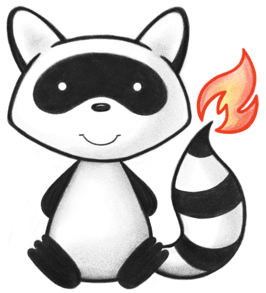
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A financial tool for tracking value accrued for a particular purpose. In the 048 * healthcare field, used to track charges for a patient, cost centers, etc. 049 */ 050@ResourceDef(name = "Account", profile = "http://hl7.org/fhir/StructureDefinition/Account") 051public class Account extends DomainResource { 052 053 public enum AccountStatus { 054 /** 055 * This account is active and may be used. 056 */ 057 ACTIVE, 058 /** 059 * This account is inactive and should not be used to track financial 060 * information. 061 */ 062 INACTIVE, 063 /** 064 * This instance should not have been part of this patient's medical record. 065 */ 066 ENTEREDINERROR, 067 /** 068 * This account is on hold. 069 */ 070 ONHOLD, 071 /** 072 * The account status is unknown. 073 */ 074 UNKNOWN, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static AccountStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("inactive".equals(codeString)) 086 return INACTIVE; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if ("on-hold".equals(codeString)) 090 return ONHOLD; 091 if ("unknown".equals(codeString)) 092 return UNKNOWN; 093 if (Configuration.isAcceptInvalidEnums()) 094 return null; 095 else 096 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case ACTIVE: 102 return "active"; 103 case INACTIVE: 104 return "inactive"; 105 case ENTEREDINERROR: 106 return "entered-in-error"; 107 case ONHOLD: 108 return "on-hold"; 109 case UNKNOWN: 110 return "unknown"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case ACTIVE: 121 return "http://hl7.org/fhir/account-status"; 122 case INACTIVE: 123 return "http://hl7.org/fhir/account-status"; 124 case ENTEREDINERROR: 125 return "http://hl7.org/fhir/account-status"; 126 case ONHOLD: 127 return "http://hl7.org/fhir/account-status"; 128 case UNKNOWN: 129 return "http://hl7.org/fhir/account-status"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case ACTIVE: 140 return "This account is active and may be used."; 141 case INACTIVE: 142 return "This account is inactive and should not be used to track financial information."; 143 case ENTEREDINERROR: 144 return "This instance should not have been part of this patient's medical record."; 145 case ONHOLD: 146 return "This account is on hold."; 147 case UNKNOWN: 148 return "The account status is unknown."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case ACTIVE: 159 return "Active"; 160 case INACTIVE: 161 return "Inactive"; 162 case ENTEREDINERROR: 163 return "Entered in error"; 164 case ONHOLD: 165 return "On Hold"; 166 case UNKNOWN: 167 return "Unknown"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class AccountStatusEnumFactory implements EnumFactory<AccountStatus> { 177 public AccountStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("active".equals(codeString)) 182 return AccountStatus.ACTIVE; 183 if ("inactive".equals(codeString)) 184 return AccountStatus.INACTIVE; 185 if ("entered-in-error".equals(codeString)) 186 return AccountStatus.ENTEREDINERROR; 187 if ("on-hold".equals(codeString)) 188 return AccountStatus.ONHOLD; 189 if ("unknown".equals(codeString)) 190 return AccountStatus.UNKNOWN; 191 throw new IllegalArgumentException("Unknown AccountStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<AccountStatus> fromType(PrimitiveType<?> code) throws FHIRException { 195 if (code == null) 196 return null; 197 if (code.isEmpty()) 198 return new Enumeration<AccountStatus>(this, AccountStatus.NULL, code); 199 String codeString = code.asStringValue(); 200 if (codeString == null || "".equals(codeString)) 201 return new Enumeration<AccountStatus>(this, AccountStatus.NULL, code); 202 if ("active".equals(codeString)) 203 return new Enumeration<AccountStatus>(this, AccountStatus.ACTIVE, code); 204 if ("inactive".equals(codeString)) 205 return new Enumeration<AccountStatus>(this, AccountStatus.INACTIVE, code); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<AccountStatus>(this, AccountStatus.ENTEREDINERROR, code); 208 if ("on-hold".equals(codeString)) 209 return new Enumeration<AccountStatus>(this, AccountStatus.ONHOLD, code); 210 if ("unknown".equals(codeString)) 211 return new Enumeration<AccountStatus>(this, AccountStatus.UNKNOWN, code); 212 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 213 } 214 215 public String toCode(AccountStatus code) { 216 if (code == AccountStatus.NULL) 217 return null; 218 if (code == AccountStatus.ACTIVE) 219 return "active"; 220 if (code == AccountStatus.INACTIVE) 221 return "inactive"; 222 if (code == AccountStatus.ENTEREDINERROR) 223 return "entered-in-error"; 224 if (code == AccountStatus.ONHOLD) 225 return "on-hold"; 226 if (code == AccountStatus.UNKNOWN) 227 return "unknown"; 228 return "?"; 229 } 230 231 public String toSystem(AccountStatus code) { 232 return code.getSystem(); 233 } 234 } 235 236 @Block() 237 public static class CoverageComponent extends BackboneElement implements IBaseBackboneElement { 238 /** 239 * The party(s) that contribute to payment (or part of) of the charges applied 240 * to this account (including self-pay). 241 * 242 * A coverage may only be responsible for specific types of charges, and the 243 * sequence of the coverages in the account could be important when processing 244 * billing. 245 */ 246 @Child(name = "coverage", type = { Coverage.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 247 @Description(shortDefinition = "The party(s), such as insurances, that may contribute to the payment of this account", formalDefinition = "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.") 248 protected Reference coverage; 249 250 /** 251 * The actual object that is the target of the reference (The party(s) that 252 * contribute to payment (or part of) of the charges applied to this account 253 * (including self-pay). 254 * 255 * A coverage may only be responsible for specific types of charges, and the 256 * sequence of the coverages in the account could be important when processing 257 * billing.) 258 */ 259 protected Coverage coverageTarget; 260 261 /** 262 * The priority of the coverage in the context of this account. 263 */ 264 @Child(name = "priority", type = { 265 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 266 @Description(shortDefinition = "The priority of the coverage in the context of this account", formalDefinition = "The priority of the coverage in the context of this account.") 267 protected PositiveIntType priority; 268 269 private static final long serialVersionUID = -1046265008L; 270 271 /** 272 * Constructor 273 */ 274 public CoverageComponent() { 275 super(); 276 } 277 278 /** 279 * Constructor 280 */ 281 public CoverageComponent(Reference coverage) { 282 super(); 283 this.coverage = coverage; 284 } 285 286 /** 287 * @return {@link #coverage} (The party(s) that contribute to payment (or part 288 * of) of the charges applied to this account (including self-pay). 289 * 290 * A coverage may only be responsible for specific types of charges, and 291 * the sequence of the coverages in the account could be important when 292 * processing billing.) 293 */ 294 public Reference getCoverage() { 295 if (this.coverage == null) 296 if (Configuration.errorOnAutoCreate()) 297 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 298 else if (Configuration.doAutoCreate()) 299 this.coverage = new Reference(); // cc 300 return this.coverage; 301 } 302 303 public boolean hasCoverage() { 304 return this.coverage != null && !this.coverage.isEmpty(); 305 } 306 307 /** 308 * @param value {@link #coverage} (The party(s) that contribute to payment (or 309 * part of) of the charges applied to this account (including 310 * self-pay). 311 * 312 * A coverage may only be responsible for specific types of 313 * charges, and the sequence of the coverages in the account could 314 * be important when processing billing.) 315 */ 316 public CoverageComponent setCoverage(Reference value) { 317 this.coverage = value; 318 return this; 319 } 320 321 /** 322 * @return {@link #coverage} The actual object that is the target of the 323 * reference. The reference library doesn't populate this, but you can 324 * use it to hold the resource if you resolve it. (The party(s) that 325 * contribute to payment (or part of) of the charges applied to this 326 * account (including self-pay). 327 * 328 * A coverage may only be responsible for specific types of charges, and 329 * the sequence of the coverages in the account could be important when 330 * processing billing.) 331 */ 332 public Coverage getCoverageTarget() { 333 if (this.coverageTarget == null) 334 if (Configuration.errorOnAutoCreate()) 335 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 336 else if (Configuration.doAutoCreate()) 337 this.coverageTarget = new Coverage(); // aa 338 return this.coverageTarget; 339 } 340 341 /** 342 * @param value {@link #coverage} The actual object that is the target of the 343 * reference. The reference library doesn't use these, but you can 344 * use it to hold the resource if you resolve it. (The party(s) 345 * that contribute to payment (or part of) of the charges applied 346 * to this account (including self-pay). 