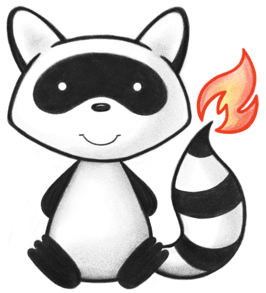
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A homogeneous material with a definite composition. 048 */ 049@ResourceDef(name = "Substance", profile = "http://hl7.org/fhir/Profile/Substance") 050public class Substance extends DomainResource { 051 052 @Block() 053 public static class SubstanceInstanceComponent extends BackboneElement implements IBaseBackboneElement { 054 /** 055 * Identifier associated with the package/container (usually a label affixed 056 * directly). 057 */ 058 @Child(name = "identifier", type = { 059 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "Identifier of the package/container", formalDefinition = "Identifier associated with the package/container (usually a label affixed directly).") 061 protected Identifier identifier; 062 063 /** 064 * When the substance is no longer valid to use. For some substances, a single 065 * arbitrary date is used for expiry. 066 */ 067 @Child(name = "expiry", type = { 068 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 069 @Description(shortDefinition = "When no longer valid to use", formalDefinition = "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.") 070 protected DateTimeType expiry; 071 072 /** 073 * The amount of the substance. 074 */ 075 @Child(name = "quantity", type = { 076 SimpleQuantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 077 @Description(shortDefinition = "Amount of substance in the package", formalDefinition = "The amount of the substance.") 078 protected SimpleQuantity quantity; 079 080 private static final long serialVersionUID = -794314734L; 081 082 /* 083 * Constructor 084 */ 085 public SubstanceInstanceComponent() { 086 super(); 087 } 088 089 /** 090 * @return {@link #identifier} (Identifier associated with the package/container 091 * (usually a label affixed directly).) 092 */ 093 public Identifier getIdentifier() { 094 if (this.identifier == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create SubstanceInstanceComponent.identifier"); 097 else if (Configuration.doAutoCreate()) 098 this.identifier = new Identifier(); // cc 099 return this.identifier; 100 } 101 102 public boolean hasIdentifier() { 103 return this.identifier != null && !this.identifier.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #identifier} (Identifier associated with the 108 * package/container (usually a label affixed directly).) 109 */ 110 public SubstanceInstanceComponent setIdentifier(Identifier value) { 111 this.identifier = value; 112 return this; 113 } 114 115 /** 116 * @return {@link #expiry} (When the substance is no longer valid to use. For 117 * some substances, a single arbitrary date is used for expiry.). This 118 * is the underlying object with id, value and extensions. The accessor 119 * "getExpiry" gives direct access to the value 120 */ 121 public DateTimeType getExpiryElement() { 122 if (this.expiry == null) 123 if (Configuration.errorOnAutoCreate()) 124 throw new Error("Attempt to auto-create SubstanceInstanceComponent.expiry"); 125 else if (Configuration.doAutoCreate()) 126 this.expiry = new DateTimeType(); // bb 127 return this.expiry; 128 } 129 130 public boolean hasExpiryElement() { 131 return this.expiry != null && !this.expiry.isEmpty(); 132 } 133 134 public boolean hasExpiry() { 135 return this.expiry != null && !this.expiry.isEmpty(); 136 } 137 138 /** 139 * @param value {@link #expiry} (When the substance is no longer valid to use. 140 * For some substances, a single arbitrary date is used for 141 * expiry.). This is the underlying object with id, value and 142 * extensions. The accessor "getExpiry" gives direct access to the 143 * value 144 */ 145 public SubstanceInstanceComponent setExpiryElement(DateTimeType value) { 146 this.expiry = value; 147 return this; 148 } 149 150 /** 151 * @return When the substance is no longer valid to use. For some substances, a 152 * single arbitrary date is used for expiry. 153 */ 154 public Date getExpiry() { 155 return this.expiry == null ? null : this.expiry.getValue(); 156 } 157 158 /** 159 * @param value When the substance is no longer valid to use. For some 160 * substances, a single arbitrary date is used for expiry. 