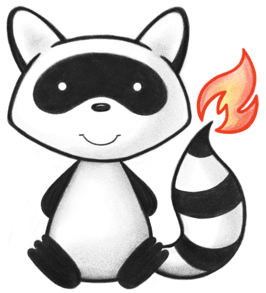
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * A record of a request for a medication, substance or device used in the 047 * healthcare setting. 048 */ 049@ResourceDef(name = "SupplyRequest", profile = "http://hl7.org/fhir/Profile/SupplyRequest") 050public class SupplyRequest extends DomainResource { 051 052 public enum SupplyRequestStatus { 053 /** 054 * Supply has been requested, but not dispensed. 055 */ 056 REQUESTED, 057 /** 058 * Supply has been received by the requestor. 059 */ 060 COMPLETED, 061 /** 062 * The supply will not be completed because the supplier was unable or unwilling 063 * to supply the item. 064 */ 065 FAILED, 066 /** 067 * The orderer of the supply cancelled the request. 068 */ 069 CANCELLED, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static SupplyRequestStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("requested".equals(codeString)) 079 return REQUESTED; 080 if ("completed".equals(codeString)) 081 return COMPLETED; 082 if ("failed".equals(codeString)) 083 return FAILED; 084 if ("cancelled".equals(codeString)) 085 return CANCELLED; 086 throw new FHIRException("Unknown SupplyRequestStatus code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case REQUESTED: 092 return "requested"; 093 case COMPLETED: 094 return "completed"; 095 case FAILED: 096 return "failed"; 097 case CANCELLED: 098 return "cancelled"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 switch (this) { 108 case REQUESTED: 109 return "http://hl7.org/fhir/supplyrequest-status"; 110 case COMPLETED: 111 return "http://hl7.org/fhir/supplyrequest-status"; 112 case FAILED: 113 return "http://hl7.org/fhir/supplyrequest-status"; 114 case CANCELLED: 115 return "http://hl7.org/fhir/supplyrequest-status"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case REQUESTED: 126 return "Supply has been requested, but not dispensed."; 127 case COMPLETED: 128 return "Supply has been received by the requestor."; 129 case FAILED: 130 return "The supply will not be completed because the supplier was unable or unwilling to supply the item."; 131 case CANCELLED: 132 return "The orderer of the supply cancelled the request."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case REQUESTED: 143 return "Requested"; 144 case COMPLETED: 145 return "Received"; 146 case FAILED: 147 return "Failed"; 148 case CANCELLED: 149 return "Cancelled"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class SupplyRequestStatusEnumFactory implements EnumFactory<SupplyRequestStatus> { 159 public SupplyRequestStatus fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("requested".equals(codeString)) 164 return SupplyRequestStatus.REQUESTED; 165 if ("completed".equals(codeString)) 166 return SupplyRequestStatus.COMPLETED; 167 if ("failed".equals(codeString)) 168 return SupplyRequestStatus.FAILED; 169 if ("cancelled".equals(codeString)) 170 return SupplyRequestStatus.CANCELLED; 171 throw new IllegalArgumentException("Unknown SupplyRequestStatus code '" + codeString + "'"); 172 } 173 174 public Enumeration<SupplyRequestStatus> fromType(Base code) throws FHIRException { 175 if (code == null || code.isEmpty()) 176 return null; 177 String codeString = ((PrimitiveType) code).asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("requested".equals(codeString)) 181 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.REQUESTED); 182 if ("completed".equals(codeString)) 183 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.COMPLETED); 184 if ("failed".equals(codeString)) 185 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.FAILED); 186 if ("cancelled".equals(codeString)) 187 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.CANCELLED); 188 throw new FHIRException("Unknown SupplyRequestStatus code '" + codeString + "'"); 189 } 190 191 public String toCode(SupplyRequestStatus code) 192 { 193 if (code == SupplyRequestStatus.NULL) 194 return null; 195 if (code == SupplyRequestStatus.REQUESTED) 196 return "requested"; 197 if (code == SupplyRequestStatus.COMPLETED) 198 return "completed"; 199 if (code == SupplyRequestStatus.FAILED) 200 return "failed"; 201 if (code == SupplyRequestStatus.CANCELLED) 202 return "cancelled"; 203 return "?"; 204 } 205 } 206 207 @Block() 208 public static class SupplyRequestWhenComponent extends BackboneElement implements IBaseBackboneElement { 209 /** 210 * Code indicating when the request should be fulfilled. 