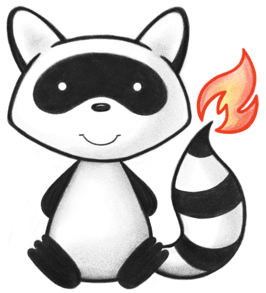
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * An authorization for the supply of glasses and/or contact lenses to a 050 * patient. 051 */ 052@ResourceDef(name = "VisionPrescription", profile = "http://hl7.org/fhir/Profile/VisionPrescription") 053public class VisionPrescription extends DomainResource { 054 055 public enum VisionEyes { 056 /** 057 * Right Eye 058 */ 059 RIGHT, 060 /** 061 * Left Eye 062 */ 063 LEFT, 064 /** 065 * added to help the parsers 066 */ 067 NULL; 068 069 public static VisionEyes fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("right".equals(codeString)) 073 return RIGHT; 074 if ("left".equals(codeString)) 075 return LEFT; 076 throw new FHIRException("Unknown VisionEyes code '" + codeString + "'"); 077 } 078 079 public String toCode() { 080 switch (this) { 081 case RIGHT: 082 return "right"; 083 case LEFT: 084 return "left"; 085 case NULL: 086 return null; 087 default: 088 return "?"; 089 } 090 } 091 092 public String getSystem() { 093 switch (this) { 094 case RIGHT: 095 return "http://hl7.org/fhir/vision-eye-codes"; 096 case LEFT: 097 return "http://hl7.org/fhir/vision-eye-codes"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getDefinition() { 106 switch (this) { 107 case RIGHT: 108 return "Right Eye"; 109 case LEFT: 110 return "Left Eye"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getDisplay() { 119 switch (this) { 120 case RIGHT: 121 return "Right Eye"; 122 case LEFT: 123 return "Left Eye"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 } 131 132 public static class VisionEyesEnumFactory implements EnumFactory<VisionEyes> { 133 public VisionEyes fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("right".equals(codeString)) 138 return VisionEyes.RIGHT; 139 if ("left".equals(codeString)) 140 return VisionEyes.LEFT; 141 throw new IllegalArgumentException("Unknown VisionEyes code '" + codeString + "'"); 142 } 143 144 public Enumeration<VisionEyes> fromType(Base code) throws FHIRException { 145 if (code == null || code.isEmpty()) 146 return null; 147 String codeString = ((PrimitiveType) code).asStringValue(); 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("right".equals(codeString)) 151 return new Enumeration<VisionEyes>(this, VisionEyes.RIGHT); 152 if ("left".equals(codeString)) 153 return new Enumeration<VisionEyes>(this, VisionEyes.LEFT); 154 throw new FHIRException("Unknown VisionEyes code '" + codeString + "'"); 155 } 156 157 public String toCode(VisionEyes code) 158 { 159 if (code == VisionEyes.NULL) 160 return null; 161 if (code == VisionEyes.RIGHT) 162 return "right"; 163 if (code == VisionEyes.LEFT) 164 return "left"; 165 return "?"; 166 } 167 } 168 169 public enum VisionBase { 170 /** 171 * top 172 */ 173 UP, 174 /** 175 * bottom 176 */ 177 DOWN, 178 /** 179 * inner edge 180 */ 181 IN, 182 /** 183 * outer edge 184 */ 185 OUT, 186 /** 187 * added to help the parsers 188 */ 189 NULL; 190 191 public static VisionBase fromCode(String codeString) throws FHIRException { 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("up".equals(codeString)) 195 return UP; 196 if ("down".equals(codeString)) 197 return DOWN; 198 if ("in".equals(codeString)) 199 return IN; 200 if ("out".equals(codeString)) 201 return OUT; 202 throw new FHIRException("Unknown VisionBase code '" + codeString + "'"); 203 } 204 205 public String toCode() { 206 switch (this) { 207 case UP: 208 return "up"; 209 case DOWN: 210 return "down"; 211 case IN: 212 return "in"; 213 case OUT: 214 return "out"; 215 case NULL: 216 return null; 217 default: 218 return "?"; 219 } 220 } 221 222 public String getSystem() { 223 switch (this) { 224 case UP: 225 return "http://hl7.org/fhir/vision-base-codes"; 226 case DOWN: 227 return "http://hl7.org/fhir/vision-base-codes"; 228 case IN: 229 return "http://hl7.org/fhir/vision-base-codes"; 230 case OUT: 231 return "http://hl7.org/fhir/vision-base-codes"; 232 case NULL: 233 return null; 234 default: 235 return "?"; 236 } 237 } 238 239 public String getDefinition() { 240 switch (this) { 241 case UP: 242 return "top"; 243 case DOWN: 244 return "bottom"; 245 case IN: 246 return "inner edge"; 247 case OUT: 248 return "outer edge"; 249 case NULL: 250 return null; 251 default: 252 return "?"; 253 } 254 } 255 256 public String getDisplay() { 257 switch (this) { 258 case UP: 259 return "Up"; 260 case DOWN: 261 return "Down"; 262 case IN: 263 return "In"; 264 case OUT: 265 return "Out"; 266 case NULL: 267 return null; 268 default: 269 return "?"; 270 } 271 } 272 } 273 274 public static class VisionBaseEnumFactory implements EnumFactory<VisionBase> { 275 public VisionBase fromCode(String codeString) throws IllegalArgumentException { 276 if (codeString == null || "".equals(codeString)) 277 if (codeString == null || "".equals(codeString)) 278 return null; 279 if ("up".equals(codeString)) 280 return VisionBase.UP; 281 if ("down".equals(codeString)) 282 return VisionBase.DOWN; 283 if ("in".equals(codeString)) 284 return VisionBase.IN; 285 if ("out".equals(codeString)) 286 return VisionBase.OUT; 287 throw new IllegalArgumentException("Unknown VisionBase code '" + codeString + "'"); 288 } 289 290 public Enumeration<VisionBase> fromType(Base code) throws FHIRException { 291 if (code == null || code.isEmpty()) 292 return null; 293 String codeString = ((PrimitiveType) code).asStringValue(); 294 if (codeString == null || "".equals(codeString)) 295 return null; 296 if ("up".equals(codeString)) 297 return new Enumeration<VisionBase>(this, VisionBase.UP); 298 if ("down".equals(codeString)) 299 return new Enumeration<VisionBase>(this, VisionBase.DOWN); 300 if ("in".equals(codeString)) 301 return new Enumeration<VisionBase>(this, VisionBase.IN); 302 if ("out".equals(codeString)) 303 return new Enumeration<VisionBase>(this, VisionBase.OUT); 304 throw new FHIRException("Unknown VisionBase code '" + codeString + "'"); 305 } 306 307 public String toCode(VisionBase code) 308 { 309 if (code == VisionBase.NULL) 310 return null; 311 if (code == VisionBase.UP) 312 return "up"; 313 if (code == VisionBase.DOWN) 314 return "down"; 315 if (code == VisionBase.IN) 316 return "in"; 317 if (code == VisionBase.OUT) 318 return "out"; 319 return "?"; 320 } 321 } 322 323 @Block() 324 public static class VisionPrescriptionDispenseComponent extends BackboneElement implements IBaseBackboneElement { 325 /** 326 * Identifies the type of vision correction product which is required for the 327 * patient. 328 */ 329 @Child(name = "product", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 330 @Description(shortDefinition = "Product to be supplied", formalDefinition = "Identifies the type of vision correction product which is required for the patient.") 331 protected Coding product; 332 333 /** 334 * The eye for which the lens applies. 335 */ 336 @Child(name = "eye", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 337 @Description(shortDefinition = "right | left", formalDefinition = "The eye for which the lens applies.") 338 protected Enumeration<VisionEyes> eye; 339 340 /** 341 * Lens power measured in diopters (0.25 units). 342 */ 343 @Child(name = "sphere", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 344 @Description(shortDefinition = "Lens sphere", formalDefinition = "Lens power measured in diopters (0.25 units).") 345 protected DecimalType sphere; 346 347 /** 348 * Power adjustment for astigmatism measured in diopters (0.25 units). 349 */ 350 @Child(name = "cylinder", type = { 351 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 352 @Description(shortDefinition = "Lens cylinder", formalDefinition = "Power adjustment for astigmatism measured in diopters (0.25 units).") 353 protected DecimalType cylinder; 354 355 /** 356 * Adjustment for astigmatism measured in integer degrees. 357 */ 358 @Child(name = "axis", type = { IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 359 @Description(shortDefinition = "Lens axis", formalDefinition = "Adjustment for astigmatism measured in integer degrees.") 360 protected IntegerType axis; 361 362 /** 363 * Amount of prism to compensate for eye alignment in fractional units. 364 */ 365 @Child(name = "prism", type = { DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 366 @Description(shortDefinition = "Lens prism", formalDefinition = "Amount of prism to compensate for eye alignment in fractional units.") 