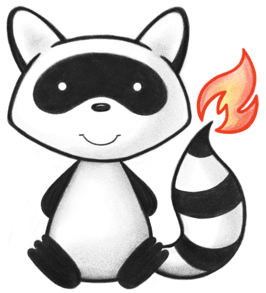
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * TestScript is a resource that specifies a suite of tests against a FHIR 050 * server implementation to determine compliance against the FHIR specification. 051 */ 052@ResourceDef(name = "TestScript", profile = "http://hl7.org/fhir/Profile/TestScript") 053public class TestScript extends DomainResource { 054 055 public enum ContentType { 056 /** 057 * XML content-type corresponding to the application/xml+fhir mime-type. 058 */ 059 XML, 060 /** 061 * JSON content-type corresponding to the application/json+fhir mime-type. 062 */ 063 JSON, 064 /** 065 * added to help the parsers 066 */ 067 NULL; 068 069 public static ContentType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("xml".equals(codeString)) 073 return XML; 074 if ("json".equals(codeString)) 075 return JSON; 076 throw new FHIRException("Unknown ContentType code '" + codeString + "'"); 077 } 078 079 public String toCode() { 080 switch (this) { 081 case XML: 082 return "xml"; 083 case JSON: 084 return "json"; 085 case NULL: 086 return null; 087 default: 088 return "?"; 089 } 090 } 091 092 public String getSystem() { 093 switch (this) { 094 case XML: 095 return "http://hl7.org/fhir/content-type"; 096 case JSON: 097 return "http://hl7.org/fhir/content-type"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getDefinition() { 106 switch (this) { 107 case XML: 108 return "XML content-type corresponding to the application/xml+fhir mime-type."; 109 case JSON: 110 return "JSON content-type corresponding to the application/json+fhir mime-type."; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getDisplay() { 119 switch (this) { 120 case XML: 121 return "xml"; 122 case JSON: 123 return "json"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 } 131 132 public static class ContentTypeEnumFactory implements EnumFactory<ContentType> { 133 public ContentType fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("xml".equals(codeString)) 138 return ContentType.XML; 139 if ("json".equals(codeString)) 140 return ContentType.JSON; 141 throw new IllegalArgumentException("Unknown ContentType code '" + codeString + "'"); 142 } 143 144 public Enumeration<ContentType> fromType(Base code) throws FHIRException { 145 if (code == null || code.isEmpty()) 146 return null; 147 String codeString = ((PrimitiveType) code).asStringValue(); 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("xml".equals(codeString)) 151 return new Enumeration<ContentType>(this, ContentType.XML); 152 if ("json".equals(codeString)) 153 return new Enumeration<ContentType>(this, ContentType.JSON); 154 throw new FHIRException("Unknown ContentType code '" + codeString + "'"); 155 } 156 157 public String toCode(ContentType code) 158 { 159 if (code == ContentType.NULL) 160 return null; 161 if (code == ContentType.XML) 162 return "xml"; 163 if (code == ContentType.JSON) 164 return "json"; 165 return "?"; 166 } 167 } 168 169 public enum AssertionDirectionType { 170 /** 171 * The assertion is evaluated on the response. This is the default value. 172 */ 173 RESPONSE, 174 /** 175 * The assertion is evaluated on the request. 176 */ 177 REQUEST, 178 /** 179 * added to help the parsers 180 */ 181 NULL; 182 183 public static AssertionDirectionType fromCode(String codeString) throws FHIRException { 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("response".equals(codeString)) 187 return RESPONSE; 188 if ("request".equals(codeString)) 189 return REQUEST; 190 throw new FHIRException("Unknown AssertionDirectionType code '" + codeString + "'"); 191 } 192 193 public String toCode() { 194 switch (this) { 195 case RESPONSE: 196 return "response"; 197 case REQUEST: 198 return "request"; 199 case NULL: 200 return null; 201 default: 202 return "?"; 203 } 204 } 205 206 public String getSystem() { 207 switch (this) { 208 case RESPONSE: 209 return "http://hl7.org/fhir/assert-direction-codes"; 210 case REQUEST: 211 return "http://hl7.org/fhir/assert-direction-codes"; 212 case NULL: 213 return null; 214 default: 215 return "?"; 216 } 217 } 218 219 public String getDefinition() { 220 switch (this) { 221 case RESPONSE: 222 return "The assertion is evaluated on the response. This is the default value."; 223 case REQUEST: 224 return "The assertion is evaluated on the request."; 225 case NULL: 226 return null; 227 default: 228 return "?"; 229 } 230 } 231 232 public String getDisplay() { 233 switch (this) { 234 case RESPONSE: 235 return "response"; 236 case REQUEST: 237 return "request"; 238 case NULL: 239 return null; 240 default: 241 return "?"; 242 } 243 } 244 } 245 246 public static class AssertionDirectionTypeEnumFactory implements EnumFactory<AssertionDirectionType> { 247 public AssertionDirectionType fromCode(String codeString) throws IllegalArgumentException { 248 if (codeString == null || "".equals(codeString)) 249 if (codeString == null || "".equals(codeString)) 250 return null; 251 if ("response".equals(codeString)) 252 return AssertionDirectionType.RESPONSE; 253 if ("request".equals(codeString)) 254 return AssertionDirectionType.REQUEST; 255 throw new IllegalArgumentException("Unknown AssertionDirectionType code '" + codeString + "'"); 256 } 257 258 public Enumeration<AssertionDirectionType> fromType(Base code) throws FHIRException { 259 if (code == null || code.isEmpty()) 260 return null; 261 String codeString = ((PrimitiveType) code).asStringValue(); 262 if (codeString == null || "".equals(codeString)) 263 return null; 264 if ("response".equals(codeString)) 265 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.RESPONSE); 266 if ("request".equals(codeString)) 267 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.REQUEST); 268 throw new FHIRException("Unknown AssertionDirectionType code '" + codeString + "'"); 269 } 270 271 public String toCode(AssertionDirectionType code) 272 { 273 if (code == AssertionDirectionType.NULL) 274 return null; 275 if (code == AssertionDirectionType.RESPONSE) 276 return "response"; 277 if (code == AssertionDirectionType.REQUEST) 278 return "request"; 279 return "?"; 280 } 281 } 282 283 public enum AssertionOperatorType { 284 /** 285 * Default value. Equals comparison. 286 */ 287 EQUALS, 288 /** 289 * Not equals comparison. 290 */ 291 NOTEQUALS, 292 /** 293 * Compare value within a known set of values. 294 */ 295 IN, 296 /** 297 * Compare value not within a known set of values. 298 */ 299 NOTIN, 300 /** 301 * Compare value to be greater than a known value. 302 */ 303 GREATERTHAN, 304 /** 305 * Compare value to be less than a known value. 306 */ 307 LESSTHAN, 308 /** 309 * Compare value is empty. 310 */ 311 EMPTY, 312 /** 313 * Compare value is not empty. 314 */ 315 NOTEMPTY, 316 /** 317 * Compare value string contains a known value. 318 */ 319 CONTAINS, 320 /** 321 * Compare value string does not contain a known value. 322 */ 323 NOTCONTAINS, 324 /** 325 * added to help the parsers 326 */ 327 NULL; 328 329 public static AssertionOperatorType fromCode(String codeString) throws FHIRException { 330 if (codeString == null || "".equals(codeString)) 331 return null; 332 if ("equals".equals(codeString)) 333 return EQUALS; 334 if ("notEquals".equals(codeString)) 335 return NOTEQUALS; 336 if ("in".equals(codeString)) 337 return IN; 338 if ("notIn".equals(codeString)) 339 return NOTIN; 340 if ("greaterThan".equals(codeString)) 341 return GREATERTHAN; 342 if ("lessThan".equals(codeString)) 343 return LESSTHAN; 344 if ("empty".equals(codeString)) 345 return EMPTY; 346 if ("notEmpty".equals(codeString)) 347 return NOTEMPTY; 348 if ("contains".equals(codeString)) 349 return CONTAINS; 350 if ("notContains".equals(codeString)) 351 return NOTCONTAINS; 352 throw new FHIRException("Unknown AssertionOperatorType code '" + codeString + "'"); 353 } 354 355 public String toCode() { 356 switch (this) { 357 case EQUALS: 358 return "equals"; 359 case NOTEQUALS: 360 return "notEquals"; 361 case IN: 362 return "in"; 363 case NOTIN: 364 return "notIn"; 365 case GREATERTHAN: 366 return "greaterThan"; 367 case LESSTHAN: 368 return "lessThan"; 369 case EMPTY: 370 return "empty"; 371 case NOTEMPTY: 372 return "notEmpty"; 373 case CONTAINS: 374 return "contains"; 375 case NOTCONTAINS: 376 return "notContains"; 377 case NULL: 378 return null; 379 default: 380 return "?"; 381 } 382 } 383 384 public String getSystem() { 385 switch (this) { 386 case EQUALS: 387 return "http://hl7.org/fhir/assert-operator-codes"; 388 case NOTEQUALS: 389 return "http://hl7.org/fhir/assert-operator-codes"; 390 case IN: 391 return "http://hl7.org/fhir/assert-operator-codes"; 392 case NOTIN: 393 return "http://hl7.org/fhir/assert-operator-codes"; 394 case GREATERTHAN: 395 return "http://hl7.org/fhir/assert-operator-codes"; 396 case LESSTHAN: 397 return "http://hl7.org/fhir/assert-operator-codes"; 398 case EMPTY: 399 return "http://hl7.org/fhir/assert-operator-codes"; 400 case NOTEMPTY: 401 return "http://hl7.org/fhir/assert-operator-codes"; 402 case CONTAINS: 403 return "http://hl7.org/fhir/assert-operator-codes"; 404 case NOTCONTAINS: 405 return "http://hl7.org/fhir/assert-operator-codes"; 406 case NULL: 407 return null; 408 default: 409 return "?"; 410 } 411 } 412 413 public String getDefinition() { 414 switch (this) { 415 case EQUALS: 416 return "Default value. Equals comparison."; 417 case NOTEQUALS: 418 return "Not equals comparison."; 419 case IN: 420 return "Compare value within a known set of values."; 421 case NOTIN: 422 return "Compare value not within a known set of values."; 423 case GREATERTHAN: 424 return "Compare value to be greater than a known value."; 425 case LESSTHAN: 426 return "Compare value to be less than a known value."; 427 case EMPTY: 428 return "Compare value is empty."; 429 case NOTEMPTY: 430 return "Compare value is not empty."; 431 case CONTAINS: 432 return "Compare value string contains a known value."; 433 case NOTCONTAINS: 434 return "Compare value string does not contain a known value."; 435 case NULL: 436 return null; 437 default: 438 return "?"; 439 } 440 } 441 442 public String getDisplay() { 443 switch (this) { 444 case EQUALS: 445 return "equals"; 446 case NOTEQUALS: 447 return "notEquals"; 448 case IN: 449 return "in"; 450 case NOTIN: 451 return "notIn"; 452 case GREATERTHAN: 453 return "greaterThan"; 454 case LESSTHAN: 455 return "lessThan"; 456 case EMPTY: 457 return "empty"; 458 case NOTEMPTY: 459 return "notEmpty"; 460 case CONTAINS: 461 return "contains"; 462 case NOTCONTAINS: 463 return "notContains"; 464 case NULL: 465 return null; 466 default: 467 return "?"; 468 } 469 } 470 } 471 472 public static class AssertionOperatorTypeEnumFactory implements EnumFactory<AssertionOperatorType> { 473 public AssertionOperatorType fromCode(String codeString) throws IllegalArgumentException { 474 if (codeString == null || "".equals(codeString)) 475 if (codeString == null || "".equals(codeString)) 476 return null; 477 if ("equals".equals(codeString)) 478 return AssertionOperatorType.EQUALS; 479 if ("notEquals".equals(codeString)) 480 return AssertionOperatorType.NOTEQUALS; 481 if ("in".equals(codeString)) 482 return AssertionOperatorType.IN; 483 if ("notIn".equals(codeString)) 484 return AssertionOperatorType.NOTIN; 485 if ("greaterThan".equals(codeString)) 486 return AssertionOperatorType.GREATERTHAN; 487 if ("lessThan".equals(codeString)) 488 return AssertionOperatorType.LESSTHAN; 489 if ("empty".equals(codeString)) 490 return AssertionOperatorType.EMPTY; 491 if ("notEmpty".equals(codeString)) 492 return AssertionOperatorType.NOTEMPTY; 493 if ("contains".equals(codeString)) 494 return AssertionOperatorType.CONTAINS; 495 if ("notContains".equals(codeString)) 496 return AssertionOperatorType.NOTCONTAINS; 497 throw new IllegalArgumentException("Unknown AssertionOperatorType code '" + codeString + "'"); 498 } 499 500 public Enumeration<AssertionOperatorType> fromType(Base code) throws FHIRException { 501 if (code == null || code.isEmpty()) 502 return null; 503 String codeString = ((PrimitiveType) code).asStringValue(); 504 if (codeString == null || "".equals(codeString)) 505 return null; 506 if ("equals".equals(codeString)) 507 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EQUALS); 508 if ("notEquals".equals(codeString)) 509 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEQUALS); 510 if ("in".equals(codeString)) 511 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.IN); 512 if ("notIn".equals(codeString)) 513 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTIN); 514 if ("greaterThan".equals(codeString)) 515 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.GREATERTHAN); 516 if ("lessThan".equals(codeString)) 517 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.LESSTHAN); 518 if ("empty".equals(codeString)) 519 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EMPTY); 520 if ("notEmpty".equals(codeString)) 521 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEMPTY); 522 if ("contains".equals(codeString)) 523 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.CONTAINS); 524 if ("notContains".equals(codeString)) 525 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTCONTAINS); 526 throw new FHIRException("Unknown AssertionOperatorType code '" + codeString + "'"); 527 } 528 529 public String toCode(AssertionOperatorType code) 530 { 531 if (code == AssertionOperatorType.NULL) 532 return null; 533 if (code == AssertionOperatorType.EQUALS) 534 return "equals"; 535 if (code == AssertionOperatorType.NOTEQUALS) 536 return "notEquals"; 537 if (code == AssertionOperatorType.IN) 538 return "in"; 539 if (code == AssertionOperatorType.NOTIN) 540 return "notIn"; 541 if (code == AssertionOperatorType.GREATERTHAN) 542 return "greaterThan"; 543 if (code == AssertionOperatorType.LESSTHAN) 544 return "lessThan"; 545 if (code == AssertionOperatorType.EMPTY) 546 return "empty"; 547 if (code == AssertionOperatorType.NOTEMPTY) 548 return "notEmpty"; 549 if (code == AssertionOperatorType.CONTAINS) 550 return "contains"; 551 if (code == AssertionOperatorType.NOTCONTAINS) 552 return "notContains"; 553 return "?"; 554 } 555 } 556 557 public enum AssertionResponseTypes { 558 /** 559 * Response code is 200. 560 */ 561 OKAY, 562 /** 563 * Response code is 201. 564 */ 565 CREATED, 566 /** 567 * Response code is 204. 568 */ 569 NOCONTENT, 570 /** 571 * Response code is 304. 572 */ 573 NOTMODIFIED, 574 /** 575 * Response code is 400. 576 */ 577 BAD, 578 /** 579 * Response code is 403. 580 */ 581 FORBIDDEN, 582 /** 583 * Response code is 404. 584 */ 585 NOTFOUND, 586 /** 587 * Response code is 405. 588 */ 589 METHODNOTALLOWED, 590 /** 591 * Response code is 409. 592 */ 593 CONFLICT, 594 /** 595 * Response code is 410. 596 */ 597 GONE, 598 /** 599 * Response code is 412. 600 */ 601 PRECONDITIONFAILED, 602 /** 603 * Response code is 422. 604 */ 605 UNPROCESSABLE, 606 /** 607 * added to help the parsers 608 */ 609 NULL; 610 611 public static AssertionResponseTypes fromCode(String codeString) throws FHIRException { 612 if (codeString == null || "".equals(codeString)) 613 return null; 614 if ("okay".equals(codeString)) 615 return OKAY; 616 if ("created".equals(codeString)) 617 return CREATED; 618 if ("noContent".equals(codeString)) 619 return NOCONTENT; 620 if ("notModified".equals(codeString)) 621 return NOTMODIFIED; 622 if ("bad".equals(codeString)) 623 return BAD; 624 if ("forbidden".equals(codeString)) 625 return FORBIDDEN; 626 if ("notFound".equals(codeString)) 627 return NOTFOUND; 628 if ("methodNotAllowed".equals(codeString)) 629 return METHODNOTALLOWED; 630 if ("conflict".equals(codeString)) 631 return CONFLICT; 632 if ("gone".equals(codeString)) 633 return GONE; 634 if ("preconditionFailed".equals(codeString)) 635 return PRECONDITIONFAILED; 636 if ("unprocessable".equals(codeString)) 637 return UNPROCESSABLE; 638 throw new FHIRException("Unknown AssertionResponseTypes code '" + codeString + "'"); 639 } 640 641 public String toCode() { 642 switch (this) { 643 case OKAY: 644 return "okay"; 645 case CREATED: 646 return "created"; 647 case NOCONTENT: 648 return "noContent"; 649 case NOTMODIFIED: 650 return "notModified"; 651 case BAD: 652 return "bad"; 653 case FORBIDDEN: 654 return "forbidden"; 655 case NOTFOUND: 656 return "notFound"; 657 case METHODNOTALLOWED: 658 return "methodNotAllowed"; 659 case CONFLICT: 660 return "conflict"; 661 case GONE: 662 return "gone"; 663 case PRECONDITIONFAILED: 664 return "preconditionFailed"; 665 case UNPROCESSABLE: 666 return "unprocessable"; 667 case NULL: 668 return null; 669 default: 670 return "?"; 671 } 672 } 673 674 public String getSystem() { 675 switch (this) { 676 case OKAY: 677 return "http://hl7.org/fhir/assert-response-code-types"; 678 case CREATED: 679 return "http://hl7.org/fhir/assert-response-code-types"; 680 case NOCONTENT: 681 return "http://hl7.org/fhir/assert-response-code-types"; 682 case NOTMODIFIED: 683 return "http://hl7.org/fhir/assert-response-code-types"; 684 case BAD: 685 return "http://hl7.org/fhir/assert-response-code-types"; 686 case FORBIDDEN: 687 return "http://hl7.org/fhir/assert-response-code-types"; 688 case NOTFOUND: 689 return "http://hl7.org/fhir/assert-response-code-types"; 690 case METHODNOTALLOWED: 691 return "http://hl7.org/fhir/assert-response-code-types"; 692 case CONFLICT: 693 return "http://hl7.org/fhir/assert-response-code-types"; 694 case GONE: 695 return "http://hl7.org/fhir/assert-response-code-types"; 696 case PRECONDITIONFAILED: 697 return "http://hl7.org/fhir/assert-response-code-types"; 698 case UNPROCESSABLE: 699 return "http://hl7.org/fhir/assert-response-code-types"; 700 case NULL: 701 return null; 702 default: 703 return "?"; 704 } 705 } 706 707 public String getDefinition() { 708 switch (this) { 709 case OKAY: 710 return "Response code is 200."; 711 case CREATED: 712 return "Response code is 201."; 713 case NOCONTENT: 714 return "Response code is 204."; 715 case NOTMODIFIED: 716 return "Response code is 304."; 717 case BAD: 718 return "Response code is 400."; 719 case FORBIDDEN: 720 return "Response code is 403."; 721 case NOTFOUND: 722 return "Response code is 404."; 723 case METHODNOTALLOWED: 724 return "Response code is 405."; 725 case CONFLICT: 726 return "Response code is 409."; 727 case GONE: 728 return "Response code is 410."; 729 case PRECONDITIONFAILED: 730 return "Response code is 412."; 731 case UNPROCESSABLE: 732 return "Response code is 422."; 733 case NULL: 734 return null; 735 default: 736 return "?"; 737 } 738 } 739 740 public String getDisplay() { 741 switch (this) { 742 case OKAY: 743 return "okay"; 744 case CREATED: 745 return "created"; 746 case NOCONTENT: 747 return "noContent"; 748 case NOTMODIFIED: 749 return "notModified"; 750 case BAD: 751 return "bad"; 752 case FORBIDDEN: 753 return "forbidden"; 754 case NOTFOUND: 755 return "notFound"; 756 case METHODNOTALLOWED: 757 return "methodNotAllowed"; 758 case CONFLICT: 759 return "conflict"; 760 case GONE: 761 return "gone"; 762 case PRECONDITIONFAILED: 763 return "preconditionFailed"; 764 case UNPROCESSABLE: 765 return "unprocessable"; 766 case NULL: 767 return null; 768 default: 769 return "?"; 770 } 771 } 772 } 773 774 public static class AssertionResponseTypesEnumFactory implements EnumFactory<AssertionResponseTypes> { 775 public AssertionResponseTypes fromCode(String codeString) throws IllegalArgumentException { 776 if (codeString == null || "".equals(codeString)) 777 if (codeString == null || "".equals(codeString)) 778 return null; 779 if ("okay".equals(codeString)) 780 return AssertionResponseTypes.OKAY; 781 if ("created".equals(codeString)) 782 return AssertionResponseTypes.CREATED; 783 if ("noContent".equals(codeString)) 784 return AssertionResponseTypes.NOCONTENT; 785 if ("notModified".equals(codeString)) 786 return AssertionResponseTypes.NOTMODIFIED; 787 if ("bad".equals(codeString)) 788 return AssertionResponseTypes.BAD; 789 if ("forbidden".equals(codeString)) 790 return AssertionResponseTypes.FORBIDDEN; 791 if ("notFound".equals(codeString)) 792 return AssertionResponseTypes.NOTFOUND; 793 if ("methodNotAllowed".equals(codeString)) 794 return AssertionResponseTypes.METHODNOTALLOWED; 795 if ("conflict".equals(codeString)) 796 return AssertionResponseTypes.CONFLICT; 797 if ("gone".equals(codeString)) 798 return AssertionResponseTypes.GONE; 799 if ("preconditionFailed".equals(codeString)) 800 return AssertionResponseTypes.PRECONDITIONFAILED; 801 if ("unprocessable".equals(codeString)) 802 return AssertionResponseTypes.UNPROCESSABLE; 803 throw new IllegalArgumentException("Unknown AssertionResponseTypes code '" + codeString + "'"); 804 } 805 806 public Enumeration<AssertionResponseTypes> fromType(Base code) throws FHIRException { 807 if (code == null || code.isEmpty()) 808 return null; 809 String codeString = ((PrimitiveType) code).asStringValue(); 810 if (codeString == null || "".equals(codeString)) 811 return null; 812 if ("okay".equals(codeString)) 813 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.OKAY); 814 if ("created".equals(codeString)) 815 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CREATED); 816 if ("noContent".equals(codeString)) 817 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOCONTENT); 818 if ("notModified".equals(codeString)) 819 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTMODIFIED); 820 if ("bad".equals(codeString)) 821 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.BAD); 822 if ("forbidden".equals(codeString)) 823 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.FORBIDDEN); 824 if ("notFound".equals(codeString)) 825 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTFOUND); 826 if ("methodNotAllowed".equals(codeString)) 827 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.METHODNOTALLOWED); 828 if ("conflict".equals(codeString)) 829 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CONFLICT); 830 if ("gone".equals(codeString)) 831 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.GONE); 832 if ("preconditionFailed".equals(codeString)) 833 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PRECONDITIONFAILED); 834 if ("unprocessable".equals(codeString)) 835 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UNPROCESSABLE); 836 throw new FHIRException("Unknown AssertionResponseTypes code '" + codeString + "'"); 837 } 838 839 public String toCode(AssertionResponseTypes code) 840 { 841 if (code == AssertionResponseTypes.NULL) 842 return null; 843 if (code == AssertionResponseTypes.OKAY) 844 return "okay"; 845 if (code == AssertionResponseTypes.CREATED) 846 return "created"; 847 if (code == AssertionResponseTypes.NOCONTENT) 848 return "noContent"; 849 if (code == AssertionResponseTypes.NOTMODIFIED) 850 return "notModified"; 851 if (code == AssertionResponseTypes.BAD) 852 return "bad"; 853 if (code == AssertionResponseTypes.FORBIDDEN) 854 return "forbidden"; 855 if (code == AssertionResponseTypes.NOTFOUND) 856 return "notFound"; 857 if (code == AssertionResponseTypes.METHODNOTALLOWED) 858 return "methodNotAllowed"; 859 if (code == AssertionResponseTypes.CONFLICT) 860 return "conflict"; 861 if (code == AssertionResponseTypes.GONE) 862 return "gone"; 863 if (code == AssertionResponseTypes.PRECONDITIONFAILED) 864 return "preconditionFailed"; 865 if (code == AssertionResponseTypes.UNPROCESSABLE) 866 return "unprocessable"; 867 return "?"; 868 } 869 } 870 871 @Block() 872 public static class TestScriptContactComponent extends BackboneElement implements IBaseBackboneElement { 873 /** 874 * The name of an individual to contact regarding the Test Script. 875 */ 876 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 877 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the Test Script.") 878 protected StringType name; 879 880 /** 881 * Contact details for individual (if a name was provided) or the publisher. 882 */ 883 @Child(name = "telecom", type = { 884 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 885 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 886 protected List<ContactPoint> telecom; 887 888 private static final long serialVersionUID = -1179697803L; 889 890 /* 891 * Constructor 892 */ 893 public TestScriptContactComponent() { 894 super(); 895 } 896 897 /** 898 * @return {@link #name} (The name of an individual to contact regarding the 899 * Test Script.). This is the underlying object with id, value and 900 * extensions. The accessor "getName" gives direct access to the value 901 */ 902 public StringType getNameElement() { 903 if (this.name == null) 904 if (Configuration.errorOnAutoCreate()) 905 throw new Error("Attempt to auto-create TestScriptContactComponent.name"); 906 else if (Configuration.doAutoCreate()) 907 this.name = new StringType(); // bb 908 return this.name; 909 } 910 911 public boolean hasNameElement() { 912 return this.name != null && !this.name.isEmpty(); 913 } 914 915 public boolean hasName() { 916 return this.name != null && !this.name.isEmpty(); 917 } 918 919 /** 920 * @param value {@link #name} (The name of an individual to contact regarding 921 * the Test Script.). This is the underlying object with id, value 922 * and extensions. The accessor "getName" gives direct access to 923 * the value 924 */ 925 public TestScriptContactComponent setNameElement(StringType value) { 926 this.name = value; 927 return this; 928 } 929 930 /** 931 * @return The name of an individual to contact regarding the Test Script. 932 */ 933 public String getName() { 934 return this.name == null ? null : this.name.getValue(); 935 } 936 937 /** 938 * @param value The name of an individual to contact regarding the Test Script. 939 */ 940 public TestScriptContactComponent setName(String value) { 941 if (Utilities.noString(value)) 942 this.name = null; 943 else { 944 if (this.name == null) 945 this.name = new StringType(); 946 this.name.setValue(value); 947 } 948 return this; 949 } 950 951 /** 952 * @return {@link #telecom} (Contact details for individual (if a name was 953 * provided) or the publisher.) 954 */ 955 public List<ContactPoint> getTelecom() { 956 if (this.telecom == null) 957 this.telecom = new ArrayList<ContactPoint>(); 958 return this.telecom; 959 } 960 961 public boolean hasTelecom() { 962 if (this.telecom == null) 963 return false; 964 for (ContactPoint item : this.telecom) 965 if (!item.isEmpty()) 966 return true; 967 return false; 968 } 969 970 /** 971 * @return {@link #telecom} (Contact details for individual (if a name was 972 * provided) or the publisher.) 973 */ 974 // syntactic sugar 975 public ContactPoint addTelecom() { // 3 976 ContactPoint t = new ContactPoint(); 977 if (this.telecom == null) 978 this.telecom = new ArrayList<ContactPoint>(); 979 this.telecom.add(t); 980 return t; 981 } 982 983 // syntactic sugar 984 public TestScriptContactComponent addTelecom(ContactPoint t) { // 3 985 if (t == null) 986 return this; 987 if (this.telecom == null) 988 this.telecom = new ArrayList<ContactPoint>(); 989 this.telecom.add(t); 990 return this; 991 } 992 993 protected void listChildren(List<Property> childrenList) { 994 super.listChildren(childrenList); 995 childrenList.add(new Property("name", "string", "The name of an individual to contact regarding the Test Script.", 996 0, java.lang.Integer.MAX_VALUE, name)); 997 childrenList.add(new Property("telecom", "ContactPoint", 998 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 999 telecom)); 1000 } 1001 1002 @Override 1003 public void setProperty(String name, Base value) throws FHIRException { 1004 if (name.equals("name")) 1005 this.name = castToString(value); // StringType 1006 else if (name.equals("telecom")) 1007 this.getTelecom().add(castToContactPoint(value)); 1008 else 1009 super.setProperty(name, value); 1010 } 1011 1012 @Override 1013 public Base addChild(String name) throws FHIRException { 1014 if (name.equals("name")) { 1015 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 1016 } else if (name.equals("telecom")) { 1017 return addTelecom(); 1018 } else 1019 return super.addChild(name); 1020 } 1021 1022 public TestScriptContactComponent copy() { 1023 TestScriptContactComponent dst = new TestScriptContactComponent(); 1024 copyValues(dst); 1025 dst.name = name == null ? null : name.copy(); 1026 if (telecom != null) { 1027 dst.telecom = new ArrayList<ContactPoint>(); 1028 for (ContactPoint i : telecom) 1029 dst.telecom.add(i.copy()); 1030 } 1031 ; 1032 return dst; 1033 } 1034 1035 @Override 1036 public boolean equalsDeep(Base other) { 1037 if (!super.equalsDeep(other)) 1038 return false; 1039 if (!(other instanceof TestScriptContactComponent)) 1040 return false; 1041 TestScriptContactComponent o = (TestScriptContactComponent) other; 1042 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 1043 } 1044 1045 @Override 1046 public boolean equalsShallow(Base other) { 1047 if (!super.equalsShallow(other)) 1048 return false; 1049 if (!(other instanceof TestScriptContactComponent)) 1050 return false; 1051 TestScriptContactComponent o = (TestScriptContactComponent) other; 1052 return compareValues(name, o.name, true); 1053 } 1054 1055 public boolean isEmpty() { 1056 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 1057 } 1058 1059 public String fhirType() { 1060 return "TestScript.contact"; 1061 1062 } 1063 1064 } 1065 1066 @Block() 1067 public static class TestScriptMetadataComponent extends BackboneElement implements IBaseBackboneElement { 1068 /** 1069 * A link to the FHIR specification that this test is covering. 1070 */ 1071 @Child(name = "link", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1072 @Description(shortDefinition = "Links to the FHIR specification", formalDefinition = "A link to the FHIR specification that this test is covering.") 1073 protected List<TestScriptMetadataLinkComponent> link; 1074 1075 /** 1076 * Capabilities that must exist and are assumed to function correctly on the 1077 * FHIR server being tested. 1078 */ 1079 @Child(name = "capability", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1080 @Description(shortDefinition = "Capabilities that are assumed to function correctly on the FHIR server being tested", formalDefinition = "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.") 1081 protected List<TestScriptMetadataCapabilityComponent> capability; 1082 1083 private static final long serialVersionUID = 745183328L; 1084 1085 /* 1086 * Constructor 1087 */ 1088 public TestScriptMetadataComponent() { 1089 super(); 1090 } 1091 1092 /** 1093 * @return {@link #link} (A link to the FHIR specification that this test is 1094 * covering.) 1095 */ 1096 public List<TestScriptMetadataLinkComponent> getLink() { 1097 if (this.link == null) 1098 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1099 return this.link; 1100 } 1101 1102 public boolean hasLink() { 1103 if (this.link == null) 1104 return false; 1105 for (TestScriptMetadataLinkComponent item : this.link) 1106 if (!item.isEmpty()) 1107 return true; 1108 return false; 1109 } 1110 1111 /** 1112 * @return {@link #link} (A link to the FHIR specification that this test is 1113 * covering.) 1114 */ 1115 // syntactic sugar 1116 public TestScriptMetadataLinkComponent addLink() { // 3 1117 TestScriptMetadataLinkComponent t = new TestScriptMetadataLinkComponent(); 1118 if (this.link == null) 1119 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1120 this.link.add(t); 1121 return t; 1122 } 1123 1124 // syntactic sugar 1125 public TestScriptMetadataComponent addLink(TestScriptMetadataLinkComponent t) { // 3 1126 if (t == null) 1127 return this; 1128 if (this.link == null) 1129 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1130 this.link.add(t); 1131 return this; 1132 } 1133 1134 /** 1135 * @return {@link #capability} (Capabilities that must exist and are assumed to 1136 * function correctly on the FHIR server being tested.) 1137 */ 1138 public List<TestScriptMetadataCapabilityComponent> getCapability() { 1139 if (this.capability == null) 1140 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1141 return this.capability; 1142 } 1143 1144 public boolean hasCapability() { 1145 if (this.capability == null) 1146 return false; 1147 for (TestScriptMetadataCapabilityComponent item : this.capability) 1148 if (!item.isEmpty()) 1149 return true; 1150 return false; 1151 } 1152 1153 /** 1154 * @return {@link #capability} (Capabilities that must exist and are assumed to 1155 * function correctly on the FHIR server being tested.) 1156 */ 1157 // syntactic sugar 1158 public TestScriptMetadataCapabilityComponent addCapability() { // 3 1159 TestScriptMetadataCapabilityComponent t = new TestScriptMetadataCapabilityComponent(); 1160 if (this.capability == null) 1161 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1162 this.capability.add(t); 1163 return t; 1164 } 1165 1166 // syntactic sugar 1167 public TestScriptMetadataComponent addCapability(TestScriptMetadataCapabilityComponent t) { // 3 1168 if (t == null) 1169 return this; 1170 if (this.capability == null) 1171 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1172 this.capability.add(t); 1173 return this; 1174 } 1175 1176 protected void listChildren(List<Property> childrenList) { 1177 super.listChildren(childrenList); 1178 childrenList.add(new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, 1179 java.lang.Integer.MAX_VALUE, link)); 1180 childrenList.add(new Property("capability", "", 1181 "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1182 java.lang.Integer.MAX_VALUE, capability)); 1183 } 1184 1185 @Override 1186 public void setProperty(String name, Base value) throws FHIRException { 1187 if (name.equals("link")) 1188 this.getLink().add((TestScriptMetadataLinkComponent) value); 1189 else if (name.equals("capability")) 1190 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); 1191 else 1192 super.setProperty(name, value); 1193 } 1194 1195 @Override 1196 public Base addChild(String name) throws FHIRException { 1197 if (name.equals("link")) { 1198 return addLink(); 1199 } else if (name.equals("capability")) { 1200 return addCapability(); 1201 } else 1202 return super.addChild(name); 1203 } 1204 1205 public TestScriptMetadataComponent copy() { 1206 TestScriptMetadataComponent dst = new TestScriptMetadataComponent(); 1207 copyValues(dst); 1208 if (link != null) { 1209 dst.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1210 for (TestScriptMetadataLinkComponent i : link) 1211 dst.link.add(i.copy()); 1212 } 1213 ; 1214 if (capability != null) { 1215 dst.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1216 for (TestScriptMetadataCapabilityComponent i : capability) 1217 dst.capability.add(i.copy()); 1218 } 1219 ; 1220 return dst; 1221 } 1222 1223 @Override 1224 public boolean equalsDeep(Base other) { 1225 if (!super.equalsDeep(other)) 1226 return false; 1227 if (!(other instanceof TestScriptMetadataComponent)) 1228 return false; 1229 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other; 1230 return compareDeep(link, o.link, true) && compareDeep(capability, o.capability, true); 1231 } 1232 1233 @Override 1234 public boolean equalsShallow(Base other) { 1235 if (!super.equalsShallow(other)) 1236 return false; 1237 if (!(other instanceof TestScriptMetadataComponent)) 1238 return false; 1239 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other; 1240 return true; 1241 } 1242 1243 public boolean isEmpty() { 1244 return super.isEmpty() && (link == null || link.isEmpty()) && (capability == null || capability.isEmpty()); 1245 } 1246 1247 public String fhirType() { 1248 return "TestScript.metadata"; 1249 1250 } 1251 1252 } 1253 1254 @Block() 1255 public static class TestScriptMetadataLinkComponent extends BackboneElement implements IBaseBackboneElement { 1256 /** 1257 * URL to a particular requirement or feature within the FHIR specification. 1258 */ 1259 @Child(name = "url", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1260 @Description(shortDefinition = "URL to the specification", formalDefinition = "URL to a particular requirement or feature within the FHIR specification.") 