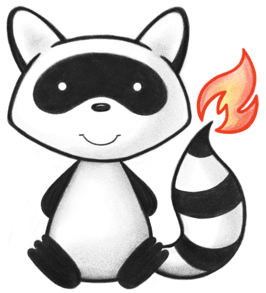
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * This resource allows for the definition of some activity to be performed, 052 * independent of a particular patient, practitioner, or other performance 053 * context. 054 */ 055@ResourceDef(name = "ActivityDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ActivityDefinition") 056@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "status", "experimental", 057 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 058 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 059 "endorser", "relatedArtifact", "library", "kind", "profile", "code", "intent", "priority", "doNotPerform", 060 "timing[x]", "location", "participant", "product[x]", "quantity", "dosage", "bodySite", "specimenRequirement", 061 "observationRequirement", "observationResultRequirement", "transform", "dynamicValue" }) 062public class ActivityDefinition extends MetadataResource { 063 064 public enum ActivityDefinitionKind { 065 /** 066 * A booking of a healthcare event among patient(s), practitioner(s), related 067 * person(s) and/or device(s) for a specific date/time. This may result in one 068 * or more Encounter(s). 069 */ 070 APPOINTMENT, 071 /** 072 * A reply to an appointment request for a patient and/or practitioner(s), such 073 * as a confirmation or rejection. 074 */ 075 APPOINTMENTRESPONSE, 076 /** 077 * Healthcare plan for patient or group. 078 */ 079 CAREPLAN, 080 /** 081 * Claim, Pre-determination or Pre-authorization. 082 */ 083 CLAIM, 084 /** 085 * A request for information to be sent to a receiver. 086 */ 087 COMMUNICATIONREQUEST, 088 /** 089 * Legal Agreement. 090 */ 091 CONTRACT, 092 /** 093 * Medical device request. 094 */ 095 DEVICEREQUEST, 096 /** 097 * Enrollment request. 098 */ 099 ENROLLMENTREQUEST, 100 /** 101 * Guidance or advice relating to an immunization. 102 */ 103 IMMUNIZATIONRECOMMENDATION, 104 /** 105 * Ordering of medication for patient or group. 106 */ 107 MEDICATIONREQUEST, 108 /** 109 * Diet, formula or nutritional supplement request. 110 */ 111 NUTRITIONORDER, 112 /** 113 * A record of a request for service such as diagnostic investigations, 114 * treatments, or operations to be performed. 115 */ 116 SERVICEREQUEST, 117 /** 118 * Request for a medication, substance or device. 119 */ 120 SUPPLYREQUEST, 121 /** 122 * A task to be performed. 123 */ 124 TASK, 125 /** 126 * Prescription for vision correction products for a patient. 127 */ 128 VISIONPRESCRIPTION, 129 /** 130 * added to help the parsers with the generic types 131 */ 132 NULL; 133 134 public static ActivityDefinitionKind fromCode(String codeString) throws FHIRException { 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("Appointment".equals(codeString)) 138 return APPOINTMENT; 139 if ("AppointmentResponse".equals(codeString)) 140 return APPOINTMENTRESPONSE; 141 if ("CarePlan".equals(codeString)) 142 return CAREPLAN; 143 if ("Claim".equals(codeString)) 144 return CLAIM; 145 if ("CommunicationRequest".equals(codeString)) 146 return COMMUNICATIONREQUEST; 147 if ("Contract".equals(codeString)) 148 return CONTRACT; 149 if ("DeviceRequest".equals(codeString)) 150 return DEVICEREQUEST; 151 if ("EnrollmentRequest".equals(codeString)) 152 return ENROLLMENTREQUEST; 153 if ("ImmunizationRecommendation".equals(codeString)) 154 return IMMUNIZATIONRECOMMENDATION; 155 if ("MedicationRequest".equals(codeString)) 156 return MEDICATIONREQUEST; 157 if ("NutritionOrder".equals(codeString)) 158 return NUTRITIONORDER; 159 if ("ServiceRequest".equals(codeString)) 160 return SERVICEREQUEST; 161 if ("SupplyRequest".equals(codeString)) 162 return SUPPLYREQUEST; 163 if ("Task".equals(codeString)) 164 return TASK; 165 if ("VisionPrescription".equals(codeString)) 166 return VISIONPRESCRIPTION; 167 if (Configuration.isAcceptInvalidEnums()) 168 return null; 169 else 170 throw new FHIRException("Unknown ActivityDefinitionKind code '" + codeString + "'"); 171 } 172 173 public String toCode() { 174 switch (this) { 175 case APPOINTMENT: 176 return "Appointment"; 177 case APPOINTMENTRESPONSE: 178 return "AppointmentResponse"; 179 case CAREPLAN: 180 return "CarePlan"; 181 case CLAIM: 182 return "Claim"; 183 case COMMUNICATIONREQUEST: 184 return "CommunicationRequest"; 185 case CONTRACT: 186 return "Contract"; 187 case DEVICEREQUEST: 188 return "DeviceRequest"; 189 case ENROLLMENTREQUEST: 190 return "EnrollmentRequest"; 191 case IMMUNIZATIONRECOMMENDATION: 192 return "ImmunizationRecommendation"; 193 case MEDICATIONREQUEST: 194 return "MedicationRequest"; 195 case NUTRITIONORDER: 196 return "NutritionOrder"; 197 case SERVICEREQUEST: 198 return "ServiceRequest"; 199 case SUPPLYREQUEST: 200 return "SupplyRequest"; 201 case TASK: 202 return "Task"; 203 case VISIONPRESCRIPTION: 204 return "VisionPrescription"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getSystem() { 213 switch (this) { 214 case APPOINTMENT: 215 return "http://hl7.org/fhir/request-resource-types"; 216 case APPOINTMENTRESPONSE: 217 return "http://hl7.org/fhir/request-resource-types"; 218 case CAREPLAN: 219 return "http://hl7.org/fhir/request-resource-types"; 220 case CLAIM: 221 return "http://hl7.org/fhir/request-resource-types"; 222 case COMMUNICATIONREQUEST: 223 return "http://hl7.org/fhir/request-resource-types"; 224 case CONTRACT: 225 return "http://hl7.org/fhir/request-resource-types"; 226 case DEVICEREQUEST: 227 return "http://hl7.org/fhir/request-resource-types"; 228 case ENROLLMENTREQUEST: 229 return "http://hl7.org/fhir/request-resource-types"; 230 case IMMUNIZATIONRECOMMENDATION: 231 return "http://hl7.org/fhir/request-resource-types"; 232 case MEDICATIONREQUEST: 233 return "http://hl7.org/fhir/request-resource-types"; 234 case NUTRITIONORDER: 235 return "http://hl7.org/fhir/request-resource-types"; 236 case SERVICEREQUEST: 237 return "http://hl7.org/fhir/request-resource-types"; 238 case SUPPLYREQUEST: 239 return "http://hl7.org/fhir/request-resource-types"; 240 case TASK: 241 return "http://hl7.org/fhir/request-resource-types"; 242 case VISIONPRESCRIPTION: 243 return "http://hl7.org/fhir/request-resource-types"; 244 case NULL: 245 return null; 246 default: 247 return "?"; 248 } 249 } 250 251 public String getDefinition() { 252 switch (this) { 253 case APPOINTMENT: 254 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 255 case APPOINTMENTRESPONSE: 256 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 257 case CAREPLAN: 258 return "Healthcare plan for patient or group."; 259 case CLAIM: 260 return "Claim, Pre-determination or Pre-authorization."; 261 case COMMUNICATIONREQUEST: 262 return "A request for information to be sent to a receiver."; 263 case CONTRACT: 264 return "Legal Agreement."; 265 case DEVICEREQUEST: 266 return "Medical device request."; 267 case ENROLLMENTREQUEST: 268 return "Enrollment request."; 269 case IMMUNIZATIONRECOMMENDATION: 270 return "Guidance or advice relating to an immunization."; 271 case MEDICATIONREQUEST: 272 return "Ordering of medication for patient or group."; 273 case NUTRITIONORDER: 274 return "Diet, formula or nutritional supplement request."; 275 case SERVICEREQUEST: 276 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 277 case SUPPLYREQUEST: 278 return "Request for a medication, substance or device."; 279 case TASK: 280 return "A task to be performed."; 281 case VISIONPRESCRIPTION: 282 return "Prescription for vision correction products for a patient."; 283 case NULL: 284 return null; 285 default: 286 return "?"; 287 } 288 } 289 290 public String getDisplay() { 291 switch (this) { 292 case APPOINTMENT: 293 return "Appointment"; 294 case APPOINTMENTRESPONSE: 295 return "AppointmentResponse"; 296 case CAREPLAN: 297 return "CarePlan"; 298 case CLAIM: 299 return "Claim"; 300 case COMMUNICATIONREQUEST: 301 return "CommunicationRequest"; 302 case CONTRACT: 303 return "Contract"; 304 case DEVICEREQUEST: 305 return "DeviceRequest"; 306 case ENROLLMENTREQUEST: 307 return "EnrollmentRequest"; 308 case IMMUNIZATIONRECOMMENDATION: 309 return "ImmunizationRecommendation"; 310 case MEDICATIONREQUEST: 311 return "MedicationRequest"; 312 case NUTRITIONORDER: 313 return "NutritionOrder"; 314 case SERVICEREQUEST: 315 return "ServiceRequest"; 316 case SUPPLYREQUEST: 317 return "SupplyRequest"; 318 case TASK: 319 return "Task"; 320 case VISIONPRESCRIPTION: 321 return "VisionPrescription"; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 } 329 330 public static class ActivityDefinitionKindEnumFactory implements EnumFactory<ActivityDefinitionKind> { 331 public ActivityDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 332 if (codeString == null || "".equals(codeString)) 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("Appointment".equals(codeString)) 336 return ActivityDefinitionKind.APPOINTMENT; 337 if ("AppointmentResponse".equals(codeString)) 338 return ActivityDefinitionKind.APPOINTMENTRESPONSE; 339 if ("CarePlan".equals(codeString)) 340 return ActivityDefinitionKind.CAREPLAN; 341 if ("Claim".equals(codeString)) 342 return ActivityDefinitionKind.CLAIM; 343 if ("CommunicationRequest".equals(codeString)) 344 return ActivityDefinitionKind.COMMUNICATIONREQUEST; 345 if ("Contract".equals(codeString)) 346 return ActivityDefinitionKind.CONTRACT; 347 if ("DeviceRequest".equals(codeString)) 348 return ActivityDefinitionKind.DEVICEREQUEST; 349 if ("EnrollmentRequest".equals(codeString)) 350 return ActivityDefinitionKind.ENROLLMENTREQUEST; 351 if ("ImmunizationRecommendation".equals(codeString)) 352 return ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION; 353 if ("MedicationRequest".equals(codeString)) 354 return ActivityDefinitionKind.MEDICATIONREQUEST; 355 if ("NutritionOrder".equals(codeString)) 356 return ActivityDefinitionKind.NUTRITIONORDER; 357 if ("ServiceRequest".equals(codeString)) 358 return ActivityDefinitionKind.SERVICEREQUEST; 359 if ("SupplyRequest".equals(codeString)) 360 return ActivityDefinitionKind.SUPPLYREQUEST; 361 if ("Task".equals(codeString)) 362 return ActivityDefinitionKind.TASK; 363 if ("VisionPrescription".equals(codeString)) 364 return ActivityDefinitionKind.VISIONPRESCRIPTION; 365 throw new IllegalArgumentException("Unknown ActivityDefinitionKind code '" + codeString + "'"); 366 } 367 368 public Enumeration<ActivityDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 369 if (code == null) 370 return null; 371 if (code.isEmpty()) 372 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NULL, code); 373 String codeString = code.asStringValue(); 374 if (codeString == null || "".equals(codeString)) 375 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NULL, code); 376 if ("Appointment".equals(codeString)) 377 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.APPOINTMENT, code); 378 if ("AppointmentResponse".equals(codeString)) 379 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.APPOINTMENTRESPONSE, code); 380 if ("CarePlan".equals(codeString)) 381 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CAREPLAN, code); 382 if ("Claim".equals(codeString)) 383 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CLAIM, code); 384 if ("CommunicationRequest".equals(codeString)) 385 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COMMUNICATIONREQUEST, code); 386 if ("Contract".equals(codeString)) 387 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CONTRACT, code); 388 if ("DeviceRequest".equals(codeString)) 389 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICEREQUEST, code); 390 if ("EnrollmentRequest".equals(codeString)) 391 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ENROLLMENTREQUEST, code); 392 if ("ImmunizationRecommendation".equals(codeString)) 393 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION, code); 394 if ("MedicationRequest".equals(codeString)) 395 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATIONREQUEST, code); 396 if ("NutritionOrder".equals(codeString)) 397 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NUTRITIONORDER, code); 398 if ("ServiceRequest".equals(codeString)) 399 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SERVICEREQUEST, code); 400 if ("SupplyRequest".equals(codeString)) 401 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SUPPLYREQUEST, code); 402 if ("Task".equals(codeString)) 403 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.TASK, code); 404 if ("VisionPrescription".equals(codeString)) 405 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.VISIONPRESCRIPTION, code); 406 throw new FHIRException("Unknown ActivityDefinitionKind code '" + codeString + "'"); 407 } 408 409 public String toCode(ActivityDefinitionKind code) { 410 if (code == ActivityDefinitionKind.NULL) 411 return null; 412 if (code == ActivityDefinitionKind.APPOINTMENT) 413 return "Appointment"; 414 if (code == ActivityDefinitionKind.APPOINTMENTRESPONSE) 415 return "AppointmentResponse"; 416 if (code == ActivityDefinitionKind.CAREPLAN) 417 return "CarePlan"; 418 if (code == ActivityDefinitionKind.CLAIM) 419 return "Claim"; 420 if (code == ActivityDefinitionKind.COMMUNICATIONREQUEST) 421 return "CommunicationRequest"; 422 if (code == ActivityDefinitionKind.CONTRACT) 423 return "Contract"; 424 if (code == ActivityDefinitionKind.DEVICEREQUEST) 425 return "DeviceRequest"; 426 if (code == ActivityDefinitionKind.ENROLLMENTREQUEST) 427 return "EnrollmentRequest"; 428 if (code == ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION) 429 return "ImmunizationRecommendation"; 430 if (code == ActivityDefinitionKind.MEDICATIONREQUEST) 431 return "MedicationRequest"; 432 if (code == ActivityDefinitionKind.NUTRITIONORDER) 433 return "NutritionOrder"; 434 if (code == ActivityDefinitionKind.SERVICEREQUEST) 435 return "ServiceRequest"; 436 if (code == ActivityDefinitionKind.SUPPLYREQUEST) 437 return "SupplyRequest"; 438 if (code == ActivityDefinitionKind.TASK) 439 return "Task"; 440 if (code == ActivityDefinitionKind.VISIONPRESCRIPTION) 441 return "VisionPrescription"; 442 return "?"; 443 } 444 445 public String toSystem(ActivityDefinitionKind code) { 446 return code.getSystem(); 447 } 448 } 449 450 public enum RequestIntent { 451 /** 452 * The request is a suggestion made by someone/something that does not have an 453 * intention to ensure it occurs and without providing an authorization to act. 454 */ 455 PROPOSAL, 456 /** 457 * The request represents an intention to ensure something occurs without 458 * providing an authorization for others to act. 459 */ 460 PLAN, 461 /** 462 * The request represents a legally binding instruction authored by a Patient or 463 * RelatedPerson. 464 */ 465 DIRECTIVE, 466 /** 467 * The request represents a request/demand and authorization for action by a 468 * Practitioner. 469 */ 470 ORDER, 471 /** 472 * The request represents an original authorization for action. 473 */ 474 ORIGINALORDER, 475 /** 476 * The request represents an automatically generated supplemental authorization 477 * for action based on a parent authorization together with initial results of 478 * the action taken against that parent authorization. 479 */ 480 REFLEXORDER, 481 /** 482 * The request represents the view of an authorization instantiated by a 483 * fulfilling system representing the details of the fulfiller's intention to 484 * act upon a submitted order. 485 */ 486 FILLERORDER, 487 /** 488 * An order created in fulfillment of a broader order that represents the 489 * authorization for a single activity occurrence. E.g. The administration of a 490 * single dose of a drug. 491 */ 492 INSTANCEORDER, 493 /** 494 * The request represents a component or option for a RequestGroup that 495 * establishes timing, conditionality and/or other constraints among a set of 496 * requests. Refer to [[[RequestGroup]]] for additional information on how this 497 * status is used. 498 */ 499 OPTION, 500 /** 501 * added to help the parsers with the generic types 502 */ 503 NULL; 504 505 public static RequestIntent fromCode(String codeString) throws FHIRException { 506 if (codeString == null || "".equals(codeString)) 507 return null; 508 if ("proposal".equals(codeString)) 509 return PROPOSAL; 510 if ("plan".equals(codeString)) 511 return PLAN; 512 if ("directive".equals(codeString)) 513 return DIRECTIVE; 514 if ("order".equals(codeString)) 515 return ORDER; 516 if ("original-order".equals(codeString)) 517 return ORIGINALORDER; 518 if ("reflex-order".equals(codeString)) 519 return REFLEXORDER; 520 if ("filler-order".equals(codeString)) 521 return FILLERORDER; 522 if ("instance-order".equals(codeString)) 523 return INSTANCEORDER; 524 if ("option".equals(codeString)) 525 return OPTION; 526 if (Configuration.isAcceptInvalidEnums()) 527 return null; 528 else 529 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 530 } 531 532 public String toCode() { 533 switch (this) { 534 case PROPOSAL: 535 return "proposal"; 536 case PLAN: 537 return "plan"; 538 case DIRECTIVE: 539 return "directive"; 540 case ORDER: 541 return "order"; 542 case ORIGINALORDER: 543 return "original-order"; 544 case REFLEXORDER: 545 return "reflex-order"; 546 case FILLERORDER: 547 return "filler-order"; 548 case INSTANCEORDER: 549 return "instance-order"; 550 case OPTION: 551 return "option"; 552 case NULL: 553 return null; 554 default: 555 return "?"; 556 } 557 } 558 559 public String getSystem() { 560 switch (this) { 561 case PROPOSAL: 562 return "http://hl7.org/fhir/request-intent"; 563 case PLAN: 564 return "http://hl7.org/fhir/request-intent"; 565 case DIRECTIVE: 566 return "http://hl7.org/fhir/request-intent"; 567 case ORDER: 568 return "http://hl7.org/fhir/request-intent"; 569 case ORIGINALORDER: 570 return "http://hl7.org/fhir/request-intent"; 571 case REFLEXORDER: 572 return "http://hl7.org/fhir/request-intent"; 573 case FILLERORDER: 574 return "http://hl7.org/fhir/request-intent"; 575 case INSTANCEORDER: 576 return "http://hl7.org/fhir/request-intent"; 577 case OPTION: 578 return "http://hl7.org/fhir/request-intent"; 579 case NULL: 580 return null; 581 default: 582 return "?"; 583 } 584 } 585 586 public String getDefinition() { 587 switch (this) { 588 case PROPOSAL: 589 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 590 case PLAN: 591 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 592 case DIRECTIVE: 593 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 594 case ORDER: 595 return "The request represents a request/demand and authorization for action by a Practitioner."; 596 case ORIGINALORDER: 597 return "The request represents an original authorization for action."; 598 case REFLEXORDER: 599 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 600 case FILLERORDER: 601 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 602 case INSTANCEORDER: 603 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 604 case OPTION: 605 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 606 case NULL: 607 return null; 608 default: 609 return "?"; 610 } 611 } 612 613 public String getDisplay() { 614 switch (this) { 615 case PROPOSAL: 616 return "Proposal"; 617 case PLAN: 618 return "Plan"; 619 case DIRECTIVE: 620 return "Directive"; 621 case ORDER: 622 return "Order"; 623 case ORIGINALORDER: 624 return "Original Order"; 625 case REFLEXORDER: 626 return "Reflex Order"; 627 case FILLERORDER: 628 return "Filler Order"; 629 case INSTANCEORDER: 630 return "Instance Order"; 631 case OPTION: 632 return "Option"; 633 case NULL: 634 return null; 635 default: 636 return "?"; 637 } 638 } 639 } 640 641 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 642 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 643 if (codeString == null || "".equals(codeString)) 644 if (codeString == null || "".equals(codeString)) 645 return null; 646 if ("proposal".equals(codeString)) 647 return RequestIntent.PROPOSAL; 648 if ("plan".equals(codeString)) 649 return RequestIntent.PLAN; 650 if ("directive".equals(codeString)) 651 return RequestIntent.DIRECTIVE; 652 if ("order".equals(codeString)) 653 return RequestIntent.ORDER; 654 if ("original-order".equals(codeString)) 655 return RequestIntent.ORIGINALORDER; 656 if ("reflex-order".equals(codeString)) 657 return RequestIntent.REFLEXORDER; 658 if ("filler-order".equals(codeString)) 659 return RequestIntent.FILLERORDER; 660 if ("instance-order".equals(codeString)) 661 return RequestIntent.INSTANCEORDER; 662 if ("option".equals(codeString)) 663 return RequestIntent.OPTION; 664 throw new IllegalArgumentException("Unknown RequestIntent code '" + codeString + "'"); 665 } 666 667 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 668 if (code == null) 669 return null; 670 if (code.isEmpty()) 671 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 672 String codeString = code.asStringValue(); 673 if (codeString == null || "".equals(codeString)) 674 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 675 if ("proposal".equals(codeString)) 676 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 677 if ("plan".equals(codeString)) 678 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 679 if ("directive".equals(codeString)) 680 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 681 if ("order".equals(codeString)) 682 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 683 if ("original-order".equals(codeString)) 684 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 685 if ("reflex-order".equals(codeString)) 686 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 687 if ("filler-order".equals(codeString)) 688 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 689 if ("instance-order".equals(codeString)) 690 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 691 if ("option".equals(codeString)) 692 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 693 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 694 } 695 696 public String toCode(RequestIntent code) { 697 if (code == RequestIntent.NULL) 698 return null; 699 if (code == RequestIntent.PROPOSAL) 700 return "proposal"; 701 if (code == RequestIntent.PLAN) 702 return "plan"; 703 if (code == RequestIntent.DIRECTIVE) 704 return "directive"; 705 if (code == RequestIntent.ORDER) 706 return "order"; 707 if (code == RequestIntent.ORIGINALORDER) 708 return "original-order"; 709 if (code == RequestIntent.REFLEXORDER) 710 return "reflex-order"; 711 if (code == RequestIntent.FILLERORDER) 712 return "filler-order"; 713 if (code == RequestIntent.INSTANCEORDER) 714 return "instance-order"; 715 if (code == RequestIntent.OPTION) 716 return "option"; 717 return "?"; 718 } 719 720 public String toSystem(RequestIntent code) { 721 return code.getSystem(); 722 } 723 } 724 725 public enum RequestPriority { 726 /** 727 * The request has normal priority. 728 */ 729 ROUTINE, 730 /** 731 * The request should be actioned promptly - higher priority than routine. 732 */ 733 URGENT, 734 /** 735 * The request should be actioned as soon as possible - higher priority than 736 * urgent. 737 */ 738 ASAP, 739 /** 740 * The request should be actioned immediately - highest possible priority. E.g. 741 * an emergency. 742 */ 743 STAT, 744 /** 745 * added to help the parsers with the generic types 746 */ 747 NULL; 748 749 public static RequestPriority fromCode(String codeString) throws FHIRException { 750 if (codeString == null || "".equals(codeString)) 751 return null; 752 if ("routine".equals(codeString)) 753 return ROUTINE; 754 if ("urgent".equals(codeString)) 755 return URGENT; 756 if ("asap".equals(codeString)) 757 return ASAP; 758 if ("stat".equals(codeString)) 759 return STAT; 760 if (Configuration.isAcceptInvalidEnums()) 761 return null; 762 else 763 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 764 } 765 766 public String toCode() { 767 switch (this) { 768 case ROUTINE: 769 return "routine"; 770 case URGENT: 771 return "urgent"; 772 case ASAP: 773 return "asap"; 774 case STAT: 775 return "stat"; 776 case NULL: 777 return null; 778 default: 779 return "?"; 780 } 781 } 782 783 public String getSystem() { 784 switch (this) { 785 case ROUTINE: 786 return "http://hl7.org/fhir/request-priority"; 787 case URGENT: 788 return "http://hl7.org/fhir/request-priority"; 789 case ASAP: 790 return "http://hl7.org/fhir/request-priority"; 791 case STAT: 792 return "http://hl7.org/fhir/request-priority"; 793 case NULL: 794 return null; 795 default: 796 return "?"; 797 } 798 } 799 800 public String getDefinition() { 801 switch (this) { 802 case ROUTINE: 803 return "The request has normal priority."; 804 case URGENT: 805 return "The request should be actioned promptly - higher priority than routine."; 806 case ASAP: 807 return "The request should be actioned as soon as possible - higher priority than urgent."; 808 case STAT: 809 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 810 case NULL: 811 return null; 812 default: 813 return "?"; 814 } 815 } 816 817 public String getDisplay() { 818 switch (this) { 819 case ROUTINE: 820 return "Routine"; 821 case URGENT: 822 return "Urgent"; 823 case ASAP: 824 return "ASAP"; 825 case STAT: 826 return "STAT"; 827 case NULL: 828 return null; 829 default: 830 return "?"; 831 } 832 } 833 } 834 835 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 836 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 837 if (codeString == null || "".equals(codeString)) 838 if (codeString == null || "".equals(codeString)) 839 return null; 840 if ("routine".equals(codeString)) 841 return RequestPriority.ROUTINE; 842 if ("urgent".equals(codeString)) 843 return RequestPriority.URGENT; 844 if ("asap".equals(codeString)) 845 return RequestPriority.ASAP; 846 if ("stat".equals(codeString)) 847 return RequestPriority.STAT; 848 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 849 } 850 851 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 852 if (code == null) 853 return null; 854 if (code.isEmpty()) 855 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 856 String codeString = code.asStringValue(); 857 if (codeString == null || "".equals(codeString)) 858 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 859 if ("routine".equals(codeString)) 860 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 861 if ("urgent".equals(codeString)) 862 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 863 if ("asap".equals(codeString)) 864 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 865 if ("stat".equals(codeString)) 866 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 867 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 868 } 869 870 public String toCode(RequestPriority code) { 871 if (code == RequestPriority.NULL) 872 return null; 873 if (code == RequestPriority.ROUTINE) 874 return "routine"; 875 if (code == RequestPriority.URGENT) 876 return "urgent"; 877 if (code == RequestPriority.ASAP) 878 return "asap"; 879 if (code == RequestPriority.STAT) 880 return "stat"; 881 return "?"; 882 } 883 884 public String toSystem(RequestPriority code) { 885 return code.getSystem(); 886 } 887 } 888 889 public enum ActivityParticipantType { 890 /** 891 * The participant is the patient under evaluation. 892 */ 893 PATIENT, 894 /** 895 * The participant is a practitioner involved in the patient's care. 896 */ 897 PRACTITIONER, 898 /** 899 * The participant is a person related to the patient. 900 */ 901 RELATEDPERSON, 902 /** 903 * The participant is a system or device used in the care of the patient. 904 */ 905 DEVICE, 906 /** 907 * added to help the parsers with the generic types 908 */ 909 NULL; 910 911 public static ActivityParticipantType fromCode(String codeString) throws FHIRException { 912 if (codeString == null || "".equals(codeString)) 913 return null; 914 if ("patient".equals(codeString)) 915 return PATIENT; 916 if ("practitioner".equals(codeString)) 917 return PRACTITIONER; 918 if ("related-person".equals(codeString)) 919 return RELATEDPERSON; 920 if ("device".equals(codeString)) 921 return DEVICE; 922 if (Configuration.isAcceptInvalidEnums()) 923 return null; 924 else 925 throw new FHIRException("Unknown ActivityParticipantType code '" + codeString + "'"); 926 } 927 928 public String toCode() { 929 switch (this) { 930 case PATIENT: 931 return "patient"; 932 case PRACTITIONER: 933 return "practitioner"; 934 case RELATEDPERSON: 935 return "related-person"; 936 case DEVICE: 937 return "device"; 938 case NULL: 939 return null; 940 default: 941 return "?"; 942 } 943 } 944 945 public String getSystem() { 946 switch (this) { 947 case PATIENT: 948 return "http://hl7.org/fhir/action-participant-type"; 949 case PRACTITIONER: 950 return "http://hl7.org/fhir/action-participant-type"; 951 case RELATEDPERSON: 952 return "http://hl7.org/fhir/action-participant-type"; 953 case DEVICE: 954 return "http://hl7.org/fhir/action-participant-type"; 955 case NULL: 956 return null; 957 default: 958 return "?"; 959 } 960 } 961 962 public String getDefinition() { 963 switch (this) { 964 case PATIENT: 965 return "The participant is the patient under evaluation."; 966 case PRACTITIONER: 967 return "The participant is a practitioner involved in the patient's care."; 968 case RELATEDPERSON: 969 return "The participant is a person related to the patient."; 970 case DEVICE: 971 return "The participant is a system or device used in the care of the patient."; 972 case NULL: 973 return null; 974 default: 975 return "?"; 976 } 977 } 978 979 public String getDisplay() { 980 switch (this) { 981 case PATIENT: 982 return "Patient"; 983 case PRACTITIONER: 984 return "Practitioner"; 985 case RELATEDPERSON: 986 return "Related Person"; 987 case DEVICE: 988 return "Device"; 989 case NULL: 990 return null; 991 default: 992 return "?"; 993 } 994 } 995 } 996 997 public static class ActivityParticipantTypeEnumFactory implements EnumFactory<ActivityParticipantType> { 998 public ActivityParticipantType fromCode(String codeString) throws IllegalArgumentException { 999 if (codeString == null || "".equals(codeString)) 1000 if (codeString == null || "".equals(codeString)) 1001 return null; 1002 if ("patient".equals(codeString)) 1003 return ActivityParticipantType.PATIENT; 1004 if ("practitioner".equals(codeString)) 1005 return ActivityParticipantType.PRACTITIONER; 1006 if ("related-person".equals(codeString)) 1007 return ActivityParticipantType.RELATEDPERSON; 1008 if ("device".equals(codeString)) 1009 return ActivityParticipantType.DEVICE; 1010 throw new IllegalArgumentException("Unknown ActivityParticipantType code '" + codeString + "'"); 1011 } 1012 1013 public Enumeration<ActivityParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 1014 if (code == null) 1015 return null; 1016 if (code.isEmpty()) 1017 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.NULL, code); 1018 String codeString = code.asStringValue(); 1019 if (codeString == null || "".equals(codeString)) 1020 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.NULL, code); 1021 if ("patient".equals(codeString)) 1022 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.PATIENT, code); 1023 if ("practitioner".equals(codeString)) 1024 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.PRACTITIONER, code); 1025 if ("related-person".equals(codeString)) 1026 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.RELATEDPERSON, code); 1027 if ("device".equals(codeString)) 1028 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.DEVICE, code); 1029 throw new FHIRException("Unknown ActivityParticipantType code '" + codeString + "'"); 1030 } 1031 1032 public String toCode(ActivityParticipantType code) { 1033 if (code == ActivityParticipantType.NULL) 1034 return null; 1035 if (code == ActivityParticipantType.PATIENT) 1036 return "patient"; 1037 if (code == ActivityParticipantType.PRACTITIONER) 1038 return "practitioner"; 1039 if (code == ActivityParticipantType.RELATEDPERSON) 1040 return "related-person"; 1041 if (code == ActivityParticipantType.DEVICE) 1042 return "device"; 1043 return "?"; 1044 } 1045 1046 public String toSystem(ActivityParticipantType code) { 1047 return code.getSystem(); 1048 } 1049 } 1050 1051 @Block() 1052 public static class ActivityDefinitionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 1053 /** 1054 * The type of participant in the action. 