367 protected DecimalType prism; 368 369 /** 370 * The relative base, or reference lens edge, for the prism. 371 */ 372 @Child(name = "base", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 373 @Description(shortDefinition = "up | down | in | out", formalDefinition = "The relative base, or reference lens edge, for the prism.") 374 protected Enumeration<VisionBase> base; 375 376 /** 377 * Power adjustment for multifocal lenses measured in diopters (0.25 units). 378 */ 379 @Child(name = "add", type = { DecimalType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 380 @Description(shortDefinition = "Lens add", formalDefinition = "Power adjustment for multifocal lenses measured in diopters (0.25 units).") 381 protected DecimalType add; 382 383 /** 384 * Contact lens power measured in diopters (0.25 units). 385 */ 386 @Child(name = "power", type = { DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 387 @Description(shortDefinition = "Contact lens power", formalDefinition = "Contact lens power measured in diopters (0.25 units).") 388 protected DecimalType power; 389 390 /** 391 * Back curvature measured in millimeters. 392 */ 393 @Child(name = "backCurve", type = { 394 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 395 @Description(shortDefinition = "Contact lens back curvature", formalDefinition = "Back curvature measured in millimeters.") 396 protected DecimalType backCurve; 397 398 /** 399 * Contact lens diameter measured in millimeters. 400 */ 401 @Child(name = "diameter", type = { 402 DecimalType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 403 @Description(shortDefinition = "Contact lens diameter", formalDefinition = "Contact lens diameter measured in millimeters.") 404 protected DecimalType diameter; 405 406 /** 407 * The recommended maximum wear period for the lens. 408 */ 409 @Child(name = "duration", type = { 410 SimpleQuantity.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 411 @Description(shortDefinition = "Lens wear duration", formalDefinition = "The recommended maximum wear period for the lens.") 412 protected SimpleQuantity duration; 413 414 /** 415 * Special color or pattern. 416 */ 417 @Child(name = "color", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 418 @Description(shortDefinition = "Lens add", formalDefinition = "Special color or pattern.") 419 protected StringType color; 420 421 /** 422 * Brand recommendations or restrictions. 423 */ 424 @Child(name = "brand", type = { StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 425 @Description(shortDefinition = "Lens add", formalDefinition = "Brand recommendations or restrictions.") 426 protected StringType brand; 427 428 /** 429 * Notes for special requirements such as coatings and lens materials. 430 */ 431 @Child(name = "notes", type = { StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 432 @Description(shortDefinition = "Notes for coatings", formalDefinition = "Notes for special requirements such as coatings and lens materials.") 433 protected StringType notes; 434 435 private static final long serialVersionUID = -1586392610L; 436 437 /* 438 * Constructor 439 */ 440 public VisionPrescriptionDispenseComponent() { 441 super(); 442 } 443 444 /* 445 * Constructor 446 */ 447 public VisionPrescriptionDispenseComponent(Coding product) { 448 super(); 449 this.product = product; 450 } 451 452 /** 453 * @return {@link #product} (Identifies the type of vision correction product 454 * which is required for the patient.) 455 */ 456 public Coding getProduct() { 457 if (this.product == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.product"); 460 else if (Configuration.doAutoCreate()) 461 this.product = new Coding(); // cc 462 return this.product; 463 } 464 465 public boolean hasProduct() { 466 return this.product != null && !this.product.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #product} (Identifies the type of vision correction 471 * product which is required for the patient.) 472 */ 473 public VisionPrescriptionDispenseComponent setProduct(Coding value) { 474 this.product = value; 475 return this; 476 } 477 478 /** 479 * @return {@link #eye} (The eye for which the lens applies.). This is the 480 * underlying object with id, value and extensions. The accessor 481 * "getEye" gives direct access to the value 482 */ 483 public Enumeration<VisionEyes> getEyeElement() { 484 if (this.eye == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.eye"); 487 else if (Configuration.doAutoCreate()) 488 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); // bb 489 return this.eye; 490 } 491 492 public boolean hasEyeElement() { 493 return this.eye != null && !this.eye.isEmpty(); 494 } 495 496 public boolean hasEye() { 497 return this.eye != null && !this.eye.isEmpty(); 498 } 499 500 /** 501 * @param value {@link #eye} (The eye for which the lens applies.). This is the 502 * underlying object with id, value and extensions. The accessor 503 * "getEye" gives direct access to the value 504 */ 505 public VisionPrescriptionDispenseComponent setEyeElement(Enumeration<VisionEyes> value) { 506 this.eye = value; 507 return this; 508 } 509 510 /** 511 * @return The eye for which the lens applies. 512 */ 513 public VisionEyes getEye() { 514 return this.eye == null ? null : this.eye.getValue(); 515 } 516 517 /** 518 * @param value The eye for which the lens applies. 519 */ 520 public VisionPrescriptionDispenseComponent setEye(VisionEyes value) { 521 if (value == null) 522 this.eye = null; 523 else { 524 if (this.eye == null) 525 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); 526 this.eye.setValue(value); 527 } 528 return this; 529 } 530 531 /** 532 * @return {@link #sphere} (Lens power measured in diopters (0.25 units).). This 533 * is the underlying object with id, value and extensions. The accessor 534 * "getSphere" gives direct access to the value 535 */ 536 public DecimalType getSphereElement() { 537 if (this.sphere == null) 538 if (Configuration.errorOnAutoCreate()) 539 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.sphere"); 540 else if (Configuration.doAutoCreate()) 541 this.sphere = new DecimalType(); // bb 542 return this.sphere; 543 } 544 545 public boolean hasSphereElement() { 546 return this.sphere != null && !this.sphere.isEmpty(); 547 } 548 549 public boolean hasSphere() { 550 return this.sphere != null && !this.sphere.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #sphere} (Lens power measured in diopters (0.25 units).). 555 * This is the underlying object with id, value and extensions. The 556 * accessor "getSphere" gives direct access to the value 557 */ 558 public VisionPrescriptionDispenseComponent setSphereElement(DecimalType value) { 559 this.sphere = value; 560 return this; 561 } 562 563 /** 564 * @return Lens power measured in diopters (0.25 units). 565 */ 566 public BigDecimal getSphere() { 567 return this.sphere == null ? null : this.sphere.getValue(); 568 } 569 570 /** 571 * @param value Lens power measured in diopters (0.25 units). 572 */ 573 public VisionPrescriptionDispenseComponent setSphere(BigDecimal value) { 574 if (value == null) 575 this.sphere = null; 576 else { 577 if (this.sphere == null) 578 this.sphere = new DecimalType(); 579 this.sphere.setValue(value); 580 } 581 return this; 582 } 583 584 /** 585 * @return {@link #cylinder} (Power adjustment for astigmatism measured in 586 * diopters (0.25 units).). This is the underlying object with id, value 587 * and extensions. The accessor "getCylinder" gives direct access to the 588 * value 589 */ 590 public DecimalType getCylinderElement() { 591 if (this.cylinder == null) 592 if (Configuration.errorOnAutoCreate()) 593 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.cylinder"); 594 else if (Configuration.doAutoCreate()) 595 this.cylinder = new DecimalType(); // bb 596 return this.cylinder; 597 } 598 599 public boolean hasCylinderElement() { 600 return this.cylinder != null && !this.cylinder.isEmpty(); 601 } 602 603 public boolean hasCylinder() { 604 return this.cylinder != null && !this.cylinder.isEmpty(); 605 } 606 607 /** 608 * @param value {@link #cylinder} (Power adjustment for astigmatism measured in 609 * diopters (0.25 units).). This is the underlying object with id, 610 * value and extensions. The accessor "getCylinder" gives direct 611 * access to the value 612 */ 613 public VisionPrescriptionDispenseComponent setCylinderElement(DecimalType value) { 614 this.cylinder = value; 615 return this; 616 } 617 618 /** 619 * @return Power adjustment for astigmatism measured in diopters (0.25 units). 