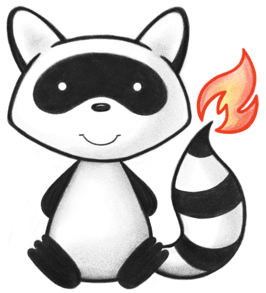
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A financial tool for tracking value accrued for a particular purpose. In the 048 * healthcare field, used to track charges for a patient, cost centers, etc. 049 */ 050@ResourceDef(name = "Account", profile = "http://hl7.org/fhir/StructureDefinition/Account") 051public class Account extends DomainResource { 052 053 public enum AccountStatus { 054 /** 055 * This account is active and may be used. 056 */ 057 ACTIVE, 058 /** 059 * This account is inactive and should not be used to track financial 060 * information. 061 */ 062 INACTIVE, 063 /** 064 * This instance should not have been part of this patient's medical record. 065 */ 066 ENTEREDINERROR, 067 /** 068 * This account is on hold. 069 */ 070 ONHOLD, 071 /** 072 * The account status is unknown. 073 */ 074 UNKNOWN, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static AccountStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("inactive".equals(codeString)) 086 return INACTIVE; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if ("on-hold".equals(codeString)) 090 return ONHOLD; 091 if ("unknown".equals(codeString)) 092 return UNKNOWN; 093 if (Configuration.isAcceptInvalidEnums()) 094 return null; 095 else 096 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case ACTIVE: 102 return "active"; 103 case INACTIVE: 104 return "inactive"; 105 case ENTEREDINERROR: 106 return "entered-in-error"; 107 case ONHOLD: 108 return "on-hold"; 109 case UNKNOWN: 110 return "unknown"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case ACTIVE: 121 return "http://hl7.org/fhir/account-status"; 122 case INACTIVE: 123 return "http://hl7.org/fhir/account-status"; 124 case ENTEREDINERROR: 125 return "http://hl7.org/fhir/account-status"; 126 case ONHOLD: 127 return "http://hl7.org/fhir/account-status"; 128 case UNKNOWN: 129 return "http://hl7.org/fhir/account-status"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case ACTIVE: 140 return "This account is active and may be used."; 141 case INACTIVE: 142 return "This account is inactive and should not be used to track financial information."; 143 case ENTEREDINERROR: 144 return "This instance should not have been part of this patient's medical record."; 145 case ONHOLD: 146 return "This account is on hold."; 147 case UNKNOWN: 148 return "The account status is unknown."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case ACTIVE: 159 return "Active"; 160 case INACTIVE: 161 return "Inactive"; 162 case ENTEREDINERROR: 163 return "Entered in error"; 164 case ONHOLD: 165 return "On Hold"; 166 case UNKNOWN: 167 return "Unknown"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class AccountStatusEnumFactory implements EnumFactory<AccountStatus> { 177 public AccountStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("active".equals(codeString)) 182 return AccountStatus.ACTIVE; 183 if ("inactive".equals(codeString)) 184 return AccountStatus.INACTIVE; 185 if ("entered-in-error".equals(codeString)) 186 return AccountStatus.ENTEREDINERROR; 187 if ("on-hold".equals(codeString)) 188 return AccountStatus.ONHOLD; 189 if ("unknown".equals(codeString)) 190 return AccountStatus.UNKNOWN; 191 throw new IllegalArgumentException("Unknown AccountStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<AccountStatus> fromType(PrimitiveType<?> code) throws FHIRException { 195 if (code == null) 196 return null; 197 if (code.isEmpty()) 198 return new Enumeration<AccountStatus>(this, AccountStatus.NULL, code); 199 String codeString = code.asStringValue(); 200 if (codeString == null || "".equals(codeString)) 201 return new Enumeration<AccountStatus>(this, AccountStatus.NULL, code); 202 if ("active".equals(codeString)) 203 return new Enumeration<AccountStatus>(this, AccountStatus.ACTIVE, code); 204 if ("inactive".equals(codeString)) 205 return new Enumeration<AccountStatus>(this, AccountStatus.INACTIVE, code); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<AccountStatus>(this, AccountStatus.ENTEREDINERROR, code); 208 if ("on-hold".equals(codeString)) 209 return new Enumeration<AccountStatus>(this, AccountStatus.ONHOLD, code); 210 if ("unknown".equals(codeString)) 211 return new Enumeration<AccountStatus>(this, AccountStatus.UNKNOWN, code); 212 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 213 } 214 215 public String toCode(AccountStatus code) { 216 if (code == AccountStatus.ACTIVE) 217 return "active"; 218 if (code == AccountStatus.INACTIVE) 219 return "inactive"; 220 if (code == AccountStatus.ENTEREDINERROR) 221 return "entered-in-error"; 222 if (code == AccountStatus.ONHOLD) 223 return "on-hold"; 224 if (code == AccountStatus.UNKNOWN) 225 return "unknown"; 226 return "?"; 227 } 228 229 public String toSystem(AccountStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class CoverageComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * The party(s) that contribute to payment (or part of) of the charges applied 238 * to this account (including self-pay). 239 * 240 * A coverage may only be responsible for specific types of charges, and the 241 * sequence of the coverages in the account could be important when processing 242 * billing. 243 */ 244 @Child(name = "coverage", type = { Coverage.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 245 @Description(shortDefinition = "The party(s), such as insurances, that may contribute to the payment of this account", formalDefinition = "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.") 246 protected Reference coverage; 247 248 /** 249 * The actual object that is the target of the reference (The party(s) that 250 * contribute to payment (or part of) of the charges applied to this account 251 * (including self-pay). 252 * 253 * A coverage may only be responsible for specific types of charges, and the 254 * sequence of the coverages in the account could be important when processing 255 * billing.) 256 */ 257 protected Coverage coverageTarget; 258 259 /** 260 * The priority of the coverage in the context of this account. 261 */ 262 @Child(name = "priority", type = { 263 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 264 @Description(shortDefinition = "The priority of the coverage in the context of this account", formalDefinition = "The priority of the coverage in the context of this account.") 265 protected PositiveIntType priority; 266 267 private static final long serialVersionUID = -1046265008L; 268 269 /** 270 * Constructor 271 */ 272 public CoverageComponent() { 273 super(); 274 } 275 276 /** 277 * Constructor 278 */ 279 public CoverageComponent(Reference coverage) { 280 super(); 281 this.coverage = coverage; 282 } 283 284 /** 285 * @return {@link #coverage} (The party(s) that contribute to payment (or part 286 * of) of the charges applied to this account (including self-pay). 287 * 288 * A coverage may only be responsible for specific types of charges, and 289 * the sequence of the coverages in the account could be important when 290 * processing billing.) 291 */ 292 public Reference getCoverage() { 293 if (this.coverage == null) 294 if (Configuration.errorOnAutoCreate()) 295 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 296 else if (Configuration.doAutoCreate()) 297 this.coverage = new Reference(); // cc 298 return this.coverage; 299 } 300 301 public boolean hasCoverage() { 302 return this.coverage != null && !this.coverage.isEmpty(); 303 } 304 305 /** 306 * @param value {@link #coverage} (The party(s) that contribute to payment (or 307 * part of) of the charges applied to this account (including 308 * self-pay). 309 * 310 * A coverage may only be responsible for specific types of 311 * charges, and the sequence of the coverages in the account could 312 * be important when processing billing.) 313 */ 314 public CoverageComponent setCoverage(Reference value) { 315 this.coverage = value; 316 return this; 317 } 318 319 /** 320 * @return {@link #coverage} The actual object that is the target of the 321 * reference. The reference library doesn't populate this, but you can 322 * use it to hold the resource if you resolve it. (The party(s) that 323 * contribute to payment (or part of) of the charges applied to this 324 * account (including self-pay). 325 * 326 * A coverage may only be responsible for specific types of charges, and 327 * the sequence of the coverages in the account could be important when 328 * processing billing.) 