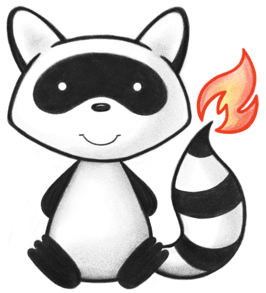
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A value set specifies a set of codes drawn from one or more code systems. 050 */ 051@ResourceDef(name = "ValueSet", profile = "http://hl7.org/fhir/Profile/ValueSet") 052public class ValueSet extends DomainResource { 053 054 public enum FilterOperator { 055 /** 056 * The specified property of the code equals the provided value. 057 */ 058 EQUAL, 059 /** 060 * Includes all concept ids that have a transitive is-a relationship with the 061 * concept Id provided as the value, including the provided concept itself. 062 */ 063 ISA, 064 /** 065 * The specified property of the code does not have an is-a relationship with 066 * the provided value. 067 */ 068 ISNOTA, 069 /** 070 * The specified property of the code matches the regex specified in the 071 * provided value. 072 */ 073 REGEX, 074 /** 075 * The specified property of the code is in the set of codes or concepts 076 * specified in the provided value (comma separated list). 077 */ 078 IN, 079 /** 080 * The specified property of the code is not in the set of codes or concepts 081 * specified in the provided value (comma separated list). 082 */ 083 NOTIN, 084 /** 085 * added to help the parsers 086 */ 087 NULL; 088 089 public static FilterOperator fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("=".equals(codeString)) 093 return EQUAL; 094 if ("is-a".equals(codeString)) 095 return ISA; 096 if ("is-not-a".equals(codeString)) 097 return ISNOTA; 098 if ("regex".equals(codeString)) 099 return REGEX; 100 if ("in".equals(codeString)) 101 return IN; 102 if ("not-in".equals(codeString)) 103 return NOTIN; 104 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case EQUAL: 110 return "="; 111 case ISA: 112 return "is-a"; 113 case ISNOTA: 114 return "is-not-a"; 115 case REGEX: 116 return "regex"; 117 case IN: 118 return "in"; 119 case NOTIN: 120 return "not-in"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getSystem() { 129 switch (this) { 130 case EQUAL: 131 return "http://hl7.org/fhir/filter-operator"; 132 case ISA: 133 return "http://hl7.org/fhir/filter-operator"; 134 case ISNOTA: 135 return "http://hl7.org/fhir/filter-operator"; 136 case REGEX: 137 return "http://hl7.org/fhir/filter-operator"; 138 case IN: 139 return "http://hl7.org/fhir/filter-operator"; 140 case NOTIN: 141 return "http://hl7.org/fhir/filter-operator"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case EQUAL: 152 return "The specified property of the code equals the provided value."; 153 case ISA: 154 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself."; 155 case ISNOTA: 156 return "The specified property of the code does not have an is-a relationship with the provided value."; 157 case REGEX: 158 return "The specified property of the code matches the regex specified in the provided value."; 159 case IN: 160 return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 161 case NOTIN: 162 return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getDisplay() { 171 switch (this) { 172 case EQUAL: 173 return "Equals"; 174 case ISA: 175 return "Is A (by subsumption)"; 176 case ISNOTA: 177 return "Not (Is A) (by subsumption)"; 178 case REGEX: 179 return "Regular Expression"; 180 case IN: 181 return "In Set"; 182 case NOTIN: 183 return "Not in Set"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 } 191 192 public static class FilterOperatorEnumFactory implements EnumFactory<FilterOperator> { 193 public FilterOperator fromCode(String codeString) throws IllegalArgumentException { 194 if (codeString == null || "".equals(codeString)) 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("=".equals(codeString)) 198 return FilterOperator.EQUAL; 199 if ("is-a".equals(codeString)) 200 return FilterOperator.ISA; 201 if ("is-not-a".equals(codeString)) 202 return FilterOperator.ISNOTA; 203 if ("regex".equals(codeString)) 204 return FilterOperator.REGEX; 205 if ("in".equals(codeString)) 206 return FilterOperator.IN; 207 if ("not-in".equals(codeString)) 208 return FilterOperator.NOTIN; 209 throw new IllegalArgumentException("Unknown FilterOperator code '" + codeString + "'"); 210 } 211 212 public Enumeration<FilterOperator> fromType(Base code) throws FHIRException { 213 if (code == null || code.isEmpty()) 214 return null; 215 String codeString = ((PrimitiveType) code).asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return null; 218 if ("=".equals(codeString)) 219 return new Enumeration<FilterOperator>(this, FilterOperator.EQUAL); 220 if ("is-a".equals(codeString)) 221 return new Enumeration<FilterOperator>(this, FilterOperator.ISA); 222 if ("is-not-a".equals(codeString)) 223 return new Enumeration<FilterOperator>(this, FilterOperator.ISNOTA); 224 if ("regex".equals(codeString)) 225 return new Enumeration<FilterOperator>(this, FilterOperator.REGEX); 226 if ("in".equals(codeString)) 227 return new Enumeration<FilterOperator>(this, FilterOperator.IN); 228 if ("not-in".equals(codeString)) 229 return new Enumeration<FilterOperator>(this, FilterOperator.NOTIN); 230 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 231 } 232 233 public String toCode(FilterOperator code) 234 { 235 if (code == FilterOperator.NULL) 236 return null; 237 if (code == FilterOperator.EQUAL) 238 return "="; 239 if (code == FilterOperator.ISA) 240 return "is-a"; 241 if (code == FilterOperator.ISNOTA) 242 return "is-not-a"; 243 if (code == FilterOperator.REGEX) 244 return "regex"; 245 if (code == FilterOperator.IN) 246 return "in"; 247 if (code == FilterOperator.NOTIN) 248 return "not-in"; 249 return "?"; 250 } 251 } 252 253 @Block() 254 public static class ValueSetContactComponent extends BackboneElement implements IBaseBackboneElement { 255 /** 256 * The name of an individual to contact regarding the value set. 257 */ 258 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 259 @Description(shortDefinition = "Name of an individual to contact", formalDefinition = "The name of an individual to contact regarding the value set.") 260 protected StringType name; 261 262 /** 263 * Contact details for individual (if a name was provided) or the publisher. 264 */ 265 @Child(name = "telecom", type = { 266 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 267 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 268 protected List<ContactPoint> telecom; 269 270 private static final long serialVersionUID = -1179697803L; 271 272 /* 273 * Constructor 274 */ 275 public ValueSetContactComponent() { 276 super(); 277 } 278 279 /** 280 * @return {@link #name} (The name of an individual to contact regarding the 281 * value set.). This is the underlying object with id, value and 282 * extensions. The accessor "getName" gives direct access to the value 283 */ 284 public StringType getNameElement() { 285 if (this.name == null) 286 if (Configuration.errorOnAutoCreate()) 287 throw new Error("Attempt to auto-create ValueSetContactComponent.name"); 288 else if (Configuration.doAutoCreate()) 289 this.name = new StringType(); // bb 290 return this.name; 291 } 292 293 public boolean hasNameElement() { 294 return this.name != null && !this.name.isEmpty(); 295 } 296 297 public boolean hasName() { 298 return this.name != null && !this.name.isEmpty(); 299 } 300 301 /** 302 * @param value {@link #name} (The name of an individual to contact regarding 303 * the value set.). This is the underlying object with id, value 304 * and extensions. The accessor "getName" gives direct access to 305 * the value 306 */ 307 public ValueSetContactComponent setNameElement(StringType value) { 308 this.name = value; 309 return this; 310 } 311 312 /** 313 * @return The name of an individual to contact regarding the value set. 314 */ 315 public String getName() { 316 return this.name == null ? null : this.name.getValue(); 317 } 318 319 /** 320 * @param value The name of an individual to contact regarding the value set. 321 */ 322 public ValueSetContactComponent setName(String value) { 323 if (Utilities.noString(value)) 324 this.name = null; 325 else { 326 if (this.name == null) 327 this.name = new StringType(); 328 this.name.setValue(value); 329 } 330 return this; 331 } 332 333 /** 334 * @return {@link #telecom} (Contact details for individual (if a name was 335 * provided) or the publisher.) 336 */ 337 public List<ContactPoint> getTelecom() { 338 if (this.telecom == null) 339 this.telecom = new ArrayList<ContactPoint>(); 340 return this.telecom; 341 } 342 343 public boolean hasTelecom() { 344 if (this.telecom == null) 345 return false; 346 for (ContactPoint item : this.telecom) 347 if (!item.isEmpty()) 348 return true; 349 return false; 350 } 351 352 /** 353 * @return {@link #telecom} (Contact details for individual (if a name was 354 * provided) or the publisher.) 355 */ 356 // syntactic sugar 357 public ContactPoint addTelecom() { // 3 358 ContactPoint t = new ContactPoint(); 359 if (this.telecom == null) 360 this.telecom = new ArrayList<ContactPoint>(); 361 this.telecom.add(t); 362 return t; 363 } 364 365 // syntactic sugar 366 public ValueSetContactComponent addTelecom(ContactPoint t) { // 3 367 if (t == null) 368 return this; 369 if (this.telecom == null) 370 this.telecom = new ArrayList<ContactPoint>(); 371 this.telecom.add(t); 372 return this; 373 } 374 375 protected void listChildren(List<Property> childrenList) { 376 super.listChildren(childrenList); 377 childrenList.add(new Property("name", "string", "The name of an individual to contact regarding the value set.", 378 0, java.lang.Integer.MAX_VALUE, name)); 379 childrenList.add(new Property("telecom", "ContactPoint", 380 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 381 telecom)); 382 } 383 384 @Override 385 public void setProperty(String name, Base value) throws FHIRException { 386 if (name.equals("name")) 387 this.name = castToString(value); // StringType 388 else if (name.equals("telecom")) 389 this.getTelecom().add(castToContactPoint(value)); 390 else 391 super.setProperty(name, value); 392 } 393 394 @Override 395 public Base addChild(String name) throws FHIRException { 396 if (name.equals("name")) { 397 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 398 } else if (name.equals("telecom")) { 399 return addTelecom(); 400 } else 401 return super.addChild(name); 402 } 403 404 public ValueSetContactComponent copy() { 405 ValueSetContactComponent dst = new ValueSetContactComponent(); 406 copyValues(dst); 407 dst.name = name == null ? null : name.copy(); 408 if (telecom != null) { 409 dst.telecom = new ArrayList<ContactPoint>(); 410 for (ContactPoint i : telecom) 411 dst.telecom.add(i.copy()); 412 } 413 ; 414 return dst; 415 } 416 417 @Override 418 public boolean equalsDeep(Base other) { 419 if (!super.equalsDeep(other)) 420 return false; 421 if (!(other instanceof ValueSetContactComponent)) 422 return false; 423 ValueSetContactComponent o = (ValueSetContactComponent) other; 424 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 425 } 426 427 @Override 428 public boolean equalsShallow(Base other) { 429 if (!super.equalsShallow(other)) 430 return false; 431 if (!(other instanceof ValueSetContactComponent)) 432 return false; 433 ValueSetContactComponent o = (ValueSetContactComponent) other; 434 return compareValues(name, o.name, true); 435 } 436 437 public boolean isEmpty() { 438 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 439 } 440 441 public String fhirType() { 442 return "ValueSet.contact"; 443 444 } 445 446 } 447 448 @Block() 449 public static class ValueSetCodeSystemComponent extends BackboneElement implements IBaseBackboneElement { 450 /** 451 * An absolute URI that is used to reference this code system, including in 452 * [Coding]{datatypes.html#Coding}.system. 453 */ 454 @Child(name = "system", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 455 @Description(shortDefinition = "URI to identify the code system (e.g. in Coding.system)", formalDefinition = "An absolute URI that is used to reference this code system, including in [Coding]{datatypes.html#Coding}.system.") 456 protected UriType system; 457 458 /** 459 * The version of this code system that defines the codes. Note that the version 460 * is optional because a well maintained code system does not suffer from 461 * versioning, and therefore the version does not need to be maintained. However 462 * many code systems are not well maintained, and the version needs to be 463 * defined and tracked. 464 */ 465 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 466 @Description(shortDefinition = "Version (for use in Coding.version)", formalDefinition = "The version of this code system that defines the codes. Note that the version is optional because a well maintained code system does not suffer from versioning, and therefore the version does not need to be maintained. However many code systems are not well maintained, and the version needs to be defined and tracked.") 467 protected StringType version; 468 469 /** 470 * If code comparison is case sensitive when codes within this system are 471 * compared to each other. 472 */ 473 @Child(name = "caseSensitive", type = { 474 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 475 @Description(shortDefinition = "If code comparison is case sensitive", formalDefinition = "If code comparison is case sensitive when codes within this system are compared to each other.") 476 protected BooleanType caseSensitive; 477 478 /** 479 * Concepts that are in the code system. The concept definitions are inherently 480 * hierarchical, but the definitions must be consulted to determine what the 481 * meaning of the hierarchical relationships are. 482 */ 483 @Child(name = "concept", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 484 @Description(shortDefinition = "Concepts in the code system", formalDefinition = "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are.") 485 protected List<ConceptDefinitionComponent> concept; 486 487 private static final long serialVersionUID = -1109401192L; 488 489 /* 490 * Constructor 491 */ 492 public ValueSetCodeSystemComponent() { 493 super(); 494 } 495 496 /* 497 * Constructor 498 */ 499 public ValueSetCodeSystemComponent(UriType system) { 500 super(); 501 this.system = system; 502 } 503 504 /** 505 * @return {@link #system} (An absolute URI that is used to reference this code 506 * system, including in [Coding]{datatypes.html#Coding}.system.). This 507 * is the underlying object with id, value and extensions. The accessor 508 * "getSystem" gives direct access to the value 509 */ 510 public UriType getSystemElement() { 511 if (this.system == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create ValueSetCodeSystemComponent.system"); 514 else if (Configuration.doAutoCreate()) 515 this.system = new UriType(); // bb 516 return this.system; 517 } 518 519 public boolean hasSystemElement() { 520 return this.system != null && !this.system.isEmpty(); 521 } 522 523 public boolean hasSystem() { 524 return this.system != null && !this.system.isEmpty(); 525 } 526 527 /** 528 * @param value {@link #system} (An absolute URI that is used to reference this 529 * code system, including in 530 * [Coding]{datatypes.html#Coding}.system.). This is the underlying 531 * object with id, value and extensions. The accessor "getSystem" 532 * gives direct access to the value 533 */ 534 public ValueSetCodeSystemComponent setSystemElement(UriType value) { 535 this.system = value; 536 return this; 537 } 538 539 /** 540 * @return An absolute URI that is used to reference this code system, including 541 * in [Coding]{datatypes.html#Coding}.system. 542 */ 543 public String getSystem() { 544 return this.system == null ? null : this.system.getValue(); 545 } 546 547 /** 548 * @param value An absolute URI that is used to reference this code system, 549 * including in [Coding]{datatypes.html#Coding}.system. 550 */ 551 public ValueSetCodeSystemComponent setSystem(String value) { 552 if (this.system == null) 553 this.system = new UriType(); 554 this.system.setValue(value); 555 return this; 556 } 557 558 /** 559 * @return {@link #version} (The version of this code system that defines the 560 * codes. Note that the version is optional because a well maintained 561 * code system does not suffer from versioning, and therefore the 562 * version does not need to be maintained. However many code systems are 563 * not well maintained, and the version needs to be defined and 564 * tracked.). This is the underlying object with id, value and 565 * extensions. The accessor "getVersion" gives direct access to the 566 * value 567 */ 568 public StringType getVersionElement() { 569 if (this.version == null) 570 if (Configuration.errorOnAutoCreate()) 571 throw new Error("Attempt to auto-create ValueSetCodeSystemComponent.version"); 572 else if (Configuration.doAutoCreate()) 573 this.version = new StringType(); // bb 574 return this.version; 575 } 576 577 public boolean hasVersionElement() { 578 return this.version != null && !this.version.isEmpty(); 579 } 580 581 public boolean hasVersion() { 582 return this.version != null && !this.version.isEmpty(); 583 } 584 585 /** 586 * @param value {@link #version} (The version of this code system that defines 587 * the codes. Note that the version is optional because a well 588 * maintained code system does not suffer from versioning, and 589 * therefore the version does not need to be maintained. However 590 * many code systems are not well maintained, and the version needs 591 * to be defined and tracked.). This is the underlying object with 592 * id, value and extensions. The accessor "getVersion" gives direct 593 * access to the value 594 */ 595 public ValueSetCodeSystemComponent setVersionElement(StringType value) { 596 this.version = value; 597 return this; 598 } 599 600 /** 601 * @return The version of this code system that defines the codes. Note that the 602 * version is optional because a well maintained code system does not 603 * suffer from versioning, and therefore the version does not need to be 604 * maintained. However many code systems are not well maintained, and 605 * the version needs to be defined and tracked. 606 */ 607 public String getVersion() { 608 return this.version == null ? null : this.version.getValue(); 609 } 610 611 /** 612 * @param value The version of this code system that defines the codes. Note 613 * that the version is optional because a well maintained code 614 * system does not suffer from versioning, and therefore the 615 * version does not need to be maintained. However many code 616 * systems are not well maintained, and the version needs to be 617 * defined and tracked. 618 */ 619 public ValueSetCodeSystemComponent setVersion(String value) { 620 if (Utilities.noString(value)) 621 this.version = null; 622 else { 623 if (this.version == null) 624 this.version = new StringType(); 625 this.version.setValue(value); 626 } 627 return this; 628 } 629 630 /** 631 * @return {@link #caseSensitive} (If code comparison is case sensitive when 632 * codes within this system are compared to each other.). This is the 633 * underlying object with id, value and extensions. The accessor 634 * "getCaseSensitive" gives direct access to the value 635 */ 636 public BooleanType getCaseSensitiveElement() { 637 if (this.caseSensitive == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create ValueSetCodeSystemComponent.caseSensitive"); 640 else if (Configuration.doAutoCreate()) 641 this.caseSensitive = new BooleanType(); // bb 642 return this.caseSensitive; 643 } 644 645 public boolean hasCaseSensitiveElement() { 646 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 647 } 648 649 public boolean hasCaseSensitive() { 650 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #caseSensitive} (If code comparison is case sensitive 655 * when codes within this system are compared to each other.). This 656 * is the underlying object with id, value and extensions. The 657 * accessor "getCaseSensitive" gives direct access to the value 658 */ 659 public ValueSetCodeSystemComponent setCaseSensitiveElement(BooleanType value) { 660 this.caseSensitive = value; 661 return this; 662 } 663 664 /** 665 * @return If code comparison is case sensitive when codes within this system 666 * are compared to each other. 667 */ 668 public boolean getCaseSensitive() { 669 return this.caseSensitive == null || this.caseSensitive.isEmpty() ? false : this.caseSensitive.getValue(); 670 } 671 672 /** 673 * @param value If code comparison is case sensitive when codes within this 674 * system are compared to each other. 675 */ 676 public ValueSetCodeSystemComponent setCaseSensitive(boolean value) { 677 if (this.caseSensitive == null) 678 this.caseSensitive = new BooleanType(); 679 this.caseSensitive.setValue(value); 680 return this; 681 } 682 683 /** 684 * @return {@link #concept} (Concepts that are in the code system. The concept 685 * definitions are inherently hierarchical, but the definitions must be 686 * consulted to determine what the meaning of the hierarchical 687 * relationships are.) 688 */ 689 public List<ConceptDefinitionComponent> getConcept() { 690 if (this.concept == null) 691 this.concept = new ArrayList<ConceptDefinitionComponent>(); 692 return this.concept; 693 } 694 695 public boolean hasConcept() { 696 if (this.concept == null) 697 return false; 698 for (ConceptDefinitionComponent item : this.concept) 699 if (!item.isEmpty()) 700 return true; 701 return false; 702 } 703 704 /** 705 * @return {@link #concept} (Concepts that are in the code system. The concept 706 * definitions are inherently hierarchical, but the definitions must be 707 * consulted to determine what the meaning of the hierarchical 708 * relationships are.) 709 */ 710 // syntactic sugar 711 public ConceptDefinitionComponent addConcept() { // 3 712 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 713 if (this.concept == null) 714 this.concept = new ArrayList<ConceptDefinitionComponent>(); 715 this.concept.add(t); 716 return t; 717 } 718 719 // syntactic sugar 720 public ValueSetCodeSystemComponent addConcept(ConceptDefinitionComponent t) { // 3 721 if (t == null) 722 return this; 723 if (this.concept == null) 724 this.concept = new ArrayList<ConceptDefinitionComponent>(); 725 this.concept.add(t); 726 return this; 727 } 728 729 protected void listChildren(List<Property> childrenList) { 730 super.listChildren(childrenList); 731 childrenList.add(new Property("system", "uri", 732 "An absolute URI that is used to reference this code system, including in [Coding]{datatypes.html#Coding}.system.", 733 0, java.lang.Integer.MAX_VALUE, system)); 734 childrenList.add(new Property("version", "string", 735 "The version of this code system that defines the codes. Note that the version is optional because a well maintained code system does not suffer from versioning, and therefore the version does not need to be maintained. However many code systems are not well maintained, and the version needs to be defined and tracked.", 736 0, java.lang.Integer.MAX_VALUE, version)); 737 childrenList.add(new Property("caseSensitive", "boolean", 738 "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 739 java.lang.Integer.MAX_VALUE, caseSensitive)); 740 childrenList.add(new Property("concept", "", 741 "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meaning of the hierarchical relationships are.", 742 0, java.lang.Integer.MAX_VALUE, concept)); 743 } 744 745 @Override 746 public void setProperty(String name, Base value) throws FHIRException { 747 if (name.equals("system")) 748 this.system = castToUri(value); // UriType 749 else if (name.equals("version")) 750 this.version = castToString(value); // StringType 751 else if (name.equals("caseSensitive")) 752 this.caseSensitive = castToBoolean(value); // BooleanType 753 else if (name.equals("concept")) 754 this.getConcept().add((ConceptDefinitionComponent) value); 755 else 756 super.setProperty(name, value); 757 } 758 759 @Override 760 public Base addChild(String name) throws FHIRException { 761 if (name.equals("system")) { 762 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 763 } else if (name.equals("version")) { 764 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 765 } else if (name.equals("caseSensitive")) { 766 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.caseSensitive"); 767 } else if (name.equals("concept")) { 768 return addConcept(); 769 } else 770 return super.addChild(name); 771 } 772 773 public ValueSetCodeSystemComponent copy() { 774 ValueSetCodeSystemComponent dst = new ValueSetCodeSystemComponent(); 775 copyValues(dst); 776 dst.system = system == null ? null : system.copy(); 777 dst.version = version == null ? null : version.copy(); 778 dst.caseSensitive = caseSensitive == null ? null : caseSensitive.copy(); 779 if (concept != null) { 780 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 781 for (ConceptDefinitionComponent i : concept) 782 dst.concept.add(i.copy()); 783 } 784 ; 785 return dst; 786 } 787 788 @Override 789 public boolean equalsDeep(Base other) { 790 if (!super.equalsDeep(other)) 791 return false; 792 if (!(other instanceof ValueSetCodeSystemComponent)) 793 return false; 794 ValueSetCodeSystemComponent o = (ValueSetCodeSystemComponent) other; 795 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) 796 && compareDeep(caseSensitive, o.caseSensitive, true) && compareDeep(concept, o.concept, true); 797 } 798 799 @Override 800 public boolean equalsShallow(Base other) { 801 if (!super.equalsShallow(other)) 802 return false; 803 if (!