161 */ 162 public SubstanceInstanceComponent setExpiry(Date value) { 163 if (value == null) 164 this.expiry = null; 165 else { 166 if (this.expiry == null) 167 this.expiry = new DateTimeType(); 168 this.expiry.setValue(value); 169 } 170 return this; 171 } 172 173 /** 174 * @return {@link #quantity} (The amount of the substance.) 175 */ 176 public SimpleQuantity getQuantity() { 177 if (this.quantity == null) 178 if (Configuration.errorOnAutoCreate()) 179 throw new Error("Attempt to auto-create SubstanceInstanceComponent.quantity"); 180 else if (Configuration.doAutoCreate()) 181 this.quantity = new SimpleQuantity(); // cc 182 return this.quantity; 183 } 184 185 public boolean hasQuantity() { 186 return this.quantity != null && !this.quantity.isEmpty(); 187 } 188 189 /** 190 * @param value {@link #quantity} (The amount of the substance.) 191 */ 192 public SubstanceInstanceComponent setQuantity(SimpleQuantity value) { 193 this.quantity = value; 194 return this; 195 } 196 197 protected void listChildren(List<Property> childrenList) { 198 super.listChildren(childrenList); 199 childrenList.add(new Property("identifier", "Identifier", 200 "Identifier associated with the package/container (usually a label affixed directly).", 0, 201 java.lang.Integer.MAX_VALUE, identifier)); 202 childrenList.add(new Property("expiry", "dateTime", 203 "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 204 0, java.lang.Integer.MAX_VALUE, expiry)); 205 childrenList.add(new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 206 java.lang.Integer.MAX_VALUE, quantity)); 207 } 208 209 @Override 210 public void setProperty(String name, Base value) throws FHIRException { 211 if (name.equals("identifier")) 212 this.identifier = castToIdentifier(value); // Identifier 213 else if (name.equals("expiry")) 214 this.expiry = castToDateTime(value); // DateTimeType 215 else if (name.equals("quantity")) 216 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 217 else 218 super.setProperty(name, value); 219 } 220 221 @Override 222 public Base addChild(String name) throws FHIRException { 223 if (name.equals("identifier")) { 224 this.identifier = new Identifier(); 225 return this.identifier; 226 } else if (name.equals("expiry")) { 227 throw new FHIRException("Cannot call addChild on a singleton property Substance.expiry"); 228 } else if (name.equals("quantity")) { 229 this.quantity = new SimpleQuantity(); 230 return this.quantity; 231 } else 232 return super.addChild(name); 233 } 234 235 public SubstanceInstanceComponent copy() { 236 SubstanceInstanceComponent dst = new SubstanceInstanceComponent(); 237 copyValues(dst); 238 dst.identifier = identifier == null ? null : identifier.copy(); 239 dst.expiry = expiry == null ? null : expiry.copy(); 240 dst.quantity = quantity == null ? null : quantity.copy(); 241 return dst; 242 } 243 244 @Override 245 public boolean equalsDeep(Base other) { 246 if (!super.equalsDeep(other)) 247 return false; 248 if (!(other instanceof SubstanceInstanceComponent)) 249 return false; 250 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other; 251 return compareDeep(identifier, o.identifier, true) && compareDeep(expiry, o.expiry, true) 252 && compareDeep(quantity, o.quantity, true); 253 } 254 255 @Override 256 public boolean equalsShallow(Base other) { 257 if (!super.equalsShallow(other)) 258 return false; 259 if (!(other instanceof SubstanceInstanceComponent)) 260 return false; 261 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other; 262 return compareValues(expiry, o.expiry, true); 263 } 264 265 public boolean isEmpty() { 266 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (expiry == null || expiry.isEmpty()) 267 && (quantity == null || quantity.isEmpty()); 268 } 269 270 public String fhirType() { 271 return "Substance.instance"; 272 273 } 274 275 } 276 277 @Block() 278 public static class SubstanceIngredientComponent extends BackboneElement implements IBaseBackboneElement { 279 /** 280 * The amount of the ingredient in the substance - a concentration ratio. 281 */ 282 @Child(name = "quantity", type = { Ratio.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 283 @Description(shortDefinition = "Optional amount (concentration)", formalDefinition = "The amount of the ingredient in the substance - a concentration ratio.") 284 protected Ratio quantity; 285 286 /** 287 * Another substance that is a component of this substance. 288 */ 289 @Child(name = "substance", type = { 290 Substance.