329 */ 330 public Coverage getCoverageTarget() { 331 if (this.coverageTarget == null) 332 if (Configuration.errorOnAutoCreate()) 333 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 334 else if (Configuration.doAutoCreate()) 335 this.coverageTarget = new Coverage(); // aa 336 return this.coverageTarget; 337 } 338 339 /** 340 * @param value {@link #coverage} The actual object that is the target of the 341 * reference. The reference library doesn't use these, but you can 342 * use it to hold the resource if you resolve it. (The party(s) 343 * that contribute to payment (or part of) of the charges applied 344 * to this account (including self-pay). 345 * 346 * A coverage may only be responsible for specific types of 347 * charges, and the sequence of the coverages in the account could 348 * be important when processing billing.) 349 */ 350 public CoverageComponent setCoverageTarget(Coverage value) { 351 this.coverageTarget = value; 352 return this; 353 } 354 355 /** 356 * @return {@link #priority} (The priority of the coverage in the context of 357 * this account.). This is the underlying object with id, value and 358 * extensions. The accessor "getPriority" gives direct access to the 359 * value 360 */ 361 public PositiveIntType getPriorityElement() { 362 if (this.priority == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create CoverageComponent.priority"); 365 else if (Configuration.doAutoCreate()) 366 this.priority = new PositiveIntType(); // bb 367 return this.priority; 368 } 369 370 public boolean hasPriorityElement() { 371 return this.priority != null && !this.priority.isEmpty(); 372 } 373 374 public boolean hasPriority() { 375 return this.priority != null && !this.priority.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #priority} (The priority of the coverage in the context 380 * of this account.). This is the underlying object with id, value 381 * and extensions. The accessor "getPriority" gives direct access 382 * to the value 383 */ 384 public CoverageComponent setPriorityElement(PositiveIntType value) { 385 this.priority = value; 386 return this; 387 } 388 389 /** 390 * @return The priority of the coverage in the context of this account. 391 */ 392 public int getPriority() { 393 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 394 } 395 396 /** 397 * @param value The priority of the coverage in the context of this account. 398 */ 399 public CoverageComponent setPriority(int value) { 400 if (this.priority == null) 401 this.priority = new PositiveIntType(); 402 this.priority.setValue(value); 403 return this; 404 } 405 406 protected void listChildren(List<Property> children) { 407 super.listChildren(children); 408 children.add(new Property("coverage", "Reference(Coverage)", 409 "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 410 0, 1, coverage)); 411 children.add(new Property("priority", "positiveInt", 412 "The priority of the coverage in the context of this account.", 0, 1, priority)); 413 } 414 415 @Override 416 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 417 switch (_hash) { 418 case -351767064: 419 /* coverage */ return new Property("coverage", "Reference(Coverage)", 420 "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 421 0, 1, coverage); 422 case -1165461084: 423 /* priority */ return new Property("priority", "positiveInt", 424 "The priority of the coverage in the context of this account.", 0, 1, priority); 425 default: 426 return super.getNamedProperty(_hash, _name, _checkValid); 427 } 428 429 } 430 431 @Override 432 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 433 switch (hash) { 434 case -351767064: 435 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 436 case -1165461084: 437 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // PositiveIntType 438 default: 439 return super.getProperty(hash, name, checkValid); 440 } 441 442 } 443 444 @Override 445 public Base setProperty(int hash, String name, Base value) throws FHIRException { 446 switch (hash) { 447 case -351767064: // coverage 448 this.coverage = castToReference(value); // Reference 449 return value; 450 case -1165461084: // priority 451 this.priority = castToPositiveInt(value); // PositiveIntType 452 return value; 453 default: 454 return super.setProperty(hash, name, value); 455 } 456 457 } 458 459 @Override 460 public Base setProperty(String name, Base value) throws FHIRException { 461 if (name.equals("coverage")) { 462 this.coverage = castToReference(value); // Reference 463 } else if (name.equals("priority")) { 464 this.priority = castToPositiveInt(value); // PositiveIntType 465 } else 466 return super.setProperty(name, value); 467 return value; 468 } 469 470 @Override 471 public Base makeProperty(int hash, String name) throws FHIRException { 472 switch (hash) { 473 case -351767064: 474 return getCoverage(); 475 case -1165461084: 476 return getPriorityElement(); 477 default: 478 return super.makeProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case -351767064: 487 /* coverage */ return new String[] { "Reference" }; 488 case -1165461084: 489 /* priority */ return new String[] { "positiveInt" }; 490 default: 491 return super.getTypesForProperty(hash, name); 492 } 493 494 } 495 496 @Override 497 public Base addChild(String name) throws FHIRException { 498 if (name.equals("coverage")) { 499 this.coverage = new Reference(); 500 return this.coverage; 501 } else if (name.equals("priority")) { 502 throw new FHIRException("Cannot call addChild on a singleton property Account.priority"); 503 } else 504 return super.addChild(name); 505 } 506 507 public CoverageComponent copy() { 508 CoverageComponent dst = new CoverageComponent(); 509 copyValues(dst); 510 return dst; 511 } 512 513 public void copyValues(CoverageComponent dst) { 514 super.copyValues(dst); 515 dst.coverage = coverage == null ? null : coverage.copy(); 516 dst.priority = priority == null ? null : priority.copy(); 517 } 518 519 @Override 520 public boolean equalsDeep(Base other_) { 521 if (!super.equalsDeep(other_)) 522 return false; 523 if (!(other_ instanceof CoverageComponent)) 524 return false; 525 CoverageComponent o = (CoverageComponent) other_; 526 return compareDeep(coverage, o.coverage, true) && compareDeep(priority, o.priority, true); 527 } 528 529 @Override 530 public boolean equalsShallow(Base other_) { 531 if (!super.equalsShallow(other_)) 532 return false; 533 if (!(other_ instanceof CoverageComponent)) 534 return false; 535 CoverageComponent o = (CoverageComponent) other_; 536 return compareValues(priority, o.priority, true); 537 } 538 539 public boolean isEmpty() { 540 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, priority); 541 } 542 543 public String fhirType() { 544 return "Account.coverage"; 545 546 } 547 548 } 549 550 @Block() 551 public static class GuarantorComponent extends BackboneElement implements IBaseBackboneElement { 552 /** 553 * The entity who is responsible. 554 */ 555 @Child(name = "party", type = { Patient.class, RelatedPerson.class, 556 Organization.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 557 @Description(shortDefinition = "Responsible entity", formalDefinition = "The entity who is responsible.") 558 protected Reference party; 559 560 /** 561 * The actual object that is the target of the reference (The entity who is 562 * responsible.) 563 */ 564 protected Resource partyTarget; 565 566 /** 567 * A guarantor may be placed on credit hold or otherwise have their role 568 * temporarily suspended. 569 */ 570 @Child(name = "onHold", type = { 571 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 572 @Description(shortDefinition = "Credit or other hold applied", formalDefinition = "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.") 573 protected BooleanType onHold; 574 575 /** 576 * The timeframe during which the guarantor accepts responsibility for the 577 * account. 578 */ 579 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 580 @Description(shortDefinition = "Guarantee account during", formalDefinition = "The timeframe during which the guarantor accepts responsibility for the account.") 581 protected Period period; 582 583 private static final long serialVersionUID = -1012345396L; 584 585 /** 586 * Constructor 587 */ 588 public GuarantorComponent() { 589 super(); 590 } 591 592 /** 593 * Constructor 594 */ 595 public GuarantorComponent(Reference party) { 596 super(); 597 this.party = party; 598 } 599 600 /** 601 * @return {@link #party} (The entity who is responsible.) 602 */ 603 public Reference getParty() { 604 if (this.party == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create GuarantorComponent.party"); 607 else if (Configuration.doAutoCreate()) 608 this.party = new Reference(); // cc 609 return this.party; 610 } 611 612 public boolean hasParty() { 613 return this.party != null && !this.party.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #party} (The entity who is responsible.) 