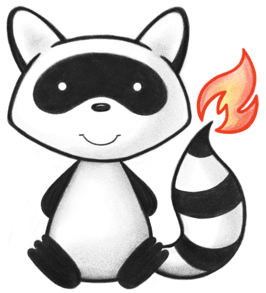
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * An address expressed using postal conventions (as opposed to GPS or other 046 * location definition formats). This data type may be used to convey addresses 047 * for use in delivering mail as well as for visiting locations which might not 048 * be valid for mail delivery. There are a variety of postal address formats 049 * defined around the world. 050 */ 051@DatatypeDef(name = "Address") 052public class Address extends Type implements ICompositeType { 053 054 public enum AddressUse { 055 /** 056 * A communication address at a home. 057 */ 058 HOME, 059 /** 060 * An office address. First choice for business related contacts during business 061 * hours. 062 */ 063 WORK, 064 /** 065 * A temporary address. The period can provide more detailed information. 066 */ 067 TEMP, 068 /** 069 * This address is no longer in use (or was never correct but retained for 070 * records). 071 */ 072 OLD, 073 /** 074 * An address to be used to send bills, invoices, receipts etc. 075 */ 076 BILLING, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static AddressUse fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("home".equals(codeString)) 086 return HOME; 087 if ("work".equals(codeString)) 088 return WORK; 089 if ("temp".equals(codeString)) 090 return TEMP; 091 if ("old".equals(codeString)) 092 return OLD; 093 if ("billing".equals(codeString)) 094 return BILLING; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown AddressUse code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case HOME: 104 return "home"; 105 case WORK: 106 return "work"; 107 case TEMP: 108 return "temp"; 109 case OLD: 110 return "old"; 111 case BILLING: 112 return "billing"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case HOME: 123 return "http://hl7.org/fhir/address-use"; 124 case WORK: 125 return "http://hl7.org/fhir/address-use"; 126 case TEMP: 127 return "http://hl7.org/fhir/address-use"; 128 case OLD: 129 return "http://hl7.org/fhir/address-use"; 130 case BILLING: 131 return "http://hl7.org/fhir/address-use"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case HOME: 142 return "A communication address at a home."; 143 case WORK: 144 return "An office address. First choice for business related contacts during business hours."; 145 case TEMP: 146 return "A temporary address. The period can provide more detailed information."; 147 case OLD: 148 return "This address is no longer in use (or was never correct but retained for records)."; 149 case BILLING: 150 return "An address to be used to send bills, invoices, receipts etc."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case HOME: 161 return "Home"; 162 case WORK: 163 return "Work"; 164 case TEMP: 165 return "Temporary"; 166 case OLD: 167 return "Old / Incorrect"; 168 case BILLING: 169 return "Billing"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class AddressUseEnumFactory implements EnumFactory<AddressUse> { 179 public AddressUse fromCode(String codeString) throws IllegalArgumentException { 180 if (codeString == null || "".equals(codeString)) 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("home".equals(codeString)) 184 return AddressUse.HOME; 185 if ("work".equals(codeString)) 186 return AddressUse.WORK; 187 if ("temp".equals(codeString)) 188 return AddressUse.TEMP; 189 if ("old".equals(codeString)) 190 return AddressUse.OLD; 191 if ("billing".equals(codeString)) 192 return AddressUse.BILLING; 193 throw new IllegalArgumentException("Unknown AddressUse code '" + codeString + "'"); 194 } 195 196 public Enumeration<AddressUse> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<AddressUse>(this, AddressUse.NULL, code); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<AddressUse>(this, AddressUse.NULL, code); 204 if ("home".equals(codeString)) 205 return new Enumeration<AddressUse>(this, AddressUse.HOME, code); 206 if ("work".equals(codeString)) 207 return new Enumeration<AddressUse>(this, AddressUse.WORK, code); 208 if ("temp".equals(codeString)) 209 return new Enumeration<AddressUse>(this, AddressUse.TEMP, code); 210 if ("old".equals(codeString)) 211 return new Enumeration<AddressUse>(this, AddressUse.OLD, code); 212 if ("billing".equals(codeString)) 213 return new Enumeration<AddressUse>(this, AddressUse.BILLING, code); 214 throw new FHIRException("Unknown AddressUse code '" + codeString + "'"); 215 } 216 217 public String toCode(AddressUse code) { 218 if (code == AddressUse.NULL) 219 return null; 220 if (code == AddressUse.HOME) 221 return "home"; 222 if (code == AddressUse.WORK) 223 return "work"; 224 if (code == AddressUse.TEMP) 225 return "temp"; 226 if (code == AddressUse.OLD) 227 return "old"; 228 if (code == AddressUse.BILLING) 229 return "billing"; 230 return "?"; 231 } 232 233 public String toSystem(AddressUse code) { 234 return code.getSystem(); 235 } 236 } 237 238 public enum AddressType { 239 /** 240 * Mailing addresses - PO Boxes and care-of addresses. 241 */ 242 POSTAL, 243 /** 244 * A physical address that can be visited. 245 */ 246 PHYSICAL, 247 /** 248 * An address that is both physical and postal. 