211 */ 212 @Child(name = "code", type = { 213 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 214 @Description(shortDefinition = "Fulfilment code", formalDefinition = "Code indicating when the request should be fulfilled.") 215 protected CodeableConcept code; 216 217 /** 218 * Formal fulfillment schedule. 219 */ 220 @Child(name = "schedule", type = { Timing.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 221 @Description(shortDefinition = "Formal fulfillment schedule", formalDefinition = "Formal fulfillment schedule.") 222 protected Timing schedule; 223 224 private static final long serialVersionUID = 307115287L; 225 226 /* 227 * Constructor 228 */ 229 public SupplyRequestWhenComponent() { 230 super(); 231 } 232 233 /** 234 * @return {@link #code} (Code indicating when the request should be fulfilled.) 235 */ 236 public CodeableConcept getCode() { 237 if (this.code == null) 238 if (Configuration.errorOnAutoCreate()) 239 throw new Error("Attempt to auto-create SupplyRequestWhenComponent.code"); 240 else if (Configuration.doAutoCreate()) 241 this.code = new CodeableConcept(); // cc 242 return this.code; 243 } 244 245 public boolean hasCode() { 246 return this.code != null && !this.code.isEmpty(); 247 } 248 249 /** 250 * @param value {@link #code} (Code indicating when the request should be 251 * fulfilled.) 252 */ 253 public SupplyRequestWhenComponent setCode(CodeableConcept value) { 254 this.code = value; 255 return this; 256 } 257 258 /** 259 * @return {@link #schedule} (Formal fulfillment schedule.) 260 */ 261 public Timing getSchedule() { 262 if (this.schedule == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create SupplyRequestWhenComponent.schedule"); 265 else if (Configuration.doAutoCreate()) 266 this.schedule = new Timing(); // cc 267 return this.schedule; 268 } 269 270 public boolean hasSchedule() { 271 return this.schedule != null && !this.schedule.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #schedule} (Formal fulfillment schedule.) 276 */ 277 public SupplyRequestWhenComponent setSchedule(Timing value) { 278 this.schedule = value; 279 return this; 280 } 281 282 protected void listChildren(List<Property> childrenList) { 283 super.listChildren(childrenList); 284 childrenList.add(new Property("code", "CodeableConcept", "Code indicating when the request should be fulfilled.", 285 0, java.lang.Integer.MAX_VALUE, code)); 286 childrenList.add( 287 new Property("schedule", "Timing", "Formal fulfillment schedule.", 0, java.lang.Integer.MAX_VALUE, schedule)); 288 } 289 290 @Override 291 public void setProperty(String name, Base value) throws FHIRException { 292 if (name.equals("code")) 293 this.code = castToCodeableConcept(value); // CodeableConcept 294 else if (name.equals("schedule")) 295 this.schedule = castToTiming(value); // Timing 296 else 297 super.setProperty(name, value); 298 } 299 300 @Override 301 public Base addChild(String name) throws FHIRException { 302 if (name.equals("code")) { 303 this.code = new CodeableConcept(); 304 return this.code; 305 } else if (name.equals("schedule")) { 306 this.schedule = new Timing(); 307 return this.schedule; 308 } else 309 return super.addChild(name); 310 } 311 312 public SupplyRequestWhenComponent copy() { 313 SupplyRequestWhenComponent dst = new SupplyRequestWhenComponent(); 314 copyValues(dst); 315 dst.code = code == null ? null : code.copy(); 316 dst.schedule = schedule == null ? null : schedule.copy(); 317 return dst; 318 } 319 320 @Override 321 public boolean equalsDeep(Base other) { 322 if (!super.equalsDeep(other)) 323 return false; 324 if (!(other instanceof SupplyRequestWhenComponent)) 325 return false; 326 SupplyRequestWhenComponent o = (SupplyRequestWhenComponent) other; 327 return compareDeep(code, o.code, true) && compareDeep(schedule, o.schedule, true); 328 } 329 330 @Override 331 public boolean equalsShallow(Base other) { 332 if (!super.equalsShallow(other)) 333 return false; 334 if (!(other instanceof SupplyRequestWhenComponent)) 335 return false; 336 SupplyRequestWhenComponent o = (SupplyRequestWhenComponent) other; 337 return true; 338 } 339 340 public boolean isEmpty() { 341 return super.isEmpty() && (code == null || code.isEmpty()) && (schedule == null || schedule.isEmpty()); 342 } 343 344 public String fhirType() { 345 return "SupplyRequest.when"; 346 347 } 348 349 } 350 351 /** 352 * A link to a resource representing the person whom the ordered item is for. 353 */ 354 @Child(name = "patient", type = { Patient.