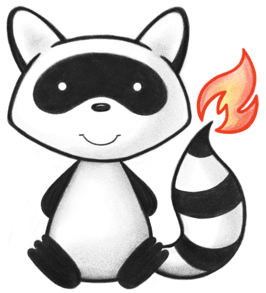
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Risk of harmful or undesirable, physiological response which is unique to an 049 * individual and associated with exposure to a substance. 050 */ 051@ResourceDef(name = "AllergyIntolerance", profile = "http://hl7.org/fhir/StructureDefinition/AllergyIntolerance") 052public class AllergyIntolerance extends DomainResource { 053 054 public enum AllergyIntoleranceType { 055 /** 056 * A propensity for hypersensitive reaction(s) to a substance. These reactions 057 * are most typically type I hypersensitivity, plus other "allergy-like" 058 * reactions, including pseudoallergy. 059 */ 060 ALLERGY, 061 /** 062 * A propensity for adverse reactions to a substance that is not judged to be 063 * allergic or "allergy-like". These reactions are typically (but not 064 * necessarily) non-immune. They are to some degree idiosyncratic and/or 065 * patient-specific (i.e. are not a reaction that is expected to occur with most 066 * or all patients given similar circumstances). 067 */ 068 INTOLERANCE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 074 public static AllergyIntoleranceType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("allergy".equals(codeString)) 078 return ALLERGY; 079 if ("intolerance".equals(codeString)) 080 return INTOLERANCE; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case ALLERGY: 090 return "allergy"; 091 case INTOLERANCE: 092 return "intolerance"; 093 case NULL: 094 return null; 095 default: 096 return "?"; 097 } 098 } 099 100 public String getSystem() { 101 switch (this) { 102 case ALLERGY: 103 return "http://hl7.org/fhir/allergy-intolerance-type"; 104 case INTOLERANCE: 105 return "http://hl7.org/fhir/allergy-intolerance-type"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getDefinition() { 114 switch (this) { 115 case ALLERGY: 116 return "A propensity for hypersensitive reaction(s) to a substance. These reactions are most typically type I hypersensitivity, plus other \"allergy-like\" reactions, including pseudoallergy."; 117 case INTOLERANCE: 118 return "A propensity for adverse reactions to a substance that is not judged to be allergic or \"allergy-like\". These reactions are typically (but not necessarily) non-immune. They are to some degree idiosyncratic and/or patient-specific (i.e. are not a reaction that is expected to occur with most or all patients given similar circumstances)."; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDisplay() { 127 switch (this) { 128 case ALLERGY: 129 return "Allergy"; 130 case INTOLERANCE: 131 return "Intolerance"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 } 139 140 public static class AllergyIntoleranceTypeEnumFactory implements EnumFactory<AllergyIntoleranceType> { 141 public AllergyIntoleranceType fromCode(String codeString) throws IllegalArgumentException { 142 if (codeString == null || "".equals(codeString)) 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("allergy".equals(codeString)) 146 return AllergyIntoleranceType.ALLERGY; 147 if ("intolerance".equals(codeString)) 148 return AllergyIntoleranceType.INTOLERANCE; 149 throw new IllegalArgumentException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 150 } 151 152 public Enumeration<AllergyIntoleranceType> fromType(PrimitiveType<?> code) throws FHIRException { 153 if (code == null) 154 return null; 155 if (code.isEmpty()) 156 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.NULL, code); 157 String codeString = code.asStringValue(); 158 if (codeString == null || "".equals(codeString)) 159 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.NULL, code); 160 if ("allergy".equals(codeString)) 161 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.ALLERGY, code); 162 if ("intolerance".equals(codeString)) 163 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.INTOLERANCE, code); 164 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 165 } 166 167 public String toCode(AllergyIntoleranceType code) { 168 if (code == AllergyIntoleranceType.ALLERGY) 169 return "allergy"; 170 if (code == AllergyIntoleranceType.INTOLERANCE) 171 return "intolerance"; 172 return "?"; 173 } 174 175 public String toSystem(AllergyIntoleranceType code) { 176 return code.getSystem(); 177 } 178 } 179 180 public enum AllergyIntoleranceCategory { 181 /** 182 * Any substance consumed to provide nutritional support for the body. 183 */ 184 FOOD, 185 /** 186 * Substances administered to achieve a physiological effect. 187 */ 188 MEDICATION, 189 /** 190 * Any substances that are encountered in the environment, including any 191 * substance not already classified as food, medication, or biologic. 192 */ 193 ENVIRONMENT, 194 /** 195 * A preparation that is synthesized from living organisms or their products, 196 * especially a human or animal protein, such as a hormone or antitoxin, that is 197 * used as a diagnostic, preventive, or therapeutic agent. Examples of biologic 198 * medications include: vaccines; allergenic extracts, which are used for both 199 * diagnosis and treatment (for example, allergy shots); gene therapies; 200 * cellular therapies. There are other biologic products, such as tissues, which 201 * are not typically associated with allergies. 202 */ 203 BIOLOGIC, 204 /** 205 * added to help the parsers with the generic types 206 */ 207 NULL; 208 209 public static AllergyIntoleranceCategory fromCode(String codeString) throws FHIRException { 210 if (codeString == null || "".equals(codeString)) 211 return null; 212 if ("food".equals(codeString)) 213 return FOOD; 214 if ("medication".equals(codeString)) 215 return MEDICATION; 216 if ("environment".equals(codeString)) 217 return ENVIRONMENT; 218 if ("biologic".equals(codeString)) 219 return BIOLOGIC; 220 if (Configuration.isAcceptInvalidEnums()) 221 return null; 222 else 223 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 224 } 225 226 public String toCode() { 227 switch (this) { 228 case FOOD: 229 return "food"; 230 case MEDICATION: 231 return "medication"; 232 case ENVIRONMENT: 233 return "environment"; 234 case BIOLOGIC: 235 return "biologic"; 236 case NULL: 237 return null; 238 default: 239 return "?"; 240 } 241 } 242 243 public String getSystem() { 244 switch (this) { 245 case FOOD: 246 return "http://hl7.org/fhir/allergy-intolerance-category"; 247 case MEDICATION: 248 return "http://hl7.org/fhir/allergy-intolerance-category"; 249 case ENVIRONMENT: 250 return "http://hl7.org/fhir/allergy-intolerance-category"; 251 case BIOLOGIC: 252 return "http://hl7.org/fhir/allergy-intolerance-category"; 253 case NULL: 254 return null; 255 default: 256 return "?"; 257 } 258 } 259 260 public String getDefinition() { 261 switch (this) { 262 case FOOD: 263 return "Any substance consumed to provide nutritional support for the body."; 264 case MEDICATION: 265 return "Substances administered to achieve a physiological effect."; 266 case ENVIRONMENT: 267 return "Any substances that are encountered in the environment, including any substance not already classified as food, medication, or biologic."; 268 case BIOLOGIC: 269 return "A preparation that is synthesized from living organisms or their products, especially a human or animal protein, such as a hormone or antitoxin, that is used as a diagnostic, preventive, or therapeutic agent. Examples of biologic medications include: vaccines; allergenic extracts, which are used for both diagnosis and treatment (for example, allergy shots); gene therapies; cellular therapies. There are other biologic products, such as tissues, which are not typically associated with allergies."; 270 case NULL: 271 return null; 272 default: 273 return "?"; 274 } 275 } 276 277 public String getDisplay() { 278 switch (this) { 279 case FOOD: 280 return "Food"; 281 case MEDICATION: 282 return "Medication"; 283 case ENVIRONMENT: 284 return "Environment"; 285 case BIOLOGIC: 286 return "Biologic"; 287 case NULL: 288 return null; 289 default: 290 return "?"; 291 } 292 } 293 } 294 295 public static class AllergyIntoleranceCategoryEnumFactory implements EnumFactory<AllergyIntoleranceCategory> { 296 public AllergyIntoleranceCategory fromCode(String codeString) throws IllegalArgumentException { 297 if (codeString == null || "".equals(codeString)) 298 if (codeString == null || "".equals(codeString)) 299 return null; 300 if ("food".equals(codeString)) 301 return AllergyIntoleranceCategory.FOOD; 302 if ("medication".equals(codeString)) 303 return AllergyIntoleranceCategory.MEDICATION; 304 if ("environment".equals(codeString)) 305 return AllergyIntoleranceCategory.ENVIRONMENT; 306 if ("biologic".equals(codeString)) 307 return AllergyIntoleranceCategory.BIOLOGIC; 308 throw new IllegalArgumentException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 309 } 310 311 public Enumeration<AllergyIntoleranceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 312 if (code == null) 313 return null; 314 if (code.isEmpty()) 315 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.NULL, code); 316 String codeString = code.asStringValue(); 317 if (codeString == null || "".equals(codeString)) 318 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.NULL, code); 319 if ("food".equals(codeString)) 320 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.FOOD, code); 321 if ("medication".equals(codeString)) 322 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.MEDICATION, code); 323 if ("environment".equals(codeString)) 324 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.ENVIRONMENT, code); 325 if ("biologic".equals(codeString)) 326 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.BIOLOGIC, code); 327 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 328 } 329 330 public String toCode(AllergyIntoleranceCategory code) { 331 if (code == AllergyIntoleranceCategory.FOOD) 332 return "food"; 333 if (code == AllergyIntoleranceCategory.MEDICATION) 334 return "medication"; 335 if (code == AllergyIntoleranceCategory.ENVIRONMENT) 336 return "environment"; 337 if (code == AllergyIntoleranceCategory.BIOLOGIC) 338 return "biologic"; 339 return "?"; 340 } 341 342 public String toSystem(AllergyIntoleranceCategory code) { 343 return code.getSystem(); 344 } 345 } 346 347 public enum AllergyIntoleranceCriticality { 348 /** 349 * Worst case result of a future exposure is not assessed to be life-threatening 350 * or having high potential for organ system failure. 351 */ 352 LOW, 353 /** 354 * Worst case result of a future exposure is assessed to be life-threatening or 355 * having high potential for organ system failure. 356 */ 357 HIGH, 358 /** 359 * Unable to assess the worst case result of a future exposure. 360 */ 361 UNABLETOASSESS, 362 /** 363 * added to help the parsers with the generic types 364 */ 365 NULL; 366 367 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 368 if (codeString == null || "".equals(codeString)) 369 return null; 370 if ("low".equals(codeString)) 371 return LOW; 372 if ("high".equals(codeString)) 373 return HIGH; 374 if ("unable-to-assess".equals(codeString)) 375 return UNABLETOASSESS; 376 if (Configuration.isAcceptInvalidEnums()) 377 return null; 378 else 379 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 380 } 381 382 public String toCode() { 383 switch (this) { 384 case LOW: 385 return "low"; 386 case HIGH: 387 return "high"; 388 case UNABLETOASSESS: 389 return "unable-to-assess"; 390 case NULL: 391 return null; 392 default: 393 return "?"; 394 } 395 } 396 397 public String getSystem() { 398 switch (this) { 399 case LOW: 400 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 401 case HIGH: 402 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 403 case UNABLETOASSESS: 404 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 405 case NULL: 406 return null; 407 default: 408 return "?"; 409 } 410 } 411 412 public String getDefinition() { 413 switch (this) { 414 case LOW: 415 return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 416 case HIGH: 417 return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 418 case UNABLETOASSESS: 419 return "Unable to assess the worst case result of a future exposure."; 420 case NULL: 421 return null; 422 default: 423 return "?"; 424 } 425 } 426 427 public String getDisplay() { 428 switch (this) { 429 case LOW: 430 return "Low Risk"; 431 case HIGH: 432 return "High Risk"; 433 case UNABLETOASSESS: 434 return "Unable to Assess Risk"; 435 case NULL: 436 return null; 437 default: 438 return "?"; 439 } 440 } 441 } 442 443 public static class AllergyIntoleranceCriticalityEnumFactory implements EnumFactory<AllergyIntoleranceCriticality> { 444 public AllergyIntoleranceCriticality fromCode(String codeString) throws IllegalArgumentException { 445 if (codeString == null || "".equals(codeString)) 446 if (codeString == null || "".equals(codeString)) 447 return null; 448 if ("low".equals(codeString)) 449 return AllergyIntoleranceCriticality.LOW; 450 if ("high".equals(codeString)) 451 return AllergyIntoleranceCriticality.HIGH; 452 if ("unable-to-assess".equals(codeString)) 453 return AllergyIntoleranceCriticality.UNABLETOASSESS; 454 throw new IllegalArgumentException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 455 } 456 457 public Enumeration<AllergyIntoleranceCriticality> fromType(PrimitiveType<?> code) throws FHIRException { 458 if (code == null) 459 return null; 460 if (code.isEmpty()) 461 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.NULL, code); 462 String codeString = code.asStringValue(); 463 if (codeString == null || "".equals(codeString)) 464 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.NULL, code); 465 if ("low".equals(codeString)) 466 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.LOW, code); 467 if ("high".equals(codeString)) 468 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.HIGH, code); 469 if ("unable-to-assess".equals(codeString)) 470 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.UNABLETOASSESS, code); 471 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 472 } 473 474 public String toCode(AllergyIntoleranceCriticality code) { 475 if (code == AllergyIntoleranceCriticality.LOW) 476 return "low"; 477 if (code == AllergyIntoleranceCriticality.HIGH) 478 return "high"; 479 if (code == AllergyIntoleranceCriticality.UNABLETOASSESS) 480 return "unable-to-assess"; 481 return "?"; 482 } 483 484 public String toSystem(AllergyIntoleranceCriticality code) { 485 return code.getSystem(); 486 } 487 } 488 489 public enum AllergyIntoleranceSeverity { 490 /** 491 * Causes mild physiological effects. 492 */ 493 MILD, 494 /** 495 * Causes moderate physiological effects. 496 */ 497 MODERATE, 498 /** 499 * Causes severe physiological effects. 