618 */ 619 public GuarantorComponent setParty(Reference value) { 620 this.party = value; 621 return this; 622 } 623 624 /** 625 * @return {@link #party} The actual object that is the target of the reference. 626 * The reference library doesn't populate this, but you can use it to 627 * hold the resource if you resolve it. (The entity who is responsible.) 628 */ 629 public Resource getPartyTarget() { 630 return this.partyTarget; 631 } 632 633 /** 634 * @param value {@link #party} The actual object that is the target of the 635 * reference. The reference library doesn't use these, but you can 636 * use it to hold the resource if you resolve it. (The entity who 637 * is responsible.) 638 */ 639 public GuarantorComponent setPartyTarget(Resource value) { 640 this.partyTarget = value; 641 return this; 642 } 643 644 /** 645 * @return {@link #onHold} (A guarantor may be placed on credit hold or 646 * otherwise have their role temporarily suspended.). This is the 647 * underlying object with id, value and extensions. The accessor 648 * "getOnHold" gives direct access to the value 649 */ 650 public BooleanType getOnHoldElement() { 651 if (this.onHold == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create GuarantorComponent.onHold"); 654 else if (Configuration.doAutoCreate()) 655 this.onHold = new BooleanType(); // bb 656 return this.onHold; 657 } 658 659 public boolean hasOnHoldElement() { 660 return this.onHold != null && !this.onHold.isEmpty(); 661 } 662 663 public boolean hasOnHold() { 664 return this.onHold != null && !this.onHold.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #onHold} (A guarantor may be placed on credit hold or 669 * otherwise have their role temporarily suspended.). This is the 670 * underlying object with id, value and extensions. The accessor 671 * "getOnHold" gives direct access to the value 672 */ 673 public GuarantorComponent setOnHoldElement(BooleanType value) { 674 this.onHold = value; 675 return this; 676 } 677 678 /** 679 * @return A guarantor may be placed on credit hold or otherwise have their role 680 * temporarily suspended. 681 */ 682 public boolean getOnHold() { 683 return this.onHold == null || this.onHold.isEmpty() ? false : this.onHold.getValue(); 684 } 685 686 /** 687 * @param value A guarantor may be placed on credit hold or otherwise have their 688 * role temporarily suspended. 689 */ 690 public GuarantorComponent setOnHold(boolean value) { 691 if (this.onHold == null) 692 this.onHold = new BooleanType(); 693 this.onHold.setValue(value); 694 return this; 695 } 696 697 /** 698 * @return {@link #period} (The timeframe during which the guarantor accepts 699 * responsibility for the account.) 700 */ 701 public Period getPeriod() { 702 if (this.period == null) 703 if (Configuration.errorOnAutoCreate()) 704 throw new Error("Attempt to auto-create GuarantorComponent.period"); 705 else if (Configuration.doAutoCreate()) 706 this.period = new Period(); // cc 707 return this.period; 708 } 709 710 public boolean hasPeriod() { 711 return this.period != null && !this.period.isEmpty(); 712 } 713 714 /** 715 * @param value {@link #period} (The timeframe during which the guarantor 716 * accepts responsibility for the account.) 717 */ 718 public GuarantorComponent setPeriod(Period value) { 719 this.period = value; 720 return this; 721 } 722 723 protected void listChildren(List<Property> children) { 724 super.listChildren(children); 725 children.add(new Property("party", "Reference(Patient|RelatedPerson|Organization)", 726 "The entity who is responsible.", 0, 1, party)); 727 children.add(new Property("onHold", "boolean", 728 "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, 729 onHold)); 730 children.add(new Property("period", "Period", 731 "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period)); 732 } 733 734 @Override 735 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 736 switch (_hash) { 737 case 106437350: 738 /* party */ return new Property("party", "Reference(Patient|RelatedPerson|Organization)", 739 "The entity who is responsible.", 0, 1, party); 740 case -1013289154: 741 /* onHold */ return new Property("onHold", "boolean", 742 "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, 743 onHold); 744 case -991726143: 745 /* period */ return new Property("period", "Period", 746 "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period); 747 default: 748 return super.getNamedProperty(_hash, _name, _checkValid); 749 } 750 751 } 752 753 @Override 754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 755 switch (hash) { 756 case 106437350: 757 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 758 case -1013289154: 759 /* onHold */ return this.onHold == null ? new Base[0] : new Base[] { this.onHold }; // BooleanType 760 case -991726143: 761 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 762 default: 763 return super.getProperty(hash, name, checkValid); 764 } 765 766 } 767 768 @Override 769 public Base setProperty(int hash, String name, Base value) throws FHIRException { 770 switch (hash) { 771 case 106437350: // party 772 this.party = castToReference(value); // Reference 773 return value; 774 case -1013289154: // onHold 775 this.onHold = castToBoolean(value); // BooleanType 776 return value; 777 case -991726143: // period 778 this.period = castToPeriod(value); // Period 779 return value; 780 default: 781 return super.setProperty(hash, name, value); 782 } 783 784 } 785 786 @Override 787 public Base setProperty(String name, Base value) throws FHIRException { 788 if (name.equals("party")) { 789 this.party = castToReference(value); // Reference 790 } else if (name.equals("onHold")) { 791 this.onHold = castToBoolean(value); // BooleanType 792 } else if (name.equals("period")) { 793 this.period = castToPeriod(value); // Period 794 } else 795 return super.setProperty(name, value); 796 return value; 797 } 798 799 @Override 800 public Base makeProperty(int hash, String name) throws FHIRException { 801 switch (hash) { 802 case 106437350: 803 return getParty(); 804 case -1013289154: 805 return getOnHoldElement(); 806 case -991726143: 807 return getPeriod(); 808 default: 809 return super.makeProperty(hash, name); 810 } 811 812 } 813 814 @Override 815 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 816 switch (hash) { 817 case 106437350: 818 /* party */ return new String[] { "Reference" }; 819 case -1013289154: 820 /* onHold */ return new String[] { "boolean" }; 821 case -991726143: 822 /* period */ return new String[] { "Period" }; 823 default: 824 return super.getTypesForProperty(hash, name); 825 } 826 827 } 828 829 @Override 830 public Base addChild(String name) throws FHIRException { 831 if (name.equals("party")) { 832 this.party = new Reference(); 833 return this.party; 834 } else if (name.equals("onHold")) { 835 throw new FHIRException("Cannot call addChild on a singleton property Account.onHold"); 836 } else if (name.equals("period")) { 837 this.period = new Period(); 838 return this.period; 839 } else 840 return super.addChild(name); 841 } 842 843 public GuarantorComponent copy() { 844 GuarantorComponent dst = new GuarantorComponent(); 845 copyValues(dst); 846 return dst; 847 } 848 849 public void copyValues(GuarantorComponent dst) { 850 super.copyValues(dst); 851 dst.party = party == null ? null : party.copy(); 852 dst.onHold = onHold == null ? null : onHold.copy(); 853 dst.period = period == null ? null : period.copy(); 854 } 855 856 @Override 857 public boolean equalsDeep(Base other_) { 858 if (!super.equalsDeep(other_)) 859 return false; 860 if (!(other_ instanceof GuarantorComponent)) 861 return false; 862 GuarantorComponent o = (GuarantorComponent) other_; 863 return compareDeep(party, o.party, true) && compareDeep(onHold, o.onHold, true) 864 && compareDeep(period, o.period, true); 865 } 866 867 @Override 868 public boolean equalsShallow(Base other_) { 869 if (!super.equalsShallow(other_)) 870 return false; 871 if (!(other_ instanceof GuarantorComponent)) 872 return false; 873 GuarantorComponent o = (GuarantorComponent) other_; 874 return compareValues(onHold, o.onHold, true); 875 } 876 877 public boolean isEmpty() { 878 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(party, onHold, period); 879 } 880 881 public String fhirType() { 882 return "Account.guarantor"; 883 884 } 885 886 } 887 888 /** 889 * Unique identifier used to reference the account. Might or might not be 890 * intended for human use (e.g. credit card number). 891 */ 892 @Child(name = "identifier", type = { 893 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 894 @Description(shortDefinition = "Account number", formalDefinition = "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).") 