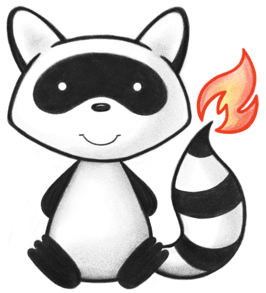
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A booking of a healthcare event among patient(s), practitioner(s), related 049 * person(s) and/or device(s) for a specific date/time. This may result in one 050 * or more Encounter(s). 051 */ 052@ResourceDef(name = "Appointment", profile = "http://hl7.org/fhir/StructureDefinition/Appointment") 053public class Appointment extends DomainResource { 054 055 public enum AppointmentStatus { 056 /** 057 * None of the participant(s) have finalized their acceptance of the appointment 058 * request, and the start/end time might not be set yet. 059 */ 060 PROPOSED, 061 /** 062 * Some or all of the participant(s) have not finalized their acceptance of the 063 * appointment request. 064 */ 065 PENDING, 066 /** 067 * All participant(s) have been considered and the appointment is confirmed to 068 * go ahead at the date/times specified. 069 */ 070 BOOKED, 071 /** 072 * The patient/patients has/have arrived and is/are waiting to be seen. 073 */ 074 ARRIVED, 075 /** 076 * The planning stages of the appointment are now complete, the encounter 077 * resource will exist and will track further status changes. Note that an 078 * encounter may exist before the appointment status is fulfilled for many 079 * reasons. 080 */ 081 FULFILLED, 082 /** 083 * The appointment has been cancelled. 084 */ 085 CANCELLED, 086 /** 087 * Some or all of the participant(s) have not/did not appear for the appointment 088 * (usually the patient). 089 */ 090 NOSHOW, 091 /** 092 * This instance should not have been part of this patient's medical record. 093 */ 094 ENTEREDINERROR, 095 /** 096 * When checked in, all pre-encounter administrative work is complete, and the 097 * encounter may begin. (where multiple patients are involved, they are all 098 * present). 099 */ 100 CHECKEDIN, 101 /** 102 * The appointment has been placed on a waitlist, to be scheduled/confirmed in 103 * the future when a slot/service is available. A specific time might or might 104 * not be pre-allocated. 105 */ 106 WAITLIST, 107 /** 108 * added to help the parsers with the generic types 109 */ 110 NULL; 111 112 public static AppointmentStatus fromCode(String codeString) throws FHIRException { 113 if (codeString == null || "".equals(codeString)) 114 return null; 115 if ("proposed".equals(codeString)) 116 return PROPOSED; 117 if ("pending".equals(codeString)) 118 return PENDING; 119 if ("booked".equals(codeString)) 120 return BOOKED; 121 if ("arrived".equals(codeString)) 122 return ARRIVED; 123 if ("fulfilled".equals(codeString)) 124 return FULFILLED; 125 if ("cancelled".equals(codeString)) 126 return CANCELLED; 127 if ("noshow".equals(codeString)) 128 return NOSHOW; 129 if ("entered-in-error".equals(codeString)) 130 return ENTEREDINERROR; 131 if ("checked-in".equals(codeString)) 132 return CHECKEDIN; 133 if ("waitlist".equals(codeString)) 134 return WAITLIST; 135 if (Configuration.isAcceptInvalidEnums()) 136 return null; 137 else 138 throw new FHIRException("Unknown AppointmentStatus code '" + codeString + "'"); 139 } 140 141 public String toCode() { 142 switch (this) { 143 case PROPOSED: 144 return "proposed"; 145 case PENDING: 146 return "pending"; 147 case BOOKED: 148 return "booked"; 149 case ARRIVED: 150 return "arrived"; 151 case FULFILLED: 152 return "fulfilled"; 153 case CANCELLED: 154 return "cancelled"; 155 case NOSHOW: 156 return "noshow"; 157 case ENTEREDINERROR: 158 return "entered-in-error"; 159 case CHECKEDIN: 160 return "checked-in"; 161 case WAITLIST: 162 return "waitlist"; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getSystem() { 171 switch (this) { 172 case PROPOSED: 173 return "http://hl7.org/fhir/appointmentstatus"; 174 case PENDING: 175 return "http://hl7.org/fhir/appointmentstatus"; 176 case BOOKED: 177 return "http://hl7.org/fhir/appointmentstatus"; 178 case ARRIVED: 179 return "http://hl7.org/fhir/appointmentstatus"; 180 case FULFILLED: 181 return "http://hl7.org/fhir/appointmentstatus"; 182 case CANCELLED: 183 return "http://hl7.org/fhir/appointmentstatus"; 184 case NOSHOW: 185 return "http://hl7.org/fhir/appointmentstatus"; 186 case ENTEREDINERROR: 187 return "http://hl7.org/fhir/appointmentstatus"; 188 case CHECKEDIN: 189 return "http://hl7.org/fhir/appointmentstatus"; 190 case WAITLIST: 191 return "http://hl7.org/fhir/appointmentstatus"; 192 case NULL: 193 return null; 194 default: 195 return "?"; 196 } 197 } 198 199 public String getDefinition() { 200 switch (this) { 201 case PROPOSED: 202 return "None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time might not be set yet."; 203 case PENDING: 204 return "Some or all of the participant(s) have not finalized their acceptance of the appointment request."; 205 case BOOKED: 206 return "All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified."; 207 case ARRIVED: 208 return "The patient/patients has/have arrived and is/are waiting to be seen."; 209 case FULFILLED: 210 return "The planning stages of the appointment are now complete, the encounter resource will exist and will track further status changes. Note that an encounter may exist before the appointment status is fulfilled for many reasons."; 211 case CANCELLED: 212 return "The appointment has been cancelled."; 213 case NOSHOW: 214 return "Some or all of the participant(s) have not/did not appear for the appointment (usually the patient)."; 215 case ENTEREDINERROR: 216 return "This instance should not have been part of this patient's medical record."; 217 case CHECKEDIN: 218 return "When checked in, all pre-encounter administrative work is complete, and the encounter may begin. (where multiple patients are involved, they are all present)."; 219 case WAITLIST: 220 return "The appointment has been placed on a waitlist, to be scheduled/confirmed in the future when a slot/service is available.