249 */ 250 BOTH, 251 /** 252 * added to help the parsers with the generic types 253 */ 254 NULL; 255 256 public static AddressType fromCode(String codeString) throws FHIRException { 257 if (codeString == null || "".equals(codeString)) 258 return null; 259 if ("postal".equals(codeString)) 260 return POSTAL; 261 if ("physical".equals(codeString)) 262 return PHYSICAL; 263 if ("both".equals(codeString)) 264 return BOTH; 265 if (Configuration.isAcceptInvalidEnums()) 266 return null; 267 else 268 throw new FHIRException("Unknown AddressType code '" + codeString + "'"); 269 } 270 271 public String toCode() { 272 switch (this) { 273 case POSTAL: 274 return "postal"; 275 case PHYSICAL: 276 return "physical"; 277 case BOTH: 278 return "both"; 279 case NULL: 280 return null; 281 default: 282 return "?"; 283 } 284 } 285 286 public String getSystem() { 287 switch (this) { 288 case POSTAL: 289 return "http://hl7.org/fhir/address-type"; 290 case PHYSICAL: 291 return "http://hl7.org/fhir/address-type"; 292 case BOTH: 293 return "http://hl7.org/fhir/address-type"; 294 case NULL: 295 return null; 296 default: 297 return "?"; 298 } 299 } 300 301 public String getDefinition() { 302 switch (this) { 303 case POSTAL: 304 return "Mailing addresses - PO Boxes and care-of addresses."; 305 case PHYSICAL: 306 return "A physical address that can be visited."; 307 case BOTH: 308 return "An address that is both physical and postal."; 309 case NULL: 310 return null; 311 default: 312 return "?"; 313 } 314 } 315 316 public String getDisplay() { 317 switch (this) { 318 case POSTAL: 319 return "Postal"; 320 case PHYSICAL: 321 return "Physical"; 322 case BOTH: 323 return "Postal & Physical"; 324 case NULL: 325 return null; 326 default: 327 return "?"; 328 } 329 } 330 } 331 332 public static class AddressTypeEnumFactory implements EnumFactory<AddressType> { 333 public AddressType fromCode(String codeString) throws IllegalArgumentException { 334 if (codeString == null || "".equals(codeString)) 335 if (codeString == null || "".equals(codeString)) 336 return null; 337 if ("postal".equals(codeString)) 338 return AddressType.POSTAL; 339 if ("physical".equals(codeString)) 340 return AddressType.PHYSICAL; 341 if ("both".equals(codeString)) 342 return AddressType.BOTH; 343 throw new IllegalArgumentException("Unknown AddressType code '" + codeString + "'"); 344 } 345 346 public Enumeration<AddressType> fromType(PrimitiveType<?> code) throws FHIRException { 347 if (code == null) 348 return null; 349 if (code.isEmpty()) 350 return new Enumeration<AddressType>(this, AddressType.NULL, code); 351 String codeString = code.asStringValue(); 352 if (codeString == null || "".equals(codeString)) 353 return new Enumeration<AddressType>(this, AddressType.NULL, code); 354 if ("postal".equals(codeString)) 355 return new Enumeration<AddressType>(this, AddressType.POSTAL, code); 356 if ("physical".equals(codeString)) 357 return new Enumeration<AddressType>(this, AddressType.PHYSICAL, code); 358 if ("both".equals(codeString)) 359 return new Enumeration<AddressType>(this, AddressType.BOTH, code); 360 throw new FHIRException("Unknown AddressType code '" + codeString + "'"); 361 } 362 363 public String toCode(AddressType code) { 364 if (code == AddressType.NULL) 365 return null; 366 if (code == AddressType.POSTAL) 367 return "postal"; 368 if (code == AddressType.PHYSICAL) 369 return "physical"; 370 if (code == AddressType.BOTH) 371 return "both"; 372 return "?"; 373 } 374 375 public String toSystem(AddressType code) { 376 return code.getSystem(); 377 } 378 } 379 380 /** 381 * The purpose of this address. 382 */ 383 @Child(name = "use", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = true, summary = true) 384 @Description(shortDefinition = "home | work | temp | old | billing - purpose of this address", formalDefinition = "The purpose of this address.") 385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/address-use") 386 protected Enumeration<AddressUse> use; 387 388 /** 389 * Distinguishes between physical addresses (those you can visit) and mailing 390 * addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 391 */ 392 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 393 @Description(shortDefinition = "postal | physical | both", formalDefinition = "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.") 394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/address-type") 395 protected Enumeration<AddressType> type; 396 397 /** 398 * Specifies the entire address as it should be displayed e.g. on a postal 399 * label. This may be provided instead of or as well as the specific parts. 400 */ 401 @Child(name = "text", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 402 @Description(shortDefinition = "Text representation of the address", formalDefinition = "Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.") 403 protected StringType text; 404 405 /** 406 * This component contains the house number, apartment number, street name, 407 * street direction, P.O. Box number, delivery hints, and similar address 408 * information. 409 */ 410 @Child(name = "line", type = { 411 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 412 @Description(shortDefinition = "Street name, number, direction & P.O. Box etc.", formalDefinition = "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.") 413 protected List<StringType> line; 414 415 /** 416 * The name of the city, town, suburb, village or other community or delivery 417 * center. 418 */ 419 @Child(name = "city", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 420 @Description(shortDefinition = "Name of city, town etc.", formalDefinition = "The name of the city, town, suburb, village or other community or delivery center.") 