1055 */ 1056 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1057 @Description(shortDefinition = "patient | practitioner | related-person | device", formalDefinition = "The type of participant in the action.") 1058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-type") 1059 protected Enumeration<ActivityParticipantType> type; 1060 1061 /** 1062 * The role the participant should play in performing the described action. 1063 */ 1064 @Child(name = "role", type = { 1065 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1066 @Description(shortDefinition = "E.g. Nurse, Surgeon, Parent, etc.", formalDefinition = "The role the participant should play in performing the described action.") 1067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-role") 1068 protected CodeableConcept role; 1069 1070 private static final long serialVersionUID = -1450932564L; 1071 1072 /** 1073 * Constructor 1074 */ 1075 public ActivityDefinitionParticipantComponent() { 1076 super(); 1077 } 1078 1079 /** 1080 * Constructor 1081 */ 1082 public ActivityDefinitionParticipantComponent(Enumeration<ActivityParticipantType> type) { 1083 super(); 1084 this.type = type; 1085 } 1086 1087 /** 1088 * @return {@link #type} (The type of participant in the action.). This is the 1089 * underlying object with id, value and extensions. The accessor 1090 * "getType" gives direct access to the value 1091 */ 1092 public Enumeration<ActivityParticipantType> getTypeElement() { 1093 if (this.type == null) 1094 if (Configuration.errorOnAutoCreate()) 1095 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.type"); 1096 else if (Configuration.doAutoCreate()) 1097 this.type = new Enumeration<ActivityParticipantType>(new ActivityParticipantTypeEnumFactory()); // bb 1098 return this.type; 1099 } 1100 1101 public boolean hasTypeElement() { 1102 return this.type != null && !this.type.isEmpty(); 1103 } 1104 1105 public boolean hasType() { 1106 return this.type != null && !this.type.isEmpty(); 1107 } 1108 1109 /** 1110 * @param value {@link #type} (The type of participant in the action.). This is 1111 * the underlying object with id, value and extensions. The 1112 * accessor "getType" gives direct access to the value 1113 */ 1114 public ActivityDefinitionParticipantComponent setTypeElement(Enumeration<ActivityParticipantType> value) { 1115 this.type = value; 1116 return this; 1117 } 1118 1119 /** 1120 * @return The type of participant in the action. 1121 */ 1122 public ActivityParticipantType getType() { 1123 return this.type == null ? null : this.type.getValue(); 1124 } 1125 1126 /** 1127 * @param value The type of participant in the action. 1128 */ 1129 public ActivityDefinitionParticipantComponent setType(ActivityParticipantType value) { 1130 if (this.type == null) 1131 this.type = new Enumeration<ActivityParticipantType>(new ActivityParticipantTypeEnumFactory()); 1132 this.type.setValue(value); 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #role} (The role the participant should play in performing the 1138 * described action.) 1139 */ 1140 public CodeableConcept getRole() { 1141 if (this.role == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.role"); 1144 else if (Configuration.doAutoCreate()) 1145 this.role = new CodeableConcept(); // cc 1146 return this.role; 1147 } 1148 1149 public boolean hasRole() { 1150 return this.role != null && !this.role.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #role} (The role the participant should play in 1155 * performing the described action.) 1156 */ 1157 public ActivityDefinitionParticipantComponent setRole(CodeableConcept value) { 1158 this.role = value; 1159 return this; 1160 } 1161 1162 protected void listChildren(List<Property> children) { 1163 super.listChildren(children); 1164 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 1165 children.add(new Property("role", "CodeableConcept", 1166 "The role the participant should play in performing the described action.", 0, 1, role)); 1167 } 1168 1169 @Override 1170 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1171 switch (_hash) { 1172 case 3575610: 1173 /* type */ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 1174 case 3506294: 1175 /* role */ return new Property("role", "CodeableConcept", 1176 "The role the participant should play in performing the described action.", 0, 1, role); 1177 default: 1178 return super.getNamedProperty(_hash, _name, _checkValid); 1179 } 1180 1181 } 1182 1183 @Override 1184 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1185 switch (hash) { 1186 case 3575610: 1187 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ActivityParticipantType> 1188 case 3506294: 1189 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 1190 default: 1191 return super.getProperty(hash, name, checkValid); 1192 } 1193 1194 } 1195 1196 @Override 1197 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1198 switch (hash) { 1199 case 3575610: // type 1200 value = new ActivityParticipantTypeEnumFactory().fromType(castToCode(value)); 1201 this.type = (Enumeration) value; // Enumeration<ActivityParticipantType> 1202 return value; 1203 case 3506294: // role 1204 this.role = castToCodeableConcept(value); // CodeableConcept 1205 return value; 1206 default: 1207 return super.setProperty(hash, name, value); 1208 } 1209 1210 } 1211 1212 @Override 1213 public Base setProperty(String name, Base value) throws FHIRException { 1214 if (name.equals("type")) { 1215 value = new ActivityParticipantTypeEnumFactory().fromType(castToCode(value)); 1216 this.type = (Enumeration) value; // Enumeration<ActivityParticipantType> 1217 } else if (name.equals("role")) { 1218 this.role = castToCodeableConcept(value); // CodeableConcept 1219 } else 1220 return super.setProperty(name, value); 1221 return value; 1222 } 1223 1224 @Override 1225 public void removeChild(String name, Base value) throws FHIRException { 1226 if (name.equals("type")) { 1227 this.type = null; 1228 } else if (name.equals("role")) { 1229 this.role = null; 1230 } else 1231 super.removeChild(name, value); 1232 1233 } 1234 1235 @Override 1236 public Base makeProperty(int hash, String name) throws FHIRException { 1237 switch (hash) { 1238 case 3575610: 1239 return getTypeElement(); 1240 case 3506294: 1241 return getRole(); 1242 default: 1243 return super.makeProperty(hash, name); 1244 } 1245 1246 } 1247 1248 @Override 1249 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1250 switch (hash) { 1251 case 3575610: 1252 /* type */ return new String[] { "code" }; 1253 case 3506294: 1254 /* role */ return new String[] { "CodeableConcept" }; 1255 default: 1256 return super.getTypesForProperty(hash, name); 1257 } 1258 1259 } 1260 1261 @Override 1262 public Base addChild(String name) throws FHIRException { 1263 if (name.equals("type")) { 1264 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.type"); 1265 } else if (name.equals("role")) { 1266 this.role = new CodeableConcept(); 1267 return this.role; 1268 } else 1269 return super.addChild(name); 1270 } 1271 1272 public ActivityDefinitionParticipantComponent copy() { 1273 ActivityDefinitionParticipantComponent dst = new ActivityDefinitionParticipantComponent(); 1274 copyValues(dst); 1275 return dst; 1276 } 1277 1278 public void copyValues(ActivityDefinitionParticipantComponent dst) { 1279 super.copyValues(dst); 1280 dst.type = type == null ? null : type.copy(); 1281 dst.role = role == null ? null : role.copy(); 1282 } 1283 1284 @Override 1285 public boolean equalsDeep(Base other_) { 1286 if (!super.equalsDeep(other_)) 1287 return false; 1288 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 1289 return false; 1290 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 1291 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true); 1292 } 1293 1294 @Override 1295 public boolean equalsShallow(Base other_) { 1296 if (!super.equalsShallow(other_)) 1297 return false; 1298 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 1299 return false; 1300 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 1301 return compareValues(type, o.type, true); 1302 } 1303 1304 public boolean isEmpty() { 1305 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role); 1306 } 1307 1308 public String fhirType() { 1309 return "ActivityDefinition.participant"; 1310 1311 } 1312 1313 } 1314 1315 @Block() 1316 public static class ActivityDefinitionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 1317 /** 1318 * The path to the element to be customized. This is the path on the resource 1319 * that will hold the result of the calculation defined by the expression. The 1320 * specified path SHALL be a FHIRPath resolveable on the specified target type 1321 * of the ActivityDefinition, and SHALL consist only of identifiers, constant 1322 * indexers, and a restricted subset of functions. The path is allowed to 1323 * contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 1324 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 1325 * Profile](fhirpath.html#simple) for full details). 1326 */ 1327 @Child(name = "path", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1328 @Description(shortDefinition = "The path to the element to be set dynamically", formalDefinition = "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).") 1329 protected StringType path; 1330 1331 /** 1332 * An expression specifying the value of the customized element. 1333 */ 1334 @Child(name = "expression", type = { 1335 Expression.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1336 @Description(shortDefinition = "An expression that provides the dynamic value for the customization", formalDefinition = "An expression specifying the value of the customized element.") 1337 protected Expression expression; 1338 1339 private static final long serialVersionUID = 1064529082L; 1340 1341 /** 1342 * Constructor 1343 */ 1344 public ActivityDefinitionDynamicValueComponent() { 1345 super(); 1346 } 1347 1348 /** 1349 * Constructor 1350 */ 1351 public ActivityDefinitionDynamicValueComponent(StringType path, Expression expression) { 1352 super(); 1353 this.path = path; 1354 this.expression = expression; 1355 } 1356 1357 /** 1358 * @return {@link #path} (The path to the element to be customized. This is the 1359 * path on the resource that will hold the result of the calculation 1360 * defined by the expression. The specified path SHALL be a FHIRPath 1361 * resolveable on the specified target type of the ActivityDefinition, 1362 * and SHALL consist only of identifiers, constant indexers, and a 1363 * restricted subset of functions. The path is allowed to contain 1364 * qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 1365 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 1366 * Profile](fhirpath.html#simple) for full details).). This is the 1367 * underlying object with id, value and extensions. The accessor 1368 * "getPath" gives direct access to the value 1369 */ 1370 public StringType getPathElement() { 1371 if (this.path == null) 1372 if (Configuration.errorOnAutoCreate()) 1373 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.path"); 1374 else if (Configuration.doAutoCreate()) 1375 this.path = new StringType(); // bb 1376 return this.path; 1377 } 1378 1379 public boolean hasPathElement() { 1380 return this.path != null && !this.path.isEmpty(); 1381 } 1382 1383 public boolean hasPath() { 1384 return this.path != null && !this.path.isEmpty(); 1385 } 1386 1387 /** 1388 * @param value {@link #path} (The path to the element to be customized. This is 1389 * the path on the resource that will hold the result of the 1390 * calculation defined by the expression. The specified path SHALL 1391 * be a FHIRPath resolveable on the specified target type of the 1392 * ActivityDefinition, and SHALL consist only of identifiers, 1393 * constant indexers, and a restricted subset of functions. The 1394 * path is allowed to contain qualifiers (.) to traverse 1395 * sub-elements, as well as indexers ([x]) to traverse 1396 * multiple-cardinality sub-elements (see the [Simple FHIRPath 1397 * Profile](fhirpath.html#simple) for full details).). This is the 1398 * underlying object with id, value and extensions. The accessor 1399 * "getPath" gives direct access to the value 1400 */ 1401 public ActivityDefinitionDynamicValueComponent setPathElement(StringType value) { 1402 this.path = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return The path to the element to be customized. This is the path on the 1408 * resource that will hold the result of the calculation defined by the 1409 * expression. The specified path SHALL be a FHIRPath resolveable on the 1410 * specified target type of the ActivityDefinition, and SHALL consist 1411 * only of identifiers, constant indexers, and a restricted subset of 1412 * functions. The path is allowed to contain qualifiers (.) to traverse 1413 * sub-elements, as well as indexers ([x]) to traverse 1414 * multiple-cardinality sub-elements (see the [Simple FHIRPath 1415 * Profile](fhirpath.html#simple) for full details). 1416 */ 1417 public String getPath() { 1418 return this.path == null ? null : this.path.getValue(); 1419 } 1420 1421 /** 1422 * @param value The path to the element to be customized. This is the path on 1423 * the resource that will hold the result of the calculation 1424 * defined by the expression. The specified path SHALL be a 1425 * FHIRPath resolveable on the specified target type of the 1426 * ActivityDefinition, and SHALL consist only of identifiers, 1427 * constant indexers, and a restricted subset of functions. The 1428 * path is allowed to contain qualifiers (.) to traverse 1429 * sub-elements, as well as indexers ([x]) to traverse 1430 * multiple-cardinality sub-elements (see the [Simple FHIRPath 1431 * Profile](fhirpath.html#simple) for full details). 1432 */ 1433 public ActivityDefinitionDynamicValueComponent setPath(String value) { 1434 if (this.path == null) 1435 this.path = new StringType(); 1436 this.path.setValue(value); 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #expression} (An expression specifying the value of the 1442 * customized element.) 1443 */ 1444 public Expression getExpression() { 1445 if (this.expression == null) 1446 if (Configuration.errorOnAutoCreate()) 1447 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.expression"); 1448 else if (Configuration.doAutoCreate()) 1449 this.expression = new Expression(); // cc 1450 return this.expression; 1451 } 1452 1453 public boolean hasExpression() { 1454 return this.expression != null && !this.expression.isEmpty(); 1455 } 1456 1457 /** 1458 * @param value {@link #expression} (An expression specifying the value of the 1459 * customized element.) 1460 */ 1461 public ActivityDefinitionDynamicValueComponent setExpression(Expression value) { 1462 this.expression = value; 1463 return this; 1464 } 1465 1466 protected void listChildren(List<Property> children) { 1467 super.listChildren(children); 1468 children.add(new Property("path", "string", 1469 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 1470 0, 1, path)); 1471 children.add(new Property("expression", "Expression", 1472 "An expression specifying the value of the customized element.", 0, 1, expression)); 1473 } 1474 1475 @Override 1476 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1477 switch (_hash) { 1478 case 3433509: 1479 /* path */ return new Property("path", "string", 1480 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 1481 0, 1, path); 1482 case -1795452264: 1483 /* expression */ return new Property("expression", "Expression", 1484 "An expression specifying the value of the customized element.", 0, 1, expression); 1485 default: 1486 return super.getNamedProperty(_hash, _name, _checkValid); 1487 } 1488 1489 } 1490 1491 @Override 1492 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1493 switch (hash) { 1494 case 3433509: 1495 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1496 case -1795452264: 1497 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 1498 default: 1499 return super.getProperty(hash, name, checkValid); 1500 } 1501 1502 } 1503 1504 @Override 1505 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1506 switch (hash) { 1507 case 3433509: // path 1508 this.path = castToString(value); // StringType 1509 return value; 1510 case -1795452264: // expression 1511 this.expression = castToExpression(value); // Expression 1512 return value; 1513 default: 1514 return super.setProperty(hash, name, value); 1515 } 1516 1517 } 1518 1519 @Override 1520 public Base setProperty(String name, Base value) throws FHIRException { 1521 if (name.equals("path")) { 1522 this.path = castToString(value); // StringType 1523 } else if (name.equals("expression")) { 1524 this.expression = castToExpression(value); // Expression 1525 } else 1526 return super.setProperty(name, value); 1527 return value; 1528 } 1529 1530 @Override 1531 public void removeChild(String name, Base value) throws FHIRException { 1532 if (name.equals("path")) { 1533 this.path = null; 1534 } else if (name.equals("expression")) { 1535 this.expression = null; 1536 } else 1537 super.removeChild(name, value); 1538 1539 } 1540 1541 @Override 1542 public Base makeProperty(int hash, String name) throws FHIRException { 1543 switch (hash) { 1544 case 3433509: 1545 return getPathElement(); 1546 case -1795452264: 1547 return getExpression(); 1548 default: 1549 return super.makeProperty(hash, name); 1550 } 1551 1552 } 1553 1554 @Override 1555 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1556 switch (hash) { 1557 case 3433509: 1558 /* path */ return new String[] { "string" }; 1559 case -1795452264: 1560 /* expression */ return new String[] { "Expression" }; 1561 default: 1562 return super.getTypesForProperty(hash, name); 1563 } 1564 1565 } 1566 1567 @Override 1568 public Base addChild(String name) throws FHIRException { 1569 if (name.equals("path")) { 1570 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.path"); 1571 } else if (name.equals("expression")) { 1572 this.expression = new Expression(); 1573 return this.expression; 1574 } else 1575 return super.addChild(name); 1576 } 1577 1578 public ActivityDefinitionDynamicValueComponent copy() { 1579 ActivityDefinitionDynamicValueComponent dst = new ActivityDefinitionDynamicValueComponent(); 1580 copyValues(dst); 1581 return dst; 1582 } 1583 1584 public void copyValues(ActivityDefinitionDynamicValueComponent dst) { 1585 super.copyValues(dst); 1586 dst.path = path == null ? null : path.copy(); 1587 dst.expression = expression == null ? null : expression.copy(); 1588 } 1589 1590 @Override 1591 public boolean equalsDeep(Base other_) { 1592 if (!super.equalsDeep(other_)) 1593 return false; 1594 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 1595 return false; 1596 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 1597 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 1598 } 1599 1600 @Override 1601 public boolean equalsShallow(Base other_) { 1602 if (!super.equalsShallow(other_)) 1603 return false; 1604 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 1605 return false; 1606 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 1607 return compareValues(path, o.path, true); 1608 } 1609 1610 public boolean isEmpty() { 1611 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 1612 } 1613 1614 public String fhirType() { 1615 return "ActivityDefinition.dynamicValue"; 1616 1617 } 1618 1619 } 1620 1621 /** 1622 * A formal identifier that is used to identify this activity definition when it 1623 * is represented in other formats, or referenced in a specification, model, 1624 * design or an instance. 1625 */ 1626 @Child(name = "identifier", type = { 1627 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1628 @Description(shortDefinition = "Additional identifier for the activity definition", formalDefinition = "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1629 protected List<Identifier> identifier; 1630 1631 /** 1632 * An explanatory or alternate title for the activity definition giving 1633 * additional information about its content. 1634 */ 1635 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1636 @Description(shortDefinition = "Subordinate title of the activity definition", formalDefinition = "An explanatory or alternate title for the activity definition giving additional information about its content.") 1637 protected StringType subtitle; 1638 1639 /** 1640 * A code or group definition that describes the intended subject of the 1641 * activity being defined. 1642 */ 1643 @Child(name = "subject", type = { CodeableConcept.class, 1644 Group.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1645 @Description(shortDefinition = "Type of individual the activity definition is intended for", formalDefinition = "A code or group definition that describes the intended subject of the activity being defined.") 1646 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 1647 protected Type subject; 1648 1649 /** 1650 * Explanation of why this activity definition is needed and why it has been 1651 * designed as it has. 1652 */ 1653 @Child(name = "purpose", type = { 1654 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1655 @Description(shortDefinition = "Why this activity definition is defined", formalDefinition = "Explanation of why this activity definition is needed and why it has been designed as it has.") 1656 protected MarkdownType purpose; 1657 1658 /** 1659 * A detailed description of how the activity definition is used from a clinical 1660 * perspective. 1661 */ 1662 @Child(name = "usage", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1663 @Description(shortDefinition = "Describes the clinical usage of the activity definition", formalDefinition = "A detailed description of how the activity definition is used from a clinical perspective.") 1664 protected StringType usage; 1665 1666 /** 1667 * A copyright statement relating to the activity definition and/or its 1668 * contents. Copyright statements are generally legal restrictions on the use 1669 * and publishing of the activity definition. 1670 */ 1671 @Child(name = "copyright", type = { 1672 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1673 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.") 1674 protected MarkdownType copyright; 1675 1676 /** 1677 * The date on which the resource content was approved by the publisher. 1678 * Approval happens once when the content is officially approved for usage. 1679 */ 1680 @Child(name = "approvalDate", type = { 1681 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1682 @Description(shortDefinition = "When the activity definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1683 protected DateType approvalDate; 1684 1685 /** 1686 * The date on which the resource content was last reviewed. Review happens 1687 * periodically after approval but does not change the original approval date. 1688 */ 1689 @Child(name = "lastReviewDate", type = { 1690 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1691 @Description(shortDefinition = "When the activity definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1692 protected DateType lastReviewDate; 1693 1694 /** 1695 * The period during which the activity definition content was or is planned to 1696 * be in active use. 1697 */ 1698 @Child(name = "effectivePeriod", type = { 1699 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1700 @Description(shortDefinition = "When the activity definition is expected to be used", formalDefinition = "The period during which the activity definition content was or is planned to be in active use.") 1701 protected Period effectivePeriod; 1702 1703 /** 1704 * Descriptive topics related to the content of the activity. Topics provide a 1705 * high-level categorization of the activity that can be useful for filtering 1706 * and searching. 1707 */ 1708 @Child(name = "topic", type = { 1709 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1710 @Description(shortDefinition = "E.g. Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.") 1711 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 1712 protected List<CodeableConcept> topic; 1713 1714 /** 1715 * An individiual or organization primarily involved in the creation and 1716 * maintenance of the content. 1717 */ 1718 @Child(name = "author", type = { 1719 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1720 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 1721 protected List<ContactDetail> author; 1722 1723 /** 1724 * An individual or organization primarily responsible for internal coherence of 1725 * the content. 1726 */ 1727 @Child(name = "editor", type = { 1728 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1729 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 1730 protected List<ContactDetail> editor; 1731 1732 /** 1733 * An individual or organization primarily responsible for review of some aspect 1734 * of the content. 1735 */ 1736 @Child(name = "reviewer", type = { 1737 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1738 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 1739 protected List<ContactDetail> reviewer; 1740 1741 /** 1742 * An individual or organization responsible for officially endorsing the 1743 * content for use in some setting. 1744 */ 1745 @Child(name = "endorser", type = { 1746 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1747 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 1748 protected List<ContactDetail> endorser; 1749 1750 /** 1751 * Related artifacts such as additional documentation, justification, or 1752 * bibliographic references. 1753 */ 1754 @Child(name = "relatedArtifact", type = { 1755 RelatedArtifact.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1756 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 1757 protected List<RelatedArtifact> relatedArtifact; 1758 1759 /** 1760 * A reference to a Library resource containing any formal logic used by the 1761 * activity definition. 1762 */ 1763 @Child(name = "library", type = { 1764 CanonicalType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1765 @Description(shortDefinition = "Logic used by the activity definition", formalDefinition = "A reference to a Library resource containing any formal logic used by the activity definition.") 1766 protected List<CanonicalType> library; 1767 1768 /** 1769 * A description of the kind of resource the activity definition is 1770 * representing. For example, a MedicationRequest, a ServiceRequest, or a 1771 * CommunicationRequest. Typically, but not always, this is a Request resource. 1772 */ 1773 @Child(name = "kind", type = { CodeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 1774 @Description(shortDefinition = "Kind of resource", formalDefinition = "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.") 1775 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-resource-types") 1776 protected Enumeration<ActivityDefinitionKind> kind; 1777 1778 /** 1779 * A profile to which the target of the activity definition is expected to 1780 * conform. 1781 */ 1782 @Child(name = "profile", type = { 1783 CanonicalType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1784 @Description(shortDefinition = "What profile the resource needs to conform to", formalDefinition = "A profile to which the target of the activity definition is expected to conform.") 1785 protected CanonicalType profile; 1786 1787 /** 1788 * Detailed description of the type of activity; e.g. What lab test, what 1789 * procedure, what kind of encounter. 1790 */ 1791 @Child(name = "code", type = { 1792 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 1793 @Description(shortDefinition = "Detail type of activity", formalDefinition = "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.") 1794 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 1795 protected CodeableConcept code; 1796 1797 /** 1798 * Indicates the level of authority/intentionality associated with the activity 1799 * and where the request should fit into the workflow chain. 1800 */ 1801 @Child(name = "intent", type = { CodeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 1802 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.") 1803 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 1804 protected Enumeration<RequestIntent> intent; 1805 1806 /** 1807 * Indicates how quickly the activity should be addressed with respect to other 1808 * requests. 1809 */ 1810 @Child(name = "priority", type = { CodeType.