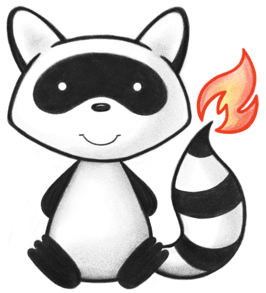
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Risk of harmful or undesirable, physiological response which is unique to an 049 * individual and associated with exposure to a substance. 050 */ 051@ResourceDef(name = "AllergyIntolerance", profile = "http://hl7.org/fhir/StructureDefinition/AllergyIntolerance") 052public class AllergyIntolerance extends DomainResource { 053 054 public enum AllergyIntoleranceType { 055 /** 056 * A propensity for hypersensitive reaction(s) to a substance. These reactions 057 * are most typically type I hypersensitivity, plus other "allergy-like" 058 * reactions, including pseudoallergy. 059 */ 060 ALLERGY, 061 /** 062 * A propensity for adverse reactions to a substance that is not judged to be 063 * allergic or "allergy-like". These reactions are typically (but not 064 * necessarily) non-immune. They are to some degree idiosyncratic and/or 065 * patient-specific (i.e. are not a reaction that is expected to occur with most 066 * or all patients given similar circumstances). 067 */ 068 INTOLERANCE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 074 public static AllergyIntoleranceType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("allergy".equals(codeString)) 078 return ALLERGY; 079 if ("intolerance".equals(codeString)) 080 return INTOLERANCE; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case ALLERGY: 090 return "allergy"; 091 case INTOLERANCE: 092 return "intolerance"; 093 case NULL: 094 return null; 095 default: 096 return "?"; 097 } 098 } 099 100 public String getSystem() { 101 switch (this) { 102 case ALLERGY: 103 return "http://hl7.org/fhir/allergy-intolerance-type"; 104 case INTOLERANCE: 105 return "http://hl7.org/fhir/allergy-intolerance-type"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getDefinition() { 114 switch (this) { 115 case ALLERGY: 116 return "A propensity for hypersensitive reaction(s) to a substance. These reactions are most typically type I hypersensitivity, plus other \"allergy-like\" reactions, including pseudoallergy."; 117 case INTOLERANCE: 118 return "A propensity for adverse reactions to a substance that is not judged to be allergic or \"allergy-like\". These reactions are typically (but not necessarily) non-immune. They are to some degree idiosyncratic and/or patient-specific (i.e. are not a reaction that is expected to occur with most or all patients given similar circumstances)."; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDisplay() { 127 switch (this) { 128 case ALLERGY: 129 return "Allergy"; 130 case INTOLERANCE: 131 return "Intolerance"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 } 139 140 public static class AllergyIntoleranceTypeEnumFactory implements EnumFactory<AllergyIntoleranceType> { 141 public AllergyIntoleranceType fromCode(String codeString) throws IllegalArgumentException { 142 if (codeString == null || "".equals(codeString)) 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("allergy".equals(codeString)) 146 return AllergyIntoleranceType.ALLERGY; 147 if ("intolerance".equals(codeString)) 148 return AllergyIntoleranceType.INTOLERANCE; 149 throw new IllegalArgumentException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 150 } 151 152 public Enumeration<AllergyIntoleranceType> fromType(PrimitiveType<?> code) throws FHIRException { 153 if (code == null) 154 return null; 155 if (code.isEmpty()) 156 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.NULL, code); 157 String codeString = code.asStringValue(); 158 if (codeString == null || "".equals(codeString)) 159 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.NULL, code); 160 if ("allergy".equals(codeString)) 161 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.ALLERGY, code); 162 if ("intolerance".equals(codeString)) 163 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.INTOLERANCE, code); 164 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 165 } 166 167 public String toCode(AllergyIntoleranceType code) { 168 if (code == AllergyIntoleranceType.NULL) 169 return null; 170 if (code == AllergyIntoleranceType.ALLERGY) 171 return "allergy"; 172 if (code == AllergyIntoleranceType.INTOLERANCE) 173 return "intolerance"; 174 return "?"; 175 } 176 177 public String toSystem(AllergyIntoleranceType code) { 178 return code.getSystem(); 179 } 180 } 181 182 public enum AllergyIntoleranceCategory { 183 /** 184 * Any substance consumed to provide nutritional support for the body. 185 */ 186 FOOD, 187 /** 188 * Substances administered to achieve a physiological effect. 189 */ 190 MEDICATION, 191 /** 192 * Any substances that are encountered in the environment, including any 193 * substance not already classified as food, medication, or biologic. 194 */ 195 ENVIRONMENT, 196 /** 197 * A preparation that is synthesized from living organisms or their products, 198 * especially a human or animal protein, such as a hormone or antitoxin, that is 199 * used as a diagnostic, preventive, or therapeutic agent. Examples of biologic 200 * medications include: vaccines; allergenic extracts, which are used for both 201 * diagnosis and treatment (for example, allergy shots); gene therapies; 202 * cellular therapies. There are other biologic products, such as tissues, which 203 * are not typically associated with allergies. 204 */ 205 BIOLOGIC, 206 /** 207 * added to help the parsers with the generic types 208 */ 209 NULL; 210 211 public static AllergyIntoleranceCategory fromCode(String codeString) throws FHIRException { 212 if (codeString == null || "".equals(codeString)) 213 return null; 214 if ("food".equals(codeString)) 215 return FOOD; 216 if ("medication".equals(codeString)) 217 return MEDICATION; 218 if ("environment".equals(codeString)) 219 return ENVIRONMENT; 220 if ("biologic".equals(codeString)) 221 return BIOLOGIC; 222 if (Configuration.isAcceptInvalidEnums()) 223 return null; 224 else 225 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 226 } 227 228 public String toCode() { 229 switch (this) { 230 case FOOD: 231 return "food"; 232 case MEDICATION: 233 return "medication"; 234 case ENVIRONMENT: 235 return "environment"; 236 case BIOLOGIC: 237 return "biologic"; 238 case NULL: 239 return null; 240 default: 241 return "?"; 242 } 243 } 244 245 public String getSystem() { 246 switch (this) { 247 case FOOD: 248 return "http://hl7.org/fhir/allergy-intolerance-category"; 249 case MEDICATION: 250 return "http://hl7.org/fhir/allergy-intolerance-category"; 251 case ENVIRONMENT: 252 return "http://hl7.org/fhir/allergy-intolerance-category"; 253 case BIOLOGIC: 254 return "http://hl7.org/fhir/allergy-intolerance-category"; 255 case NULL: 256 return null; 257 default: 258 return "?"; 259 } 260 } 261 262 public String getDefinition() { 263 switch (this) { 264 case FOOD: 265 return "Any substance consumed to provide nutritional support for the body."; 266 case MEDICATION: 267 return "Substances administered to achieve a physiological effect."; 268 case ENVIRONMENT: 269 return "Any substances that are encountered in the environment, including any substance not already classified as food, medication, or biologic."; 270 case BIOLOGIC: 271 return "A preparation that is synthesized from living organisms or their products, especially a human or animal protein, such as a hormone or antitoxin, that is used as a diagnostic, preventive, or therapeutic agent. Examples of biologic medications include: vaccines; allergenic extracts, which are used for both diagnosis and treatment (for example, allergy shots); gene therapies; cellular therapies. There are other biologic products, such as tissues, which are not typically associated with allergies."; 272 case NULL: 273 return null; 274 default: 275 return "?"; 276 } 277 } 278 279 public String getDisplay() { 280 switch (this) { 281 case FOOD: 282 return "Food"; 283 case MEDICATION: 284 return "Medication"; 285 case ENVIRONMENT: 286 return "Environment"; 287 case BIOLOGIC: 288 return "Biologic"; 289 case NULL: 290 return null; 291 default: 292 return "?"; 293 } 294 } 295 } 296 297 public static class AllergyIntoleranceCategoryEnumFactory implements EnumFactory<AllergyIntoleranceCategory> { 298 public AllergyIntoleranceCategory fromCode(String codeString) throws IllegalArgumentException { 299 if (codeString == null || "".equals(codeString)) 300 if (codeString == null || "".equals(codeString)) 301 return null; 302 if ("food".equals(codeString)) 303 return AllergyIntoleranceCategory.FOOD; 304 if ("medication".equals(codeString)) 305 return AllergyIntoleranceCategory.MEDICATION; 306 if ("environment".equals(codeString)) 307 return AllergyIntoleranceCategory.ENVIRONMENT; 308 if ("biologic".equals(codeString)) 309 return AllergyIntoleranceCategory.BIOLOGIC; 310 throw new IllegalArgumentException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 311 } 312 313 public Enumeration<AllergyIntoleranceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 314 if (code == null) 315 return null; 316 if (code.isEmpty()) 317 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.NULL, code); 318 String codeString = code.asStringValue(); 319 if (codeString == null || "".equals(codeString)) 320 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.NULL, code); 321 if ("food".equals(codeString)) 322 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.FOOD, code); 323 if ("medication".equals(codeString)) 324 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.MEDICATION, code); 325 if ("environment".equals(codeString)) 326 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.ENVIRONMENT, code); 327 if ("biologic".equals(codeString)) 328 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.BIOLOGIC, code); 329 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 330 } 331 332 public String toCode(AllergyIntoleranceCategory code) { 333 if (code == AllergyIntoleranceCategory.NULL) 334 return null; 335 if (code == AllergyIntoleranceCategory.FOOD) 336 return "food"; 337 if (code == AllergyIntoleranceCategory.MEDICATION) 338 return "medication"; 339 if (code == AllergyIntoleranceCategory.ENVIRONMENT) 340 return "environment"; 341 if (code == AllergyIntoleranceCategory.BIOLOGIC) 342 return "biologic"; 343 return "?"; 344 } 345 346 public String toSystem(AllergyIntoleranceCategory code) { 347 return code.getSystem(); 348 } 349 } 350 351 public enum AllergyIntoleranceCriticality { 352 /** 353 * Worst case result of a future exposure is not assessed to be life-threatening 354 * or having high potential for organ system failure. 355 */ 356 LOW, 357 /** 358 * Worst case result of a future exposure is assessed to be life-threatening or 359 * having high potential for organ system failure. 360 */ 361 HIGH, 362 /** 363 * Unable to assess the worst case result of a future exposure. 364 */ 365 UNABLETOASSESS, 366 /** 367 * added to help the parsers with the generic types 368 */ 369 NULL; 370 371 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 372 if (codeString == null || "".equals(codeString)) 373 return null; 374 if ("low".equals(codeString)) 375 return LOW; 376 if ("high".equals(codeString)) 377 return HIGH; 378 if ("unable-to-assess".equals(codeString)) 379 return UNABLETOASSESS; 380 if (Configuration.isAcceptInvalidEnums()) 381 return null; 382 else 383 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 384 } 385 386 public String toCode() { 387 switch (this) { 388 case LOW: 389 return "low"; 390 case HIGH: 391 return "high"; 392 case UNABLETOASSESS: 393 return "unable-to-assess"; 394 case NULL: 395 return null; 396 default: 397 return "?"; 398 } 399 } 400 401 public String getSystem() { 402 switch (this) { 403 case LOW: 404 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 405 case HIGH: 406 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 407 case UNABLETOASSESS: 408 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 409 case NULL: 410 return null; 411 default: 412 return "?"; 413 } 414 } 415 416 public String getDefinition() { 417 switch (this) { 418 case LOW: 419 return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 420 case HIGH: 421 return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 422 case UNABLETOASSESS: 423 return "Unable to assess the worst case result of a future exposure."; 424 case NULL: 425 return null; 426 default: 427 return "?"; 428 } 429 } 430 431 public String getDisplay() { 432 switch (this) { 433 case LOW: 434 return "Low Risk"; 435 case HIGH: 436 return "High Risk"; 437 case UNABLETOASSESS: 438 return "Unable to Assess Risk"; 439 case NULL: 440 return null; 441 default: 442 return "?"; 443 } 444 } 445 } 446 447 public static class AllergyIntoleranceCriticalityEnumFactory implements EnumFactory<AllergyIntoleranceCriticality> { 448 public AllergyIntoleranceCriticality fromCode(String codeString) throws IllegalArgumentException { 449 if (codeString == null || "".equals(codeString)) 450 if (codeString == null || "".equals(codeString)) 451 return null; 452 if ("low".equals(codeString)) 453 return AllergyIntoleranceCriticality.LOW; 454 if ("high".equals(codeString)) 455 return AllergyIntoleranceCriticality.HIGH; 456 if ("unable-to-assess".equals(codeString)) 457 return AllergyIntoleranceCriticality.UNABLETOASSESS; 458 throw new IllegalArgumentException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 459 } 460 461 public Enumeration<AllergyIntoleranceCriticality> fromType(PrimitiveType<?> code) throws FHIRException { 462 if (code == null) 463 return null; 464 if (code.isEmpty()) 465 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.NULL, code); 466 String codeString = code.asStringValue(); 467 if (codeString == null || "".equals(codeString)) 468 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.NULL, code); 469 if ("low".equals(codeString)) 470 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.LOW, code); 471 if ("high".equals(codeString)) 472 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.HIGH, code); 473 if ("unable-to-assess".equals(codeString)) 474 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.UNABLETOASSESS, code); 475 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 476 } 477 478 public String toCode(AllergyIntoleranceCriticality code) { 479 if (code == AllergyIntoleranceCriticality.NULL) 480 return null; 481 if (code == AllergyIntoleranceCriticality.LOW) 482 return "low"; 483 if (code == AllergyIntoleranceCriticality.HIGH) 484 return "high"; 485 if (code == AllergyIntoleranceCriticality.UNABLETOASSESS) 486 return "unable-to-assess"; 487 return "?"; 488 } 489 490 public String toSystem(AllergyIntoleranceCriticality code) { 491 return code.getSystem(); 492 } 493 } 494 495 public enum AllergyIntoleranceSeverity { 496 /** 497 * Causes mild physiological effects. 498 */ 499 MILD, 500 /** 501 * Causes moderate physiological effects. 502 */ 503 MODERATE, 504 /** 505 * Causes severe physiological effects. 