421 protected StringType city; 422 423 /** 424 * The name of the administrative area (county). 425 */ 426 @Child(name = "district", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 427 @Description(shortDefinition = "District name (aka county)", formalDefinition = "The name of the administrative area (county).") 428 protected StringType district; 429 430 /** 431 * Sub-unit of a country with limited sovereignty in a federally organized 432 * country. A code may be used if codes are in common use (e.g. US 2 letter 433 * state codes). 434 */ 435 @Child(name = "state", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 436 @Description(shortDefinition = "Sub-unit of country (abbreviations ok)", formalDefinition = "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).") 437 protected StringType state; 438 439 /** 440 * A postal code designating a region defined by the postal service. 441 */ 442 @Child(name = "postalCode", type = { 443 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 444 @Description(shortDefinition = "Postal code for area", formalDefinition = "A postal code designating a region defined by the postal service.") 445 protected StringType postalCode; 446 447 /** 448 * Country - a nation as commonly understood or generally accepted. 449 */ 450 @Child(name = "country", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 451 @Description(shortDefinition = "Country (e.g. can be ISO 3166 2 or 3 letter code)", formalDefinition = "Country - a nation as commonly understood or generally accepted.") 452 protected StringType country; 453 454 /** 455 * Time period when address was/is in use. 456 */ 457 @Child(name = "period", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 458 @Description(shortDefinition = "Time period when address was/is in use", formalDefinition = "Time period when address was/is in use.") 459 protected Period period; 460 461 private static final long serialVersionUID = 561490318L; 462 463 /** 464 * Constructor 465 */ 466 public Address() { 467 super(); 468 } 469 470 /** 471 * @return {@link #use} (The purpose of this address.). This is the underlying 472 * object with id, value and extensions. The accessor "getUse" gives 473 * direct access to the value 474 */ 475 public Enumeration<AddressUse> getUseElement() { 476 if (this.use == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create Address.use"); 479 else if (Configuration.doAutoCreate()) 480 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); // bb 481 return this.use; 482 } 483 484 public boolean hasUseElement() { 485 return this.use != null && !this.use.isEmpty(); 486 } 487 488 public boolean hasUse() { 489 return this.use != null && !this.use.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #use} (The purpose of this address.). This is the 494 * underlying object with id, value and extensions. The accessor 495 * "getUse" gives direct access to the value 496 */ 497 public Address setUseElement(Enumeration<AddressUse> value) { 498 this.use = value; 499 return this; 500 } 501 502 /** 503 * @return The purpose of this address. 504 */ 505 public AddressUse getUse() { 506 return this.use == null ? null : this.use.getValue(); 507 } 508 509 /** 510 * @param value The purpose of this address. 511 */ 512 public Address setUse(AddressUse value) { 513 if (value == null) 514 this.use = null; 515 else { 516 if (this.use == null) 517 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); 518 this.use.setValue(value); 519 } 520 return this; 521 } 522 523 /** 524 * @return {@link #type} (Distinguishes between physical addresses (those you 525 * can visit) and mailing addresses (e.g. PO Boxes and care-of 526 * addresses). Most addresses are both.). This is the underlying object 527 * with id, value and extensions. The accessor "getType" gives direct 528 * access to the value 529 */ 530 public Enumeration<AddressType> getTypeElement() { 531 if (this.type == null) 532 if (Configuration.errorOnAutoCreate()) 533 throw new Error("Attempt to auto-create Address.type"); 534 else if (Configuration.doAutoCreate()) 535 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); // bb 536 return this.type; 537 } 538 539 public boolean hasTypeElement() { 540 return this.type != null && !this.type.isEmpty(); 541 } 542 543 public boolean hasType() { 544 return this.type != null && !this.type.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #type} (Distinguishes between physical addresses (those 549 * you can visit) and mailing addresses (e.g. PO Boxes and care-of 550 * addresses). Most addresses are both.). This is the underlying 551 * object with id, value and extensions. The accessor "getType" 552 * gives direct access to the value 553 */ 554 public Address setTypeElement(Enumeration<AddressType> value) { 555 this.type = value; 556 return this; 557 } 558 559 /** 560 * @return Distinguishes between physical addresses (those you can visit) and 561 * mailing addresses (e.g. PO Boxes and care-of addresses). Most 562 * addresses are both. 563 */ 564 public AddressType getType() { 565 return this.type == null ? null : this.type.getValue(); 566 } 567 568 /** 569 * @param value Distinguishes between physical addresses (those you can visit) 570 * and mailing addresses (e.g. PO Boxes and care-of addresses). 