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 355 @Description(shortDefinition = "Patient for whom the item is supplied", formalDefinition = "A link to a resource representing the person whom the ordered item is for.") 356 protected Reference patient; 357 358 /** 359 * The actual object that is the target of the reference (A link to a resource 360 * representing the person whom the ordered item is for.) 361 */ 362 protected Patient patientTarget; 363 364 /** 365 * The Practitioner , Organization or Patient who initiated this order for the 366 * supply. 367 */ 368 @Child(name = "source", type = { Practitioner.class, Organization.class, 369 Patient.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 370 @Description(shortDefinition = "Who initiated this order", formalDefinition = "The Practitioner , Organization or Patient who initiated this order for the supply.") 371 protected Reference source; 372 373 /** 374 * The actual object that is the target of the reference (The Practitioner , 375 * Organization or Patient who initiated this order for the supply.) 376 */ 377 protected Resource sourceTarget; 378 379 /** 380 * When the request was made. 381 */ 382 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 383 @Description(shortDefinition = "When the request was made", formalDefinition = "When the request was made.") 384 protected DateTimeType date; 385 386 /** 387 * Unique identifier for this supply request. 388 */ 389 @Child(name = "identifier", type = { 390 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 391 @Description(shortDefinition = "Unique identifier", formalDefinition = "Unique identifier for this supply request.") 392 protected Identifier identifier; 393 394 /** 395 * Status of the supply request. 396 */ 397 @Child(name = "status", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = true, summary = true) 398 @Description(shortDefinition = "requested | completed | failed | cancelled", formalDefinition = "Status of the supply request.") 399 protected Enumeration<SupplyRequestStatus> status; 400 401 /** 402 * Category of supply, e.g. central, non-stock, etc. This is used to support 403 * work flows associated with the supply process. 404 */ 405 @Child(name = "kind", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 406 @Description(shortDefinition = "The kind of supply (central, non-stock, etc.)", formalDefinition = "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.") 407 protected CodeableConcept kind; 408 409 /** 410 * The item that is requested to be supplied. 411 */ 412 @Child(name = "orderedItem", type = { Medication.class, Substance.class, 413 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 414 @Description(shortDefinition = "Medication, Substance, or Device requested to be supplied", formalDefinition = "The item that is requested to be supplied.") 415 protected Reference orderedItem; 416 417 /** 418 * The actual object that is the target of the reference (The item that is 419 * requested to be supplied.) 420 */ 421 protected Resource orderedItemTarget; 422 423 /** 424 * Who is intended to fulfill the request. 425 */ 426 @Child(name = "supplier", type = { 427 Organization.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 428 @Description(shortDefinition = "Who is intended to fulfill the request", formalDefinition = "Who is intended to fulfill the request.") 429 protected List<Reference> supplier; 430 /** 431 * The actual objects that are the target of the reference (Who is intended to 432 * fulfill the request.) 433 */ 434 protected List<Organization> supplierTarget; 435 436 /** 437 * Why the supply item was requested. 438 */ 439 @Child(name = "reason", type = { 440 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 441 @Description(shortDefinition = "Why the supply item was requested", formalDefinition = "Why the supply item was requested.") 442 protected Type reason; 443 444 /** 445 * When the request should be fulfilled. 446 */ 447 @Child(name = "when", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = true) 448 @Description(shortDefinition = "When the request should be fulfilled", formalDefinition = "When the request should be fulfilled.") 