506 */ 507 SEVERE, 508 /** 509 * added to help the parsers with the generic types 510 */ 511 NULL; 512 513 public static AllergyIntoleranceSeverity fromCode(String codeString) throws FHIRException { 514 if (codeString == null || "".equals(codeString)) 515 return null; 516 if ("mild".equals(codeString)) 517 return MILD; 518 if ("moderate".equals(codeString)) 519 return MODERATE; 520 if ("severe".equals(codeString)) 521 return SEVERE; 522 if (Configuration.isAcceptInvalidEnums()) 523 return null; 524 else 525 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 526 } 527 528 public String toCode() { 529 switch (this) { 530 case MILD: 531 return "mild"; 532 case MODERATE: 533 return "moderate"; 534 case SEVERE: 535 return "severe"; 536 case NULL: 537 return null; 538 default: 539 return "?"; 540 } 541 } 542 543 public String getSystem() { 544 switch (this) { 545 case MILD: 546 return "http://hl7.org/fhir/reaction-event-severity"; 547 case MODERATE: 548 return "http://hl7.org/fhir/reaction-event-severity"; 549 case SEVERE: 550 return "http://hl7.org/fhir/reaction-event-severity"; 551 case NULL: 552 return null; 553 default: 554 return "?"; 555 } 556 } 557 558 public String getDefinition() { 559 switch (this) { 560 case MILD: 561 return "Causes mild physiological effects."; 562 case MODERATE: 563 return "Causes moderate physiological effects."; 564 case SEVERE: 565 return "Causes severe physiological effects."; 566 case NULL: 567 return null; 568 default: 569 return "?"; 570 } 571 } 572 573 public String getDisplay() { 574 switch (this) { 575 case MILD: 576 return "Mild"; 577 case MODERATE: 578 return "Moderate"; 579 case SEVERE: 580 return "Severe"; 581 case NULL: 582 return null; 583 default: 584 return "?"; 585 } 586 } 587 } 588 589 public static class AllergyIntoleranceSeverityEnumFactory implements EnumFactory<AllergyIntoleranceSeverity> { 590 public AllergyIntoleranceSeverity fromCode(String codeString) throws IllegalArgumentException { 591 if (codeString == null || "".equals(codeString)) 592 if (codeString == null || "".equals(codeString)) 593 return null; 594 if ("mild".equals(codeString)) 595 return AllergyIntoleranceSeverity.MILD; 596 if ("moderate".equals(codeString)) 597 return AllergyIntoleranceSeverity.MODERATE; 598 if ("severe".equals(codeString)) 599 return AllergyIntoleranceSeverity.SEVERE; 600 throw new IllegalArgumentException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 601 } 602 603 public Enumeration<AllergyIntoleranceSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 604 if (code == null) 605 return null; 606 if (code.isEmpty()) 607 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.NULL, code); 608 String codeString = code.asStringValue(); 609 if (codeString == null || "".equals(codeString)) 610 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.NULL, code); 611 if ("mild".equals(codeString)) 612 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MILD, code); 613 if ("moderate".equals(codeString)) 614 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MODERATE, code); 615 if ("severe".equals(codeString)) 616 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.SEVERE, code); 617 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 618 } 619 620 public String toCode(AllergyIntoleranceSeverity code) { 621 if (code == AllergyIntoleranceSeverity.NULL) 622 return null; 623 if (code == AllergyIntoleranceSeverity.MILD) 624 return "mild"; 625 if (code == AllergyIntoleranceSeverity.MODERATE) 626 return "moderate"; 627 if (code == AllergyIntoleranceSeverity.SEVERE) 628 return "severe"; 629 return "?"; 630 } 631 632 public String toSystem(AllergyIntoleranceSeverity code) { 633 return code.getSystem(); 634 } 635 } 636 637 @Block() 638 public static class AllergyIntoleranceReactionComponent extends BackboneElement implements IBaseBackboneElement { 639 /** 640 * Identification of the specific substance (or pharmaceutical product) 641 * considered to be responsible for the Adverse Reaction event. Note: the 642 * substance for a specific reaction may be different from the substance 643 * identified as the cause of the risk, but it must be consistent with it. For 644 * instance, it may be a more specific substance (e.g. a brand medication) or a 645 * composite product that includes the identified substance. It must be 646 * clinically safe to only process the 'code' and ignore the 647 * 'reaction.substance'. If a receiving system is unable to confirm that 648 * AllergyIntolerance.reaction.substance falls within the semantic scope of 649 * AllergyIntolerance.code, then the receiving system should ignore 650 * AllergyIntolerance.reaction.substance. 651 */ 652 @Child(name = "substance", type = { 653 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 654 @Description(shortDefinition = "Specific substance or pharmaceutical product considered to be responsible for event", formalDefinition = "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.") 655 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/substance-code") 656 protected CodeableConcept substance; 657 658 /** 659 * Clinical symptoms and/or signs that are observed or associated with the 660 * adverse reaction event. 661 */ 662 @Child(name = "manifestation", type = { 663 CodeableConcept.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 664 @Description(shortDefinition = "Clinical symptoms/signs associated with the Event", formalDefinition = "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.") 665 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 666 protected List<CodeableConcept> manifestation; 667 668 /** 669 * Text description about the reaction as a whole, including details of the 670 * manifestation if required. 671 */ 672 @Child(name = "description", type = { 673 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 674 @Description(shortDefinition = "Description of the event as a whole", formalDefinition = "Text description about the reaction as a whole, including details of the manifestation if required.") 675 protected StringType description; 676 677 /** 678 * Record of the date and/or time of the onset of the Reaction. 679 */ 680 @Child(name = "onset", type = { 681 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 682 @Description(shortDefinition = "Date(/time) when manifestations showed", formalDefinition = "Record of the date and/or time of the onset of the Reaction.") 683 protected DateTimeType onset; 684 685 /** 686 * Clinical assessment of the severity of the reaction event as a whole, 687 * potentially considering multiple different manifestations. 688 */ 689 @Child(name = "severity", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 690 @Description(shortDefinition = "mild | moderate | severe (of event as a whole)", formalDefinition = "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.") 691 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reaction-event-severity") 692 protected Enumeration<AllergyIntoleranceSeverity> severity; 693 694 /** 695 * Identification of the route by which the subject was exposed to the 696 * substance. 697 */ 698 @Child(name = "exposureRoute", type = { 699 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 700 @Description(shortDefinition = "How the subject was exposed to the substance", formalDefinition = "Identification of the route by which the subject was exposed to the substance.") 701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/route-codes") 702 protected CodeableConcept exposureRoute; 703 704 /** 705 * Additional text about the adverse reaction event not captured in other 706 * fields. 707 */ 708 @Child(name = "note", type = { 709 Annotation.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 710 @Description(shortDefinition = "Text about event not captured in other fields", formalDefinition = "Additional text about the adverse reaction event not captured in other fields.") 711 protected List<Annotation> note; 712 713 private static final long serialVersionUID = -752118516L; 714 715 /** 716 * Constructor 717 */ 718 public AllergyIntoleranceReactionComponent() { 719 super(); 720 } 721 722 /** 723 * @return {@link #substance} (Identification of the specific substance (or 724 * pharmaceutical product) considered to be responsible for the Adverse 725 * Reaction event. Note: the substance for a specific reaction may be 726 * different from the substance identified as the cause of the risk, but 727 * it must be consistent with it. For instance, it may be a more 728 * specific substance (e.g. a brand medication) or a composite product 729 * that includes the identified substance. It must be clinically safe to 730 * only process the 'code' and ignore the 'reaction.substance'. If a 731 * receiving system is unable to confirm that 732 * AllergyIntolerance.reaction.substance falls within the semantic scope 733 * of AllergyIntolerance.code, then the receiving system should ignore 734 * AllergyIntolerance.reaction.substance.) 735 */ 736 public CodeableConcept getSubstance() { 737 if (this.substance == null) 738 if (Configuration.errorOnAutoCreate()) 739 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.substance"); 740 else if (Configuration.doAutoCreate()) 741 this.substance = new CodeableConcept(); // cc 742 return this.substance; 743 } 744 745 public boolean hasSubstance() { 746 return this.substance != null && !this.substance.isEmpty(); 747 } 748 749 /** 750 * @param value {@link #substance} (Identification of the specific substance (or 751 * pharmaceutical product) considered to be responsible for the 752 * Adverse Reaction event. Note: the substance for a specific 753 * reaction may be different from the substance identified as the 754 * cause of the risk, but it must be consistent with it. For 755 * instance, it may be a more specific substance (e.g. a brand 756 * medication) or a composite product that includes the identified 757 * substance. It must be clinically safe to only process the 'code' 758 * and ignore the 'reaction.substance'. If a receiving system is 759 * unable to confirm that AllergyIntolerance.reaction.substance 760 * falls within the semantic scope of AllergyIntolerance.code, then 761 * the receiving system should ignore 762 * AllergyIntolerance.reaction.substance.) 763 */ 764 public AllergyIntoleranceReactionComponent setSubstance(CodeableConcept value) { 765 this.substance = value; 766 return this; 767 } 768 769 /** 770 * @return {@link #manifestation} (Clinical symptoms and/or signs that are 771 * observed or associated with the adverse reaction event.) 772 */ 773 public List<CodeableConcept> getManifestation() { 774 if (this.manifestation == null) 775 this.manifestation = new ArrayList<CodeableConcept>(); 776 return this.manifestation; 777 } 778 779 /** 780 * @return Returns a reference to <code>this</code> for easy method chaining 781 */ 782 public AllergyIntoleranceReactionComponent setManifestation(List<CodeableConcept> theManifestation) { 783 this.manifestation = theManifestation; 784 return this; 785 } 786 787 public boolean hasManifestation() { 788 if (this.manifestation == null) 789 return false; 790 for (CodeableConcept item : this.manifestation) 791 if (!item.isEmpty()) 792 return true; 793 return false; 794 } 795 796 public CodeableConcept addManifestation() { // 3 797 CodeableConcept t = new CodeableConcept(); 798 if (this.manifestation == null) 799 this.manifestation = new ArrayList<CodeableConcept>(); 800 this.manifestation.add(t); 801 return t; 802 } 803 804 public AllergyIntoleranceReactionComponent addManifestation(CodeableConcept t) { // 3 805 if (t == null) 806 return this; 807 if (this.manifestation == null) 808 this.manifestation = new ArrayList<CodeableConcept>(); 809 this.manifestation.add(t); 810 return this; 811 } 812 813 /** 814 * @return The first repetition of repeating field {@link #manifestation}, 815 * creating it if it does not already exist 816 */ 817 public CodeableConcept getManifestationFirstRep() { 818 if (getManifestation().isEmpty()) { 819 addManifestation(); 820 } 821 return getManifestation().get(0); 822 } 823 824 /** 825 * @return {@link #description} (Text description about the reaction as a whole, 826 * including details of the manifestation if required.). This is the 827 * underlying object with id, value and extensions. The accessor 828 * "getDescription" gives direct access to the value 829 */ 830 public StringType getDescriptionElement() { 831 if (this.description == null) 832 if (Configuration.errorOnAutoCreate()) 833 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.description"); 834 else if (Configuration.doAutoCreate()) 835 this.description = new StringType(); // bb 836 return this.description; 837 } 838 839 public boolean hasDescriptionElement() { 840 return this.description != null && !this.description.isEmpty(); 841 } 842 843 public boolean hasDescription() { 844 return this.description != null && !this.description.isEmpty(); 845 } 846 847 /** 848 * @param value {@link #description} (Text description about the reaction as a 849 * whole, including details of the manifestation if required.). 850 * This is the underlying object with id, value and extensions. The 851 * accessor "getDescription" gives direct access to the value 852 */ 853 public AllergyIntoleranceReactionComponent setDescriptionElement(StringType value) { 854 this.description = value; 855 return this; 856 } 857 858 /** 859 * @return Text description about the reaction as a whole, including details of 860 * the manifestation if required. 861 */ 862 public String getDescription() { 863 return this.description == null ? null : this.description.getValue(); 864 } 865 866 /** 867 * @param value Text description about the reaction as a whole, including 868 * details of the manifestation if required. 869 */ 870 public AllergyIntoleranceReactionComponent setDescription(String value) { 871 if (Utilities.noString(value)) 872 this.description = null; 873 else { 874 if (this.description == null) 875 this.description = new StringType(); 876 this.description.setValue(value); 877 } 878 return this; 879 } 880 881 /** 882 * @return {@link #onset} (Record of the date and/or time of the onset of the 883 * Reaction.). This is the underlying object with id, value and 884 * extensions. The accessor "getOnset" gives direct access to the value 885 */ 886 public DateTimeType getOnsetElement() { 887 if (this.onset == null) 888 if (Configuration.errorOnAutoCreate()) 889 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.onset"); 890 else if (Configuration.doAutoCreate()) 891 this.onset = new DateTimeType(); // bb 892 return this.onset; 893 } 894 895 public boolean hasOnsetElement() { 896 return this.onset != null && !this.onset.isEmpty(); 897 } 898 899 public boolean hasOnset() { 900 return this.onset != null && !this.onset.isEmpty(); 901 } 902 903 /** 904 * @param value {@link #onset} (Record of the date and/or time of the onset of 905 * the Reaction.). This is the underlying object with id, value and 906 * extensions. The accessor "getOnset" gives direct access to the 907 * value 908 */ 909 public AllergyIntoleranceReactionComponent setOnsetElement(DateTimeType value) { 910 this.onset = value; 911 return this; 912 } 913 914 /** 915 * @return Record of the date and/or time of the onset of the Reaction. 916 */ 917 public Date getOnset() { 918 return this.onset == null ? null : this.onset.getValue(); 919 } 920 921 /** 922 * @param value Record of the date and/or time of the onset of the Reaction. 923 */ 924 public AllergyIntoleranceReactionComponent setOnset(Date value) { 925 if (value == null) 926 this.onset = null; 927 else { 928 if (this.onset == null) 929 this.onset = new DateTimeType(); 930 this.onset.setValue(value); 931 } 932 return this; 933 } 934 935 /** 936 * @return {@link #severity} (Clinical assessment of the severity of the 937 * reaction event as a whole, potentially considering multiple different 938 * manifestations.). This is the underlying object with id, value and 939 * extensions. The accessor "getSeverity" gives direct access to the 940 * value 941 */ 942 public Enumeration<AllergyIntoleranceSeverity> getSeverityElement() { 943 if (this.severity == null) 944 if (Configuration.errorOnAutoCreate()) 945 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.severity"); 946 else if (Configuration.doAutoCreate()) 947 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); // bb 948 return this.severity; 949 } 950 951 public boolean hasSeverityElement() { 952 return this.severity != null && !this.severity.isEmpty(); 953 } 954 955 public boolean hasSeverity() { 956 return this.severity != null && !this.severity.