895 protected List<Identifier> identifier; 896 897 /** 898 * Indicates whether the account is presently used/usable or not. 899 */ 900 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 901 @Description(shortDefinition = "active | inactive | entered-in-error | on-hold | unknown", formalDefinition = "Indicates whether the account is presently used/usable or not.") 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/account-status") 903 protected Enumeration<AccountStatus> status; 904 905 /** 906 * Categorizes the account for reporting and searching purposes. 907 */ 908 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 909 @Description(shortDefinition = "E.g. patient, expense, depreciation", formalDefinition = "Categorizes the account for reporting and searching purposes.") 910 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/account-type") 911 protected CodeableConcept type; 912 913 /** 914 * Name used for the account when displaying it to humans in reports, etc. 915 */ 916 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 917 @Description(shortDefinition = "Human-readable label", formalDefinition = "Name used for the account when displaying it to humans in reports, etc.") 918 protected StringType name; 919 920 /** 921 * Identifies the entity which incurs the expenses. While the immediate 922 * recipients of services or goods might be entities related to the subject, the 923 * expenses were ultimately incurred by the subject of the Account. 924 */ 925 @Child(name = "subject", type = { Patient.class, Device.class, Practitioner.class, PractitionerRole.class, 926 Location.class, HealthcareService.class, 927 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 928 @Description(shortDefinition = "The entity that caused the expenses", formalDefinition = "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.") 929 protected List<Reference> subject; 930 /** 931 * The actual objects that are the target of the reference (Identifies the 932 * entity which incurs the expenses. While the immediate recipients of services 933 * or goods might be entities related to the subject, the expenses were 934 * ultimately incurred by the subject of the Account.) 935 */ 936 protected List<Resource> subjectTarget; 937 938 /** 939 * The date range of services associated with this account. 940 */ 941 @Child(name = "servicePeriod", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 942 @Description(shortDefinition = "Transaction window", formalDefinition = "The date range of services associated with this account.") 943 protected Period servicePeriod; 944 945 /** 946 * The party(s) that are responsible for covering the payment of this account, 947 * and what order should they be applied to the account. 948 */ 949 @Child(name = "coverage", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 950 @Description(shortDefinition = "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account", formalDefinition = "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.") 951 protected List<CoverageComponent> coverage; 952 953 /** 954 * Indicates the service area, hospital, department, etc. with responsibility 955 * for managing the Account. 956 */ 957 @Child(name = "owner", type = { Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 958 @Description(shortDefinition = "Entity managing the Account", formalDefinition = "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.") 959 protected Reference owner; 960 961 /** 962 * The actual object that is the target of the reference (Indicates the service 963 * area, hospital, department, etc. with responsibility for managing the 964 * Account.) 965 */ 966 protected Organization ownerTarget; 967 968 /** 969 * Provides additional information about what the account tracks and how it is 970 * used. 971 */ 972 @Child(name = "description", type = { 973 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 974 @Description(shortDefinition = "Explanation of purpose/use", formalDefinition = "Provides additional information about what the account tracks and how it is used.") 975 protected StringType description; 976 977 /** 978 * The parties responsible for balancing the account if other payment options 979 * fall short. 980 */ 981 @Child(name = "guarantor", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 982 @Description(shortDefinition = "The parties ultimately responsible for balancing the Account", formalDefinition = "The parties responsible for balancing the account if other payment options fall short.") 983 protected List<GuarantorComponent> guarantor; 984 985 /** 986 * Reference to a parent Account. 987 */ 988 @Child(name = "partOf", type = { Account.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 989 @Description(shortDefinition = "Reference to a parent Account", formalDefinition = "Reference to a parent Account.") 990 protected Reference partOf; 991 992 /** 993 * The actual object that is the target of the reference (Reference to a parent 994 * Account.) 995 */ 996 protected Account partOfTarget; 997 998 private static final long serialVersionUID = 1211238069L; 999 1000 /** 1001 * Constructor 1002 */ 1003 public Account() { 1004 super(); 1005 } 1006 1007 /** 1008 * Constructor 1009 */ 1010 public Account(Enumeration<AccountStatus> status) { 1011 super(); 1012 this.status = status; 1013 } 1014 1015 /** 1016 * @return {@link #identifier} (Unique identifier used to reference the account. 1017 * Might or might not be intended for human use (e.g. credit card 1018 * number).) 1019 */ 1020 public List<Identifier> getIdentifier() { 1021 if (this.identifier == null) 1022 this.identifier = new ArrayList<Identifier>(); 1023 return this.identifier; 1024 } 1025 1026 /** 1027 * @return Returns a reference to <code>this</code> for easy method chaining 1028 */ 1029 public Account setIdentifier(List<Identifier> theIdentifier) { 1030 this.identifier = theIdentifier; 1031 return this; 1032 } 1033 1034 public boolean hasIdentifier() { 1035 if (this.identifier == null) 1036 return false; 1037 for (Identifier item : this.identifier) 1038 if (!item.isEmpty()) 1039 return true; 1040 return false; 1041 } 1042 1043 public Identifier addIdentifier() { // 3 1044 Identifier t = new Identifier(); 1045 if (this.identifier == null) 1046 this.identifier = new ArrayList<Identifier>(); 1047 this.identifier.add(t); 1048 return t; 1049 } 1050 1051 public Account addIdentifier(Identifier t) { // 3 1052 if (t == null) 1053 return this; 1054 if (this.identifier == null) 1055 this.identifier = new ArrayList<Identifier>(); 1056 this.identifier.add(t); 1057 return this; 1058 } 1059 1060 /** 1061 * @return The first repetition of repeating field {@link #identifier}, creating 1062 * it if it does not already exist 1063 */ 1064 public Identifier getIdentifierFirstRep() { 1065 if (getIdentifier().isEmpty()) { 1066 addIdentifier(); 1067 } 1068 return getIdentifier().get(0); 1069 } 1070 1071 /** 1072 * @return {@link #status} (Indicates whether the account is presently 1073 * used/usable or not.). This is the underlying object with id, value 1074 * and extensions. The accessor "getStatus" gives direct access to the 1075 * value 1076 */ 1077 public Enumeration<AccountStatus> getStatusElement() { 1078 if (this.status == null) 1079 if (Configuration.errorOnAutoCreate()) 1080 throw new Error("Attempt to auto-create Account.status"); 1081 else if (Configuration.doAutoCreate()) 1082 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); // bb 1083 return this.status; 1084 } 1085 1086 public boolean hasStatusElement() { 1087 return this.status != null && !this.status.isEmpty(); 1088 } 1089 1090 public boolean hasStatus() { 1091 return this.status != null && !this.status.isEmpty(); 1092 } 1093 1094 /** 1095 * @param value {@link #status} (Indicates whether the account is presently 1096 * used/usable or not.). This is the underlying object with id, 1097 * value and extensions. The accessor "getStatus" gives direct 1098 * access to the value 1099 */ 1100 public Account setStatusElement(Enumeration<AccountStatus> value) { 1101 this.status = value; 1102 return this; 1103 } 1104 1105 /** 1106 * @return Indicates whether the account is presently used/usable or not. 1107 */ 1108 public AccountStatus getStatus() { 1109 return this.status == null ? null : this.status.getValue(); 1110 } 1111 1112 /** 1113 * @param value Indicates whether the account is presently used/usable or not. 1114 */ 1115 public Account setStatus(AccountStatus value) { 1116 if (this.status == null) 1117 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); 1118 this.status.setValue(value); 1119 return this; 1120 } 1121 1122 /** 1123 * @return {@link #type} (Categorizes the account for reporting and searching 1124 * purposes.) 