHIRException { 686 if (name.equals("type")) { 687 throw new FHIRException("Cannot call addChild on a singleton property Subscription.type"); 688 } else if (name.equals("endpoint")) { 689 throw new FHIRException("Cannot call addChild on a singleton property Subscription.endpoint"); 690 } else if (name.equals("payload")) { 691 throw new FHIRException("Cannot call addChild on a singleton property Subscription.payload"); 692 } else if (name.equals("header")) { 693 throw new FHIRException("Cannot call addChild on a singleton property Subscription.header"); 694 } else 695 return super.addChild(name); 696 } 697 698 public SubscriptionChannelComponent copy() { 699 SubscriptionChannelComponent dst = new SubscriptionChannelComponent(); 700 copyValues(dst); 701 dst.type = type == null ? null : type.copy(); 702 dst.endpoint = endpoint == null ? null : endpoint.copy(); 703 dst.payload = payload == null ? null : payload.copy(); 704 dst.header = header == null ? null : header.copy(); 705 return dst; 706 } 707 708 @Override 709 public boolean equalsDeep(Base other) { 710 if (!super.equalsDeep(other)) 711 return false; 712 if (!(other instanceof SubscriptionChannelComponent)) 713 return false; 714 SubscriptionChannelComponent o = (SubscriptionChannelComponent) other; 715 return compareDeep(type, o.type, true) && compareDeep(endpoint, o.endpoint, true) 716 && compareDeep(payload, o.payload, true) && compareDeep(header, o.header, true); 717 } 718 719 @Override 720 public boolean equalsShallow(Base other) { 721 if (!super.equalsShallow(other)) 722 return false; 723 if (!(other instanceof SubscriptionChannelComponent)) 724 return false; 725 SubscriptionChannelComponent o = (SubscriptionChannelComponent) other; 726 return compareValues(type, o.type, true) && compareValues(endpoint, o.endpoint, true) 727 && compareValues(payload, o.payload, true) && compareValues(header, o.header, true); 728 } 729 730 public boolean isEmpty() { 731 return super.isEmpty() && (type == null || type.isEmpty()) && (endpoint == null || endpoint.isEmpty()) 732 && (payload == null || payload.isEmpty()) && (header == null || header.isEmpty()); 733 } 734 735 public String fhirType() { 736 return "Subscription.channel"; 737 738 } 739 740 } 741 742 /** 743 * The rules that the server should use to determine when to generate 744 * notifications for this subscription. 745 */ 746 @Child(name = "criteria", type = { StringType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 747 @Description(shortDefinition = "Rule for server push criteria", formalDefinition = "The rules that the server should use to determine when to generate notifications for this subscription.") 748 protected StringType criteria; 749 750 /** 751 * Contact details for a human to contact about the subscription. The primary 752 * use of this for system administrator troubleshooting. 753 */ 754 @Child(name = "contact", type = { 755 ContactPoint.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 756 @Description(shortDefinition = "Contact details for source (e.g. troubleshooting)", formalDefinition = "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.") 757 protected List<ContactPoint> contact; 758 759 /** 760 * A description of why this subscription is defined. 761 */ 762 @Child(name = "reason", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 763 @Description(shortDefinition = "Description of why this subscription was created", formalDefinition = "A description of why this subscription is defined.") 764 protected StringType reason; 765 766 /** 767 * The status of the subscription, which marks the server state for managing the 768 * subscription. 769 */ 770 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 771 @Description(shortDefinition = "requested | active | error | off", formalDefinition = "The status of the subscription, which marks the server state for managing the subscription.") 772 protected Enumeration<SubscriptionStatus> status; 773 774 /** 775 * A record of the last error that occurred when the server processed a 776 * notification. 777 */ 778 @Child(name = "error", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 779 @Description(shortDefinition = "Latest error note", formalDefinition = "A record of the last error that occurred when the server processed a notification.") 