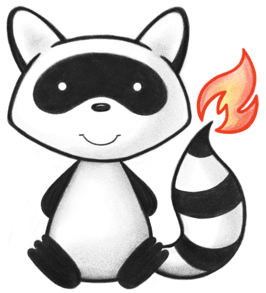
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2015 University Health Network 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.r4.hapi.rest.server; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.api.BundleInclusionRule; 024import ca.uhn.fhir.model.api.Include; 025import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 026import ca.uhn.fhir.model.valueset.BundleEntrySearchModeEnum; 027import ca.uhn.fhir.model.valueset.BundleEntryTransactionMethodEnum; 028import ca.uhn.fhir.model.valueset.BundleTypeEnum; 029import ca.uhn.fhir.rest.api.BundleLinks; 030import ca.uhn.fhir.rest.api.Constants; 031import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 032import ca.uhn.fhir.rest.server.RestfulServerUtils; 033import ca.uhn.fhir.util.ResourceReferenceInfo; 034import jakarta.annotation.Nonnull; 035import jakarta.annotation.Nullable; 036import org.hl7.fhir.instance.model.api.IAnyResource; 037import org.hl7.fhir.instance.model.api.IBaseResource; 038import org.hl7.fhir.instance.model.api.IIdType; 039import org.hl7.fhir.instance.model.api.IPrimitiveType; 040import org.hl7.fhir.r4.model.Bundle; 041import org.hl7.fhir.r4.model.Bundle.BundleEntryComponent; 042import org.hl7.fhir.r4.model.Bundle.BundleLinkComponent; 043import org.hl7.fhir.r4.model.Bundle.SearchEntryMode; 044import org.hl7.fhir.r4.model.DomainResource; 045import org.hl7.fhir.r4.model.IdType; 046import org.hl7.fhir.r4.model.Resource; 047 048import java.math.BigDecimal; 049import java.util.ArrayList; 050import java.util.Date; 051import java.util.HashSet; 052import java.util.List; 053import java.util.Set; 054import java.util.UUID; 055 056import static org.apache.commons.lang3.StringUtils.isNotBlank; 057 058@SuppressWarnings("Duplicates") 059public class R4BundleFactory implements IVersionSpecificBundleFactory { 060 private String myBase; 061 private Bundle myBundle; 062 private final FhirContext myContext; 063 064 public R4BundleFactory(FhirContext theContext) { 065 myContext = theContext; 066 } 067 068 @Override 069 public void addResourcesToBundle( 070 List<IBaseResource> theResult, 071 BundleTypeEnum theBundleType, 072 String theServerBase, 073 BundleInclusionRule theBundleInclusionRule, 074 Set<Include> theIncludes) { 075 ensureBundle(); 076 077 List<IAnyResource> includedResources = new ArrayList<>(); 078 Set<IIdType> addedResourceIds = new HashSet<>(); 079 080 for (IBaseResource next : theResult) { 081 if (!next.getIdElement().isEmpty()) { 082 addedResourceIds.add(next.getIdElement()); 083 } 084 } 085 086 for (IBaseResource next : theResult) { 087 088 Set<String> containedIds = new HashSet<>(); 089 090 if (next instanceof DomainResource) { 091 for (Resource nextContained : ((DomainResource) next).getContained()) { 092 if (isNotBlank(nextContained.getId())) { 093 containedIds.add(nextContained.getId()); 094 } 095 } 096 } 097 098 List<ResourceReferenceInfo> references = myContext.newTerser().getAllResourceReferences(next); 099 do { 100 List<IAnyResource> addedResourcesThisPass = new ArrayList<>(); 101 102 for (ResourceReferenceInfo nextRefInfo : references) { 103 if (theBundleInclusionRule != null 104 && !theBundleInclusionRule.shouldIncludeReferencedResource(nextRefInfo, theIncludes)) { 105 continue; 106 } 107 108 IAnyResource nextRes = 109 (IAnyResource) nextRefInfo.getResourceReference().getResource(); 110 if (nextRes != null) { 111 if (nextRes.getIdElement().hasIdPart()) { 112 if (containedIds.contains(nextRes.getIdElement().getValue())) { 113 // Don't add contained IDs as top level resources 114 continue; 115 } 116 117 IIdType id = nextRes.getIdElement(); 118 if (!id.hasResourceType()) { 119 String resName = myContext.getResourceType(nextRes); 120 id = id.withResourceType(resName); 121 } 122 123 if (!addedResourceIds.contains(id)) { 124 addedResourceIds.add(id); 125 addedResourcesThisPass.add(nextRes); 126 } 127 } 128 } 129 } 130 131 includedResources.addAll(addedResourcesThisPass); 132 133 // Linked resources may themselves have linked resources 134 references = new ArrayList<>(); 135 for (IAnyResource iResource : addedResourcesThisPass) { 136 List<ResourceReferenceInfo> newReferences = 137 myContext.newTerser().getAllResourceReferences(iResource); 138 references.addAll(newReferences); 139 } 140 } while (!references.isEmpty()); 141 142 BundleEntryComponent entry = myBundle.addEntry().setResource((Resource) next); 143 Resource nextAsResource = (Resource) next; 144 IIdType id = populateBundleEntryFullUrl(next, entry); 145 146 // Populate Request 147 BundleEntryTransactionMethodEnum httpVerb = 148 ResourceMetadataKeyEnum.ENTRY_TRANSACTION_METHOD.get(nextAsResource); 149 if (httpVerb != null) { 150 entry.getRequest().getMethodElement().setValueAsString(httpVerb.name()); 151 if (id != null) { 152 entry.getRequest().setUrl(id.toUnqualified().getValue()); 153 } 154 } 155 if (BundleEntryTransactionMethodEnum.DELETE.equals(httpVerb)) { 156 entry.setResource(null); 157 } 158 159 // Populate Bundle.entry.response 160 if (theBundleType != null) { 161 switch (theBundleType) { 162 case BATCH_RESPONSE: 163 case TRANSACTION_RESPONSE: 164 case HISTORY: 165 if (id != null) { 166 String version = id.getVersionIdPart(); 167 if ("1".equals(version)) { 168 entry.getResponse().setStatus("201 Created"); 169 } else if (isNotBlank(version)) { 170 entry.getResponse().setStatus("200 OK"); 171 } 172 if (isNotBlank(version)) { 173 entry.getResponse().setEtag(RestfulServerUtils.createEtag(version)); 174 } 175 } 176 break; 177 } 178 } 179 180 // Populate Bundle.entry.search 181 BundleEntrySearchModeEnum searchMode = ResourceMetadataKeyEnum.ENTRY_SEARCH_MODE.get(nextAsResource); 182 if (searchMode != null) { 183 entry.getSearch().getModeElement().setValueAsString(searchMode.getCode()); 184 } 185 BigDecimal searchScore = ResourceMetadataKeyEnum.ENTRY_SEARCH_SCORE.get(nextAsResource); 186 if (searchScore != null) { 187 entry.getSearch().getScoreElement().setValue(searchScore); 188 } 189 } 190 191 /* 192 * Actually add the resources to the bundle 193 */ 194 for (IAnyResource next : includedResources) { 195 BundleEntryComponent entry = myBundle.addEntry(); 196 entry.setResource((Resource) next).getSearch().setMode(SearchEntryMode.INCLUDE); 197 populateBundleEntryFullUrl(next, entry); 198 } 199 } 200 201 @Override 202 public void addRootPropertiesToBundle( 203 String theId, 204 @Nonnull BundleLinks theBundleLinks, 205 Integer theTotalResults, 206 IPrimitiveType<Date> theLastUpdated) { 207 ensureBundle(); 208 209 myBase = theBundleLinks.serverBase; 210 211 if (myBundle.getIdElement().isEmpty()) { 212 myBundle.setId(theId); 