\nA specific time might or might not be pre-allocated."; 221 case NULL: 222 return null; 223 default: 224 return "?"; 225 } 226 } 227 228 public String getDisplay() { 229 switch (this) { 230 case PROPOSED: 231 return "Proposed"; 232 case PENDING: 233 return "Pending"; 234 case BOOKED: 235 return "Booked"; 236 case ARRIVED: 237 return "Arrived"; 238 case FULFILLED: 239 return "Fulfilled"; 240 case CANCELLED: 241 return "Cancelled"; 242 case NOSHOW: 243 return "No Show"; 244 case ENTEREDINERROR: 245 return "Entered in error"; 246 case CHECKEDIN: 247 return "Checked In"; 248 case WAITLIST: 249 return "Waitlisted"; 250 case NULL: 251 return null; 252 default: 253 return "?"; 254 } 255 } 256 } 257 258 public static class AppointmentStatusEnumFactory implements EnumFactory<AppointmentStatus> { 259 public AppointmentStatus fromCode(String codeString) throws IllegalArgumentException { 260 if (codeString == null || "".equals(codeString)) 261 if (codeString == null || "".equals(codeString)) 262 return null; 263 if ("proposed".equals(codeString)) 264 return AppointmentStatus.PROPOSED; 265 if ("pending".equals(codeString)) 266 return AppointmentStatus.PENDING; 267 if ("booked".equals(codeString)) 268 return AppointmentStatus.BOOKED; 269 if ("arrived".equals(codeString)) 270 return AppointmentStatus.ARRIVED; 271 if ("fulfilled".equals(codeString)) 272 return AppointmentStatus.FULFILLED; 273 if ("cancelled".equals(codeString)) 274 return AppointmentStatus.CANCELLED; 275 if ("noshow".equals(codeString)) 276 return AppointmentStatus.NOSHOW; 277 if ("entered-in-error".equals(codeString)) 278 return AppointmentStatus.ENTEREDINERROR; 279 if ("checked-in".equals(codeString)) 280 return AppointmentStatus.CHECKEDIN; 281 if ("waitlist".equals(codeString)) 282 return AppointmentStatus.WAITLIST; 283 throw new IllegalArgumentException("Unknown AppointmentStatus code '" + codeString + "'"); 284 } 285 286 public Enumeration<AppointmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 287 if (code == null) 288 return null; 289 if (code.isEmpty()) 290 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NULL, code); 291 String codeString = code.asStringValue(); 292 if (codeString == null || "".equals(codeString)) 293 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NULL, code); 294 if ("proposed".equals(codeString)) 295 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PROPOSED, code); 296 if ("pending".equals(codeString)) 297 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PENDING, code); 298 if ("booked".equals(codeString)) 299 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.BOOKED, code); 300 if ("arrived".equals(codeString)) 301 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ARRIVED, code); 302 if ("fulfilled".equals(codeString)) 303 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.FULFILLED, code); 304 if ("cancelled".equals(codeString)) 305 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CANCELLED, code); 306 if ("noshow".equals(codeString)) 307 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NOSHOW, code); 308 if ("entered-in-error".equals(codeString)) 309 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ENTEREDINERROR, code); 310 if ("checked-in".equals(codeString)) 311 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CHECKEDIN, code); 312 if ("waitlist".equals(codeString)) 313 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.WAITLIST, code); 314 throw new FHIRException("Unknown AppointmentStatus code '" + codeString + "'"); 315 } 316 317 public String toCode(AppointmentStatus code) { 318 if (code == AppointmentStatus.NULL) 319 return null; 320 if (code == AppointmentStatus.PROPOSED) 321 return "proposed"; 322 if (code == AppointmentStatus.PENDING) 323 return "pending"; 324 if (code == AppointmentStatus.BOOKED) 325 return "booked"; 326 if (code == AppointmentStatus.ARRIVED) 327 return "arrived"; 328 if (code == AppointmentStatus.FULFILLED) 329 return "fulfilled"; 330 if (code == AppointmentStatus.CANCELLED) 331 return "cancelled"; 332 if (code == AppointmentStatus.NOSHOW) 333 return "noshow"; 334 if (code == AppointmentStatus.ENTEREDINERROR) 335 return "entered-in-error"; 336 if (code == AppointmentStatus.CHECKEDIN) 337 return "checked-in"; 338 if (code == AppointmentStatus.WAITLIST) 339 return "waitlist"; 340 return "?"; 341 } 342 343 public String toSystem(AppointmentStatus code) { 344 return code.getSystem(); 345 } 346 } 347 348 public enum ParticipantRequired { 349 /** 350 * The participant is required to attend the appointment. 351 */ 352 REQUIRED, 353 /** 354 * The participant may optionally attend the appointment. 355 */ 356 OPTIONAL, 357 /** 358 * The participant is excluded from the appointment, and might not be informed 359 * of the appointment taking place. (Appointment is about them, not for them - 360 * such as 2 doctors discussing results about a patient's test). 361 */ 362 INFORMATIONONLY, 363 /** 364 * added to help the parsers with the generic types 365 */ 366 NULL; 367 368 public static ParticipantRequired fromCode(String codeString) throws FHIRException { 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("required".equals(codeString)) 372 return REQUIRED; 373 if ("optional".equals(codeString)) 374 return OPTIONAL; 375 if ("information-only".equals(codeString)) 376 return INFORMATIONONLY; 377 if (Configuration.isAcceptInvalidEnums()) 378 return null; 379 else 380 throw new FHIRException("Unknown ParticipantRequired code '" + codeString + "'"); 381 } 382 383 public String toCode() { 384 switch (this) { 385 case REQUIRED: 386 return "required"; 387 case OPTIONAL: 388 return "optional"; 389 case INFORMATIONONLY: 390 return "information-only"; 391 case NULL: 392 return null; 393 default: 394 return "?"; 395 } 396 } 397 398 public String getSystem() { 399 switch (this) { 400 case REQUIRED: 401 return "http://hl7.org/fhir/participantrequired"; 402 case OPTIONAL: 403 return "http://hl7.org/fhir/participantrequired"; 404 case INFORMATIONONLY: 405 return "http://hl7.org/fhir/participantrequired"; 406 case NULL: 407 return null; 408 default: 409 return "?"; 410 } 411 } 412 413 public String getDefinition() { 414 switch (this) { 415 case REQUIRED: 416 return "The participant is required to attend the appointment."; 417 case OPTIONAL: 418 return "The participant may optionally attend the appointment."; 419 case INFORMATIONONLY: 420 return "The participant is excluded from the appointment, and might not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test)."; 421 case NULL: 422 return null; 423 default: 424 return "?"; 425 } 426 } 427 428 public String getDisplay() { 429 switch (this) { 430 case REQUIRED: 431 return "Required"; 432 case OPTIONAL: 433 return "Optional"; 434 case INFORMATIONONLY: 435 return "Information Only"; 436 case NULL: 437 return null; 438 default: 439 return "?"; 440 } 441 } 442 } 443 444 public static class ParticipantRequiredEnumFactory implements EnumFactory<ParticipantRequired> { 445 public ParticipantRequired fromCode(String codeString) throws IllegalArgumentException { 446 if (codeString == null || "".equals(codeString)) 447 if (codeString == null || "".equals(codeString)) 448 return null; 449 if ("required".equals(codeString)) 450 return ParticipantRequired.REQUIRED; 451 if ("optional".equals(codeString)) 452 return ParticipantRequired.OPTIONAL; 453 if ("information-only".equals(codeString)) 454 return ParticipantRequired.INFORMATIONONLY; 455 throw new IllegalArgumentException("Unknown ParticipantRequired code '" + codeString + "'"); 456 } 457 458 public Enumeration<ParticipantRequired> fromType(PrimitiveType<?