780 protected StringType error; 781 782 /** 783 * Details where to send notifications when resources are received that meet the 784 * criteria. 785 */ 786 @Child(name = "channel", type = {}, order = 5, min = 1, max = 1, modifier = false, summary = true) 787 @Description(shortDefinition = "The channel on which to report matches to the criteria", formalDefinition = "Details where to send notifications when resources are received that meet the criteria.") 788 protected SubscriptionChannelComponent channel; 789 790 /** 791 * The time for the server to turn the subscription off. 792 */ 793 @Child(name = "end", type = { InstantType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 794 @Description(shortDefinition = "When to automatically delete the subscription", formalDefinition = "The time for the server to turn the subscription off.") 795 protected InstantType end; 796 797 /** 798 * A tag to add to any resource that matches the criteria, after the 799 * subscription is processed. 800 */ 801 @Child(name = "tag", type = { 802 Coding.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 803 @Description(shortDefinition = "A tag to add to matching resources", formalDefinition = "A tag to add to any resource that matches the criteria, after the subscription is processed.") 804 protected List<Coding> tag; 805 806 private static final long serialVersionUID = -1390870804L; 807 808 /* 809 * Constructor 810 */ 811 public Subscription() { 812 super(); 813 } 814 815 /* 816 * Constructor 817 */ 818 public Subscription(StringType criteria, StringType reason, Enumeration<SubscriptionStatus> status, 819 SubscriptionChannelComponent channel) { 820 super(); 821 this.criteria = criteria; 822 this.reason = reason; 823 this.status = status; 824 this.channel = channel; 825 } 826 827 /** 828 * @return {@link #criteria} (The rules that the server should use to determine 829 * when to generate notifications for this subscription.). This is the 830 * underlying object with id, value and extensions. The accessor 831 * "getCriteria" gives direct access to the value 832 */ 833 public StringType getCriteriaElement() { 834 if (this.criteria == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create Subscription.criteria"); 837 else if (Configuration.doAutoCreate()) 838 this.criteria = new StringType(); // bb 839 return this.criteria; 840 } 841 842 public boolean hasCriteriaElement() { 843 return this.criteria != null && !this.criteria.isEmpty(); 844 } 845 846 public boolean hasCriteria() { 847 return this.criteria != null && !this.criteria.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #criteria} (The rules that the server should use to 852 * determine when to generate notifications for this 853 * subscription.). This is the underlying object with id, value and 854 * extensions. The accessor "getCriteria" gives direct access to 855 * the value 856 */ 857 public Subscription setCriteriaElement(StringType value) { 858 this.criteria = value; 859 return this; 860 } 861 862 /** 863 * @return The rules that the server should use to determine when to generate 864 * notifications for this subscription. 865 */ 866 public String getCriteria() { 867 return this.criteria == null ? null : this.criteria.getValue(); 868 } 869 870 /** 871 * @param value The rules that the server should use to determine when to 872 * generate notifications for this subscription. 873 */ 874 public Subscription setCriteria(String value) { 875 if (this.criteria == null) 876 this.criteria = new StringType(); 877 this.criteria.setValue(value); 878 return this; 879 } 880 881 /** 882 * @return {@link #contact} (Contact details for a human to contact about the 883 * subscription. The primary use of this for system administrator 884 * troubleshooting.) 885 */ 886 public List<ContactPoint> getContact() { 887 if (this.contact == null) 888 this.contact = new ArrayList<ContactPoint>(); 889 return this.contact; 890 } 891 892 public boolean hasContact() { 893 if (this.contact == null) 894 return false; 895 for (ContactPoint item : this.contact) 896 if (!item.isEmpty()) 897 return true; 898 return false; 899 } 900 901 /** 902 * @return {@link #contact} (Contact details for a human to contact about the 903 * subscription. The primary use of this for system administrator 904 * troubleshooting.) 