620 */ 621 public BigDecimal getCylinder() { 622 return this.cylinder == null ? null : this.cylinder.getValue(); 623 } 624 625 /** 626 * @param value Power adjustment for astigmatism measured in diopters (0.25 627 * units). 628 */ 629 public VisionPrescriptionDispenseComponent setCylinder(BigDecimal value) { 630 if (value == null) 631 this.cylinder = null; 632 else { 633 if (this.cylinder == null) 634 this.cylinder = new DecimalType(); 635 this.cylinder.setValue(value); 636 } 637 return this; 638 } 639 640 /** 641 * @return {@link #axis} (Adjustment for astigmatism measured in integer 642 * degrees.). This is the underlying object with id, value and 643 * extensions. The accessor "getAxis" gives direct access to the value 644 */ 645 public IntegerType getAxisElement() { 646 if (this.axis == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.axis"); 649 else if (Configuration.doAutoCreate()) 650 this.axis = new IntegerType(); // bb 651 return this.axis; 652 } 653 654 public boolean hasAxisElement() { 655 return this.axis != null && !this.axis.isEmpty(); 656 } 657 658 public boolean hasAxis() { 659 return this.axis != null && !this.axis.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #axis} (Adjustment for astigmatism measured in integer 664 * degrees.). This is the underlying object with id, value and 665 * extensions. The accessor "getAxis" gives direct access to the 666 * value 667 */ 668 public VisionPrescriptionDispenseComponent setAxisElement(IntegerType value) { 669 this.axis = value; 670 return this; 671 } 672 673 /** 674 * @return Adjustment for astigmatism measured in integer degrees. 675 */ 676 public int getAxis() { 677 return this.axis == null || this.axis.isEmpty() ? 0 : this.axis.getValue(); 678 } 679 680 /** 681 * @param value Adjustment for astigmatism measured in integer degrees. 682 */ 683 public VisionPrescriptionDispenseComponent setAxis(int value) { 684 if (this.axis == null) 685 this.axis = new IntegerType(); 686 this.axis.setValue(value); 687 return this; 688 } 689 690 /** 691 * @return {@link #prism} (Amount of prism to compensate for eye alignment in 692 * fractional units.). This is the underlying object with id, value and 693 * extensions. The accessor "getPrism" gives direct access to the value 694 */ 695 public DecimalType getPrismElement() { 696 if (this.prism == null) 697 if (Configuration.errorOnAutoCreate()) 698 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.prism"); 699 else if (Configuration.doAutoCreate()) 700 this.prism = new DecimalType(); // bb 701 return this.prism; 702 } 703 704 public boolean hasPrismElement() { 705 return this.prism != null && !this.prism.isEmpty(); 706 } 707 708 public boolean hasPrism() { 709 return this.prism != null && !this.prism.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #prism} (Amount of prism to compensate for eye alignment 714 * in fractional units.). This is the underlying object with id, 715 * value and extensions. The accessor "getPrism" gives direct 716 * access to the value 717 */ 718 public VisionPrescriptionDispenseComponent setPrismElement(DecimalType value) { 719 this.prism = value; 720 return this; 721 } 722 723 /** 724 * @return Amount of prism to compensate for eye alignment in fractional units. 725 */ 726 public BigDecimal getPrism() { 727 return this.prism == null ? null : this.prism.getValue(); 728 } 729 730 /** 731 * @param value Amount of prism to compensate for eye alignment in fractional 732 * units. 733 */ 734 public VisionPrescriptionDispenseComponent setPrism(BigDecimal value) { 735 if (value == null) 736 this.prism = null; 737 else { 738 if (this.prism == null) 739 this.prism = new DecimalType(); 740 this.prism.setValue(value); 741 } 742 return this; 743 } 744 745 /** 746 * @return {@link #base} (The relative base, or reference lens edge, for the 747 * prism.). This is the underlying object with id, value and extensions. 748 * The accessor "getBase" gives direct access to the value 749 */ 750 public Enumeration<VisionBase> getBaseElement() { 751 if (this.base == null) 752 if (Configuration.errorOnAutoCreate()) 753 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.base"); 754 else if (Configuration.doAutoCreate()) 755 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); // bb 756 return this.base; 757 } 758 759 public boolean hasBaseElement() { 760 return this.base != null && !this.base.isEmpty(); 761 } 762 763 public boolean hasBase() { 764 return this.base != null && !this.base.isEmpty(); 765 } 766 767 /** 768 * @param value {@link #base} (The relative base, or reference lens edge, for 769 * the prism.). This is the underlying object with id, value and 770 * extensions. The accessor "getBase" gives direct access to the 771 * value 772 */ 773 public VisionPrescriptionDispenseComponent setBaseElement(Enumeration<VisionBase> value) { 774 this.base = value; 775 return this; 776 } 777 778 /** 779 * @return The relative base, or reference lens edge, for the prism. 780 */ 781 public VisionBase getBase() { 782 return this.base == null ? null : this.base.getValue(); 783 } 784 785 /** 786 * @param value The relative base, or reference lens edge, for the prism. 787 */ 788 public VisionPrescriptionDispenseComponent setBase(VisionBase value) { 789 if (value == null) 790 this.base = null; 791 else { 792 if (this.base == null) 793 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); 794 this.base.setValue(value); 795 } 796 return this; 797 } 798 799 /** 800 * @return {@link #add} (Power adjustment for multifocal lenses measured in 801 * diopters (0.25 units).). This is the underlying object with id, value 802 * and extensions. The accessor "getAdd" gives direct access to the 803 * value 804 */ 805 public DecimalType getAddElement() { 806 if (this.add == null) 807 if (Configuration.errorOnAutoCreate()) 808 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.add"); 809 else if (Configuration.doAutoCreate()) 810 this.add = new DecimalType(); // bb 811 return this.add; 812 } 813 814 public boolean hasAddElement() { 815 return this.add != null && !this.add.isEmpty(); 816 } 817 818 public boolean hasAdd() { 819 return this.add != null && !this.add.isEmpty(); 820 } 821 822 /** 823 * @param value {@link #add} (Power adjustment for multifocal lenses measured in 824 * diopters (0.25 units).). This is the underlying object with id, 825 * value and extensions. The accessor "getAdd" gives direct access 826 * to the value 827 */ 828 public VisionPrescriptionDispenseComponent setAddElement(DecimalType value) { 829 this.add = value; 830 return this; 831 } 832 833 /** 834 * @return Power adjustment for multifocal lenses measured in diopters (0.25 835 * units). 836 */ 837 public BigDecimal getAdd() { 838 return this.add == null ? null : this.add.getValue(); 839 } 840 841 /** 842 * @param value Power adjustment for multifocal lenses measured in diopters 843 * (0.25 units). 844 */ 845 public VisionPrescriptionDispenseComponent setAdd(BigDecimal value) { 846 if (value == null) 847 this.add = null; 848 else { 849 if (this.add == null) 850 this.add = new DecimalType(); 851 this.add.setValue(value); 852 } 853 return this; 854 } 855 856 /** 857 * @return {@link #power} (Contact lens power measured in diopters (0.25 858 * units).). This is the underlying object with id, value and 859 * extensions. The accessor "getPower" gives direct access to the value 860 */ 861 public DecimalType getPowerElement() { 862 if (this.power == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.power"); 865 else if (Configuration.doAutoCreate()) 866 this.power = new DecimalType(); // bb 867 return this.power; 868 } 869 870 public boolean hasPowerElement() { 871 return this.power != null && !this.power.isEmpty(); 872 } 873 874 public boolean hasPower() { 875 return this.power != null && !this.power.isEmpty(); 876 } 877 878 /** 879 * @param value {@link #power} (Contact lens power measured in diopters (0.25 880 * units).). This is the underlying object with id, value and 881 * extensions. The accessor "getPower" gives direct access to the 882 * value 883 */ 884 public VisionPrescriptionDispenseComponent setPowerElement(DecimalType value) { 885 this.power = value; 886 return this; 887 } 888 889 /** 890 * @return Contact lens power measured in diopters (0.25 units). 