> code) throws FHIRException { 459 if (code == null) 460 return null; 461 if (code.isEmpty()) 462 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.NULL, code); 463 String codeString = code.asStringValue(); 464 if (codeString == null || "".equals(codeString)) 465 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.NULL, code); 466 if ("required".equals(codeString)) 467 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.REQUIRED, code); 468 if ("optional".equals(codeString)) 469 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.OPTIONAL, code); 470 if ("information-only".equals(codeString)) 471 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.INFORMATIONONLY, code); 472 throw new FHIRException("Unknown ParticipantRequired code '" + codeString + "'"); 473 } 474 475 public String toCode(ParticipantRequired code) { 476 if (code == ParticipantRequired.NULL) 477 return null; 478 if (code == ParticipantRequired.REQUIRED) 479 return "required"; 480 if (code == ParticipantRequired.OPTIONAL) 481 return "optional"; 482 if (code == ParticipantRequired.INFORMATIONONLY) 483 return "information-only"; 484 return "?"; 485 } 486 487 public String toSystem(ParticipantRequired code) { 488 return code.getSystem(); 489 } 490 } 491 492 public enum ParticipationStatus { 493 /** 494 * The participant has accepted the appointment. 495 */ 496 ACCEPTED, 497 /** 498 * The participant has declined the appointment and will not participate in the 499 * appointment. 500 */ 501 DECLINED, 502 /** 503 * The participant has tentatively accepted the appointment. This could be 504 * automatically created by a system and requires further processing before it 505 * can be accepted. There is no commitment that attendance will occur. 506 */ 507 TENTATIVE, 508 /** 509 * The participant needs to indicate if they accept the appointment by changing 510 * this status to one of the other statuses. 511 */ 512 NEEDSACTION, 513 /** 514 * added to help the parsers with the generic types 515 */ 516 NULL; 517 518 public static ParticipationStatus fromCode(String codeString) throws FHIRException { 519 if (codeString == null || "".equals(codeString)) 520 return null; 521 if ("accepted".equals(codeString)) 522 return ACCEPTED; 523 if ("declined".equals(codeString)) 524 return DECLINED; 525 if ("tentative".equals(codeString)) 526 return TENTATIVE; 527 if ("needs-action".equals(codeString)) 528 return NEEDSACTION; 529 if (Configuration.isAcceptInvalidEnums()) 530 return null; 531 else 532 throw new FHIRException("Unknown ParticipationStatus code '" + codeString + "'"); 533 } 534 535 public String toCode() { 536 switch (this) { 537 case ACCEPTED: 538 return "accepted"; 539 case DECLINED: 540 return "declined"; 541 case TENTATIVE: 542 return "tentative"; 543 case NEEDSACTION: 544 return "needs-action"; 545 case NULL: 546 return null; 547 default: 548 return "?"; 549 } 550 } 551 552 public String getSystem() { 553 switch (this) { 554 case ACCEPTED: 555 return "http://hl7.org/fhir/participationstatus"; 556 case DECLINED: 557 return "http://hl7.org/fhir/participationstatus"; 558 case TENTATIVE: 559 return "http://hl7.org/fhir/participationstatus"; 560 case NEEDSACTION: 561 return "http://hl7.org/fhir/participationstatus"; 562 case NULL: 563 return null; 564 default: 565 return "?"; 566 } 567 } 568 569 public String getDefinition() { 570 switch (this) { 571 case ACCEPTED: 572 return "The participant has accepted the appointment."; 573 case DECLINED: 574 return "The participant has declined the appointment and will not participate in the appointment."; 575 case TENTATIVE: 576 return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 577 case NEEDSACTION: 578 return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 579 case NULL: 580 return null; 581 default: 582 return "?"; 583 } 584 } 585 586 public String getDisplay() { 587 switch (this) { 588 case ACCEPTED: 589 return "Accepted"; 590 case DECLINED: 591 return "Declined"; 592 case TENTATIVE: 593 return "Tentative"; 594 case NEEDSACTION: 595 return "Needs Action"; 596 case NULL: 597 return null; 598 default: 599 return "?"; 600 } 601 } 602 } 603 604 public static class ParticipationStatusEnumFactory implements EnumFactory<ParticipationStatus> { 605 public ParticipationStatus fromCode(String codeString) throws IllegalArgumentException { 606 if (codeString == null || "".equals(codeString)) 607 if (codeString == null || "".equals(codeString)) 608 return null; 609 if ("accepted".equals(codeString)) 610 return ParticipationStatus.ACCEPTED; 611 if ("declined".equals(codeString)) 612 return ParticipationStatus.DECLINED; 613 if ("tentative".equals(codeString)) 614 return ParticipationStatus.TENTATIVE; 615 if ("needs-action".equals(codeString)) 616 return ParticipationStatus.NEEDSACTION; 617 throw new IllegalArgumentException("Unknown ParticipationStatus code '" + codeString + "'"); 618 } 619 620 public Enumeration<ParticipationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 621 if (code == null) 622 return null; 623 if (code.isEmpty()) 624 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NULL, code); 625 String codeString = code.asStringValue(); 626 if (codeString == null || "".equals(codeString)) 627 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NULL, code); 628 if ("accepted".equals(codeString)) 629 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.ACCEPTED, code); 630 if ("declined".equals(codeString)) 631 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.DECLINED, code); 632 if ("tentative".equals(codeString)) 633 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.TENTATIVE, code); 634 if ("needs-action".equals(codeString)) 635 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NEEDSACTION, code); 636 throw new FHIRException("Unknown ParticipationStatus code '" + codeString + "'"); 637 } 638 639 public String toCode(ParticipationStatus code) { 640 if (code == ParticipationStatus.NULL) 641 return null; 642 if (code == ParticipationStatus.ACCEPTED) 643 return "accepted"; 644 if (code == ParticipationStatus.DECLINED) 645 return "declined"; 646 if (code == ParticipationStatus.TENTATIVE) 647 return "tentative"; 648 if (code == ParticipationStatus.NEEDSACTION) 649 return "needs-action"; 650 return "?"; 651 } 652 653 public String toSystem(ParticipationStatus code) { 654 return code.getSystem(); 655 } 656 } 657 658 @Block() 659 public static class AppointmentParticipantComponent extends BackboneElement implements IBaseBackboneElement { 660 /** 661 * Role of participant in the appointment. 662 */ 663 @Child(name = "type", type = { 664 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 665 @Description(shortDefinition = "Role of participant in the appointment", formalDefinition = "Role of participant in the appointment.") 