1261 protected UriType url; 1262 1263 /** 1264 * Short description of the link. 1265 */ 1266 @Child(name = "description", type = { 1267 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1268 @Description(shortDefinition = "Short description", formalDefinition = "Short description of the link.") 1269 protected StringType description; 1270 1271 private static final long serialVersionUID = 213372298L; 1272 1273 /* 1274 * Constructor 1275 */ 1276 public TestScriptMetadataLinkComponent() { 1277 super(); 1278 } 1279 1280 /* 1281 * Constructor 1282 */ 1283 public TestScriptMetadataLinkComponent(UriType url) { 1284 super(); 1285 this.url = url; 1286 } 1287 1288 /** 1289 * @return {@link #url} (URL to a particular requirement or feature within the 1290 * FHIR specification.). This is the underlying object with id, value 1291 * and extensions. The accessor "getUrl" gives direct access to the 1292 * value 1293 */ 1294 public UriType getUrlElement() { 1295 if (this.url == null) 1296 if (Configuration.errorOnAutoCreate()) 1297 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.url"); 1298 else if (Configuration.doAutoCreate()) 1299 this.url = new UriType(); // bb 1300 return this.url; 1301 } 1302 1303 public boolean hasUrlElement() { 1304 return this.url != null && !this.url.isEmpty(); 1305 } 1306 1307 public boolean hasUrl() { 1308 return this.url != null && !this.url.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #url} (URL to a particular requirement or feature within 1313 * the FHIR specification.). This is the underlying object with id, 1314 * value and extensions. The accessor "getUrl" gives direct access 1315 * to the value 1316 */ 1317 public TestScriptMetadataLinkComponent setUrlElement(UriType value) { 1318 this.url = value; 1319 return this; 1320 } 1321 1322 /** 1323 * @return URL to a particular requirement or feature within the FHIR 1324 * specification. 1325 */ 1326 public String getUrl() { 1327 return this.url == null ? null : this.url.getValue(); 1328 } 1329 1330 /** 1331 * @param value URL to a particular requirement or feature within the FHIR 1332 * specification. 1333 */ 1334 public TestScriptMetadataLinkComponent setUrl(String value) { 1335 if (this.url == null) 1336 this.url = new UriType(); 1337 this.url.setValue(value); 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #description} (Short description of the link.). This is the 1343 * underlying object with id, value and extensions. The accessor 1344 * "getDescription" gives direct access to the value 1345 */ 1346 public StringType getDescriptionElement() { 1347 if (this.description == null) 1348 if (Configuration.errorOnAutoCreate()) 1349 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.description"); 1350 else if (Configuration.doAutoCreate()) 1351 this.description = new StringType(); // bb 1352 return this.description; 1353 } 1354 1355 public boolean hasDescriptionElement() { 1356 return this.description != null && !this.description.isEmpty(); 1357 } 1358 1359 public boolean hasDescription() { 1360 return this.description != null && !this.description.isEmpty(); 1361 } 1362 1363 /** 1364 * @param value {@link #description} (Short description of the link.). This is 1365 * the underlying object with id, value and extensions. The 1366 * accessor "getDescription" gives direct access to the value 1367 */ 1368 public TestScriptMetadataLinkComponent setDescriptionElement(StringType value) { 1369 this.description = value; 1370 return this; 1371 } 1372 1373 /** 1374 * @return Short description of the link. 1375 */ 1376 public String getDescription() { 1377 return this.description == null ? null : this.description.getValue(); 1378 } 1379 1380 /** 1381 * @param value Short description of the link. 1382 */ 1383 public TestScriptMetadataLinkComponent setDescription(String value) { 1384 if (Utilities.noString(value)) 1385 this.description = null; 1386 else { 1387 if (this.description == null) 1388 this.description = new StringType(); 1389 this.description.setValue(value); 1390 } 1391 return this; 1392 } 1393 1394 protected void listChildren(List<Property> childrenList) { 1395 super.listChildren(childrenList); 1396 childrenList 1397 .add(new Property("url", "uri", "URL to a particular requirement or feature within the FHIR specification.", 1398 0, java.lang.Integer.MAX_VALUE, url)); 1399 childrenList.add(new Property("description", "string", "Short description of the link.", 0, 1400 java.lang.Integer.MAX_VALUE, description)); 1401 } 1402 1403 @Override 1404 public void setProperty(String name, Base value) throws FHIRException { 1405 if (name.equals("url")) 1406 this.url = castToUri(value); // UriType 1407 else if (name.equals("description")) 1408 this.description = castToString(value); // StringType 1409 else 1410 super.setProperty(name, value); 1411 } 1412 1413 @Override 1414 public Base addChild(String name) throws FHIRException { 1415 if (name.equals("url")) { 1416 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 1417 } else if (name.equals("description")) { 1418 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 1419 } else 1420 return super.addChild(name); 1421 } 1422 1423 public TestScriptMetadataLinkComponent copy() { 1424 TestScriptMetadataLinkComponent dst = new TestScriptMetadataLinkComponent(); 1425 copyValues(dst); 1426 dst.url = url == null ? null : url.copy(); 1427 dst.description = description == null ? null : description.copy(); 1428 return dst; 1429 } 1430 1431 @Override 1432 public boolean equalsDeep(Base other) { 1433 if (!super.equalsDeep(other)) 1434 return false; 1435 if (!(other instanceof TestScriptMetadataLinkComponent)) 1436 return false; 1437 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other; 1438 return compareDeep(url, o.url, true) && compareDeep(description, o.description, true); 1439 } 1440 1441 @Override 1442 public boolean equalsShallow(Base other) { 1443 if (!super.equalsShallow(other)) 1444 return false; 1445 if (!(other instanceof TestScriptMetadataLinkComponent)) 1446 return false; 1447 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other; 1448 return compareValues(url, o.url, true) && compareValues(description, o.description, true); 1449 } 1450 1451 public boolean isEmpty() { 1452 return super.isEmpty() && (url == null || url.isEmpty()) && (description == null || description.isEmpty()); 1453 } 1454 1455 public String fhirType() { 1456 return "TestScript.metadata.link"; 1457 1458 } 1459 1460 } 1461 1462 @Block() 1463 public static class TestScriptMetadataCapabilityComponent extends BackboneElement implements IBaseBackboneElement { 1464 /** 1465 * Whether or not the test execution will require the given capabilities of the 1466 * server in order for this test script to execute. 1467 */ 1468 @Child(name = "required", type = { 1469 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1470 @Description(shortDefinition = "Are the capabilities required?", formalDefinition = "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.") 1471 protected BooleanType required; 1472 1473 /** 1474 * Whether or not the test execution will validate the given capabilities of the 1475 * server in order for this test script to execute. 1476 */ 1477 @Child(name = "validated", type = { 1478 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1479 @Description(shortDefinition = "Are the capabilities validated?", formalDefinition = "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.") 1480 protected BooleanType validated; 1481 1482 /** 1483 * Description of the capabilities that this test script is requiring the server 1484 * to support. 1485 */ 1486 @Child(name = "description", type = { 1487 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1488 @Description(shortDefinition = "The expected capabilities of the server", formalDefinition = "Description of the capabilities that this test script is requiring the server to support.") 1489 protected StringType description; 1490 1491 /** 1492 * Which server these requirements apply to. 1493 */ 1494 @Child(name = "destination", type = { 1495 IntegerType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1496 @Description(shortDefinition = "Which server these requirements apply to", formalDefinition = "Which server these requirements apply to.") 1497 protected IntegerType destination; 1498 1499 /** 1500 * Links to the FHIR specification that describes this interaction and the 1501 * resources involved in more detail. 1502 */ 1503 @Child(name = "link", type = { 1504 UriType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1505 @Description(shortDefinition = "Links to the FHIR specification", formalDefinition = "Links to the FHIR specification that describes this interaction and the resources involved in more detail.") 1506 protected List<UriType> link; 1507 1508 /** 1509 * Minimum conformance required of server for test script to execute 1510 * successfully. If server does not meet at a minimum the reference conformance 1511 * definition, then all tests in this script are skipped. 1512 */ 1513 @Child(name = "conformance", type = { 1514 Conformance.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 1515 @Description(shortDefinition = "Required Conformance", formalDefinition = "Minimum conformance required of server for test script to execute successfully. If server does not meet at a minimum the reference conformance definition, then all tests in this script are skipped.") 1516 protected Reference conformance; 1517 1518 /** 1519 * The actual object that is the target of the reference (Minimum conformance 1520 * required of server for test script to execute successfully. If server does 1521 * not meet at a minimum the reference conformance definition, then all tests in 1522 * this script are skipped.) 1523 */ 1524 protected Conformance conformanceTarget; 1525 1526 private static final long serialVersionUID = 1318523355L; 1527 1528 /* 1529 * Constructor 1530 */ 1531 public TestScriptMetadataCapabilityComponent() { 1532 super(); 1533 } 1534 1535 /* 1536 * Constructor 1537 */ 1538 public TestScriptMetadataCapabilityComponent(Reference conformance) { 1539 super(); 1540 this.conformance = conformance; 1541 } 1542 1543 /** 1544 * @return {@link #required} (Whether or not the test execution will require the 1545 * given capabilities of the server in order for this test script to 1546 * execute.). This is the underlying object with id, value and 1547 * extensions. The accessor "getRequired" gives direct access to the 1548 * value 1549 */ 1550 public BooleanType getRequiredElement() { 1551 if (this.required == null) 1552 if (Configuration.errorOnAutoCreate()) 1553 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.required"); 1554 else if (Configuration.doAutoCreate()) 1555 this.required = new BooleanType(); // bb 1556 return this.required; 1557 } 1558 1559 public boolean hasRequiredElement() { 1560 return this.required != null && !this.required.isEmpty(); 1561 } 1562 1563 public boolean hasRequired() { 1564 return this.required != null && !this.required.isEmpty(); 1565 } 1566 1567 /** 1568 * @param value {@link #required} (Whether or not the test execution will 1569 * require the given capabilities of the server in order for this 1570 * test script to execute.). This is the underlying object with id, 1571 * value and extensions. The accessor "getRequired" gives direct 1572 * access to the value 1573 */ 1574 public TestScriptMetadataCapabilityComponent setRequiredElement(BooleanType value) { 1575 this.required = value; 1576 return this; 1577 } 1578 1579 /** 1580 * @return Whether or not the test execution will require the given capabilities 1581 * of the server in order for this test script to execute. 1582 */ 1583 public boolean getRequired() { 1584 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 1585 } 1586 1587 /** 1588 * @param value Whether or not the test execution will require the given 1589 * capabilities of the server in order for this test script to 1590 * execute. 1591 */ 1592 public TestScriptMetadataCapabilityComponent setRequired(boolean value) { 1593 if (this.required == null) 1594 this.required = new BooleanType(); 1595 this.required.setValue(value); 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #validated} (Whether or not the test execution will validate 1601 * the given capabilities of the server in order for this test script to 1602 * execute.). This is the underlying object with id, value and 1603 * extensions. The accessor "getValidated" gives direct access to the 1604 * value 1605 */ 1606 public BooleanType getValidatedElement() { 1607 if (this.validated == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.validated"); 1610 else if (Configuration.doAutoCreate()) 1611 this.validated = new BooleanType(); // bb 1612 return this.validated; 1613 } 1614 1615 public boolean hasValidatedElement() { 1616 return this.validated != null && !this.validated.isEmpty(); 1617 } 1618 1619 public boolean hasValidated() { 1620 return this.validated != null && !this.validated.isEmpty(); 1621 } 1622 1623 /** 1624 * @param value {@link #validated} (Whether or not the test execution will 1625 * validate the given capabilities of the server in order for this 1626 * test script to execute.). This is the underlying object with id, 1627 * value and extensions. The accessor "getValidated" gives direct 1628 * access to the value 1629 */ 1630 public TestScriptMetadataCapabilityComponent setValidatedElement(BooleanType value) { 1631 this.validated = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return Whether or not the test execution will validate the given 1637 * capabilities of the server in order for this test script to execute. 1638 */ 1639 public boolean getValidated() { 1640 return this.validated == null || this.validated.isEmpty() ? false : this.validated.getValue(); 1641 } 1642 1643 /** 1644 * @param value Whether or not the test execution will validate the given 1645 * capabilities of the server in order for this test script to 1646 * execute. 1647 */ 1648 public TestScriptMetadataCapabilityComponent setValidated(boolean value) { 1649 if (this.validated == null) 1650 this.validated = new BooleanType(); 1651 this.validated.setValue(value); 1652 return this; 1653 } 1654 1655 /** 1656 * @return {@link #description} (Description of the capabilities that this test 1657 * script is requiring the server to support.). This is the underlying 1658 * object with id, value and extensions. The accessor "getDescription" 1659 * gives direct access to the value 1660 */ 1661 public StringType getDescriptionElement() { 1662 if (this.description == null) 1663 if (Configuration.errorOnAutoCreate()) 1664 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.description"); 1665 else if (Configuration.doAutoCreate()) 1666 this.description = new StringType(); // bb 1667 return this.description; 1668 } 1669 1670 public boolean hasDescriptionElement() { 1671 return this.description != null && !this.description.isEmpty(); 1672 } 1673 1674 public boolean hasDescription() { 1675 return this.description != null && !this.description.isEmpty(); 1676 } 1677 1678 /** 1679 * @param value {@link #description} (Description of the capabilities that this 1680 * test script is requiring the server to support.). This is the 1681 * underlying object with id, value and extensions. The accessor 1682 * "getDescription" gives direct access to the value 1683 */ 1684 public TestScriptMetadataCapabilityComponent setDescriptionElement(StringType value) { 1685 this.description = value; 1686 return this; 1687 } 1688 1689 /** 1690 * @return Description of the capabilities that this test script is requiring 1691 * the server to support. 1692 */ 1693 public String getDescription() { 1694 return this.description == null ? null : this.description.getValue(); 1695 } 1696 1697 /** 1698 * @param value Description of the capabilities that this test script is 1699 * requiring the server to support. 1700 */ 1701 public TestScriptMetadataCapabilityComponent setDescription(String value) { 1702 if (Utilities.noString(value)) 1703 this.description = null; 1704 else { 1705 if (this.description == null) 1706 this.description = new StringType(); 1707 this.description.setValue(value); 1708 } 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #destination} (Which server these requirements apply to.). 1714 * This is the underlying object with id, value and extensions. The 1715 * accessor "getDestination" gives direct access to the value 1716 */ 1717 public IntegerType getDestinationElement() { 1718 if (this.destination == null) 1719 if (Configuration.errorOnAutoCreate()) 1720 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.destination"); 1721 else if (Configuration.doAutoCreate()) 1722 this.destination = new IntegerType(); // bb 1723 return this.destination; 1724 } 1725 1726 public boolean hasDestinationElement() { 1727 return this.destination != null && !this.destination.isEmpty(); 1728 } 1729 1730 public boolean hasDestination() { 1731 return this.destination != null && !this.destination.isEmpty(); 1732 } 1733 1734 /** 1735 * @param value {@link #destination} (Which server these requirements apply 1736 * to.). This is the underlying object with id, value and 1737 * extensions. The accessor "getDestination" gives direct access to 1738 * the value 1739 */ 1740 public TestScriptMetadataCapabilityComponent setDestinationElement(IntegerType value) { 1741 this.destination = value; 1742 return this; 1743 } 1744 1745 /** 1746 * @return Which server these requirements apply to. 1747 */ 1748 public int getDestination() { 1749 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 1750 } 1751 1752 /** 1753 * @param value Which server these requirements apply to. 1754 */ 1755 public TestScriptMetadataCapabilityComponent setDestination(int value) { 1756 if (this.destination == null) 1757 this.destination = new IntegerType(); 1758 this.destination.setValue(value); 1759 return this; 1760 } 1761 1762 /** 1763 * @return {@link #link} (Links to the FHIR specification that describes this 1764 * interaction and the resources involved in more detail.) 1765 */ 1766 public List<UriType> getLink() { 1767 if (this.link == null) 1768 this.link = new ArrayList<UriType>(); 1769 return this.link; 1770 } 1771 1772 public boolean hasLink() { 1773 if (this.link == null) 1774 return false; 1775 for (UriType item : this.link) 1776 if (!item.isEmpty()) 1777 return true; 1778 return false; 1779 } 1780 1781 /** 1782 * @return {@link #link} (Links to the FHIR specification that describes this 1783 * interaction and the resources involved in more detail.) 1784 */ 1785 // syntactic sugar 1786 public UriType addLinkElement() {// 2 1787 UriType t = new UriType(); 1788 if (this.link == null) 1789 this.link = new ArrayList<UriType>(); 1790 this.link.add(t); 1791 return t; 1792 } 1793 1794 /** 1795 * @param value {@link #link} (Links to the FHIR specification that describes 1796 * this interaction and the resources involved in more detail.) 1797 */ 1798 public TestScriptMetadataCapabilityComponent addLink(String value) { // 1 1799 UriType t = new UriType(); 1800 t.setValue(value); 1801 if (this.link == null) 1802 this.link = new ArrayList<UriType>(); 1803 this.link.add(t); 1804 return this; 1805 } 1806 1807 /** 1808 * @param value {@link #link} (Links to the FHIR specification that describes 1809 * this interaction and the resources involved in more detail.) 1810 */ 1811 public boolean hasLink(String value) { 1812 if (this.link == null) 1813 return false; 1814 for (UriType v : this.link) 1815 if (v.equals(value)) // uri 1816 return true; 1817 return false; 1818 } 1819 1820 /** 1821 * @return {@link #conformance} (Minimum conformance required of server for test 1822 * script to execute successfully. If server does not meet at a minimum 1823 * the reference conformance definition, then all tests in this script 1824 * are skipped.) 1825 */ 1826 public Reference getConformance() { 1827 if (this.conformance == null) 1828 if (Configuration.errorOnAutoCreate()) 1829 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.conformance"); 1830 else if (Configuration.doAutoCreate()) 1831 this.conformance = new Reference(); // cc 1832 return this.conformance; 1833 } 1834 1835 public boolean hasConformance() { 1836 return this.conformance != null && !this.conformance.isEmpty(); 1837 } 1838 1839 /** 1840 * @param value {@link #conformance} (Minimum conformance required of server for 1841 * test script to execute successfully. If server does not meet at 1842 * a minimum the reference conformance definition, then all tests 1843 * in this script are skipped.) 1844 */ 1845 public TestScriptMetadataCapabilityComponent setConformance(Reference value) { 1846 this.conformance = value; 1847 return this; 1848 } 1849 1850 /** 1851 * @return {@link #conformance} The actual object that is the target of the 1852 * reference. The reference library doesn't populate this, but you can 1853 * use it to hold the resource if you resolve it. (Minimum conformance 1854 * required of server for test script to execute successfully. If server 1855 * does not meet at a minimum the reference conformance definition, then 1856 * all tests in this script are skipped.) 1857 */ 1858 public Conformance getConformanceTarget() { 1859 if (this.conformanceTarget == null) 1860 if (Configuration.errorOnAutoCreate()) 1861 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.conformance"); 1862 else if (Configuration.doAutoCreate()) 1863 this.conformanceTarget = new Conformance(); // aa 1864 return this.conformanceTarget; 1865 } 1866 1867 /** 1868 * @param value {@link #conformance} The actual object that is the target of the 1869 * reference. The reference library doesn't use these, but you can 1870 * use it to hold the resource if you resolve it. (Minimum 1871 * conformance required of server for test script to execute 1872 * successfully. If server does not meet at a minimum the reference 1873 * conformance definition, then all tests in this script are 1874 * skipped.) 1875 */ 1876 public TestScriptMetadataCapabilityComponent setConformanceTarget(Conformance value) { 1877 this.conformanceTarget = value; 1878 return this; 1879 } 1880 1881 protected void listChildren(List<Property> childrenList) { 1882 super.listChildren(childrenList); 1883 childrenList.add(new Property("required", "boolean", 1884 "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 1885 0, java.lang.Integer.MAX_VALUE, required)); 1886 childrenList.add(new Property("validated", "boolean", 1887 "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 1888 0, java.lang.Integer.MAX_VALUE, validated)); 1889 childrenList.add(new Property("description", "string", 1890 "Description of the capabilities that this test script is requiring the server to support.", 0, 1891 java.lang.Integer.MAX_VALUE, description)); 1892 childrenList.add(new Property("destination", "integer", "Which server these requirements apply to.", 0, 1893 java.lang.Integer.MAX_VALUE, destination)); 1894 childrenList.add(new Property("link", "uri", 1895 "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 1896 0, java.lang.Integer.MAX_VALUE, link)); 1897 childrenList.add(new Property("conformance", "Reference(Conformance)", 1898 "Minimum conformance required of server for test script to execute successfully. If server does not meet at a minimum the reference conformance definition, then all tests in this script are skipped.", 1899 0, java.lang.Integer.MAX_VALUE, conformance)); 1900 } 1901 1902 @Override 1903 public void setProperty(String name, Base value) throws FHIRException { 1904 if (name.equals("required")) 1905 this.required = castToBoolean(value); // BooleanType 1906 else if (name.equals("validated")) 1907 this.validated = castToBoolean(value); // BooleanType 1908 else if (name.equals("description")) 1909 this.description = castToString(value); // StringType 1910 else if (name.equals("destination")) 1911 this.destination = castToInteger(value); // IntegerType 1912 else if (name.equals("link")) 1913 this.getLink().add(castToUri(value)); 1914 else if (name.equals("conformance")) 1915 this.conformance = castToReference(value); // Reference 1916 else 1917 super.setProperty(name, value); 1918 } 1919 1920 @Override 1921 public Base addChild(String name) throws FHIRException { 1922 if (name.equals("required")) { 1923 throw new FHIRException("Cannot call addChild on a singleton property TestScript.required"); 1924 } else if (name.equals("validated")) { 1925 throw new FHIRException("Cannot call addChild on a singleton property TestScript.validated"); 1926 } else if (name.equals("description")) { 1927 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 1928 } else if (name.equals("destination")) { 1929 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination"); 1930 } else if (name.equals("link")) { 1931 throw new FHIRException("Cannot call addChild on a singleton property TestScript.link"); 1932 } else if (name.equals("conformance")) { 1933 this.conformance = new Reference(); 1934 return this.conformance; 1935 } else 1936 return super.addChild(name); 1937 } 1938 1939 public TestScriptMetadataCapabilityComponent copy() { 1940 TestScriptMetadataCapabilityComponent dst = new TestScriptMetadataCapabilityComponent(); 1941 copyValues(dst); 1942 dst.required = required == null ? null : required.copy(); 1943 dst.validated = validated == null ? null : validated.copy(); 1944 dst.description = description == null ? null : description.copy(); 1945 dst.destination = destination == null ? null : destination.copy(); 1946 if (link != null) { 1947 dst.link = new ArrayList<UriType>(); 1948 for (UriType i : link) 1949 dst.link.add(i.copy()); 1950 } 1951 ; 1952 dst.conformance = conformance == null ? null : conformance.copy(); 1953 return dst; 1954 } 1955 1956 @Override 1957 public boolean equalsDeep(Base other) { 1958 if (!super.equalsDeep(other)) 1959 return false; 1960 if (!(other instanceof TestScriptMetadataCapabilityComponent)) 1961 return false; 1962 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other; 1963 return compareDeep(required, o.required, true) && compareDeep(validated, o.validated, true) 1964 && compareDeep(description, o.description, true) && compareDeep(destination, o.destination, true) 1965 && compareDeep(link, o.link, true) && compareDeep(conformance, o.conformance, true); 1966 } 1967 1968 @Override 1969 public boolean equalsShallow(Base other) { 1970 if (!super.equalsShallow(other)) 1971 return false; 1972 if (!(other instanceof TestScriptMetadataCapabilityComponent)) 1973 return false; 1974 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other; 1975 return compareValues(required, o.required, true) && compareValues(validated, o.validated, true) 1976 && compareValues(description, o.description, true) && compareValues(destination, o.destination, true) 1977 && compareValues(link, o.link, true); 1978 } 1979 1980 public boolean isEmpty() { 1981 return super.isEmpty() && (required == null || required.isEmpty()) && (validated == null || validated.isEmpty()) 1982 && (description == null || description.isEmpty()) && (destination == null || destination.isEmpty()) 1983 && (link == null || link.isEmpty()) && (conformance == null || conformance.isEmpty()); 1984 } 1985 1986 public String fhirType() { 1987 return "TestScript.metadata.capability"; 1988 1989 } 1990 1991 } 1992 1993 @Block() 1994 public static class TestScriptFixtureComponent extends BackboneElement implements IBaseBackboneElement { 1995 /** 1996 * Whether or not to implicitly create the fixture during setup. If true, the 1997 * fixture is automatically created on each server being tested during setup, 1998 * therefore no create operation is required for this fixture in the 1999 * TestScript.setup section. 2000 */ 2001 @Child(name = "autocreate", type = { 2002 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2003 @Description(shortDefinition = "Whether or not to implicitly create the fixture during setup", formalDefinition = "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.") 2004 protected BooleanType autocreate; 2005 2006 /** 2007 * Whether or not to implicitly delete the fixture during teardown If true, the 2008 * fixture is automatically deleted on each server being tested during teardown, 2009 * therefore no delete operation is required for this fixture in the 2010 * TestScript.teardown section. 2011 */ 2012 @Child(name = "autodelete", type = { 2013 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2014 @Description(shortDefinition = "Whether or not to implicitly delete the fixture during teardown", formalDefinition = "Whether or not to implicitly delete the fixture during teardown If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.") 2015 protected BooleanType autodelete; 2016 2017 /** 2018 * Reference to the resource (containing the contents of the resource needed for 2019 * operations). 2020 */ 2021 @Child(name = "resource", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 2022 @Description(shortDefinition = "Reference of the resource", formalDefinition = "Reference to the resource (containing the contents of the resource needed for operations).") 2023 protected Reference resource; 2024 2025 /** 2026 * The actual object that is the target of the reference (Reference to the 2027 * resource (containing the contents of the resource needed for operations).) 2028 */ 2029 protected Resource resourceTarget; 2030 2031 private static final long serialVersionUID = 1110683307L; 2032 2033 /* 2034 * Constructor 2035 */ 2036 public TestScriptFixtureComponent() { 2037 super(); 2038 } 2039 2040 /** 2041 * @return {@link #autocreate} (Whether or not to implicitly create the fixture 2042 * during setup. If true, the fixture is automatically created on each 2043 * server being tested during setup, therefore no create operation is 2044 * required for this fixture in the TestScript.setup section.). This is 2045 * the underlying object with id, value and extensions. The accessor 2046 * "getAutocreate" gives direct access to the value 2047 */ 2048 public BooleanType getAutocreateElement() { 2049 if (this.autocreate == null) 2050 if (Configuration.errorOnAutoCreate()) 2051 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autocreate"); 2052 else if (Configuration.doAutoCreate()) 2053 this.autocreate = new BooleanType(); // bb 2054 return this.autocreate; 2055 } 2056 2057 public boolean hasAutocreateElement() { 2058 return this.autocreate != null && !this.autocreate.isEmpty(); 2059 } 2060 2061 public boolean hasAutocreate() { 2062 return this.autocreate != null && !this.autocreate.isEmpty(); 2063 } 2064 2065 /** 2066 * @param value {@link #autocreate} (Whether or not to implicitly create the 2067 * fixture during setup. If true, the fixture is automatically 2068 * created on each server being tested during setup, therefore no 2069 * create operation is required for this fixture in the 2070 * TestScript.setup section.). This is the underlying object with 2071 * id, value and extensions. The accessor "getAutocreate" gives 2072 * direct access to the value 2073 */ 2074 public TestScriptFixtureComponent setAutocreateElement(BooleanType value) { 2075 this.autocreate = value; 2076 return this; 2077 } 2078 2079 /** 2080 * @return Whether or not to implicitly create the fixture during setup. If 2081 * true, the fixture is automatically created on each server being 2082 * tested during setup, therefore no create operation is required for 2083 * this fixture in the TestScript.setup section. 2084 */ 2085 public boolean getAutocreate() { 2086 return this.autocreate == null || this.autocreate.isEmpty() ? false : this.autocreate.getValue(); 2087 } 2088 2089 /** 2090 * @param value Whether or not to implicitly create the fixture during setup. If 2091 * true, the fixture is automatically created on each server being 2092 * tested during setup, therefore no create operation is required 2093 * for this fixture in the TestScript.setup section. 2094 */ 2095 public TestScriptFixtureComponent setAutocreate(boolean value) { 2096 if (this.autocreate == null) 2097 this.autocreate = new BooleanType(); 2098 this.autocreate.setValue(value); 2099 return this; 2100 } 2101 2102 /** 2103 * @return {@link #autodelete} (Whether or not to implicitly delete the fixture 2104 * during teardown If true, the fixture is automatically deleted on each 2105 * server being tested during teardown, therefore no delete operation is 2106 * required for this fixture in the TestScript.teardown section.). This 2107 * is the underlying object with id, value and extensions. The accessor 2108 * "getAutodelete" gives direct access to the value 2109 */ 2110 public BooleanType getAutodeleteElement() { 2111 if (this.autodelete == null) 2112 if (Configuration.errorOnAutoCreate()) 2113 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autodelete"); 2114 else if (Configuration.doAutoCreate()) 2115 this.autodelete = new BooleanType(); // bb 2116 return this.autodelete; 2117 } 2118 2119 public boolean hasAutodeleteElement() { 2120 return this.autodelete != null && !this.autodelete.isEmpty(); 2121 } 2122 2123 public boolean hasAutodelete() { 2124 return this.autodelete != null && !this.autodelete.isEmpty(); 2125 } 2126 2127 /** 2128 * @param value {@link #autodelete} (Whether or not to implicitly delete the 2129 * fixture during teardown If true, the fixture is automatically 2130 * deleted on each server being tested during teardown, therefore 2131 * no delete operation is required for this fixture in the 2132 * TestScript.teardown section.). This is the underlying object 2133 * with id, value and extensions. The accessor "getAutodelete" 2134 * gives direct access to the value 2135 */ 2136 public TestScriptFixtureComponent setAutodeleteElement(BooleanType value) { 2137 this.autodelete = value; 2138 return this; 2139 } 2140 2141 /** 2142 * @return Whether or not to implicitly delete the fixture during teardown If 2143 * true, the fixture is automatically deleted on each server being 2144 * tested during teardown, therefore no delete operation is required for 2145 * this fixture in the TestScript.teardown section. 2146 */ 2147 public boolean getAutodelete() { 2148 return this.autodelete == null || this.autodelete.isEmpty() ? false : this.autodelete.getValue(); 2149 } 2150 2151 /** 2152 * @param value Whether or not to implicitly delete the fixture during teardown 2153 * If true, the fixture is automatically deleted on each server 2154 * being tested during teardown, therefore no delete operation is 2155 * required for this fixture in the TestScript.teardown section. 2156 */ 2157 public TestScriptFixtureComponent setAutodelete(boolean value) { 2158 if (this.autodelete == null) 2159 this.autodelete = new BooleanType(); 2160 this.autodelete.setValue(value); 2161 return this; 2162 } 2163 2164 /** 2165 * @return {@link #resource} (Reference to the resource (containing the contents 2166 * of the resource needed for operations).) 2167 */ 2168 public Reference getResource() { 2169 if (this.resource == null) 2170 if (Configuration.errorOnAutoCreate()) 2171 throw new Error("Attempt to auto-create TestScriptFixtureComponent.resource"); 2172 else if (Configuration.doAutoCreate()) 2173 this.resource = new Reference(); // cc 2174 return this.resource; 2175 } 2176 2177 public boolean hasResource() { 2178 return this.resource != null && !this.resource.isEmpty(); 2179 } 2180 2181 /** 2182 * @param value {@link #resource} (Reference to the resource (containing the 2183 * contents of the resource needed for operations).) 2184 */ 2185 public TestScriptFixtureComponent setResource(Reference value) { 2186 this.resource = value; 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #resource} The actual object that is the target of the 2192 * reference. The reference library doesn't populate this, but you can 2193 * use it to hold the resource if you resolve it. (Reference to the 2194 * resource (containing the contents of the resource needed for 2195 * operations).) 