891 */ 892 public BigDecimal getPower() { 893 return this.power == null ? null : this.power.getValue(); 894 } 895 896 /** 897 * @param value Contact lens power measured in diopters (0.25 units). 898 */ 899 public VisionPrescriptionDispenseComponent setPower(BigDecimal value) { 900 if (value == null) 901 this.power = null; 902 else { 903 if (this.power == null) 904 this.power = new DecimalType(); 905 this.power.setValue(value); 906 } 907 return this; 908 } 909 910 /** 911 * @return {@link #backCurve} (Back curvature measured in millimeters.). This is 912 * the underlying object with id, value and extensions. The accessor 913 * "getBackCurve" gives direct access to the value 914 */ 915 public DecimalType getBackCurveElement() { 916 if (this.backCurve == null) 917 if (Configuration.errorOnAutoCreate()) 918 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.backCurve"); 919 else if (Configuration.doAutoCreate()) 920 this.backCurve = new DecimalType(); // bb 921 return this.backCurve; 922 } 923 924 public boolean hasBackCurveElement() { 925 return this.backCurve != null && !this.backCurve.isEmpty(); 926 } 927 928 public boolean hasBackCurve() { 929 return this.backCurve != null && !this.backCurve.isEmpty(); 930 } 931 932 /** 933 * @param value {@link #backCurve} (Back curvature measured in millimeters.). 934 * This is the underlying object with id, value and extensions. The 935 * accessor "getBackCurve" gives direct access to the value 936 */ 937 public VisionPrescriptionDispenseComponent setBackCurveElement(DecimalType value) { 938 this.backCurve = value; 939 return this; 940 } 941 942 /** 943 * @return Back curvature measured in millimeters. 944 */ 945 public BigDecimal getBackCurve() { 946 return this.backCurve == null ? null : this.backCurve.getValue(); 947 } 948 949 /** 950 * @param value Back curvature measured in millimeters. 951 */ 952 public VisionPrescriptionDispenseComponent setBackCurve(BigDecimal value) { 953 if (value == null) 954 this.backCurve = null; 955 else { 956 if (this.backCurve == null) 957 this.backCurve = new DecimalType(); 958 this.backCurve.setValue(value); 959 } 960 return this; 961 } 962 963 /** 964 * @return {@link #diameter} (Contact lens diameter measured in millimeters.). 965 * This is the underlying object with id, value and extensions. The 966 * accessor "getDiameter" gives direct access to the value 967 */ 968 public DecimalType getDiameterElement() { 969 if (this.diameter == null) 970 if (Configuration.errorOnAutoCreate()) 971 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.diameter"); 972 else if (Configuration.doAutoCreate()) 973 this.diameter = new DecimalType(); // bb 974 return this.diameter; 975 } 976 977 public boolean hasDiameterElement() { 978 return this.diameter != null && !this.diameter.isEmpty(); 979 } 980 981 public boolean hasDiameter() { 982 return this.diameter != null && !this.diameter.isEmpty(); 983 } 984 985 /** 986 * @param value {@link #diameter} (Contact lens diameter measured in 987 * millimeters.). This is the underlying object with id, value and 988 * extensions. The accessor "getDiameter" gives direct access to 989 * the value 990 */ 991 public VisionPrescriptionDispenseComponent setDiameterElement(DecimalType value) { 992 this.diameter = value; 993 return this; 994 } 995 996 /** 997 * @return Contact lens diameter measured in millimeters. 998 */ 999 public BigDecimal getDiameter() { 1000 return this.diameter == null ? null : this.diameter.getValue(); 1001 } 1002 1003 /** 1004 * @param value Contact lens diameter measured in millimeters. 1005 */ 1006 public VisionPrescriptionDispenseComponent setDiameter(BigDecimal value) { 1007 if (value == null) 1008 this.diameter = null; 1009 else { 1010 if (this.diameter == null) 1011 this.diameter = new DecimalType(); 1012 this.diameter.setValue(value); 1013 } 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #duration} (The recommended maximum wear period for the lens.) 1019 */ 1020 public SimpleQuantity getDuration() { 1021 if (this.duration == null) 1022 if (Configuration.errorOnAutoCreate()) 1023 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.duration"); 1024 else if (Configuration.doAutoCreate()) 1025 this.duration = new SimpleQuantity(); // cc 1026 return this.duration; 1027 } 1028 1029 public boolean hasDuration() { 1030 return this.duration != null && !this.duration.isEmpty(); 1031 } 1032 1033 /** 1034 * @param value {@link #duration} (The recommended maximum wear period for the 1035 * lens.) 1036 */ 1037 public VisionPrescriptionDispenseComponent setDuration(SimpleQuantity value) { 1038 this.duration = value; 1039 return this; 1040 } 1041 1042 /** 1043 * @return {@link #color} (Special color or pattern.). This is the underlying 1044 * object with id, value and extensions. The accessor "getColor" gives 1045 * direct access to the value 1046 */ 1047 public StringType getColorElement() { 1048 if (this.color == null) 1049 if (Configuration.errorOnAutoCreate()) 1050 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.color"); 1051 else if (Configuration.doAutoCreate()) 1052 this.color = new StringType(); // bb 1053 return this.color; 1054 } 1055 1056 public boolean hasColorElement() { 1057 return this.color != null && !this.color.isEmpty(); 1058 } 1059 1060 public boolean hasColor() { 1061 return this.color != null && !this.color.isEmpty(); 1062 } 1063 1064 /** 1065 * @param value {@link #color} (Special color or pattern.). This is the 1066 * underlying object with id, value and extensions. The accessor 1067 * "getColor" gives direct access to the value 1068 */ 1069 public VisionPrescriptionDispenseComponent setColorElement(StringType value) { 1070 this.color = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return Special color or pattern. 1076 */ 1077 public String getColor() { 1078 return this.color == null ? null : this.color.getValue(); 1079 } 1080 1081 /** 1082 * @param value Special color or pattern. 1083 */ 1084 public VisionPrescriptionDispenseComponent setColor(String value) { 1085 if (Utilities.noString(value)) 1086 this.color = null; 1087 else { 1088 if (this.color == null) 1089 this.color = new StringType(); 1090 this.color.setValue(value); 1091 } 1092 return this; 1093 } 1094 1095 /** 1096 * @return {@link #brand} (Brand recommendations or restrictions.). This is the 1097 * underlying object with id, value and extensions. The accessor 1098 * "getBrand" gives direct access to the value 1099 */ 1100 public StringType getBrandElement() { 1101 if (this.brand == null) 1102 if (Configuration.errorOnAutoCreate()) 1103 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.brand"); 1104 else if (Configuration.doAutoCreate()) 1105 this.brand = new StringType(); // bb 1106 return this.brand; 1107 } 1108 1109 public boolean hasBrandElement() { 1110 return this.brand != null && !this.brand.isEmpty(); 1111 } 1112 1113 public boolean hasBrand() { 1114 return this.brand != null && !this.brand.isEmpty(); 1115 } 1116 1117 /** 1118 * @param value {@link #brand} (Brand recommendations or restrictions.). This is 1119 * the underlying object with id, value and extensions. The 1120 * accessor "getBrand" gives direct access to the value 1121 */ 1122 public VisionPrescriptionDispenseComponent setBrandElement(StringType value) { 1123 this.brand = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return Brand recommendations or restrictions. 1129 */ 1130 public String getBrand() { 1131 return this.brand == null ? null : this.brand.getValue(); 1132 } 1133 1134 /** 1135 * @param value Brand recommendations or restrictions. 