666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-participant-type") 667 protected List<CodeableConcept> type; 668 669 /** 670 * A Person, Location/HealthcareService or Device that is participating in the 671 * appointment. 672 */ 673 @Child(name = "actor", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 674 Device.class, HealthcareService.class, 675 Location.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 676 @Description(shortDefinition = "Person, Location/HealthcareService or Device", formalDefinition = "A Person, Location/HealthcareService or Device that is participating in the appointment.") 677 protected Reference actor; 678 679 /** 680 * The actual object that is the target of the reference (A Person, 681 * Location/HealthcareService or Device that is participating in the 682 * appointment.) 683 */ 684 protected Resource actorTarget; 685 686 /** 687 * Whether this participant is required to be present at the meeting. This 688 * covers a use-case where two doctors need to meet to discuss the results for a 689 * specific patient, and the patient is not required to be present. 690 */ 691 @Child(name = "required", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 692 @Description(shortDefinition = "required | optional | information-only", formalDefinition = "Whether this participant is required to be present at the meeting. This covers a use-case where two doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.") 693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participantrequired") 694 protected Enumeration<ParticipantRequired> required; 695 696 /** 697 * Participation status of the actor. 698 */ 699 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 700 @Description(shortDefinition = "accepted | declined | tentative | needs-action", formalDefinition = "Participation status of the actor.") 701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participationstatus") 702 protected Enumeration<ParticipationStatus> status; 703 704 /** 705 * Participation period of the actor. 706 */ 707 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 708 @Description(shortDefinition = "Participation period of the actor", formalDefinition = "Participation period of the actor.") 709 protected Period period; 710 711 private static final long serialVersionUID = -1939292177L; 712 713 /** 714 * Constructor 715 */ 716 public AppointmentParticipantComponent() { 717 super(); 718 } 719 720 /** 721 * Constructor 722 */ 723 public AppointmentParticipantComponent(Enumeration<ParticipationStatus> status) { 724 super(); 725 this.status = status; 726 } 727 728 /** 729 * @return {@link #type} (Role of participant in the appointment.) 730 */ 731 public List<CodeableConcept> getType() { 732 if (this.type == null) 733 this.type = new ArrayList<CodeableConcept>(); 734 return this.type; 735 } 736 737 /** 738 * @return Returns a reference to <code>this</code> for easy method chaining 739 */ 740 public AppointmentParticipantComponent setType(List<CodeableConcept> theType) { 741 this.type = theType; 742 return this; 743 } 744 745 public boolean hasType() { 746 if (this.type == null) 747 return false; 748 for (CodeableConcept item : this.type) 749 if (!item.isEmpty()) 750 return true; 751 return false; 752 } 753 754 public CodeableConcept addType() { // 3 755 CodeableConcept t = new CodeableConcept(); 756 if (this.type == null) 757 this.type = new ArrayList<CodeableConcept>(); 758 this.type.add(t); 759 return t; 760 } 761 762 public AppointmentParticipantComponent addType(CodeableConcept t) { // 3 763 if (t == null) 764 return this; 765 if (this.type == null) 766 this.type = new ArrayList<CodeableConcept>(); 767 this.type.add(t); 768 return this; 769 } 770 771 /** 772 * @return The first repetition of repeating field {@link #type}, creating it if 773 * it does not already exist 774 */ 775 public CodeableConcept getTypeFirstRep() { 776 if (getType().isEmpty()) { 777 addType(); 778 } 779 return getType().get(0); 780 } 781 782 /** 783 * @return {@link #actor} (A Person, Location/HealthcareService or Device that 784 * is participating in the appointment.) 785 */ 786 public Reference getActor() { 787 if (this.actor == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create AppointmentParticipantComponent.actor"); 790 else if (Configuration.doAutoCreate()) 791 this.actor = new Reference(); // cc 792 return this.actor; 793 } 794 795 public boolean hasActor() { 796 return this.actor != null && !this.actor.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #actor} (A Person, Location/HealthcareService or Device 801 * that is participating in the appointment.) 802 */ 803 public AppointmentParticipantComponent setActor(Reference value) { 804 this.actor = value; 805 return this; 806 } 807 808 /** 809 * @return {@link #actor} The actual object that is the target of the reference. 810 * The reference library doesn't populate this, but you can use it to 811 * hold the resource if you resolve it. (A Person, 812 * Location/HealthcareService or Device that is participating in the 813 * appointment.) 814 */ 815 public Resource getActorTarget() { 816 return this.actorTarget; 817 } 818 819 /** 820 * @param value {@link #actor} The actual object that is the target of the 821 * reference. The reference library doesn't use these, but you can 822 * use it to hold the resource if you resolve it. (A Person, 823 * Location/HealthcareService or Device that is participating in 824 * the appointment.) 825 */ 826 public AppointmentParticipantComponent setActorTarget(Resource value) { 827 this.actorTarget = value; 828 return this; 829 } 830 831 /** 832 * @return {@link #required} (Whether this participant is required to be present 833 * at the meeting. This covers a use-case where two doctors need to meet 834 * to discuss the results for a specific patient, and the patient is not 835 * required to be present.). This is the underlying object with id, 836 * value and extensions. The accessor "getRequired" gives direct access 837 * to the value 838 */ 839 public Enumeration<ParticipantRequired> getRequiredElement() { 840 if (this.required == null) 841 if (Configuration.errorOnAutoCreate()) 842 throw new Error("Attempt to auto-create AppointmentParticipantComponent.required"); 843 else if (Configuration.doAutoCreate()) 844 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); // bb 845 return this.required; 846 } 847 848 public boolean hasRequiredElement() { 849 return this.required != null && !this.required.isEmpty(); 850 } 851 852 public boolean hasRequired() { 853 return this.required != null && !this.required.isEmpty(); 854 } 855 856 /** 857 * @param value {@link #required} (Whether this participant is required to be 858 * present at the meeting. This covers a use-case where two doctors 859 * need to meet to discuss the results for a specific patient, and 860 * the patient is not required to be present.). This is the 861 * underlying object with id, value and extensions. The accessor 862 * "getRequired" gives direct access to the value 863 */ 864 public AppointmentParticipantComponent setRequiredElement(Enumeration<ParticipantRequired> value) { 865 this.required = value; 866 return this; 867 } 868 869 /** 870 * @return Whether this participant is required to be present at the meeting. 