500 */ 501 SEVERE, 502 /** 503 * added to help the parsers with the generic types 504 */ 505 NULL; 506 507 public static AllergyIntoleranceSeverity fromCode(String codeString) throws FHIRException { 508 if (codeString == null || "".equals(codeString)) 509 return null; 510 if ("mild".equals(codeString)) 511 return MILD; 512 if ("moderate".equals(codeString)) 513 return MODERATE; 514 if ("severe".equals(codeString)) 515 return SEVERE; 516 if (Configuration.isAcceptInvalidEnums()) 517 return null; 518 else 519 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 520 } 521 522 public String toCode() { 523 switch (this) { 524 case MILD: 525 return "mild"; 526 case MODERATE: 527 return "moderate"; 528 case SEVERE: 529 return "severe"; 530 case NULL: 531 return null; 532 default: 533 return "?"; 534 } 535 } 536 537 public String getSystem() { 538 switch (this) { 539 case MILD: 540 return "http://hl7.org/fhir/reaction-event-severity"; 541 case MODERATE: 542 return "http://hl7.org/fhir/reaction-event-severity"; 543 case SEVERE: 544 return "http://hl7.org/fhir/reaction-event-severity"; 545 case NULL: 546 return null; 547 default: 548 return "?"; 549 } 550 } 551 552 public String getDefinition() { 553 switch (this) { 554 case MILD: 555 return "Causes mild physiological effects."; 556 case MODERATE: 557 return "Causes moderate physiological effects."; 558 case SEVERE: 559 return "Causes severe physiological effects."; 560 case NULL: 561 return null; 562 default: 563 return "?"; 564 } 565 } 566 567 public String getDisplay() { 568 switch (this) { 569 case MILD: 570 return "Mild"; 571 case MODERATE: 572 return "Moderate"; 573 case SEVERE: 574 return "Severe"; 575 case NULL: 576 return null; 577 default: 578 return "?"; 579 } 580 } 581 } 582 583 public static class AllergyIntoleranceSeverityEnumFactory implements EnumFactory<AllergyIntoleranceSeverity> { 584 public AllergyIntoleranceSeverity fromCode(String codeString) throws IllegalArgumentException { 585 if (codeString == null || "".equals(codeString)) 586 if (codeString == null || "".equals(codeString)) 587 return null; 588 if ("mild".equals(codeString)) 589 return AllergyIntoleranceSeverity.MILD; 590 if ("moderate".equals(codeString)) 591 return AllergyIntoleranceSeverity.MODERATE; 592 if ("severe".equals(codeString)) 593 return AllergyIntoleranceSeverity.SEVERE; 594 throw new IllegalArgumentException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 595 } 596 597 public Enumeration<AllergyIntoleranceSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 598 if (code == null) 599 return null; 600 if (code.isEmpty()) 601 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.NULL, code); 602 String codeString = code.asStringValue(); 603 if (codeString == null || "".equals(codeString)) 604 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.NULL, code); 605 if ("mild".equals(codeString)) 606 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MILD, code); 607 if ("moderate".equals(codeString)) 608 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MODERATE, code); 609 if ("severe".equals(codeString)) 610 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.SEVERE, code); 611 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 612 } 613 614 public String toCode(AllergyIntoleranceSeverity code) { 615 if (code == AllergyIntoleranceSeverity.MILD) 616 return "mild"; 617 if (code == AllergyIntoleranceSeverity.MODERATE) 618 return "moderate"; 619 if (code == AllergyIntoleranceSeverity.SEVERE) 620 return "severe"; 621 return "?"; 622 } 623 624 public String toSystem(AllergyIntoleranceSeverity code) { 625 return code.getSystem(); 626 } 627 } 628 629 @Block() 630 public static class AllergyIntoleranceReactionComponent extends BackboneElement implements IBaseBackboneElement { 631 /** 632 * Identification of the specific substance (or pharmaceutical product) 633 * considered to be responsible for the Adverse Reaction event. Note: the 634 * substance for a specific reaction may be different from the substance 635 * identified as the cause of the risk, but it must be consistent with it. For 636 * instance, it may be a more specific substance (e.g. a brand medication) or a 637 * composite product that includes the identified substance. It must be 638 * clinically safe to only process the 'code' and ignore the 639 * 'reaction.substance'. If a receiving system is unable to confirm that 640 * AllergyIntolerance.reaction.substance falls within the semantic scope of 641 * AllergyIntolerance.code, then the receiving system should ignore 642 * AllergyIntolerance.reaction.substance. 643 */ 644 @Child(name = "substance", type = { 645 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 646 @Description(shortDefinition = "Specific substance or pharmaceutical product considered to be responsible for event", formalDefinition = "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.") 647 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/substance-code") 648 protected CodeableConcept substance; 649 650 /** 651 * Clinical symptoms and/or signs that are observed or associated with the 652 * adverse reaction event. 653 */ 654 @Child(name = "manifestation", type = { 655 CodeableConcept.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 656 @Description(shortDefinition = "Clinical symptoms/signs associated with the Event", formalDefinition = "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.") 657 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 658 protected List<CodeableConcept> manifestation; 659 660 /** 661 * Text description about the reaction as a whole, including details of the 662 * manifestation if required. 663 */ 664 @Child(name = "description", type = { 665 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 666 @Description(shortDefinition = "Description of the event as a whole", formalDefinition = "Text description about the reaction as a whole, including details of the manifestation if required.") 667 protected StringType description; 668 669 /** 670 * Record of the date and/or time of the onset of the Reaction. 671 */ 672 @Child(name = "onset", type = { 673 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 674 @Description(shortDefinition = "Date(/time) when manifestations showed", formalDefinition = "Record of the date and/or time of the onset of the Reaction.") 675 protected DateTimeType onset; 676 677 /** 678 * Clinical assessment of the severity of the reaction event as a whole, 679 * potentially considering multiple different manifestations. 680 */ 681 @Child(name = "severity", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 682 @Description(shortDefinition = "mild | moderate | severe (of event as a whole)", formalDefinition = "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.") 683 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reaction-event-severity") 684 protected Enumeration<AllergyIntoleranceSeverity> severity; 685 686 /** 687 * Identification of the route by which the subject was exposed to the 688 * substance. 689 */ 690 @Child(name = "exposureRoute", type = { 691 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 692 @Description(shortDefinition = "How the subject was exposed to the substance", formalDefinition = "Identification of the route by which the subject was exposed to the substance.") 693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/route-codes") 694 protected CodeableConcept exposureRoute; 695 696 /** 697 * Additional text about the adverse reaction event not captured in other 698 * fields. 699 */ 700 @Child(name = "note", type = { 701 Annotation.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 702 @Description(shortDefinition = "Text about event not captured in other fields", formalDefinition = "Additional text about the adverse reaction event not captured in other fields.") 703 protected List<Annotation> note; 704 705 private static final long serialVersionUID = -752118516L; 706 707 /** 708 * Constructor 709 */ 710 public AllergyIntoleranceReactionComponent() { 711 super(); 712 } 713 714 /** 715 * @return {@link #substance} (Identification of the specific substance (or 716 * pharmaceutical product) considered to be responsible for the Adverse 717 * Reaction event. Note: the substance for a specific reaction may be 718 * different from the substance identified as the cause of the risk, but 719 * it must be consistent with it. For instance, it may be a more 720 * specific substance (e.g. a brand medication) or a composite product 721 * that includes the identified substance. It must be clinically safe to 722 * only process the 'code' and ignore the 'reaction.substance'. If a 723 * receiving system is unable to confirm that 724 * AllergyIntolerance.reaction.substance falls within the semantic scope 725 * of AllergyIntolerance.code, then the receiving system should ignore 726 * AllergyIntolerance.reaction.substance.) 727 */ 728 public CodeableConcept getSubstance() { 729 if (this.substance == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.substance"); 732 else if (Configuration.doAutoCreate()) 733 this.substance = new CodeableConcept(); // cc 734 return this.substance; 735 } 736 737 public boolean hasSubstance() { 738 return this.substance != null && !this.substance.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #substance} (Identification of the specific substance (or 743 * pharmaceutical product) considered to be responsible for the 744 * Adverse Reaction event. Note: the substance for a specific 745 * reaction may be different from the substance identified as the 746 * cause of the risk, but it must be consistent with it. For 747 * instance, it may be a more specific substance (e.g. a brand 748 * medication) or a composite product that includes the identified 749 * substance. It must be clinically safe to only process the 'code' 750 * and ignore the 'reaction.substance'. If a receiving system is 751 * unable to confirm that AllergyIntolerance.reaction.substance 752 * falls within the semantic scope of AllergyIntolerance.code, then 753 * the receiving system should ignore 754 * AllergyIntolerance.reaction.substance.) 755 */ 756 public AllergyIntoleranceReactionComponent setSubstance(CodeableConcept value) { 757 this.substance = value; 758 return this; 759 } 760 761 /** 762 * @return {@link #manifestation} (Clinical symptoms and/or signs that are 763 * observed or associated with the adverse reaction event.) 764 */ 765 public List<CodeableConcept> getManifestation() { 766 if (this.manifestation == null) 767 this.manifestation = new ArrayList<CodeableConcept>(); 768 return this.manifestation; 769 } 770 771 /** 772 * @return Returns a reference to <code>this</code> for easy method chaining 773 */ 774 public AllergyIntoleranceReactionComponent setManifestation(List<CodeableConcept> theManifestation) { 775 this.manifestation = theManifestation; 776 return this; 777 } 778 779 public boolean hasManifestation() { 780 if (this.manifestation == null) 781 return false; 782 for (CodeableConcept item : this.manifestation) 783 if (!item.isEmpty()) 784 return true; 785 return false; 786 } 787 788 public CodeableConcept addManifestation() { // 3 789 CodeableConcept t = new CodeableConcept(); 790 if (this.manifestation == null) 791 this.manifestation = new ArrayList<CodeableConcept>(); 792 this.manifestation.add(t); 793 return t; 794 } 795 796 public AllergyIntoleranceReactionComponent addManifestation(CodeableConcept t) { // 3 797 if (t == null) 798 return this; 799 if (this.manifestation == null) 800 this.manifestation = new ArrayList<CodeableConcept>(); 801 this.manifestation.add(t); 802 return this; 803 } 804 805 /** 806 * @return The first repetition of repeating field {@link #manifestation}, 807 * creating it if it does not already exist 808 */ 809 public CodeableConcept getManifestationFirstRep() { 810 if (getManifestation().isEmpty()) { 811 addManifestation(); 812 } 813 return getManifestation().get(0); 814 } 815 816 /** 817 * @return {@link #description} (Text description about the reaction as a whole, 818 * including details of the manifestation if required.). This is the 819 * underlying object with id, value and extensions. The accessor 820 * "getDescription" gives direct access to the value 821 */ 822 public StringType getDescriptionElement() { 823 if (this.description == null) 824 if (Configuration.errorOnAutoCreate()) 825 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.description"); 826 else if (Configuration.doAutoCreate()) 827 this.description = new StringType(); // bb 828 return this.description; 829 } 830 831 public boolean hasDescriptionElement() { 832 return this.description != null && !this.description.isEmpty(); 833 } 834 835 public boolean hasDescription() { 836 return this.description != null && !this.description.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #description} (Text description about the reaction as a 841 * whole, including details of the manifestation if required.). 842 * This is the underlying object with id, value and extensions. The 843 * accessor "getDescription" gives direct access to the value 844 */ 845 public AllergyIntoleranceReactionComponent setDescriptionElement(StringType value) { 846 this.description = value; 847 return this; 848 } 849 850 /** 851 * @return Text description about the reaction as a whole, including details of 852 * the manifestation if required. 853 */ 854 public String getDescription() { 855 return this.description == null ? null : this.description.getValue(); 856 } 857 858 /** 859 * @param value Text description about the reaction as a whole, including 860 * details of the manifestation if required. 861 */ 862 public AllergyIntoleranceReactionComponent setDescription(String value) { 863 if (Utilities.noString(value)) 864 this.description = null; 865 else { 866 if (this.description == null) 867 this.description = new StringType(); 868 this.description.setValue(value); 869 } 870 return this; 871 } 872 873 /** 874 * @return {@link #onset} (Record of the date and/or time of the onset of the 875 * Reaction.). This is the underlying object with id, value and 876 * extensions. The accessor "getOnset" gives direct access to the value 877 */ 878 public DateTimeType getOnsetElement() { 879 if (this.onset == null) 880 if (Configuration.errorOnAutoCreate()) 881 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.onset"); 882 else if (Configuration.doAutoCreate()) 883 this.onset = new DateTimeType(); // bb 884 return this.onset; 885 } 886 887 public boolean hasOnsetElement() { 888 return this.onset != null && !this.onset.isEmpty(); 889 } 890 891 public boolean hasOnset() { 892 return this.onset != null && !this.onset.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #onset} (Record of the date and/or time of the onset of 897 * the Reaction.). This is the underlying object with id, value and 898 * extensions. The accessor "getOnset" gives direct access to the 899 * value 900 */ 901 public AllergyIntoleranceReactionComponent setOnsetElement(DateTimeType value) { 902 this.onset = value; 903 return this; 904 } 905 906 /** 907 * @return Record of the date and/or time of the onset of the Reaction. 908 */ 909 public Date getOnset() { 910 return this.onset == null ? null : this.onset.getValue(); 911 } 912 913 /** 914 * @param value Record of the date and/or time of the onset of the Reaction. 