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 1811 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the activity should be addressed with respect to other requests.") 1812 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 1813 protected Enumeration<RequestPriority> priority; 1814 1815 /** 1816 * Set this to true if the definition is to indicate that a particular activity 1817 * should NOT be performed. If true, this element should be interpreted to 1818 * reinforce a negative coding. For example NPO as a code with a doNotPerform of 1819 * true would still indicate to NOT perform the action. 1820 */ 1821 @Child(name = "doNotPerform", type = { 1822 BooleanType.class }, order = 21, min = 0, max = 1, modifier = true, summary = true) 1823 @Description(shortDefinition = "True if the activity should not be performed", formalDefinition = "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.") 1824 protected BooleanType doNotPerform; 1825 1826 /** 1827 * The period, timing or frequency upon which the described activity is to 1828 * occur. 1829 */ 1830 @Child(name = "timing", type = { Timing.class, DateTimeType.class, Age.class, Period.class, Range.class, 1831 Duration.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 1832 @Description(shortDefinition = "When activity is to occur", formalDefinition = "The period, timing or frequency upon which the described activity is to occur.") 1833 protected Type timing; 1834 1835 /** 1836 * Identifies the facility where the activity will occur; e.g. home, hospital, 1837 * specific clinic, etc. 1838 */ 1839 @Child(name = "location", type = { Location.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 1840 @Description(shortDefinition = "Where it should happen", formalDefinition = "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.") 1841 protected Reference location; 1842 1843 /** 1844 * The actual object that is the target of the reference (Identifies the 1845 * facility where the activity will occur; e.g. home, hospital, specific clinic, 1846 * etc.) 1847 */ 1848 protected Location locationTarget; 1849 1850 /** 1851 * Indicates who should participate in performing the action described. 1852 */ 1853 @Child(name = "participant", type = {}, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1854 @Description(shortDefinition = "Who should participate in the action", formalDefinition = "Indicates who should participate in performing the action described.") 1855 protected List<ActivityDefinitionParticipantComponent> participant; 1856 1857 /** 1858 * Identifies the food, drug or other product being consumed or supplied in the 1859 * activity. 1860 */ 1861 @Child(name = "product", type = { Medication.class, Substance.class, 1862 CodeableConcept.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 1863 @Description(shortDefinition = "What's administered/supplied", formalDefinition = "Identifies the food, drug or other product being consumed or supplied in the activity.") 1864 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1865 protected Type product; 1866 1867 /** 1868 * Identifies the quantity expected to be consumed at once (per dose, per meal, 1869 * etc.). 1870 */ 1871 @Child(name = "quantity", type = { Quantity.class }, order = 26, min = 0, max = 1, modifier = false, summary = false) 1872 @Description(shortDefinition = "How much is administered/consumed/supplied", formalDefinition = "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).") 1873 protected Quantity quantity; 1874 1875 /** 1876 * Provides detailed dosage instructions in the same way that they are described 1877 * for MedicationRequest resources. 1878 */ 1879 @Child(name = "dosage", type = { 1880 Dosage.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1881 @Description(shortDefinition = "Detailed dosage instructions", formalDefinition = "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.") 1882 protected List<Dosage> dosage; 1883 1884 /** 1885 * Indicates the sites on the subject's body where the procedure should be 1886 * performed (I.e. the target sites). 1887 */ 1888 @Child(name = "bodySite", type = { 1889 CodeableConcept.class }, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1890 @Description(shortDefinition = "What part of body to perform on", formalDefinition = "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).") 1891 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 1892 protected List<CodeableConcept> bodySite; 1893 1894 /** 1895 * Defines specimen requirements for the action to be performed, such as 1896 * required specimens for a lab test. 1897 */ 1898 @Child(name = "specimenRequirement", type = { 1899 SpecimenDefinition.class }, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1900 @Description(shortDefinition = "What specimens are required to perform this action", formalDefinition = "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.") 1901 protected List<Reference> specimenRequirement; 1902 /** 1903 * The actual objects that are the target of the reference (Defines specimen 1904 * requirements for the action to be performed, such as required specimens for a 1905 * lab test.) 1906 */ 1907 protected List<SpecimenDefinition> specimenRequirementTarget; 1908 1909 /** 1910 * Defines observation requirements for the action to be performed, such as body 1911 * weight or surface area. 1912 */ 1913 @Child(name = "observationRequirement", type = { 1914 ObservationDefinition.class }, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1915 @Description(shortDefinition = "What observations are required to perform this action", formalDefinition = "Defines observation requirements for the action to be performed, such as body weight or surface area.") 1916 protected List<Reference> observationRequirement; 1917 /** 1918 * The actual objects that are the target of the reference (Defines observation 1919 * requirements for the action to be performed, such as body weight or surface 1920 * area.) 1921 */ 1922 protected List<ObservationDefinition> observationRequirementTarget; 1923 1924 /** 1925 * Defines the observations that are expected to be produced by the action. 1926 */ 1927 @Child(name = "observationResultRequirement", type = { 1928 ObservationDefinition.class }, order = 31, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1929 @Description(shortDefinition = "What observations must be produced by this action", formalDefinition = "Defines the observations that are expected to be produced by the action.") 1930 protected List<Reference> observationResultRequirement; 1931 /** 1932 * The actual objects that are the target of the reference (Defines the 1933 * observations that are expected to be produced by the action.) 1934 */ 1935 protected List<ObservationDefinition> observationResultRequirementTarget; 1936 1937 /** 1938 * A reference to a StructureMap resource that defines a transform that can be 1939 * executed to produce the intent resource using the ActivityDefinition instance 1940 * as the input. 1941 */ 1942 @Child(name = "transform", type = { 1943 CanonicalType.class }, order = 32, min = 0, max = 1, modifier = false, summary = false) 1944 @Description(shortDefinition = "Transform to apply the template", formalDefinition = "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.") 1945 protected CanonicalType transform; 1946 1947 /** 1948 * Dynamic values that will be evaluated to produce values for elements of the 1949 * resulting resource. For example, if the dosage of a medication must be 1950 * computed based on the patient's weight, a dynamic value would be used to 1951 * specify an expression that calculated the weight, and the path on the request 1952 * resource that would contain the result. 1953 */ 1954 @Child(name = "dynamicValue", type = {}, order = 33, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1955 @Description(shortDefinition = "Dynamic aspects of the definition", formalDefinition = "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.") 1956 protected List<ActivityDefinitionDynamicValueComponent> dynamicValue; 1957 1958 private static final long serialVersionUID = 1488459022L; 1959 1960 /** 1961 * Constructor 1962 */ 1963 public ActivityDefinition() { 1964 super(); 1965 } 1966 1967 /** 1968 * Constructor 1969 */ 1970 public ActivityDefinition(Enumeration<PublicationStatus> status) { 1971 super(); 1972 this.status = status; 1973 } 1974 1975 /** 1976 * @return {@link #url} (An absolute URI that is used to identify this activity 1977 * definition when it is referenced in a specification, model, design or 1978 * an instance; also called its canonical identifier. This SHOULD be 1979 * globally unique and SHOULD be a literal address at which at which an 1980 * authoritative instance of this activity definition is (or will be) 1981 * published. This URL can be the target of a canonical reference. It 1982 * SHALL remain the same when the activity definition is stored on 1983 * different servers.). This is the underlying object with id, value and 1984 * extensions. The accessor "getUrl" gives direct access to the value 1985 */ 1986 public UriType getUrlElement() { 1987 if (this.url == null) 1988 if (Configuration.errorOnAutoCreate()) 1989 throw new Error("Attempt to auto-create ActivityDefinition.url"); 1990 else if (Configuration.doAutoCreate()) 1991 this.url = new UriType(); // bb 1992 return this.url; 1993 } 1994 1995 public boolean hasUrlElement() { 1996 return this.url != null && !this.url.isEmpty(); 1997 } 1998 1999 public boolean hasUrl() { 2000 return this.url != null && !this.url.isEmpty(); 2001 } 2002 2003 /** 2004 * @param value {@link #url} (An absolute URI that is used to identify this 2005 * activity definition when it is referenced in a specification, 2006 * model, design or an instance; also called its canonical 2007 * identifier. This SHOULD be globally unique and SHOULD be a 2008 * literal address at which at which an authoritative instance of 2009 * this activity definition is (or will be) published. This URL can 2010 * be the target of a canonical reference. It SHALL remain the same 2011 * when the activity definition is stored on different servers.). 2012 * This is the underlying object with id, value and extensions. The 2013 * accessor "getUrl" gives direct access to the value 2014 */ 2015 public ActivityDefinition setUrlElement(UriType value) { 2016 this.url = value; 2017 return this; 2018 } 2019 2020 /** 2021 * @return An absolute URI that is used to identify this activity definition 2022 * when it is referenced in a specification, model, design or an 2023 * instance; also called its canonical identifier. This SHOULD be 2024 * globally unique and SHOULD be a literal address at which at which an 2025 * authoritative instance of this activity definition is (or will be) 2026 * published. This URL can be the target of a canonical reference. It 2027 * SHALL remain the same when the activity definition is stored on 2028 * different servers. 2029 */ 2030 public String getUrl() { 2031 return this.url == null ? null : this.url.getValue(); 2032 } 2033 2034 /** 2035 * @param value An absolute URI that is used to identify this activity 2036 * definition when it is referenced in a specification, model, 2037 * design or an instance; also called its canonical identifier. 2038 * This SHOULD be globally unique and SHOULD be a literal address 2039 * at which at which an authoritative instance of this activity 2040 * definition is (or will be) published. This URL can be the target 2041 * of a canonical reference. It SHALL remain the same when the 2042 * activity definition is stored on different servers. 2043 */ 2044 public ActivityDefinition setUrl(String value) { 2045 if (Utilities.noString(value)) 2046 this.url = null; 2047 else { 2048 if (this.url == null) 2049 this.url = new UriType(); 2050 this.url.setValue(value); 2051 } 2052 return this; 2053 } 2054 2055 /** 2056 * @return {@link #identifier} (A formal identifier that is used to identify 2057 * this activity definition when it is represented in other formats, or 2058 * referenced in a specification, model, design or an instance.) 2059 */ 2060 public List<Identifier> getIdentifier() { 2061 if (this.identifier == null) 2062 this.identifier = new ArrayList<Identifier>(); 2063 return this.identifier; 2064 } 2065 2066 /** 2067 * @return Returns a reference to <code>this</code> for easy method chaining 2068 */ 2069 public ActivityDefinition setIdentifier(List<Identifier> theIdentifier) { 2070 this.identifier = theIdentifier; 2071 return this; 2072 } 2073 2074 public boolean hasIdentifier() { 2075 if (this.identifier == null) 2076 return false; 2077 for (Identifier item : this.identifier) 2078 if (!item.isEmpty()) 2079 return true; 2080 return false; 2081 } 2082 2083 public Identifier addIdentifier() { // 3 2084 Identifier t = new Identifier(); 2085 if (this.identifier == null) 2086 this.identifier = new ArrayList<Identifier>(); 2087 this.identifier.add(t); 2088 return t; 2089 } 2090 2091 public ActivityDefinition addIdentifier(Identifier t) { // 3 2092 if (t == null) 2093 return this; 2094 if (this.identifier == null) 2095 this.identifier = new ArrayList<Identifier>(); 2096 this.identifier.add(t); 2097 return this; 2098 } 2099 2100 /** 2101 * @return The first repetition of repeating field {@link #identifier}, creating 2102 * it if it does not already exist 2103 */ 2104 public Identifier getIdentifierFirstRep() { 2105 if (getIdentifier().isEmpty()) { 2106 addIdentifier(); 2107 } 2108 return getIdentifier().get(0); 2109 } 2110 2111 /** 2112 * @return {@link #version} (The identifier that is used to identify this 2113 * version of the activity definition when it is referenced in a 2114 * specification, model, design or instance. This is an arbitrary value 2115 * managed by the activity definition author and is not expected to be 2116 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 2117 * if a managed version is not available. There is also no expectation 2118 * that versions can be placed in a lexicographical sequence. To provide 2119 * a version consistent with the Decision Support Service specification, 2120 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 2121 * information on versioning knowledge assets, refer to the Decision 2122 * Support Service specification. Note that a version is required for 2123 * non-experimental active assets.). This is the underlying object with 2124 * id, value and extensions. The accessor "getVersion" gives direct 2125 * access to the value 2126 */ 2127 public StringType getVersionElement() { 2128 if (this.version == null) 2129 if (Configuration.errorOnAutoCreate()) 2130 throw new Error("Attempt to auto-create ActivityDefinition.version"); 2131 else if (Configuration.doAutoCreate()) 2132 this.version = new StringType(); // bb 2133 return this.version; 2134 } 2135 2136 public boolean hasVersionElement() { 2137 return this.version != null && !this.version.isEmpty(); 2138 } 2139 2140 public boolean hasVersion() { 2141 return this.version != null && !this.version.isEmpty(); 2142 } 2143 2144 /** 2145 * @param value {@link #version} (The identifier that is used to identify this 2146 * version of the activity definition when it is referenced in a 2147 * specification, model, design or instance. This is an arbitrary 2148 * value managed by the activity definition author and is not 2149 * expected to be globally unique. For example, it might be a 2150 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2151 * There is also no expectation that versions can be placed in a 2152 * lexicographical sequence. To provide a version consistent with 2153 * the Decision Support Service specification, use the format 2154 * Major.Minor.Revision (e.g. 1.0.0). For more information on 2155 * versioning knowledge assets, refer to the Decision Support 2156 * Service specification. Note that a version is required for 2157 * non-experimental active assets.). This is the underlying object 2158 * with id, value and extensions. The accessor "getVersion" gives 2159 * direct access to the value 2160 */ 2161 public ActivityDefinition setVersionElement(StringType value) { 2162 this.version = value; 2163 return this; 2164 } 2165 2166 /** 2167 * @return The identifier that is used to identify this version of the activity 2168 * definition when it is referenced in a specification, model, design or 2169 * instance. This is an arbitrary value managed by the activity 2170 * definition author and is not expected to be globally unique. For 2171 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 2172 * is not available. There is also no expectation that versions can be 2173 * placed in a lexicographical sequence. To provide a version consistent 2174 * with the Decision Support Service specification, use the format 2175 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 2176 * knowledge assets, refer to the Decision Support Service 2177 * specification. Note that a version is required for non-experimental 2178 * active assets. 2179 */ 2180 public String getVersion() { 2181 return this.version == null ? null : this.version.getValue(); 2182 } 2183 2184 /** 2185 * @param value The identifier that is used to identify this version of the 2186 * activity definition when it is referenced in a specification, 2187 * model, design or instance. This is an arbitrary value managed by 2188 * the activity definition author and is not expected to be 2189 * globally unique. For example, it might be a timestamp (e.g. 2190 * yyyymmdd) if a managed version is not available. There is also 2191 * no expectation that versions can be placed in a lexicographical 2192 * sequence. To provide a version consistent with the Decision 2193 * Support Service specification, use the format 2194 * Major.Minor.Revision (e.g. 1.0.0). For more information on 2195 * versioning knowledge assets, refer to the Decision Support 2196 * Service specification. Note that a version is required for 2197 * non-experimental active assets. 2198 */ 2199 public ActivityDefinition setVersion(String value) { 2200 if (Utilities.noString(value)) 2201 this.version = null; 2202 else { 2203 if (this.version == null) 2204 this.version = new StringType(); 2205 this.version.setValue(value); 2206 } 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #name} (A natural language name identifying the activity 2212 * definition. This name should be usable as an identifier for the 2213 * module by machine processing applications such as code generation.). 2214 * This is the underlying object with id, value and extensions. The 2215 * accessor "getName" gives direct access to the value 2216 */ 2217 public StringType getNameElement() { 2218 if (this.name == null) 2219 if (Configuration.errorOnAutoCreate()) 2220 throw new Error("Attempt to auto-create ActivityDefinition.name"); 2221 else if (Configuration.doAutoCreate()) 2222 this.name = new StringType(); // bb 2223 return this.name; 2224 } 2225 2226 public boolean hasNameElement() { 2227 return this.name != null && !this.name.isEmpty(); 2228 } 2229 2230 public boolean hasName() { 2231 return this.name != null && !this.name.isEmpty(); 2232 } 2233 2234 /** 2235 * @param value {@link #name} (A natural language name identifying the activity 2236 * definition. This name should be usable as an identifier for the 2237 * module by machine processing applications such as code 2238 * generation.). This is the underlying object with id, value and 2239 * extensions. The accessor "getName" gives direct access to the 2240 * value 2241 */ 2242 public ActivityDefinition setNameElement(StringType value) { 2243 this.name = value; 2244 return this; 2245 } 2246 2247 /** 2248 * @return A natural language name identifying the activity definition. This 2249 * name should be usable as an identifier for the module by machine 2250 * processing applications such as code generation. 2251 */ 2252 public String getName() { 2253 return this.name == null ? null : this.name.getValue(); 2254 } 2255 2256 /** 2257 * @param value A natural language name identifying the activity definition. 2258 * This name should be usable as an identifier for the module by 2259 * machine processing applications such as code generation. 2260 */ 2261 public ActivityDefinition setName(String value) { 2262 if (Utilities.noString(value)) 2263 this.name = null; 2264 else { 2265 if (this.name == null) 2266 this.name = new StringType(); 2267 this.name.setValue(value); 2268 } 2269 return this; 2270 } 2271 2272 /** 2273 * @return {@link #title} (A short, descriptive, user-friendly title for the 2274 * activity definition.). This is the underlying object with id, value 2275 * and extensions. The accessor "getTitle" gives direct access to the 2276 * value 2277 */ 2278 public StringType getTitleElement() { 2279 if (this.title == null) 2280 if (Configuration.errorOnAutoCreate()) 2281 throw new Error("Attempt to auto-create ActivityDefinition.title"); 2282 else if (Configuration.doAutoCreate()) 2283 this.title = new StringType(); // bb 2284 return this.title; 2285 } 2286 2287 public boolean hasTitleElement() { 2288 return this.title != null && !this.title.isEmpty(); 2289 } 2290 2291 public boolean hasTitle() { 2292 return this.title != null && !this.title.isEmpty(); 2293 } 2294 2295 /** 2296 * @param value {@link #title} (A short, descriptive, user-friendly title for 2297 * the activity definition.). This is the underlying object with 2298 * id, value and extensions. The accessor "getTitle" gives direct 2299 * access to the value 2300 */ 2301 public ActivityDefinition setTitleElement(StringType value) { 2302 this.title = value; 2303 return this; 2304 } 2305 2306 /** 2307 * @return A short, descriptive, user-friendly title for the activity 2308 * definition. 2309 */ 2310 public String getTitle() { 2311 return this.title == null ? null : this.title.getValue(); 2312 } 2313 2314 /** 2315 * @param value A short, descriptive, user-friendly title for the activity 2316 * definition. 2317 */ 2318 public ActivityDefinition setTitle(String value) { 2319 if (Utilities.noString(value)) 2320 this.title = null; 2321 else { 2322 if (this.title == null) 2323 this.title = new StringType(); 2324 this.title.setValue(value); 2325 } 2326 return this; 2327 } 2328 2329 /** 2330 * @return {@link #subtitle} (An explanatory or alternate title for the activity 2331 * definition giving additional information about its content.). This is 2332 * the underlying object with id, value and extensions. The accessor 2333 * "getSubtitle" gives direct access to the value 2334 */ 2335 public StringType getSubtitleElement() { 2336 if (this.subtitle == null) 2337 if (Configuration.errorOnAutoCreate()) 2338 throw new Error("Attempt to auto-create ActivityDefinition.subtitle"); 2339 else if (Configuration.doAutoCreate()) 2340 this.subtitle = new StringType(); // bb 2341 return this.subtitle; 2342 } 2343 2344 public boolean hasSubtitleElement() { 2345 return this.subtitle != null && !this.subtitle.isEmpty(); 2346 } 2347 2348 public boolean hasSubtitle() { 2349 return this.subtitle != null && !this.subtitle.isEmpty(); 2350 } 2351 2352 /** 2353 * @param value {@link #subtitle} (An explanatory or alternate title for the 2354 * activity definition giving additional information about its 2355 * content.). This is the underlying object with id, value and 2356 * extensions. The accessor "getSubtitle" gives direct access to 2357 * the value 2358 */ 2359 public ActivityDefinition setSubtitleElement(StringType value) { 2360 this.subtitle = value; 2361 return this; 2362 } 2363 2364 /** 2365 * @return An explanatory or alternate title for the activity definition giving 2366 * additional information about its content. 2367 */ 2368 public String getSubtitle() { 2369 return this.subtitle == null ? null : this.subtitle.getValue(); 2370 } 2371 2372 /** 2373 * @param value An explanatory or alternate title for the activity definition 2374 * giving additional information about its content. 2375 */ 2376 public ActivityDefinition setSubtitle(String value) { 2377 if (Utilities.noString(value)) 2378 this.subtitle = null; 2379 else { 2380 if (this.subtitle == null) 2381 this.subtitle = new StringType(); 2382 this.subtitle.setValue(value); 2383 } 2384 return this; 2385 } 2386 2387 /** 2388 * @return {@link #status} (The status of this activity definition. Enables 2389 * tracking the life-cycle of the content.). This is the underlying 2390 * object with id, value and extensions. The accessor "getStatus" gives 2391 * direct access to the value 2392 */ 2393 public Enumeration<PublicationStatus> getStatusElement() { 2394 if (this.status == null) 2395 if (Configuration.errorOnAutoCreate()) 2396 throw new Error("Attempt to auto-create ActivityDefinition.status"); 2397 else if (Configuration.doAutoCreate()) 2398 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2399 return this.status; 2400 } 2401 2402 public boolean hasStatusElement() { 2403 return this.status != null && !this.status.isEmpty(); 2404 } 2405 2406 public boolean hasStatus() { 2407 return this.status != null && !this.status.isEmpty(); 2408 } 2409 2410 /** 2411 * @param value {@link #status} (The status of this activity definition. Enables 2412 * tracking the life-cycle of the content.). This is the underlying 2413 * object with id, value and extensions. The accessor "getStatus" 2414 * gives direct access to the value 2415 */ 2416 public ActivityDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2417 this.status = value; 2418 return this; 2419 } 2420 2421 /** 2422 * @return The status of this activity definition. Enables tracking the 2423 * life-cycle of the content. 2424 */ 2425 public PublicationStatus getStatus() { 2426 return this.status == null ? null : this.status.getValue(); 2427 } 2428 2429 /** 2430 * @param value The status of this activity definition. Enables tracking the 2431 * life-cycle of the content. 2432 */ 2433 public ActivityDefinition setStatus(PublicationStatus value) { 2434 if (this.status == null) 2435 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2436 this.status.setValue(value); 2437 return this; 2438 } 2439 2440 /** 2441 * @return {@link #experimental} (A Boolean value to indicate that this activity 2442 * definition is authored for testing purposes (or 2443 * education/evaluation/marketing) and is not intended to be used for 2444 * genuine usage.). This is the underlying object with id, value and 2445 * extensions. The accessor "getExperimental" gives direct access to the 2446 * value 2447 */ 2448 public BooleanType getExperimentalElement() { 2449 if (this.experimental == null) 2450 if (Configuration.errorOnAutoCreate()) 2451 throw new Error("Attempt to auto-create ActivityDefinition.experimental"); 2452 else if (Configuration.doAutoCreate()) 2453 this.experimental = new BooleanType(); // bb 2454 return this.experimental; 2455 } 2456 2457 public boolean hasExperimentalElement() { 2458 return this.experimental != null && !this.experimental.isEmpty(); 2459 } 2460 2461 public boolean hasExperimental() { 2462 return this.experimental != null && !this.experimental.isEmpty(); 2463 } 2464 2465 /** 2466 * @param value {@link #experimental} (A Boolean value to indicate that this 2467 * activity definition is authored for testing purposes (or 2468 * education/evaluation/marketing) and is not intended to be used 2469 * for genuine usage.). This is the underlying object with id, 2470 * value and extensions. The accessor "getExperimental" gives 2471 * direct access to the value 2472 */ 2473 public ActivityDefinition setExperimentalElement(BooleanType value) { 2474 this.experimental = value; 2475 return this; 2476 } 2477 2478 /** 2479 * @return A Boolean value to indicate that this activity definition is authored 2480 * for testing purposes (or education/evaluation/marketing) and is not 2481 * intended to be used for genuine usage. 2482 */ 2483 public boolean getExperimental() { 2484 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2485 } 2486 2487 /** 2488 * @param value A Boolean value to indicate that this activity definition is 2489 * authored for testing purposes (or 2490 * education/evaluation/marketing) and is not intended to be used 2491 * for genuine usage. 2492 */ 2493 public ActivityDefinition setExperimental(boolean value) { 2494 if (this.experimental == null) 2495 this.experimental = new BooleanType(); 2496 this.experimental.setValue(value); 2497 return this; 2498 } 2499 2500 /** 2501 * @return {@link #subject} (A code or group definition that describes the 2502 * intended subject of the activity being defined.) 2503 */ 2504 public Type getSubject() { 2505 return this.subject; 2506 } 2507 2508 /** 2509 * @return {@link #subject} (A code or group definition that describes the 2510 * intended subject of the activity being defined.) 2511 */ 2512 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2513 if (this.subject == null) 2514 this.subject = new CodeableConcept(); 2515 if (!(this.subject instanceof CodeableConcept)) 2516 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2517 + this.subject.getClass().getName() + " was encountered"); 2518 return (CodeableConcept) this.subject; 2519 } 2520 2521 public boolean hasSubjectCodeableConcept() { 2522 return this != null && this.subject instanceof CodeableConcept; 2523 } 2524 2525 /** 2526 * @return {@link #subject} (A code or group definition that describes the 2527 * intended subject of the activity being defined.) 2528 */ 2529 public Reference getSubjectReference() throws FHIRException { 2530 if (this.subject == null) 2531 this.subject = new Reference(); 2532 if (!(this.subject instanceof Reference)) 2533 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 2534 + " was encountered"); 2535 return (Reference) this.subject; 2536 } 2537 2538 public boolean hasSubjectReference() { 2539 return this != null && this.subject instanceof Reference; 2540 } 2541 2542 public boolean hasSubject() { 2543 return this.subject != null && !this.subject.isEmpty(); 2544 } 2545 2546 /** 2547 * @param value {@link #subject} (A code or group definition that describes the 2548 * intended subject of the activity being defined.) 