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 291 @Description(shortDefinition = "A component of the substance", formalDefinition = "Another substance that is a component of this substance.") 292 protected Reference substance; 293 294 /** 295 * The actual object that is the target of the reference (Another substance that 296 * is a component of this substance.) 297 */ 298 protected Substance substanceTarget; 299 300 private static final long serialVersionUID = -1783242034L; 301 302 /* 303 * Constructor 304 */ 305 public SubstanceIngredientComponent() { 306 super(); 307 } 308 309 /* 310 * Constructor 311 */ 312 public SubstanceIngredientComponent(Reference substance) { 313 super(); 314 this.substance = substance; 315 } 316 317 /** 318 * @return {@link #quantity} (The amount of the ingredient in the substance - a 319 * concentration ratio.) 320 */ 321 public Ratio getQuantity() { 322 if (this.quantity == null) 323 if (Configuration.errorOnAutoCreate()) 324 throw new Error("Attempt to auto-create SubstanceIngredientComponent.quantity"); 325 else if (Configuration.doAutoCreate()) 326 this.quantity = new Ratio(); // cc 327 return this.quantity; 328 } 329 330 public boolean hasQuantity() { 331 return this.quantity != null && !this.quantity.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #quantity} (The amount of the ingredient in the substance 336 * - a concentration ratio.) 337 */ 338 public SubstanceIngredientComponent setQuantity(Ratio value) { 339 this.quantity = value; 340 return this; 341 } 342 343 /** 344 * @return {@link #substance} (Another substance that is a component of this 345 * substance.) 346 */ 347 public Reference getSubstance() { 348 if (this.substance == null) 349 if (Configuration.errorOnAutoCreate()) 350 throw new Error("Attempt to auto-create SubstanceIngredientComponent.substance"); 351 else if (Configuration.doAutoCreate()) 352 this.substance = new Reference(); // cc 353 return this.substance; 354 } 355 356 public boolean hasSubstance() { 357 return this.substance != null && !this.substance.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #substance} (Another substance that is a component of 362 * this substance.) 363 */ 364 public SubstanceIngredientComponent setSubstance(Reference value) { 365 this.substance = value; 366 return this; 367 } 368 369 /** 370 * @return {@link #substance} The actual object that is the target of the 371 * reference. The reference library doesn't populate this, but you can 372 * use it to hold the resource if you resolve it. (Another substance 373 * that is a component of this substance.) 374 */ 375 public Substance getSubstanceTarget() { 376 if (this.substanceTarget == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create SubstanceIngredientComponent.substance"); 379 else if (Configuration.doAutoCreate()) 380 this.substanceTarget = new Substance(); // aa 381 return this.substanceTarget; 382 } 383 384 /** 385 * @param value {@link #substance} The actual object that is the target of the 386 * reference. The reference library doesn't use these, but you can 387 * use it to hold the resource if you resolve it. (Another 388 * substance that is a component of this substance.) 389 */ 390 public SubstanceIngredientComponent setSubstanceTarget(Substance value) { 391 this.substanceTarget = value; 392 return this; 393 } 394 395 protected void listChildren(List<Property> childrenList) { 396 super.listChildren(childrenList); 397 childrenList.add( 398 new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 399 java.lang.Integer.MAX_VALUE, quantity)); 400 childrenList.add(new Property("substance", "Reference(Substance)", 401 "Another substance that is a component of this substance.", 0, java.lang.Integer.MAX_VALUE, substance)); 402 } 403 404 @Override 405 public void setProperty(String name, Base value) throws FHIRException { 406 if (name.equals("quantity")) 407 this.quantity = castToRatio(value); // Ratio 408 else if (name.equals("substance")) 409 this.substance = castToReference(value); // Reference 410 else 411 super.setProperty(name, value); 412 } 413 414 @Override 415 public Base addChild(String name) throws FHIRException { 416 if (name.equals("quantity")) { 417 this.quantity = new Ratio(); 418 return this.quantity; 419 } else if (name.equals("substance")) { 420 this.substance = new Reference(); 421 return this.substance; 422 } else 423 return super.addChild(name); 424 } 425 426 public SubstanceIngredientComponent copy() { 427 SubstanceIngredientComponent dst = new SubstanceIngredientComponent(); 428 copyValues(dst); 429 dst.quantity = quantity == null ? null : quantity.copy(); 430 dst.substance = substance == null ? null : substance.copy(); 431 return dst; 432 } 433 434 @Override 435 public boolean equalsDeep(Base other) { 436 if (!super.equalsDeep(other)) 437 return false; 438 if (!