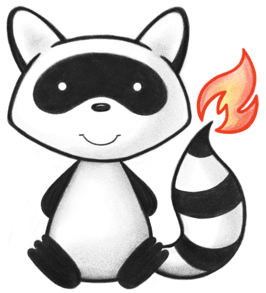
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 044import org.hl7.fhir.instance.model.api.ICompositeType; 045import org.hl7.fhir.exceptions.FHIRException; 046 047/** 048 * Specifies an event that may occur multiple times. Timing schedules are used 049 * to record when things are expected or requested to occur. The most common 050 * usage is in dosage instructions for medications. They are also used when 051 * planning care of various kinds. 052 */ 053@DatatypeDef(name = "Timing") 054public class Timing extends Type implements ICompositeType { 055 056 public enum UnitsOfTime { 057 /** 058 * null 059 */ 060 S, 061 /** 062 * null 063 */ 064 MIN, 065 /** 066 * null 067 */ 068 H, 069 /** 070 * null 071 */ 072 D, 073 /** 074 * null 075 */ 076 WK, 077 /** 078 * null 079 */ 080 MO, 081 /** 082 * null 083 */ 084 A, 085 /** 086 * added to help the parsers 087 */ 088 NULL; 089 090 public static UnitsOfTime fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("s".equals(codeString)) 094 return S; 095 if ("min".equals(codeString)) 096 return MIN; 097 if ("h".equals(codeString)) 098 return H; 099 if ("d".equals(codeString)) 100 return D; 101 if ("wk".equals(codeString)) 102 return WK; 103 if ("mo".equals(codeString)) 104 return MO; 105 if ("a".equals(codeString)) 106 return A; 107 throw new FHIRException("Unknown UnitsOfTime code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case S: 113 return "s"; 114 case MIN: 115 return "min"; 116 case H: 117 return "h"; 118 case D: 119 return "d"; 120 case WK: 121 return "wk"; 122 case MO: 123 return "mo"; 124 case A: 125 return "a"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getSystem() { 134 switch (this) { 135 case S: 136 return "http://unitsofmeasure.org"; 137 case MIN: 138 return "http://unitsofmeasure.org"; 139 case H: 140 return "http://unitsofmeasure.org"; 141 case D: 142 return "http://unitsofmeasure.org"; 143 case WK: 144 return "http://unitsofmeasure.org"; 145 case MO: 146 return "http://unitsofmeasure.org"; 147 case A: 148 return "http://unitsofmeasure.org"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDefinition() { 157 switch (this) { 158 case S: 159 return ""; 160 case MIN: 161 return ""; 162 case H: 163 return ""; 164 case D: 165 return ""; 166 case WK: 167 return ""; 168 case MO: 169 return ""; 170 case A: 171 return ""; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDisplay() { 180 switch (this) { 181 case S: 182 return "s"; 183 case MIN: 184 return "min"; 185 case H: 186 return "h"; 187 case D: 188 return "d"; 189 case WK: 190 return "wk"; 191 case MO: 192 return "mo"; 193 case A: 194 return "a"; 195 case NULL: 196 return null; 197 default: 198 return "?"; 199 } 200 } 201 } 202 203 public static class UnitsOfTimeEnumFactory implements EnumFactory<UnitsOfTime> { 204 public UnitsOfTime fromCode(String codeString) throws IllegalArgumentException { 205 if (codeString == null || "".equals(codeString)) 206 if (codeString == null || "".equals(codeString)) 207 return null; 208 if ("s".equals(codeString)) 209 return UnitsOfTime.S; 210 if ("min".equals(codeString)) 211 return UnitsOfTime.MIN; 212 if ("h".equals(codeString)) 213 return UnitsOfTime.H; 214 if ("d".equals(codeString)) 215 return UnitsOfTime.D; 216 if ("wk".equals(codeString)) 217 return UnitsOfTime.WK; 218 if ("mo".equals(codeString)) 219 return UnitsOfTime.MO; 220 if ("a".equals(codeString)) 221 return UnitsOfTime.A; 222 throw new IllegalArgumentException("Unknown UnitsOfTime code '" + codeString + "'"); 223 } 224 225 public Enumeration<UnitsOfTime> fromType(Base code) throws FHIRException { 226 if (code == null || code.isEmpty()) 227 return null; 228 String codeString = ((PrimitiveType) code).asStringValue(); 229 if (codeString == null || "".equals(codeString)) 230 return null; 231 if ("s".equals(codeString)) 232 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.S); 233 if ("min".equals(codeString)) 234 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MIN); 235 if ("h".equals(codeString)) 236 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.H); 237 if ("d".equals(codeString)) 238 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.D); 239 if ("wk".equals(codeString)) 240 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.WK); 241 if ("mo".equals(codeString)) 242 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MO); 243 if ("a".equals(codeString)) 244 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.A); 245 throw new FHIRException("Unknown UnitsOfTime code '" + codeString + "'"); 246 } 247 248 public String toCode(UnitsOfTime code) { 249 if (code == UnitsOfTime.S) 250 return "s"; 251 if (code == UnitsOfTime.MIN) 252 return "min"; 253 if (code == UnitsOfTime.H) 254 return "h"; 255 if (code == UnitsOfTime.D) 256 return "d"; 257 if (code == UnitsOfTime.WK) 258 return "wk"; 259 if (code == UnitsOfTime.MO) 260 return "mo"; 261 if (code == UnitsOfTime.A) 262 return "a"; 263 return "?"; 264 } 265 } 266 267 public enum EventTiming { 268 /** 269 * null 270 */ 271 HS, 272 /** 273 * null 274 */ 275 WAKE, 276 /** 277 * null 278 */ 279 C, 280 /** 281 * null 282 */ 283 CM, 284 /** 285 * null 286 */ 287 CD, 288 /** 289 * null 290 */ 291 CV, 292 /** 293 * null 294 */ 295 AC, 296 /** 297 * null 298 */ 299 ACM, 300 /** 301 * null 302 */ 303 ACD, 304 /** 305 * null 306 */ 307 ACV, 308 /** 309 * null 310 */ 311 PC, 312 /** 313 * null 314 */ 315 PCM, 316 /** 317 * null 318 */ 319 PCD, 320 /** 321 * null 322 */ 323 PCV, 324 /** 325 * added to help the parsers 326 */ 327 NULL; 328 329 public static EventTiming fromCode(String codeString) throws FHIRException { 330 if (codeString == null || "".equals(codeString)) 331 return null; 332 if ("HS".equals(codeString)) 333 return HS; 334 if ("WAKE".equals(codeString)) 335 return WAKE; 336 if ("C".equals(codeString)) 337 return C; 338 if ("CM".equals(codeString)) 339 return CM; 340 if ("CD".equals(codeString)) 341 return CD; 342 if ("CV".equals(codeString)) 343 return CV; 344 if ("AC".equals(codeString)) 345 return AC; 346 if ("ACM".equals(codeString)) 347 return ACM; 348 if ("ACD".equals(codeString)) 349 return ACD; 350 if ("ACV".equals(codeString)) 351 return ACV; 352 if ("PC".equals(codeString)) 353 return PC; 354 if ("PCM".equals(codeString)) 355 return PCM; 356 if ("PCD".equals(codeString)) 357 return PCD; 358 if ("PCV".equals(codeString)) 359 return PCV; 360 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 361 } 362 363 public String toCode() { 364 switch (this) { 365 case HS: 366 return "HS"; 367 case WAKE: 368 return "WAKE"; 369 case C: 370 return "C"; 371 case CM: 372 return "CM"; 373 case CD: 374 return "CD"; 375 case CV: 376 return "CV"; 377 case AC: 378 return "AC"; 379 case ACM: 380 return "ACM"; 381 case ACD: 382 return "ACD"; 383 case ACV: 384 return "ACV"; 385 case PC: 386 return "PC"; 387 case PCM: 388 return "PCM"; 