1125 */ 1126 public CodeableConcept getType() { 1127 if (this.type == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create Account.type"); 1130 else if (Configuration.doAutoCreate()) 1131 this.type = new CodeableConcept(); // cc 1132 return this.type; 1133 } 1134 1135 public boolean hasType() { 1136 return this.type != null && !this.type.isEmpty(); 1137 } 1138 1139 /** 1140 * @param value {@link #type} (Categorizes the account for reporting and 1141 * searching purposes.) 1142 */ 1143 public Account setType(CodeableConcept value) { 1144 this.type = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #name} (Name used for the account when displaying it to humans 1150 * in reports, etc.). This is the underlying object with id, value and 1151 * extensions. The accessor "getName" gives direct access to the value 1152 */ 1153 public StringType getNameElement() { 1154 if (this.name == null) 1155 if (Configuration.errorOnAutoCreate()) 1156 throw new Error("Attempt to auto-create Account.name"); 1157 else if (Configuration.doAutoCreate()) 1158 this.name = new StringType(); // bb 1159 return this.name; 1160 } 1161 1162 public boolean hasNameElement() { 1163 return this.name != null && !this.name.isEmpty(); 1164 } 1165 1166 public boolean hasName() { 1167 return this.name != null && !this.name.isEmpty(); 1168 } 1169 1170 /** 1171 * @param value {@link #name} (Name used for the account when displaying it to 1172 * humans in reports, etc.). This is the underlying object with id, 1173 * value and extensions. The accessor "getName" gives direct access 1174 * to the value 1175 */ 1176 public Account setNameElement(StringType value) { 1177 this.name = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return Name used for the account when displaying it to humans in reports, 1183 * etc. 1184 */ 1185 public String getName() { 1186 return this.name == null ? null : this.name.getValue(); 1187 } 1188 1189 /** 1190 * @param value Name used for the account when displaying it to humans in 1191 * reports, etc. 1192 */ 1193 public Account setName(String value) { 1194 if (Utilities.noString(value)) 1195 this.name = null; 1196 else { 1197 if (this.name == null) 1198 this.name = new StringType(); 1199 this.name.setValue(value); 1200 } 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #subject} (Identifies the entity which incurs the expenses. 1206 * While the immediate recipients of services or goods might be entities 1207 * related to the subject, the expenses were ultimately incurred by the 1208 * subject of the Account.) 1209 */ 1210 public List<Reference> getSubject() { 1211 if (this.subject == null) 1212 this.subject = new ArrayList<Reference>(); 1213 return this.subject; 1214 } 1215 1216 /** 1217 * @return Returns a reference to <code>this</code> for easy method chaining 1218 */ 1219 public Account setSubject(List<Reference> theSubject) { 1220 this.subject = theSubject; 1221 return this; 1222 } 1223 1224 public boolean hasSubject() { 1225 if (this.subject == null) 1226 return false; 1227 for (Reference item : this.subject) 1228 if (!item.isEmpty()) 1229 return true; 1230 return false; 1231 } 1232 1233 public Reference addSubject() { // 3 1234 Reference t = new Reference(); 1235 if (this.subject == null) 1236 this.subject = new ArrayList<Reference>(); 1237 this.subject.add(t); 1238 return t; 1239 } 1240 1241 public Account addSubject(Reference t) { // 3 1242 if (t == null) 1243 return this; 1244 if (this.subject == null) 1245 this.subject = new ArrayList<Reference>(); 1246 this.subject.add(t); 1247 return this; 1248 } 1249 1250 /** 1251 * @return The first repetition of repeating field {@link #subject}, creating it 1252 * if it does not already exist 1253 */ 1254 public Reference getSubjectFirstRep() { 1255 if (getSubject().isEmpty()) { 1256 addSubject(); 1257 } 1258 return getSubject().get(0); 1259 } 1260 1261 /** 1262 * @deprecated Use Reference#setResource(IBaseResource) instead 1263 */ 1264 @Deprecated 1265 public List<Resource> getSubjectTarget() { 1266 if (this.subjectTarget == null) 1267 this.subjectTarget = new ArrayList<Resource>(); 1268 return this.subjectTarget; 1269 } 1270 1271 /** 1272 * @return {@link #servicePeriod} (The date range of services associated with 1273 * this account.) 1274 */ 1275 public Period getServicePeriod() { 1276 if (this.servicePeriod == null) 1277 if (Configuration.errorOnAutoCreate()) 1278 throw new Error("Attempt to auto-create Account.servicePeriod"); 1279 else if (Configuration.doAutoCreate()) 1280 this.servicePeriod = new Period(); // cc 1281 return this.servicePeriod; 1282 } 1283 1284 public boolean hasServicePeriod() { 1285 return this.servicePeriod != null && !this.servicePeriod.isEmpty(); 1286 } 1287 1288 /** 1289 * @param value {@link #servicePeriod} (The date range of services associated 1290 * with this account.) 1291 */ 1292 public Account setServicePeriod(Period value) { 1293 this.servicePeriod = value; 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #coverage} (The party(s) that are responsible for covering the 1299 * payment of this account, and what order should they be applied to the 1300 * account.) 1301 */ 1302 public List<CoverageComponent> getCoverage() { 1303 if (this.coverage == null) 1304 this.coverage = new ArrayList<CoverageComponent>(); 1305 return this.coverage; 1306 } 1307 1308 /** 1309 * @return Returns a reference to <code>this</code> for easy method chaining 1310 */ 1311 public Account setCoverage(List<CoverageComponent> theCoverage) { 1312 this.coverage = theCoverage; 1313 return this; 1314 } 1315 1316 public boolean hasCoverage() { 1317 if (this.coverage == null) 1318 return false; 1319 for (CoverageComponent item : this.coverage) 1320 if (!item.isEmpty()) 1321 return true; 1322 return false; 1323 } 1324 1325 public CoverageComponent addCoverage() { // 3 1326 CoverageComponent t = new CoverageComponent(); 1327 if (this.coverage == null) 1328 this.coverage = new ArrayList<CoverageComponent>(); 1329 this.coverage.add(t); 1330 return t; 1331 } 1332 1333 public Account addCoverage(CoverageComponent t) { // 3 1334 if (t == null) 1335 return this; 1336 if (this.coverage == null) 1337 this.coverage = new ArrayList<CoverageComponent>(); 1338 this.coverage.add(t); 1339 return this; 1340 } 1341 1342 /** 1343 * @return The first repetition of repeating field {@link #coverage}, creating 1344 * it if it does not already exist 1345 */ 1346 public CoverageComponent getCoverageFirstRep() { 1347 if (getCoverage().isEmpty()) { 1348 addCoverage(); 1349 } 1350 return getCoverage().get(0); 1351 } 1352 1353 /** 1354 * @return {@link #owner} (Indicates the service area, hospital, department, 1355 * etc. with responsibility for managing the Account.) 1356 */ 1357 public Reference getOwner() { 1358 if (this.owner == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create Account.owner"); 1361 else if (Configuration.doAutoCreate()) 1362 this.owner = new Reference(); // cc 1363 return this.owner; 1364 } 1365 1366 public boolean hasOwner() { 1367 return this.owner != null && !this.owner.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #owner} (Indicates the service area, hospital, 1372 * department, etc. with responsibility for managing the Account.) 1373 */ 1374 public Account setOwner(Reference value) { 1375 this.owner = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #owner} The actual object that is the target of the reference. 1381 * The reference library doesn't populate this, but you can use it to 1382 * hold the resource if you resolve it. (Indicates the service area, 1383 * hospital, department, etc. with responsibility for managing the 1384 * Account.) 1385 */ 1386 public Organization getOwnerTarget() { 1387 if (this.ownerTarget == null) 1388 if (Configuration.errorOnAutoCreate()) 1389 throw new Error("Attempt to auto-create Account.owner"); 1390 else if (Configuration.doAutoCreate()) 1391 this.ownerTarget = new Organization(); // aa 1392 return this.ownerTarget; 1393 } 1394 1395 /** 1396 * @param value {@link #owner} The actual object that is the target of the 1397 * reference. The reference library doesn't use these, but you can 1398 * use it to hold the resource if you resolve it. (Indicates the 1399 * service area, hospital, department, etc. with responsibility for 1400 * managing the Account.) 