(other instanceof ValueSetCodeSystemComponent)) 804 return false; 805 ValueSetCodeSystemComponent o = (ValueSetCodeSystemComponent) other; 806 return compareValues(system, o.system, true) && compareValues(version, o.version, true) 807 && compareValues(caseSensitive, o.caseSensitive, true); 808 } 809 810 public boolean isEmpty() { 811 return super.isEmpty() && (system == null || system.isEmpty()) && (version == null || version.isEmpty()) 812 && (caseSensitive == null || caseSensitive.isEmpty()) && (concept == null || concept.isEmpty()); 813 } 814 815 public String fhirType() { 816 return "ValueSet.codeSystem"; 817 818 } 819 820 } 821 822 @Block() 823 public static class ConceptDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 824 /** 825 * A code - a text symbol - that uniquely identifies the concept within the code 826 * system. 827 */ 828 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 829 @Description(shortDefinition = "Code that identifies concept", formalDefinition = "A code - a text symbol - that uniquely identifies the concept within the code system.") 830 protected CodeType code; 831 832 /** 833 * If this code is not for use as a real concept. 834 */ 835 @Child(name = "abstract", type = { 836 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 837 @Description(shortDefinition = "If this code is not for use as a real concept", formalDefinition = "If this code is not for use as a real concept.") 838 protected BooleanType abstract_; 839 840 /** 841 * A human readable string that is the recommended default way to present this 842 * concept to a user. 843 */ 844 @Child(name = "display", type = { 845 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 846 @Description(shortDefinition = "Text to display to the user", formalDefinition = "A human readable string that is the recommended default way to present this concept to a user.") 847 protected StringType display; 848 849 /** 850 * The formal definition of the concept. The value set resource does not make 851 * formal definitions required, because of the prevalence of legacy systems. 852 * However, they are highly recommended, as without them there is no formal 853 * meaning associated with the concept. 854 */ 855 @Child(name = "definition", type = { 856 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 857 @Description(shortDefinition = "Formal definition", formalDefinition = "The formal definition of the concept. The value set resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.") 858 protected StringType definition; 859 860 /** 861 * Additional representations for the concept - other languages, aliases, 862 * specialized purposes, used for particular purposes, etc. 863 */ 864 @Child(name = "designation", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 865 @Description(shortDefinition = "Additional representations for the concept", formalDefinition = "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.") 866 protected List<ConceptDefinitionDesignationComponent> designation; 867 868 /** 869 * Defines children of a concept to produce a hierarchy of concepts. The nature 870 * of the relationships is variable (is-a/contains/categorizes) and can only be 871 * determined by examining the definitions of the concepts. 872 */ 873 @Child(name = "concept", type = { 874 ConceptDefinitionComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 875 @Description(shortDefinition = "Child Concepts (is-a/contains/categorizes)", formalDefinition = "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) and can only be determined by examining the definitions of the concepts.") 876 protected List<ConceptDefinitionComponent> concept; 877 878 private static final long serialVersionUID = -318560292L; 879 880 /* 881 * Constructor 882 */ 883 public ConceptDefinitionComponent() { 884 super(); 885 } 886 887 /* 888 * Constructor 889 */ 890 public ConceptDefinitionComponent(CodeType code) { 891 super(); 892 this.code = code; 893 } 894 895 /** 896 * @return {@link #code} (A code - a text symbol - that uniquely identifies the 897 * concept within the code system.). This is the underlying object with 898 * id, value and extensions. The accessor "getCode" gives direct access 899 * to the value 900 */ 901 public CodeType getCodeElement() { 902 if (this.code == null) 903 if (Configuration.errorOnAutoCreate()) 904 throw new Error("Attempt to auto-create ConceptDefinitionComponent.code"); 905 else if (Configuration.doAutoCreate()) 906 this.code = new CodeType(); // bb 907 return this.code; 908 } 909 910 public boolean hasCodeElement() { 911 return this.code != null && !this.code.isEmpty(); 912 } 913 914 public boolean hasCode() { 915 return this.code != null && !this.code.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #code} (A code - a text symbol - that uniquely identifies 920 * the concept within the code system.). This is the underlying 921 * object with id, value and extensions. The accessor "getCode" 922 * gives direct access to the value 923 */ 924 public ConceptDefinitionComponent setCodeElement(CodeType value) { 925 this.code = value; 926 return this; 927 } 928 929 /** 930 * @return A code - a text symbol - that uniquely identifies the concept within 931 * the code system. 932 */ 933 public String getCode() { 934 return this.code == null ? null : this.code.getValue(); 935 } 936 937 /** 938 * @param value A code - a text symbol - that uniquely identifies the concept 939 * within the code system. 940 */ 941 public ConceptDefinitionComponent setCode(String value) { 942 if (this.code == null) 943 this.code = new CodeType(); 944 this.code.setValue(value); 945 return this; 946 } 947 948 /** 949 * @return {@link #abstract_} (If this code is not for use as a real concept.). 950 * This is the underlying object with id, value and extensions. The 951 * accessor "getAbstract" gives direct access to the value 952 */ 953 public BooleanType getAbstractElement() { 954 if (this.abstract_ == null) 955 if (Configuration.errorOnAutoCreate()) 956 throw new Error("Attempt to auto-create ConceptDefinitionComponent.abstract_"); 957 else if (Configuration.doAutoCreate()) 958 this.abstract_ = new BooleanType(); // bb 959 return this.abstract_; 960 } 961 962 public boolean hasAbstractElement() { 963 return this.abstract_ != null && !this.abstract_.isEmpty(); 964 } 965 966 public boolean hasAbstract() { 967 return this.abstract_ != null && !this.abstract_.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #abstract_} (If this code is not for use as a real 972 * concept.). This is the underlying object with id, value and 973 * extensions. The accessor "getAbstract" gives direct access to 974 * the value 975 */ 976 public ConceptDefinitionComponent setAbstractElement(BooleanType value) { 977 this.abstract_ = value; 978 return this; 979 } 980 981 /** 982 * @return If this code is not for use as a real concept. 983 */ 984 public boolean getAbstract() { 985 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 986 } 987 988 /** 989 * @param value If this code is not for use as a real concept. 990 */ 991 public ConceptDefinitionComponent setAbstract(boolean value) { 992 if (this.abstract_ == null) 993 this.abstract_ = new BooleanType(); 994 this.abstract_.setValue(value); 995 return this; 996 } 997 998 /** 999 * @return {@link #display} (A human readable string that is the recommended 1000 * default way to present this concept to a user.). This is the 1001 * underlying object with id, value and extensions. The accessor 1002 * "getDisplay" gives direct access to the value 1003 */ 1004 public StringType getDisplayElement() { 1005 if (this.display == null) 1006 if (Configuration.errorOnAutoCreate()) 1007 throw new Error("Attempt to auto-create ConceptDefinitionComponent.display"); 1008 else if (Configuration.doAutoCreate()) 1009 this.display = new StringType(); // bb 1010 return this.display; 1011 } 1012 1013 public boolean hasDisplayElement() { 1014 return this.display != null && !this.display.isEmpty(); 1015 } 1016 1017 public boolean hasDisplay() { 1018 return this.display != null && !this.display.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #display} (A human readable string that is the 1023 * recommended default way to present this concept to a user.). 1024 * This is the underlying object with id, value and extensions. The 1025 * accessor "getDisplay" gives direct access to the value 1026 */ 1027 public ConceptDefinitionComponent setDisplayElement(StringType value) { 1028 this.display = value; 1029 return this; 1030 } 1031 1032 /** 1033 * @return A human readable string that is the recommended default way to 1034 * present this concept to a user. 1035 */ 1036 public String getDisplay() { 1037 return this.display == null ? null : this.display.getValue(); 1038 } 1039 1040 /** 1041 * @param value A human readable string that is the recommended default way to 1042 * present this concept to a user. 1043 */ 1044 public ConceptDefinitionComponent setDisplay(String value) { 1045 if (Utilities.noString(value)) 1046 this.display = null; 1047 else { 1048 if (this.display == null) 1049 this.display = new StringType(); 1050 this.display.setValue(value); 1051 } 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #definition} (The formal definition of the concept. The value 1057 * set resource does not make formal definitions required, because of 1058 * the prevalence of legacy systems. However, they are highly 1059 * recommended, as without them there is no formal meaning associated 1060 * with the concept.). This is the underlying object with id, value and 1061 * extensions. The accessor "getDefinition" gives direct access to the 1062 * value 1063 */ 1064 public StringType getDefinitionElement() { 1065 if (this.definition == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create ConceptDefinitionComponent.definition"); 1068 else if (Configuration.doAutoCreate()) 1069 this.definition = new StringType(); // bb 1070 return this.definition; 1071 } 1072 1073 public boolean hasDefinitionElement() { 1074 return this.definition != null && !this.definition.isEmpty(); 1075 } 1076 1077 public boolean hasDefinition() { 1078 return this.definition != null && !this.definition.isEmpty(); 1079 } 1080 1081 /** 1082 * @param value {@link #definition} (The formal definition of the concept. The 1083 * value set resource does not make formal definitions required, 1084 * because of the prevalence of legacy systems. However, they are 1085 * highly recommended, as without them there is no formal meaning 1086 * associated with the concept.). This is the underlying object 1087 * with id, value and extensions. The accessor "getDefinition" 1088 * gives direct access to the value 1089 */ 1090 public ConceptDefinitionComponent setDefinitionElement(StringType value) { 1091 this.definition = value; 1092 return this; 1093 } 1094 1095 /** 1096 * @return The formal definition of the concept. The value set resource does not 1097 * make formal definitions required, because of the prevalence of legacy 1098 * systems. However, they are highly recommended, as without them there 1099 * is no formal meaning associated with the concept. 1100 */ 1101 public String getDefinition() { 1102 return this.definition == null ? null : this.definition.getValue(); 1103 } 1104 1105 /** 1106 * @param value The formal definition of the concept. The value set resource 1107 * does not make formal definitions required, because of the 1108 * prevalence of legacy systems. However, they are highly 1109 * recommended, as without them there is no formal meaning 1110 * associated with the concept. 1111 */ 1112 public ConceptDefinitionComponent setDefinition(String value) { 1113 if (Utilities.noString(value)) 1114 this.definition = null; 1115 else { 1116 if (this.definition == null) 1117 this.definition = new StringType(); 1118 this.definition.setValue(value); 1119 } 1120 return this; 1121 } 1122 1123 /** 1124 * @return {@link #designation} (Additional representations for the concept - 1125 * other languages, aliases, specialized purposes, used for particular 1126 * purposes, etc.) 1127 */ 1128 public List<ConceptDefinitionDesignationComponent> getDesignation() { 1129 if (this.designation == null) 1130 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1131 return this.designation; 1132 } 1133 1134 public boolean hasDesignation() { 1135 if (this.designation == null) 1136 return false; 1137 for (ConceptDefinitionDesignationComponent item : this.designation) 1138 if (!item.isEmpty()) 1139 return true; 1140 return false; 1141 } 1142 1143 /** 1144 * @return {@link #designation} (Additional representations for the concept - 1145 * other languages, aliases, specialized purposes, used for particular 1146 * purposes, etc.) 1147 */ 1148 // syntactic sugar 1149 public ConceptDefinitionDesignationComponent addDesignation() { // 3 1150 ConceptDefinitionDesignationComponent t = new ConceptDefinitionDesignationComponent(); 1151 if (this.designation == null) 1152 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1153 this.designation.add(t); 1154 return t; 1155 } 1156 1157 // syntactic sugar 1158 public ConceptDefinitionComponent addDesignation(ConceptDefinitionDesignationComponent t) { // 3 1159 if (t == null) 1160 return this; 1161 if (this.designation == null) 1162 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1163 this.designation.add(t); 1164 return this; 1165 } 1166 1167 /** 1168 * @return {@link #concept} (Defines children of a concept to produce a 1169 * hierarchy of concepts. The nature of the relationships is variable 1170 * (is-a/contains/categorizes) and can only be determined by examining 1171 * the definitions of the concepts.) 1172 */ 1173 public List<ConceptDefinitionComponent> getConcept() { 1174 if (this.concept == null) 1175 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1176 return this.concept; 1177 } 1178 1179 public boolean hasConcept() { 1180 if (this.concept == null) 1181 return false; 1182 for (ConceptDefinitionComponent item : this.concept) 1183 if (!item.isEmpty()) 1184 return true; 1185 return false; 1186 } 1187 1188 /** 1189 * @return {@link #concept} (Defines children of a concept to produce a 1190 * hierarchy of concepts. The nature of the relationships is variable 1191 * (is-a/contains/categorizes) and can only be determined by examining 1192 * the definitions of the concepts.) 1193 */ 1194 // syntactic sugar 1195 public ConceptDefinitionComponent addConcept() { // 3 1196 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 1197 if (this.concept == null) 1198 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1199 this.concept.add(t); 1200 return t; 1201 } 1202 1203 // syntactic sugar 1204 public ConceptDefinitionComponent addConcept(ConceptDefinitionComponent t) { // 3 1205 if (t == null) 1206 return this; 1207 if (this.concept == null) 1208 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1209 this.concept.add(t); 1210 return this; 1211 } 1212 1213 protected void listChildren(List<Property> childrenList) { 1214 super.listChildren(childrenList); 1215 childrenList.add(new Property("code", "code", 1216 "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1217 java.lang.Integer.MAX_VALUE, code)); 1218 childrenList.add(new Property("abstract", "boolean", "If this code is not for use as a real concept.", 0, 1219 java.lang.Integer.MAX_VALUE, abstract_)); 1220 childrenList.add(new Property("display", "string", 1221 "A human readable string that is the recommended default way to present this concept to a user.", 0, 1222 java.lang.Integer.MAX_VALUE, display)); 1223 childrenList.add(new Property("definition", "string", 1224 "The formal definition of the concept. The value set resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 1225 0, java.lang.Integer.MAX_VALUE, definition)); 1226 childrenList.add(new Property("designation", "", 1227 "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 1228 0, java.lang.Integer.MAX_VALUE, designation)); 1229 childrenList.add(new Property("concept", "@ValueSet.codeSystem.concept", 1230 "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) and can only be determined by examining the definitions of the concepts.", 1231 0, java.lang.Integer.MAX_VALUE, concept)); 1232 } 1233 1234 @Override 1235 public void setProperty(String name, Base value) throws FHIRException { 1236 if (name.equals("code")) 1237 this.code = castToCode(value); // CodeType 1238 else if (name.equals("abstract")) 1239 this.abstract_ = castToBoolean(value); // BooleanType 1240 else if (name.equals("display")) 1241 this.display = castToString(value); // StringType 1242 else if (name.equals("definition")) 1243 this.definition = castToString(value); // StringType 1244 else if (name.equals("designation")) 1245 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); 1246 else if (name.equals("concept")) 1247 this.getConcept().add((ConceptDefinitionComponent) value); 1248 else 1249 super.setProperty(name, value); 1250 } 1251 1252 @Override 1253 public Base addChild(String name) throws FHIRException { 1254 if (name.equals("code")) { 1255 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 1256 } else if (name.equals("abstract")) { 1257 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.abstract"); 1258 } else if (name.equals("display")) { 1259 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 1260 } else if (name.equals("definition")) { 1261 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.definition"); 1262 } else if (name.equals("designation")) { 1263 return addDesignation(); 1264 } else if (name.equals("concept")) { 1265 return addConcept(); 1266 } else 1267 return super.addChild(name); 1268 } 1269 1270 public ConceptDefinitionComponent copy() { 1271 ConceptDefinitionComponent dst = new ConceptDefinitionComponent(); 1272 copyValues(dst); 1273 dst.code = code == null ? null : code.copy(); 1274 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 1275 dst.display = display == null ? null : display.copy(); 1276 dst.definition = definition == null ? null : definition.copy(); 1277 if (designation != null) { 1278 dst.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1279 for (ConceptDefinitionDesignationComponent i : designation) 1280 dst.designation.add(i.copy()); 1281 } 1282 ; 1283 if (concept != null) { 1284 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 1285 for (ConceptDefinitionComponent i : concept) 1286 dst.concept.add(i.copy()); 1287 } 1288 ; 1289 return dst; 1290 } 1291 1292 @Override 1293 public boolean equalsDeep(Base other) { 1294 if (!super.equalsDeep(other)) 1295 return false; 1296 if (!(other instanceof ConceptDefinitionComponent)) 1297 return false; 1298 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other; 1299 return compareDeep(code, o.code, true) && compareDeep(abstract_, o.abstract_, true) 1300 && compareDeep(display, o.display, true) && compareDeep(definition, o.definition, true) 1301 && compareDeep(designation, o.designation, true) && compareDeep(concept, o.concept, true); 1302 } 1303 1304 @Override 1305 public boolean equalsShallow(Base other) { 1306 if (!super.equalsShallow(other)) 1307 return false; 1308 if (!(other instanceof ConceptDefinitionComponent)) 1309 return false; 1310 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other; 1311 return compareValues(code, o.code, true) && compareValues(abstract_, o.abstract_, true) 1312 && compareValues(display, o.display, true) && compareValues(definition, o.definition, true); 1313 } 1314 1315 public boolean isEmpty() { 1316 return super.isEmpty() && (code == null || code.isEmpty()) && (abstract_ == null || abstract_.isEmpty()) 1317 && (display == null || display.isEmpty()) && (definition == null || definition.isEmpty()) 1318 && (designation == null || designation.isEmpty()) && (concept == null || concept.isEmpty()); 1319 } 1320 1321 public String fhirType() { 1322 return "ValueSet.codeSystem.concept"; 1323 1324 } 1325 1326 } 1327 1328 @Block() 1329 public static class ConceptDefinitionDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1330 /** 1331 * The language this designation is defined for. 1332 */ 1333 @Child(name = "language", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1334 @Description(shortDefinition = "Human language of the designation", formalDefinition = "The language this designation is defined for.") 1335 protected CodeType language; 1336 1337 /** 1338 * A code that details how this designation would be used. 1339 */ 1340 @Child(name = "use", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1341 @Description(shortDefinition = "Details how this designation would be used", formalDefinition = "A code that details how this designation would be used.") 1342 protected Coding use; 1343 1344 /** 1345 * The text value for this designation. 1346 */ 1347 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1348 @Description(shortDefinition = "The text value for this designation", formalDefinition = "The text value for this designation.") 1349 protected StringType value; 1350 1351 private static final long serialVersionUID = 1515662414L; 1352 1353 /* 1354 * Constructor 1355 */ 1356 public ConceptDefinitionDesignationComponent() { 1357 super(); 1358 } 1359 1360 /* 1361 * Constructor 1362 */ 1363 public ConceptDefinitionDesignationComponent(StringType value) { 1364 super(); 1365 this.value = value; 1366 } 1367 1368 /** 1369 * @return {@link #language} (The language this designation is defined for.). 1370 * This is the underlying object with id, value and extensions. The 1371 * accessor "getLanguage" gives direct access to the value 1372 */ 1373 public CodeType getLanguageElement() { 1374 if (this.language == null) 1375 if (Configuration.errorOnAutoCreate()) 1376 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.language"); 1377 else if (Configuration.doAutoCreate()) 1378 this.language = new CodeType(); // bb 1379 return this.language; 1380 } 1381 1382 public boolean hasLanguageElement() { 1383 return this.language != null && !this.language.isEmpty(); 1384 } 1385 1386 public boolean hasLanguage() { 1387 return this.language != null && !this.language.isEmpty(); 1388 } 1389 1390 /** 1391 * @param value {@link #language} (The language this designation is defined 1392 * for.). This is the underlying object with id, value and 1393 * extensions. The accessor "getLanguage" gives direct access to 1394 * the value 1395 */ 1396 public ConceptDefinitionDesignationComponent setLanguageElement(CodeType value) { 1397 this.language = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return The language this designation is defined for. 1403 */ 1404 public String getLanguage() { 1405 return this.language == null ? null : this.language.getValue(); 1406 } 1407 1408 /** 1409 * @param value The language this designation is defined for. 1410 */ 1411 public ConceptDefinitionDesignationComponent setLanguage(String value) { 1412 if (Utilities.noString(value)) 1413 this.language = null; 1414 else { 1415 if (this.language == null) 1416 this.language = new CodeType(); 1417 this.language.setValue(value); 1418 } 1419 return this; 1420 } 1421 1422 /** 1423 * @return {@link #use} (A code that details how this designation would be 1424 * used.) 1425 */ 1426 public Coding getUse() { 1427 if (this.use == null) 1428 if (Configuration.errorOnAutoCreate()) 1429 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.use"); 1430 else if (Configuration.doAutoCreate()) 1431 this.use = new Coding(); // cc 1432 return this.use; 1433 } 1434 1435 public boolean hasUse() { 1436 return this.use != null && !this.use.isEmpty(); 1437 } 1438 1439 /** 1440 * @param value {@link #use} (A code that details how this designation would be 1441 * used.) 1442 */ 1443 public ConceptDefinitionDesignationComponent setUse(Coding value) { 1444 this.use = value; 1445 return this; 1446 } 1447 1448 /** 1449 * @return {@link #value} (The text value for this designation.). This is the 1450 * underlying object with id, value and extensions. The accessor 1451 * "getValue" gives direct access to the value 1452 */ 1453 public StringType getValueElement() { 1454 if (this.value == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.value"); 1457 else if (Configuration.doAutoCreate()) 1458 this.value = new StringType(); // bb 1459 return this.value; 1460 } 1461 1462 public boolean hasValueElement() { 1463 return this.value != null && !this.value.isEmpty(); 1464 } 1465 1466 public boolean hasValue() { 1467 return this.value != null && !this.value.isEmpty(); 1468 } 1469 1470 /** 1471 * @param value {@link #value} (The text value for this designation.). This is 1472 * the underlying object with id, value and extensions. The 1473 * accessor "getValue" gives direct access to the value 1474 */ 1475 public ConceptDefinitionDesignationComponent setValueElement(StringType value) { 1476 this.value = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return The text value for this designation. 1482 */ 1483 public String getValue() { 1484 return this.value == null ? null : this.value.getValue(); 1485 } 1486 1487 /** 1488 * @param value The text value for this designation. 