915 */ 916 public AllergyIntoleranceReactionComponent setOnset(Date value) { 917 if (value == null) 918 this.onset = null; 919 else { 920 if (this.onset == null) 921 this.onset = new DateTimeType(); 922 this.onset.setValue(value); 923 } 924 return this; 925 } 926 927 /** 928 * @return {@link #severity} (Clinical assessment of the severity of the 929 * reaction event as a whole, potentially considering multiple different 930 * manifestations.). This is the underlying object with id, value and 931 * extensions. The accessor "getSeverity" gives direct access to the 932 * value 933 */ 934 public Enumeration<AllergyIntoleranceSeverity> getSeverityElement() { 935 if (this.severity == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.severity"); 938 else if (Configuration.doAutoCreate()) 939 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); // bb 940 return this.severity; 941 } 942 943 public boolean hasSeverityElement() { 944 return this.severity != null && !this.severity.isEmpty(); 945 } 946 947 public boolean hasSeverity() { 948 return this.severity != null && !this.severity.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #severity} (Clinical assessment of the severity of the 953 * reaction event as a whole, potentially considering multiple 954 * different manifestations.). This is the underlying object with 955 * id, value and extensions. The accessor "getSeverity" gives 956 * direct access to the value 957 */ 958 public AllergyIntoleranceReactionComponent setSeverityElement(Enumeration<AllergyIntoleranceSeverity> value) { 959 this.severity = value; 960 return this; 961 } 962 963 /** 964 * @return Clinical assessment of the severity of the reaction event as a whole, 965 * potentially considering multiple different manifestations. 966 */ 967 public AllergyIntoleranceSeverity getSeverity() { 968 return this.severity == null ? null : this.severity.getValue(); 969 } 970 971 /** 972 * @param value Clinical assessment of the severity of the reaction event as a 973 * whole, potentially considering multiple different 974 * manifestations. 975 */ 976 public AllergyIntoleranceReactionComponent setSeverity(AllergyIntoleranceSeverity value) { 977 if (value == null) 978 this.severity = null; 979 else { 980 if (this.severity == null) 981 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); 982 this.severity.setValue(value); 983 } 984 return this; 985 } 986 987 /** 988 * @return {@link #exposureRoute} (Identification of the route by which the 989 * subject was exposed to the substance.) 990 */ 991 public CodeableConcept getExposureRoute() { 992 if (this.exposureRoute == null) 993 if (Configuration.errorOnAutoCreate()) 994 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.exposureRoute"); 995 else if (Configuration.doAutoCreate()) 996 this.exposureRoute = new CodeableConcept(); // cc 997 return this.exposureRoute; 998 } 999 1000 public boolean hasExposureRoute() { 1001 return this.exposureRoute != null && !this.exposureRoute.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #exposureRoute} (Identification of the route by which the 1006 * subject was exposed to the substance.) 1007 */ 1008 public AllergyIntoleranceReactionComponent setExposureRoute(CodeableConcept value) { 1009 this.exposureRoute = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return {@link #note} (Additional text about the adverse reaction event not 1015 * captured in other fields.) 1016 */ 1017 public List<Annotation> getNote() { 1018 if (this.note == null) 1019 this.note = new ArrayList<Annotation>(); 1020 return this.note; 1021 } 1022 1023 /** 1024 * @return Returns a reference to <code>this</code> for easy method chaining 1025 */ 1026 public AllergyIntoleranceReactionComponent setNote(List<Annotation> theNote) { 1027 this.note = theNote; 1028 return this; 1029 } 1030 1031 public boolean hasNote() { 1032 if (this.note == null) 1033 return false; 1034 for (Annotation item : this.note) 1035 if (!item.isEmpty()) 1036 return true; 1037 return false; 1038 } 1039 1040 public Annotation addNote() { // 3 1041 Annotation t = new Annotation(); 1042 if (this.note == null) 1043 this.note = new ArrayList<Annotation>(); 1044 this.note.add(t); 1045 return t; 1046 } 1047 1048 public AllergyIntoleranceReactionComponent addNote(Annotation t) { // 3 1049 if (t == null) 1050 return this; 1051 if (this.note == null) 1052 this.note = new ArrayList<Annotation>(); 1053 this.note.add(t); 1054 return this; 1055 } 1056 1057 /** 1058 * @return The first repetition of repeating field {@link #note}, creating it if 1059 * it does not already exist 1060 */ 1061 public Annotation getNoteFirstRep() { 1062 if (getNote().isEmpty()) { 1063 addNote(); 1064 } 1065 return getNote().get(0); 1066 } 1067 1068 protected void listChildren(List<Property> children) { 1069 super.listChildren(children); 1070 children.add(new Property("substance", "CodeableConcept", 1071 "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 1072 0, 1, substance)); 1073 children.add(new Property("manifestation", "CodeableConcept", 1074 "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, 1075 java.lang.Integer.MAX_VALUE, manifestation)); 1076 children.add(new Property("description", "string", 1077 "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, 1078 description)); 1079 children.add(new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 0, 1080 1, onset)); 1081 children.add(new Property("severity", "code", 1082 "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 1083 0, 1, severity)); 1084 children.add(new Property("exposureRoute", "CodeableConcept", 1085 "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute)); 1086 children.add(new Property("note", "Annotation", 1087 "Additional text about the adverse reaction event not captured in other fields.", 0, 1088 java.lang.Integer.MAX_VALUE, note)); 1089 } 1090 1091 @Override 1092 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1093 switch (_hash) { 1094 case 530040176: 1095 /* substance */ return new Property("substance", "CodeableConcept", 1096 "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 1097 0, 1, substance); 1098 case 1115984422: 1099 /* manifestation */ return new Property("manifestation", "CodeableConcept", 1100 "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, 1101 java.lang.Integer.MAX_VALUE, manifestation); 1102 case -1724546052: 1103 /* description */ return new Property("description", "string", 1104 "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, 1105 description); 1106 case 105901603: 1107 /* onset */ return new Property("onset", "dateTime", 1108 "Record of the date and/or time of the onset of the Reaction.", 0, 1, onset); 1109 case 1478300413: 1110 /* severity */ return new Property("severity", "code", 1111 "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 1112 0, 1, severity); 1113 case 421286274: 1114 /* exposureRoute */ return new Property("exposureRoute", "CodeableConcept", 1115 "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute); 1116 case 3387378: 1117 /* note */ return new Property("note", "Annotation", 1118 "Additional text about the adverse reaction event not captured in other fields.", 0, 1119 java.lang.Integer.MAX_VALUE, note); 1120 default: 1121 return super.getNamedProperty(_hash, _name, _checkValid); 1122 } 1123 1124 } 1125 1126 @Override 1127 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1128 switch (hash) { 1129 case 530040176: 1130 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // CodeableConcept 1131 case 1115984422: 1132 /* manifestation */ return this.manifestation == null ? new Base[0] 1133 : this.manifestation.toArray(new Base[this.manifestation.size()]); // CodeableConcept 1134 case -1724546052: 1135 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1136 case 105901603: 1137 /* onset */ return this.onset == null ? new Base[0] : new Base[] { this.onset }; // DateTimeType 1138 case 1478300413: 1139 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<AllergyIntoleranceSeverity> 1140 case 421286274: 1141 /* exposureRoute */ return this.exposureRoute == null ? new Base[0] : new Base[] { this.exposureRoute }; // CodeableConcept 1142 case 3387378: 1143 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1144 default: 1145 return super.getProperty(hash, name, checkValid); 1146 } 1147 1148 } 1149 1150 @Override 1151 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1152 switch (hash) { 1153 case 530040176: // substance 1154 this.substance = castToCodeableConcept(value); // CodeableConcept 1155 return value; 1156 case 1115984422: // manifestation 1157 this.getManifestation().add(castToCodeableConcept(value)); // CodeableConcept 1158 return value; 1159 case -1724546052: // description 1160 this.description = castToString(value); // StringType 1161 return value; 1162 case 105901603: // onset 1163 this.onset = castToDateTime(value); // DateTimeType 1164 return value; 1165 case 1478300413: // severity 1166 value = new AllergyIntoleranceSeverityEnumFactory().fromType(castToCode(value)); 1167 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1168 return value; 1169 case 421286274: // exposureRoute 1170 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1171 return value; 1172 case 3387378: // note 1173 this.getNote().add(castToAnnotation(value)); // Annotation 1174 return value; 1175 default: 1176 return super.setProperty(hash, name, value); 1177 } 1178 1179 } 1180 1181 @Override 1182 public Base setProperty(String name, Base value) throws FHIRException { 1183 if (name.equals("substance")) { 1184 this.substance = castToCodeableConcept(value); // CodeableConcept 1185 } else if (name.equals("manifestation")) { 1186 this.getManifestation().add(castToCodeableConcept(value)); 1187 } else if (name.equals("description")) { 1188 this.description = castToString(value); // StringType 1189 } else if (name.equals("onset")) { 1190 this.onset = castToDateTime(value); // DateTimeType 1191 } else if (name.equals("severity")) { 1192 value = new AllergyIntoleranceSeverityEnumFactory().fromType(castToCode(value)); 1193 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1194 } else if (name.equals("exposureRoute")) { 1195 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1196 } else if (name.equals("note")) { 1197 this.getNote().add(castToAnnotation(value)); 1198 } else 1199 return super.setProperty(name, value); 1200 return value; 1201 } 1202 1203 @Override 1204 public Base makeProperty(int hash, String name) throws FHIRException { 1205 switch (hash) { 1206 case 530040176: 1207 return getSubstance(); 1208 case 1115984422: 1209 return addManifestation(); 1210 case -1724546052: 1211 return getDescriptionElement(); 1212 case 105901603: 1213 return getOnsetElement(); 1214 case 1478300413: 1215 return getSeverityElement(); 1216 case 421286274: 1217 return getExposureRoute(); 1218 case 3387378: 1219 return addNote(); 1220 default: 1221 return super.makeProperty(hash, name); 1222 } 1223 1224 } 1225 1226 @Override 1227 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1228 switch (hash) { 1229 case 530040176: 1230 /* substance */ return new String[] { "CodeableConcept" }; 1231 case 1115984422: 1232 /* manifestation */ return new String[] { "CodeableConcept" }; 1233 case -1724546052: 1234 /* description */ return new String[] { "string" }; 1235 case 105901603: 1236 /* onset */ return new String[] { "dateTime" }; 1237 case 1478300413: 1238 /* severity */ return new String[] { "code" }; 1239 case 421286274: 1240 /* exposureRoute */ return new String[] { "CodeableConcept" }; 1241 case 3387378: 1242 /* note */ return new String[] { "Annotation" }; 1243 default: 1244 return super.getTypesForProperty(hash, name); 1245 } 1246 1247 } 1248 1249 @Override 1250 public Base addChild(String name) throws FHIRException { 1251 if (name.equals("substance")) { 1252 this.substance = new CodeableConcept(); 1253 return this.substance; 1254 } else if (name.equals("manifestation")) { 1255 return addManifestation(); 1256 } else if (name.equals("description")) { 1257 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.description"); 1258 } else if (name.equals("onset")) { 1259 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 1260 } else if (name.equals("severity")) { 1261 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.severity"); 1262 } else if (name.equals("exposureRoute")) { 1263 this.exposureRoute = new CodeableConcept(); 1264 return this.exposureRoute; 1265 } else if (name.equals("note")) { 1266 return addNote(); 1267 } else 1268 return super.addChild(name); 1269 } 1270 1271 public AllergyIntoleranceReactionComponent copy() { 1272 AllergyIntoleranceReactionComponent dst = new AllergyIntoleranceReactionComponent(); 1273 copyValues(dst); 1274 return dst; 1275 } 1276 1277 public void copyValues(AllergyIntoleranceReactionComponent dst) { 1278 super.copyValues(dst); 1279 dst.substance = substance == null ? null : substance.copy(); 1280 if (manifestation != null) { 1281 dst.manifestation = new ArrayList<CodeableConcept>(); 1282 for (CodeableConcept i : manifestation) 1283 dst.manifestation.add(i.copy()); 1284 } 1285 ; 1286 dst.description = description == null ? null : description.copy(); 1287 dst.onset = onset == null ? null : onset.copy(); 1288 dst.severity = severity == null ? null : severity.copy(); 1289 dst.exposureRoute = exposureRoute == null ? null : exposureRoute.copy(); 1290 if (note != null) { 1291 dst.note = new ArrayList<Annotation>(); 1292 for (Annotation i : note) 1293 dst.note.add(i.copy()); 1294 } 1295 ; 1296 } 1297 1298 @Override 1299 public boolean equalsDeep(Base other_) { 1300 if (!super.equalsDeep(other_)) 1301 return false; 1302 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1303 return false; 1304 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1305 return compareDeep(substance, o.substance, true) && compareDeep(manifestation, o.manifestation, true) 1306 && compareDeep(description, o.description, true) && compareDeep(onset, o.onset, true) 1307 && compareDeep(severity, o.severity, true) && compareDeep(exposureRoute, o.exposureRoute, true) 1308 && compareDeep(note, o.note, true); 1309 } 1310 1311 @Override 1312 public boolean equalsShallow(Base other_) { 1313 if (!super.equalsShallow(other_)) 1314 return false; 1315 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1316 return false; 1317 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1318 return compareValues(description, o.description, true) && compareValues(onset, o.onset, true) 1319 && compareValues(severity, o.severity, true); 1320 } 1321 1322 public boolean isEmpty() { 1323 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, manifestation, description, onset, 1324 severity, exposureRoute, note); 1325 } 1326 1327 public String fhirType() { 1328 return "AllergyIntolerance.reaction"; 1329 1330 } 1331 1332 } 1333 1334 /** 1335 * Business identifiers assigned to this AllergyIntolerance by the performer or 1336 * other systems which remain constant as the resource is updated and propagates 1337 * from server to server. 1338 */ 1339 @Child(name = "identifier", type = { 1340 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1341 @Description(shortDefinition = "External ids for this item", formalDefinition = "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 1342 protected List<Identifier> identifier; 1343 1344 /** 1345 * The clinical status of the allergy or intolerance. 1346 */ 1347 @Child(name = "clinicalStatus", type = { 1348 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1349 @Description(shortDefinition = "active | inactive | resolved", formalDefinition = "The clinical status of the allergy or intolerance.") 