2549 */ 2550 public ActivityDefinition setSubject(Type value) { 2551 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2552 throw new Error("Not the right type for ActivityDefinition.subject[x]: " + value.fhirType()); 2553 this.subject = value; 2554 return this; 2555 } 2556 2557 /** 2558 * @return {@link #date} (The date (and optionally time) when the activity 2559 * definition was published. The date must change when the business 2560 * version changes and it must change if the status code changes. In 2561 * addition, it should change when the substantive content of the 2562 * activity definition changes.). This is the underlying object with id, 2563 * value and extensions. The accessor "getDate" gives direct access to 2564 * the value 2565 */ 2566 public DateTimeType getDateElement() { 2567 if (this.date == null) 2568 if (Configuration.errorOnAutoCreate()) 2569 throw new Error("Attempt to auto-create ActivityDefinition.date"); 2570 else if (Configuration.doAutoCreate()) 2571 this.date = new DateTimeType(); // bb 2572 return this.date; 2573 } 2574 2575 public boolean hasDateElement() { 2576 return this.date != null && !this.date.isEmpty(); 2577 } 2578 2579 public boolean hasDate() { 2580 return this.date != null && !this.date.isEmpty(); 2581 } 2582 2583 /** 2584 * @param value {@link #date} (The date (and optionally time) when the activity 2585 * definition was published. The date must change when the business 2586 * version changes and it must change if the status code changes. 2587 * In addition, it should change when the substantive content of 2588 * the activity definition changes.). This is the underlying object 2589 * with id, value and extensions. The accessor "getDate" gives 2590 * direct access to the value 2591 */ 2592 public ActivityDefinition setDateElement(DateTimeType value) { 2593 this.date = value; 2594 return this; 2595 } 2596 2597 /** 2598 * @return The date (and optionally time) when the activity definition was 2599 * published. The date must change when the business version changes and 2600 * it must change if the status code changes. In addition, it should 2601 * change when the substantive content of the activity definition 2602 * changes. 2603 */ 2604 public Date getDate() { 2605 return this.date == null ? null : this.date.getValue(); 2606 } 2607 2608 /** 2609 * @param value The date (and optionally time) when the activity definition was 2610 * published. The date must change when the business version 2611 * changes and it must change if the status code changes. In 2612 * addition, it should change when the substantive content of the 2613 * activity definition changes. 2614 */ 2615 public ActivityDefinition setDate(Date value) { 2616 if (value == null) 2617 this.date = null; 2618 else { 2619 if (this.date == null) 2620 this.date = new DateTimeType(); 2621 this.date.setValue(value); 2622 } 2623 return this; 2624 } 2625 2626 /** 2627 * @return {@link #publisher} (The name of the organization or individual that 2628 * published the activity definition.). This is the underlying object 2629 * with id, value and extensions. The accessor "getPublisher" gives 2630 * direct access to the value 2631 */ 2632 public StringType getPublisherElement() { 2633 if (this.publisher == null) 2634 if (Configuration.errorOnAutoCreate()) 2635 throw new Error("Attempt to auto-create ActivityDefinition.publisher"); 2636 else if (Configuration.doAutoCreate()) 2637 this.publisher = new StringType(); // bb 2638 return this.publisher; 2639 } 2640 2641 public boolean hasPublisherElement() { 2642 return this.publisher != null && !this.publisher.isEmpty(); 2643 } 2644 2645 public boolean hasPublisher() { 2646 return this.publisher != null && !this.publisher.isEmpty(); 2647 } 2648 2649 /** 2650 * @param value {@link #publisher} (The name of the organization or individual 2651 * that published the activity definition.). This is the underlying 2652 * object with id, value and extensions. The accessor 2653 * "getPublisher" gives direct access to the value 2654 */ 2655 public ActivityDefinition setPublisherElement(StringType value) { 2656 this.publisher = value; 2657 return this; 2658 } 2659 2660 /** 2661 * @return The name of the organization or individual that published the 2662 * activity definition. 2663 */ 2664 public String getPublisher() { 2665 return this.publisher == null ? null : this.publisher.getValue(); 2666 } 2667 2668 /** 2669 * @param value The name of the organization or individual that published the 2670 * activity definition. 2671 */ 2672 public ActivityDefinition setPublisher(String value) { 2673 if (Utilities.noString(value)) 2674 this.publisher = null; 2675 else { 2676 if (this.publisher == null) 2677 this.publisher = new StringType(); 2678 this.publisher.setValue(value); 2679 } 2680 return this; 2681 } 2682 2683 /** 2684 * @return {@link #contact} (Contact details to assist a user in finding and 2685 * communicating with the publisher.) 2686 */ 2687 public List<ContactDetail> getContact() { 2688 if (this.contact == null) 2689 this.contact = new ArrayList<ContactDetail>(); 2690 return this.contact; 2691 } 2692 2693 /** 2694 * @return Returns a reference to <code>this</code> for easy method chaining 2695 */ 2696 public ActivityDefinition setContact(List<ContactDetail> theContact) { 2697 this.contact = theContact; 2698 return this; 2699 } 2700 2701 public boolean hasContact() { 2702 if (this.contact == null) 2703 return false; 2704 for (ContactDetail item : this.contact) 2705 if (!item.isEmpty()) 2706 return true; 2707 return false; 2708 } 2709 2710 public ContactDetail addContact() { // 3 2711 ContactDetail t = new ContactDetail(); 2712 if (this.contact == null) 2713 this.contact = new ArrayList<ContactDetail>(); 2714 this.contact.add(t); 2715 return t; 2716 } 2717 2718 public ActivityDefinition addContact(ContactDetail t) { // 3 2719 if (t == null) 2720 return this; 2721 if (this.contact == null) 2722 this.contact = new ArrayList<ContactDetail>(); 2723 this.contact.add(t); 2724 return this; 2725 } 2726 2727 /** 2728 * @return The first repetition of repeating field {@link #contact}, creating it 2729 * if it does not already exist 2730 */ 2731 public ContactDetail getContactFirstRep() { 2732 if (getContact().isEmpty()) { 2733 addContact(); 2734 } 2735 return getContact().get(0); 2736 } 2737 2738 /** 2739 * @return {@link #description} (A free text natural language description of the 2740 * activity definition from a consumer's perspective.). This is the 2741 * underlying object with id, value and extensions. The accessor 2742 * "getDescription" gives direct access to the value 2743 */ 2744 public MarkdownType getDescriptionElement() { 2745 if (this.description == null) 2746 if (Configuration.errorOnAutoCreate()) 2747 throw new Error("Attempt to auto-create ActivityDefinition.description"); 2748 else if (Configuration.doAutoCreate()) 2749 this.description = new MarkdownType(); // bb 2750 return this.description; 2751 } 2752 2753 public boolean hasDescriptionElement() { 2754 return this.description != null && !this.description.isEmpty(); 2755 } 2756 2757 public boolean hasDescription() { 2758 return this.description != null && !this.description.isEmpty(); 2759 } 2760 2761 /** 2762 * @param value {@link #description} (A free text natural language description 2763 * of the activity definition from a consumer's perspective.). This 2764 * is the underlying object with id, value and extensions. The 2765 * accessor "getDescription" gives direct access to the value 2766 */ 2767 public ActivityDefinition setDescriptionElement(MarkdownType value) { 2768 this.description = value; 2769 return this; 2770 } 2771 2772 /** 2773 * @return A free text natural language description of the activity definition 2774 * from a consumer's perspective. 2775 */ 2776 public String getDescription() { 2777 return this.description == null ? null : this.description.getValue(); 2778 } 2779 2780 /** 2781 * @param value A free text natural language description of the activity 2782 * definition from a consumer's perspective. 2783 */ 2784 public ActivityDefinition setDescription(String value) { 2785 if (value == null) 2786 this.description = null; 2787 else { 2788 if (this.description == null) 2789 this.description = new MarkdownType(); 2790 this.description.setValue(value); 2791 } 2792 return this; 2793 } 2794 2795 /** 2796 * @return {@link #useContext} (The content was developed with a focus and 2797 * intent of supporting the contexts that are listed. These contexts may 2798 * be general categories (gender, age, ...) or may be references to 2799 * specific programs (insurance plans, studies, ...) and may be used to 2800 * assist with indexing and searching for appropriate activity 2801 * definition instances.) 2802 */ 2803 public List<UsageContext> getUseContext() { 2804 if (this.useContext == null) 2805 this.useContext = new ArrayList<UsageContext>(); 2806 return this.useContext; 2807 } 2808 2809 /** 2810 * @return Returns a reference to <code>this</code> for easy method chaining 2811 */ 2812 public ActivityDefinition setUseContext(List<UsageContext> theUseContext) { 2813 this.useContext = theUseContext; 2814 return this; 2815 } 2816 2817 public boolean hasUseContext() { 2818 if (this.useContext == null) 2819 return false; 2820 for (UsageContext item : this.useContext) 2821 if (!item.isEmpty()) 2822 return true; 2823 return false; 2824 } 2825 2826 public UsageContext addUseContext() { // 3 2827 UsageContext t = new UsageContext(); 2828 if (this.useContext == null) 2829 this.useContext = new ArrayList<UsageContext>(); 2830 this.useContext.add(t); 2831 return t; 2832 } 2833 2834 public ActivityDefinition addUseContext(UsageContext t) { // 3 2835 if (t == null) 2836 return this; 2837 if (this.useContext == null) 2838 this.useContext = new ArrayList<UsageContext>(); 2839 this.useContext.add(t); 2840 return this; 2841 } 2842 2843 /** 2844 * @return The first repetition of repeating field {@link #useContext}, creating 2845 * it if it does not already exist 2846 */ 2847 public UsageContext getUseContextFirstRep() { 2848 if (getUseContext().isEmpty()) { 2849 addUseContext(); 2850 } 2851 return getUseContext().get(0); 2852 } 2853 2854 /** 2855 * @return {@link #jurisdiction} (A legal or geographic region in which the 2856 * activity definition is intended to be used.) 2857 */ 2858 public List<CodeableConcept> getJurisdiction() { 2859 if (this.jurisdiction == null) 2860 this.jurisdiction = new ArrayList<CodeableConcept>(); 2861 return this.jurisdiction; 2862 } 2863 2864 /** 2865 * @return Returns a reference to <code>this</code> for easy method chaining 2866 */ 2867 public ActivityDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2868 this.jurisdiction = theJurisdiction; 2869 return this; 2870 } 2871 2872 public boolean hasJurisdiction() { 2873 if (this.jurisdiction == null) 2874 return false; 2875 for (CodeableConcept item : this.jurisdiction) 2876 if (!item.isEmpty()) 2877 return true; 2878 return false; 2879 } 2880 2881 public CodeableConcept addJurisdiction() { // 3 2882 CodeableConcept t = new CodeableConcept(); 2883 if (this.jurisdiction == null) 2884 this.jurisdiction = new ArrayList<CodeableConcept>(); 2885 this.jurisdiction.add(t); 2886 return t; 2887 } 2888 2889 public ActivityDefinition addJurisdiction(CodeableConcept t) { // 3 2890 if (t == null) 2891 return this; 2892 if (this.jurisdiction == null) 2893 this.jurisdiction = new ArrayList<CodeableConcept>(); 2894 this.jurisdiction.add(t); 2895 return this; 2896 } 2897 2898 /** 2899 * @return The first repetition of repeating field {@link #jurisdiction}, 2900 * creating it if it does not already exist 2901 */ 2902 public CodeableConcept getJurisdictionFirstRep() { 2903 if (getJurisdiction().isEmpty()) { 2904 addJurisdiction(); 2905 } 2906 return getJurisdiction().get(0); 2907 } 2908 2909 /** 2910 * @return {@link #purpose} (Explanation of why this activity definition is 2911 * needed and why it has been designed as it has.). This is the 2912 * underlying object with id, value and extensions. The accessor 2913 * "getPurpose" gives direct access to the value 2914 */ 2915 public MarkdownType getPurposeElement() { 2916 if (this.purpose == null) 2917 if (Configuration.errorOnAutoCreate()) 2918 throw new Error("Attempt to auto-create ActivityDefinition.purpose"); 2919 else if (Configuration.doAutoCreate()) 2920 this.purpose = new MarkdownType(); // bb 2921 return this.purpose; 2922 } 2923 2924 public boolean hasPurposeElement() { 2925 return this.purpose != null && !this.purpose.isEmpty(); 2926 } 2927 2928 public boolean hasPurpose() { 2929 return this.purpose != null && !this.purpose.isEmpty(); 2930 } 2931 2932 /** 2933 * @param value {@link #purpose} (Explanation of why this activity definition is 2934 * needed and why it has been designed as it has.). This is the 2935 * underlying object with id, value and extensions. The accessor 2936 * "getPurpose" gives direct access to the value 2937 */ 2938 public ActivityDefinition setPurposeElement(MarkdownType value) { 2939 this.purpose = value; 2940 return this; 2941 } 2942 2943 /** 2944 * @return Explanation of why this activity definition is needed and why it has 2945 * been designed as it has. 2946 */ 2947 public String getPurpose() { 2948 return this.purpose == null ? null : this.purpose.getValue(); 2949 } 2950 2951 /** 2952 * @param value Explanation of why this activity definition is needed and why it 2953 * has been designed as it has. 2954 */ 2955 public ActivityDefinition setPurpose(String value) { 2956 if (value == null) 2957 this.purpose = null; 2958 else { 2959 if (this.purpose == null) 2960 this.purpose = new MarkdownType(); 2961 this.purpose.setValue(value); 2962 } 2963 return this; 2964 } 2965 2966 /** 2967 * @return {@link #usage} (A detailed description of how the activity definition 2968 * is used from a clinical perspective.). This is the underlying object 2969 * with id, value and extensions. The accessor "getUsage" gives direct 2970 * access to the value 2971 */ 2972 public StringType getUsageElement() { 2973 if (this.usage == null) 2974 if (Configuration.errorOnAutoCreate()) 2975 throw new Error("Attempt to auto-create ActivityDefinition.usage"); 2976 else if (Configuration.doAutoCreate()) 2977 this.usage = new StringType(); // bb 2978 return this.usage; 2979 } 2980 2981 public boolean hasUsageElement() { 2982 return this.usage != null && !this.usage.isEmpty(); 2983 } 2984 2985 public boolean hasUsage() { 2986 return this.usage != null && !this.usage.isEmpty(); 2987 } 2988 2989 /** 2990 * @param value {@link #usage} (A detailed description of how the activity 2991 * definition is used from a clinical perspective.). This is the 2992 * underlying object with id, value and extensions. The accessor 2993 * "getUsage" gives direct access to the value 2994 */ 2995 public ActivityDefinition setUsageElement(StringType value) { 2996 this.usage = value; 2997 return this; 2998 } 2999 3000 /** 3001 * @return A detailed description of how the activity definition is used from a 3002 * clinical perspective. 3003 */ 3004 public String getUsage() { 3005 return this.usage == null ? null : this.usage.getValue(); 3006 } 3007 3008 /** 3009 * @param value A detailed description of how the activity definition is used 3010 * from a clinical perspective. 3011 */ 3012 public ActivityDefinition setUsage(String value) { 3013 if (Utilities.noString(value)) 3014 this.usage = null; 3015 else { 3016 if (this.usage == null) 3017 this.usage = new StringType(); 3018 this.usage.setValue(value); 3019 } 3020 return this; 3021 } 3022 3023 /** 3024 * @return {@link #copyright} (A copyright statement relating to the activity 3025 * definition and/or its contents. Copyright statements are generally 3026 * legal restrictions on the use and publishing of the activity 3027 * definition.). This is the underlying object with id, value and 3028 * extensions. The accessor "getCopyright" gives direct access to the 3029 * value 3030 */ 3031 public MarkdownType getCopyrightElement() { 3032 if (this.copyright == null) 3033 if (Configuration.errorOnAutoCreate()) 3034 throw new Error("Attempt to auto-create ActivityDefinition.copyright"); 3035 else if (Configuration.doAutoCreate()) 3036 this.copyright = new MarkdownType(); // bb 3037 return this.copyright; 3038 } 3039 3040 public boolean hasCopyrightElement() { 3041 return this.copyright != null && !this.copyright.isEmpty(); 3042 } 3043 3044 public boolean hasCopyright() { 3045 return this.copyright != null && !this.copyright.isEmpty(); 3046 } 3047 3048 /** 3049 * @param value {@link #copyright} (A copyright statement relating to the 3050 * activity definition and/or its contents. Copyright statements 3051 * are generally legal restrictions on the use and publishing of 3052 * the activity definition.). This is the underlying object with 3053 * id, value and extensions. The accessor "getCopyright" gives 3054 * direct access to the value 3055 */ 3056 public ActivityDefinition setCopyrightElement(MarkdownType value) { 3057 this.copyright = value; 3058 return this; 3059 } 3060 3061 /** 3062 * @return A copyright statement relating to the activity definition and/or its 3063 * contents. Copyright statements are generally legal restrictions on 3064 * the use and publishing of the activity definition. 3065 */ 3066 public String getCopyright() { 3067 return this.copyright == null ? null : this.copyright.getValue(); 3068 } 3069 3070 /** 3071 * @param value A copyright statement relating to the activity definition and/or 3072 * its contents. Copyright statements are generally legal 3073 * restrictions on the use and publishing of the activity 3074 * definition. 3075 */ 3076 public ActivityDefinition setCopyright(String value) { 3077 if (value == null) 3078 this.copyright = null; 3079 else { 3080 if (this.copyright == null) 3081 this.copyright = new MarkdownType(); 3082 this.copyright.setValue(value); 3083 } 3084 return this; 3085 } 3086 3087 /** 3088 * @return {@link #approvalDate} (The date on which the resource content was 3089 * approved by the publisher. Approval happens once when the content is 3090 * officially approved for usage.). This is the underlying object with 3091 * id, value and extensions. The accessor "getApprovalDate" gives direct 3092 * access to the value 3093 */ 3094 public DateType getApprovalDateElement() { 3095 if (this.approvalDate == null) 3096 if (Configuration.errorOnAutoCreate()) 3097 throw new Error("Attempt to auto-create ActivityDefinition.approvalDate"); 3098 else if (Configuration.doAutoCreate()) 3099 this.approvalDate = new DateType(); // bb 3100 return this.approvalDate; 3101 } 3102 3103 public boolean hasApprovalDateElement() { 3104 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3105 } 3106 3107 public boolean hasApprovalDate() { 3108 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3109 } 3110 3111 /** 3112 * @param value {@link #approvalDate} (The date on which the resource content 3113 * was approved by the publisher. Approval happens once when the 3114 * content is officially approved for usage.). This is the 3115 * underlying object with id, value and extensions. The accessor 3116 * "getApprovalDate" gives direct access to the value 3117 */ 3118 public ActivityDefinition setApprovalDateElement(DateType value) { 3119 this.approvalDate = value; 3120 return this; 3121 } 3122 3123 /** 3124 * @return The date on which the resource content was approved by the publisher. 3125 * Approval happens once when the content is officially approved for 3126 * usage. 3127 */ 3128 public Date getApprovalDate() { 3129 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3130 } 3131 3132 /** 3133 * @param value The date on which the resource content was approved by the 3134 * publisher. Approval happens once when the content is officially 3135 * approved for usage. 3136 */ 3137 public ActivityDefinition setApprovalDate(Date value) { 3138 if (value == null) 3139 this.approvalDate = null; 3140 else { 3141 if (this.approvalDate == null) 3142 this.approvalDate = new DateType(); 3143 this.approvalDate.setValue(value); 3144 } 3145 return this; 3146 } 3147 3148 /** 3149 * @return {@link #lastReviewDate} (The date on which the resource content was 3150 * last reviewed. Review happens periodically after approval but does 3151 * not change the original approval date.). This is the underlying 3152 * object with id, value and extensions. The accessor 3153 * "getLastReviewDate" gives direct access to the value 3154 */ 3155 public DateType getLastReviewDateElement() { 3156 if (this.lastReviewDate == null) 3157 if (Configuration.errorOnAutoCreate()) 3158 throw new Error("Attempt to auto-create ActivityDefinition.lastReviewDate"); 3159 else if (Configuration.doAutoCreate()) 3160 this.lastReviewDate = new DateType(); // bb 3161 return this.lastReviewDate; 3162 } 3163 3164 public boolean hasLastReviewDateElement() { 3165 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3166 } 3167 3168 public boolean hasLastReviewDate() { 3169 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3170 } 3171 3172 /** 3173 * @param value {@link #lastReviewDate} (The date on which the resource content 3174 * was last reviewed. Review happens periodically after approval 3175 * but does not change the original approval date.). This is the 3176 * underlying object with id, value and extensions. The accessor 3177 * "getLastReviewDate" gives direct access to the value 3178 */ 3179 public ActivityDefinition setLastReviewDateElement(DateType value) { 3180 this.lastReviewDate = value; 3181 return this; 3182 } 3183 3184 /** 3185 * @return The date on which the resource content was last reviewed. Review 3186 * happens periodically after approval but does not change the original 3187 * approval date. 3188 */ 3189 public Date getLastReviewDate() { 3190 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3191 } 3192 3193 /** 3194 * @param value The date on which the resource content was last reviewed. Review 3195 * happens periodically after approval but does not change the 3196 * original approval date. 3197 */ 3198 public ActivityDefinition setLastReviewDate(Date value) { 3199 if (value == null) 3200 this.lastReviewDate = null; 3201 else { 3202 if (this.lastReviewDate == null) 3203 this.lastReviewDate = new DateType(); 3204 this.lastReviewDate.setValue(value); 3205 } 3206 return this; 3207 } 3208 3209 /** 3210 * @return {@link #effectivePeriod} (The period during which the activity 3211 * definition content was or is planned to be in active use.) 3212 */ 3213 public Period getEffectivePeriod() { 3214 if (this.effectivePeriod == null) 3215 if (Configuration.errorOnAutoCreate()) 3216 throw new Error("Attempt to auto-create ActivityDefinition.effectivePeriod"); 3217 else if (Configuration.doAutoCreate()) 3218 this.effectivePeriod = new Period(); // cc 3219 return this.effectivePeriod; 3220 } 3221 3222 public boolean hasEffectivePeriod() { 3223 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3224 } 3225 3226 /** 3227 * @param value {@link #effectivePeriod} (The period during which the activity 3228 * definition content was or is planned to be in active use.) 3229 */ 3230 public ActivityDefinition setEffectivePeriod(Period value) { 3231 this.effectivePeriod = value; 3232 return this; 3233 } 3234 3235 /** 3236 * @return {@link #topic} (Descriptive topics related to the content of the 3237 * activity. Topics provide a high-level categorization of the activity 3238 * that can be useful for filtering and searching.) 3239 */ 3240 public List<CodeableConcept> getTopic() { 3241 if (this.topic == null) 3242 this.topic = new ArrayList<CodeableConcept>(); 3243 return this.topic; 3244 } 3245 3246 /** 3247 * @return Returns a reference to <code>this</code> for easy method chaining 3248 */ 3249 public ActivityDefinition setTopic(List<CodeableConcept> theTopic) { 3250 this.topic = theTopic; 3251 return this; 3252 } 3253 3254 public boolean hasTopic() { 3255 if (this.topic == null) 3256 return false; 3257 for (CodeableConcept item : this.topic) 3258 if (!item.isEmpty()) 3259 return true; 3260 return false; 3261 } 3262 3263 public CodeableConcept addTopic() { // 3 3264 CodeableConcept t = new CodeableConcept(); 3265 if (this.topic == null) 3266 this.topic = new ArrayList<CodeableConcept>(); 3267 this.topic.add(t); 3268 return t; 3269 } 3270 3271 public ActivityDefinition addTopic(CodeableConcept t) { // 3 3272 if (t == null) 3273 return this; 3274 if (this.topic == null) 3275 this.topic = new ArrayList<CodeableConcept>(); 3276 this.topic.add(t); 3277 return this; 3278 } 3279 3280 /** 3281 * @return The first repetition of repeating field {@link #topic}, creating it 3282 * if it does not already exist 3283 */ 3284 public CodeableConcept getTopicFirstRep() { 3285 if (getTopic().isEmpty()) { 3286 addTopic(); 3287 } 3288 return getTopic().get(0); 3289 } 3290 3291 /** 3292 * @return {@link #author} (An individiual or organization primarily involved in 3293 * the creation and maintenance of the content.) 3294 */ 3295 public List<ContactDetail> getAuthor() { 3296 if (this.author == null) 3297 this.author = new ArrayList<ContactDetail>(); 3298 return this.author; 3299 } 3300 3301 /** 3302 * @return Returns a reference to <code>this</code> for easy method chaining 3303 */ 3304 public ActivityDefinition setAuthor(List<ContactDetail> theAuthor) { 3305 this.author = theAuthor; 3306 return this; 3307 } 3308 3309 public boolean hasAuthor() { 3310 if (this.author == null) 3311 return false; 3312 for (ContactDetail item : this.author) 3313 if (!item.isEmpty()) 3314 return true; 3315 return false; 3316 } 3317 3318 public ContactDetail addAuthor() { // 3 3319 ContactDetail t = new ContactDetail(); 3320 if (this.author == null) 3321 this.author = new ArrayList<ContactDetail>(); 3322 this.author.add(t); 3323 return t; 3324 } 3325 3326 public ActivityDefinition addAuthor(ContactDetail t) { // 3 3327 if (t == null) 3328 return this; 3329 if (this.author == null) 3330 this.author = new ArrayList<ContactDetail>(); 3331 this.author.add(t); 3332 return this; 3333 } 3334 3335 /** 3336 * @return The first repetition of repeating field {@link #author}, creating it 3337 * if it does not already exist 3338 */ 3339 public ContactDetail getAuthorFirstRep() { 3340 if (getAuthor().isEmpty()) { 3341 addAuthor(); 3342 } 3343 return getAuthor().get(0); 3344 } 3345 3346 /** 3347 * @return {@link #editor} (An individual or organization primarily responsible 3348 * for internal coherence of the content.) 3349 */ 3350 public List<ContactDetail> getEditor() { 3351 if (this.editor == null) 3352 this.editor = new ArrayList<ContactDetail>(); 3353 return this.editor; 3354 } 3355 3356 /** 3357 * @return Returns a reference to <code>this</code> for easy method chaining 3358 */ 3359 public ActivityDefinition setEditor(List<ContactDetail> theEditor) { 3360 this.editor = theEditor; 3361 return this; 3362 } 3363 3364 public boolean hasEditor() { 3365 if (this.editor == null) 3366 return false; 3367 for (ContactDetail item : this.editor) 3368 if (!item.isEmpty()) 3369 return true; 3370 return false; 3371 } 3372 3373 public ContactDetail addEditor() { // 3 3374 ContactDetail t = new ContactDetail(); 3375 if (this.editor == null) 3376 this.editor = new ArrayList<ContactDetail>(); 3377 this.editor.add(t); 3378 return t; 3379 } 3380 3381 public ActivityDefinition addEditor(ContactDetail t) { // 3 3382 if (t == null) 3383 return this; 3384 if (this.editor == null) 3385 this.editor = new ArrayList<ContactDetail>(); 3386 this.editor.add(t); 3387 return this; 3388 } 3389 3390 /** 3391 * @return The first repetition of repeating field {@link #editor}, creating it 3392 * if it does not already exist 3393 */ 3394 public ContactDetail getEditorFirstRep() { 3395 if (getEditor().isEmpty()) { 3396 addEditor(); 3397 } 3398 return getEditor().get(0); 3399 } 3400 3401 /** 3402 * @return {@link #reviewer} (An individual or organization primarily 3403 * responsible for review of some aspect of the content.) 3404 */ 3405 public List<ContactDetail> getReviewer() { 3406 if (this.reviewer == null) 3407 this.reviewer = new ArrayList<ContactDetail>(); 3408 return this.reviewer; 3409 } 3410 3411 /** 3412 * @return Returns a reference to <code>this</code> for easy method chaining 3413 */ 3414 public ActivityDefinition setReviewer(List<ContactDetail> theReviewer) { 3415 this.reviewer = theReviewer; 3416 return this; 3417 } 3418 3419 public boolean hasReviewer() { 3420 if (this.reviewer == null) 3421 return false; 3422 for (ContactDetail item : this.reviewer) 3423 if (!item.isEmpty()) 3424 return true; 3425 return false; 3426 } 3427 3428 public ContactDetail addReviewer() { // 3 3429 ContactDetail t = new ContactDetail(); 3430 if (this.reviewer == null) 3431 this.reviewer = new ArrayList<ContactDetail>(); 3432 this.reviewer.add(t); 3433 return t; 3434 } 3435 3436 public ActivityDefinition addReviewer(ContactDetail t) { // 3 3437 if (t == null) 3438 return this; 3439 if (this.reviewer == null) 3440 this.reviewer = new ArrayList<ContactDetail>(); 3441 this.reviewer.add(t); 3442 return this; 3443 } 3444 3445 /** 3446 * @return The first repetition of repeating field {@link #reviewer}, creating 3447 * it if it does not already exist 3448 */ 3449 public ContactDetail getReviewerFirstRep() { 3450 if (getReviewer().isEmpty()) { 3451 addReviewer(); 3452 } 3453 return getReviewer().get(0); 3454 } 3455 3456 /** 3457 * @return {@link #endorser} (An individual or organization responsible for 3458 * officially endorsing the content for use in some setting.) 3459 */ 3460 public List<ContactDetail> getEndorser() { 3461 if (this.endorser == null) 3462 this.endorser = new ArrayList<ContactDetail>(); 3463 return this.endorser; 3464 } 3465 3466 /** 3467 * @return Returns a reference to <code>this</code> for easy method chaining 3468 */ 3469 public ActivityDefinition setEndorser(List<ContactDetail> theEndorser) { 3470 this.endorser = theEndorser; 3471 return this; 3472 } 3473 3474 public boolean hasEndorser() { 3475 if (this.endorser == null) 3476 return false; 3477 for (ContactDetail item : this.endorser) 3478 if (!item.isEmpty()) 3479 return true; 3480 return false; 3481 } 3482 3483 public ContactDetail addEndorser() { // 3 3484 ContactDetail t = new ContactDetail(); 3485 if (this.endorser == null) 3486 this.endorser = new ArrayList<ContactDetail>(); 3487 this.endorser.add(t); 3488 return t; 3489 } 3490 3491 public ActivityDefinition addEndorser(ContactDetail t) { // 3 3492 if (t == null) 3493 return this; 3494 if (this.endorser == null) 3495 this.endorser = new ArrayList<ContactDetail>(); 3496 this.endorser.add(t); 3497 return this; 3498 } 3499 3500 /** 3501 * @return The first repetition of repeating field {@link #endorser}, creating 3502 * it if it does not already exist 3503 */ 3504 public ContactDetail getEndorserFirstRep() { 3505 if (getEndorser().isEmpty()) { 3506 addEndorser(); 3507 } 3508 return getEndorser().get(0); 3509 } 3510 3511 /** 3512 * @return {@link #relatedArtifact} (Related artifacts such as additional 3513 * documentation, justification, or bibliographic references.) 