2196 */ 2197 public Resource getResourceTarget() { 2198 return this.resourceTarget; 2199 } 2200 2201 /** 2202 * @param value {@link #resource} The actual object that is the target of the 2203 * reference. The reference library doesn't use these, but you can 2204 * use it to hold the resource if you resolve it. (Reference to the 2205 * resource (containing the contents of the resource needed for 2206 * operations).) 2207 */ 2208 public TestScriptFixtureComponent setResourceTarget(Resource value) { 2209 this.resourceTarget = value; 2210 return this; 2211 } 2212 2213 protected void listChildren(List<Property> childrenList) { 2214 super.listChildren(childrenList); 2215 childrenList.add(new Property("autocreate", "boolean", 2216 "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 2217 0, java.lang.Integer.MAX_VALUE, autocreate)); 2218 childrenList.add(new Property("autodelete", "boolean", 2219 "Whether or not to implicitly delete the fixture during teardown If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 2220 0, java.lang.Integer.MAX_VALUE, autodelete)); 2221 childrenList.add(new Property("resource", "Reference(Any)", 2222 "Reference to the resource (containing the contents of the resource needed for operations).", 0, 2223 java.lang.Integer.MAX_VALUE, resource)); 2224 } 2225 2226 @Override 2227 public void setProperty(String name, Base value) throws FHIRException { 2228 if (name.equals("autocreate")) 2229 this.autocreate = castToBoolean(value); // BooleanType 2230 else if (name.equals("autodelete")) 2231 this.autodelete = castToBoolean(value); // BooleanType 2232 else if (name.equals("resource")) 2233 this.resource = castToReference(value); // Reference 2234 else 2235 super.setProperty(name, value); 2236 } 2237 2238 @Override 2239 public Base addChild(String name) throws FHIRException { 2240 if (name.equals("autocreate")) { 2241 throw new FHIRException("Cannot call addChild on a singleton property TestScript.autocreate"); 2242 } else if (name.equals("autodelete")) { 2243 throw new FHIRException("Cannot call addChild on a singleton property TestScript.autodelete"); 2244 } else if (name.equals("resource")) { 2245 this.resource = new Reference(); 2246 return this.resource; 2247 } else 2248 return super.addChild(name); 2249 } 2250 2251 public TestScriptFixtureComponent copy() { 2252 TestScriptFixtureComponent dst = new TestScriptFixtureComponent(); 2253 copyValues(dst); 2254 dst.autocreate = autocreate == null ? null : autocreate.copy(); 2255 dst.autodelete = autodelete == null ? null : autodelete.copy(); 2256 dst.resource = resource == null ? null : resource.copy(); 2257 return dst; 2258 } 2259 2260 @Override 2261 public boolean equalsDeep(Base other) { 2262 if (!super.equalsDeep(other)) 2263 return false; 2264 if (!(other instanceof TestScriptFixtureComponent)) 2265 return false; 2266 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other; 2267 return compareDeep(autocreate, o.autocreate, true) && compareDeep(autodelete, o.autodelete, true) 2268 && compareDeep(resource, o.resource, true); 2269 } 2270 2271 @Override 2272 public boolean equalsShallow(Base other) { 2273 if (!super.equalsShallow(other)) 2274 return false; 2275 if (!(other instanceof TestScriptFixtureComponent)) 2276 return false; 2277 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other; 2278 return compareValues(autocreate, o.autocreate, true) && compareValues(autodelete, o.autodelete, true); 2279 } 2280 2281 public boolean isEmpty() { 2282 return super.isEmpty() && (autocreate == null || autocreate.isEmpty()) 2283 && (autodelete == null || autodelete.isEmpty()) && (resource == null || resource.isEmpty()); 2284 } 2285 2286 public String fhirType() { 2287 return "TestScript.fixture"; 2288 2289 } 2290 2291 } 2292 2293 @Block() 2294 public static class TestScriptVariableComponent extends BackboneElement implements IBaseBackboneElement { 2295 /** 2296 * Descriptive name for this variable. 2297 */ 2298 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2299 @Description(shortDefinition = "Descriptive name for this variable", formalDefinition = "Descriptive name for this variable.") 2300 protected StringType name; 2301 2302 /** 2303 * Will be used to grab the HTTP header field value from the headers that 2304 * sourceId is pointing to. 2305 */ 2306 @Child(name = "headerField", type = { 2307 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2308 @Description(shortDefinition = "HTTP header field name for source", formalDefinition = "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.") 2309 protected StringType headerField; 2310 2311 /** 2312 * XPath or JSONPath against the fixture body. When variables are defined, 2313 * either headerField must be specified or path, but not both. 2314 */ 2315 @Child(name = "path", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2316 @Description(shortDefinition = "XPath or JSONPath against the fixture body", formalDefinition = "XPath or JSONPath against the fixture body. When variables are defined, either headerField must be specified or path, but not both.") 2317 protected StringType path; 2318 2319 /** 2320 * Fixture to evaluate the XPath/JSONPath expression or the headerField against 2321 * within this variable. 2322 */ 2323 @Child(name = "sourceId", type = { IdType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2324 @Description(shortDefinition = "Fixture Id of source expression or headerField within this variable", formalDefinition = "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.") 2325 protected IdType sourceId; 2326 2327 private static final long serialVersionUID = 1128806685L; 2328 2329 /* 2330 * Constructor 2331 */ 2332 public TestScriptVariableComponent() { 2333 super(); 2334 } 2335 2336 /* 2337 * Constructor 2338 */ 2339 public TestScriptVariableComponent(StringType name) { 2340 super(); 2341 this.name = name; 2342 } 2343 2344 /** 2345 * @return {@link #name} (Descriptive name for this variable.). This is the 2346 * underlying object with id, value and extensions. The accessor 2347 * "getName" gives direct access to the value 2348 */ 2349 public StringType getNameElement() { 2350 if (this.name == null) 2351 if (Configuration.errorOnAutoCreate()) 2352 throw new Error("Attempt to auto-create TestScriptVariableComponent.name"); 2353 else if (Configuration.doAutoCreate()) 2354 this.name = new StringType(); // bb 2355 return this.name; 2356 } 2357 2358 public boolean hasNameElement() { 2359 return this.name != null && !this.name.isEmpty(); 2360 } 2361 2362 public boolean hasName() { 2363 return this.name != null && !this.name.isEmpty(); 2364 } 2365 2366 /** 2367 * @param value {@link #name} (Descriptive name for this variable.). This is the 2368 * underlying object with id, value and extensions. The accessor 2369 * "getName" gives direct access to the value 2370 */ 2371 public TestScriptVariableComponent setNameElement(StringType value) { 2372 this.name = value; 2373 return this; 2374 } 2375 2376 /** 2377 * @return Descriptive name for this variable. 2378 */ 2379 public String getName() { 2380 return this.name == null ? null : this.name.getValue(); 2381 } 2382 2383 /** 2384 * @param value Descriptive name for this variable. 2385 */ 2386 public TestScriptVariableComponent setName(String value) { 2387 if (this.name == null) 2388 this.name = new StringType(); 2389 this.name.setValue(value); 2390 return this; 2391 } 2392 2393 /** 2394 * @return {@link #headerField} (Will be used to grab the HTTP header field 2395 * value from the headers that sourceId is pointing to.). This is the 2396 * underlying object with id, value and extensions. The accessor 2397 * "getHeaderField" gives direct access to the value 2398 */ 2399 public StringType getHeaderFieldElement() { 2400 if (this.headerField == null) 2401 if (Configuration.errorOnAutoCreate()) 2402 throw new Error("Attempt to auto-create TestScriptVariableComponent.headerField"); 2403 else if (Configuration.doAutoCreate()) 2404 this.headerField = new StringType(); // bb 2405 return this.headerField; 2406 } 2407 2408 public boolean hasHeaderFieldElement() { 2409 return this.headerField != null && !this.headerField.isEmpty(); 2410 } 2411 2412 public boolean hasHeaderField() { 2413 return this.headerField != null && !this.headerField.isEmpty(); 2414 } 2415 2416 /** 2417 * @param value {@link #headerField} (Will be used to grab the HTTP header field 2418 * value from the headers that sourceId is pointing to.). This is 2419 * the underlying object with id, value and extensions. The 2420 * accessor "getHeaderField" gives direct access to the value 2421 */ 2422 public TestScriptVariableComponent setHeaderFieldElement(StringType value) { 2423 this.headerField = value; 2424 return this; 2425 } 2426 2427 /** 2428 * @return Will be used to grab the HTTP header field value from the headers 2429 * that sourceId is pointing to. 2430 */ 2431 public String getHeaderField() { 2432 return this.headerField == null ? null : this.headerField.getValue(); 2433 } 2434 2435 /** 2436 * @param value Will be used to grab the HTTP header field value from the 2437 * headers that sourceId is pointing to. 2438 */ 2439 public TestScriptVariableComponent setHeaderField(String value) { 2440 if (Utilities.noString(value)) 2441 this.headerField = null; 2442 else { 2443 if (this.headerField == null) 2444 this.headerField = new StringType(); 2445 this.headerField.setValue(value); 2446 } 2447 return this; 2448 } 2449 2450 /** 2451 * @return {@link #path} (XPath or JSONPath against the fixture body. When 2452 * variables are defined, either headerField must be specified or path, 2453 * but not both.). This is the underlying object with id, value and 2454 * extensions. The accessor "getPath" gives direct access to the value 2455 */ 2456 public StringType getPathElement() { 2457 if (this.path == null) 2458 if (Configuration.errorOnAutoCreate()) 2459 throw new Error("Attempt to auto-create TestScriptVariableComponent.path"); 2460 else if (Configuration.doAutoCreate()) 2461 this.path = new StringType(); // bb 2462 return this.path; 2463 } 2464 2465 public boolean hasPathElement() { 2466 return this.path != null && !this.path.isEmpty(); 2467 } 2468 2469 public boolean hasPath() { 2470 return this.path != null && !this.path.isEmpty(); 2471 } 2472 2473 /** 2474 * @param value {@link #path} (XPath or JSONPath against the fixture body. When 2475 * variables are defined, either headerField must be specified or 2476 * path, but not both.). This is the underlying object with id, 2477 * value and extensions. The accessor "getPath" gives direct access 2478 * to the value 2479 */ 2480 public TestScriptVariableComponent setPathElement(StringType value) { 2481 this.path = value; 2482 return this; 2483 } 2484 2485 /** 2486 * @return XPath or JSONPath against the fixture body. When variables are 2487 * defined, either headerField must be specified or path, but not both. 2488 */ 2489 public String getPath() { 2490 return this.path == null ? null : this.path.getValue(); 2491 } 2492 2493 /** 2494 * @param value XPath or JSONPath against the fixture body. When variables are 2495 * defined, either headerField must be specified or path, but not 2496 * both. 2497 */ 2498 public TestScriptVariableComponent setPath(String value) { 2499 if (Utilities.noString(value)) 2500 this.path = null; 2501 else { 2502 if (this.path == null) 2503 this.path = new StringType(); 2504 this.path.setValue(value); 2505 } 2506 return this; 2507 } 2508 2509 /** 2510 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression 2511 * or the headerField against within this variable.). This is the 2512 * underlying object with id, value and extensions. The accessor 2513 * "getSourceId" gives direct access to the value 2514 */ 2515 public IdType getSourceIdElement() { 2516 if (this.sourceId == null) 2517 if (Configuration.errorOnAutoCreate()) 2518 throw new Error("Attempt to auto-create TestScriptVariableComponent.sourceId"); 2519 else if (Configuration.doAutoCreate()) 2520 this.sourceId = new IdType(); // bb 2521 return this.sourceId; 2522 } 2523 2524 public boolean hasSourceIdElement() { 2525 return this.sourceId != null && !this.sourceId.isEmpty(); 2526 } 2527 2528 public boolean hasSourceId() { 2529 return this.sourceId != null && !this.sourceId.isEmpty(); 2530 } 2531 2532 /** 2533 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath 2534 * expression or the headerField against within this variable.). 2535 * This is the underlying object with id, value and extensions. The 2536 * accessor "getSourceId" gives direct access to the value 2537 */ 2538 public TestScriptVariableComponent setSourceIdElement(IdType value) { 2539 this.sourceId = value; 2540 return this; 2541 } 2542 2543 /** 2544 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField 2545 * against within this variable. 2546 */ 2547 public String getSourceId() { 2548 return this.sourceId == null ? null : this.sourceId.getValue(); 2549 } 2550 2551 /** 2552 * @param value Fixture to evaluate the XPath/JSONPath expression or the 2553 * headerField against within this variable. 2554 */ 2555 public TestScriptVariableComponent setSourceId(String value) { 2556 if (Utilities.noString(value)) 2557 this.sourceId = null; 2558 else { 2559 if (this.sourceId == null) 2560 this.sourceId = new IdType(); 2561 this.sourceId.setValue(value); 2562 } 2563 return this; 2564 } 2565 2566 protected void listChildren(List<Property> childrenList) { 2567 super.listChildren(childrenList); 2568 childrenList.add( 2569 new Property("name", "string", "Descriptive name for this variable.", 0, java.lang.Integer.MAX_VALUE, name)); 2570 childrenList.add(new Property("headerField", "string", 2571 "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 2572 java.lang.Integer.MAX_VALUE, headerField)); 2573 childrenList.add(new Property("path", "string", 2574 "XPath or JSONPath against the fixture body. When variables are defined, either headerField must be specified or path, but not both.", 2575 0, java.lang.Integer.MAX_VALUE, path)); 2576 childrenList.add(new Property("sourceId", "id", 2577 "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 2578 java.lang.Integer.MAX_VALUE, sourceId)); 2579 } 2580 2581 @Override 2582 public void setProperty(String name, Base value) throws FHIRException { 2583 if (name.equals("name")) 2584 this.name = castToString(value); // StringType 2585 else if (name.equals("headerField")) 2586 this.headerField = castToString(value); // StringType 2587 else if (name.equals("path")) 2588 this.path = castToString(value); // StringType 2589 else if (name.equals("sourceId")) 2590 this.sourceId = castToId(value); // IdType 2591 else 2592 super.setProperty(name, value); 2593 } 2594 2595 @Override 2596 public Base addChild(String name) throws FHIRException { 2597 if (name.equals("name")) { 2598 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 2599 } else if (name.equals("headerField")) { 2600 throw new FHIRException("Cannot call addChild on a singleton property TestScript.headerField"); 2601 } else if (name.equals("path")) { 2602 throw new FHIRException("Cannot call addChild on a singleton property TestScript.path"); 2603 } else if (name.equals("sourceId")) { 2604 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 2605 } else 2606 return super.addChild(name); 2607 } 2608 2609 public TestScriptVariableComponent copy() { 2610 TestScriptVariableComponent dst = new TestScriptVariableComponent(); 2611 copyValues(dst); 2612 dst.name = name == null ? null : name.copy(); 2613 dst.headerField = headerField == null ? null : headerField.copy(); 2614 dst.path = path == null ? null : path.copy(); 2615 dst.sourceId = sourceId == null ? null : sourceId.copy(); 2616 return dst; 2617 } 2618 2619 @Override 2620 public boolean equalsDeep(Base other) { 2621 if (!super.equalsDeep(other)) 2622 return false; 2623 if (!(other instanceof TestScriptVariableComponent)) 2624 return false; 2625 TestScriptVariableComponent o = (TestScriptVariableComponent) other; 2626 return compareDeep(name, o.name, true) && compareDeep(headerField, o.headerField, true) 2627 && compareDeep(path, o.path, true) && compareDeep(sourceId, o.sourceId, true); 2628 } 2629 2630 @Override 2631 public boolean equalsShallow(Base other) { 2632 if (!super.equalsShallow(other)) 2633 return false; 2634 if (!(other instanceof TestScriptVariableComponent)) 2635 return false; 2636 TestScriptVariableComponent o = (TestScriptVariableComponent) other; 2637 return compareValues(name, o.name, true) && compareValues(headerField, o.headerField, true) 2638 && compareValues(path, o.path, true) && compareValues(sourceId, o.sourceId, true); 2639 } 2640 2641 public boolean isEmpty() { 2642 return super.isEmpty() && (name == null || name.isEmpty()) && (headerField == null || headerField.isEmpty()) 2643 && (path == null || path.isEmpty()) && (sourceId == null || sourceId.isEmpty()); 2644 } 2645 2646 public String fhirType() { 2647 return "TestScript.variable"; 2648 2649 } 2650 2651 } 2652 2653 @Block() 2654 public static class TestScriptSetupComponent extends BackboneElement implements IBaseBackboneElement { 2655 /** 2656 * Capabilities that must exist and are assumed to function correctly on the 2657 * FHIR server being tested. 2658 */ 2659 @Child(name = "metadata", type = { 2660 TestScriptMetadataComponent.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2661 @Description(shortDefinition = "Capabilities that are assumed to function correctly on the FHIR server being tested", formalDefinition = "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.") 2662 protected TestScriptMetadataComponent metadata; 2663 2664 /** 2665 * Action would contain either an operation or an assertion. 2666 */ 2667 @Child(name = "action", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2668 @Description(shortDefinition = "A setup operation or assert to perform", formalDefinition = "Action would contain either an operation or an assertion.") 2669 protected List<TestScriptSetupActionComponent> action; 2670 2671 private static final long serialVersionUID = -1836543723L; 2672 2673 /* 2674 * Constructor 2675 */ 2676 public TestScriptSetupComponent() { 2677 super(); 2678 } 2679 2680 /** 2681 * @return {@link #metadata} (Capabilities that must exist and are assumed to 2682 * function correctly on the FHIR server being tested.) 2683 */ 2684 public TestScriptMetadataComponent getMetadata() { 2685 if (this.metadata == null) 2686 if (Configuration.errorOnAutoCreate()) 2687 throw new Error("Attempt to auto-create TestScriptSetupComponent.metadata"); 2688 else if (Configuration.doAutoCreate()) 2689 this.metadata = new TestScriptMetadataComponent(); // cc 2690 return this.metadata; 2691 } 2692 2693 public boolean hasMetadata() { 2694 return this.metadata != null && !this.metadata.isEmpty(); 2695 } 2696 2697 /** 2698 * @param value {@link #metadata} (Capabilities that must exist and are assumed 2699 * to function correctly on the FHIR server being tested.) 2700 */ 2701 public TestScriptSetupComponent setMetadata(TestScriptMetadataComponent value) { 2702 this.metadata = value; 2703 return this; 2704 } 2705 2706 /** 2707 * @return {@link #action} (Action would contain either an operation or an 2708 * assertion.) 2709 */ 2710 public List<TestScriptSetupActionComponent> getAction() { 2711 if (this.action == null) 2712 this.action = new ArrayList<TestScriptSetupActionComponent>(); 2713 return this.action; 2714 } 2715 2716 public boolean hasAction() { 2717 if (this.action == null) 2718 return false; 2719 for (TestScriptSetupActionComponent item : this.action) 2720 if (!item.isEmpty()) 2721 return true; 2722 return false; 2723 } 2724 2725 /** 2726 * @return {@link #action} (Action would contain either an operation or an 2727 * assertion.) 2728 */ 2729 // syntactic sugar 2730 public TestScriptSetupActionComponent addAction() { // 3 2731 TestScriptSetupActionComponent t = new TestScriptSetupActionComponent(); 2732 if (this.action == null) 2733 this.action = new ArrayList<TestScriptSetupActionComponent>(); 2734 this.action.add(t); 2735 return t; 2736 } 2737 2738 // syntactic sugar 2739 public TestScriptSetupComponent addAction(TestScriptSetupActionComponent t) { // 3 2740 if (t == null) 2741 return this; 2742 if (this.action == null) 2743 this.action = new ArrayList<TestScriptSetupActionComponent>(); 2744 this.action.add(t); 2745 return this; 2746 } 2747 2748 protected void listChildren(List<Property> childrenList) { 2749 super.listChildren(childrenList); 2750 childrenList.add(new Property("metadata", "@TestScript.metadata", 2751 "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, 2752 java.lang.Integer.MAX_VALUE, metadata)); 2753 childrenList.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, 2754 java.lang.Integer.MAX_VALUE, action)); 2755 } 2756 2757 @Override 2758 public void setProperty(String name, Base value) throws FHIRException { 2759 if (name.equals("metadata")) 2760 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 2761 else if (name.equals("action")) 2762 this.getAction().add((TestScriptSetupActionComponent) value); 2763 else 2764 super.setProperty(name, value); 2765 } 2766 2767 @Override 2768 public Base addChild(String name) throws FHIRException { 2769 if (name.equals("metadata")) { 2770 this.metadata = new TestScriptMetadataComponent(); 2771 return this.metadata; 2772 } else if (name.equals("action")) { 2773 return addAction(); 2774 } else 2775 return super.addChild(name); 2776 } 2777 2778 public TestScriptSetupComponent copy() { 2779 TestScriptSetupComponent dst = new TestScriptSetupComponent(); 2780 copyValues(dst); 2781 dst.metadata = metadata == null ? null : metadata.copy(); 2782 if (action != null) { 2783 dst.action = new ArrayList<TestScriptSetupActionComponent>(); 2784 for (TestScriptSetupActionComponent i : action) 2785 dst.action.add(i.copy()); 2786 } 2787 ; 2788 return dst; 2789 } 2790 2791 @Override 2792 public boolean equalsDeep(Base other) { 2793 if (!super.equalsDeep(other)) 2794 return false; 2795 if (!(other instanceof TestScriptSetupComponent)) 2796 return false; 2797 TestScriptSetupComponent o = (TestScriptSetupComponent) other; 2798 return compareDeep(metadata, o.metadata, true) && compareDeep(action, o.action, true); 2799 } 2800 2801 @Override 2802 public boolean equalsShallow(Base other) { 2803 if (!super.equalsShallow(other)) 2804 return false; 2805 if (!(other instanceof TestScriptSetupComponent)) 2806 return false; 2807 TestScriptSetupComponent o = (TestScriptSetupComponent) other; 2808 return true; 2809 } 2810 2811 public boolean isEmpty() { 2812 return super.isEmpty() && (metadata == null || metadata.isEmpty()) && (action == null || action.isEmpty()); 2813 } 2814 2815 public String fhirType() { 2816 return "TestScript.setup"; 2817 2818 } 2819 2820 } 2821 2822 @Block() 2823 public static class TestScriptSetupActionComponent extends BackboneElement implements IBaseBackboneElement { 2824 /** 2825 * The operation to perform. 2826 */ 2827 @Child(name = "operation", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = false) 2828 @Description(shortDefinition = "The setup operation to perform", formalDefinition = "The operation to perform.") 2829 protected TestScriptSetupActionOperationComponent operation; 2830 2831 /** 2832 * Evaluates the results of previous operations to determine if the server under 2833 * test behaves appropriately. 2834 */ 2835 @Child(name = "assert", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = false) 2836 @Description(shortDefinition = "The assertion to perform", formalDefinition = "Evaluates the results of previous operations to determine if the server under test behaves appropriately.") 2837 protected TestScriptSetupActionAssertComponent assert_; 2838 2839 private static final long serialVersionUID = 1411550037L; 2840 2841 /* 2842 * Constructor 2843 */ 2844 public TestScriptSetupActionComponent() { 2845 super(); 2846 } 2847 2848 /** 2849 * @return {@link #operation} (The operation to perform.) 2850 */ 2851 public TestScriptSetupActionOperationComponent getOperation() { 2852 if (this.operation == null) 2853 if (Configuration.errorOnAutoCreate()) 2854 throw new Error("Attempt to auto-create TestScriptSetupActionComponent.operation"); 2855 else if (Configuration.doAutoCreate()) 2856 this.operation = new TestScriptSetupActionOperationComponent(); // cc 2857 return this.operation; 2858 } 2859 2860 public boolean hasOperation() { 2861 return this.operation != null && !this.operation.isEmpty(); 2862 } 2863 2864 /** 2865 * @param value {@link #operation} (The operation to perform.) 2866 */ 2867 public TestScriptSetupActionComponent setOperation(TestScriptSetupActionOperationComponent value) { 2868 this.operation = value; 2869 return this; 2870 } 2871 2872 /** 2873 * @return {@link #assert_} (Evaluates the results of previous operations to 2874 * determine if the server under test behaves appropriately.) 2875 */ 2876 public TestScriptSetupActionAssertComponent getAssert() { 2877 if (this.assert_ == null) 2878 if (Configuration.errorOnAutoCreate()) 2879 throw new Error("Attempt to auto-create TestScriptSetupActionComponent.assert_"); 2880 else if (Configuration.doAutoCreate()) 2881 this.assert_ = new TestScriptSetupActionAssertComponent(); // cc 2882 return this.assert_; 2883 } 2884 2885 public boolean hasAssert() { 2886 return this.assert_ != null && !this.assert_.isEmpty(); 2887 } 2888 2889 /** 2890 * @param value {@link #assert_} (Evaluates the results of previous operations 2891 * to determine if the server under test behaves appropriately.) 2892 */ 2893 public TestScriptSetupActionComponent setAssert(TestScriptSetupActionAssertComponent value) { 2894 this.assert_ = value; 2895 return this; 2896 } 2897 2898 protected void listChildren(List<Property> childrenList) { 2899 super.listChildren(childrenList); 2900 childrenList 2901 .add(new Property("operation", "", "The operation to perform.", 0, java.lang.Integer.MAX_VALUE, operation)); 2902 childrenList.add(new Property("assert", "", 2903 "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 2904 0, java.lang.Integer.MAX_VALUE, assert_)); 2905 } 2906 2907 @Override 2908 public void setProperty(String name, Base value) throws FHIRException { 2909 if (name.equals("operation")) 2910 this.operation = (TestScriptSetupActionOperationComponent) value; // TestScriptSetupActionOperationComponent 2911 else if (name.equals("assert")) 2912 this.assert_ = (TestScriptSetupActionAssertComponent) value; // TestScriptSetupActionAssertComponent 2913 else 2914 super.setProperty(name, value); 2915 } 2916 2917 @Override 2918 public Base addChild(String name) throws FHIRException { 2919 if (name.equals("operation")) { 2920 this.operation = new TestScriptSetupActionOperationComponent(); 2921 return this.operation; 2922 } else if (name.equals("assert")) { 2923 this.assert_ = new TestScriptSetupActionAssertComponent(); 2924 return this.assert_; 2925 } else 2926 return super.addChild(name); 2927 } 2928 2929 public TestScriptSetupActionComponent copy() { 2930 TestScriptSetupActionComponent dst = new TestScriptSetupActionComponent(); 2931 copyValues(dst); 2932 dst.operation = operation == null ? null : operation.copy(); 2933 dst.assert_ = assert_ == null ? null : assert_.copy(); 2934 return dst; 2935 } 2936 2937 @Override 2938 public boolean equalsDeep(Base other) { 2939 if (!super.equalsDeep(other)) 2940 return false; 2941 if (!(other instanceof TestScriptSetupActionComponent)) 2942 return false; 2943 TestScriptSetupActionComponent o = (TestScriptSetupActionComponent) other; 2944 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 2945 } 2946 2947 @Override 2948 public boolean equalsShallow(Base other) { 2949 if (!super.equalsShallow(other)) 2950 return false; 2951 if (!(other instanceof TestScriptSetupActionComponent)) 2952 return false; 2953 TestScriptSetupActionComponent o = (TestScriptSetupActionComponent) other; 2954 return true; 2955 } 2956 2957 public boolean isEmpty() { 2958 return super.isEmpty() && (operation == null || operation.isEmpty()) && (assert_ == null || assert_.isEmpty()); 2959 } 2960 2961 public String fhirType() { 2962 return "TestScript.setup.action"; 2963 2964 } 2965 2966 } 2967 2968 @Block() 2969 public static class TestScriptSetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 2970 /** 2971 * Server interaction or operation type. 2972 */ 2973 @Child(name = "type", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2974 @Description(shortDefinition = "The setup operation type that will be executed", formalDefinition = "Server interaction or operation type.") 2975 protected Coding type; 2976 2977 /** 2978 * The type of the resource. See http://hl7-fhir.github.io/resourcelist.html. 2979 */ 2980 @Child(name = "resource", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2981 @Description(shortDefinition = "Resource type", formalDefinition = "The type of the resource. See http://hl7-fhir.github.io/resourcelist.html.") 2982 protected CodeType resource; 2983 2984 /** 2985 * The label would be used for tracking/logging purposes by test engines. 2986 */ 2987 @Child(name = "label", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2988 @Description(shortDefinition = "Tracking/logging operation label", formalDefinition = "The label would be used for tracking/logging purposes by test engines.") 2989 protected StringType label; 2990 2991 /** 2992 * The description would be used by test engines for tracking and reporting 2993 * purposes. 2994 */ 2995 @Child(name = "description", type = { 2996 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2997 @Description(shortDefinition = "Tracking/reporting operation description", formalDefinition = "The description would be used by test engines for tracking and reporting purposes.") 2998 protected StringType description; 2999 3000 /** 3001 * The content-type or mime-type to use for RESTful operation in the 'Accept' 3002 * header. 3003 */ 3004 @Child(name = "accept", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3005 @Description(shortDefinition = "xml | json", formalDefinition = "The content-type or mime-type to use for RESTful operation in the 'Accept' header.") 3006 protected Enumeration<ContentType> accept; 3007 3008 /** 3009 * The content-type or mime-type to use for RESTful operation in the 3010 * 'Content-Type' header. 3011 */ 3012 @Child(name = "contentType", type = { 3013 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3014 @Description(shortDefinition = "xml | json", formalDefinition = "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.") 3015 protected Enumeration<ContentType> contentType; 3016 3017 /** 3018 * Which server to perform the operation on. 3019 */ 3020 @Child(name = "destination", type = { 3021 IntegerType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3022 @Description(shortDefinition = "Which server to perform the operation on", formalDefinition = "Which server to perform the operation on.") 3023 protected IntegerType destination; 3024 3025 /** 3026 * Whether or not to implicitly send the request url in encoded format. The 3027 * default is true to match the standard RESTful client behavior. Set to false 3028 * when communicating with a server that does not support encoded url paths. 3029 */ 3030 @Child(name = "encodeRequestUrl", type = { 3031 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3032 @Description(shortDefinition = "Whether or not to send the request url in encoded format", formalDefinition = "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.") 3033 protected BooleanType encodeRequestUrl; 3034 3035 /** 3036 * Path plus parameters after [type]. Used to set parts of the request URL 3037 * explicitly. 3038 */ 3039 @Child(name = "params", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 3040 @Description(shortDefinition = "Explicitly defined path parameters", formalDefinition = "Path plus parameters after [type]. Used to set parts of the request URL explicitly.") 3041 protected StringType params; 3042 3043 /** 3044 * Header elements would be used to set HTTP headers. 3045 */ 3046 @Child(name = "requestHeader", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3047 @Description(shortDefinition = "Each operation can have one ore more header elements", formalDefinition = "Header elements would be used to set HTTP headers.") 3048 protected List<TestScriptSetupActionOperationRequestHeaderComponent> requestHeader; 3049 3050 /** 3051 * The fixture id (maybe new) to map to the response. 3052 */ 3053 @Child(name = "responseId", type = { 3054 IdType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 3055 @Description(shortDefinition = "Fixture Id of mapped response", formalDefinition = "The fixture id (maybe new) to map to the response.") 3056 protected IdType responseId; 3057 3058 /** 3059 * The id of the fixture used as the body of a PUT or POST request. 3060 */ 3061 @Child(name = "sourceId", type = { IdType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 3062 @Description(shortDefinition = "Fixture Id of body for PUT and POST requests", formalDefinition = "The id of the fixture used as the body of a PUT or POST request.") 3063 protected IdType sourceId; 3064 3065 /** 3066 * Id of fixture used for extracting the [id], [type], and [vid] for GET 3067 * requests. 3068 */ 3069 @Child(name = "targetId", type = { IdType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 3070 @Description(shortDefinition = "Id of fixture used for extracting the [id], [type], and [vid] for GET requests", formalDefinition = "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.") 3071 protected IdType targetId; 3072 3073 /** 3074 * Complete request URL. 3075 */ 3076 @Child(name = "url", type = { StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 3077 @Description(shortDefinition = "Request URL", formalDefinition = "Complete request URL.") 3078 protected StringType url; 3079 3080 private static final long serialVersionUID = -590188078L; 3081 3082 /* 3083 * Constructor 3084 */ 3085 public TestScriptSetupActionOperationComponent() { 3086 super(); 3087 } 3088 3089 /** 3090 * @return {@link #type} (Server interaction or operation type.) 3091 */ 3092 public Coding getType() { 3093 if (this.type == null) 3094 if (Configuration.errorOnAutoCreate()) 3095 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.type"); 3096 else if (Configuration.doAutoCreate()) 3097 this.type = new Coding(); // cc 3098 return this.type; 3099 } 3100 3101 public boolean hasType() { 3102 return this.type != null && !this.type.isEmpty(); 3103 } 3104 3105 /** 3106 * @param value {@link #type} (Server interaction or operation type.) 3107 */ 3108 public TestScriptSetupActionOperationComponent setType(Coding value) { 3109 this.type = value; 3110 return this; 3111 } 3112 3113 /** 3114 * @return {@link #resource} (The type of the resource. See 3115 * http://hl7-fhir.github.io/resourcelist.html.). This is the underlying 3116 * object with id, value and extensions. The accessor "getResource" 3117 * gives direct access to the value 3118 */ 3119 public CodeType getResourceElement() { 3120 if (this.resource == null) 3121 if (Configuration.errorOnAutoCreate()) 3122 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.resource"); 3123 else if (Configuration.doAutoCreate()) 3124 this.resource = new CodeType(); // bb 3125 return this.resource; 3126 } 3127 3128 public boolean hasResourceElement() { 3129 return this.resource != null && !this.resource.isEmpty(); 3130 } 3131 3132 public boolean hasResource() { 3133 return this.resource != null && !this.resource.isEmpty(); 3134 } 3135 3136 /** 3137 * @param value {@link #resource} (The type of the resource. See 3138 * http://hl7-fhir.github.io/resourcelist.html.). This is the 3139 * underlying object with id, value and extensions. The accessor 3140 * "getResource" gives direct access to the value 3141 */ 3142 public TestScriptSetupActionOperationComponent setResourceElement(CodeType value) { 3143 this.resource = value; 3144 return this; 3145 } 3146 3147 /** 3148 * @return The type of the resource. See 3149 * http://hl7-fhir.github.io/resourcelist.html. 3150 */ 3151 public String getResource() { 3152 return this.resource == null ? null : this.resource.getValue(); 3153 } 3154 3155 /** 3156 * @param value The type of the resource. See 3157 * http://hl7-fhir.github.io/resourcelist.html. 3158 */ 3159 public TestScriptSetupActionOperationComponent setResource(String value) { 3160 if (Utilities.noString(value)) 3161 this.resource = null; 3162 else { 3163 if (this.resource == null) 3164 this.resource = new CodeType(); 3165 this.resource.setValue(value); 3166 } 3167 return this; 3168 } 3169 3170 /** 3171 * @return {@link #label} (The label would be used for tracking/logging purposes 3172 * by test engines.). This is the underlying object with id, value and 3173 * extensions. The accessor "getLabel" gives direct access to the value 3174 */ 3175 public StringType getLabelElement() { 3176 if (this.label == null) 3177 if (Configuration.errorOnAutoCreate()) 3178 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.label"); 3179 else if (Configuration.doAutoCreate()) 3180 this.label = new StringType(); // bb 3181 return this.label; 3182 } 3183 3184 public boolean hasLabelElement() { 3185 return this.label != null && !this.label.isEmpty(); 3186 } 3187 3188 public boolean hasLabel() { 3189 return this.label != null && !this.label.isEmpty(); 3190 } 3191 3192 /** 3193 * @param value {@link #label} (The label would be used for tracking/logging 3194 * purposes by test engines.). This is the underlying object with 3195 * id, value and extensions. The accessor "getLabel" gives direct 3196 * access to the value 3197 */ 3198 public TestScriptSetupActionOperationComponent setLabelElement(StringType value) { 3199 this.label = value; 3200 return this; 3201 } 3202 3203 /** 3204 * @return The label would be used for tracking/logging purposes by test 3205 * engines. 