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #severity} (Clinical assessment of the severity of the 961 * reaction event as a whole, potentially considering multiple 962 * different manifestations.). This is the underlying object with 963 * id, value and extensions. The accessor "getSeverity" gives 964 * direct access to the value 965 */ 966 public AllergyIntoleranceReactionComponent setSeverityElement(Enumeration<AllergyIntoleranceSeverity> value) { 967 this.severity = value; 968 return this; 969 } 970 971 /** 972 * @return Clinical assessment of the severity of the reaction event as a whole, 973 * potentially considering multiple different manifestations. 974 */ 975 public AllergyIntoleranceSeverity getSeverity() { 976 return this.severity == null ? null : this.severity.getValue(); 977 } 978 979 /** 980 * @param value Clinical assessment of the severity of the reaction event as a 981 * whole, potentially considering multiple different 982 * manifestations. 983 */ 984 public AllergyIntoleranceReactionComponent setSeverity(AllergyIntoleranceSeverity value) { 985 if (value == null) 986 this.severity = null; 987 else { 988 if (this.severity == null) 989 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); 990 this.severity.setValue(value); 991 } 992 return this; 993 } 994 995 /** 996 * @return {@link #exposureRoute} (Identification of the route by which the 997 * subject was exposed to the substance.) 998 */ 999 public CodeableConcept getExposureRoute() { 1000 if (this.exposureRoute == null) 1001 if (Configuration.errorOnAutoCreate()) 1002 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.exposureRoute"); 1003 else if (Configuration.doAutoCreate()) 1004 this.exposureRoute = new CodeableConcept(); // cc 1005 return this.exposureRoute; 1006 } 1007 1008 public boolean hasExposureRoute() { 1009 return this.exposureRoute != null && !this.exposureRoute.isEmpty(); 1010 } 1011 1012 /** 1013 * @param value {@link #exposureRoute} (Identification of the route by which the 1014 * subject was exposed to the substance.) 1015 */ 1016 public AllergyIntoleranceReactionComponent setExposureRoute(CodeableConcept value) { 1017 this.exposureRoute = value; 1018 return this; 1019 } 1020 1021 /** 1022 * @return {@link #note} (Additional text about the adverse reaction event not 1023 * captured in other fields.) 1024 */ 1025 public List<Annotation> getNote() { 1026 if (this.note == null) 1027 this.note = new ArrayList<Annotation>(); 1028 return this.note; 1029 } 1030 1031 /** 1032 * @return Returns a reference to <code>this</code> for easy method chaining 1033 */ 1034 public AllergyIntoleranceReactionComponent setNote(List<Annotation> theNote) { 1035 this.note = theNote; 1036 return this; 1037 } 1038 1039 public boolean hasNote() { 1040 if (this.note == null) 1041 return false; 1042 for (Annotation item : this.note) 1043 if (!item.isEmpty()) 1044 return true; 1045 return false; 1046 } 1047 1048 public Annotation addNote() { // 3 1049 Annotation t = new Annotation(); 1050 if (this.note == null) 1051 this.note = new ArrayList<Annotation>(); 1052 this.note.add(t); 1053 return t; 1054 } 1055 1056 public AllergyIntoleranceReactionComponent addNote(Annotation t) { // 3 1057 if (t == null) 1058 return this; 1059 if (this.note == null) 1060 this.note = new ArrayList<Annotation>(); 1061 this.note.add(t); 1062 return this; 1063 } 1064 1065 /** 1066 * @return The first repetition of repeating field {@link #note}, creating it if 1067 * it does not already exist 1068 */ 1069 public Annotation getNoteFirstRep() { 1070 if (getNote().isEmpty()) { 1071 addNote(); 1072 } 1073 return getNote().get(0); 1074 } 1075 1076 protected void listChildren(List<Property> children) { 1077 super.listChildren(children); 1078 children.add(new Property("substance", "CodeableConcept", 1079 "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 1080 0, 1, substance)); 1081 children.add(new Property("manifestation", "CodeableConcept", 1082 "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, 1083 java.lang.Integer.MAX_VALUE, manifestation)); 1084 children.add(new Property("description", "string", 1085 "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, 1086 description)); 1087 children.add(new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 0, 1088 1, onset)); 1089 children.add(new Property("severity", "code", 1090 "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 1091 0, 1, severity)); 1092 children.add(new Property("exposureRoute", "CodeableConcept", 1093 "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute)); 1094 children.add(new Property("note", "Annotation", 1095 "Additional text about the adverse reaction event not captured in other fields.", 0, 1096 java.lang.Integer.MAX_VALUE, note)); 1097 } 1098 1099 @Override 1100 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1101 switch (_hash) { 1102 case 530040176: 1103 /* substance */ return new Property("substance", "CodeableConcept", 1104 "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 1105 0, 1, substance); 1106 case 1115984422: 1107 /* manifestation */ return new Property("manifestation", "CodeableConcept", 1108 "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, 1109 java.lang.Integer.MAX_VALUE, manifestation); 1110 case -1724546052: 1111 /* description */ return new Property("description", "string", 1112 "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, 1113 description); 1114 case 105901603: 1115 /* onset */ return new Property("onset", "dateTime", 1116 "Record of the date and/or time of the onset of the Reaction.", 0, 1, onset); 1117 case 1478300413: 1118 /* severity */ return new Property("severity", "code", 1119 "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 1120 0, 1, severity); 1121 case 421286274: 1122 /* exposureRoute */ return new Property("exposureRoute", "CodeableConcept", 1123 "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute); 1124 case 3387378: 1125 /* note */ return new Property("note", "Annotation", 1126 "Additional text about the adverse reaction event not captured in other fields.", 0, 1127 java.lang.Integer.MAX_VALUE, note); 1128 default: 1129 return super.getNamedProperty(_hash, _name, _checkValid); 1130 } 1131 1132 } 1133 1134 @Override 1135 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1136 switch (hash) { 1137 case 530040176: 1138 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // CodeableConcept 1139 case 1115984422: 1140 /* manifestation */ return this.manifestation == null ? new Base[0] 1141 : this.manifestation.toArray(new Base[this.manifestation.size()]); // CodeableConcept 1142 case -1724546052: 1143 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1144 case 105901603: 1145 /* onset */ return this.onset == null ? new Base[0] : new Base[] { this.onset }; // DateTimeType 1146 case 1478300413: 1147 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<AllergyIntoleranceSeverity> 1148 case 421286274: 1149 /* exposureRoute */ return this.exposureRoute == null ? new Base[0] : new Base[] { this.exposureRoute }; // CodeableConcept 1150 case 3387378: 1151 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1152 default: 1153 return super.getProperty(hash, name, checkValid); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1160 switch (hash) { 1161 case 530040176: // substance 1162 this.substance = castToCodeableConcept(value); // CodeableConcept 1163 return value; 1164 case 1115984422: // manifestation 1165 this.getManifestation().add(castToCodeableConcept(value)); // CodeableConcept 1166 return value; 1167 case -1724546052: // description 1168 this.description = castToString(value); // StringType 1169 return value; 1170 case 105901603: // onset 1171 this.onset = castToDateTime(value); // DateTimeType 1172 return value; 1173 case 1478300413: // severity 1174 value = new AllergyIntoleranceSeverityEnumFactory().fromType(castToCode(value)); 1175 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1176 return value; 1177 case 421286274: // exposureRoute 1178 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1179 return value; 1180 case 3387378: // note 1181 this.getNote().add(castToAnnotation(value)); // Annotation 1182 return value; 1183 default: 1184 return super.setProperty(hash, name, value); 1185 } 1186 1187 } 1188 1189 @Override 1190 public Base setProperty(String name, Base value) throws FHIRException { 1191 if (name.equals("substance")) { 1192 this.substance = castToCodeableConcept(value); // CodeableConcept 1193 } else if (name.equals("manifestation")) { 1194 this.getManifestation().add(castToCodeableConcept(value)); 1195 } else if (name.equals("description")) { 1196 this.description = castToString(value); // StringType 1197 } else if (name.equals("onset")) { 1198 this.onset = castToDateTime(value); // DateTimeType 1199 } else if (name.equals("severity")) { 1200 value = new AllergyIntoleranceSeverityEnumFactory().fromType(castToCode(value)); 1201 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1202 } else if (name.equals("exposureRoute")) { 1203 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1204 } else if (name.equals("note")) { 1205 this.getNote().add(castToAnnotation(value)); 1206 } else 1207 return super.setProperty(name, value); 1208 return value; 1209 } 1210 1211 @Override 1212 public void removeChild(String name, Base value) throws FHIRException { 1213 if (name.equals("substance")) { 1214 this.substance = null; 1215 } else if (name.equals("manifestation")) { 1216 this.getManifestation().remove(castToCodeableConcept(value)); 1217 } else if (name.equals("description")) { 1218 this.description = null; 1219 } else if (name.equals("onset")) { 1220 this.onset = null; 1221 } else if (name.equals("severity")) { 1222 this.severity = null; 1223 } else if (name.equals("exposureRoute")) { 1224 this.exposureRoute = null; 1225 } else if (name.equals("note")) { 1226 this.getNote().remove(castToAnnotation(value)); 1227 } else 1228 super.removeChild(name, value); 1229 1230 } 1231 1232 @Override 1233 public Base makeProperty(int hash, String name) throws FHIRException { 1234 switch (hash) { 1235 case 530040176: 1236 return getSubstance(); 1237 case 1115984422: 1238 return addManifestation(); 1239 case -1724546052: 1240 return getDescriptionElement(); 1241 case 105901603: 1242 return getOnsetElement(); 1243 case 1478300413: 1244 return getSeverityElement(); 1245 case 421286274: 1246 return getExposureRoute(); 1247 case 3387378: 1248 return addNote(); 1249 default: 1250 return super.makeProperty(hash, name); 1251 } 1252 1253 } 1254 1255 @Override 1256 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1257 switch (hash) { 1258 case 530040176: 1259 /* substance */ return new String[] { "CodeableConcept" }; 1260 case 1115984422: 1261 /* manifestation */ return new String[] { "CodeableConcept" }; 1262 case -1724546052: 1263 /* description */ return new String[] { "string" }; 1264 case 105901603: 1265 /* onset */ return new String[] { "dateTime" }; 1266 case 1478300413: 1267 /* severity */ return new String[] { "code" }; 1268 case 421286274: 1269 /* exposureRoute */ return new String[] { "CodeableConcept" }; 1270 case 3387378: 1271 /* note */ return new String[] { "Annotation" }; 1272 default: 1273 return super.getTypesForProperty(hash, name); 1274 } 1275 1276 } 1277 1278 @Override 1279 public Base addChild(String name) throws FHIRException { 1280 if (name.equals("substance")) { 1281 this.substance = new CodeableConcept(); 1282 return this.substance; 1283 } else if (name.equals("manifestation")) { 1284 return addManifestation(); 1285 } else if (name.equals("description")) { 1286 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.description"); 1287 } else if (name.equals("onset")) { 1288 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 1289 } else if (name.equals("severity")) { 1290 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.severity"); 1291 } else if (name.equals("exposureRoute")) { 1292 this.exposureRoute = new CodeableConcept(); 1293 return this.exposureRoute; 1294 } else if (name.equals("note")) { 1295 return addNote(); 1296 } else 1297 return super.addChild(name); 1298 } 1299 1300 public AllergyIntoleranceReactionComponent copy() { 1301 AllergyIntoleranceReactionComponent dst = new AllergyIntoleranceReactionComponent(); 1302 copyValues(dst); 1303 return dst; 1304 } 1305 1306 public void copyValues(AllergyIntoleranceReactionComponent dst) { 1307 super.copyValues(dst); 1308 dst.substance = substance == null ? null : substance.copy(); 1309 if (manifestation != null) { 1310 dst.manifestation = new ArrayList<CodeableConcept>(); 1311 for (CodeableConcept i : manifestation) 1312 dst.manifestation.add(i.copy()); 1313 } 1314 ; 1315 dst.description = description == null ? null : description.copy(); 1316 dst.onset = onset == null ? null : onset.copy(); 1317 dst.severity = severity == null ? null : severity.copy(); 1318 dst.exposureRoute = exposureRoute == null ? null : exposureRoute.copy(); 1319 if (note != null) { 1320 dst.note = new ArrayList<Annotation>(); 1321 for (Annotation i : note) 1322 dst.note.add(i.copy()); 1323 } 1324 ; 1325 } 1326 1327 @Override 1328 public boolean equalsDeep(Base other_) { 1329 if (!super.equalsDeep(other_)) 1330 return false; 1331 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1332 return false; 1333 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1334 return compareDeep(substance, o.substance, true) && compareDeep(manifestation, o.manifestation, true) 1335 && compareDeep(description, o.description, true) && compareDeep(onset, o.onset, true) 1336 && compareDeep(severity, o.severity, true) && compareDeep(exposureRoute, o.exposureRoute, true) 1337 && compareDeep(note, o.note, true); 1338 } 1339 1340 @Override 1341 public boolean equalsShallow(Base other_) { 1342 if (!super.equalsShallow(other_)) 1343 return false; 1344 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1345 return false; 1346 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1347 return compareValues(description, o.description, true) && compareValues(onset, o.onset, true) 1348 && compareValues(severity, o.severity, true); 1349 } 1350 1351 public boolean isEmpty() { 1352 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, manifestation, description, onset, 1353 severity, exposureRoute, note); 1354 } 1355 1356 public String fhirType() { 1357 return "AllergyIntolerance.reaction"; 1358 1359 } 1360 1361 } 1362 1363 /** 1364 * Business identifiers assigned to this AllergyIntolerance by the performer or 1365 * other systems which remain constant as the resource is updated and propagates 1366 * from server to server. 1367 */ 1368 @Child(name = "identifier", type = { 1369 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1370 @Description(shortDefinition = "External ids for this item", formalDefinition = "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 1371 protected List<Identifier> identifier; 1372 1373 /** 1374 * The clinical status of the allergy or intolerance. 