213 } 214 215 if (myBundle.getMeta().getLastUpdated() == null && theLastUpdated != null) { 216 myBundle.getMeta().getLastUpdatedElement().setValueAsString(theLastUpdated.getValueAsString()); 217 } 218 219 if (hasNoLinkOfType(Constants.LINK_SELF, myBundle) && isNotBlank(theBundleLinks.getSelf())) { 220 myBundle.addLink().setRelation(Constants.LINK_SELF).setUrl(theBundleLinks.getSelf()); 221 } 222 if (hasNoLinkOfType(Constants.LINK_NEXT, myBundle) && isNotBlank(theBundleLinks.getNext())) { 223 myBundle.addLink().setRelation(Constants.LINK_NEXT).setUrl(theBundleLinks.getNext()); 224 } 225 if (hasNoLinkOfType(Constants.LINK_PREVIOUS, myBundle) && isNotBlank(theBundleLinks.getPrev())) { 226 myBundle.addLink().setRelation(Constants.LINK_PREVIOUS).setUrl(theBundleLinks.getPrev()); 227 } 228 229 addTotalResultsToBundle(theTotalResults, theBundleLinks.bundleType); 230 } 231 232 @Override 233 public void addTotalResultsToBundle(Integer theTotalResults, BundleTypeEnum theBundleType) { 234 ensureBundle(); 235 236 if (myBundle.getIdElement().isEmpty()) { 237 myBundle.setId(UUID.randomUUID().toString()); 238 } 239 240 if (myBundle.getTypeElement().isEmpty() && theBundleType != null) { 241 myBundle.getTypeElement().setValueAsString(theBundleType.getCode()); 242 } 243 244 if (myBundle.getTotalElement().isEmpty() && theTotalResults != null) { 245 myBundle.getTotalElement().setValue(theTotalResults); 246 } 247 } 248 249 private void ensureBundle() { 250 if (myBundle == null) { 251 myBundle = new Bundle(); 252 } 253 } 254 255 @Override 256 public IBaseResource getResourceBundle() { 257 return myBundle; 258 } 259 260 private boolean hasNoLinkOfType(String theLinkType, Bundle theBundle) { 261 for (BundleLinkComponent next : theBundle.getLink()) { 262 if (theLinkType.equals(next.getRelation())) { 263 return false; 264 } 265 } 266 return true; 267 } 268 269 @Override 270 public void initializeWithBundleResource(IBaseResource theBundle) { 271 myBundle = (Bundle) theBundle; 272 } 273 274 @Nullable 275 private IIdType populateBundleEntryFullUrl(IBaseResource theResource, BundleEntryComponent theEntry) { 276 final IIdType idElement; 277 if (theResource.getIdElement().hasBaseUrl()) { 278 idElement = theResource.getIdElement(); 279 theEntry.setFullUrl(idElement.toVersionless().getValue()); 280 } else { 281 if (isNotBlank(myBase) && theResource.getIdElement().hasIdPart()) { 282 idElement = theResource.getIdElement().withServerBase(myBase, myContext.getResourceType(theResource)); 283 theEntry.setFullUrl(idElement.toVersionless().getValue()); 284 } else { 285 idElement = null; 286 } 287 } 288 return idElement; 289 } 290 291 @Override 292 public List<IBaseResource> toListOfResources() { 293 ArrayList<IBaseResource> retVal = new ArrayList<>(); 294 for (BundleEntryComponent next : myBundle.getEntry()) { 295 if (next.getResource() != null) { 296 retVal.add(next.getResource()); 297 } else if (!next.getResponse().getLocationElement().isEmpty()) { 298 IdType id = new IdType(next.getResponse().getLocation()); 299 String resourceType = id.getResourceType(); 300 if (isNotBlank(resourceType)) { 301 IAnyResource res = (IAnyResource) 302 myContext.getResourceDefinition(resourceType).newInstance(); 303 res.setId(id); 304 retVal.add(res); 305 } 306 } 307 } 308 return retVal; 309 } 310}