1401 */ 1402 public Account setOwnerTarget(Organization value) { 1403 this.ownerTarget = value; 1404 return this; 1405 } 1406 1407 /** 1408 * @return {@link #description} (Provides additional information about what the 1409 * account tracks and how it is used.). This is the underlying object 1410 * with id, value and extensions. The accessor "getDescription" gives 1411 * direct access to the value 1412 */ 1413 public StringType getDescriptionElement() { 1414 if (this.description == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create Account.description"); 1417 else if (Configuration.doAutoCreate()) 1418 this.description = new StringType(); // bb 1419 return this.description; 1420 } 1421 1422 public boolean hasDescriptionElement() { 1423 return this.description != null && !this.description.isEmpty(); 1424 } 1425 1426 public boolean hasDescription() { 1427 return this.description != null && !this.description.isEmpty(); 1428 } 1429 1430 /** 1431 * @param value {@link #description} (Provides additional information about what 1432 * the account tracks and how it is used.). This is the underlying 1433 * object with id, value and extensions. The accessor 1434 * "getDescription" gives direct access to the value 1435 */ 1436 public Account setDescriptionElement(StringType value) { 1437 this.description = value; 1438 return this; 1439 } 1440 1441 /** 1442 * @return Provides additional information about what the account tracks and how 1443 * it is used. 1444 */ 1445 public String getDescription() { 1446 return this.description == null ? null : this.description.getValue(); 1447 } 1448 1449 /** 1450 * @param value Provides additional information about what the account tracks 1451 * and how it is used. 1452 */ 1453 public Account setDescription(String value) { 1454 if (Utilities.noString(value)) 1455 this.description = null; 1456 else { 1457 if (this.description == null) 1458 this.description = new StringType(); 1459 this.description.setValue(value); 1460 } 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #guarantor} (The parties responsible for balancing the account 1466 * if other payment options fall short.) 1467 */ 1468 public List<GuarantorComponent> getGuarantor() { 1469 if (this.guarantor == null) 1470 this.guarantor = new ArrayList<GuarantorComponent>(); 1471 return this.guarantor; 1472 } 1473 1474 /** 1475 * @return Returns a reference to <code>this</code> for easy method chaining 1476 */ 1477 public Account setGuarantor(List<GuarantorComponent> theGuarantor) { 1478 this.guarantor = theGuarantor; 1479 return this; 1480 } 1481 1482 public boolean hasGuarantor() { 1483 if (this.guarantor == null) 1484 return false; 1485 for (GuarantorComponent item : this.guarantor) 1486 if (!item.isEmpty()) 1487 return true; 1488 return false; 1489 } 1490 1491 public GuarantorComponent addGuarantor() { // 3 1492 GuarantorComponent t = new GuarantorComponent(); 1493 if (this.guarantor == null) 1494 this.guarantor = new ArrayList<GuarantorComponent>(); 1495 this.guarantor.add(t); 1496 return t; 1497 } 1498 1499 public Account addGuarantor(GuarantorComponent t) { // 3 1500 if (t == null) 1501 return this; 1502 if (this.guarantor == null) 1503 this.guarantor = new ArrayList<GuarantorComponent>(); 1504 this.guarantor.add(t); 1505 return this; 1506 } 1507 1508 /** 1509 * @return The first repetition of repeating field {@link #guarantor}, creating 1510 * it if it does not already exist 1511 */ 1512 public GuarantorComponent getGuarantorFirstRep() { 1513 if (getGuarantor().isEmpty()) { 1514 addGuarantor(); 1515 } 1516 return getGuarantor().get(0); 1517 } 1518 1519 /** 1520 * @return {@link #partOf} (Reference to a parent Account.) 1521 */ 1522 public Reference getPartOf() { 1523 if (this.partOf == null) 1524 if (Configuration.errorOnAutoCreate()) 1525 throw new Error("Attempt to auto-create Account.partOf"); 1526 else if (Configuration.doAutoCreate()) 1527 this.partOf = new Reference(); // cc 1528 return this.partOf; 1529 } 1530 1531 public boolean hasPartOf() { 1532 return this.partOf != null && !this.partOf.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #partOf} (Reference to a parent Account.) 1537 */ 1538 public Account setPartOf(Reference value) { 1539 this.partOf = value; 1540 return this; 1541 } 1542 1543 /** 1544 * @return {@link #partOf} The actual object that is the target of the 1545 * reference. The reference library doesn't populate this, but you can 1546 * use it to hold the resource if you resolve it. (Reference to a parent 1547 * Account.) 1548 */ 1549 public Account getPartOfTarget() { 1550 if (this.partOfTarget == null) 1551 if (Configuration.errorOnAutoCreate()) 1552 throw new Error("Attempt to auto-create Account.partOf"); 1553 else if (Configuration.doAutoCreate()) 1554 this.partOfTarget = new Account(); // aa 1555 return this.partOfTarget; 1556 } 1557 1558 /** 1559 * @param value {@link #partOf} The actual object that is the target of the 1560 * reference. The reference library doesn't use these, but you can 1561 * use it to hold the resource if you resolve it. (Reference to a 1562 * parent Account.) 1563 */ 1564 public Account setPartOfTarget(Account value) { 1565 this.partOfTarget = value; 1566 return this; 1567 } 1568 1569 protected void listChildren(List<Property> children) { 1570 super.listChildren(children); 1571 children.add(new Property("identifier", "Identifier", 1572 "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).", 1573 0, java.lang.Integer.MAX_VALUE, identifier)); 1574 children.add( 1575 new Property("status", "code", "Indicates whether the account is presently used/usable or not.", 0, 1, status)); 1576 children.add(new Property("type", "CodeableConcept", 1577 "Categorizes the account for reporting and searching purposes.", 0, 1, type)); 1578 children.add(new Property("name", "string", 1579 "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name)); 1580 children.add(new Property("subject", 1581 "Reference(Patient|Device|Practitioner|PractitionerRole|Location|HealthcareService|Organization)", 1582 "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.", 1583 0, java.lang.Integer.MAX_VALUE, subject)); 1584 children.add(new Property("servicePeriod", "Period", "The date range of services associated with this account.", 0, 1585 1, servicePeriod)); 1586 children.add(new Property("coverage", "", 1587 "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 1588 0, java.lang.Integer.MAX_VALUE, coverage)); 1589 children.add(new Property("owner", "Reference(Organization)", 1590 "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.", 0, 1, 1591 owner)); 1592 children.add(new Property("description", "string", 1593 "Provides additional information about what the account tracks and how it is used.", 0, 1, description)); 1594 children.add(new Property("guarantor", "", 1595 "The parties responsible for balancing the account if other payment options fall short.", 0, 1596 java.lang.Integer.MAX_VALUE, guarantor)); 1597 children.add(new Property("partOf", "Reference(Account)", "Reference to a parent Account.", 0, 1, partOf)); 1598 } 1599 1600 @Override 1601 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1602 switch (_hash) { 1603 case -1618432855: 1604 /* identifier */ return new Property("identifier", "Identifier", 1605 "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).", 1606 0, java.lang.Integer.MAX_VALUE, identifier); 1607 case -892481550: 1608 /* status */ return new Property("status", "code", 1609 "Indicates whether the account is presently used/usable or not.", 0, 1, status); 1610 case 3575610: 1611 /* type */ return new Property("type", "CodeableConcept", 1612 "Categorizes the account for reporting and searching purposes.", 0, 1, type); 1613 case 3373707: 1614 /* name */ return new Property("name", "string", 1615 "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name); 1616 case -1867885268: 1617 /* subject */ return new Property("subject", 1618 "Reference(Patient|Device|Practitioner|PractitionerRole|Location|HealthcareService|Organization)", 1619 "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.", 1620 0, java.lang.Integer.MAX_VALUE, subject); 1621 case 2129104086: 1622 /* servicePeriod */ return new Property("servicePeriod", "Period", 1623 "The date range of services associated with this account.", 0, 1, servicePeriod); 1624 case -351767064: 1625 /* coverage */ return new Property("coverage", "", 1626 "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 1627 0, java.lang.Integer.MAX_VALUE, coverage); 1628 case 106164915: 1629 /* owner */ return new Property("owner", "Reference(Organization)", 1630 "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.", 0, 1, 1631 owner); 1632 case -1724546052: 1633 /* description */ return new Property("description", "string", 1634 "Provides additional information about what the account tracks and how it is used.", 