389 case PCD: 390 return "PCD"; 391 case PCV: 392 return "PCV"; 393 case NULL: 394 return null; 395 default: 396 return "?"; 397 } 398 } 399 400 public String getSystem() { 401 switch (this) { 402 case HS: 403 return "http://hl7.org/fhir/v3/TimingEvent"; 404 case WAKE: 405 return "http://hl7.org/fhir/v3/TimingEvent"; 406 case C: 407 return "http://hl7.org/fhir/v3/TimingEvent"; 408 case CM: 409 return "http://hl7.org/fhir/v3/TimingEvent"; 410 case CD: 411 return "http://hl7.org/fhir/v3/TimingEvent"; 412 case CV: 413 return "http://hl7.org/fhir/v3/TimingEvent"; 414 case AC: 415 return "http://hl7.org/fhir/v3/TimingEvent"; 416 case ACM: 417 return "http://hl7.org/fhir/v3/TimingEvent"; 418 case ACD: 419 return "http://hl7.org/fhir/v3/TimingEvent"; 420 case ACV: 421 return "http://hl7.org/fhir/v3/TimingEvent"; 422 case PC: 423 return "http://hl7.org/fhir/v3/TimingEvent"; 424 case PCM: 425 return "http://hl7.org/fhir/v3/TimingEvent"; 426 case PCD: 427 return "http://hl7.org/fhir/v3/TimingEvent"; 428 case PCV: 429 return "http://hl7.org/fhir/v3/TimingEvent"; 430 case NULL: 431 return null; 432 default: 433 return "?"; 434 } 435 } 436 437 public String getDefinition() { 438 switch (this) { 439 case HS: 440 return ""; 441 case WAKE: 442 return ""; 443 case C: 444 return ""; 445 case CM: 446 return ""; 447 case CD: 448 return ""; 449 case CV: 450 return ""; 451 case AC: 452 return ""; 453 case ACM: 454 return ""; 455 case ACD: 456 return ""; 457 case ACV: 458 return ""; 459 case PC: 460 return ""; 461 case PCM: 462 return ""; 463 case PCD: 464 return ""; 465 case PCV: 466 return ""; 467 case NULL: 468 return null; 469 default: 470 return "?"; 471 } 472 } 473 474 public String getDisplay() { 475 switch (this) { 476 case HS: 477 return "HS"; 478 case WAKE: 479 return "WAKE"; 480 case C: 481 return "C"; 482 case CM: 483 return "CM"; 484 case CD: 485 return "CD"; 486 case CV: 487 return "CV"; 488 case AC: 489 return "AC"; 490 case ACM: 491 return "ACM"; 492 case ACD: 493 return "ACD"; 494 case ACV: 495 return "ACV"; 496 case PC: 497 return "PC"; 498 case PCM: 499 return "PCM"; 500 case PCD: 501 return "PCD"; 502 case PCV: 503 return "PCV"; 504 case NULL: 505 return null; 506 default: 507 return "?"; 508 } 509 } 510 } 511 512 public static class EventTimingEnumFactory implements EnumFactory<EventTiming> { 513 public EventTiming fromCode(String codeString) throws IllegalArgumentException { 514 if (codeString == null || "".equals(codeString)) 515 if (codeString == null || "".equals(codeString)) 516 return null; 517 if ("HS".equals(codeString)) 518 return EventTiming.HS; 519 if ("WAKE".equals(codeString)) 520 return EventTiming.WAKE; 521 if ("C".equals(codeString)) 522 return EventTiming.C; 523 if ("CM".equals(codeString)) 524 return EventTiming.CM; 525 if ("CD".equals(codeString)) 526 return EventTiming.CD; 527 if ("CV".equals(codeString)) 528 return EventTiming.CV; 529 if ("AC".equals(codeString)) 530 return EventTiming.AC; 531 if ("ACM".equals(codeString)) 532 return EventTiming.ACM; 533 if ("ACD".equals(codeString)) 534 return EventTiming.ACD; 535 if ("ACV".equals(codeString)) 536 return EventTiming.ACV; 537 if ("PC".equals(codeString)) 538 return EventTiming.PC; 539 if ("PCM".equals(codeString)) 540 return EventTiming.PCM; 541 if ("PCD".equals(codeString)) 542 return EventTiming.PCD; 543 if ("PCV".equals(codeString)) 544 return EventTiming.PCV; 545 throw new IllegalArgumentException("Unknown EventTiming code '" + codeString + "'"); 546 } 547 548 public Enumeration<EventTiming> fromType(Base code) throws FHIRException { 549 if (code == null || code.isEmpty()) 550 return null; 551 String codeString = ((PrimitiveType) code).asStringValue(); 552 if (codeString == null || "".equals(codeString)) 553 return null; 554 if ("HS".equals(codeString)) 555 return new Enumeration<EventTiming>(this, EventTiming.HS); 556 if ("WAKE".equals(codeString)) 557 return new Enumeration<EventTiming>(this, EventTiming.WAKE); 558 if ("C".equals(codeString)) 559 return new Enumeration<EventTiming>(this, EventTiming.C); 560 if ("CM".equals(codeString)) 561 return new Enumeration<EventTiming>(this, EventTiming.CM); 562 if ("CD".equals(codeString)) 563 return new Enumeration<EventTiming>(this, EventTiming.CD); 564 if ("CV".equals(codeString)) 565 return new Enumeration<EventTiming>(this, EventTiming.CV); 566 if ("AC".equals(codeString)) 567 return new Enumeration<EventTiming>(this, EventTiming.AC); 568 if ("ACM".equals(codeString)) 569 return new Enumeration<EventTiming>(this, EventTiming.ACM); 570 if ("ACD".equals(codeString)) 571 return new Enumeration<EventTiming>(this, EventTiming.ACD); 572 if ("ACV".equals(codeString)) 573 return new Enumeration<EventTiming>(this, EventTiming.ACV); 574 if ("PC".equals(codeString)) 575 return new Enumeration<EventTiming>(this, EventTiming.PC); 576 if ("PCM".equals(codeString)) 577 return new Enumeration<EventTiming>(this, EventTiming.PCM); 578 if ("PCD".equals(codeString)) 579 return new Enumeration<EventTiming>(this, EventTiming.PCD); 580 if ("PCV".equals(codeString)) 581 return new Enumeration<EventTiming>(this, EventTiming.PCV); 582 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 583 } 584 585 public String toCode(EventTiming code) { 586 if (code == EventTiming.HS) 587 return "HS"; 588 if (code == EventTiming.WAKE) 589 return "WAKE"; 590 if (code == EventTiming.C) 591 return "C"; 592 if (code == EventTiming.CM) 593 return "CM"; 594 if (code == EventTiming.CD) 595 return "CD"; 596 if (code == EventTiming.CV) 597 return "CV"; 598 if (code == EventTiming.AC) 599 return "AC"; 600 if (code == EventTiming.ACM) 601 return "ACM"; 602 if (code == EventTiming.ACD) 603 return "ACD"; 604 if (code == EventTiming.ACV) 605 return "ACV"; 606 if (code == EventTiming.PC) 607 return "PC"; 608 if (code == EventTiming.PCM) 609 return "PCM"; 610 if (code == EventTiming.PCD) 611 return "PCD"; 612 if (code == EventTiming.PCV) 613 return "PCV"; 614 return "?"; 615 } 616 } 617 618 @Block() 619 public static class TimingRepeatComponent extends Element implements IBaseDatatypeElement { 620 /** 621 * Either a duration for the length of the timing schedule, a range of possible 622 * length, or outer bounds for start and/or end limits of the timing schedule. 623 */ 624 @Child(name = "bounds", type = { Duration.class, Range.class, 625 Period.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 626 @Description(shortDefinition = "Length/Range of lengths, or (Start and/or end) limits", formalDefinition = "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.") 627 protected Type bounds; 628 629 /** 630 * A total count of the desired number of repetitions. 631 */ 632 @Child(name = "count", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 633 @Description(shortDefinition = "Number of times to repeat", formalDefinition = "A total count of the desired number of repetitions.") 