1489 */ 1490 public ConceptDefinitionDesignationComponent setValue(String value) { 1491 if (this.value == null) 1492 this.value = new StringType(); 1493 this.value.setValue(value); 1494 return this; 1495 } 1496 1497 protected void listChildren(List<Property> childrenList) { 1498 super.listChildren(childrenList); 1499 childrenList.add(new Property("language", "code", "The language this designation is defined for.", 0, 1500 java.lang.Integer.MAX_VALUE, language)); 1501 childrenList.add(new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1502 java.lang.Integer.MAX_VALUE, use)); 1503 childrenList.add(new Property("value", "string", "The text value for this designation.", 0, 1504 java.lang.Integer.MAX_VALUE, value)); 1505 } 1506 1507 @Override 1508 public void setProperty(String name, Base value) throws FHIRException { 1509 if (name.equals("language")) 1510 this.language = castToCode(value); // CodeType 1511 else if (name.equals("use")) 1512 this.use = castToCoding(value); // Coding 1513 else if (name.equals("value")) 1514 this.value = castToString(value); // StringType 1515 else 1516 super.setProperty(name, value); 1517 } 1518 1519 @Override 1520 public Base addChild(String name) throws FHIRException { 1521 if (name.equals("language")) { 1522 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.language"); 1523 } else if (name.equals("use")) { 1524 this.use = new Coding(); 1525 return this.use; 1526 } else if (name.equals("value")) { 1527 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.value"); 1528 } else 1529 return super.addChild(name); 1530 } 1531 1532 public ConceptDefinitionDesignationComponent copy() { 1533 ConceptDefinitionDesignationComponent dst = new ConceptDefinitionDesignationComponent(); 1534 copyValues(dst); 1535 dst.language = language == null ? null : language.copy(); 1536 dst.use = use == null ? null : use.copy(); 1537 dst.value = value == null ? null : value.copy(); 1538 return dst; 1539 } 1540 1541 @Override 1542 public boolean equalsDeep(Base other) { 1543 if (!super.equalsDeep(other)) 1544 return false; 1545 if (!(other instanceof ConceptDefinitionDesignationComponent)) 1546 return false; 1547 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other; 1548 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) 1549 && compareDeep(value, o.value, true); 1550 } 1551 1552 @Override 1553 public boolean equalsShallow(Base other) { 1554 if (!super.equalsShallow(other)) 1555 return false; 1556 if (!(other instanceof ConceptDefinitionDesignationComponent)) 1557 return false; 1558 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other; 1559 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 1560 } 1561 1562 public boolean isEmpty() { 1563 return super.isEmpty() && (language == null || language.isEmpty()) && (use == null || use.isEmpty()) 1564 && (value == null || value.isEmpty()); 1565 } 1566 1567 public String fhirType() { 1568 return "ValueSet.codeSystem.concept.designation"; 1569 1570 } 1571 1572 } 1573 1574 @Block() 1575 public static class ValueSetComposeComponent extends BackboneElement implements IBaseBackboneElement { 1576 /** 1577 * Includes the contents of the referenced value set as a part of the contents 1578 * of this value set. This is an absolute URI that is a reference to 1579 * ValueSet.uri. 1580 */ 1581 @Child(name = "import", type = { 1582 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1583 @Description(shortDefinition = "Import the contents of another value set", formalDefinition = "Includes the contents of the referenced value set as a part of the contents of this value set. This is an absolute URI that is a reference to ValueSet.uri.") 1584 protected List<UriType> import_; 1585 1586 /** 1587 * Include one or more codes from a code system. 1588 */ 1589 @Child(name = "include", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1590 @Description(shortDefinition = "Include one or more codes from a code system", formalDefinition = "Include one or more codes from a code system.") 1591 protected List<ConceptSetComponent> include; 1592 1593 /** 1594 * Exclude one or more codes from the value set. 1595 */ 1596 @Child(name = "exclude", type = { 1597 ConceptSetComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1598 @Description(shortDefinition = "Explicitly exclude codes", formalDefinition = "Exclude one or more codes from the value set.") 1599 protected List<ConceptSetComponent> exclude; 1600 1601 private static final long serialVersionUID = -703166694L; 1602 1603 /* 1604 * Constructor 1605 */ 1606 public ValueSetComposeComponent() { 1607 super(); 1608 } 1609 1610 /** 1611 * @return {@link #import_} (Includes the contents of the referenced value set 1612 * as a part of the contents of this value set. This is an absolute URI 1613 * that is a reference to ValueSet.uri.) 1614 */ 1615 public List<UriType> getImport() { 1616 if (this.import_ == null) 1617 this.import_ = new ArrayList<UriType>(); 1618 return this.import_; 1619 } 1620 1621 public boolean hasImport() { 1622 if (this.import_ == null) 1623 return false; 1624 for (UriType item : this.import_) 1625 if (!item.isEmpty()) 1626 return true; 1627 return false; 1628 } 1629 1630 /** 1631 * @return {@link #import_} (Includes the contents of the referenced value set 1632 * as a part of the contents of this value set. This is an absolute URI 1633 * that is a reference to ValueSet.uri.) 1634 */ 1635 // syntactic sugar 1636 public UriType addImportElement() {// 2 1637 UriType t = new UriType(); 1638 if (this.import_ == null) 1639 this.import_ = new ArrayList<UriType>(); 1640 this.import_.add(t); 1641 return t; 1642 } 1643 1644 /** 1645 * @param value {@link #import_} (Includes the contents of the referenced value 1646 * set as a part of the contents of this value set. This is an 1647 * absolute URI that is a reference to ValueSet.uri.) 1648 */ 1649 public ValueSetComposeComponent addImport(String value) { // 1 1650 UriType t = new UriType(); 1651 t.setValue(value); 1652 if (this.import_ == null) 1653 this.import_ = new ArrayList<UriType>(); 1654 this.import_.add(t); 1655 return this; 1656 } 1657 1658 /** 1659 * @param value {@link #import_} (Includes the contents of the referenced value 1660 * set as a part of the contents of this value set. This is an 1661 * absolute URI that is a reference to ValueSet.uri.) 1662 */ 1663 public boolean hasImport(String value) { 1664 if (this.import_ == null) 1665 return false; 1666 for (UriType v : this.import_) 1667 if (v.equals(value)) // uri 1668 return true; 1669 return false; 1670 } 1671 1672 /** 1673 * @return {@link #include} (Include one or more codes from a code system.) 1674 */ 1675 public List<ConceptSetComponent> getInclude() { 1676 if (this.include == null) 1677 this.include = new ArrayList<ConceptSetComponent>(); 1678 return this.include; 1679 } 1680 1681 public boolean hasInclude() { 1682 if (this.include == null) 1683 return false; 1684 for (ConceptSetComponent item : this.include) 1685 if (!item.isEmpty()) 1686 return true; 1687 return false; 1688 } 1689 1690 /** 1691 * @return {@link #include} (Include one or more codes from a code system.) 1692 */ 1693 // syntactic sugar 1694 public ConceptSetComponent addInclude() { // 3 1695 ConceptSetComponent t = new ConceptSetComponent(); 1696 if (this.include == null) 1697 this.include = new ArrayList<ConceptSetComponent>(); 1698 this.include.add(t); 1699 return t; 1700 } 1701 1702 // syntactic sugar 1703 public ValueSetComposeComponent addInclude(ConceptSetComponent t) { // 3 1704 if (t == null) 1705 return this; 1706 if (this.include == null) 1707 this.include = new ArrayList<ConceptSetComponent>(); 1708 this.include.add(t); 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #exclude} (Exclude one or more codes from the value set.) 1714 */ 1715 public List<ConceptSetComponent> getExclude() { 1716 if (this.exclude == null) 1717 this.exclude = new ArrayList<ConceptSetComponent>(); 1718 return this.exclude; 1719 } 1720 1721 public boolean hasExclude() { 1722 if (this.exclude == null) 1723 return false; 1724 for (ConceptSetComponent item : this.exclude) 1725 if (!item.isEmpty()) 1726 return true; 1727 return false; 1728 } 1729 1730 /** 1731 * @return {@link #exclude} (Exclude one or more codes from the value set.) 1732 */ 1733 // syntactic sugar 1734 public ConceptSetComponent addExclude() { // 3 1735 ConceptSetComponent t = new ConceptSetComponent(); 1736 if (this.exclude == null) 1737 this.exclude = new ArrayList<ConceptSetComponent>(); 1738 this.exclude.add(t); 1739 return t; 1740 } 1741 1742 // syntactic sugar 1743 public ValueSetComposeComponent addExclude(ConceptSetComponent t) { // 3 1744 if (t == null) 1745 return this; 1746 if (this.exclude == null) 1747 this.exclude = new ArrayList<ConceptSetComponent>(); 1748 this.exclude.add(t); 1749 return this; 1750 } 1751 1752 protected void listChildren(List<Property> childrenList) { 1753 super.listChildren(childrenList); 1754 childrenList.add(new Property("import", "uri", 1755 "Includes the contents of the referenced value set as a part of the contents of this value set. This is an absolute URI that is a reference to ValueSet.uri.", 1756 0, java.lang.Integer.MAX_VALUE, import_)); 1757 childrenList.add(new Property("include", "", "Include one or more codes from a code system.", 0, 1758 java.lang.Integer.MAX_VALUE, include)); 1759 childrenList.add(new Property("exclude", "@ValueSet.compose.include", 1760 "Exclude one or more codes from the value set.", 0, java.lang.Integer.MAX_VALUE, exclude)); 1761 } 1762 1763 @Override 1764 public void setProperty(String name, Base value) throws FHIRException { 1765 if (name.equals("import")) 1766 this.getImport().add(castToUri(value)); 1767 else if (name.equals("include")) 1768 this.getInclude().add((ConceptSetComponent) value); 1769 else if (name.equals("exclude")) 1770 this.getExclude().add((ConceptSetComponent) value); 1771 else 1772 super.setProperty(name, value); 1773 } 1774 1775 @Override 1776 public Base addChild(String name) throws FHIRException { 1777 if (name.equals("import")) { 1778 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.import"); 1779 } else if (name.equals("include")) { 1780 return addInclude(); 1781 } else if (name.equals("exclude")) { 1782 return addExclude(); 1783 } else 1784 return super.addChild(name); 1785 } 1786 1787 public ValueSetComposeComponent copy() { 1788 ValueSetComposeComponent dst = new ValueSetComposeComponent(); 1789 copyValues(dst); 1790 if (import_ != null) { 1791 dst.import_ = new ArrayList<UriType>(); 1792 for (UriType i : import_) 1793 dst.import_.add(i.copy()); 1794 } 1795 ; 1796 if (include != null) { 1797 dst.include = new ArrayList<ConceptSetComponent>(); 1798 for (ConceptSetComponent i : include) 1799 dst.include.add(i.copy()); 1800 } 1801 ; 1802 if (exclude != null) { 1803 dst.exclude = new ArrayList<ConceptSetComponent>(); 1804 for (ConceptSetComponent i : exclude) 1805 dst.exclude.add(i.copy()); 1806 } 1807 ; 1808 return dst; 1809 } 1810 1811 @Override 1812 public boolean equalsDeep(Base other) { 1813 if (!super.equalsDeep(other)) 1814 return false; 1815 if (!(other instanceof ValueSetComposeComponent)) 1816 return false; 1817 ValueSetComposeComponent o = (ValueSetComposeComponent) other; 1818 return compareDeep(import_, o.import_, true) && compareDeep(include, o.include, true) 1819 && compareDeep(exclude, o.exclude, true); 1820 } 1821 1822 @Override 1823 public boolean equalsShallow(Base other) { 1824 if (!super.equalsShallow(other)) 1825 return false; 1826 if (!(other instanceof ValueSetComposeComponent)) 1827 return false; 1828 ValueSetComposeComponent o = (ValueSetComposeComponent) other; 1829 return compareValues(import_, o.import_, true); 1830 } 1831 1832 public boolean isEmpty() { 1833 return super.isEmpty() && (import_ == null || import_.isEmpty()) && (include == null || include.isEmpty()) 1834 && (exclude == null || exclude.isEmpty()); 1835 } 1836 1837 public String fhirType() { 1838 return "ValueSet.compose"; 1839 1840 } 1841 1842 } 1843 1844 @Block() 1845 public static class ConceptSetComponent extends BackboneElement implements IBaseBackboneElement { 1846 /** 1847 * An absolute URI which is the code system from which the selected codes come 1848 * from. 1849 */ 1850 @Child(name = "system", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1851 @Description(shortDefinition = "The system the codes come from", formalDefinition = "An absolute URI which is the code system from which the selected codes come from.") 1852 protected UriType system; 1853 1854 /** 1855 * The version of the code system that the codes are selected from. 1856 */ 1857 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1858 @Description(shortDefinition = "Specific version of the code system referred to", formalDefinition = "The version of the code system that the codes are selected from.") 1859 protected StringType version; 1860 1861 /** 1862 * Specifies a concept to be included or excluded. 1863 */ 1864 @Child(name = "concept", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1865 @Description(shortDefinition = "A concept defined in the system", formalDefinition = "Specifies a concept to be included or excluded.") 1866 protected List<ConceptReferenceComponent> concept; 1867 1868 /** 1869 * Select concepts by specify a matching criteria based on the properties 1870 * (including relationships) defined by the system. If multiple filters are 1871 * specified, they SHALL all be true. 1872 */ 1873 @Child(name = "filter", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1874 @Description(shortDefinition = "Select codes/concepts by their properties (including relationships)", formalDefinition = "Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true.") 1875 protected List<ConceptSetFilterComponent> filter; 1876 1877 private static final long serialVersionUID = -196054471L; 1878 1879 /* 1880 * Constructor 1881 */ 1882 public ConceptSetComponent() { 1883 super(); 1884 } 1885 1886 /* 1887 * Constructor 1888 */ 1889 public ConceptSetComponent(UriType system) { 1890 super(); 1891 this.system = system; 1892 } 1893 1894 /** 1895 * @return {@link #system} (An absolute URI which is the code system from which 1896 * the selected codes come from.). This is the underlying object with 1897 * id, value and extensions. The accessor "getSystem" gives direct 1898 * access to the value 1899 */ 1900 public UriType getSystemElement() { 1901 if (this.system == null) 1902 if (Configuration.errorOnAutoCreate()) 1903 throw new Error("Attempt to auto-create ConceptSetComponent.system"); 1904 else if (Configuration.doAutoCreate()) 1905 this.system = new UriType(); // bb 1906 return this.system; 1907 } 1908 1909 public boolean hasSystemElement() { 1910 return this.system != null && !this.system.isEmpty(); 1911 } 1912 1913 public boolean hasSystem() { 1914 return this.system != null && !this.system.isEmpty(); 1915 } 1916 1917 /** 1918 * @param value {@link #system} (An absolute URI which is the code system from 1919 * which the selected codes come from.). This is the underlying 1920 * object with id, value and extensions. The accessor "getSystem" 1921 * gives direct access to the value 1922 */ 1923 public ConceptSetComponent setSystemElement(UriType value) { 1924 this.system = value; 1925 return this; 1926 } 1927 1928 /** 1929 * @return An absolute URI which is the code system from which the selected 1930 * codes come from. 1931 */ 1932 public String getSystem() { 1933 return this.system == null ? null : this.system.getValue(); 1934 } 1935 1936 /** 1937 * @param value An absolute URI which is the code system from which the selected 1938 * codes come from. 1939 */ 1940 public ConceptSetComponent setSystem(String value) { 1941 if (this.system == null) 1942 this.system = new UriType(); 1943 this.system.setValue(value); 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #version} (The version of the code system that the codes are 1949 * selected from.). This is the underlying object with id, value and 1950 * extensions. The accessor "getVersion" gives direct access to the 1951 * value 1952 */ 1953 public StringType getVersionElement() { 1954 if (this.version == null) 1955 if (Configuration.errorOnAutoCreate()) 1956 throw new Error("Attempt to auto-create ConceptSetComponent.version"); 1957 else if (Configuration.doAutoCreate()) 1958 this.version = new StringType(); // bb 1959 return this.version; 1960 } 1961 1962 public boolean hasVersionElement() { 1963 return this.version != null && !this.version.isEmpty(); 1964 } 1965 1966 public boolean hasVersion() { 1967 return this.version != null && !this.version.isEmpty(); 1968 } 1969 1970 /** 1971 * @param value {@link #version} (The version of the code system that the codes 1972 * are selected from.). This is the underlying object with id, 1973 * value and extensions. The accessor "getVersion" gives direct 1974 * access to the value 1975 */ 1976 public ConceptSetComponent setVersionElement(StringType value) { 1977 this.version = value; 1978 return this; 1979 } 1980 1981 /** 1982 * @return The version of the code system that the codes are selected from. 1983 */ 1984 public String getVersion() { 1985 return this.version == null ? null : this.version.getValue(); 1986 } 1987 1988 /** 1989 * @param value The version of the code system that the codes are selected from. 1990 */ 1991 public ConceptSetComponent setVersion(String value) { 1992 if (Utilities.noString(value)) 1993 this.version = null; 1994 else { 1995 if (this.version == null) 1996 this.version = new StringType(); 1997 this.version.setValue(value); 1998 } 1999 return this; 2000 } 2001 2002 /** 2003 * @return {@link #concept} (Specifies a concept to be included or excluded.) 2004 */ 2005 public List<ConceptReferenceComponent> getConcept() { 2006 if (this.concept == null) 2007 this.concept = new ArrayList<ConceptReferenceComponent>(); 2008 return this.concept; 2009 } 2010 2011 public boolean hasConcept() { 2012 if (this.concept == null) 2013 return false; 2014 for (ConceptReferenceComponent item : this.concept) 2015 if (!item.isEmpty()) 2016 return true; 2017 return false; 2018 } 2019 2020 /** 2021 * @return {@link #concept} (Specifies a concept to be included or excluded.) 2022 */ 2023 // syntactic sugar 2024 public ConceptReferenceComponent addConcept() { // 3 2025 ConceptReferenceComponent t = new ConceptReferenceComponent(); 2026 if (this.concept == null) 2027 this.concept = new ArrayList<ConceptReferenceComponent>(); 2028 this.concept.add(t); 2029 return t; 2030 } 2031 2032 // syntactic sugar 2033 public ConceptSetComponent addConcept(ConceptReferenceComponent t) { // 3 2034 if (t == null) 2035 return this; 2036 if (this.concept == null) 2037 this.concept = new ArrayList<ConceptReferenceComponent>(); 2038 this.concept.add(t); 2039 return this; 2040 } 2041 2042 /** 2043 * @return {@link #filter} (Select concepts by specify a matching criteria based 2044 * on the properties (including relationships) defined by the system. If 2045 * multiple filters are specified, they SHALL all be true.) 2046 */ 2047 public List<ConceptSetFilterComponent> getFilter() { 2048 if (this.filter == null) 2049 this.filter = new ArrayList<ConceptSetFilterComponent>(); 2050 return this.filter; 2051 } 2052 2053 public boolean hasFilter() { 2054 if (this.filter == null) 2055 return false; 2056 for (ConceptSetFilterComponent item : this.filter) 2057 if (!item.isEmpty()) 2058 return true; 2059 return false; 2060 } 2061 2062 /** 2063 * @return {@link #filter} (Select concepts by specify a matching criteria based 2064 * on the properties (including relationships) defined by the system. If 2065 * multiple filters are specified, they SHALL all be true.) 2066 */ 2067 // syntactic sugar 2068 public ConceptSetFilterComponent addFilter() { // 3 2069 ConceptSetFilterComponent t = new ConceptSetFilterComponent(); 2070 if (this.filter == null) 2071 this.filter = new ArrayList<ConceptSetFilterComponent>(); 2072 this.filter.add(t); 2073 return t; 2074 } 2075 2076 // syntactic sugar 2077 public ConceptSetComponent addFilter(ConceptSetFilterComponent t) { // 3 2078 if (t == null) 2079 return this; 2080 if (this.filter == null) 2081 this.filter = new ArrayList<ConceptSetFilterComponent>(); 2082 this.filter.add(t); 2083 return this; 2084 } 2085 2086 protected void listChildren(List<Property> childrenList) { 2087 super.listChildren(childrenList); 2088 childrenList.add(new Property("system", "uri", 2089 "An absolute URI which is the code system from which the selected codes come from.", 0, 2090 java.lang.Integer.MAX_VALUE, system)); 2091 childrenList.add(new Property("version", "string", 2092 "The version of the code system that the codes are selected from.", 0, java.lang.Integer.MAX_VALUE, version)); 2093 childrenList.add(new Property("concept", "", "Specifies a concept to be included or excluded.", 0, 2094 java.lang.Integer.MAX_VALUE, concept)); 2095 childrenList.add(new Property("filter", "", 2096 "Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true.", 2097 0, java.lang.Integer.MAX_VALUE, filter)); 2098 } 2099 2100 @Override 2101 public void setProperty(String name, Base value) throws FHIRException { 2102 if (name.equals("system")) 2103 this.system = castToUri(value); // UriType 2104 else if (name.equals("version")) 2105 this.version = castToString(value); // StringType 2106 else if (name.equals("concept")) 2107 this.getConcept().add((ConceptReferenceComponent) value); 2108 else if (name.equals("filter")) 2109 this.getFilter().add((ConceptSetFilterComponent) value); 2110 else 2111 super.setProperty(name, value); 2112 } 2113 2114 @Override 2115 public Base addChild(String name) throws FHIRException { 2116 if (name.equals("system")) { 2117 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 2118 } else if (name.equals("version")) { 2119 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 2120 } else if (name.equals("concept")) { 2121 return addConcept(); 2122 } else if (name.equals("filter")) { 2123 return addFilter(); 2124 } else 2125 return super.addChild(name); 2126 } 2127 2128 public ConceptSetComponent copy() { 2129 ConceptSetComponent dst = new ConceptSetComponent(); 2130 copyValues(dst); 2131 dst.system = system == null ? null : system.copy(); 2132 dst.version = version == null ? null : version.copy(); 2133 if (concept != null) { 2134 dst.concept = new ArrayList<ConceptReferenceComponent>(); 2135 for (ConceptReferenceComponent i : concept) 2136 dst.concept.add(i.copy()); 2137 } 2138 ; 2139 if (filter != null) { 2140 dst.filter = new ArrayList<ConceptSetFilterComponent>(); 2141 for (ConceptSetFilterComponent i : filter) 2142 dst.filter.add(i.copy()); 2143 } 2144 ; 2145 return dst; 2146 } 2147 2148 @Override 2149 public boolean equalsDeep(Base other) { 2150 if (!super.equalsDeep(other)) 2151 return false; 2152 if (!(other instanceof ConceptSetComponent)) 2153 return false; 2154 ConceptSetComponent o = (ConceptSetComponent) other; 2155 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) 2156 && compareDeep(concept, o.concept, true) && compareDeep(filter, o.filter, true); 2157 } 2158 2159 @Override 2160 public boolean equalsShallow(Base other) { 2161 if (!super.equalsShallow(other)) 2162 return false; 2163 if (!(other instanceof ConceptSetComponent)) 2164 return false; 2165 ConceptSetComponent o = (ConceptSetComponent) other; 2166 return compareValues(system, o.system, true) && compareValues(version, o.version, true); 2167 } 2168 2169 public boolean isEmpty() { 2170 return super.isEmpty() && (system == null || system.isEmpty()) && (version == null || version.isEmpty()) 2171 && (concept == null || concept.isEmpty()) && (filter == null || filter.isEmpty()); 2172 } 2173 2174 public String fhirType() { 2175 return "ValueSet.compose.include"; 2176 2177 } 2178 2179 } 2180 2181 @Block() 2182 public static class ConceptReferenceComponent extends BackboneElement implements IBaseBackboneElement { 2183 /** 2184 * Specifies a code for the concept to be included or excluded. 2185 */ 2186 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2187 @Description(shortDefinition = "Code or expression from system", formalDefinition = "Specifies a code for the concept to be included or excluded.") 2188 protected CodeType code; 2189 2190 /** 2191 * The text to display to the user for this concept in the context of this 2192 * valueset. If no display is provided, then applications using the value set 2193 * use the display specified for the code by the system. 2194 */ 2195 @Child(name = "display", type = { 2196 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2197 @Description(shortDefinition = "Test to display for this code for this value set", formalDefinition = "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.") 2198 protected StringType display; 2199 2200 /** 2201 * Additional representations for this concept when used in this value set - 2202 * other languages, aliases, specialized purposes, used for particular purposes, 2203 * etc. 2204 */ 2205 @Child(name = "designation", type = { 2206 ConceptDefinitionDesignationComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2207 @Description(shortDefinition = "Additional representations for this valueset", formalDefinition = "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.") 