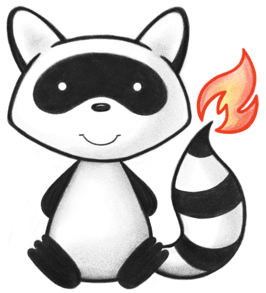
001package org.hl7.fhir.r4.formats; 002 003import java.io.IOException; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034import java.io.InputStream; 035import java.io.OutputStream; 036import java.io.UnsupportedEncodingException; 037 038import org.hl7.fhir.exceptions.FHIRFormatError; 039import org.hl7.fhir.r4.model.Resource; 040import org.hl7.fhir.r4.model.Type; 041import org.xmlpull.v1.XmlPullParserException; 042 043/** 044 * General interface - either an XML or JSON parser: read or write instances 045 * 046 * Defined to allow a factory to create a parser of the right type 047 */ 048public interface IParser { 049 050 /** 051 * check what kind of parser this is 052 * 053 * @return what kind of parser this is 054 */ 055 public ParserType getType(); 056 057 // -- Parser Configuration ---------------------------------- 058 /** 059 * Whether to parse or ignore comments - either reading or writing 060 */ 061 public boolean getHandleComments(); 062 063 public IParser setHandleComments(boolean value); 064 065 /** 066 * @param allowUnknownContent Whether to throw an exception if unknown content 067 * is found (or just skip it) when parsing 068 */ 069 public boolean isAllowUnknownContent(); 070 071 public IParser setAllowUnknownContent(boolean value); 072 073 public enum OutputStyle { 074 /** 075 * Produce normal output - no whitespace, except in HTML where whitespace is 076 * untouched 077 */ 078 NORMAL, 079 080 /** 081 * Produce pretty output - human readable whitespace, HTML whitespace untouched 082 */ 083 PRETTY, 084 085 /** 086 * Produce canonical output - no comments, no whitspace, HTML whitespace 087 * normlised, JSON attributes sorted alphabetically (slightly slower) 088 */ 089 CANONICAL, 090 } 091 092 /** 093 * Writing: 094 */ 095 public OutputStyle getOutputStyle(); 096 097 public IParser setOutputStyle(OutputStyle value); 098 099 /** 100 * This method is used by the publication tooling to stop the xhrtml narrative 101 * being generated. It is not valid to use in production use. The tooling uses 102 * it to generate json/xml representations in html that are not cluttered by 103 * escaped html representations of the html representation 104 */ 105 public IParser setSuppressXhtml(String message); 106 107 // -- Reading methods ---------------------------------------- 108 109 /** 110 * parse content that is known to be a resource 111 * 112 * @throws XmlPullParserException 113 * @throws FHIRFormatError 114 * @throws IOException 115 */ 116 public Resource parse(InputStream input) throws IOException, FHIRFormatError; 117 118 /** 119 * parse content that is known to be a resource 120 * 121 * @throws UnsupportedEncodingException 122 * @throws IOException 123 * @throws FHIRFormatError 124 */ 125 public Resource parse(String input) throws UnsupportedEncodingException, FHIRFormatError, IOException; 126 127 /** 128 * parse content that is known to be a resource 129 * 130 * @throws IOException 131 * @throws FHIRFormatError 132 */ 133 public Resource parse(byte[] bytes) throws FHIRFormatError, IOException; 134 135 /** 136 * This is used to parse a type - a fragment of a resource. There's no reason to 137 * use this in production - it's used in the build tools 138 * 139 * Not supported by all implementations 140 * 141 * @param input 142 * @param knownType. if this is blank, the parser may try to infer the type (xml 143 * only) 144 * @return 145 * @throws XmlPullParserException 146 * @throws FHIRFormatError 147 * @throws IOException 148 */ 149 public Type parseType(InputStream input, String knownType) throws IOException, FHIRFormatError; 150 151 public Type parseAnyType(InputStream input, String knownType) throws IOException, FHIRFormatError; 152 153 /** 154 * This is used to parse a type - a fragment of a resource. There's no reason to 155 * use this in production - it's used in the build tools 156 * 157 * Not supported by all implementations 158 * 159 * @param input 160 * @param knownType. if this is blank, the parser may try to infer the type (xml 161 * only) 162 * @return 163 * @throws UnsupportedEncodingException 164 * @throws IOException 165 * @throws FHIRFormatError 166 */ 167 public Type parseType(String input, String knownType) 168 throws UnsupportedEncodingException, FHIRFormatError, IOException; 169 170 /** 171 * This is used to parse a type - a fragment of a resource. There's no reason to 172 * use this in production - it's used in the build tools 173 * 174 * Not supported by all implementations 175 * 176 * @param input 177 * @param knownType. if this is blank, the parser may try to infer the type (xml 178 * only) 179 * @return 180 * @throws IOException 181 * @throws FHIRFormatError 182 */ 183 public Type parseType(byte[] bytes, String knownType) throws FHIRFormatError, IOException; 184 185 // -- Writing methods ---------------------------------------- 186 187 /** 188 * Compose a resource to a stream, possibly using pretty presentation for a 189 * human reader (used in the spec, for example, but not normally in production) 190 * 191 * @throws IOException 192 */ 193 public void compose(OutputStream stream, Resource resource) throws IOException; 194 195 /** 196 * Compose a resource to a stream, possibly using pretty presentation for a 197 * human reader (used in the spec, for example, but not normally in production) 198 * 199 * @throws IOException 200 */ 201 public String composeString(Resource resource) throws IOException; 202 203 /** 204 * Compose a resource to a stream, possibly using pretty presentation for a 205 * human reader (used in the spec, for example, but not normally in production) 206 * 207 * @throws IOException 208 */ 209 public byte[] composeBytes(Resource resource) throws IOException; 210 211 /** 212 * Compose a type to a stream, possibly using pretty presentation for a human 213 * reader (used in the spec, for example, but not normally in production) 214 * 215 * Not supported by all implementations. rootName is ignored in the JSON format 216 * 217 * @throws XmlPullParserException 218 * @throws FHIRFormatError 219 * @throws IOException 220 */ 221 public void compose(OutputStream stream, Type type, String rootName) throws IOException; 222 223 /** 224 * Compose a type to a stream, possibly using pretty presentation for a human 225 * reader (used in the spec, for example, but not normally in production) 226 * 227 * Not supported by all implementations. rootName is ignored in the JSON format 228 * 229 * @throws IOException 230 */ 231 public String composeString(Type type, String rootName) throws IOException; 232 233 /** 234 * Compose a type to a stream, possibly using pretty presentation for a human 235 * reader (used in the spec, for example, but not normally in production) 236 * 237 * Not supported by all implementations. rootName is ignored in the JSON format 238 * 239 * @throws IOException 240 */ 241 public byte[] composeBytes(Type type, String rootName) throws IOException; 242 243}