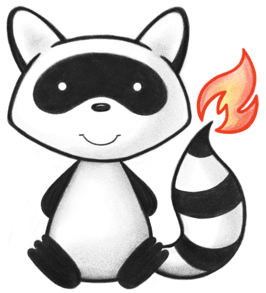
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * Record of delivery of what is supplied. 045 */ 046@ResourceDef(name = "SupplyDelivery", profile = "http://hl7.org/fhir/Profile/SupplyDelivery") 047public class SupplyDelivery extends DomainResource { 048 049 public enum SupplyDeliveryStatus { 050 /** 051 * Supply has been requested, but not delivered. 052 */ 053 INPROGRESS, 054 /** 055 * Supply has been delivered ("completed"). 056 */ 057 COMPLETED, 058 /** 059 * Dispensing was not completed. 060 */ 061 ABANDONED, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 067 public static SupplyDeliveryStatus fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("in-progress".equals(codeString)) 071 return INPROGRESS; 072 if ("completed".equals(codeString)) 073 return COMPLETED; 074 if ("abandoned".equals(codeString)) 075 return ABANDONED; 076 throw new FHIRException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 077 } 078 079 public String toCode() { 080 switch (this) { 081 case INPROGRESS: 082 return "in-progress"; 083 case COMPLETED: 084 return "completed"; 085 case ABANDONED: 086 return "abandoned"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getSystem() { 095 switch (this) { 096 case INPROGRESS: 097 return "http://hl7.org/fhir/supplydelivery-status"; 098 case COMPLETED: 099 return "http://hl7.org/fhir/supplydelivery-status"; 100 case ABANDONED: 101 return "http://hl7.org/fhir/supplydelivery-status"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getDefinition() { 110 switch (this) { 111 case INPROGRESS: 112 return "Supply has been requested, but not delivered."; 113 case COMPLETED: 114 return "Supply has been delivered (\"completed\")."; 115 case ABANDONED: 116 return "Dispensing was not completed."; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDisplay() { 125 switch (this) { 126 case INPROGRESS: 127 return "In Progress"; 128 case COMPLETED: 129 return "Delivered"; 130 case ABANDONED: 131 return "Abandoned"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 } 139 140 public static class SupplyDeliveryStatusEnumFactory implements EnumFactory<SupplyDeliveryStatus> { 141 public SupplyDeliveryStatus fromCode(String codeString) throws IllegalArgumentException { 142 if (codeString == null || "".equals(codeString)) 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("in-progress".equals(codeString)) 146 return SupplyDeliveryStatus.INPROGRESS; 147 if ("completed".equals(codeString)) 148 return SupplyDeliveryStatus.COMPLETED; 149 if ("abandoned".equals(codeString)) 150 return SupplyDeliveryStatus.ABANDONED; 151 throw new IllegalArgumentException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 152 } 153 154 public Enumeration<SupplyDeliveryStatus> fromType(Base code) throws FHIRException { 155 if (code == null || code.isEmpty()) 156 return null; 157 String codeString = ((PrimitiveType) code).asStringValue(); 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("in-progress".equals(codeString)) 161 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.INPROGRESS); 162 if ("completed".equals(codeString)) 163 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.COMPLETED); 164 if ("abandoned".equals(codeString)) 165 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ABANDONED); 166 throw new FHIRException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 167 } 168 169 public String toCode(SupplyDeliveryStatus code) 170 { 171 if (code == SupplyDeliveryStatus.NULL) 172 return null; 173 if (code == SupplyDeliveryStatus.INPROGRESS) 174 return "in-progress"; 175 if (code == SupplyDeliveryStatus.COMPLETED) 176 return "completed"; 177 if (code == SupplyDeliveryStatus.ABANDONED) 178 return "abandoned"; 179 return "?"; 180 } 181 } 182 183 /** 184 * Identifier assigned by the dispensing facility when the item(s) is dispensed. 185 */ 186 @Child(name = "identifier", type = { 187 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 188 @Description(shortDefinition = "External identifier", formalDefinition = "Identifier assigned by the dispensing facility when the item(s) is dispensed.") 189 protected Identifier identifier; 190 191 /** 192 * A code specifying the state of the dispense event. 