1136 */ 1137 public VisionPrescriptionDispenseComponent setBrand(String value) { 1138 if (Utilities.noString(value)) 1139 this.brand = null; 1140 else { 1141 if (this.brand == null) 1142 this.brand = new StringType(); 1143 this.brand.setValue(value); 1144 } 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #notes} (Notes for special requirements such as coatings and 1150 * lens materials.). This is the underlying object with id, value and 1151 * extensions. The accessor "getNotes" gives direct access to the value 1152 */ 1153 public StringType getNotesElement() { 1154 if (this.notes == null) 1155 if (Configuration.errorOnAutoCreate()) 1156 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.notes"); 1157 else if (Configuration.doAutoCreate()) 1158 this.notes = new StringType(); // bb 1159 return this.notes; 1160 } 1161 1162 public boolean hasNotesElement() { 1163 return this.notes != null && !this.notes.isEmpty(); 1164 } 1165 1166 public boolean hasNotes() { 1167 return this.notes != null && !this.notes.isEmpty(); 1168 } 1169 1170 /** 1171 * @param value {@link #notes} (Notes for special requirements such as coatings 1172 * and lens materials.). This is the underlying object with id, 1173 * value and extensions. The accessor "getNotes" gives direct 1174 * access to the value 1175 */ 1176 public VisionPrescriptionDispenseComponent setNotesElement(StringType value) { 1177 this.notes = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return Notes for special requirements such as coatings and lens materials. 1183 */ 1184 public String getNotes() { 1185 return this.notes == null ? null : this.notes.getValue(); 1186 } 1187 1188 /** 1189 * @param value Notes for special requirements such as coatings and lens 1190 * materials. 1191 */ 1192 public VisionPrescriptionDispenseComponent setNotes(String value) { 1193 if (Utilities.noString(value)) 1194 this.notes = null; 1195 else { 1196 if (this.notes == null) 1197 this.notes = new StringType(); 1198 this.notes.setValue(value); 1199 } 1200 return this; 1201 } 1202 1203 protected void listChildren(List<Property> childrenList) { 1204 super.listChildren(childrenList); 1205 childrenList.add(new Property("product", "Coding", 1206 "Identifies the type of vision correction product which is required for the patient.", 0, 1207 java.lang.Integer.MAX_VALUE, product)); 1208 childrenList 1209 .add(new Property("eye", "code", "The eye for which the lens applies.", 0, java.lang.Integer.MAX_VALUE, eye)); 1210 childrenList.add(new Property("sphere", "decimal", "Lens power measured in diopters (0.25 units).", 0, 1211 java.lang.Integer.MAX_VALUE, sphere)); 1212 childrenList.add( 1213 new Property("cylinder", "decimal", "Power adjustment for astigmatism measured in diopters (0.25 units).", 0, 1214 java.lang.Integer.MAX_VALUE, cylinder)); 1215 childrenList.add(new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1216 java.lang.Integer.MAX_VALUE, axis)); 1217 childrenList 1218 .add(new Property("prism", "decimal", "Amount of prism to compensate for eye alignment in fractional units.", 1219 0, java.lang.Integer.MAX_VALUE, prism)); 1220 childrenList.add(new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1221 java.lang.Integer.MAX_VALUE, base)); 1222 childrenList.add( 1223 new Property("add", "decimal", "Power adjustment for multifocal lenses measured in diopters (0.25 units).", 0, 1224 java.lang.Integer.MAX_VALUE, add)); 1225 childrenList.add(new Property("power", "decimal", "Contact lens power measured in diopters (0.25 units).", 0, 1226 java.lang.Integer.MAX_VALUE, power)); 1227 childrenList.add(new Property("backCurve", "decimal", "Back curvature measured in millimeters.", 0, 1228 java.lang.Integer.MAX_VALUE, backCurve)); 1229 childrenList.add(new Property("diameter", "decimal", "Contact lens diameter measured in millimeters.", 0, 1230 java.lang.Integer.MAX_VALUE, diameter)); 1231 childrenList.add(new Property("duration", "SimpleQuantity", "The recommended maximum wear period for the lens.", 1232 0, java.lang.Integer.MAX_VALUE, duration)); 1233 childrenList 1234 .add(new Property("color", "string", "Special color or pattern.", 0, java.lang.Integer.MAX_VALUE, color)); 1235 childrenList.add(new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1236 java.lang.Integer.MAX_VALUE, brand)); 1237 childrenList 1238 .add(new Property("notes", "string", "Notes for special requirements such as coatings and lens materials.", 0, 1239 java.lang.Integer.MAX_VALUE, notes)); 1240 } 1241 1242 @Override 1243 public void setProperty(String name, Base value) throws FHIRException { 1244 if (name.equals("product")) 1245 this.product = castToCoding(value); // Coding 1246 else if (name.equals("eye")) 1247 this.eye = new VisionEyesEnumFactory().fromType(value); // Enumeration<VisionEyes> 1248 else if (name.equals("sphere")) 1249 this.sphere = castToDecimal(value); // DecimalType 1250 else if (name.equals("cylinder")) 1251 this.cylinder = castToDecimal(value); // DecimalType 1252 else if (name.equals("axis")) 1253 this.axis = castToInteger(value); // IntegerType 1254 else if (name.equals("prism")) 1255 this.prism = castToDecimal(value); // DecimalType 1256 else if (name.equals("base")) 1257 this.base = new VisionBaseEnumFactory().fromType(value); // Enumeration<VisionBase> 1258 else if (name.equals("add")) 1259 this.add = castToDecimal(value); // DecimalType 1260 else if (name.equals("power")) 1261 this.power = castToDecimal(value); // DecimalType 1262 else if (name.equals("backCurve")) 1263 this.backCurve = castToDecimal(value); // DecimalType 1264 else if (name.equals("diameter")) 1265 this.diameter = castToDecimal(value); // DecimalType 1266 else if (name.equals("duration")) 1267 this.duration = castToSimpleQuantity(value); // SimpleQuantity 1268 else if (name.equals("color")) 1269 this.color = castToString(value); // StringType 1270 else if (name.equals("brand")) 1271 this.brand = castToString(value); // StringType 1272 else if (name.equals("notes")) 1273 this.notes = castToString(value); // StringType 1274 else 1275 super.setProperty(name, value); 1276 } 1277 1278 @Override 1279 public Base addChild(String name) throws FHIRException { 1280 if (name.equals("product")) { 1281 this.product = new Coding(); 1282 return this.product; 1283 } else if (name.equals("eye")) { 1284 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.eye"); 1285 } else if (name.equals("sphere")) { 1286 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.sphere"); 1287 } else if (name.equals("cylinder")) { 1288 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.cylinder"); 1289 } else if (name.equals("axis")) { 1290 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.axis"); 1291 } else if (name.equals("prism")) { 1292 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.prism"); 1293 } else if (name.equals("base")) { 1294 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.base"); 1295 } else if (name.equals("add")) { 1296 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.add"); 1297 } else if (name.equals("power")) { 1298 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.power"); 1299 } else if (name.equals("backCurve")) { 1300 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.backCurve"); 1301 } else if (name.equals("diameter")) { 1302 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.diameter"); 1303 } else if (name.equals("duration")) { 1304 this.duration = new SimpleQuantity(); 1305 return this.duration; 1306 } else if (name.equals("color")) { 1307 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.color"); 1308 } else if (name.equals("brand")) { 1309 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.brand"); 1310 } else if (name.equals("notes")) { 1311 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.notes"); 1312 } else 1313 return super.addChild(name); 1314 } 1315 1316 public VisionPrescriptionDispenseComponent copy() { 1317 VisionPrescriptionDispenseComponent dst = new VisionPrescriptionDispenseComponent(); 1318 copyValues(dst); 1319 dst.product = product == null ? null : product.copy(); 1320 dst.eye = eye == null ? null : eye.copy(); 1321 dst.sphere = sphere == null ? null : sphere.copy(); 1322 dst.cylinder = cylinder == null ? null : cylinder.copy(); 1323 dst.axis = axis == null ? null : axis.copy(); 1324 dst.prism = prism == null ? null : prism.copy(); 1325 dst.base = base == null ? null : base.copy(); 1326 dst.add = add == null ? null : add.copy(); 1327 dst.power = power == null ? null : power.copy(); 1328 dst.backCurve = backCurve == null ? null : backCurve.copy(); 1329 dst.diameter = diameter == null ? null : diameter.copy(); 1330 dst.duration = duration == null ? null : duration.copy(); 1331 dst.color = color == null ? null : color.copy(); 1332 dst.brand = brand == null ? null : brand.copy(); 1333 dst.notes = notes == null ? null : notes.copy(); 1334 return dst; 1335 } 1336 1337 @Override 1338 public boolean equalsDeep(Base other) { 1339 if (!super.equalsDeep(other)) 1340 return false; 1341 if (!