2208 protected List<ConceptDefinitionDesignationComponent> designation; 2209 2210 private static final long serialVersionUID = -1513912691L; 2211 2212 /* 2213 * Constructor 2214 */ 2215 public ConceptReferenceComponent() { 2216 super(); 2217 } 2218 2219 /* 2220 * Constructor 2221 */ 2222 public ConceptReferenceComponent(CodeType code) { 2223 super(); 2224 this.code = code; 2225 } 2226 2227 /** 2228 * @return {@link #code} (Specifies a code for the concept to be included or 2229 * excluded.). This is the underlying object with id, value and 2230 * extensions. The accessor "getCode" gives direct access to the value 2231 */ 2232 public CodeType getCodeElement() { 2233 if (this.code == null) 2234 if (Configuration.errorOnAutoCreate()) 2235 throw new Error("Attempt to auto-create ConceptReferenceComponent.code"); 2236 else if (Configuration.doAutoCreate()) 2237 this.code = new CodeType(); // bb 2238 return this.code; 2239 } 2240 2241 public boolean hasCodeElement() { 2242 return this.code != null && !this.code.isEmpty(); 2243 } 2244 2245 public boolean hasCode() { 2246 return this.code != null && !this.code.isEmpty(); 2247 } 2248 2249 /** 2250 * @param value {@link #code} (Specifies a code for the concept to be included 2251 * or excluded.). This is the underlying object with id, value and 2252 * extensions. The accessor "getCode" gives direct access to the 2253 * value 2254 */ 2255 public ConceptReferenceComponent setCodeElement(CodeType value) { 2256 this.code = value; 2257 return this; 2258 } 2259 2260 /** 2261 * @return Specifies a code for the concept to be included or excluded. 2262 */ 2263 public String getCode() { 2264 return this.code == null ? null : this.code.getValue(); 2265 } 2266 2267 /** 2268 * @param value Specifies a code for the concept to be included or excluded. 2269 */ 2270 public ConceptReferenceComponent setCode(String value) { 2271 if (this.code == null) 2272 this.code = new CodeType(); 2273 this.code.setValue(value); 2274 return this; 2275 } 2276 2277 /** 2278 * @return {@link #display} (The text to display to the user for this concept in 2279 * the context of this valueset. If no display is provided, then 2280 * applications using the value set use the display specified for the 2281 * code by the system.). This is the underlying object with id, value 2282 * and extensions. The accessor "getDisplay" gives direct access to the 2283 * value 2284 */ 2285 public StringType getDisplayElement() { 2286 if (this.display == null) 2287 if (Configuration.errorOnAutoCreate()) 2288 throw new Error("Attempt to auto-create ConceptReferenceComponent.display"); 2289 else if (Configuration.doAutoCreate()) 2290 this.display = new StringType(); // bb 2291 return this.display; 2292 } 2293 2294 public boolean hasDisplayElement() { 2295 return this.display != null && !this.display.isEmpty(); 2296 } 2297 2298 public boolean hasDisplay() { 2299 return this.display != null && !this.display.isEmpty(); 2300 } 2301 2302 /** 2303 * @param value {@link #display} (The text to display to the user for this 2304 * concept in the context of this valueset. If no display is 2305 * provided, then applications using the value set use the display 2306 * specified for the code by the system.). This is the underlying 2307 * object with id, value and extensions. The accessor "getDisplay" 2308 * gives direct access to the value 2309 */ 2310 public ConceptReferenceComponent setDisplayElement(StringType value) { 2311 this.display = value; 2312 return this; 2313 } 2314 2315 /** 2316 * @return The text to display to the user for this concept in the context of 2317 * this valueset. If no display is provided, then applications using the 2318 * value set use the display specified for the code by the system. 2319 */ 2320 public String getDisplay() { 2321 return this.display == null ? null : this.display.getValue(); 2322 } 2323 2324 /** 2325 * @param value The text to display to the user for this concept in the context 2326 * of this valueset. If no display is provided, then applications 2327 * using the value set use the display specified for the code by 2328 * the system. 2329 */ 2330 public ConceptReferenceComponent setDisplay(String value) { 2331 if (Utilities.noString(value)) 2332 this.display = null; 2333 else { 2334 if (this.display == null) 2335 this.display = new StringType(); 2336 this.display.setValue(value); 2337 } 2338 return this; 2339 } 2340 2341 /** 2342 * @return {@link #designation} (Additional representations for this concept 2343 * when used in this value set - other languages, aliases, specialized 2344 * purposes, used for particular purposes, etc.) 2345 */ 2346 public List<ConceptDefinitionDesignationComponent> getDesignation() { 2347 if (this.designation == null) 2348 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2349 return this.designation; 2350 } 2351 2352 public boolean hasDesignation() { 2353 if (this.designation == null) 2354 return false; 2355 for (ConceptDefinitionDesignationComponent item : this.designation) 2356 if (!item.isEmpty()) 2357 return true; 2358 return false; 2359 } 2360 2361 /** 2362 * @return {@link #designation} (Additional representations for this concept 2363 * when used in this value set - other languages, aliases, specialized 2364 * purposes, used for particular purposes, etc.) 2365 */ 2366 // syntactic sugar 2367 public ConceptDefinitionDesignationComponent addDesignation() { // 3 2368 ConceptDefinitionDesignationComponent t = new ConceptDefinitionDesignationComponent(); 2369 if (this.designation == null) 2370 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2371 this.designation.add(t); 2372 return t; 2373 } 2374 2375 // syntactic sugar 2376 public ConceptReferenceComponent addDesignation(ConceptDefinitionDesignationComponent t) { // 3 2377 if (t == null) 2378 return this; 2379 if (this.designation == null) 2380 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2381 this.designation.add(t); 2382 return this; 2383 } 2384 2385 protected void listChildren(List<Property> childrenList) { 2386 super.listChildren(childrenList); 2387 childrenList.add(new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 0, 2388 java.lang.Integer.MAX_VALUE, code)); 2389 childrenList.add(new Property("display", "string", 2390 "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 2391 0, java.lang.Integer.MAX_VALUE, display)); 2392 childrenList.add(new Property("designation", "@ValueSet.codeSystem.concept.designation", 2393 "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 2394 0, java.lang.Integer.MAX_VALUE, designation)); 2395 } 2396 2397 @Override 2398 public void setProperty(String name, Base value) throws FHIRException { 2399 if (name.equals("code")) 2400 this.code = castToCode(value); // CodeType 2401 else if (name.equals("display")) 2402 this.display = castToString(value); // StringType 2403 else if (name.equals("designation")) 2404 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); 2405 else 2406 super.setProperty(name, value); 2407 } 2408 2409 @Override 2410 public Base addChild(String name) throws FHIRException { 2411 if (name.equals("code")) { 2412 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 2413 } else if (name.equals("display")) { 2414 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 2415 } else if (name.equals("designation")) { 2416 return addDesignation(); 2417 } else 2418 return super.addChild(name); 2419 } 2420 2421 public ConceptReferenceComponent copy() { 2422 ConceptReferenceComponent dst = new ConceptReferenceComponent(); 2423 copyValues(dst); 2424 dst.code = code == null ? null : code.copy(); 2425 dst.display = display == null ? null : display.copy(); 2426 if (designation != null) { 2427 dst.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2428 for (ConceptDefinitionDesignationComponent i : designation) 2429 dst.designation.add(i.copy()); 2430 } 2431 ; 2432 return dst; 2433 } 2434 2435 @Override 2436 public boolean equalsDeep(Base other) { 2437 if (!super.equalsDeep(other)) 2438 return false; 2439 if (!(other instanceof ConceptReferenceComponent)) 2440 return false; 2441 ConceptReferenceComponent o = (ConceptReferenceComponent) other; 2442 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 2443 && compareDeep(designation, o.designation, true); 2444 } 2445 2446 @Override 2447 public boolean equalsShallow(Base other) { 2448 if (!super.equalsShallow(other)) 2449 return false; 2450 if (!(other instanceof ConceptReferenceComponent)) 2451 return false; 2452 ConceptReferenceComponent o = (ConceptReferenceComponent) other; 2453 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 2454 } 2455 2456 public boolean isEmpty() { 2457 return super.isEmpty() && (code == null || code.isEmpty()) && (display == null || display.isEmpty()) 2458 && (designation == null || designation.isEmpty()); 2459 } 2460 2461 public String fhirType() { 2462 return "ValueSet.compose.include.concept"; 2463 2464 } 2465 2466 } 2467 2468 @Block() 2469 public static class ConceptSetFilterComponent extends BackboneElement implements IBaseBackboneElement { 2470 /** 2471 * A code that identifies a property defined in the code system. 2472 */ 2473 @Child(name = "property", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2474 @Description(shortDefinition = "A property defined by the code system", formalDefinition = "A code that identifies a property defined in the code system.") 2475 protected CodeType property; 2476 2477 /** 2478 * The kind of operation to perform as a part of the filter criteria. 2479 */ 2480 @Child(name = "op", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2481 @Description(shortDefinition = "= | is-a | is-not-a | regex | in | not-in", formalDefinition = "The kind of operation to perform as a part of the filter criteria.") 2482 protected Enumeration<FilterOperator> op; 2483 2484 /** 2485 * The match value may be either a code defined by the system, or a string 2486 * value, which is a regex match on the literal string of the property value. 2487 */ 2488 @Child(name = "value", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 2489 @Description(shortDefinition = "Code from the system, or regex criteria", formalDefinition = "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value.") 2490 protected CodeType value; 2491 2492 private static final long serialVersionUID = 1985515000L; 2493 2494 /* 2495 * Constructor 2496 */ 2497 public ConceptSetFilterComponent() { 2498 super(); 2499 } 2500 2501 /* 2502 * Constructor 2503 */ 2504 public ConceptSetFilterComponent(CodeType property, Enumeration<FilterOperator> op, CodeType value) { 2505 super(); 2506 this.property = property; 2507 this.op = op; 2508 this.value = value; 2509 } 2510 2511 /** 2512 * @return {@link #property} (A code that identifies a property defined in the 2513 * code system.). This is the underlying object with id, value and 2514 * extensions. The accessor "getProperty" gives direct access to the 2515 * value 2516 */ 2517 public CodeType getPropertyElement() { 2518 if (this.property == null) 2519 if (Configuration.errorOnAutoCreate()) 2520 throw new Error("Attempt to auto-create ConceptSetFilterComponent.property"); 2521 else if (Configuration.doAutoCreate()) 2522 this.property = new CodeType(); // bb 2523 return this.property; 2524 } 2525 2526 public boolean hasPropertyElement() { 2527 return this.property != null && !this.property.isEmpty(); 2528 } 2529 2530 public boolean hasProperty() { 2531 return this.property != null && !this.property.isEmpty(); 2532 } 2533 2534 /** 2535 * @param value {@link #property} (A code that identifies a property defined in 2536 * the code system.). This is the underlying object with id, value 2537 * and extensions. The accessor "getProperty" gives direct access 2538 * to the value 2539 */ 2540 public ConceptSetFilterComponent setPropertyElement(CodeType value) { 2541 this.property = value; 2542 return this; 2543 } 2544 2545 /** 2546 * @return A code that identifies a property defined in the code system. 2547 */ 2548 public String getProperty() { 2549 return this.property == null ? null : this.property.getValue(); 2550 } 2551 2552 /** 2553 * @param value A code that identifies a property defined in the code system. 2554 */ 2555 public ConceptSetFilterComponent setProperty(String value) { 2556 if (this.property == null) 2557 this.property = new CodeType(); 2558 this.property.setValue(value); 2559 return this; 2560 } 2561 2562 /** 2563 * @return {@link #op} (The kind of operation to perform as a part of the filter 2564 * criteria.). This is the underlying object with id, value and 2565 * extensions. The accessor "getOp" gives direct access to the value 2566 */ 2567 public Enumeration<FilterOperator> getOpElement() { 2568 if (this.op == null) 2569 if (Configuration.errorOnAutoCreate()) 2570 throw new Error("Attempt to auto-create ConceptSetFilterComponent.op"); 2571 else if (Configuration.doAutoCreate()) 2572 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); // bb 2573 return this.op; 2574 } 2575 2576 public boolean hasOpElement() { 2577 return this.op != null && !this.op.isEmpty(); 2578 } 2579 2580 public boolean hasOp() { 2581 return this.op != null && !this.op.isEmpty(); 2582 } 2583 2584 /** 2585 * @param value {@link #op} (The kind of operation to perform as a part of the 2586 * filter criteria.). This is the underlying object with id, value 2587 * and extensions. The accessor "getOp" gives direct access to the 2588 * value 2589 */ 2590 public ConceptSetFilterComponent setOpElement(Enumeration<FilterOperator> value) { 2591 this.op = value; 2592 return this; 2593 } 2594 2595 /** 2596 * @return The kind of operation to perform as a part of the filter criteria. 2597 */ 2598 public FilterOperator getOp() { 2599 return this.op == null ? null : this.op.getValue(); 2600 } 2601 2602 /** 2603 * @param value The kind of operation to perform as a part of the filter 2604 * criteria. 2605 */ 2606 public ConceptSetFilterComponent setOp(FilterOperator value) { 2607 if (this.op == null) 2608 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 2609 this.op.setValue(value); 2610 return this; 2611 } 2612 2613 /** 2614 * @return {@link #value} (The match value may be either a code defined by the 2615 * system, or a string value, which is a regex match on the literal 2616 * string of the property value.). This is the underlying object with 2617 * id, value and extensions. The accessor "getValue" gives direct access 2618 * to the value 2619 */ 2620 public CodeType getValueElement() { 2621 if (this.value == null) 2622 if (Configuration.errorOnAutoCreate()) 2623 throw new Error("Attempt to auto-create ConceptSetFilterComponent.value"); 2624 else if (Configuration.doAutoCreate()) 2625 this.value = new CodeType(); // bb 2626 return this.value; 2627 } 2628 2629 public boolean hasValueElement() { 2630 return this.value != null && !this.value.isEmpty(); 2631 } 2632 2633 public boolean hasValue() { 2634 return this.value != null && !this.value.isEmpty(); 2635 } 2636 2637 /** 2638 * @param value {@link #value} (The match value may be either a code defined by 2639 * the system, or a string value, which is a regex match on the 2640 * literal string of the property value.). This is the underlying 2641 * object with id, value and extensions. The accessor "getValue" 2642 * gives direct access to the value 2643 */ 2644 public ConceptSetFilterComponent setValueElement(CodeType value) { 2645 this.value = value; 2646 return this; 2647 } 2648 2649 /** 2650 * @return The match value may be either a code defined by the system, or a 2651 * string value, which is a regex match on the literal string of the 2652 * property value. 2653 */ 2654 public String getValue() { 2655 return this.value == null ? null : this.value.getValue(); 2656 } 2657 2658 /** 2659 * @param value The match value may be either a code defined by the system, or a 2660 * string value, which is a regex match on the literal string of 2661 * the property value. 2662 */ 2663 public ConceptSetFilterComponent setValue(String value) { 2664 if (this.value == null) 2665 this.value = new CodeType(); 2666 this.value.setValue(value); 2667 return this; 2668 } 2669 2670 protected void listChildren(List<Property> childrenList) { 2671 super.listChildren(childrenList); 2672 childrenList.add(new Property("property", "code", "A code that identifies a property defined in the code system.", 2673 0, java.lang.Integer.MAX_VALUE, property)); 2674 childrenList.add(new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 2675 0, java.lang.Integer.MAX_VALUE, op)); 2676 childrenList.add(new Property("value", "code", 2677 "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value.", 2678 0, java.lang.Integer.MAX_VALUE, value)); 2679 } 2680 2681 @Override 2682 public void setProperty(String name, Base value) throws FHIRException { 2683 if (name.equals("property")) 2684 this.property = castToCode(value); // CodeType 2685 else if (name.equals("op")) 2686 this.op = new FilterOperatorEnumFactory().fromType(value); // Enumeration<FilterOperator> 2687 else if (name.equals("value")) 2688 this.value = castToCode(value); // CodeType 2689 else 2690 super.setProperty(name, value); 2691 } 2692 2693 @Override 2694 public Base addChild(String name) throws FHIRException { 2695 if (name.equals("property")) { 2696 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.property"); 2697 } else if (name.equals("op")) { 2698 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.op"); 2699 } else if (name.equals("value")) { 2700 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.value"); 2701 } else 2702 return super.addChild(name); 2703 } 2704 2705 public ConceptSetFilterComponent copy() { 2706 ConceptSetFilterComponent dst = new ConceptSetFilterComponent(); 2707 copyValues(dst); 2708 dst.property = property == null ? null : property.copy(); 2709 dst.op = op == null ? null : op.copy(); 2710 dst.value = value == null ? null : value.copy(); 2711 return dst; 2712 } 2713 2714 @Override 2715 public boolean equalsDeep(Base other) { 2716 if (!super.equalsDeep(other)) 2717 return false; 2718 if (!(other instanceof ConceptSetFilterComponent)) 2719 return false; 2720 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other; 2721 return compareDeep(property, o.property, true) && compareDeep(op, o.op, true) 2722 && compareDeep(value, o.value, true); 2723 } 2724 2725 @Override 2726 public boolean equalsShallow(Base other) { 2727 if (!super.equalsShallow(other)) 2728 return false; 2729 if (!(other instanceof ConceptSetFilterComponent)) 2730 return false; 2731 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other; 2732 return compareValues(property, o.property, true) && compareValues(op, o.op, true) 2733 && compareValues(value, o.value, true); 2734 } 2735 2736 public boolean isEmpty() { 2737 return super.isEmpty() && (property == null || property.isEmpty()) && (op == null || op.isEmpty()) 2738 && (value == null || value.isEmpty()); 2739 } 2740 2741 public String fhirType() { 2742 return "ValueSet.compose.include.filter"; 2743 2744 } 2745 2746 } 2747 2748 @Block() 2749 public static class ValueSetExpansionComponent extends BackboneElement implements IBaseBackboneElement { 2750 /** 2751 * An identifier that uniquely identifies this expansion of the valueset. 2752 * Systems may re-use the same identifier as long as the expansion and the 2753 * definition remain the same, but are not required to do so. 2754 */ 2755 @Child(name = "identifier", type = { 2756 UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2757 @Description(shortDefinition = "Uniquely identifies this expansion", formalDefinition = "An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so.") 2758 protected UriType identifier; 2759 2760 /** 2761 * The time at which the expansion was produced by the expanding system. 2762 */ 2763 @Child(name = "timestamp", type = { 2764 DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2765 @Description(shortDefinition = "Time ValueSet expansion happened", formalDefinition = "The time at which the expansion was produced by the expanding system.") 2766 protected DateTimeType timestamp; 2767 2768 /** 2769 * The total number of concepts in the expansion. If the number of concept nodes 2770 * in this resource is less than the stated number, then the server can return 2771 * more using the offset parameter. 2772 */ 2773 @Child(name = "total", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2774 @Description(shortDefinition = "Total number of codes in the expansion", formalDefinition = "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.") 2775 protected IntegerType total; 2776 2777 /** 2778 * If paging is being used, the offset at which this resource starts. I.e. this 2779 * resource is a partial view into the expansion. If paging is not being used, 2780 * this element SHALL not be present. 2781 */ 2782 @Child(name = "offset", type = { 2783 IntegerType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2784 @Description(shortDefinition = "Offset at which this resource starts", formalDefinition = "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present.") 2785 protected IntegerType offset; 2786 2787 /** 2788 * A parameter that controlled the expansion process. These parameters may be 2789 * used by users of expanded value sets to check whether the expansion is 2790 * suitable for a particular purpose, or to pick the correct expansion. 2791 */ 2792 @Child(name = "parameter", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2793 @Description(shortDefinition = "Parameter that controlled the expansion process", formalDefinition = "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.") 2794 protected List<ValueSetExpansionParameterComponent> parameter; 2795 2796 /** 2797 * The codes that are contained in the value set expansion. 2798 */ 2799 @Child(name = "contains", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2800 @Description(shortDefinition = "Codes in the value set", formalDefinition = "The codes that are contained in the value set expansion.") 2801 protected List<ValueSetExpansionContainsComponent> contains; 2802 2803 private static final long serialVersionUID = -43471993L; 2804 2805 /* 2806 * Constructor 2807 */ 2808 public ValueSetExpansionComponent() { 2809 super(); 2810 } 2811 2812 /* 2813 * Constructor 2814 */ 2815 public ValueSetExpansionComponent(UriType identifier, DateTimeType timestamp) { 2816 super(); 2817 this.identifier = identifier; 2818 this.timestamp = timestamp; 2819 } 2820 2821 /** 2822 * @return {@link #identifier} (An identifier that uniquely identifies this 2823 * expansion of the valueset. Systems may re-use the same identifier as 2824 * long as the expansion and the definition remain the same, but are not 2825 * required to do so.). This is the underlying object with id, value and 2826 * extensions. The accessor "getIdentifier" gives direct access to the 2827 * value 2828 */ 2829 public UriType getIdentifierElement() { 2830 if (this.identifier == null) 2831 if (Configuration.errorOnAutoCreate()) 2832 throw new Error("Attempt to auto-create ValueSetExpansionComponent.identifier"); 2833 else if (Configuration.doAutoCreate()) 2834 this.identifier = new UriType(); // bb 2835 return this.identifier; 2836 } 2837 2838 public boolean hasIdentifierElement() { 2839 return this.identifier != null && !this.identifier.isEmpty(); 2840 } 2841 2842 public boolean hasIdentifier() { 2843 return this.identifier != null && !this.identifier.isEmpty(); 2844 } 2845 2846 /** 2847 * @param value {@link #identifier} (An identifier that uniquely identifies this 2848 * expansion of the valueset. Systems may re-use the same 2849 * identifier as long as the expansion and the definition remain 2850 * the same, but are not required to do so.). This is the 2851 * underlying object with id, value and extensions. The accessor 2852 * "getIdentifier" gives direct access to the value 2853 */ 2854 public ValueSetExpansionComponent setIdentifierElement(UriType value) { 2855 this.identifier = value; 2856 return this; 2857 } 2858 2859 /** 2860 * @return An identifier that uniquely identifies this expansion of the 2861 * valueset. Systems may re-use the same identifier as long as the 2862 * expansion and the definition remain the same, but are not required to 2863 * do so. 2864 */ 2865 public String getIdentifier() { 2866 return this.identifier == null ? null : this.identifier.getValue(); 2867 } 2868 2869 /** 2870 * @param value An identifier that uniquely identifies this expansion of the 2871 * valueset. Systems may re-use the same identifier as long as the 2872 * expansion and the definition remain the same, but are not 2873 * required to do so. 2874 */ 2875 public ValueSetExpansionComponent setIdentifier(String value) { 2876 if (this.identifier == null) 2877 this.identifier = new UriType(); 2878 this.identifier.setValue(value); 2879 return this; 2880 } 2881 2882 /** 2883 * @return {@link #timestamp} (The time at which the expansion was produced by 2884 * the expanding system.). This is the underlying object with id, value 2885 * and extensions. The accessor "getTimestamp" gives direct access to 2886 * the value 2887 */ 2888 public DateTimeType getTimestampElement() { 2889 if (this.timestamp == null) 2890 if (Configuration.errorOnAutoCreate()) 2891 throw new Error("Attempt to auto-create ValueSetExpansionComponent.timestamp"); 2892 else if (Configuration.doAutoCreate()) 2893 this.timestamp = new DateTimeType(); // bb 2894 return this.timestamp; 2895 } 2896 2897 public boolean hasTimestampElement() { 2898 return this.timestamp != null && !this.timestamp.isEmpty(); 2899 } 2900 2901 public boolean hasTimestamp() { 2902 return this.timestamp != null && !this.timestamp.isEmpty(); 2903 } 2904 2905 /** 2906 * @param value {@link #timestamp} (The time at which the expansion was produced 2907 * by the expanding system.). This is the underlying object with 2908 * id, value and extensions. The accessor "getTimestamp" gives 2909 * direct access to the value 2910 */ 2911 public ValueSetExpansionComponent setTimestampElement(DateTimeType value) { 2912 this.timestamp = value; 2913 return this; 2914 } 2915 2916 /** 2917 * @return The time at which the expansion was produced by the expanding system. 