871 * This covers a use-case where two doctors need to meet to discuss the 872 * results for a specific patient, and the patient is not required to be 873 * present. 874 */ 875 public ParticipantRequired getRequired() { 876 return this.required == null ? null : this.required.getValue(); 877 } 878 879 /** 880 * @param value Whether this participant is required to be present at the 881 * meeting. This covers a use-case where two doctors need to meet 882 * to discuss the results for a specific patient, and the patient 883 * is not required to be present. 884 */ 885 public AppointmentParticipantComponent setRequired(ParticipantRequired value) { 886 if (value == null) 887 this.required = null; 888 else { 889 if (this.required == null) 890 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); 891 this.required.setValue(value); 892 } 893 return this; 894 } 895 896 /** 897 * @return {@link #status} (Participation status of the actor.). This is the 898 * underlying object with id, value and extensions. The accessor 899 * "getStatus" gives direct access to the value 900 */ 901 public Enumeration<ParticipationStatus> getStatusElement() { 902 if (this.status == null) 903 if (Configuration.errorOnAutoCreate()) 904 throw new Error("Attempt to auto-create AppointmentParticipantComponent.status"); 905 else if (Configuration.doAutoCreate()) 906 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); // bb 907 return this.status; 908 } 909 910 public boolean hasStatusElement() { 911 return this.status != null && !this.status.isEmpty(); 912 } 913 914 public boolean hasStatus() { 915 return this.status != null && !this.status.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #status} (Participation status of the actor.). This is 920 * the underlying object with id, value and extensions. The 921 * accessor "getStatus" gives direct access to the value 922 */ 923 public AppointmentParticipantComponent setStatusElement(Enumeration<ParticipationStatus> value) { 924 this.status = value; 925 return this; 926 } 927 928 /** 929 * @return Participation status of the actor. 930 */ 931 public ParticipationStatus getStatus() { 932 return this.status == null ? null : this.status.getValue(); 933 } 934 935 /** 936 * @param value Participation status of the actor. 937 */ 938 public AppointmentParticipantComponent setStatus(ParticipationStatus value) { 939 if (this.status == null) 940 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); 941 this.status.setValue(value); 942 return this; 943 } 944 945 /** 946 * @return {@link #period} (Participation period of the actor.) 947 */ 948 public Period getPeriod() { 949 if (this.period == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create AppointmentParticipantComponent.period"); 952 else if (Configuration.doAutoCreate()) 953 this.period = new Period(); // cc 954 return this.period; 955 } 956 957 public boolean hasPeriod() { 958 return this.period != null && !this.period.isEmpty(); 959 } 960 961 /** 962 * @param value {@link #period} (Participation period of the actor.) 963 */ 964 public AppointmentParticipantComponent setPeriod(Period value) { 965 this.period = value; 966 return this; 967 } 968 969 protected void listChildren(List<Property> children) { 970 super.listChildren(children); 971 children.add(new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, 972 java.lang.Integer.MAX_VALUE, type)); 973 children.add(new Property("actor", 974 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 975 "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor)); 976 children.add(new Property("required", "code", 977 "Whether this participant is required to be present at the meeting. This covers a use-case where two doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 978 0, 1, required)); 979 children.add(new Property("status", "code", "Participation status of the actor.", 0, 1, status)); 980 children.add(new Property("period", "Period", "Participation period of the actor.", 0, 1, period)); 981 } 982 983 @Override 984 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 985 switch (_hash) { 986 case 3575610: 987 /* type */ return new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, 988 java.lang.Integer.MAX_VALUE, type); 989 case 92645877: 990 /* actor */ return new Property("actor", 991 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 992 "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor); 993 case -393139297: 994 /* required */ return new Property("required", "code", 995 "Whether this participant is required to be present at the meeting. This covers a use-case where two doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 996 0, 1, required); 997 case -892481550: 998 /* status */ return new Property("status", "code", "Participation status of the actor.", 0, 1, status); 999 case -991726143: 1000 /* period */ return new Property("period", "Period", "Participation period of the actor.", 0, 1, period); 1001 default: 1002 return super.getNamedProperty(_hash, _name, _checkValid); 1003 } 1004 1005 } 1006 1007 @Override 1008 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1009 switch (hash) { 1010 case 3575610: 1011 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1012 case 92645877: 1013 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 1014 case -393139297: 1015 /* required */ return this.required == null ? new Base[0] : new Base[] { this.required }; // Enumeration<ParticipantRequired> 1016 case -892481550: 1017 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ParticipationStatus> 1018 case -991726143: 1019 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1020 default: 1021 return super.getProperty(hash, name, checkValid); 1022 } 1023 1024 } 1025 1026 @Override 1027 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1028 switch (hash) { 1029 case 3575610: // type 1030 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1031 return value; 1032 case 92645877: // actor 1033 this.actor = castToReference(value); // Reference 1034 return value; 1035 case -393139297: // required 1036 value = new ParticipantRequiredEnumFactory().fromType(castToCode(value)); 1037 this.required = (Enumeration) value; // Enumeration<ParticipantRequired> 1038 return value; 1039 