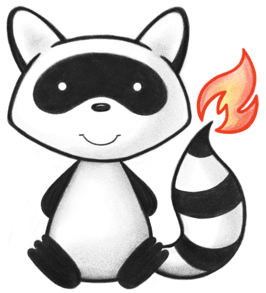
001package org.hl7.fhir.dstu2.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.FileNotFoundException; 033import java.io.IOException; 034import java.util.HashMap; 035import java.util.Map; 036 037import javax.xml.parsers.DocumentBuilder; 038import javax.xml.parsers.DocumentBuilderFactory; 039import javax.xml.parsers.ParserConfigurationException; 040 041import org.hl7.fhir.utilities.filesystem.CSFileInputStream; 042import org.hl7.fhir.utilities.xml.XMLUtil; 043import org.w3c.dom.Document; 044import org.w3c.dom.Element; 045import org.xml.sax.SAXException; 046 047public class Translations { 048 049 private String[] lang; 050 private Map<String, Element> messages = new HashMap<String, Element>(); 051 052 /** 053 * Set a default language to use 054 * 055 * @param lang 056 */ 057 public void setLang(String lang) { 058 this.lang = lang.split("[.;]"); 059 } 060 061 /** 062 * Load from the XML translations file maintained by the FHIR project 063 * 064 * @param filename 065 * @throws IOException 066 * @throws SAXException 067 * @throws FileNotFoundException 068 * @throws ParserConfigurationException 069 * @throws Exception 070 */ 071 public void load(String filename) 072 throws FileNotFoundException, SAXException, IOException, ParserConfigurationException { 073 DocumentBuilderFactory factory = XMLUtil.newXXEProtectedDocumentBuilderFactory(); 074 DocumentBuilder builder = factory.newDocumentBuilder(); 075 loadMessages(builder.parse(new CSFileInputStream(filename))); 076 } 077 078 private void loadMessages(Document doc) { 079 // TODO Auto-generated method stub 080 Element element = XMLUtil.getFirstChild(doc.getDocumentElement()); 081 while (element != null) { 082 messages.put(element.getAttribute("id"), element); 083 element = XMLUtil.getNextSibling(element); 084 } 085 } 086 087 public boolean hasTranslation(String id) { 088 return messages.containsKey(id); 089 } 090 091 /** 092 * use configured language 093 * 094 * @param id - the id of the message to retrieve 095 * @param defaultMsg - string to use if the message is not defined or a language 096 * match is not found (if null, then will default to english) 097 * @return the message 098 */ 099 public String getMessage(String id, String defaultMsg) { 100 return getMessage(id, lang, defaultMsg); 101 } 102 103 /** 104 * return the message in a specified language 105 * 106 * @param id - the id of the message to retrieve 107 * @param lang - a language string from a browser 108 * @param defaultMsg - string to use if the message is not defined or a language 109 * match is not found (if null, then will default to the 110 * english message) 111 * @return the message 112 */ 113 public String getMessage(String id, String lang, String defaultMsg) { 114 return getMessage(id, lang.split("[.;]"), defaultMsg); 115 } 116 117 private String getMessage(String id, String[] lang, String defaultMsg) { 118 Element msg = messages.get(id); 119 if (msg == null) 120 return defaultMsg; 121 for (String l : lang) { 122 String res = getByLang(msg, l); 123 if (res != null) 124 return res; 125 } 126 if (defaultMsg == null) { 127 String res = getByLang(msg, "en"); 128 if (res != null) 129 return res; 130 } 131 return defaultMsg; 132 } 133 134 private String getByLang(Element msg, String lang) { 135 Element c = XMLUtil.getFirstChild(msg); 136 while (c != null) { 137 if (c.getAttribute("lang").equals(lang)) 138 return c.getTextContent(); 139 c = XMLUtil.getNextSibling(c); 140 } 141 return null; 142 } 143 144 // http://en.wikipedia.org/wiki/List_of_ISO_639-1_codes 145 public String getLangDesc(String s) { 146 if (s.equals("en")) 147 return "English"; 148 if (s.equals("nl")) 149 return "Nederlands (Dutch)"; 150 if (s.equals("de")) 151 return "Deutsch (German)"; 152 if (s.equals("du")) 153 return "\u0440\u0443\u0301\u0441\u0441\u043a\u0438\u0439 (Russian)"; 154 return "\"" + s + "\""; 155 } 156 157}