1350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergyintolerance-clinical") 1351 protected CodeableConcept clinicalStatus; 1352 1353 /** 1354 * Assertion about certainty associated with the propensity, or potential risk, 1355 * of a reaction to the identified substance (including pharmaceutical product). 1356 */ 1357 @Child(name = "verificationStatus", type = { 1358 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 1359 @Description(shortDefinition = "unconfirmed | confirmed | refuted | entered-in-error", formalDefinition = "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).") 1360 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergyintolerance-verification") 1361 protected CodeableConcept verificationStatus; 1362 1363 /** 1364 * Identification of the underlying physiological mechanism for the reaction 1365 * risk. 1366 */ 1367 @Child(name = "type", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1368 @Description(shortDefinition = "allergy | intolerance - Underlying mechanism (if known)", formalDefinition = "Identification of the underlying physiological mechanism for the reaction risk.") 1369 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergy-intolerance-type") 1370 protected Enumeration<AllergyIntoleranceType> type; 1371 1372 /** 1373 * Category of the identified substance. 1374 */ 1375 @Child(name = "category", type = { 1376 CodeType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1377 @Description(shortDefinition = "food | medication | environment | biologic", formalDefinition = "Category of the identified substance.") 1378 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergy-intolerance-category") 1379 protected List<Enumeration<AllergyIntoleranceCategory>> category; 1380 1381 /** 1382 * Estimate of the potential clinical harm, or seriousness, of the reaction to 1383 * the identified substance. 1384 */ 1385 @Child(name = "criticality", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1386 @Description(shortDefinition = "low | high | unable-to-assess", formalDefinition = "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.") 1387 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergy-intolerance-criticality") 1388 protected Enumeration<AllergyIntoleranceCriticality> criticality; 1389 1390 /** 1391 * Code for an allergy or intolerance statement (either a positive or a 1392 * negated/excluded statement). This may be a code for a substance or 1393 * pharmaceutical product that is considered to be responsible for the adverse 1394 * reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., 1395 * "Latex allergy"), or a negated/excluded code for a specific substance or 1396 * class (e.g., "No latex allergy") or a general or categorical negated 1397 * statement (e.g., "No known allergy", "No known drug allergies"). Note: the 1398 * substance for a specific reaction may be different from the substance 1399 * identified as the cause of the risk, but it must be consistent with it. For 1400 * instance, it may be a more specific substance (e.g. a brand medication) or a 1401 * composite product that includes the identified substance. It must be 1402 * clinically safe to only process the 'code' and ignore the 1403 * 'reaction.substance'. If a receiving system is unable to confirm that 1404 * AllergyIntolerance.reaction.substance falls within the semantic scope of 1405 * AllergyIntolerance.code, then the receiving system should ignore 1406 * AllergyIntolerance.reaction.substance. 1407 */ 1408 @Child(name = "code", type = { CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1409 @Description(shortDefinition = "Code that identifies the allergy or intolerance", formalDefinition = "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.") 1410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergyintolerance-code") 1411 protected CodeableConcept code; 1412 1413 /** 1414 * The patient who has the allergy or intolerance. 1415 */ 1416 @Child(name = "patient", type = { Patient.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1417 @Description(shortDefinition = "Who the sensitivity is for", formalDefinition = "The patient who has the allergy or intolerance.") 1418 protected Reference patient; 1419 1420 /** 1421 * The actual object that is the target of the reference (The patient who has 1422 * the allergy or intolerance.) 1423 */ 1424 protected Patient patientTarget; 1425 1426 /** 1427 * The encounter when the allergy or intolerance was asserted. 1428 */ 1429 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1430 @Description(shortDefinition = "Encounter when the allergy or intolerance was asserted", formalDefinition = "The encounter when the allergy or intolerance was asserted.") 1431 protected Reference encounter; 1432 1433 /** 1434 * The actual object that is the target of the reference (The encounter when the 1435 * allergy or intolerance was asserted.) 1436 */ 1437 protected Encounter encounterTarget; 1438 1439 /** 1440 * Estimated or actual date, date-time, or age when allergy or intolerance was 1441 * identified. 1442 */ 1443 @Child(name = "onset", type = { DateTimeType.class, Age.class, Period.class, Range.class, 1444 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1445 @Description(shortDefinition = "When allergy or intolerance was identified", formalDefinition = "Estimated or actual date, date-time, or age when allergy or intolerance was identified.") 1446 protected Type onset; 1447 1448 /** 1449 * The recordedDate represents when this particular AllergyIntolerance record 1450 * was created in the system, which is often a system-generated date. 1451 */ 1452 @Child(name = "recordedDate", type = { 1453 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1454 @Description(shortDefinition = "Date first version of the resource instance was recorded", formalDefinition = "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.") 1455 protected DateTimeType recordedDate; 1456 1457 /** 1458 * Individual who recorded the record and takes responsibility for its content. 1459 */ 1460 @Child(name = "recorder", type = { Practitioner.class, PractitionerRole.class, Patient.class, 1461 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1462 @Description(shortDefinition = "Who recorded the sensitivity", formalDefinition = "Individual who recorded the record and takes responsibility for its content.") 1463 protected Reference recorder; 1464 1465 /** 1466 * The actual object that is the target of the reference (Individual who 1467 * recorded the record and takes responsibility for its content.) 1468 */ 1469 protected Resource recorderTarget; 1470 1471 /** 1472 * The source of the information about the allergy that is recorded. 1473 */ 1474 @Child(name = "asserter", type = { Patient.class, RelatedPerson.class, Practitioner.class, 1475 PractitionerRole.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1476 @Description(shortDefinition = "Source of the information about the allergy", formalDefinition = "The source of the information about the allergy that is recorded.") 1477 protected Reference asserter; 1478 1479 /** 1480 * The actual object that is the target of the reference (The source of the 1481 * information about the allergy that is recorded.) 1482 */ 1483 protected Resource asserterTarget; 1484 1485 /** 1486 * Represents the date and/or time of the last known occurrence of a reaction 1487 * event. 1488 */ 1489 @Child(name = "lastOccurrence", type = { 1490 DateTimeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1491 @Description(shortDefinition = "Date(/time) of last known occurrence of a reaction", formalDefinition = "Represents the date and/or time of the last known occurrence of a reaction event.") 1492 protected DateTimeType lastOccurrence; 1493 1494 /** 1495 * Additional narrative about the propensity for the Adverse Reaction, not 1496 * captured in other fields. 1497 */ 1498 @Child(name = "note", type = { 1499 Annotation.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1500 @Description(shortDefinition = "Additional text not captured in other fields", formalDefinition = "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.") 1501 protected List<Annotation> note; 1502 1503 /** 1504 * Details about each adverse reaction event linked to exposure to the 1505 * identified substance. 1506 */ 1507 @Child(name = "reaction", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1508 @Description(shortDefinition = "Adverse Reaction Events linked to exposure to substance", formalDefinition = "Details about each adverse reaction event linked to exposure to the identified substance.") 1509 protected List<AllergyIntoleranceReactionComponent> reaction; 1510 1511 private static final long serialVersionUID = 393192289L; 1512 1513 /** 1514 * Constructor 1515 */ 1516 public AllergyIntolerance() { 1517 super(); 1518 } 1519 1520 /** 1521 * Constructor 1522 */ 1523 public AllergyIntolerance(Reference patient) { 1524 super(); 1525 this.patient = patient; 1526 } 1527 1528 /** 1529 * @return {@link #identifier} (Business identifiers assigned to this 1530 * AllergyIntolerance by the performer or other systems which remain 1531 * constant as the resource is updated and propagates from server to 1532 * server.) 1533 */ 1534 public List<Identifier> getIdentifier() { 1535 if (this.identifier == null) 1536 this.identifier = new ArrayList<Identifier>(); 1537 return this.identifier; 1538 } 1539 1540 /** 1541 * @return Returns a reference to <code>this</code> for easy method chaining 1542 */ 1543 public AllergyIntolerance setIdentifier(List<Identifier> theIdentifier) { 1544 this.identifier = theIdentifier; 1545 return this; 1546 } 1547 1548 public boolean hasIdentifier() { 1549 if (this.identifier == null) 1550 return false; 1551 for (Identifier item : this.identifier) 1552 if (!item.isEmpty()) 1553 return true; 1554 return false; 1555 } 1556 1557 public Identifier addIdentifier() { // 3 1558 Identifier t = new Identifier(); 1559 if (this.identifier == null) 1560 this.identifier = new ArrayList<Identifier>(); 1561 this.identifier.add(t); 1562 return t; 1563 } 1564 1565 public AllergyIntolerance addIdentifier(Identifier t) { // 3 1566 if (t == null) 1567 return this; 1568 if (this.identifier == null) 1569 this.identifier = new ArrayList<Identifier>(); 1570 this.identifier.add(t); 1571 return this; 1572 } 1573 1574 /** 1575 * @return The first repetition of repeating field {@link #identifier}, creating 1576 * it if it does not already exist 1577 */ 1578 public Identifier getIdentifierFirstRep() { 1579 if (getIdentifier().isEmpty()) { 1580 addIdentifier(); 1581 } 1582 return getIdentifier().get(0); 1583 } 1584 1585 /** 1586 * @return {@link #clinicalStatus} (The clinical status of the allergy or 1587 * intolerance.) 1588 */ 1589 public CodeableConcept getClinicalStatus() { 1590 if (this.clinicalStatus == null) 1591 if (Configuration.errorOnAutoCreate()) 1592 throw new Error("Attempt to auto-create AllergyIntolerance.clinicalStatus"); 1593 else if (Configuration.doAutoCreate()) 1594 this.clinicalStatus = new CodeableConcept(); // cc 1595 return this.clinicalStatus; 1596 } 1597 1598 public boolean hasClinicalStatus() { 1599 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1600 } 1601 1602 /** 1603 * @param value {@link #clinicalStatus} (The clinical status of the allergy or 1604 * intolerance.) 1605 */ 1606 public AllergyIntolerance setClinicalStatus(CodeableConcept value) { 1607 this.clinicalStatus = value; 1608 return this; 1609 } 1610 1611 /** 1612 * @return {@link #verificationStatus} (Assertion about certainty associated 1613 * with the propensity, or potential risk, of a reaction to the 1614 * identified substance (including pharmaceutical product).) 1615 */ 1616 public CodeableConcept getVerificationStatus() { 1617 if (this.verificationStatus == null) 1618 if (Configuration.errorOnAutoCreate()) 1619 throw new Error("Attempt to auto-create AllergyIntolerance.verificationStatus"); 1620 else if (Configuration.doAutoCreate()) 1621 this.verificationStatus = new CodeableConcept(); // cc 1622 return this.verificationStatus; 1623 } 1624 1625 public boolean hasVerificationStatus() { 1626 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1627 } 1628 1629 /** 1630 * @param value {@link #verificationStatus} (Assertion about certainty 1631 * associated with the propensity, or potential risk, of a reaction 1632 * to the identified substance (including pharmaceutical product).) 1633 */ 1634 public AllergyIntolerance setVerificationStatus(CodeableConcept value) { 1635 this.verificationStatus = value; 1636 return this; 1637 } 1638 1639 /** 1640 * @return {@link #type} (Identification of the underlying physiological 1641 * mechanism for the reaction risk.). This is the underlying object with 1642 * id, value and extensions. The accessor "getType" gives direct access 1643 * to the value 1644 */ 1645 public Enumeration<AllergyIntoleranceType> getTypeElement() { 1646 if (this.type == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create AllergyIntolerance.type"); 1649 else if (Configuration.doAutoCreate()) 1650 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); // bb 1651 return this.type; 1652 } 1653 1654 public boolean hasTypeElement() { 1655 return this.type != null && !this.type.isEmpty(); 1656 } 1657 1658 public boolean hasType() { 1659 return this.type != null && !this.type.isEmpty(); 1660 } 1661 1662 /** 1663 * @param value {@link #type} (Identification of the underlying physiological 1664 * mechanism for the reaction risk.). This is the underlying object 1665 * with id, value and extensions. The accessor "getType" gives 1666 * direct access to the value 1667 */ 1668 public AllergyIntolerance setTypeElement(Enumeration<AllergyIntoleranceType> value) { 1669 this.type = value; 1670 return this; 1671 } 1672 1673 /** 1674 * @return Identification of the underlying physiological mechanism for the 1675 * reaction risk. 1676 */ 1677 public AllergyIntoleranceType getType() { 1678 return this.type == null ? null : this.type.getValue(); 1679 } 1680 1681 /** 1682 * @param value Identification of the underlying physiological mechanism for the 1683 * reaction risk. 1684 */ 1685 public AllergyIntolerance setType(AllergyIntoleranceType value) { 1686 if (value == null) 1687 this.type = null; 1688 else { 1689 if (this.type == null) 1690 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); 1691 this.type.setValue(value); 1692 } 1693 return this; 1694 } 1695 1696 /** 1697 * @return {@link #category} (Category of the identified substance.) 1698 */ 1699 public List<Enumeration<AllergyIntoleranceCategory>> getCategory() { 1700 if (this.category == null) 1701 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1702 return this.category; 1703 } 1704 1705 /** 1706 * @return Returns a reference to <code>this</code> for easy method chaining 1707 */ 1708 public AllergyIntolerance setCategory(List<Enumeration<AllergyIntoleranceCategory>> theCategory) { 1709 this.category = theCategory; 1710 return this; 1711 } 1712 1713 public boolean hasCategory() { 1714 if (this.category == null) 1715 return false; 1716 for (Enumeration<AllergyIntoleranceCategory> item : this.category) 1717 if (!item.isEmpty()) 1718 return true; 1719 return false; 1720 } 1721 1722 /** 1723 * @return {@link #category} (Category of the identified substance.) 