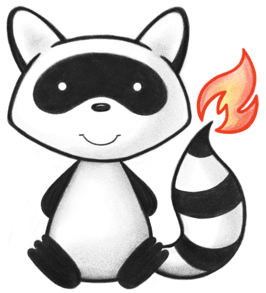
001package org.hl7.fhir.dstu2.utils.validation; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.List; 033 034import org.hl7.fhir.dstu2.model.StructureDefinition; 035import org.hl7.fhir.dstu2.utils.validation.constants.BestPracticeWarningLevel; 036import org.hl7.fhir.dstu2.utils.validation.constants.CheckDisplayOption; 037import org.hl7.fhir.dstu2.utils.validation.constants.IdStatus; 038import org.hl7.fhir.utilities.validation.ValidationMessage; 039import org.w3c.dom.Document; 040import org.w3c.dom.Element; 041 042import com.google.gson.JsonObject; 043 044@Deprecated 045public interface IResourceValidator { 046 047 /** 048 * how much to check displays for coded elements 049 * 050 * @return 051 */ 052 CheckDisplayOption getCheckDisplay(); 053 054 /** 055 * how much to check displays for coded elements 056 * 057 * @return 058 */ 059 void setCheckDisplay(CheckDisplayOption checkDisplay); 060 061 /** 062 * whether the resource must have an id or not (depends on context) 063 * 064 * @return 065 */ 066 067 IdStatus getResourceIdRule(); 068 069 void setResourceIdRule(IdStatus resourceIdRule); 070 071 BestPracticeWarningLevel getBasePracticeWarningLevel(); 072 073 void setBestPracticeWarningLevel(BestPracticeWarningLevel value); 074 075 /** 076 * Given a DOM element, return a list of errors in the resource @- if the 077 * underlying infrastructure fails (not if the resource is invalid) 078 */ 079 void validate(List<ValidationMessage> errors, Element element) throws Exception; 080 081 /** 082 * Given a JSON Object, return a list of errors in the resource @- if the 083 * underlying infrastructure fails (not if the resource is invalid) 084 */ 085 void validate(List<ValidationMessage> errors, JsonObject object) throws Exception; 086 087 /** 088 * Given a DOM element, return a list of errors in the resource @- if the 089 * underlying infrastructure fails (not if the resource is invalid) 090 */ 091 List<ValidationMessage> validate(Element element) throws Exception; 092 093 /** 094 * Given a DOM element, return a list of errors in the resource @- if the 095 * underlying infrastructure fails (not if the resource is invalid) 096 */ 097 List<ValidationMessage> validate(JsonObject object) throws Exception; 098 099 /** 100 * Given a DOM element, return a list of errors in the resource with regard to 101 * the specified profile (by logical identifier) @- if the underlying 102 * infrastructure fails, or the profile can't be found (not if the resource is 103 * invalid) 104 */ 105 void validate(List<ValidationMessage> errors, Element element, String profile) throws Exception; 106 107 /** 108 * Given a DOM element, return a list of errors in the resource with regard to 109 * the specified profile (by logical identifier) @- if the underlying 110 * infrastructure fails, or the profile can't be found (not if the resource is 111 * invalid) 112 */ 113 List<ValidationMessage> validate(Element element, String profile) throws Exception; 114 115 /** 116 * Given a DOM element, return a list of errors in the resource with regard to 117 * the specified profile (by logical identifier) @- if the underlying 118 * infrastructure fails, or the profile can't be found (not if the resource is 119 * invalid) 120 */ 121 List<ValidationMessage> validate(JsonObject object, StructureDefinition profile) throws Exception; 122 123 /** 124 * Given a DOM element, return a list of errors in the resource with regard to 125 * the specified profile (by logical identifier) @- if the underlying 126 * infrastructure fails, or the profile can't be found (not if the resource is 127 * invalid) 128 */ 129 List<ValidationMessage> validate(JsonObject object, String profile) throws Exception; 130 131 /** 132 * Given a DOM element, return a list of errors in the resource with regard to 133 * the specified profile @- if the underlying infrastructure fails (not if the 134 * resource is invalid) 135 */ 136 void validate(List<ValidationMessage> errors, Element element, StructureDefinition profile) throws Exception; 137 138 /** 139 * Given a DOM element, return a list of errors in the resource with regard to 140 * the specified profile @- if the underlying infrastructure fails (not if the 141 * resource is invalid) 142 */ 143 void validate(List<ValidationMessage> errors, JsonObject object, StructureDefinition profile) throws Exception; 144 145 /** 146 * Given a DOM element, return a list of errors in the resource with regard to 147 * the specified profile @- if the underlying infrastructure fails (not if the 148 * resource is invalid) 149 */ 150 void validate(List<ValidationMessage> errors, JsonObject object, String profile) throws Exception; 151 152 /** 153 * Given a DOM element, return a list of errors in the resource with regard to 154 * the specified profile @- if the underlying infrastructure fails (not if the 155 * resource is invalid) 156 */ 157 List<ValidationMessage> validate(Element element, StructureDefinition profile) throws Exception; 158 159 /** 160 * Given a DOM document, return a list of errors in the resource @- if the 161 * underlying infrastructure fails (not if the resource is invalid) 162 */ 163 void validate(List<ValidationMessage> errors, Document document) throws Exception; 164 165 /** 166 * Given a DOM document, return a list of errors in the resource @- if the 167 * underlying infrastructure fails (not if the resource is invalid) 168 */ 169 List<ValidationMessage> validate(Document document) throws Exception; 170 171 /** 172 * Given a DOM document, return a list of errors in the resource with regard to 173 * the specified profile (by logical identifier) @- if the underlying 174 * infrastructure fails, or the profile can't be found (not if the resource is 175 * invalid) 176 */ 177 void validate(List<ValidationMessage> errors, Document document, String profile) throws Exception; 178 179 /** 180 * Given a DOM document, return a list of errors in the resource with regard to 181 * the specified profile (by logical identifier) @- if the underlying 182 * infrastructure fails, or the profile can't be found (not if the resource is 183 * invalid) 184 */ 185 List<ValidationMessage> validate(Document document, String profile) throws Exception; 186 187 /** 188 * Given a DOM document, return a list of errors in the resource with regard to 189 * the specified profile @- if the underlying infrastructure fails (not if the 190 * resource is invalid) 191 */ 192 void validate(List<ValidationMessage> errors, Document document, StructureDefinition profile) throws Exception; 193 194 /** 195 * Given a DOM document, return a list of errors in the resource with regard to 196 * the specified profile @- if the underlying infrastructure fails (not if the 197 * resource is invalid) 198 */ 199 List<ValidationMessage> validate(Document document, StructureDefinition profile) throws Exception; 200 201}