3514 */ 3515 public List<RelatedArtifact> getRelatedArtifact() { 3516 if (this.relatedArtifact == null) 3517 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3518 return this.relatedArtifact; 3519 } 3520 3521 /** 3522 * @return Returns a reference to <code>this</code> for easy method chaining 3523 */ 3524 public ActivityDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3525 this.relatedArtifact = theRelatedArtifact; 3526 return this; 3527 } 3528 3529 public boolean hasRelatedArtifact() { 3530 if (this.relatedArtifact == null) 3531 return false; 3532 for (RelatedArtifact item : this.relatedArtifact) 3533 if (!item.isEmpty()) 3534 return true; 3535 return false; 3536 } 3537 3538 public RelatedArtifact addRelatedArtifact() { // 3 3539 RelatedArtifact t = new RelatedArtifact(); 3540 if (this.relatedArtifact == null) 3541 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3542 this.relatedArtifact.add(t); 3543 return t; 3544 } 3545 3546 public ActivityDefinition addRelatedArtifact(RelatedArtifact t) { // 3 3547 if (t == null) 3548 return this; 3549 if (this.relatedArtifact == null) 3550 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3551 this.relatedArtifact.add(t); 3552 return this; 3553 } 3554 3555 /** 3556 * @return The first repetition of repeating field {@link #relatedArtifact}, 3557 * creating it if it does not already exist 3558 */ 3559 public RelatedArtifact getRelatedArtifactFirstRep() { 3560 if (getRelatedArtifact().isEmpty()) { 3561 addRelatedArtifact(); 3562 } 3563 return getRelatedArtifact().get(0); 3564 } 3565 3566 /** 3567 * @return {@link #library} (A reference to a Library resource containing any 3568 * formal logic used by the activity definition.) 3569 */ 3570 public List<CanonicalType> getLibrary() { 3571 if (this.library == null) 3572 this.library = new ArrayList<CanonicalType>(); 3573 return this.library; 3574 } 3575 3576 /** 3577 * @return Returns a reference to <code>this</code> for easy method chaining 3578 */ 3579 public ActivityDefinition setLibrary(List<CanonicalType> theLibrary) { 3580 this.library = theLibrary; 3581 return this; 3582 } 3583 3584 public boolean hasLibrary() { 3585 if (this.library == null) 3586 return false; 3587 for (CanonicalType item : this.library) 3588 if (!item.isEmpty()) 3589 return true; 3590 return false; 3591 } 3592 3593 /** 3594 * @return {@link #library} (A reference to a Library resource containing any 3595 * formal logic used by the activity definition.) 3596 */ 3597 public CanonicalType addLibraryElement() {// 2 3598 CanonicalType t = new CanonicalType(); 3599 if (this.library == null) 3600 this.library = new ArrayList<CanonicalType>(); 3601 this.library.add(t); 3602 return t; 3603 } 3604 3605 /** 3606 * @param value {@link #library} (A reference to a Library resource containing 3607 * any formal logic used by the activity definition.) 3608 */ 3609 public ActivityDefinition addLibrary(String value) { // 1 3610 CanonicalType t = new CanonicalType(); 3611 t.setValue(value); 3612 if (this.library == null) 3613 this.library = new ArrayList<CanonicalType>(); 3614 this.library.add(t); 3615 return this; 3616 } 3617 3618 /** 3619 * @param value {@link #library} (A reference to a Library resource containing 3620 * any formal logic used by the activity definition.) 3621 */ 3622 public boolean hasLibrary(String value) { 3623 if (this.library == null) 3624 return false; 3625 for (CanonicalType v : this.library) 3626 if (v.getValue().equals(value)) // canonical(Library) 3627 return true; 3628 return false; 3629 } 3630 3631 /** 3632 * @return {@link #kind} (A description of the kind of resource the activity 3633 * definition is representing. For example, a MedicationRequest, a 3634 * ServiceRequest, or a CommunicationRequest. Typically, but not always, 3635 * this is a Request resource.). This is the underlying object with id, 3636 * value and extensions. The accessor "getKind" gives direct access to 3637 * the value 3638 */ 3639 public Enumeration<ActivityDefinitionKind> getKindElement() { 3640 if (this.kind == null) 3641 if (Configuration.errorOnAutoCreate()) 3642 throw new Error("Attempt to auto-create ActivityDefinition.kind"); 3643 else if (Configuration.doAutoCreate()) 3644 this.kind = new Enumeration<ActivityDefinitionKind>(new ActivityDefinitionKindEnumFactory()); // bb 3645 return this.kind; 3646 } 3647 3648 public boolean hasKindElement() { 3649 return this.kind != null && !this.kind.isEmpty(); 3650 } 3651 3652 public boolean hasKind() { 3653 return this.kind != null && !this.kind.isEmpty(); 3654 } 3655 3656 /** 3657 * @param value {@link #kind} (A description of the kind of resource the 3658 * activity definition is representing. For example, a 3659 * MedicationRequest, a ServiceRequest, or a CommunicationRequest. 3660 * Typically, but not always, this is a Request resource.). This is 3661 * the underlying object with id, value and extensions. The 3662 * accessor "getKind" gives direct access to the value 3663 */ 3664 public ActivityDefinition setKindElement(Enumeration<ActivityDefinitionKind> value) { 3665 this.kind = value; 3666 return this; 3667 } 3668 3669 /** 3670 * @return A description of the kind of resource the activity definition is 3671 * representing. For example, a MedicationRequest, a ServiceRequest, or 3672 * a CommunicationRequest. Typically, but not always, this is a Request 3673 * resource. 3674 */ 3675 public ActivityDefinitionKind getKind() { 3676 return this.kind == null ? null : this.kind.getValue(); 3677 } 3678 3679 /** 3680 * @param value A description of the kind of resource the activity definition is 3681 * representing. For example, a MedicationRequest, a 3682 * ServiceRequest, or a CommunicationRequest. Typically, but not 3683 * always, this is a Request resource. 3684 */ 3685 public ActivityDefinition setKind(ActivityDefinitionKind value) { 3686 if (value == null) 3687 this.kind = null; 3688 else { 3689 if (this.kind == null) 3690 this.kind = new Enumeration<ActivityDefinitionKind>(new ActivityDefinitionKindEnumFactory()); 3691 this.kind.setValue(value); 3692 } 3693 return this; 3694 } 3695 3696 /** 3697 * @return {@link #profile} (A profile to which the target of the activity 3698 * definition is expected to conform.). This is the underlying object 3699 * with id, value and extensions. The accessor "getProfile" gives direct 3700 * access to the value 3701 */ 3702 public CanonicalType getProfileElement() { 3703 if (this.profile == null) 3704 if (Configuration.errorOnAutoCreate()) 3705 throw new Error("Attempt to auto-create ActivityDefinition.profile"); 3706 else if (Configuration.doAutoCreate()) 3707 this.profile = new CanonicalType(); // bb 3708 return this.profile; 3709 } 3710 3711 public boolean hasProfileElement() { 3712 return this.profile != null && !this.profile.isEmpty(); 3713 } 3714 3715 public boolean hasProfile() { 3716 return this.profile != null && !this.profile.isEmpty(); 3717 } 3718 3719 /** 3720 * @param value {@link #profile} (A profile to which the target of the activity 3721 * definition is expected to conform.). This is the underlying 3722 * object with id, value and extensions. The accessor "getProfile" 3723 * gives direct access to the value 3724 */ 3725 public ActivityDefinition setProfileElement(CanonicalType value) { 3726 this.profile = value; 3727 return this; 3728 } 3729 3730 /** 3731 * @return A profile to which the target of the activity definition is expected 3732 * to conform. 3733 */ 3734 public String getProfile() { 3735 return this.profile == null ? null : this.profile.getValue(); 3736 } 3737 3738 /** 3739 * @param value A profile to which the target of the activity definition is 3740 * expected to conform. 3741 */ 3742 public ActivityDefinition setProfile(String value) { 3743 if (Utilities.noString(value)) 3744 this.profile = null; 3745 else { 3746 if (this.profile == null) 3747 this.profile = new CanonicalType(); 3748 this.profile.setValue(value); 3749 } 3750 return this; 3751 } 3752 3753 /** 3754 * @return {@link #code} (Detailed description of the type of activity; e.g. 3755 * What lab test, what procedure, what kind of encounter.) 3756 */ 3757 public CodeableConcept getCode() { 3758 if (this.code == null) 3759 if (Configuration.errorOnAutoCreate()) 3760 throw new Error("Attempt to auto-create ActivityDefinition.code"); 3761 else if (Configuration.doAutoCreate()) 3762 this.code = new CodeableConcept(); // cc 3763 return this.code; 3764 } 3765 3766 public boolean hasCode() { 3767 return this.code != null && !this.code.isEmpty(); 3768 } 3769 3770 /** 3771 * @param value {@link #code} (Detailed description of the type of activity; 3772 * e.g. What lab test, what procedure, what kind of encounter.) 3773 */ 3774 public ActivityDefinition setCode(CodeableConcept value) { 3775 this.code = value; 3776 return this; 3777 } 3778 3779 /** 3780 * @return {@link #intent} (Indicates the level of authority/intentionality 3781 * associated with the activity and where the request should fit into 3782 * the workflow chain.). This is the underlying object with id, value 3783 * and extensions. The accessor "getIntent" gives direct access to the 3784 * value 3785 */ 3786 public Enumeration<RequestIntent> getIntentElement() { 3787 if (this.intent == null) 3788 if (Configuration.errorOnAutoCreate()) 3789 throw new Error("Attempt to auto-create ActivityDefinition.intent"); 3790 else if (Configuration.doAutoCreate()) 3791 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 3792 return this.intent; 3793 } 3794 3795 public boolean hasIntentElement() { 3796 return this.intent != null && !this.intent.isEmpty(); 3797 } 3798 3799 public boolean hasIntent() { 3800 return this.intent != null && !this.intent.isEmpty(); 3801 } 3802 3803 /** 3804 * @param value {@link #intent} (Indicates the level of authority/intentionality 3805 * associated with the activity and where the request should fit 3806 * into the workflow chain.). This is the underlying object with 3807 * id, value and extensions. The accessor "getIntent" gives direct 3808 * access to the value 3809 */ 3810 public ActivityDefinition setIntentElement(Enumeration<RequestIntent> value) { 3811 this.intent = value; 3812 return this; 3813 } 3814 3815 /** 3816 * @return Indicates the level of authority/intentionality associated with the 3817 * activity and where the request should fit into the workflow chain. 3818 */ 3819 public RequestIntent getIntent() { 3820 return this.intent == null ? null : this.intent.getValue(); 3821 } 3822 3823 /** 3824 * @param value Indicates the level of authority/intentionality associated with 3825 * the activity and where the request should fit into the workflow 3826 * chain. 3827 */ 3828 public ActivityDefinition setIntent(RequestIntent value) { 3829 if (value == null) 3830 this.intent = null; 3831 else { 3832 if (this.intent == null) 3833 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 3834 this.intent.setValue(value); 3835 } 3836 return this; 3837 } 3838 3839 /** 3840 * @return {@link #priority} (Indicates how quickly the activity should be 3841 * addressed with respect to other requests.). This is the underlying 3842 * object with id, value and extensions. The accessor "getPriority" 3843 * gives direct access to the value 3844 */ 3845 public Enumeration<RequestPriority> getPriorityElement() { 3846 if (this.priority == null) 3847 if (Configuration.errorOnAutoCreate()) 3848 throw new Error("Attempt to auto-create ActivityDefinition.priority"); 3849 else if (Configuration.doAutoCreate()) 3850 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 3851 return this.priority; 3852 } 3853 3854 public boolean hasPriorityElement() { 3855 return this.priority != null && !this.priority.isEmpty(); 3856 } 3857 3858 public boolean hasPriority() { 3859 return this.priority != null && !this.priority.isEmpty(); 3860 } 3861 3862 /** 3863 * @param value {@link #priority} (Indicates how quickly the activity should be 3864 * addressed with respect to other requests.). This is the 3865 * underlying object with id, value and extensions. The accessor 3866 * "getPriority" gives direct access to the value 3867 */ 3868 public ActivityDefinition setPriorityElement(Enumeration<RequestPriority> value) { 3869 this.priority = value; 3870 return this; 3871 } 3872 3873 /** 3874 * @return Indicates how quickly the activity should be addressed with respect 3875 * to other requests. 3876 */ 3877 public RequestPriority getPriority() { 3878 return this.priority == null ? null : this.priority.getValue(); 3879 } 3880 3881 /** 3882 * @param value Indicates how quickly the activity should be addressed with 3883 * respect to other requests. 3884 */ 3885 public ActivityDefinition setPriority(RequestPriority value) { 3886 if (value == null) 3887 this.priority = null; 3888 else { 3889 if (this.priority == null) 3890 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 3891 this.priority.setValue(value); 3892 } 3893 return this; 3894 } 3895 3896 /** 3897 * @return {@link #doNotPerform} (Set this to true if the definition is to 3898 * indicate that a particular activity should NOT be performed. If true, 3899 * this element should be interpreted to reinforce a negative coding. 3900 * For example NPO as a code with a doNotPerform of true would still 3901 * indicate to NOT perform the action.). This is the underlying object 3902 * with id, value and extensions. The accessor "getDoNotPerform" gives 3903 * direct access to the value 3904 */ 3905 public BooleanType getDoNotPerformElement() { 3906 if (this.doNotPerform == null) 3907 if (Configuration.errorOnAutoCreate()) 3908 throw new Error("Attempt to auto-create ActivityDefinition.doNotPerform"); 3909 else if (Configuration.doAutoCreate()) 3910 this.doNotPerform = new BooleanType(); // bb 3911 return this.doNotPerform; 3912 } 3913 3914 public boolean hasDoNotPerformElement() { 3915 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 3916 } 3917 3918 public boolean hasDoNotPerform() { 3919 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 3920 } 3921 3922 /** 3923 * @param value {@link #doNotPerform} (Set this to true if the definition is to 3924 * indicate that a particular activity should NOT be performed. If 3925 * true, this element should be interpreted to reinforce a negative 3926 * coding. For example NPO as a code with a doNotPerform of true 3927 * would still indicate to NOT perform the action.). This is the 3928 * underlying object with id, value and extensions. The accessor 3929 * "getDoNotPerform" gives direct access to the value 3930 */ 3931 public ActivityDefinition setDoNotPerformElement(BooleanType value) { 3932 this.doNotPerform = value; 3933 return this; 3934 } 3935 3936 /** 3937 * @return Set this to true if the definition is to indicate that a particular 3938 * activity should NOT be performed. If true, this element should be 3939 * interpreted to reinforce a negative coding. For example NPO as a code 3940 * with a doNotPerform of true would still indicate to NOT perform the 3941 * action. 3942 */ 3943 public boolean getDoNotPerform() { 3944 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 3945 } 3946 3947 /** 3948 * @param value Set this to true if the definition is to indicate that a 3949 * particular activity should NOT be performed. If true, this 3950 * element should be interpreted to reinforce a negative coding. 3951 * For example NPO as a code with a doNotPerform of true would 3952 * still indicate to NOT perform the action. 3953 */ 3954 public ActivityDefinition setDoNotPerform(boolean value) { 3955 if (this.doNotPerform == null) 3956 this.doNotPerform = new BooleanType(); 3957 this.doNotPerform.setValue(value); 3958 return this; 3959 } 3960 3961 /** 3962 * @return {@link #timing} (The period, timing or frequency upon which the 3963 * described activity is to occur.) 3964 */ 3965 public Type getTiming() { 3966 return this.timing; 3967 } 3968 3969 /** 3970 * @return {@link #timing} (The period, timing or frequency upon which the 3971 * described activity is to occur.) 3972 */ 3973 public Timing getTimingTiming() throws FHIRException { 3974 if (this.timing == null) 3975 this.timing = new Timing(); 3976 if (!(this.timing instanceof Timing)) 3977 throw new FHIRException( 3978 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 3979 return (Timing) this.timing; 3980 } 3981 3982 public boolean hasTimingTiming() { 3983 return this != null && this.timing instanceof Timing; 3984 } 3985 3986 /** 3987 * @return {@link #timing} (The period, timing or frequency upon which the 3988 * described activity is to occur.) 3989 */ 3990 public DateTimeType getTimingDateTimeType() throws FHIRException { 3991 if (this.timing == null) 3992 this.timing = new DateTimeType(); 3993 if (!(this.timing instanceof DateTimeType)) 3994 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3995 + this.timing.getClass().getName() + " was encountered"); 3996 return (DateTimeType) this.timing; 3997 } 3998 3999 public boolean hasTimingDateTimeType() { 4000 return this != null && this.timing instanceof DateTimeType; 4001 } 4002 4003 /** 4004 * @return {@link #timing} (The period, timing or frequency upon which the 4005 * described activity is to occur.) 4006 */ 4007 public Age getTimingAge() throws FHIRException { 4008 if (this.timing == null) 4009 this.timing = new Age(); 4010 if (!(this.timing instanceof Age)) 4011 throw new FHIRException( 4012 "Type mismatch: the type Age was expected, but " + this.timing.getClass().getName() + " was encountered"); 4013 return (Age) this.timing; 4014 } 4015 4016 public boolean hasTimingAge() { 4017 return this != null && this.timing instanceof Age; 4018 } 4019 4020 /** 4021 * @return {@link #timing} (The period, timing or frequency upon which the 4022 * described activity is to occur.) 4023 */ 4024 public Period getTimingPeriod() throws FHIRException { 4025 if (this.timing == null) 4026 this.timing = new Period(); 4027 if (!(this.timing instanceof Period)) 4028 throw new FHIRException( 4029 "Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() + " was encountered"); 4030 return (Period) this.timing; 4031 } 4032 4033 public boolean hasTimingPeriod() { 4034 return this != null && this.timing instanceof Period; 4035 } 4036 4037 /** 4038 * @return {@link #timing} (The period, timing or frequency upon which the 4039 * described activity is to occur.) 4040 */ 4041 public Range getTimingRange() throws FHIRException { 4042 if (this.timing == null) 4043 this.timing = new Range(); 4044 if (!(this.timing instanceof Range)) 4045 throw new FHIRException( 4046 "Type mismatch: the type Range was expected, but " + this.timing.getClass().getName() + " was encountered"); 4047 return (Range) this.timing; 4048 } 4049 4050 public boolean hasTimingRange() { 4051 return this != null && this.timing instanceof Range; 4052 } 4053 4054 /** 4055 * @return {@link #timing} (The period, timing or frequency upon which the 4056 * described activity is to occur.) 4057 */ 4058 public Duration getTimingDuration() throws FHIRException { 4059 if (this.timing == null) 4060 this.timing = new Duration(); 4061 if (!(this.timing instanceof Duration)) 4062 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.timing.getClass().getName() 4063 + " was encountered"); 4064 return (Duration) this.timing; 4065 } 4066 4067 public boolean hasTimingDuration() { 4068 return this != null && this.timing instanceof Duration; 4069 } 4070 4071 public boolean hasTiming() { 4072 return this.timing != null && !this.timing.isEmpty(); 4073 } 4074 4075 /** 4076 * @param value {@link #timing} (The period, timing or frequency upon which the 4077 * described activity is to occur.) 4078 */ 4079 public ActivityDefinition setTiming(Type value) { 4080 if (value != null && !(value instanceof Timing || value instanceof DateTimeType || value instanceof Age 4081 || value instanceof Period || value instanceof Range || value instanceof Duration)) 4082 throw new Error("Not the right type for ActivityDefinition.timing[x]: " + value.fhirType()); 4083 this.timing = value; 4084 return this; 4085 } 4086 4087 /** 4088 * @return {@link #location} (Identifies the facility where the activity will 4089 * occur; e.g. home, hospital, specific clinic, etc.) 4090 */ 4091 public Reference getLocation() { 4092 if (this.location == null) 4093 if (Configuration.errorOnAutoCreate()) 4094 throw new Error("Attempt to auto-create ActivityDefinition.location"); 4095 else if (Configuration.doAutoCreate()) 4096 this.location = new Reference(); // cc 4097 return this.location; 4098 } 4099 4100 public boolean hasLocation() { 4101 return this.location != null && !this.location.isEmpty(); 4102 } 4103 4104 /** 4105 * @param value {@link #location} (Identifies the facility where the activity 4106 * will occur; e.g. home, hospital, specific clinic, etc.) 4107 */ 4108 public ActivityDefinition setLocation(Reference value) { 4109 this.location = value; 4110 return this; 4111 } 4112 4113 /** 4114 * @return {@link #location} The actual object that is the target of the 4115 * reference. The reference library doesn't populate this, but you can 4116 * use it to hold the resource if you resolve it. (Identifies the 4117 * facility where the activity will occur; e.g. home, hospital, specific 4118 * clinic, etc.) 4119 */ 4120 public Location getLocationTarget() { 4121 if (this.locationTarget == null) 4122 if (Configuration.errorOnAutoCreate()) 4123 throw new Error("Attempt to auto-create ActivityDefinition.location"); 4124 else if (Configuration.doAutoCreate()) 4125 this.locationTarget = new Location(); // aa 4126 return this.locationTarget; 4127 } 4128 4129 /** 4130 * @param value {@link #location} The actual object that is the target of the 4131 * reference. The reference library doesn't use these, but you can 4132 * use it to hold the resource if you resolve it. (Identifies the 4133 * facility where the activity will occur; e.g. home, hospital, 4134 * specific clinic, etc.) 4135 */ 4136 public ActivityDefinition setLocationTarget(Location value) { 4137 this.locationTarget = value; 4138 return this; 4139 } 4140 4141 /** 4142 * @return {@link #participant} (Indicates who should participate in performing 4143 * the action described.) 4144 */ 4145 public List<ActivityDefinitionParticipantComponent> getParticipant() { 4146 if (this.participant == null) 4147 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4148 return this.participant; 4149 } 4150 4151 /** 4152 * @return Returns a reference to <code>this</code> for easy method chaining 4153 */ 4154 public ActivityDefinition setParticipant(List<ActivityDefinitionParticipantComponent> theParticipant) { 4155 this.participant = theParticipant; 4156 return this; 4157 } 4158 4159 public boolean hasParticipant() { 4160 if (this.participant == null) 4161 return false; 4162 for (ActivityDefinitionParticipantComponent item : this.participant) 4163 if (!item.isEmpty()) 4164 return true; 4165 return false; 4166 } 4167 4168 public ActivityDefinitionParticipantComponent addParticipant() { // 3 4169 ActivityDefinitionParticipantComponent t = new ActivityDefinitionParticipantComponent(); 4170 if (this.participant == null) 4171 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4172 this.participant.add(t); 4173 return t; 4174 } 4175 4176 public ActivityDefinition addParticipant(ActivityDefinitionParticipantComponent t) { // 3 4177 if (t == null) 4178 return this; 4179 if (this.participant == null) 4180 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4181 this.participant.add(t); 4182 return this; 4183 } 4184 4185 /** 4186 * @return The first repetition of repeating field {@link #participant}, 4187 * creating it if it does not already exist 4188 */ 4189 public ActivityDefinitionParticipantComponent getParticipantFirstRep() { 4190 if (getParticipant().isEmpty()) { 4191 addParticipant(); 4192 } 4193 return getParticipant().get(0); 4194 } 4195 4196 /** 4197 * @return {@link #product} (Identifies the food, drug or other product being 4198 * consumed or supplied in the activity.) 4199 */ 4200 public Type getProduct() { 4201 return this.product; 4202 } 4203 4204 /** 4205 * @return {@link #product} (Identifies the food, drug or other product being 4206 * consumed or supplied in the activity.) 4207 */ 4208 public Reference getProductReference() throws FHIRException { 4209 if (this.product == null) 4210 this.product = new Reference(); 4211 if (!(this.product instanceof Reference)) 4212 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.product.getClass().getName() 4213 + " was encountered"); 4214 return (Reference) this.product; 4215 } 4216 4217 public boolean hasProductReference() { 4218 return this != null && this.product instanceof Reference; 4219 } 4220 4221 /** 4222 * @return {@link #product} (Identifies the food, drug or other product being 4223 * consumed or supplied in the activity.) 4224 */ 4225 public CodeableConcept getProductCodeableConcept() throws FHIRException { 4226 if (this.product == null) 4227 this.product = new CodeableConcept(); 4228 if (!(this.product instanceof CodeableConcept)) 4229 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 4230 + this.product.getClass().getName() + " was encountered"); 4231 return (CodeableConcept) this.product; 4232 } 4233 4234 public boolean hasProductCodeableConcept() { 4235 return this != null && this.product instanceof CodeableConcept; 4236 } 4237 4238 public boolean hasProduct() { 4239 return this.product != null && !this.product.isEmpty(); 4240 } 4241 4242 /** 4243 * @param value {@link #product} (Identifies the food, drug or other product 4244 * being consumed or supplied in the activity.) 4245 */ 4246 public ActivityDefinition setProduct(Type value) { 4247 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 4248 throw new Error("Not the right type for ActivityDefinition.product[x]: " + value.fhirType()); 4249 this.product = value; 4250 return this; 4251 } 4252 4253 /** 4254 * @return {@link #quantity} (Identifies the quantity expected to be consumed at 4255 * once (per dose, per meal, etc.).) 4256 */ 4257 public Quantity getQuantity() { 4258 if (this.quantity == null) 4259 if (Configuration.errorOnAutoCreate()) 4260 throw new Error("Attempt to auto-create ActivityDefinition.quantity"); 4261 else if (Configuration.doAutoCreate()) 4262 this.quantity = new Quantity(); // cc 4263 return this.quantity; 4264 } 4265 4266 public boolean hasQuantity() { 4267 return this.quantity != null && !this.quantity.isEmpty(); 4268 } 4269 4270 /** 4271 * @param value {@link #quantity} (Identifies the quantity expected to be 4272 * consumed at once (per dose, per meal, etc.).) 4273 */ 4274 public ActivityDefinition setQuantity(Quantity value) { 4275 this.quantity = value; 4276 return this; 4277 } 4278 4279 /** 4280 * @return {@link #dosage} (Provides detailed dosage instructions in the same 4281 * way that they are described for MedicationRequest resources.) 4282 */ 4283 public List<Dosage> getDosage() { 4284 if (this.dosage == null) 4285 this.dosage = new ArrayList<Dosage>(); 4286 return this.dosage; 4287 } 4288 4289 /** 4290 * @return Returns a reference to <code>this</code> for easy method chaining 4291 */ 4292 public ActivityDefinition setDosage(List<Dosage> theDosage) { 4293 this.dosage = theDosage; 4294 return this; 4295 } 4296 4297 public boolean hasDosage() { 4298 if (this.dosage == null) 4299 return false; 4300 for (Dosage item : this.dosage) 4301 if (!item.isEmpty()) 4302 return true; 4303 return false; 4304 } 4305 4306 public Dosage addDosage() { // 3 4307 Dosage t = new Dosage(); 4308 if (this.dosage == null) 4309 this.dosage = new ArrayList<Dosage>(); 4310 this.dosage.add(t); 4311 return t; 4312 } 4313 4314 public ActivityDefinition addDosage(Dosage t) { // 3 4315 if (t == null) 4316 return this; 4317 if (this.dosage == null) 4318 this.dosage = new ArrayList<Dosage>(); 4319 this.dosage.add(t); 4320 return this; 4321 } 4322 4323 /** 4324 * @return The first repetition of repeating field {@link #dosage}, creating it 4325 * if it does not already exist 4326 */ 4327 public Dosage getDosageFirstRep() { 4328 if (getDosage().isEmpty()) { 4329 addDosage(); 4330 } 4331 return getDosage().get(0); 4332 } 4333 4334 /** 4335 * @return {@link #bodySite} (Indicates the sites on the subject's body where 4336 * the procedure should be performed (I.e. the target sites).) 