634 protected IntegerType count; 635 636 /** 637 * How long this thing happens for when it happens. 638 */ 639 @Child(name = "duration", type = { 640 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 641 @Description(shortDefinition = "How long when it happens", formalDefinition = "How long this thing happens for when it happens.") 642 protected DecimalType duration; 643 644 /** 645 * The upper limit of how long this thing happens for when it happens. 646 */ 647 @Child(name = "durationMax", type = { 648 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 649 @Description(shortDefinition = "How long when it happens (Max)", formalDefinition = "The upper limit of how long this thing happens for when it happens.") 650 protected DecimalType durationMax; 651 652 /** 653 * The units of time for the duration, in UCUM units. 654 */ 655 @Child(name = "durationUnits", type = { 656 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 657 @Description(shortDefinition = "s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition = "The units of time for the duration, in UCUM units.") 658 protected Enumeration<UnitsOfTime> durationUnits; 659 660 /** 661 * The number of times to repeat the action within the specified period / period 662 * range (i.e. both period and periodMax provided). 663 */ 664 @Child(name = "frequency", type = { 665 IntegerType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 666 @Description(shortDefinition = "Event occurs frequency times per period", formalDefinition = "The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided).") 667 protected IntegerType frequency; 668 669 /** 670 * If present, indicates that the frequency is a range - so repeat between 671 * [frequency] and [frequencyMax] times within the period or period range. 672 */ 673 @Child(name = "frequencyMax", type = { 674 IntegerType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 675 @Description(shortDefinition = "Event occurs up to frequencyMax times per period", formalDefinition = "If present, indicates that the frequency is a range - so repeat between [frequency] and [frequencyMax] times within the period or period range.") 676 protected IntegerType frequencyMax; 677 678 /** 679 * Indicates the duration of time over which repetitions are to occur; e.g. to 680 * express "3 times per day", 3 would be the frequency and "1 day" would be the 681 * period. 682 */ 683 @Child(name = "period", type = { DecimalType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 684 @Description(shortDefinition = "Event occurs frequency times per period", formalDefinition = "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period.") 685 protected DecimalType period; 686 687 /** 688 * If present, indicates that the period is a range from [period] to 689 * [periodMax], allowing expressing concepts such as "do this once every 3-5 690 * days. 691 */ 692 @Child(name = "periodMax", type = { 693 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 694 @Description(shortDefinition = "Upper limit of period (3-4 hours)", formalDefinition = "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.") 695 protected DecimalType periodMax; 696 697 /** 698 * The units of time for the period in UCUM units. 699 */ 700 @Child(name = "periodUnits", type = { 701 CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 702 @Description(shortDefinition = "s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition = "The units of time for the period in UCUM units.") 703 protected Enumeration<UnitsOfTime> periodUnits; 704 705 /** 706 * A real world event that the occurrence of the event should be tied to. 707 */ 708 @Child(name = "when", type = { CodeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 709 @Description(shortDefinition = "Regular life events the event is tied to", formalDefinition = "A real world event that the occurrence of the event should be tied to.") 710 protected Enumeration<EventTiming> when; 711 712 private static final long serialVersionUID = -585686982L; 713 714 /* 715 * Constructor 716 */ 717 public TimingRepeatComponent() { 718 super(); 719 } 720 721 /** 722 * @return {@link #bounds} (Either a duration for the length of the timing 723 * schedule, a range of possible length, or outer bounds for start 724 * and/or end limits of the timing schedule.) 725 */ 726 public Type getBounds() { 727 return this.bounds; 728 } 729 730 /** 731 * @return {@link #bounds} (Either a duration for the length of the timing 732 * schedule, a range of possible length, or outer bounds for start 733 * and/or end limits of the timing schedule.) 734 */ 735 public Duration getBoundsDuration() throws FHIRException { 736 if (!(this.bounds instanceof Duration)) 737 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.bounds.getClass().getName() 738 + " was encountered"); 739 return (Duration) this.bounds; 740 } 741 742 public boolean hasBoundsDuration() { 743 return this.bounds instanceof Duration; 744 } 745 746 /** 747 * @return {@link #bounds} (Either a duration for the length of the timing 748 * schedule, a range of possible length, or outer bounds for start 749 * and/or end limits of the timing schedule.) 750 */ 751 public Range getBoundsRange() throws FHIRException { 752 if (!(this.bounds instanceof Range)) 753 throw new FHIRException( 754 "Type mismatch: the type Range was expected, but " + this.bounds.getClass().getName() + " was encountered"); 755 return (Range) this.bounds; 756 } 757 758 public boolean hasBoundsRange() { 759 return this.bounds instanceof Range; 760 } 761 762 /** 763 * @return {@link #bounds} (Either a duration for the length of the timing 764 * schedule, a range of possible length, or outer bounds for start 765 * and/or end limits of the timing schedule.) 766 */ 767 public Period getBoundsPeriod() throws FHIRException { 768 if (!(this.bounds instanceof Period)) 769 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.bounds.getClass().getName() 770 + " was encountered"); 771 return (Period) this.bounds; 772 } 773 774 public boolean hasBoundsPeriod() { 775 return this.bounds instanceof Period; 776 } 777 778 public boolean hasBounds() { 779 return this.bounds != null && !this.bounds.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #bounds} (Either a duration for the length of the timing 784 * schedule, a range of possible length, or outer bounds for start 785 * and/or end limits of the timing schedule.) 786 */ 787 public TimingRepeatComponent setBounds(Type value) { 788 this.bounds = value; 789 return this; 790 } 791 792 /** 793 * @return {@link #count} (A total count of the desired number of repetitions.). 