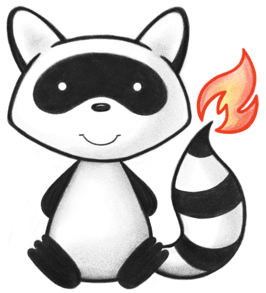
001package org.hl7.fhir.dstu2.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.List; 033 034import org.hl7.fhir.dstu2.model.UriType; 035import org.hl7.fhir.dstu2.model.ValueSet; 036import org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent; 037import org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent; 038import org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent; 039import org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent; 040import org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent; 041import org.hl7.fhir.dstu2.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 042import org.hl7.fhir.dstu2.utils.EOperationOutcome; 043import org.hl7.fhir.dstu2.utils.IWorkerContext; 044import org.hl7.fhir.dstu2.utils.IWorkerContext.ValidationResult; 045 046@Deprecated 047public class ValueSetCheckerSimple implements ValueSetChecker { 048 049 private ValueSet valueset; 050 private ValueSetExpanderFactory factory; 051 private IWorkerContext context; 052 053 public ValueSetCheckerSimple(ValueSet source, ValueSetExpanderFactory factory, IWorkerContext context) { 054 this.valueset = source; 055 this.factory = factory; 056 this.context = context; 057 } 058 059 @Override 060 public boolean codeInValueSet(String system, String code) throws EOperationOutcome, Exception { 061 if (valueset.hasCodeSystem() && system.equals(valueset.getCodeSystem().getSystem()) 062 && codeInDefine(valueset.getCodeSystem().getConcept(), code, valueset.getCodeSystem().getCaseSensitive())) 063 return true; 064 065 if (valueset.hasCompose()) { 066 boolean ok = false; 067 for (UriType uri : valueset.getCompose().getImport()) { 068 ok = ok || inImport(uri.getValue(), system, code); 069 } 070 for (ConceptSetComponent vsi : valueset.getCompose().getInclude()) { 071 ok = ok || inComponent(vsi, system, code); 072 } 073 for (ConceptSetComponent vsi : valueset.getCompose().getExclude()) { 074 ok = ok && !inComponent(vsi, system, code); 075 } 076 } 077 078 return false; 079 } 080 081 private boolean inImport(String uri, String system, String code) throws EOperationOutcome, Exception { 082 ValueSet vs = context.fetchResource(ValueSet.class, uri); 083 if (vs == null) 084 return false; // we can't tell 085 return codeInExpansion(factory.getExpander().expand(vs), system, code); 086 } 087 088 private boolean codeInExpansion(ValueSetExpansionOutcome vso, String system, String code) 089 throws EOperationOutcome, Exception { 090 if (vso.getService() != null) { 091 return vso.getService().codeInValueSet(system, code); 092 } else { 093 for (ValueSetExpansionContainsComponent c : vso.getValueset().getExpansion().getContains()) { 094 if (code.equals(c.getCode()) && (system == null || system.equals(c.getSystem()))) 095 return true; 096 if (codeinExpansion(c, system, code)) 097 return true; 098 } 099 } 100 return false; 101 } 102 103 private boolean codeinExpansion(ValueSetExpansionContainsComponent cnt, String system, String code) { 104 for (ValueSetExpansionContainsComponent c : cnt.getContains()) { 105 if (code.equals(c.getCode()) && system.equals(c.getSystem().toString())) 106 return true; 107 if (codeinExpansion(c, system, code)) 108 return true; 109 } 110 return false; 111 } 112 113 private boolean inComponent(ConceptSetComponent vsi, String system, String code) { 114 if (!vsi.getSystem().equals(system)) 115 return false; 116 // whether we know the system or not, we'll accept the stated codes at face 117 // value 118 for (ConceptReferenceComponent cc : vsi.getConcept()) 119 if (cc.getCode().equals(code)) { 120 return true; 121 } 122 123 ValueSet def = context.fetchCodeSystem(system); 124 if (def != null) { 125 if (!def.getCodeSystem().getCaseSensitive()) { 126 // well, ok, it's not case sensitive - we'll check that too now 127 for (ConceptReferenceComponent cc : vsi.getConcept()) 128 if (cc.getCode().equalsIgnoreCase(code)) { 129 return false; 130 } 131 } 132 if (vsi.getConcept().isEmpty() && vsi.getFilter().isEmpty()) { 133 return codeInDefine(def.getCodeSystem().getConcept(), code, def.getCodeSystem().getCaseSensitive()); 134 } 135 for (ConceptSetFilterComponent f : vsi.getFilter()) 136 throw new Error("not done yet: " + f.getValue()); 137 138 return false; 139 } else if (context.supportsSystem(system)) { 140 ValidationResult vv = context.validateCode(system, code, null, vsi); 141 return vv.isOk(); 142 } else 143 // we don't know this system, and can't resolve it 144 return false; 145 } 146 147 private boolean codeInDefine(List<ConceptDefinitionComponent> concepts, String code, boolean caseSensitive) { 148 for (ConceptDefinitionComponent c : concepts) { 149 if (caseSensitive && code.equals(c.getCode())) 150 return true; 151 if (!caseSensitive && code.equalsIgnoreCase(c.getCode())) 152 return true; 153 if (codeInDefine(c.getConcept(), code, caseSensitive)) 154 return true; 155 } 156 return false; 157 } 158 159}