1724 */ 1725 public Enumeration<AllergyIntoleranceCategory> addCategoryElement() {// 2 1726 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>( 1727 new AllergyIntoleranceCategoryEnumFactory()); 1728 if (this.category == null) 1729 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1730 this.category.add(t); 1731 return t; 1732 } 1733 1734 /** 1735 * @param value {@link #category} (Category of the identified substance.) 1736 */ 1737 public AllergyIntolerance addCategory(AllergyIntoleranceCategory value) { // 1 1738 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>( 1739 new AllergyIntoleranceCategoryEnumFactory()); 1740 t.setValue(value); 1741 if (this.category == null) 1742 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1743 this.category.add(t); 1744 return this; 1745 } 1746 1747 /** 1748 * @param value {@link #category} (Category of the identified substance.) 1749 */ 1750 public boolean hasCategory(AllergyIntoleranceCategory value) { 1751 if (this.category == null) 1752 return false; 1753 for (Enumeration<AllergyIntoleranceCategory> v : this.category) 1754 if (v.getValue().equals(value)) // code 1755 return true; 1756 return false; 1757 } 1758 1759 /** 1760 * @return {@link #criticality} (Estimate of the potential clinical harm, or 1761 * seriousness, of the reaction to the identified substance.). This is 1762 * the underlying object with id, value and extensions. The accessor 1763 * "getCriticality" gives direct access to the value 1764 */ 1765 public Enumeration<AllergyIntoleranceCriticality> getCriticalityElement() { 1766 if (this.criticality == null) 1767 if (Configuration.errorOnAutoCreate()) 1768 throw new Error("Attempt to auto-create AllergyIntolerance.criticality"); 1769 else if (Configuration.doAutoCreate()) 1770 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 1771 new AllergyIntoleranceCriticalityEnumFactory()); // bb 1772 return this.criticality; 1773 } 1774 1775 public boolean hasCriticalityElement() { 1776 return this.criticality != null && !this.criticality.isEmpty(); 1777 } 1778 1779 public boolean hasCriticality() { 1780 return this.criticality != null && !this.criticality.isEmpty(); 1781 } 1782 1783 /** 1784 * @param value {@link #criticality} (Estimate of the potential clinical harm, 1785 * or seriousness, of the reaction to the identified substance.). 1786 * This is the underlying object with id, value and extensions. The 1787 * accessor "getCriticality" gives direct access to the value 1788 */ 1789 public AllergyIntolerance setCriticalityElement(Enumeration<AllergyIntoleranceCriticality> value) { 1790 this.criticality = value; 1791 return this; 1792 } 1793 1794 /** 1795 * @return Estimate of the potential clinical harm, or seriousness, of the 1796 * reaction to the identified substance. 1797 */ 1798 public AllergyIntoleranceCriticality getCriticality() { 1799 return this.criticality == null ? null : this.criticality.getValue(); 1800 } 1801 1802 /** 1803 * @param value Estimate of the potential clinical harm, or seriousness, of the 1804 * reaction to the identified substance. 1805 */ 1806 public AllergyIntolerance setCriticality(AllergyIntoleranceCriticality value) { 1807 if (value == null) 1808 this.criticality = null; 1809 else { 1810 if (this.criticality == null) 1811 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 1812 new AllergyIntoleranceCriticalityEnumFactory()); 1813 this.criticality.setValue(value); 1814 } 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #code} (Code for an allergy or intolerance statement (either a 1820 * positive or a negated/excluded statement). This may be a code for a 1821 * substance or pharmaceutical product that is considered to be 1822 * responsible for the adverse reaction risk (e.g., "Latex"), an allergy 1823 * or intolerance condition (e.g., "Latex allergy"), or a 1824 * negated/excluded code for a specific substance or class (e.g., "No 1825 * latex allergy") or a general or categorical negated statement (e.g., 1826 * "No known allergy", "No known drug allergies"). Note: the substance 1827 * for a specific reaction may be different from the substance 1828 * identified as the cause of the risk, but it must be consistent with 1829 * it. For instance, it may be a more specific substance (e.g. a brand 1830 * medication) or a composite product that includes the identified 1831 * substance. It must be clinically safe to only process the 'code' and 1832 * ignore the 'reaction.substance'. If a receiving system is unable to 1833 * confirm that AllergyIntolerance.reaction.substance falls within the 1834 * semantic scope of AllergyIntolerance.code, then the receiving system 1835 * should ignore AllergyIntolerance.reaction.substance.) 1836 */ 1837 public CodeableConcept getCode() { 1838 if (this.code == null) 1839 if (Configuration.errorOnAutoCreate()) 1840 throw new Error("Attempt to auto-create AllergyIntolerance.code"); 1841 else if (Configuration.doAutoCreate()) 1842 this.code = new CodeableConcept(); // cc 1843 return this.code; 1844 } 1845 1846 public boolean hasCode() { 1847 return this.code != null && !this.code.isEmpty(); 1848 } 1849 1850 /** 1851 * @param value {@link #code} (Code for an allergy or intolerance statement 1852 * (either a positive or a negated/excluded statement). This may be 1853 * a code for a substance or pharmaceutical product that is 1854 * considered to be responsible for the adverse reaction risk 1855 * (e.g., "Latex"), an allergy or intolerance condition (e.g., 1856 * "Latex allergy"), or a negated/excluded code for a specific 1857 * substance or class (e.g., "No latex allergy") or a general or 1858 * categorical negated statement (e.g., "No known allergy", "No 1859 * known drug allergies"). Note: the substance for a specific 1860 * reaction may be different from the substance identified as the 1861 * cause of the risk, but it must be consistent with it. For 1862 * instance, it may be a more specific substance (e.g. a brand 1863 * medication) or a composite product that includes the identified 1864 * substance. It must be clinically safe to only process the 'code' 1865 * and ignore the 'reaction.substance'. If a receiving system is 1866 * unable to confirm that AllergyIntolerance.reaction.substance 1867 * falls within the semantic scope of AllergyIntolerance.code, then 1868 * the receiving system should ignore 1869 * AllergyIntolerance.reaction.substance.) 1870 */ 1871 public AllergyIntolerance setCode(CodeableConcept value) { 1872 this.code = value; 1873 return this; 1874 } 1875 1876 /** 1877 * @return {@link #patient} (The patient who has the allergy or intolerance.) 1878 */ 1879 public Reference getPatient() { 1880 if (this.patient == null) 1881 if (Configuration.errorOnAutoCreate()) 1882 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1883 else if (Configuration.doAutoCreate()) 1884 this.patient = new Reference(); // cc 1885 return this.patient; 1886 } 1887 1888 public boolean hasPatient() { 1889 return this.patient != null && !this.patient.isEmpty(); 1890 } 1891 1892 /** 1893 * @param value {@link #patient} (The patient who has the allergy or 1894 * intolerance.) 1895 */ 1896 public AllergyIntolerance setPatient(Reference value) { 1897 this.patient = value; 1898 return this; 1899 } 1900 1901 /** 1902 * @return {@link #patient} The actual object that is the target of the 1903 * reference. The reference library doesn't populate this, but you can 1904 * use it to hold the resource if you resolve it. (The patient who has 1905 * the allergy or intolerance.) 1906 */ 1907 public Patient getPatientTarget() { 1908 if (this.patientTarget == null) 1909 if (Configuration.errorOnAutoCreate()) 1910 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1911 else if (Configuration.doAutoCreate()) 1912 this.patientTarget = new Patient(); // aa 1913 return this.patientTarget; 1914 } 1915 1916 /** 1917 * @param value {@link #patient} The actual object that is the target of the 1918 * reference. The reference library doesn't use these, but you can 1919 * use it to hold the resource if you resolve it. (The patient who 1920 * has the allergy or intolerance.) 1921 */ 1922 public AllergyIntolerance setPatientTarget(Patient value) { 1923 this.patientTarget = value; 1924 return this; 1925 } 1926 1927 /** 1928 * @return {@link #encounter} (The encounter when the allergy or intolerance was 1929 * asserted.) 1930 */ 1931 public Reference getEncounter() { 1932 if (this.encounter == null) 1933 if (Configuration.errorOnAutoCreate()) 1934 throw new Error("Attempt to auto-create AllergyIntolerance.encounter"); 1935 else if (Configuration.doAutoCreate()) 1936 this.encounter = new Reference(); // cc 1937 return this.encounter; 1938 } 1939 1940 public boolean hasEncounter() { 1941 return this.encounter != null && !this.encounter.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #encounter} (The encounter when the allergy or 1946 * intolerance was asserted.) 1947 */ 1948 public AllergyIntolerance setEncounter(Reference value) { 1949 this.encounter = value; 1950 return this; 1951 } 1952 1953 /** 1954 * @return {@link #encounter} The actual object that is the target of the 1955 * reference. The reference library doesn't populate this, but you can 1956 * use it to hold the resource if you resolve it. (The encounter when 1957 * the allergy or intolerance was asserted.) 1958 */ 1959 public Encounter getEncounterTarget() { 1960 if (this.encounterTarget == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create AllergyIntolerance.encounter"); 1963 else if (Configuration.doAutoCreate()) 1964 this.encounterTarget = new Encounter(); // aa 1965 return this.encounterTarget; 1966 } 1967 1968 /** 1969 * @param value {@link #encounter} The actual object that is the target of the 1970 * reference. The reference library doesn't use these, but you can 1971 * use it to hold the resource if you resolve it. (The encounter 1972 * when the allergy or intolerance was asserted.) 1973 */ 1974 public AllergyIntolerance setEncounterTarget(Encounter value) { 1975 this.encounterTarget = value; 1976 return this; 1977 } 1978 1979 /** 1980 * @return {@link #onset} (Estimated or actual date, date-time, or age when 1981 * allergy or intolerance was identified.) 1982 */ 1983 public Type getOnset() { 1984 return this.onset; 1985 } 1986 1987 /** 1988 * @return {@link #onset} (Estimated or actual date, date-time, or age when 1989 * allergy or intolerance was identified.) 1990 */ 1991 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1992 if (this.onset == null) 1993 this.onset = new DateTimeType(); 1994 if (!(this.onset instanceof DateTimeType)) 1995 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1996 + this.onset.getClass().getName() + " was encountered"); 1997 return (DateTimeType) this.onset; 1998 } 1999 2000 public boolean hasOnsetDateTimeType() { 2001 return this != null && this.onset instanceof DateTimeType; 2002 } 2003 2004 /** 2005 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2006 * allergy or intolerance was identified.) 2007 */ 2008 public Age getOnsetAge() throws FHIRException { 2009 if (this.onset == null) 2010 this.onset = new Age(); 2011 if (!(this.onset instanceof Age)) 2012 throw new FHIRException( 2013 "Type mismatch: the type Age was expected, but " + this.onset.getClass().getName() + " was encountered"); 2014 return (Age) this.onset; 2015 } 2016 2017 public boolean hasOnsetAge() { 2018 return this != null && this.onset instanceof Age; 2019 } 2020 2021 /** 2022 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2023 * allergy or intolerance was identified.) 2024 */ 2025 public Period getOnsetPeriod() throws FHIRException { 2026 if (this.onset == null) 2027 this.onset = new Period(); 2028 if (!(this.onset instanceof Period)) 2029 throw new FHIRException( 2030 "Type mismatch: the type Period was expected, but " + this.onset.getClass().getName() + " was encountered"); 2031 return (Period) this.onset; 2032 } 2033 2034 public boolean hasOnsetPeriod() { 2035 return this != null && this.onset instanceof Period; 2036 } 2037 2038 /** 2039 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2040 * allergy or intolerance was identified.) 2041 */ 2042 public Range getOnsetRange() throws FHIRException { 2043 if (this.onset == null) 2044 this.onset = new Range(); 2045 if (!(this.onset instanceof Range)) 2046 throw new FHIRException( 2047 "Type mismatch: the type Range was expected, but " + this.onset.getClass().getName() + " was encountered"); 2048 return (Range) this.onset; 2049 } 2050 2051 public boolean hasOnsetRange() { 2052 return this != null && this.onset instanceof Range; 2053 } 2054 2055 /** 2056 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2057 * allergy or intolerance was identified.) 2058 */ 2059 public StringType getOnsetStringType() throws FHIRException { 2060 if (this.onset == null) 2061 this.onset = new StringType(); 2062 if (!(this.onset instanceof StringType)) 2063 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.onset.getClass().getName() 2064 + " was encountered"); 2065 return (StringType) this.onset; 2066 } 2067 2068 public boolean hasOnsetStringType() { 2069 return this != null && this.onset instanceof StringType; 2070 } 2071 2072 public boolean hasOnset() { 2073 return this.onset != null && !this.onset.isEmpty(); 2074 } 2075 2076 /** 2077 * @param value {@link #onset} (Estimated or actual date, date-time, or age when 2078 * allergy or intolerance was identified.) 2079 */ 2080 public AllergyIntolerance setOnset(Type value) { 2081 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 2082 || value instanceof Range || value instanceof StringType)) 2083 throw new Error("Not the right type for AllergyIntolerance.onset[x]: " + value.fhirType()); 2084 this.onset = value; 2085 return this; 2086 } 2087 2088 /** 2089 * @return {@link #recordedDate} (The recordedDate represents when this 2090 * particular AllergyIntolerance record was created in the system, which 2091 * is often a system-generated date.). This is the underlying object 2092 * with id, value and extensions. The accessor "getRecordedDate" gives 2093 * direct access to the value 2094 */ 2095 public DateTimeType getRecordedDateElement() { 2096 if (this.recordedDate == null) 2097 if (Configuration.errorOnAutoCreate()) 2098 throw new Error("Attempt to auto-create AllergyIntolerance.recordedDate"); 2099 else if (Configuration.doAutoCreate()) 2100 this.recordedDate = new DateTimeType(); // bb 2101 return this.recordedDate; 2102 } 2103 2104 public boolean hasRecordedDateElement() { 2105 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2106 } 2107 2108 public boolean hasRecordedDate() { 2109 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2110 } 2111 2112 /** 2113 * @param value {@link #recordedDate} (The recordedDate represents when this 2114 * particular AllergyIntolerance record was created in the system, 2115 * which is often a system-generated date.). This is the underlying 2116 * object with id, value and extensions. The accessor 2117 * "getRecordedDate" gives direct access to the value 2118 */ 2119 public AllergyIntolerance setRecordedDateElement(DateTimeType value) { 2120 this.recordedDate = value; 2121 return this; 2122 } 2123 2124 /** 2125 * @return The recordedDate represents when this particular AllergyIntolerance 2126 * record was created in the system, which is often a system-generated 2127 * date. 2128 */ 2129 public Date getRecordedDate() { 2130 return this.recordedDate == null ? null : this.recordedDate.getValue(); 2131 } 2132 2133 /** 2134 * @param value The recordedDate represents when this particular 2135 * AllergyIntolerance record was created in the system, which is 2136 * often a system-generated date. 2137 */ 2138 public AllergyIntolerance setRecordedDate(Date value) { 2139 if (value == null) 2140 this.recordedDate = null; 2141 else { 2142 if (this.recordedDate == null) 2143 this.recordedDate = new DateTimeType(); 2144 this.recordedDate.setValue(value); 2145 } 2146 return this; 2147 } 2148 2149 /** 2150 * @return {@link #recorder} (Individual who recorded the record and takes 2151 * responsibility for its content.) 