794 * This is the underlying object with id, value and extensions. The 795 * accessor "getCount" gives direct access to the value 796 */ 797 public IntegerType getCountElement() { 798 if (this.count == null) 799 if (Configuration.errorOnAutoCreate()) 800 throw new Error("Attempt to auto-create TimingRepeatComponent.count"); 801 else if (Configuration.doAutoCreate()) 802 this.count = new IntegerType(); // bb 803 return this.count; 804 } 805 806 public boolean hasCountElement() { 807 return this.count != null && !this.count.isEmpty(); 808 } 809 810 public boolean hasCount() { 811 return this.count != null && !this.count.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #count} (A total count of the desired number of 816 * repetitions.). This is the underlying object with id, value and 817 * extensions. The accessor "getCount" gives direct access to the 818 * value 819 */ 820 public TimingRepeatComponent setCountElement(IntegerType value) { 821 this.count = value; 822 return this; 823 } 824 825 /** 826 * @return A total count of the desired number of repetitions. 827 */ 828 public int getCount() { 829 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 830 } 831 832 /** 833 * @param value A total count of the desired number of repetitions. 834 */ 835 public TimingRepeatComponent setCount(int value) { 836 if (this.count == null) 837 this.count = new IntegerType(); 838 this.count.setValue(value); 839 return this; 840 } 841 842 /** 843 * @return {@link #duration} (How long this thing happens for when it happens.). 844 * This is the underlying object with id, value and extensions. The 845 * accessor "getDuration" gives direct access to the value 846 */ 847 public DecimalType getDurationElement() { 848 if (this.duration == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create TimingRepeatComponent.duration"); 851 else if (Configuration.doAutoCreate()) 852 this.duration = new DecimalType(); // bb 853 return this.duration; 854 } 855 856 public boolean hasDurationElement() { 857 return this.duration != null && !this.duration.isEmpty(); 858 } 859 860 public boolean hasDuration() { 861 return this.duration != null && !this.duration.isEmpty(); 862 } 863 864 /** 865 * @param value {@link #duration} (How long this thing happens for when it 866 * happens.). This is the underlying object with id, value and 867 * extensions. The accessor "getDuration" gives direct access to 868 * the value 869 */ 870 public TimingRepeatComponent setDurationElement(DecimalType value) { 871 this.duration = value; 872 return this; 873 } 874 875 /** 876 * @return How long this thing happens for when it happens. 877 */ 878 public BigDecimal getDuration() { 879 return this.duration == null ? null : this.duration.getValue(); 880 } 881 882 /** 883 * @param value How long this thing happens for when it happens. 884 */ 885 public TimingRepeatComponent setDuration(BigDecimal value) { 886 if (value == null) 887 this.duration = null; 888 else { 889 if (this.duration == null) 890 this.duration = new DecimalType(); 891 this.duration.setValue(value); 892 } 893 return this; 894 } 895 896 /** 897 * @return {@link #durationMax} (The upper limit of how long this thing happens 898 * for when it happens.). This is the underlying object with id, value 899 * and extensions. The accessor "getDurationMax" gives direct access to 900 * the value 901 */ 902 public DecimalType getDurationMaxElement() { 903 if (this.durationMax == null) 904 if (Configuration.errorOnAutoCreate()) 905 throw new Error("Attempt to auto-create TimingRepeatComponent.durationMax"); 906 else if (Configuration.doAutoCreate()) 907 this.durationMax = new DecimalType(); // bb 908 return this.durationMax; 909 } 910 911 public boolean hasDurationMaxElement() { 912 return this.durationMax != null && !this.durationMax.isEmpty(); 913 } 914 915 public boolean hasDurationMax() { 916 return this.durationMax != null && !this.durationMax.isEmpty(); 917 } 918 919 /** 920 * @param value {@link #durationMax} (The upper limit of how long this thing 921 * happens for when it happens.). This is the underlying object 922 * with id, value and extensions. The accessor "getDurationMax" 923 * gives direct access to the value 924 */ 925 public TimingRepeatComponent setDurationMaxElement(DecimalType value) { 926 this.durationMax = value; 927 return this; 928 } 929 930 /** 931 * @return The upper limit of how long this thing happens for when it happens. 932 */ 933 public BigDecimal getDurationMax() { 934 return this.durationMax == null ? null : this.durationMax.getValue(); 935 } 936 937 /** 938 * @param value The upper limit of how long this thing happens for when it 939 * happens. 940 */ 941 public TimingRepeatComponent setDurationMax(BigDecimal value) { 942 if (value == null) 943 this.durationMax = null; 944 else { 945 if (this.durationMax == null) 946 this.durationMax = new DecimalType(); 947 this.durationMax.setValue(value); 948 } 949 return this; 950 } 951 952 /** 953 * @return {@link #durationUnits} (The units of time for the duration, in UCUM 954 * units.). This is the underlying object with id, value and extensions. 955 * The accessor "getDurationUnits" gives direct access to the value 956 */ 957 public Enumeration<UnitsOfTime> getDurationUnitsElement() { 958 if (this.durationUnits == null) 959 if (Configuration.errorOnAutoCreate()) 960 throw new Error("Attempt to auto-create TimingRepeatComponent.durationUnits"); 961 else if (Configuration.doAutoCreate()) 962 this.durationUnits = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 963 return this.durationUnits; 964 } 965 966 public boolean hasDurationUnitsElement() { 967 return this.durationUnits != null && !this.durationUnits.isEmpty(); 968 } 969 970 public boolean hasDurationUnits() { 971 return this.durationUnits != null && !this.durationUnits.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #durationUnits} (The units of time for the duration, in 976 * UCUM units.). This is the underlying object with id, value and 977 * extensions. The accessor "getDurationUnits" gives direct access 978 * to the value 979 */ 980 public TimingRepeatComponent setDurationUnitsElement(Enumeration<UnitsOfTime> value) { 981 this.durationUnits = value; 982 return this; 983 } 984 985 /** 986 * @return The units of time for the duration, in UCUM units. 987 */ 988 public UnitsOfTime getDurationUnits() { 989 return this.durationUnits == null ? null : this.durationUnits.getValue(); 990 } 991 992 /** 993 * @param value The units of time for the duration, in UCUM units. 