(other instanceof VisionPrescriptionDispenseComponent)) 1342 return false; 1343 VisionPrescriptionDispenseComponent o = (VisionPrescriptionDispenseComponent) other; 1344 return compareDeep(product, o.product, true) && compareDeep(eye, o.eye, true) 1345 && compareDeep(sphere, o.sphere, true) && compareDeep(cylinder, o.cylinder, true) 1346 && compareDeep(axis, o.axis, true) && compareDeep(prism, o.prism, true) && compareDeep(base, o.base, true) 1347 && compareDeep(add, o.add, true) && compareDeep(power, o.power, true) 1348 && compareDeep(backCurve, o.backCurve, true) && compareDeep(diameter, o.diameter, true) 1349 && compareDeep(duration, o.duration, true) && compareDeep(color, o.color, true) 1350 && compareDeep(brand, o.brand, true) && compareDeep(notes, o.notes, true); 1351 } 1352 1353 @Override 1354 public boolean equalsShallow(Base other) { 1355 if (!super.equalsShallow(other)) 1356 return false; 1357 if (!(other instanceof VisionPrescriptionDispenseComponent)) 1358 return false; 1359 VisionPrescriptionDispenseComponent o = (VisionPrescriptionDispenseComponent) other; 1360 return compareValues(eye, o.eye, true) && compareValues(sphere, o.sphere, true) 1361 && compareValues(cylinder, o.cylinder, true) && compareValues(axis, o.axis, true) 1362 && compareValues(prism, o.prism, true) && compareValues(base, o.base, true) && compareValues(add, o.add, true) 1363 && compareValues(power, o.power, true) && compareValues(backCurve, o.backCurve, true) 1364 && compareValues(diameter, o.diameter, true) && compareValues(color, o.color, true) 1365 && compareValues(brand, o.brand, true) && compareValues(notes, o.notes, true); 1366 } 1367 1368 public boolean isEmpty() { 1369 return super.isEmpty() && (product == null || product.isEmpty()) && (eye == null || eye.isEmpty()) 1370 && (sphere == null || sphere.isEmpty()) && (cylinder == null || cylinder.isEmpty()) 1371 && (axis == null || axis.isEmpty()) && (prism == null || prism.isEmpty()) && (base == null || base.isEmpty()) 1372 && (add == null || add.isEmpty()) && (power == null || power.isEmpty()) 1373 && (backCurve == null || backCurve.isEmpty()) && (diameter == null || diameter.isEmpty()) 1374 && (duration == null || duration.isEmpty()) && (color == null || color.isEmpty()) 1375 && (brand == null || brand.isEmpty()) && (notes == null || notes.isEmpty()); 1376 } 1377 1378 public String fhirType() { 1379 return "VisionPrescription.dispense"; 1380 1381 } 1382 1383 } 1384 1385 /** 1386 * Business identifier which may be used by other parties to reference or 1387 * identify the prescription. 1388 */ 1389 @Child(name = "identifier", type = { 1390 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1391 @Description(shortDefinition = "Business identifier", formalDefinition = "Business identifier which may be used by other parties to reference or identify the prescription.") 1392 protected List<Identifier> identifier; 1393 1394 /** 1395 * The date (and perhaps time) when the prescription was written. 1396 */ 1397 @Child(name = "dateWritten", type = { 1398 DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1399 @Description(shortDefinition = "When prescription was authorized", formalDefinition = "The date (and perhaps time) when the prescription was written.") 1400 protected DateTimeType dateWritten; 1401 1402 /** 1403 * A link to a resource representing the person to whom the vision products will 1404 * be supplied. 1405 */ 1406 @Child(name = "patient", type = { Patient.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1407 @Description(shortDefinition = "Who prescription is for", formalDefinition = "A link to a resource representing the person to whom the vision products will be supplied.") 1408 protected Reference patient; 1409 1410 /** 1411 * The actual object that is the target of the reference (A link to a resource 1412 * representing the person to whom the vision products will be supplied.) 1413 */ 1414 protected Patient patientTarget; 1415 1416 /** 1417 * The healthcare professional responsible for authorizing the prescription. 1418 */ 1419 @Child(name = "prescriber", type = { 1420 Practitioner.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1421 @Description(shortDefinition = "Who authorizes the vision product", formalDefinition = "The healthcare professional responsible for authorizing the prescription.") 1422 protected Reference prescriber; 1423 1424 /** 1425 * The actual object that is the target of the reference (The healthcare 1426 * professional responsible for authorizing the prescription.) 1427 */ 1428 protected Practitioner prescriberTarget; 1429 1430 /** 1431 * A link to a resource that identifies the particular occurrence of contact 1432 * between patient and health care provider. 1433 */ 1434 @Child(name = "encounter", type = { Encounter.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1435 @Description(shortDefinition = "Created during encounter / admission / stay", formalDefinition = "A link to a resource that identifies the particular occurrence of contact between patient and health care provider.") 1436 protected Reference encounter; 1437 1438 /** 1439 * The actual object that is the target of the reference (A link to a resource 1440 * that identifies the particular occurrence of contact between patient and 1441 * health care provider.) 1442 */ 1443 protected Encounter encounterTarget; 1444 1445 /** 1446 * Can be the reason or the indication for writing the prescription. 1447 */ 1448 @Child(name = "reason", type = { CodeableConcept.class, 1449 Condition.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1450 @Description(shortDefinition = "Reason or indication for writing the prescription", formalDefinition = "Can be the reason or the indication for writing the prescription.") 1451 protected Type reason; 1452 1453 /** 1454 * Deals with details of the dispense part of the supply specification. 1455 */ 1456 @Child(name = "dispense", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1457 @Description(shortDefinition = "Vision supply authorization", formalDefinition = "Deals with details of the dispense part of the supply specification.") 1458 protected List<VisionPrescriptionDispenseComponent> dispense; 1459 1460 private static final long serialVersionUID = -1108276057L; 1461 1462 /* 1463 * Constructor 1464 */ 1465 public VisionPrescription() { 1466 super(); 1467 } 1468 1469 /** 1470 * @return {@link #identifier} (Business identifier which may be used by other 1471 * parties to reference or identify the prescription.) 1472 */ 1473 public List<Identifier> getIdentifier() { 1474 if (this.identifier == null) 1475 this.identifier = new ArrayList<Identifier>(); 1476 return this.identifier; 1477 } 1478 1479 public boolean hasIdentifier() { 1480 if (this.identifier == null) 1481 return false; 1482 for (Identifier item : this.identifier) 1483 if (!item.isEmpty()) 1484 return true; 1485 return false; 1486 } 1487 1488 /** 1489 * @return {@link #identifier} (Business identifier which may be used by other 1490 * parties to reference or identify the prescription.) 1491 */ 1492 // syntactic sugar 1493 public Identifier addIdentifier() { // 3 1494 Identifier t = new Identifier(); 1495 if (this.identifier == null) 1496 this.identifier = new ArrayList<Identifier>(); 1497 this.identifier.add(t); 1498 return t; 1499 } 1500 1501 // syntactic sugar 1502 public VisionPrescription addIdentifier(Identifier t) { // 3 1503 if (t == null) 1504 return this; 1505 if (this.identifier == null) 1506 this.identifier = new ArrayList<Identifier>(); 1507 this.identifier.add(t); 1508 return this; 1509 } 1510 1511 /** 1512 * @return {@link #dateWritten} (The date (and perhaps time) when the 1513 * prescription was written.). This is the underlying object with id, 1514 * value and extensions. The accessor "getDateWritten" gives direct 1515 * access to the value 1516 */ 1517 public DateTimeType getDateWrittenElement() { 1518 if (this.dateWritten == null) 1519 if (Configuration.errorOnAutoCreate()) 1520 throw new Error("Attempt to auto-create VisionPrescription.dateWritten"); 1521 else if (Configuration.doAutoCreate()) 1522 this.dateWritten = new DateTimeType(); // bb 1523 return this.dateWritten; 1524 } 1525 1526 public boolean hasDateWrittenElement() { 1527 return this.dateWritten != null && !this.dateWritten.isEmpty(); 1528 } 1529 1530 public boolean hasDateWritten() { 1531 return this.dateWritten != null && !this.dateWritten.isEmpty(); 1532 } 1533 1534 /** 1535 * @param value {@link #dateWritten} (The date (and perhaps time) when the 1536 * prescription was written.). This is the underlying object with 1537 * id, value and extensions. The accessor "getDateWritten" gives 1538 * direct access to the value 1539 */ 1540 public VisionPrescription setDateWrittenElement(DateTimeType value) { 1541 this.dateWritten = value; 1542 return this; 1543 } 1544 1545 /** 1546 * @return The date (and perhaps time) when the prescription was written. 