571 * Most addresses are both. 572 */ 573 public Address setType(AddressType value) { 574 if (value == null) 575 this.type = null; 576 else { 577 if (this.type == null) 578 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); 579 this.type.setValue(value); 580 } 581 return this; 582 } 583 584 /** 585 * @return {@link #text} (Specifies the entire address as it should be displayed 586 * e.g. on a postal label. This may be provided instead of or as well as 587 * the specific parts.). This is the underlying object with id, value 588 * and extensions. The accessor "getText" gives direct access to the 589 * value 590 */ 591 public StringType getTextElement() { 592 if (this.text == null) 593 if (Configuration.errorOnAutoCreate()) 594 throw new Error("Attempt to auto-create Address.text"); 595 else if (Configuration.doAutoCreate()) 596 this.text = new StringType(); // bb 597 return this.text; 598 } 599 600 public boolean hasTextElement() { 601 return this.text != null && !this.text.isEmpty(); 602 } 603 604 public boolean hasText() { 605 return this.text != null && !this.text.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #text} (Specifies the entire address as it should be 610 * displayed e.g. on a postal label. This may be provided instead 611 * of or as well as the specific parts.). This is the underlying 612 * object with id, value and extensions. The accessor "getText" 613 * gives direct access to the value 614 */ 615 public Address setTextElement(StringType value) { 616 this.text = value; 617 return this; 618 } 619 620 /** 621 * @return Specifies the entire address as it should be displayed e.g. on a 622 * postal label. This may be provided instead of or as well as the 623 * specific parts. 624 */ 625 public String getText() { 626 return this.text == null ? null : this.text.getValue(); 627 } 628 629 /** 630 * @param value Specifies the entire address as it should be displayed e.g. on a 631 * postal label. This may be provided instead of or as well as the 632 * specific parts. 633 */ 634 public Address setText(String value) { 635 if (Utilities.noString(value)) 636 this.text = null; 637 else { 638 if (this.text == null) 639 this.text = new StringType(); 640 this.text.setValue(value); 641 } 642 return this; 643 } 644 645 /** 646 * @return {@link #line} (This component contains the house number, apartment 647 * number, street name, street direction, P.O. Box number, delivery 648 * hints, and similar address information.) 649 */ 650 public List<StringType> getLine() { 651 if (this.line == null) 652 this.line = new ArrayList<StringType>(); 653 return this.line; 654 } 655 656 /** 657 * @return Returns a reference to <code>this</code> for easy method chaining 658 */ 659 public Address setLine(List<StringType> theLine) { 660 this.line = theLine; 661 return this; 662 } 663 664 public boolean hasLine() { 665 if (this.line == null) 666 return false; 667 for (StringType item : this.line) 668 if (!item.isEmpty()) 669 return true; 670 return false; 671 } 672 673 /** 674 * @return {@link #line} (This component contains the house number, apartment 675 * number, street name, street direction, P.O. Box number, delivery 676 * hints, and similar address information.) 677 */ 678 public StringType addLineElement() {// 2 679 StringType t = new StringType(); 680 if (this.line == null) 681 this.line = new ArrayList<StringType>(); 682 this.line.add(t); 683 return t; 684 } 685 686 /** 687 * @param value {@link #line} (This component contains the house number, 688 * apartment number, street name, street direction, P.O. Box 689 * number, delivery hints, and similar address information.) 690 */ 691 public Address addLine(String value) { // 1 692 StringType t = new StringType(); 693 t.setValue(value); 694 if (this.line == null) 695 this.line = new ArrayList<StringType>(); 696 this.line.add(t); 697 return this; 698 } 699 700 /** 701 * @param value {@link #line} (This component contains the house number, 702 * apartment number, street name, street direction, P.O. Box 703 * number, delivery hints, and similar address information.) 704 */ 705 public boolean hasLine(String value) { 706 if (this.line == null) 707 return false; 708 for (StringType v : this.line) 709 if (v.getValue().equals(value)) // string 710 return true; 711 return false; 712 } 713 714 /** 715 * @return {@link #city} (The name of the city, town, suburb, village or other 716 * community or delivery center.). This is the underlying object with 717 * id, value and extensions. The accessor "getCity" gives direct access 718 * to the value 719 */ 720 public StringType getCityElement() { 721 if (this.city == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create Address.city"); 724 else if (Configuration.doAutoCreate()) 725 this.city = new StringType(); // bb 726 return this.city; 727 } 728 729 public boolean hasCityElement() { 730 return this.city != null && !this.city.isEmpty(); 731 } 732 733 public boolean hasCity() { 734 return this.city != null && !this.city.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #city} (The name of the city, town, suburb, village or 739 * other community or delivery center.). This is the underlying 740 * object with id, value and extensions. The accessor "getCity" 741 * gives direct access to the value 742 */ 743 public Address setCityElement(StringType value) { 744 this.city = value; 745 return this; 746 } 747 748 /** 749 * @return The name of the city, town, suburb, village or other community or 750 * delivery center. 