2152 */ 2153 public Reference getRecorder() { 2154 if (this.recorder == null) 2155 if (Configuration.errorOnAutoCreate()) 2156 throw new Error("Attempt to auto-create AllergyIntolerance.recorder"); 2157 else if (Configuration.doAutoCreate()) 2158 this.recorder = new Reference(); // cc 2159 return this.recorder; 2160 } 2161 2162 public boolean hasRecorder() { 2163 return this.recorder != null && !this.recorder.isEmpty(); 2164 } 2165 2166 /** 2167 * @param value {@link #recorder} (Individual who recorded the record and takes 2168 * responsibility for its content.) 2169 */ 2170 public AllergyIntolerance setRecorder(Reference value) { 2171 this.recorder = value; 2172 return this; 2173 } 2174 2175 /** 2176 * @return {@link #recorder} The actual object that is the target of the 2177 * reference. The reference library doesn't populate this, but you can 2178 * use it to hold the resource if you resolve it. (Individual who 2179 * recorded the record and takes responsibility for its content.) 2180 */ 2181 public Resource getRecorderTarget() { 2182 return this.recorderTarget; 2183 } 2184 2185 /** 2186 * @param value {@link #recorder} The actual object that is the target of the 2187 * reference. The reference library doesn't use these, but you can 2188 * use it to hold the resource if you resolve it. (Individual who 2189 * recorded the record and takes responsibility for its content.) 2190 */ 2191 public AllergyIntolerance setRecorderTarget(Resource value) { 2192 this.recorderTarget = value; 2193 return this; 2194 } 2195 2196 /** 2197 * @return {@link #asserter} (The source of the information about the allergy 2198 * that is recorded.) 2199 */ 2200 public Reference getAsserter() { 2201 if (this.asserter == null) 2202 if (Configuration.errorOnAutoCreate()) 2203 throw new Error("Attempt to auto-create AllergyIntolerance.asserter"); 2204 else if (Configuration.doAutoCreate()) 2205 this.asserter = new Reference(); // cc 2206 return this.asserter; 2207 } 2208 2209 public boolean hasAsserter() { 2210 return this.asserter != null && !this.asserter.isEmpty(); 2211 } 2212 2213 /** 2214 * @param value {@link #asserter} (The source of the information about the 2215 * allergy that is recorded.) 2216 */ 2217 public AllergyIntolerance setAsserter(Reference value) { 2218 this.asserter = value; 2219 return this; 2220 } 2221 2222 /** 2223 * @return {@link #asserter} The actual object that is the target of the 2224 * reference. The reference library doesn't populate this, but you can 2225 * use it to hold the resource if you resolve it. (The source of the 2226 * information about the allergy that is recorded.) 2227 */ 2228 public Resource getAsserterTarget() { 2229 return this.asserterTarget; 2230 } 2231 2232 /** 2233 * @param value {@link #asserter} The actual object that is the target of the 2234 * reference. The reference library doesn't use these, but you can 2235 * use it to hold the resource if you resolve it. (The source of 2236 * the information about the allergy that is recorded.) 2237 */ 2238 public AllergyIntolerance setAsserterTarget(Resource value) { 2239 this.asserterTarget = value; 2240 return this; 2241 } 2242 2243 /** 2244 * @return {@link #lastOccurrence} (Represents the date and/or time of the last 2245 * known occurrence of a reaction event.). This is the underlying object 2246 * with id, value and extensions. The accessor "getLastOccurrence" gives 2247 * direct access to the value 2248 */ 2249 public DateTimeType getLastOccurrenceElement() { 2250 if (this.lastOccurrence == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create AllergyIntolerance.lastOccurrence"); 2253 else if (Configuration.doAutoCreate()) 2254 this.lastOccurrence = new DateTimeType(); // bb 2255 return this.lastOccurrence; 2256 } 2257 2258 public boolean hasLastOccurrenceElement() { 2259 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 2260 } 2261 2262 public boolean hasLastOccurrence() { 2263 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 2264 } 2265 2266 /** 2267 * @param value {@link #lastOccurrence} (Represents the date and/or time of the 2268 * last known occurrence of a reaction event.). This is the 2269 * underlying object with id, value and extensions. The accessor 2270 * "getLastOccurrence" gives direct access to the value 2271 */ 2272 public AllergyIntolerance setLastOccurrenceElement(DateTimeType value) { 2273 this.lastOccurrence = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return Represents the date and/or time of the last known occurrence of a 2279 * reaction event. 2280 */ 2281 public Date getLastOccurrence() { 2282 return this.lastOccurrence == null ? null : this.lastOccurrence.getValue(); 2283 } 2284 2285 /** 2286 * @param value Represents the date and/or time of the last known occurrence of 2287 * a reaction event. 2288 */ 2289 public AllergyIntolerance setLastOccurrence(Date value) { 2290 if (value == null) 2291 this.lastOccurrence = null; 2292 else { 2293 if (this.lastOccurrence == null) 2294 this.lastOccurrence = new DateTimeType(); 2295 this.lastOccurrence.setValue(value); 2296 } 2297 return this; 2298 } 2299 2300 /** 2301 * @return {@link #note} (Additional narrative about the propensity for the 2302 * Adverse Reaction, not captured in other fields.) 2303 */ 2304 public List<Annotation> getNote() { 2305 if (this.note == null) 2306 this.note = new ArrayList<Annotation>(); 2307 return this.note; 2308 } 2309 2310 /** 2311 * @return Returns a reference to <code>this</code> for easy method chaining 2312 */ 2313 public AllergyIntolerance setNote(List<Annotation> theNote) { 2314 this.note = theNote; 2315 return this; 2316 } 2317 2318 public boolean hasNote() { 2319 if (this.note == null) 2320 return false; 2321 for (Annotation item : this.note) 2322 if (!item.isEmpty()) 2323 return true; 2324 return false; 2325 } 2326 2327 public Annotation addNote() { // 3 2328 Annotation t = new Annotation(); 2329 if (this.note == null) 2330 this.note = new ArrayList<Annotation>(); 2331 this.note.add(t); 2332 return t; 2333 } 2334 2335 public AllergyIntolerance addNote(Annotation t) { // 3 2336 if (t == null) 2337 return this; 2338 if (this.note == null) 2339 this.note = new ArrayList<Annotation>(); 2340 this.note.add(t); 2341 return this; 2342 } 2343 2344 /** 2345 * @return The first repetition of repeating field {@link #note}, creating it if 2346 * it does not already exist 2347 */ 2348 public Annotation getNoteFirstRep() { 2349 if (getNote().isEmpty()) { 2350 addNote(); 2351 } 2352 return getNote().get(0); 2353 } 2354 2355 /** 2356 * @return {@link #reaction} (Details about each adverse reaction event linked 2357 * to exposure to the identified substance.) 2358 */ 2359 public List<AllergyIntoleranceReactionComponent> getReaction() { 2360 if (this.reaction == null) 2361 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2362 return this.reaction; 2363 } 2364 2365 /** 2366 * @return Returns a reference to <code>this</code> for easy method chaining 2367 */ 2368 public AllergyIntolerance setReaction(List<AllergyIntoleranceReactionComponent> theReaction) { 2369 this.reaction = theReaction; 2370 return this; 2371 } 2372 2373 public boolean hasReaction() { 2374 if (this.reaction == null) 2375 return false; 2376 for (AllergyIntoleranceReactionComponent item : this.reaction) 2377 if (!item.isEmpty()) 2378 return true; 2379 return false; 2380 } 2381 2382 public AllergyIntoleranceReactionComponent addReaction() { // 3 2383 AllergyIntoleranceReactionComponent t = new AllergyIntoleranceReactionComponent(); 2384 if (this.reaction == null) 2385 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2386 this.reaction.add(t); 2387 return t; 2388 } 2389 2390 public AllergyIntolerance addReaction(AllergyIntoleranceReactionComponent t) { // 3 2391 if (t == null) 2392 return this; 2393 if (this.reaction == null) 2394 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2395 this.reaction.add(t); 2396 return this; 2397 } 2398 2399 /** 2400 * @return The first repetition of repeating field {@link #reaction}, creating 2401 * it if it does not already exist 2402 */ 2403 public AllergyIntoleranceReactionComponent getReactionFirstRep() { 2404 if (getReaction().isEmpty()) { 2405 addReaction(); 2406 } 2407 return getReaction().get(0); 2408 } 2409 2410 protected void listChildren(List<Property> children) { 2411 super.listChildren(children); 2412 children.add(new Property("identifier", "Identifier", 2413 "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2414 0, java.lang.Integer.MAX_VALUE, identifier)); 2415 children.add(new Property("clinicalStatus", "CodeableConcept", "The clinical status of the allergy or intolerance.", 2416 0, 1, clinicalStatus)); 2417 children.add(new Property("verificationStatus", "CodeableConcept", 2418 "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).", 2419 0, 1, verificationStatus)); 2420 children.add(new Property("type", "code", 2421 "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type)); 2422 children.add(new Property("category", "code", "Category of the identified substance.", 0, 2423 java.lang.Integer.MAX_VALUE, category)); 2424 children.add(new Property("criticality", "code", 2425 "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, 2426 criticality)); 2427 children.add(new Property("code", "CodeableConcept", 2428 "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 2429 0, 1, code)); 2430 children.add(new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 1, 2431 patient)); 2432 children.add(new Property("encounter", "Reference(Encounter)", 2433 "The encounter when the allergy or intolerance was asserted.", 0, 1, encounter)); 2434 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", 2435 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset)); 2436 children.add(new Property("recordedDate", "dateTime", 2437 "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.", 2438 0, 1, recordedDate)); 2439 children.add(new Property("recorder", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 2440 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder)); 2441 children.add(new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 2442 "The source of the information about the allergy that is recorded.", 0, 1, asserter)); 2443 children.add(new Property("lastOccurrence", "dateTime", 2444 "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence)); 2445 children.add(new Property("note", "Annotation", 2446 "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, 2447 java.lang.Integer.MAX_VALUE, note)); 2448 children.add(new Property("reaction", "", 2449 "Details about each adverse reaction event linked to exposure to the identified substance.", 0, 2450 java.lang.Integer.MAX_VALUE, reaction)); 2451 } 2452 2453 @Override 2454 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2455 switch (_hash) { 2456 case -1618432855: 2457 /* identifier */ return new Property("identifier", "Identifier", 2458 "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2459 0, java.lang.Integer.MAX_VALUE, identifier); 2460 case -462853915: 2461 /* clinicalStatus */ return new Property("clinicalStatus", "CodeableConcept", 2462 "The clinical status of the allergy or intolerance.", 0, 1, clinicalStatus); 2463 case -842509843: 2464 /* verificationStatus */ return new Property("verificationStatus", "CodeableConcept", 2465 "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).", 2466 0, 1, verificationStatus); 2467 case 3575610: 2468 /* type */ return new Property("type", "code", 2469 "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type); 2470 case 50511102: 2471 /* category */ return new Property("category", "code", "Category of the identified substance.", 0, 2472 java.lang.Integer.MAX_VALUE, category); 2473 case -1608054609: 2474 /* criticality */ return new Property("criticality", "code", 2475 "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, 2476 criticality); 2477 case 3059181: 2478 /* code */ return new Property("code", "CodeableConcept", 2479 "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 2480 0, 1, code); 2481 case -791418107: 2482 /* patient */ return new Property("patient", "Reference(Patient)", 2483 "The patient who has the allergy or intolerance.", 0, 1, patient); 2484 case 1524132147: 2485 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2486 "The encounter when the allergy or intolerance was asserted.", 0, 1, encounter); 2487 case -1886216323: 2488 /* onset[x] */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2489 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2490 case 105901603: 2491 /* onset */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2492 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2493 case -1701663010: 2494 /* onsetDateTime */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2495 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2496 case -1886241828: 2497 /* onsetAge */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2498 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2499 case -1545082428: 2500 /* onsetPeriod */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2501 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2502 case -186664742: 2503 /* onsetRange */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2504 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2505 case -1445342188: 2506 /* onsetString */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2507 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2508 case -1952893826: 2509 /* recordedDate */ return new Property("recordedDate", "dateTime", 2510 "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.", 2511 0, 1, recordedDate); 2512 case -799233858: 2513 /* recorder */ return new Property("recorder", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 2514 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder); 2515 case -373242253: 2516 /* asserter */ return new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 2517 "The source of the information about the allergy that is recorded.", 0, 1, asserter); 2518 case 1896977671: 2519 /* lastOccurrence */ return new Property("lastOccurrence", "dateTime", 2520 "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence); 