1547 */ 1548 public Date getDateWritten() { 1549 return this.dateWritten == null ? null : this.dateWritten.getValue(); 1550 } 1551 1552 /** 1553 * @param value The date (and perhaps time) when the prescription was written. 1554 */ 1555 public VisionPrescription setDateWritten(Date value) { 1556 if (value == null) 1557 this.dateWritten = null; 1558 else { 1559 if (this.dateWritten == null) 1560 this.dateWritten = new DateTimeType(); 1561 this.dateWritten.setValue(value); 1562 } 1563 return this; 1564 } 1565 1566 /** 1567 * @return {@link #patient} (A link to a resource representing the person to 1568 * whom the vision products will be supplied.) 1569 */ 1570 public Reference getPatient() { 1571 if (this.patient == null) 1572 if (Configuration.errorOnAutoCreate()) 1573 throw new Error("Attempt to auto-create VisionPrescription.patient"); 1574 else if (Configuration.doAutoCreate()) 1575 this.patient = new Reference(); // cc 1576 return this.patient; 1577 } 1578 1579 public boolean hasPatient() { 1580 return this.patient != null && !this.patient.isEmpty(); 1581 } 1582 1583 /** 1584 * @param value {@link #patient} (A link to a resource representing the person 1585 * to whom the vision products will be supplied.) 1586 */ 1587 public VisionPrescription setPatient(Reference value) { 1588 this.patient = value; 1589 return this; 1590 } 1591 1592 /** 1593 * @return {@link #patient} The actual object that is the target of the 1594 * reference. The reference library doesn't populate this, but you can 1595 * use it to hold the resource if you resolve it. (A link to a resource 1596 * representing the person to whom the vision products will be 1597 * supplied.) 1598 */ 1599 public Patient getPatientTarget() { 1600 if (this.patientTarget == null) 1601 if (Configuration.errorOnAutoCreate()) 1602 throw new Error("Attempt to auto-create VisionPrescription.patient"); 1603 else if (Configuration.doAutoCreate()) 1604 this.patientTarget = new Patient(); // aa 1605 return this.patientTarget; 1606 } 1607 1608 /** 1609 * @param value {@link #patient} The actual object that is the target of the 1610 * reference. The reference library doesn't use these, but you can 1611 * use it to hold the resource if you resolve it. (A link to a 1612 * resource representing the person to whom the vision products 1613 * will be supplied.) 1614 */ 1615 public VisionPrescription setPatientTarget(Patient value) { 1616 this.patientTarget = value; 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #prescriber} (The healthcare professional responsible for 1622 * authorizing the prescription.) 1623 */ 1624 public Reference getPrescriber() { 1625 if (this.prescriber == null) 1626 if (Configuration.errorOnAutoCreate()) 1627 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 1628 else if (Configuration.doAutoCreate()) 1629 this.prescriber = new Reference(); // cc 1630 return this.prescriber; 1631 } 1632 1633 public boolean hasPrescriber() { 1634 return this.prescriber != null && !this.prescriber.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #prescriber} (The healthcare professional responsible for 1639 * authorizing the prescription.) 1640 */ 1641 public VisionPrescription setPrescriber(Reference value) { 1642 this.prescriber = value; 1643 return this; 1644 } 1645 1646 /** 1647 * @return {@link #prescriber} The actual object that is the target of the 1648 * reference. The reference library doesn't populate this, but you can 1649 * use it to hold the resource if you resolve it. (The healthcare 1650 * professional responsible for authorizing the prescription.) 1651 */ 1652 public Practitioner getPrescriberTarget() { 1653 if (this.prescriberTarget == null) 1654 if (Configuration.errorOnAutoCreate()) 1655 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 1656 else if (Configuration.doAutoCreate()) 1657 this.prescriberTarget = new Practitioner(); // aa 1658 return this.prescriberTarget; 1659 } 1660 1661 /** 1662 * @param value {@link #prescriber} The actual object that is the target of the 1663 * reference. The reference library doesn't use these, but you can 1664 * use it to hold the resource if you resolve it. (The healthcare 1665 * professional responsible for authorizing the prescription.) 1666 */ 1667 public VisionPrescription setPrescriberTarget(Practitioner value) { 1668 this.prescriberTarget = value; 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #encounter} (A link to a resource that identifies the 1674 * particular occurrence of contact between patient and health care 1675 * provider.) 1676 */ 1677 public Reference getEncounter() { 1678 if (this.encounter == null) 1679 if (Configuration.errorOnAutoCreate()) 1680 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 1681 else if (Configuration.doAutoCreate()) 1682 this.encounter = new Reference(); // cc 1683 return this.encounter; 1684 } 1685 1686 public boolean hasEncounter() { 1687 return this.encounter != null && !this.encounter.isEmpty(); 1688 } 1689 1690 /** 1691 * @param value {@link #encounter} (A link to a resource that identifies the 1692 * particular occurrence of contact between patient and health care 1693 * provider.) 1694 */ 1695 public VisionPrescription setEncounter(Reference value) { 1696 this.encounter = value; 1697 return this; 1698 } 1699 1700 /** 1701 * @return {@link #encounter} The actual object that is the target of the 1702 * reference. The reference library doesn't populate this, but you can 1703 * use it to hold the resource if you resolve it. (A link to a resource 1704 * that identifies the particular occurrence of contact between patient 1705 * and health care provider.) 1706 */ 1707 public Encounter getEncounterTarget() { 1708 if (this.encounterTarget == null) 1709 if (Configuration.errorOnAutoCreate()) 1710 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 1711 else if (Configuration.doAutoCreate()) 1712 this.encounterTarget = new Encounter(); // aa 1713 return this.encounterTarget; 1714 } 1715 1716 /** 1717 * @param value {@link #encounter} The actual object that is the target of the 1718 * reference. The reference library doesn't use these, but you can 1719 * use it to hold the resource if you resolve it. (A link to a 1720 * resource that identifies the particular occurrence of contact 1721 * between patient and health care provider.) 1722 */ 1723 public VisionPrescription setEncounterTarget(Encounter value) { 1724 this.encounterTarget = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return {@link #reason} (Can be the reason or the indication for writing the 1730 * prescription.) 1731 */ 1732 public Type getReason() { 1733 return this.reason; 1734 } 1735 1736 /** 1737 * @return {@link #reason} (Can be the reason or the indication for writing the 1738 * prescription.) 1739 */ 1740 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 1741 if (!(this.reason instanceof CodeableConcept)) 1742 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1743 + this.reason.getClass().getName() + " was encountered"); 1744 return (CodeableConcept) this.reason; 1745 } 1746 1747 public boolean hasReasonCodeableConcept() { 1748 return this.reason instanceof CodeableConcept; 1749 } 1750 1751 /** 1752 * @return {@link #reason} (Can be the reason or the indication for writing the 1753 * prescription.) 1754 */ 1755 public Reference getReasonReference() throws FHIRException { 1756 if (!(this.reason instanceof Reference)) 1757 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.reason.getClass().getName() 1758 + " was encountered"); 1759 return (Reference) this.reason; 1760 } 1761 1762 public boolean hasReasonReference() { 1763 return this.reason instanceof Reference; 1764 } 1765 1766 public boolean hasReason() { 1767 return this.reason != null && !this.reason.isEmpty(); 1768 } 1769 1770 /** 1771 * @param value {@link #reason} (Can be the reason or the indication for writing 1772 * the prescription.) 