2918 */ 2919 public Date getTimestamp() { 2920 return this.timestamp == null ? null : this.timestamp.getValue(); 2921 } 2922 2923 /** 2924 * @param value The time at which the expansion was produced by the expanding 2925 * system. 2926 */ 2927 public ValueSetExpansionComponent setTimestamp(Date value) { 2928 if (this.timestamp == null) 2929 this.timestamp = new DateTimeType(); 2930 this.timestamp.setValue(value); 2931 return this; 2932 } 2933 2934 /** 2935 * @return {@link #total} (The total number of concepts in the expansion. If the 2936 * number of concept nodes in this resource is less than the stated 2937 * number, then the server can return more using the offset parameter.). 2938 * This is the underlying object with id, value and extensions. The 2939 * accessor "getTotal" gives direct access to the value 2940 */ 2941 public IntegerType getTotalElement() { 2942 if (this.total == null) 2943 if (Configuration.errorOnAutoCreate()) 2944 throw new Error("Attempt to auto-create ValueSetExpansionComponent.total"); 2945 else if (Configuration.doAutoCreate()) 2946 this.total = new IntegerType(); // bb 2947 return this.total; 2948 } 2949 2950 public boolean hasTotalElement() { 2951 return this.total != null && !this.total.isEmpty(); 2952 } 2953 2954 public boolean hasTotal() { 2955 return this.total != null && !this.total.isEmpty(); 2956 } 2957 2958 /** 2959 * @param value {@link #total} (The total number of concepts in the expansion. 2960 * If the number of concept nodes in this resource is less than the 2961 * stated number, then the server can return more using the offset 2962 * parameter.). This is the underlying object with id, value and 2963 * extensions. The accessor "getTotal" gives direct access to the 2964 * value 2965 */ 2966 public ValueSetExpansionComponent setTotalElement(IntegerType value) { 2967 this.total = value; 2968 return this; 2969 } 2970 2971 /** 2972 * @return The total number of concepts in the expansion. If the number of 2973 * concept nodes in this resource is less than the stated number, then 2974 * the server can return more using the offset parameter. 2975 */ 2976 public int getTotal() { 2977 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 2978 } 2979 2980 /** 2981 * @param value The total number of concepts in the expansion. If the number of 2982 * concept nodes in this resource is less than the stated number, 2983 * then the server can return more using the offset parameter. 2984 */ 2985 public ValueSetExpansionComponent setTotal(int value) { 2986 if (this.total == null) 2987 this.total = new IntegerType(); 2988 this.total.setValue(value); 2989 return this; 2990 } 2991 2992 /** 2993 * @return {@link #offset} (If paging is being used, the offset at which this 2994 * resource starts. I.e. this resource is a partial view into the 2995 * expansion. If paging is not being used, this element SHALL not be 2996 * present.). This is the underlying object with id, value and 2997 * extensions. The accessor "getOffset" gives direct access to the value 2998 */ 2999 public IntegerType getOffsetElement() { 3000 if (this.offset == null) 3001 if (Configuration.errorOnAutoCreate()) 3002 throw new Error("Attempt to auto-create ValueSetExpansionComponent.offset"); 3003 else if (Configuration.doAutoCreate()) 3004 this.offset = new IntegerType(); // bb 3005 return this.offset; 3006 } 3007 3008 public boolean hasOffsetElement() { 3009 return this.offset != null && !this.offset.isEmpty(); 3010 } 3011 3012 public boolean hasOffset() { 3013 return this.offset != null && !this.offset.isEmpty(); 3014 } 3015 3016 /** 3017 * @param value {@link #offset} (If paging is being used, the offset at which 3018 * this resource starts. I.e. this resource is a partial view into 3019 * the expansion. If paging is not being used, this element SHALL 3020 * not be present.). This is the underlying object with id, value 3021 * and extensions. The accessor "getOffset" gives direct access to 3022 * the value 3023 */ 3024 public ValueSetExpansionComponent setOffsetElement(IntegerType value) { 3025 this.offset = value; 3026 return this; 3027 } 3028 3029 /** 3030 * @return If paging is being used, the offset at which this resource starts. 3031 * I.e. this resource is a partial view into the expansion. If paging is 3032 * not being used, this element SHALL not be present. 3033 */ 3034 public int getOffset() { 3035 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 3036 } 3037 3038 /** 3039 * @param value If paging is being used, the offset at which this resource 3040 * starts. I.e. this resource is a partial view into the expansion. 3041 * If paging is not being used, this element SHALL not be present. 3042 */ 3043 public ValueSetExpansionComponent setOffset(int value) { 3044 if (this.offset == null) 3045 this.offset = new IntegerType(); 3046 this.offset.setValue(value); 3047 return this; 3048 } 3049 3050 /** 3051 * @return {@link #parameter} (A parameter that controlled the expansion 3052 * process. These parameters may be used by users of expanded value sets 3053 * to check whether the expansion is suitable for a particular purpose, 3054 * or to pick the correct expansion.) 3055 */ 3056 public List<ValueSetExpansionParameterComponent> getParameter() { 3057 if (this.parameter == null) 3058 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 3059 return this.parameter; 3060 } 3061 3062 public boolean hasParameter() { 3063 if (this.parameter == null) 3064 return false; 3065 for (ValueSetExpansionParameterComponent item : this.parameter) 3066 if (!item.isEmpty()) 3067 return true; 3068 return false; 3069 } 3070 3071 /** 3072 * @return {@link #parameter} (A parameter that controlled the expansion 3073 * process. These parameters may be used by users of expanded value sets 3074 * to check whether the expansion is suitable for a particular purpose, 3075 * or to pick the correct expansion.) 3076 */ 3077 // syntactic sugar 3078 public ValueSetExpansionParameterComponent addParameter() { // 3 3079 ValueSetExpansionParameterComponent t = new ValueSetExpansionParameterComponent(); 3080 if (this.parameter == null) 3081 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 3082 this.parameter.add(t); 3083 return t; 3084 } 3085 3086 // syntactic sugar 3087 public ValueSetExpansionComponent addParameter(ValueSetExpansionParameterComponent t) { // 3 3088 if (t == null) 3089 return this; 3090 if (this.parameter == null) 3091 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 3092 this.parameter.add(t); 3093 return this; 3094 } 3095 3096 /** 3097 * @return {@link #contains} (The codes that are contained in the value set 3098 * expansion.) 3099 */ 3100 public List<ValueSetExpansionContainsComponent> getContains() { 3101 if (this.contains == null) 3102 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3103 return this.contains; 3104 } 3105 3106 public boolean hasContains() { 3107 if (this.contains == null) 3108 return false; 3109 for (ValueSetExpansionContainsComponent item : this.contains) 3110 if (!item.isEmpty()) 3111 return true; 3112 return false; 3113 } 3114 3115 /** 3116 * @return {@link #contains} (The codes that are contained in the value set 3117 * expansion.) 3118 */ 3119 // syntactic sugar 3120 public ValueSetExpansionContainsComponent addContains() { // 3 3121 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 3122 if (this.contains == null) 3123 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3124 this.contains.add(t); 3125 return t; 3126 } 3127 3128 // syntactic sugar 3129 public ValueSetExpansionComponent addContains(ValueSetExpansionContainsComponent t) { // 3 3130 if (t == null) 3131 return this; 3132 if (this.contains == null) 3133 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3134 this.contains.add(t); 3135 return this; 3136 } 3137 3138 protected void listChildren(List<Property> childrenList) { 3139 super.listChildren(childrenList); 3140 childrenList.add(new Property("identifier", "uri", 3141 "An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so.", 3142 0, java.lang.Integer.MAX_VALUE, identifier)); 3143 childrenList.add( 3144 new Property("timestamp", "dateTime", "The time at which the expansion was produced by the expanding system.", 3145 0, java.lang.Integer.MAX_VALUE, timestamp)); 3146 childrenList.add(new Property("total", "integer", 3147 "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 3148 0, java.lang.Integer.MAX_VALUE, total)); 3149 childrenList.add(new Property("offset", "integer", 3150 "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present.", 3151 0, java.lang.Integer.MAX_VALUE, offset)); 3152 childrenList.add(new Property("parameter", "", 3153 "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 3154 0, java.lang.Integer.MAX_VALUE, parameter)); 3155 childrenList.add(new Property("contains", "", "The codes that are contained in the value set expansion.", 0, 3156 java.lang.Integer.MAX_VALUE, contains)); 3157 } 3158 3159 @Override 3160 public void setProperty(String name, Base value) throws FHIRException { 3161 if (name.equals("identifier")) 3162 this.identifier = castToUri(value); // UriType 3163 else if (name.equals("timestamp")) 3164 this.timestamp = castToDateTime(value); // DateTimeType 3165 else if (name.equals("total")) 3166 this.total = castToInteger(value); // IntegerType 3167 else if (name.equals("offset")) 3168 this.offset = castToInteger(value); // IntegerType 3169 else if (name.equals("parameter")) 3170 this.getParameter().add((ValueSetExpansionParameterComponent) value); 3171 else if (name.equals("contains")) 3172 this.getContains().add((ValueSetExpansionContainsComponent) value); 3173 else 3174 super.setProperty(name, value); 3175 } 3176 3177 @Override 3178 public Base addChild(String name) throws FHIRException { 3179 if (name.equals("identifier")) { 3180 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.identifier"); 3181 } else if (name.equals("timestamp")) { 3182 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.timestamp"); 3183 } else if (name.equals("total")) { 3184 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.total"); 3185 } else if (name.equals("offset")) { 3186 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.offset"); 3187 } else if (name.equals("parameter")) { 3188 return addParameter(); 3189 } else if (name.equals("contains")) { 3190 return addContains(); 3191 } else 3192 return super.addChild(name); 3193 } 3194 3195 public ValueSetExpansionComponent copy() { 3196 ValueSetExpansionComponent dst = new ValueSetExpansionComponent(); 3197 copyValues(dst); 3198 dst.identifier = identifier == null ? null : identifier.copy(); 3199 dst.timestamp = timestamp == null ? null : timestamp.copy(); 3200 dst.total = total == null ? null : total.copy(); 3201 dst.offset = offset == null ? null : offset.copy(); 3202 if (parameter != null) { 3203 dst.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 3204 for (ValueSetExpansionParameterComponent i : parameter) 3205 dst.parameter.add(i.copy()); 3206 } 3207 ; 3208 if (contains != null) { 3209 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3210 for (ValueSetExpansionContainsComponent i : contains) 3211 dst.contains.add(i.copy()); 3212 } 3213 ; 3214 return dst; 3215 } 3216 3217 @Override 3218 public boolean equalsDeep(Base other) { 3219 if (!super.equalsDeep(other)) 3220 return false; 3221 if (!(other instanceof ValueSetExpansionComponent)) 3222 return false; 3223 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other; 3224 return compareDeep(identifier, o.identifier, true) && compareDeep(timestamp, o.timestamp, true) 3225 && compareDeep(total, o.total, true) && compareDeep(offset, o.offset, true) 3226 && compareDeep(parameter, o.parameter, true) && compareDeep(contains, o.contains, true); 3227 } 3228 3229 @Override 3230 public boolean equalsShallow(Base other) { 3231 if (!super.equalsShallow(other)) 3232 return false; 3233 if (!(other instanceof ValueSetExpansionComponent)) 3234 return false; 3235 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other; 3236 return compareValues(identifier, o.identifier, true) && compareValues(timestamp, o.timestamp, true) 3237 && compareValues(total, o.total, true) && compareValues(offset, o.offset, true); 3238 } 3239 3240 public boolean isEmpty() { 3241 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 3242 && (timestamp == null || timestamp.isEmpty()) && (total == null || total.isEmpty()) 3243 && (offset == null || offset.isEmpty()) && (parameter == null || parameter.isEmpty()) 3244 && (contains == null || contains.isEmpty()); 3245 } 3246 3247 public String fhirType() { 3248 return "ValueSet.expansion"; 3249 3250 } 3251 3252 } 3253 3254 @Block() 3255 public static class ValueSetExpansionParameterComponent extends BackboneElement implements IBaseBackboneElement { 3256 /** 3257 * The name of the parameter. 3258 */ 3259 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3260 @Description(shortDefinition = "Name as assigned by the server", formalDefinition = "The name of the parameter.") 3261 protected StringType name; 3262 3263 /** 3264 * The value of the parameter. 3265 */ 3266 @Child(name = "value", type = { StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, 3267 UriType.class, CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3268 @Description(shortDefinition = "Value of the named parameter", formalDefinition = "The value of the parameter.") 3269 protected Type value; 3270 3271 private static final long serialVersionUID = 1172641169L; 3272 3273 /* 3274 * Constructor 3275 */ 3276 public ValueSetExpansionParameterComponent() { 3277 super(); 3278 } 3279 3280 /* 3281 * Constructor 3282 */ 3283 public ValueSetExpansionParameterComponent(StringType name) { 3284 super(); 3285 this.name = name; 3286 } 3287 3288 /** 3289 * @return {@link #name} (The name of the parameter.). This is the underlying 3290 * object with id, value and extensions. The accessor "getName" gives 3291 * direct access to the value 3292 */ 3293 public StringType getNameElement() { 3294 if (this.name == null) 3295 if (Configuration.errorOnAutoCreate()) 3296 throw new Error("Attempt to auto-create ValueSetExpansionParameterComponent.name"); 3297 else if (Configuration.doAutoCreate()) 3298 this.name = new StringType(); // bb 3299 return this.name; 3300 } 3301 3302 public boolean hasNameElement() { 3303 return this.name != null && !this.name.isEmpty(); 3304 } 3305 3306 public boolean hasName() { 3307 return this.name != null && !this.name.isEmpty(); 3308 } 3309 3310 /** 3311 * @param value {@link #name} (The name of the parameter.). This is the 3312 * underlying object with id, value and extensions. The accessor 3313 * "getName" gives direct access to the value 3314 */ 3315 public ValueSetExpansionParameterComponent setNameElement(StringType value) { 3316 this.name = value; 3317 return this; 3318 } 3319 3320 /** 3321 * @return The name of the parameter. 3322 */ 3323 public String getName() { 3324 return this.name == null ? null : this.name.getValue(); 3325 } 3326 3327 /** 3328 * @param value The name of the parameter. 3329 */ 3330 public ValueSetExpansionParameterComponent setName(String value) { 3331 if (this.name == null) 3332 this.name = new StringType(); 3333 this.name.setValue(value); 3334 return this; 3335 } 3336 3337 /** 3338 * @return {@link #value} (The value of the parameter.) 3339 */ 3340 public Type getValue() { 3341 return this.value; 3342 } 3343 3344 /** 3345 * @return {@link #value} (The value of the parameter.) 3346 */ 3347 public StringType getValueStringType() throws FHIRException { 3348 if (!(this.value instanceof StringType)) 3349 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3350 + this.value.getClass().getName() + " was encountered"); 3351 return (StringType) this.value; 3352 } 3353 3354 public boolean hasValueStringType() { 3355 return this.value instanceof StringType; 3356 } 3357 3358 /** 3359 * @return {@link #value} (The value of the parameter.) 3360 */ 3361 public BooleanType getValueBooleanType() throws FHIRException { 3362 if (!(this.value instanceof BooleanType)) 3363 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 3364 + this.value.getClass().getName() + " was encountered"); 3365 return (BooleanType) this.value; 3366 } 3367 3368 public boolean hasValueBooleanType() { 3369 return this.value instanceof BooleanType; 3370 } 3371 3372 /** 3373 * @return {@link #value} (The value of the parameter.) 3374 */ 3375 public IntegerType getValueIntegerType() throws FHIRException { 3376 if (!(this.value instanceof IntegerType)) 3377 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 3378 + this.value.getClass().getName() + " was encountered"); 3379 return (IntegerType) this.value; 3380 } 3381 3382 public boolean hasValueIntegerType() { 3383 return this.value instanceof IntegerType; 3384 } 3385 3386 /** 3387 * @return {@link #value} (The value of the parameter.) 3388 */ 3389 public DecimalType getValueDecimalType() throws FHIRException { 3390 if (!(this.value instanceof DecimalType)) 3391 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 3392 + this.value.getClass().getName() + " was encountered"); 3393 return (DecimalType) this.value; 3394 } 3395 3396 public boolean hasValueDecimalType() { 3397 return this.value instanceof DecimalType; 3398 } 3399 3400 /** 3401 * @return {@link #value} (The value of the parameter.) 3402 */ 3403 public UriType getValueUriType() throws FHIRException { 3404 if (!(this.value instanceof UriType)) 3405 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 3406 + " was encountered"); 3407 return (UriType) this.value; 3408 } 3409 3410 public boolean hasValueUriType() { 3411 return this.value instanceof UriType; 3412 } 3413 3414 /** 3415 * @return {@link #value} (The value of the parameter.) 3416 */ 3417 public CodeType getValueCodeType() throws FHIRException { 3418 if (!(this.value instanceof CodeType)) 3419 throw new FHIRException("Type mismatch: the type CodeType was expected, but " + this.value.getClass().getName() 3420 + " was encountered"); 3421 return (CodeType) this.value; 3422 } 3423 3424 public boolean hasValueCodeType() { 3425 return this.value instanceof CodeType; 3426 } 3427 3428 public boolean hasValue() { 3429 return this.value != null && !this.value.isEmpty(); 3430 } 3431 3432 /** 3433 * @param value {@link #value} (The value of the parameter.) 3434 */ 3435 public ValueSetExpansionParameterComponent setValue(Type value) { 3436 this.value = value; 3437 return this; 3438 } 3439 3440 protected void listChildren(List<Property> childrenList) { 3441 super.listChildren(childrenList); 3442 childrenList 3443 .add(new Property("name", "string", "The name of the parameter.", 0, java.lang.Integer.MAX_VALUE, name)); 3444 childrenList.add(new Property("value[x]", "string|boolean|integer|decimal|uri|code", 3445 "The value of the parameter.", 0, java.lang.Integer.MAX_VALUE, value)); 3446 } 3447 3448 @Override 3449 public void setProperty(String name, Base value) throws FHIRException { 3450 if (name.equals("name")) 3451 this.name = castToString(value); // StringType 3452 else if (name.equals("value[x]")) 3453 this.value = (Type) value; // Type 3454 else 3455 super.setProperty(name, value); 3456 } 3457 3458 @Override 3459 public Base addChild(String name) throws FHIRException { 3460 if (name.equals("name")) { 3461 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 3462 } else if (name.equals("valueString")) { 3463 this.value = new StringType(); 3464 return this.value; 3465 } else if (name.equals("valueBoolean")) { 3466 this.value = new BooleanType(); 3467 return this.value; 3468 } else if (name.equals("valueInteger")) { 3469 this.value = new IntegerType(); 3470 return this.value; 3471 } else if (name.equals("valueDecimal")) { 3472 this.value = new DecimalType(); 3473 return this.value; 3474 } else if (name.equals("valueUri")) { 3475 this.value = new UriType(); 3476 return this.value; 3477 } else if (name.equals("valueCode")) { 3478 this.value = new CodeType(); 3479 return this.value; 3480 } else 3481 return super.addChild(name); 3482 } 3483 3484 public ValueSetExpansionParameterComponent copy() { 3485 ValueSetExpansionParameterComponent dst = new ValueSetExpansionParameterComponent(); 3486 copyValues(dst); 3487 dst.name = name == null ? null : name.copy(); 3488 dst.value = value == null ? null : value.copy(); 3489 return dst; 3490 } 3491 3492 @Override 3493 public boolean equalsDeep(Base other) { 3494 if (!super.equalsDeep(other)) 3495 return false; 3496 if (!(other instanceof ValueSetExpansionParameterComponent)) 3497 return false; 3498 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other; 3499 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 3500 } 3501 3502 @Override 3503 public boolean equalsShallow(Base other) { 3504 if (!super.equalsShallow(other)) 3505 return false; 3506 if (!(other instanceof ValueSetExpansionParameterComponent)) 3507 return false; 3508 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other; 3509 return compareValues(name, o.name, true); 3510 } 3511 3512 public boolean isEmpty() { 3513 return super.isEmpty() && (name == null || name.isEmpty()) && (value == null || value.isEmpty()); 3514 } 3515 3516 public String fhirType() { 3517 return "ValueSet.expansion.parameter"; 3518 3519 } 3520 3521 } 3522 3523 @Block() 3524 public static class ValueSetExpansionContainsComponent extends BackboneElement implements IBaseBackboneElement { 3525 /** 3526 * An absolute URI which is the code system in which the code for this item in 3527 * the expansion is defined. 3528 */ 3529 @Child(name = "system", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3530 @Description(shortDefinition = "System value for the code", formalDefinition = "An absolute URI which is the code system in which the code for this item in the expansion is defined.") 3531 protected UriType system; 3532 3533 /** 3534 * If true, this entry is included in the expansion for navigational purposes, 3535 * and the user cannot select the code directly as a proper value. 3536 */ 3537 @Child(name = "abstract", type = { 3538 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3539 @Description(shortDefinition = "If user cannot select this entry", formalDefinition = "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.") 3540 protected BooleanType abstract_; 3541 3542 /** 3543 * The version of this code system that defined this code and/or display. This 3544 * should only be used with code systems that do not enforce concept permanence. 3545 */ 3546 @Child(name = "version", type = { 3547 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3548 @Description(shortDefinition = "Version in which this code/display is defined", formalDefinition = "The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence.") 3549 protected StringType version; 3550 3551 /** 3552 * The code for this item in the expansion hierarchy. If this code is missing 3553 * the entry in the hierarchy is a place holder (abstract) and does not 3554 * represent a valid code in the value set. 3555 */ 3556 @Child(name = "code", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3557 @Description(shortDefinition = "Code - if blank, this is not a selectable code", formalDefinition = "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.") 3558 protected CodeType code; 3559 3560 /** 3561 * The recommended display for this item in the expansion. 3562 */ 3563 @Child(name = "display", type = { 3564 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3565 @Description(shortDefinition = "User display for the concept", formalDefinition = "The recommended display for this item in the expansion.") 3566 protected StringType display; 3567 3568 /** 3569 * Other codes and entries contained under this entry in the hierarchy. 3570 */ 3571 @Child(name = "contains", type = { 3572 ValueSetExpansionContainsComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3573 @Description(shortDefinition = "Codes contained under this entry", formalDefinition = "Other codes and entries contained under this entry in the hierarchy.") 3574 protected List<ValueSetExpansionContainsComponent> contains; 3575 3576 private static final long serialVersionUID = -2038349483L; 3577 3578 /* 3579 * Constructor 3580 */ 3581 public ValueSetExpansionContainsComponent() { 3582 super(); 3583 } 3584 3585 /** 3586 * @return {@link #system} (An absolute URI which is the code system in which 3587 * the code for this item in the expansion is defined.). This is the 3588 * underlying object with id, value and extensions. The accessor 3589 * "getSystem" gives direct access to the value 3590 */ 3591 public UriType getSystemElement() { 3592 if (this.system == null) 3593 if (Configuration.errorOnAutoCreate()) 3594 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.system"); 3595 else if (Configuration.doAutoCreate()) 3596 this.system = new UriType(); // bb 3597 return this.system; 3598 } 3599 3600 public boolean hasSystemElement() { 3601 return this.system != null && !this.system.isEmpty(); 3602 } 3603 3604 public boolean hasSystem() { 3605 return this.system != null && !this.system.isEmpty(); 3606 } 3607 3608 /** 3609 * @param value {@link #system} (An absolute URI which is the code system in 3610 * which the code for this item in the expansion is defined.). This 3611 * is the underlying object with id, value and extensions. The 3612 * accessor "getSystem" gives direct access to the value 3613 */ 3614 public ValueSetExpansionContainsComponent setSystemElement(UriType value) { 3615 this.system = value; 3616 return this; 3617 } 3618 3619 /** 3620 * @return An absolute URI which is the code system in which the code for this 3621 * item in the expansion is defined. 