449 protected SupplyRequestWhenComponent when; 450 451 private static final long serialVersionUID = 1649766198L; 452 453 /* 454 * Constructor 455 */ 456 public SupplyRequest() { 457 super(); 458 } 459 460 /** 461 * @return {@link #patient} (A link to a resource representing the person whom 462 * the ordered item is for.) 463 */ 464 public Reference getPatient() { 465 if (this.patient == null) 466 if (Configuration.errorOnAutoCreate()) 467 throw new Error("Attempt to auto-create SupplyRequest.patient"); 468 else if (Configuration.doAutoCreate()) 469 this.patient = new Reference(); // cc 470 return this.patient; 471 } 472 473 public boolean hasPatient() { 474 return this.patient != null && !this.patient.isEmpty(); 475 } 476 477 /** 478 * @param value {@link #patient} (A link to a resource representing the person 479 * whom the ordered item is for.) 480 */ 481 public SupplyRequest setPatient(Reference value) { 482 this.patient = value; 483 return this; 484 } 485 486 /** 487 * @return {@link #patient} The actual object that is the target of the 488 * reference. The reference library doesn't populate this, but you can 489 * use it to hold the resource if you resolve it. (A link to a resource 490 * representing the person whom the ordered item is for.) 491 */ 492 public Patient getPatientTarget() { 493 if (this.patientTarget == null) 494 if (Configuration.errorOnAutoCreate()) 495 throw new Error("Attempt to auto-create SupplyRequest.patient"); 496 else if (Configuration.doAutoCreate()) 497 this.patientTarget = new Patient(); // aa 498 return this.patientTarget; 499 } 500 501 /** 502 * @param value {@link #patient} The actual object that is the target of the 503 * reference. The reference library doesn't use these, but you can 504 * use it to hold the resource if you resolve it. (A link to a 505 * resource representing the person whom the ordered item is for.) 506 */ 507 public SupplyRequest setPatientTarget(Patient value) { 508 this.patientTarget = value; 509 return this; 510 } 511 512 /** 513 * @return {@link #source} (The Practitioner , Organization or Patient who 514 * initiated this order for the supply.) 515 */ 516 public Reference getSource() { 517 if (this.source == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create SupplyRequest.source"); 520 else if (Configuration.doAutoCreate()) 521 this.source = new Reference(); // cc 522 return this.source; 523 } 524 525 public boolean hasSource() { 526 return this.source != null && !this.source.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #source} (The Practitioner , Organization or Patient who 531 * initiated this order for the supply.) 532 */ 533 public SupplyRequest setSource(Reference value) { 534 this.source = value; 535 return this; 536 } 537 538 /** 539 * @return {@link #source} The actual object that is the target of the 540 * reference. The reference library doesn't populate this, but you can 541 * use it to hold the resource if you resolve it. (The Practitioner , 542 * Organization or Patient who initiated this order for the supply.) 543 */ 544 public Resource getSourceTarget() { 545 return this.sourceTarget; 546 } 547 548 /** 549 * @param value {@link #source} The actual object that is the target of the 550 * reference. The reference library doesn't use these, but you can 551 * use it to hold the resource if you resolve it. (The Practitioner 552 * , Organization or Patient who initiated this order for the 553 * supply.) 554 */ 555 public SupplyRequest setSourceTarget(Resource value) { 556 this.sourceTarget = value; 557 return this; 558 } 559 560 /** 561 * @return {@link #date} (When the request was made.). This is the underlying 562 * object with id, value and extensions. The accessor "getDate" gives 563 * direct access to the value 564 */ 565 public DateTimeType getDateElement() { 566 if (this.date == null) 567 if (Configuration.errorOnAutoCreate()) 568 throw new Error("Attempt to auto-create SupplyRequest.date"); 569 else if (Configuration.doAutoCreate()) 570 this.date = new DateTimeType(); // bb 571 return this.date; 572 } 573 574 public boolean hasDateElement() { 575 return this.date != null && !this.date.isEmpty(); 576 } 577 578 public boolean hasDate() { 579 return this.date != null && !this.date.isEmpty(); 580 } 581 582 /** 583 * @param value {@link #date} (When the request was made.). This is the 584 * underlying object with id, value and extensions. The accessor 585 * "getDate" gives direct access to the value 586 */ 587 public SupplyRequest setDateElement(DateTimeType value) { 588 this.date = value; 589 return this; 590 } 591 592 /** 593 * @return When the request was made. 594 */ 595 public Date getDate() { 596 return this.date == null ? null : this.date.getValue(); 597 } 598 599 /** 600 * @param value When the request was made. 601 */ 602 public SupplyRequest setDate(Date value) { 603 if (value == null) 604 this.date = null; 605 else { 606 if (this.date == null) 607 this.date = new DateTimeType(); 608 this.date.setValue(value); 609 } 610 return this; 611 } 612 613 /** 614 * @return {@link #identifier} (Unique identifier for this supply request.) 615 */ 616 public Identifier getIdentifier() { 617 if (this.identifier == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create SupplyRequest.identifier"); 620 else if (Configuration.doAutoCreate()) 621 this.identifier = new Identifier(); // cc 622 return this.identifier; 623 } 624 625 public boolean hasIdentifier() { 626 return this.identifier != null && !this.identifier.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #identifier} (Unique identifier for this supply request.) 631 */ 632 public SupplyRequest setIdentifier(Identifier value) { 633 this.identifier = value; 634 return this; 635 } 636 637 /** 638 * @return {@link #status} (Status of the supply request.). This is the 639 * underlying object with id, value and extensions. The accessor 640 * "getStatus" gives direct access to the value 641 */ 642 public Enumeration<SupplyRequestStatus> getStatusElement() { 643 if (this.status == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create SupplyRequest.status"); 646 else if (Configuration.doAutoCreate()) 647 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); // bb 648 return this.status; 649 } 650 651 public boolean hasStatusElement() { 652 return this.status != null && !this.status.isEmpty(); 653 } 654 655 public boolean hasStatus() { 656 return this.status != null && !this.status.isEmpty(); 657 } 658 659 /** 660 * @param value {@link #status} (Status of the supply request.). This is the 661 * underlying object with id, value and extensions. The accessor 662 * "getStatus" gives direct access to the value 663 */ 664 public SupplyRequest setStatusElement(Enumeration<SupplyRequestStatus> value) { 665 this.status = value; 666 return this; 667 } 668 669 /** 670 * @return Status of the supply request. 671 */ 672 public SupplyRequestStatus getStatus() { 673 return this.status == null ? null : this.status.getValue(); 674 } 675 676 /** 677 * @param value Status of the supply request. 678 */ 679 public SupplyRequest setStatus(SupplyRequestStatus value) { 680 if (value == null) 681 this.status = null; 682 else { 683 if (this.status == null) 684 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); 685 this.status.setValue(value); 686 } 687 return this; 688 } 689 690 /** 691 * @return {@link #kind} (Category of supply, e.g. central, non-stock, etc. This 692 * is used to support work flows associated with the supply process.) 693 */ 694 public CodeableConcept getKind() { 695 if (this.kind == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create SupplyRequest.kind"); 698 else if (Configuration.doAutoCreate()) 699 this.kind = new CodeableConcept(); // cc 700 return this.kind; 701 } 702 703 public boolean hasKind() { 704 return this.kind != null && !this.kind.isEmpty(); 705 } 706 707 /** 708 * @param value {@link #kind} (Category of supply, e.g. central, non-stock, etc. 709 * This is used to support work flows associated with the supply 710 * process.) 711 */ 712 public SupplyRequest setKind(CodeableConcept value) { 713 this.kind = value; 714 return this; 715 } 716 717 /** 718 * @return {@link #orderedItem} (The item that is requested to be supplied.) 719 */ 720 public Reference getOrderedItem() { 721 if (this.orderedItem == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create SupplyRequest.orderedItem"); 724 else if (Configuration.doAutoCreate()) 725 this.orderedItem = new Reference(); // cc 726 return this.orderedItem; 727 } 728 729 public boolean hasOrderedItem() { 730 return this.orderedItem != null && !this.orderedItem.isEmpty(); 731 } 732 733 /** 734 * @param value {@link #orderedItem} (The item that is requested to be 735 * supplied.) 