3206 */ 3207 public String getLabel() { 3208 return this.label == null ? null : this.label.getValue(); 3209 } 3210 3211 /** 3212 * @param value The label would be used for tracking/logging purposes by test 3213 * engines. 3214 */ 3215 public TestScriptSetupActionOperationComponent setLabel(String value) { 3216 if (Utilities.noString(value)) 3217 this.label = null; 3218 else { 3219 if (this.label == null) 3220 this.label = new StringType(); 3221 this.label.setValue(value); 3222 } 3223 return this; 3224 } 3225 3226 /** 3227 * @return {@link #description} (The description would be used by test engines 3228 * for tracking and reporting purposes.). This is the underlying object 3229 * with id, value and extensions. The accessor "getDescription" gives 3230 * direct access to the value 3231 */ 3232 public StringType getDescriptionElement() { 3233 if (this.description == null) 3234 if (Configuration.errorOnAutoCreate()) 3235 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.description"); 3236 else if (Configuration.doAutoCreate()) 3237 this.description = new StringType(); // bb 3238 return this.description; 3239 } 3240 3241 public boolean hasDescriptionElement() { 3242 return this.description != null && !this.description.isEmpty(); 3243 } 3244 3245 public boolean hasDescription() { 3246 return this.description != null && !this.description.isEmpty(); 3247 } 3248 3249 /** 3250 * @param value {@link #description} (The description would be used by test 3251 * engines for tracking and reporting purposes.). This is the 3252 * underlying object with id, value and extensions. The accessor 3253 * "getDescription" gives direct access to the value 3254 */ 3255 public TestScriptSetupActionOperationComponent setDescriptionElement(StringType value) { 3256 this.description = value; 3257 return this; 3258 } 3259 3260 /** 3261 * @return The description would be used by test engines for tracking and 3262 * reporting purposes. 3263 */ 3264 public String getDescription() { 3265 return this.description == null ? null : this.description.getValue(); 3266 } 3267 3268 /** 3269 * @param value The description would be used by test engines for tracking and 3270 * reporting purposes. 3271 */ 3272 public TestScriptSetupActionOperationComponent setDescription(String value) { 3273 if (Utilities.noString(value)) 3274 this.description = null; 3275 else { 3276 if (this.description == null) 3277 this.description = new StringType(); 3278 this.description.setValue(value); 3279 } 3280 return this; 3281 } 3282 3283 /** 3284 * @return {@link #accept} (The content-type or mime-type to use for RESTful 3285 * operation in the 'Accept' header.). This is the underlying object 3286 * with id, value and extensions. The accessor "getAccept" gives direct 3287 * access to the value 3288 */ 3289 public Enumeration<ContentType> getAcceptElement() { 3290 if (this.accept == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.accept"); 3293 else if (Configuration.doAutoCreate()) 3294 this.accept = new Enumeration<ContentType>(new ContentTypeEnumFactory()); // bb 3295 return this.accept; 3296 } 3297 3298 public boolean hasAcceptElement() { 3299 return this.accept != null && !this.accept.isEmpty(); 3300 } 3301 3302 public boolean hasAccept() { 3303 return this.accept != null && !this.accept.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #accept} (The content-type or mime-type to use for 3308 * RESTful operation in the 'Accept' header.). This is the 3309 * underlying object with id, value and extensions. The accessor 3310 * "getAccept" gives direct access to the value 3311 */ 3312 public TestScriptSetupActionOperationComponent setAcceptElement(Enumeration<ContentType> value) { 3313 this.accept = value; 3314 return this; 3315 } 3316 3317 /** 3318 * @return The content-type or mime-type to use for RESTful operation in the 3319 * 'Accept' header. 3320 */ 3321 public ContentType getAccept() { 3322 return this.accept == null ? null : this.accept.getValue(); 3323 } 3324 3325 /** 3326 * @param value The content-type or mime-type to use for RESTful operation in 3327 * the 'Accept' header. 3328 */ 3329 public TestScriptSetupActionOperationComponent setAccept(ContentType value) { 3330 if (value == null) 3331 this.accept = null; 3332 else { 3333 if (this.accept == null) 3334 this.accept = new Enumeration<ContentType>(new ContentTypeEnumFactory()); 3335 this.accept.setValue(value); 3336 } 3337 return this; 3338 } 3339 3340 /** 3341 * @return {@link #contentType} (The content-type or mime-type to use for 3342 * RESTful operation in the 'Content-Type' header.). This is the 3343 * underlying object with id, value and extensions. The accessor 3344 * "getContentType" gives direct access to the value 3345 */ 3346 public Enumeration<ContentType> getContentTypeElement() { 3347 if (this.contentType == null) 3348 if (Configuration.errorOnAutoCreate()) 3349 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.contentType"); 3350 else if (Configuration.doAutoCreate()) 3351 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); // bb 3352 return this.contentType; 3353 } 3354 3355 public boolean hasContentTypeElement() { 3356 return this.contentType != null && !this.contentType.isEmpty(); 3357 } 3358 3359 public boolean hasContentType() { 3360 return this.contentType != null && !this.contentType.isEmpty(); 3361 } 3362 3363 /** 3364 * @param value {@link #contentType} (The content-type or mime-type to use for 3365 * RESTful operation in the 'Content-Type' header.). This is the 3366 * underlying object with id, value and extensions. The accessor 3367 * "getContentType" gives direct access to the value 3368 */ 3369 public TestScriptSetupActionOperationComponent setContentTypeElement(Enumeration<ContentType> value) { 3370 this.contentType = value; 3371 return this; 3372 } 3373 3374 /** 3375 * @return The content-type or mime-type to use for RESTful operation in the 3376 * 'Content-Type' header. 3377 */ 3378 public ContentType getContentType() { 3379 return this.contentType == null ? null : this.contentType.getValue(); 3380 } 3381 3382 /** 3383 * @param value The content-type or mime-type to use for RESTful operation in 3384 * the 'Content-Type' header. 3385 */ 3386 public TestScriptSetupActionOperationComponent setContentType(ContentType value) { 3387 if (value == null) 3388 this.contentType = null; 3389 else { 3390 if (this.contentType == null) 3391 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); 3392 this.contentType.setValue(value); 3393 } 3394 return this; 3395 } 3396 3397 /** 3398 * @return {@link #destination} (Which server to perform the operation on.). 3399 * This is the underlying object with id, value and extensions. The 3400 * accessor "getDestination" gives direct access to the value 3401 */ 3402 public IntegerType getDestinationElement() { 3403 if (this.destination == null) 3404 if (Configuration.errorOnAutoCreate()) 3405 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.destination"); 3406 else if (Configuration.doAutoCreate()) 3407 this.destination = new IntegerType(); // bb 3408 return this.destination; 3409 } 3410 3411 public boolean hasDestinationElement() { 3412 return this.destination != null && !this.destination.isEmpty(); 3413 } 3414 3415 public boolean hasDestination() { 3416 return this.destination != null && !this.destination.isEmpty(); 3417 } 3418 3419 /** 3420 * @param value {@link #destination} (Which server to perform the operation 3421 * on.). This is the underlying object with id, value and 3422 * extensions. The accessor "getDestination" gives direct access to 3423 * the value 3424 */ 3425 public TestScriptSetupActionOperationComponent setDestinationElement(IntegerType value) { 3426 this.destination = value; 3427 return this; 3428 } 3429 3430 /** 3431 * @return Which server to perform the operation on. 3432 */ 3433 public int getDestination() { 3434 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 3435 } 3436 3437 /** 3438 * @param value Which server to perform the operation on. 3439 */ 3440 public TestScriptSetupActionOperationComponent setDestination(int value) { 3441 if (this.destination == null) 3442 this.destination = new IntegerType(); 3443 this.destination.setValue(value); 3444 return this; 3445 } 3446 3447 /** 3448 * @return {@link #encodeRequestUrl} (Whether or not to implicitly send the 3449 * request url in encoded format. The default is true to match the 3450 * standard RESTful client behavior. Set to false when communicating 3451 * with a server that does not support encoded url paths.). This is the 3452 * underlying object with id, value and extensions. The accessor 3453 * "getEncodeRequestUrl" gives direct access to the value 3454 */ 3455 public BooleanType getEncodeRequestUrlElement() { 3456 if (this.encodeRequestUrl == null) 3457 if (Configuration.errorOnAutoCreate()) 3458 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.encodeRequestUrl"); 3459 else if (Configuration.doAutoCreate()) 3460 this.encodeRequestUrl = new BooleanType(); // bb 3461 return this.encodeRequestUrl; 3462 } 3463 3464 public boolean hasEncodeRequestUrlElement() { 3465 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 3466 } 3467 3468 public boolean hasEncodeRequestUrl() { 3469 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 3470 } 3471 3472 /** 3473 * @param value {@link #encodeRequestUrl} (Whether or not to implicitly send the 3474 * request url in encoded format. The default is true to match the 3475 * standard RESTful client behavior. Set to false when 3476 * communicating with a server that does not support encoded url 3477 * paths.). This is the underlying object with id, value and 3478 * extensions. The accessor "getEncodeRequestUrl" gives direct 3479 * access to the value 3480 */ 3481 public TestScriptSetupActionOperationComponent setEncodeRequestUrlElement(BooleanType value) { 3482 this.encodeRequestUrl = value; 3483 return this; 3484 } 3485 3486 /** 3487 * @return Whether or not to implicitly send the request url in encoded format. 3488 * The default is true to match the standard RESTful client behavior. 3489 * Set to false when communicating with a server that does not support 3490 * encoded url paths. 3491 */ 3492 public boolean getEncodeRequestUrl() { 3493 return this.encodeRequestUrl == null || this.encodeRequestUrl.isEmpty() ? false 3494 : this.encodeRequestUrl.getValue(); 3495 } 3496 3497 /** 3498 * @param value Whether or not to implicitly send the request url in encoded 3499 * format. The default is true to match the standard RESTful client 3500 * behavior. Set to false when communicating with a server that 3501 * does not support encoded url paths. 3502 */ 3503 public TestScriptSetupActionOperationComponent setEncodeRequestUrl(boolean value) { 3504 if (this.encodeRequestUrl == null) 3505 this.encodeRequestUrl = new BooleanType(); 3506 this.encodeRequestUrl.setValue(value); 3507 return this; 3508 } 3509 3510 /** 3511 * @return {@link #params} (Path plus parameters after [type]. Used to set parts 3512 * of the request URL explicitly.). This is the underlying object with 3513 * id, value and extensions. The accessor "getParams" gives direct 3514 * access to the value 3515 */ 3516 public StringType getParamsElement() { 3517 if (this.params == null) 3518 if (Configuration.errorOnAutoCreate()) 3519 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.params"); 3520 else if (Configuration.doAutoCreate()) 3521 this.params = new StringType(); // bb 3522 return this.params; 3523 } 3524 3525 public boolean hasParamsElement() { 3526 return this.params != null && !this.params.isEmpty(); 3527 } 3528 3529 public boolean hasParams() { 3530 return this.params != null && !this.params.isEmpty(); 3531 } 3532 3533 /** 3534 * @param value {@link #params} (Path plus parameters after [type]. Used to set 3535 * parts of the request URL explicitly.). This is the underlying 3536 * object with id, value and extensions. The accessor "getParams" 3537 * gives direct access to the value 3538 */ 3539 public TestScriptSetupActionOperationComponent setParamsElement(StringType value) { 3540 this.params = value; 3541 return this; 3542 } 3543 3544 /** 3545 * @return Path plus parameters after [type]. Used to set parts of the request 3546 * URL explicitly. 3547 */ 3548 public String getParams() { 3549 return this.params == null ? null : this.params.getValue(); 3550 } 3551 3552 /** 3553 * @param value Path plus parameters after [type]. Used to set parts of the 3554 * request URL explicitly. 3555 */ 3556 public TestScriptSetupActionOperationComponent setParams(String value) { 3557 if (Utilities.noString(value)) 3558 this.params = null; 3559 else { 3560 if (this.params == null) 3561 this.params = new StringType(); 3562 this.params.setValue(value); 3563 } 3564 return this; 3565 } 3566 3567 /** 3568 * @return {@link #requestHeader} (Header elements would be used to set HTTP 3569 * headers.) 3570 */ 3571 public List<TestScriptSetupActionOperationRequestHeaderComponent> getRequestHeader() { 3572 if (this.requestHeader == null) 3573 this.requestHeader = new ArrayList<TestScriptSetupActionOperationRequestHeaderComponent>(); 3574 return this.requestHeader; 3575 } 3576 3577 public boolean hasRequestHeader() { 3578 if (this.requestHeader == null) 3579 return false; 3580 for (TestScriptSetupActionOperationRequestHeaderComponent item : this.requestHeader) 3581 if (!item.isEmpty()) 3582 return true; 3583 return false; 3584 } 3585 3586 /** 3587 * @return {@link #requestHeader} (Header elements would be used to set HTTP 3588 * headers.) 3589 */ 3590 // syntactic sugar 3591 public TestScriptSetupActionOperationRequestHeaderComponent addRequestHeader() { // 3 3592 TestScriptSetupActionOperationRequestHeaderComponent t = new TestScriptSetupActionOperationRequestHeaderComponent(); 3593 if (this.requestHeader == null) 3594 this.requestHeader = new ArrayList<TestScriptSetupActionOperationRequestHeaderComponent>(); 3595 this.requestHeader.add(t); 3596 return t; 3597 } 3598 3599 // syntactic sugar 3600 public TestScriptSetupActionOperationComponent addRequestHeader( 3601 TestScriptSetupActionOperationRequestHeaderComponent t) { // 3 3602 if (t == null) 3603 return this; 3604 if (this.requestHeader == null) 3605 this.requestHeader = new ArrayList<TestScriptSetupActionOperationRequestHeaderComponent>(); 3606 this.requestHeader.add(t); 3607 return this; 3608 } 3609 3610 /** 3611 * @return {@link #responseId} (The fixture id (maybe new) to map to the 3612 * response.). This is the underlying object with id, value and 3613 * extensions. The accessor "getResponseId" gives direct access to the 3614 * value 3615 */ 3616 public IdType getResponseIdElement() { 3617 if (this.responseId == null) 3618 if (Configuration.errorOnAutoCreate()) 3619 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.responseId"); 3620 else if (Configuration.doAutoCreate()) 3621 this.responseId = new IdType(); // bb 3622 return this.responseId; 3623 } 3624 3625 public boolean hasResponseIdElement() { 3626 return this.responseId != null && !this.responseId.isEmpty(); 3627 } 3628 3629 public boolean hasResponseId() { 3630 return this.responseId != null && !this.responseId.isEmpty(); 3631 } 3632 3633 /** 3634 * @param value {@link #responseId} (The fixture id (maybe new) to map to the 3635 * response.). This is the underlying object with id, value and 3636 * extensions. The accessor "getResponseId" gives direct access to 3637 * the value 3638 */ 3639 public TestScriptSetupActionOperationComponent setResponseIdElement(IdType value) { 3640 this.responseId = value; 3641 return this; 3642 } 3643 3644 /** 3645 * @return The fixture id (maybe new) to map to the response. 3646 */ 3647 public String getResponseId() { 3648 return this.responseId == null ? null : this.responseId.getValue(); 3649 } 3650 3651 /** 3652 * @param value The fixture id (maybe new) to map to the response. 3653 */ 3654 public TestScriptSetupActionOperationComponent setResponseId(String value) { 3655 if (Utilities.noString(value)) 3656 this.responseId = null; 3657 else { 3658 if (this.responseId == null) 3659 this.responseId = new IdType(); 3660 this.responseId.setValue(value); 3661 } 3662 return this; 3663 } 3664 3665 /** 3666 * @return {@link #sourceId} (The id of the fixture used as the body of a PUT or 3667 * POST request.). This is the underlying object with id, value and 3668 * extensions. The accessor "getSourceId" gives direct access to the 3669 * value 3670 */ 3671 public IdType getSourceIdElement() { 3672 if (this.sourceId == null) 3673 if (Configuration.errorOnAutoCreate()) 3674 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.sourceId"); 3675 else if (Configuration.doAutoCreate()) 3676 this.sourceId = new IdType(); // bb 3677 return this.sourceId; 3678 } 3679 3680 public boolean hasSourceIdElement() { 3681 return this.sourceId != null && !this.sourceId.isEmpty(); 3682 } 3683 3684 public boolean hasSourceId() { 3685 return this.sourceId != null && !this.sourceId.isEmpty(); 3686 } 3687 3688 /** 3689 * @param value {@link #sourceId} (The id of the fixture used as the body of a 3690 * PUT or POST request.). This is the underlying object with id, 3691 * value and extensions. The accessor "getSourceId" gives direct 3692 * access to the value 3693 */ 3694 public TestScriptSetupActionOperationComponent setSourceIdElement(IdType value) { 3695 this.sourceId = value; 3696 return this; 3697 } 3698 3699 /** 3700 * @return The id of the fixture used as the body of a PUT or POST request. 3701 */ 3702 public String getSourceId() { 3703 return this.sourceId == null ? null : this.sourceId.getValue(); 3704 } 3705 3706 /** 3707 * @param value The id of the fixture used as the body of a PUT or POST request. 3708 */ 3709 public TestScriptSetupActionOperationComponent setSourceId(String value) { 3710 if (Utilities.noString(value)) 3711 this.sourceId = null; 3712 else { 3713 if (this.sourceId == null) 3714 this.sourceId = new IdType(); 3715 this.sourceId.setValue(value); 3716 } 3717 return this; 3718 } 3719 3720 /** 3721 * @return {@link #targetId} (Id of fixture used for extracting the [id], 3722 * [type], and [vid] for GET requests.). This is the underlying object 3723 * with id, value and extensions. The accessor "getTargetId" gives 3724 * direct access to the value 3725 */ 3726 public IdType getTargetIdElement() { 3727 if (this.targetId == null) 3728 if (Configuration.errorOnAutoCreate()) 3729 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.targetId"); 3730 else if (Configuration.doAutoCreate()) 3731 this.targetId = new IdType(); // bb 3732 return this.targetId; 3733 } 3734 3735 public boolean hasTargetIdElement() { 3736 return this.targetId != null && !this.targetId.isEmpty(); 3737 } 3738 3739 public boolean hasTargetId() { 3740 return this.targetId != null && !this.targetId.isEmpty(); 3741 } 3742 3743 /** 3744 * @param value {@link #targetId} (Id of fixture used for extracting the [id], 3745 * [type], and [vid] for GET requests.). This is the underlying 3746 * object with id, value and extensions. The accessor "getTargetId" 3747 * gives direct access to the value 3748 */ 3749 public TestScriptSetupActionOperationComponent setTargetIdElement(IdType value) { 3750 this.targetId = value; 3751 return this; 3752 } 3753 3754 /** 3755 * @return Id of fixture used for extracting the [id], [type], and [vid] for GET 3756 * requests. 3757 */ 3758 public String getTargetId() { 3759 return this.targetId == null ? null : this.targetId.getValue(); 3760 } 3761 3762 /** 3763 * @param value Id of fixture used for extracting the [id], [type], and [vid] 3764 * for GET requests. 3765 */ 3766 public TestScriptSetupActionOperationComponent setTargetId(String value) { 3767 if (Utilities.noString(value)) 3768 this.targetId = null; 3769 else { 3770 if (this.targetId == null) 3771 this.targetId = new IdType(); 3772 this.targetId.setValue(value); 3773 } 3774 return this; 3775 } 3776 3777 /** 3778 * @return {@link #url} (Complete request URL.). This is the underlying object 3779 * with id, value and extensions. The accessor "getUrl" gives direct 3780 * access to the value 3781 */ 3782 public StringType getUrlElement() { 3783 if (this.url == null) 3784 if (Configuration.errorOnAutoCreate()) 3785 throw new Error("Attempt to auto-create TestScriptSetupActionOperationComponent.url"); 3786 else if (Configuration.doAutoCreate()) 3787 this.url = new StringType(); // bb 3788 return this.url; 3789 } 3790 3791 public boolean hasUrlElement() { 3792 return this.url != null && !this.url.isEmpty(); 3793 } 3794 3795 public boolean hasUrl() { 3796 return this.url != null && !this.url.isEmpty(); 3797 } 3798 3799 /** 3800 * @param value {@link #url} (Complete request URL.). This is the underlying 3801 * object with id, value and extensions. The accessor "getUrl" 3802 * gives direct access to the value 3803 */ 3804 public TestScriptSetupActionOperationComponent setUrlElement(StringType value) { 3805 this.url = value; 3806 return this; 3807 } 3808 3809 /** 3810 * @return Complete request URL. 3811 */ 3812 public String getUrl() { 3813 return this.url == null ? null : this.url.getValue(); 3814 } 3815 3816 /** 3817 * @param value Complete request URL. 3818 */ 3819 public TestScriptSetupActionOperationComponent setUrl(String value) { 3820 if (Utilities.noString(value)) 3821 this.url = null; 3822 else { 3823 if (this.url == null) 3824 this.url = new StringType(); 3825 this.url.setValue(value); 3826 } 3827 return this; 3828 } 3829 3830 protected void listChildren(List<Property> childrenList) { 3831 super.listChildren(childrenList); 3832 childrenList.add(new Property("type", "Coding", "Server interaction or operation type.", 0, 3833 java.lang.Integer.MAX_VALUE, type)); 3834 childrenList.add(new Property("resource", "code", 3835 "The type of the resource. See http://hl7-fhir.github.io/resourcelist.html.", 0, java.lang.Integer.MAX_VALUE, 3836 resource)); 3837 childrenList 3838 .add(new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 3839 0, java.lang.Integer.MAX_VALUE, label)); 3840 childrenList.add(new Property("description", "string", 3841 "The description would be used by test engines for tracking and reporting purposes.", 0, 3842 java.lang.Integer.MAX_VALUE, description)); 3843 childrenList.add(new Property("accept", "code", 3844 "The content-type or mime-type to use for RESTful operation in the 'Accept' header.", 0, 3845 java.lang.Integer.MAX_VALUE, accept)); 3846 childrenList.add(new Property("contentType", "code", 3847 "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 3848 java.lang.Integer.MAX_VALUE, contentType)); 3849 childrenList.add(new Property("destination", "integer", "Which server to perform the operation on.", 0, 3850 java.lang.Integer.MAX_VALUE, destination)); 3851 childrenList.add(new Property("encodeRequestUrl", "boolean", 3852 "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 3853 0, java.lang.Integer.MAX_VALUE, encodeRequestUrl)); 3854 childrenList.add(new Property("params", "string", 3855 "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 3856 java.lang.Integer.MAX_VALUE, params)); 3857 childrenList.add(new Property("requestHeader", "", "Header elements would be used to set HTTP headers.", 0, 3858 java.lang.Integer.MAX_VALUE, requestHeader)); 3859 childrenList.add(new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 0, 3860 java.lang.Integer.MAX_VALUE, responseId)); 3861 childrenList 3862 .add(new Property("sourceId", "id", "The id of the fixture used as the body of a PUT or POST request.", 0, 3863 java.lang.Integer.MAX_VALUE, sourceId)); 3864 childrenList.add(new Property("targetId", "id", 3865 "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 3866 java.lang.Integer.MAX_VALUE, targetId)); 3867 childrenList.add(new Property("url", "string", "Complete request URL.", 0, java.lang.Integer.MAX_VALUE, url)); 3868 } 3869 3870 @Override 3871 public void setProperty(String name, Base value) throws FHIRException { 3872 if (name.equals("type")) 3873 this.type = castToCoding(value); // Coding 3874 else if (name.equals("resource")) 3875 this.resource = castToCode(value); // CodeType 3876 else if (name.equals("label")) 3877 this.label = castToString(value); // StringType 3878 else if (name.equals("description")) 3879 this.description = castToString(value); // StringType 3880 else if (name.equals("accept")) 3881 this.accept = new ContentTypeEnumFactory().fromType(value); // Enumeration<ContentType> 3882 else if (name.equals("contentType")) 3883 this.contentType = new ContentTypeEnumFactory().fromType(value); // Enumeration<ContentType> 3884 else if (name.equals("destination")) 3885 this.destination = castToInteger(value); // IntegerType 3886 else if (name.equals("encodeRequestUrl")) 3887 this.encodeRequestUrl = castToBoolean(value); // BooleanType 3888 else if (name.equals("params")) 3889 this.params = castToString(value); // StringType 3890 else if (name.equals("requestHeader")) 3891 this.getRequestHeader().add((TestScriptSetupActionOperationRequestHeaderComponent) value); 3892 else if (name.equals("responseId")) 3893 this.responseId = castToId(value); // IdType 3894 else if (name.equals("sourceId")) 3895 this.sourceId = castToId(value); // IdType 3896 else if (name.equals("targetId")) 3897 this.targetId = castToId(value); // IdType 3898 else if (name.equals("url")) 3899 this.url = castToString(value); // StringType 3900 else 3901 super.setProperty(name, value); 3902 } 3903 3904 @Override 3905 public Base addChild(String name) throws FHIRException { 3906 if (name.equals("type")) { 3907 this.type = new Coding(); 3908 return this.type; 3909 } else if (name.equals("resource")) { 3910 throw new FHIRException("Cannot call addChild on a singleton property TestScript.resource"); 3911 } else if (name.equals("label")) { 3912 throw new FHIRException("Cannot call addChild on a singleton property TestScript.label"); 3913 } else if (name.equals("description")) { 3914 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 3915 } else if (name.equals("accept")) { 3916 throw new FHIRException("Cannot call addChild on a singleton property TestScript.accept"); 3917 } else if (name.equals("contentType")) { 3918 throw new FHIRException("Cannot call addChild on a singleton property TestScript.contentType"); 3919 } else if (name.equals("destination")) { 3920 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination"); 3921 } else if (name.equals("encodeRequestUrl")) { 3922 throw new FHIRException("Cannot call addChild on a singleton property TestScript.encodeRequestUrl"); 3923 } else if (name.equals("params")) { 3924 throw new FHIRException("Cannot call addChild on a singleton property TestScript.params"); 3925 } else if (name.equals("requestHeader")) { 3926 return addRequestHeader(); 3927 } else if (name.equals("responseId")) { 3928 throw new FHIRException("Cannot call addChild on a singleton property TestScript.responseId"); 3929 } else if (name.equals("sourceId")) { 3930 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 3931 } else if (name.equals("targetId")) { 3932 throw new FHIRException("Cannot call addChild on a singleton property TestScript.targetId"); 3933 } else if (name.equals("url")) { 3934 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 3935 } else 3936 return super.addChild(name); 3937 } 3938 3939 public TestScriptSetupActionOperationComponent copy() { 3940 TestScriptSetupActionOperationComponent dst = new TestScriptSetupActionOperationComponent(); 3941 copyValues(dst); 3942 dst.type = type == null ? null : type.copy(); 3943 dst.resource = resource == null ? null : resource.copy(); 3944 dst.label = label == null ? null : label.copy(); 3945 dst.description = description == null ? null : description.copy(); 3946 dst.accept = accept == null ? null : accept.copy(); 3947 dst.contentType = contentType == null ? null : contentType.copy(); 3948 dst.destination = destination == null ? null : destination.copy(); 3949 dst.encodeRequestUrl = encodeRequestUrl == null ? null : encodeRequestUrl.copy(); 3950 dst.params = params == null ? null : params.copy(); 3951 if (requestHeader != null) { 3952 dst.requestHeader = new ArrayList<TestScriptSetupActionOperationRequestHeaderComponent>(); 3953 for (TestScriptSetupActionOperationRequestHeaderComponent i : requestHeader) 3954 dst.requestHeader.add(i.copy()); 3955 } 3956 ; 3957 dst.responseId = responseId == null ? null : responseId.copy(); 3958 dst.sourceId = sourceId == null ? null : sourceId.copy(); 3959 dst.targetId = targetId == null ? null : targetId.copy(); 3960 dst.url = url == null ? null : url.copy(); 3961 return dst; 3962 } 3963 3964 @Override 3965 public boolean equalsDeep(Base other) { 3966 if (!super.equalsDeep(other)) 3967 return false; 3968 if (!(other instanceof TestScriptSetupActionOperationComponent)) 3969 return false; 3970 TestScriptSetupActionOperationComponent o = (TestScriptSetupActionOperationComponent) other; 3971 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true) 3972 && compareDeep(label, o.label, true) && compareDeep(description, o.description, true) 3973 && compareDeep(accept, o.accept, true) && compareDeep(contentType, o.contentType, true) 3974 && compareDeep(destination, o.destination, true) && compareDeep(encodeRequestUrl, o.encodeRequestUrl, true) 3975 && compareDeep(params, o.params, true) && compareDeep(requestHeader, o.requestHeader, true) 3976 && compareDeep(responseId, o.responseId, true) && compareDeep(sourceId, o.sourceId, true) 3977 && compareDeep(targetId, o.targetId, true) && compareDeep(url, o.url, true); 3978 } 3979 3980 @Override 3981 public boolean equalsShallow(Base other) { 3982 if (!super.equalsShallow(other)) 3983 return false; 3984 if (!(other instanceof TestScriptSetupActionOperationComponent)) 3985 return false; 3986 TestScriptSetupActionOperationComponent o = (TestScriptSetupActionOperationComponent) other; 3987 return compareValues(resource, o.resource, true) && compareValues(label, o.label, true) 3988 && compareValues(description, o.description, true) && compareValues(accept, o.accept, true) 3989 && compareValues(contentType, o.contentType, true) && compareValues(destination, o.destination, true) 3990 && compareValues(encodeRequestUrl, o.encodeRequestUrl, true) && compareValues(params, o.params, true) 3991 && compareValues(responseId, o.responseId, true) && compareValues(sourceId, o.sourceId, true) 3992 && compareValues(targetId, o.targetId, true) && compareValues(url, o.url, true); 3993 } 3994 3995 public boolean isEmpty() { 3996 return super.isEmpty() && (type == null || type.isEmpty()) && (resource == null || resource.isEmpty()) 3997 && (label == null || label.isEmpty()) && (description == null || description.isEmpty()) 3998 && (accept == null || accept.isEmpty()) && (contentType == null || contentType.isEmpty()) 3999 && (destination == null || destination.isEmpty()) && (encodeRequestUrl == null || encodeRequestUrl.isEmpty()) 4000 && (params == null || params.isEmpty()) && (requestHeader == null || requestHeader.isEmpty()) 4001 && (responseId == null || responseId.isEmpty()) && (sourceId == null || sourceId.isEmpty()) 4002 && (targetId == null || targetId.isEmpty()) && (url == null || url.isEmpty()); 4003 } 4004 4005 public String fhirType() { 4006 return "TestScript.setup.action.operation"; 4007 4008 } 4009 4010 } 4011 4012 @Block() 4013 public static class TestScriptSetupActionOperationRequestHeaderComponent extends BackboneElement 4014 implements IBaseBackboneElement { 4015 /** 4016 * The HTTP header field e.g. "Accept". 4017 */ 4018 @Child(name = "field", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4019 @Description(shortDefinition = "HTTP header field name", formalDefinition = "The HTTP header field e.g. \"Accept\".") 4020 protected StringType field; 4021 4022 /** 4023 * The value of the header e.g. "application/xml". 4024 */ 4025 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4026 @Description(shortDefinition = "HTTP headerfield value", formalDefinition = "The value of the header e.g. \"application/xml\".") 4027 protected StringType value; 4028 4029 private static final long serialVersionUID = 274395337L; 4030 4031 /* 4032 * Constructor 4033 */ 4034 public TestScriptSetupActionOperationRequestHeaderComponent() { 4035 super(); 4036 } 4037 4038 /* 4039 * Constructor 4040 */ 4041 public TestScriptSetupActionOperationRequestHeaderComponent(StringType field, StringType value) { 4042 super(); 4043 this.field = field; 4044 this.value = value; 4045 } 4046 4047 /** 4048 * @return {@link #field} (The HTTP header field e.g. "Accept".). This is the 4049 * underlying object with id, value and extensions. The accessor 4050 * "getField" gives direct access to the value 4051 */ 4052 public StringType getFieldElement() { 4053 if (this.field == null) 4054 if (Configuration.errorOnAutoCreate()) 4055 throw new Error("Attempt to auto-create TestScriptSetupActionOperationRequestHeaderComponent.field"); 4056 else if (Configuration.doAutoCreate()) 4057 this.field = new StringType(); // bb 4058 return this.field; 4059 } 4060 4061 public boolean hasFieldElement() { 4062 return this.field != null && !this.field.isEmpty(); 4063 } 4064 4065 public boolean hasField() { 4066 return this.field != null && !this.field.isEmpty(); 4067 } 4068 4069 /** 4070 * @param value {@link #field} (The HTTP header field e.g. "Accept".). This is 4071 * the underlying object with id, value and extensions. The 4072 * accessor "getField" gives direct access to the value 4073 */ 4074 public TestScriptSetupActionOperationRequestHeaderComponent setFieldElement(StringType value) { 4075 this.field = value; 4076 return this; 4077 } 4078 4079 /** 4080 * @return The HTTP header field e.g. "Accept". 4081 */ 4082 public String getField() { 4083 return this.field == null ? null : this.field.getValue(); 4084 } 4085 4086 /** 4087 * @param value The HTTP header field e.g. "Accept". 4088 */ 4089 public TestScriptSetupActionOperationRequestHeaderComponent setField(String value) { 4090 if (this.field == null) 4091 this.field = new StringType(); 4092 this.field.setValue(value); 4093 return this; 4094 } 4095 4096 /** 4097 * @return {@link #value} (The value of the header e.g. "application/xml".). 