3622 */ 3623 public String getSystem() { 3624 return this.system == null ? null : this.system.getValue(); 3625 } 3626 3627 /** 3628 * @param value An absolute URI which is the code system in which the code for 3629 * this item in the expansion is defined. 3630 */ 3631 public ValueSetExpansionContainsComponent setSystem(String value) { 3632 if (Utilities.noString(value)) 3633 this.system = null; 3634 else { 3635 if (this.system == null) 3636 this.system = new UriType(); 3637 this.system.setValue(value); 3638 } 3639 return this; 3640 } 3641 3642 /** 3643 * @return {@link #abstract_} (If true, this entry is included in the expansion 3644 * for navigational purposes, and the user cannot select the code 3645 * directly as a proper value.). This is the underlying object with id, 3646 * value and extensions. The accessor "getAbstract" gives direct access 3647 * to the value 3648 */ 3649 public BooleanType getAbstractElement() { 3650 if (this.abstract_ == null) 3651 if (Configuration.errorOnAutoCreate()) 3652 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.abstract_"); 3653 else if (Configuration.doAutoCreate()) 3654 this.abstract_ = new BooleanType(); // bb 3655 return this.abstract_; 3656 } 3657 3658 public boolean hasAbstractElement() { 3659 return this.abstract_ != null && !this.abstract_.isEmpty(); 3660 } 3661 3662 public boolean hasAbstract() { 3663 return this.abstract_ != null && !this.abstract_.isEmpty(); 3664 } 3665 3666 /** 3667 * @param value {@link #abstract_} (If true, this entry is included in the 3668 * expansion for navigational purposes, and the user cannot select 3669 * the code directly as a proper value.). This is the underlying 3670 * object with id, value and extensions. The accessor "getAbstract" 3671 * gives direct access to the value 3672 */ 3673 public ValueSetExpansionContainsComponent setAbstractElement(BooleanType value) { 3674 this.abstract_ = value; 3675 return this; 3676 } 3677 3678 /** 3679 * @return If true, this entry is included in the expansion for navigational 3680 * purposes, and the user cannot select the code directly as a proper 3681 * value. 3682 */ 3683 public boolean getAbstract() { 3684 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 3685 } 3686 3687 /** 3688 * @param value If true, this entry is included in the expansion for 3689 * navigational purposes, and the user cannot select the code 3690 * directly as a proper value. 3691 */ 3692 public ValueSetExpansionContainsComponent setAbstract(boolean value) { 3693 if (this.abstract_ == null) 3694 this.abstract_ = new BooleanType(); 3695 this.abstract_.setValue(value); 3696 return this; 3697 } 3698 3699 /** 3700 * @return {@link #version} (The version of this code system that defined this 3701 * code and/or display. This should only be used with code systems that 3702 * do not enforce concept permanence.). This is the underlying object 3703 * with id, value and extensions. The accessor "getVersion" gives direct 3704 * access to the value 3705 */ 3706 public StringType getVersionElement() { 3707 if (this.version == null) 3708 if (Configuration.errorOnAutoCreate()) 3709 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.version"); 3710 else if (Configuration.doAutoCreate()) 3711 this.version = new StringType(); // bb 3712 return this.version; 3713 } 3714 3715 public boolean hasVersionElement() { 3716 return this.version != null && !this.version.isEmpty(); 3717 } 3718 3719 public boolean hasVersion() { 3720 return this.version != null && !this.version.isEmpty(); 3721 } 3722 3723 /** 3724 * @param value {@link #version} (The version of this code system that defined 3725 * this code and/or display. This should only be used with code 3726 * systems that do not enforce concept permanence.). This is the 3727 * underlying object with id, value and extensions. The accessor 3728 * "getVersion" gives direct access to the value 3729 */ 3730 public ValueSetExpansionContainsComponent setVersionElement(StringType value) { 3731 this.version = value; 3732 return this; 3733 } 3734 3735 /** 3736 * @return The version of this code system that defined this code and/or 3737 * display. This should only be used with code systems that do not 3738 * enforce concept permanence. 3739 */ 3740 public String getVersion() { 3741 return this.version == null ? null : this.version.getValue(); 3742 } 3743 3744 /** 3745 * @param value The version of this code system that defined this code and/or 3746 * display. This should only be used with code systems that do not 3747 * enforce concept permanence. 3748 */ 3749 public ValueSetExpansionContainsComponent setVersion(String value) { 3750 if (Utilities.noString(value)) 3751 this.version = null; 3752 else { 3753 if (this.version == null) 3754 this.version = new StringType(); 3755 this.version.setValue(value); 3756 } 3757 return this; 3758 } 3759 3760 /** 3761 * @return {@link #code} (The code for this item in the expansion hierarchy. If 3762 * this code is missing the entry in the hierarchy is a place holder 3763 * (abstract) and does not represent a valid code in the value set.). 3764 * This is the underlying object with id, value and extensions. The 3765 * accessor "getCode" gives direct access to the value 3766 */ 3767 public CodeType getCodeElement() { 3768 if (this.code == null) 3769 if (Configuration.errorOnAutoCreate()) 3770 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.code"); 3771 else if (Configuration.doAutoCreate()) 3772 this.code = new CodeType(); // bb 3773 return this.code; 3774 } 3775 3776 public boolean hasCodeElement() { 3777 return this.code != null && !this.code.isEmpty(); 3778 } 3779 3780 public boolean hasCode() { 3781 return this.code != null && !this.code.isEmpty(); 3782 } 3783 3784 /** 3785 * @param value {@link #code} (The code for this item in the expansion 3786 * hierarchy. If this code is missing the entry in the hierarchy is 3787 * a place holder (abstract) and does not represent a valid code in 3788 * the value set.). This is the underlying object with id, value 3789 * and extensions. The accessor "getCode" gives direct access to 3790 * the value 3791 */ 3792 public ValueSetExpansionContainsComponent setCodeElement(CodeType value) { 3793 this.code = value; 3794 return this; 3795 } 3796 3797 /** 3798 * @return The code for this item in the expansion hierarchy. If this code is 3799 * missing the entry in the hierarchy is a place holder (abstract) and 3800 * does not represent a valid code in the value set. 3801 */ 3802 public String getCode() { 3803 return this.code == null ? null : this.code.getValue(); 3804 } 3805 3806 /** 3807 * @param value The code for this item in the expansion hierarchy. If this code 3808 * is missing the entry in the hierarchy is a place holder 3809 * (abstract) and does not represent a valid code in the value set. 3810 */ 3811 public ValueSetExpansionContainsComponent setCode(String value) { 3812 if (Utilities.noString(value)) 3813 this.code = null; 3814 else { 3815 if (this.code == null) 3816 this.code = new CodeType(); 3817 this.code.setValue(value); 3818 } 3819 return this; 3820 } 3821 3822 /** 3823 * @return {@link #display} (The recommended display for this item in the 3824 * expansion.). This is the underlying object with id, value and 3825 * extensions. The accessor "getDisplay" gives direct access to the 3826 * value 3827 */ 3828 public StringType getDisplayElement() { 3829 if (this.display == null) 3830 if (Configuration.errorOnAutoCreate()) 3831 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.display"); 3832 else if (Configuration.doAutoCreate()) 3833 this.display = new StringType(); // bb 3834 return this.display; 3835 } 3836 3837 public boolean hasDisplayElement() { 3838 return this.display != null && !this.display.isEmpty(); 3839 } 3840 3841 public boolean hasDisplay() { 3842 return this.display != null && !this.display.isEmpty(); 3843 } 3844 3845 /** 3846 * @param value {@link #display} (The recommended display for this item in the 3847 * expansion.). This is the underlying object with id, value and 3848 * extensions. The accessor "getDisplay" gives direct access to the 3849 * value 3850 */ 3851 public ValueSetExpansionContainsComponent setDisplayElement(StringType value) { 3852 this.display = value; 3853 return this; 3854 } 3855 3856 /** 3857 * @return The recommended display for this item in the expansion. 3858 */ 3859 public String getDisplay() { 3860 return this.display == null ? null : this.display.getValue(); 3861 } 3862 3863 /** 3864 * @param value The recommended display for this item in the expansion. 3865 */ 3866 public ValueSetExpansionContainsComponent setDisplay(String value) { 3867 if (Utilities.noString(value)) 3868 this.display = null; 3869 else { 3870 if (this.display == null) 3871 this.display = new StringType(); 3872 this.display.setValue(value); 3873 } 3874 return this; 3875 } 3876 3877 /** 3878 * @return {@link #contains} (Other codes and entries contained under this entry 3879 * in the hierarchy.) 3880 */ 3881 public List<ValueSetExpansionContainsComponent> getContains() { 3882 if (this.contains == null) 3883 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3884 return this.contains; 3885 } 3886 3887 public boolean hasContains() { 3888 if (this.contains == null) 3889 return false; 3890 for (ValueSetExpansionContainsComponent item : this.contains) 3891 if (!item.isEmpty()) 3892 return true; 3893 return false; 3894 } 3895 3896 /** 3897 * @return {@link #contains} (Other codes and entries contained under this entry 3898 * in the hierarchy.) 3899 */ 3900 // syntactic sugar 3901 public ValueSetExpansionContainsComponent addContains() { // 3 3902 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 3903 if (this.contains == null) 3904 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3905 this.contains.add(t); 3906 return t; 3907 } 3908 3909 // syntactic sugar 3910 public ValueSetExpansionContainsComponent addContains(ValueSetExpansionContainsComponent t) { // 3 3911 if (t == null) 3912 return this; 3913 if (this.contains == null) 3914 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3915 this.contains.add(t); 3916 return this; 3917 } 3918 3919 protected void listChildren(List<Property> childrenList) { 3920 super.listChildren(childrenList); 3921 childrenList.add(new Property("system", "uri", 3922 "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 3923 java.lang.Integer.MAX_VALUE, system)); 3924 childrenList.add(new Property("abstract", "boolean", 3925 "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 3926 0, java.lang.Integer.MAX_VALUE, abstract_)); 3927 childrenList.add(new Property("version", "string", 3928 "The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence.", 3929 0, java.lang.Integer.MAX_VALUE, version)); 3930 childrenList.add(new Property("code", "code", 3931 "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 3932 0, java.lang.Integer.MAX_VALUE, code)); 3933 childrenList.add(new Property("display", "string", "The recommended display for this item in the expansion.", 0, 3934 java.lang.Integer.MAX_VALUE, display)); 3935 childrenList.add(new Property("contains", "@ValueSet.expansion.contains", 3936 "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, 3937 contains)); 3938 } 3939 3940 @Override 3941 public void setProperty(String name, Base value) throws FHIRException { 3942 if (name.equals("system")) 3943 this.system = castToUri(value); // UriType 3944 else if (name.equals("abstract")) 3945 this.abstract_ = castToBoolean(value); // BooleanType 3946 else if (name.equals("version")) 3947 this.version = castToString(value); // StringType 3948 else if (name.equals("code")) 3949 this.code = castToCode(value); // CodeType 3950 else if (name.equals("display")) 3951 this.display = castToString(value); // StringType 3952 else if (name.equals("contains")) 3953 this.getContains().add((ValueSetExpansionContainsComponent) value); 3954 else 3955 super.setProperty(name, value); 3956 } 3957 3958 @Override 3959 public Base addChild(String name) throws FHIRException { 3960 if (name.equals("system")) { 3961 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 3962 } else if (name.equals("abstract")) { 3963 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.abstract"); 3964 } else if (name.equals("version")) { 3965 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 3966 } else if (name.equals("code")) { 3967 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 3968 } else if (name.equals("display")) { 3969 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 3970 } else if (name.equals("contains")) { 3971 return addContains(); 3972 } else 3973 return super.addChild(name); 3974 } 3975 3976 public ValueSetExpansionContainsComponent copy() { 3977 ValueSetExpansionContainsComponent dst = new ValueSetExpansionContainsComponent(); 3978 copyValues(dst); 3979 dst.system = system == null ? null : system.copy(); 3980 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 3981 dst.version = version == null ? null : version.copy(); 3982 dst.code = code == null ? null : code.copy(); 3983 dst.display = display == null ? null : display.copy(); 3984 if (contains != null) { 3985 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3986 for (ValueSetExpansionContainsComponent i : contains) 3987 dst.contains.add(i.copy()); 3988 } 3989 ; 3990 return dst; 3991 } 3992 3993 @Override 3994 public boolean equalsDeep(Base other) { 3995 if (!super.equalsDeep(other)) 3996 return false; 3997 if (!(other instanceof ValueSetExpansionContainsComponent)) 3998 return false; 3999 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other; 4000 return compareDeep(system, o.system, true) && compareDeep(abstract_, o.abstract_, true) 4001 && compareDeep(version, o.version, true) && compareDeep(code, o.code, true) 4002 && compareDeep(display, o.display, true) && compareDeep(contains, o.contains, true); 4003 } 4004 4005 @Override 4006 public boolean equalsShallow(Base other) { 4007 if (!super.equalsShallow(other)) 4008 return false; 4009 if (!(other instanceof ValueSetExpansionContainsComponent)) 4010 return false; 4011 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other; 4012 return compareValues(system, o.system, true) && compareValues(abstract_, o.abstract_, true) 4013 && compareValues(version, o.version, true) && compareValues(code, o.code, true) 4014 && compareValues(display, o.display, true); 4015 } 4016 4017 public boolean isEmpty() { 4018 return super.isEmpty() && (system == null || system.isEmpty()) && (abstract_ == null || abstract_.isEmpty()) 4019 && (version == null || version.isEmpty()) && (code == null || code.isEmpty()) 4020 && (display == null || display.isEmpty()) && (contains == null || contains.isEmpty()); 4021 } 4022 4023 public String fhirType() { 4024 return "ValueSet.expansion.contains"; 4025 4026 } 4027 4028 } 4029 4030 /** 4031 * An absolute URL that is used to identify this value set when it is referenced 4032 * in a specification, model, design or an instance. This SHALL be a URL, SHOULD 4033 * be globally unique, and SHOULD be an address at which this value set is (or 4034 * will be) published. 4035 */ 4036 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 4037 @Description(shortDefinition = "Globally unique logical identifier for value set", formalDefinition = "An absolute URL that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published.") 4038 protected UriType url; 4039 4040 /** 4041 * Formal identifier that is used to identify this value set when it is 4042 * represented in other formats, or referenced in a specification, model, design 4043 * or an instance. 4044 */ 4045 @Child(name = "identifier", type = { 4046 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 4047 @Description(shortDefinition = "Additional identifier for the value set (e.g. HL7 v2 / CDA)", formalDefinition = "Formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.") 4048 protected Identifier identifier; 4049 4050 /** 4051 * Used to identify this version of the value set when it is referenced in a 4052 * specification, model, design or instance. This is an arbitrary value managed 4053 * by the profile author manually and the value should be a timestamp. 4054 */ 4055 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4056 @Description(shortDefinition = "Logical identifier for this version of the value set", formalDefinition = "Used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.") 4057 protected StringType version; 4058 4059 /** 4060 * A free text natural language name describing the value set. 4061 */ 4062 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4063 @Description(shortDefinition = "Informal name for this value set", formalDefinition = "A free text natural language name describing the value set.") 4064 protected StringType name; 4065 4066 /** 4067 * The status of the value set. 4068 */ 4069 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 4070 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the value set.") 4071 protected Enumeration<ConformanceResourceStatus> status; 4072 4073 /** 4074 * This valueset was authored for testing purposes (or 4075 * education/evaluation/marketing), and is not intended to be used for genuine 4076 * usage. 4077 */ 4078 @Child(name = "experimental", type = { 4079 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4080 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "This valueset was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 4081 protected BooleanType experimental; 4082 4083 /** 4084 * The name of the individual or organization that published the value set. 4085 */ 4086 @Child(name = "publisher", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 4087 @Description(shortDefinition = "Name of the publisher (organization or individual)", formalDefinition = "The name of the individual or organization that published the value set.") 4088 protected StringType publisher; 4089 4090 /** 4091 * Contacts to assist a user in finding and communicating with the publisher. 4092 */ 4093 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4094 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 4095 protected List<ValueSetContactComponent> contact; 4096 4097 /** 4098 * The date that the value set status was last changed. The date must change 4099 * when the business version changes, if it does, and it must change if the 4100 * status code changes. In addition, it should change when the substantive 4101 * content of the implementation guide changes (e.g. the 'content logical 4102 * definition'). 4103 */ 4104 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 4105 @Description(shortDefinition = "Date for given status", formalDefinition = "The date that the value set status was last changed. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes (e.g. the 'content logical definition').") 4106 protected DateTimeType date; 4107 4108 /** 4109 * If a locked date is defined, then the Content Logical Definition must be 4110 * evaluated using the current version of all referenced code system(s) and 4111 * value set instances as of the locked date. 4112 */ 4113 @Child(name = "lockedDate", type = { DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 4114 @Description(shortDefinition = "Fixed date for all referenced code systems and value sets", formalDefinition = "If a locked date is defined, then the Content Logical Definition must be evaluated using the current version of all referenced code system(s) and value set instances as of the locked date.") 4115 protected DateType lockedDate; 4116 4117 /** 4118 * A free text natural language description of the use of the value set - reason 4119 * for definition, "the semantic space" to be included in the value set, 4120 * conditions of use, etc. The description may include a list of expected usages 4121 * for the value set and can also describe the approach taken to build the value 4122 * set. 4123 */ 4124 @Child(name = "description", type = { 4125 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 4126 @Description(shortDefinition = "Human language description of the value set", formalDefinition = "A free text natural language description of the use of the value set - reason for definition, \"the semantic space\" to be included in the value set, conditions of use, etc. The description may include a list of expected usages for the value set and can also describe the approach taken to build the value set.") 4127 protected StringType description; 4128 4129 /** 4130 * The content was developed with a focus and intent of supporting the contexts 4131 * that are listed. These terms may be used to assist with indexing and 4132 * searching of value set definitions. 4133 */ 4134 @Child(name = "useContext", type = { 4135 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4136 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of value set definitions.") 4137 protected List<CodeableConcept> useContext; 4138 4139 /** 4140 * If this is set to 'true', then no new versions of the content logical 4141 * definition can be created. Note: Other metadata might still change. 4142 */ 4143 @Child(name = "immutable", type = { 4144 BooleanType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 4145 @Description(shortDefinition = "Indicates whether or not any change to the content logical definition may occur", formalDefinition = "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.") 4146 protected BooleanType immutable; 4147 4148 /** 4149 * Explains why this value set is needed and why it has been constrained as it 4150 * has. 4151 */ 4152 @Child(name = "requirements", type = { 4153 StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4154 @Description(shortDefinition = "Why needed", formalDefinition = "Explains why this value set is needed and why it has been constrained as it has.") 4155 protected StringType requirements; 4156 4157 /** 4158 * A copyright statement relating to the value set and/or its contents. 4159 * Copyright statements are generally legal restrictions on the use and 4160 * publishing of the value set. 4161 */ 4162 @Child(name = "copyright", type = { 4163 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 4164 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.") 4165 protected StringType copyright; 4166 4167 /** 4168 * Whether this is intended to be used with an extensible binding or not. 4169 */ 4170 @Child(name = "extensible", type = { 4171 BooleanType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 4172 @Description(shortDefinition = "Whether this is intended to be used with an extensible binding", formalDefinition = "Whether this is intended to be used with an extensible binding or not.") 4173 protected BooleanType extensible; 4174 4175 /** 4176 * A definition of a code system, inlined into the value set (as a packaging 4177 * convenience). Note that the inline code system may be used from other value 4178 * sets by referring to its (codeSystem.system) directly. 4179 */ 4180 @Child(name = "codeSystem", type = {}, order = 16, min = 0, max = 1, modifier = false, summary = true) 4181 @Description(shortDefinition = "An inline code system, which is part of this value set", formalDefinition = "A definition of a code system, inlined into the value set (as a packaging convenience). Note that the inline code system may be used from other value sets by referring to its (codeSystem.system) directly.") 4182 protected ValueSetCodeSystemComponent codeSystem; 4183 4184 /** 4185 * A set of criteria that provide the content logical definition of the value 4186 * set by including or excluding codes from outside this value set. 4187 */ 4188 @Child(name = "compose", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = false) 4189 @Description(shortDefinition = "When value set includes codes from elsewhere", formalDefinition = "A set of criteria that provide the content logical definition of the value set by including or excluding codes from outside this value set.") 4190 protected ValueSetComposeComponent compose; 4191 4192 /** 4193 * A value set can also be "expanded", where the value set is turned into a 4194 * simple collection of enumerated codes. This element holds the expansion, if 4195 * it has been performed. 4196 */ 4197 @Child(name = "expansion", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = false) 4198 @Description(shortDefinition = "Used when the value set is \"expanded\"", formalDefinition = "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.") 