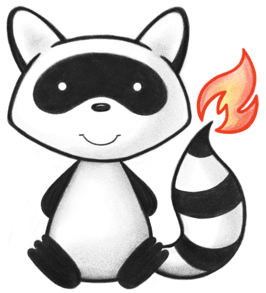
001package org.hl7.fhir.dstu2.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.dstu2.model.CodeableConcept; 033import org.hl7.fhir.dstu2.model.OperationOutcome; 034import org.hl7.fhir.dstu2.model.OperationOutcome.IssueSeverity; 035import org.hl7.fhir.dstu2.model.OperationOutcome.IssueType; 036import org.hl7.fhir.dstu2.model.OperationOutcome.OperationOutcomeIssueComponent; 037import org.hl7.fhir.dstu2.model.StringType; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.utilities.validation.ValidationMessage; 040 041@Deprecated 042public class OperationOutcomeUtilities { 043 044 public static OperationOutcomeIssueComponent convertToIssue(ValidationMessage message, OperationOutcome op) { 045 OperationOutcomeIssueComponent issue = new OperationOutcome.OperationOutcomeIssueComponent(); 046 issue.setCode(convert(message.getType())); 047 if (message.getLocation() != null) { 048 StringType s = new StringType(); 049 s.setValue(Utilities.fhirPathToXPath(message.getLocation()) + (message.getLine() >= 0 && message.getCol() >= 0 050 ? " (line " + Integer.toString(message.getLine()) + ", col" + Integer.toString(message.getCol()) + ")" 051 : "")); 052 issue.getLocation().add(s); 053 } 054 issue.setSeverity(convert(message.getLevel())); 055 CodeableConcept c = new CodeableConcept(); 056 c.setText(message.getMessage()); 057 issue.setDetails(c); 058 if (message.getSource() != null) { 059 issue.getExtension().add(ToolingExtensions.makeIssueSource(message.getSource())); 060 } 061 return issue; 062 } 063 064 private static IssueSeverity convert(org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity level) { 065 switch (level) { 066 case FATAL: 067 return IssueSeverity.FATAL; 068 case ERROR: 069 return IssueSeverity.ERROR; 070 case WARNING: 071 return IssueSeverity.WARNING; 072 case INFORMATION: 073 return IssueSeverity.INFORMATION; 074 } 075 return IssueSeverity.NULL; 076 } 077 078 private static IssueType convert(org.hl7.fhir.utilities.validation.ValidationMessage.IssueType type) { 079 switch (type) { 080 case INVALID: 081 case STRUCTURE: 082 return IssueType.STRUCTURE; 083 case REQUIRED: 084 return IssueType.REQUIRED; 085 case VALUE: 086 return IssueType.VALUE; 087 case INVARIANT: 088 return IssueType.INVARIANT; 089 case SECURITY: 090 return IssueType.SECURITY; 091 case LOGIN: 092 return IssueType.LOGIN; 093 case UNKNOWN: 094 return IssueType.UNKNOWN; 095 case EXPIRED: 096 return IssueType.EXPIRED; 097 case FORBIDDEN: 098 return IssueType.FORBIDDEN; 099 case SUPPRESSED: 100 return IssueType.SUPPRESSED; 101 case PROCESSING: 102 return IssueType.PROCESSING; 103 case NOTSUPPORTED: 104 return IssueType.NOTSUPPORTED; 105 case DUPLICATE: 106 return IssueType.DUPLICATE; 107 case NOTFOUND: 108 return IssueType.NOTFOUND; 109 case TOOLONG: 110 return IssueType.TOOLONG; 111 case CODEINVALID: 112 return IssueType.CODEINVALID; 113 case EXTENSION: 114 return IssueType.EXTENSION; 115 case TOOCOSTLY: 116 return IssueType.TOOCOSTLY; 117 case BUSINESSRULE: 118 return IssueType.BUSINESSRULE; 119 case CONFLICT: 120 return IssueType.CONFLICT; 121 case INCOMPLETE: 122 return IssueType.INCOMPLETE; 123 case TRANSIENT: 124 return IssueType.TRANSIENT; 125 case LOCKERROR: 126 return IssueType.LOCKERROR; 127 case NOSTORE: 128 return IssueType.NOSTORE; 129 case EXCEPTION: 130 return IssueType.EXCEPTION; 131 case TIMEOUT: 132 return IssueType.TIMEOUT; 133 case THROTTLED: 134 return IssueType.THROTTLED; 135 case INFORMATIONAL: 136 return IssueType.INFORMATIONAL; 137 } 138 return IssueType.NULL; 139 } 140}