905 */ 906 // syntactic sugar 907 public ContactPoint addContact() { // 3 908 ContactPoint t = new ContactPoint(); 909 if (this.contact == null) 910 this.contact = new ArrayList<ContactPoint>(); 911 this.contact.add(t); 912 return t; 913 } 914 915 // syntactic sugar 916 public Subscription addContact(ContactPoint t) { // 3 917 if (t == null) 918 return this; 919 if (this.contact == null) 920 this.contact = new ArrayList<ContactPoint>(); 921 this.contact.add(t); 922 return this; 923 } 924 925 /** 926 * @return {@link #reason} (A description of why this subscription is defined.). 927 * This is the underlying object with id, value and extensions. The 928 * accessor "getReason" gives direct access to the value 929 */ 930 public StringType getReasonElement() { 931 if (this.reason == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create Subscription.reason"); 934 else if (Configuration.doAutoCreate()) 935 this.reason = new StringType(); // bb 936 return this.reason; 937 } 938 939 public boolean hasReasonElement() { 940 return this.reason != null && !this.reason.isEmpty(); 941 } 942 943 public boolean hasReason() { 944 return this.reason != null && !this.reason.isEmpty(); 945 } 946 947 /** 948 * @param value {@link #reason} (A description of why this subscription is 949 * defined.). This is the underlying object with id, value and 950 * extensions. The accessor "getReason" gives direct access to the 951 * value 952 */ 953 public Subscription setReasonElement(StringType value) { 954 this.reason = value; 955 return this; 956 } 957 958 /** 959 * @return A description of why this subscription is defined. 960 */ 961 public String getReason() { 962 return this.reason == null ? null : this.reason.getValue(); 963 } 964 965 /** 966 * @param value A description of why this subscription is defined. 967 */ 968 public Subscription setReason(String value) { 969 if (this.reason == null) 970 this.reason = new StringType(); 971 this.reason.setValue(value); 972 return this; 973 } 974 975 /** 976 * @return {@link #status} (The status of the subscription, which marks the 977 * server state for managing the subscription.). This is the underlying 978 * object with id, value and extensions. The accessor "getStatus" gives 979 * direct access to the value 980 */ 981 public Enumeration<SubscriptionStatus> getStatusElement() { 982 if (this.status == null) 983 if (Configuration.errorOnAutoCreate()) 984 throw new Error("Attempt to auto-create Subscription.status"); 985 else if (Configuration.doAutoCreate()) 986 this.status = new Enumeration<SubscriptionStatus>(new SubscriptionStatusEnumFactory()); // bb 987 return this.status; 988 } 989 990 public boolean hasStatusElement() { 991 return this.status != null && !this.status.isEmpty(); 992 } 993 994 public boolean hasStatus() { 995 return this.status != null && !this.status.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #status} (The status of the subscription, which marks the 1000 * server state for managing the subscription.). This is the 1001 * underlying object with id, value and extensions. The accessor 1002 * "getStatus" gives direct access to the value 1003 */ 1004 public Subscription setStatusElement(Enumeration<SubscriptionStatus> value) { 1005 this.status = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return The status of the subscription, which marks the server state for 1011 * managing the subscription. 1012 */ 1013 public SubscriptionStatus getStatus() { 1014 return this.status == null ? null : this.status.getValue(); 1015 } 1016 1017 /** 1018 * @param value The status of the subscription, which marks the server state for 1019 * managing the subscription. 