347 * 348 * A coverage may only be responsible for specific types of 349 * charges, and the sequence of the coverages in the account could 350 * be important when processing billing.) 351 */ 352 public CoverageComponent setCoverageTarget(Coverage value) { 353 this.coverageTarget = value; 354 return this; 355 } 356 357 /** 358 * @return {@link #priority} (The priority of the coverage in the context of 359 * this account.). This is the underlying object with id, value and 360 * extensions. The accessor "getPriority" gives direct access to the 361 * value 362 */ 363 public PositiveIntType getPriorityElement() { 364 if (this.priority == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create CoverageComponent.priority"); 367 else if (Configuration.doAutoCreate()) 368 this.priority = new PositiveIntType(); // bb 369 return this.priority; 370 } 371 372 public boolean hasPriorityElement() { 373 return this.priority != null && !this.priority.isEmpty(); 374 } 375 376 public boolean hasPriority() { 377 return this.priority != null && !this.priority.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #priority} (The priority of the coverage in the context 382 * of this account.). This is the underlying object with id, value 383 * and extensions. The accessor "getPriority" gives direct access 384 * to the value 385 */ 386 public CoverageComponent setPriorityElement(PositiveIntType value) { 387 this.priority = value; 388 return this; 389 } 390 391 /** 392 * @return The priority of the coverage in the context of this account. 393 */ 394 public int getPriority() { 395 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 396 } 397 398 /** 399 * @param value The priority of the coverage in the context of this account. 400 */ 401 public CoverageComponent setPriority(int value) { 402 if (this.priority == null) 403 this.priority = new PositiveIntType(); 404 this.priority.setValue(value); 405 return this; 406 } 407 408 protected void listChildren(List<Property> children) { 409 super.listChildren(children); 410 children.add(new Property("coverage", "Reference(Coverage)", 411 "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 412 0, 1, coverage)); 413 children.add(new Property("priority", "positiveInt", 414 "The priority of the coverage in the context of this account.", 0, 1, priority)); 415 } 416 417 @Override 418 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 419 switch (_hash) { 420 case -351767064: 421 /* coverage */ return new Property("coverage", "Reference(Coverage)", 422 "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 423 0, 1, coverage); 424 case -1165461084: 425 /* priority */ return new Property("priority", "positiveInt", 426 "The priority of the coverage in the context of this account.", 0, 1, priority); 427 default: 428 return super.getNamedProperty(_hash, _name, _checkValid); 429 } 430 431 } 432 433 @Override 434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 435 switch (hash) { 436 case -351767064: 437 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 438 case -1165461084: 439 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // PositiveIntType 440 default: 441 return super.getProperty(hash, name, checkValid); 442 } 443 444 } 445 446 @Override 447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 448 switch (hash) { 449 case -351767064: // coverage 450 this.coverage = castToReference(value); // Reference 451 return value; 452 case -1165461084: // priority 453 this.priority = castToPositiveInt(value); // PositiveIntType 454 return value; 455 default: 456 return super.setProperty(hash, name, value); 457 } 458 459 } 460 461 @Override 462 public Base setProperty(String name, Base value) throws FHIRException { 463 if (name.equals("coverage")) { 464 this.coverage = castToReference(value); // Reference 465 } else if (name.equals("priority")) { 466 this.priority = castToPositiveInt(value); // PositiveIntType 467 } else 468 return super.setProperty(name, value); 469 return value; 470 } 471 472 @Override 473 public void removeChild(String name, Base value) throws FHIRException { 474 if (name.equals("coverage")) { 475 this.coverage = null; 476 } else if (name.equals("priority")) { 477 this.priority = null; 478 } else 479 super.removeChild(name, value); 480 481 } 482 483 @Override 484 public Base makeProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case -351767064: 487 return getCoverage(); 488 case -1165461084: 489 return getPriorityElement(); 490 default: 491 return super.makeProperty(hash, name); 492 } 493 494 } 495 496 @Override 497 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 498 switch (hash) { 499 case -351767064: 500 /* coverage */ return new String[] { "Reference" }; 501 case -1165461084: 502 /* priority */ return new String[] { "positiveInt" }; 503 default: 504 return super.getTypesForProperty(hash, name); 505 } 506 507 } 508 509 @Override 510 public Base addChild(String name) throws FHIRException { 511 if (name.equals("coverage")) { 512 this.coverage = new Reference(); 513 return this.coverage; 514 } else if (name.equals("priority")) { 515 throw new FHIRException("Cannot call addChild on a singleton property Account.priority"); 516 } else 517 return super.addChild(name); 518 } 519 520 public CoverageComponent copy() { 521 CoverageComponent dst = new CoverageComponent(); 522 copyValues(dst); 523 return dst; 524 } 525 526 public void copyValues(CoverageComponent dst) { 527 super.copyValues(dst); 528 dst.coverage = coverage == null ? null : coverage.copy(); 529 dst.priority = priority == null ? null : priority.copy(); 530 } 531 532 @Override 533 public boolean equalsDeep(Base other_) { 534 if (!super.equalsDeep(other_)) 535 return false; 536 if (!(other_ instanceof CoverageComponent)) 537 return false; 538 CoverageComponent o = (CoverageComponent) other_; 539 return compareDeep(coverage, o.coverage, true) && compareDeep(priority, o.priority, true); 540 } 541 542 @Override 543 public boolean equalsShallow(Base other_) { 544 if (!super.equalsShallow(other_)) 545 return false; 546 if (!(other_ instanceof CoverageComponent)) 547 return false; 548 CoverageComponent o = (CoverageComponent) other_; 549 return compareValues(priority, o.priority, true); 550 } 551 552 public boolean isEmpty() { 553 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, priority); 554 } 555 556 public String fhirType() { 557 return "Account.coverage"; 558 559 } 560 561 } 562 563 @Block() 564 public static class GuarantorComponent extends BackboneElement implements IBaseBackboneElement { 565 /** 566 * The entity who is responsible. 567 */ 568 @Child(name = "party", type = { Patient.class, RelatedPerson.class, 569 Organization.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 570 @Description(shortDefinition = "Responsible entity", formalDefinition = "The entity who is responsible.") 571 protected Reference party; 572 573 /** 574 * The actual object that is the target of the reference (The entity who is 575 * responsible.) 576 */ 577 protected Resource partyTarget; 578 579 /** 580 * A guarantor may be placed on credit hold or otherwise have their role 581 * temporarily suspended. 582 */ 583 @Child(name = "onHold", type = { 584 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 585 @Description(shortDefinition = "Credit or other hold applied", formalDefinition = "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.") 586 protected BooleanType onHold; 587 588 /** 589 * The timeframe during which the guarantor accepts responsibility for the 590 * account. 591 */ 592 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 593 @Description(shortDefinition = "Guarantee account during", formalDefinition = "The timeframe during which the guarantor accepts responsibility for the account.") 594 protected Period period; 595 596 private static final long serialVersionUID = -1012345396L; 597 598 /** 599 * Constructor 600 */ 601 public GuarantorComponent() { 602 super(); 603 } 604 605 /** 606 * Constructor 607 */ 608 public GuarantorComponent(Reference party) { 609 super(); 610 this.party = party; 611 } 612 613 /** 614 * @return {@link #party} (The entity who is responsible.) 615 */ 616 public Reference getParty() { 617 if (this.party == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create GuarantorComponent.party"); 620 else if (Configuration.doAutoCreate()) 621 this.party = new Reference(); // cc 622 return this.party; 623 } 624 625 public boolean hasParty() { 626 return this.party != null && !this.party.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #party} (The entity who is responsible.) 