736 */ 737 public SupplyRequest setOrderedItem(Reference value) { 738 this.orderedItem = value; 739 return this; 740 } 741 742 /** 743 * @return {@link #orderedItem} The actual object that is the target of the 744 * reference. The reference library doesn't populate this, but you can 745 * use it to hold the resource if you resolve it. (The item that is 746 * requested to be supplied.) 747 */ 748 public Resource getOrderedItemTarget() { 749 return this.orderedItemTarget; 750 } 751 752 /** 753 * @param value {@link #orderedItem} The actual object that is the target of the 754 * reference. The reference library doesn't use these, but you can 755 * use it to hold the resource if you resolve it. (The item that is 756 * requested to be supplied.) 757 */ 758 public SupplyRequest setOrderedItemTarget(Resource value) { 759 this.orderedItemTarget = value; 760 return this; 761 } 762 763 /** 764 * @return {@link #supplier} (Who is intended to fulfill the request.) 765 */ 766 public List<Reference> getSupplier() { 767 if (this.supplier == null) 768 this.supplier = new ArrayList<Reference>(); 769 return this.supplier; 770 } 771 772 public boolean hasSupplier() { 773 if (this.supplier == null) 774 return false; 775 for (Reference item : this.supplier) 776 if (!item.isEmpty()) 777 return true; 778 return false; 779 } 780 781 /** 782 * @return {@link #supplier} (Who is intended to fulfill the request.) 783 */ 784 // syntactic sugar 785 public Reference addSupplier() { // 3 786 Reference t = new Reference(); 787 if (this.supplier == null) 788 this.supplier = new ArrayList<Reference>(); 789 this.supplier.add(t); 790 return t; 791 } 792 793 // syntactic sugar 794 public SupplyRequest addSupplier(Reference t) { // 3 795 if (t == null) 796 return this; 797 if (this.supplier == null) 798 this.supplier = new ArrayList<Reference>(); 799 this.supplier.add(t); 800 return this; 801 } 802 803 /** 804 * @return {@link #supplier} (The actual objects that are the target of the 805 * reference. The reference library doesn't populate this, but you can 806 * use this to hold the resources if you resolvethemt. Who is intended 807 * to fulfill the request.) 808 */ 809 public List<Organization> getSupplierTarget() { 810 if (this.supplierTarget == null) 811 this.supplierTarget = new ArrayList<Organization>(); 812 return this.supplierTarget; 813 } 814 815 // syntactic sugar 816 /** 817 * @return {@link #supplier} (Add an actual object that is the target of the 818 * reference. The reference library doesn't use these, but you can use 819 * this to hold the resources if you resolvethemt. Who is intended to 820 * fulfill the request.) 821 */ 822 public Organization addSupplierTarget() { 823 Organization r = new Organization(); 824 if (this.supplierTarget == null) 825 this.supplierTarget = new ArrayList<Organization>(); 826 this.supplierTarget.add(r); 827 return r; 828 } 829 830 /** 831 * @return {@link #reason} (Why the supply item was requested.) 832 */ 833 public Type getReason() { 834 return this.reason; 835 } 836 837 /** 838 * @return {@link #reason} (Why the supply item was requested.) 839 */ 840 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 841 if (!(this.reason instanceof CodeableConcept)) 842 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 843 + this.reason.getClass().getName() + " was encountered"); 844 return (CodeableConcept) this.reason; 845 } 846 847 public boolean hasReasonCodeableConcept() { 848 return this.reason instanceof CodeableConcept; 849 } 850 851 /** 852 * @return {@link #reason} (Why the supply item was requested.) 853 */ 854 public Reference getReasonReference() throws FHIRException { 855 if (!(this.reason instanceof Reference)) 856 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.reason.getClass().getName() 857 + " was encountered"); 858 return (Reference) this.reason; 859 } 860 861 public boolean hasReasonReference() { 862 return this.reason instanceof Reference; 863 } 864 865 public boolean hasReason() { 866 return this.reason != null && !this.reason.isEmpty(); 867 } 868 869 /** 870 * @param value {@link #reason} (Why the supply item was requested.) 871 */ 872 public SupplyRequest setReason(Type value) { 873 this.reason = value; 874 return this; 875 } 876 877 /** 878 * @return {@link #when} (When the request should be fulfilled.) 