4098 * This is the underlying object with id, value and extensions. The 4099 * accessor "getValue" gives direct access to the value 4100 */ 4101 public StringType getValueElement() { 4102 if (this.value == null) 4103 if (Configuration.errorOnAutoCreate()) 4104 throw new Error("Attempt to auto-create TestScriptSetupActionOperationRequestHeaderComponent.value"); 4105 else if (Configuration.doAutoCreate()) 4106 this.value = new StringType(); // bb 4107 return this.value; 4108 } 4109 4110 public boolean hasValueElement() { 4111 return this.value != null && !this.value.isEmpty(); 4112 } 4113 4114 public boolean hasValue() { 4115 return this.value != null && !this.value.isEmpty(); 4116 } 4117 4118 /** 4119 * @param value {@link #value} (The value of the header e.g. 4120 * "application/xml".). This is the underlying object with id, 4121 * value and extensions. The accessor "getValue" gives direct 4122 * access to the value 4123 */ 4124 public TestScriptSetupActionOperationRequestHeaderComponent setValueElement(StringType value) { 4125 this.value = value; 4126 return this; 4127 } 4128 4129 /** 4130 * @return The value of the header e.g. "application/xml". 4131 */ 4132 public String getValue() { 4133 return this.value == null ? null : this.value.getValue(); 4134 } 4135 4136 /** 4137 * @param value The value of the header e.g. "application/xml". 4138 */ 4139 public TestScriptSetupActionOperationRequestHeaderComponent setValue(String value) { 4140 if (this.value == null) 4141 this.value = new StringType(); 4142 this.value.setValue(value); 4143 return this; 4144 } 4145 4146 protected void listChildren(List<Property> childrenList) { 4147 super.listChildren(childrenList); 4148 childrenList.add(new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 4149 java.lang.Integer.MAX_VALUE, field)); 4150 childrenList.add(new Property("value", "string", "The value of the header e.g. \"application/xml\".", 0, 4151 java.lang.Integer.MAX_VALUE, value)); 4152 } 4153 4154 @Override 4155 public void setProperty(String name, Base value) throws FHIRException { 4156 if (name.equals("field")) 4157 this.field = castToString(value); // StringType 4158 else if (name.equals("value")) 4159 this.value = castToString(value); // StringType 4160 else 4161 super.setProperty(name, value); 4162 } 4163 4164 @Override 4165 public Base addChild(String name) throws FHIRException { 4166 if (name.equals("field")) { 4167 throw new FHIRException("Cannot call addChild on a singleton property TestScript.field"); 4168 } else if (name.equals("value")) { 4169 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 4170 } else 4171 return super.addChild(name); 4172 } 4173 4174 public TestScriptSetupActionOperationRequestHeaderComponent copy() { 4175 TestScriptSetupActionOperationRequestHeaderComponent dst = new TestScriptSetupActionOperationRequestHeaderComponent(); 4176 copyValues(dst); 4177 dst.field = field == null ? null : field.copy(); 4178 dst.value = value == null ? null : value.copy(); 4179 return dst; 4180 } 4181 4182 @Override 4183 public boolean equalsDeep(Base other) { 4184 if (!super.equalsDeep(other)) 4185 return false; 4186 if (!(other instanceof TestScriptSetupActionOperationRequestHeaderComponent)) 4187 return false; 4188 TestScriptSetupActionOperationRequestHeaderComponent o = (TestScriptSetupActionOperationRequestHeaderComponent) other; 4189 return compareDeep(field, o.field, true) && compareDeep(value, o.value, true); 4190 } 4191 4192 @Override 4193 public boolean equalsShallow(Base other) { 4194 if (!super.equalsShallow(other)) 4195 return false; 4196 if (!(other instanceof TestScriptSetupActionOperationRequestHeaderComponent)) 4197 return false; 4198 TestScriptSetupActionOperationRequestHeaderComponent o = (TestScriptSetupActionOperationRequestHeaderComponent) other; 4199 return compareValues(field, o.field, true) && compareValues(value, o.value, true); 4200 } 4201 4202 public boolean isEmpty() { 4203 return super.isEmpty() && (field == null || field.isEmpty()) && (value == null || value.isEmpty()); 4204 } 4205 4206 public String fhirType() { 4207 return "TestScript.setup.action.operation.requestHeader"; 4208 4209 } 4210 4211 } 4212 4213 @Block() 4214 public static class TestScriptSetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 4215 /** 4216 * The label would be used for tracking/logging purposes by test engines. 4217 */ 4218 @Child(name = "label", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 4219 @Description(shortDefinition = "Tracking/logging assertion label", formalDefinition = "The label would be used for tracking/logging purposes by test engines.") 4220 protected StringType label; 4221 4222 /** 4223 * The description would be used by test engines for tracking and reporting 4224 * purposes. 4225 */ 4226 @Child(name = "description", type = { 4227 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4228 @Description(shortDefinition = "Tracking/reporting assertion description", formalDefinition = "The description would be used by test engines for tracking and reporting purposes.") 4229 protected StringType description; 4230 4231 /** 4232 * The direction to use for the assertion. 4233 */ 4234 @Child(name = "direction", type = { 4235 CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4236 @Description(shortDefinition = "response | request", formalDefinition = "The direction to use for the assertion.") 4237 protected Enumeration<AssertionDirectionType> direction; 4238 4239 /** 4240 * Id of fixture used to compare the "sourceId/path" evaluations to. 4241 */ 4242 @Child(name = "compareToSourceId", type = { 4243 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 4244 @Description(shortDefinition = "Id of fixture used to compare the \"sourceId/path\" evaluations to", formalDefinition = "Id of fixture used to compare the \"sourceId/path\" evaluations to.") 4245 protected StringType compareToSourceId; 4246 4247 /** 4248 * XPath or JSONPath expression against fixture used to compare the 4249 * "sourceId/path" evaluations to. 4250 */ 4251 @Child(name = "compareToSourcePath", type = { 4252 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4253 @Description(shortDefinition = "XPath or JSONPath expression against fixture used to compare the \"sourceId/path\" evaluations to", formalDefinition = "XPath or JSONPath expression against fixture used to compare the \"sourceId/path\" evaluations to.") 4254 protected StringType compareToSourcePath; 4255 4256 /** 4257 * The content-type or mime-type to use for RESTful operation in the 4258 * 'Content-Type' header. 4259 */ 4260 @Child(name = "contentType", type = { 4261 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4262 @Description(shortDefinition = "xml | json", formalDefinition = "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.") 4263 protected Enumeration<ContentType> contentType; 4264 4265 /** 4266 * The HTTP header field name e.g. 'Location'. 4267 */ 4268 @Child(name = "headerField", type = { 4269 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4270 @Description(shortDefinition = "HTTP header field name", formalDefinition = "The HTTP header field name e.g. 'Location'.") 4271 protected StringType headerField; 4272 4273 /** 4274 * The ID of a fixture. Asserts that the response contains at a minimumId the 4275 * fixture specified by minimumId. 4276 */ 4277 @Child(name = "minimumId", type = { 4278 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 4279 @Description(shortDefinition = "Fixture Id of minimum content resource", formalDefinition = "The ID of a fixture. Asserts that the response contains at a minimumId the fixture specified by minimumId.") 4280 protected StringType minimumId; 4281 4282 /** 4283 * Whether or not the test execution performs validation on the bundle 4284 * navigation links. 4285 */ 4286 @Child(name = "navigationLinks", type = { 4287 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 4288 @Description(shortDefinition = "Perform validation on navigation links?", formalDefinition = "Whether or not the test execution performs validation on the bundle navigation links.") 4289 protected BooleanType navigationLinks; 4290 4291 /** 4292 * The operator type. 4293 */ 4294 @Child(name = "operator", type = { 4295 CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 4296 @Description(shortDefinition = "equals | notEquals | in | notIn | greaterThan | lessThan | empty | notEmpty | contains | notContains", formalDefinition = "The operator type.") 4297 protected Enumeration<AssertionOperatorType> operator; 4298 4299 /** 4300 * The XPath or JSONPath expression to be evaluated against the fixture 4301 * representing the response received from server. 4302 */ 4303 @Child(name = "path", type = { StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4304 @Description(shortDefinition = "XPath or JSONPath expression", formalDefinition = "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.") 4305 protected StringType path; 4306 4307 /** 4308 * The type of the resource. See http://hl7-fhir.github.io/resourcelist.html. 4309 */ 4310 @Child(name = "resource", type = { 4311 CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 4312 @Description(shortDefinition = "Resource type", formalDefinition = "The type of the resource. See http://hl7-fhir.github.io/resourcelist.html.") 4313 protected CodeType resource; 4314 4315 /** 4316 * okay | created | noContent | notModified | bad | forbidden | notFound | 4317 * methodNotAllowed | conflict | gone | preconditionFailed | unprocessable. 4318 */ 4319 @Child(name = "response", type = { 4320 CodeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4321 @Description(shortDefinition = "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable", formalDefinition = "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.") 4322 protected Enumeration<AssertionResponseTypes> response; 4323 4324 /** 4325 * The value of the HTTP response code to be tested. 4326 */ 4327 @Child(name = "responseCode", type = { 4328 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 4329 @Description(shortDefinition = "HTTP response code to test", formalDefinition = "The value of the HTTP response code to be tested.") 4330 protected StringType responseCode; 4331 4332 /** 4333 * Fixture to evaluate the XPath/JSONPath expression or the headerField against. 4334 */ 4335 @Child(name = "sourceId", type = { IdType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 4336 @Description(shortDefinition = "Fixture Id of source expression or headerField", formalDefinition = "Fixture to evaluate the XPath/JSONPath expression or the headerField against.") 4337 protected IdType sourceId; 4338 4339 /** 4340 * The ID of the Profile to validate against. 4341 */ 4342 @Child(name = "validateProfileId", type = { 4343 IdType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 4344 @Description(shortDefinition = "Profile Id of validation profile reference", formalDefinition = "The ID of the Profile to validate against.") 4345 protected IdType validateProfileId; 4346 4347 /** 4348 * The value to compare to. 4349 */ 4350 @Child(name = "value", type = { StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 4351 @Description(shortDefinition = "The value to compare to", formalDefinition = "The value to compare to.") 4352 protected StringType value; 4353 4354 /** 4355 * Whether or not the test execution will produce a warning only on error for 4356 * this assert. 4357 */ 4358 @Child(name = "warningOnly", type = { 4359 BooleanType.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 4360 @Description(shortDefinition = "Will this assert produce a warning only on error?", formalDefinition = "Whether or not the test execution will produce a warning only on error for this assert.") 4361 protected BooleanType warningOnly; 4362 4363 private static final long serialVersionUID = -607939856L; 4364 4365 /* 4366 * Constructor 4367 */ 4368 public TestScriptSetupActionAssertComponent() { 4369 super(); 4370 } 4371 4372 /** 4373 * @return {@link #label} (The label would be used for tracking/logging purposes 4374 * by test engines.). This is the underlying object with id, value and 4375 * extensions. The accessor "getLabel" gives direct access to the value 4376 */ 4377 public StringType getLabelElement() { 4378 if (this.label == null) 4379 if (Configuration.errorOnAutoCreate()) 4380 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.label"); 4381 else if (Configuration.doAutoCreate()) 4382 this.label = new StringType(); // bb 4383 return this.label; 4384 } 4385 4386 public boolean hasLabelElement() { 4387 return this.label != null && !this.label.isEmpty(); 4388 } 4389 4390 public boolean hasLabel() { 4391 return this.label != null && !this.label.isEmpty(); 4392 } 4393 4394 /** 4395 * @param value {@link #label} (The label would be used for tracking/logging 4396 * purposes by test engines.). This is the underlying object with 4397 * id, value and extensions. The accessor "getLabel" gives direct 4398 * access to the value 4399 */ 4400 public TestScriptSetupActionAssertComponent setLabelElement(StringType value) { 4401 this.label = value; 4402 return this; 4403 } 4404 4405 /** 4406 * @return The label would be used for tracking/logging purposes by test 4407 * engines. 4408 */ 4409 public String getLabel() { 4410 return this.label == null ? null : this.label.getValue(); 4411 } 4412 4413 /** 4414 * @param value The label would be used for tracking/logging purposes by test 4415 * engines. 4416 */ 4417 public TestScriptSetupActionAssertComponent setLabel(String value) { 4418 if (Utilities.noString(value)) 4419 this.label = null; 4420 else { 4421 if (this.label == null) 4422 this.label = new StringType(); 4423 this.label.setValue(value); 4424 } 4425 return this; 4426 } 4427 4428 /** 4429 * @return {@link #description} (The description would be used by test engines 4430 * for tracking and reporting purposes.). This is the underlying object 4431 * with id, value and extensions. The accessor "getDescription" gives 4432 * direct access to the value 4433 */ 4434 public StringType getDescriptionElement() { 4435 if (this.description == null) 4436 if (Configuration.errorOnAutoCreate()) 4437 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.description"); 4438 else if (Configuration.doAutoCreate()) 4439 this.description = new StringType(); // bb 4440 return this.description; 4441 } 4442 4443 public boolean hasDescriptionElement() { 4444 return this.description != null && !this.description.isEmpty(); 4445 } 4446 4447 public boolean hasDescription() { 4448 return this.description != null && !this.description.isEmpty(); 4449 } 4450 4451 /** 4452 * @param value {@link #description} (The description would be used by test 4453 * engines for tracking and reporting purposes.). This is the 4454 * underlying object with id, value and extensions. The accessor 4455 * "getDescription" gives direct access to the value 4456 */ 4457 public TestScriptSetupActionAssertComponent setDescriptionElement(StringType value) { 4458 this.description = value; 4459 return this; 4460 } 4461 4462 /** 4463 * @return The description would be used by test engines for tracking and 4464 * reporting purposes. 4465 */ 4466 public String getDescription() { 4467 return this.description == null ? null : this.description.getValue(); 4468 } 4469 4470 /** 4471 * @param value The description would be used by test engines for tracking and 4472 * reporting purposes. 4473 */ 4474 public TestScriptSetupActionAssertComponent setDescription(String value) { 4475 if (Utilities.noString(value)) 4476 this.description = null; 4477 else { 4478 if (this.description == null) 4479 this.description = new StringType(); 4480 this.description.setValue(value); 4481 } 4482 return this; 4483 } 4484 4485 /** 4486 * @return {@link #direction} (The direction to use for the assertion.). This is 4487 * the underlying object with id, value and extensions. The accessor 4488 * "getDirection" gives direct access to the value 4489 */ 4490 public Enumeration<AssertionDirectionType> getDirectionElement() { 4491 if (this.direction == null) 4492 if (Configuration.errorOnAutoCreate()) 4493 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.direction"); 4494 else if (Configuration.doAutoCreate()) 4495 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); // bb 4496 return this.direction; 4497 } 4498 4499 public boolean hasDirectionElement() { 4500 return this.direction != null && !this.direction.isEmpty(); 4501 } 4502 4503 public boolean hasDirection() { 4504 return this.direction != null && !this.direction.isEmpty(); 4505 } 4506 4507 /** 4508 * @param value {@link #direction} (The direction to use for the assertion.). 4509 * This is the underlying object with id, value and extensions. The 4510 * accessor "getDirection" gives direct access to the value 4511 */ 4512 public TestScriptSetupActionAssertComponent setDirectionElement(Enumeration<AssertionDirectionType> value) { 4513 this.direction = value; 4514 return this; 4515 } 4516 4517 /** 4518 * @return The direction to use for the assertion. 4519 */ 4520 public AssertionDirectionType getDirection() { 4521 return this.direction == null ? null : this.direction.getValue(); 4522 } 4523 4524 /** 4525 * @param value The direction to use for the assertion. 4526 */ 4527 public TestScriptSetupActionAssertComponent setDirection(AssertionDirectionType value) { 4528 if (value == null) 4529 this.direction = null; 4530 else { 4531 if (this.direction == null) 4532 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); 4533 this.direction.setValue(value); 4534 } 4535 return this; 4536 } 4537 4538 /** 4539 * @return {@link #compareToSourceId} (Id of fixture used to compare the 4540 * "sourceId/path" evaluations to.). This is the underlying object with 4541 * id, value and extensions. The accessor "getCompareToSourceId" gives 4542 * direct access to the value 4543 */ 4544 public StringType getCompareToSourceIdElement() { 4545 if (this.compareToSourceId == null) 4546 if (Configuration.errorOnAutoCreate()) 4547 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.compareToSourceId"); 4548 else if (Configuration.doAutoCreate()) 4549 this.compareToSourceId = new StringType(); // bb 4550 return this.compareToSourceId; 4551 } 4552 4553 public boolean hasCompareToSourceIdElement() { 4554 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 4555 } 4556 4557 public boolean hasCompareToSourceId() { 4558 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 4559 } 4560 4561 /** 4562 * @param value {@link #compareToSourceId} (Id of fixture used to compare the 4563 * "sourceId/path" evaluations to.). This is the underlying object 4564 * with id, value and extensions. The accessor 4565 * "getCompareToSourceId" gives direct access to the value 4566 */ 4567 public TestScriptSetupActionAssertComponent setCompareToSourceIdElement(StringType value) { 4568 this.compareToSourceId = value; 4569 return this; 4570 } 4571 4572 /** 4573 * @return Id of fixture used to compare the "sourceId/path" evaluations to. 4574 */ 4575 public String getCompareToSourceId() { 4576 return this.compareToSourceId == null ? null : this.compareToSourceId.getValue(); 4577 } 4578 4579 /** 4580 * @param value Id of fixture used to compare the "sourceId/path" evaluations 4581 * to. 4582 */ 4583 public TestScriptSetupActionAssertComponent setCompareToSourceId(String value) { 4584 if (Utilities.noString(value)) 4585 this.compareToSourceId = null; 4586 else { 4587 if (this.compareToSourceId == null) 4588 this.compareToSourceId = new StringType(); 4589 this.compareToSourceId.setValue(value); 4590 } 4591 return this; 4592 } 4593 4594 /** 4595 * @return {@link #compareToSourcePath} (XPath or JSONPath expression against 4596 * fixture used to compare the "sourceId/path" evaluations to.). This is 4597 * the underlying object with id, value and extensions. The accessor 4598 * "getCompareToSourcePath" gives direct access to the value 4599 */ 4600 public StringType getCompareToSourcePathElement() { 4601 if (this.compareToSourcePath == null) 4602 if (Configuration.errorOnAutoCreate()) 4603 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.compareToSourcePath"); 4604 else if (Configuration.doAutoCreate()) 4605 this.compareToSourcePath = new StringType(); // bb 4606 return this.compareToSourcePath; 4607 } 4608 4609 public boolean hasCompareToSourcePathElement() { 4610 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 4611 } 4612 4613 public boolean hasCompareToSourcePath() { 4614 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 4615 } 4616 4617 /** 4618 * @param value {@link #compareToSourcePath} (XPath or JSONPath expression 4619 * against fixture used to compare the "sourceId/path" evaluations 4620 * to.). This is the underlying object with id, value and 4621 * extensions. The accessor "getCompareToSourcePath" gives direct 4622 * access to the value 4623 */ 4624 public TestScriptSetupActionAssertComponent setCompareToSourcePathElement(StringType value) { 4625 this.compareToSourcePath = value; 4626 return this; 4627 } 4628 4629 /** 4630 * @return XPath or JSONPath expression against fixture used to compare the 4631 * "sourceId/path" evaluations to. 4632 */ 4633 public String getCompareToSourcePath() { 4634 return this.compareToSourcePath == null ? null : this.compareToSourcePath.getValue(); 4635 } 4636 4637 /** 4638 * @param value XPath or JSONPath expression against fixture used to compare the 4639 * "sourceId/path" evaluations to. 4640 */ 4641 public TestScriptSetupActionAssertComponent setCompareToSourcePath(String value) { 4642 if (Utilities.noString(value)) 4643 this.compareToSourcePath = null; 4644 else { 4645 if (this.compareToSourcePath == null) 4646 this.compareToSourcePath = new StringType(); 4647 this.compareToSourcePath.setValue(value); 4648 } 4649 return this; 4650 } 4651 4652 /** 4653 * @return {@link #contentType} (The content-type or mime-type to use for 4654 * RESTful operation in the 'Content-Type' header.). This is the 4655 * underlying object with id, value and extensions. The accessor 4656 * "getContentType" gives direct access to the value 4657 */ 4658 public Enumeration<ContentType> getContentTypeElement() { 4659 if (this.contentType == null) 4660 if (Configuration.errorOnAutoCreate()) 4661 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.contentType"); 4662 else if (Configuration.doAutoCreate()) 4663 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); // bb 4664 return this.contentType; 4665 } 4666 4667 public boolean hasContentTypeElement() { 4668 return this.contentType != null && !this.contentType.isEmpty(); 4669 } 4670 4671 public boolean hasContentType() { 4672 return this.contentType != null && !this.contentType.isEmpty(); 4673 } 4674 4675 /** 4676 * @param value {@link #contentType} (The content-type or mime-type to use for 4677 * RESTful operation in the 'Content-Type' header.). This is the 4678 * underlying object with id, value and extensions. The accessor 4679 * "getContentType" gives direct access to the value 4680 */ 4681 public TestScriptSetupActionAssertComponent setContentTypeElement(Enumeration<ContentType> value) { 4682 this.contentType = value; 4683 return this; 4684 } 4685 4686 /** 4687 * @return The content-type or mime-type to use for RESTful operation in the 4688 * 'Content-Type' header. 4689 */ 4690 public ContentType getContentType() { 4691 return this.contentType == null ? null : this.contentType.getValue(); 4692 } 4693 4694 /** 4695 * @param value The content-type or mime-type to use for RESTful operation in 4696 * the 'Content-Type' header. 4697 */ 4698 public TestScriptSetupActionAssertComponent setContentType(ContentType value) { 4699 if (value == null) 4700 this.contentType = null; 4701 else { 4702 if (this.contentType == null) 4703 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); 4704 this.contentType.setValue(value); 4705 } 4706 return this; 4707 } 4708 4709 /** 4710 * @return {@link #headerField} (The HTTP header field name e.g. 'Location'.). 4711 * This is the underlying object with id, value and extensions. The 4712 * accessor "getHeaderField" gives direct access to the value 4713 */ 4714 public StringType getHeaderFieldElement() { 4715 if (this.headerField == null) 4716 if (Configuration.errorOnAutoCreate()) 4717 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.headerField"); 4718 else if (Configuration.doAutoCreate()) 4719 this.headerField = new StringType(); // bb 4720 return this.headerField; 4721 } 4722 4723 public boolean hasHeaderFieldElement() { 4724 return this.headerField != null && !this.headerField.isEmpty(); 4725 } 4726 4727 public boolean hasHeaderField() { 4728 return this.headerField != null && !this.headerField.isEmpty(); 4729 } 4730 4731 /** 4732 * @param value {@link #headerField} (The HTTP header field name e.g. 4733 * 'Location'.). This is the underlying object with id, value and 4734 * extensions. The accessor "getHeaderField" gives direct access to 4735 * the value 4736 */ 4737 public TestScriptSetupActionAssertComponent setHeaderFieldElement(StringType value) { 4738 this.headerField = value; 4739 return this; 4740 } 4741 4742 /** 4743 * @return The HTTP header field name e.g. 'Location'. 4744 */ 4745 public String getHeaderField() { 4746 return this.headerField == null ? null : this.headerField.getValue(); 4747 } 4748 4749 /** 4750 * @param value The HTTP header field name e.g. 'Location'. 4751 */ 4752 public TestScriptSetupActionAssertComponent setHeaderField(String value) { 4753 if (Utilities.noString(value)) 4754 this.headerField = null; 4755 else { 4756 if (this.headerField == null) 4757 this.headerField = new StringType(); 4758 this.headerField.setValue(value); 4759 } 4760 return this; 4761 } 4762 4763 /** 4764 * @return {@link #minimumId} (The ID of a fixture. Asserts that the response 4765 * contains at a minimumId the fixture specified by minimumId.). This is 4766 * the underlying object with id, value and extensions. The accessor 4767 * "getMinimumId" gives direct access to the value 4768 */ 4769 public StringType getMinimumIdElement() { 4770 if (this.minimumId == null) 4771 if (Configuration.errorOnAutoCreate()) 4772 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.minimumId"); 4773 else if (Configuration.doAutoCreate()) 4774 this.minimumId = new StringType(); // bb 4775 return this.minimumId; 4776 } 4777 4778 public boolean hasMinimumIdElement() { 4779 return this.minimumId != null && !this.minimumId.isEmpty(); 4780 } 4781 4782 public boolean hasMinimumId() { 4783 return this.minimumId != null && !this.minimumId.isEmpty(); 4784 } 4785 4786 /** 4787 * @param value {@link #minimumId} (The ID of a fixture. Asserts that the 4788 * response contains at a minimumId the fixture specified by 4789 * minimumId.). This is the underlying object with id, value and 4790 * extensions. The accessor "getMinimumId" gives direct access to 4791 * the value 4792 */ 4793 public TestScriptSetupActionAssertComponent setMinimumIdElement(StringType value) { 4794 this.minimumId = value; 4795 return this; 4796 } 4797 4798 /** 4799 * @return The ID of a fixture. Asserts that the response contains at a 4800 * minimumId the fixture specified by minimumId. 4801 */ 4802 public String getMinimumId() { 4803 return this.minimumId == null ? null : this.minimumId.getValue(); 4804 } 4805 4806 /** 4807 * @param value The ID of a fixture. Asserts that the response contains at a 4808 * minimumId the fixture specified by minimumId. 4809 */ 4810 public TestScriptSetupActionAssertComponent setMinimumId(String value) { 4811 if (Utilities.noString(value)) 4812 this.minimumId = null; 4813 else { 4814 if (this.minimumId == null) 4815 this.minimumId = new StringType(); 4816 this.minimumId.setValue(value); 4817 } 4818 return this; 4819 } 4820 4821 /** 4822 * @return {@link #navigationLinks} (Whether or not the test execution performs 4823 * validation on the bundle navigation links.). This is the underlying 4824 * object with id, value and extensions. The accessor 4825 * "getNavigationLinks" gives direct access to the value 4826 */ 4827 public BooleanType getNavigationLinksElement() { 4828 if (this.navigationLinks == null) 4829 if (Configuration.errorOnAutoCreate()) 4830 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.navigationLinks"); 4831 else if (Configuration.doAutoCreate()) 4832 this.navigationLinks = new BooleanType(); // bb 4833 return this.navigationLinks; 4834 } 4835 4836 public boolean hasNavigationLinksElement() { 4837 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 4838 } 4839 4840 public boolean hasNavigationLinks() { 4841 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 4842 } 4843 4844 /** 4845 * @param value {@link #navigationLinks} (Whether or not the test execution 4846 * performs validation on the bundle navigation links.). This is 4847 * the underlying object with id, value and extensions. The 4848 * accessor "getNavigationLinks" gives direct access to the value 4849 */ 4850 public TestScriptSetupActionAssertComponent setNavigationLinksElement(BooleanType value) { 4851 this.navigationLinks = value; 4852 return this; 4853 } 4854 4855 /** 4856 * @return Whether or not the test execution performs validation on the bundle 4857 * navigation links. 4858 */ 4859 public boolean getNavigationLinks() { 4860 return this.navigationLinks == null || this.navigationLinks.isEmpty() ? false : this.navigationLinks.getValue(); 4861 } 4862 4863 /** 4864 * @param value Whether or not the test execution performs validation on the 4865 * bundle navigation links. 4866 */ 4867 public TestScriptSetupActionAssertComponent setNavigationLinks(boolean value) { 4868 if (this.navigationLinks == null) 4869 this.navigationLinks = new BooleanType(); 4870 this.navigationLinks.setValue(value); 4871 return this; 4872 } 4873 4874 /** 4875 * @return {@link #operator} (The operator type.). This is the underlying object 4876 * with id, value and extensions. The accessor "getOperator" gives 4877 * direct access to the value 4878 */ 4879 public Enumeration<AssertionOperatorType> getOperatorElement() { 4880 if (this.operator == null) 4881 if (Configuration.errorOnAutoCreate()) 4882 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.operator"); 4883 else if (Configuration.doAutoCreate()) 4884 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); // bb 4885 return this.operator; 4886 } 4887 4888 public boolean hasOperatorElement() { 4889 return this.operator != null && !this.operator.isEmpty(); 4890 } 4891 4892 public boolean hasOperator() { 4893 return this.operator != null && !this.operator.isEmpty(); 4894 } 4895 4896 /** 4897 * @param value {@link #operator} (The operator type.). This is the underlying 4898 * object with id, value and extensions. The accessor "getOperator" 4899 * gives direct access to the value 4900 */ 4901 public TestScriptSetupActionAssertComponent setOperatorElement(Enumeration<AssertionOperatorType> value) { 4902 this.operator = value; 4903 return this; 4904 } 4905 4906 /** 4907 * @return The operator type. 4908 */ 4909 public AssertionOperatorType getOperator() { 4910 return this.operator == null ? null : this.operator.getValue(); 4911 } 4912 4913 /** 4914 * @param value The operator type. 4915 */ 4916 public TestScriptSetupActionAssertComponent setOperator(AssertionOperatorType value) { 4917 if (value == null) 4918 this.operator = null; 4919 else { 4920 if (this.operator == null) 4921 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); 4922 this.operator.setValue(value); 4923 } 4924 return this; 4925 } 4926 4927 /** 4928 * @return {@link #path} (The XPath or JSONPath expression to be evaluated 4929 * against the fixture representing the response received from server.). 4930 * This is the underlying object with id, value and extensions. The 4931 * accessor "getPath" gives direct access to the value 4932 */ 4933 public StringType getPathElement() { 4934 if (this.path == null) 4935 if (Configuration.errorOnAutoCreate()) 4936 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.path"); 4937 else if (Configuration.doAutoCreate()) 4938 this.path = new StringType(); // bb 4939 return this.path; 4940 } 4941 4942 public boolean hasPathElement() { 4943 return this.path != null && !this.path.isEmpty(); 4944 } 4945 4946 public boolean hasPath() { 4947 return this.path != null && !this.path.isEmpty(); 4948 } 4949 4950 /** 4951 * @param value {@link #path} (The XPath or JSONPath expression to be evaluated 4952 * against the fixture representing the response received from 4953 * server.). This is the underlying object with id, value and 4954 * extensions. The accessor "getPath" gives direct access to the 4955 * value 4956 */ 4957 public TestScriptSetupActionAssertComponent setPathElement(StringType value) { 4958 this.path = value; 4959 return this; 4960 } 4961 4962 /** 4963 * @return The XPath or JSONPath expression to be evaluated against the fixture 4964 * representing the response received from server. 4965 */ 4966 public String getPath() { 4967 return this.path == null ? null : this.path.getValue(); 4968 } 4969 4970 /** 4971 * @param value The XPath or JSONPath expression to be evaluated against the 4972 * fixture representing the response received from server. 4973 */ 4974 public TestScriptSetupActionAssertComponent setPath(String value) { 4975 if (Utilities.noString(value)) 4976 this.path = null; 4977 else { 4978 if (this.path == null) 4979 this.path = new StringType(); 4980 this.path.setValue(value); 4981 } 4982 return this; 4983 } 4984 4985 /** 4986 * @return {@link #resource} (The type of the resource. See 4987 * http://hl7-fhir.github.io/resourcelist.html.). This is the underlying 4988 * object with id, value and extensions. The accessor "getResource" 4989 * gives direct access to the value 4990 */ 4991 public CodeType getResourceElement() { 4992 if (this.resource == null) 4993 if (Configuration.errorOnAutoCreate()) 4994 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.resource"); 4995 else if (Configuration.doAutoCreate()) 4996 this.resource = new CodeType(); // bb 4997 return this.resource; 4998 } 4999 5000 public boolean hasResourceElement() { 5001 return this.resource != null && !this.resource.isEmpty(); 5002 } 5003 5004 public boolean hasResource() { 5005 return this.resource != null && !this.resource.isEmpty(); 5006 } 5007 5008 /** 5009 * @param value {@link #resource} (The type of the resource. See 5010 * http://hl7-fhir.github.io/resourcelist.html.). This is the 5011 * underlying object with id, value and extensions. The accessor 5012 * "getResource" gives direct access to the value 5013 */ 5014 public TestScriptSetupActionAssertComponent setResourceElement(CodeType value) { 5015 this.resource = value; 5016 return this; 5017 } 5018 5019 /** 5020 * @return The type of the resource. See 5021 * http://hl7-fhir.github.io/resourcelist.html. 5022 */ 5023 public String getResource() { 5024 return this.resource == null ? null : this.resource.getValue(); 5025 } 5026 5027 /** 5028 * @param value The type of the resource. See 5029 * http://hl7-fhir.github.io/resourcelist.html. 5030 */ 5031 public TestScriptSetupActionAssertComponent setResource(String value) { 5032 if (Utilities.noString(value)) 5033 this.resource = null; 5034 else { 5035 if (this.resource == null) 5036 this.resource = new CodeType(); 5037 this.resource.setValue(value); 5038 } 5039 return this; 5040 } 5041 5042 /** 5043 * @return {@link #response} (okay | created | noContent | notModified | bad | 5044 * forbidden | notFound | methodNotAllowed | conflict | gone | 5045 * preconditionFailed | unprocessable.). This is the underlying object 5046 * with id, value and extensions. The accessor "getResponse" gives 5047 * direct access to the value 5048 */ 5049 public Enumeration<AssertionResponseTypes> getResponseElement() { 5050 if (this.response == null) 5051 if (Configuration.errorOnAutoCreate()) 5052 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.response"); 5053 else if (Configuration.doAutoCreate()) 5054 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); // bb 5055 return this.response; 5056 } 5057 5058 public boolean hasResponseElement() { 5059 return this.response != null && !this.response.isEmpty(); 5060 } 5061 5062 public boolean hasResponse() { 5063 return this.response != null && !this.response.isEmpty(); 5064 } 5065 5066 /** 5067 * @param value {@link #response} (okay | created | noContent | notModified | 5068 * bad | forbidden | notFound | methodNotAllowed | conflict | gone 5069 * | preconditionFailed | unprocessable.). This is the underlying 5070 * object with id, value and extensions. The accessor "getResponse" 5071 * gives direct access to the value 5072 */ 5073 public TestScriptSetupActionAssertComponent setResponseElement(Enumeration<AssertionResponseTypes> value) { 5074 this.response = value; 5075 return this; 5076 } 5077 5078 /** 5079 * @return okay | created | noContent | notModified | bad | forbidden | notFound 5080 * | methodNotAllowed | conflict | gone | preconditionFailed | 5081 * unprocessable. 