case -892481550: // status 1040 value = new ParticipationStatusEnumFactory().fromType(castToCode(value)); 1041 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 1042 return value; 1043 case -991726143: // period 1044 this.period = castToPeriod(value); // Period 1045 return value; 1046 default: 1047 return super.setProperty(hash, name, value); 1048 } 1049 1050 } 1051 1052 @Override 1053 public Base setProperty(String name, Base value) throws FHIRException { 1054 if (name.equals("type")) { 1055 this.getType().add(castToCodeableConcept(value)); 1056 } else if (name.equals("actor")) { 1057 this.actor = castToReference(value); // Reference 1058 } else if (name.equals("required")) { 1059 value = new ParticipantRequiredEnumFactory().fromType(castToCode(value)); 1060 this.required = (Enumeration) value; // Enumeration<ParticipantRequired> 1061 } else if (name.equals("status")) { 1062 value = new ParticipationStatusEnumFactory().fromType(castToCode(value)); 1063 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 1064 } else if (name.equals("period")) { 1065 this.period = castToPeriod(value); // Period 1066 } else 1067 return super.setProperty(name, value); 1068 return value; 1069 } 1070 1071 @Override 1072 public void removeChild(String name, Base value) throws FHIRException { 1073 if (name.equals("type")) { 1074 this.getType().remove(castToCodeableConcept(value)); 1075 } else if (name.equals("actor")) { 1076 this.actor = null; 1077 } else if (name.equals("required")) { 1078 this.required = null; 1079 } else if (name.equals("status")) { 1080 this.status = null; 1081 } else if (name.equals("period")) { 1082 this.period = null; 1083 } else 1084 super.removeChild(name, value); 1085 1086 } 1087 1088 @Override 1089 public Base makeProperty(int hash, String name) throws FHIRException { 1090 switch (hash) { 1091 case 3575610: 1092 return addType(); 1093 case 92645877: 1094 return getActor(); 1095 case -393139297: 1096 return getRequiredElement(); 1097 case -892481550: 1098 return getStatusElement(); 1099 case -991726143: 1100 return getPeriod(); 1101 default: 1102 return super.makeProperty(hash, name); 1103 } 1104 1105 } 1106 1107 @Override 1108 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1109 switch (hash) { 1110 case 3575610: 1111 /* type */ return new String[] { "CodeableConcept" }; 1112 case 92645877: 1113 /* actor */ return new String[] { "Reference" }; 1114 case -393139297: 1115 /* required */ return new String[] { "code" }; 1116 case -892481550: 1117 /* status */ return new String[] { "code" }; 1118 case -991726143: 1119 /* period */ return new String[] { "Period" }; 1120 default: 1121 return super.getTypesForProperty(hash, name); 1122 } 1123 1124 } 1125 1126 @Override 1127 public Base addChild(String name) throws FHIRException { 1128 if (name.equals("type")) { 1129 return addType(); 1130 } else if (name.equals("actor")) { 1131 this.actor = new Reference(); 1132 return this.actor; 1133 } else if (name.equals("required")) { 1134 throw new FHIRException("Cannot call addChild on a singleton property Appointment.required"); 1135 } else if (name.equals("status")) { 1136 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 1137 } else if (name.equals("period")) { 1138 this.period = new Period(); 1139 return this.period; 1140 } else 1141 return super.addChild(name); 1142 } 1143 1144 public AppointmentParticipantComponent copy() { 1145 AppointmentParticipantComponent dst = new AppointmentParticipantComponent(); 1146 copyValues(dst); 1147 return dst; 1148 } 1149 1150 public void copyValues(AppointmentParticipantComponent dst) { 1151 super.copyValues(dst); 1152 if (type != null) { 1153 dst.type = new ArrayList<CodeableConcept>(); 1154 for (CodeableConcept i : type) 1155 dst.type.add(i.copy()); 1156 } 1157 ; 1158 dst.actor = actor == null ? null : actor.copy(); 1159 dst.required = required == null ? null : required.copy(); 1160 dst.status = status == null ? null : status.copy(); 1161 dst.period = period == null ? null : period.copy(); 1162 } 1163 1164 @Override 1165 public boolean equalsDeep(Base other_) { 1166 if (!super.equalsDeep(other_)) 1167 return false; 1168 if (!(other_ instanceof AppointmentParticipantComponent)) 1169 return false; 1170 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 1171 return compareDeep(type, o.type, true) && compareDeep(actor, o.actor, true) 1172 && compareDeep(required, o.required, true) && compareDeep(status, o.status, true) 1173 && compareDeep(period, o.period, true); 1174 } 1175 1176 @Override 1177 public boolean equalsShallow(Base other_) { 1178 if (!super.equalsShallow(other_)) 1179 return false; 1180 if (!(other_ instanceof AppointmentParticipantComponent)) 1181 return false; 1182 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 1183 return compareValues(required, o.required, true) && compareValues(status, o.status, true); 1184 } 1185 1186 public boolean isEmpty() { 1187 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, actor, required, status, period); 1188 } 1189 1190 public String fhirType() { 1191 return "Appointment.participant"; 1192 1193 } 1194 1195 } 1196 1197 /** 1198 * This records identifiers associated with this appointment concern that are 1199 * defined by business processes and/or used to refer to it when a direct URL 1200 * reference to the resource itself is not appropriate (e.g. in CDA documents, 1201 * or in written / printed documentation). 1202 */ 1203 @Child(name = "identifier", type = { 1204 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1205 @Description(shortDefinition = "External Ids for this item", formalDefinition = "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 1206 protected List<Identifier> identifier; 1207 1208 /** 1209 * The overall status of the Appointment. Each of the participants has their own 1210 * participation status which indicates their involvement in the process, 1211 * however this status indicates the shared status. 1212 */ 1213 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1214 @Description(shortDefinition = "proposed | pending | booked | arrived | fulfilled | cancelled | noshow | entered-in-error | checked-in | waitlist", formalDefinition = "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.") 1215 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/appointmentstatus") 1216 protected Enumeration<AppointmentStatus> status; 1217 1218 /** 1219 * The coded reason for the appointment being cancelled. This is often used in 1220 * reporting/billing/futher processing to determine if further actions are 1221 * required, or specific fees apply. 1222 */ 1223 @Child(name = "cancelationReason", type = { 1224 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1225 @Description(shortDefinition = "The coded reason for the appointment being cancelled", formalDefinition = "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.") 