1375 */ 1376 @Child(name = "clinicalStatus", type = { 1377 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1378 @Description(shortDefinition = "active | inactive | resolved", formalDefinition = "The clinical status of the allergy or intolerance.") 1379 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergyintolerance-clinical") 1380 protected CodeableConcept clinicalStatus; 1381 1382 /** 1383 * Assertion about certainty associated with the propensity, or potential risk, 1384 * of a reaction to the identified substance (including pharmaceutical product). 1385 */ 1386 @Child(name = "verificationStatus", type = { 1387 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 1388 @Description(shortDefinition = "unconfirmed | confirmed | refuted | entered-in-error", formalDefinition = "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).") 1389 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergyintolerance-verification") 1390 protected CodeableConcept verificationStatus; 1391 1392 /** 1393 * Identification of the underlying physiological mechanism for the reaction 1394 * risk. 1395 */ 1396 @Child(name = "type", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1397 @Description(shortDefinition = "allergy | intolerance - Underlying mechanism (if known)", formalDefinition = "Identification of the underlying physiological mechanism for the reaction risk.") 1398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergy-intolerance-type") 1399 protected Enumeration<AllergyIntoleranceType> type; 1400 1401 /** 1402 * Category of the identified substance. 1403 */ 1404 @Child(name = "category", type = { 1405 CodeType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1406 @Description(shortDefinition = "food | medication | environment | biologic", formalDefinition = "Category of the identified substance.") 1407 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergy-intolerance-category") 1408 protected List<Enumeration<AllergyIntoleranceCategory>> category; 1409 1410 /** 1411 * Estimate of the potential clinical harm, or seriousness, of the reaction to 1412 * the identified substance. 1413 */ 1414 @Child(name = "criticality", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1415 @Description(shortDefinition = "low | high | unable-to-assess", formalDefinition = "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.") 1416 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergy-intolerance-criticality") 1417 protected Enumeration<AllergyIntoleranceCriticality> criticality; 1418 1419 /** 1420 * Code for an allergy or intolerance statement (either a positive or a 1421 * negated/excluded statement). This may be a code for a substance or 1422 * pharmaceutical product that is considered to be responsible for the adverse 1423 * reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., 1424 * "Latex allergy"), or a negated/excluded code for a specific substance or 1425 * class (e.g., "No latex allergy") or a general or categorical negated 1426 * statement (e.g., "No known allergy", "No known drug allergies"). Note: the 1427 * substance for a specific reaction may be different from the substance 1428 * identified as the cause of the risk, but it must be consistent with it. For 1429 * instance, it may be a more specific substance (e.g. a brand medication) or a 1430 * composite product that includes the identified substance. It must be 1431 * clinically safe to only process the 'code' and ignore the 1432 * 'reaction.substance'. If a receiving system is unable to confirm that 1433 * AllergyIntolerance.reaction.substance falls within the semantic scope of 1434 * AllergyIntolerance.code, then the receiving system should ignore 1435 * AllergyIntolerance.reaction.substance. 1436 */ 1437 @Child(name = "code", type = { CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1438 @Description(shortDefinition = "Code that identifies the allergy or intolerance", formalDefinition = "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.") 1439 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/allergyintolerance-code") 1440 protected CodeableConcept code; 1441 1442 /** 1443 * The patient who has the allergy or intolerance. 1444 */ 1445 @Child(name = "patient", type = { Patient.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1446 @Description(shortDefinition = "Who the sensitivity is for", formalDefinition = "The patient who has the allergy or intolerance.") 1447 protected Reference patient; 1448 1449 /** 1450 * The actual object that is the target of the reference (The patient who has 1451 * the allergy or intolerance.) 1452 */ 1453 protected Patient patientTarget; 1454 1455 /** 1456 * The encounter when the allergy or intolerance was asserted. 1457 */ 1458 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1459 @Description(shortDefinition = "Encounter when the allergy or intolerance was asserted", formalDefinition = "The encounter when the allergy or intolerance was asserted.") 1460 protected Reference encounter; 1461 1462 /** 1463 * The actual object that is the target of the reference (The encounter when the 1464 * allergy or intolerance was asserted.) 1465 */ 1466 protected Encounter encounterTarget; 1467 1468 /** 1469 * Estimated or actual date, date-time, or age when allergy or intolerance was 1470 * identified. 1471 */ 1472 @Child(name = "onset", type = { DateTimeType.class, Age.class, Period.class, Range.class, 1473 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1474 @Description(shortDefinition = "When allergy or intolerance was identified", formalDefinition = "Estimated or actual date, date-time, or age when allergy or intolerance was identified.") 1475 protected Type onset; 1476 1477 /** 1478 * The recordedDate represents when this particular AllergyIntolerance record 1479 * was created in the system, which is often a system-generated date. 1480 */ 1481 @Child(name = "recordedDate", type = { 1482 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1483 @Description(shortDefinition = "Date first version of the resource instance was recorded", formalDefinition = "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.") 1484 protected DateTimeType recordedDate; 1485 1486 /** 1487 * Individual who recorded the record and takes responsibility for its content. 1488 */ 1489 @Child(name = "recorder", type = { Practitioner.class, PractitionerRole.class, Patient.class, 1490 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1491 @Description(shortDefinition = "Who recorded the sensitivity", formalDefinition = "Individual who recorded the record and takes responsibility for its content.") 1492 protected Reference recorder; 1493 1494 /** 1495 * The actual object that is the target of the reference (Individual who 1496 * recorded the record and takes responsibility for its content.) 1497 */ 1498 protected Resource recorderTarget; 1499 1500 /** 1501 * The source of the information about the allergy that is recorded. 1502 */ 1503 @Child(name = "asserter", type = { Patient.class, RelatedPerson.class, Practitioner.class, 1504 PractitionerRole.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1505 @Description(shortDefinition = "Source of the information about the allergy", formalDefinition = "The source of the information about the allergy that is recorded.") 1506 protected Reference asserter; 1507 1508 /** 1509 * The actual object that is the target of the reference (The source of the 1510 * information about the allergy that is recorded.) 1511 */ 1512 protected Resource asserterTarget; 1513 1514 /** 1515 * Represents the date and/or time of the last known occurrence of a reaction 1516 * event. 1517 */ 1518 @Child(name = "lastOccurrence", type = { 1519 DateTimeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1520 @Description(shortDefinition = "Date(/time) of last known occurrence of a reaction", formalDefinition = "Represents the date and/or time of the last known occurrence of a reaction event.") 1521 protected DateTimeType lastOccurrence; 1522 1523 /** 1524 * Additional narrative about the propensity for the Adverse Reaction, not 1525 * captured in other fields. 1526 */ 1527 @Child(name = "note", type = { 1528 Annotation.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1529 @Description(shortDefinition = "Additional text not captured in other fields", formalDefinition = "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.") 1530 protected List<Annotation> note; 1531 1532 /** 1533 * Details about each adverse reaction event linked to exposure to the 1534 * identified substance. 1535 */ 1536 @Child(name = "reaction", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1537 @Description(shortDefinition = "Adverse Reaction Events linked to exposure to substance", formalDefinition = "Details about each adverse reaction event linked to exposure to the identified substance.") 1538 protected List<AllergyIntoleranceReactionComponent> reaction; 1539 1540 private static final long serialVersionUID = 393192289L; 1541 1542 /** 1543 * Constructor 1544 */ 1545 public AllergyIntolerance() { 1546 super(); 1547 } 1548 1549 /** 1550 * Constructor 1551 */ 1552 public AllergyIntolerance(Reference patient) { 1553 super(); 1554 this.patient = patient; 1555 } 1556 1557 /** 1558 * @return {@link #identifier} (Business identifiers assigned to this 1559 * AllergyIntolerance by the performer or other systems which remain 1560 * constant as the resource is updated and propagates from server to 1561 * server.) 1562 */ 1563 public List<Identifier> getIdentifier() { 1564 if (this.identifier == null) 1565 this.identifier = new ArrayList<Identifier>(); 1566 return this.identifier; 1567 } 1568 1569 /** 1570 * @return Returns a reference to <code>this</code> for easy method chaining 1571 */ 1572 public AllergyIntolerance setIdentifier(List<Identifier> theIdentifier) { 1573 this.identifier = theIdentifier; 1574 return this; 1575 } 1576 1577 public boolean hasIdentifier() { 1578 if (this.identifier == null) 1579 return false; 1580 for (Identifier item : this.identifier) 1581 if (!item.isEmpty()) 1582 return true; 1583 return false; 1584 } 1585 1586 public Identifier addIdentifier() { // 3 1587 Identifier t = new Identifier(); 1588 if (this.identifier == null) 1589 this.identifier = new ArrayList<Identifier>(); 1590 this.identifier.add(t); 1591 return t; 1592 } 1593 1594 public AllergyIntolerance addIdentifier(Identifier t) { // 3 1595 if (t == null) 1596 return this; 1597 if (this.identifier == null) 1598 this.identifier = new ArrayList<Identifier>(); 1599 this.identifier.add(t); 1600 return this; 1601 } 1602 1603 /** 1604 * @return The first repetition of repeating field {@link #identifier}, creating 1605 * it if it does not already exist 1606 */ 1607 public Identifier getIdentifierFirstRep() { 1608 if (getIdentifier().isEmpty()) { 1609 addIdentifier(); 1610 } 1611 return getIdentifier().get(0); 1612 } 1613 1614 /** 1615 * @return {@link #clinicalStatus} (The clinical status of the allergy or 1616 * intolerance.) 1617 */ 1618 public CodeableConcept getClinicalStatus() { 1619 if (this.clinicalStatus == null) 1620 if (Configuration.errorOnAutoCreate()) 1621 throw new Error("Attempt to auto-create AllergyIntolerance.clinicalStatus"); 1622 else if (Configuration.doAutoCreate()) 1623 this.clinicalStatus = new CodeableConcept(); // cc 1624 return this.clinicalStatus; 1625 } 1626 1627 public boolean hasClinicalStatus() { 1628 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1629 } 1630 1631 /** 1632 * @param value {@link #clinicalStatus} (The clinical status of the allergy or 1633 * intolerance.) 1634 */ 1635 public AllergyIntolerance setClinicalStatus(CodeableConcept value) { 1636 this.clinicalStatus = value; 1637 return this; 1638 } 1639 1640 /** 1641 * @return {@link #verificationStatus} (Assertion about certainty associated 1642 * with the propensity, or potential risk, of a reaction to the 1643 * identified substance (including pharmaceutical product).) 1644 */ 1645 public CodeableConcept getVerificationStatus() { 1646 if (this.verificationStatus == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create AllergyIntolerance.verificationStatus"); 1649 else if (Configuration.doAutoCreate()) 1650 this.verificationStatus = new CodeableConcept(); // cc 1651 return this.verificationStatus; 1652 } 1653 1654 public boolean hasVerificationStatus() { 1655 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #verificationStatus} (Assertion about certainty 1660 * associated with the propensity, or potential risk, of a reaction 1661 * to the identified substance (including pharmaceutical product).) 1662 */ 1663 public AllergyIntolerance setVerificationStatus(CodeableConcept value) { 1664 this.verificationStatus = value; 1665 return this; 1666 } 1667 1668 /** 1669 * @return {@link #type} (Identification of the underlying physiological 1670 * mechanism for the reaction risk.). This is the underlying object with 1671 * id, value and extensions. The accessor "getType" gives direct access 1672 * to the value 1673 */ 1674 public Enumeration<AllergyIntoleranceType> getTypeElement() { 1675 if (this.type == null) 1676 if (Configuration.errorOnAutoCreate()) 1677 throw new Error("Attempt to auto-create AllergyIntolerance.type"); 1678 else if (Configuration.doAutoCreate()) 1679 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); // bb 1680 return this.type; 1681 } 1682 1683 public boolean hasTypeElement() { 1684 return this.type != null && !this.type.isEmpty(); 1685 } 1686 1687 public boolean hasType() { 1688 return this.type != null && !this.type.isEmpty(); 1689 } 1690 1691 /** 1692 * @param value {@link #type} (Identification of the underlying physiological 1693 * mechanism for the reaction risk.). This is the underlying object 1694 * with id, value and extensions. The accessor "getType" gives 1695 * direct access to the value 1696 */ 1697 public AllergyIntolerance setTypeElement(Enumeration<AllergyIntoleranceType> value) { 1698 this.type = value; 1699 return this; 1700 } 1701 1702 /** 1703 * @return Identification of the underlying physiological mechanism for the 1704 * reaction risk. 1705 */ 1706 public AllergyIntoleranceType getType() { 1707 return this.type == null ? null : this.type.getValue(); 1708 } 1709 1710 /** 1711 * @param value Identification of the underlying physiological mechanism for the 1712 * reaction risk. 1713 */ 1714 public AllergyIntolerance setType(AllergyIntoleranceType value) { 1715 if (value == null) 1716 this.type = null; 1717 else { 1718 if (this.type == null) 1719 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); 1720 this.type.setValue(value); 1721 } 1722 return this; 1723 } 1724 1725 /** 1726 * @return {@link #category} (Category of the identified substance.) 1727 */ 1728 public List<Enumeration<AllergyIntoleranceCategory>> getCategory() { 1729 if (this.category == null) 1730 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1731 return this.category; 1732 } 1733 1734 /** 1735 * @return Returns a reference to <code>this</code> for easy method chaining 1736 */ 1737 public AllergyIntolerance setCategory(List<Enumeration<AllergyIntoleranceCategory>> theCategory) { 1738 this.category = theCategory; 1739 return this; 1740 } 1741 1742 public boolean hasCategory() { 1743 if (this.category == null) 1744 return false; 1745 for (Enumeration<AllergyIntoleranceCategory> item : this.category) 1746 if (!item.isEmpty()) 1747 return true; 1748 return false; 1749 } 1750 1751 /** 1752 * @return {@link #category} (Category of the identified substance.) 