5082 */ 5083 public AssertionResponseTypes getResponse() { 5084 return this.response == null ? null : this.response.getValue(); 5085 } 5086 5087 /** 5088 * @param value okay | created | noContent | notModified | bad | forbidden | 5089 * notFound | methodNotAllowed | conflict | gone | 5090 * preconditionFailed | unprocessable. 5091 */ 5092 public TestScriptSetupActionAssertComponent setResponse(AssertionResponseTypes value) { 5093 if (value == null) 5094 this.response = null; 5095 else { 5096 if (this.response == null) 5097 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); 5098 this.response.setValue(value); 5099 } 5100 return this; 5101 } 5102 5103 /** 5104 * @return {@link #responseCode} (The value of the HTTP response code to be 5105 * tested.). This is the underlying object with id, value and 5106 * extensions. The accessor "getResponseCode" gives direct access to the 5107 * value 5108 */ 5109 public StringType getResponseCodeElement() { 5110 if (this.responseCode == null) 5111 if (Configuration.errorOnAutoCreate()) 5112 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.responseCode"); 5113 else if (Configuration.doAutoCreate()) 5114 this.responseCode = new StringType(); // bb 5115 return this.responseCode; 5116 } 5117 5118 public boolean hasResponseCodeElement() { 5119 return this.responseCode != null && !this.responseCode.isEmpty(); 5120 } 5121 5122 public boolean hasResponseCode() { 5123 return this.responseCode != null && !this.responseCode.isEmpty(); 5124 } 5125 5126 /** 5127 * @param value {@link #responseCode} (The value of the HTTP response code to be 5128 * tested.). This is the underlying object with id, value and 5129 * extensions. The accessor "getResponseCode" gives direct access 5130 * to the value 5131 */ 5132 public TestScriptSetupActionAssertComponent setResponseCodeElement(StringType value) { 5133 this.responseCode = value; 5134 return this; 5135 } 5136 5137 /** 5138 * @return The value of the HTTP response code to be tested. 5139 */ 5140 public String getResponseCode() { 5141 return this.responseCode == null ? null : this.responseCode.getValue(); 5142 } 5143 5144 /** 5145 * @param value The value of the HTTP response code to be tested. 5146 */ 5147 public TestScriptSetupActionAssertComponent setResponseCode(String value) { 5148 if (Utilities.noString(value)) 5149 this.responseCode = null; 5150 else { 5151 if (this.responseCode == null) 5152 this.responseCode = new StringType(); 5153 this.responseCode.setValue(value); 5154 } 5155 return this; 5156 } 5157 5158 /** 5159 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression 5160 * or the headerField against.). This is the underlying object with id, 5161 * value and extensions. The accessor "getSourceId" gives direct access 5162 * to the value 5163 */ 5164 public IdType getSourceIdElement() { 5165 if (this.sourceId == null) 5166 if (Configuration.errorOnAutoCreate()) 5167 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.sourceId"); 5168 else if (Configuration.doAutoCreate()) 5169 this.sourceId = new IdType(); // bb 5170 return this.sourceId; 5171 } 5172 5173 public boolean hasSourceIdElement() { 5174 return this.sourceId != null && !this.sourceId.isEmpty(); 5175 } 5176 5177 public boolean hasSourceId() { 5178 return this.sourceId != null && !this.sourceId.isEmpty(); 5179 } 5180 5181 /** 5182 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath 5183 * expression or the headerField against.). This is the underlying 5184 * object with id, value and extensions. The accessor "getSourceId" 5185 * gives direct access to the value 5186 */ 5187 public TestScriptSetupActionAssertComponent setSourceIdElement(IdType value) { 5188 this.sourceId = value; 5189 return this; 5190 } 5191 5192 /** 5193 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField 5194 * against. 5195 */ 5196 public String getSourceId() { 5197 return this.sourceId == null ? null : this.sourceId.getValue(); 5198 } 5199 5200 /** 5201 * @param value Fixture to evaluate the XPath/JSONPath expression or the 5202 * headerField against. 5203 */ 5204 public TestScriptSetupActionAssertComponent setSourceId(String value) { 5205 if (Utilities.noString(value)) 5206 this.sourceId = null; 5207 else { 5208 if (this.sourceId == null) 5209 this.sourceId = new IdType(); 5210 this.sourceId.setValue(value); 5211 } 5212 return this; 5213 } 5214 5215 /** 5216 * @return {@link #validateProfileId} (The ID of the Profile to validate 5217 * against.). This is the underlying object with id, value and 5218 * extensions. The accessor "getValidateProfileId" gives direct access 5219 * to the value 5220 */ 5221 public IdType getValidateProfileIdElement() { 5222 if (this.validateProfileId == null) 5223 if (Configuration.errorOnAutoCreate()) 5224 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.validateProfileId"); 5225 else if (Configuration.doAutoCreate()) 5226 this.validateProfileId = new IdType(); // bb 5227 return this.validateProfileId; 5228 } 5229 5230 public boolean hasValidateProfileIdElement() { 5231 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 5232 } 5233 5234 public boolean hasValidateProfileId() { 5235 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 5236 } 5237 5238 /** 5239 * @param value {@link #validateProfileId} (The ID of the Profile to validate 5240 * against.). This is the underlying object with id, value and 5241 * extensions. The accessor "getValidateProfileId" gives direct 5242 * access to the value 5243 */ 5244 public TestScriptSetupActionAssertComponent setValidateProfileIdElement(IdType value) { 5245 this.validateProfileId = value; 5246 return this; 5247 } 5248 5249 /** 5250 * @return The ID of the Profile to validate against. 5251 */ 5252 public String getValidateProfileId() { 5253 return this.validateProfileId == null ? null : this.validateProfileId.getValue(); 5254 } 5255 5256 /** 5257 * @param value The ID of the Profile to validate against. 5258 */ 5259 public TestScriptSetupActionAssertComponent setValidateProfileId(String value) { 5260 if (Utilities.noString(value)) 5261 this.validateProfileId = null; 5262 else { 5263 if (this.validateProfileId == null) 5264 this.validateProfileId = new IdType(); 5265 this.validateProfileId.setValue(value); 5266 } 5267 return this; 5268 } 5269 5270 /** 5271 * @return {@link #value} (The value to compare to.). This is the underlying 5272 * object with id, value and extensions. The accessor "getValue" gives 5273 * direct access to the value 5274 */ 5275 public StringType getValueElement() { 5276 if (this.value == null) 5277 if (Configuration.errorOnAutoCreate()) 5278 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.value"); 5279 else if (Configuration.doAutoCreate()) 5280 this.value = new StringType(); // bb 5281 return this.value; 5282 } 5283 5284 public boolean hasValueElement() { 5285 return this.value != null && !this.value.isEmpty(); 5286 } 5287 5288 public boolean hasValue() { 5289 return this.value != null && !this.value.isEmpty(); 5290 } 5291 5292 /** 5293 * @param value {@link #value} (The value to compare to.). This is the 5294 * underlying object with id, value and extensions. The accessor 5295 * "getValue" gives direct access to the value 5296 */ 5297 public TestScriptSetupActionAssertComponent setValueElement(StringType value) { 5298 this.value = value; 5299 return this; 5300 } 5301 5302 /** 5303 * @return The value to compare to. 5304 */ 5305 public String getValue() { 5306 return this.value == null ? null : this.value.getValue(); 5307 } 5308 5309 /** 5310 * @param value The value to compare to. 5311 */ 5312 public TestScriptSetupActionAssertComponent setValue(String value) { 5313 if (Utilities.noString(value)) 5314 this.value = null; 5315 else { 5316 if (this.value == null) 5317 this.value = new StringType(); 5318 this.value.setValue(value); 5319 } 5320 return this; 5321 } 5322 5323 /** 5324 * @return {@link #warningOnly} (Whether or not the test execution will produce 5325 * a warning only on error for this assert.). This is the underlying 5326 * object with id, value and extensions. The accessor "getWarningOnly" 5327 * gives direct access to the value 5328 */ 5329 public BooleanType getWarningOnlyElement() { 5330 if (this.warningOnly == null) 5331 if (Configuration.errorOnAutoCreate()) 5332 throw new Error("Attempt to auto-create TestScriptSetupActionAssertComponent.warningOnly"); 5333 else if (Configuration.doAutoCreate()) 5334 this.warningOnly = new BooleanType(); // bb 5335 return this.warningOnly; 5336 } 5337 5338 public boolean hasWarningOnlyElement() { 5339 return this.warningOnly != null && !this.warningOnly.isEmpty(); 5340 } 5341 5342 public boolean hasWarningOnly() { 5343 return this.warningOnly != null && !this.warningOnly.isEmpty(); 5344 } 5345 5346 /** 5347 * @param value {@link #warningOnly} (Whether or not the test execution will 5348 * produce a warning only on error for this assert.). This is the 5349 * underlying object with id, value and extensions. The accessor 5350 * "getWarningOnly" gives direct access to the value 5351 */ 5352 public TestScriptSetupActionAssertComponent setWarningOnlyElement(BooleanType value) { 5353 this.warningOnly = value; 5354 return this; 5355 } 5356 5357 /** 5358 * @return Whether or not the test execution will produce a warning only on 5359 * error for this assert. 5360 */ 5361 public boolean getWarningOnly() { 5362 return this.warningOnly == null || this.warningOnly.isEmpty() ? false : this.warningOnly.getValue(); 5363 } 5364 5365 /** 5366 * @param value Whether or not the test execution will produce a warning only on 5367 * error for this assert. 5368 */ 5369 public TestScriptSetupActionAssertComponent setWarningOnly(boolean value) { 5370 if (this.warningOnly == null) 5371 this.warningOnly = new BooleanType(); 5372 this.warningOnly.setValue(value); 5373 return this; 5374 } 5375 5376 protected void listChildren(List<Property> childrenList) { 5377 super.listChildren(childrenList); 5378 childrenList 5379 .add(new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 5380 0, java.lang.Integer.MAX_VALUE, label)); 5381 childrenList.add(new Property("description", "string", 5382 "The description would be used by test engines for tracking and reporting purposes.", 0, 5383 java.lang.Integer.MAX_VALUE, description)); 5384 childrenList.add(new Property("direction", "code", "The direction to use for the assertion.", 0, 5385 java.lang.Integer.MAX_VALUE, direction)); 5386 childrenList.add(new Property("compareToSourceId", "string", 5387 "Id of fixture used to compare the \"sourceId/path\" evaluations to.", 0, java.lang.Integer.MAX_VALUE, 5388 compareToSourceId)); 5389 childrenList.add(new Property("compareToSourcePath", "string", 5390 "XPath or JSONPath expression against fixture used to compare the \"sourceId/path\" evaluations to.", 0, 5391 java.lang.Integer.MAX_VALUE, compareToSourcePath)); 5392 childrenList.add(new Property("contentType", "code", 5393 "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 5394 java.lang.Integer.MAX_VALUE, contentType)); 5395 childrenList.add(new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 5396 java.lang.Integer.MAX_VALUE, headerField)); 5397 childrenList.add(new Property("minimumId", "string", 5398 "The ID of a fixture. Asserts that the response contains at a minimumId the fixture specified by minimumId.", 5399 0, java.lang.Integer.MAX_VALUE, minimumId)); 5400 childrenList.add(new Property("navigationLinks", "boolean", 5401 "Whether or not the test execution performs validation on the bundle navigation links.", 0, 5402 java.lang.Integer.MAX_VALUE, navigationLinks)); 5403 childrenList 5404 .add(new Property("operator", "code", "The operator type.", 0, java.lang.Integer.MAX_VALUE, operator)); 5405 childrenList.add(new Property("path", "string", 5406 "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 5407 0, java.lang.Integer.MAX_VALUE, path)); 5408 childrenList.add(new Property("resource", "code", 5409 "The type of the resource. See http://hl7-fhir.github.io/resourcelist.html.", 0, java.lang.Integer.MAX_VALUE, 5410 resource)); 5411 childrenList.add(new Property("response", "code", 5412 "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.", 5413 0, java.lang.Integer.MAX_VALUE, response)); 5414 childrenList.add(new Property("responseCode", "string", "The value of the HTTP response code to be tested.", 0, 5415 java.lang.Integer.MAX_VALUE, responseCode)); 5416 childrenList.add(new Property("sourceId", "id", 5417 "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 5418 java.lang.Integer.MAX_VALUE, sourceId)); 5419 childrenList.add(new Property("validateProfileId", "id", "The ID of the Profile to validate against.", 0, 5420 java.lang.Integer.MAX_VALUE, validateProfileId)); 5421 childrenList 5422 .add(new Property("value", "string", "The value to compare to.", 0, java.lang.Integer.MAX_VALUE, value)); 5423 childrenList.add(new Property("warningOnly", "boolean", 5424 "Whether or not the test execution will produce a warning only on error for this assert.", 0, 5425 java.lang.Integer.MAX_VALUE, warningOnly)); 5426 } 5427 5428 @Override 5429 public void setProperty(String name, Base value) throws FHIRException { 5430 if (name.equals("label")) 5431 this.label = castToString(value); // StringType 5432 else if (name.equals("description")) 5433 this.description = castToString(value); // StringType 5434 else if (name.equals("direction")) 5435 this.direction = new AssertionDirectionTypeEnumFactory().fromType(value); // Enumeration<AssertionDirectionType> 5436 else if (name.equals("compareToSourceId")) 5437 this.compareToSourceId = castToString(value); // StringType 5438 else if (name.equals("compareToSourcePath")) 5439 this.compareToSourcePath = castToString(value); // StringType 5440 else if (name.equals("contentType")) 5441 this.contentType = new ContentTypeEnumFactory().fromType(value); // Enumeration<ContentType> 5442 else if (name.equals("headerField")) 5443 this.headerField = castToString(value); // StringType 5444 else if (name.equals("minimumId")) 5445 this.minimumId = castToString(value); // StringType 5446 else if (name.equals("navigationLinks")) 5447 this.navigationLinks = castToBoolean(value); // BooleanType 5448 else if (name.equals("operator")) 5449 this.operator = new AssertionOperatorTypeEnumFactory().fromType(value); // Enumeration<AssertionOperatorType> 5450 else if (name.equals("path")) 5451 this.path = castToString(value); // StringType 5452 else if (name.equals("resource")) 5453 this.resource = castToCode(value); // CodeType 5454 else if (name.equals("response")) 5455 this.response = new AssertionResponseTypesEnumFactory().fromType(value); // Enumeration<AssertionResponseTypes> 5456 else if (name.equals("responseCode")) 5457 this.responseCode = castToString(value); // StringType 5458 else if (name.equals("sourceId")) 5459 this.sourceId = castToId(value); // IdType 5460 else if (name.equals("validateProfileId")) 5461 this.validateProfileId = castToId(value); // IdType 5462 else if (name.equals("value")) 5463 this.value = castToString(value); // StringType 5464 else if (name.equals("warningOnly")) 5465 this.warningOnly = castToBoolean(value); // BooleanType 5466 else 5467 super.setProperty(name, value); 5468 } 5469 5470 @Override 5471 public Base addChild(String name) throws FHIRException { 5472 if (name.equals("label")) { 5473 throw new FHIRException("Cannot call addChild on a singleton property TestScript.label"); 5474 } else if (name.equals("description")) { 5475 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 5476 } else if (name.equals("direction")) { 5477 throw new FHIRException("Cannot call addChild on a singleton property TestScript.direction"); 5478 } else if (name.equals("compareToSourceId")) { 5479 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourceId"); 5480 } else if (name.equals("compareToSourcePath")) { 5481 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourcePath"); 5482 } else if (name.equals("contentType")) { 5483 throw new FHIRException("Cannot call addChild on a singleton property TestScript.contentType"); 5484 } else if (name.equals("headerField")) { 5485 throw new FHIRException("Cannot call addChild on a singleton property TestScript.headerField"); 5486 } else if (name.equals("minimumId")) { 5487 throw new FHIRException("Cannot call addChild on a singleton property TestScript.minimumId"); 5488 } else if (name.equals("navigationLinks")) { 5489 throw new FHIRException("Cannot call addChild on a singleton property TestScript.navigationLinks"); 5490 } else if (name.equals("operator")) { 5491 throw new FHIRException("Cannot call addChild on a singleton property TestScript.operator"); 5492 } else if (name.equals("path")) { 5493 throw new FHIRException("Cannot call addChild on a singleton property TestScript.path"); 5494 } else if (name.equals("resource")) { 5495 throw new FHIRException("Cannot call addChild on a singleton property TestScript.resource"); 5496 } else if (name.equals("response")) { 5497 throw new FHIRException("Cannot call addChild on a singleton property TestScript.response"); 5498 } else if (name.equals("responseCode")) { 5499 throw new FHIRException("Cannot call addChild on a singleton property TestScript.responseCode"); 5500 } else if (name.equals("sourceId")) { 5501 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 5502 } else if (name.equals("validateProfileId")) { 5503 throw new FHIRException("Cannot call addChild on a singleton property TestScript.validateProfileId"); 5504 } else if (name.equals("value")) { 5505 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 5506 } else if (name.equals("warningOnly")) { 5507 throw new FHIRException("Cannot call addChild on a singleton property TestScript.warningOnly"); 5508 } else 5509 return super.addChild(name); 5510 } 5511 5512 public TestScriptSetupActionAssertComponent copy() { 5513 TestScriptSetupActionAssertComponent dst = new TestScriptSetupActionAssertComponent(); 5514 copyValues(dst); 5515 dst.label = label == null ? null : label.copy(); 5516 dst.description = description == null ? null : description.copy(); 5517 dst.direction = direction == null ? null : direction.copy(); 5518 dst.compareToSourceId = compareToSourceId == null ? null : compareToSourceId.copy(); 5519 dst.compareToSourcePath = compareToSourcePath == null ? null : compareToSourcePath.copy(); 5520 dst.contentType = contentType == null ? null : contentType.copy(); 5521 dst.headerField = headerField == null ? null : headerField.copy(); 5522 dst.minimumId = minimumId == null ? null : minimumId.copy(); 5523 dst.navigationLinks = navigationLinks == null ? null : navigationLinks.copy(); 5524 dst.operator = operator == null ? null : operator.copy(); 5525 dst.path = path == null ? null : path.copy(); 5526 dst.resource = resource == null ? null : resource.copy(); 5527 dst.response = response == null ? null : response.copy(); 5528 dst.responseCode = responseCode == null ? null : responseCode.copy(); 5529 dst.sourceId = sourceId == null ? null : sourceId.copy(); 5530 dst.validateProfileId = validateProfileId == null ? null : validateProfileId.copy(); 5531 dst.value = value == null ? null : value.copy(); 5532 dst.warningOnly = warningOnly == null ? null : warningOnly.copy(); 5533 return dst; 5534 } 5535 5536 @Override 5537 public boolean equalsDeep(Base other) { 5538 if (!super.equalsDeep(other)) 5539 return false; 5540 if (!(other instanceof TestScriptSetupActionAssertComponent)) 5541 return false; 5542 TestScriptSetupActionAssertComponent o = (TestScriptSetupActionAssertComponent) other; 5543 return compareDeep(label, o.label, true) && compareDeep(description, o.description, true) 5544 && compareDeep(direction, o.direction, true) && compareDeep(compareToSourceId, o.compareToSourceId, true) 5545 && compareDeep(compareToSourcePath, o.compareToSourcePath, true) 5546 && compareDeep(contentType, o.contentType, true) && compareDeep(headerField, o.headerField, true) 5547 && compareDeep(minimumId, o.minimumId, true) && compareDeep(navigationLinks, o.navigationLinks, true) 5548 && compareDeep(operator, o.operator, true) && compareDeep(path, o.path, true) 5549 && compareDeep(resource, o.resource, true) && compareDeep(response, o.response, true) 5550 && compareDeep(responseCode, o.responseCode, true) && compareDeep(sourceId, o.sourceId, true) 5551 && compareDeep(validateProfileId, o.validateProfileId, true) && compareDeep(value, o.value, true) 5552 && compareDeep(warningOnly, o.warningOnly, true); 5553 } 5554 5555 @Override 5556 public boolean equalsShallow(Base other) { 5557 if (!super.equalsShallow(other)) 5558 return false; 5559 if (!(other instanceof TestScriptSetupActionAssertComponent)) 5560 return false; 5561 TestScriptSetupActionAssertComponent o = (TestScriptSetupActionAssertComponent) other; 5562 return compareValues(label, o.label, true) && compareValues(description, o.description, true) 5563 && compareValues(direction, o.direction, true) && compareValues(compareToSourceId, o.compareToSourceId, true) 5564 && compareValues(compareToSourcePath, o.compareToSourcePath, true) 5565 && compareValues(contentType, o.contentType, true) && compareValues(headerField, o.headerField, true) 5566 && compareValues(minimumId, o.minimumId, true) && compareValues(navigationLinks, o.navigationLinks, true) 5567 && compareValues(operator, o.operator, true) && compareValues(path, o.path, true) 5568 && compareValues(resource, o.resource, true) && compareValues(response, o.response, true) 5569 && compareValues(responseCode, o.responseCode, true) && compareValues(sourceId, o.sourceId, true) 5570 && compareValues(validateProfileId, o.validateProfileId, true) && compareValues(value, o.value, true) 5571 && compareValues(warningOnly, o.warningOnly, true); 5572 } 5573 5574 public boolean isEmpty() { 5575 return super.isEmpty() && (label == null || label.isEmpty()) && (description == null || description.isEmpty()) 5576 && (direction == null || direction.isEmpty()) && (compareToSourceId == null || compareToSourceId.isEmpty()) 5577 && (compareToSourcePath == null || compareToSourcePath.isEmpty()) 5578 && (contentType == null || contentType.isEmpty()) && (headerField == null || headerField.isEmpty()) 5579 && (minimumId == null || minimumId.isEmpty()) && (navigationLinks == null || navigationLinks.isEmpty()) 5580 && (operator == null || operator.isEmpty()) && (path == null || path.isEmpty()) 5581 && (resource == null || resource.isEmpty()) && (response == null || response.isEmpty()) 5582 && (responseCode == null || responseCode.isEmpty()) && (sourceId == null || sourceId.isEmpty()) 5583 && (validateProfileId == null || validateProfileId.isEmpty()) && (value == null || value.isEmpty()) 5584 && (warningOnly == null || warningOnly.isEmpty()); 5585 } 5586 5587 public String fhirType() { 5588 return "TestScript.setup.action.assert"; 5589 5590 } 5591 5592 } 5593 5594 @Block() 5595 public static class TestScriptTestComponent extends BackboneElement implements IBaseBackboneElement { 5596 /** 5597 * The name of this test used for tracking/logging purposes by test engines. 5598 */ 5599 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 5600 @Description(shortDefinition = "Tracking/logging name of this test", formalDefinition = "The name of this test used for tracking/logging purposes by test engines.") 5601 protected StringType name; 5602 5603 /** 5604 * A short description of the test used by test engines for tracking and 5605 * reporting purposes. 5606 */ 5607 @Child(name = "description", type = { 5608 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5609 @Description(shortDefinition = "Tracking/reporting short description of the test", formalDefinition = "A short description of the test used by test engines for tracking and reporting purposes.") 5610 protected StringType description; 5611 5612 /** 5613 * Capabilities that must exist and are assumed to function correctly on the 5614 * FHIR server being tested. 5615 */ 5616 @Child(name = "metadata", type = { 5617 TestScriptMetadataComponent.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 5618 @Description(shortDefinition = "Capabilities that are expected to function correctly on the FHIR server being tested", formalDefinition = "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.") 5619 protected TestScriptMetadataComponent metadata; 5620 5621 /** 5622 * Action would contain either an operation or an assertion. 5623 */ 5624 @Child(name = "action", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5625 @Description(shortDefinition = "A test operation or assert to perform", formalDefinition = "Action would contain either an operation or an assertion.") 5626 protected List<TestScriptTestActionComponent> action; 5627 5628 private static final long serialVersionUID = 408339297L; 5629 5630 /* 5631 * Constructor 5632 */ 5633 public TestScriptTestComponent() { 5634 super(); 5635 } 5636 5637 /** 5638 * @return {@link #name} (The name of this test used for tracking/logging 5639 * purposes by test engines.). This is the underlying object with id, 5640 * value and extensions. The accessor "getName" gives direct access to 5641 * the value 5642 */ 5643 public StringType getNameElement() { 5644 if (this.name == null) 5645 if (Configuration.errorOnAutoCreate()) 5646 throw new Error("Attempt to auto-create TestScriptTestComponent.name"); 5647 else if (Configuration.doAutoCreate()) 5648 this.name = new StringType(); // bb 5649 return this.name; 5650 } 5651 5652 public boolean hasNameElement() { 5653 return this.name != null && !this.name.isEmpty(); 5654 } 5655 5656 public boolean hasName() { 5657 return this.name != null && !this.name.isEmpty(); 5658 } 5659 5660 /** 5661 * @param value {@link #name} (The name of this test used for tracking/logging 5662 * purposes by test engines.). This is the underlying object with 5663 * id, value and extensions. The accessor "getName" gives direct 5664 * access to the value 5665 */ 5666 public TestScriptTestComponent setNameElement(StringType value) { 5667 this.name = value; 5668 return this; 5669 } 5670 5671 /** 5672 * @return The name of this test used for tracking/logging purposes by test 5673 * engines. 5674 */ 5675 public String getName() { 5676 return this.name == null ? null : this.name.getValue(); 5677 } 5678 5679 /** 5680 * @param value The name of this test used for tracking/logging purposes by test 5681 * engines. 5682 */ 5683 public TestScriptTestComponent setName(String value) { 5684 if (Utilities.noString(value)) 5685 this.name = null; 5686 else { 5687 if (this.name == null) 5688 this.name = new StringType(); 5689 this.name.setValue(value); 5690 } 5691 return this; 5692 } 5693 5694 /** 5695 * @return {@link #description} (A short description of the test used by test 5696 * engines for tracking and reporting purposes.). This is the underlying 5697 * object with id, value and extensions. The accessor "getDescription" 5698 * gives direct access to the value 5699 */ 5700 public StringType getDescriptionElement() { 5701 if (this.description == null) 5702 if (Configuration.errorOnAutoCreate()) 5703 throw new Error("Attempt to auto-create TestScriptTestComponent.description"); 5704 else if (Configuration.doAutoCreate()) 5705 this.description = new StringType(); // bb 5706 return this.description; 5707 } 5708 5709 public boolean hasDescriptionElement() { 5710 return this.description != null && !this.description.isEmpty(); 5711 } 5712 5713 public boolean hasDescription() { 5714 return this.description != null && !this.description.isEmpty(); 5715 } 5716 5717 /** 5718 * @param value {@link #description} (A short description of the test used by 5719 * test engines for tracking and reporting purposes.). This is the 5720 * underlying object with id, value and extensions. The accessor 5721 * "getDescription" gives direct access to the value 5722 */ 5723 public TestScriptTestComponent setDescriptionElement(StringType value) { 5724 this.description = value; 5725 return this; 5726 } 5727 5728 /** 5729 * @return A short description of the test used by test engines for tracking and 5730 * reporting purposes. 5731 */ 5732 public String getDescription() { 5733 return this.description == null ? null : this.description.getValue(); 5734 } 5735 5736 /** 5737 * @param value A short description of the test used by test engines for 5738 * tracking and reporting purposes. 5739 */ 5740 public TestScriptTestComponent setDescription(String value) { 5741 if (Utilities.noString(value)) 5742 this.description = null; 5743 else { 5744 if (this.description == null) 5745 this.description = new StringType(); 5746 this.description.setValue(value); 5747 } 5748 return this; 5749 } 5750 5751 /** 5752 * @return {@link #metadata} (Capabilities that must exist and are assumed to 5753 * function correctly on the FHIR server being tested.) 5754 */ 5755 public TestScriptMetadataComponent getMetadata() { 5756 if (this.metadata == null) 5757 if (Configuration.errorOnAutoCreate()) 5758 throw new Error("Attempt to auto-create TestScriptTestComponent.metadata"); 5759 else if (Configuration.doAutoCreate()) 5760 this.metadata = new TestScriptMetadataComponent(); // cc 5761 return this.metadata; 5762 } 5763 5764 public boolean hasMetadata() { 5765 return this.metadata != null && !this.metadata.isEmpty(); 5766 } 5767 5768 /** 5769 * @param value {@link #metadata} (Capabilities that must exist and are assumed 5770 * to function correctly on the FHIR server being tested.) 5771 */ 5772 public TestScriptTestComponent setMetadata(TestScriptMetadataComponent value) { 5773 this.metadata = value; 5774 return this; 5775 } 5776 5777 /** 5778 * @return {@link #action} (Action would contain either an operation or an 5779 * assertion.) 5780 */ 5781 public List<TestScriptTestActionComponent> getAction() { 5782 if (this.action == null) 5783 this.action = new ArrayList<TestScriptTestActionComponent>(); 5784 return this.action; 5785 } 5786 5787 public boolean hasAction() { 5788 if (this.action == null) 5789 return false; 5790 for (TestScriptTestActionComponent item : this.action) 5791 if (!item.isEmpty()) 5792 return true; 5793 return false; 5794 } 5795 5796 /** 5797 * @return {@link #action} (Action would contain either an operation or an 5798 * assertion.) 5799 */ 5800 // syntactic sugar 5801 public TestScriptTestActionComponent addAction() { // 3 5802 TestScriptTestActionComponent t = new TestScriptTestActionComponent(); 5803 if (this.action == null) 5804 this.action = new ArrayList<TestScriptTestActionComponent>(); 5805 this.action.add(t); 5806 return t; 5807 } 5808 5809 // syntactic sugar 5810 public TestScriptTestComponent addAction(TestScriptTestActionComponent t) { // 3 5811 if (t == null) 5812 return this; 5813 if (this.action == null) 5814 this.action = new ArrayList<TestScriptTestActionComponent>(); 5815 this.action.add(t); 5816 return this; 5817 } 5818 5819 protected void listChildren(List<Property> childrenList) { 5820 super.listChildren(childrenList); 5821 childrenList.add( 5822 new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 5823 java.lang.Integer.MAX_VALUE, name)); 5824 childrenList.add(new Property("description", "string", 5825 "A short description of the test used by test engines for tracking and reporting purposes.", 0, 5826 java.lang.Integer.MAX_VALUE, description)); 5827 childrenList.add(new Property("metadata", "@TestScript.metadata", 5828 "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, 5829 java.lang.Integer.MAX_VALUE, metadata)); 5830 childrenList.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, 5831 java.lang.Integer.MAX_VALUE, action)); 5832 } 5833 5834 @Override 5835 public void setProperty(String name, Base value) throws FHIRException { 5836 if (name.equals("name")) 5837 this.name = castToString(value); // StringType 5838 else if (name.equals("description")) 5839 this.description = castToString(value); // StringType 5840 else if (name.equals("metadata")) 5841 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 5842 else if (name.equals("action")) 5843 this.getAction().add((TestScriptTestActionComponent) value); 5844 else 5845 super.setProperty(name, value); 5846 } 5847 5848 @Override 5849 public Base addChild(String name) throws FHIRException { 5850 if (name.equals("name")) { 5851 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 5852 } else if (name.equals("description")) { 5853 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 5854 } else if (name.equals("metadata")) { 5855 this.metadata = new TestScriptMetadataComponent(); 5856 return this.metadata; 5857 } else if (name.equals("action")) { 5858 return addAction(); 5859 } else 5860 return super.addChild(name); 5861 } 5862 5863 public TestScriptTestComponent copy() { 5864 TestScriptTestComponent dst = new TestScriptTestComponent(); 5865 copyValues(dst); 5866 dst.name = name == null ? null : name.copy(); 5867 dst.description = description == null ? null : description.copy(); 5868 dst.metadata = metadata == null ? null : metadata.copy(); 5869 if (action != null) { 5870 dst.action = new ArrayList<TestScriptTestActionComponent>(); 5871 for (TestScriptTestActionComponent i : action) 5872 dst.action.add(i.copy()); 5873 } 5874 ; 5875 return dst; 5876 } 5877 5878 @Override 5879 public boolean equalsDeep(Base other) { 5880 if (!super.equalsDeep(other)) 5881 return false; 5882 if (!(other instanceof TestScriptTestComponent)) 5883 return false; 5884 TestScriptTestComponent o = (TestScriptTestComponent) other; 5885 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 5886 && compareDeep(metadata, o.metadata, true) && compareDeep(action, o.action, true); 5887 } 5888 5889 @Override 5890 public boolean equalsShallow(Base other) { 5891 if (!super.equalsShallow(other)) 5892 return false; 5893 if (!(other instanceof TestScriptTestComponent)) 5894 return false; 5895 TestScriptTestComponent o = (TestScriptTestComponent) other; 5896 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 5897 } 5898 5899 public boolean isEmpty() { 5900 return super.isEmpty() && (name == null || name.isEmpty()) && (description == null || description.isEmpty()) 5901 && (metadata == null || metadata.isEmpty()) && (action == null || action.isEmpty()); 5902 } 5903 5904 public String fhirType() { 5905 return "TestScript.test"; 5906 5907 } 5908 5909 } 5910 5911 @Block() 5912 public static class TestScriptTestActionComponent extends BackboneElement implements IBaseBackboneElement { 5913 /** 5914 * An operation would involve a REST request to a server. 5915 */ 5916 @Child(name = "operation", type = { 5917 TestScriptSetupActionOperationComponent.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 5918 @Description(shortDefinition = "The setup operation to perform", formalDefinition = "An operation would involve a REST request to a server.") 5919 protected TestScriptSetupActionOperationComponent operation; 5920 5921 /** 5922 * Evaluates the results of previous operations to determine if the server under 5923 * test behaves appropriately. 