193 */ 194 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 195 @Description(shortDefinition = "in-progress | completed | abandoned", formalDefinition = "A code specifying the state of the dispense event.") 196 protected Enumeration<SupplyDeliveryStatus> status; 197 198 /** 199 * A link to a resource representing the person whom the delivered item is for. 200 */ 201 @Child(name = "patient", type = { Patient.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 202 @Description(shortDefinition = "Patient for whom the item is supplied", formalDefinition = "A link to a resource representing the person whom the delivered item is for.") 203 protected Reference patient; 204 205 /** 206 * The actual object that is the target of the reference (A link to a resource 207 * representing the person whom the delivered item is for.) 208 */ 209 protected Patient patientTarget; 210 211 /** 212 * Indicates the type of dispensing event that is performed. Examples include: 213 * Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 214 */ 215 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 216 @Description(shortDefinition = "Category of dispense event", formalDefinition = "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.") 217 protected CodeableConcept type; 218 219 /** 220 * The amount of supply that has been dispensed. Includes unit of measure. 221 */ 222 @Child(name = "quantity", type = { 223 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "Amount dispensed", formalDefinition = "The amount of supply that has been dispensed. Includes unit of measure.") 225 protected SimpleQuantity quantity; 226 227 /** 228 * Identifies the medication, substance or device being dispensed. This is 229 * either a link to a resource representing the details of the item or a simple 230 * attribute carrying a code that identifies the item from a known list. 231 */ 232 @Child(name = "suppliedItem", type = { Medication.class, Substance.class, 233 Device.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 234 @Description(shortDefinition = "Medication, Substance, or Device supplied", formalDefinition = "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a simple attribute carrying a code that identifies the item from a known list.") 235 protected Reference suppliedItem; 236 237 /** 238 * The actual object that is the target of the reference (Identifies the 239 * medication, substance or device being dispensed. This is either a link to a 240 * resource representing the details of the item or a simple attribute carrying 241 * a code that identifies the item from a known list.) 242 */ 243 protected Resource suppliedItemTarget; 244 245 /** 246 * The individual responsible for dispensing the medication, supplier or device. 247 */ 248 @Child(name = "supplier", type = { 249 Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 250 @Description(shortDefinition = "Dispenser", formalDefinition = "The individual responsible for dispensing the medication, supplier or device.") 251 protected Reference supplier; 252 253 /** 254 * The actual object that is the target of the reference (The individual 255 * responsible for dispensing the medication, supplier or device.) 256 */ 257 protected Practitioner supplierTarget; 258 259 /** 260 * The time the dispense event occurred. 261 */ 262 @Child(name = "whenPrepared", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 263 @Description(shortDefinition = "Dispensing time", formalDefinition = "The time the dispense event occurred.") 264 protected Period whenPrepared; 265 266 /** 267 * The time the dispensed item was sent or handed to the patient (or agent). 268 */ 269 @Child(name = "time", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 270 @Description(shortDefinition = "Handover time", formalDefinition = "The time the dispensed item was sent or handed to the patient (or agent).") 271 protected DateTimeType time; 272 273 /** 274 * Identification of the facility/location where the Supply was shipped to, as 275 * part of the dispense event. 276 */ 277 @Child(name = "destination", type = { Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 278 @Description(shortDefinition = "Where the Supply was sent", formalDefinition = "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.") 