751 */ 752 public String getCity() { 753 return this.city == null ? null : this.city.getValue(); 754 } 755 756 /** 757 * @param value The name of the city, town, suburb, village or other community 758 * or delivery center. 759 */ 760 public Address setCity(String value) { 761 if (Utilities.noString(value)) 762 this.city = null; 763 else { 764 if (this.city == null) 765 this.city = new StringType(); 766 this.city.setValue(value); 767 } 768 return this; 769 } 770 771 /** 772 * @return {@link #district} (The name of the administrative area (county).). 773 * This is the underlying object with id, value and extensions. The 774 * accessor "getDistrict" gives direct access to the value 775 */ 776 public StringType getDistrictElement() { 777 if (this.district == null) 778 if (Configuration.errorOnAutoCreate()) 779 throw new Error("Attempt to auto-create Address.district"); 780 else if (Configuration.doAutoCreate()) 781 this.district = new StringType(); // bb 782 return this.district; 783 } 784 785 public boolean hasDistrictElement() { 786 return this.district != null && !this.district.isEmpty(); 787 } 788 789 public boolean hasDistrict() { 790 return this.district != null && !this.district.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #district} (The name of the administrative area 795 * (county).). This is the underlying object with id, value and 796 * extensions. The accessor "getDistrict" gives direct access to 797 * the value 798 */ 799 public Address setDistrictElement(StringType value) { 800 this.district = value; 801 return this; 802 } 803 804 /** 805 * @return The name of the administrative area (county). 806 */ 807 public String getDistrict() { 808 return this.district == null ? null : this.district.getValue(); 809 } 810 811 /** 812 * @param value The name of the administrative area (county). 813 */ 814 public Address setDistrict(String value) { 815 if (Utilities.noString(value)) 816 this.district = null; 817 else { 818 if (this.district == null) 819 this.district = new StringType(); 820 this.district.setValue(value); 821 } 822 return this; 823 } 824 825 /** 826 * @return {@link #state} (Sub-unit of a country with limited sovereignty in a 827 * federally organized country. A code may be used if codes are in 828 * common use (e.g. US 2 letter state codes).). This is the underlying 829 * object with id, value and extensions. The accessor "getState" gives 830 * direct access to the value 831 */ 832 public StringType getStateElement() { 833 if (this.state == null) 834 if (Configuration.errorOnAutoCreate()) 835 throw new Error("Attempt to auto-create Address.state"); 836 else if (Configuration.doAutoCreate()) 837 this.state = new StringType(); // bb 838 return this.state; 839 } 840 841 public boolean hasStateElement() { 842 return this.state != null && !this.state.isEmpty(); 843 } 844 845 public boolean hasState() { 846 return this.state != null && !this.state.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #state} (Sub-unit of a country with limited sovereignty 851 * in a federally organized country. A code may be used if codes 852 * are in common use (e.g. US 2 letter state codes).). This is the 853 * underlying object with id, value and extensions. The accessor 854 * "getState" gives direct access to the value 855 */ 856 public Address setStateElement(StringType value) { 857 this.state = value; 858 return this; 859 } 860 861 /** 862 * @return Sub-unit of a country with limited sovereignty in a federally 863 * organized country. A code may be used if codes are in common use 864 * (e.g. US 2 letter state codes). 865 */ 866 public String getState() { 867 return this.state == null ? null : this.state.getValue(); 868 } 869 870 /** 871 * @param value Sub-unit of a country with limited sovereignty in a federally 872 * organized country. A code may be used if codes are in common use 873 * (e.g. US 2 letter state codes). 874 */ 875 public Address setState(String value) { 876 if (Utilities.noString(value)) 877 this.state = null; 878 else { 879 if (this.state == null) 880 this.state = new StringType(); 881 this.state.setValue(value); 882 } 883 return this; 884 } 885 886 /** 887 * @return {@link #postalCode} (A postal code designating a region defined by 888 * the postal service.). This is the underlying object with id, value 889 * and extensions. The accessor "getPostalCode" gives direct access to 890 * the value 891 */ 892 public StringType getPostalCodeElement() { 893 if (this.postalCode == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create Address.postalCode"); 896 else if (Configuration.doAutoCreate()) 897 this.postalCode = new StringType(); // bb 898 return this.postalCode; 899 } 900 901 public boolean hasPostalCodeElement() { 902 return this.postalCode != null && !this.postalCode.isEmpty(); 903 } 904 905 public boolean hasPostalCode() { 906 return this.postalCode != null && !this.postalCode.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #postalCode} (A postal code designating a region defined 911 * by the postal service.). This is the underlying object with id, 912 * value and extensions. The accessor "getPostalCode" gives direct 913 * access to the value 914 */ 915 public Address setPostalCodeElement(StringType value) { 916 this.postalCode = value; 917 return this; 918 } 919 920 /** 921 * @return A postal code designating a region defined by the postal service. 