5924 */ 5925 @Child(name = "assert", type = { 5926 TestScriptSetupActionAssertComponent.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5927 @Description(shortDefinition = "The setup assertion to perform", formalDefinition = "Evaluates the results of previous operations to determine if the server under test behaves appropriately.") 5928 protected TestScriptSetupActionAssertComponent assert_; 5929 5930 private static final long serialVersionUID = 1411550037L; 5931 5932 /* 5933 * Constructor 5934 */ 5935 public TestScriptTestActionComponent() { 5936 super(); 5937 } 5938 5939 /** 5940 * @return {@link #operation} (An operation would involve a REST request to a 5941 * server.) 5942 */ 5943 public TestScriptSetupActionOperationComponent getOperation() { 5944 if (this.operation == null) 5945 if (Configuration.errorOnAutoCreate()) 5946 throw new Error("Attempt to auto-create TestScriptTestActionComponent.operation"); 5947 else if (Configuration.doAutoCreate()) 5948 this.operation = new TestScriptSetupActionOperationComponent(); // cc 5949 return this.operation; 5950 } 5951 5952 public boolean hasOperation() { 5953 return this.operation != null && !this.operation.isEmpty(); 5954 } 5955 5956 /** 5957 * @param value {@link #operation} (An operation would involve a REST request to 5958 * a server.) 5959 */ 5960 public TestScriptTestActionComponent setOperation(TestScriptSetupActionOperationComponent value) { 5961 this.operation = value; 5962 return this; 5963 } 5964 5965 /** 5966 * @return {@link #assert_} (Evaluates the results of previous operations to 5967 * determine if the server under test behaves appropriately.) 5968 */ 5969 public TestScriptSetupActionAssertComponent getAssert() { 5970 if (this.assert_ == null) 5971 if (Configuration.errorOnAutoCreate()) 5972 throw new Error("Attempt to auto-create TestScriptTestActionComponent.assert_"); 5973 else if (Configuration.doAutoCreate()) 5974 this.assert_ = new TestScriptSetupActionAssertComponent(); // cc 5975 return this.assert_; 5976 } 5977 5978 public boolean hasAssert() { 5979 return this.assert_ != null && !this.assert_.isEmpty(); 5980 } 5981 5982 /** 5983 * @param value {@link #assert_} (Evaluates the results of previous operations 5984 * to determine if the server under test behaves appropriately.) 5985 */ 5986 public TestScriptTestActionComponent setAssert(TestScriptSetupActionAssertComponent value) { 5987 this.assert_ = value; 5988 return this; 5989 } 5990 5991 protected void listChildren(List<Property> childrenList) { 5992 super.listChildren(childrenList); 5993 childrenList.add(new Property("operation", "@TestScript.setup.action.operation", 5994 "An operation would involve a REST request to a server.", 0, java.lang.Integer.MAX_VALUE, operation)); 5995 childrenList.add(new Property("assert", "@TestScript.setup.action.assert", 5996 "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 5997 0, java.lang.Integer.MAX_VALUE, assert_)); 5998 } 5999 6000 @Override 6001 public void setProperty(String name, Base value) throws FHIRException { 6002 if (name.equals("operation")) 6003 this.operation = (TestScriptSetupActionOperationComponent) value; // TestScriptSetupActionOperationComponent 6004 else if (name.equals("assert")) 6005 this.assert_ = (TestScriptSetupActionAssertComponent) value; // TestScriptSetupActionAssertComponent 6006 else 6007 super.setProperty(name, value); 6008 } 6009 6010 @Override 6011 public Base addChild(String name) throws FHIRException { 6012 if (name.equals("operation")) { 6013 this.operation = new TestScriptSetupActionOperationComponent(); 6014 return this.operation; 6015 } else if (name.equals("assert")) { 6016 this.assert_ = new TestScriptSetupActionAssertComponent(); 6017 return this.assert_; 6018 } else 6019 return super.addChild(name); 6020 } 6021 6022 public TestScriptTestActionComponent copy() { 6023 TestScriptTestActionComponent dst = new TestScriptTestActionComponent(); 6024 copyValues(dst); 6025 dst.operation = operation == null ? null : operation.copy(); 6026 dst.assert_ = assert_ == null ? null : assert_.copy(); 6027 return dst; 6028 } 6029 6030 @Override 6031 public boolean equalsDeep(Base other) { 6032 if (!super.equalsDeep(other)) 6033 return false; 6034 if (!(other instanceof TestScriptTestActionComponent)) 6035 return false; 6036 TestScriptTestActionComponent o = (TestScriptTestActionComponent) other; 6037 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 6038 } 6039 6040 @Override 6041 public boolean equalsShallow(Base other) { 6042 if (!super.equalsShallow(other)) 6043 return false; 6044 if (!(other instanceof TestScriptTestActionComponent)) 6045 return false; 6046 TestScriptTestActionComponent o = (TestScriptTestActionComponent) other; 6047 return true; 6048 } 6049 6050 public boolean isEmpty() { 6051 return super.isEmpty() && (operation == null || operation.isEmpty()) && (assert_ == null || assert_.isEmpty()); 6052 } 6053 6054 public String fhirType() { 6055 return "TestScript.test.action"; 6056 6057 } 6058 6059 } 6060 6061 @Block() 6062 public static class TestScriptTeardownComponent extends BackboneElement implements IBaseBackboneElement { 6063 /** 6064 * The teardown action will only contain an operation. 6065 */ 6066 @Child(name = "action", type = {}, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6067 @Description(shortDefinition = "One or more teardown operations to perform", formalDefinition = "The teardown action will only contain an operation.") 6068 protected List<TestScriptTeardownActionComponent> action; 6069 6070 private static final long serialVersionUID = 1850225254L; 6071 6072 /* 6073 * Constructor 6074 */ 6075 public TestScriptTeardownComponent() { 6076 super(); 6077 } 6078 6079 /** 6080 * @return {@link #action} (The teardown action will only contain an operation.) 6081 */ 6082 public List<TestScriptTeardownActionComponent> getAction() { 6083 if (this.action == null) 6084 this.action = new ArrayList<TestScriptTeardownActionComponent>(); 6085 return this.action; 6086 } 6087 6088 public boolean hasAction() { 6089 if (this.action == null) 6090 return false; 6091 for (TestScriptTeardownActionComponent item : this.action) 6092 if (!item.isEmpty()) 6093 return true; 6094 return false; 6095 } 6096 6097 /** 6098 * @return {@link #action} (The teardown action will only contain an operation.) 6099 */ 6100 // syntactic sugar 6101 public TestScriptTeardownActionComponent addAction() { // 3 6102 TestScriptTeardownActionComponent t = new TestScriptTeardownActionComponent(); 6103 if (this.action == null) 6104 this.action = new ArrayList<TestScriptTeardownActionComponent>(); 6105 this.action.add(t); 6106 return t; 6107 } 6108 6109 // syntactic sugar 6110 public TestScriptTeardownComponent addAction(TestScriptTeardownActionComponent t) { // 3 6111 if (t == null) 6112 return this; 6113 if (this.action == null) 6114 this.action = new ArrayList<TestScriptTeardownActionComponent>(); 6115 this.action.add(t); 6116 return this; 6117 } 6118 6119 protected void listChildren(List<Property> childrenList) { 6120 super.listChildren(childrenList); 6121 childrenList.add(new Property("action", "", "The teardown action will only contain an operation.", 0, 6122 java.lang.Integer.MAX_VALUE, action)); 6123 } 6124 6125 @Override 6126 public void setProperty(String name, Base value) throws FHIRException { 6127 if (name.equals("action")) 6128 this.getAction().add((TestScriptTeardownActionComponent) value); 6129 else 6130 super.setProperty(name, value); 6131 } 6132 6133 @Override 6134 public Base addChild(String name) throws FHIRException { 6135 if (name.equals("action")) { 6136 return addAction(); 6137 } else 6138 return super.addChild(name); 6139 } 6140 6141 public TestScriptTeardownComponent copy() { 6142 TestScriptTeardownComponent dst = new TestScriptTeardownComponent(); 6143 copyValues(dst); 6144 if (action != null) { 6145 dst.action = new ArrayList<TestScriptTeardownActionComponent>(); 6146 for (TestScriptTeardownActionComponent i : action) 6147 dst.action.add(i.copy()); 6148 } 6149 ; 6150 return dst; 6151 } 6152 6153 @Override 6154 public boolean equalsDeep(Base other) { 6155 if (!super.equalsDeep(other)) 6156 return false; 6157 if (!(other instanceof TestScriptTeardownComponent)) 6158 return false; 6159 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other; 6160 return compareDeep(action, o.action, true); 6161 } 6162 6163 @Override 6164 public boolean equalsShallow(Base other) { 6165 if (!super.equalsShallow(other)) 6166 return false; 6167 if (!(other instanceof TestScriptTeardownComponent)) 6168 return false; 6169 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other; 6170 return true; 6171 } 6172 6173 public boolean isEmpty() { 6174 return super.isEmpty() && (action == null || action.isEmpty()); 6175 } 6176 6177 public String fhirType() { 6178 return "TestScript.teardown"; 6179 6180 } 6181 6182 } 6183 6184 @Block() 6185 public static class TestScriptTeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 6186 /** 6187 * An operation would involve a REST request to a server. 6188 */ 6189 @Child(name = "operation", type = { 6190 TestScriptSetupActionOperationComponent.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6191 @Description(shortDefinition = "The teardown operation to perform", formalDefinition = "An operation would involve a REST request to a server.") 6192 protected TestScriptSetupActionOperationComponent operation; 6193 6194 private static final long serialVersionUID = 1684092023L; 6195 6196 /* 6197 * Constructor 6198 */ 6199 public TestScriptTeardownActionComponent() { 6200 super(); 6201 } 6202 6203 /** 6204 * @return {@link #operation} (An operation would involve a REST request to a 6205 * server.) 6206 */ 6207 public TestScriptSetupActionOperationComponent getOperation() { 6208 if (this.operation == null) 6209 if (Configuration.errorOnAutoCreate()) 6210 throw new Error("Attempt to auto-create TestScriptTeardownActionComponent.operation"); 6211 else if (Configuration.doAutoCreate()) 6212 this.operation = new TestScriptSetupActionOperationComponent(); // cc 6213 return this.operation; 6214 } 6215 6216 public boolean hasOperation() { 6217 return this.operation != null && !this.operation.isEmpty(); 6218 } 6219 6220 /** 6221 * @param value {@link #operation} (An operation would involve a REST request to 6222 * a server.) 6223 */ 6224 public TestScriptTeardownActionComponent setOperation(TestScriptSetupActionOperationComponent value) { 6225 this.operation = value; 6226 return this; 6227 } 6228 6229 protected void listChildren(List<Property> childrenList) { 6230 super.listChildren(childrenList); 6231 childrenList.add(new Property("operation", "@TestScript.setup.action.operation", 6232 "An operation would involve a REST request to a server.", 0, java.lang.Integer.MAX_VALUE, operation)); 6233 } 6234 6235 @Override 6236 public void setProperty(String name, Base value) throws FHIRException { 6237 if (name.equals("operation")) 6238 this.operation = (TestScriptSetupActionOperationComponent) value; // TestScriptSetupActionOperationComponent 6239 else 6240 super.setProperty(name, value); 6241 } 6242 6243 @Override 6244 public Base addChild(String name) throws FHIRException { 6245 if (name.equals("operation")) { 6246 this.operation = new TestScriptSetupActionOperationComponent(); 6247 return this.operation; 6248 } else 6249 return super.addChild(name); 6250 } 6251 6252 public TestScriptTeardownActionComponent copy() { 6253 TestScriptTeardownActionComponent dst = new TestScriptTeardownActionComponent(); 6254 copyValues(dst); 6255 dst.operation = operation == null ? null : operation.copy(); 6256 return dst; 6257 } 6258 6259 @Override 6260 public boolean equalsDeep(Base other) { 6261 if (!super.equalsDeep(other)) 6262 return false; 6263 if (!(other instanceof TestScriptTeardownActionComponent)) 6264 return false; 6265 TestScriptTeardownActionComponent o = (TestScriptTeardownActionComponent) other; 6266 return compareDeep(operation, o.operation, true); 6267 } 6268 6269 @Override 6270 public boolean equalsShallow(Base other) { 6271 if (!super.equalsShallow(other)) 6272 return false; 6273 if (!(other instanceof TestScriptTeardownActionComponent)) 6274 return false; 6275 TestScriptTeardownActionComponent o = (TestScriptTeardownActionComponent) other; 6276 return true; 6277 } 6278 6279 public boolean isEmpty() { 6280 return super.isEmpty() && (operation == null || operation.isEmpty()); 6281 } 6282 6283 public String fhirType() { 6284 return "TestScript.teardown.action"; 6285 6286 } 6287 6288 } 6289 6290 /** 6291 * An absolute URL that is used to identify this Test Script. This SHALL be a 6292 * URL, SHOULD be globally unique, and SHOULD be an address at which this Test 6293 * Script is (or will be) published. 6294 */ 6295 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 6296 @Description(shortDefinition = "Absolute URL used to reference this TestScript", formalDefinition = "An absolute URL that is used to identify this Test Script. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this Test Script is (or will be) published.") 6297 protected UriType url; 6298 6299 /** 6300 * The identifier that is used to identify this version of the TestScript. This 6301 * is an arbitrary value managed by the TestScript author manually. 6302 */ 6303 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 6304 @Description(shortDefinition = "Logical id for this version of the TestScript", formalDefinition = "The identifier that is used to identify this version of the TestScript. This is an arbitrary value managed by the TestScript author manually.") 6305 protected StringType version; 6306 6307 /** 6308 * A free text natural language name identifying the TestScript. 6309 */ 6310 @Child(name = "name", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 6311 @Description(shortDefinition = "Informal name for this TestScript", formalDefinition = "A free text natural language name identifying the TestScript.") 6312 protected StringType name; 6313 6314 /** 6315 * The status of the TestScript. 6316 */ 6317 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 6318 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the TestScript.") 6319 protected Enumeration<ConformanceResourceStatus> status; 6320 6321 /** 6322 * Identifier for the TestScript assigned for external purposes outside the 6323 * context of FHIR. 6324 */ 6325 @Child(name = "identifier", type = { 6326 Identifier.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 6327 @Description(shortDefinition = "External identifier", formalDefinition = "Identifier for the TestScript assigned for external purposes outside the context of FHIR.") 6328 protected Identifier identifier; 6329 6330 /** 6331 * This TestScript was authored for testing purposes (or 6332 * education/evaluation/marketing), and is not intended to be used for genuine 6333 * usage. 6334 */ 6335 @Child(name = "experimental", type = { 6336 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 6337 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "This TestScript was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 6338 protected BooleanType experimental; 6339 6340 /** 6341 * The name of the individual or organization that published the Test Script. 6342 */ 6343 @Child(name = "publisher", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 6344 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the Test Script.") 6345 protected StringType publisher; 6346 6347 /** 6348 * Contacts to assist a user in finding and communicating with the publisher. 6349 */ 6350 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6351 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 6352 protected List<TestScriptContactComponent> contact; 6353 6354 /** 6355 * The date this version of the test tcript was published. The date must change 6356 * when the business version changes, if it does, and it must change if the 6357 * status code changes. In addition, it should change when the substantive 6358 * content of the test cases change. 6359 */ 6360 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 6361 @Description(shortDefinition = "Date for this version of the TestScript", formalDefinition = "The date this version of the test tcript was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the test cases change.") 6362 protected DateTimeType date; 6363 6364 /** 6365 * A free text natural language description of the TestScript and its use. 6366 */ 6367 @Child(name = "description", type = { 6368 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 6369 @Description(shortDefinition = "Natural language description of the TestScript", formalDefinition = "A free text natural language description of the TestScript and its use.") 6370 protected StringType description; 6371 6372 /** 6373 * The content was developed with a focus and intent of supporting the contexts 6374 * that are listed. These terms may be used to assist with indexing and 6375 * searching of Test Scripts. 6376 */ 6377 @Child(name = "useContext", type = { 6378 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6379 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of Test Scripts.") 6380 protected List<CodeableConcept> useContext; 6381 6382 /** 6383 * Explains why this Test Script is needed and why it's been constrained as it 6384 * has. 6385 */ 6386 @Child(name = "requirements", type = { 6387 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 6388 @Description(shortDefinition = "Scope and Usage this Test Script is for", formalDefinition = "Explains why this Test Script is needed and why it's been constrained as it has.") 6389 protected StringType requirements; 6390 6391 /** 6392 * A copyright statement relating to the Test Script and/or its contents. 6393 * Copyright statements are generally legal restrictions on the use and 6394 * publishing of the details of the constraints and mappings. 6395 */ 6396 @Child(name = "copyright", type = { 6397 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 6398 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the Test Script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the constraints and mappings.") 6399 protected StringType copyright; 6400 6401 /** 6402 * The required capability must exist and are assumed to function correctly on 6403 * the FHIR server being tested. 6404 */ 6405 @Child(name = "metadata", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = false) 6406 @Description(shortDefinition = "Required capability that is assumed to function correctly on the FHIR server being tested", formalDefinition = "The required capability must exist and are assumed to function correctly on the FHIR server being tested.") 6407 protected TestScriptMetadataComponent metadata; 6408 6409 /** 6410 * If the tests apply to more than one FHIR server (e.g. cross-server 6411 * interoperability tests) then multiserver=true. Defaults to false if value is 6412 * unspecified. 6413 */ 6414 @Child(name = "multiserver", type = { 6415 BooleanType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 6416 @Description(shortDefinition = "Whether or not the tests apply to more than one FHIR server", formalDefinition = "If the tests apply to more than one FHIR server (e.g. cross-server interoperability tests) then multiserver=true. Defaults to false if value is unspecified.") 6417 protected BooleanType multiserver; 6418 6419 /** 6420 * Fixture in the test script - by reference (uri). All fixtures are required 6421 * for the test script to execute. 6422 */ 6423 @Child(name = "fixture", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6424 @Description(shortDefinition = "Fixture in the test script - by reference (uri)", formalDefinition = "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.") 6425 protected List<TestScriptFixtureComponent> fixture; 6426 6427 /** 6428 * Reference to the profile to be used for validation. 6429 */ 6430 @Child(name = "profile", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6431 @Description(shortDefinition = "Reference of the validation profile", formalDefinition = "Reference to the profile to be used for validation.") 6432 protected List<Reference> profile; 6433 /** 6434 * The actual objects that are the target of the reference (Reference to the 6435 * profile to be used for validation.) 6436 */ 6437 protected List<Resource> profileTarget; 6438 6439 /** 6440 * Variable is set based either on element value in response body or on header 6441 * field value in the response headers. 6442 */ 6443 @Child(name = "variable", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6444 @Description(shortDefinition = "Placeholder for evaluated elements", formalDefinition = "Variable is set based either on element value in response body or on header field value in the response headers.") 6445 protected List<TestScriptVariableComponent> variable; 6446 6447 /** 6448 * A series of required setup operations before tests are executed. 6449 */ 6450 @Child(name = "setup", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = false) 6451 @Description(shortDefinition = "A series of required setup operations before tests are executed", formalDefinition = "A series of required setup operations before tests are executed.") 6452 protected TestScriptSetupComponent setup; 6453 6454 /** 6455 * A test in this script. 6456 */ 6457 @Child(name = "test", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6458 @Description(shortDefinition = "A test in this script", formalDefinition = "A test in this script.") 6459 protected List<TestScriptTestComponent> test; 6460 6461 /** 6462 * A series of operations required to clean up after the all the tests are 6463 * executed (successfully or otherwise). 6464 */ 6465 @Child(name = "teardown", type = {}, order = 20, min = 0, max = 1, modifier = false, summary = false) 6466 @Description(shortDefinition = "A series of required clean up steps", formalDefinition = "A series of operations required to clean up after the all the tests are executed (successfully or otherwise).") 6467 protected TestScriptTeardownComponent teardown; 6468 6469 private static final long serialVersionUID = -2049162917L; 6470 6471 /* 6472 * Constructor 6473 */ 6474 public TestScript() { 6475 super(); 6476 } 6477 6478 /* 6479 * Constructor 6480 */ 6481 public TestScript(UriType url, StringType name, Enumeration<ConformanceResourceStatus> status) { 6482 super(); 6483 this.url = url; 6484 this.name = name; 6485 this.status = status; 6486 } 6487 6488 /** 6489 * @return {@link #url} (An absolute URL that is used to identify this Test 6490 * Script. This SHALL be a URL, SHOULD be globally unique, and SHOULD be 6491 * an address at which this Test Script is (or will be) published.). 6492 * This is the underlying object with id, value and extensions. The 6493 * accessor "getUrl" gives direct access to the value 6494 */ 6495 public UriType getUrlElement() { 6496 if (this.url == null) 6497 if (Configuration.errorOnAutoCreate()) 6498 throw new Error("Attempt to auto-create TestScript.url"); 6499 else if (Configuration.doAutoCreate()) 6500 this.url = new UriType(); // bb 6501 return this.url; 6502 } 6503 6504 public boolean hasUrlElement() { 6505 return this.url != null && !this.url.isEmpty(); 6506 } 6507 6508 public boolean hasUrl() { 6509 return this.url != null && !this.url.isEmpty(); 6510 } 6511 6512 /** 6513 * @param value {@link #url} (An absolute URL that is used to identify this Test 6514 * Script. This SHALL be a URL, SHOULD be globally unique, and 6515 * SHOULD be an address at which this Test Script is (or will be) 6516 * published.). This is the underlying object with id, value and 6517 * extensions. The accessor "getUrl" gives direct access to the 6518 * value 6519 */ 6520 public TestScript setUrlElement(UriType value) { 6521 this.url = value; 6522 return this; 6523 } 6524 6525 /** 6526 * @return An absolute URL that is used to identify this Test Script. This SHALL 6527 * be a URL, SHOULD be globally unique, and SHOULD be an address at 6528 * which this Test Script is (or will be) published. 6529 */ 6530 public String getUrl() { 6531 return this.url == null ? null : this.url.getValue(); 6532 } 6533 6534 /** 6535 * @param value An absolute URL that is used to identify this Test Script. This 6536 * SHALL be a URL, SHOULD be globally unique, and SHOULD be an 6537 * address at which this Test Script is (or will be) published. 6538 */ 6539 public TestScript setUrl(String value) { 6540 if (this.url == null) 6541 this.url = new UriType(); 6542 this.url.setValue(value); 6543 return this; 6544 } 6545 6546 /** 6547 * @return {@link #version} (The identifier that is used to identify this 6548 * version of the TestScript. This is an arbitrary value managed by the 6549 * TestScript author manually.). This is the underlying object with id, 6550 * value and extensions. The accessor "getVersion" gives direct access 6551 * to the value 6552 */ 6553 public StringType getVersionElement() { 6554 if (this.version == null) 6555 if (Configuration.errorOnAutoCreate()) 6556 throw new Error("Attempt to auto-create TestScript.version"); 6557 else if (Configuration.doAutoCreate()) 6558 this.version = new StringType(); // bb 6559 return this.version; 6560 } 6561 6562 public boolean hasVersionElement() { 6563 return this.version != null && !this.version.isEmpty(); 6564 } 6565 6566 public boolean hasVersion() { 6567 return this.version != null && !this.version.isEmpty(); 6568 } 6569 6570 /** 6571 * @param value {@link #version} (The identifier that is used to identify this 6572 * version of the TestScript. This is an arbitrary value managed by 6573 * the TestScript author manually.). This is the underlying object 6574 * with id, value and extensions. The accessor "getVersion" gives 6575 * direct access to the value 6576 */ 6577 public TestScript setVersionElement(StringType value) { 6578 this.version = value; 6579 return this; 6580 } 6581 6582 /** 6583 * @return The identifier that is used to identify this version of the 6584 * TestScript. This is an arbitrary value managed by the TestScript 6585 * author manually. 6586 */ 6587 public String getVersion() { 6588 return this.version == null ? null : this.version.getValue(); 6589 } 6590 6591 /** 6592 * @param value The identifier that is used to identify this version of the 6593 * TestScript. This is an arbitrary value managed by the TestScript 6594 * author manually. 6595 */ 6596 public TestScript setVersion(String value) { 6597 if (Utilities.noString(value)) 6598 this.version = null; 6599 else { 6600 if (this.version == null) 6601 this.version = new StringType(); 6602 this.version.setValue(value); 6603 } 6604 return this; 6605 } 6606 6607 /** 6608 * @return {@link #name} (A free text natural language name identifying the 6609 * TestScript.). This is the underlying object with id, value and 6610 * extensions. The accessor "getName" gives direct access to the value 6611 */ 6612 public StringType getNameElement() { 6613 if (this.name == null) 6614 if (Configuration.errorOnAutoCreate()) 6615 throw new Error("Attempt to auto-create TestScript.name"); 6616 else if (Configuration.doAutoCreate()) 6617 this.name = new StringType(); // bb 6618 return this.name; 6619 } 6620 6621 public boolean hasNameElement() { 6622 return this.name != null && !this.name.isEmpty(); 6623 } 6624 6625 public boolean hasName() { 6626 return this.name != null && !this.name.isEmpty(); 6627 } 6628 6629 /** 6630 * @param value {@link #name} (A free text natural language name identifying the 6631 * TestScript.). This is the underlying object with id, value and 6632 * extensions. The accessor "getName" gives direct access to the 6633 * value 6634 */ 6635 public TestScript setNameElement(StringType value) { 6636 this.name = value; 6637 return this; 6638 } 6639 6640 /** 6641 * @return A free text natural language name identifying the TestScript. 6642 */ 6643 public String getName() { 6644 return this.name == null ? null : this.name.getValue(); 6645 } 6646 6647 /** 6648 * @param value A free text natural language name identifying the TestScript. 6649 */ 6650 public TestScript setName(String value) { 6651 if (this.name == null) 6652 this.name = new StringType(); 6653 this.name.setValue(value); 6654 return this; 6655 } 6656 6657 /** 6658 * @return {@link #status} (The status of the TestScript.). This is the 6659 * underlying object with id, value and extensions. The accessor 6660 * "getStatus" gives direct access to the value 6661 */ 6662 public Enumeration<ConformanceResourceStatus> getStatusElement() { 6663 if (this.status == null) 6664 if (Configuration.errorOnAutoCreate()) 6665 throw new Error("Attempt to auto-create TestScript.status"); 6666 else if (Configuration.doAutoCreate()) 6667 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 6668 return this.status; 6669 } 6670 6671 public boolean hasStatusElement() { 6672 return this.status != null && !this.status.isEmpty(); 6673 } 6674 6675 public boolean hasStatus() { 6676 return this.status != null && !this.status.isEmpty(); 6677 } 6678 6679 /** 6680 * @param value {@link #status} (The status of the TestScript.). This is the 6681 * underlying object with id, value and extensions. The accessor 6682 * "getStatus" gives direct access to the value 6683 */ 6684 public TestScript setStatusElement(Enumeration<ConformanceResourceStatus> value) { 6685 this.status = value; 6686 return this; 6687 } 6688 6689 /** 6690 * @return The status of the TestScript. 6691 */ 6692 public ConformanceResourceStatus getStatus() { 6693 return this.status == null ? null : this.status.getValue(); 6694 } 6695 6696 /** 6697 * @param value The status of the TestScript. 6698 */ 6699 public TestScript setStatus(ConformanceResourceStatus value) { 6700 if (this.status == null) 6701 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 6702 this.status.setValue(value); 6703 return this; 6704 } 6705 6706 /** 6707 * @return {@link #identifier} (Identifier for the TestScript assigned for 6708 * external purposes outside the context of FHIR.) 6709 */ 6710 public Identifier getIdentifier() { 6711 if (this.identifier == null) 6712 if (Configuration.errorOnAutoCreate()) 6713 throw new Error("Attempt to auto-create TestScript.identifier"); 6714 else if (Configuration.doAutoCreate()) 6715 this.identifier = new Identifier(); // cc 6716 return this.identifier; 6717 } 6718 6719 public boolean hasIdentifier() { 6720 return this.identifier != null && !this.identifier.isEmpty(); 6721 } 6722 6723 /** 6724 * @param value {@link #identifier} (Identifier for the TestScript assigned for 6725 * external purposes outside the context of FHIR.) 6726 */ 6727 public TestScript setIdentifier(Identifier value) { 6728 this.identifier = value; 6729 return this; 6730 } 6731 6732 /** 6733 * @return {@link #experimental} (This TestScript was authored for testing 6734 * purposes (or education/evaluation/marketing), and is not intended to 6735 * be used for genuine usage.). This is the underlying object with id, 6736 * value and extensions. The accessor "getExperimental" gives direct 6737 * access to the value 6738 */ 6739 public BooleanType getExperimentalElement() { 6740 if (this.experimental == null) 6741 if (Configuration.errorOnAutoCreate()) 6742 throw new Error("Attempt to auto-create TestScript.experimental"); 6743 else if (Configuration.doAutoCreate()) 6744 this.experimental = new BooleanType(); // bb 6745 return this.experimental; 6746 } 6747 6748 public boolean hasExperimentalElement() { 6749 return this.experimental != null && !this.experimental.isEmpty(); 6750 } 6751 6752 public boolean hasExperimental() { 6753 return this.experimental != null && !this.experimental.isEmpty(); 6754 } 6755 6756 /** 6757 * @param value {@link #experimental} (This TestScript was authored for testing 6758 * purposes (or education/evaluation/marketing), and is not 6759 * intended to be used for genuine usage.). This is the underlying 6760 * object with id, value and extensions. The accessor 6761 * "getExperimental" gives direct access to the value 6762 */ 6763 public TestScript setExperimentalElement(BooleanType value) { 6764 this.experimental = value; 6765 return this; 6766 } 6767 6768 /** 6769 * @return This TestScript was authored for testing purposes (or 6770 * education/evaluation/marketing), and is not intended to be used for 6771 * genuine usage. 6772 */ 6773 public boolean getExperimental() { 6774 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 6775 } 6776 6777 /** 6778 * @param value This TestScript was authored for testing purposes (or 6779 * education/evaluation/marketing), and is not intended to be used 6780 * for genuine usage. 6781 */ 6782 public TestScript setExperimental(boolean value) { 6783 if (this.experimental == null) 6784 this.experimental = new BooleanType(); 6785 this.experimental.setValue(value); 6786 return this; 6787 } 6788 6789 /** 6790 * @return {@link #publisher} (The name of the individual or organization that 6791 * published the Test Script.). This is the underlying object with id, 6792 * value and extensions. The accessor "getPublisher" gives direct access 6793 * to the value 6794 */ 6795 public StringType getPublisherElement() { 6796 if (this.publisher == null) 6797 if (Configuration.errorOnAutoCreate()) 6798 throw new Error("Attempt to auto-create TestScript.publisher"); 6799 else if (Configuration.doAutoCreate()) 6800 this.publisher = new StringType(); // bb 6801 return this.publisher; 6802 } 6803 6804 public boolean hasPublisherElement() { 6805 return this.publisher != null && !this.publisher.isEmpty(); 6806 } 6807 6808 public boolean hasPublisher() { 6809 return this.publisher != null && !this.publisher.isEmpty(); 6810 } 6811 6812 /** 6813 * @param value {@link #publisher} (The name of the individual or organization 6814 * that published the Test Script.). This is the underlying object 6815 * with id, value and extensions. The accessor "getPublisher" gives 6816 * direct access to the value 6817 */ 6818 public TestScript setPublisherElement(StringType value) { 6819 this.publisher = value; 6820 return this; 6821 } 6822 6823 /** 6824 * @return The name of the individual or organization that published the Test 6825 * Script. 6826 */ 6827 public String getPublisher() { 6828 return this.publisher == null ? null : this.publisher.getValue(); 6829 } 6830 6831 /** 6832 * @param value The name of the individual or organization that published the 6833 * Test Script. 6834 */ 6835 public TestScript setPublisher(String value) { 6836 if (Utilities.noString(value)) 6837 this.publisher = null; 6838 else { 6839 if (this.publisher == null) 6840 this.publisher = new StringType(); 6841 this.publisher.setValue(value); 6842 } 6843 return this; 6844 } 6845 6846 /** 6847 * @return {@link #contact} (Contacts to assist a user in finding and 6848 * communicating with the publisher.) 6849 */ 6850 public List<TestScriptContactComponent> getContact() { 6851 if (this.contact == null) 6852 this.contact = new ArrayList<TestScriptContactComponent>(); 6853 return this.contact; 6854 } 6855 6856 public boolean hasContact() { 6857 if (this.contact == null) 6858 return false; 6859 for (TestScriptContactComponent item : this.contact) 6860 if (!item.isEmpty()) 6861 return true; 6862 return false; 6863 } 6864 6865 /** 6866 * @return {@link #contact} (Contacts to assist a user in finding and 6867 * communicating with the publisher.) 