279 protected Reference destination; 280 281 /** 282 * The actual object that is the target of the reference (Identification of the 283 * facility/location where the Supply was shipped to, as part of the dispense 284 * event.) 285 */ 286 protected Location destinationTarget; 287 288 /** 289 * Identifies the person who picked up the Supply. 290 */ 291 @Child(name = "receiver", type = { 292 Practitioner.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 293 @Description(shortDefinition = "Who collected the Supply", formalDefinition = "Identifies the person who picked up the Supply.") 294 protected List<Reference> receiver; 295 /** 296 * The actual objects that are the target of the reference (Identifies the 297 * person who picked up the Supply.) 298 */ 299 protected List<Practitioner> receiverTarget; 300 301 private static final long serialVersionUID = -1520129707L; 302 303 /* 304 * Constructor 305 */ 306 public SupplyDelivery() { 307 super(); 308 } 309 310 /** 311 * @return {@link #identifier} (Identifier assigned by the dispensing facility 312 * when the item(s) is dispensed.) 313 */ 314 public Identifier getIdentifier() { 315 if (this.identifier == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create SupplyDelivery.identifier"); 318 else if (Configuration.doAutoCreate()) 319 this.identifier = new Identifier(); // cc 320 return this.identifier; 321 } 322 323 public boolean hasIdentifier() { 324 return this.identifier != null && !this.identifier.isEmpty(); 325 } 326 327 /** 328 * @param value {@link #identifier} (Identifier assigned by the dispensing 329 * facility when the item(s) is dispensed.) 330 */ 331 public SupplyDelivery setIdentifier(Identifier value) { 332 this.identifier = value; 333 return this; 334 } 335 336 /** 337 * @return {@link #status} (A code specifying the state of the dispense event.). 338 * This is the underlying object with id, value and extensions. The 339 * accessor "getStatus" gives direct access to the value 340 */ 341 public Enumeration<SupplyDeliveryStatus> getStatusElement() { 342 if (this.status == null) 343 if (Configuration.errorOnAutoCreate()) 344 throw new Error("Attempt to auto-create SupplyDelivery.status"); 345 else if (Configuration.doAutoCreate()) 346 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); // bb 347 return this.status; 348 } 349 350 public boolean hasStatusElement() { 351 return this.status != null && !this.status.isEmpty(); 352 } 353 354 public boolean hasStatus() { 355 return this.status != null && !this.status.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #status} (A code specifying the state of the dispense 360 * event.). This is the underlying object with id, value and 361 * extensions. The accessor "getStatus" gives direct access to the 362 * value 363 */ 364 public SupplyDelivery setStatusElement(Enumeration<SupplyDeliveryStatus> value) { 365 this.status = value; 366 return this; 367 } 368 369 /** 370 * @return A code specifying the state of the dispense event. 371 */ 372 public SupplyDeliveryStatus getStatus() { 373 return this.status == null ? null : this.status.getValue(); 374 } 375 376 /** 377 * @param value A code specifying the state of the dispense event. 378 */ 379 public SupplyDelivery setStatus(SupplyDeliveryStatus value) { 380 if (value == null) 381 this.status = null; 382 else { 383 if (this.status == null) 384 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); 385 this.status.setValue(value); 386 } 387 return this; 388 } 389 390 /** 391 * @return {@link #patient} (A link to a resource representing the person whom 392 * the delivered item is for.) 393 */ 394 public Reference getPatient() { 395 if (this.patient == null) 396 if (Configuration.errorOnAutoCreate()) 397 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 398 else if (Configuration.doAutoCreate()) 399 this.patient = new Reference(); // cc 400 return this.patient; 401 } 402 403 public boolean hasPatient() { 404 return this.patient != null && !this.patient.isEmpty(); 405 } 406 407 /** 408 * @param value {@link #patient} (A link to a resource representing the person 409 * whom the delivered item is for.) 410 */ 411 public SupplyDelivery setPatient(Reference value) { 412 this.patient = value; 413 return this; 414 } 415 416 /** 417 * @return {@link #patient} The actual object that is the target of the 418 * reference. The reference library doesn't populate this, but you can 419 * use it to hold the resource if you resolve it. (A link to a resource 420 * representing the person whom the delivered item is for.) 421 */ 422 public Patient getPatientTarget() { 423 if (this.patientTarget == null) 424 if (Configuration.errorOnAutoCreate()) 425 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 426 else if (Configuration.doAutoCreate()) 427 this.patientTarget = new Patient(); // aa 428 return this.patientTarget; 429 } 430 431 /** 432 * @param value {@link #patient} The actual object that is the target of the 433 * reference. The reference library doesn't use these, but you can 434 * use it to hold the resource if you resolve it. (A link to a 435 * resource representing the person whom the delivered item is 436 * for.) 437 */ 438 public SupplyDelivery setPatientTarget(Patient value) { 439 this.patientTarget = value; 440 return this; 441 } 442 443 /** 444 * @return {@link #type} (Indicates the type of dispensing event that is 445 * performed. Examples include: Trial Fill, Completion of Trial, Partial 446 * Fill, Emergency Fill, Samples, etc.) 447 */ 448 public CodeableConcept getType() { 449 if (this.type == null) 450 if (Configuration.errorOnAutoCreate()) 451 throw new Error("Attempt to auto-create SupplyDelivery.type"); 452 else if (Configuration.doAutoCreate()) 453 this.type = new CodeableConcept(); // cc 454 return this.type; 455 } 456 457 public boolean hasType() { 458 return this.type != null && !this.type.isEmpty(); 459 } 460 461 /** 462 * @param value {@link #type} (Indicates the type of dispensing event that is 463 * performed. Examples include: Trial Fill, Completion of Trial, 464 * Partial Fill, Emergency Fill, Samples, etc.) 465 */ 466 public SupplyDelivery setType(CodeableConcept value) { 467 this.type = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #quantity} (The amount of supply that has been dispensed. 473 * Includes unit of measure.) 474 */ 475 public SimpleQuantity getQuantity() { 476 if (this.quantity == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create SupplyDelivery.quantity"); 479 else if (Configuration.doAutoCreate()) 480 this.quantity = new SimpleQuantity(); // cc 481 return this.quantity; 482 } 483 484 public boolean hasQuantity() { 485 return this.quantity != null && !this.quantity.isEmpty(); 486 } 487 488 /** 489 * @param value {@link #quantity} (The amount of supply that has been dispensed. 490 * Includes unit of measure.) 491 */ 492 public SupplyDelivery setQuantity(SimpleQuantity value) { 493 this.quantity = value; 494 return this; 495 } 496 497 /** 498 * @return {@link #suppliedItem} (Identifies the medication, substance or device 499 * being dispensed. This is either a link to a resource representing the 500 * details of the item or a simple attribute carrying a code that 501 * identifies the item from a known list.) 502 */ 503 public Reference getSuppliedItem() { 504 if (this.suppliedItem == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create SupplyDelivery.suppliedItem"); 507 else if (Configuration.doAutoCreate()) 508 this.suppliedItem = new Reference(); // cc 509 return this.suppliedItem; 510 } 511 512 public boolean hasSuppliedItem() { 513 return this.suppliedItem != null && !this.suppliedItem.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #suppliedItem} (Identifies the medication, substance or 518 * device being dispensed. This is either a link to a resource 519 * representing the details of the item or a simple attribute 520 * carrying a code that identifies the item from a known list.) 521 */ 522 public SupplyDelivery setSuppliedItem(Reference value) { 523 this.suppliedItem = value; 524 return this; 525 } 526 527 /** 528 * @return {@link #suppliedItem} The actual object that is the target of the 529 * reference. The reference library doesn't populate this, but you can 530 * use it to hold the resource if you resolve it. (Identifies the 531 * medication, substance or device being dispensed. This is either a 532 * link to a resource representing the details of the item or a simple 533 * attribute carrying a code that identifies the item from a known 