922 */ 923 public String getPostalCode() { 924 return this.postalCode == null ? null : this.postalCode.getValue(); 925 } 926 927 /** 928 * @param value A postal code designating a region defined by the postal 929 * service. 930 */ 931 public Address setPostalCode(String value) { 932 if (Utilities.noString(value)) 933 this.postalCode = null; 934 else { 935 if (this.postalCode == null) 936 this.postalCode = new StringType(); 937 this.postalCode.setValue(value); 938 } 939 return this; 940 } 941 942 /** 943 * @return {@link #country} (Country - a nation as commonly understood or 944 * generally accepted.). This is the underlying object with id, value 945 * and extensions. The accessor "getCountry" gives direct access to the 946 * value 947 */ 948 public StringType getCountryElement() { 949 if (this.country == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create Address.country"); 952 else if (Configuration.doAutoCreate()) 953 this.country = new StringType(); // bb 954 return this.country; 955 } 956 957 public boolean hasCountryElement() { 958 return this.country != null && !this.country.isEmpty(); 959 } 960 961 public boolean hasCountry() { 962 return this.country != null && !this.country.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #country} (Country - a nation as commonly understood or 967 * generally accepted.). This is the underlying object with id, 968 * value and extensions. The accessor "getCountry" gives direct 969 * access to the value 970 */ 971 public Address setCountryElement(StringType value) { 972 this.country = value; 973 return this; 974 } 975 976 /** 977 * @return Country - a nation as commonly understood or generally accepted. 978 */ 979 public String getCountry() { 980 return this.country == null ? null : this.country.getValue(); 981 } 982 983 /** 984 * @param value Country - a nation as commonly understood or generally accepted. 985 */ 986 public Address setCountry(String value) { 987 if (Utilities.noString(value)) 988 this.country = null; 989 else { 990 if (this.country == null) 991 this.country = new StringType(); 992 this.country.setValue(value); 993 } 994 return this; 995 } 996 997 /** 998 * @return {@link #period} (Time period when address was/is in use.) 999 */ 1000 public Period getPeriod() { 1001 if (this.period == null) 1002 if (Configuration.errorOnAutoCreate()) 1003 throw new Error("Attempt to auto-create Address.period"); 1004 else if (Configuration.doAutoCreate()) 1005 this.period = new Period(); // cc 1006 return this.period; 1007 } 1008 1009 public boolean hasPeriod() { 1010 return this.period != null && !this.period.isEmpty(); 1011 } 1012 1013 /** 1014 * @param value {@link #period} (Time period when address was/is in use.) 1015 */ 1016 public Address setPeriod(Period value) { 1017 this.period = value; 1018 return this; 1019 } 1020 1021 protected void listChildren(List<Property> children) { 1022 super.listChildren(children); 1023 children.add(new Property("use", "code", "The purpose of this address.", 0, 1, use)); 1024 children.add(new Property("type", "code", 1025 "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 1026 0, 1, type)); 1027 children.add(new Property("text", "string", 1028 "Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.", 1029 0, 1, text)); 1030 children.add(new Property("line", "string", 1031 "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 1032 0, java.lang.Integer.MAX_VALUE, line)); 1033 children.add(new Property("city", "string", 1034 "The name of the city, town, suburb, village or other community or delivery center.", 0, 1, city)); 1035 children.add(new Property("district", "string", "The name of the administrative area (county).", 0, 1, district)); 1036 children.add(new Property("state", "string", 1037 "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).", 1038 0, 1, state)); 1039 children.add(new Property("postalCode", "string", 1040 "A postal code designating a region defined by the postal service.", 0, 1, postalCode)); 1041 children.add(new Property("country", "string", "Country - a nation as commonly understood or generally accepted.", 1042 0, 1, country)); 1043 children.add(new Property("period", "Period", "Time period when address was/is in use.", 0, 1, period)); 1044 } 1045 1046 @Override 1047 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1048 switch (_hash) { 1049 case 116103: 1050 /* use */ return new Property("use", "code", "The purpose of this address.", 0, 1, use); 1051 case 3575610: 1052 /* type */ return new Property("type", "code", 1053 "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 1054 0, 1, type); 1055 case 3556653: 1056 /* text */ return new Property("text", "string", 1057 "Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.", 1058 0, 1, text); 1059 case 3321844: 1060 /* line */ return new Property("line", "string", 1061 "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 1062 0, java.lang.Integer.MAX_VALUE, line); 1063 case 3053931: 1064 /* city */ return new Property("city", "string", 1065 "The name of the city, town, suburb, village or other community or delivery center.", 0, 1, city); 1066 case 288961422: 1067 /* district */ return new Property("district", "string", "The name of the administrative area (county).", 0, 1, 1068 district); 1069 case 109757585: 1070 /* state */ return new Property("state", "string", 1071 "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).", 1072 0, 1, state); 1073 case 2011152728: 1074 /* postalCode */ return new Property("postalCode", "string", 1075 "A postal code designating a region defined by the postal service.", 0, 1, postalCode); 1076 case 957831062: 1077 /* country */ return new Property("country", "string", 1078 "Country - a nation as commonly understood or generally accepted.", 0, 1, country); 1079 case -991726143: 1080 /* period */ return new Property("period", "Period", "Time period when address was/is in use.", 0, 1, period); 1081 default: 1082 return super.getNamedProperty(_hash, _name, _checkValid); 1083 } 1084 1085 } 1086 1087 @Override 1088 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1089 switch (hash) { 1090 case 116103: 1091 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<AddressUse> 1092 case 3575610: 1093 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<AddressType> 1094 case 3556653: 1095 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 1096 case 3321844: 1097 /* line */ return this.line == null ? new Base[0] : this.line.toArray(new Base[this.line.size()]); // StringType 1098 case 3053931: 1099 /* city */ return this.city == null ? new Base[0] : new Base[] { this.city }; // StringType 1100 case 288961422: 1101 /* district */ return this.district == null ? new Base[0] : new Base[] { this.district }; // StringType 1102 case 109757585: 1103 /* state */ return this.state == null ? new Base[0] : new Base[] { this.state }; // StringType 1104 case 2011152728: 1105 /* postalCode */ return this.postalCode == null ? new Base[0] : new Base[] { this.postalCode }; // StringType 1106 case 957831062: 1107 /* country */ return this.country == null ? new Base[0] : new Base[] { this.country }; // StringType 1108 case -991726143: 1109 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1110 default: 1111 return super.getProperty(hash, name, checkValid); 1112 } 1113 1114 } 1115 1116 @Override 1117 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1118 switch (hash) { 1119 case 116103: // use 1120 value = new AddressUseEnumFactory().fromType(castToCode(value)); 1121 this.use = (Enumeration) value; // Enumeration<AddressUse> 1122 return value; 1123 case 3575610: // type 1124 value = new AddressTypeEnumFactory().fromType(castToCode(value)); 1125 this.type = (Enumeration) value; // Enumeration<AddressType> 1126 return value; 1127 case 3556653: // text 1128 this.text = castToString(value); // StringType 1129 return value; 1130 case 3321844: // line 1131 this.getLine().add(castToString(value)); // StringType 1132 return value; 1133 case 3053931: // city 1134 this.city = castToString(value); // StringType 1135 return value; 1136 case 288961422: // district 1137 this.district = castToString(value); // StringType 1138 return value; 1139 case 109757585: // state 1140 this.state = castToString(value); // StringType 1141 return value; 1142 case 2011152728: // postalCode 1143 this.postalCode = castToString(value); // StringType 1144 return value; 1145 case 957831062: // country 1146 this.country = castToString(value); // StringType 1147 return value; 1148 case -991726143: // period 1149 this.period = castToPeriod(value); // Period 1150 return value; 1151 default: 1152 return super.setProperty(hash, name, value); 1153 } 1154 1155 } 1156 1157 @Override 1158 public Base setProperty(String name, Base value) throws FHIRException { 1159 if (name.equals("use")) { 1160 value = new AddressUseEnumFactory().fromType(castToCode(value)); 1161 this.use = (Enumeration) value; // Enumeration<AddressUse> 1162 } else if (name.equals("type")) { 1163 value = new AddressTypeEnumFactory().fromType(castToCode(value)); 1164 this.type = (Enumeration) value; // Enumeration<AddressType> 1165 } else if (name.equals("text")) { 1166 this.text = castToString(value); // StringType 1167 } else if (name.equals("line")) { 1168 this.getLine().add(castToString(value)); 1169 } else if (name.equals("city")) { 1170 this.city = castToString(value); // StringType 1171 } else if (name.equals("district")) { 1172 this.district = castToString(value); // StringType 1173 } else if (name.equals("state")) { 1174 this.state = castToString(value); // StringType 1175 } else if (name.equals("postalCode")) { 1176 this.postalCode = castToString(value); // StringType 1177 } else if (name.equals("country")) { 1178 this.country = castToString(value); // StringType 1179 } else if (name.equals("period")) { 1180 this.period = castToPeriod(value); // Period 1181 } else 1182 return super.setProperty(name, value); 1183 return value; 1184 } 1185 1186 @Override 1187 public void removeChild(String name, Base value) throws FHIRException { 1188 if (name.equals("use")) { 1189 this.use = null; 1190 } else if (name.equals("type")) { 1191 this.type = null; 1192 } else if (name.equals("text")) { 1193 this.text = null; 1194 } else if (name.equals("line")) { 1195 this.getLine().remove(castToString(value)); 1196 } else if (name.equals("city")) { 1197 this.city = null; 1198 } else if (name.equals("district")) { 1199 this.district = null; 1200 } else if (name.equals("state")) { 1201 this.state = null; 1202 } else if (name.equals("postalCode")) { 1203 this.postalCode = null; 1204 } else if (name.equals("country")) { 1205 this.country = null; 1206 } else if (name.equals("period")) { 1207 this.period = null; 1208 } else 1209 super.removeChild(name, value); 