994 */ 995 public TimingRepeatComponent setDurationUnits(UnitsOfTime value) { 996 if (value == null) 997 this.durationUnits = null; 998 else { 999 if (this.durationUnits == null) 1000 this.durationUnits = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1001 this.durationUnits.setValue(value); 1002 } 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #frequency} (The number of times to repeat the action within 1008 * the specified period / period range (i.e. both period and periodMax 1009 * provided).). This is the underlying object with id, value and 1010 * extensions. The accessor "getFrequency" gives direct access to the 1011 * value 1012 */ 1013 public IntegerType getFrequencyElement() { 1014 if (this.frequency == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create TimingRepeatComponent.frequency"); 1017 else if (Configuration.doAutoCreate()) 1018 this.frequency = new IntegerType(); // bb 1019 return this.frequency; 1020 } 1021 1022 public boolean hasFrequencyElement() { 1023 return this.frequency != null && !this.frequency.isEmpty(); 1024 } 1025 1026 public boolean hasFrequency() { 1027 return this.frequency != null && !this.frequency.isEmpty(); 1028 } 1029 1030 /** 1031 * @param value {@link #frequency} (The number of times to repeat the action 1032 * within the specified period / period range (i.e. both period and 1033 * periodMax provided).). This is the underlying object with id, 1034 * value and extensions. The accessor "getFrequency" gives direct 1035 * access to the value 1036 */ 1037 public TimingRepeatComponent setFrequencyElement(IntegerType value) { 1038 this.frequency = value; 1039 return this; 1040 } 1041 1042 /** 1043 * @return The number of times to repeat the action within the specified period 1044 * / period range (i.e. both period and periodMax provided). 1045 */ 1046 public int getFrequency() { 1047 return this.frequency == null || this.frequency.isEmpty() ? 0 : this.frequency.getValue(); 1048 } 1049 1050 /** 1051 * @param value The number of times to repeat the action within the specified 1052 * period / period range (i.e. both period and periodMax provided). 1053 */ 1054 public TimingRepeatComponent setFrequency(int value) { 1055 if (this.frequency == null) 1056 this.frequency = new IntegerType(); 1057 this.frequency.setValue(value); 1058 return this; 1059 } 1060 1061 /** 1062 * @return {@link #frequencyMax} (If present, indicates that the frequency is a 1063 * range - so repeat between [frequency] and [frequencyMax] times within 1064 * the period or period range.). This is the underlying object with id, 1065 * value and extensions. The accessor "getFrequencyMax" gives direct 1066 * access to the value 1067 */ 1068 public IntegerType getFrequencyMaxElement() { 1069 if (this.frequencyMax == null) 1070 if (Configuration.errorOnAutoCreate()) 1071 throw new Error("Attempt to auto-create TimingRepeatComponent.frequencyMax"); 1072 else if (Configuration.doAutoCreate()) 1073 this.frequencyMax = new IntegerType(); // bb 1074 return this.frequencyMax; 1075 } 1076 1077 public boolean hasFrequencyMaxElement() { 1078 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1079 } 1080 1081 public boolean hasFrequencyMax() { 1082 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1083 } 1084 1085 /** 1086 * @param value {@link #frequencyMax} (If present, indicates that the frequency 1087 * is a range - so repeat between [frequency] and [frequencyMax] 1088 * times within the period or period range.). This is the 1089 * underlying object with id, value and extensions. The accessor 1090 * "getFrequencyMax" gives direct access to the value 1091 */ 1092 public TimingRepeatComponent setFrequencyMaxElement(IntegerType value) { 1093 this.frequencyMax = value; 1094 return this; 1095 } 1096 1097 /** 1098 * @return If present, indicates that the frequency is a range - so repeat 1099 * between [frequency] and [frequencyMax] times within the period or 1100 * period range. 1101 */ 1102 public int getFrequencyMax() { 1103 return this.frequencyMax == null || this.frequencyMax.isEmpty() ? 0 : this.frequencyMax.getValue(); 1104 } 1105 1106 /** 1107 * @param value If present, indicates that the frequency is a range - so repeat 1108 * between [frequency] and [frequencyMax] times within the period 1109 * or period range. 1110 */ 1111 public TimingRepeatComponent setFrequencyMax(int value) { 1112 if (this.frequencyMax == null) 1113 this.frequencyMax = new IntegerType(); 1114 this.frequencyMax.setValue(value); 1115 return this; 1116 } 1117 1118 /** 1119 * @return {@link #period} (Indicates the duration of time over which 1120 * repetitions are to occur; e.g. to express "3 times per day", 3 would 1121 * be the frequency and "1 day" would be the period.). This is the 1122 * underlying object with id, value and extensions. The accessor 1123 * "getPeriod" gives direct access to the value 1124 */ 1125 public DecimalType getPeriodElement() { 1126 if (this.period == null) 1127 if (Configuration.errorOnAutoCreate()) 1128 throw new Error("Attempt to auto-create TimingRepeatComponent.period"); 1129 else if (Configuration.doAutoCreate()) 1130 this.period = new DecimalType(); // bb 1131 return this.period; 1132 } 1133 1134 public boolean hasPeriodElement() { 1135 return this.period != null && !this.period.isEmpty(); 1136 } 1137 1138 public boolean hasPeriod() { 1139 return this.period != null && !this.period.isEmpty(); 1140 } 1141 1142 /** 1143 * @param value {@link #period} (Indicates the duration of time over which 1144 * repetitions are to occur; e.g. to express "3 times per day", 3 1145 * would be the frequency and "1 day" would be the period.). This 1146 * is the underlying object with id, value and extensions. The 1147 * accessor "getPeriod" gives direct access to the value 1148 */ 1149 public TimingRepeatComponent setPeriodElement(DecimalType value) { 1150 this.period = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return Indicates the duration of time over which repetitions are to occur; 1156 * e.g. to express "3 times per day", 3 would be the frequency and "1 1157 * day" would be the period. 1158 */ 1159 public BigDecimal getPeriod() { 1160 return this.period == null ? null : this.period.getValue(); 1161 } 1162 1163 /** 1164 * @param value Indicates the duration of time over which repetitions are to 1165 * occur; e.g. to express "3 times per day", 3 would be the 1166 * frequency and "1 day" would be the period. 1167 */ 1168 public TimingRepeatComponent setPeriod(BigDecimal value) { 1169 if (value == null) 1170 this.period = null; 1171 else { 1172 if (this.period == null) 1173 this.period = new DecimalType(); 1174 this.period.setValue(value); 1175 } 1176 return this; 1177 } 1178 1179 /** 1180 * @return {@link #periodMax} (If present, indicates that the period is a range 1181 * from [period] to [periodMax], allowing expressing concepts such as 1182 * "do this once every 3-5 days.). This is the underlying object with 1183 * id, value and extensions. The accessor "getPeriodMax" gives direct 1184 * access to the value 1185 */ 1186 public DecimalType getPeriodMaxElement() { 1187 if (this.periodMax == null) 1188 if (Configuration.errorOnAutoCreate()) 1189 throw new Error("Attempt to auto-create TimingRepeatComponent.periodMax"); 1190 else if (Configuration.doAutoCreate()) 1191 this.periodMax = new DecimalType(); // bb 1192 return this.periodMax; 1193 } 1194 1195 public boolean hasPeriodMaxElement() { 1196 return this.periodMax != null && !this.periodMax.isEmpty(); 1197 } 1198 1199 public boolean hasPeriodMax() { 1200 return this.periodMax != null && !this.periodMax.isEmpty(); 1201 } 1202 1203 /** 1204 * @param value {@link #periodMax} (If present, indicates that the period is a 1205 * range from [period] to [periodMax], allowing expressing concepts 1206 * such as "do this once every 3-5 days.). This is the underlying 1207 * object with id, value and extensions. The accessor 1208 * "getPeriodMax" gives direct access to the value 1209 */ 1210 public TimingRepeatComponent setPeriodMaxElement(DecimalType value) { 1211 this.periodMax = value; 1212 return this; 1213 } 1214 1215 /** 1216 * @return If present, indicates that the period is a range from [period] to 1217 * [periodMax], allowing expressing concepts such as "do this once every 1218 * 3-5 days. 1219 */ 1220 public BigDecimal getPeriodMax() { 1221 return this.periodMax == null ? null : this.periodMax.getValue(); 1222 } 1223 1224 /** 1225 * @param value If present, indicates that the period is a range from [period] 1226 * to [periodMax], allowing expressing concepts such as "do this 1227 * once every 3-5 days. 1228 */ 1229 public TimingRepeatComponent setPeriodMax(BigDecimal value) { 1230 if (value == null) 1231 this.periodMax = null; 1232 else { 1233 if (this.periodMax == null) 1234 this.periodMax = new DecimalType(); 1235 this.periodMax.setValue(value); 1236 } 1237 return this; 1238 } 1239 1240 /** 1241 * @return {@link #periodUnits} (The units of time for the period in UCUM 1242 * units.). This is the underlying object with id, value and extensions. 1243 * The accessor "getPeriodUnits" gives direct access to the value 1244 */ 1245 public Enumeration<UnitsOfTime> getPeriodUnitsElement() { 1246 if (this.periodUnits == null) 1247 if (Configuration.errorOnAutoCreate()) 1248 throw new Error("Attempt to auto-create TimingRepeatComponent.periodUnits"); 1249 else if (Configuration.doAutoCreate()) 1250 this.periodUnits = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1251 return this.periodUnits; 1252 } 1253 1254 public boolean hasPeriodUnitsElement() { 1255 return this.periodUnits != null && !this.periodUnits.isEmpty(); 1256 } 1257 1258 public boolean hasPeriodUnits() { 1259 return this.periodUnits != null && !this.periodUnits.isEmpty(); 1260 } 1261 1262 /** 1263 * @param value {@link #periodUnits} (The units of time for the period in UCUM 1264 * units.). This is the underlying object with id, value and 1265 * extensions. The accessor "getPeriodUnits" gives direct access to 1266 * the value 1267 */ 1268 public TimingRepeatComponent setPeriodUnitsElement(Enumeration<UnitsOfTime> value) { 1269 this.periodUnits = value; 1270 return this; 1271 } 1272 1273 /** 1274 * @return The units of time for the period in UCUM units. 1275 */ 1276 public UnitsOfTime getPeriodUnits() { 1277 return this.periodUnits == null ? null : this.periodUnits.getValue(); 1278 } 1279 1280 /** 1281 * @param value The units of time for the period in UCUM units. 1282 */ 1283 public TimingRepeatComponent setPeriodUnits(UnitsOfTime value) { 1284 if (value == null) 1285 this.periodUnits = null; 1286 else { 1287 if (this.periodUnits == null) 1288 this.periodUnits = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1289 this.periodUnits.setValue(value); 1290 } 1291 return this; 1292 } 1293 1294 /** 1295 * @return {@link #when} (A real world event that the occurrence of the event 1296 * should be tied to.). This is the underlying object with id, value and 1297 * extensions. The accessor "getWhen" gives direct access to the value 1298 */ 1299 public Enumeration<EventTiming> getWhenElement() { 1300 if (this.when == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create TimingRepeatComponent.when"); 1303 else if (Configuration.doAutoCreate()) 1304 this.when = new Enumeration<EventTiming>(new EventTimingEnumFactory()); // bb 1305 return this.when; 1306 } 1307 1308 public boolean hasWhenElement() { 1309 return this.when != null && !this.when.isEmpty(); 1310 } 1311 1312 public boolean hasWhen() { 1313 return this.when != null && !this.when.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #when} (A real world event that the occurrence of the 1318 * event should be tied to.). This is the underlying object with 1319 * id, value and extensions. The accessor "getWhen" gives direct 1320 * access to the value 1321 */ 1322 public TimingRepeatComponent setWhenElement(Enumeration<EventTiming> value) { 1323 this.when = value; 1324 return this; 1325 } 1326 1327 /** 1328 * @return A real world event that the occurrence of the event should be tied 1329 * to. 1330 */ 1331 public EventTiming getWhen() { 1332 return this.when == null ? null : this.when.getValue(); 1333 } 1334 1335 /** 1336 * @param value A real world event that the occurrence of the event should be 1337 * tied to. 1338 */ 1339 public TimingRepeatComponent setWhen(EventTiming value) { 1340 if (value == null) 1341 this.when = null; 1342 else { 1343 if (this.when == null) 1344 this.when = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 1345 this.when.setValue(value); 1346 } 1347 return this; 1348 } 1349 1350 protected void listChildren(List<Property> childrenList) { 1351 super.listChildren(childrenList); 1352 childrenList.add(new Property("bounds[x]", "Duration|Range|Period", 1353 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 1354 0, java.lang.Integer.MAX_VALUE, bounds)); 1355 childrenList.add(new Property("count", "integer", "A total count of the desired number of repetitions.", 0, 1356 java.lang.Integer.MAX_VALUE, count)); 1357 childrenList.add(new Property("duration", "decimal", "How long this thing happens for when it happens.", 0, 1358 java.lang.Integer.MAX_VALUE, duration)); 1359 childrenList.add( 1360 new Property("durationMax", "decimal", "The upper limit of how long this thing happens for when it happens.", 1361 0, java.lang.Integer.MAX_VALUE, durationMax)); 1362 childrenList.add(new Property("durationUnits", "code", "The units of time for the duration, in UCUM units.", 0, 1363 java.lang.Integer.MAX_VALUE, durationUnits)); 1364 childrenList.add(new Property("frequency", "integer", 1365 "The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided).", 1366 0, java.lang.Integer.MAX_VALUE, frequency)); 1367 childrenList.add(new Property("frequencyMax", "integer", 1368 "If present, indicates that the frequency is a range - so repeat between [frequency] and [frequencyMax] times within the period or period range.", 1369 0, java.lang.Integer.MAX_VALUE, frequencyMax)); 1370 childrenList.add(new Property("period", "decimal", 1371 "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period.", 1372 0, java.lang.Integer.MAX_VALUE, period)); 1373 childrenList.add(new Property("periodMax", "decimal", 1374 "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 1375 0, java.lang.Integer.MAX_VALUE, periodMax)); 1376 childrenList.add(new