534 * list.) 535 */ 536 public Resource getSuppliedItemTarget() { 537 return this.suppliedItemTarget; 538 } 539 540 /** 541 * @param value {@link #suppliedItem} The actual object that is the target of 542 * the reference. The reference library doesn't use these, but you 543 * can use it to hold the resource if you resolve it. (Identifies 544 * the medication, substance or device being dispensed. This is 545 * either a link to a resource representing the details of the item 546 * or a simple attribute carrying a code that identifies the item 547 * from a known list.) 548 */ 549 public SupplyDelivery setSuppliedItemTarget(Resource value) { 550 this.suppliedItemTarget = value; 551 return this; 552 } 553 554 /** 555 * @return {@link #supplier} (The individual responsible for dispensing the 556 * medication, supplier or device.) 557 */ 558 public Reference getSupplier() { 559 if (this.supplier == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 562 else if (Configuration.doAutoCreate()) 563 this.supplier = new Reference(); // cc 564 return this.supplier; 565 } 566 567 public boolean hasSupplier() { 568 return this.supplier != null && !this.supplier.isEmpty(); 569 } 570 571 /** 572 * @param value {@link #supplier} (The individual responsible for dispensing the 573 * medication, supplier or device.) 574 */ 575 public SupplyDelivery setSupplier(Reference value) { 576 this.supplier = value; 577 return this; 578 } 579 580 /** 581 * @return {@link #supplier} The actual object that is the target of the 582 * reference. The reference library doesn't populate this, but you can 583 * use it to hold the resource if you resolve it. (The individual 584 * responsible for dispensing the medication, supplier or device.) 585 */ 586 public Practitioner getSupplierTarget() { 587 if (this.supplierTarget == null) 588 if (Configuration.errorOnAutoCreate()) 589 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 590 else if (Configuration.doAutoCreate()) 591 this.supplierTarget = new Practitioner(); // aa 592 return this.supplierTarget; 593 } 594 595 /** 596 * @param value {@link #supplier} The actual object that is the target of the 597 * reference. The reference library doesn't use these, but you can 598 * use it to hold the resource if you resolve it. (The individual 599 * responsible for dispensing the medication, supplier or device.) 600 */ 601 public SupplyDelivery setSupplierTarget(Practitioner value) { 602 this.supplierTarget = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #whenPrepared} (The time the dispense event occurred.) 608 */ 609 public Period getWhenPrepared() { 610 if (this.whenPrepared == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create SupplyDelivery.whenPrepared"); 613 else if (Configuration.doAutoCreate()) 614 this.whenPrepared = new Period(); // cc 615 return this.whenPrepared; 616 } 617 618 public boolean hasWhenPrepared() { 619 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #whenPrepared} (The time the dispense event occurred.) 624 */ 625 public SupplyDelivery setWhenPrepared(Period value) { 626 this.whenPrepared = value; 627 return this; 628 } 629 630 /** 631 * @return {@link #time} (The time the dispensed item was sent or handed to the 632 * patient (or agent).). This is the underlying object with id, value 633 * and extensions. The accessor "getTime" gives direct access to the 634 * value 635 */ 636 public DateTimeType getTimeElement() { 637 if (this.time == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create SupplyDelivery.time"); 640 else if (Configuration.doAutoCreate()) 641 this.time = new DateTimeType(); // bb 642 return this.time; 643 } 644 645 public boolean hasTimeElement() { 646 return this.time != null && !this.time.isEmpty(); 647 } 648 649 public boolean hasTime() { 650 return this.time != null && !this.time.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #time} (The time the dispensed item was sent or handed to 655 * the patient (or agent).). This is the underlying object with id, 656 * value and extensions. The accessor "getTime" gives direct access 657 * to the value 658 */ 659 public SupplyDelivery setTimeElement(DateTimeType value) { 660 this.time = value; 661 return this; 662 } 663 664 /** 665 * @return The time the dispensed item was sent or handed to the patient (or 666 * agent). 