2521 case 3387378: 2522 /* note */ return new Property("note", "Annotation", 2523 "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, 2524 java.lang.Integer.MAX_VALUE, note); 2525 case -867509719: 2526 /* reaction */ return new Property("reaction", "", 2527 "Details about each adverse reaction event linked to exposure to the identified substance.", 0, 2528 java.lang.Integer.MAX_VALUE, reaction); 2529 default: 2530 return super.getNamedProperty(_hash, _name, _checkValid); 2531 } 2532 2533 } 2534 2535 @Override 2536 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2537 switch (hash) { 2538 case -1618432855: 2539 /* identifier */ return this.identifier == null ? new Base[0] 2540 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2541 case -462853915: 2542 /* clinicalStatus */ return this.clinicalStatus == null ? new Base[0] : new Base[] { this.clinicalStatus }; // CodeableConcept 2543 case -842509843: 2544 /* verificationStatus */ return this.verificationStatus == null ? new Base[0] 2545 : new Base[] { this.verificationStatus }; // CodeableConcept 2546 case 3575610: 2547 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<AllergyIntoleranceType> 2548 case 50511102: 2549 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // Enumeration<AllergyIntoleranceCategory> 2550 case -1608054609: 2551 /* criticality */ return this.criticality == null ? new Base[0] : new Base[] { this.criticality }; // Enumeration<AllergyIntoleranceCriticality> 2552 case 3059181: 2553 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2554 case -791418107: 2555 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2556 case 1524132147: 2557 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2558 case 105901603: 2559 /* onset */ return this.onset == null ? new Base[0] : new Base[] { this.onset }; // Type 2560 case -1952893826: 2561 /* recordedDate */ return this.recordedDate == null ? new Base[0] : new Base[] { this.recordedDate }; // DateTimeType 2562 case -799233858: 2563 /* recorder */ return this.recorder == null ? new Base[0] : new Base[] { this.recorder }; // Reference 2564 case -373242253: 2565 /* asserter */ return this.asserter == null ? new Base[0] : new Base[] { this.asserter }; // Reference 2566 case 1896977671: 2567 /* lastOccurrence */ return this.lastOccurrence == null ? new Base[0] : new Base[] { this.lastOccurrence }; // DateTimeType 2568 case 3387378: 2569 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2570 case -867509719: 2571 /* reaction */ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // AllergyIntoleranceReactionComponent 2572 default: 2573 return super.getProperty(hash, name, checkValid); 2574 } 2575 2576 } 2577 2578 @Override 2579 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2580 switch (hash) { 2581 case -1618432855: // identifier 2582 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2583 return value; 2584 case -462853915: // clinicalStatus 2585 this.clinicalStatus = castToCodeableConcept(value); // CodeableConcept 2586 return value; 2587 case -842509843: // verificationStatus 2588 this.verificationStatus = castToCodeableConcept(value); // CodeableConcept 2589 return value; 2590 case 3575610: // type 2591 value = new AllergyIntoleranceTypeEnumFactory().fromType(castToCode(value)); 2592 this.type = (Enumeration) value; // Enumeration<AllergyIntoleranceType> 2593 return value; 2594 case 50511102: // category 2595 value = new AllergyIntoleranceCategoryEnumFactory().fromType(castToCode(value)); 2596 this.getCategory().add((Enumeration) value); // Enumeration<AllergyIntoleranceCategory> 2597 return value; 2598 case -1608054609: // criticality 2599 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(castToCode(value)); 2600 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2601 return value; 2602 case 3059181: // code 2603 this.code = castToCodeableConcept(value); // CodeableConcept 2604 return value; 2605 case -791418107: // patient 2606 this.patient = castToReference(value); // Reference 2607 return value; 2608 case 1524132147: // encounter 2609 this.encounter = castToReference(value); // Reference 2610 return value; 2611 case 105901603: // onset 2612 this.onset = castToType(value); // Type 2613 return value; 2614 case -1952893826: // recordedDate 2615 this.recordedDate = castToDateTime(value); // DateTimeType 2616 return value; 2617 case -799233858: // recorder 2618 this.recorder = castToReference(value); // Reference 2619 return value; 2620 case -373242253: // asserter 2621 this.asserter = castToReference(value); // Reference 2622 return value; 2623 case 1896977671: // lastOccurrence 2624 this.lastOccurrence = castToDateTime(value); // DateTimeType 2625 return value; 2626 case 3387378: // note 2627 this.getNote().add(castToAnnotation(value)); // Annotation 2628 return value; 2629 case -867509719: // reaction 2630 this.getReaction().add((AllergyIntoleranceReactionComponent) value); // AllergyIntoleranceReactionComponent 2631 return value; 2632 default: 2633 return super.setProperty(hash, name, value); 2634 } 2635 2636 } 2637 2638 @Override 2639 public Base setProperty(String name, Base value) throws FHIRException { 2640 if (name.equals("identifier")) { 2641 this.getIdentifier().add(castToIdentifier(value)); 2642 } else if (name.equals("clinicalStatus")) { 2643 this.clinicalStatus = castToCodeableConcept(value); // CodeableConcept 2644 } else if (name.equals("verificationStatus")) { 2645 this.verificationStatus = castToCodeableConcept(value); // CodeableConcept 2646 } else if (name.equals("type")) { 2647 value = new AllergyIntoleranceTypeEnumFactory().fromType(castToCode(value)); 2648 this.type = (Enumeration) value; // Enumeration<AllergyIntoleranceType> 2649 } else if (name.equals("category")) { 2650 value = new AllergyIntoleranceCategoryEnumFactory().fromType(castToCode(value)); 2651 this.getCategory().add((Enumeration) value); 2652 } else if (name.equals("criticality")) { 2653 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(castToCode(value)); 2654 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2655 } else if (name.equals("code")) { 2656 this.code = castToCodeableConcept(value); // CodeableConcept 2657 } else if (name.equals("patient")) { 2658 this.patient = castToReference(value); // Reference 2659 } else if (name.equals("encounter")) { 2660 this.encounter = castToReference(value); // Reference 2661 } else if (name.equals("onset[x]")) { 2662 this.onset = castToType(value); // Type 2663 } else if (name.equals("recordedDate")) { 2664 this.recordedDate = castToDateTime(value); // DateTimeType 2665 } else if (name.equals("recorder")) { 2666 this.recorder = castToReference(value); // Reference 2667 } else if (name.equals("asserter")) { 2668 this.asserter = castToReference(value); // Reference 2669 } else if (name.equals("lastOccurrence")) { 2670 this.lastOccurrence = castToDateTime(value); // DateTimeType 2671 } else if (name.equals("note")) { 2672 this.getNote().add(castToAnnotation(value)); 2673 } else if (name.equals("reaction")) { 2674 this.getReaction().add((AllergyIntoleranceReactionComponent) value); 2675 } else 2676 return super.setProperty(name, value); 2677 return value; 2678 } 2679 2680 @Override 2681 public Base makeProperty(int hash, String name) throws FHIRException { 2682 switch (hash) { 2683 case -1618432855: 2684 return addIdentifier(); 2685 case -462853915: 2686 return getClinicalStatus(); 2687 case -842509843: 2688 return getVerificationStatus(); 2689 case 3575610: 2690 return getTypeElement(); 2691 case 50511102: 2692 return addCategoryElement(); 2693 case -1608054609: 2694 return getCriticalityElement(); 2695 case 3059181: 2696 return getCode(); 2697 case -791418107: 2698 return getPatient(); 2699 case 1524132147: 2700 return getEncounter(); 2701 case -1886216323: 2702 return getOnset(); 2703 case 105901603: 2704 return getOnset(); 2705 case -1952893826: 2706 return getRecordedDateElement(); 2707 case -799233858: 2708 return getRecorder(); 2709 case -373242253: 2710 return getAsserter(); 2711 case 1896977671: 2712 return getLastOccurrenceElement(); 2713 case 3387378: 2714 return addNote(); 2715 case -867509719: 2716 return addReaction(); 2717 default: 2718 return super.makeProperty(hash, name); 2719 } 2720 2721 } 2722 2723 @Override 2724 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2725 switch (hash) { 2726 case -1618432855: 2727 /* identifier */ return new String[] { "Identifier" }; 2728 case -462853915: 2729 /* clinicalStatus */ return new String[] { "CodeableConcept" }; 2730 case -842509843: 2731 /* verificationStatus */ return new String[] { "CodeableConcept" }; 2732 case 3575610: 2733 /* type */ return new String[] { "code" }; 2734 case 50511102: 2735 /* category */ return new String[] { "code" }; 2736 case -1608054609: 2737 /* criticality */ return new String[] { "code" }; 2738 case 3059181: 2739 /* code */ return new String[] { "CodeableConcept" }; 2740 case -791418107: 2741 /* patient */ return new String[] { "Reference" }; 2742 case 1524132147: 2743 /* encounter */ return new String[] { "Reference" }; 2744 case 105901603: 2745 /* onset */ return new String[] { "dateTime", "Age", "Period", "Range", "string" }; 2746 case -1952893826: 2747 /* recordedDate */ return new String[] { "dateTime" }; 2748 case -799233858: 2749 /* recorder */ return new String[] { "Reference" }; 2750 case -373242253: 2751 /* asserter */ return new String[] { "Reference" }; 2752 case 1896977671: 2753 /* lastOccurrence */ return new String[] { "dateTime" }; 2754 case 3387378: 2755 /* note */ return new String[] { "Annotation" }; 2756 case -867509719: 2757 /* reaction */ return new String[] {}; 2758 default: 2759 return super.getTypesForProperty(hash, name); 2760 } 2761 2762 } 2763 2764 @Override 2765 public Base addChild(String name) throws FHIRException { 2766 if (name.equals("identifier")) { 2767 return addIdentifier(); 2768 } else if (name.equals("clinicalStatus")) { 2769 this.clinicalStatus = new CodeableConcept(); 2770 return this.clinicalStatus; 2771 } else if (name.equals("verificationStatus")) { 2772 this.verificationStatus = new CodeableConcept(); 2773 return this.verificationStatus; 2774 } else if (name.equals("type")) { 2775 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.type"); 2776 } else if (name.equals("category")) { 2777 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.category"); 2778 } else if (name.equals("criticality")) { 2779 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.criticality"); 2780 } else if (name.equals("code")) { 2781 this.code = new CodeableConcept(); 2782 return this.code; 2783 } else if (name.equals("patient")) { 2784 this.patient = new Reference(); 2785 return this.patient; 2786 } else if (name.equals("encounter")) { 2787 this.encounter = new Reference(); 2788 return this.encounter; 2789 } else if (name.equals("onsetDateTime")) { 2790 this.onset = new DateTimeType(); 2791 return this.onset; 2792 } else if (name.equals("onsetAge")) { 2793 this.onset = new Age(); 2794 return this.onset; 2795 } else if (name.equals("onsetPeriod")) { 2796 this.onset = new Period(); 2797 return this.onset; 2798 } else if (name.equals("onsetRange")) { 2799 this.onset = new Range(); 2800 return this.onset; 2801 } else if (name.equals("onsetString")) { 2802 this.onset = new StringType(); 2803 return this.onset; 2804 } else if (name.equals("recordedDate")) { 2805 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.recordedDate"); 2806 } else if (name.equals("recorder")) { 2807 this.recorder = new Reference(); 2808 return this.recorder; 2809 } else if (name.equals("asserter")) { 2810 this.asserter = new Reference(); 2811 return this.asserter; 2812 } else if (name.equals("lastOccurrence")) { 2813 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.lastOccurrence"); 2814 } else if (name.equals("note")) { 2815 return addNote(); 2816 } else if (name.equals("reaction")) { 2817 return addReaction(); 2818 } else 2819 return super.addChild(name); 2820 } 2821 2822 public String fhirType() { 2823 return "AllergyIntolerance"; 2824 2825 } 2826 2827 public AllergyIntolerance copy() { 2828 AllergyIntolerance dst = new AllergyIntolerance(); 2829 copyValues(dst); 2830 return dst; 2831 } 2832 2833 public void copyValues(AllergyIntolerance dst) { 2834 super.copyValues(dst); 2835 if (identifier != null) { 2836 dst.identifier = new ArrayList<Identifier>(); 2837 for (Identifier i : identifier) 2838 dst.identifier.add(i.copy()); 2839 } 2840 ; 2841 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 2842 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 2843 dst.type = type == null ? null : type.copy(); 2844 if (category != null) { 2845 dst.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 2846 for (Enumeration<AllergyIntoleranceCategory> i : category) 2847 dst.category.add(i.copy()); 2848 } 2849 ; 2850 dst.criticality = criticality == null ? null : criticality.copy(); 2851 dst.code = code == null ? null : code.copy(); 2852 dst.patient = patient == null ? null : patient.copy(); 2853 dst.encounter = encounter == null ? null : encounter.copy(); 2854 dst.onset = onset == null ? null : onset.copy(); 2855 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2856 dst.recorder = recorder == null ? null : recorder.copy(); 2857 dst.asserter = asserter == null ? null : asserter.copy(); 2858 dst.lastOccurrence = lastOccurrence == null ? null : lastOccurrence.copy(); 2859 if (note != null) { 2860 dst.note = new ArrayList<Annotation>(); 2861 for (Annotation i : note) 2862 dst.note.add(i.copy()); 2863 } 2864 ; 2865 if (reaction != null) { 2866 dst.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2867 for (AllergyIntoleranceReactionComponent i : reaction) 2868 dst.reaction.add(i.copy()); 2869 } 2870 ; 2871 } 2872 2873 protected AllergyIntolerance typedCopy() { 2874 return copy(); 2875 } 2876 2877 @Override 2878 public boolean equalsDeep(Base other_) { 2879 if (!super.equalsDeep(other_)) 2880 return false; 2881 if (!(other_ instanceof AllergyIntolerance)) 2882 return false; 2883 AllergyIntolerance o = (AllergyIntolerance) other_; 2884 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 2885 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(type, o.type, true) 2886 && compareDeep(category, o.category, true) && compareDeep(criticality, o.criticality, true) 2887 && compareDeep(code, o.code, true) && compareDeep(patient, o.patient, true) 2888 && compareDeep(encounter, o.encounter, true) && compareDeep(onset, o.onset, true) 2889 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(recorder, o.recorder, true) 2890 && compareDeep(asserter, o.asserter, true) && compareDeep(lastOccurrence, o.lastOccurrence, true) 2891 && compareDeep(note, o.note, true) && compareDeep(reaction, o.reaction, true); 2892 } 2893 2894 @Override 2895 public boolean equalsShallow(Base other_) { 2896 if (!super.equalsShallow(other_)) 2897 return false; 2898 if (!