0, 1, description); 1635 case -188629045: 1636 /* guarantor */ return new Property("guarantor", "", 1637 "The parties responsible for balancing the account if other payment options fall short.", 0, 1638 java.lang.Integer.MAX_VALUE, guarantor); 1639 case -995410646: 1640 /* partOf */ return new Property("partOf", "Reference(Account)", "Reference to a parent Account.", 0, 1, partOf); 1641 default: 1642 return super.getNamedProperty(_hash, _name, _checkValid); 1643 } 1644 1645 } 1646 1647 @Override 1648 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1649 switch (hash) { 1650 case -1618432855: 1651 /* identifier */ return this.identifier == null ? new Base[0] 1652 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1653 case -892481550: 1654 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<AccountStatus> 1655 case 3575610: 1656 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1657 case 3373707: 1658 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1659 case -1867885268: 1660 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 1661 case 2129104086: 1662 /* servicePeriod */ return this.servicePeriod == null ? new Base[0] : new Base[] { this.servicePeriod }; // Period 1663 case -351767064: 1664 /* coverage */ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // CoverageComponent 1665 case 106164915: 1666 /* owner */ return this.owner == null ? new Base[0] : new Base[] { this.owner }; // Reference 1667 case -1724546052: 1668 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1669 case -188629045: 1670 /* guarantor */ return this.guarantor == null ? new Base[0] 1671 : this.guarantor.toArray(new Base[this.guarantor.size()]); // GuarantorComponent 1672 case -995410646: 1673 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 1674 default: 1675 return super.getProperty(hash, name, checkValid); 1676 } 1677 1678 } 1679 1680 @Override 1681 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1682 switch (hash) { 1683 case -1618432855: // identifier 1684 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1685 return value; 1686 case -892481550: // status 1687 value = new AccountStatusEnumFactory().fromType(castToCode(value)); 1688 this.status = (Enumeration) value; // Enumeration<AccountStatus> 1689 return value; 1690 case 3575610: // type 1691 this.type = castToCodeableConcept(value); // CodeableConcept 1692 return value; 1693 case 3373707: // name 1694 this.name = castToString(value); // StringType 1695 return value; 1696 case -1867885268: // subject 1697 this.getSubject().add(castToReference(value)); // Reference 1698 return value; 1699 case 2129104086: // servicePeriod 1700 this.servicePeriod = castToPeriod(value); // Period 1701 return value; 1702 case -351767064: // coverage 1703 this.getCoverage().add((CoverageComponent) value); // CoverageComponent 1704 return value; 1705 case 106164915: // owner 1706 this.owner = castToReference(value); // Reference 1707 return value; 1708 case -1724546052: // description 1709 this.description = castToString(value); // StringType 1710 return value; 1711 case -188629045: // guarantor 1712 this.getGuarantor().add((GuarantorComponent) value); // GuarantorComponent 1713 return value; 1714 case -995410646: // partOf 1715 this.partOf = castToReference(value); // Reference 1716 return value; 1717 default: 1718 return super.setProperty(hash, name, value); 1719 } 1720 1721 } 1722 1723 @Override 1724 public Base setProperty(String name, Base value) throws FHIRException { 1725 if (name.equals("identifier")) { 1726 this.getIdentifier().add(castToIdentifier(value)); 1727 } else if (name.equals("status")) { 1728 value = new AccountStatusEnumFactory().fromType(castToCode(value)); 1729 this.status = (Enumeration) value; // Enumeration<AccountStatus> 1730 } else if (name.equals("type")) { 1731 this.type = castToCodeableConcept(value); // CodeableConcept 1732 } else if (name.equals("name")) { 1733 this.name = castToString(value); // StringType 1734 } else if (name.equals("subject")) { 1735 this.getSubject().add(castToReference(value)); 1736 } else if (name.equals("servicePeriod")) { 1737 this.servicePeriod = castToPeriod(value); // Period 1738 } else if (name.equals("coverage")) { 1739 this.getCoverage().add((CoverageComponent) value); 1740 } else if (name.equals("owner")) { 1741 this.owner = castToReference(value); // Reference 1742 } else if (name.equals("description")) { 1743 this.description = castToString(value); // StringType 1744 } else if (name.equals("guarantor")) { 1745 this.getGuarantor().add((GuarantorComponent) value); 1746 } else if (name.equals("partOf")) { 1747 this.partOf = castToReference(value); // Reference 1748 } else 1749 return super.setProperty(name, value); 1750 return value; 1751 } 1752 1753 @Override 1754 public Base makeProperty(int hash, String name) throws FHIRException { 1755 switch (hash) { 1756 case -1618432855: 1757 return addIdentifier(); 1758 case -892481550: 1759 return getStatusElement(); 1760 case 3575610: 1761 return getType(); 1762 case 3373707: 1763 return getNameElement(); 1764 case -1867885268: 1765 return addSubject(); 1766 case 2129104086: 1767 return getServicePeriod(); 1768 case -351767064: 1769 return addCoverage(); 1770 case 106164915: 1771 return getOwner(); 1772 case -1724546052: 1773 return getDescriptionElement(); 1774 case -188629045: 1775 return addGuarantor(); 1776 case -995410646: 1777 return getPartOf(); 1778 default: 1779 return super.makeProperty(hash, name); 1780 } 1781 1782 } 1783 1784 @Override 1785 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1786 switch (hash) { 1787 case -1618432855: 1788 /* identifier */ return new String[] { "Identifier" }; 1789 case -892481550: 1790 /* status */ return new String[] { "code" }; 1791 case 3575610: 1792 /* type */ return new String[] { "CodeableConcept" }; 1793 case 3373707: 1794 /* name */ return new String[] { "string" }; 1795 case -1867885268: 1796 /* subject */ return new String[] { "Reference" }; 1797 case 2129104086: 1798 /* servicePeriod */ return new String[] { "Period" }; 1799 case -351767064: 1800 /* coverage */ return new String[] {}; 1801 case 106164915: 1802 /* owner */ return new String[] { "Reference" }; 1803 case -1724546052: 1804 /* description */ return new String[] { "string" }; 1805 case -188629045: 1806 /* guarantor */ return new String[] {}; 1807 case -995410646: 1808 /* partOf */ return new String[] { "Reference" }; 1809 default: 1810 return super.getTypesForProperty(hash, name); 1811 } 1812 1813 } 1814 1815 @Override 1816 public Base addChild(String name) throws FHIRException { 1817 if (name.equals("identifier")) { 1818 return addIdentifier(); 1819 } else if (name.equals("status")) { 1820 throw new FHIRException("Cannot call addChild on a singleton property Account.status"); 1821 } else if (name.equals("type")) { 1822 this.type = new CodeableConcept(); 1823 return this.type; 1824 } else if (name.equals("name")) { 1825 throw new FHIRException("Cannot call addChild on a singleton property Account.name"); 1826 } else if (name.equals("subject")) { 1827 return addSubject(); 1828 } else if (name.equals("servicePeriod")) { 1829 this.servicePeriod = new Period(); 1830 return this.servicePeriod; 1831 } else if (name.equals("coverage")) { 1832 return addCoverage(); 1833 } else if (name.equals("owner")) { 1834 this.owner = new Reference(); 1835 return this.owner; 1836 } else if (name.equals("description")) { 1837 throw new FHIRException("Cannot call addChild on a singleton property Account.description"); 1838 } else if (name.equals("guarantor")) { 1839 return addGuarantor(); 1840 } else if (name.equals("partOf")) { 1841 this.partOf = new Reference(); 1842 return this.partOf; 1843 } else 1844 return super.addChild(name); 1845 } 1846 1847 public String fhirType() { 1848 return "Account"; 1849 1850 } 1851 1852 public Account copy() { 1853 Account dst = new Account(); 1854 copyValues(dst); 1855 return dst; 1856 } 1857 1858 public void copyValues(Account dst) { 1859 super.copyValues(dst); 1860 if (identifier != null) { 1861 dst.identifier = new ArrayList<Identifier>(); 1862 for (Identifier i : identifier) 1863 dst.identifier.add(i.copy()); 1864 } 1865 ; 1866 dst.status = status == null ? null : status.copy(); 1867 dst.type = type == null ? null : type.copy(); 1868 dst.name = name == null ? null : name.copy(); 1869 if (subject != null) { 1870 dst.subject = new ArrayList<Reference>(); 1871 for (Reference i : subject) 1872 dst.subject.add(i.copy()); 1873 } 1874 ; 1875 dst.servicePeriod = servicePeriod == null ? null : servicePeriod.copy(); 1876 if (coverage != null) { 1877 dst.coverage = new ArrayList<CoverageComponent>(); 1878 for (CoverageComponent i : coverage) 1879 dst.coverage.add(i.copy()); 1880 } 1881 ; 1882 dst.owner = owner == null ? null : owner.copy(); 1883 dst.description = description == null ? null : description.copy(); 1884 if (guarantor != null) { 1885 dst.guarantor = new ArrayList<GuarantorComponent>(); 1886 for (GuarantorComponent i : guarantor) 1887 dst.guarantor.add(i.copy()); 1888 } 1889 ; 1890 dst.partOf = partOf == null ? null : partOf.copy(); 1891 } 1892 1893 protected Account typedCopy() { 1894 return copy(); 1895 } 1896 1897 @Override 1898 public boolean equalsDeep(Base other_) { 1899 if (!super.equalsDeep(other_)) 1900 return false; 1901 if (!