1753 */ 1754 public Enumeration<AllergyIntoleranceCategory> addCategoryElement() {// 2 1755 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>( 1756 new AllergyIntoleranceCategoryEnumFactory()); 1757 if (this.category == null) 1758 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1759 this.category.add(t); 1760 return t; 1761 } 1762 1763 /** 1764 * @param value {@link #category} (Category of the identified substance.) 1765 */ 1766 public AllergyIntolerance addCategory(AllergyIntoleranceCategory value) { // 1 1767 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>( 1768 new AllergyIntoleranceCategoryEnumFactory()); 1769 t.setValue(value); 1770 if (this.category == null) 1771 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1772 this.category.add(t); 1773 return this; 1774 } 1775 1776 /** 1777 * @param value {@link #category} (Category of the identified substance.) 1778 */ 1779 public boolean hasCategory(AllergyIntoleranceCategory value) { 1780 if (this.category == null) 1781 return false; 1782 for (Enumeration<AllergyIntoleranceCategory> v : this.category) 1783 if (v.getValue().equals(value)) // code 1784 return true; 1785 return false; 1786 } 1787 1788 /** 1789 * @return {@link #criticality} (Estimate of the potential clinical harm, or 1790 * seriousness, of the reaction to the identified substance.). This is 1791 * the underlying object with id, value and extensions. The accessor 1792 * "getCriticality" gives direct access to the value 1793 */ 1794 public Enumeration<AllergyIntoleranceCriticality> getCriticalityElement() { 1795 if (this.criticality == null) 1796 if (Configuration.errorOnAutoCreate()) 1797 throw new Error("Attempt to auto-create AllergyIntolerance.criticality"); 1798 else if (Configuration.doAutoCreate()) 1799 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 1800 new AllergyIntoleranceCriticalityEnumFactory()); // bb 1801 return this.criticality; 1802 } 1803 1804 public boolean hasCriticalityElement() { 1805 return this.criticality != null && !this.criticality.isEmpty(); 1806 } 1807 1808 public boolean hasCriticality() { 1809 return this.criticality != null && !this.criticality.isEmpty(); 1810 } 1811 1812 /** 1813 * @param value {@link #criticality} (Estimate of the potential clinical harm, 1814 * or seriousness, of the reaction to the identified substance.). 1815 * This is the underlying object with id, value and extensions. The 1816 * accessor "getCriticality" gives direct access to the value 1817 */ 1818 public AllergyIntolerance setCriticalityElement(Enumeration<AllergyIntoleranceCriticality> value) { 1819 this.criticality = value; 1820 return this; 1821 } 1822 1823 /** 1824 * @return Estimate of the potential clinical harm, or seriousness, of the 1825 * reaction to the identified substance. 1826 */ 1827 public AllergyIntoleranceCriticality getCriticality() { 1828 return this.criticality == null ? null : this.criticality.getValue(); 1829 } 1830 1831 /** 1832 * @param value Estimate of the potential clinical harm, or seriousness, of the 1833 * reaction to the identified substance. 1834 */ 1835 public AllergyIntolerance setCriticality(AllergyIntoleranceCriticality value) { 1836 if (value == null) 1837 this.criticality = null; 1838 else { 1839 if (this.criticality == null) 1840 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 1841 new AllergyIntoleranceCriticalityEnumFactory()); 1842 this.criticality.setValue(value); 1843 } 1844 return this; 1845 } 1846 1847 /** 1848 * @return {@link #code} (Code for an allergy or intolerance statement (either a 1849 * positive or a negated/excluded statement). This may be a code for a 1850 * substance or pharmaceutical product that is considered to be 1851 * responsible for the adverse reaction risk (e.g., "Latex"), an allergy 1852 * or intolerance condition (e.g., "Latex allergy"), or a 1853 * negated/excluded code for a specific substance or class (e.g., "No 1854 * latex allergy") or a general or categorical negated statement (e.g., 1855 * "No known allergy", "No known drug allergies"). Note: the substance 1856 * for a specific reaction may be different from the substance 1857 * identified as the cause of the risk, but it must be consistent with 1858 * it. For instance, it may be a more specific substance (e.g. a brand 1859 * medication) or a composite product that includes the identified 1860 * substance. It must be clinically safe to only process the 'code' and 1861 * ignore the 'reaction.substance'. If a receiving system is unable to 1862 * confirm that AllergyIntolerance.reaction.substance falls within the 1863 * semantic scope of AllergyIntolerance.code, then the receiving system 1864 * should ignore AllergyIntolerance.reaction.substance.) 1865 */ 1866 public CodeableConcept getCode() { 1867 if (this.code == null) 1868 if (Configuration.errorOnAutoCreate()) 1869 throw new Error("Attempt to auto-create AllergyIntolerance.code"); 1870 else if (Configuration.doAutoCreate()) 1871 this.code = new CodeableConcept(); // cc 1872 return this.code; 1873 } 1874 1875 public boolean hasCode() { 1876 return this.code != null && !this.code.isEmpty(); 1877 } 1878 1879 /** 1880 * @param value {@link #code} (Code for an allergy or intolerance statement 1881 * (either a positive or a negated/excluded statement). This may be 1882 * a code for a substance or pharmaceutical product that is 1883 * considered to be responsible for the adverse reaction risk 1884 * (e.g., "Latex"), an allergy or intolerance condition (e.g., 1885 * "Latex allergy"), or a negated/excluded code for a specific 1886 * substance or class (e.g., "No latex allergy") or a general or 1887 * categorical negated statement (e.g., "No known allergy", "No 1888 * known drug allergies"). Note: the substance for a specific 1889 * reaction may be different from the substance identified as the 1890 * cause of the risk, but it must be consistent with it. For 1891 * instance, it may be a more specific substance (e.g. a brand 1892 * medication) or a composite product that includes the identified 1893 * substance. It must be clinically safe to only process the 'code' 1894 * and ignore the 'reaction.substance'. If a receiving system is 1895 * unable to confirm that AllergyIntolerance.reaction.substance 1896 * falls within the semantic scope of AllergyIntolerance.code, then 1897 * the receiving system should ignore 1898 * AllergyIntolerance.reaction.substance.) 1899 */ 1900 public AllergyIntolerance setCode(CodeableConcept value) { 1901 this.code = value; 1902 return this; 1903 } 1904 1905 /** 1906 * @return {@link #patient} (The patient who has the allergy or intolerance.) 1907 */ 1908 public Reference getPatient() { 1909 if (this.patient == null) 1910 if (Configuration.errorOnAutoCreate()) 1911 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1912 else if (Configuration.doAutoCreate()) 1913 this.patient = new Reference(); // cc 1914 return this.patient; 1915 } 1916 1917 public boolean hasPatient() { 1918 return this.patient != null && !this.patient.isEmpty(); 1919 } 1920 1921 /** 1922 * @param value {@link #patient} (The patient who has the allergy or 1923 * intolerance.) 1924 */ 1925 public AllergyIntolerance setPatient(Reference value) { 1926 this.patient = value; 1927 return this; 1928 } 1929 1930 /** 1931 * @return {@link #patient} The actual object that is the target of the 1932 * reference. The reference library doesn't populate this, but you can 1933 * use it to hold the resource if you resolve it. (The patient who has 1934 * the allergy or intolerance.) 1935 */ 1936 public Patient getPatientTarget() { 1937 if (this.patientTarget == null) 1938 if (Configuration.errorOnAutoCreate()) 1939 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1940 else if (Configuration.doAutoCreate()) 1941 this.patientTarget = new Patient(); // aa 1942 return this.patientTarget; 1943 } 1944 1945 /** 1946 * @param value {@link #patient} The actual object that is the target of the 1947 * reference. The reference library doesn't use these, but you can 1948 * use it to hold the resource if you resolve it. (The patient who 1949 * has the allergy or intolerance.) 1950 */ 1951 public AllergyIntolerance setPatientTarget(Patient value) { 1952 this.patientTarget = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return {@link #encounter} (The encounter when the allergy or intolerance was 1958 * asserted.) 1959 */ 1960 public Reference getEncounter() { 1961 if (this.encounter == null) 1962 if (Configuration.errorOnAutoCreate()) 1963 throw new Error("Attempt to auto-create AllergyIntolerance.encounter"); 1964 else if (Configuration.doAutoCreate()) 1965 this.encounter = new Reference(); // cc 1966 return this.encounter; 1967 } 1968 1969 public boolean hasEncounter() { 1970 return this.encounter != null && !this.encounter.isEmpty(); 1971 } 1972 1973 /** 1974 * @param value {@link #encounter} (The encounter when the allergy or 1975 * intolerance was asserted.) 1976 */ 1977 public AllergyIntolerance setEncounter(Reference value) { 1978 this.encounter = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return {@link #encounter} The actual object that is the target of the 1984 * reference. The reference library doesn't populate this, but you can 1985 * use it to hold the resource if you resolve it. (The encounter when 1986 * the allergy or intolerance was asserted.) 1987 */ 1988 public Encounter getEncounterTarget() { 1989 if (this.encounterTarget == null) 1990 if (Configuration.errorOnAutoCreate()) 1991 throw new Error("Attempt to auto-create AllergyIntolerance.encounter"); 1992 else if (Configuration.doAutoCreate()) 1993 this.encounterTarget = new Encounter(); // aa 1994 return this.encounterTarget; 1995 } 1996 1997 /** 1998 * @param value {@link #encounter} The actual object that is the target of the 1999 * reference. The reference library doesn't use these, but you can 2000 * use it to hold the resource if you resolve it. (The encounter 2001 * when the allergy or intolerance was asserted.) 2002 */ 2003 public AllergyIntolerance setEncounterTarget(Encounter value) { 2004 this.encounterTarget = value; 2005 return this; 2006 } 2007 2008 /** 2009 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2010 * allergy or intolerance was identified.) 2011 */ 2012 public Type getOnset() { 2013 return this.onset; 2014 } 2015 2016 /** 2017 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2018 * allergy or intolerance was identified.) 2019 */ 2020 public DateTimeType getOnsetDateTimeType() throws FHIRException { 2021 if (this.onset == null) 2022 this.onset = new DateTimeType(); 2023 if (!(this.onset instanceof DateTimeType)) 2024 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2025 + this.onset.getClass().getName() + " was encountered"); 2026 return (DateTimeType) this.onset; 2027 } 2028 2029 public boolean hasOnsetDateTimeType() { 2030 return this != null && this.onset instanceof DateTimeType; 2031 } 2032 2033 /** 2034 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2035 * allergy or intolerance was identified.) 2036 */ 2037 public Age getOnsetAge() throws FHIRException { 2038 if (this.onset == null) 2039 this.onset = new Age(); 2040 if (!(this.onset instanceof Age)) 2041 throw new FHIRException( 2042 "Type mismatch: the type Age was expected, but " + this.onset.getClass().getName() + " was encountered"); 2043 return (Age) this.onset; 2044 } 2045 2046 public boolean hasOnsetAge() { 2047 return this != null && this.onset instanceof Age; 2048 } 2049 2050 /** 2051 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2052 * allergy or intolerance was identified.) 2053 */ 2054 public Period getOnsetPeriod() throws FHIRException { 2055 if (this.onset == null) 2056 this.onset = new Period(); 2057 if (!(this.onset instanceof Period)) 2058 throw new FHIRException( 2059 "Type mismatch: the type Period was expected, but " + this.onset.getClass().getName() + " was encountered"); 2060 return (Period) this.onset; 2061 } 2062 2063 public boolean hasOnsetPeriod() { 2064 return this != null && this.onset instanceof Period; 2065 } 2066 2067 /** 2068 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2069 * allergy or intolerance was identified.) 2070 */ 2071 public Range getOnsetRange() throws FHIRException { 2072 if (this.onset == null) 2073 this.onset = new Range(); 2074 if (!(this.onset instanceof Range)) 2075 throw new FHIRException( 2076 "Type mismatch: the type Range was expected, but " + this.onset.getClass().getName() + " was encountered"); 2077 return (Range) this.onset; 2078 } 2079 2080 public boolean hasOnsetRange() { 2081 return this != null && this.onset instanceof Range; 2082 } 2083 2084 /** 2085 * @return {@link #onset} (Estimated or actual date, date-time, or age when 2086 * allergy or intolerance was identified.) 2087 */ 2088 public StringType getOnsetStringType() throws FHIRException { 2089 if (this.onset == null) 2090 this.onset = new StringType(); 2091 if (!(this.onset instanceof StringType)) 2092 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.onset.getClass().getName() 2093 + " was encountered"); 2094 return (StringType) this.onset; 2095 } 2096 2097 public boolean hasOnsetStringType() { 2098 return this != null && this.onset instanceof StringType; 2099 } 2100 2101 public boolean hasOnset() { 2102 return this.onset != null && !this.onset.isEmpty(); 2103 } 2104 2105 /** 2106 * @param value {@link #onset} (Estimated or actual date, date-time, or age when 2107 * allergy or intolerance was identified.) 2108 */ 2109 public AllergyIntolerance setOnset(Type value) { 2110 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 2111 || value instanceof Range || value instanceof StringType)) 2112 throw new Error("Not the right type for AllergyIntolerance.onset[x]: " + value.fhirType()); 2113 this.onset = value; 2114 return this; 2115 } 2116 2117 /** 2118 * @return {@link #recordedDate} (The recordedDate represents when this 2119 * particular AllergyIntolerance record was created in the system, which 2120 * is often a system-generated date.). This is the underlying object 2121 * with id, value and extensions. The accessor "getRecordedDate" gives 2122 * direct access to the value 2123 */ 2124 public DateTimeType getRecordedDateElement() { 2125 if (this.recordedDate == null) 2126 if (Configuration.errorOnAutoCreate()) 2127 throw new Error("Attempt to auto-create AllergyIntolerance.recordedDate"); 2128 else if (Configuration.doAutoCreate()) 2129 this.recordedDate = new DateTimeType(); // bb 2130 return this.recordedDate; 2131 } 2132 2133 public boolean hasRecordedDateElement() { 2134 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2135 } 2136 2137 public boolean hasRecordedDate() { 2138 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2139 } 2140 2141 /** 2142 * @param value {@link #recordedDate} (The recordedDate represents when this 2143 * particular AllergyIntolerance record was created in the system, 2144 * which is often a system-generated date.). This is the underlying 2145 * object with id, value and extensions. The accessor 2146 * "getRecordedDate" gives direct access to the value 2147 */ 2148 public AllergyIntolerance setRecordedDateElement(DateTimeType value) { 2149 this.recordedDate = value; 2150 return this; 2151 } 2152 2153 /** 2154 * @return The recordedDate represents when this particular AllergyIntolerance 2155 * record was created in the system, which is often a system-generated 2156 * date. 2157 */ 2158 public Date getRecordedDate() { 2159 return this.recordedDate == null ? null : this.recordedDate.getValue(); 2160 } 2161 2162 /** 2163 * @param value The recordedDate represents when this particular 2164 * AllergyIntolerance record was created in the system, which is 2165 * often a system-generated date. 2166 */ 2167 public AllergyIntolerance setRecordedDate(Date value) { 2168 if (value == null) 2169 this.recordedDate = null; 2170 else { 2171 if (this.recordedDate == null) 2172 this.recordedDate = new DateTimeType(); 2173 this.recordedDate.setValue(value); 2174 } 2175 return this; 2176 } 2177 2178 /** 2179 * @return {@link #recorder} (Individual who recorded the record and takes 2180 * responsibility for its content.) 