631 */ 632 public GuarantorComponent setParty(Reference value) { 633 this.party = value; 634 return this; 635 } 636 637 /** 638 * @return {@link #party} The actual object that is the target of the reference. 639 * The reference library doesn't populate this, but you can use it to 640 * hold the resource if you resolve it. (The entity who is responsible.) 641 */ 642 public Resource getPartyTarget() { 643 return this.partyTarget; 644 } 645 646 /** 647 * @param value {@link #party} The actual object that is the target of the 648 * reference. The reference library doesn't use these, but you can 649 * use it to hold the resource if you resolve it. (The entity who 650 * is responsible.) 651 */ 652 public GuarantorComponent setPartyTarget(Resource value) { 653 this.partyTarget = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #onHold} (A guarantor may be placed on credit hold or 659 * otherwise have their role temporarily suspended.). This is the 660 * underlying object with id, value and extensions. The accessor 661 * "getOnHold" gives direct access to the value 662 */ 663 public BooleanType getOnHoldElement() { 664 if (this.onHold == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create GuarantorComponent.onHold"); 667 else if (Configuration.doAutoCreate()) 668 this.onHold = new BooleanType(); // bb 669 return this.onHold; 670 } 671 672 public boolean hasOnHoldElement() { 673 return this.onHold != null && !this.onHold.isEmpty(); 674 } 675 676 public boolean hasOnHold() { 677 return this.onHold != null && !this.onHold.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #onHold} (A guarantor may be placed on credit hold or 682 * otherwise have their role temporarily suspended.). This is the 683 * underlying object with id, value and extensions. The accessor 684 * "getOnHold" gives direct access to the value 685 */ 686 public GuarantorComponent setOnHoldElement(BooleanType value) { 687 this.onHold = value; 688 return this; 689 } 690 691 /** 692 * @return A guarantor may be placed on credit hold or otherwise have their role 693 * temporarily suspended. 694 */ 695 public boolean getOnHold() { 696 return this.onHold == null || this.onHold.isEmpty() ? false : this.onHold.getValue(); 697 } 698 699 /** 700 * @param value A guarantor may be placed on credit hold or otherwise have their 701 * role temporarily suspended. 702 */ 703 public GuarantorComponent setOnHold(boolean value) { 704 if (this.onHold == null) 705 this.onHold = new BooleanType(); 706 this.onHold.setValue(value); 707 return this; 708 } 709 710 /** 711 * @return {@link #period} (The timeframe during which the guarantor accepts 712 * responsibility for the account.) 713 */ 714 public Period getPeriod() { 715 if (this.period == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create GuarantorComponent.period"); 718 else if (Configuration.doAutoCreate()) 719 this.period = new Period(); // cc 720 return this.period; 721 } 722 723 public boolean hasPeriod() { 724 return this.period != null && !this.period.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #period} (The timeframe during which the guarantor 729 * accepts responsibility for the account.) 730 */ 731 public GuarantorComponent setPeriod(Period value) { 732 this.period = value; 733 return this; 734 } 735 736 protected void listChildren(List<Property> children) { 737 super.listChildren(children); 738 children.add(new Property("party", "Reference(Patient|RelatedPerson|Organization)", 739 "The entity who is responsible.", 0, 1, party)); 740 children.add(new Property("onHold", "boolean", 741 "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, 742 onHold)); 743 children.add(new Property("period", "Period", 744 "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period)); 745 } 746 747 @Override 748 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 749 switch (_hash) { 750 case 106437350: 751 /* party */ return new Property("party", "Reference(Patient|RelatedPerson|Organization)", 752 "The entity who is responsible.", 0, 1, party); 753 case -1013289154: 754 /* onHold */ return new Property("onHold", "boolean", 755 "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, 756 onHold); 757 case -991726143: 758 /* period */ return new Property("period", "Period", 759 "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period); 760 default: 761 return super.getNamedProperty(_hash, _name, _checkValid); 762 } 763 764 } 765 766 @Override 767 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 768 switch (hash) { 769 case 106437350: 770 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 771 case -1013289154: 772 /* onHold */ return this.onHold == null ? new Base[0] : new Base[] { this.onHold }; // BooleanType 773 case -991726143: 774 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 775 default: 776 return super.getProperty(hash, name, checkValid); 777 } 778 779 } 780 781 @Override 782 public Base setProperty(int hash, String name, Base value) throws FHIRException { 783 switch (hash) { 784 case 106437350: // party 785 this.party = castToReference(value); // Reference 786 return value; 787 case -1013289154: // onHold 788 this.onHold = castToBoolean(value); // BooleanType 789 return value; 790 case -991726143: // period 791 this.period = castToPeriod(value); // Period 792 return value; 793 default: 794 return super.setProperty(hash, name, value); 795 } 796 797 } 798 799 @Override 800 public Base setProperty(String name, Base value) throws FHIRException { 801 if (name.equals("party")) { 802 this.party = castToReference(value); // Reference 803 } else if (name.equals("onHold")) { 804 this.onHold = castToBoolean(value); // BooleanType 805 } else if (name.equals("period")) { 806 this.period = castToPeriod(value); // Period 807 } else 808 return super.setProperty(name, value); 809 return value; 810 } 811 812 @Override 813 public void removeChild(String name, Base value) throws FHIRException { 814 if (name.equals("party")) { 815 this.party = null; 816 } else if (name.equals("onHold")) { 817 this.onHold = null; 818 } else if (name.equals("period")) { 819 this.period = null; 820 } else 821 super.removeChild(name, value); 822 823 } 824 825 @Override 826 public Base makeProperty(int hash, String name) throws FHIRException { 827 switch (hash) { 828 case 106437350: 829 return getParty(); 830 case -1013289154: 831 return getOnHoldElement(); 832 case -991726143: 833 return getPeriod(); 834 default: 835 return super.makeProperty(hash, name); 836 } 837 838 } 839 840 @Override 841 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 842 switch (hash) { 843 case 106437350: 844 /* party */ return new String[] { "Reference" }; 845 case -1013289154: 846 /* onHold */ return new String[] { "boolean" }; 847 case -991726143: 848 /* period */ return new String[] { "Period" }; 849 default: 850 return super.getTypesForProperty(hash, name); 851 } 852 853 } 854 855 @Override 856 public Base addChild(String name) throws FHIRException { 857 if (name.equals("party")) { 858 this.party = new Reference(); 859 return this.party; 860 } else if (name.equals("onHold")) { 861 throw new FHIRException("Cannot call addChild on a singleton property Account.onHold"); 862 } else if (name.equals("period")) { 863 this.period = new Period(); 864 return this.period; 865 } else 866 return super.addChild(name); 867 } 868 869 public GuarantorComponent copy() { 870 GuarantorComponent dst = new GuarantorComponent(); 871 copyValues(dst); 872 return dst; 873 } 874 875 public void copyValues(GuarantorComponent dst) { 876 super.copyValues(dst); 877 dst.party = party == null ? null : party.copy(); 878 dst.onHold = onHold == null ? null : onHold.copy(); 879 dst.period = period == null ? null : period.copy(); 880 } 881 882 @Override 883 public boolean equalsDeep(Base other_) { 884 if (!super.equalsDeep(other_)) 885 return false; 886 if (!(other_ instanceof GuarantorComponent)) 887 return false; 888 GuarantorComponent o = (GuarantorComponent) other_; 889 return compareDeep(party, o.party, true) && compareDeep(onHold, o.onHold, true) 890 && compareDeep(period, o.period, true); 891 } 892 893 @Override 894 public boolean equalsShallow(Base other_) { 895 if (!super.equalsShallow(other_)) 896 return false; 897 if (!(other_ instanceof GuarantorComponent)) 898 return false; 899 GuarantorComponent o = (GuarantorComponent) other_; 900 return compareValues(onHold, o.onHold, true); 901 } 902 903 public boolean isEmpty() { 904 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(party, onHold, period); 905 } 906 907 public String fhirType() { 908 return "Account.guarantor"; 909 910 } 911 912 } 913 914 /** 915 * Unique identifier used to reference the account. Might or might not be 916 * intended for human use (e.g. credit card number). 917 */ 918 @Child(name = "identifier", type = { 919 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 920 @Description(shortDefinition = "Account number", formalDefinition = "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).") 