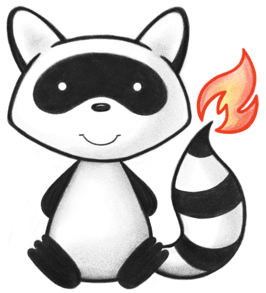
001package org.hl7.fhir.r4.fhirpath; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.exceptions.FHIRException; 007import org.hl7.fhir.r4.model.Base; 008import org.hl7.fhir.r4.model.Element; 009import org.hl7.fhir.r4.model.ElementDefinition; 010import org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent; 011import org.hl7.fhir.r4.model.Property; 012import org.hl7.fhir.r4.model.Resource; 013import org.hl7.fhir.r4.model.StringType; 014import org.hl7.fhir.r4.model.StructureDefinition; 015import org.hl7.fhir.utilities.Utilities; 016 017public class FHIRPathUtilityClasses { 018 019 020 public static class FHIRConstant extends Base { 021 022 private static final long serialVersionUID = -8933773658248269439L; 023 private String value; 024 025 public FHIRConstant(String value) { 026 this.value = value; 027 } 028 029 @Override 030 public String fhirType() { 031 return "%constant"; 032 } 033 034 @Override 035 protected void listChildren(List<Property> result) { 036 } 037 038 @Override 039 public String getIdBase() { 040 return null; 041 } 042 043 @Override 044 public void setIdBase(String value) { 045 } 046 047 public String getValue() { 048 return value; 049 } 050 051 @Override 052 public String primitiveValue() { 053 return value; 054 } 055 056 @Override 057 public Base copy() { 058 throw new Error("Not Implemented"); 059 } 060 061 } 062 063 public static class ClassTypeInfo extends Base { 064 private static final long serialVersionUID = 4909223114071029317L; 065 private Base instance; 066 067 public ClassTypeInfo(Base instance) { 068 super(); 069 this.instance = instance; 070 } 071 072 @Override 073 public String fhirType() { 074 return "ClassInfo"; 075 } 076 077 @Override 078 protected void listChildren(List<Property> result) { 079 } 080 081 @Override 082 public String getIdBase() { 083 return null; 084 } 085 086 @Override 087 public void setIdBase(String value) { 088 } 089 090 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 091 if (name.equals("name")) { 092 return new Base[] { new StringType(getName()) }; 093 } else if (name.equals("namespace")) { 094 return new Base[] { new StringType(getNamespace()) }; 095 } else { 096 return super.getProperty(hash, name, checkValid); 097 } 098 } 099 100 private String getNamespace() { 101 if ((instance instanceof Resource)) { 102 return "FHIR"; 103 } else if (!(instance instanceof Element) || ((Element) instance).isDisallowExtensions()) { 104 return "System"; 105 } else { 106 return "FHIR"; 107 } 108 } 109 110 private String getName() { 111 if ((instance instanceof Resource)) { 112 return instance.fhirType(); 113 } else if (!(instance instanceof Element) || ((Element) instance).isDisallowExtensions()) { 114 return Utilities.capitalize(instance.fhirType()); 115 } else { 116 return instance.fhirType(); 117 } 118 } 119 120 @Override 121 public Base copy() { 122 throw new Error("Not Implemented"); 123 } 124 125 } 126 127 public static class TypedElementDefinition { 128 private ElementDefinition element; 129 private String type; 130 private StructureDefinition src; 131 132 public TypedElementDefinition(StructureDefinition src, ElementDefinition element, String type) { 133 super(); 134 this.element = element; 135 this.type = type; 136 this.src = src; 137 } 138 139 public TypedElementDefinition(ElementDefinition element) { 140 super(); 141 this.element = element; 142 } 143 144 public ElementDefinition getElement() { 145 return element; 146 } 147 148 public String getType() { 149 return type; 150 } 151 152 public List<TypeRefComponent> getTypes() { 153 List<TypeRefComponent> res = new ArrayList<ElementDefinition.TypeRefComponent>(); 154 for (TypeRefComponent tr : element.getType()) { 155 if (type == null || type.equals(tr.getCode())) { 156 res.add(tr); 157 } 158 } 159 return res; 160 } 161 162 public boolean hasType(String tn) { 163 if (type != null) { 164 return tn.equals(type); 165 } else { 166 for (TypeRefComponent t : element.getType()) { 167 if (tn.equals(t.getCode())) { 168 return true; 169 } 170 } 171 return false; 172 } 173 } 174 175 public StructureDefinition getSrc() { 176 return src; 177 } 178 } 179 180 public static class FunctionDetails { 181 private String description; 182 private int minParameters; 183 private int maxParameters; 184 185 public FunctionDetails(String description, int minParameters, int maxParameters) { 186 super(); 187 this.description = description; 188 this.minParameters = minParameters; 189 this.maxParameters = maxParameters; 190 } 191 192 public String getDescription() { 193 return description; 194 } 195 196 public int getMinParameters() { 197 return minParameters; 198 } 199 200 public int getMaxParameters() { 201 return maxParameters; 202 } 203 204 } 205}