1020 */ 1021 public Subscription setStatus(SubscriptionStatus value) { 1022 if (this.status == null) 1023 this.status = new Enumeration<SubscriptionStatus>(new SubscriptionStatusEnumFactory()); 1024 this.status.setValue(value); 1025 return this; 1026 } 1027 1028 /** 1029 * @return {@link #error} (A record of the last error that occurred when the 1030 * server processed a notification.). This is the underlying object with 1031 * id, value and extensions. The accessor "getError" gives direct access 1032 * to the value 1033 */ 1034 public StringType getErrorElement() { 1035 if (this.error == null) 1036 if (Configuration.errorOnAutoCreate()) 1037 throw new Error("Attempt to auto-create Subscription.error"); 1038 else if (Configuration.doAutoCreate()) 1039 this.error = new StringType(); // bb 1040 return this.error; 1041 } 1042 1043 public boolean hasErrorElement() { 1044 return this.error != null && !this.error.isEmpty(); 1045 } 1046 1047 public boolean hasError() { 1048 return this.error != null && !this.error.isEmpty(); 1049 } 1050 1051 /** 1052 * @param value {@link #error} (A record of the last error that occurred when 1053 * the server processed a notification.). This is the underlying 1054 * object with id, value and extensions. The accessor "getError" 1055 * gives direct access to the value 1056 */ 1057 public Subscription setErrorElement(StringType value) { 1058 this.error = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return A record of the last error that occurred when the server processed a 1064 * notification. 1065 */ 1066 public String getError() { 1067 return this.error == null ? null : this.error.getValue(); 1068 } 1069 1070 /** 1071 * @param value A record of the last error that occurred when the server 1072 * processed a notification. 1073 */ 1074 public Subscription setError(String value) { 1075 if (Utilities.noString(value)) 1076 this.error = null; 1077 else { 1078 if (this.error == null) 1079 this.error = new StringType(); 1080 this.error.setValue(value); 1081 } 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #channel} (Details where to send notifications when resources 1087 * are received that meet the criteria.) 1088 */ 1089 public SubscriptionChannelComponent getChannel() { 1090 if (this.channel == null) 1091 if (Configuration.errorOnAutoCreate()) 1092 throw new Error("Attempt to auto-create Subscription.channel"); 1093 else if (Configuration.doAutoCreate()) 1094 this.channel = new SubscriptionChannelComponent(); // cc 1095 return this.channel; 1096 } 1097 1098 public boolean hasChannel() { 1099 return this.channel != null && !this.channel.isEmpty(); 1100 } 1101 1102 /** 1103 * @param value {@link #channel} (Details where to send notifications when 1104 * resources are received that meet the criteria.) 1105 */ 1106 public Subscription setChannel(SubscriptionChannelComponent value) { 1107 this.channel = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return {@link #end} (The time for the server to turn the subscription off.). 1113 * This is the underlying object with id, value and extensions. The 1114 * accessor "getEnd" gives direct access to the value 1115 */ 1116 public InstantType getEndElement() { 1117 if (this.end == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create Subscription.end"); 1120 else if (Configuration.doAutoCreate()) 1121 this.end = new InstantType(); // bb 1122 return this.end; 1123 } 1124 1125 public boolean hasEndElement() { 1126 return this.end != null && !this.end.isEmpty(); 1127 } 1128 1129 public boolean hasEnd() { 1130 return this.end != null && !this.end.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #end} (The time for the server to turn the subscription 1135 * off.). This is the underlying object with id, value and 1136 * extensions. The accessor "getEnd" gives direct access to the 1137 * value 1138 */ 1139 public Subscription setEndElement(InstantType value) { 1140 this.end = value; 1141 return this; 1142 } 1143 1144 /** 1145 * @return The time for the server to turn the subscription off. 1146 */ 1147 public Date getEnd() { 1148 return this.end == null ? null : this.end.getValue(); 1149 } 1150 1151 /** 1152 * @param value The time for the server to turn the subscription off. 1153 */ 1154 public Subscription setEnd(Date value) { 1155 if (value == null) 1156 this.end = null; 1157 else { 1158 if (this.end == null) 1159 this.end = new InstantType(); 1160 this.end.setValue(value); 1161 } 1162 return this; 1163 } 1164 1165 /** 1166 * @return {@link #tag} (A tag to add to any resource that matches the criteria, 1167 * after the subscription is processed.) 