1210 1211 } 1212 1213 @Override 1214 public Base makeProperty(int hash, String name) throws FHIRException { 1215 switch (hash) { 1216 case 116103: 1217 return getUseElement(); 1218 case 3575610: 1219 return getTypeElement(); 1220 case 3556653: 1221 return getTextElement(); 1222 case 3321844: 1223 return addLineElement(); 1224 case 3053931: 1225 return getCityElement(); 1226 case 288961422: 1227 return getDistrictElement(); 1228 case 109757585: 1229 return getStateElement(); 1230 case 2011152728: 1231 return getPostalCodeElement(); 1232 case 957831062: 1233 return getCountryElement(); 1234 case -991726143: 1235 return getPeriod(); 1236 default: 1237 return super.makeProperty(hash, name); 1238 } 1239 1240 } 1241 1242 @Override 1243 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1244 switch (hash) { 1245 case 116103: 1246 /* use */ return new String[] { "code" }; 1247 case 3575610: 1248 /* type */ return new String[] { "code" }; 1249 case 3556653: 1250 /* text */ return new String[] { "string" }; 1251 case 3321844: 1252 /* line */ return new String[] { "string" }; 1253 case 3053931: 1254 /* city */ return new String[] { "string" }; 1255 case 288961422: 1256 /* district */ return new String[] { "string" }; 1257 case 109757585: 1258 /* state */ return new String[] { "string" }; 1259 case 2011152728: 1260 /* postalCode */ return new String[] { "string" }; 1261 case 957831062: 1262 /* country */ return new String[] { "string" }; 1263 case -991726143: 1264 /* period */ return new String[] { "Period" }; 1265 default: 1266 return super.getTypesForProperty(hash, name); 1267 } 1268 1269 } 1270 1271 @Override 1272 public Base addChild(String name) throws FHIRException { 1273 if (name.equals("use")) { 1274 throw new FHIRException("Cannot call addChild on a singleton property Address.use"); 1275 } else if (name.equals("type")) { 1276 throw new FHIRException("Cannot call addChild on a singleton property Address.type"); 1277 } else if (name.equals("text")) { 1278 throw new FHIRException("Cannot call addChild on a singleton property Address.text"); 1279 } else if (name.equals("line")) { 1280 throw new FHIRException("Cannot call addChild on a singleton property Address.line"); 1281 } else if (name.equals("city")) { 1282 throw new FHIRException("Cannot call addChild on a singleton property Address.city"); 1283 } else if (name.equals("district")) { 1284 throw new FHIRException("Cannot call addChild on a singleton property Address.district"); 1285 } else if (name.equals("state")) { 1286 throw new FHIRException("Cannot call addChild on a singleton property Address.state"); 1287 } else if (name.equals("postalCode")) { 1288 throw new FHIRException("Cannot call addChild on a singleton property Address.postalCode"); 1289 } else if (name.equals("country")) { 1290 throw new FHIRException("Cannot call addChild on a singleton property Address.country"); 1291 } else if (name.equals("period")) { 1292 this.period = new Period(); 1293 return this.period; 1294 } else 1295 return super.addChild(name); 1296 } 1297 1298 public String fhirType() { 1299 return "Address"; 1300 1301 } 1302 1303 public Address copy() { 1304 Address dst = new Address(); 1305 copyValues(dst); 1306 return dst; 1307 } 1308 1309 public void copyValues(Address dst) { 1310 super.copyValues(dst); 1311 dst.use = use == null ? null : use.copy(); 1312 dst.type = type == null ? null : type.copy(); 1313 dst.text = text == null ? null : text.copy(); 1314 if (line != null) { 1315 dst.line = new ArrayList<StringType>(); 1316 for (StringType i : line) 1317 dst.line.add(i.copy()); 1318 } 1319 ; 1320 dst.city = city == null ? null : city.copy(); 1321 dst.district = district == null ? null : district.copy(); 1322 dst.state = state == null ? null : state.copy(); 1323 dst.postalCode = postalCode == null ? null : postalCode.copy(); 1324 dst.country = country == null ? null : country.copy(); 1325 dst.period = period == null ? null : period.copy(); 1326 } 1327 1328 protected Address typedCopy() { 1329 return copy(); 1330 } 1331 1332 @Override 1333 public boolean equalsDeep(Base other_) { 1334 if (!super.equalsDeep(other_)) 1335 return false; 1336 if (!(other_ instanceof Address)) 1337 return false; 1338 Address o = (Address) other_; 1339 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 1340 && compareDeep(line, o.line, true) && compareDeep(city, o.city, true) && compareDeep(district, o.district, true) 1341 && compareDeep(state, o.state, true) && compareDeep(postalCode, o.postalCode, true) 1342 && compareDeep(country, o.country, true) && compareDeep(period, o.period, true); 1343 } 1344 1345 @Override 1346 public boolean equalsShallow(Base other_) { 1347 if (!super.equalsShallow(other_)) 1348 return false; 1349 if (!(other_ instanceof Address)) 1350 return false; 1351 Address o = (Address) other_; 1352 return compareValues(use, o.use, true) && compareValues(type, o.type, true) && compareValues(text, o.text, true) 1353 && compareValues(line, o.line, true) && compareValues(city, o.city, true) 1354 && compareValues(district, o.district, true) && compareValues(state, o.state, true) 1355 && compareValues(postalCode, o.postalCode, true) && compareValues(country, o.country, true); 1356 } 1357 1358 public boolean isEmpty() { 1359 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, type, text, line, city, district, state, 1360 postalCode, country, period); 1361 } 1362 1363}