667 */ 668 public Date getTime() { 669 return this.time == null ? null : this.time.getValue(); 670 } 671 672 /** 673 * @param value The time the dispensed item was sent or handed to the patient 674 * (or agent). 675 */ 676 public SupplyDelivery setTime(Date value) { 677 if (value == null) 678 this.time = null; 679 else { 680 if (this.time == null) 681 this.time = new DateTimeType(); 682 this.time.setValue(value); 683 } 684 return this; 685 } 686 687 /** 688 * @return {@link #destination} (Identification of the facility/location where 689 * the Supply was shipped to, as part of the dispense event.) 690 */ 691 public Reference getDestination() { 692 if (this.destination == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 695 else if (Configuration.doAutoCreate()) 696 this.destination = new Reference(); // cc 697 return this.destination; 698 } 699 700 public boolean hasDestination() { 701 return this.destination != null && !this.destination.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #destination} (Identification of the facility/location 706 * where the Supply was shipped to, as part of the dispense event.) 707 */ 708 public SupplyDelivery setDestination(Reference value) { 709 this.destination = value; 710 return this; 711 } 712 713 /** 714 * @return {@link #destination} The actual object that is the target of the 715 * reference. The reference library doesn't populate this, but you can 716 * use it to hold the resource if you resolve it. (Identification of the 717 * facility/location where the Supply was shipped to, as part of the 718 * dispense event.) 719 */ 720 public Location getDestinationTarget() { 721 if (this.destinationTarget == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 724 else if (Configuration.doAutoCreate()) 725 this.destinationTarget = new Location(); // aa 726 return this.destinationTarget; 727 } 728 729 /** 730 * @param value {@link #destination} The actual object that is the target of the 731 * reference. The reference library doesn't use these, but you can 732 * use it to hold the resource if you resolve it. (Identification 733 * of the facility/location where the Supply was shipped to, as 734 * part of the dispense event.) 735 */ 736 public SupplyDelivery setDestinationTarget(Location value) { 737 this.destinationTarget = value; 738 return this; 739 } 740 741 /** 742 * @return {@link #receiver} (Identifies the person who picked up the Supply.) 743 */ 744 public List<Reference> getReceiver() { 745 if (this.receiver == null) 746 this.receiver = new ArrayList<Reference>(); 747 return this.receiver; 748 } 749 750 public boolean hasReceiver() { 751 if (this.receiver == null) 752 return false; 753 for (Reference item : this.receiver) 754 if (!item.isEmpty()) 755 return true; 756 return false; 757 } 758 759 /** 760 * @return {@link #receiver} (Identifies the person who picked up the Supply.) 761 */ 762 // syntactic sugar 763 public Reference addReceiver() { // 3 764 Reference t = new Reference(); 765 if (this.receiver == null) 766 this.receiver = new ArrayList<Reference>(); 767 this.receiver.add(t); 768 return t; 769 } 770 771 // syntactic sugar 772 public SupplyDelivery addReceiver(Reference t) { // 3 773 if (t == null) 774 return this; 775 if (this.receiver == null) 776 this.receiver = new ArrayList<Reference>(); 777 this.receiver.add(t); 778 return this; 779 } 780 781 /** 782 * @return {@link #receiver} (The actual objects that are the target of the 783 * reference. The reference library doesn't populate this, but you can 784 * use this to hold the resources if you resolvethemt. Identifies the 785 * person who picked up the Supply.) 786 */ 787 public List<Practitioner> getReceiverTarget() { 788 if (this.receiverTarget == null) 789 this.receiverTarget = new ArrayList<Practitioner>(); 790 return this.receiverTarget; 791 } 792 793 // syntactic sugar 794 /** 795 * @return {@link #receiver} (Add an actual object that is the target of the 796 * reference. The reference library doesn't use these, but you can use 797 * this to hold the resources if you resolvethemt. Identifies the person 798 * who picked up the Supply.) 