(other instanceof SubstanceIngredientComponent)) 439 return false; 440 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other; 441 return compareDeep(quantity, o.quantity, true) && compareDeep(substance, o.substance, true); 442 } 443 444 @Override 445 public boolean equalsShallow(Base other) { 446 if (!super.equalsShallow(other)) 447 return false; 448 if (!(other instanceof SubstanceIngredientComponent)) 449 return false; 450 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other; 451 return true; 452 } 453 454 public boolean isEmpty() { 455 return super.isEmpty() && (quantity == null || quantity.isEmpty()) && (substance == null || substance.isEmpty()); 456 } 457 458 public String fhirType() { 459 return "Substance.ingredient"; 460 461 } 462 463 } 464 465 /** 466 * Unique identifier for the substance. 467 */ 468 @Child(name = "identifier", type = { 469 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 470 @Description(shortDefinition = "Unique identifier", formalDefinition = "Unique identifier for the substance.") 471 protected List<Identifier> identifier; 472 473 /** 474 * A code that classifies the general type of substance. This is used for 475 * searching, sorting and display purposes. 476 */ 477 @Child(name = "category", type = { 478 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 479 @Description(shortDefinition = "What class/type of substance this is", formalDefinition = "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.") 480 protected List<CodeableConcept> category; 481 482 /** 483 * A code (or set of codes) that identify this substance. 484 */ 485 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 486 @Description(shortDefinition = "What substance this is", formalDefinition = "A code (or set of codes) that identify this substance.") 487 protected CodeableConcept code; 488 489 /** 490 * A description of the substance - its appearance, handling requirements, and 491 * other usage notes. 492 */ 493 @Child(name = "description", type = { 494 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 495 @Description(shortDefinition = "Textual description of the substance, comments", formalDefinition = "A description of the substance - its appearance, handling requirements, and other usage notes.") 496 protected StringType description; 497 498 /** 499 * Substance may be used to describe a kind of substance, or a specific 500 * package/container of the substance: an instance. 501 */ 502 @Child(name = "instance", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 503 @Description(shortDefinition = "If this describes a specific package/container of the substance", formalDefinition = "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.") 504 protected List<SubstanceInstanceComponent> instance; 505 506 /** 507 * A substance can be composed of other substances. 508 */ 509 @Child(name = "ingredient", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 510 @Description(shortDefinition = "Composition information about the substance", formalDefinition = "A substance can be composed of other substances.") 511 protected List<SubstanceIngredientComponent> ingredient; 512 513 private static final long serialVersionUID = -1653977206L; 514 515 /* 516 * Constructor 517 */ 518 public Substance() { 519 super(); 520 } 521 522 /* 523 * Constructor 524 */ 525 public Substance(CodeableConcept code) { 526 super(); 527 this.code = code; 528 } 529 530 /** 531 * @return {@link #identifier} (Unique identifier for the substance.) 532 */ 533 public List<Identifier> getIdentifier() { 534 if (this.identifier == null) 535 this.identifier = new ArrayList<Identifier>(); 536 return this.identifier; 537 } 538 539 public boolean hasIdentifier() { 540 if (this.identifier == null) 541 return false; 542 for (Identifier item : this.identifier) 543 if (!item.isEmpty()) 544 return true; 545 return false; 546 } 547 548 /** 549 * @return {@link #identifier} (Unique identifier for the substance.) 