921 protected List<Identifier> identifier; 922 923 /** 924 * Indicates whether the account is presently used/usable or not. 925 */ 926 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 927 @Description(shortDefinition = "active | inactive | entered-in-error | on-hold | unknown", formalDefinition = "Indicates whether the account is presently used/usable or not.") 928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/account-status") 929 protected Enumeration<AccountStatus> status; 930 931 /** 932 * Categorizes the account for reporting and searching purposes. 933 */ 934 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 935 @Description(shortDefinition = "E.g. patient, expense, depreciation", formalDefinition = "Categorizes the account for reporting and searching purposes.") 936 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/account-type") 937 protected CodeableConcept type; 938 939 /** 940 * Name used for the account when displaying it to humans in reports, etc. 941 */ 942 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 943 @Description(shortDefinition = "Human-readable label", formalDefinition = "Name used for the account when displaying it to humans in reports, etc.") 944 protected StringType name; 945 946 /** 947 * Identifies the entity which incurs the expenses. While the immediate 948 * recipients of services or goods might be entities related to the subject, the 949 * expenses were ultimately incurred by the subject of the Account. 950 */ 951 @Child(name = "subject", type = { Patient.class, Device.class, Practitioner.class, PractitionerRole.class, 952 Location.class, HealthcareService.class, 953 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 954 @Description(shortDefinition = "The entity that caused the expenses", formalDefinition = "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.") 955 protected List<Reference> subject; 956 /** 957 * The actual objects that are the target of the reference (Identifies the 958 * entity which incurs the expenses. While the immediate recipients of services 959 * or goods might be entities related to the subject, the expenses were 960 * ultimately incurred by the subject of the Account.) 961 */ 962 protected List<Resource> subjectTarget; 963 964 /** 965 * The date range of services associated with this account. 966 */ 967 @Child(name = "servicePeriod", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 968 @Description(shortDefinition = "Transaction window", formalDefinition = "The date range of services associated with this account.") 969 protected Period servicePeriod; 970 971 /** 972 * The party(s) that are responsible for covering the payment of this account, 973 * and what order should they be applied to the account. 974 */ 975 @Child(name = "coverage", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 976 @Description(shortDefinition = "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account", formalDefinition = "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.") 977 protected List<CoverageComponent> coverage; 978 979 /** 980 * Indicates the service area, hospital, department, etc. with responsibility 981 * for managing the Account. 982 */ 983 @Child(name = "owner", type = { Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 984 @Description(shortDefinition = "Entity managing the Account", formalDefinition = "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.") 985 protected Reference owner; 986 987 /** 988 * The actual object that is the target of the reference (Indicates the service 989 * area, hospital, department, etc. with responsibility for managing the 990 * Account.) 991 */ 992 protected Organization ownerTarget; 993 994 /** 995 * Provides additional information about what the account tracks and how it is 996 * used. 997 */ 998 @Child(name = "description", type = { 999 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1000 @Description(shortDefinition = "Explanation of purpose/use", formalDefinition = "Provides additional information about what the account tracks and how it is used.") 1001 protected StringType description; 1002 1003 /** 1004 * The parties responsible for balancing the account if other payment options 1005 * fall short. 1006 */ 1007 @Child(name = "guarantor", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1008 @Description(shortDefinition = "The parties ultimately responsible for balancing the Account", formalDefinition = "The parties responsible for balancing the account if other payment options fall short.") 1009 protected List<GuarantorComponent> guarantor; 1010 1011 /** 1012 * Reference to a parent Account. 1013 */ 1014 @Child(name = "partOf", type = { Account.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1015 @Description(shortDefinition = "Reference to a parent Account", formalDefinition = "Reference to a parent Account.") 1016 protected Reference partOf; 1017 1018 /** 1019 * The actual object that is the target of the reference (Reference to a parent 1020 * Account.) 1021 */ 1022 protected Account partOfTarget; 1023 1024 private static final long serialVersionUID = 1211238069L; 1025 1026 /** 1027 * Constructor 1028 */ 1029 public Account() { 1030 super(); 1031 } 1032 1033 /** 1034 * Constructor 1035 */ 1036 public Account(Enumeration<AccountStatus> status) { 1037 super(); 1038 this.status = status; 1039 } 1040 1041 /** 1042 * @return {@link #identifier} (Unique identifier used to reference the account. 1043 * Might or might not be intended for human use (e.g. credit card 1044 * number).) 1045 */ 1046 public List<Identifier> getIdentifier() { 1047 if (this.identifier == null) 1048 this.identifier = new ArrayList<Identifier>(); 1049 return this.identifier; 1050 } 1051 1052 /** 1053 * @return Returns a reference to <code>this</code> for easy method chaining 1054 */ 1055 public Account setIdentifier(List<Identifier> theIdentifier) { 1056 this.identifier = theIdentifier; 1057 return this; 1058 } 1059 1060 public boolean hasIdentifier() { 1061 if (this.identifier == null) 1062 return false; 1063 for (Identifier item : this.identifier) 1064 if (!item.isEmpty()) 1065 return true; 1066 return false; 1067 } 1068 1069 public Identifier addIdentifier() { // 3 1070 Identifier t = new Identifier(); 1071 if (this.identifier == null) 1072 this.identifier = new ArrayList<Identifier>(); 1073 this.identifier.add(t); 1074 return t; 1075 } 1076 1077 public Account addIdentifier(Identifier t) { // 3 1078 if (t == null) 1079 return this; 1080 if (this.identifier == null) 1081 this.identifier = new ArrayList<Identifier>(); 1082 this.identifier.add(t); 1083 return this; 1084 } 1085 1086 /** 1087 * @return The first repetition of repeating field {@link #identifier}, creating 1088 * it if it does not already exist 1089 */ 1090 public Identifier getIdentifierFirstRep() { 1091 if (getIdentifier().isEmpty()) { 1092 addIdentifier(); 1093 } 1094 return getIdentifier().get(0); 1095 } 1096 1097 /** 1098 * @return {@link #status} (Indicates whether the account is presently 1099 * used/usable or not.). This is the underlying object with id, value 1100 * and extensions. The accessor "getStatus" gives direct access to the 1101 * value 1102 */ 1103 public Enumeration<AccountStatus> getStatusElement() { 1104 if (this.status == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create Account.status"); 1107 else if (Configuration.doAutoCreate()) 1108 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); // bb 1109 return this.status; 1110 } 1111 1112 public boolean hasStatusElement() { 1113 return this.status != null && !this.status.isEmpty(); 1114 } 1115 1116 public boolean hasStatus() { 1117 return this.status != null && !this.status.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #status} (Indicates whether the account is presently 1122 * used/usable or not.). This is the underlying object with id, 1123 * value and extensions. The accessor "getStatus" gives direct 1124 * access to the value 1125 */ 1126 public Account setStatusElement(Enumeration<AccountStatus> value) { 1127 this.status = value; 1128 return this; 1129 } 1130 1131 /** 1132 * @return Indicates whether the account is presently used/usable or not. 1133 */ 1134 public AccountStatus getStatus() { 1135 return this.status == null ? null : this.status.getValue(); 1136 } 1137 1138 /** 1139 * @param value Indicates whether the account is presently used/usable or not. 1140 */ 1141 public Account setStatus(AccountStatus value) { 1142 if (this.status == null) 1143 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); 1144 this.status.setValue(value); 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #type} (Categorizes the account for reporting and searching 1150 * purposes.) 