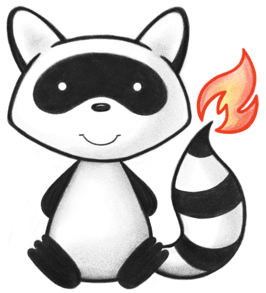
001package org.hl7.fhir.r4.formats; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.IOException; 033import java.io.Writer; 034import java.math.BigDecimal; 035 036import org.hl7.fhir.utilities.Utilities; 037 038/** 039 * A little implementation of a json write to replace Gson .... because Gson 040 * screws up decimal values, and *we care* 041 * 042 * @author Grahame Grieve 043 * 044 */ 045public class JsonCreatorDirect implements JsonCreator { 046 047 private Writer writer; 048 private boolean pretty; 049 private boolean named; 050 private boolean valued; 051 private int indent; 052 053 public JsonCreatorDirect(Writer writer) { 054 super(); 055 this.writer = writer; 056 } 057 058 @Override 059 public void setIndent(String indent) { 060 this.pretty = !Utilities.noString(indent); 061 } 062 063 @Override 064 public void beginObject() throws IOException { 065 checkState(); 066 writer.write("{"); 067 stepIn(); 068 } 069 070 public void stepIn() throws IOException { 071 if (pretty) { 072 indent++; 073 writer.write("\r\n"); 074 for (int i = 0; i < indent; i++) { 075 writer.write(" "); 076 } 077 } 078 } 079 080 public void stepOut() throws IOException { 081 if (pretty) { 082 indent--; 083 writer.write("\r\n"); 084 for (int i = 0; i < indent; i++) { 085 writer.write(" "); 086 } 087 } 088 } 089 090 private void checkState() throws IOException { 091 if (named) { 092 if (pretty) 093 writer.write(" : "); 094 else 095 writer.write(":"); 096 named = false; 097 } 098 if (valued) { 099 writer.write(","); 100 if (pretty) { 101 writer.write("\r\n"); 102 for (int i = 0; i < indent; i++) { 103 writer.write(" "); 104 } 105 } 106 valued = false; 107 } 108 } 109 110 @Override 111 public void endObject() throws IOException { 112 stepOut(); 113 writer.write("}"); 114 } 115 116 @Override 117 public void nullValue() throws IOException { 118 checkState(); 119 writer.write("null"); 120 valued = true; 121 } 122 123 @Override 124 public void name(String name) throws IOException { 125 checkState(); 126 writer.write("\"" + name + "\""); 127 named = true; 128 } 129 130 @Override 131 public void value(String value) throws IOException { 132 checkState(); 133 writer.write("\"" + Utilities.escapeJson(value) + "\""); 134 valued = true; 135 } 136 137 @Override 138 public void value(Boolean value) throws IOException { 139 checkState(); 140 if (value == null) 141 writer.write("null"); 142 else if (value.booleanValue()) 143 writer.write("true"); 144 else 145 writer.write("false"); 146 valued = true; 147 } 148 149 @Override 150 public void value(BigDecimal value) throws IOException { 151 checkState(); 152 if (value == null) 153 writer.write("null"); 154 else 155 writer.write(value.toString()); 156 valued = true; 157 } 158 159 @Override 160 public void valueNum(String value) throws IOException { 161 checkState(); 162 if (value == null) 163 writer.write("null"); 164 else 165 writer.write(value); 166 valued = true; 167 } 168 169 @Override 170 public void value(Integer value) throws IOException { 171 checkState(); 172 if (value == null) 173 writer.write("null"); 174 else 175 writer.write(value.toString()); 176 valued = true; 177 } 178 179 @Override 180 public void beginArray() throws IOException { 181 checkState(); 182 writer.write("["); 183 } 184 185 @Override 186 public void endArray() throws IOException { 187 writer.write("]"); 188 } 189 190 @Override 191 public void finish() throws IOException { 192 // nothing 193 } 194 195 @Override 196 public void link(String href) { 197 // not used 198 199 } 200 201}