1226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/appointment-cancellation-reason") 1227 protected CodeableConcept cancelationReason; 1228 1229 /** 1230 * A broad categorization of the service that is to be performed during this 1231 * appointment. 1232 */ 1233 @Child(name = "serviceCategory", type = { 1234 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1235 @Description(shortDefinition = "A broad categorization of the service that is to be performed during this appointment", formalDefinition = "A broad categorization of the service that is to be performed during this appointment.") 1236 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 1237 protected List<CodeableConcept> serviceCategory; 1238 1239 /** 1240 * The specific service that is to be performed during this appointment. 1241 */ 1242 @Child(name = "serviceType", type = { 1243 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1244 @Description(shortDefinition = "The specific service that is to be performed during this appointment", formalDefinition = "The specific service that is to be performed during this appointment.") 1245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 1246 protected List<CodeableConcept> serviceType; 1247 1248 /** 1249 * The specialty of a practitioner that would be required to perform the service 1250 * requested in this appointment. 1251 */ 1252 @Child(name = "specialty", type = { 1253 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1254 @Description(shortDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment.") 1255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 1256 protected List<CodeableConcept> specialty; 1257 1258 /** 1259 * The style of appointment or patient that has been booked in the slot (not 1260 * service type). 1261 */ 1262 @Child(name = "appointmentType", type = { 1263 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1264 @Description(shortDefinition = "The style of appointment or patient that has been booked in the slot (not service type)", formalDefinition = "The style of appointment or patient that has been booked in the slot (not service type).") 1265 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0276") 1266 protected CodeableConcept appointmentType; 1267 1268 /** 1269 * The coded reason that this appointment is being scheduled. This is more 1270 * clinical than administrative. 1271 */ 1272 @Child(name = "reasonCode", type = { 1273 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1274 @Description(shortDefinition = "Coded reason this appointment is scheduled", formalDefinition = "The coded reason that this appointment is being scheduled. This is more clinical than administrative.") 1275 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-reason") 1276 protected List<CodeableConcept> reasonCode; 1277 1278 /** 1279 * Reason the appointment has been scheduled to take place, as specified using 1280 * information from another resource. When the patient arrives and the encounter 1281 * begins it may be used as the admission diagnosis. The indication will 1282 * typically be a Condition (with other resources referenced in the 1283 * evidence.detail), or a Procedure. 1284 */ 1285 @Child(name = "reasonReference", type = { Condition.class, Procedure.class, Observation.class, 1286 ImmunizationRecommendation.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1287 @Description(shortDefinition = "Reason the appointment is to take place (resource)", formalDefinition = "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.") 1288 protected List<Reference> reasonReference; 1289 /** 1290 * The actual objects that are the target of the reference (Reason the 1291 * appointment has been scheduled to take place, as specified using information 1292 * from another resource. When the patient arrives and the encounter begins it 1293 * may be used as the admission diagnosis. The indication will typically be a 1294 * Condition (with other resources referenced in the evidence.detail), or a 1295 * Procedure.) 1296 */ 1297 protected List<Resource> reasonReferenceTarget; 1298 1299 /** 1300 * The priority of the appointment. Can be used to make informed decisions if 1301 * needing to re-prioritize appointments. (The iCal Standard specifies 0 as 1302 * undefined, 1 as highest, 9 as lowest priority). 1303 */ 1304 @Child(name = "priority", type = { 1305 UnsignedIntType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1306 @Description(shortDefinition = "Used to make informed decisions if needing to re-prioritize", formalDefinition = "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).") 1307 protected UnsignedIntType priority; 1308 1309 /** 1310 * The brief description of the appointment as would be shown on a subject line 1311 * in a meeting request, or appointment list. Detailed or expanded information 1312 * should be put in the comment field. 1313 */ 1314 @Child(name = "description", type = { 1315 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1316 @Description(shortDefinition = "Shown on a subject line in a meeting request, or appointment list", formalDefinition = "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.") 1317 protected StringType description; 1318 1319 /** 1320 * Additional information to support the appointment provided when making the 1321 * appointment. 1322 */ 1323 @Child(name = "supportingInformation", type = { 1324 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1325 @Description(shortDefinition = "Additional information to support the appointment", formalDefinition = "Additional information to support the appointment provided when making the appointment.") 1326 protected List<Reference> supportingInformation; 1327 /** 1328 * The actual objects that are the target of the reference (Additional 1329 * information to support the appointment provided when making the appointment.) 1330 */ 1331 protected List<Resource> supportingInformationTarget; 1332 1333 /** 1334 * Date/Time that the appointment is to take place. 1335 */ 1336 @Child(name = "start", type = { InstantType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1337 @Description(shortDefinition = "When appointment is to take place", formalDefinition = "Date/Time that the appointment is to take place.") 1338 protected InstantType start; 1339 1340 /** 1341 * Date/Time that the appointment is to conclude. 1342 */ 1343 @Child(name = "end", type = { InstantType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1344 @Description(shortDefinition = "When appointment is to conclude", formalDefinition = "Date/Time that the appointment is to conclude.") 