1151 */ 1152 public CodeableConcept getType() { 1153 if (this.type == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create Account.type"); 1156 else if (Configuration.doAutoCreate()) 1157 this.type = new CodeableConcept(); // cc 1158 return this.type; 1159 } 1160 1161 public boolean hasType() { 1162 return this.type != null && !this.type.isEmpty(); 1163 } 1164 1165 /** 1166 * @param value {@link #type} (Categorizes the account for reporting and 1167 * searching purposes.) 1168 */ 1169 public Account setType(CodeableConcept value) { 1170 this.type = value; 1171 return this; 1172 } 1173 1174 /** 1175 * @return {@link #name} (Name used for the account when displaying it to humans 1176 * in reports, etc.). This is the underlying object with id, value and 1177 * extensions. The accessor "getName" gives direct access to the value 1178 */ 1179 public StringType getNameElement() { 1180 if (this.name == null) 1181 if (Configuration.errorOnAutoCreate()) 1182 throw new Error("Attempt to auto-create Account.name"); 1183 else if (Configuration.doAutoCreate()) 1184 this.name = new StringType(); // bb 1185 return this.name; 1186 } 1187 1188 public boolean hasNameElement() { 1189 return this.name != null && !this.name.isEmpty(); 1190 } 1191 1192 public boolean hasName() { 1193 return this.name != null && !this.name.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #name} (Name used for the account when displaying it to 1198 * humans in reports, etc.). This is the underlying object with id, 1199 * value and extensions. The accessor "getName" gives direct access 1200 * to the value 1201 */ 1202 public Account setNameElement(StringType value) { 1203 this.name = value; 1204 return this; 1205 } 1206 1207 /** 1208 * @return Name used for the account when displaying it to humans in reports, 1209 * etc. 1210 */ 1211 public String getName() { 1212 return this.name == null ? null : this.name.getValue(); 1213 } 1214 1215 /** 1216 * @param value Name used for the account when displaying it to humans in 1217 * reports, etc. 1218 */ 1219 public Account setName(String value) { 1220 if (Utilities.noString(value)) 1221 this.name = null; 1222 else { 1223 if (this.name == null) 1224 this.name = new StringType(); 1225 this.name.setValue(value); 1226 } 1227 return this; 1228 } 1229 1230 /** 1231 * @return {@link #subject} (Identifies the entity which incurs the expenses. 1232 * While the immediate recipients of services or goods might be entities 1233 * related to the subject, the expenses were ultimately incurred by the 1234 * subject of the Account.) 1235 */ 1236 public List<Reference> getSubject() { 1237 if (this.subject == null) 1238 this.subject = new ArrayList<Reference>(); 1239 return this.subject; 1240 } 1241 1242 /** 1243 * @return Returns a reference to <code>this</code> for easy method chaining 1244 */ 1245 public Account setSubject(List<Reference> theSubject) { 1246 this.subject = theSubject; 1247 return this; 1248 } 1249 1250 public boolean hasSubject() { 1251 if (this.subject == null) 1252 return false; 1253 for (Reference item : this.subject) 1254 if (!item.isEmpty()) 1255 return true; 1256 return false; 1257 } 1258 1259 public Reference addSubject() { // 3 1260 Reference t = new Reference(); 1261 if (this.subject == null) 1262 this.subject = new ArrayList<Reference>(); 1263 this.subject.add(t); 1264 return t; 1265 } 1266 1267 public Account addSubject(Reference t) { // 3 1268 if (t == null) 1269 return this; 1270 if (this.subject == null) 1271 this.subject = new ArrayList<Reference>(); 1272 this.subject.add(t); 1273 return this; 1274 } 1275 1276 /** 1277 * @return The first repetition of repeating field {@link #subject}, creating it 1278 * if it does not already exist 1279 */ 1280 public Reference getSubjectFirstRep() { 1281 if (getSubject().isEmpty()) { 1282 addSubject(); 1283 } 1284 return getSubject().get(0); 1285 } 1286 1287 /** 1288 * @deprecated Use Reference#setResource(IBaseResource) instead 1289 */ 1290 @Deprecated 1291 public List<Resource> getSubjectTarget() { 1292 if (this.subjectTarget == null) 1293 this.subjectTarget = new ArrayList<Resource>(); 1294 return this.subjectTarget; 1295 } 1296 1297 /** 1298 * @return {@link #servicePeriod} (The date range of services associated with 1299 * this account.) 1300 */ 1301 public Period getServicePeriod() { 1302 if (this.servicePeriod == null) 1303 if (Configuration.errorOnAutoCreate()) 1304 throw new Error("Attempt to auto-create Account.servicePeriod"); 1305 else if (Configuration.doAutoCreate()) 1306 this.servicePeriod = new Period(); // cc 1307 return this.servicePeriod; 1308 } 1309 1310 public boolean hasServicePeriod() { 1311 return this.servicePeriod != null && !this.servicePeriod.isEmpty(); 1312 } 1313 1314 /** 1315 * @param value {@link #servicePeriod} (The date range of services associated 1316 * with this account.) 1317 */ 1318 public Account setServicePeriod(Period value) { 1319 this.servicePeriod = value; 1320 return this; 1321 } 1322 1323 /** 1324 * @return {@link #coverage} (The party(s) that are responsible for covering the 1325 * payment of this account, and what order should they be applied to the 1326 * account.) 1327 */ 1328 public List<CoverageComponent> getCoverage() { 1329 if (this.coverage == null) 1330 this.coverage = new ArrayList<CoverageComponent>(); 1331 return this.coverage; 1332 } 1333 1334 /** 1335 * @return Returns a reference to <code>this</code> for easy method chaining 1336 */ 1337 public Account setCoverage(List<CoverageComponent> theCoverage) { 1338 this.coverage = theCoverage; 1339 return this; 1340 } 1341 1342 public boolean hasCoverage() { 1343 if (this.coverage == null) 1344 return false; 1345 for (CoverageComponent item : this.coverage) 1346 if (!item.isEmpty()) 1347 return true; 1348 return false; 1349 } 1350 1351 public CoverageComponent addCoverage() { // 3 1352 CoverageComponent t = new CoverageComponent(); 1353 if (this.coverage == null) 1354 this.coverage = new ArrayList<CoverageComponent>(); 1355 this.coverage.add(t); 1356 return t; 1357 } 1358 1359 public Account addCoverage(CoverageComponent t) { // 3 1360 if (t == null) 1361 return this; 1362 if (this.coverage == null) 1363 this.coverage = new ArrayList<CoverageComponent>(); 1364 this.coverage.add(t); 1365 return this; 1366 } 1367 1368 /** 1369 * @return The first repetition of repeating field {@link #coverage}, creating 1370 * it if it does not already exist 1371 */ 1372 public CoverageComponent getCoverageFirstRep() { 1373 if (getCoverage().isEmpty()) { 1374 addCoverage(); 1375 } 1376 return getCoverage().get(0); 1377 } 1378 1379 /** 1380 * @return {@link #owner} (Indicates the service area, hospital, department, 1381 * etc. with responsibility for managing the Account.) 1382 */ 1383 public Reference getOwner() { 1384 if (this.owner == null) 1385 if (Configuration.errorOnAutoCreate()) 1386 throw new Error("Attempt to auto-create Account.owner"); 1387 else if (Configuration.doAutoCreate()) 1388 this.owner = new Reference(); // cc 1389 return this.owner; 1390 } 1391 1392 public boolean hasOwner() { 1393 return this.owner != null && !this.owner.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #owner} (Indicates the service area, hospital, 1398 * department, etc. with responsibility for managing the Account.) 1399 */ 1400 public Account setOwner(Reference value) { 1401 this.owner = value; 1402 return this; 1403 } 1404 1405 /** 1406 * @return {@link #owner} The actual object that is the target of the reference. 1407 * The reference library doesn't populate this, but you can use it to 1408 * hold the resource if you resolve it. (Indicates the service area, 1409 * hospital, department, etc. with responsibility for managing the 1410 * Account.) 1411 */ 1412 public Organization getOwnerTarget() { 1413 if (this.ownerTarget == null) 1414 if (Configuration.errorOnAutoCreate()) 1415 throw new Error("Attempt to auto-create Account.owner"); 1416 else if (Configuration.doAutoCreate()) 1417 this.ownerTarget = new Organization(); // aa 1418 return this.ownerTarget; 1419 } 1420 1421 /** 1422 * @param value {@link #owner} The actual object that is the target of the 1423 * reference. The reference library doesn't use these, but you can 1424 * use it to hold the resource if you resolve it. (Indicates the 1425 * service area, hospital, department, etc. with responsibility for 1426 * managing the Account.) 1427 */ 1428 public Account setOwnerTarget(Organization value) { 1429 this.ownerTarget = value; 1430 return this; 1431 } 1432 1433 /** 1434 * @return {@link #description} (Provides additional information about what the 1435 * account tracks and how it is used.). This is the underlying object 1436 * with id, value and extensions. The accessor "getDescription" gives 1437 * direct access to the value 1438 */ 1439 public StringType getDescriptionElement() { 1440 if (this.description == null) 1441 if (Configuration.errorOnAutoCreate()) 1442 throw new Error("Attempt to auto-create Account.description"); 1443 else if (Configuration.doAutoCreate()) 1444 this.description = new StringType(); // bb 1445 return this.description; 1446 } 1447 1448 public boolean hasDescriptionElement() { 1449 return this.description != null && !this.description.isEmpty(); 1450 } 1451 1452 public boolean hasDescription() { 1453 return this.description != null && !this.description.