(other_ instanceof AllergyIntolerance)) 2899 return false; 2900 AllergyIntolerance o = (AllergyIntolerance) other_; 2901 return compareValues(type, o.type, true) && compareValues(category, o.category, true) 2902 && compareValues(criticality, o.criticality, true) && compareValues(recordedDate, o.recordedDate, true) 2903 && compareValues(lastOccurrence, o.lastOccurrence, true); 2904 } 2905 2906 public boolean isEmpty() { 2907 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus, verificationStatus, type, 2908 category, criticality, code, patient, encounter, onset, recordedDate, recorder, asserter, lastOccurrence, note, 2909 reaction); 2910 } 2911 2912 @Override 2913 public ResourceType getResourceType() { 2914 return ResourceType.AllergyIntolerance; 2915 } 2916 2917 /** 2918 * Search parameter: <b>severity</b> 2919 * <p> 2920 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2921 * Type: <b>token</b><br> 2922 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2923 * </p> 2924 */ 2925 @SearchParamDefinition(name = "severity", path = "AllergyIntolerance.reaction.severity", description = "mild | moderate | severe (of event as a whole)", type = "token") 2926 public static final String SP_SEVERITY = "severity"; 2927 /** 2928 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2929 * <p> 2930 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2931 * Type: <b>token</b><br> 2932 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2933 * </p> 2934 */ 2935 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2936 SP_SEVERITY); 2937 2938 /** 2939 * Search parameter: <b>date</b> 2940 * <p> 2941 * Description: <b>Date first version of the resource instance was 2942 * recorded</b><br> 2943 * Type: <b>date</b><br> 2944 * Path: <b>AllergyIntolerance.recordedDate</b><br> 2945 * </p> 2946 */ 2947 @SearchParamDefinition(name = "date", path = "AllergyIntolerance.recordedDate", description = "Date first version of the resource instance was recorded", type = "date") 2948 public static final String SP_DATE = "date"; 2949 /** 2950 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2951 * <p> 2952 * Description: <b>Date first version of the resource instance was 2953 * recorded</b><br> 2954 * Type: <b>date</b><br> 2955 * Path: <b>AllergyIntolerance.recordedDate</b><br> 2956 * </p> 2957 */ 2958 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2959 SP_DATE); 2960 2961 /** 2962 * Search parameter: <b>identifier</b> 2963 * <p> 2964 * Description: <b>External ids for this item</b><br> 2965 * Type: <b>token</b><br> 2966 * Path: <b>AllergyIntolerance.identifier</b><br> 2967 * </p> 2968 */ 2969 @SearchParamDefinition(name = "identifier", path = "AllergyIntolerance.identifier", description = "External ids for this item", type = "token") 2970 public static final String SP_IDENTIFIER = "identifier"; 2971 /** 2972 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2973 * <p> 2974 * Description: <b>External ids for this item</b><br> 2975 * Type: <b>token</b><br> 2976 * Path: <b>AllergyIntolerance.identifier</b><br> 2977 * </p> 2978 */ 2979 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2980 SP_IDENTIFIER); 2981 2982 /** 2983 * Search parameter: <b>manifestation</b> 2984 * <p> 2985 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2986 * Type: <b>token</b><br> 2987 * Path: <b>AllergyIntolerance.reaction.manifestation</b><br> 2988 * </p> 2989 */ 2990 @SearchParamDefinition(name = "manifestation", path = "AllergyIntolerance.reaction.manifestation", description = "Clinical symptoms/signs associated with the Event", type = "token") 2991 public static final String SP_MANIFESTATION = "manifestation"; 2992 /** 2993 * <b>Fluent Client</b> search parameter constant for <b>manifestation</b> 2994 * <p> 2995 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2996 * Type: <b>token</b><br> 2997 * Path: <b>AllergyIntolerance.reaction.manifestation</b><br> 2998 * </p> 2999 */ 3000 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MANIFESTATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3001 SP_MANIFESTATION); 3002 3003 /** 3004 * Search parameter: <b>recorder</b> 3005 * <p> 3006 * Description: <b>Who recorded the sensitivity</b><br> 3007 * Type: <b>reference</b><br> 3008 * Path: <b>AllergyIntolerance.recorder</b><br> 3009 * </p> 3010 */ 3011 @SearchParamDefinition(name = "recorder", path = "AllergyIntolerance.recorder", description = "Who recorded the sensitivity", type = "reference", providesMembershipIn = { 3012 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3013 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Patient.class, 3014 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3015 public static final String SP_RECORDER = "recorder"; 3016 /** 3017 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 3018 * <p> 3019 * Description: <b>Who recorded the sensitivity</b><br> 3020 * Type: <b>reference</b><br> 3021 * Path: <b>AllergyIntolerance.recorder</b><br> 3022 * </p> 3023 */ 3024 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3025 SP_RECORDER); 3026 3027 /** 3028 * Constant for fluent queries to be used to add include statements. Specifies 3029 * the path value of "<b>AllergyIntolerance:recorder</b>". 3030 */ 3031 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include( 3032 "AllergyIntolerance:recorder").toLocked(); 3033 3034 /** 3035 * Search parameter: <b>code</b> 3036 * <p> 3037 * Description: <b>Code that identifies the allergy or intolerance</b><br> 3038 * Type: <b>token</b><br> 3039 * Path: <b>AllergyIntolerance.code, 3040 * AllergyIntolerance.reaction.substance</b><br> 3041 * </p> 3042 */ 3043 @SearchParamDefinition(name = "code", path = "AllergyIntolerance.code | AllergyIntolerance.reaction.substance", description = "Code that identifies the allergy or intolerance", type = "token") 3044 public static final String SP_CODE = "code"; 3045 /** 3046 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3047 * <p> 3048 * Description: <b>Code that identifies the allergy or intolerance</b><br> 3049 * Type: <b>token</b><br> 3050 * Path: <b>AllergyIntolerance.code, 3051 * AllergyIntolerance.reaction.substance</b><br> 3052 * </p> 3053 */ 3054 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3055 SP_CODE); 3056 3057 /** 3058 * Search parameter: <b>verification-status</b> 3059 * <p> 3060 * Description: <b>unconfirmed | confirmed | refuted | entered-in-error</b><br> 3061 * Type: <b>token</b><br> 3062 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 3063 * </p> 3064 */ 3065 @SearchParamDefinition(name = "verification-status", path = "AllergyIntolerance.verificationStatus", description = "unconfirmed | confirmed | refuted | entered-in-error", type = "token") 3066 public static final String SP_VERIFICATION_STATUS = "verification-status"; 3067 /** 3068 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 3069 * <p> 3070 * Description: <b>unconfirmed | confirmed | refuted | entered-in-error</b><br> 3071 * Type: <b>token</b><br> 3072 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 3073 * </p> 3074 */ 3075 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3076 SP_VERIFICATION_STATUS); 3077 3078 /** 3079 * Search parameter: <b>criticality</b> 3080 * <p> 3081 * Description: <b>low | high | unable-to-assess</b><br> 3082 * Type: <b>token</b><br> 3083 * Path: <b>AllergyIntolerance.criticality</b><br> 3084 * </p> 3085 */ 3086 @SearchParamDefinition(name = "criticality", path = "AllergyIntolerance.criticality", description = "low | high | unable-to-assess", type = "token") 3087 public static final String SP_CRITICALITY = "criticality"; 3088 /** 3089 * <b>Fluent Client</b> search parameter constant for <b>criticality</b> 3090 * <p> 3091 * Description: <b>low | high | unable-to-assess</b><br> 3092 * Type: <b>token</b><br> 3093 * Path: <b>AllergyIntolerance.criticality</b><br> 3094 * </p> 3095 */ 3096 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CRITICALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3097 SP_CRITICALITY); 3098 3099 /** 3100 * Search parameter: <b>clinical-status</b> 3101 * <p> 3102 * Description: <b>active | inactive | resolved</b><br> 3103 * Type: <b>token</b><br> 3104 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 3105 * </p> 3106 */ 3107 @SearchParamDefinition(name = "clinical-status", path = "AllergyIntolerance.clinicalStatus", description = "active | inactive | resolved", type = "token") 3108 public static final String SP_CLINICAL_STATUS = "clinical-status"; 3109 /** 3110 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 3111 * <p> 3112 * Description: <b>active | inactive | resolved</b><br> 3113 * Type: <b>token</b><br> 3114 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 3115 * </p> 3116 */ 3117 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3118 SP_CLINICAL_STATUS); 3119 3120 /** 3121 * Search parameter: <b>type</b> 3122 * <p> 3123 * Description: <b>allergy | intolerance - Underlying mechanism (if 3124 * known)</b><br> 3125 * Type: <b>token</b><br> 3126 * Path: <b>AllergyIntolerance.type</b><br> 3127 * </p> 3128 */ 3129 @SearchParamDefinition(name = "type", path = "AllergyIntolerance.type", description = "allergy | intolerance - Underlying mechanism (if known)", type = "token") 3130 public static final String SP_TYPE = "type"; 3131 /** 3132 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3133 * <p> 3134 * Description: <b>allergy | intolerance - Underlying mechanism (if 3135 * known)</b><br> 3136 * Type: <b>token</b><br> 3137 * Path: <b>AllergyIntolerance.type</b><br> 3138 * </p> 3139 */ 3140 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3141 SP_TYPE); 3142 3143 /** 3144 * Search parameter: <b>onset</b> 3145 * <p> 3146 * Description: <b>Date(/time) when manifestations showed</b><br> 3147 * Type: <b>date</b><br> 3148 * Path: <b>AllergyIntolerance.reaction.onset</b><br> 3149 * </p> 3150 */ 3151 @SearchParamDefinition(name = "onset", path = "AllergyIntolerance.reaction.onset", description = "Date(/time) when manifestations showed", type = "date") 3152 public static final String SP_ONSET = "onset"; 3153 /** 3154 * <b>Fluent Client</b> search parameter constant for <b>onset</b> 3155 * <p> 3156 * Description: <b>Date(/time) when manifestations showed</b><br> 3157 * Type: <b>date</b><br> 3158 * Path: <b>AllergyIntolerance.reaction.onset</b><br> 3159 * </p> 3160 */ 3161 public static final ca.uhn.fhir.rest.gclient.DateClientParam ONSET = new ca.uhn.fhir.rest.gclient.DateClientParam( 3162 SP_ONSET); 3163 3164 /** 3165 * Search parameter: <b>route</b> 3166 * <p> 3167 * Description: <b>How the subject was exposed to the substance</b><br> 3168 * Type: <b>token</b><br> 3169 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 3170 * </p> 3171 */ 3172 @SearchParamDefinition(name = "route", path = "AllergyIntolerance.reaction.exposureRoute", description = "How the subject was exposed to the substance", type = "token") 3173 public static final String SP_ROUTE = "route"; 3174 /** 3175 * <b>Fluent Client</b> search parameter constant for <b>route</b> 3176 * <p> 3177 * Description: <b>How the subject was exposed to the substance</b><br> 3178 * Type: <b>token</b><br> 3179 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 3180 * </p> 3181 */ 3182 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3183 SP_ROUTE); 3184 3185 /** 3186 * Search parameter: <b>asserter</b> 3187 * <p> 3188 * Description: <b>Source of the information about the allergy</b><br> 3189 * Type: <b>reference</b><br> 3190 * Path: <b>AllergyIntolerance.asserter</b><br> 3191 * </p> 3192 */ 3193 @SearchParamDefinition(name = "asserter", path = "AllergyIntolerance.asserter", description = "Source of the information about the allergy", type = "reference", providesMembershipIn = { 3194 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3195 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3196 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 3197 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3198 public static final String SP_ASSERTER = "asserter"; 3199 /** 3200 * <b>Fluent Client</b> search parameter constant for <b>asserter</b> 3201 * <p> 3202 * Description: <b>Source of the information about the allergy</b><br> 3203 * Type: <b>reference</b><br> 3204 * Path: <b>AllergyIntolerance.asserter</b><br> 3205 * </p> 3206 */ 3207 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSERTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3208 SP_ASSERTER); 3209 3210 /** 3211 * Constant for fluent queries to be used to add include statements. Specifies 3212 * the path value of "<b>AllergyIntolerance:asserter</b>". 3213 */ 3214 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSERTER = new ca.uhn.fhir.model.api.Include( 3215 "AllergyIntolerance:asserter").toLocked(); 3216 3217 /** 3218 * Search parameter: <b>patient</b> 3219 * <p> 3220 * Description: <b>Who the sensitivity is for</b><br> 3221 * Type: <b>reference</b><br> 3222 * Path: <b>AllergyIntolerance.patient</b><br> 3223 * </p> 3224 */ 3225 @SearchParamDefinition(name = "patient", path = "AllergyIntolerance.patient", description = "Who the sensitivity is for", type = "reference", providesMembershipIn = { 3226 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3227 public static final String SP_PATIENT = "patient"; 3228 /** 3229 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3230 * <p> 3231 * Description: <b>Who the sensitivity is for</b><br> 3232 * Type: <b>reference</b><br> 3233 * Path: <b>AllergyIntolerance.patient</b><br> 3234 * </p> 3235 */ 3236 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3237 SP_PATIENT); 3238 3239 /** 3240 * Constant for fluent queries to be used to add include statements. Specifies 3241 * the path value of "<b>AllergyIntolerance:patient</b>". 3242 */ 3243 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3244 "AllergyIntolerance:patient").toLocked(); 3245 3246 /** 3247 * Search parameter: <b>category</b> 3248 * <p> 3249 * Description: <b>food | medication | environment | biologic</b><br> 3250 * Type: <b>token</b><br> 3251 * Path: <b>AllergyIntolerance.category</b><br> 3252 * </p> 3253 */ 3254 @SearchParamDefinition(name = "category", path = "AllergyIntolerance.category", description = "food | medication | environment | biologic", type = "token") 3255 public static final String SP_CATEGORY = "category"; 3256 /** 3257 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3258 * <p> 3259 * Description: <b>food | medication | environment | biologic</b><br> 3260 * Type: <b>token</b><br> 3261 * Path: <b>AllergyIntolerance.category</b><br> 3262 * </p> 3263 */ 3264 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3265 SP_CATEGORY); 3266 3267 /** 3268 * Search parameter: <b>last-date</b> 3269 * <p> 3270 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 3271 * Type: <b>date</b><br> 3272 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 3273 * </p> 3274 */ 3275 @SearchParamDefinition(name = "last-date", path = "AllergyIntolerance.lastOccurrence", description = "Date(/time) of last known occurrence of a reaction", type = "date") 3276 public static final String SP_LAST_DATE = "last-date"; 3277 /** 3278 * <b>Fluent Client</b> search parameter constant for <b>last-date</b> 3279 * <p> 3280 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 3281 * Type: <b>date</b><br> 3282 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 3283 * </p> 3284 */ 3285 public static final ca.uhn.fhir.rest.gclient.DateClientParam LAST_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3286 SP_LAST_DATE); 3287 3288}