(other_ instanceof Account)) 1902 return false; 1903 Account o = (Account) other_; 1904 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1905 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) 1906 && compareDeep(servicePeriod, o.servicePeriod, true) && compareDeep(coverage, o.coverage, true) 1907 && compareDeep(owner, o.owner, true) && compareDeep(description, o.description, true) 1908 && compareDeep(guarantor, o.guarantor, true) && compareDeep(partOf, o.partOf, true); 1909 } 1910 1911 @Override 1912 public boolean equalsShallow(Base other_) { 1913 if (!super.equalsShallow(other_)) 1914 return false; 1915 if (!(other_ instanceof Account)) 1916 return false; 1917 Account o = (Account) other_; 1918 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 1919 && compareValues(description, o.description, true); 1920 } 1921 1922 public boolean isEmpty() { 1923 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, name, subject, 1924 servicePeriod, coverage, owner, description, guarantor, partOf); 1925 } 1926 1927 @Override 1928 public ResourceType getResourceType() { 1929 return ResourceType.Account; 1930 } 1931 1932 /** 1933 * Search parameter: <b>owner</b> 1934 * <p> 1935 * Description: <b>Entity managing the Account</b><br> 1936 * Type: <b>reference</b><br> 1937 * Path: <b>Account.owner</b><br> 1938 * </p> 1939 */ 1940 @SearchParamDefinition(name = "owner", path = "Account.owner", description = "Entity managing the Account", type = "reference", target = { 1941 Organization.class }) 1942 public static final String SP_OWNER = "owner"; 1943 /** 1944 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 1945 * <p> 1946 * Description: <b>Entity managing the Account</b><br> 1947 * Type: <b>reference</b><br> 1948 * Path: <b>Account.owner</b><br> 1949 * </p> 1950 */ 1951 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1952 SP_OWNER); 1953 1954 /** 1955 * Constant for fluent queries to be used to add include statements. Specifies 1956 * the path value of "<b>Account:owner</b>". 1957 */ 1958 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Account:owner") 1959 .toLocked(); 1960 1961 /** 1962 * Search parameter: <b>identifier</b> 1963 * <p> 1964 * Description: <b>Account number</b><br> 1965 * Type: <b>token</b><br> 1966 * Path: <b>Account.identifier</b><br> 1967 * </p> 1968 */ 1969 @SearchParamDefinition(name = "identifier", path = "Account.identifier", description = "Account number", type = "token") 1970 public static final String SP_IDENTIFIER = "identifier"; 1971 /** 1972 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1973 * <p> 1974 * Description: <b>Account number</b><br> 1975 * Type: <b>token</b><br> 1976 * Path: <b>Account.identifier</b><br> 1977 * </p> 1978 */ 1979 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1980 SP_IDENTIFIER); 1981 1982 /** 1983 * Search parameter: <b>period</b> 1984 * <p> 1985 * Description: <b>Transaction window</b><br> 1986 * Type: <b>date</b><br> 1987 * Path: <b>Account.servicePeriod</b><br> 1988 * </p> 1989 */ 1990 @SearchParamDefinition(name = "period", path = "Account.servicePeriod", description = "Transaction window", type = "date") 1991 public static final String SP_PERIOD = "period"; 1992 /** 1993 * <b>Fluent Client</b> search parameter constant for <b>period</b> 1994 * <p> 1995 * Description: <b>Transaction window</b><br> 1996 * Type: <b>date</b><br> 1997 * Path: <b>Account.servicePeriod</b><br> 1998 * </p> 1999 */ 2000 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 2001 SP_PERIOD); 2002 2003 /** 2004 * Search parameter: <b>subject</b> 2005 * <p> 2006 * Description: <b>The entity that caused the expenses</b><br> 2007 * Type: <b>reference</b><br> 2008 * Path: <b>Account.subject</b><br> 2009 * </p> 2010 */ 2011 @SearchParamDefinition(name = "subject", path = "Account.subject", description = "The entity that caused the expenses", type = "reference", providesMembershipIn = { 2012 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2013 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2014 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2015 HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, 2016 PractitionerRole.class }) 2017 public static final String SP_SUBJECT = "subject"; 2018 /** 2019 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2020 * <p> 2021 * Description: <b>The entity that caused the expenses</b><br> 2022 * Type: <b>reference</b><br> 2023 * Path: <b>Account.subject</b><br> 2024 * </p> 2025 */ 2026 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2027 SP_SUBJECT); 2028 2029 /** 2030 * Constant for fluent queries to be used to add include statements. Specifies 2031 * the path value of "<b>Account:subject</b>". 2032 */ 2033 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2034 "Account:subject").toLocked(); 2035 2036 /** 2037 * Search parameter: <b>patient</b> 2038 * <p> 2039 * Description: <b>The entity that caused the expenses</b><br> 2040 * Type: <b>reference</b><br> 2041 * Path: <b>Account.subject</b><br> 2042 * </p> 2043 */ 2044 @SearchParamDefinition(name = "patient", path = "Account.subject.where(resolve() is Patient)", description = "The entity that caused the expenses", type = "reference", target = { 2045 Patient.class }) 2046 public static final String SP_PATIENT = "patient"; 2047 /** 2048 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2049 * <p> 2050 * Description: <b>The entity that caused the expenses</b><br> 2051 * Type: <b>reference</b><br> 2052 * Path: <b>Account.subject</b><br> 2053 * </p> 2054 */ 2055 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2056 SP_PATIENT); 2057 2058 /** 2059 * Constant for fluent queries to be used to add include statements. Specifies 2060 * the path value of "<b>Account:patient</b>". 2061 */ 2062 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2063 "Account:patient").toLocked(); 2064 2065 /** 2066 * Search parameter: <b>name</b> 2067 * <p> 2068 * Description: <b>Human-readable label</b><br> 2069 * Type: <b>string</b><br> 2070 * Path: <b>Account.name</b><br> 2071 * </p> 2072 */ 2073 @SearchParamDefinition(name = "name", path = "Account.name", description = "Human-readable label", type = "string") 2074 public static final String SP_NAME = "name"; 2075 /** 2076 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2077 * <p> 2078 * Description: <b>Human-readable label</b><br> 2079 * Type: <b>string</b><br> 2080 * Path: <b>Account.name</b><br> 2081 * </p> 2082 */ 2083 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 2084 SP_NAME); 2085 2086 /** 2087 * Search parameter: <b>type</b> 2088 * <p> 2089 * Description: <b>E.g. patient, expense, depreciation</b><br> 2090 * Type: <b>token</b><br> 2091 * Path: <b>Account.type</b><br> 2092 * </p> 2093 */ 2094 @SearchParamDefinition(name = "type", path = "Account.type", description = "E.g. patient, expense, depreciation", type = "token") 2095 public static final String SP_TYPE = "type"; 2096 /** 2097 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2098 * <p> 2099 * Description: <b>E.g. patient, expense, depreciation</b><br> 2100 * Type: <b>token</b><br> 2101 * Path: <b>Account.type</b><br> 2102 * </p> 2103 */ 2104 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2105 SP_TYPE); 2106 2107 /** 2108 * Search parameter: <b>status</b> 2109 * <p> 2110 * Description: <b>active | inactive | entered-in-error | on-hold | 2111 * unknown</b><br> 2112 * Type: <b>token</b><br> 2113 * Path: <b>Account.status</b><br> 2114 * </p> 2115 */ 2116 @SearchParamDefinition(name = "status", path = "Account.status", description = "active | inactive | entered-in-error | on-hold | unknown", type = "token") 2117 public static final String SP_STATUS = "status"; 2118 /** 2119 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2120 * <p> 2121 * Description: <b>active | inactive | entered-in-error | on-hold | 2122 * unknown</b><br> 2123 * Type: <b>token</b><br> 2124 * Path: <b>Account.status</b><br> 2125 * </p> 2126 */ 2127 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2128 SP_STATUS); 2129 2130}