4199 protected ValueSetExpansionComponent expansion; 4200 4201 private static final long serialVersionUID = -467533312L; 4202 4203 /* 4204 * Constructor 4205 */ 4206 public ValueSet() { 4207 super(); 4208 } 4209 4210 /* 4211 * Constructor 4212 */ 4213 public ValueSet(Enumeration<ConformanceResourceStatus> status) { 4214 super(); 4215 this.status = status; 4216 } 4217 4218 /** 4219 * @return {@link #url} (An absolute URL that is used to identify this value set 4220 * when it is referenced in a specification, model, design or an 4221 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 4222 * be an address at which this value set is (or will be) published.). 4223 * This is the underlying object with id, value and extensions. The 4224 * accessor "getUrl" gives direct access to the value 4225 */ 4226 public UriType getUrlElement() { 4227 if (this.url == null) 4228 if (Configuration.errorOnAutoCreate()) 4229 throw new Error("Attempt to auto-create ValueSet.url"); 4230 else if (Configuration.doAutoCreate()) 4231 this.url = new UriType(); // bb 4232 return this.url; 4233 } 4234 4235 public boolean hasUrlElement() { 4236 return this.url != null && !this.url.isEmpty(); 4237 } 4238 4239 public boolean hasUrl() { 4240 return this.url != null && !this.url.isEmpty(); 4241 } 4242 4243 /** 4244 * @param value {@link #url} (An absolute URL that is used to identify this 4245 * value set when it is referenced in a specification, model, 4246 * design or an instance. This SHALL be a URL, SHOULD be globally 4247 * unique, and SHOULD be an address at which this value set is (or 4248 * will be) published.). This is the underlying object with id, 4249 * value and extensions. The accessor "getUrl" gives direct access 4250 * to the value 4251 */ 4252 public ValueSet setUrlElement(UriType value) { 4253 this.url = value; 4254 return this; 4255 } 4256 4257 /** 4258 * @return An absolute URL that is used to identify this value set when it is 4259 * referenced in a specification, model, design or an instance. This 4260 * SHALL be a URL, SHOULD be globally unique, and SHOULD be an address 4261 * at which this value set is (or will be) published. 4262 */ 4263 public String getUrl() { 4264 return this.url == null ? null : this.url.getValue(); 4265 } 4266 4267 /** 4268 * @param value An absolute URL that is used to identify this value set when it 4269 * is referenced in a specification, model, design or an instance. 4270 * This SHALL be a URL, SHOULD be globally unique, and SHOULD be an 4271 * address at which this value set is (or will be) published. 4272 */ 4273 public ValueSet setUrl(String value) { 4274 if (Utilities.noString(value)) 4275 this.url = null; 4276 else { 4277 if (this.url == null) 4278 this.url = new UriType(); 4279 this.url.setValue(value); 4280 } 4281 return this; 4282 } 4283 4284 /** 4285 * @return {@link #identifier} (Formal identifier that is used to identify this 4286 * value set when it is represented in other formats, or referenced in a 4287 * specification, model, design or an instance.) 4288 */ 4289 public Identifier getIdentifier() { 4290 if (this.identifier == null) 4291 if (Configuration.errorOnAutoCreate()) 4292 throw new Error("Attempt to auto-create ValueSet.identifier"); 4293 else if (Configuration.doAutoCreate()) 4294 this.identifier = new Identifier(); // cc 4295 return this.identifier; 4296 } 4297 4298 public boolean hasIdentifier() { 4299 return this.identifier != null && !this.identifier.isEmpty(); 4300 } 4301 4302 /** 4303 * @param value {@link #identifier} (Formal identifier that is used to identify 4304 * this value set when it is represented in other formats, or 4305 * referenced in a specification, model, design or an instance.) 4306 */ 4307 public ValueSet setIdentifier(Identifier value) { 4308 this.identifier = value; 4309 return this; 4310 } 4311 4312 /** 4313 * @return {@link #version} (Used to identify this version of the value set when 4314 * it is referenced in a specification, model, design or instance. This 4315 * is an arbitrary value managed by the profile author manually and the 4316 * value should be a timestamp.). This is the underlying object with id, 4317 * value and extensions. The accessor "getVersion" gives direct access 4318 * to the value 4319 */ 4320 public StringType getVersionElement() { 4321 if (this.version == null) 4322 if (Configuration.errorOnAutoCreate()) 4323 throw new Error("Attempt to auto-create ValueSet.version"); 4324 else if (Configuration.doAutoCreate()) 4325 this.version = new StringType(); // bb 4326 return this.version; 4327 } 4328 4329 public boolean hasVersionElement() { 4330 return this.version != null && !this.version.isEmpty(); 4331 } 4332 4333 public boolean hasVersion() { 4334 return this.version != null && !this.version.isEmpty(); 4335 } 4336 4337 /** 4338 * @param value {@link #version} (Used to identify this version of the value set 4339 * when it is referenced in a specification, model, design or 4340 * instance. This is an arbitrary value managed by the profile 4341 * author manually and the value should be a timestamp.). This is 4342 * the underlying object with id, value and extensions. The 4343 * accessor "getVersion" gives direct access to the value 4344 */ 4345 public ValueSet setVersionElement(StringType value) { 4346 this.version = value; 4347 return this; 4348 } 4349 4350 /** 4351 * @return Used to identify this version of the value set when it is referenced 4352 * in a specification, model, design or instance. This is an arbitrary 4353 * value managed by the profile author manually and the value should be 4354 * a timestamp. 4355 */ 4356 public String getVersion() { 4357 return this.version == null ? null : this.version.getValue(); 4358 } 4359 4360 /** 4361 * @param value Used to identify this version of the value set when it is 4362 * referenced in a specification, model, design or instance. This 4363 * is an arbitrary value managed by the profile author manually and 4364 * the value should be a timestamp. 4365 */ 4366 public ValueSet setVersion(String value) { 4367 if (Utilities.noString(value)) 4368 this.version = null; 4369 else { 4370 if (this.version == null) 4371 this.version = new StringType(); 4372 this.version.setValue(value); 4373 } 4374 return this; 4375 } 4376 4377 /** 4378 * @return {@link #name} (A free text natural language name describing the value 4379 * set.). This is the underlying object with id, value and extensions. 4380 * The accessor "getName" gives direct access to the value 4381 */ 4382 public StringType getNameElement() { 4383 if (this.name == null) 4384 if (Configuration.errorOnAutoCreate()) 4385 throw new Error("Attempt to auto-create ValueSet.name"); 4386 else if (Configuration.doAutoCreate()) 4387 this.name = new StringType(); // bb 4388 return this.name; 4389 } 4390 4391 public boolean hasNameElement() { 4392 return this.name != null && !this.name.isEmpty(); 4393 } 4394 4395 public boolean hasName() { 4396 return this.name != null && !this.name.isEmpty(); 4397 } 4398 4399 /** 4400 * @param value {@link #name} (A free text natural language name describing the 4401 * value set.). This is the underlying object with id, value and 4402 * extensions. The accessor "getName" gives direct access to the 4403 * value 4404 */ 4405 public ValueSet setNameElement(StringType value) { 4406 this.name = value; 4407 return this; 4408 } 4409 4410 /** 4411 * @return A free text natural language name describing the value set. 4412 */ 4413 public String getName() { 4414 return this.name == null ? null : this.name.getValue(); 4415 } 4416 4417 /** 4418 * @param value A free text natural language name describing the value set. 4419 */ 4420 public ValueSet setName(String value) { 4421 if (Utilities.noString(value)) 4422 this.name = null; 4423 else { 4424 if (this.name == null) 4425 this.name = new StringType(); 4426 this.name.setValue(value); 4427 } 4428 return this; 4429 } 4430 4431 /** 4432 * @return {@link #status} (The status of the value set.). This is the 4433 * underlying object with id, value and extensions. The accessor 4434 * "getStatus" gives direct access to the value 4435 */ 4436 public Enumeration<ConformanceResourceStatus> getStatusElement() { 4437 if (this.status == null) 4438 if (Configuration.errorOnAutoCreate()) 4439 throw new Error("Attempt to auto-create ValueSet.status"); 4440 else if (Configuration.doAutoCreate()) 4441 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 4442 return this.status; 4443 } 4444 4445 public boolean hasStatusElement() { 4446 return this.status != null && !this.status.isEmpty(); 4447 } 4448 4449 public boolean hasStatus() { 4450 return this.status != null && !this.status.isEmpty(); 4451 } 4452 4453 /** 4454 * @param value {@link #status} (The status of the value set.). This is the 4455 * underlying object with id, value and extensions. The accessor 4456 * "getStatus" gives direct access to the value 4457 */ 4458 public ValueSet setStatusElement(Enumeration<ConformanceResourceStatus> value) { 4459 this.status = value; 4460 return this; 4461 } 4462 4463 /** 4464 * @return The status of the value set. 4465 */ 4466 public ConformanceResourceStatus getStatus() { 4467 return this.status == null ? null : this.status.getValue(); 4468 } 4469 4470 /** 4471 * @param value The status of the value set. 4472 */ 4473 public ValueSet setStatus(ConformanceResourceStatus value) { 4474 if (this.status == null) 4475 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 4476 this.status.setValue(value); 4477 return this; 4478 } 4479 4480 /** 4481 * @return {@link #experimental} (This valueset was authored for testing 4482 * purposes (or education/evaluation/marketing), and is not intended to 4483 * be used for genuine usage.). This is the underlying object with id, 4484 * value and extensions. The accessor "getExperimental" gives direct 4485 * access to the value 4486 */ 4487 public BooleanType getExperimentalElement() { 4488 if (this.experimental == null) 4489 if (Configuration.errorOnAutoCreate()) 4490 throw new Error("Attempt to auto-create ValueSet.experimental"); 4491 else if (Configuration.doAutoCreate()) 4492 this.experimental = new BooleanType(); // bb 4493 return this.experimental; 4494 } 4495 4496 public boolean hasExperimentalElement() { 4497 return this.experimental != null && !this.experimental.isEmpty(); 4498 } 4499 4500 public boolean hasExperimental() { 4501 return this.experimental != null && !this.experimental.isEmpty(); 4502 } 4503 4504 /** 4505 * @param value {@link #experimental} (This valueset was authored for testing 4506 * purposes (or education/evaluation/marketing), and is not 4507 * intended to be used for genuine usage.). This is the underlying 4508 * object with id, value and extensions. The accessor 4509 * "getExperimental" gives direct access to the value 4510 */ 4511 public ValueSet setExperimentalElement(BooleanType value) { 4512 this.experimental = value; 4513 return this; 4514 } 4515 4516 /** 4517 * @return This valueset was authored for testing purposes (or 4518 * education/evaluation/marketing), and is not intended to be used for 4519 * genuine usage. 4520 */ 4521 public boolean getExperimental() { 4522 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4523 } 4524 4525 /** 4526 * @param value This valueset was authored for testing purposes (or 4527 * education/evaluation/marketing), and is not intended to be used 4528 * for genuine usage. 4529 */ 4530 public ValueSet setExperimental(boolean value) { 4531 if (this.experimental == null) 4532 this.experimental = new BooleanType(); 4533 this.experimental.setValue(value); 4534 return this; 4535 } 4536 4537 /** 4538 * @return {@link #publisher} (The name of the individual or organization that 4539 * published the value set.). This is the underlying object with id, 4540 * value and extensions. The accessor "getPublisher" gives direct access 4541 * to the value 4542 */ 4543 public StringType getPublisherElement() { 4544 if (this.publisher == null) 4545 if (Configuration.errorOnAutoCreate()) 4546 throw new Error("Attempt to auto-create ValueSet.publisher"); 4547 else if (Configuration.doAutoCreate()) 4548 this.publisher = new StringType(); // bb 4549 return this.publisher; 4550 } 4551 4552 public boolean hasPublisherElement() { 4553 return this.publisher != null && !this.publisher.isEmpty(); 4554 } 4555 4556 public boolean hasPublisher() { 4557 return this.publisher != null && !this.publisher.isEmpty(); 4558 } 4559 4560 /** 4561 * @param value {@link #publisher} (The name of the individual or organization 4562 * that published the value set.). This is the underlying object 4563 * with id, value and extensions. The accessor "getPublisher" gives 4564 * direct access to the value 4565 */ 4566 public ValueSet setPublisherElement(StringType value) { 4567 this.publisher = value; 4568 return this; 4569 } 4570 4571 /** 4572 * @return The name of the individual or organization that published the value 4573 * set. 4574 */ 4575 public String getPublisher() { 4576 return this.publisher == null ? null : this.publisher.getValue(); 4577 } 4578 4579 /** 4580 * @param value The name of the individual or organization that published the 4581 * value set. 4582 */ 4583 public ValueSet setPublisher(String value) { 4584 if (Utilities.noString(value)) 4585 this.publisher = null; 4586 else { 4587 if (this.publisher == null) 4588 this.publisher = new StringType(); 4589 this.publisher.setValue(value); 4590 } 4591 return this; 4592 } 4593 4594 /** 4595 * @return {@link #contact} (Contacts to assist a user in finding and 4596 * communicating with the publisher.) 4597 */ 4598 public List<ValueSetContactComponent> getContact() { 4599 if (this.contact == null) 4600 this.contact = new ArrayList<ValueSetContactComponent>(); 4601 return this.contact; 4602 } 4603 4604 public boolean hasContact() { 4605 if (this.contact == null) 4606 return false; 4607 for (ValueSetContactComponent item : this.contact) 4608 if (!item.isEmpty()) 4609 return true; 4610 return false; 4611 } 4612 4613 /** 4614 * @return {@link #contact} (Contacts to assist a user in finding and 4615 * communicating with the publisher.) 4616 */ 4617 // syntactic sugar 4618 public ValueSetContactComponent addContact() { // 3 4619 ValueSetContactComponent t = new ValueSetContactComponent(); 4620 if (this.contact == null) 4621 this.contact = new ArrayList<ValueSetContactComponent>(); 4622 this.contact.add(t); 4623 return t; 4624 } 4625 4626 // syntactic sugar 4627 public ValueSet addContact(ValueSetContactComponent t) { // 3 4628 if (t == null) 4629 return this; 4630 if (this.contact == null) 4631 this.contact = new ArrayList<ValueSetContactComponent>(); 4632 this.contact.add(t); 4633 return this; 4634 } 4635 4636 /** 4637 * @return {@link #date} (The date that the value set status was last changed. 4638 * The date must change when the business version changes, if it does, 4639 * and it must change if the status code changes. In addition, it should 4640 * change when the substantive content of the implementation guide 4641 * changes (e.g. the 'content logical definition').). This is the 4642 * underlying object with id, value and extensions. The accessor 4643 * "getDate" gives direct access to the value 4644 */ 4645 public DateTimeType getDateElement() { 4646 if (this.date == null) 4647 if (Configuration.errorOnAutoCreate()) 4648 throw new Error("Attempt to auto-create ValueSet.date"); 4649 else if (Configuration.doAutoCreate()) 4650 this.date = new DateTimeType(); // bb 4651 return this.date; 4652 } 4653 4654 public boolean hasDateElement() { 4655 return this.date != null && !this.date.isEmpty(); 4656 } 4657 4658 public boolean hasDate() { 4659 return this.date != null && !this.date.isEmpty(); 4660 } 4661 4662 /** 4663 * @param value {@link #date} (The date that the value set status was last 4664 * changed. The date must change when the business version changes, 4665 * if it does, and it must change if the status code changes. In 4666 * addition, it should change when the substantive content of the 4667 * implementation guide changes (e.g. the 'content logical 4668 * definition').). This is the underlying object with id, value and 4669 * extensions. The accessor "getDate" gives direct access to the 4670 * value 4671 */ 4672 public ValueSet setDateElement(DateTimeType value) { 4673 this.date = value; 4674 return this; 4675 } 4676 4677 /** 4678 * @return The date that the value set status was last changed. The date must 4679 * change when the business version changes, if it does, and it must 4680 * change if the status code changes. In addition, it should change when 4681 * the substantive content of the implementation guide changes (e.g. the 4682 * 'content logical definition'). 4683 */ 4684 public Date getDate() { 4685 return this.date == null ? null : this.date.getValue(); 4686 } 4687 4688 /** 4689 * @param value The date that the value set status was last changed. The date 4690 * must change when the business version changes, if it does, and 4691 * it must change if the status code changes. In addition, it 4692 * should change when the substantive content of the implementation 4693 * guide changes (e.g. the 'content logical definition'). 4694 */ 4695 public ValueSet setDate(Date value) { 4696 if (value == null) 4697 this.date = null; 4698 else { 4699 if (this.date == null) 4700 this.date = new DateTimeType(); 4701 this.date.setValue(value); 4702 } 4703 return this; 4704 } 4705 4706 /** 4707 * @return {@link #lockedDate} (If a locked date is defined, then the Content 4708 * Logical Definition must be evaluated using the current version of all 4709 * referenced code system(s) and value set instances as of the locked 4710 * date.). This is the underlying object with id, value and extensions. 4711 * The accessor "getLockedDate" gives direct access to the value 4712 */ 4713 public DateType getLockedDateElement() { 4714 if (this.lockedDate == null) 4715 if (Configuration.errorOnAutoCreate()) 4716 throw new Error("Attempt to auto-create ValueSet.lockedDate"); 4717 else if (Configuration.doAutoCreate()) 4718 this.lockedDate = new DateType(); // bb 4719 return this.lockedDate; 4720 } 4721 4722 public boolean hasLockedDateElement() { 4723 return this.lockedDate != null && !this.lockedDate.isEmpty(); 4724 } 4725 4726 public boolean hasLockedDate() { 4727 return this.lockedDate != null && !this.lockedDate.isEmpty(); 4728 } 4729 4730 /** 4731 * @param value {@link #lockedDate} (If a locked date is defined, then the 4732 * Content Logical Definition must be evaluated using the current 4733 * version of all referenced code system(s) and value set instances 4734 * as of the locked date.). This is the underlying object with id, 4735 * value and extensions. The accessor "getLockedDate" gives direct 4736 * access to the value 4737 */ 4738 public ValueSet setLockedDateElement(DateType value) { 4739 this.lockedDate = value; 4740 return this; 4741 } 4742 4743 /** 4744 * @return If a locked date is defined, then the Content Logical Definition must 4745 * be evaluated using the current version of all referenced code 4746 * system(s) and value set instances as of the locked date. 4747 */ 4748 public Date getLockedDate() { 4749 return this.lockedDate == null ? null : this.lockedDate.getValue(); 4750 } 4751 4752 /** 4753 * @param value If a locked date is defined, then the Content Logical Definition 4754 * must be evaluated using the current version of all referenced 4755 * code system(s) and value set instances as of the locked date. 4756 */ 4757 public ValueSet setLockedDate(Date value) { 4758 if (value == null) 4759 this.lockedDate = null; 4760 else { 4761 if (this.lockedDate == null) 4762 this.lockedDate = new DateType(); 4763 this.lockedDate.setValue(value); 4764 } 4765 return this; 4766 } 4767 4768 /** 4769 * @return {@link #description} (A free text natural language description of the 4770 * use of the value set - reason for definition, "the semantic space" to 4771 * be included in the value set, conditions of use, etc. The description 4772 * may include a list of expected usages for the value set and can also 4773 * describe the approach taken to build the value set.). This is the 4774 * underlying object with id, value and extensions. The accessor 4775 * "getDescription" gives direct access to the value 4776 */ 4777 public StringType getDescriptionElement() { 4778 if (this.description == null) 4779 if (Configuration.errorOnAutoCreate()) 4780 throw new Error("Attempt to auto-create ValueSet.description"); 4781 else if (Configuration.doAutoCreate()) 4782 this.description = new StringType(); // bb 4783 return this.description; 4784 } 4785 4786 public boolean hasDescriptionElement() { 4787 return this.description != null && !this.description.isEmpty(); 4788 } 4789 4790 public boolean hasDescription() { 4791 return this.description != null && !this.description.isEmpty(); 4792 } 4793 4794 /** 4795 * @param value {@link #description} (A free text natural language description 4796 * of the use of the value set - reason for definition, "the 4797 * semantic space" to be included in the value set, conditions of 4798 * use, etc. The description may include a list of expected usages 4799 * for the value set and can also describe the approach taken to 4800 * build the value set.). This is the underlying object with id, 4801 * value and extensions. The accessor "getDescription" gives direct 4802 * access to the value 4803 */ 4804 public ValueSet setDescriptionElement(StringType value) { 4805 this.description = value; 4806 return this; 4807 } 4808 4809 /** 4810 * @return A free text natural language description of the use of the value set 4811 * - reason for definition, "the semantic space" to be included in the 4812 * value set, conditions of use, etc. The description may include a list 4813 * of expected usages for the value set and can also describe the 4814 * approach taken to build the value set. 4815 */ 4816 public String getDescription() { 4817 return this.description == null ? null : this.description.getValue(); 4818 } 4819 4820 /** 4821 * @param value A free text natural language description of the use of the value 4822 * set - reason for definition, "the semantic space" to be included 4823 * in the value set, conditions of use, etc. The description may 4824 * include a list of expected usages for the value set and can also 4825 * describe the approach taken to build the value set. 4826 */ 4827 public ValueSet setDescription(String value) { 4828 if (Utilities.noString(value)) 4829 this.description = null; 4830 else { 4831 if (this.description == null) 4832 this.description = new StringType(); 4833 this.description.setValue(value); 4834 } 4835 return this; 4836 } 4837 4838 /** 4839 * @return {@link #useContext} (The content was developed with a focus and 4840 * intent of supporting the contexts that are listed. These terms may be 4841 * used to assist with indexing and searching of value set definitions.) 4842 */ 4843 public List<CodeableConcept> getUseContext() { 4844 if (this.useContext == null) 4845 this.useContext = new ArrayList<CodeableConcept>(); 4846 return this.useContext; 4847 } 4848 4849 public boolean hasUseContext() { 4850 if (this.useContext == null) 4851 return false; 4852 for (CodeableConcept item : this.useContext) 4853 if (!item.isEmpty()) 4854 return true; 4855 return false; 4856 } 4857 4858 /** 4859 * @return {@link #useContext} (The content was developed with a focus and 4860 * intent of supporting the contexts that are listed. These terms may be 4861 * used to assist with indexing and searching of value set definitions.) 4862 */ 4863 // syntactic sugar 4864 public CodeableConcept addUseContext() { // 3 4865 CodeableConcept t = new CodeableConcept(); 4866 if (this.useContext == null) 4867 this.useContext = new ArrayList<CodeableConcept>(); 4868 this.useContext.add(t); 4869 return t; 4870 } 4871 4872 // syntactic sugar 4873 public ValueSet addUseContext(CodeableConcept t) { // 3 4874 if (t == null) 4875 return this; 4876 if (this.useContext == null) 4877 this.useContext = new ArrayList<CodeableConcept>(); 4878 this.useContext.add(t); 4879 return this; 4880 } 4881 4882 /** 4883 * @return {@link #immutable} (If this is set to 'true', then no new versions of 4884 * the content logical definition can be created. Note: Other metadata 4885 * might still change.). This is the underlying object with id, value 4886 * and extensions. The accessor "getImmutable" gives direct access to 4887 * the value 4888 */ 4889 public BooleanType getImmutableElement() { 4890 if (this.immutable == null) 4891 if (Configuration.errorOnAutoCreate()) 4892 throw new Error("Attempt to auto-create ValueSet.immutable"); 4893 else if (Configuration.doAutoCreate()) 4894 this.immutable = new BooleanType(); // bb 4895 return this.immutable; 4896 } 4897 4898 public boolean hasImmutableElement() { 4899 return this.immutable != null && !this.immutable.isEmpty(); 4900 } 4901 4902 public boolean hasImmutable() { 4903 return this.immutable != null && !this.immutable.isEmpty(); 4904 } 4905 4906 /** 4907 * @param value {@link #immutable} (If this is set to 'true', then no new 4908 * versions of the content logical definition can be created. Note: 4909 * Other metadata might still change.). This is the underlying 4910 * object with id, value and extensions. The accessor 4911 * "getImmutable" gives direct access to the value 4912 */ 4913 public ValueSet setImmutableElement(BooleanType value) { 4914 this.immutable = value; 4915 return this; 4916 } 4917 4918 /** 4919 * @return If this is set to 'true', then no new versions of the content logical 4920 * definition can be created. Note: Other metadata might still change. 