Property("periodUnits", "code", "The units of time for the period in UCUM units.", 0, 1377 java.lang.Integer.MAX_VALUE, periodUnits)); 1378 childrenList 1379 .add(new Property("when", "code", "A real world event that the occurrence of the event should be tied to.", 0, 1380 java.lang.Integer.MAX_VALUE, when)); 1381 } 1382 1383 @Override 1384 public void setProperty(String name, Base value) throws FHIRException { 1385 if (name.equals("bounds[x]")) 1386 this.bounds = (Type) value; // Type 1387 else if (name.equals("count")) 1388 this.count = castToInteger(value); // IntegerType 1389 else if (name.equals("duration")) 1390 this.duration = castToDecimal(value); // DecimalType 1391 else if (name.equals("durationMax")) 1392 this.durationMax = castToDecimal(value); // DecimalType 1393 else if (name.equals("durationUnits")) 1394 this.durationUnits = new UnitsOfTimeEnumFactory().fromType(value); // Enumeration<UnitsOfTime> 1395 else if (name.equals("frequency")) 1396 this.frequency = castToInteger(value); // IntegerType 1397 else if (name.equals("frequencyMax")) 1398 this.frequencyMax = castToInteger(value); // IntegerType 1399 else if (name.equals("period")) 1400 this.period = castToDecimal(value); // DecimalType 1401 else if (name.equals("periodMax")) 1402 this.periodMax = castToDecimal(value); // DecimalType 1403 else if (name.equals("periodUnits")) 1404 this.periodUnits = new UnitsOfTimeEnumFactory().fromType(value); // Enumeration<UnitsOfTime> 1405 else if (name.equals("when")) 1406 this.when = new EventTimingEnumFactory().fromType(value); // Enumeration<EventTiming> 1407 else 1408 super.setProperty(name, value); 1409 } 1410 1411 @Override 1412 public Base addChild(String name) throws FHIRException { 1413 if (name.equals("boundsDuration")) { 1414 this.bounds = new Duration(); 1415 return this.bounds; 1416 } else if (name.equals("boundsRange")) { 1417 this.bounds = new Range(); 1418 return this.bounds; 1419 } else if (name.equals("boundsPeriod")) { 1420 this.bounds = new Period(); 1421 return this.bounds; 1422 } else if (name.equals("count")) { 1423 throw new FHIRException("Cannot call addChild on a singleton property Timing.count"); 1424 } else if (name.equals("duration")) { 1425 throw new FHIRException("Cannot call addChild on a singleton property Timing.duration"); 1426 } else if (name.equals("durationMax")) { 1427 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationMax"); 1428 } else if (name.equals("durationUnits")) { 1429 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationUnits"); 1430 } else if (name.equals("frequency")) { 1431 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequency"); 1432 } else if (name.equals("frequencyMax")) { 1433 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequencyMax"); 1434 } else if (name.equals("period")) { 1435 throw new FHIRException("Cannot call addChild on a singleton property Timing.period"); 1436 } else if (name.equals("periodMax")) { 1437 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodMax"); 1438 } else if (name.equals("periodUnits")) { 1439 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodUnits"); 1440 } else if (name.equals("when")) { 1441 throw new FHIRException("Cannot call addChild on a singleton property Timing.when"); 1442 } else 1443 return super.addChild(name); 1444 } 1445 1446 public TimingRepeatComponent copy() { 1447 TimingRepeatComponent dst = new TimingRepeatComponent(); 1448 copyValues(dst); 1449 dst.bounds = bounds == null ? null : bounds.copy(); 1450 dst.count = count == null ? null : count.copy(); 1451 dst.duration = duration == null ? null : duration.copy(); 1452 dst.durationMax = durationMax == null ? null : durationMax.copy(); 1453 dst.durationUnits = durationUnits == null ? null : durationUnits.copy(); 1454 dst.frequency = frequency == null ? null : frequency.copy(); 1455 dst.frequencyMax = frequencyMax == null ? null : frequencyMax.copy(); 1456 dst.period = period == null ? null : period.copy(); 1457 dst.periodMax = periodMax == null ? null : periodMax.copy(); 1458 dst.periodUnits = periodUnits == null ? null : periodUnits.copy(); 1459 dst.when = when == null ? null : when.copy(); 1460 return dst; 1461 } 1462 1463 @Override 1464 public boolean equalsDeep(Base other) { 1465 if (!super.equalsDeep(other)) 1466 return false; 1467 if (!(other instanceof TimingRepeatComponent)) 1468 return false; 1469 TimingRepeatComponent o = (TimingRepeatComponent) other; 1470 return compareDeep(bounds, o.bounds, true) && compareDeep(count, o.count, true) 1471 && compareDeep(duration, o.duration, true) && compareDeep(durationMax, o.durationMax, true) 1472 && compareDeep(durationUnits, o.durationUnits, true) && compareDeep(frequency, o.frequency, true) 1473 && compareDeep(frequencyMax, o.frequencyMax, true) && compareDeep(period, o.period, true) 1474 && compareDeep(periodMax, o.periodMax, true) && compareDeep(periodUnits, o.periodUnits, true) 1475 && compareDeep(when, o.when, true); 1476 } 1477 1478 @Override 1479 public boolean equalsShallow(Base other) { 1480 if (!super.equalsShallow(other)) 1481 return false; 1482 if (!(other instanceof TimingRepeatComponent)) 1483 return false; 1484 TimingRepeatComponent o = (TimingRepeatComponent) other; 1485 return compareValues(count, o.count, true) && compareValues(duration, o.duration, true) 1486 && compareValues(durationMax, o.durationMax, true) && compareValues(durationUnits, o.durationUnits, true) 1487 && compareValues(frequency, o.frequency, true) && compareValues(frequencyMax, o.frequencyMax, true) 1488 && compareValues(period, o.period, true) && compareValues(periodMax, o.periodMax, true) 1489 && compareValues(periodUnits, o.periodUnits, true) && compareValues(when, o.when, true); 1490 } 1491 1492 public boolean isEmpty() { 1493 return super.isEmpty() && (bounds == null || bounds.isEmpty()) && (count == null || count.isEmpty()) 1494 && (duration == null || duration.isEmpty()) && (durationMax == null || durationMax.isEmpty()) 1495 && (durationUnits == null || durationUnits.isEmpty()) && (frequency == null || frequency.isEmpty()) 1496 && (frequencyMax == null || frequencyMax.isEmpty()) && (period == null || period.isEmpty()) 1497 && (periodMax == null || periodMax.isEmpty()) && (periodUnits == null || periodUnits.isEmpty()) 1498 && (when == null || when.isEmpty()); 1499 } 1500 1501 public String fhirType() { 1502 return "Timing.repeat"; 1503 1504 } 1505 1506 } 1507 1508 /** 1509 * Identifies specific times when the event occurs. 1510 */ 1511 @Child(name = "event", type = { 1512 DateTimeType.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1513 @Description(shortDefinition = "When the event occurs", formalDefinition = "Identifies specific times when the event occurs.") 1514 protected List<DateTimeType> event; 1515 1516 /** 1517 * A set of rules that describe when the event should occur. 