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #description} (Provides additional information about what 1458 * the account tracks and how it is used.). This is the underlying 1459 * object with id, value and extensions. The accessor 1460 * "getDescription" gives direct access to the value 1461 */ 1462 public Account setDescriptionElement(StringType value) { 1463 this.description = value; 1464 return this; 1465 } 1466 1467 /** 1468 * @return Provides additional information about what the account tracks and how 1469 * it is used. 1470 */ 1471 public String getDescription() { 1472 return this.description == null ? null : this.description.getValue(); 1473 } 1474 1475 /** 1476 * @param value Provides additional information about what the account tracks 1477 * and how it is used. 1478 */ 1479 public Account setDescription(String value) { 1480 if (Utilities.noString(value)) 1481 this.description = null; 1482 else { 1483 if (this.description == null) 1484 this.description = new StringType(); 1485 this.description.setValue(value); 1486 } 1487 return this; 1488 } 1489 1490 /** 1491 * @return {@link #guarantor} (The parties responsible for balancing the account 1492 * if other payment options fall short.) 1493 */ 1494 public List<GuarantorComponent> getGuarantor() { 1495 if (this.guarantor == null) 1496 this.guarantor = new ArrayList<GuarantorComponent>(); 1497 return this.guarantor; 1498 } 1499 1500 /** 1501 * @return Returns a reference to <code>this</code> for easy method chaining 1502 */ 1503 public Account setGuarantor(List<GuarantorComponent> theGuarantor) { 1504 this.guarantor = theGuarantor; 1505 return this; 1506 } 1507 1508 public boolean hasGuarantor() { 1509 if (this.guarantor == null) 1510 return false; 1511 for (GuarantorComponent item : this.guarantor) 1512 if (!item.isEmpty()) 1513 return true; 1514 return false; 1515 } 1516 1517 public GuarantorComponent addGuarantor() { // 3 1518 GuarantorComponent t = new GuarantorComponent(); 1519 if (this.guarantor == null) 1520 this.guarantor = new ArrayList<GuarantorComponent>(); 1521 this.guarantor.add(t); 1522 return t; 1523 } 1524 1525 public Account addGuarantor(GuarantorComponent t) { // 3 1526 if (t == null) 1527 return this; 1528 if (this.guarantor == null) 1529 this.guarantor = new ArrayList<GuarantorComponent>(); 1530 this.guarantor.add(t); 1531 return this; 1532 } 1533 1534 /** 1535 * @return The first repetition of repeating field {@link #guarantor}, creating 1536 * it if it does not already exist 1537 */ 1538 public GuarantorComponent getGuarantorFirstRep() { 1539 if (getGuarantor().isEmpty()) { 1540 addGuarantor(); 1541 } 1542 return getGuarantor().get(0); 1543 } 1544 1545 /** 1546 * @return {@link #partOf} (Reference to a parent Account.) 1547 */ 1548 public Reference getPartOf() { 1549 if (this.partOf == null) 1550 if (Configuration.errorOnAutoCreate()) 1551 throw new Error("Attempt to auto-create Account.partOf"); 1552 else if (Configuration.doAutoCreate()) 1553 this.partOf = new Reference(); // cc 1554 return this.partOf; 1555 } 1556 1557 public boolean hasPartOf() { 1558 return this.partOf != null && !this.partOf.isEmpty(); 1559 } 1560 1561 /** 1562 * @param value {@link #partOf} (Reference to a parent Account.) 1563 */ 1564 public Account setPartOf(Reference value) { 1565 this.partOf = value; 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #partOf} The actual object that is the target of the 1571 * reference. The reference library doesn't populate this, but you can 1572 * use it to hold the resource if you resolve it. (Reference to a parent 1573 * Account.) 1574 */ 1575 public Account getPartOfTarget() { 1576 if (this.partOfTarget == null) 1577 if (Configuration.errorOnAutoCreate()) 1578 throw new Error("Attempt to auto-create Account.partOf"); 1579 else if (Configuration.doAutoCreate()) 1580 this.partOfTarget = new Account(); // aa 1581 return this.partOfTarget; 1582 } 1583 1584 /** 1585 * @param value {@link #partOf} The actual object that is the target of the 1586 * reference. The reference library doesn't use these, but you can 1587 * use it to hold the resource if you resolve it. (Reference to a 1588 * parent Account.) 1589 */ 1590 public Account setPartOfTarget(Account value) { 1591 this.partOfTarget = value; 1592 return this; 1593 } 1594 1595 protected void listChildren(List<Property> children) { 1596 super.listChildren(children); 1597 children.add(new Property("identifier", "Identifier", 1598 "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).", 1599 0, java.lang.Integer.MAX_VALUE, identifier)); 1600 children.add( 1601 new Property("status", "code", "Indicates whether the account is presently used/usable or not.", 0, 1, status)); 1602 children.add(new Property("type", "CodeableConcept", 1603 "Categorizes the account for reporting and searching purposes.", 0, 1, type)); 1604 children.add(new Property("name", "string", 1605 "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name)); 1606 children.add(new Property("subject", 1607 "Reference(Patient|Device|Practitioner|PractitionerRole|Location|HealthcareService|Organization)", 1608 "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.", 1609 0, java.lang.Integer.MAX_VALUE, subject)); 1610 children.add(new Property("servicePeriod", "Period", "The date range of services associated with this account.", 0, 1611 1, servicePeriod)); 1612 children.add(new Property("coverage", "", 1613 "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 1614 0, java.lang.Integer.MAX_VALUE, coverage)); 1615 children.add(new Property("owner", "Reference(Organization)", 1616 "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.", 0, 1, 1617 owner)); 1618 children.add(new Property("description", "string", 1619 "Provides additional information about what the account tracks and how it is used.", 0, 1, description)); 1620 children.add(new Property("guarantor", "", 1621 "The parties responsible for balancing the account if other payment options fall short.", 0, 1622 java.lang.Integer.MAX_VALUE, guarantor)); 1623 children.add(new Property("partOf", "Reference(Account)", "Reference to a parent Account.", 0, 1, partOf)); 1624 } 1625 1626 @Override 1627 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1628 switch (_hash) { 1629 case -1618432855: 1630 /* identifier */ return new Property("identifier", "Identifier", 1631 "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).", 1632 0, java.lang.Integer.MAX_VALUE, identifier); 1633 case -892481550: 1634 /* status */ return new Property("status", "code", 1635 "Indicates whether the account is presently used/usable or not.", 0, 1, status); 1636 case 3575610: 1637 /* type */ return new Property("type", "CodeableConcept", 1638 "Categorizes the account for reporting and searching purposes.", 0, 1, type); 1639 case 3373707: 1640 /* name */ return new Property("name", "string", 1641 "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name); 1642 case -1867885268: 1643 /* subject */ return new Property("subject", 1644 "Reference(Patient|Device|Practitioner|PractitionerRole|Location|HealthcareService|Organization)", 1645 "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.", 1646 0, java.lang.Integer.MAX_VALUE, subject); 1647 case 2129104086: 1648 /* servicePeriod */ return new Property("servicePeriod", "Period", 1649 "The date range of services associated with this account.", 0, 1, servicePeriod); 1650 case -351767064: 1651 /* coverage */ return new Property("coverage", "", 1652 "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 1653 0, java.lang.Integer.MAX_VALUE, coverage); 1654 case 106164915: 1655 /* owner */ return new Property("owner", "Reference(Organization)", 1656 "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.", 0, 1, 1657 owner); 1658 case -1724546052: 1659 /* description */ return new Property("description", "string", 1660 "Provides additional information about what the account tracks and how it is used.", 0, 1, description); 1661 case -188629045: 1662 /* guarantor */ return new Property("guarantor", "", 1663 "The parties responsible for balancing the account if other payment options fall short.", 0, 1664 java.lang.Integer.MAX_VALUE, guarantor); 1665 case -995410646: 1666 /* partOf */ return new Property("partOf", "Reference(Account)", "Reference to a parent Account.", 0, 1, partOf); 1667 default: 1668 return super.getNamedProperty(_hash, _name, _checkValid); 1669 } 1670 1671 } 1672 1673 @Override 1674 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1675 switch (hash) { 1676 case -1618432855: 1677 /* identifier */ return this.identifier == null ? new Base[0] 1678 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1679 case -892481550: 1680 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<AccountStatus> 1681 case 3575610: 1682 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1683 case 3373707: 1684 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1685 case -1867885268: 1686 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 1687 case 2129104086: 1688 /* servicePeriod */ return this.servicePeriod == null ? new Base[0] : new Base[] { this.servicePeriod }; // Period 1689 case -351767064: 1690 /* coverage */ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // CoverageComponent 1691 case 106164915: 1692 /* owner */ return this.owner == null ? new Base[0] : new Base[] { this.owner }; // Reference 1693 case -1724546052: 1694 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1695 case -188629045: 1696 /* guarantor */ return this.guarantor == null ? new Base[0] 1697 : this.guarantor.toArray(new Base[this.guarantor.size()]); // GuarantorComponent 1698 case -995410646: 1699 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 1700 default: 1701 return super.getProperty(hash, name, checkValid); 1702 } 1703 1704 } 1705 1706 @Override 1707 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1708 switch (hash) { 1709 case -1618432855: // identifier 1710 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1711 return value; 1712 case -892481550: // status 1713 value = new AccountStatusEnumFactory().fromType(castToCode(value)); 1714 this.status = (Enumeration) value; // Enumeration<AccountStatus> 1715 return value; 1716 case 3575610: // type 1717 this.type = castToCodeableConcept(value); // CodeableConcept 1718 return value; 1719 case 3373707: // name 1720 this.name = castToString(value); // StringType 1721 return value; 1722 case -1867885268: // subject 1723 this.getSubject().add(castToReference(value)); // Reference 1724 return value; 1725 case 2129104086: // servicePeriod 1726 this.servicePeriod = castToPeriod(value); // Period 1727 return value; 1728 case -351767064: // coverage 1729 this.getCoverage().add((CoverageComponent) value); // CoverageComponent 1730 return value; 1731 case 106164915: // owner 1732 this.owner = castToReference(value); // Reference 1733 return value; 1734 case -1724546052: // description 1735 this.description = castToString(value); // StringType 1736 return value; 1737 case -188629045: // guarantor 1738 this.getGuarantor().add((GuarantorComponent) value); // GuarantorComponent 1739 return value; 1740 case -995410646: // partOf 1741 this.partOf = castToReference(value); // Reference 1742 return value; 1743 default: 1744 return super.setProperty(hash, name, value); 1745 } 1746 1747 } 1748 1749 @Override 1750 public Base setProperty(String name, Base value) throws FHIRException { 1751 if (name.equals("identifier")) { 1752 this.getIdentifier().add(castToIdentifier(value)); 1753 } else if (name.equals("status")) { 1754 value = new AccountStatusEnumFactory().fromType(castToCode(value)); 1755 this.status = (Enumeration) value; // Enumeration<AccountStatus> 1756 } else if (name.equals("type")) { 1757 this.type = castToCodeableConcept(value); // CodeableConcept 1758 } else if (name.equals("name")) { 1759 this.name = castToString(value); // StringType 1760 } else if (name.equals("subject")) { 1761 this.getSubject().add(castToReference(value)); 1762 } else if (name.equals("servicePeriod")) { 1763 this.servicePeriod = castToPeriod(value); // Period 1764 } else if (name.equals("coverage")) { 1765 this.getCoverage().add((CoverageComponent) value); 1766 } else if (name.equals("owner")) { 1767 this.owner = castToReference(value); // Reference 1768 } else if (name.equals("description")) { 1769 this.description = castToString(value); // StringType 1770 } else if (name.equals("guarantor")) { 1771 this.getGuarantor().add((GuarantorComponent) value); 1772 } else if (name.equals("partOf")) { 1773 this.partOf = castToReference(value); // Reference 1774 } else 1775 return super.setProperty(name, value); 1776 return value; 1777 } 1778 1779 @Override 1780 public void removeChild(String name, Base value) throws FHIRException { 1781 if (name.equals("identifier")) { 1782 this.getIdentifier().remove(castToIdentifier(value)); 1783 } else if (name.equals("status")) { 1784 this.status = null; 1785 } else if (name.equals("type")) { 1786 this.type = null; 1787 } else if (name.equals("name")) { 1788 this.name = null; 1789 } else if (name.equals("subject")) { 1790 this.getSubject().remove(castToReference(value)); 1791 } else if (name.equals("servicePeriod")) { 1792 this.servicePeriod = null; 1793 } else if (name.equals("coverage")) { 1794 this.getCoverage().remove((CoverageComponent) value); 1795 } else if (name.equals("owner")) { 1796 this.owner = null; 1797 } else if (name.equals("description")) { 1798 this.description = null; 1799 } else if (name.equals("guarantor")) { 1800 this.getGuarantor().remove((GuarantorComponent) value); 1801 } else if (name.equals("partOf")) { 1802 this.partOf = null; 1803 } else 1804 super.removeChild(name, value); 1805 1806 } 1807 1808 @Override 1809 public Base makeProperty(int hash, String name) throws FHIRException { 1810 switch (hash) { 1811 case -1618432855: 1812 return addIdentifier(); 1813 case -892481550: 1814 return getStatusElement(); 1815 case 3575610: 1816 return getType(); 1817 case 3373707: 1818 return getNameElement(); 1819 case -1867885268: 1820 return addSubject(); 1821 case 2129104086: 1822 return getServicePeriod(); 1823 case -351767064: 1824 return addCoverage(); 1825 case 106164915: 1826 return getOwner(); 1827 case -1724546052: 1828 return getDescriptionElement(); 1829 case -188629045: 1830 return addGuarantor(); 1831 case -995410646: 1832 return getPartOf(); 1833 default: 1834 return super.makeProperty(hash, name); 1835 } 1836 1837 } 1838 1839 @Override 1840 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1841 switch (hash) { 1842 case -1618432855: 1843 /* identifier */ return new String[] { "Identifier" }; 1844 case -892481550: 1845 /* status */ return new String[] { "code" }; 1846 case 3575610: 1847 /* type */ return new String[] { "CodeableConcept" }; 1848 case 3373707: 1849 /* name */ return new String[] { "string" }; 1850 case -1867885268: 1851 /* subject */ return new String[] { "Reference" }; 1852 case 2129104086: 1853 /* servicePeriod */ return new String[] { "Period" }; 1854 case -351767064: 1855 /* coverage */ return new String[] {}; 1856 case 106164915: 1857 /* owner */ return new String[] { "Reference" }; 1858 case -1724546052: 1859 /* description */ return new String[] { "string" }; 1860 case -188629045: 1861 /* guarantor */ return new String[] {}; 1862 case -995410646: 1863 /* partOf */ return new String[] { "Reference" }; 1864 default: 1865 return super.getTypesForProperty(hash, name); 1866 } 1867 1868 } 1869 1870 @Override 1871 public Base addChild(String name) throws FHIRException { 1872 if (name.equals("identifier")) { 1873 return addIdentifier(); 1874 } else if (name.equals("status")) { 1875 throw new FHIRException("Cannot call addChild on a singleton property Account.status"); 1876 } else if (name.equals("type")) { 1877 this.type = new CodeableConcept(); 1878 return this.type; 1879 } else if (name.equals("name")) { 1880 throw new FHIRException("Cannot call addChild on a singleton property Account.name"); 1881 } else if (name.equals("subject")) { 1882 return addSubject(); 1883 } else if (name.equals("servicePeriod")) { 1884 this.servicePeriod = new Period(); 1885 return this.servicePeriod; 1886 } else if (name.equals("coverage")) { 1887 return addCoverage(); 1888 } else if (name.equals("owner")) { 1889 this.owner = new Reference(); 1890 return this.owner; 1891 } else if (name.equals("description")) { 1892 throw new FHIRException("Cannot call addChild on a singleton property Account.description"); 1893 } else if (name.equals("guarantor")) { 1894 return addGuarantor(); 1895 } else if (name.equals("partOf")) { 1896 this.partOf = new Reference(); 1897 return this.partOf; 1898 } else 1899 return super.addChild(name); 1900 } 1901 1902 public String fhirType() { 1903 return "Account"; 1904 1905 } 1906 1907 public Account copy() { 1908 Account dst = new Account(); 1909 copyValues(dst); 1910 return dst; 1911 } 1912 1913 public void copyValues(Account dst) { 1914 super.copyValues(dst); 1915 if (identifier != null) { 1916 dst.identifier = new ArrayList<Identifier>(); 1917 for (Identifier i : identifier) 1918 dst.identifier.add(i.copy()); 1919 } 1920 ; 1921 dst.status = status == null ? null : status.copy(); 1922 dst.type = type == null ? null : type.copy(); 1923 dst.name = name == null ? null : name.copy(); 1924 if (subject != null) { 1925 dst.subject = new ArrayList<Reference>(); 1926 for (Reference i : subject) 1927 dst.subject.add(i.copy()); 1928 } 1929 ; 1930 dst.servicePeriod = servicePeriod == null ? null : servicePeriod.copy(); 1931 if (coverage != null) { 1932 dst.coverage = new ArrayList<CoverageComponent>(); 1933 for (CoverageComponent i : coverage) 1934 dst.coverage.add(i.copy()); 1935 } 1936 ; 1937 dst.owner = owner == null ? null : owner.copy(); 1938 dst.description = description == null ? null : description.copy(); 1939 if (guarantor != null) { 1940 dst.guarantor = new ArrayList<GuarantorComponent>(); 1941 for (GuarantorComponent i : guarantor) 1942 dst.guarantor.add(i.copy()); 1943 } 1944 ; 1945 dst.partOf = partOf == null ? null : partOf.copy(); 1946 } 1947 1948 protected Account typedCopy() { 1949 return copy(); 1950 } 1951 1952 @Override 1953 public boolean equalsDeep(Base other_) { 1954 if (!super.equalsDeep(other_)) 1955 return false; 1956 if (!