4921 */ 4922 public boolean getImmutable() { 4923 return this.immutable == null || this.immutable.isEmpty() ? false : this.immutable.getValue(); 4924 } 4925 4926 /** 4927 * @param value If this is set to 'true', then no new versions of the content 4928 * logical definition can be created. Note: Other metadata might 4929 * still change. 4930 */ 4931 public ValueSet setImmutable(boolean value) { 4932 if (this.immutable == null) 4933 this.immutable = new BooleanType(); 4934 this.immutable.setValue(value); 4935 return this; 4936 } 4937 4938 /** 4939 * @return {@link #requirements} (Explains why this value set is needed and why 4940 * it has been constrained as it has.). This is the underlying object 4941 * with id, value and extensions. The accessor "getRequirements" gives 4942 * direct access to the value 4943 */ 4944 public StringType getRequirementsElement() { 4945 if (this.requirements == null) 4946 if (Configuration.errorOnAutoCreate()) 4947 throw new Error("Attempt to auto-create ValueSet.requirements"); 4948 else if (Configuration.doAutoCreate()) 4949 this.requirements = new StringType(); // bb 4950 return this.requirements; 4951 } 4952 4953 public boolean hasRequirementsElement() { 4954 return this.requirements != null && !this.requirements.isEmpty(); 4955 } 4956 4957 public boolean hasRequirements() { 4958 return this.requirements != null && !this.requirements.isEmpty(); 4959 } 4960 4961 /** 4962 * @param value {@link #requirements} (Explains why this value set is needed and 4963 * why it has been constrained as it has.). This is the underlying 4964 * object with id, value and extensions. The accessor 4965 * "getRequirements" gives direct access to the value 4966 */ 4967 public ValueSet setRequirementsElement(StringType value) { 4968 this.requirements = value; 4969 return this; 4970 } 4971 4972 /** 4973 * @return Explains why this value set is needed and why it has been constrained 4974 * as it has. 4975 */ 4976 public String getRequirements() { 4977 return this.requirements == null ? null : this.requirements.getValue(); 4978 } 4979 4980 /** 4981 * @param value Explains why this value set is needed and why it has been 4982 * constrained as it has. 4983 */ 4984 public ValueSet setRequirements(String value) { 4985 if (Utilities.noString(value)) 4986 this.requirements = null; 4987 else { 4988 if (this.requirements == null) 4989 this.requirements = new StringType(); 4990 this.requirements.setValue(value); 4991 } 4992 return this; 4993 } 4994 4995 /** 4996 * @return {@link #copyright} (A copyright statement relating to the value set 4997 * and/or its contents. Copyright statements are generally legal 4998 * restrictions on the use and publishing of the value set.). This is 4999 * the underlying object with id, value and extensions. The accessor 5000 * "getCopyright" gives direct access to the value 5001 */ 5002 public StringType getCopyrightElement() { 5003 if (this.copyright == null) 5004 if (Configuration.errorOnAutoCreate()) 5005 throw new Error("Attempt to auto-create ValueSet.copyright"); 5006 else if (Configuration.doAutoCreate()) 5007 this.copyright = new StringType(); // bb 5008 return this.copyright; 5009 } 5010 5011 public boolean hasCopyrightElement() { 5012 return this.copyright != null && !this.copyright.isEmpty(); 5013 } 5014 5015 public boolean hasCopyright() { 5016 return this.copyright != null && !this.copyright.isEmpty(); 5017 } 5018 5019 /** 5020 * @param value {@link #copyright} (A copyright statement relating to the value 5021 * set and/or its contents. Copyright statements are generally 5022 * legal restrictions on the use and publishing of the value set.). 5023 * This is the underlying object with id, value and extensions. The 5024 * accessor "getCopyright" gives direct access to the value 5025 */ 5026 public ValueSet setCopyrightElement(StringType value) { 5027 this.copyright = value; 5028 return this; 5029 } 5030 5031 /** 5032 * @return A copyright statement relating to the value set and/or its contents. 5033 * Copyright statements are generally legal restrictions on the use and 5034 * publishing of the value set. 5035 */ 5036 public String getCopyright() { 5037 return this.copyright == null ? null : this.copyright.getValue(); 5038 } 5039 5040 /** 5041 * @param value A copyright statement relating to the value set and/or its 5042 * contents. Copyright statements are generally legal restrictions 5043 * on the use and publishing of the value set. 5044 */ 5045 public ValueSet setCopyright(String value) { 5046 if (Utilities.noString(value)) 5047 this.copyright = null; 5048 else { 5049 if (this.copyright == null) 5050 this.copyright = new StringType(); 5051 this.copyright.setValue(value); 5052 } 5053 return this; 5054 } 5055 5056 /** 5057 * @return {@link #extensible} (Whether this is intended to be used with an 5058 * extensible binding or not.). This is the underlying object with id, 5059 * value and extensions. The accessor "getExtensible" gives direct 5060 * access to the value 5061 */ 5062 public BooleanType getExtensibleElement() { 5063 if (this.extensible == null) 5064 if (Configuration.errorOnAutoCreate()) 5065 throw new Error("Attempt to auto-create ValueSet.extensible"); 5066 else if (Configuration.doAutoCreate()) 5067 this.extensible = new BooleanType(); // bb 5068 return this.extensible; 5069 } 5070 5071 public boolean hasExtensibleElement() { 5072 return this.extensible != null && !this.extensible.isEmpty(); 5073 } 5074 5075 public boolean hasExtensible() { 5076 return this.extensible != null && !this.extensible.isEmpty(); 5077 } 5078 5079 /** 5080 * @param value {@link #extensible} (Whether this is intended to be used with an 5081 * extensible binding or not.). This is the underlying object with 5082 * id, value and extensions. The accessor "getExtensible" gives 5083 * direct access to the value 5084 */ 5085 public ValueSet setExtensibleElement(BooleanType value) { 5086 this.extensible = value; 5087 return this; 5088 } 5089 5090 /** 5091 * @return Whether this is intended to be used with an extensible binding or 5092 * not. 5093 */ 5094 public boolean getExtensible() { 5095 return this.extensible == null || this.extensible.isEmpty() ? false : this.extensible.getValue(); 5096 } 5097 5098 /** 5099 * @param value Whether this is intended to be used with an extensible binding 5100 * or not. 5101 */ 5102 public ValueSet setExtensible(boolean value) { 5103 if (this.extensible == null) 5104 this.extensible = new BooleanType(); 5105 this.extensible.setValue(value); 5106 return this; 5107 } 5108 5109 /** 5110 * @return {@link #codeSystem} (A definition of a code system, inlined into the 5111 * value set (as a packaging convenience). Note that the inline code 5112 * system may be used from other value sets by referring to its 5113 * (codeSystem.system) directly.) 5114 */ 5115 public ValueSetCodeSystemComponent getCodeSystem() { 5116 if (this.codeSystem == null) 5117 if (Configuration.errorOnAutoCreate()) 5118 throw new Error("Attempt to auto-create ValueSet.codeSystem"); 5119 else if (Configuration.doAutoCreate()) 5120 this.codeSystem = new ValueSetCodeSystemComponent(); // cc 5121 return this.codeSystem; 5122 } 5123 5124 public boolean hasCodeSystem() { 5125 return this.codeSystem != null && !this.codeSystem.isEmpty(); 5126 } 5127 5128 /** 5129 * @param value {@link #codeSystem} (A definition of a code system, inlined into 5130 * the value set (as a packaging convenience). Note that the inline 5131 * code system may be used from other value sets by referring to 5132 * its (codeSystem.system) directly.) 5133 */ 5134 public ValueSet setCodeSystem(ValueSetCodeSystemComponent value) { 5135 this.codeSystem = value; 5136 return this; 5137 } 5138 5139 /** 5140 * @return {@link #compose} (A set of criteria that provide the content logical 5141 * definition of the value set by including or excluding codes from 5142 * outside this value set.) 5143 */ 5144 public ValueSetComposeComponent getCompose() { 5145 if (this.compose == null) 5146 if (Configuration.errorOnAutoCreate()) 5147 throw new Error("Attempt to auto-create ValueSet.compose"); 5148 else if (Configuration.doAutoCreate()) 5149 this.compose = new ValueSetComposeComponent(); // cc 5150 return this.compose; 5151 } 5152 5153 public boolean hasCompose() { 5154 return this.compose != null && !this.compose.isEmpty(); 5155 } 5156 5157 /** 5158 * @param value {@link #compose} (A set of criteria that provide the content 5159 * logical definition of the value set by including or excluding 5160 * codes from outside this value set.) 5161 */ 5162 public ValueSet setCompose(ValueSetComposeComponent value) { 5163 this.compose = value; 5164 return this; 5165 } 5166 5167 /** 5168 * @return {@link #expansion} (A value set can also be "expanded", where the 5169 * value set is turned into a simple collection of enumerated codes. 5170 * This element holds the expansion, if it has been performed.) 5171 */ 5172 public ValueSetExpansionComponent getExpansion() { 5173 if (this.expansion == null) 5174 if (Configuration.errorOnAutoCreate()) 5175 throw new Error("Attempt to auto-create ValueSet.expansion"); 5176 else if (Configuration.doAutoCreate()) 5177 this.expansion = new ValueSetExpansionComponent(); // cc 5178 return this.expansion; 5179 } 5180 5181 public boolean hasExpansion() { 5182 return this.expansion != null && !this.expansion.isEmpty(); 5183 } 5184 5185 /** 5186 * @param value {@link #expansion} (A value set can also be "expanded", where 5187 * the value set is turned into a simple collection of enumerated 5188 * codes. This element holds the expansion, if it has been 5189 * performed.) 5190 */ 5191 public ValueSet setExpansion(ValueSetExpansionComponent value) { 5192 this.expansion = value; 5193 return this; 5194 } 5195 5196 protected void listChildren(List<Property> childrenList) { 5197 super.listChildren(childrenList); 5198 childrenList.add(new Property("url", "uri", 5199 "An absolute URL that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published.", 5200 0, java.lang.Integer.MAX_VALUE, url)); 5201 childrenList.add(new Property("identifier", "Identifier", 5202 "Formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5203 0, java.lang.Integer.MAX_VALUE, identifier)); 5204 childrenList.add(new Property("version", "string", 5205 "Used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.", 5206 0, java.lang.Integer.MAX_VALUE, version)); 5207 childrenList.add(new Property("name", "string", "A free text natural language name describing the value set.", 0, 5208 java.lang.Integer.MAX_VALUE, name)); 5209 childrenList 5210 .add(new Property("status", "code", "The status of the value set.", 0, java.lang.Integer.MAX_VALUE, status)); 5211 childrenList.add(new Property("experimental", "boolean", 5212 "This valueset was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 5213 0, java.lang.Integer.MAX_VALUE, experimental)); 5214 childrenList.add( 5215 new Property("publisher", "string", "The name of the individual or organization that published the value set.", 5216 0, java.lang.Integer.MAX_VALUE, publisher)); 5217 childrenList 5218 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 5219 0, java.lang.Integer.MAX_VALUE, contact)); 5220 childrenList.add(new Property("date", "dateTime", 5221 "The date that the value set status was last changed. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes (e.g. the 'content logical definition').", 5222 0, java.lang.Integer.MAX_VALUE, date)); 5223 childrenList.add(new Property("lockedDate", "date", 5224 "If a locked date is defined, then the Content Logical Definition must be evaluated using the current version of all referenced code system(s) and value set instances as of the locked date.", 5225 0, java.lang.Integer.MAX_VALUE, lockedDate)); 5226 childrenList.add(new Property("description", "string", 5227 "A free text natural language description of the use of the value set - reason for definition, \"the semantic space\" to be included in the value set, conditions of use, etc. The description may include a list of expected usages for the value set and can also describe the approach taken to build the value set.", 5228 0, java.lang.Integer.MAX_VALUE, description)); 5229 childrenList.add(new Property("useContext", "CodeableConcept", 5230 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of value set definitions.", 5231 0, java.lang.Integer.MAX_VALUE, useContext)); 5232 childrenList.add(new Property("immutable", "boolean", 5233 "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 5234 0, java.lang.Integer.MAX_VALUE, immutable)); 5235 childrenList.add(new Property("requirements", "string", 5236 "Explains why this value set is needed and why it has been constrained as it has.", 0, 5237 java.lang.Integer.MAX_VALUE, requirements)); 5238 childrenList.add(new Property("copyright", "string", 5239 "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 5240 0, java.lang.Integer.MAX_VALUE, copyright)); 5241 childrenList.add( 5242 new Property("extensible", "boolean", "Whether this is intended to be used with an extensible binding or not.", 5243 0, java.lang.Integer.MAX_VALUE, extensible)); 5244 childrenList.add(new Property("codeSystem", "", 5245 "A definition of a code system, inlined into the value set (as a packaging convenience). Note that the inline code system may be used from other value sets by referring to its (codeSystem.system) directly.", 5246 0, java.lang.Integer.MAX_VALUE, codeSystem)); 5247 childrenList.add(new Property("compose", "", 5248 "A set of criteria that provide the content logical definition of the value set by including or excluding codes from outside this value set.", 5249 0, java.lang.Integer.MAX_VALUE, compose)); 5250 childrenList.add(new Property("expansion", "", 5251 "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 5252 0, java.lang.Integer.MAX_VALUE, expansion)); 5253 } 5254 5255 @Override 5256 public void setProperty(String name, Base value) throws FHIRException { 5257 if (name.equals("url")) 5258 this.url = castToUri(value); // UriType 5259 else if (name.equals("identifier")) 5260 this.identifier = castToIdentifier(value); // Identifier 5261 else if (name.equals("version")) 5262 this.version = castToString(value); // StringType 5263 else if (name.equals("name")) 5264 this.name = castToString(value); // StringType 5265 else if (name.equals("status")) 5266 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 5267 else if (name.equals("experimental")) 5268 this.experimental = castToBoolean(value); // BooleanType 5269 else if (name.equals("publisher")) 5270 this.publisher = castToString(value); // StringType 5271 else if (name.equals("contact")) 5272 this.getContact().add((ValueSetContactComponent) value); 5273 else if (name.equals("date")) 5274 this.date = castToDateTime(value); // DateTimeType 5275 else if (name.equals("lockedDate")) 5276 this.lockedDate = castToDate(value); // DateType 5277 else if (name.equals("description")) 5278 this.description = castToString(value); // StringType 5279 else if (name.equals("useContext")) 5280 this.getUseContext().add(castToCodeableConcept(value)); 5281 else if (name.equals("immutable")) 5282 this.immutable = castToBoolean(value); // BooleanType 5283 else if (name.equals("requirements")) 5284 this.requirements = castToString(value); // StringType 5285 else if (name.equals("copyright")) 5286 this.copyright = castToString(value); // StringType 5287 else if (name.equals("extensible")) 5288 this.extensible = castToBoolean(value); // BooleanType 5289 else if (name.equals("codeSystem")) 5290 this.codeSystem = (ValueSetCodeSystemComponent) value; // ValueSetCodeSystemComponent 5291 else if (name.equals("compose")) 5292 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 5293 else if (name.equals("expansion")) 5294 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 5295 else 5296 super.setProperty(name, value); 5297 } 5298 5299 @Override 5300 public Base addChild(String name) throws FHIRException { 5301 if (name.equals("url")) { 5302 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.url"); 5303 } else if (name.equals("identifier")) { 5304 this.identifier = new Identifier(); 5305 return this.identifier; 5306 } else if (name.equals("version")) { 5307 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 5308 } else if (name.equals("name")) { 5309 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 5310 } else if (name.equals("status")) { 5311 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.status"); 5312 } else if (name.equals("experimental")) { 5313 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.experimental"); 5314 } else if (name.equals("publisher")) { 5315 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.publisher"); 5316 } else if (name.equals("contact")) { 5317 return addContact(); 5318 } else if (name.equals("date")) { 5319 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.date"); 5320 } else if (name.equals("lockedDate")) { 5321 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.lockedDate"); 5322 } else if (name.equals("description")) { 5323 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.description"); 5324 } else if (name.equals("useContext")) { 5325 return addUseContext(); 5326 } else if (name.equals("immutable")) { 5327 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.immutable"); 5328 } else if (name.equals("requirements")) { 5329 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.requirements"); 5330 } else if (name.equals("copyright")) { 5331 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.copyright"); 5332 } else if (name.equals("extensible")) { 5333 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.extensible"); 5334 } else if (name.equals("codeSystem")) { 5335 this.codeSystem = new ValueSetCodeSystemComponent(); 5336 return this.codeSystem; 5337 } else if (name.equals("compose")) { 5338 this.compose = new ValueSetComposeComponent(); 5339 return this.compose; 5340 } else if (name.equals("expansion")) { 5341 this.expansion = new ValueSetExpansionComponent(); 5342 return this.expansion; 5343 } else 5344 return super.addChild(name); 5345 } 5346 5347 public String fhirType() { 5348 return "ValueSet"; 5349 5350 } 5351 5352 public ValueSet copy() { 5353 ValueSet dst = new ValueSet(); 5354 copyValues(dst); 5355 dst.url = url == null ? null : url.copy(); 5356 dst.identifier = identifier == null ? null : identifier.copy(); 5357 dst.version = version == null ? null : version.copy(); 5358 dst.name = name == null ? null : name.copy(); 5359 dst.status = status == null ? null : status.copy(); 5360 dst.experimental = experimental == null ? null : experimental.copy(); 5361 dst.publisher = publisher == null ? null : publisher.copy(); 5362 if (contact != null) { 5363 dst.contact = new ArrayList<ValueSetContactComponent>(); 5364 for (ValueSetContactComponent i : contact) 5365 dst.contact.add(i.copy()); 5366 } 5367 ; 5368 dst.date = date == null ? null : date.copy(); 5369 dst.lockedDate = lockedDate == null ? null : lockedDate.copy(); 5370 dst.description = description == null ? null : description.copy(); 5371 if (useContext != null) { 5372 dst.useContext = new ArrayList<CodeableConcept>(); 5373 for (CodeableConcept i : useContext) 5374 dst.useContext.add(i.copy()); 5375 } 5376 ; 5377 dst.immutable = immutable == null ? null : immutable.copy(); 5378 dst.requirements = requirements == null ? null : requirements.copy(); 5379 dst.copyright = copyright == null ? null : copyright.copy(); 5380 dst.extensible = extensible == null ? null : extensible.copy(); 5381 dst.codeSystem = codeSystem == null ? null : codeSystem.copy(); 5382 dst.compose = compose == null ? null : compose.copy(); 5383 dst.expansion = expansion == null ? null : expansion.copy(); 5384 return dst; 5385 } 5386 5387 protected ValueSet typedCopy() { 5388 return copy(); 5389 } 5390 5391 @Override 5392 public boolean equalsDeep(Base other) { 5393 if (!super.equalsDeep(other)) 5394 return false; 5395 if (!(other instanceof ValueSet)) 5396 return false; 5397 ValueSet o = (ValueSet) other; 5398 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) 5399 && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 5400 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 5401 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 5402 && compareDeep(date, o.date, true) && compareDeep(lockedDate, o.lockedDate, true) 5403 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 5404 && compareDeep(immutable, o.immutable, true) && compareDeep(requirements, o.requirements, true) 5405 && compareDeep(copyright, o.copyright, true) && compareDeep(extensible, o.extensible, true) 5406 && compareDeep(codeSystem, o.codeSystem, true) && compareDeep(compose, o.compose, true) 5407 && compareDeep(expansion, o.expansion, true); 5408 } 5409 5410 @Override 5411 public boolean equalsShallow(Base other) { 5412 if (!super.equalsShallow(other)) 5413 return false; 5414 if (!(other instanceof ValueSet)) 5415 return false; 5416 ValueSet o = (ValueSet) other; 5417 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 5418 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 5419 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 5420 && compareValues(date, o.date, true) && compareValues(lockedDate, o.lockedDate, true) 5421 && compareValues(description, o.description, true) && compareValues(immutable, o.immutable, true) 5422 && compareValues(requirements, o.requirements, true) && compareValues(copyright, o.copyright, true) 5423 && compareValues(extensible, o.extensible, true); 5424 } 5425 5426 public boolean isEmpty() { 5427 return super.isEmpty() && (url == null || url.isEmpty()) && (identifier == null || identifier.isEmpty()) 5428 && (version == null || version.isEmpty()) && (name == null || name.isEmpty()) 5429 && (status == null || status.isEmpty()) && (experimental == null || experimental.isEmpty()) 5430 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 5431 && (date == null || date.isEmpty()) && (lockedDate == null || lockedDate.isEmpty()) 5432 && (description == null || description.isEmpty()) && (useContext == null || useContext.isEmpty()) 5433 && (immutable == null || immutable.isEmpty()) && (requirements == null || requirements.isEmpty()) 5434 && (copyright == null || copyright.isEmpty()) && (extensible == null || extensible.isEmpty()) 5435 && (codeSystem == null || codeSystem.isEmpty()) && (compose == null || compose.isEmpty()) 5436 && (expansion == null || expansion.isEmpty()); 5437 } 5438 5439 @Override 5440 public ResourceType getResourceType() { 5441 return ResourceType.ValueSet; 5442 } 5443 5444 @SearchParamDefinition(name = "date", path = "ValueSet.date", description = "The value set publication date", type = "date") 5445 public static final String SP_DATE = "date"; 5446 @SearchParamDefinition(name = "identifier", path = "ValueSet.identifier", description = "The identifier for the value set", type = "token") 5447 public static final String SP_IDENTIFIER = "identifier"; 5448 @SearchParamDefinition(name = "code", path = "ValueSet.codeSystem.concept.code", description = "A code defined in the value set", type = "token") 5449 public static final String SP_CODE = "code"; 5450 @SearchParamDefinition(name = "description", path = "ValueSet.description", description = "Text search in the description of the value set", type = "string") 5451 public static final String SP_DESCRIPTION = "description"; 5452 @SearchParamDefinition(name = "version", path = "ValueSet.version", description = "The version identifier of the value set", type = "token") 5453 public static final String SP_VERSION = "version"; 5454 @SearchParamDefinition(name = "url", path = "ValueSet.url", description = "The logical URL for the value set", type = "uri") 5455 public static final String SP_URL = "url"; 5456 @SearchParamDefinition(name = "expansion", path = "ValueSet.expansion.identifier", description = "Uniquely identifies this expansion", type = "uri") 5457 public static final String SP_EXPANSION = "expansion"; 5458 @SearchParamDefinition(name = "reference", path = "ValueSet.compose.include.system", description = "A code system included or excluded in the value set or an imported value set", type = "uri") 5459 public static final String SP_REFERENCE = "reference"; 5460 @SearchParamDefinition(name = "system", path = "ValueSet.codeSystem.system", description = "The system for any codes defined by this value set", type = "uri") 5461 public static final String SP_SYSTEM = "system"; 5462 @SearchParamDefinition(name = "name", path = "ValueSet.name", description = "The name of the value set", type = "string") 5463 public static final String SP_NAME = "name"; 5464 @SearchParamDefinition(name = "context", path = "ValueSet.useContext", description = "A use context assigned to the value set", type = "token") 5465 public static final String SP_CONTEXT = "context"; 5466 @SearchParamDefinition(name = "publisher", path = "ValueSet.publisher", description = "Name of the publisher of the value set", type = "string") 5467 public static final String SP_PUBLISHER = "publisher"; 5468 @SearchParamDefinition(name = "status", path = "ValueSet.status", description = "The status of the value set", type = "token") 5469 public static final String SP_STATUS = "status"; 5470 5471}