2181 */ 2182 public Reference getRecorder() { 2183 if (this.recorder == null) 2184 if (Configuration.errorOnAutoCreate()) 2185 throw new Error("Attempt to auto-create AllergyIntolerance.recorder"); 2186 else if (Configuration.doAutoCreate()) 2187 this.recorder = new Reference(); // cc 2188 return this.recorder; 2189 } 2190 2191 public boolean hasRecorder() { 2192 return this.recorder != null && !this.recorder.isEmpty(); 2193 } 2194 2195 /** 2196 * @param value {@link #recorder} (Individual who recorded the record and takes 2197 * responsibility for its content.) 2198 */ 2199 public AllergyIntolerance setRecorder(Reference value) { 2200 this.recorder = value; 2201 return this; 2202 } 2203 2204 /** 2205 * @return {@link #recorder} The actual object that is the target of the 2206 * reference. The reference library doesn't populate this, but you can 2207 * use it to hold the resource if you resolve it. (Individual who 2208 * recorded the record and takes responsibility for its content.) 2209 */ 2210 public Resource getRecorderTarget() { 2211 return this.recorderTarget; 2212 } 2213 2214 /** 2215 * @param value {@link #recorder} The actual object that is the target of the 2216 * reference. The reference library doesn't use these, but you can 2217 * use it to hold the resource if you resolve it. (Individual who 2218 * recorded the record and takes responsibility for its content.) 2219 */ 2220 public AllergyIntolerance setRecorderTarget(Resource value) { 2221 this.recorderTarget = value; 2222 return this; 2223 } 2224 2225 /** 2226 * @return {@link #asserter} (The source of the information about the allergy 2227 * that is recorded.) 2228 */ 2229 public Reference getAsserter() { 2230 if (this.asserter == null) 2231 if (Configuration.errorOnAutoCreate()) 2232 throw new Error("Attempt to auto-create AllergyIntolerance.asserter"); 2233 else if (Configuration.doAutoCreate()) 2234 this.asserter = new Reference(); // cc 2235 return this.asserter; 2236 } 2237 2238 public boolean hasAsserter() { 2239 return this.asserter != null && !this.asserter.isEmpty(); 2240 } 2241 2242 /** 2243 * @param value {@link #asserter} (The source of the information about the 2244 * allergy that is recorded.) 2245 */ 2246 public AllergyIntolerance setAsserter(Reference value) { 2247 this.asserter = value; 2248 return this; 2249 } 2250 2251 /** 2252 * @return {@link #asserter} The actual object that is the target of the 2253 * reference. The reference library doesn't populate this, but you can 2254 * use it to hold the resource if you resolve it. (The source of the 2255 * information about the allergy that is recorded.) 2256 */ 2257 public Resource getAsserterTarget() { 2258 return this.asserterTarget; 2259 } 2260 2261 /** 2262 * @param value {@link #asserter} The actual object that is the target of the 2263 * reference. The reference library doesn't use these, but you can 2264 * use it to hold the resource if you resolve it. (The source of 2265 * the information about the allergy that is recorded.) 2266 */ 2267 public AllergyIntolerance setAsserterTarget(Resource value) { 2268 this.asserterTarget = value; 2269 return this; 2270 } 2271 2272 /** 2273 * @return {@link #lastOccurrence} (Represents the date and/or time of the last 2274 * known occurrence of a reaction event.). This is the underlying object 2275 * with id, value and extensions. The accessor "getLastOccurrence" gives 2276 * direct access to the value 2277 */ 2278 public DateTimeType getLastOccurrenceElement() { 2279 if (this.lastOccurrence == null) 2280 if (Configuration.errorOnAutoCreate()) 2281 throw new Error("Attempt to auto-create AllergyIntolerance.lastOccurrence"); 2282 else if (Configuration.doAutoCreate()) 2283 this.lastOccurrence = new DateTimeType(); // bb 2284 return this.lastOccurrence; 2285 } 2286 2287 public boolean hasLastOccurrenceElement() { 2288 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 2289 } 2290 2291 public boolean hasLastOccurrence() { 2292 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 2293 } 2294 2295 /** 2296 * @param value {@link #lastOccurrence} (Represents the date and/or time of the 2297 * last known occurrence of a reaction event.). This is the 2298 * underlying object with id, value and extensions. The accessor 2299 * "getLastOccurrence" gives direct access to the value 2300 */ 2301 public AllergyIntolerance setLastOccurrenceElement(DateTimeType value) { 2302 this.lastOccurrence = value; 2303 return this; 2304 } 2305 2306 /** 2307 * @return Represents the date and/or time of the last known occurrence of a 2308 * reaction event. 2309 */ 2310 public Date getLastOccurrence() { 2311 return this.lastOccurrence == null ? null : this.lastOccurrence.getValue(); 2312 } 2313 2314 /** 2315 * @param value Represents the date and/or time of the last known occurrence of 2316 * a reaction event. 2317 */ 2318 public AllergyIntolerance setLastOccurrence(Date value) { 2319 if (value == null) 2320 this.lastOccurrence = null; 2321 else { 2322 if (this.lastOccurrence == null) 2323 this.lastOccurrence = new DateTimeType(); 2324 this.lastOccurrence.setValue(value); 2325 } 2326 return this; 2327 } 2328 2329 /** 2330 * @return {@link #note} (Additional narrative about the propensity for the 2331 * Adverse Reaction, not captured in other fields.) 2332 */ 2333 public List<Annotation> getNote() { 2334 if (this.note == null) 2335 this.note = new ArrayList<Annotation>(); 2336 return this.note; 2337 } 2338 2339 /** 2340 * @return Returns a reference to <code>this</code> for easy method chaining 2341 */ 2342 public AllergyIntolerance setNote(List<Annotation> theNote) { 2343 this.note = theNote; 2344 return this; 2345 } 2346 2347 public boolean hasNote() { 2348 if (this.note == null) 2349 return false; 2350 for (Annotation item : this.note) 2351 if (!item.isEmpty()) 2352 return true; 2353 return false; 2354 } 2355 2356 public Annotation addNote() { // 3 2357 Annotation t = new Annotation(); 2358 if (this.note == null) 2359 this.note = new ArrayList<Annotation>(); 2360 this.note.add(t); 2361 return t; 2362 } 2363 2364 public AllergyIntolerance addNote(Annotation t) { // 3 2365 if (t == null) 2366 return this; 2367 if (this.note == null) 2368 this.note = new ArrayList<Annotation>(); 2369 this.note.add(t); 2370 return this; 2371 } 2372 2373 /** 2374 * @return The first repetition of repeating field {@link #note}, creating it if 2375 * it does not already exist 2376 */ 2377 public Annotation getNoteFirstRep() { 2378 if (getNote().isEmpty()) { 2379 addNote(); 2380 } 2381 return getNote().get(0); 2382 } 2383 2384 /** 2385 * @return {@link #reaction} (Details about each adverse reaction event linked 2386 * to exposure to the identified substance.) 2387 */ 2388 public List<AllergyIntoleranceReactionComponent> getReaction() { 2389 if (this.reaction == null) 2390 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2391 return this.reaction; 2392 } 2393 2394 /** 2395 * @return Returns a reference to <code>this</code> for easy method chaining 2396 */ 2397 public AllergyIntolerance setReaction(List<AllergyIntoleranceReactionComponent> theReaction) { 2398 this.reaction = theReaction; 2399 return this; 2400 } 2401 2402 public boolean hasReaction() { 2403 if (this.reaction == null) 2404 return false; 2405 for (AllergyIntoleranceReactionComponent item : this.reaction) 2406 if (!item.isEmpty()) 2407 return true; 2408 return false; 2409 } 2410 2411 public AllergyIntoleranceReactionComponent addReaction() { // 3 2412 AllergyIntoleranceReactionComponent t = new AllergyIntoleranceReactionComponent(); 2413 if (this.reaction == null) 2414 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2415 this.reaction.add(t); 2416 return t; 2417 } 2418 2419 public AllergyIntolerance addReaction(AllergyIntoleranceReactionComponent t) { // 3 2420 if (t == null) 2421 return this; 2422 if (this.reaction == null) 2423 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2424 this.reaction.add(t); 2425 return this; 2426 } 2427 2428 /** 2429 * @return The first repetition of repeating field {@link #reaction}, creating 2430 * it if it does not already exist 2431 */ 2432 public AllergyIntoleranceReactionComponent getReactionFirstRep() { 2433 if (getReaction().isEmpty()) { 2434 addReaction(); 2435 } 2436 return getReaction().get(0); 2437 } 2438 2439 protected void listChildren(List<Property> children) { 2440 super.listChildren(children); 2441 children.add(new Property("identifier", "Identifier", 2442 "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2443 0, java.lang.Integer.MAX_VALUE, identifier)); 2444 children.add(new Property("clinicalStatus", "CodeableConcept", "The clinical status of the allergy or intolerance.", 2445 0, 1, clinicalStatus)); 2446 children.add(new Property("verificationStatus", "CodeableConcept", 2447 "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).", 2448 0, 1, verificationStatus)); 2449 children.add(new Property("type", "code", 2450 "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type)); 2451 children.add(new Property("category", "code", "Category of the identified substance.", 0, 2452 java.lang.Integer.MAX_VALUE, category)); 2453 children.add(new Property("criticality", "code", 2454 "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, 2455 criticality)); 2456 children.add(new Property("code", "CodeableConcept", 2457 "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 2458 0, 1, code)); 2459 children.add(new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 1, 2460 patient)); 2461 children.add(new Property("encounter", "Reference(Encounter)", 2462 "The encounter when the allergy or intolerance was asserted.", 0, 1, encounter)); 2463 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", 2464 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset)); 2465 children.add(new Property("recordedDate", "dateTime", 2466 "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.", 2467 0, 1, recordedDate)); 2468 children.add(new Property("recorder", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 2469 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder)); 2470 children.add(new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 2471 "The source of the information about the allergy that is recorded.", 0, 1, asserter)); 2472 children.add(new Property("lastOccurrence", "dateTime", 2473 "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence)); 2474 children.add(new Property("note", "Annotation", 2475 "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, 2476 java.lang.Integer.MAX_VALUE, note)); 2477 children.add(new Property("reaction", "", 2478 "Details about each adverse reaction event linked to exposure to the identified substance.", 0, 2479 java.lang.Integer.MAX_VALUE, reaction)); 2480 } 2481 2482 @Override 2483 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2484 switch (_hash) { 2485 case -1618432855: 2486 /* identifier */ return new Property("identifier", "Identifier", 2487 "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2488 0, java.lang.Integer.MAX_VALUE, identifier); 2489 case -462853915: 2490 /* clinicalStatus */ return new Property("clinicalStatus", "CodeableConcept", 2491 "The clinical status of the allergy or intolerance.", 0, 1, clinicalStatus); 2492 case -842509843: 2493 /* verificationStatus */ return new Property("verificationStatus", "CodeableConcept", 2494 "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product).", 2495 0, 1, verificationStatus); 2496 case 3575610: 2497 /* type */ return new Property("type", "code", 2498 "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type); 2499 case 50511102: 2500 /* category */ return new Property("category", "code", "Category of the identified substance.", 0, 2501 java.lang.Integer.MAX_VALUE, category); 2502 case -1608054609: 2503 /* criticality */ return new Property("criticality", "code", 2504 "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, 2505 criticality); 2506 case 3059181: 2507 /* code */ return new Property("code", "CodeableConcept", 2508 "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 2509 0, 1, code); 2510 case -791418107: 2511 /* patient */ return new Property("patient", "Reference(Patient)", 2512 "The patient who has the allergy or intolerance.", 0, 1, patient); 2513 case 1524132147: 2514 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2515 "The encounter when the allergy or intolerance was asserted.", 0, 1, encounter); 2516 case -1886216323: 2517 /* onset[x] */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2518 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2519 case 105901603: 2520 /* onset */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2521 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2522 case -1701663010: 2523 /* onsetDateTime */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2524 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2525 case -1886241828: 2526 /* onsetAge */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2527 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2528 case -1545082428: 2529 /* onsetPeriod */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2530 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2531 case -186664742: 2532 /* onsetRange */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2533 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2534 case -1445342188: 2535 /* onsetString */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 2536 "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2537 case -1952893826: 2538 /* recordedDate */ return new Property("recordedDate", "dateTime", 2539 "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.", 2540 0, 1, recordedDate); 2541 case -799233858: 2542 /* recorder */ return new Property("recorder", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 2543 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder); 2544 case -373242253: 2545 /* asserter */ return new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 2546 "The source of the information about the allergy that is recorded.", 0, 1, asserter); 2547 case 1896977671: 2548 /* lastOccurrence */ return new Property("lastOccurrence", "dateTime", 2549 "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence); 2550 case 3387378: 2551 /* note */ return new Property("note", "Annotation", 2552 "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, 2553 java.lang.Integer.MAX_VALUE, note); 2554 case -867509719: 2555 /* reaction */ return new Property("reaction", "", 2556 "Details about each adverse reaction event linked to exposure to the identified substance.", 0, 2557 java.lang.Integer.MAX_VALUE, reaction); 2558 default: 2559 return super.getNamedProperty(_hash, _name, _checkValid); 2560 } 2561 2562 } 2563 2564 @Override 2565 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2566 switch (hash) { 2567 case -1618432855: 2568 /* identifier */ return this.identifier == null ? new Base[0] 2569 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2570 case -462853915: 2571 /* clinicalStatus */ return this.clinicalStatus == null ? new Base[0] : new Base[] { this.clinicalStatus }; // CodeableConcept 2572 case -842509843: 2573 /* verificationStatus */ return this.verificationStatus == null ? new Base[0] 2574 : new Base[] { this.verificationStatus }; // CodeableConcept 2575 case 3575610: 2576 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<AllergyIntoleranceType> 2577 case 50511102: 2578 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // Enumeration<AllergyIntoleranceCategory> 2579 case -1608054609: 2580 /* criticality */ return this.criticality == null ? new Base[0] : new Base[] { this.criticality }; // Enumeration<AllergyIntoleranceCriticality> 2581 case 3059181: 2582 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2583 case -791418107: 2584 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2585 case 1524132147: 2586 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2587 case 105901603: 2588 /* onset */ return this.onset == null ? new Base[0] : new Base[] { this.onset }; // Type 2589 case -1952893826: 2590 /* recordedDate */ return this.recordedDate == null ? new Base[0] : new Base[] { this.recordedDate }; // DateTimeType 2591 case -799233858: 2592 /* recorder */ return this.recorder == null ? new Base[0] : new Base[] { this.recorder }; // Reference 2593 case -373242253: 2594 /* asserter */ return this.asserter == null ? new Base[0] : new Base[] { this.asserter }; // Reference 2595 case 1896977671: 2596 /* lastOccurrence */ return this.lastOccurrence == null ? new Base[0] : new Base[] { this.lastOccurrence }; // DateTimeType 2597 case 3387378: 2598 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2599 case -867509719: 2600 /* reaction */ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // AllergyIntoleranceReactionComponent 2601 default: 2602 return super.getProperty(hash, name, checkValid); 2603 } 2604 2605 } 2606 2607 @Override 2608 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2609 switch (hash) { 2610 case -1618432855: // identifier 2611 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2612 return value; 2613 case -462853915: // clinicalStatus 2614 this.clinicalStatus = castToCodeableConcept(value); // CodeableConcept 2615 return value; 2616 case -842509843: // verificationStatus 2617 this.verificationStatus = castToCodeableConcept(value); // CodeableConcept 2618 return value; 2619 case 3575610: // type 2620 value = new AllergyIntoleranceTypeEnumFactory().fromType(castToCode(value)); 2621 this.type = (Enumeration) value; // Enumeration<AllergyIntoleranceType> 2622 return value; 2623 case 50511102: // category 2624 value = new AllergyIntoleranceCategoryEnumFactory().fromType(castToCode(value)); 2625 this.getCategory().add((Enumeration) value); // Enumeration<AllergyIntoleranceCategory> 2626 return value; 2627 case -1608054609: // criticality 2628 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(castToCode(value)); 2629 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2630 return value; 2631 case 3059181: // code 2632 this.code = castToCodeableConcept(value); // CodeableConcept 2633 return value; 2634 case -791418107: // patient 2635 this.patient = castToReference(value); // Reference 2636 return value; 2637 case 1524132147: // encounter 2638 this.encounter = castToReference(value); // Reference 2639 return value; 2640 case 105901603: // onset 2641 this.onset = castToType(value); // Type 2642 return value; 2643 case -1952893826: // recordedDate 2644 this.recordedDate = castToDateTime(value); // DateTimeType 2645 return value; 2646 case -799233858: // recorder 2647 this.recorder = castToReference(value); // Reference 2648 return value; 2649 case -373242253: // asserter 2650 this.asserter = castToReference(value); // Reference 2651 return value; 2652 case 1896977671: // lastOccurrence 2653 this.lastOccurrence = castToDateTime(value); // DateTimeType 2654 return value; 2655 case 3387378: // note 2656 this.getNote().add(castToAnnotation(value)); // Annotation 2657 return value; 2658 case -867509719: // reaction 2659 this.getReaction().add((AllergyIntoleranceReactionComponent) value); // AllergyIntoleranceReactionComponent 2660 return value; 2661 default: 2662 return super.setProperty(hash, name, value); 2663 } 2664 2665 } 2666 2667 @Override 2668 public Base setProperty(String name, Base value) throws FHIRException { 2669 if (name.equals("identifier")) { 2670 this.getIdentifier().add(castToIdentifier(value)); 2671 } else if (name.equals("clinicalStatus")) { 2672 this.clinicalStatus = castToCodeableConcept(value); // CodeableConcept 2673 } else if (name.equals("verificationStatus")) { 2674 this.verificationStatus = castToCodeableConcept(value); // CodeableConcept 2675 } else if (name.equals("type")) { 2676 value = new AllergyIntoleranceTypeEnumFactory().fromType(castToCode(value)); 2677 this.type = (Enumeration) value; // Enumeration<AllergyIntoleranceType> 2678 } else if (name.equals("category")) { 2679 value = new AllergyIntoleranceCategoryEnumFactory().fromType(castToCode(value)); 2680 this.getCategory().add((Enumeration) value); 2681 } else if (name.equals("criticality")) { 2682 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(castToCode(value)); 2683 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2684 } else if (name.equals("code")) { 2685 this.code = castToCodeableConcept(value); // CodeableConcept 2686 } else if (name.equals("patient")) { 2687 this.patient = castToReference(value); // Reference 2688 } else if (name.equals("encounter")) { 2689 this.encounter = castToReference(value); // Reference 2690 } else if (name.equals("onset[x]")) { 2691 this.onset = castToType(value); // Type 2692 } else if (name.equals("recordedDate")) { 2693 this.recordedDate = castToDateTime(value); // DateTimeType 2694 } else if (name.equals("recorder")) { 2695 this.recorder = castToReference(value); // Reference 2696 } else if (name.equals("asserter")) { 2697 this.asserter = castToReference(value); // Reference 2698 } else if (name.equals("lastOccurrence")) { 2699 this.lastOccurrence = castToDateTime(value); // DateTimeType 2700 } else if (name.equals("note")) { 2701 this.getNote().add(castToAnnotation(value)); 2702 } else if (name.equals("reaction")) { 2703 this.getReaction().add((AllergyIntoleranceReactionComponent) value); 2704 } else 2705 return super.setProperty(name, value); 2706 return value; 2707 } 2708 2709 @Override 2710 public void removeChild(String name, Base value) throws FHIRException { 2711 if (name.equals("identifier")) { 2712 this.getIdentifier().remove(castToIdentifier(value)); 2713 } else if (name.equals("clinicalStatus")) { 2714 this.clinicalStatus = null; 2715 } else if (name.equals("verificationStatus")) { 2716 this.verificationStatus = null; 2717 } else if (name.equals("type")) { 2718 this.type = null; 2719 } else if (name.equals("category")) { 2720 this.getCategory().remove((Enumeration) value); 2721 } else if (name.equals("criticality")) { 2722 value = null; 2723 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2724 } else if (name.equals("code")) { 2725 this.code = null; 2726 } else if (name.equals("patient")) { 2727 this.patient = null; 2728 } else if (name.equals("encounter")) { 2729 this.encounter = null; 2730 } else if (name.equals("onset[x]")) { 2731 this.onset = null; 2732 } else if (name.equals("recordedDate")) { 2733 this.recordedDate = null; 2734 } else if (name.equals("recorder")) { 2735 this.recorder = null; 2736 } else if (name.equals("asserter")) { 2737 this.asserter = null; 2738 } else if (name.equals("lastOccurrence")) { 2739 this.lastOccurrence = null; 2740 } else if (name.equals("note")) { 2741 this.getNote().remove(castToAnnotation(value)); 2742 } else if (name.equals("reaction")) { 2743 this.getReaction().remove((AllergyIntoleranceReactionComponent) value); 2744 } else 2745 super.removeChild(name, value); 2746 2747 } 2748 2749 @Override 2750 public Base makeProperty(int hash, String name) throws FHIRException { 2751 switch (hash) { 2752 case -1618432855: 2753 return addIdentifier(); 2754 case -462853915: 2755 return getClinicalStatus(); 2756 case -842509843: 2757 return getVerificationStatus(); 2758 case 3575610: 2759 return getTypeElement(); 2760 case 50511102: 2761 return addCategoryElement(); 2762 case -1608054609: 2763 return getCriticalityElement(); 2764 case 3059181: 2765 return getCode(); 2766 case -791418107: 2767 return getPatient(); 2768 case 1524132147: 2769 return getEncounter(); 2770 case -1886216323: 2771 return getOnset(); 2772 case 105901603: 2773 return getOnset(); 2774 case -1952893826: 2775 return getRecordedDateElement(); 2776 case -799233858: 2777 return getRecorder(); 2778 case -373242253: 2779 return getAsserter(); 2780 case 1896977671: 2781 return getLastOccurrenceElement(); 2782 case 3387378: 2783 return addNote(); 2784 case -867509719: 2785 return addReaction(); 2786 default: 2787 return super.makeProperty(hash, name); 2788 } 2789 2790 } 2791 2792 @Override 2793 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2794 switch (hash) { 2795 case -1618432855: 2796 /* identifier */ return new String[] { "Identifier" }; 2797 case -462853915: 2798 /* clinicalStatus */ return new String[] { "CodeableConcept" }; 2799 case -842509843: 2800 /* verificationStatus */ return new String[] { "CodeableConcept" }; 2801 case 3575610: 2802 /* type */ return new String[] { "code" }; 2803 case 50511102: 2804 /* category */ return new String[] { "code" }; 2805 case -1608054609: 2806 /* criticality */ return new String[] { "code" }; 2807 case 3059181: 2808 /* code */ return new String[] { "CodeableConcept" }; 2809 case -791418107: 2810 /* patient */ return new String[] { "Reference" }; 2811 case 1524132147: 2812 /* encounter */ return new String[] { "Reference" }; 2813 case 105901603: 2814 /* onset */ return new String[] { "dateTime", "Age", "Period", "Range", "string" }; 2815 case -1952893826: 2816 /* recordedDate */ return new String[] { "dateTime" }; 2817 case -799233858: 2818 /* recorder */ return new String[] { "Reference" }; 2819 case -373242253: 2820 /* asserter */ return new String[] { "Reference" }; 2821 case 1896977671: 2822 /* lastOccurrence */ return new String[] { "dateTime" }; 2823 case 3387378: 2824 /* note */ return new String[] { "Annotation" }; 2825 case -867509719: 2826 /* reaction */ return new String[] {}; 2827 default: 2828 return super.getTypesForProperty(hash, name); 2829 } 2830 2831 } 2832 2833 @Override 2834 public Base addChild(String name) throws FHIRException { 2835 if (name.equals("identifier")) { 2836 return addIdentifier(); 2837 } else if (name.equals("clinicalStatus")) { 2838 this.clinicalStatus = new CodeableConcept(); 2839 return this.clinicalStatus; 2840 } else if (name.equals("verificationStatus")) { 2841 this.verificationStatus = new CodeableConcept(); 2842 return this.verificationStatus; 2843 } else if (name.equals("type")) { 2844 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.type"); 2845 } else if (name.equals("category")) { 2846 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.category"); 2847 } else if (name.equals("criticality")) { 2848 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.criticality"); 2849 } else if (name.equals("code")) { 2850 this.code = new CodeableConcept(); 2851 return this.code; 2852 } else if (name.equals("patient")) { 2853 this.patient = new Reference(); 2854 return this.patient; 2855 } else if (name.equals("encounter")) { 2856 this.encounter = new Reference(); 2857 return this.encounter; 2858 } else if (name.equals("onsetDateTime")) { 2859 this.onset = new DateTimeType(); 2860 return this.onset; 2861 } else if (name.equals("onsetAge")) { 2862 this.onset = new Age(); 2863 return this.onset; 2864 } else if (name.equals("onsetPeriod")) { 2865 this.onset = new Period(); 2866 return this.onset; 2867 } else if (name.equals("onsetRange")) { 2868 this.onset = new Range(); 2869 return this.onset; 2870 } else if (name.equals("onsetString")) { 2871 this.onset = new StringType(); 2872 return this.onset; 2873 } else if (name.equals("recordedDate")) { 2874 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.recordedDate"); 2875 } else if (name.equals("recorder")) { 2876 this.recorder = new Reference(); 2877 return this.recorder; 2878 } else if (name.equals("asserter")) { 2879 this.asserter = new Reference(); 2880 return this.asserter; 2881 } else if (name.equals("lastOccurrence")) { 2882 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.lastOccurrence"); 2883 } else if (name.equals("note")) { 2884 return addNote(); 2885 } else if (name.equals("reaction")) { 2886 return addReaction(); 2887 } else 2888 return super.addChild(name); 2889 } 2890 2891 public String fhirType() { 2892 return "AllergyIntolerance"; 2893 2894 } 2895 2896 public AllergyIntolerance copy() { 2897 AllergyIntolerance dst = new AllergyIntolerance(); 2898 copyValues(dst); 2899 return dst; 2900 } 2901 2902 public void copyValues(AllergyIntolerance dst) { 2903 super.copyValues(dst); 2904 if (identifier != null) { 2905 dst.identifier = new ArrayList<Identifier>(); 2906 for (Identifier i : identifier) 2907 dst.identifier.add(i.copy()); 2908 } 2909 ; 2910 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 2911 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 2912 dst.type = type == null ? null : type.copy(); 2913 if (category != null) { 2914 dst.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 2915 for (Enumeration<AllergyIntoleranceCategory> i : category) 2916 dst.category.add(i.copy()); 2917 } 2918 ; 2919 dst.criticality = criticality == null ? null : criticality.copy(); 2920 dst.code = code == null ? null : code.copy(); 2921 dst.patient = patient == null ? null : patient.copy(); 2922 dst.encounter = encounter == null ? null : encounter.copy(); 2923 dst.onset = onset == null ? null : onset.copy(); 2924 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2925 dst.recorder = recorder == null ? null : recorder.copy(); 2926 dst.asserter = asserter == null ? null : asserter.copy(); 2927 dst.lastOccurrence = lastOccurrence == null ? null : lastOccurrence.copy(); 2928 if (note != null) { 2929 dst.note = new ArrayList<Annotation>(); 2930 for (Annotation i : note) 2931 dst.note.add(i.copy()); 2932 } 2933 ; 2934 if (reaction != null) { 2935 dst.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2936 for (AllergyIntoleranceReactionComponent i : reaction) 2937 dst.reaction.add(i.copy()); 2938 } 2939 ; 2940 } 2941 2942 protected AllergyIntolerance typedCopy() { 2943 return copy(); 2944 } 2945 2946 @Override 2947 public boolean equalsDeep(Base other_) { 2948 if (!super.equalsDeep(other_)) 2949 return false; 2950 if (!(other_ instanceof AllergyIntolerance)) 2951 return false; 2952 AllergyIntolerance o = (AllergyIntolerance) other_; 2953 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 2954 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(type, o.type, true) 2955 && compareDeep(category, o.category, true) && compareDeep(criticality, o.criticality, true) 2956 && compareDeep(code, o.code, true) && compareDeep(patient, o.patient, true) 2957 && compareDeep(encounter, o.encounter, true) && compareDeep(onset, o.onset, true) 2958 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(recorder, o.recorder, true) 2959 && compareDeep(asserter, o.asserter, true) && compareDeep(lastOccurrence, o.lastOccurrence, true) 2960 && compareDeep(note, o.note, true) && compareDeep(reaction, o.reaction, true); 2961 } 2962 2963 @Override 2964 public boolean equalsShallow(Base other_) { 2965 if (!super.equalsShallow(other_)) 2966 return false; 2967 if (!