550 */ 551 // syntactic sugar 552 public Identifier addIdentifier() { // 3 553 Identifier t = new Identifier(); 554 if (this.identifier == null) 555 this.identifier = new ArrayList<Identifier>(); 556 this.identifier.add(t); 557 return t; 558 } 559 560 // syntactic sugar 561 public Substance addIdentifier(Identifier t) { // 3 562 if (t == null) 563 return this; 564 if (this.identifier == null) 565 this.identifier = new ArrayList<Identifier>(); 566 this.identifier.add(t); 567 return this; 568 } 569 570 /** 571 * @return {@link #category} (A code that classifies the general type of 572 * substance. This is used for searching, sorting and display purposes.) 573 */ 574 public List<CodeableConcept> getCategory() { 575 if (this.category == null) 576 this.category = new ArrayList<CodeableConcept>(); 577 return this.category; 578 } 579 580 public boolean hasCategory() { 581 if (this.category == null) 582 return false; 583 for (CodeableConcept item : this.category) 584 if (!item.isEmpty()) 585 return true; 586 return false; 587 } 588 589 /** 590 * @return {@link #category} (A code that classifies the general type of 591 * substance. This is used for searching, sorting and display purposes.) 592 */ 593 // syntactic sugar 594 public CodeableConcept addCategory() { // 3 595 CodeableConcept t = new CodeableConcept(); 596 if (this.category == null) 597 this.category = new ArrayList<CodeableConcept>(); 598 this.category.add(t); 599 return t; 600 } 601 602 // syntactic sugar 603 public Substance addCategory(CodeableConcept t) { // 3 604 if (t == null) 605 return this; 606 if (this.category == null) 607 this.category = new ArrayList<CodeableConcept>(); 608 this.category.add(t); 609 return this; 610 } 611 612 /** 613 * @return {@link #code} (A code (or set of codes) that identify this 614 * substance.) 615 */ 616 public CodeableConcept getCode() { 617 if (this.code == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create Substance.code"); 620 else if (Configuration.doAutoCreate()) 621 this.code = new CodeableConcept(); // cc 622 return this.code; 623 } 624 625 public boolean hasCode() { 626 return this.code != null && !this.code.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #code} (A code (or set of codes) that identify this 631 * substance.) 632 */ 633 public Substance setCode(CodeableConcept value) { 634 this.code = value; 635 return this; 636 } 637 638 /** 639 * @return {@link #description} (A description of the substance - its 640 * appearance, handling requirements, and other usage notes.). This is 641 * the underlying object with id, value and extensions. The accessor 642 * "getDescription" gives direct access to the value 643 */ 644 public StringType getDescriptionElement() { 645 if (this.description == null) 646 if (Configuration.errorOnAutoCreate()) 647 throw new Error("Attempt to auto-create Substance.description"); 648 else if (Configuration.doAutoCreate()) 649 this.description = new StringType(); // bb 650 return this.description; 651 } 652 653 public boolean hasDescriptionElement() { 654 return this.description != null && !this.description.isEmpty(); 655 } 656 657 public boolean hasDescription() { 658 return this.description != null && !this.description.isEmpty(); 659 } 660 661 /** 662 * @param value {@link #description} (A description of the substance - its 663 * appearance, handling requirements, and other usage notes.). This 664 * is the underlying object with id, value and extensions. The 665 * accessor "getDescription" gives direct access to the value 666 */ 667 public Substance setDescriptionElement(StringType value) { 668 this.description = value; 669 return this; 670 } 671 672 /** 673 * @return A description of the substance - its appearance, handling 674 * requirements, and other usage notes. 675 */ 676 public String getDescription() { 677 return this.description == null ? null : this.description.getValue(); 678 } 679 680 /** 681 * @param value A description of the substance - its appearance, handling 682 * requirements, and other usage notes. 683 */ 684 public Substance setDescription(String value) { 685 if (Utilities.noString(value)) 686 this.description = null; 687 else { 688 if (this.description == null) 689 this.description = new StringType(); 690 this.description.setValue(value); 691 } 692 return this; 693 } 694 695 /** 696 * @return {@link #instance} (Substance may be used to describe a kind of 697 * substance, or a specific package/container of the substance: an 698 * instance.) 