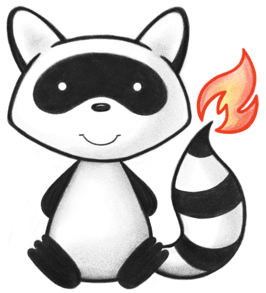
001package org.hl7.fhir.dstu2.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.List; 033 034import java.util.Locale; 035import org.hl7.fhir.dstu2.formats.IParser; 036import org.hl7.fhir.dstu2.formats.ParserType; 037import org.hl7.fhir.dstu2.model.CodeableConcept; 038import org.hl7.fhir.dstu2.model.Coding; 039import org.hl7.fhir.dstu2.model.ConceptMap; 040import org.hl7.fhir.dstu2.model.Resource; 041import org.hl7.fhir.dstu2.model.StructureDefinition; 042import org.hl7.fhir.dstu2.model.ValueSet; 043import org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent; 044import org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent; 045import org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent; 046import org.hl7.fhir.dstu2.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 047import org.hl7.fhir.dstu2.utils.validation.IResourceValidator; 048import org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity; 049 050/** 051 * This is the standard interface used for access to underlying FHIR services 052 * through the tools and utilities provided by the reference implementation. 053 * 054 * The functionality it provides is - get access to parsers, validators, 055 * narrative builders etc (you can't create these directly because they need 056 * access to the right context for their information) 057 * 058 * - find resources that the tools need to carry out their tasks 059 * 060 * - provide access to terminology services they need. (typically, these 061 * terminology service requests are just passed through to the local 062 * implementation's terminology service) 063 * 064 * @author Grahame 065 */ 066public interface IWorkerContext { 067 068 // -- Parsers (read and write instances) 069 // ---------------------------------------- 070 071 /** 072 * Get a parser to read/write instances. Use the defined type (will be extended 073 * as further types are added, though the only currently anticipate type is RDF) 074 * 075 * XML/JSON - the standard renderers XHTML - render the narrative only (generate 076 * it if necessary) 077 * 078 * @param type 079 * @return 080 */ 081 public IParser getParser(ParserType type); 082 083 /** 084 * Get a parser to read/write instances. Determine the type from the stated 085 * type. Supported value for type: - the recommended MIME types - variants of 086 * application/xml and application/json - _format values xml, json 087 * 088 * @param type 089 * @return 090 */ 091 public IParser getParser(String type); 092 093 /** 094 * Get a JSON parser 095 * 096 * @return 097 */ 098 public IParser newJsonParser(); 099 100 /** 101 * Get an XML parser 102 * 103 * @return 104 */ 105 public IParser newXmlParser(); 106 107 /** 108 * Get a generator that can generate narrative for the instance 109 * 110 * @return a prepared generator 111 */ 112 public INarrativeGenerator getNarrativeGenerator(String prefix, String basePath); 113 114 /** 115 * Get a validator that can check whether a resource is valid 116 * 117 * @return a prepared generator @ 118 */ 119 public IResourceValidator newValidator(); 120 121 // -- resource fetchers --------------------------------------------------- 122 123 /** 124 * Find an identified resource. The most common use of this is to access the the 125 * standard conformance resources that are part of the standard - structure 126 * definitions, value sets, concept maps, etc. 127 * 128 * Also, the narrative generator uses this, and may access any kind of resource 129 * 130 * The URI is called speculatively for things that might exist, so not finding a 131 * matching resouce, return null, not an error 132 * 133 * The URI can have one of 3 formats: - a full URL e.g. 134 * http://acme.org/fhir/ValueSet/[id] - a relative URL e.g. ValueSet/[id] - a 135 * logical id e.g. [id] 136 * 137 * It's an error if the second form doesn't agree with class_. It's an error if 138 * class_ is null for the last form 139 * 140 * @param resource 141 * @param Reference 142 * @return 143 * @throws Exception 144 */ 145 public <T extends Resource> T fetchResource(Class<T> class_, String uri); 146 147 /** 148 * find whether a resource is available. 