4337 */ 4338 public List<CodeableConcept> getBodySite() { 4339 if (this.bodySite == null) 4340 this.bodySite = new ArrayList<CodeableConcept>(); 4341 return this.bodySite; 4342 } 4343 4344 /** 4345 * @return Returns a reference to <code>this</code> for easy method chaining 4346 */ 4347 public ActivityDefinition setBodySite(List<CodeableConcept> theBodySite) { 4348 this.bodySite = theBodySite; 4349 return this; 4350 } 4351 4352 public boolean hasBodySite() { 4353 if (this.bodySite == null) 4354 return false; 4355 for (CodeableConcept item : this.bodySite) 4356 if (!item.isEmpty()) 4357 return true; 4358 return false; 4359 } 4360 4361 public CodeableConcept addBodySite() { // 3 4362 CodeableConcept t = new CodeableConcept(); 4363 if (this.bodySite == null) 4364 this.bodySite = new ArrayList<CodeableConcept>(); 4365 this.bodySite.add(t); 4366 return t; 4367 } 4368 4369 public ActivityDefinition addBodySite(CodeableConcept t) { // 3 4370 if (t == null) 4371 return this; 4372 if (this.bodySite == null) 4373 this.bodySite = new ArrayList<CodeableConcept>(); 4374 this.bodySite.add(t); 4375 return this; 4376 } 4377 4378 /** 4379 * @return The first repetition of repeating field {@link #bodySite}, creating 4380 * it if it does not already exist 4381 */ 4382 public CodeableConcept getBodySiteFirstRep() { 4383 if (getBodySite().isEmpty()) { 4384 addBodySite(); 4385 } 4386 return getBodySite().get(0); 4387 } 4388 4389 /** 4390 * @return {@link #specimenRequirement} (Defines specimen requirements for the 4391 * action to be performed, such as required specimens for a lab test.) 4392 */ 4393 public List<Reference> getSpecimenRequirement() { 4394 if (this.specimenRequirement == null) 4395 this.specimenRequirement = new ArrayList<Reference>(); 4396 return this.specimenRequirement; 4397 } 4398 4399 /** 4400 * @return Returns a reference to <code>this</code> for easy method chaining 4401 */ 4402 public ActivityDefinition setSpecimenRequirement(List<Reference> theSpecimenRequirement) { 4403 this.specimenRequirement = theSpecimenRequirement; 4404 return this; 4405 } 4406 4407 public boolean hasSpecimenRequirement() { 4408 if (this.specimenRequirement == null) 4409 return false; 4410 for (Reference item : this.specimenRequirement) 4411 if (!item.isEmpty()) 4412 return true; 4413 return false; 4414 } 4415 4416 public Reference addSpecimenRequirement() { // 3 4417 Reference t = new Reference(); 4418 if (this.specimenRequirement == null) 4419 this.specimenRequirement = new ArrayList<Reference>(); 4420 this.specimenRequirement.add(t); 4421 return t; 4422 } 4423 4424 public ActivityDefinition addSpecimenRequirement(Reference t) { // 3 4425 if (t == null) 4426 return this; 4427 if (this.specimenRequirement == null) 4428 this.specimenRequirement = new ArrayList<Reference>(); 4429 this.specimenRequirement.add(t); 4430 return this; 4431 } 4432 4433 /** 4434 * @return The first repetition of repeating field {@link #specimenRequirement}, 4435 * creating it if it does not already exist 4436 */ 4437 public Reference getSpecimenRequirementFirstRep() { 4438 if (getSpecimenRequirement().isEmpty()) { 4439 addSpecimenRequirement(); 4440 } 4441 return getSpecimenRequirement().get(0); 4442 } 4443 4444 /** 4445 * @deprecated Use Reference#setResource(IBaseResource) instead 4446 */ 4447 @Deprecated 4448 public List<SpecimenDefinition> getSpecimenRequirementTarget() { 4449 if (this.specimenRequirementTarget == null) 4450 this.specimenRequirementTarget = new ArrayList<SpecimenDefinition>(); 4451 return this.specimenRequirementTarget; 4452 } 4453 4454 /** 4455 * @deprecated Use Reference#setResource(IBaseResource) instead 4456 */ 4457 @Deprecated 4458 public SpecimenDefinition addSpecimenRequirementTarget() { 4459 SpecimenDefinition r = new SpecimenDefinition(); 4460 if (this.specimenRequirementTarget == null) 4461 this.specimenRequirementTarget = new ArrayList<SpecimenDefinition>(); 4462 this.specimenRequirementTarget.add(r); 4463 return r; 4464 } 4465 4466 /** 4467 * @return {@link #observationRequirement} (Defines observation requirements for 4468 * the action to be performed, such as body weight or surface area.) 4469 */ 4470 public List<Reference> getObservationRequirement() { 4471 if (this.observationRequirement == null) 4472 this.observationRequirement = new ArrayList<Reference>(); 4473 return this.observationRequirement; 4474 } 4475 4476 /** 4477 * @return Returns a reference to <code>this</code> for easy method chaining 4478 */ 4479 public ActivityDefinition setObservationRequirement(List<Reference> theObservationRequirement) { 4480 this.observationRequirement = theObservationRequirement; 4481 return this; 4482 } 4483 4484 public boolean hasObservationRequirement() { 4485 if (this.observationRequirement == null) 4486 return false; 4487 for (Reference item : this.observationRequirement) 4488 if (!item.isEmpty()) 4489 return true; 4490 return false; 4491 } 4492 4493 public Reference addObservationRequirement() { // 3 4494 Reference t = new Reference(); 4495 if (this.observationRequirement == null) 4496 this.observationRequirement = new ArrayList<Reference>(); 4497 this.observationRequirement.add(t); 4498 return t; 4499 } 4500 4501 public ActivityDefinition addObservationRequirement(Reference t) { // 3 4502 if (t == null) 4503 return this; 4504 if (this.observationRequirement == null) 4505 this.observationRequirement = new ArrayList<Reference>(); 4506 this.observationRequirement.add(t); 4507 return this; 4508 } 4509 4510 /** 4511 * @return The first repetition of repeating field 4512 * {@link #observationRequirement}, creating it if it does not already 4513 * exist 4514 */ 4515 public Reference getObservationRequirementFirstRep() { 4516 if (getObservationRequirement().isEmpty()) { 4517 addObservationRequirement(); 4518 } 4519 return getObservationRequirement().get(0); 4520 } 4521 4522 /** 4523 * @deprecated Use Reference#setResource(IBaseResource) instead 4524 */ 4525 @Deprecated 4526 public List<ObservationDefinition> getObservationRequirementTarget() { 4527 if (this.observationRequirementTarget == null) 4528 this.observationRequirementTarget = new ArrayList<ObservationDefinition>(); 4529 return this.observationRequirementTarget; 4530 } 4531 4532 /** 4533 * @deprecated Use Reference#setResource(IBaseResource) instead 4534 */ 4535 @Deprecated 4536 public ObservationDefinition addObservationRequirementTarget() { 4537 ObservationDefinition r = new ObservationDefinition(); 4538 if (this.observationRequirementTarget == null) 4539 this.observationRequirementTarget = new ArrayList<ObservationDefinition>(); 4540 this.observationRequirementTarget.add(r); 4541 return r; 4542 } 4543 4544 /** 4545 * @return {@link #observationResultRequirement} (Defines the observations that 4546 * are expected to be produced by the action.) 4547 */ 4548 public List<Reference> getObservationResultRequirement() { 4549 if (this.observationResultRequirement == null) 4550 this.observationResultRequirement = new ArrayList<Reference>(); 4551 return this.observationResultRequirement; 4552 } 4553 4554 /** 4555 * @return Returns a reference to <code>this</code> for easy method chaining 4556 */ 4557 public ActivityDefinition setObservationResultRequirement(List<Reference> theObservationResultRequirement) { 4558 this.observationResultRequirement = theObservationResultRequirement; 4559 return this; 4560 } 4561 4562 public boolean hasObservationResultRequirement() { 4563 if (this.observationResultRequirement == null) 4564 return false; 4565 for (Reference item : this.observationResultRequirement) 4566 if (!item.isEmpty()) 4567 return true; 4568 return false; 4569 } 4570 4571 public Reference addObservationResultRequirement() { // 3 4572 Reference t = new Reference(); 4573 if (this.observationResultRequirement == null) 4574 this.observationResultRequirement = new ArrayList<Reference>(); 4575 this.observationResultRequirement.add(t); 4576 return t; 4577 } 4578 4579 public ActivityDefinition addObservationResultRequirement(Reference t) { // 3 4580 if (t == null) 4581 return this; 4582 if (this.observationResultRequirement == null) 4583 this.observationResultRequirement = new ArrayList<Reference>(); 4584 this.observationResultRequirement.add(t); 4585 return this; 4586 } 4587 4588 /** 4589 * @return The first repetition of repeating field 4590 * {@link #observationResultRequirement}, creating it if it does not 4591 * already exist 4592 */ 4593 public Reference getObservationResultRequirementFirstRep() { 4594 if (getObservationResultRequirement().isEmpty()) { 4595 addObservationResultRequirement(); 4596 } 4597 return getObservationResultRequirement().get(0); 4598 } 4599 4600 /** 4601 * @deprecated Use Reference#setResource(IBaseResource) instead 4602 */ 4603 @Deprecated 4604 public List<ObservationDefinition> getObservationResultRequirementTarget() { 4605 if (this.observationResultRequirementTarget == null) 4606 this.observationResultRequirementTarget = new ArrayList<ObservationDefinition>(); 4607 return this.observationResultRequirementTarget; 4608 } 4609 4610 /** 4611 * @deprecated Use Reference#setResource(IBaseResource) instead 4612 */ 4613 @Deprecated 4614 public ObservationDefinition addObservationResultRequirementTarget() { 4615 ObservationDefinition r = new ObservationDefinition(); 4616 if (this.observationResultRequirementTarget == null) 4617 this.observationResultRequirementTarget = new ArrayList<ObservationDefinition>(); 4618 this.observationResultRequirementTarget.add(r); 4619 return r; 4620 } 4621 4622 /** 4623 * @return {@link #transform} (A reference to a StructureMap resource that 4624 * defines a transform that can be executed to produce the intent 4625 * resource using the ActivityDefinition instance as the input.). This 4626 * is the underlying object with id, value and extensions. The accessor 4627 * "getTransform" gives direct access to the value 4628 */ 4629 public CanonicalType getTransformElement() { 4630 if (this.transform == null) 4631 if (Configuration.errorOnAutoCreate()) 4632 throw new Error("Attempt to auto-create ActivityDefinition.transform"); 4633 else if (Configuration.doAutoCreate()) 4634 this.transform = new CanonicalType(); // bb 4635 return this.transform; 4636 } 4637 4638 public boolean hasTransformElement() { 4639 return this.transform != null && !this.transform.isEmpty(); 4640 } 4641 4642 public boolean hasTransform() { 4643 return this.transform != null && !this.transform.isEmpty(); 4644 } 4645 4646 /** 4647 * @param value {@link #transform} (A reference to a StructureMap resource that 4648 * defines a transform that can be executed to produce the intent 4649 * resource using the ActivityDefinition instance as the input.). 4650 * This is the underlying object with id, value and extensions. The 4651 * accessor "getTransform" gives direct access to the value 4652 */ 4653 public ActivityDefinition setTransformElement(CanonicalType value) { 4654 this.transform = value; 4655 return this; 4656 } 4657 4658 /** 4659 * @return A reference to a StructureMap resource that defines a transform that 4660 * can be executed to produce the intent resource using the 4661 * ActivityDefinition instance as the input. 4662 */ 4663 public String getTransform() { 4664 return this.transform == null ? null : this.transform.getValue(); 4665 } 4666 4667 /** 4668 * @param value A reference to a StructureMap resource that defines a transform 4669 * that can be executed to produce the intent resource using the 4670 * ActivityDefinition instance as the input. 4671 */ 4672 public ActivityDefinition setTransform(String value) { 4673 if (Utilities.noString(value)) 4674 this.transform = null; 4675 else { 4676 if (this.transform == null) 4677 this.transform = new CanonicalType(); 4678 this.transform.setValue(value); 4679 } 4680 return this; 4681 } 4682 4683 /** 4684 * @return {@link #dynamicValue} (Dynamic values that will be evaluated to 4685 * produce values for elements of the resulting resource. For example, 4686 * if the dosage of a medication must be computed based on the patient's 4687 * weight, a dynamic value would be used to specify an expression that 4688 * calculated the weight, and the path on the request resource that 4689 * would contain the result.) 4690 */ 4691 public List<ActivityDefinitionDynamicValueComponent> getDynamicValue() { 4692 if (this.dynamicValue == null) 4693 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4694 return this.dynamicValue; 4695 } 4696 4697 /** 4698 * @return Returns a reference to <code>this</code> for easy method chaining 4699 */ 4700 public ActivityDefinition setDynamicValue(List<ActivityDefinitionDynamicValueComponent> theDynamicValue) { 4701 this.dynamicValue = theDynamicValue; 4702 return this; 4703 } 4704 4705 public boolean hasDynamicValue() { 4706 if (this.dynamicValue == null) 4707 return false; 4708 for (ActivityDefinitionDynamicValueComponent item : this.dynamicValue) 4709 if (!item.isEmpty()) 4710 return true; 4711 return false; 4712 } 4713 4714 public ActivityDefinitionDynamicValueComponent addDynamicValue() { // 3 4715 ActivityDefinitionDynamicValueComponent t = new ActivityDefinitionDynamicValueComponent(); 4716 if (this.dynamicValue == null) 4717 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4718 this.dynamicValue.add(t); 4719 return t; 4720 } 4721 4722 public ActivityDefinition addDynamicValue(ActivityDefinitionDynamicValueComponent t) { // 3 4723 if (t == null) 4724 return this; 4725 if (this.dynamicValue == null) 4726 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4727 this.dynamicValue.add(t); 4728 return this; 4729 } 4730 4731 /** 4732 * @return The first repetition of repeating field {@link #dynamicValue}, 4733 * creating it if it does not already exist 4734 */ 4735 public ActivityDefinitionDynamicValueComponent getDynamicValueFirstRep() { 4736 if (getDynamicValue().isEmpty()) { 4737 addDynamicValue(); 4738 } 4739 return getDynamicValue().get(0); 4740 } 4741 4742 protected void listChildren(List<Property> children) { 4743 super.listChildren(children); 4744 children.add(new Property("url", "uri", 4745 "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.", 4746 0, 1, url)); 4747 children.add(new Property("identifier", "Identifier", 4748 "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4749 0, java.lang.Integer.MAX_VALUE, identifier)); 4750 children.add(new Property("version", "string", 4751 "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 4752 0, 1, version)); 4753 children.add(new Property("name", "string", 4754 "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4755 0, 1, name)); 4756 children.add(new Property("title", "string", 4757 "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title)); 4758 children.add(new Property("subtitle", "string", 4759 "An explanatory or alternate title for the activity definition giving additional information about its content.", 4760 0, 1, subtitle)); 4761 children.add(new Property("status", "code", 4762 "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 4763 children.add(new Property("experimental", "boolean", 4764 "A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4765 0, 1, experimental)); 4766 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4767 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4768 subject)); 4769 children.add(new Property("date", "dateTime", 4770 "The date (and optionally time) when the activity definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 4771 0, 1, date)); 4772 children.add(new Property("publisher", "string", 4773 "The name of the organization or individual that published the activity definition.", 0, 1, publisher)); 4774 children.add(new Property("contact", "ContactDetail", 4775 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4776 java.lang.Integer.MAX_VALUE, contact)); 4777 children.add(new Property("description", "markdown", 4778 "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, 4779 description)); 4780 children.add(new Property("useContext", "UsageContext", 4781 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.", 4782 0, java.lang.Integer.MAX_VALUE, useContext)); 4783 children.add(new Property("jurisdiction", "CodeableConcept", 4784 "A legal or geographic region in which the activity definition is intended to be used.", 0, 4785 java.lang.Integer.MAX_VALUE, jurisdiction)); 4786 children.add(new Property("purpose", "markdown", 4787 "Explanation of why this activity definition is needed and why it has been designed as it has.", 0, 1, 4788 purpose)); 4789 children.add(new Property("usage", "string", 4790 "A detailed description of how the activity definition is used from a clinical perspective.", 0, 1, usage)); 4791 children.add(new Property("copyright", "markdown", 4792 "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 4793 0, 1, copyright)); 4794 children.add(new Property("approvalDate", "date", 4795 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4796 0, 1, approvalDate)); 4797 children.add(new Property("lastReviewDate", "date", 4798 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4799 0, 1, lastReviewDate)); 4800 children.add(new Property("effectivePeriod", "Period", 4801 "The period during which the activity definition content was or is planned to be in active use.", 0, 1, 4802 effectivePeriod)); 4803 children.add(new Property("topic", "CodeableConcept", 4804 "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 4805 0, java.lang.Integer.MAX_VALUE, topic)); 4806 children.add(new Property("author", "ContactDetail", 4807 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4808 java.lang.Integer.MAX_VALUE, author)); 4809 children.add(new Property("editor", "ContactDetail", 4810 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4811 java.lang.Integer.MAX_VALUE, editor)); 4812 children.add(new Property("reviewer", "ContactDetail", 4813 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4814 java.lang.Integer.MAX_VALUE, reviewer)); 4815 children.add(new Property("endorser", "ContactDetail", 4816 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4817 java.lang.Integer.MAX_VALUE, endorser)); 4818 children.add(new Property("relatedArtifact", "RelatedArtifact", 4819 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4820 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4821 children.add(new Property("library", "canonical(Library)", 4822 "A reference to a Library resource containing any formal logic used by the activity definition.", 0, 4823 java.lang.Integer.MAX_VALUE, library)); 4824 children.add(new Property("kind", "code", 4825 "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.", 4826 0, 1, kind)); 4827 children.add(new Property("profile", "canonical(StructureDefinition)", 4828 "A profile to which the target of the activity definition is expected to conform.", 0, 1, profile)); 4829 children.add(new Property("code", "CodeableConcept", 4830 "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 4831 1, code)); 4832 children.add(new Property("intent", "code", 4833 "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.", 4834 0, 1, intent)); 4835 children.add(new Property("priority", "code", 4836 "Indicates how quickly the activity should be addressed with respect to other requests.", 0, 1, priority)); 4837 children.add(new Property("doNotPerform", "boolean", 4838 "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.", 4839 0, 1, doNotPerform)); 4840 children.add(new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 4841 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing)); 4842 children.add(new Property("location", "Reference(Location)", 4843 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 4844 location)); 4845 children.add(new Property("participant", "", "Indicates who should participate in performing the action described.", 4846 0, java.lang.Integer.MAX_VALUE, participant)); 4847 children.add(new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 4848 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product)); 4849 children.add(new Property("quantity", "SimpleQuantity", 4850 "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity)); 4851 children.add(new Property("dosage", "Dosage", 4852 "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 4853 0, java.lang.Integer.MAX_VALUE, dosage)); 4854 children.add(new Property("bodySite", "CodeableConcept", 4855 "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, 4856 java.lang.Integer.MAX_VALUE, bodySite)); 4857 children.add(new Property("specimenRequirement", "Reference(SpecimenDefinition)", 4858 "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.", 0, 4859 java.lang.Integer.MAX_VALUE, specimenRequirement)); 4860 children.add(new Property("observationRequirement", "Reference(ObservationDefinition)", 4861 "Defines observation requirements for the action to be performed, such as body weight or surface area.", 0, 4862 java.lang.Integer.MAX_VALUE, observationRequirement)); 4863 children.add(new Property("observationResultRequirement", "Reference(ObservationDefinition)", 4864 "Defines the observations that are expected to be produced by the action.", 0, java.lang.Integer.MAX_VALUE, 4865 observationResultRequirement)); 4866 children.add(new Property("transform", "canonical(StructureMap)", 4867 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 4868 0, 1, transform)); 4869 children.add(new Property("dynamicValue", "", 4870 "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.", 4871 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 4872 } 4873 4874 @Override 4875 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4876 switch (_hash) { 4877 case 116079: 4878 /* url */ return new Property("url", "uri", 4879 "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.", 4880 0, 1, url); 4881 case -1618432855: 4882 /* identifier */ return new Property("identifier", "Identifier", 4883 "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4884 0, java.lang.Integer.MAX_VALUE, identifier); 4885 case 351608024: 4886 /* version */ return new Property("version", "string", 4887 "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 4888 0, 1, version); 4889 case 3373707: 4890 /* name */ return new Property("name", "string", 4891 "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4892 0, 1, name); 4893 case 110371416: 4894 /* title */ return new Property("title", "string", 4895 "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title); 4896 case -2060497896: 4897 /* subtitle */ return new Property("subtitle", "string", 4898 "An explanatory or alternate title for the activity definition giving additional information about its content.", 4899 0, 1, subtitle); 4900 case -892481550: 4901 /* status */ return new Property("status", "code", 4902 "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status); 4903 case -404562712: 4904 /* experimental */ return new Property("experimental", "boolean", 4905 "A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4906 0, 1, experimental); 4907 case -573640748: 4908 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4909 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4910 subject); 4911 case -1867885268: 4912 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4913 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4914 subject); 4915 case -1257122603: 4916 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4917 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4918 subject); 4919 case 772938623: 4920 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4921 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4922 subject); 4923 case 3076014: 4924 /* date */ return new Property("date", "dateTime", 4925 "The date (and optionally time) when the activity definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 4926 0, 1, date); 4927 case 1447404028: 4928 /* publisher */ return new Property("publisher", "string", 4929 "The name of the organization or individual that published the activity definition.", 0, 1, publisher); 4930 case 951526432: 4931 /* contact */ return new Property("contact", "ContactDetail", 4932 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4933 java.lang.Integer.MAX_VALUE, contact); 4934 case -1724546052: 4935 /* description */ return new Property("description", "markdown", 4936 "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, 4937 description); 4938 case -669707736: 4939 /* useContext */ return new Property("useContext", "UsageContext", 4940 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.", 4941 0, java.lang.Integer.MAX_VALUE, useContext); 4942 case -507075711: 4943 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4944 "A legal or geographic region in which the activity definition is intended to be used.", 0, 4945 java.lang.Integer.MAX_VALUE, jurisdiction); 4946 case -220463842: 4947 /* purpose */ return new Property("purpose", "markdown", 4948 "Explanation of why this activity definition is needed and why it has been designed as it has.", 0, 1, 4949 purpose); 4950 case 111574433: 4951 /* usage */ return new Property("usage", "string", 4952 "A detailed description of how the activity definition is used from a clinical perspective.", 0, 1, usage); 4953 case 1522889671: 4954 /* copyright */ return new Property("copyright", "markdown", 4955 "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 4956 0, 1, copyright); 4957 case 223539345: 4958 /* approvalDate */ return new Property("approvalDate", "date", 4959 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4960 0, 1, approvalDate); 4961 case -1687512484: 4962 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4963 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4964 0, 1, lastReviewDate); 4965 case -403934648: 4966 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4967 "The period during which the activity definition content was or is planned to be in active use.", 0, 1, 4968 effectivePeriod); 4969 case 110546223: 4970 /* topic */ return new Property("topic", "CodeableConcept", 4971 "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 4972 0, java.lang.Integer.MAX_VALUE, topic); 4973 case -1406328437: 4974 /* author */ return new Property("author", "ContactDetail", 4975 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4976 java.lang.Integer.MAX_VALUE, author); 4977 case -1307827859: 4978 /* editor */ return new Property("editor", "ContactDetail", 4979 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4980 java.lang.Integer.MAX_VALUE, editor); 4981 case -261190139: 4982 /* reviewer */ return new Property("reviewer", "ContactDetail", 4983 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4984 java.lang.Integer.MAX_VALUE, reviewer); 4985 case 1740277666: 4986 /* endorser */ return new Property("endorser", "ContactDetail", 4987 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4988 java.lang.Integer.MAX_VALUE, endorser); 4989 case 666807069: 4990 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4991 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4992 java.lang.Integer.MAX_VALUE, relatedArtifact); 4993 case 166208699: 4994 /* library */ return new Property("library", "canonical(Library)", 4995 "A reference to a Library resource containing any formal logic used by the activity definition.", 0, 4996 java.lang.Integer.MAX_VALUE, library); 4997 case 3292052: 4998 /* kind */ return new Property("kind", "code", 4999 "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.", 5000 0, 1, kind); 5001 case -309425751: 5002 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 5003 "A profile to which the target of the activity definition is expected to conform.", 0, 1, profile); 5004 case 3059181: 5005 /* code */ return new Property("code", "CodeableConcept", 5006 "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 5007 0, 1, code); 5008 case -1183762788: 5009 /* intent */ return new Property("intent", "code", 5010 "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.", 5011 0, 1, intent); 5012 case -1165461084: 5013 /* priority */ return new Property("priority", "code", 5014 "Indicates how quickly the activity should be addressed with respect to other requests.", 0, 1, priority); 5015 case -1788508167: 5016 /* doNotPerform */ return new Property("doNotPerform", "boolean", 5017 "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.", 5018 0, 1, doNotPerform); 5019 case 164632566: 5020 /* timing[x] */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5021 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5022 case -873664438: 5023 /* timing */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5024 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5025 case -497554124: 5026 /* timingTiming */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5027 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5028 case -1837458939: 5029 /* timingDateTime */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5030 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5031 case 164607061: 5032 /* timingAge */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5033 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5034 case -615615829: 5035 /* timingPeriod */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5036 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5037 case -710871277: 5038 /* timingRange */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5039 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5040 case -1327253506: 5041 /* timingDuration */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5042 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5043 case 1901043637: 5044 /* location */ return new Property("location", "Reference(Location)", 5045 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 5046 location); 5047 case 767422259: 5048 /* participant */ return new Property("participant", "", 5049 "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, 5050 participant); 5051 case 1753005361: 5052 /* product[x] */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5053 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5054 case -309474065: 5055 /* product */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5056 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5057 case -669667556: 5058 /* productReference */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5059 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5060 case 906854066: 5061 /* productCodeableConcept */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5062 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5063 case -1285004149: 5064 /* quantity */ return new Property("quantity", "SimpleQuantity", 5065 "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity); 5066 case -1326018889: 5067 /* dosage */ return new Property("dosage", "Dosage", 5068 "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 5069 0, java.lang.Integer.MAX_VALUE, dosage); 5070 case 1702620169: 5071 /* bodySite */ return new Property("bodySite", "CodeableConcept", 5072 "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 5073 0, java.lang.Integer.MAX_VALUE, bodySite); 5074 case 1498467355: 5075 /* specimenRequirement */ return new Property("specimenRequirement", "Reference(SpecimenDefinition)", 5076 "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.", 0, 5077 java.lang.Integer.MAX_VALUE, specimenRequirement); 5078 case 362354807: 5079 /* observationRequirement */ return new Property("observationRequirement", "Reference(ObservationDefinition)", 5080 "Defines observation requirements for the action to be performed, such as body weight or surface area.", 0, 5081 java.lang.Integer.MAX_VALUE, observationRequirement); 5082 case 395230490: 5083 /* observationResultRequirement */ return new Property("observationResultRequirement", 5084 "Reference(ObservationDefinition)", 5085 "Defines the observations that are expected to be produced by the action.", 0, java.lang.Integer.MAX_VALUE, 5086 observationResultRequirement); 5087 case 1052666732: 5088 /* transform */ return new Property("transform", "canonical(StructureMap)", 5089 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 5090 0, 1, transform); 5091 case 572625010: 5092 /* dynamicValue */ return new Property("dynamicValue", "", 5093 "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.", 5094 0, java.lang.Integer.MAX_VALUE, dynamicValue); 5095 default: 5096 return super.getNamedProperty(_hash, _name, _checkValid); 5097 } 5098 5099 } 5100 5101 @Override 5102 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5103 switch (hash) { 5104 case 116079: 5105 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5106 case -1618432855: 5107 /* identifier */ return this.identifier == null ? new Base[0] 5108 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5109 case 351608024: 5110 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5111 case 3373707: 5112 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5113 case 110371416: 5114 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5115 case -2060497896: 5116 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 5117 case -892481550: 5118 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5119 case -404562712: 5120 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 5121 case -1867885268: 5122 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 5123 case 3076014: 5124 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5125 case 1447404028: 5126 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5127 case 951526432: 5128 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5129 case -1724546052: 5130 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5131 case -669707736: 5132 /* useContext */ return this.useContext == null ? new Base[0] 5133 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5134 case -507075711: 5135 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5136 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5137 case -220463842: 5138 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5139 case 111574433: 5140 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 5141 case 1522889671: 5142 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5143 case 223539345: 5144 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 5145 case -1687512484: 5146 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 5147 case -403934648: 5148 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 5149 case 110546223: 5150 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 5151 case -1406328437: 5152 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5153 case -1307827859: 5154 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5155 case -261190139: 5156 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5157 case 1740277666: 5158 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5159 case 666807069: 5160 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 5161 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5162 case 166208699: 5163 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 5164 case 3292052: 5165 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<ActivityDefinitionKind> 5166 case -309425751: 5167 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 5168 case 3059181: 5169 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 5170 case -1183762788: 5171 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<RequestIntent> 5172 case -1165461084: 5173 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 5174 case -1788508167: 5175 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 5176 case -873664438: 5177 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 5178 case 1901043637: 5179 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 5180 case 767422259: 5181 /* participant */ return this.participant == null ? new Base[0] 5182 : this.participant.toArray(new Base[this.participant.size()]); // ActivityDefinitionParticipantComponent 5183 case -309474065: 5184 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // Type 5185 case -1285004149: 5186 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5187 case -1326018889: 5188 /* dosage */ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 5189 case 1702620169: 5190 /* bodySite */ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 5191 case 1498467355: 5192 /* specimenRequirement */ return this.specimenRequirement == null ? new Base[0] 5193 : this.specimenRequirement.toArray(new Base[this.specimenRequirement.size()]); // Reference 5194 case 362354807: 5195 /* observationRequirement */ return this.observationRequirement == null ? new Base[0] 5196 : this.observationRequirement.toArray(new Base[this.observationRequirement.size()]); // Reference 5197 case 395230490: 5198 /* observationResultRequirement */ return this.observationResultRequirement == null ? new Base[0] 5199 : this.observationResultRequirement.toArray(new Base[this.observationResultRequirement.size()]); // Reference 5200 case 1052666732: 5201 /* transform */ return this.transform == null ? new Base[0] : new Base[] { this.transform }; // CanonicalType 5202 case 572625010: 5203 /* dynamicValue */ return this.dynamicValue == null ? new Base[0] 5204 : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // ActivityDefinitionDynamicValueComponent 5205 default: 5206 return super.getProperty(hash, name, checkValid); 5207 } 5208 5209 } 5210 5211 @Override 5212 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5213 switch (hash) { 5214 case 116079: // url 5215 this.url = castToUri(value); // UriType 5216 return value; 5217 case -1618432855: // identifier 5218 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5219 return value; 5220 case 351608024: // version 5221 this.version = castToString(value); // StringType 5222 return value; 5223 case 3373707: // name 5224 this.name = castToString(value); // StringType 5225 return value; 5226 case 110371416: // title 5227 this.title = castToString(value); // StringType 5228 return value; 5229 case -2060497896: // subtitle 5230 this.subtitle = castToString(value); // StringType 5231 return value; 5232 case -892481550: // status 5233 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5234 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5235 return value; 5236 case -404562712: // experimental 5237 this.experimental = castToBoolean(value); // BooleanType 5238 return value; 5239 case -1867885268: // subject 5240 this.subject = castToType(value); // Type 5241 return value; 5242 case 3076014: // date 5243 this.date = castToDateTime(value); // DateTimeType 5244 return value; 5245 case 1447404028: // publisher 5246 this.publisher = castToString(value); // StringType 5247 return value; 5248 case 951526432: // contact 5249 this.getContact().add(castToContactDetail(value)); // ContactDetail 5250 return value; 5251 case -1724546052: // description 5252 this.description = castToMarkdown(value); // MarkdownType 5253 return value; 5254 case -669707736: // useContext 5255 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5256 return value; 5257 case -507075711: // jurisdiction 5258 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5259 return value; 5260 case -220463842: // purpose 5261 this.purpose = castToMarkdown(value); // MarkdownType 5262 return value; 5263 case 111574433: // usage 5264 this.usage = castToString(value); // StringType 5265 return value; 5266 case 1522889671: // copyright 5267 this.copyright = castToMarkdown(value); // MarkdownType 5268 return value; 5269 case 223539345: // approvalDate 5270 this.approvalDate = castToDate(value); // DateType 5271 return value; 5272 case -1687512484: // lastReviewDate 5273 this.lastReviewDate = castToDate(value); // DateType 5274 return value; 5275 case -403934648: // effectivePeriod 5276 this.effectivePeriod = castToPeriod(value); // Period 5277 return value; 5278 case 110546223: // topic 5279 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 5280 return value; 5281 case -1406328437: // author 5282 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 5283 return value; 5284 case -1307827859: // editor 5285 this.getEditor().add(castToContactDetail(value)); // ContactDetail 5286 return value; 5287 case -261190139: // reviewer 5288 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 5289 return value; 5290 case 1740277666: // endorser 5291 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 5292 return value; 5293 case 666807069: // relatedArtifact 5294 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 5295 return value; 5296 case 166208699: // library 5297 this.getLibrary().add(castToCanonical(value)); // CanonicalType 5298 return value; 5299 case 3292052: // kind 5300 value = new ActivityDefinitionKindEnumFactory().fromType(castToCode(value)); 5301 this.kind = (Enumeration) value; // Enumeration<ActivityDefinitionKind> 5302 return value; 5303 case -309425751: // profile 5304 this.profile = castToCanonical(value); // CanonicalType 5305 return value; 5306 case 3059181: // code 5307 this.code = castToCodeableConcept(value); // CodeableConcept 5308 return value; 5309 case -1183762788: // intent 5310 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5311 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5312 return value; 5313 case -1165461084: // priority 5314 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5315 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5316 return value; 5317 case -1788508167: // doNotPerform 5318 this.doNotPerform = castToBoolean(value); // BooleanType 5319 return value; 5320 case -873664438: // timing 5321 this.timing = castToType(value); // Type 5322 return value; 5323 case 1901043637: // location 5324 this.location = castToReference(value); // Reference 5325 return value; 5326 case 767422259: // participant 5327 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); // ActivityDefinitionParticipantComponent 5328 return value; 5329 case -309474065: // product 5330 this.product = castToType(value); // Type 5331 return value; 5332 case -1285004149: // quantity 5333 this.quantity = castToQuantity(value); // Quantity 5334 return value; 5335 case -1326018889: // dosage 5336 this.getDosage().add(castToDosage(value)); // Dosage 5337 return value; 5338 case 1702620169: // bodySite 5339 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 5340 return value; 5341 case 1498467355: // specimenRequirement 5342 this.getSpecimenRequirement().add(castToReference(value)); // Reference 5343 return value; 5344 case 362354807: // observationRequirement 5345 this.getObservationRequirement().add(castToReference(value)); // Reference 5346 return value; 5347 case 395230490: // observationResultRequirement 5348 this.getObservationResultRequirement().add(castToReference(value)); // Reference 5349 return value; 5350 case 1052666732: // transform 5351 this.transform = castToCanonical(value); // CanonicalType 5352 return value; 5353 case 572625010: // dynamicValue 5354 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); // ActivityDefinitionDynamicValueComponent 5355 return value; 5356 default: 5357 return super.setProperty(hash, name, value); 5358 } 5359 5360 } 5361 5362 @Override 5363 public Base setProperty(String name, Base value) throws FHIRException { 5364 if (name.equals("url")) { 5365 this.url = castToUri(value); // UriType 5366 } else if (name.equals("identifier")) { 5367 this.getIdentifier().add(castToIdentifier(value)); 5368 } else if (name.equals("version")) { 5369 this.version = castToString(value); // StringType 5370 } else if (name.equals("name")) { 5371 this.name = castToString(value); // StringType 5372 } else if (name.equals("title")) { 5373 this.title = castToString(value); // StringType 5374 } else if (name.equals("subtitle")) { 5375 this.subtitle = castToString(value); // StringType 5376 } else if (name.equals("status")) { 5377 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5378 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5379 } else if (name.equals("experimental")) { 5380 this.experimental = castToBoolean(value); // BooleanType 5381 } else if (name.equals("subject[x]")) { 5382 this.subject = castToType(value); // Type 5383 } else if (name.equals("date")) { 5384 this.date = castToDateTime(value); // DateTimeType 5385 } else if (name.equals("publisher")) { 5386 this.publisher = castToString(value); // StringType 5387 } else if (name.equals("contact")) { 5388 this.getContact().add(castToContactDetail(value)); 5389 } else if (name.equals("description")) { 5390 this.description = castToMarkdown(value); // MarkdownType 5391 } else if (name.equals("useContext")) { 5392 this.getUseContext().add(castToUsageContext(value)); 5393 } else if (name.equals("jurisdiction")) { 5394 this.getJurisdiction().add(castToCodeableConcept(value)); 5395 } else if (name.equals("purpose")) { 5396 this.purpose = castToMarkdown(value); // MarkdownType 5397 } else if (name.equals("usage")) { 5398 this.usage = castToString(value); // StringType 5399 } else if (name.equals("copyright")) { 5400 this.copyright = castToMarkdown(value); // MarkdownType 5401 } else if (name.equals("approvalDate")) { 5402 this.approvalDate = castToDate(value); // DateType 5403 } else if (name.equals("lastReviewDate")) { 5404 this.lastReviewDate = castToDate(value); // DateType 5405 } else if (name.equals("effectivePeriod")) { 5406 this.effectivePeriod = castToPeriod(value); // Period 5407 } else if (name.equals("topic")) { 5408 this.getTopic().add(castToCodeableConcept(value)); 5409 } else if (name.equals("author")) { 5410 this.getAuthor().add(castToContactDetail(value)); 5411 } else if (name.equals("editor")) { 5412 this.getEditor().add(castToContactDetail(value)); 5413 } else if (name.equals("reviewer")) { 5414 this.getReviewer().add(castToContactDetail(value)); 5415 } else if (name.equals("endorser")) { 5416 this.getEndorser().add(castToContactDetail(value)); 5417 } else if (name.equals("relatedArtifact")) { 5418 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 5419 } else if (name.equals("library")) { 5420 this.getLibrary().add(castToCanonical(value)); 5421 } else if (name.equals("kind")) { 5422 value = new ActivityDefinitionKindEnumFactory().fromType(castToCode(value)); 5423 this.kind = (Enumeration) value; // Enumeration<ActivityDefinitionKind> 5424 } else if (name.equals("profile")) { 5425 this.profile = castToCanonical(value); // CanonicalType 5426 } else if (name.equals("code")) { 5427 this.code = castToCodeableConcept(value); // CodeableConcept 5428 } else if (name.equals("intent")) { 5429 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5430 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5431 } else if (name.equals("priority")) { 5432 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5433 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5434 } else if (name.equals("doNotPerform")) { 5435 this.doNotPerform = castToBoolean(value); // BooleanType 5436 } else if (name.equals("timing[x]")) { 5437 this.timing = castToType(value); // Type 5438 } else if (name.equals("location")) { 5439 this.location = castToReference(value); // Reference 5440 } else if (name.equals("participant")) { 5441 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); 5442 } else if (name.equals("product[x]")) { 5443 this.product = castToType(value); // Type 5444 } else if (name.equals("quantity")) { 5445 this.quantity = castToQuantity(value); // Quantity 5446 } else if (name.equals("dosage")) { 5447 this.getDosage().add(castToDosage(value)); 5448 } else if (name.equals("bodySite")) { 5449 this.getBodySite().add(castToCodeableConcept(value)); 5450 } else if (name.equals("specimenRequirement")) { 5451 this.getSpecimenRequirement().add(castToReference(value)); 5452 } else if (name.equals("observationRequirement")) { 5453 this.getObservationRequirement().add(castToReference(value)); 5454 } else if (name.equals("observationResultRequirement")) { 5455 this.getObservationResultRequirement().add(castToReference(value)); 5456 } else if (name.equals("transform")) { 5457 this.transform = castToCanonical(value); // CanonicalType 5458 } else if (name.equals("dynamicValue")) { 5459 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); 5460 } else 5461 return super.setProperty(name, value); 5462 return value; 5463 } 5464 5465 @Override 5466 public void removeChild(String name, Base value) throws FHIRException { 5467 if (name.equals("url")) { 5468 this.url = null; 5469 } else if (name.equals("identifier")) { 5470 this.getIdentifier().remove(castToIdentifier(value)); 5471 } else if (name.equals("version")) { 5472 this.version = null; 5473 } else if (name.equals("name")) { 5474 this.name = null; 5475 } else if (name.equals("title")) { 5476 this.title = null; 5477 } else if (name.equals("subtitle")) { 5478 this.subtitle = null; 5479 } else if (name.equals("status")) { 5480 this.status = null; 5481 } else if (name.equals("experimental")) { 5482 this.experimental = null; 5483 } else if (name.equals("subject[x]")) { 5484 this.subject = null; 5485 } else if (name.equals("date")) { 5486 this.date = null; 5487 } else if (name.equals("publisher")) { 5488 this.publisher = null; 5489 } else if (name.equals("contact")) { 5490 this.getContact().remove(castToContactDetail(value)); 5491 } else if (name.equals("description")) { 5492 this.description = null; 5493 } else if (name.equals("useContext")) { 5494 this.getUseContext().remove(castToUsageContext(value)); 5495 } else if (name.equals("jurisdiction")) { 5496 this.getJurisdiction().remove(castToCodeableConcept(value)); 5497 } else if (name.equals("purpose")) { 5498 this.purpose = null; 5499 } else if (name.equals("usage")) { 5500 this.usage = null; 5501 } else if (name.equals("copyright")) { 5502 this.copyright = null; 5503 } else if (name.equals("approvalDate")) { 5504 this.approvalDate = null; 5505 } else if (name.equals("lastReviewDate")) { 5506 this.lastReviewDate = null; 5507 } else if (name.equals("effectivePeriod")) { 5508 this.effectivePeriod = null; 5509 } else if (name.equals("topic")) { 5510 this.getTopic().remove(castToCodeableConcept(value)); 5511 } else if (name.equals("author")) { 5512 this.getAuthor().remove(castToContactDetail(value)); 5513 } else if (name.equals("editor")) { 5514 this.getEditor().remove(castToContactDetail(value)); 5515 } else if (name.equals("reviewer")) { 5516 this.getReviewer().remove(castToContactDetail(value)); 5517 } else if (name.equals("endorser")) { 5518 this.getEndorser().remove(castToContactDetail(value)); 5519 } else if (name.equals("relatedArtifact")) { 5520 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 5521 } else if (name.equals("library")) { 5522 this.getLibrary().remove(castToCanonical(value)); 5523 } else if (name.equals("kind")) { 5524 this.kind = null; 5525 } else if (name.equals("profile")) { 5526 this.profile = null; 5527 } else if (name.equals("code")) { 5528 this.code = null; 5529 } else if (name.equals("intent")) { 5530 this.intent = null; 5531 } else if (name.equals("priority")) { 5532 this.priority = null; 5533 } else if (name.equals("doNotPerform")) { 5534 this.doNotPerform = null; 5535 } else if (name.equals("timing[x]")) { 5536 this.timing = null; 5537 } else if (name.equals("location")) { 5538 this.location = null; 5539 } else if (name.equals("participant")) { 5540 this.getParticipant().remove((ActivityDefinitionParticipantComponent) value); 5541 } else if (name.equals("product[x]")) { 5542 this.product = null; 5543 } else if (name.equals("quantity")) { 5544 this.quantity = null; 5545 } else if (name.equals("dosage")) { 5546 this.getDosage().remove(castToDosage(value)); 5547 } else if (name.equals("bodySite")) { 5548 this.getBodySite().remove(castToCodeableConcept(value)); 5549 } else if (name.equals("specimenRequirement")) { 5550 this.getSpecimenRequirement().remove(castToReference(value)); 5551 } else if (name.equals("observationRequirement")) { 5552 this.getObservationRequirement().remove(castToReference(value)); 5553 } else if (name.equals("observationResultRequirement")) { 5554 this.getObservationResultRequirement().remove(castToReference(value)); 5555 } else if (name.equals("transform")) { 5556 this.transform = null; 5557 } else if (name.equals("dynamicValue")) { 5558 this.getDynamicValue().remove((ActivityDefinitionDynamicValueComponent) value); 5559 } else 5560 super.removeChild(name, value); 5561 5562 } 5563 5564 @Override 5565 public Base makeProperty(int hash, String name) throws FHIRException { 5566 switch (hash) { 5567 case 116079: 5568 return getUrlElement(); 5569 case -1618432855: 5570 return addIdentifier(); 5571 case 351608024: 5572 return getVersionElement(); 5573 case 3373707: 5574 return getNameElement(); 5575 case 110371416: 5576 return getTitleElement(); 5577 case -2060497896: 5578 return getSubtitleElement(); 5579 case -892481550: 5580 return getStatusElement(); 5581 case -404562712: 5582 return getExperimentalElement(); 5583 case -573640748: 5584 return getSubject(); 5585 case -1867885268: 5586 return getSubject(); 5587 case 3076014: 5588 return getDateElement(); 5589 case 1447404028: 5590 return getPublisherElement(); 5591 case 951526432: 5592 return addContact(); 5593 case -1724546052: 5594 return getDescriptionElement(); 5595 case -669707736: 5596 return addUseContext(); 5597 case -507075711: 5598 return addJurisdiction(); 5599 case -220463842: 5600 return getPurposeElement(); 5601 case 111574433: 5602 return getUsageElement(); 5603 case 1522889671: 5604 return getCopyrightElement(); 5605 case 223539345: 5606 return getApprovalDateElement(); 5607 case -1687512484: 5608 return getLastReviewDateElement(); 5609 case -403934648: 5610 return getEffectivePeriod(); 5611 case 110546223: 5612 return addTopic(); 5613 case -1406328437: 5614 return addAuthor(); 5615 case -1307827859: 5616 return addEditor(); 5617 case -261190139: 5618 return addReviewer(); 5619 case 1740277666: 5620 return addEndorser(); 5621 case 666807069: 5622 return addRelatedArtifact(); 5623 case 166208699: 5624 return addLibraryElement(); 5625 case 3292052: 5626 return getKindElement(); 5627 case -309425751: 5628 return getProfileElement(); 5629 case 3059181: 5630 return getCode(); 5631 case -1183762788: 5632 return getIntentElement(); 5633 case -1165461084: 5634 return getPriorityElement(); 5635 case -1788508167: 5636 return getDoNotPerformElement(); 5637 case 164632566: 5638 return getTiming(); 5639 case -873664438: 5640 return getTiming(); 5641 case 1901043637: 5642 return getLocation(); 5643 case 767422259: 5644 return addParticipant(); 5645 case 1753005361: 5646 return getProduct(); 5647 case -309474065: 5648 return getProduct(); 5649 case -1285004149: 5650 return getQuantity(); 5651 case -1326018889: 5652 return addDosage(); 5653 case 1702620169: 5654 return addBodySite(); 5655 case 1498467355: 5656 return addSpecimenRequirement(); 5657 case 362354807: 5658 return addObservationRequirement(); 5659 case 395230490: 5660 return addObservationResultRequirement(); 5661 case 1052666732: 5662 return getTransformElement(); 5663 case 572625010: 5664 return addDynamicValue(); 5665 default: 5666 return super.makeProperty(hash, name); 5667 } 5668 5669 } 5670 5671 @Override 5672 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5673 switch (hash) { 5674 case 116079: 5675 /* url */ return new String[] { "uri" }; 5676 case -1618432855: 5677 /* identifier */ return new String[] { "Identifier" }; 5678 case 351608024: 5679 /* version */ return new String[] { "string" }; 5680 case 3373707: 5681 /* name */ return new String[] { "string" }; 5682 case 110371416: 5683 /* title */ return new String[] { "string" }; 5684 case -2060497896: 5685 /* subtitle */ return new String[] { "string" }; 5686 case -892481550: 5687 /* status */ return new String[] { "code" }; 5688 case -404562712: 5689 /* experimental */ return new String[] { "boolean" }; 5690 case -1867885268: 5691 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 5692 case 3076014: 5693 /* date */ return new String[] { "dateTime" }; 5694 case 1447404028: 5695 /* publisher */ return new String[] { "string" }; 5696 case 951526432: 5697 /* contact */ return new String[] { "ContactDetail" }; 5698 case -1724546052: 5699 /* description */ return new String[] { "markdown" }; 5700 case -669707736: 5701 /* useContext */ return new String[] { "UsageContext" }; 5702 case -507075711: 5703 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5704 case -220463842: 5705 /* purpose */ return new String[] { "markdown" }; 5706 case 111574433: 5707 /* usage */ return new String[] { "string" }; 5708 case 1522889671: 5709 /* copyright */ return new String[] { "markdown" }; 5710 case 223539345: 5711 /* approvalDate */ return new String[] { "date" }; 5712 case -1687512484: 5713 /* lastReviewDate */ return new String[] { "date" }; 5714 case -403934648: 5715 /* effectivePeriod */ return new String[] { "Period" }; 5716 case 110546223: 5717 /* topic */ return new String[] { "CodeableConcept" }; 5718 case -1406328437: 5719 /* author */ return new String[] { "ContactDetail" }; 5720 case -1307827859: 5721 /* editor */ return new String[] { "ContactDetail" }; 5722 case -261190139: 5723 /* reviewer */ return new String[] { "ContactDetail" }; 5724 case 1740277666: 5725 /* endorser */ return new String[] { "ContactDetail" }; 5726 case 666807069: 5727 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 5728 case 166208699: 5729 /* library */ return new String[] { "canonical" }; 5730 case 3292052: 5731 /* kind */ return new String[] { "code" }; 5732 case -309425751: 5733 /* profile */ return new String[] { "canonical" }; 5734 case 3059181: 5735 /* code */ return new String[] { "CodeableConcept" }; 5736 case -1183762788: 5737 /* intent */ return new String[] { "code" }; 5738 case -1165461084: 5739 /* priority */ return new String[] { "code" }; 5740 case -1788508167: 5741 /* doNotPerform */ return new String[] { "boolean" }; 5742 case -873664438: 5743 /* timing */ return new String[] { "Timing", "dateTime", "Age", "Period", "Range", "Duration" }; 5744 case 1901043637: 5745 /* location */ return new String[] { "Reference" }; 5746 case 767422259: 5747 /* participant */ return new String[] {}; 5748 case -309474065: 5749 /* product */ return new String[] { "Reference", "CodeableConcept" }; 5750 case -1285004149: 5751 /* quantity */ return new String[] { "SimpleQuantity" }; 5752 case -1326018889: 5753 /* dosage */ return new String[] { "Dosage" }; 5754 case 1702620169: 5755 /* bodySite */ return new String[] { "CodeableConcept" }; 5756 case 1498467355: 5757 /* specimenRequirement */ return new String[] { "Reference" }; 5758 case 362354807: 5759 /* observationRequirement */ return new String[] { "Reference" }; 5760 case 395230490: 5761 /* observationResultRequirement */ return new String[] { "Reference" }; 5762 case 1052666732: 5763 /* transform */ return new String[] { "canonical" }; 5764 case 572625010: 5765 /* dynamicValue */ return new String[] {}; 5766 default: 5767 return super.getTypesForProperty(hash, name); 5768 } 5769 5770 } 5771 5772 @Override 5773 public Base addChild(String name) throws FHIRException { 5774 if (name.equals("url")) { 5775 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.url"); 5776 } else if (name.equals("identifier")) { 5777 return addIdentifier(); 5778 } else if (name.equals("version")) { 5779 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.version"); 5780 } else if (name.equals("name")) { 5781 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.name"); 5782 } else if (name.equals("title")) { 5783 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.title"); 5784 } else if (name.equals("subtitle")) { 5785 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.subtitle"); 5786 } else if (name.equals("status")) { 5787 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.status"); 5788 } else if (name.equals("experimental")) { 5789 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.experimental"); 5790 } else if (name.equals("subjectCodeableConcept")) { 5791 this.subject = new CodeableConcept(); 5792 return this.subject; 5793 } else if (name.equals("subjectReference")) { 5794 this.subject = new Reference(); 5795 return this.subject; 5796 } else if (name.equals("date")) { 5797 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.date"); 5798 } else if (name.equals("publisher")) { 5799 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.publisher"); 5800 } else if (name.equals("contact")) { 5801 return addContact(); 5802 } else if (name.equals("description")) { 5803 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.description"); 5804 } else if (name.equals("useContext")) { 5805 return addUseContext(); 5806 } else if (name.equals("jurisdiction")) { 5807 return addJurisdiction(); 5808 } else if (name.equals("purpose")) { 5809 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.purpose"); 5810 } else if (name.equals("usage")) { 5811 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.usage"); 5812 } else if (name.equals("copyright")) { 5813 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.copyright"); 5814 } else if (name.equals("approvalDate")) { 5815 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.approvalDate"); 5816 } else if (name.equals("lastReviewDate")) { 5817 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.lastReviewDate"); 5818 } else if (name.equals("effectivePeriod")) { 5819 this.effectivePeriod = new Period(); 5820 return this.effectivePeriod; 5821 } else if (name.equals("topic")) { 5822 return addTopic(); 5823 } else if (name.equals("author")) { 5824 return addAuthor(); 5825 } else if (name.equals("editor")) { 5826 return addEditor(); 5827 } else if (name.equals("reviewer")) { 5828 return addReviewer(); 5829 } else if (name.equals("endorser")) { 5830 return addEndorser(); 5831 } else if (name.equals("relatedArtifact")) { 5832 return addRelatedArtifact(); 5833 } else if (name.equals("library")) { 5834 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.library"); 5835 } else if (name.equals("kind")) { 5836 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.kind"); 