6868 */ 6869 // syntactic sugar 6870 public TestScriptContactComponent addContact() { // 3 6871 TestScriptContactComponent t = new TestScriptContactComponent(); 6872 if (this.contact == null) 6873 this.contact = new ArrayList<TestScriptContactComponent>(); 6874 this.contact.add(t); 6875 return t; 6876 } 6877 6878 // syntactic sugar 6879 public TestScript addContact(TestScriptContactComponent t) { // 3 6880 if (t == null) 6881 return this; 6882 if (this.contact == null) 6883 this.contact = new ArrayList<TestScriptContactComponent>(); 6884 this.contact.add(t); 6885 return this; 6886 } 6887 6888 /** 6889 * @return {@link #date} (The date this version of the test tcript was 6890 * published. The date must change when the business version changes, if 6891 * it does, and it must change if the status code changes. In addition, 6892 * it should change when the substantive content of the test cases 6893 * change.). This is the underlying object with id, value and 6894 * extensions. The accessor "getDate" gives direct access to the value 6895 */ 6896 public DateTimeType getDateElement() { 6897 if (this.date == null) 6898 if (Configuration.errorOnAutoCreate()) 6899 throw new Error("Attempt to auto-create TestScript.date"); 6900 else if (Configuration.doAutoCreate()) 6901 this.date = new DateTimeType(); // bb 6902 return this.date; 6903 } 6904 6905 public boolean hasDateElement() { 6906 return this.date != null && !this.date.isEmpty(); 6907 } 6908 6909 public boolean hasDate() { 6910 return this.date != null && !this.date.isEmpty(); 6911 } 6912 6913 /** 6914 * @param value {@link #date} (The date this version of the test tcript was 6915 * published. The date must change when the business version 6916 * changes, if it does, and it must change if the status code 6917 * changes. In addition, it should change when the substantive 6918 * content of the test cases change.). This is the underlying 6919 * object with id, value and extensions. The accessor "getDate" 6920 * gives direct access to the value 6921 */ 6922 public TestScript setDateElement(DateTimeType value) { 6923 this.date = value; 6924 return this; 6925 } 6926 6927 /** 6928 * @return The date this version of the test tcript was published. The date must 6929 * change when the business version changes, if it does, and it must 6930 * change if the status code changes. In addition, it should change when 6931 * the substantive content of the test cases change. 6932 */ 6933 public Date getDate() { 6934 return this.date == null ? null : this.date.getValue(); 6935 } 6936 6937 /** 6938 * @param value The date this version of the test tcript was published. The date 6939 * must change when the business version changes, if it does, and 6940 * it must change if the status code changes. In addition, it 6941 * should change when the substantive content of the test cases 6942 * change. 6943 */ 6944 public TestScript setDate(Date value) { 6945 if (value == null) 6946 this.date = null; 6947 else { 6948 if (this.date == null) 6949 this.date = new DateTimeType(); 6950 this.date.setValue(value); 6951 } 6952 return this; 6953 } 6954 6955 /** 6956 * @return {@link #description} (A free text natural language description of the 6957 * TestScript and its use.). This is the underlying object with id, 6958 * value and extensions. The accessor "getDescription" gives direct 6959 * access to the value 6960 */ 6961 public StringType getDescriptionElement() { 6962 if (this.description == null) 6963 if (Configuration.errorOnAutoCreate()) 6964 throw new Error("Attempt to auto-create TestScript.description"); 6965 else if (Configuration.doAutoCreate()) 6966 this.description = new StringType(); // bb 6967 return this.description; 6968 } 6969 6970 public boolean hasDescriptionElement() { 6971 return this.description != null && !this.description.isEmpty(); 6972 } 6973 6974 public boolean hasDescription() { 6975 return this.description != null && !this.description.isEmpty(); 6976 } 6977 6978 /** 6979 * @param value {@link #description} (A free text natural language description 6980 * of the TestScript and its use.). This is the underlying object 6981 * with id, value and extensions. The accessor "getDescription" 6982 * gives direct access to the value 6983 */ 6984 public TestScript setDescriptionElement(StringType value) { 6985 this.description = value; 6986 return this; 6987 } 6988 6989 /** 6990 * @return A free text natural language description of the TestScript and its 6991 * use. 6992 */ 6993 public String getDescription() { 6994 return this.description == null ? null : this.description.getValue(); 6995 } 6996 6997 /** 6998 * @param value A free text natural language description of the TestScript and 6999 * its use. 7000 */ 7001 public TestScript setDescription(String value) { 7002 if (Utilities.noString(value)) 7003 this.description = null; 7004 else { 7005 if (this.description == null) 7006 this.description = new StringType(); 7007 this.description.setValue(value); 7008 } 7009 return this; 7010 } 7011 7012 /** 7013 * @return {@link #useContext} (The content was developed with a focus and 7014 * intent of supporting the contexts that are listed. These terms may be 7015 * used to assist with indexing and searching of Test Scripts.) 7016 */ 7017 public List<CodeableConcept> getUseContext() { 7018 if (this.useContext == null) 7019 this.useContext = new ArrayList<CodeableConcept>(); 7020 return this.useContext; 7021 } 7022 7023 public boolean hasUseContext() { 7024 if (this.useContext == null) 7025 return false; 7026 for (CodeableConcept item : this.useContext) 7027 if (!item.isEmpty()) 7028 return true; 7029 return false; 7030 } 7031 7032 /** 7033 * @return {@link #useContext} (The content was developed with a focus and 7034 * intent of supporting the contexts that are listed. These terms may be 7035 * used to assist with indexing and searching of Test Scripts.) 7036 */ 7037 // syntactic sugar 7038 public CodeableConcept addUseContext() { // 3 7039 CodeableConcept t = new CodeableConcept(); 7040 if (this.useContext == null) 7041 this.useContext = new ArrayList<CodeableConcept>(); 7042 this.useContext.add(t); 7043 return t; 7044 } 7045 7046 // syntactic sugar 7047 public TestScript addUseContext(CodeableConcept t) { // 3 7048 if (t == null) 7049 return this; 7050 if (this.useContext == null) 7051 this.useContext = new ArrayList<CodeableConcept>(); 7052 this.useContext.add(t); 7053 return this; 7054 } 7055 7056 /** 7057 * @return {@link #requirements} (Explains why this Test Script is needed and 7058 * why it's been constrained as it has.). This is the underlying object 7059 * with id, value and extensions. The accessor "getRequirements" gives 7060 * direct access to the value 7061 */ 7062 public StringType getRequirementsElement() { 7063 if (this.requirements == null) 7064 if (Configuration.errorOnAutoCreate()) 7065 throw new Error("Attempt to auto-create TestScript.requirements"); 7066 else if (Configuration.doAutoCreate()) 7067 this.requirements = new StringType(); // bb 7068 return this.requirements; 7069 } 7070 7071 public boolean hasRequirementsElement() { 7072 return this.requirements != null && !this.requirements.isEmpty(); 7073 } 7074 7075 public boolean hasRequirements() { 7076 return this.requirements != null && !this.requirements.isEmpty(); 7077 } 7078 7079 /** 7080 * @param value {@link #requirements} (Explains why this Test Script is needed 7081 * and why it's been constrained as it has.). This is the 7082 * underlying object with id, value and extensions. The accessor 7083 * "getRequirements" gives direct access to the value 7084 */ 7085 public TestScript setRequirementsElement(StringType value) { 7086 this.requirements = value; 7087 return this; 7088 } 7089 7090 /** 7091 * @return Explains why this Test Script is needed and why it's been constrained 7092 * as it has. 7093 */ 7094 public String getRequirements() { 7095 return this.requirements == null ? null : this.requirements.getValue(); 7096 } 7097 7098 /** 7099 * @param value Explains why this Test Script is needed and why it's been 7100 * constrained as it has. 7101 */ 7102 public TestScript setRequirements(String value) { 7103 if (Utilities.noString(value)) 7104 this.requirements = null; 7105 else { 7106 if (this.requirements == null) 7107 this.requirements = new StringType(); 7108 this.requirements.setValue(value); 7109 } 7110 return this; 7111 } 7112 7113 /** 7114 * @return {@link #copyright} (A copyright statement relating to the Test Script 7115 * and/or its contents. Copyright statements are generally legal 7116 * restrictions on the use and publishing of the details of the 7117 * constraints and mappings.). This is the underlying object with id, 7118 * value and extensions. The accessor "getCopyright" gives direct access 7119 * to the value 7120 */ 7121 public StringType getCopyrightElement() { 7122 if (this.copyright == null) 7123 if (Configuration.errorOnAutoCreate()) 7124 throw new Error("Attempt to auto-create TestScript.copyright"); 7125 else if (Configuration.doAutoCreate()) 7126 this.copyright = new StringType(); // bb 7127 return this.copyright; 7128 } 7129 7130 public boolean hasCopyrightElement() { 7131 return this.copyright != null && !this.copyright.isEmpty(); 7132 } 7133 7134 public boolean hasCopyright() { 7135 return this.copyright != null && !this.copyright.isEmpty(); 7136 } 7137 7138 /** 7139 * @param value {@link #copyright} (A copyright statement relating to the Test 7140 * Script and/or its contents. Copyright statements are generally 7141 * legal restrictions on the use and publishing of the details of 7142 * the constraints and mappings.). This is the underlying object 7143 * with id, value and extensions. The accessor "getCopyright" gives 7144 * direct access to the value 7145 */ 7146 public TestScript setCopyrightElement(StringType value) { 7147 this.copyright = value; 7148 return this; 7149 } 7150 7151 /** 7152 * @return A copyright statement relating to the Test Script and/or its 7153 * contents. Copyright statements are generally legal restrictions on 7154 * the use and publishing of the details of the constraints and 7155 * mappings. 7156 */ 7157 public String getCopyright() { 7158 return this.copyright == null ? null : this.copyright.getValue(); 7159 } 7160 7161 /** 7162 * @param value A copyright statement relating to the Test Script and/or its 7163 * contents. Copyright statements are generally legal restrictions 7164 * on the use and publishing of the details of the constraints and 7165 * mappings. 7166 */ 7167 public TestScript setCopyright(String value) { 7168 if (Utilities.noString(value)) 7169 this.copyright = null; 7170 else { 7171 if (this.copyright == null) 7172 this.copyright = new StringType(); 7173 this.copyright.setValue(value); 7174 } 7175 return this; 7176 } 7177 7178 /** 7179 * @return {@link #metadata} (The required capability must exist and are assumed 7180 * to function correctly on the FHIR server being tested.) 7181 */ 7182 public TestScriptMetadataComponent getMetadata() { 7183 if (this.metadata == null) 7184 if (Configuration.errorOnAutoCreate()) 7185 throw new Error("Attempt to auto-create TestScript.metadata"); 7186 else if (Configuration.doAutoCreate()) 7187 this.metadata = new TestScriptMetadataComponent(); // cc 7188 return this.metadata; 7189 } 7190 7191 public boolean hasMetadata() { 7192 return this.metadata != null && !this.metadata.isEmpty(); 7193 } 7194 7195 /** 7196 * @param value {@link #metadata} (The required capability must exist and are 7197 * assumed to function correctly on the FHIR server being tested.) 7198 */ 7199 public TestScript setMetadata(TestScriptMetadataComponent value) { 7200 this.metadata = value; 7201 return this; 7202 } 7203 7204 /** 7205 * @return {@link #multiserver} (If the tests apply to more than one FHIR server 7206 * (e.g. cross-server interoperability tests) then multiserver=true. 7207 * Defaults to false if value is unspecified.). This is the underlying 7208 * object with id, value and extensions. The accessor "getMultiserver" 7209 * gives direct access to the value 7210 */ 7211 public BooleanType getMultiserverElement() { 7212 if (this.multiserver == null) 7213 if (Configuration.errorOnAutoCreate()) 7214 throw new Error("Attempt to auto-create TestScript.multiserver"); 7215 else if (Configuration.doAutoCreate()) 7216 this.multiserver = new BooleanType(); // bb 7217 return this.multiserver; 7218 } 7219 7220 public boolean hasMultiserverElement() { 7221 return this.multiserver != null && !this.multiserver.isEmpty(); 7222 } 7223 7224 public boolean hasMultiserver() { 7225 return this.multiserver != null && !this.multiserver.isEmpty(); 7226 } 7227 7228 /** 7229 * @param value {@link #multiserver} (If the tests apply to more than one FHIR 7230 * server (e.g. cross-server interoperability tests) then 7231 * multiserver=true. Defaults to false if value is unspecified.). 7232 * This is the underlying object with id, value and extensions. The 7233 * accessor "getMultiserver" gives direct access to the value 7234 */ 7235 public TestScript setMultiserverElement(BooleanType value) { 7236 this.multiserver = value; 7237 return this; 7238 } 7239 7240 /** 7241 * @return If the tests apply to more than one FHIR server (e.g. cross-server 7242 * interoperability tests) then multiserver=true. Defaults to false if 7243 * value is unspecified. 7244 */ 7245 public boolean getMultiserver() { 7246 return this.multiserver == null || this.multiserver.isEmpty() ? false : this.multiserver.getValue(); 7247 } 7248 7249 /** 7250 * @param value If the tests apply to more than one FHIR server (e.g. 7251 * cross-server interoperability tests) then multiserver=true. 7252 * Defaults to false if value is unspecified. 7253 */ 7254 public TestScript setMultiserver(boolean value) { 7255 if (this.multiserver == null) 7256 this.multiserver = new BooleanType(); 7257 this.multiserver.setValue(value); 7258 return this; 7259 } 7260 7261 /** 7262 * @return {@link #fixture} (Fixture in the test script - by reference (uri). 7263 * All fixtures are required for the test script to execute.) 7264 */ 7265 public List<TestScriptFixtureComponent> getFixture() { 7266 if (this.fixture == null) 7267 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 7268 return this.fixture; 7269 } 7270 7271 public boolean hasFixture() { 7272 if (this.fixture == null) 7273 return false; 7274 for (TestScriptFixtureComponent item : this.fixture) 7275 if (!item.isEmpty()) 7276 return true; 7277 return false; 7278 } 7279 7280 /** 7281 * @return {@link #fixture} (Fixture in the test script - by reference (uri). 7282 * All fixtures are required for the test script to execute.) 7283 */ 7284 // syntactic sugar 7285 public TestScriptFixtureComponent addFixture() { // 3 7286 TestScriptFixtureComponent t = new TestScriptFixtureComponent(); 7287 if (this.fixture == null) 7288 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 7289 this.fixture.add(t); 7290 return t; 7291 } 7292 7293 // syntactic sugar 7294 public TestScript addFixture(TestScriptFixtureComponent t) { // 3 7295 if (t == null) 7296 return this; 7297 if (this.fixture == null) 7298 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 7299 this.fixture.add(t); 7300 return this; 7301 } 7302 7303 /** 7304 * @return {@link #profile} (Reference to the profile to be used for 7305 * validation.) 7306 */ 7307 public List<Reference> getProfile() { 7308 if (this.profile == null) 7309 this.profile = new ArrayList<Reference>(); 7310 return this.profile; 7311 } 7312 7313 public boolean hasProfile() { 7314 if (this.profile == null) 7315 return false; 7316 for (Reference item : this.profile) 7317 if (!item.isEmpty()) 7318 return true; 7319 return false; 7320 } 7321 7322 /** 7323 * @return {@link #profile} (Reference to the profile to be used for 7324 * validation.) 7325 */ 7326 // syntactic sugar 7327 public Reference addProfile() { // 3 7328 Reference t = new Reference(); 7329 if (this.profile == null) 7330 this.profile = new ArrayList<Reference>(); 7331 this.profile.add(t); 7332 return t; 7333 } 7334 7335 // syntactic sugar 7336 public TestScript addProfile(Reference t) { // 3 7337 if (t == null) 7338 return this; 7339 if (this.profile == null) 7340 this.profile = new ArrayList<Reference>(); 7341 this.profile.add(t); 7342 return this; 7343 } 7344 7345 /** 7346 * @return {@link #profile} (The actual objects that are the target of the 7347 * reference. The reference library doesn't populate this, but you can 7348 * use this to hold the resources if you resolvethemt. Reference to the 7349 * profile to be used for validation.) 7350 */ 7351 public List<Resource> getProfileTarget() { 7352 if (this.profileTarget == null) 7353 this.profileTarget = new ArrayList<Resource>(); 7354 return this.profileTarget; 7355 } 7356 7357 /** 7358 * @return {@link #variable} (Variable is set based either on element value in 7359 * response body or on header field value in the response headers.) 7360 */ 7361 public List<TestScriptVariableComponent> getVariable() { 7362 if (this.variable == null) 7363 this.variable = new ArrayList<TestScriptVariableComponent>(); 7364 return this.variable; 7365 } 7366 7367 public boolean hasVariable() { 7368 if (this.variable == null) 7369 return false; 7370 for (TestScriptVariableComponent item : this.variable) 7371 if (!item.isEmpty()) 7372 return true; 7373 return false; 7374 } 7375 7376 /** 7377 * @return {@link #variable} (Variable is set based either on element value in 7378 * response body or on header field value in the response headers.) 7379 */ 7380 // syntactic sugar 7381 public TestScriptVariableComponent addVariable() { // 3 7382 TestScriptVariableComponent t = new TestScriptVariableComponent(); 7383 if (this.variable == null) 7384 this.variable = new ArrayList<TestScriptVariableComponent>(); 7385 this.variable.add(t); 7386 return t; 7387 } 7388 7389 // syntactic sugar 7390 public TestScript addVariable(TestScriptVariableComponent t) { // 3 7391 if (t == null) 7392 return this; 7393 if (this.variable == null) 7394 this.variable = new ArrayList<TestScriptVariableComponent>(); 7395 this.variable.add(t); 7396 return this; 7397 } 7398 7399 /** 7400 * @return {@link #setup} (A series of required setup operations before tests 7401 * are executed.) 7402 */ 7403 public TestScriptSetupComponent getSetup() { 7404 if (this.setup == null) 7405 if (Configuration.errorOnAutoCreate()) 7406 throw new Error("Attempt to auto-create TestScript.setup"); 7407 else if (Configuration.doAutoCreate()) 7408 this.setup = new TestScriptSetupComponent(); // cc 7409 return this.setup; 7410 } 7411 7412 public boolean hasSetup() { 7413 return this.setup != null && !this.setup.isEmpty(); 7414 } 7415 7416 /** 7417 * @param value {@link #setup} (A series of required setup operations before 7418 * tests are executed.) 7419 */ 7420 public TestScript setSetup(TestScriptSetupComponent value) { 7421 this.setup = value; 7422 return this; 7423 } 7424 7425 /** 7426 * @return {@link #test} (A test in this script.) 7427 */ 7428 public List<TestScriptTestComponent> getTest() { 7429 if (this.test == null) 7430 this.test = new ArrayList<TestScriptTestComponent>(); 7431 return this.test; 7432 } 7433 7434 public boolean hasTest() { 7435 if (this.test == null) 7436 return false; 7437 for (TestScriptTestComponent item : this.test) 7438 if (!item.isEmpty()) 7439 return true; 7440 return false; 7441 } 7442 7443 /** 7444 * @return {@link #test} (A test in this script.) 7445 */ 7446 // syntactic sugar 7447 public TestScriptTestComponent addTest() { // 3 7448 TestScriptTestComponent t = new TestScriptTestComponent(); 7449 if (this.test == null) 7450 this.test = new ArrayList<TestScriptTestComponent>(); 7451 this.test.add(t); 7452 return t; 7453 } 7454 7455 // syntactic sugar 7456 public TestScript addTest(TestScriptTestComponent t) { // 3 7457 if (t == null) 7458 return this; 7459 if (this.test == null) 7460 this.test = new ArrayList<TestScriptTestComponent>(); 7461 this.test.add(t); 7462 return this; 7463 } 7464 7465 /** 7466 * @return {@link #teardown} (A series of operations required to clean up after 7467 * the all the tests are executed (successfully or otherwise).) 7468 */ 7469 public TestScriptTeardownComponent getTeardown() { 7470 if (this.teardown == null) 7471 if (Configuration.errorOnAutoCreate()) 7472 throw new Error("Attempt to auto-create TestScript.teardown"); 7473 else if (Configuration.doAutoCreate()) 7474 this.teardown = new TestScriptTeardownComponent(); // cc 7475 return this.teardown; 7476 } 7477 7478 public boolean hasTeardown() { 7479 return this.teardown != null && !this.teardown.isEmpty(); 7480 } 7481 7482 /** 7483 * @param value {@link #teardown} (A series of operations required to clean up 7484 * after the all the tests are executed (successfully or 7485 * otherwise).) 7486 */ 7487 public TestScript setTeardown(TestScriptTeardownComponent value) { 7488 this.teardown = value; 7489 return this; 7490 } 7491 7492 protected void listChildren(List<Property> childrenList) { 7493 super.listChildren(childrenList); 7494 childrenList.add(new Property("url", "uri", 7495 "An absolute URL that is used to identify this Test Script. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this Test Script is (or will be) published.", 7496 0, java.lang.Integer.MAX_VALUE, url)); 7497 childrenList.add(new Property("version", "string", 7498 "The identifier that is used to identify this version of the TestScript. This is an arbitrary value managed by the TestScript author manually.", 7499 0, java.lang.Integer.MAX_VALUE, version)); 7500 childrenList.add(new Property("name", "string", "A free text natural language name identifying the TestScript.", 0, 7501 java.lang.Integer.MAX_VALUE, name)); 7502 childrenList 7503 .add(new Property("status", "code", "The status of the TestScript.", 0, java.lang.Integer.MAX_VALUE, status)); 7504 childrenList.add(new Property("identifier", "Identifier", 7505 "Identifier for the TestScript assigned for external purposes outside the context of FHIR.", 0, 7506 java.lang.Integer.MAX_VALUE, identifier)); 7507 childrenList.add(new Property("experimental", "boolean", 7508 "This TestScript was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 7509 0, java.lang.Integer.MAX_VALUE, experimental)); 7510 childrenList.add(new Property("publisher", "string", 7511 "The name of the individual or organization that published the Test Script.", 0, java.lang.Integer.MAX_VALUE, 7512 publisher)); 7513 childrenList 7514 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 7515 0, java.lang.Integer.MAX_VALUE, contact)); 7516 childrenList.add(new Property("date", "dateTime", 7517 "The date this version of the test tcript was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the test cases change.", 7518 0, java.lang.Integer.MAX_VALUE, date)); 7519 childrenList.add( 7520 new Property("description", "string", "A free text natural language description of the TestScript and its use.", 7521 0, java.lang.Integer.MAX_VALUE, description)); 7522 childrenList.add(new Property("useContext", "CodeableConcept", 7523 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of Test Scripts.", 7524 0, java.lang.Integer.MAX_VALUE, useContext)); 7525 childrenList.add(new Property("requirements", "string", 7526 "Explains why this Test Script is needed and why it's been constrained as it has.", 0, 7527 java.lang.Integer.MAX_VALUE, requirements)); 7528 childrenList.add(new Property("copyright", "string", 7529 "A copyright statement relating to the Test Script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the constraints and mappings.", 7530 0, java.lang.Integer.MAX_VALUE, copyright)); 7531 childrenList.add(new Property("metadata", "", 7532 "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 0, 7533 java.lang.Integer.MAX_VALUE, metadata)); 7534 childrenList.add(new Property("multiserver", "boolean", 7535 "If the tests apply to more than one FHIR server (e.g. cross-server interoperability tests) then multiserver=true. Defaults to false if value is unspecified.", 7536 0, java.lang.Integer.MAX_VALUE, multiserver)); 7537 childrenList.add(new Property("fixture", "", 7538 "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 0, 7539 java.lang.Integer.MAX_VALUE, fixture)); 7540 childrenList.add(new Property("profile", "Reference(Any)", "Reference to the profile to be used for validation.", 0, 7541 java.lang.Integer.MAX_VALUE, profile)); 7542 childrenList.add(new Property("variable", "", 7543 "Variable is set based either on element value in response body or on header field value in the response headers.", 7544 0, java.lang.Integer.MAX_VALUE, variable)); 7545 childrenList.add(new Property("setup", "", "A series of required setup operations before tests are executed.", 0, 7546 java.lang.Integer.MAX_VALUE, setup)); 7547 childrenList.add(new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test)); 7548 childrenList.add(new Property("teardown", "", 7549 "A series of operations required to clean up after the all the tests are executed (successfully or otherwise).", 7550 0, java.lang.Integer.MAX_VALUE, teardown)); 7551 } 7552 7553 @Override 7554 public void setProperty(String name, Base value) throws FHIRException { 7555 if (name.equals("url")) 7556 this.url = castToUri(value); // UriType 7557 else if (name.equals("version")) 7558 this.version = castToString(value); // StringType 7559 else if (name.equals("name")) 7560 this.name = castToString(value); // StringType 7561 else if (name.equals("status")) 7562 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 7563 else if (name.equals("identifier")) 7564 this.identifier = castToIdentifier(value); // Identifier 7565 else if (name.equals("experimental")) 7566 this.experimental = castToBoolean(value); // BooleanType 7567 else if (name.equals("publisher")) 7568 this.publisher = castToString(value); // StringType 7569 else if (name.equals("contact")) 7570 this.getContact().add((TestScriptContactComponent) value); 7571 else if (name.equals("date")) 7572 this.date = castToDateTime(value); // DateTimeType 7573 else if (name.equals("description")) 7574 this.description = castToString(value); // StringType 7575 else if (name.equals("useContext")) 7576 this.getUseContext().add(castToCodeableConcept(value)); 7577 else if (name.equals("requirements")) 7578 this.requirements = castToString(value); // StringType 7579 else if (name.equals("copyright")) 7580 this.copyright = castToString(value); // StringType 7581 else if (name.equals("metadata")) 7582 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 7583 else if (name.equals("multiserver")) 7584 this.multiserver = castToBoolean(value); // BooleanType 7585 else if (name.equals("fixture")) 7586 this.getFixture().add((TestScriptFixtureComponent) value); 7587 else if (name.equals("profile")) 7588 this.getProfile().add(castToReference(value)); 7589 else if (name.equals("variable")) 7590 this.getVariable().add((TestScriptVariableComponent) value); 7591 else if (name.equals("setup")) 7592 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 7593 else if (name.equals("test")) 7594 this.getTest().add((TestScriptTestComponent) value); 7595 else if (name.equals("teardown")) 7596 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 7597 else 7598 super.setProperty(name, value); 7599 } 7600 7601 @Override 7602 public Base addChild(String name) throws FHIRException { 7603 if (name.equals("url")) { 7604 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 7605 } else if (name.equals("version")) { 7606 throw new FHIRException("Cannot call addChild on a singleton property TestScript.version"); 7607 } else if (name.equals("name")) { 7608 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 7609 } else if (name.equals("status")) { 7610 throw new FHIRException("Cannot call addChild on a singleton property TestScript.status"); 7611 } else if (name.equals("identifier")) { 7612 this.identifier = new Identifier(); 7613 return this.identifier; 7614 } else if (name.equals("experimental")) { 7615 throw new FHIRException("Cannot call addChild on a singleton property TestScript.experimental"); 7616 } else if (name.equals("publisher")) { 7617 throw new FHIRException("Cannot call addChild on a singleton property TestScript.publisher"); 7618 } else if (name.equals("contact")) { 7619 return addContact(); 7620 } else if (name.equals("date")) { 7621 throw new FHIRException("Cannot call addChild on a singleton property TestScript.date"); 7622 } else if (name.equals("description")) { 7623 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 7624 } else if (name.equals("useContext")) { 7625 return addUseContext(); 7626 } else if (name.equals("requirements")) { 7627 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requirements"); 7628 } else if (name.equals("copyright")) { 7629 throw new FHIRException("Cannot call addChild on a singleton property TestScript.copyright"); 7630 } else if (name.equals("metadata")) { 7631 this.metadata = new TestScriptMetadataComponent(); 7632 return this.metadata; 7633 } else if (name.equals("multiserver")) { 7634 throw new FHIRException("Cannot call addChild on a singleton property TestScript.multiserver"); 7635 } else if (name.equals("fixture")) { 7636 return addFixture(); 7637 } else if (name.equals("profile")) { 7638 return addProfile(); 7639 } else if (name.equals("variable")) { 7640 return addVariable(); 7641 } else if (name.equals("setup")) { 7642 this.setup = new TestScriptSetupComponent(); 7643 return this.setup; 7644 } else if (name.equals("test")) { 7645 return addTest(); 7646 } else if (name.equals("teardown")) { 7647 this.teardown = new TestScriptTeardownComponent(); 7648 return this.teardown; 7649 } else 7650 return super.addChild(name); 7651 } 7652 7653 public String fhirType() { 7654 return "TestScript"; 7655 7656 } 7657 7658 public TestScript copy() { 7659 TestScript dst = new TestScript(); 7660 copyValues(dst); 7661 dst.url = url == null ? null : url.copy(); 7662 dst.version = version == null ? null : version.copy(); 7663 dst.name = name == null ? null : name.copy(); 7664 dst.status = status == null ? null : status.copy(); 7665 dst.identifier = identifier == null ? null : identifier.copy(); 7666 dst.experimental = experimental == null ? null : experimental.copy(); 7667 dst.publisher = publisher == null ? null : publisher.copy(); 7668 if (contact != null) { 7669 dst.contact = new ArrayList<TestScriptContactComponent>(); 7670 for (TestScriptContactComponent i : contact) 7671 dst.contact.add(i.copy()); 7672 } 7673 ; 7674 dst.date = date == null ? null : date.copy(); 7675 dst.description = description == null ? null : description.copy(); 7676 if (useContext != null) { 7677 dst.useContext = new ArrayList<CodeableConcept>(); 7678 for (CodeableConcept i : useContext) 7679 dst.useContext.add(i.copy()); 7680 } 7681 ; 7682 dst.requirements = requirements == null ? null : requirements.copy(); 7683 dst.copyright = copyright == null ? null : copyright.copy(); 7684 dst.metadata = metadata == null ? null : metadata.copy(); 7685 dst.multiserver = multiserver == null ? null : multiserver.copy(); 7686 if (fixture != null) { 7687 dst.fixture = new ArrayList<TestScriptFixtureComponent>(); 7688 for (TestScriptFixtureComponent i : fixture) 7689 dst.fixture.add(i.copy()); 7690 } 7691 ; 7692 if (profile != null) { 7693 dst.profile = new ArrayList<Reference>(); 7694 for (Reference i : profile) 7695 dst.profile.add(i.copy()); 7696 } 7697 ; 7698 if (variable != null) { 7699 dst.variable = new ArrayList<TestScriptVariableComponent>(); 7700 for (TestScriptVariableComponent i : variable) 7701 dst.variable.add(i.copy()); 7702 } 7703 ; 7704 dst.setup = setup == null ? null : setup.copy(); 7705 if (test != null) { 7706 dst.test = new ArrayList<TestScriptTestComponent>(); 7707 for (TestScriptTestComponent i : test) 7708 dst.test.add(i.copy()); 7709 } 7710 ; 7711 dst.teardown = teardown == null ? null : teardown.copy(); 7712 return dst; 7713 } 7714 7715 protected TestScript typedCopy() { 7716 return copy(); 7717 } 7718 7719 @Override 7720 public boolean equalsDeep(Base other) { 7721 if (!super.equalsDeep(other)) 7722 return false; 7723 if (!(other instanceof TestScript)) 7724 return false; 7725 TestScript o = (TestScript) other; 7726 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 7727 && compareDeep(status, o.status, true) && compareDeep(identifier, o.identifier, true) 7728 && compareDeep(experimental, o.experimental, true) && compareDeep(publisher, o.publisher, true) 7729 && compareDeep(contact, o.contact, true) && compareDeep(date, o.date, true) 7730 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 7731 && compareDeep(requirements, o.requirements, true) && compareDeep(copyright, o.copyright, true) 7732 && compareDeep(metadata, o.metadata, true) && compareDeep(multiserver, o.multiserver, true) 7733 && compareDeep(fixture, o.fixture, true) && compareDeep(profile, o.profile, true) 7734 && compareDeep(variable, o.variable, true) && compareDeep(setup, o.setup, true) 7735 && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true); 7736 } 7737 7738 @Override 7739 public boolean equalsShallow(Base other) { 7740 if (!super.equalsShallow(other)) 7741 return false; 7742 if (!(other instanceof TestScript)) 7743 return false; 7744 TestScript o = (TestScript) other; 7745 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 7746 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 7747 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 7748 && compareValues(date, o.date, true) && compareValues(description, o.description, true) 7749 && compareValues(requirements, o.requirements, true) && compareValues(copyright, o.copyright, true) 7750 && compareValues(multiserver, o.multiserver, true); 7751 } 7752 7753 public boolean isEmpty() { 7754 return super.isEmpty() && (url == null || url.isEmpty()) && (version == null || version.isEmpty()) 7755 && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) 7756 && (identifier == null || identifier.isEmpty()) && (experimental == null || experimental.isEmpty()) 7757 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 7758 && (date == null || date.isEmpty()) && (description == null || description.isEmpty()) 7759 && (useContext == null || useContext.isEmpty()) && (requirements == null || requirements.isEmpty()) 7760 && (copyright == null || copyright.isEmpty()) && (metadata == null || metadata.isEmpty()) 7761 && (multiserver == null || multiserver.isEmpty()) && (fixture == null || fixture.isEmpty()) 7762 && (profile == null || profile.isEmpty()) && (variable == null || variable.isEmpty()) 7763 && (setup == null || setup.isEmpty()) && (test == null || test.isEmpty()) 7764 && (teardown == null || teardown.isEmpty()); 7765 } 7766 7767 @Override 7768 public ResourceType getResourceType() { 7769 return ResourceType.TestScript; 7770 } 7771 7772 @SearchParamDefinition(name = "identifier", path = "TestScript.identifier", description = "External identifier", type = "token") 7773 public static final String SP_IDENTIFIER = "identifier"; 7774 @SearchParamDefinition(name = "testscript-test-capability", path = "TestScript.test.metadata.capability.description", description = "TestScript test required and validated capability", type = "string") 7775 public static final String SP_TESTSCRIPTTESTCAPABILITY = "testscript-test-capability"; 7776 @SearchParamDefinition(name = "testscript-setup-capability", path = "TestScript.setup.metadata.capability.description", description = "TestScript setup required and validated capability", type = "string") 7777 public static final String SP_TESTSCRIPTSETUPCAPABILITY = "testscript-setup-capability"; 7778 @SearchParamDefinition(name = "name", path = "TestScript.name", description = "Informal name for this TestScript", type = "string") 7779 public static final String SP_NAME = "name"; 7780 @SearchParamDefinition(name = "description", path = "TestScript.description", description = "Natural language description of the TestScript", type = "string") 7781 public static final String SP_DESCRIPTION = "description"; 7782 @SearchParamDefinition(name = "testscript-capability", path = "TestScript.metadata.capability.description", description = "TestScript required and validated capability", type = "string") 7783 public static final String SP_TESTSCRIPTCAPABILITY = "testscript-capability"; 7784 @SearchParamDefinition(name = "url", path = "TestScript.url", description = "Absolute URL used to reference this TestScript", type = "uri") 7785 public static final String SP_URL = "url"; 7786 7787}