1518 */ 1519 @Child(name = "repeat", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = true) 1520 @Description(shortDefinition = "When the event is to occur", formalDefinition = "A set of rules that describe when the event should occur.") 1521 protected TimingRepeatComponent repeat; 1522 1523 /** 1524 * A code for the timing pattern. Some codes such as BID are ubiquitous, but 1525 * many institutions define their own additional codes. 1526 */ 1527 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1528 @Description(shortDefinition = "QD | QOD | Q4H | Q6H | BID | TID | QID | AM | PM +", formalDefinition = "A code for the timing pattern. Some codes such as BID are ubiquitous, but many institutions define their own additional codes.") 1529 protected CodeableConcept code; 1530 1531 private static final long serialVersionUID = 791565112L; 1532 1533 /* 1534 * Constructor 1535 */ 1536 public Timing() { 1537 super(); 1538 } 1539 1540 /** 1541 * @return {@link #event} (Identifies specific times when the event occurs.) 1542 */ 1543 public List<DateTimeType> getEvent() { 1544 if (this.event == null) 1545 this.event = new ArrayList<DateTimeType>(); 1546 return this.event; 1547 } 1548 1549 public boolean hasEvent() { 1550 if (this.event == null) 1551 return false; 1552 for (DateTimeType item : this.event) 1553 if (!item.isEmpty()) 1554 return true; 1555 return false; 1556 } 1557 1558 /** 1559 * @return {@link #event} (Identifies specific times when the event occurs.) 1560 */ 1561 // syntactic sugar 1562 public DateTimeType addEventElement() {// 2 1563 DateTimeType t = new DateTimeType(); 1564 if (this.event == null) 1565 this.event = new ArrayList<DateTimeType>(); 1566 this.event.add(t); 1567 return t; 1568 } 1569 1570 /** 1571 * @param value {@link #event} (Identifies specific times when the event 1572 * occurs.) 1573 */ 1574 public Timing addEvent(Date value) { // 1 1575 DateTimeType t = new DateTimeType(); 1576 t.setValue(value); 1577 if (this.event == null) 1578 this.event = new ArrayList<DateTimeType>(); 1579 this.event.add(t); 1580 return this; 1581 } 1582 1583 /** 1584 * @param value {@link #event} (Identifies specific times when the event 1585 * occurs.) 1586 */ 1587 public boolean hasEvent(Date value) { 1588 if (this.event == null) 1589 return false; 1590 for (DateTimeType v : this.event) 1591 if (v.equals(value)) // dateTime 1592 return true; 1593 return false; 1594 } 1595 1596 /** 1597 * @return {@link #repeat} (A set of rules that describe when the event should 1598 * occur.) 1599 */ 1600 public TimingRepeatComponent getRepeat() { 1601 if (this.repeat == null) 1602 if (Configuration.errorOnAutoCreate()) 1603 throw new Error("Attempt to auto-create Timing.repeat"); 1604 else if (Configuration.doAutoCreate()) 1605 this.repeat = new TimingRepeatComponent(); // cc 1606 return this.repeat; 1607 } 1608 1609 public boolean hasRepeat() { 1610 return this.repeat != null && !this.repeat.isEmpty(); 1611 } 1612 1613 /** 1614 * @param value {@link #repeat} (A set of rules that describe when the event 1615 * should occur.) 1616 */ 1617 public Timing setRepeat(TimingRepeatComponent value) { 1618 this.repeat = value; 1619 return this; 1620 } 1621 1622 /** 1623 * @return {@link #code} (A code for the timing pattern. Some codes such as BID 1624 * are ubiquitous, but many institutions define their own additional 1625 * codes.) 1626 */ 1627 public CodeableConcept getCode() { 1628 if (this.code == null) 1629 if (Configuration.errorOnAutoCreate()) 1630 throw new Error("Attempt to auto-create Timing.code"); 1631 else if (Configuration.doAutoCreate()) 1632 this.code = new CodeableConcept(); // cc 1633 return this.code; 1634 } 1635 1636 public boolean hasCode() { 1637 return this.code != null && !this.code.isEmpty(); 1638 } 1639 1640 /** 1641 * @param value {@link #code} (A code for the timing pattern. Some codes such as 1642 * BID are ubiquitous, but many institutions define their own 1643 * additional codes.) 1644 */ 1645 public Timing setCode(CodeableConcept value) { 1646 this.code = value; 1647 return this; 1648 } 1649 1650 protected void listChildren(List<Property> childrenList) { 1651 super.listChildren(childrenList); 1652 childrenList.add(new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, 1653 java.lang.Integer.MAX_VALUE, event)); 1654 childrenList.add(new Property("repeat", "", "A set of rules that describe when the event should occur.", 0, 1655 java.lang.Integer.MAX_VALUE, repeat)); 1656 childrenList.add(new Property("code", "CodeableConcept", 1657 "A code for the timing pattern. Some codes such as BID are ubiquitous, but many institutions define their own additional codes.", 1658 0, java.lang.Integer.MAX_VALUE, code)); 1659 } 1660 1661 @Override 1662 public void setProperty(String name, Base value) throws FHIRException { 1663 if (name.equals("event")) 1664 this.getEvent().add(castToDateTime(value)); 1665 else if (name.equals("repeat")) 1666 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 1667 else if (name.equals("code")) 1668 this.code = castToCodeableConcept(value); // CodeableConcept 1669 else 1670 super.setProperty(name, value); 1671 } 1672 1673 @Override 1674 public Base addChild(String name) throws FHIRException { 1675 if (name.equals("event")) { 1676 throw new FHIRException("Cannot call addChild on a singleton property Timing.event"); 1677 } else if (name.equals("repeat")) { 1678 this.repeat = new TimingRepeatComponent(); 1679 return this.repeat; 1680 } else if (name.equals("code")) { 1681 this.code = new CodeableConcept(); 1682 return this.code; 1683 } else 1684 return super.addChild(name); 1685 } 1686 1687 public String fhirType() { 1688 return "Timing"; 1689 1690 } 1691 1692 public Timing copy() { 1693 Timing dst = new Timing(); 1694 copyValues(dst); 1695 if (event != null) { 1696 dst.event = new ArrayList<DateTimeType>(); 1697 for (DateTimeType i : event) 1698 dst.event.add(i.copy()); 1699 } 1700 ; 1701 dst.repeat = repeat == null ? null : repeat.copy(); 1702 dst.code = code == null ? null : code.copy(); 1703 return dst; 1704 } 1705 1706 protected Timing typedCopy() { 1707 return copy(); 1708 } 1709 1710 @Override 1711 public boolean equalsDeep(Base other) { 1712 if (!super.equalsDeep(other)) 1713 return false; 1714 if (!(other instanceof Timing)) 1715 return false; 1716 Timing o = (Timing) other; 1717 return compareDeep(event, o.event, true) && compareDeep(repeat, o.repeat, true) && compareDeep(code, o.code, true); 1718 } 1719 1720 @Override 1721 public boolean equalsShallow(Base other) { 1722 if (!super.equalsShallow(other)) 1723 return false; 1724 if (!(other instanceof Timing)) 1725 return false; 1726 Timing o = (Timing) other; 1727 return compareValues(event, o.event, true); 1728 } 1729 1730 public boolean isEmpty() { 1731 return super.isEmpty() && (event == null || event.isEmpty()) && (repeat == null || repeat.isEmpty()) 1732 && (code == null || code.isEmpty()); 1733 } 1734 1735}