5837 } else if (name.equals("profile")) { 5838 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.profile"); 5839 } else if (name.equals("code")) { 5840 this.code = new CodeableConcept(); 5841 return this.code; 5842 } else if (name.equals("intent")) { 5843 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.intent"); 5844 } else if (name.equals("priority")) { 5845 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.priority"); 5846 } else if (name.equals("doNotPerform")) { 5847 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.doNotPerform"); 5848 } else if (name.equals("timingTiming")) { 5849 this.timing = new Timing(); 5850 return this.timing; 5851 } else if (name.equals("timingDateTime")) { 5852 this.timing = new DateTimeType(); 5853 return this.timing; 5854 } else if (name.equals("timingAge")) { 5855 this.timing = new Age(); 5856 return this.timing; 5857 } else if (name.equals("timingPeriod")) { 5858 this.timing = new Period(); 5859 return this.timing; 5860 } else if (name.equals("timingRange")) { 5861 this.timing = new Range(); 5862 return this.timing; 5863 } else if (name.equals("timingDuration")) { 5864 this.timing = new Duration(); 5865 return this.timing; 5866 } else if (name.equals("location")) { 5867 this.location = new Reference(); 5868 return this.location; 5869 } else if (name.equals("participant")) { 5870 return addParticipant(); 5871 } else if (name.equals("productReference")) { 5872 this.product = new Reference(); 5873 return this.product; 5874 } else if (name.equals("productCodeableConcept")) { 5875 this.product = new CodeableConcept(); 5876 return this.product; 5877 } else if (name.equals("quantity")) { 5878 this.quantity = new Quantity(); 5879 return this.quantity; 5880 } else if (name.equals("dosage")) { 5881 return addDosage(); 5882 } else if (name.equals("bodySite")) { 5883 return addBodySite(); 5884 } else if (name.equals("specimenRequirement")) { 5885 return addSpecimenRequirement(); 5886 } else if (name.equals("observationRequirement")) { 5887 return addObservationRequirement(); 5888 } else if (name.equals("observationResultRequirement")) { 5889 return addObservationResultRequirement(); 5890 } else if (name.equals("transform")) { 5891 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.transform"); 5892 } else if (name.equals("dynamicValue")) { 5893 return addDynamicValue(); 5894 } else 5895 return super.addChild(name); 5896 } 5897 5898 public String fhirType() { 5899 return "ActivityDefinition"; 5900 5901 } 5902 5903 public ActivityDefinition copy() { 5904 ActivityDefinition dst = new ActivityDefinition(); 5905 copyValues(dst); 5906 return dst; 5907 } 5908 5909 public void copyValues(ActivityDefinition dst) { 5910 super.copyValues(dst); 5911 dst.url = url == null ? null : url.copy(); 5912 if (identifier != null) { 5913 dst.identifier = new ArrayList<Identifier>(); 5914 for (Identifier i : identifier) 5915 dst.identifier.add(i.copy()); 5916 } 5917 ; 5918 dst.version = version == null ? null : version.copy(); 5919 dst.name = name == null ? null : name.copy(); 5920 dst.title = title == null ? null : title.copy(); 5921 dst.subtitle = subtitle == null ? null : subtitle.copy(); 5922 dst.status = status == null ? null : status.copy(); 5923 dst.experimental = experimental == null ? null : experimental.copy(); 5924 dst.subject = subject == null ? null : subject.copy(); 5925 dst.date = date == null ? null : date.copy(); 5926 dst.publisher = publisher == null ? null : publisher.copy(); 5927 if (contact != null) { 5928 dst.contact = new ArrayList<ContactDetail>(); 5929 for (ContactDetail i : contact) 5930 dst.contact.add(i.copy()); 5931 } 5932 ; 5933 dst.description = description == null ? null : description.copy(); 5934 if (useContext != null) { 5935 dst.useContext = new ArrayList<UsageContext>(); 5936 for (UsageContext i : useContext) 5937 dst.useContext.add(i.copy()); 5938 } 5939 ; 5940 if (jurisdiction != null) { 5941 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5942 for (CodeableConcept i : jurisdiction) 5943 dst.jurisdiction.add(i.copy()); 5944 } 5945 ; 5946 dst.purpose = purpose == null ? null : purpose.copy(); 5947 dst.usage = usage == null ? null : usage.copy(); 5948 dst.copyright = copyright == null ? null : copyright.copy(); 5949 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5950 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5951 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5952 if (topic != null) { 5953 dst.topic = new ArrayList<CodeableConcept>(); 5954 for (CodeableConcept i : topic) 5955 dst.topic.add(i.copy()); 5956 } 5957 ; 5958 if (author != null) { 5959 dst.author = new ArrayList<ContactDetail>(); 5960 for (ContactDetail i : author) 5961 dst.author.add(i.copy()); 5962 } 5963 ; 5964 if (editor != null) { 5965 dst.editor = new ArrayList<ContactDetail>(); 5966 for (ContactDetail i : editor) 5967 dst.editor.add(i.copy()); 5968 } 5969 ; 5970 if (reviewer != null) { 5971 dst.reviewer = new ArrayList<ContactDetail>(); 5972 for (ContactDetail i : reviewer) 5973 dst.reviewer.add(i.copy()); 5974 } 5975 ; 5976 if (endorser != null) { 5977 dst.endorser = new ArrayList<ContactDetail>(); 5978 for (ContactDetail i : endorser) 5979 dst.endorser.add(i.copy()); 5980 } 5981 ; 5982 if (relatedArtifact != null) { 5983 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5984 for (RelatedArtifact i : relatedArtifact) 5985 dst.relatedArtifact.add(i.copy()); 5986 } 5987 ; 5988 if (library != null) { 5989 dst.library = new ArrayList<CanonicalType>(); 5990 for (CanonicalType i : library) 5991 dst.library.add(i.copy()); 5992 } 5993 ; 5994 dst.kind = kind == null ? null : kind.copy(); 5995 dst.profile = profile == null ? null : profile.copy(); 5996 dst.code = code == null ? null : code.copy(); 5997 dst.intent = intent == null ? null : intent.copy(); 5998 dst.priority = priority == null ? null : priority.copy(); 5999 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 6000 dst.timing = timing == null ? null : timing.copy(); 6001 dst.location = location == null ? null : location.copy(); 6002 if (participant != null) { 6003 dst.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 6004 for (ActivityDefinitionParticipantComponent i : participant) 6005 dst.participant.add(i.copy()); 6006 } 6007 ; 6008 dst.product = product == null ? null : product.copy(); 6009 dst.quantity = quantity == null ? null : quantity.copy(); 6010 if (dosage != null) { 6011 dst.dosage = new ArrayList<Dosage>(); 6012 for (Dosage i : dosage) 6013 dst.dosage.add(i.copy()); 6014 } 6015 ; 6016 if (bodySite != null) { 6017 dst.bodySite = new ArrayList<CodeableConcept>(); 6018 for (CodeableConcept i : bodySite) 6019 dst.bodySite.add(i.copy()); 6020 } 6021 ; 6022 if (specimenRequirement != null) { 6023 dst.specimenRequirement = new ArrayList<Reference>(); 6024 for (Reference i : specimenRequirement) 6025 dst.specimenRequirement.add(i.copy()); 6026 } 6027 ; 6028 if (observationRequirement != null) { 6029 dst.observationRequirement = new ArrayList<Reference>(); 6030 for (Reference i : observationRequirement) 6031 dst.observationRequirement.add(i.copy()); 6032 } 6033 ; 6034 if (observationResultRequirement != null) { 6035 dst.observationResultRequirement = new ArrayList<Reference>(); 6036 for (Reference i : observationResultRequirement) 6037 dst.observationResultRequirement.add(i.copy()); 6038 } 6039 ; 6040 dst.transform = transform == null ? null : transform.copy(); 6041 if (dynamicValue != null) { 6042 dst.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 6043 for (ActivityDefinitionDynamicValueComponent i : dynamicValue) 6044 dst.dynamicValue.add(i.copy()); 6045 } 6046 ; 6047 } 6048 6049 protected ActivityDefinition typedCopy() { 6050 return copy(); 6051 } 6052 6053 @Override 6054 public boolean equalsDeep(Base other_) { 6055 if (!super.equalsDeep(other_)) 6056 return false; 6057 if (!(other_ instanceof ActivityDefinition)) 6058 return false; 6059 ActivityDefinition o = (ActivityDefinition) other_; 6060 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 6061 && compareDeep(subject, o.subject, true) && compareDeep(purpose, o.purpose, true) 6062 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 6063 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 6064 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 6065 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 6066 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 6067 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 6068 && compareDeep(kind, o.kind, true) && compareDeep(profile, o.profile, true) && compareDeep(code, o.code, true) 6069 && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 6070 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(timing, o.timing, true) 6071 && compareDeep(location, o.location, true) && compareDeep(participant, o.participant, true) 6072 && compareDeep(product, o.product, true) && compareDeep(quantity, o.quantity, true) 6073 && compareDeep(dosage, o.dosage, true) && compareDeep(bodySite, o.bodySite, true) 6074 && compareDeep(specimenRequirement, o.specimenRequirement, true) 6075 && compareDeep(observationRequirement, o.observationRequirement, true) 6076 && compareDeep(observationResultRequirement, o.observationResultRequirement, true) 6077 && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true); 6078 } 6079 6080 @Override 6081 public boolean equalsShallow(Base other_) { 6082 if (!super.equalsShallow(other_)) 6083 return false; 6084 if (!(other_ instanceof ActivityDefinition)) 6085 return false; 6086 ActivityDefinition o = (ActivityDefinition) other_; 6087 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 6088 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 6089 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 6090 && compareValues(kind, o.kind, true) && compareValues(intent, o.intent, true) 6091 && compareValues(priority, o.priority, true) && compareValues(doNotPerform, o.doNotPerform, true); 6092 } 6093 6094 public boolean isEmpty() { 6095 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, subject, purpose, usage, 6096 copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, 6097 relatedArtifact, library, kind, profile, code, intent, priority, doNotPerform, timing, location, participant, 6098 product, quantity, dosage, bodySite, specimenRequirement, observationRequirement, observationResultRequirement, 6099 transform, dynamicValue); 6100 } 6101 6102 @Override 6103 public ResourceType getResourceType() { 6104 return ResourceType.ActivityDefinition; 6105 } 6106 6107 /** 6108 * Search parameter: <b>date</b> 6109 * <p> 6110 * Description: <b>The activity definition publication date</b><br> 6111 * Type: <b>date</b><br> 6112 * Path: <b>ActivityDefinition.date</b><br> 6113 * </p> 6114 */ 6115 @SearchParamDefinition(name = "date", path = "ActivityDefinition.date", description = "The activity definition publication date", type = "date") 6116 public static final String SP_DATE = "date"; 6117 /** 6118 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6119 * <p> 6120 * Description: <b>The activity definition publication date</b><br> 6121 * Type: <b>date</b><br> 6122 * Path: <b>ActivityDefinition.date</b><br> 6123 * </p> 6124 */ 6125 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6126 SP_DATE); 6127 6128 /** 6129 * Search parameter: <b>identifier</b> 6130 * <p> 6131 * Description: <b>External identifier for the activity definition</b><br> 6132 * Type: <b>token</b><br> 6133 * Path: <b>ActivityDefinition.identifier</b><br> 6134 * </p> 6135 */ 6136 @SearchParamDefinition(name = "identifier", path = "ActivityDefinition.identifier", description = "External identifier for the activity definition", type = "token") 6137 public static final String SP_IDENTIFIER = "identifier"; 6138 /** 6139 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6140 * <p> 6141 * Description: <b>External identifier for the activity definition</b><br> 6142 * Type: <b>token</b><br> 6143 * Path: <b>ActivityDefinition.identifier</b><br> 6144 * </p> 6145 */ 6146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6147 SP_IDENTIFIER); 6148 6149 /** 6150 * Search parameter: <b>successor</b> 6151 * <p> 6152 * Description: <b>What resource is being referenced</b><br> 6153 * Type: <b>reference</b><br> 6154 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6155 * </p> 6156 */ 6157 @SearchParamDefinition(name = "successor", path = "ActivityDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 6158 public static final String SP_SUCCESSOR = "successor"; 6159 /** 6160 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 6161 * <p> 6162 * Description: <b>What resource is being referenced</b><br> 6163 * Type: <b>reference</b><br> 6164 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6165 * </p> 6166 */ 6167 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6168 SP_SUCCESSOR); 6169 6170 /** 6171 * Constant for fluent queries to be used to add include statements. Specifies 6172 * the path value of "<b>ActivityDefinition:successor</b>". 6173 */ 6174 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 6175 "ActivityDefinition:successor").toLocked(); 6176 6177 /** 6178 * Search parameter: <b>context-type-value</b> 6179 * <p> 6180 * Description: <b>A use context type and value assigned to the activity 6181 * definition</b><br> 6182 * Type: <b>composite</b><br> 6183 * Path: <b></b><br> 6184 * </p> 6185 */ 6186 @SearchParamDefinition(name = "context-type-value", path = "ActivityDefinition.useContext", description = "A use context type and value assigned to the activity definition", type = "composite", compositeOf = { 6187 "context-type", "context" }) 6188 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6189 /** 6190 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6191 * <p> 6192 * Description: <b>A use context type and value assigned to the activity 6193 * definition</b><br> 6194 * Type: <b>composite</b><br> 6195 * Path: <b></b><br> 6196 * </p> 6197 */ 6198 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 6199 SP_CONTEXT_TYPE_VALUE); 6200 6201 /** 6202 * Search parameter: <b>jurisdiction</b> 6203 * <p> 6204 * Description: <b>Intended jurisdiction for the activity definition</b><br> 6205 * Type: <b>token</b><br> 6206 * Path: <b>ActivityDefinition.jurisdiction</b><br> 6207 * </p> 6208 */ 6209 @SearchParamDefinition(name = "jurisdiction", path = "ActivityDefinition.jurisdiction", description = "Intended jurisdiction for the activity definition", type = "token") 6210 public static final String SP_JURISDICTION = "jurisdiction"; 6211 /** 6212 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6213 * <p> 6214 * Description: <b>Intended jurisdiction for the activity definition</b><br> 6215 * Type: <b>token</b><br> 6216 * Path: <b>ActivityDefinition.jurisdiction</b><br> 6217 * </p> 6218 */ 6219 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6220 SP_JURISDICTION); 6221 6222 /** 6223 * Search parameter: <b>description</b> 6224 * <p> 6225 * Description: <b>The description of the activity definition</b><br> 6226 * Type: <b>string</b><br> 6227 * Path: <b>ActivityDefinition.description</b><br> 6228 * </p> 6229 */ 6230 @SearchParamDefinition(name = "description", path = "ActivityDefinition.description", description = "The description of the activity definition", type = "string") 6231 public static final String SP_DESCRIPTION = "description"; 6232 /** 6233 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6234 * <p> 6235 * Description: <b>The description of the activity definition</b><br> 6236 * Type: <b>string</b><br> 6237 * Path: <b>ActivityDefinition.description</b><br> 6238 * </p> 6239 */ 6240 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 6241 SP_DESCRIPTION); 6242 6243 /** 6244 * Search parameter: <b>derived-from</b> 6245 * <p> 6246 * Description: <b>What resource is being referenced</b><br> 6247 * Type: <b>reference</b><br> 6248 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6249 * </p> 6250 */ 6251 @SearchParamDefinition(name = "derived-from", path = "ActivityDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 6252 public static final String SP_DERIVED_FROM = "derived-from"; 6253 /** 6254 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 6255 * <p> 6256 * Description: <b>What resource is being referenced</b><br> 6257 * Type: <b>reference</b><br> 6258 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6259 * </p> 6260 */ 6261 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6262 SP_DERIVED_FROM); 6263 6264 /** 6265 * Constant for fluent queries to be used to add include statements. Specifies 6266 * the path value of "<b>ActivityDefinition:derived-from</b>". 6267 */ 6268 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 6269 "ActivityDefinition:derived-from").toLocked(); 6270 6271 /** 6272 * Search parameter: <b>context-type</b> 6273 * <p> 6274 * Description: <b>A type of use context assigned to the activity 6275 * definition</b><br> 6276 * Type: <b>token</b><br> 6277 * Path: <b>ActivityDefinition.useContext.code</b><br> 6278 * </p> 6279 */ 6280 @SearchParamDefinition(name = "context-type", path = "ActivityDefinition.useContext.code", description = "A type of use context assigned to the activity definition", type = "token") 6281 public static final String SP_CONTEXT_TYPE = "context-type"; 6282 /** 6283 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6284 * <p> 6285 * Description: <b>A type of use context assigned to the activity 6286 * definition</b><br> 6287 * Type: <b>token</b><br> 6288 * Path: <b>ActivityDefinition.useContext.code</b><br> 6289 * </p> 6290 */ 6291 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6292 SP_CONTEXT_TYPE); 6293 6294 /** 6295 * Search parameter: <b>predecessor</b> 6296 * <p> 6297 * Description: <b>What resource is being referenced</b><br> 6298 * Type: <b>reference</b><br> 6299 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6300 * </p> 6301 */ 6302 @SearchParamDefinition(name = "predecessor", path = "ActivityDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 6303 public static final String SP_PREDECESSOR = "predecessor"; 6304 /** 6305 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6306 * <p> 6307 * Description: <b>What resource is being referenced</b><br> 6308 * Type: <b>reference</b><br> 6309 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6310 * </p> 6311 */ 6312 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6313 SP_PREDECESSOR); 6314 6315 /** 6316 * Constant for fluent queries to be used to add include statements. Specifies 6317 * the path value of "<b>ActivityDefinition:predecessor</b>". 6318 */ 6319 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 6320 "ActivityDefinition:predecessor").toLocked(); 6321 6322 /** 6323 * Search parameter: <b>title</b> 6324 * <p> 6325 * Description: <b>The human-friendly name of the activity definition</b><br> 6326 * Type: <b>string</b><br> 6327 * Path: <b>ActivityDefinition.title</b><br> 6328 * </p> 6329 */ 6330 @SearchParamDefinition(name = "title", path = "ActivityDefinition.title", description = "The human-friendly name of the activity definition", type = "string") 6331 public static final String SP_TITLE = "title"; 6332 /** 6333 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6334 * <p> 6335 * Description: <b>The human-friendly name of the activity definition</b><br> 6336 * Type: <b>string</b><br> 6337 * Path: <b>ActivityDefinition.title</b><br> 6338 * </p> 6339 */ 6340 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 6341 SP_TITLE); 6342 6343 /** 6344 * Search parameter: <b>composed-of</b> 6345 * <p> 6346 * Description: <b>What resource is being referenced</b><br> 6347 * Type: <b>reference</b><br> 6348 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6349 * </p> 6350 */ 6351 @SearchParamDefinition(name = "composed-of", path = "ActivityDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 6352 public static final String SP_COMPOSED_OF = "composed-of"; 6353 /** 6354 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 6355 * <p> 6356 * Description: <b>What resource is being referenced</b><br> 6357 * Type: <b>reference</b><br> 6358 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6359 * </p> 6360 */ 6361 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6362 SP_COMPOSED_OF); 6363 6364 /** 6365 * Constant for fluent queries to be used to add include statements. Specifies 6366 * the path value of "<b>ActivityDefinition:composed-of</b>". 6367 */ 6368 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 6369 "ActivityDefinition:composed-of").toLocked(); 6370 6371 /** 6372 * Search parameter: <b>version</b> 6373 * <p> 6374 * Description: <b>The business version of the activity definition</b><br> 6375 * Type: <b>token</b><br> 6376 * Path: <b>ActivityDefinition.version</b><br> 6377 * </p> 6378 */ 6379 @SearchParamDefinition(name = "version", path = "ActivityDefinition.version", description = "The business version of the activity definition", type = "token") 6380 public static final String SP_VERSION = "version"; 6381 /** 6382 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6383 * <p> 6384 * Description: <b>The business version of the activity definition</b><br> 6385 * Type: <b>token</b><br> 6386 * Path: <b>ActivityDefinition.version</b><br> 6387 * </p> 6388 */ 6389 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6390 SP_VERSION); 6391 6392 /** 6393 * Search parameter: <b>url</b> 6394 * <p> 6395 * Description: <b>The uri that identifies the activity definition</b><br> 6396 * Type: <b>uri</b><br> 6397 * Path: <b>ActivityDefinition.url</b><br> 6398 * </p> 6399 */ 6400 @SearchParamDefinition(name = "url", path = "ActivityDefinition.url", description = "The uri that identifies the activity definition", type = "uri") 6401 public static final String SP_URL = "url"; 6402 /** 6403 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6404 * <p> 6405 * Description: <b>The uri that identifies the activity definition</b><br> 6406 * Type: <b>uri</b><br> 6407 * Path: <b>ActivityDefinition.url</b><br> 6408 * </p> 6409 */ 6410 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6411 6412 /** 6413 * Search parameter: <b>context-quantity</b> 6414 * <p> 6415 * Description: <b>A quantity- or range-valued use context assigned to the 6416 * activity definition</b><br> 6417 * Type: <b>quantity</b><br> 6418 * Path: <b>ActivityDefinition.useContext.valueQuantity, 6419 * ActivityDefinition.useContext.valueRange</b><br> 6420 * </p> 6421 */ 6422 @SearchParamDefinition(name = "context-quantity", path = "(ActivityDefinition.useContext.value as Quantity) | (ActivityDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the activity definition", type = "quantity") 6423 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6424 /** 6425 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6426 * <p> 6427 * Description: <b>A quantity- or range-valued use context assigned to the 6428 * activity definition</b><br> 6429 * Type: <b>quantity</b><br> 6430 * Path: <b>ActivityDefinition.useContext.valueQuantity, 6431 * ActivityDefinition.useContext.valueRange</b><br> 6432 * </p> 6433 */ 6434 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6435 SP_CONTEXT_QUANTITY); 6436 6437 /** 6438 * Search parameter: <b>effective</b> 6439 * <p> 6440 * Description: <b>The time during which the activity definition is intended to 6441 * be in use</b><br> 6442 * Type: <b>date</b><br> 6443 * Path: <b>ActivityDefinition.effectivePeriod</b><br> 6444 * </p> 6445 */ 6446 @SearchParamDefinition(name = "effective", path = "ActivityDefinition.effectivePeriod", description = "The time during which the activity definition is intended to be in use", type = "date") 6447 public static final String SP_EFFECTIVE = "effective"; 6448 /** 6449 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6450 * <p> 6451 * Description: <b>The time during which the activity definition is intended to 6452 * be in use</b><br> 6453 * Type: <b>date</b><br> 6454 * Path: <b>ActivityDefinition.effectivePeriod</b><br> 6455 * </p> 6456 */ 6457 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6458 SP_EFFECTIVE); 6459 6460 /** 6461 * Search parameter: <b>depends-on</b> 6462 * <p> 6463 * Description: <b>What resource is being referenced</b><br> 6464 * Type: <b>reference</b><br> 6465 * Path: <b>ActivityDefinition.relatedArtifact.resource, 6466 * ActivityDefinition.library</b><br> 6467 * </p> 6468 */ 6469 @SearchParamDefinition(name = "depends-on", path = "ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library", description = "What resource is being referenced", type = "reference") 6470 public static final String SP_DEPENDS_ON = "depends-on"; 6471 /** 6472 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 6473 * <p> 6474 * Description: <b>What resource is being referenced</b><br> 6475 * Type: <b>reference</b><br> 6476 * Path: <b>ActivityDefinition.relatedArtifact.resource, 6477 * ActivityDefinition.library</b><br> 6478 * </p> 6479 */ 6480 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6481 SP_DEPENDS_ON); 6482 6483 /** 6484 * Constant for fluent queries to be used to add include statements. Specifies 6485 * the path value of "<b>ActivityDefinition:depends-on</b>". 6486 */ 6487 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 6488 "ActivityDefinition:depends-on").toLocked(); 6489 6490 /** 6491 * Search parameter: <b>name</b> 6492 * <p> 6493 * Description: <b>Computationally friendly name of the activity 6494 * definition</b><br> 6495 * Type: <b>string</b><br> 6496 * Path: <b>ActivityDefinition.name</b><br> 6497 * </p> 6498 */ 6499 @SearchParamDefinition(name = "name", path = "ActivityDefinition.name", description = "Computationally friendly name of the activity definition", type = "string") 6500 public static final String SP_NAME = "name"; 6501 /** 6502 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6503 * <p> 6504 * Description: <b>Computationally friendly name of the activity 6505 * definition</b><br> 6506 * Type: <b>string</b><br> 6507 * Path: <b>ActivityDefinition.name</b><br> 6508 * </p> 6509 */ 6510 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6511 SP_NAME); 6512 6513 /** 6514 * Search parameter: <b>context</b> 6515 * <p> 6516 * Description: <b>A use context assigned to the activity definition</b><br> 6517 * Type: <b>token</b><br> 6518 * Path: <b>ActivityDefinition.useContext.valueCodeableConcept</b><br> 6519 * </p> 6520 */ 6521 @SearchParamDefinition(name = "context", path = "(ActivityDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the activity definition", type = "token") 6522 public static final String SP_CONTEXT = "context"; 6523 /** 6524 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6525 * <p> 6526 * Description: <b>A use context assigned to the activity definition</b><br> 6527 * Type: <b>token</b><br> 6528 * Path: <b>ActivityDefinition.useContext.valueCodeableConcept</b><br> 6529 * </p> 6530 */ 6531 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6532 SP_CONTEXT); 6533 6534 /** 6535 * Search parameter: <b>publisher</b> 6536 * <p> 6537 * Description: <b>Name of the publisher of the activity definition</b><br> 6538 * Type: <b>string</b><br> 6539 * Path: <b>ActivityDefinition.publisher</b><br> 6540 * </p> 6541 */ 6542 @SearchParamDefinition(name = "publisher", path = "ActivityDefinition.publisher", description = "Name of the publisher of the activity definition", type = "string") 6543 public static final String SP_PUBLISHER = "publisher"; 6544 /** 6545 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6546 * <p> 6547 * Description: <b>Name of the publisher of the activity definition</b><br> 6548 * Type: <b>string</b><br> 6549 * Path: <b>ActivityDefinition.publisher</b><br> 6550 * </p> 6551 */ 6552 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6553 SP_PUBLISHER); 6554 6555 /** 6556 * Search parameter: <b>topic</b> 6557 * <p> 6558 * Description: <b>Topics associated with the module</b><br> 6559 * Type: <b>token</b><br> 6560 * Path: <b>ActivityDefinition.topic</b><br> 6561 * </p> 6562 */ 6563 @SearchParamDefinition(name = "topic", path = "ActivityDefinition.topic", description = "Topics associated with the module", type = "token") 6564 public static final String SP_TOPIC = "topic"; 6565 /** 6566 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 6567 * <p> 6568 * Description: <b>Topics associated with the module</b><br> 6569 * Type: <b>token</b><br> 6570 * Path: <b>ActivityDefinition.topic</b><br> 6571 * </p> 6572 */ 6573 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6574 SP_TOPIC); 6575 6576 /** 6577 * Search parameter: <b>context-type-quantity</b> 6578 * <p> 6579 * Description: <b>A use context type and quantity- or range-based value 6580 * assigned to the activity definition</b><br> 6581 * Type: <b>composite</b><br> 6582 * Path: <b></b><br> 6583 * </p> 6584 */ 6585 @SearchParamDefinition(name = "context-type-quantity", path = "ActivityDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the activity definition", type = "composite", compositeOf = { 6586 "context-type", "context-quantity" }) 6587 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6588 /** 6589 * <b>Fluent Client</b> search parameter constant for 6590 * <b>context-type-quantity</b> 6591 * <p> 6592 * Description: <b>A use context type and quantity- or range-based value 6593 * assigned to the activity definition</b><br> 6594 * Type: <b>composite</b><br> 6595 * Path: <b></b><br> 6596 * </p> 6597 */ 6598 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6599 SP_CONTEXT_TYPE_QUANTITY); 6600 6601 /** 6602 * Search parameter: <b>status</b> 6603 * <p> 6604 * Description: <b>The current status of the activity definition</b><br> 6605 * Type: <b>token</b><br> 6606 * Path: <b>ActivityDefinition.status</b><br> 6607 * </p> 6608 */ 6609 @SearchParamDefinition(name = "status", path = "ActivityDefinition.status", description = "The current status of the activity definition", type = "token") 6610 public static final String SP_STATUS = "status"; 6611 /** 6612 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6613 * <p> 6614 * Description: <b>The current status of the activity definition</b><br> 6615 * Type: <b>token</b><br> 6616 * Path: <b>ActivityDefinition.status</b><br> 6617 * </p> 6618 */ 6619 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6620 SP_STATUS); 6621 6622}