149 * 150 * Implementations of the interface can assume that if hasResource ruturns true, 151 * the resource will usually be fetched subsequently 152 * 153 * @param class_ 154 * @param uri 155 * @return 156 */ 157 public <T extends Resource> boolean hasResource(Class<T> class_, String uri); 158 159 // -- profile services --------------------------------------------------------- 160 161 public List<String> getResourceNames(); 162 163 // -- Terminology services 164 // ------------------------------------------------------ 165 166 // these are the terminology services used internally by the tools 167 /** 168 * Find a value set for the nominated system uri. return null if there isn't one 169 * (then the tool might try supportsSystem) 170 * 171 * @param system 172 * @return 173 */ 174 public ValueSet fetchCodeSystem(String system); 175 176 /** 177 * True if the underlying terminology service provider will do expansion and 178 * code validation for the terminology. Corresponds to the extension 179 * 180 * http://hl7.org/fhir/StructureDefinition/conformance-supported-system 181 * 182 * in the Conformance resource 183 * 184 * @param system 185 * @return 186 */ 187 public boolean supportsSystem(String system); 188 189 /** 190 * find concept maps for a source 191 * 192 * @param url 193 * @return 194 */ 195 public List<ConceptMap> findMapsForSource(String url); 196 197 /** 198 * ValueSet Expansion - see $expand 199 * 200 * @param source 201 * @return 202 */ 203 public ValueSetExpansionOutcome expandVS(ValueSet source, boolean cacheOk); 204 205 /** 206 * Value set expanion inside the internal expansion engine - used for references 207 * to supported system (see "supportsSystem") for which there is no value set. 208 * 209 * @param inc 210 * @return 211 */ 212 public ValueSetExpansionComponent expandVS(ConceptSetComponent inc); 213 214 Locale getLocale(); 215 216 void setLocale(Locale locale); 217 218 String formatMessage(String theMessage, Object... theMessageArguments); 219 220 void setValidationMessageLanguage(Locale locale); 221 222 public class ValidationResult { 223 private ConceptDefinitionComponent definition; 224 private IssueSeverity severity; 225 private String message; 226 227 public ValidationResult(IssueSeverity severity, String message) { 228 this.severity = severity; 229 this.message = message; 230 } 231 232 public ValidationResult(ConceptDefinitionComponent definition) { 233 this.definition = definition; 234 } 235 236 public ValidationResult(IssueSeverity severity, String message, ConceptDefinitionComponent definition) { 237 this.severity = severity; 238 this.message = message; 239 this.definition = definition; 240 } 241 242 public boolean isOk() { 243 return definition != null; 244 } 245 246 public String getDisplay() { 247 return definition == null ? "??" : definition.getDisplay(); 248 } 249 250 public ConceptDefinitionComponent asConceptDefinition() { 251 return definition; 252 } 253 254 public IssueSeverity getSeverity() { 255 return severity; 256 } 257 258 public String getMessage() { 259 return message; 260 } 261 } 262 263 /** 264 * Validation of a code - consult the terminology service to see whether it is 265 * known. If known, return a description of it 266 * 267 * note: always return a result, with either an error or a code description 268 * 269 * corresponds to 2 terminology service calls: $validate-code and $lookup 270 * 271 * @param system 272 * @param code 273 * @param display 274 * @return 275 */ 276 public ValidationResult validateCode(String system, String code, String display); 277 278 /** 279 * Validation of a code - consult the terminology service to see whether it is 280 * known. If known, return a description of it Also, check whether it's in the 281 * provided value set 282 * 283 * note: always return a result, with either an error or a code description, or 284 * both (e.g. known code, but not in the value set) 285 * 286 * corresponds to 2 terminology service calls: $validate-code and $lookup 287 * 288 * @param system 289 * @param code 290 * @param display 291 * @return 292 */ 293 public ValidationResult validateCode(String system, String code, String display, ValueSet vs); 294 295 public ValidationResult validateCode(Coding code, ValueSet vs); 296 297 public ValidationResult validateCode(CodeableConcept code, ValueSet vs); 298 299 /** 300 * Validation of a code - consult the terminology service to see whether it is 301 * known. If known, return a description of it Also, check whether it's in the 302 * provided value set fragment (for supported systems with no value set 303 * definition) 304 * 305 * note: always return a result, with either an error or a code description, or 306 * both (e.g. known code, but not in the value set) 307 * 308 * corresponds to 2 terminology service calls: $validate-code and $lookup 309 * 310 * @param system 311 * @param code 312 * @param display 313 * @return 314 */ 315 public ValidationResult validateCode(String system, String code, String display, ConceptSetComponent vsi); 316 317 /** 318 * returns the recommended tla for the type 319 * 320 * @param name 321 * @return 322 */ 323 public String getAbbreviation(String name); 324 325 public List<StructureDefinition> allStructures(); 326 327 public StructureDefinition fetchTypeDefinition(String typeName); 328 329}