(other_ instanceof Account)) 1957 return false; 1958 Account o = (Account) other_; 1959 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1960 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) 1961 && compareDeep(servicePeriod, o.servicePeriod, true) && compareDeep(coverage, o.coverage, true) 1962 && compareDeep(owner, o.owner, true) && compareDeep(description, o.description, true) 1963 && compareDeep(guarantor, o.guarantor, true) && compareDeep(partOf, o.partOf, true); 1964 } 1965 1966 @Override 1967 public boolean equalsShallow(Base other_) { 1968 if (!super.equalsShallow(other_)) 1969 return false; 1970 if (!(other_ instanceof Account)) 1971 return false; 1972 Account o = (Account) other_; 1973 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 1974 && compareValues(description, o.description, true); 1975 } 1976 1977 public boolean isEmpty() { 1978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, name, subject, 1979 servicePeriod, coverage, owner, description, guarantor, partOf); 1980 } 1981 1982 @Override 1983 public ResourceType getResourceType() { 1984 return ResourceType.Account; 1985 } 1986 1987 /** 1988 * Search parameter: <b>owner</b> 1989 * <p> 1990 * Description: <b>Entity managing the Account</b><br> 1991 * Type: <b>reference</b><br> 1992 * Path: <b>Account.owner</b><br> 1993 * </p> 1994 */ 1995 @SearchParamDefinition(name = "owner", path = "Account.owner", description = "Entity managing the Account", type = "reference", target = { 1996 Organization.class }) 1997 public static final String SP_OWNER = "owner"; 1998 /** 1999 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 2000 * <p> 2001 * Description: <b>Entity managing the Account</b><br> 2002 * Type: <b>reference</b><br> 2003 * Path: <b>Account.owner</b><br> 2004 * </p> 2005 */ 2006 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2007 SP_OWNER); 2008 2009 /** 2010 * Constant for fluent queries to be used to add include statements. Specifies 2011 * the path value of "<b>Account:owner</b>". 2012 */ 2013 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Account:owner") 2014 .toLocked(); 2015 2016 /** 2017 * Search parameter: <b>identifier</b> 2018 * <p> 2019 * Description: <b>Account number</b><br> 2020 * Type: <b>token</b><br> 2021 * Path: <b>Account.identifier</b><br> 2022 * </p> 2023 */ 2024 @SearchParamDefinition(name = "identifier", path = "Account.identifier", description = "Account number", type = "token") 2025 public static final String SP_IDENTIFIER = "identifier"; 2026 /** 2027 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2028 * <p> 2029 * Description: <b>Account number</b><br> 2030 * Type: <b>token</b><br> 2031 * Path: <b>Account.identifier</b><br> 2032 * </p> 2033 */ 2034 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2035 SP_IDENTIFIER); 2036 2037 /** 2038 * Search parameter: <b>period</b> 2039 * <p> 2040 * Description: <b>Transaction window</b><br> 2041 * Type: <b>date</b><br> 2042 * Path: <b>Account.servicePeriod</b><br> 2043 * </p> 2044 */ 2045 @SearchParamDefinition(name = "period", path = "Account.servicePeriod", description = "Transaction window", type = "date") 2046 public static final String SP_PERIOD = "period"; 2047 /** 2048 * <b>Fluent Client</b> search parameter constant for <b>period</b> 2049 * <p> 2050 * Description: <b>Transaction window</b><br> 2051 * Type: <b>date</b><br> 2052 * Path: <b>Account.servicePeriod</b><br> 2053 * </p> 2054 */ 2055 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 2056 SP_PERIOD); 2057 2058 /** 2059 * Search parameter: <b>subject</b> 2060 * <p> 2061 * Description: <b>The entity that caused the expenses</b><br> 2062 * Type: <b>reference</b><br> 2063 * Path: <b>Account.subject</b><br> 2064 * </p> 2065 */ 2066 @SearchParamDefinition(name = "subject", path = "Account.subject", description = "The entity that caused the expenses", type = "reference", providesMembershipIn = { 2067 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2068 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2069 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2070 HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, 2071 PractitionerRole.class }) 2072 public static final String SP_SUBJECT = "subject"; 2073 /** 2074 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2075 * <p> 2076 * Description: <b>The entity that caused the expenses</b><br> 2077 * Type: <b>reference</b><br> 2078 * Path: <b>Account.subject</b><br> 2079 * </p> 2080 */ 2081 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2082 SP_SUBJECT); 2083 2084 /** 2085 * Constant for fluent queries to be used to add include statements. Specifies 2086 * the path value of "<b>Account:subject</b>". 2087 */ 2088 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2089 "Account:subject").toLocked(); 2090 2091 /** 2092 * Search parameter: <b>patient</b> 2093 * <p> 2094 * Description: <b>The entity that caused the expenses</b><br> 2095 * Type: <b>reference</b><br> 2096 * Path: <b>Account.subject</b><br> 2097 * </p> 2098 */ 2099 @SearchParamDefinition(name = "patient", path = "Account.subject.where(resolve() is Patient)", description = "The entity that caused the expenses", type = "reference", target = { 2100 Patient.class }) 2101 public static final String SP_PATIENT = "patient"; 2102 /** 2103 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2104 * <p> 2105 * Description: <b>The entity that caused the expenses</b><br> 2106 * Type: <b>reference</b><br> 2107 * Path: <b>Account.subject</b><br> 2108 * </p> 2109 */ 2110 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2111 SP_PATIENT); 2112 2113 /** 2114 * Constant for fluent queries to be used to add include statements. Specifies 2115 * the path value of "<b>Account:patient</b>". 2116 */ 2117 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2118 "Account:patient").toLocked(); 2119 2120 /** 2121 * Search parameter: <b>name</b> 2122 * <p> 2123 * Description: <b>Human-readable label</b><br> 2124 * Type: <b>string</b><br> 2125 * Path: <b>Account.name</b><br> 2126 * </p> 2127 */ 2128 @SearchParamDefinition(name = "name", path = "Account.name", description = "Human-readable label", type = "string") 2129 public static final String SP_NAME = "name"; 2130 /** 2131 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2132 * <p> 2133 * Description: <b>Human-readable label</b><br> 2134 * Type: <b>string</b><br> 2135 * Path: <b>Account.name</b><br> 2136 * </p> 2137 */ 2138 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 2139 SP_NAME); 2140 2141 /** 2142 * Search parameter: <b>type</b> 2143 * <p> 2144 * Description: <b>E.g. patient, expense, depreciation</b><br> 2145 * Type: <b>token</b><br> 2146 * Path: <b>Account.type</b><br> 2147 * </p> 2148 */ 2149 @SearchParamDefinition(name = "type", path = "Account.type", description = "E.g. patient, expense, depreciation", type = "token") 2150 public static final String SP_TYPE = "type"; 2151 /** 2152 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2153 * <p> 2154 * Description: <b>E.g. patient, expense, depreciation</b><br> 2155 * Type: <b>token</b><br> 2156 * Path: <b>Account.type</b><br> 2157 * </p> 2158 */ 2159 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2160 SP_TYPE); 2161 2162 /** 2163 * Search parameter: <b>status</b> 2164 * <p> 2165 * Description: <b>active | inactive | entered-in-error | on-hold | 2166 * unknown</b><br> 2167 * Type: <b>token</b><br> 2168 * Path: <b>Account.status</b><br> 2169 * </p> 2170 */ 2171 @SearchParamDefinition(name = "status", path = "Account.status", description = "active | inactive | entered-in-error | on-hold | unknown", type = "token") 2172 public static final String SP_STATUS = "status"; 2173 /** 2174 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2175 * <p> 2176 * Description: <b>active | inactive | entered-in-error | on-hold | 2177 * unknown</b><br> 2178 * Type: <b>token</b><br> 2179 * Path: <b>Account.status</b><br> 2180 * </p> 2181 */ 2182 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2183 SP_STATUS); 2184 2185}