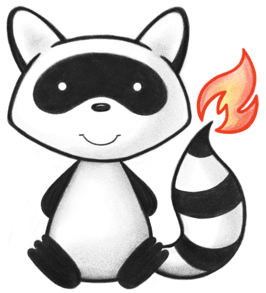
001package org.hl7.fhir.r4.context; 002 003import java.util.ArrayList; 004import java.util.HashMap; 005import java.util.HashSet; 006import java.util.List; 007import java.util.Map; 008import java.util.Set; 009 010import lombok.Getter; 011import lombok.Setter; 012import lombok.extern.slf4j.Slf4j; 013import org.hl7.fhir.exceptions.DefinitionException; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r4.conformance.ProfileUtilities; 016import org.hl7.fhir.r4.conformance.ProfileUtilities.ProfileKnowledgeProvider; 017import org.hl7.fhir.r4.model.CodeSystem; 018import org.hl7.fhir.r4.model.ElementDefinition; 019import org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionComponent; 020import org.hl7.fhir.r4.model.CodeSystem.ConceptPropertyComponent; 021import org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBindingComponent; 022import org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType; 023import org.hl7.fhir.r4.model.NamingSystem.NamingSystemUniqueIdComponent; 024import org.hl7.fhir.r4.model.Resource; 025import org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind; 026import org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule; 027import org.hl7.fhir.r4.model.StructureMap; 028import org.hl7.fhir.r4.model.Identifier; 029import org.hl7.fhir.r4.model.NamingSystem; 030import org.hl7.fhir.r4.model.StructureDefinition; 031import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 032import org.hl7.fhir.utilities.OIDUtilities; 033import org.hl7.fhir.utilities.Utilities; 034import org.hl7.fhir.utilities.VersionUtilities; 035import org.hl7.fhir.utilities.i18n.I18nConstants; 036import org.hl7.fhir.utilities.validation.ValidationMessage; 037import org.hl7.fhir.utilities.validation.ValidationMessage.IssueType; 038import org.hl7.fhir.utilities.validation.ValidationMessage.Source; 039 040@MarkedToMoveToAdjunctPackage 041@Slf4j 042public class ContextUtilities implements ProfileKnowledgeProvider { 043 044 private IWorkerContext context; 045 @Setter 046 @Deprecated 047 private boolean suppressDebugMessages; 048 049 public boolean isSuppressDebugMessages() { 050 return false; 051 } 052 053 private Map<String, String> oidCache = new HashMap<>(); 054 private List<StructureDefinition> allStructuresList = new ArrayList<StructureDefinition>(); 055 private List<String> canonicalResourceNames; 056 private List<String> concreteResourceNames; 057 private Set<String> concreteResourceNameSet; 058 059 public ContextUtilities(IWorkerContext context) { 060 super(); 061 this.context = context; 062 } 063 064 065 public String oid2Uri(String oid) { 066 if (oid != null && oid.startsWith("urn:oid:")) { 067 oid = oid.substring(8); 068 } 069 if (oidCache.containsKey(oid)) { 070 return oidCache.get(oid); 071 } 072 073 String uri = OIDUtilities.getUriForOid(oid); 074 if (uri != null) { 075 oidCache.put(oid, uri); 076 return uri; 077 } 078 CodeSystem cs = context.fetchCodeSystem("http://terminology.hl7.org/CodeSystem/v2-tables"); 079 if (cs != null) { 080 for (ConceptDefinitionComponent cc : cs.getConcept()) { 081 for (ConceptPropertyComponent cp : cc.getProperty()) { 082 if (Utilities.existsInList(cp.getCode(), "v2-table-oid", "v2-cs-oid") && oid.equals(cp.getValue().primitiveValue())) { 083 for (ConceptPropertyComponent cp2 : cc.getProperty()) { 084 if ("v2-cs-uri".equals(cp2.getCode())) { 085 oidCache.put(oid, cp2.getValue().primitiveValue()); 086 return cp2.getValue().primitiveValue(); 087 } 088 } 089 } 090 } 091 } 092 } 093 for (CodeSystem css : context.fetchResourcesByType(CodeSystem.class)) { 094 if (("urn:oid:"+oid).equals(css.getUrl())) { 095 oidCache.put(oid, css.getUrl()); 096 return css.getUrl(); 097 } 098 for (Identifier id : css.getIdentifier()) { 099 if ("urn:ietf:rfc:3986".equals(id.getSystem()) && ("urn:oid:"+oid).equals(id.getValue())) { 100 oidCache.put(oid, css.getUrl()); 101 return css.getUrl(); 102 } 103 } 104 } 105 for (NamingSystem ns : context.fetchResourcesByType(NamingSystem.class)) { 106 if (hasOid(ns, oid)) { 107 uri = getUri(ns); 108 if (uri != null) { 109 oidCache.put(oid, null); 110 return null; 111 } 112 } 113 } 114 oidCache.put(oid, null); 115 return null; 116 } 117 118 private String getUri(NamingSystem ns) { 119 for (NamingSystemUniqueIdComponent id : ns.getUniqueId()) { 120 if (id.getType() == NamingSystemIdentifierType.URI) 121 return id.getValue(); 122 } 123 return null; 124 } 125 126 private boolean hasOid(NamingSystem ns, String oid) { 127 for (NamingSystemUniqueIdComponent id : ns.getUniqueId()) { 128 if (id.getType() == NamingSystemIdentifierType.OID && id.getValue().equals(oid)) 129 return true; 130 } 131 return false; 132 } 133 134 /** 135 * @return a list of the resource and type names defined for this version 136 */ 137 public List<String> getTypeNames() { 138 Set<String> result = new HashSet<String>(); 139 for (StructureDefinition sd : context.fetchResourcesByType(StructureDefinition.class)) { 140 if (sd.getKind() != StructureDefinitionKind.LOGICAL && sd.getDerivation() == TypeDerivationRule.SPECIALIZATION) 141 result.add(sd.getName()); 142 } 143 return Utilities.sorted(result); 144 } 145 146 147 /** 148 * @return a set of the resource and type names defined for this version 149 */ 150 public Set<String> getTypeNameSet() { 151 Set<String> result = new HashSet<String>(); 152 for (StructureDefinition sd : context.fetchResourcesByType(StructureDefinition.class)) { 153 if (sd.getKind() != StructureDefinitionKind.LOGICAL && sd.getDerivation() == TypeDerivationRule.SPECIALIZATION && 154 VersionUtilities.versionsCompatible(context.getVersion(), sd.getFhirVersion().toCode())) { 155 result.add(sd.getName()); 156 } 157 } 158 return result; 159 } 160 161 public String getLinkForUrl(String corePath, String url) { 162 if (url == null) { 163 return null; 164 } 165 166 if (context.hasResource(Resource.class, url)) { 167 Resource cr = context.fetchResource(Resource.class, url); 168 return cr.getUserString("path"); 169 } 170 return null; 171 } 172 173 174 protected String tail(String url) { 175 if (Utilities.noString(url)) { 176 return "noname"; 177 } 178 if (url.contains("/")) { 179 return url.substring(url.lastIndexOf("/")+1); 180 } 181 return url; 182 } 183 184 private boolean hasUrlProperty(StructureDefinition sd) { 185 for (ElementDefinition ed : sd.getSnapshot().getElement()) { 186 if (ed.getPath().equals(sd.getType()+".url")) { 187 return true; 188 } 189 } 190 return false; 191 } 192 193 // -- profile services --------------------------------------------------------- 194 195 196 /** 197 * @return a list of the resource names that are canonical resources defined for this version 198 */ 199 public List<String> getCanonicalResourceNames() { 200 if (canonicalResourceNames == null) { 201 canonicalResourceNames = new ArrayList<>(); 202 Set<String> names = new HashSet<>(); 203 for (StructureDefinition sd : allStructures()) { 204 if (sd.getKind() == StructureDefinitionKind.RESOURCE && !sd.getAbstract() && hasUrlProperty(sd)) { 205 names.add(sd.getType()); 206 } 207 } 208 canonicalResourceNames.addAll(Utilities.sorted(names)); 209 } 210 return canonicalResourceNames; 211 } 212 213 /** 214 * @return a list of all structure definitions, with snapshots generated (if possible) 215 */ 216 public List<StructureDefinition> allStructures(){ 217 if (allStructuresList.isEmpty()) { 218 Set<StructureDefinition> set = new HashSet<StructureDefinition>(); 219 for (StructureDefinition sd : getStructures()) { 220 if (!set.contains(sd)) { 221 try { 222 generateSnapshot(sd); 223 // new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(Utilities.path("[tmp]", "snapshot", tail(sd.getUrl())+".xml")), sd); 224 } catch (Exception e) { 225 log.debug("Unable to generate snapshot @2 for "+tail(sd.getUrl()) +" from "+tail(sd.getBaseDefinition())+" because "+e.getMessage(), e); 226 } 227 allStructuresList.add(sd); 