699 */ 700 public List<SubstanceInstanceComponent> getInstance() { 701 if (this.instance == null) 702 this.instance = new ArrayList<SubstanceInstanceComponent>(); 703 return this.instance; 704 } 705 706 public boolean hasInstance() { 707 if (this.instance == null) 708 return false; 709 for (SubstanceInstanceComponent item : this.instance) 710 if (!item.isEmpty()) 711 return true; 712 return false; 713 } 714 715 /** 716 * @return {@link #instance} (Substance may be used to describe a kind of 717 * substance, or a specific package/container of the substance: an 718 * instance.) 719 */ 720 // syntactic sugar 721 public SubstanceInstanceComponent addInstance() { // 3 722 SubstanceInstanceComponent t = new SubstanceInstanceComponent(); 723 if (this.instance == null) 724 this.instance = new ArrayList<SubstanceInstanceComponent>(); 725 this.instance.add(t); 726 return t; 727 } 728 729 // syntactic sugar 730 public Substance addInstance(SubstanceInstanceComponent t) { // 3 731 if (t == null) 732 return this; 733 if (this.instance == null) 734 this.instance = new ArrayList<SubstanceInstanceComponent>(); 735 this.instance.add(t); 736 return this; 737 } 738 739 /** 740 * @return {@link #ingredient} (A substance can be composed of other 741 * substances.) 742 */ 743 public List<SubstanceIngredientComponent> getIngredient() { 744 if (this.ingredient == null) 745 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 746 return this.ingredient; 747 } 748 749 public boolean hasIngredient() { 750 if (this.ingredient == null) 751 return false; 752 for (SubstanceIngredientComponent item : this.ingredient) 753 if (!item.isEmpty()) 754 return true; 755 return false; 756 } 757 758 /** 759 * @return {@link #ingredient} (A substance can be composed of other 760 * substances.) 761 */ 762 // syntactic sugar 763 public SubstanceIngredientComponent addIngredient() { // 3 764 SubstanceIngredientComponent t = new SubstanceIngredientComponent(); 765 if (this.ingredient == null) 766 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 767 this.ingredient.add(t); 768 return t; 769 } 770 771 // syntactic sugar 772 public Substance addIngredient(SubstanceIngredientComponent t) { // 3 773 if (t == null) 774 return this; 775 if (this.ingredient == null) 776 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 777 this.ingredient.add(t); 778 return this; 779 } 780 781 protected void listChildren(List<Property> childrenList) { 782 super.listChildren(childrenList); 783 childrenList.add(new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, 784 java.lang.Integer.MAX_VALUE, identifier)); 785 childrenList.add(new Property("category", "CodeableConcept", 786 "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 787 0, java.lang.Integer.MAX_VALUE, category)); 788 childrenList.add(new Property("code", "CodeableConcept", "A code (or set of codes) that identify this substance.", 789 0, java.lang.Integer.MAX_VALUE, code)); 790 childrenList.add(new Property("description", "string", 791 "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 792 java.lang.Integer.MAX_VALUE, description)); 793 childrenList.add(new Property("instance", "", 794 "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 795 0, java.lang.Integer.MAX_VALUE, instance)); 796 childrenList.add(new Property("ingredient", "", "A substance can be composed of other substances.", 0, 797 java.lang.Integer.MAX_VALUE, ingredient)); 798 } 799 800 @Override 801 public void setProperty(String name, Base value) throws FHIRException { 802 if (name.equals("identifier")) 803 this.getIdentifier().add(castToIdentifier(value)); 804 else if (name.equals("category")) 805 this.getCategory().add(castToCodeableConcept(value)); 806 else if (name.equals("code")) 807 this.code = castToCodeableConcept(value); // CodeableConcept 808 else if (name.equals("description")) 809 this.description = castToString(value); // StringType 810 else if (name.equals("instance")) 811 this.getInstance().add((SubstanceInstanceComponent) value); 812 else if (name.equals("ingredient")) 813 this.getIngredient().add((SubstanceIngredientComponent) value); 814 else 815 super.setProperty(name, value); 816 } 817 818 @Override 819 public