799 */ 800 public Practitioner addReceiverTarget() { 801 Practitioner r = new Practitioner(); 802 if (this.receiverTarget == null) 803 this.receiverTarget = new ArrayList<Practitioner>(); 804 this.receiverTarget.add(r); 805 return r; 806 } 807 808 protected void listChildren(List<Property> childrenList) { 809 super.listChildren(childrenList); 810 childrenList.add(new Property("identifier", "Identifier", 811 "Identifier assigned by the dispensing facility when the item(s) is dispensed.", 0, java.lang.Integer.MAX_VALUE, 812 identifier)); 813 childrenList.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 814 java.lang.Integer.MAX_VALUE, status)); 815 childrenList.add(new Property("patient", "Reference(Patient)", 816 "A link to a resource representing the person whom the delivered item is for.", 0, java.lang.Integer.MAX_VALUE, 817 patient)); 818 childrenList.add(new Property("type", "CodeableConcept", 819 "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 820 0, java.lang.Integer.MAX_VALUE, type)); 821 childrenList.add(new Property("quantity", "SimpleQuantity", 822 "The amount of supply that has been dispensed. Includes unit of measure.", 0, java.lang.Integer.MAX_VALUE, 823 quantity)); 824 childrenList.add(new Property("suppliedItem", "Reference(Medication|Substance|Device)", 825 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a simple attribute carrying a code that identifies the item from a known list.", 826 0, java.lang.Integer.MAX_VALUE, suppliedItem)); 827 childrenList.add(new Property("supplier", "Reference(Practitioner)", 828 "The individual responsible for dispensing the medication, supplier or device.", 0, java.lang.Integer.MAX_VALUE, 829 supplier)); 830 childrenList.add(new Property("whenPrepared", "Period", "The time the dispense event occurred.", 0, 831 java.lang.Integer.MAX_VALUE, whenPrepared)); 832 childrenList.add( 833 new Property("time", "dateTime", "The time the dispensed item was sent or handed to the patient (or agent).", 0, 834 java.lang.Integer.MAX_VALUE, time)); 835 childrenList.add(new Property("destination", "Reference(Location)", 836 "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 837 java.lang.Integer.MAX_VALUE, destination)); 838 childrenList.add(new Property("receiver", "Reference(Practitioner)", 839 "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver)); 840 } 841 842 @Override 843 public void setProperty(String name, Base value) throws FHIRException { 844 if (name.equals("identifier")) 845 this.identifier = castToIdentifier(value); // Identifier 846 else if (name.equals("status")) 847 this.status = new SupplyDeliveryStatusEnumFactory().fromType(value); // Enumeration<SupplyDeliveryStatus> 848 else if (name.equals("patient")) 849 this.patient = castToReference(value); // Reference 850 else if (name.equals("type")) 851 this.type = castToCodeableConcept(value); // CodeableConcept 852 else if (name.equals("quantity")) 853 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 854 else if (name.equals("suppliedItem")) 855 this.suppliedItem = castToReference(value); // Reference 856 else if (name.equals("supplier")) 857 this.supplier = castToReference(value); // Reference 858 else if (name.equals("whenPrepared")) 859 this.whenPrepared = castToPeriod(value); // Period 860 else if (name.equals("time")) 861 this.time = castToDateTime(value); // DateTimeType 862 else if (name.equals("destination")) 863 this.destination = castToReference(value); // Reference 864 else if (name.equals("receiver")) 865 this.getReceiver().add(castToReference(value)); 866 else 867 super.setProperty(name, value); 868 } 869 870 @Override 871 public Base addChild(String name) throws FHIRException { 872 if (name.equals("identifier")) { 873 this.identifier = new Identifier(); 874 return this.identifier; 875 } else if (name.equals("status")) { 876 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.status"); 877 } else if (name.equals("patient")) { 878 this.patient = new Reference(); 879 return this.patient; 880 } else if (name.equals("type")) { 881 this.type = new CodeableConcept(); 882 return this.type; 883 } else if (name.equals("quantity")) { 884 this.quantity = new SimpleQuantity(); 885 return this.quantity; 886 } else if (name.equals("suppliedItem")) { 887 this.suppliedItem = new Reference(); 888 return this.suppliedItem; 