(other_ instanceof AllergyIntolerance)) 2968 return false; 2969 AllergyIntolerance o = (AllergyIntolerance) other_; 2970 return compareValues(type, o.type, true) && compareValues(category, o.category, true) 2971 && compareValues(criticality, o.criticality, true) && compareValues(recordedDate, o.recordedDate, true) 2972 && compareValues(lastOccurrence, o.lastOccurrence, true); 2973 } 2974 2975 public boolean isEmpty() { 2976 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus, verificationStatus, type, 2977 category, criticality, code, patient, encounter, onset, recordedDate, recorder, asserter, lastOccurrence, note, 2978 reaction); 2979 } 2980 2981 @Override 2982 public ResourceType getResourceType() { 2983 return ResourceType.AllergyIntolerance; 2984 } 2985 2986 /** 2987 * Search parameter: <b>severity</b> 2988 * <p> 2989 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2990 * Type: <b>token</b><br> 2991 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2992 * </p> 2993 */ 2994 @SearchParamDefinition(name = "severity", path = "AllergyIntolerance.reaction.severity", description = "mild | moderate | severe (of event as a whole)", type = "token") 2995 public static final String SP_SEVERITY = "severity"; 2996 /** 2997 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2998 * <p> 2999 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 3000 * Type: <b>token</b><br> 3001 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 3002 * </p> 3003 */ 3004 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3005 SP_SEVERITY); 3006 3007 /** 3008 * Search parameter: <b>date</b> 3009 * <p> 3010 * Description: <b>Date first version of the resource instance was 3011 * recorded</b><br> 3012 * Type: <b>date</b><br> 3013 * Path: <b>AllergyIntolerance.recordedDate</b><br> 3014 * </p> 3015 */ 3016 @SearchParamDefinition(name = "date", path = "AllergyIntolerance.recordedDate", description = "Date first version of the resource instance was recorded", type = "date") 3017 public static final String SP_DATE = "date"; 3018 /** 3019 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3020 * <p> 3021 * Description: <b>Date first version of the resource instance was 3022 * recorded</b><br> 3023 * Type: <b>date</b><br> 3024 * Path: <b>AllergyIntolerance.recordedDate</b><br> 3025 * </p> 3026 */ 3027 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3028 SP_DATE); 3029 3030 /** 3031 * Search parameter: <b>identifier</b> 3032 * <p> 3033 * Description: <b>External ids for this item</b><br> 3034 * Type: <b>token</b><br> 3035 * Path: <b>AllergyIntolerance.identifier</b><br> 3036 * </p> 3037 */ 3038 @SearchParamDefinition(name = "identifier", path = "AllergyIntolerance.identifier", description = "External ids for this item", type = "token") 3039 public static final String SP_IDENTIFIER = "identifier"; 3040 /** 3041 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3042 * <p> 3043 * Description: <b>External ids for this item</b><br> 3044 * Type: <b>token</b><br> 3045 * Path: <b>AllergyIntolerance.identifier</b><br> 3046 * </p> 3047 */ 3048 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3049 SP_IDENTIFIER); 3050 3051 /** 3052 * Search parameter: <b>manifestation</b> 3053 * <p> 3054 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 3055 * Type: <b>token</b><br> 3056 * Path: <b>AllergyIntolerance.reaction.manifestation</b><br> 3057 * </p> 3058 */ 3059 @SearchParamDefinition(name = "manifestation", path = "AllergyIntolerance.reaction.manifestation", description = "Clinical symptoms/signs associated with the Event", type = "token") 3060 public static final String SP_MANIFESTATION = "manifestation"; 3061 /** 3062 * <b>Fluent Client</b> search parameter constant for <b>manifestation</b> 3063 * <p> 3064 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 3065 * Type: <b>token</b><br> 3066 * Path: <b>AllergyIntolerance.reaction.manifestation</b><br> 3067 * </p> 3068 */ 3069 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MANIFESTATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3070 SP_MANIFESTATION); 3071 3072 /** 3073 * Search parameter: <b>recorder</b> 3074 * <p> 3075 * Description: <b>Who recorded the sensitivity</b><br> 3076 * Type: <b>reference</b><br> 3077 * Path: <b>AllergyIntolerance.recorder</b><br> 3078 * </p> 3079 */ 3080 @SearchParamDefinition(name = "recorder", path = "AllergyIntolerance.recorder", description = "Who recorded the sensitivity", type = "reference", providesMembershipIn = { 3081 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3082 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Patient.class, 3083 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3084 public static final String SP_RECORDER = "recorder"; 3085 /** 3086 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 3087 * <p> 3088 * Description: <b>Who recorded the sensitivity</b><br> 3089 * Type: <b>reference</b><br> 3090 * Path: <b>AllergyIntolerance.recorder</b><br> 3091 * </p> 3092 */ 3093 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3094 SP_RECORDER); 3095 3096 /** 3097 * Constant for fluent queries to be used to add include statements. Specifies 3098 * the path value of "<b>AllergyIntolerance:recorder</b>". 3099 */ 3100 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include( 3101 "AllergyIntolerance:recorder").toLocked(); 3102 3103 /** 3104 * Search parameter: <b>code</b> 3105 * <p> 3106 * Description: <b>Code that identifies the allergy or intolerance</b><br> 3107 * Type: <b>token</b><br> 3108 * Path: <b>AllergyIntolerance.code, 3109 * AllergyIntolerance.reaction.substance</b><br> 3110 * </p> 3111 */ 3112 @SearchParamDefinition(name = "code", path = "AllergyIntolerance.code | AllergyIntolerance.reaction.substance", description = "Code that identifies the allergy or intolerance", type = "token") 3113 public static final String SP_CODE = "code"; 3114 /** 3115 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3116 * <p> 3117 * Description: <b>Code that identifies the allergy or intolerance</b><br> 3118 * Type: <b>token</b><br> 3119 * Path: <b>AllergyIntolerance.code, 3120 * AllergyIntolerance.reaction.substance</b><br> 3121 * </p> 3122 */ 3123 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3124 SP_CODE); 3125 3126 /** 3127 * Search parameter: <b>verification-status</b> 3128 * <p> 3129 * Description: <b>unconfirmed | confirmed | refuted | entered-in-error</b><br> 3130 * Type: <b>token</b><br> 3131 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 3132 * </p> 3133 */ 3134 @SearchParamDefinition(name = "verification-status", path = "AllergyIntolerance.verificationStatus", description = "unconfirmed | confirmed | refuted | entered-in-error", type = "token") 3135 public static final String SP_VERIFICATION_STATUS = "verification-status"; 3136 /** 3137 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 3138 * <p> 3139 * Description: <b>unconfirmed | confirmed | refuted | entered-in-error</b><br> 3140 * Type: <b>token</b><br> 3141 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 3142 * </p> 3143 */ 3144 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3145 SP_VERIFICATION_STATUS); 3146 3147 /** 3148 * Search parameter: <b>criticality</b> 3149 * <p> 3150 * Description: <b>low | high | unable-to-assess</b><br> 3151 * Type: <b>token</b><br> 3152 * Path: <b>AllergyIntolerance.criticality</b><br> 3153 * </p> 3154 */ 3155 @SearchParamDefinition(name = "criticality", path = "AllergyIntolerance.criticality", description = "low | high | unable-to-assess", type = "token") 3156 public static final String SP_CRITICALITY = "criticality"; 3157 /** 3158 * <b>Fluent Client</b> search parameter constant for <b>criticality</b> 3159 * <p> 3160 * Description: <b>low | high | unable-to-assess</b><br> 3161 * Type: <b>token</b><br> 3162 * Path: <b>AllergyIntolerance.criticality</b><br> 3163 * </p> 3164 */ 3165 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CRITICALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3166 SP_CRITICALITY); 3167 3168 /** 3169 * Search parameter: <b>clinical-status</b> 3170 * <p> 3171 * Description: <b>active | inactive | resolved</b><br> 3172 * Type: <b>token</b><br> 3173 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 3174 * </p> 3175 */ 3176 @SearchParamDefinition(name = "clinical-status", path = "AllergyIntolerance.clinicalStatus", description = "active | inactive | resolved", type = "token") 3177 public static final String SP_CLINICAL_STATUS = "clinical-status"; 3178 /** 3179 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 3180 * <p> 3181 * Description: <b>active | inactive | resolved</b><br> 3182 * Type: <b>token</b><br> 3183 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 3184 * </p> 3185 */ 3186 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3187 SP_CLINICAL_STATUS); 3188 3189 /** 3190 * Search parameter: <b>type</b> 3191 * <p> 3192 * Description: <b>allergy | intolerance - Underlying mechanism (if 3193 * known)</b><br> 3194 * Type: <b>token</b><br> 3195 * Path: <b>AllergyIntolerance.type</b><br> 3196 * </p> 3197 */ 3198 @SearchParamDefinition(name = "type", path = "AllergyIntolerance.type", description = "allergy | intolerance - Underlying mechanism (if known)", type = "token") 3199 public static final String SP_TYPE = "type"; 3200 /** 3201 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3202 * <p> 3203 * Description: <b>allergy | intolerance - Underlying mechanism (if 3204 * known)</b><br> 3205 * Type: <b>token</b><br> 3206 * Path: <b>AllergyIntolerance.type</b><br> 3207 * </p> 3208 */ 3209 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3210 SP_TYPE); 3211 3212 /** 3213 * Search parameter: <b>onset</b> 3214 * <p> 3215 * Description: <b>Date(/time) when manifestations showed</b><br> 3216 * Type: <b>date</b><br> 3217 * Path: <b>AllergyIntolerance.reaction.onset</b><br> 3218 * </p> 3219 */ 3220 @SearchParamDefinition(name = "onset", path = "AllergyIntolerance.reaction.onset", description = "Date(/time) when manifestations showed", type = "date") 3221 public static final String SP_ONSET = "onset"; 3222 /** 3223 * <b>Fluent Client</b> search parameter constant for <b>onset</b> 3224 * <p> 3225 * Description: <b>Date(/time) when manifestations showed</b><br> 3226 * Type: <b>date</b><br> 3227 * Path: <b>AllergyIntolerance.reaction.onset</b><br> 3228 * </p> 3229 */ 3230 public static final ca.uhn.fhir.rest.gclient.DateClientParam ONSET = new ca.uhn.fhir.rest.gclient.DateClientParam( 3231 SP_ONSET); 3232 3233 /** 3234 * Search parameter: <b>route</b> 3235 * <p> 3236 * Description: <b>How the subject was exposed to the substance</b><br> 3237 * Type: <b>token</b><br> 3238 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 3239 * </p> 3240 */ 3241 @SearchParamDefinition(name = "route", path = "AllergyIntolerance.reaction.exposureRoute", description = "How the subject was exposed to the substance", type = "token") 3242 public static final String SP_ROUTE = "route"; 3243 /** 3244 * <b>Fluent Client</b> search parameter constant for <b>route</b> 3245 * <p> 3246 * Description: <b>How the subject was exposed to the substance</b><br> 3247 * Type: <b>token</b><br> 3248 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 3249 * </p> 3250 */ 3251 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3252 SP_ROUTE); 3253 3254 /** 3255 * Search parameter: <b>asserter</b> 3256 * <p> 3257 * Description: <b>Source of the information about the allergy</b><br> 3258 * Type: <b>reference</b><br> 3259 * Path: <b>AllergyIntolerance.asserter</b><br> 3260 * </p> 3261 */ 3262 @SearchParamDefinition(name = "asserter", path = "AllergyIntolerance.asserter", description = "Source of the information about the allergy", type = "reference", providesMembershipIn = { 3263 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3264 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3265 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 3266 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3267 public static final String SP_ASSERTER = "asserter"; 3268 /** 3269 * <b>Fluent Client</b> search parameter constant for <b>asserter</b> 3270 * <p> 3271 * Description: <b>Source of the information about the allergy</b><br> 3272 * Type: <b>reference</b><br> 3273 * Path: <b>AllergyIntolerance.asserter</b><br> 3274 * </p> 3275 */ 3276 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSERTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3277 SP_ASSERTER); 3278 3279 /** 3280 * Constant for fluent queries to be used to add include statements. Specifies 3281 * the path value of "<b>AllergyIntolerance:asserter</b>". 3282 */ 3283 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSERTER = new ca.uhn.fhir.model.api.Include( 3284 "AllergyIntolerance:asserter").toLocked(); 3285 3286 /** 3287 * Search parameter: <b>patient</b> 3288 * <p> 3289 * Description: <b>Who the sensitivity is for</b><br> 3290 * Type: <b>reference</b><br> 3291 * Path: <b>AllergyIntolerance.patient</b><br> 3292 * </p> 3293 */ 3294 @SearchParamDefinition(name = "patient", path = "AllergyIntolerance.patient", description = "Who the sensitivity is for", type = "reference", providesMembershipIn = { 3295 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3296 public static final String SP_PATIENT = "patient"; 3297 /** 3298 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3299 * <p> 3300 * Description: <b>Who the sensitivity is for</b><br> 3301 * Type: <b>reference</b><br> 3302 * Path: <b>AllergyIntolerance.patient</b><br> 3303 * </p> 3304 */ 3305 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3306 SP_PATIENT); 3307 3308 /** 3309 * Constant for fluent queries to be used to add include statements. Specifies 3310 * the path value of "<b>AllergyIntolerance:patient</b>". 3311 */ 3312 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3313 "AllergyIntolerance:patient").toLocked(); 3314 3315 /** 3316 * Search parameter: <b>category</b> 3317 * <p> 3318 * Description: <b>food | medication | environment | biologic</b><br> 3319 * Type: <b>token</b><br> 3320 * Path: <b>AllergyIntolerance.category</b><br> 3321 * </p> 3322 */ 3323 @SearchParamDefinition(name = "category", path = "AllergyIntolerance.category", description = "food | medication | environment | biologic", type = "token") 3324 public static final String SP_CATEGORY = "category"; 3325 /** 3326 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3327 * <p> 3328 * Description: <b>food | medication | environment | biologic</b><br> 3329 * Type: <b>token</b><br> 3330 * Path: <b>AllergyIntolerance.category</b><br> 3331 * </p> 3332 */ 3333 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3334 SP_CATEGORY); 3335 3336 /** 3337 * Search parameter: <b>last-date</b> 3338 * <p> 3339 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 3340 * Type: <b>date</b><br> 3341 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 3342 * </p> 3343 */ 3344 @SearchParamDefinition(name = "last-date", path = "AllergyIntolerance.lastOccurrence", description = "Date(/time) of last known occurrence of a reaction", type = "date") 3345 public static final String SP_LAST_DATE = "last-date"; 3346 /** 3347 * <b>Fluent Client</b> search parameter constant for <b>last-date</b> 3348 * <p> 3349 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 3350 * Type: <b>date</b><br> 3351 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 3352 * </p> 3353 */ 3354 public static final ca.uhn.fhir.rest.gclient.DateClientParam LAST_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3355 SP_LAST_DATE); 3356 3357}