879 */ 880 public SupplyRequestWhenComponent getWhen() { 881 if (this.when == null) 882 if (Configuration.errorOnAutoCreate()) 883 throw new Error("Attempt to auto-create SupplyRequest.when"); 884 else if (Configuration.doAutoCreate()) 885 this.when = new SupplyRequestWhenComponent(); // cc 886 return this.when; 887 } 888 889 public boolean hasWhen() { 890 return this.when != null && !this.when.isEmpty(); 891 } 892 893 /** 894 * @param value {@link #when} (When the request should be fulfilled.) 895 */ 896 public SupplyRequest setWhen(SupplyRequestWhenComponent value) { 897 this.when = value; 898 return this; 899 } 900 901 protected void listChildren(List<Property> childrenList) { 902 super.listChildren(childrenList); 903 childrenList.add(new Property("patient", "Reference(Patient)", 904 "A link to a resource representing the person whom the ordered item is for.", 0, java.lang.Integer.MAX_VALUE, 905 patient)); 906 childrenList.add(new Property("source", "Reference(Practitioner|Organization|Patient)", 907 "The Practitioner , Organization or Patient who initiated this order for the supply.", 0, 908 java.lang.Integer.MAX_VALUE, source)); 909 childrenList 910 .add(new Property("date", "dateTime", "When the request was made.", 0, java.lang.Integer.MAX_VALUE, date)); 911 childrenList.add(new Property("identifier", "Identifier", "Unique identifier for this supply request.", 0, 912 java.lang.Integer.MAX_VALUE, identifier)); 913 childrenList 914 .add(new Property("status", "code", "Status of the supply request.", 0, java.lang.Integer.MAX_VALUE, status)); 915 childrenList.add(new Property("kind", "CodeableConcept", 916 "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 917 0, java.lang.Integer.MAX_VALUE, kind)); 918 childrenList.add(new Property("orderedItem", "Reference(Medication|Substance|Device)", 919 "The item that is requested to be supplied.", 0, java.lang.Integer.MAX_VALUE, orderedItem)); 920 childrenList.add(new Property("supplier", "Reference(Organization)", "Who is intended to fulfill the request.", 0, 921 java.lang.Integer.MAX_VALUE, supplier)); 922 childrenList.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "Why the supply item was requested.", 923 0, java.lang.Integer.MAX_VALUE, reason)); 924 childrenList 925 .add(new Property("when", "", "When the request should be fulfilled.", 0, java.lang.Integer.MAX_VALUE, when)); 926 } 927 928 @Override 929 public void setProperty(String name, Base value) throws FHIRException { 930 if (name.equals("patient")) 931 this.patient = castToReference(value); // Reference 932 else if (name.equals("source")) 933 this.source = castToReference(value); // Reference 934 else if (name.equals("date")) 935 this.date = castToDateTime(value); // DateTimeType 936 else if (name.equals("identifier")) 937 this.identifier = castToIdentifier(value); // Identifier 938 else if (name.equals("status")) 939 this.status = new SupplyRequestStatusEnumFactory().fromType(value); // Enumeration<SupplyRequestStatus> 940 else if (name.equals("kind")) 941 this.kind = castToCodeableConcept(value); // CodeableConcept 942 else if (name.equals("orderedItem")) 943 this.orderedItem = castToReference(value); // Reference 944 else if (name.equals("supplier")) 945 this.getSupplier().add(castToReference(value)); 946 else if (name.equals("reason[x]")) 947 this.reason = (Type) value; // Type 948 else if (name.equals("when")) 949 this.when = (SupplyRequestWhenComponent) value; // SupplyRequestWhenComponent 950 else 951 super.setProperty(name, value); 952 } 953 954 @Override 955 public Base addChild(String name) throws FHIRException { 956 if (name.equals("patient")) { 957 this.patient = new Reference(); 958 return this.patient; 959 } else if (name.equals("source")) { 960 this.source = new Reference(); 961 return this.source; 962 } else if (name.equals("date")) { 963 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.date"); 964 } else if (name.equals("identifier")) { 965 this.identifier = new Identifier(); 966 return this.identifier; 967 } else if (name.equals("status")) { 968 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.status"); 969 } else if (name.equals("kind")) { 970 this.kind = new CodeableConcept(); 971 return this.kind; 972 } else if (name.equals("orderedItem")) { 973 this.orderedItem = new Reference(); 974 return this.orderedItem; 975 } else if (name.equals("supplier")) { 976 return