889 } else if (name.equals("supplier")) { 890 this.supplier = new Reference(); 891 return this.supplier; 892 } else if (name.equals("whenPrepared")) { 893 this.whenPrepared = new Period(); 894 return this.whenPrepared; 895 } else if (name.equals("time")) { 896 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.time"); 897 } else if (name.equals("destination")) { 898 this.destination = new Reference(); 899 return this.destination; 900 } else if (name.equals("receiver")) { 901 return addReceiver(); 902 } else 903 return super.addChild(name); 904 } 905 906 public String fhirType() { 907 return "SupplyDelivery"; 908 909 } 910 911 public SupplyDelivery copy() { 912 SupplyDelivery dst = new SupplyDelivery(); 913 copyValues(dst); 914 dst.identifier = identifier == null ? null : identifier.copy(); 915 dst.status = status == null ? null : status.copy(); 916 dst.patient = patient == null ? null : patient.copy(); 917 dst.type = type == null ? null : type.copy(); 918 dst.quantity = quantity == null ? null : quantity.copy(); 919 dst.suppliedItem = suppliedItem == null ? null : suppliedItem.copy(); 920 dst.supplier = supplier == null ? null : supplier.copy(); 921 dst.whenPrepared = whenPrepared == null ? null : whenPrepared.copy(); 922 dst.time = time == null ? null : time.copy(); 923 dst.destination = destination == null ? null : destination.copy(); 924 if (receiver != null) { 925 dst.receiver = new ArrayList<Reference>(); 926 for (Reference i : receiver) 927 dst.receiver.add(i.copy()); 928 } 929 ; 930 return dst; 931 } 932 933 protected SupplyDelivery typedCopy() { 934 return copy(); 935 } 936 937 @Override 938 public boolean equalsDeep(Base other) { 939 if (!super.equalsDeep(other)) 940 return false; 941 if (!(other instanceof SupplyDelivery)) 942 return false; 943 SupplyDelivery o = (SupplyDelivery) other; 944 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 945 && compareDeep(patient, o.patient, true) && compareDeep(type, o.type, true) 946 && compareDeep(quantity, o.quantity, true) && compareDeep(suppliedItem, o.suppliedItem, true) 947 && compareDeep(supplier, o.supplier, true) && compareDeep(whenPrepared, o.whenPrepared, true) 948 && compareDeep(time, o.time, true) && compareDeep(destination, o.destination, true) 949 && compareDeep(receiver, o.receiver, true); 950 } 951 952 @Override 953 public boolean equalsShallow(Base other) { 954 if (!super.equalsShallow(other)) 955 return false; 956 if (!(other instanceof SupplyDelivery)) 957 return false; 958 SupplyDelivery o = (SupplyDelivery) other; 959 return compareValues(status, o.status, true) && compareValues(time, o.time, true); 960 } 961 962 public boolean isEmpty() { 963 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 964 && (patient == null || patient.isEmpty()) && (type == null || type.isEmpty()) 965 && (quantity == null || quantity.isEmpty()) && (suppliedItem == null || suppliedItem.isEmpty()) 966 && (supplier == null || supplier.isEmpty()) && (whenPrepared == null || whenPrepared.isEmpty()) 967 && (time == null || time.isEmpty()) && (destination == null || destination.isEmpty()) 968 && (receiver == null || receiver.isEmpty()); 969 } 970 971 @Override 972 public ResourceType getResourceType() { 973 return ResourceType.SupplyDelivery; 974 } 975 976 @SearchParamDefinition(name = "identifier", path = "SupplyDelivery.identifier", description = "External identifier", type = "token") 977 public static final String SP_IDENTIFIER = "identifier"; 978 @SearchParamDefinition(name = "receiver", path = "SupplyDelivery.receiver", description = "Who collected the Supply", type = "reference") 979 public static final String SP_RECEIVER = "receiver"; 980 @SearchParamDefinition(name = "patient", path = "SupplyDelivery.patient", description = "Patient for whom the item is supplied", type = "reference") 981 public static final String SP_PATIENT = "patient"; 982 @SearchParamDefinition(name = "supplier", path = "SupplyDelivery.supplier", description = "Dispenser", type = "reference") 983 public static final String SP_SUPPLIER = "supplier"; 984 @SearchParamDefinition(name = "status", path = "SupplyDelivery.status", description = "in-progress | completed | abandoned", type = "token") 985 public static final String SP_STATUS = "status"; 986 987}