Base addChild(String name) throws FHIRException { 820 if (name.equals("identifier")) { 821 return addIdentifier(); 822 } else if (name.equals("category")) { 823 return addCategory(); 824 } else if (name.equals("code")) { 825 this.code = new CodeableConcept(); 826 return this.code; 827 } else if (name.equals("description")) { 828 throw new FHIRException("Cannot call addChild on a singleton property Substance.description"); 829 } else if (name.equals("instance")) { 830 return addInstance(); 831 } else if (name.equals("ingredient")) { 832 return addIngredient(); 833 } else 834 return super.addChild(name); 835 } 836 837 public String fhirType() { 838 return "Substance"; 839 840 } 841 842 public Substance copy() { 843 Substance dst = new Substance(); 844 copyValues(dst); 845 if (identifier != null) { 846 dst.identifier = new ArrayList<Identifier>(); 847 for (Identifier i : identifier) 848 dst.identifier.add(i.copy()); 849 } 850 ; 851 if (category != null) { 852 dst.category = new ArrayList<CodeableConcept>(); 853 for (CodeableConcept i : category) 854 dst.category.add(i.copy()); 855 } 856 ; 857 dst.code = code == null ? null : code.copy(); 858 dst.description = description == null ? null : description.copy(); 859 if (instance != null) { 860 dst.instance = new ArrayList<SubstanceInstanceComponent>(); 861 for (SubstanceInstanceComponent i : instance) 862 dst.instance.add(i.copy()); 863 } 864 ; 865 if (ingredient != null) { 866 dst.ingredient = new ArrayList<SubstanceIngredientComponent>(); 867 for (SubstanceIngredientComponent i : ingredient) 868 dst.ingredient.add(i.copy()); 869 } 870 ; 871 return dst; 872 } 873 874 protected Substance typedCopy() { 875 return copy(); 876 } 877 878 @Override 879 public boolean equalsDeep(Base other) { 880 if (!super.equalsDeep(other)) 881 return false; 882 if (!(other instanceof Substance)) 883 return false; 884 Substance o = (Substance) other; 885 return compareDeep(identifier, o.identifier, true) && compareDeep(category, o.category, true) 886 && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 887 && compareDeep(instance, o.instance, true) && compareDeep(ingredient, o.ingredient, true); 888 } 889 890 @Override 891 public boolean equalsShallow(Base other) { 892 if (!super.equalsShallow(other)) 893 return false; 894 if (!(other instanceof Substance)) 895 return false; 896 Substance o = (Substance) other; 897 return compareValues(description, o.description, true); 898 } 899 900 public boolean isEmpty() { 901 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (category == null || category.isEmpty()) 902 && (code == null || code.isEmpty()) && (description == null || description.isEmpty()) 903 && (instance == null || instance.isEmpty()) && (ingredient == null || ingredient.isEmpty()); 904 } 905 906 @Override 907 public ResourceType getResourceType() { 908 return ResourceType.Substance; 909 } 910 911 @SearchParamDefinition(name = "identifier", path = "Substance.identifier", description = "Unique identifier for the substance", type = "token") 912 public static final String SP_IDENTIFIER = "identifier"; 913 @SearchParamDefinition(name = "container-identifier", path = "Substance.instance.identifier", description = "Identifier of the package/container", type = "token") 914 public static final String SP_CONTAINERIDENTIFIER = "container-identifier"; 915 @SearchParamDefinition(name = "code", path = "Substance.code", description = "The code of the substance", type = "token") 916 public static final String SP_CODE = "code"; 917 @SearchParamDefinition(name = "quantity", path = "Substance.instance.quantity", description = "Amount of substance in the package", type = "quantity") 918 public static final String SP_QUANTITY = "quantity"; 919 @SearchParamDefinition(name = "substance", path = "Substance.ingredient.substance", description = "A component of the substance", type = "reference") 920 public static final String SP_SUBSTANCE = "substance"; 921 @SearchParamDefinition(name = "expiry", path = "Substance.instance.expiry", description = "Expiry date of package or container of substance", type = "date") 922 public static final String SP_EXPIRY = "expiry"; 923 @SearchParamDefinition(name = "category", path = "Substance.category", description = "The category of the substance", type = "token") 924 public static final String SP_CATEGORY = "category"; 925 926}