1168 */ 1169 public List<Coding> getTag() { 1170 if (this.tag == null) 1171 this.tag = new ArrayList<Coding>(); 1172 return this.tag; 1173 } 1174 1175 public boolean hasTag() { 1176 if (this.tag == null) 1177 return false; 1178 for (Coding item : this.tag) 1179 if (!item.isEmpty()) 1180 return true; 1181 return false; 1182 } 1183 1184 /** 1185 * @return {@link #tag} (A tag to add to any resource that matches the criteria, 1186 * after the subscription is processed.) 1187 */ 1188 // syntactic sugar 1189 public Coding addTag() { // 3 1190 Coding t = new Coding(); 1191 if (this.tag == null) 1192 this.tag = new ArrayList<Coding>(); 1193 this.tag.add(t); 1194 return t; 1195 } 1196 1197 // syntactic sugar 1198 public Subscription addTag(Coding t) { // 3 1199 if (t == null) 1200 return this; 1201 if (this.tag == null) 1202 this.tag = new ArrayList<Coding>(); 1203 this.tag.add(t); 1204 return this; 1205 } 1206 1207 protected void listChildren(List<Property> childrenList) { 1208 super.listChildren(childrenList); 1209 childrenList.add(new Property("criteria", "string", 1210 "The rules that the server should use to determine when to generate notifications for this subscription.", 0, 1211 java.lang.Integer.MAX_VALUE, criteria)); 1212 childrenList.add(new Property("contact", "ContactPoint", 1213 "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 1214 0, java.lang.Integer.MAX_VALUE, contact)); 1215 childrenList.add(new Property("reason", "string", "A description of why this subscription is defined.", 0, 1216 java.lang.Integer.MAX_VALUE, reason)); 1217 childrenList.add(new Property("status", "code", 1218 "The status of the subscription, which marks the server state for managing the subscription.", 0, 1219 java.lang.Integer.MAX_VALUE, status)); 1220 childrenList.add(new Property("error", "string", 1221 "A record of the last error that occurred when the server processed a notification.", 0, 1222 java.lang.Integer.MAX_VALUE, error)); 1223 childrenList.add(new Property("channel", "", 1224 "Details where to send notifications when resources are received that meet the criteria.", 0, 1225 java.lang.Integer.MAX_VALUE, channel)); 1226 childrenList.add(new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1227 java.lang.Integer.MAX_VALUE, end)); 1228 childrenList.add(new Property("tag", "Coding", 1229 "A tag to add to any resource that matches the criteria, after the subscription is processed.", 0, 1230 java.lang.Integer.MAX_VALUE, tag)); 1231 } 1232 1233 @Override 1234 public void setProperty(String name, Base value) throws FHIRException { 1235 if (name.equals("criteria")) 1236 this.criteria = castToString(value); // StringType 1237 else if (name.equals("contact")) 1238 this.getContact().add(castToContactPoint(value)); 1239 else if (name.equals("reason")) 1240 this.reason = castToString(value); // StringType 1241 else if (name.equals("status")) 1242 this.status = new SubscriptionStatusEnumFactory().fromType(value); // Enumeration<SubscriptionStatus> 1243 else if (name.equals("error")) 1244 this.error = castToString(value); // StringType 1245 else if (name.equals("channel")) 1246 this.channel = (SubscriptionChannelComponent) value; // SubscriptionChannelComponent 1247 else if (name.equals("end")) 1248 this.end = castToInstant(value); // InstantType 1249 else if (name.equals("tag")) 1250 this.getTag().add(castToCoding(value)); 1251 else 1252 super.setProperty(name, value); 1253 } 1254 1255 @Override 1256 public Base addChild(String name) throws FHIRException { 1257 if (name.equals("criteria")) { 1258 throw new FHIRException("Cannot call addChild on a singleton property Subscription.criteria"); 1259 } else if (name.equals("contact")) { 1260 return addContact(); 1261 } else if (name.equals("reason")) { 1262 throw new FHIRException("Cannot call addChild on a singleton property Subscription.reason"); 1263 } else if (name.equals("status")) { 1264 throw new FHIRException("Cannot call addChild on a singleton property Subscription.status"); 1265 } else if (name.equals("error")) { 1266 throw new FHIRException("Cannot call addChild on a singleton property Subscription.error"); 1267 } else if (name.equals("channel")) { 