228 set.add(sd); 229 } 230 } 231 } 232 return allStructuresList; 233 } 234 235 /** 236 * @return a list of all structure definitions, without trying to generate snapshots 237 */ 238 public List<StructureDefinition> getStructures() { 239 return context.fetchResourcesByType(StructureDefinition.class); 240 } 241 242 /** 243 * Given a structure definition, generate a snapshot (or regenerate it) 244 * @param p 245 * @throws DefinitionException 246 * @throws FHIRException 247 */ 248 public void generateSnapshot(StructureDefinition p) throws DefinitionException, FHIRException { 249 if ((!p.hasSnapshot() || isProfileNeedsRegenerate(p))) { 250 if (!p.hasBaseDefinition()) 251 throw new DefinitionException(context.formatMessage(I18nConstants.PROFILE___HAS_NO_BASE_AND_NO_SNAPSHOT, p.getName(), p.getUrl())); 252 StructureDefinition sd = context.fetchResource(StructureDefinition.class, p.getBaseDefinition(), p); 253 if (sd == null && "http://hl7.org/fhir/StructureDefinition/Base".equals(p.getBaseDefinition())) { 254 throw new Error("Not done yet"); // sd = ProfileUtilities.makeBaseDefinition(p.getFhirVersion()); 255 } 256 if (sd == null) { 257 throw new DefinitionException(context.formatMessage(I18nConstants.PROFILE___BASE__COULD_NOT_BE_RESOLVED, p.getName(), p.getUrl(), p.getBaseDefinition())); 258 } 259 List<ValidationMessage> msgs = new ArrayList<ValidationMessage>(); 260 List<String> errors = new ArrayList<String>(); 261 ProfileUtilities pu = new ProfileUtilities(context, msgs, this); 262 pu.setThrowException(false); 263 if (sd.getDerivation() == TypeDerivationRule.CONSTRAINT) { 264 pu.sortDifferential(sd, p, p.getUrl(), errors); 265 } 266 pu.setDebug(false); 267 for (String err : errors) { 268 msgs.add(new ValidationMessage(Source.ProfileValidator, IssueType.EXCEPTION, p.getUserString("path"), "Error sorting Differential: "+err, ValidationMessage.IssueSeverity.ERROR)); 269 } 270 pu.generateSnapshot(sd, p, p.getUrl(), sd.getUserString("webroot"), p.getName()); 271 for (ValidationMessage msg : msgs) { 272 if ((msg.getLevel() == ValidationMessage.IssueSeverity.ERROR) || msg.getLevel() == ValidationMessage.IssueSeverity.FATAL) { 273 if (!msg.isIgnorableError()) { 274 throw new DefinitionException(context.formatMessage(I18nConstants.PROFILE___ELEMENT__ERROR_GENERATING_SNAPSHOT_, p.getName(), p.getUrl(), msg.getLocation(), msg.getMessage())); 275 } else { 276 log.error(msg.getMessage()); 277 } 278 } 279 } 280 if (!p.hasSnapshot()) 281 throw new FHIRException(context.formatMessage(I18nConstants.PROFILE___ERROR_GENERATING_SNAPSHOT, p.getName(), p.getUrl())); 282 pu = null; 283 } 284 } 285 286 287 // work around the fact that some Implementation guides were published with old snapshot generators that left invalid snapshots behind. 288 private boolean isProfileNeedsRegenerate(StructureDefinition p) { 289 boolean needs = !p.hasUserData("hack.regnerated") && Utilities.existsInList(p.getUrl(), "http://hl7.org/fhir/uv/sdc/StructureDefinition/sdc-questionnaireresponse"); 290 if (needs) { 291 p.setUserData("hack.regnerated", "yes"); 292 } 293 return needs; 294 } 295 296 @Override 297 public boolean isPrimitiveType(String type) { 298 return context.isPrimitiveType(type); 299 } 300 301 @Override 302 public boolean isDatatype(String type) { 303 StructureDefinition sd = context.fetchTypeDefinition(type); 304 return sd != null && (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE || sd.getKind() == StructureDefinitionKind.COMPLEXTYPE) && sd.getDerivation() == TypeDerivationRule.SPECIALIZATION; 305 } 306 307 @Override 308 public boolean isResource(String t) { 309 if (getConcreteResourceSet().contains(t)) { 310 return true; 311 } 312 StructureDefinition sd; 313 try { 314 sd = context.fetchResource(StructureDefinition.class, "http://hl7.org/fhir/StructureDefinition/"+t); 315 } catch (Exception e) { 316 return false; 317 } 318 if (sd == null) 319 return false; 320 if (sd.getDerivation() == TypeDerivationRule.CONSTRAINT) 321 return false; 322 return sd.getKind() == StructureDefinitionKind.RESOURCE; 323 } 324 325 @Override 326 public boolean hasLinkFor(String typeSimple) { 327 return false; 328 } 329 330 @Override 331 public String getLinkFor(String corePath, String typeSimple) { 332 return null; 333 } 334 335 @Override 336 public BindingResolution resolveBinding(StructureDefinition profile, ElementDefinitionBindingComponent binding, String path) { 337 return null; 338 } 339 340 @Override 341 public BindingResolution resolveBinding(StructureDefinition profile, String url, String path) { 342 return null; 343 } 344 345 @Override 346 public String getLinkForProfile(StructureDefinition profile, String url) { 347 return null; 348 } 349 @Override 350 public boolean prependLinks() { 351 return false; 352 } 353 354 public StructureDefinition fetchByJsonName(String key) { 355 for (StructureDefinition sd : context.fetchResourcesByType(StructureDefinition.class)) { 356 ElementDefinition ed = sd.getSnapshot().getElementFirstRep(); 357 if (ed != null) { 358 return sd; 359 } 360 } 361 return null; 362 } 363 364 public Set<String> getConcreteResourceSet() { 365 if (concreteResourceNameSet == null) { 366 concreteResourceNameSet = new HashSet<>(); 367 for (StructureDefinition sd : getStructures()) { 368 if (sd.getKind() == StructureDefinitionKind.RESOURCE && !sd.getAbstract() && sd.getDerivation() == TypeDerivationRule.SPECIALIZATION) { 369 concreteResourceNameSet.add(sd.getType()); 370 } 371 } 372 } 373 return concreteResourceNameSet; 374 } 375 376 public List<String> getConcreteResources() { 377 if (concreteResourceNames == null) { 378 concreteResourceNames = new ArrayList<>(); 379 concreteResourceNames.addAll(Utilities.sorted(getConcreteResourceSet())); 380 } 381 return concreteResourceNames; 382 } 383 384 public List<StructureMap> listMaps(String url) { 385 List<StructureMap> res = new ArrayList<>(); 386 String start = url.substring(0, url.indexOf("*")); 387 String end = url.substring(url.indexOf("*")+1); 388 for (StructureMap map : context.fetchResourcesByType(StructureMap.class)) { 389 String u = map.getUrl(); 390 if (u.startsWith(start) && u.endsWith(end)) { 391 res.add(map); 392 } 393 } 394 return res; 395 } 396 397 public List<String> fetchCodeSystemVersions(String system) { 398 List<String> res = new ArrayList<>(); 399 for (CodeSystem cs : context.fetchResourcesByType(CodeSystem.class)) { 400 if (system.equals(cs.getUrl()) && cs.hasVersion()) { 401 res.add(cs.getVersion()); 402 } 403 } 404 return res; 405 } 406 407 public StructureDefinition findType(String typeName) { 408 StructureDefinition t = context.fetchTypeDefinition(typeName); 409 if (t != null) { 410 return t; 411 } 412 List<StructureDefinition> candidates = new ArrayList<>(); 413 for (StructureDefinition sd : getStructures()) { 414 if (sd.getType().equals(typeName)) { 415 candidates.add(sd); 416 } 417 } 418 if (candidates.size() == 1) { 419 return candidates.get(0); 420 } 421 return null; 422 } 423 424 public StructureDefinition fetchProfileByIdentifier(String tid) { 425 for (StructureDefinition sd : context.fetchResourcesByType(StructureDefinition.class)) { 426 for (Identifier ii : sd.getIdentifier()) { 427 if (tid.equals(ii.getValue())) { 428 return sd; 429 } 430 } 431 } 432 return null; 433 } 434 435 public boolean isAbstractType(String typeName) { 436 StructureDefinition sd = context.fetchTypeDefinition(typeName); 437 if (sd != null) { 438 return sd.getAbstract(); 439 } 440 return false; 441 } 442 443 public boolean isDomainResource(String typeName) { 444 StructureDefinition sd = context.fetchTypeDefinition(typeName); 445 while (sd != null) { 446 if ("DomainResource".equals(sd.getType())) { 447 return true; 448 } 449 sd = context.fetchResource(StructureDefinition.class, sd.getBaseDefinition()); 450 } 451 return false; 452 } 453 454 public IWorkerContext getWorker() { 455 return context; 456 } 457 458} 459