addSupplier(); 977 } else if (name.equals("reasonCodeableConcept")) { 978 this.reason = new CodeableConcept(); 979 return this.reason; 980 } else if (name.equals("reasonReference")) { 981 this.reason = new Reference(); 982 return this.reason; 983 } else if (name.equals("when")) { 984 this.when = new SupplyRequestWhenComponent(); 985 return this.when; 986 } else 987 return super.addChild(name); 988 } 989 990 public String fhirType() { 991 return "SupplyRequest"; 992 993 } 994 995 public SupplyRequest copy() { 996 SupplyRequest dst = new SupplyRequest(); 997 copyValues(dst); 998 dst.patient = patient == null ? null : patient.copy(); 999 dst.source = source == null ? null : source.copy(); 1000 dst.date = date == null ? null : date.copy(); 1001 dst.identifier = identifier == null ? null : identifier.copy(); 1002 dst.status = status == null ? null : status.copy(); 1003 dst.kind = kind == null ? null : kind.copy(); 1004 dst.orderedItem = orderedItem == null ? null : orderedItem.copy(); 1005 if (supplier != null) { 1006 dst.supplier = new ArrayList<Reference>(); 1007 for (Reference i : supplier) 1008 dst.supplier.add(i.copy()); 1009 } 1010 ; 1011 dst.reason = reason == null ? null : reason.copy(); 1012 dst.when = when == null ? null : when.copy(); 1013 return dst; 1014 } 1015 1016 protected SupplyRequest typedCopy() { 1017 return copy(); 1018 } 1019 1020 @Override 1021 public boolean equalsDeep(Base other) { 1022 if (!super.equalsDeep(other)) 1023 return false; 1024 if (!(other instanceof SupplyRequest)) 1025 return false; 1026 SupplyRequest o = (SupplyRequest) other; 1027 return compareDeep(patient, o.patient, true) && compareDeep(source, o.source, true) 1028 && compareDeep(date, o.date, true) && compareDeep(identifier, o.identifier, true) 1029 && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) 1030 && compareDeep(orderedItem, o.orderedItem, true) && compareDeep(supplier, o.supplier, true) 1031 && compareDeep(reason, o.reason, true) && compareDeep(when, o.when, true); 1032 } 1033 1034 @Override 1035 public boolean equalsShallow(Base other) { 1036 if (!super.equalsShallow(other)) 1037 return false; 1038 if (!(other instanceof SupplyRequest)) 1039 return false; 1040 SupplyRequest o = (SupplyRequest) other; 1041 return compareValues(date, o.date, true) && compareValues(status, o.status, true); 1042 } 1043 1044 public boolean isEmpty() { 1045 return super.isEmpty() && (patient == null || patient.isEmpty()) && (source == null || source.isEmpty()) 1046 && (date == null || date.isEmpty()) && (identifier == null || identifier.isEmpty()) 1047 && (status == null || status.isEmpty()) && (kind == null || kind.isEmpty()) 1048 && (orderedItem == null || orderedItem.isEmpty()) && (supplier == null || supplier.isEmpty()) 1049 && (reason == null || reason.isEmpty()) && (when == null || when.isEmpty()); 1050 } 1051 1052 @Override 1053 public ResourceType getResourceType() { 1054 return ResourceType.SupplyRequest; 1055 } 1056 1057 @SearchParamDefinition(name = "date", path = "SupplyRequest.date", description = "When the request was made", type = "date") 1058 public static final String SP_DATE = "date"; 1059 @SearchParamDefinition(name = "identifier", path = "SupplyRequest.identifier", description = "Unique identifier", type = "token") 1060 public static final String SP_IDENTIFIER = "identifier"; 1061 @SearchParamDefinition(name = "kind", path = "SupplyRequest.kind", description = "The kind of supply (central, non-stock, etc.)", type = "token") 1062 public static final String SP_KIND = "kind"; 1063 @SearchParamDefinition(name = "patient", path = "SupplyRequest.patient", description = "Patient for whom the item is supplied", type = "reference") 1064 public static final String SP_PATIENT = "patient"; 1065 @SearchParamDefinition(name = "supplier", path = "SupplyRequest.supplier", description = "Who is intended to fulfill the request", type = "reference") 1066 public static final String SP_SUPPLIER = "supplier"; 1067 @SearchParamDefinition(name = "source", path = "SupplyRequest.source", description = "Who initiated this order", type = "reference") 1068 public static final String SP_SOURCE = "source"; 1069 @SearchParamDefinition(name = "status", path = "SupplyRequest.status", description = "requested | completed | failed | cancelled", type = "token") 1070 public static final String SP_STATUS = "status"; 1071 1072}