1268 this.channel = new SubscriptionChannelComponent(); 1269 return this.channel; 1270 } else if (name.equals("end")) { 1271 throw new FHIRException("Cannot call addChild on a singleton property Subscription.end"); 1272 } else if (name.equals("tag")) { 1273 return addTag(); 1274 } else 1275 return super.addChild(name); 1276 } 1277 1278 public String fhirType() { 1279 return "Subscription"; 1280 1281 } 1282 1283 public Subscription copy() { 1284 Subscription dst = new Subscription(); 1285 copyValues(dst); 1286 dst.criteria = criteria == null ? null : criteria.copy(); 1287 if (contact != null) { 1288 dst.contact = new ArrayList<ContactPoint>(); 1289 for (ContactPoint i : contact) 1290 dst.contact.add(i.copy()); 1291 } 1292 ; 1293 dst.reason = reason == null ? null : reason.copy(); 1294 dst.status = status == null ? null : status.copy(); 1295 dst.error = error == null ? null : error.copy(); 1296 dst.channel = channel == null ? null : channel.copy(); 1297 dst.end = end == null ? null : end.copy(); 1298 if (tag != null) { 1299 dst.tag = new ArrayList<Coding>(); 1300 for (Coding i : tag) 1301 dst.tag.add(i.copy()); 1302 } 1303 ; 1304 return dst; 1305 } 1306 1307 protected Subscription typedCopy() { 1308 return copy(); 1309 } 1310 1311 @Override 1312 public boolean equalsDeep(Base other) { 1313 if (!super.equalsDeep(other)) 1314 return false; 1315 if (!(other instanceof Subscription)) 1316 return false; 1317 Subscription o = (Subscription) other; 1318 return compareDeep(criteria, o.criteria, true) && compareDeep(contact, o.contact, true) 1319 && compareDeep(reason, o.reason, true) && compareDeep(status, o.status, true) 1320 && compareDeep(error, o.error, true) && compareDeep(channel, o.channel, true) && compareDeep(end, o.end, true) 1321 && compareDeep(tag, o.tag, true); 1322 } 1323 1324 @Override 1325 public boolean equalsShallow(Base other) { 1326 if (!super.equalsShallow(other)) 1327 return false; 1328 if (!(other instanceof Subscription)) 1329 return false; 1330 Subscription o = (Subscription) other; 1331 return compareValues(criteria, o.criteria, true) && compareValues(reason, o.reason, true) 1332 && compareValues(status, o.status, true) && compareValues(error, o.error, true) 1333 && compareValues(end, o.end, true); 1334 } 1335 1336 public boolean isEmpty() { 1337 return super.isEmpty() && (criteria == null || criteria.isEmpty()) && (contact == null || contact.isEmpty()) 1338 && (reason == null || reason.isEmpty()) && (status == null || status.isEmpty()) 1339 && (error == null || error.isEmpty()) && (channel == null || channel.isEmpty()) 1340 && (end == null || end.isEmpty()) && (tag == null || tag.isEmpty()); 1341 } 1342 1343 @Override 1344 public ResourceType getResourceType() { 1345 return ResourceType.Subscription; 1346 } 1347 1348 @SearchParamDefinition(name = "payload", path = "Subscription.channel.payload", description = "Mimetype to send, or blank for no payload", type = "string") 1349 public static final String SP_PAYLOAD = "payload"; 1350 @SearchParamDefinition(name = "criteria", path = "Subscription.criteria", description = "Rule for server push criteria", type = "string") 1351 public static final String SP_CRITERIA = "criteria"; 1352 @SearchParamDefinition(name = "contact", path = "Subscription.contact", description = "Contact details for source (e.g. troubleshooting)", type = "token") 1353 public static final String SP_CONTACT = "contact"; 1354 @SearchParamDefinition(name = "tag", path = "Subscription.tag", description = "A tag to add to matching resources", type = "token") 1355 public static final String SP_TAG = "tag"; 1356 @SearchParamDefinition(name = "type", path = "Subscription.channel.type", description = "rest-hook | websocket | email | sms | message", type = "token") 1357 public static final String SP_TYPE = "type"; 1358 @SearchParamDefinition(name = "url", path = "Subscription.channel.endpoint", description = "Where the channel points to", type = "uri") 1359 public static final String SP_URL = "url"; 1360 @SearchParamDefinition(name = "status", path = "Subscription.status", description = "requested | active | error | off", type = "token") 1361 public static final String SP_STATUS = "status"; 1362 1363}