1773 */ 1774 public VisionPrescription setReason(Type value) { 1775 this.reason = value; 1776 return this; 1777 } 1778 1779 /** 1780 * @return {@link #dispense} (Deals with details of the dispense part of the 1781 * supply specification.) 1782 */ 1783 public List<VisionPrescriptionDispenseComponent> getDispense() { 1784 if (this.dispense == null) 1785 this.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 1786 return this.dispense; 1787 } 1788 1789 public boolean hasDispense() { 1790 if (this.dispense == null) 1791 return false; 1792 for (VisionPrescriptionDispenseComponent item : this.dispense) 1793 if (!item.isEmpty()) 1794 return true; 1795 return false; 1796 } 1797 1798 /** 1799 * @return {@link #dispense} (Deals with details of the dispense part of the 1800 * supply specification.) 1801 */ 1802 // syntactic sugar 1803 public VisionPrescriptionDispenseComponent addDispense() { // 3 1804 VisionPrescriptionDispenseComponent t = new VisionPrescriptionDispenseComponent(); 1805 if (this.dispense == null) 1806 this.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 1807 this.dispense.add(t); 1808 return t; 1809 } 1810 1811 // syntactic sugar 1812 public VisionPrescription addDispense(VisionPrescriptionDispenseComponent t) { // 3 1813 if (t == null) 1814 return this; 1815 if (this.dispense == null) 1816 this.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 1817 this.dispense.add(t); 1818 return this; 1819 } 1820 1821 protected void listChildren(List<Property> childrenList) { 1822 super.listChildren(childrenList); 1823 childrenList.add(new Property("identifier", "Identifier", 1824 "Business identifier which may be used by other parties to reference or identify the prescription.", 0, 1825 java.lang.Integer.MAX_VALUE, identifier)); 1826 childrenList.add(new Property("dateWritten", "dateTime", 1827 "The date (and perhaps time) when the prescription was written.", 0, java.lang.Integer.MAX_VALUE, dateWritten)); 1828 childrenList.add(new Property("patient", "Reference(Patient)", 1829 "A link to a resource representing the person to whom the vision products will be supplied.", 0, 1830 java.lang.Integer.MAX_VALUE, patient)); 1831 childrenList.add(new Property("prescriber", "Reference(Practitioner)", 1832 "The healthcare professional responsible for authorizing the prescription.", 0, java.lang.Integer.MAX_VALUE, 1833 prescriber)); 1834 childrenList.add(new Property("encounter", "Reference(Encounter)", 1835 "A link to a resource that identifies the particular occurrence of contact between patient and health care provider.", 1836 0, java.lang.Integer.MAX_VALUE, encounter)); 1837 childrenList.add(new Property("reason[x]", "CodeableConcept|Reference(Condition)", 1838 "Can be the reason or the indication for writing the prescription.", 0, java.lang.Integer.MAX_VALUE, reason)); 1839 childrenList 1840 .add(new Property("dispense", "", "Deals with details of the dispense part of the supply specification.", 0, 1841 java.lang.Integer.MAX_VALUE, dispense)); 1842 } 1843 1844 @Override 1845 public void setProperty(String name, Base value) throws FHIRException { 1846 if (name.equals("identifier")) 1847 this.getIdentifier().add(castToIdentifier(value)); 1848 else if (name.equals("dateWritten")) 1849 this.dateWritten = castToDateTime(value); // DateTimeType 1850 else if (name.equals("patient")) 1851 this.patient = castToReference(value); // Reference 1852 else if (name.equals("prescriber")) 1853 this.prescriber = castToReference(value); // Reference 1854 else if (name.equals("encounter")) 1855 this.encounter = castToReference(value); // Reference 1856 else if (name.equals("reason[x]")) 1857 this.reason = (Type) value; // Type 1858 else if (name.equals("dispense")) 1859 this.getDispense().add((VisionPrescriptionDispenseComponent) value); 1860 else 1861 super.setProperty(name, value); 1862 } 1863 1864 @Override 1865 public Base addChild(String name) throws FHIRException { 1866 if (name.equals("identifier")) { 1867 return addIdentifier(); 1868 } else if (name.equals("dateWritten")) { 1869 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.dateWritten"); 1870 } else if (name.equals("patient")) { 1871 this.patient = new Reference(); 1872 return this.patient; 1873 } else if (name.equals("prescriber")) { 1874 this.prescriber = new Reference(); 1875 return this.prescriber; 1876 } else if (name.equals("encounter")) { 1877 this.encounter = new Reference(); 1878 return this.encounter; 1879 } else if (name.equals("reasonCodeableConcept")) { 1880 this.reason = new CodeableConcept(); 1881 return this.reason; 1882 } else if (name.equals("reasonReference")) { 1883 this.reason = new Reference(); 1884 return this.reason; 1885 } else if (name.equals("dispense")) { 1886 return addDispense(); 1887 } else 1888 return super.addChild(name); 1889 } 1890 1891 public String fhirType() { 1892 return "VisionPrescription"; 1893 1894 } 1895 1896 public VisionPrescription copy() { 1897 VisionPrescription dst = new VisionPrescription(); 1898 copyValues(dst); 1899 if (identifier != null) { 1900 dst.identifier = new ArrayList<Identifier>(); 1901 for (Identifier i : identifier) 1902 dst.identifier.add(i.copy()); 1903 } 1904 ; 1905 dst.dateWritten = dateWritten == null ? null : dateWritten.copy(); 1906 dst.patient = patient == null ? null : patient.copy(); 1907 dst.prescriber = prescriber == null ? null : prescriber.copy(); 1908 dst.encounter = encounter == null ? null : encounter.copy(); 1909 dst.reason = reason == null ? null : reason.copy(); 1910 if (dispense != null) { 1911 dst.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 1912 for (VisionPrescriptionDispenseComponent i : dispense) 1913 dst.dispense.add(i.copy()); 1914 } 1915 ; 1916 return dst; 1917 } 1918 1919 protected VisionPrescription typedCopy() { 1920 return copy(); 1921 } 1922 1923 @Override 1924 public boolean equalsDeep(Base other) { 1925 if (!super.equalsDeep(other)) 1926 return false; 1927 if (!(other instanceof VisionPrescription)) 1928 return false; 1929 VisionPrescription o = (VisionPrescription) other; 1930 return compareDeep(identifier, o.identifier, true) && compareDeep(dateWritten, o.dateWritten, true) 1931 && compareDeep(patient, o.patient, true) && compareDeep(prescriber, o.prescriber, true) 1932 && compareDeep(encounter, o.encounter, true) && compareDeep(reason, o.reason, true) 1933 && compareDeep(dispense, o.dispense, true); 1934 } 1935 1936 @Override 1937 public boolean equalsShallow(Base other) { 1938 if (!super.equalsShallow(other)) 1939 return false; 1940 if (!(other instanceof VisionPrescription)) 1941 return false; 1942 VisionPrescription o = (VisionPrescription) other; 1943 return compareValues(dateWritten, o.dateWritten, true); 1944 } 1945 1946 public boolean isEmpty() { 1947 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 1948 && (dateWritten == null || dateWritten.isEmpty()) && (patient == null || patient.isEmpty()) 1949 && (prescriber == null || prescriber.isEmpty()) && (encounter == null || encounter.isEmpty()) 1950 && (reason == null || reason.isEmpty()) && (dispense == null || dispense.isEmpty()); 1951 } 1952 1953 @Override 1954 public ResourceType getResourceType() { 1955 return ResourceType.VisionPrescription; 1956 } 1957 1958 @SearchParamDefinition(name = "prescriber", path = "VisionPrescription.prescriber", description = "Who authorizes the vision product", type = "reference") 1959 public static final String SP_PRESCRIBER = "prescriber"; 1960 @SearchParamDefinition(name = "identifier", path = "VisionPrescription.identifier", description = "Return prescriptions with this external identifier", type = "token") 1961 public static final String SP_IDENTIFIER = "identifier"; 1962 @SearchParamDefinition(name = "patient", path = "VisionPrescription.patient", description = "The identity of a patient to list dispenses for", type = "reference") 1963 public static final String SP_PATIENT = "patient"; 1964 @SearchParamDefinition(name = "datewritten", path = "VisionPrescription.dateWritten", description = "Return prescriptions written on this date", type = "date") 1965 public static final String SP_DATEWRITTEN = "datewritten"; 1966 @SearchParamDefinition(name = "encounter", path = "VisionPrescription.encounter", description = "Return prescriptions with this encounter identifier", type = "reference") 1967 public static final String SP_ENCOUNTER = "encounter"; 1968 1969}