1345 protected InstantType end; 1346 1347 /** 1348 * Number of minutes that the appointment is to take. This can be less than the 1349 * duration between the start and end times. For example, where the actual time 1350 * of appointment is only an estimate or if a 30 minute appointment is being 1351 * requested, but any time would work. Also, if there is, for example, a planned 1352 * 15 minute break in the middle of a long appointment, the duration may be 15 1353 * minutes less than the difference between the start and end. 1354 */ 1355 @Child(name = "minutesDuration", type = { 1356 PositiveIntType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1357 @Description(shortDefinition = "Can be less than start/end (e.g. estimate)", formalDefinition = "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.") 1358 protected PositiveIntType minutesDuration; 1359 1360 /** 1361 * The slots from the participants' schedules that will be filled by the 1362 * appointment. 1363 */ 1364 @Child(name = "slot", type = { 1365 Slot.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1366 @Description(shortDefinition = "The slots that this appointment is filling", formalDefinition = "The slots from the participants' schedules that will be filled by the appointment.") 1367 protected List<Reference> slot; 1368 /** 1369 * The actual objects that are the target of the reference (The slots from the 1370 * participants' schedules that will be filled by the appointment.) 1371 */ 1372 protected List<Slot> slotTarget; 1373 1374 /** 1375 * The date that this appointment was initially created. This could be different 1376 * to the meta.lastModified value on the initial entry, as this could have been 1377 * before the resource was created on the FHIR server, and should remain 1378 * unchanged over the lifespan of the appointment. 1379 */ 1380 @Child(name = "created", type = { 1381 DateTimeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1382 @Description(shortDefinition = "The date that this appointment was initially created", formalDefinition = "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.") 1383 protected DateTimeType created; 1384 1385 /** 1386 * Additional comments about the appointment. 1387 */ 1388 @Child(name = "comment", type = { StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1389 @Description(shortDefinition = "Additional comments", formalDefinition = "Additional comments about the appointment.") 1390 protected StringType comment; 1391 1392 /** 1393 * While Appointment.comment contains information for internal use, 1394 * Appointment.patientInstructions is used to capture patient facing information 1395 * about the Appointment (e.g. please bring your referral or fast from 8pm night 1396 * before). 1397 */ 1398 @Child(name = "patientInstruction", type = { 1399 StringType.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 1400 @Description(shortDefinition = "Detailed information and instructions for the patient", formalDefinition = "While Appointment.comment contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).") 1401 protected StringType patientInstruction; 1402 1403 /** 1404 * The service request this appointment is allocated to assess (e.g. incoming 1405 * referral or procedure request). 1406 */ 1407 @Child(name = "basedOn", type = { 1408 ServiceRequest.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1409 @Description(shortDefinition = "The service request this appointment is allocated to assess", formalDefinition = "The service request this appointment is allocated to assess (e.g. incoming referral or procedure request).") 1410 protected List<Reference> basedOn; 1411 /** 1412 * The actual objects that are the target of the reference (The service request 1413 * this appointment is allocated to assess (e.g. incoming referral or procedure 1414 * request).) 1415 */ 1416 protected List<ServiceRequest> basedOnTarget; 1417 1418 /** 1419 * List of participants involved in the appointment. 1420 */ 1421 @Child(name = "participant", type = {}, order = 20, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1422 @Description(shortDefinition = "Participants involved in appointment", formalDefinition = "List of participants involved in the appointment.") 1423 protected List<AppointmentParticipantComponent> participant; 1424 1425 /** 1426 * A set of date ranges (potentially including times) that the appointment is 1427 * preferred to be scheduled within. 1428 * 1429 * The duration (usually in minutes) could also be provided to indicate the 1430 * length of the appointment to fill and populate the start/end times for the 1431 * actual allocated time. However, in other situations the duration may be 1432 * calculated by the scheduling system. 1433 */ 1434 @Child(name = "requestedPeriod", type = { 1435 Period.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1436 @Description(shortDefinition = "Potential date/time interval(s) requested to allocate the appointment within", formalDefinition = "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.") 1437 protected List<Period> requestedPeriod; 1438 1439 private static final long serialVersionUID = -1096822339L; 1440 1441 /** 1442 * Constructor 1443 */ 1444 public Appointment() { 1445 super(); 1446 } 1447 1448 /** 1449 * Constructor 1450 */ 1451 public Appointment(Enumeration<AppointmentStatus> status) { 1452 super(); 1453 this.status = status; 1454 } 1455 1456 /** 1457 * @return {@link #identifier} (This records identifiers associated with this 1458 * appointment concern that are defined by business processes and/or 1459 * used to refer to it when a direct URL reference to the resource 1460 * itself is not appropriate (e.g. in CDA documents, or in written / 1461 * printed documentation).) 1462 */ 1463 public List<Identifier> getIdentifier() { 1464 if (this.identifier == null) 1465 this.identifier = new ArrayList<Identifier>(); 1466 return this.identifier; 1467 } 1468 1469 /** 1470 * @return Returns a reference to <code>this</code> for easy method chaining 1471 */ 1472 public Appointment setIdentifier(List<Identifier> theIdentifier) { 1473 this.identifier = theIdentifier; 1474 return this; 1475 } 1476 1477 public boolean hasIdentifier() { 1478 if (this.identifier == null) 1479 return false; 1480 for (Identifier item : this.identifier) 1481 if (!item.isEmpty()) 1482 return true; 1483 return false; 1484 } 1485 1486 public Identifier addIdentifier() { // 3 1487 Identifier t = new Identifier(); 1488 if (this.identifier == null) 1489 this.identifier = new ArrayList<Identifier>(); 1490 this.identifier.add(t); 1491 return t; 1492 } 1493 1494 public Appointment addIdentifier(Identifier t) { // 3 1495 if (t == null) 1496 return this; 1497 if (this.identifier == null) 1498 this.identifier = new ArrayList<Identifier>(); 1499 this.identifier.add(t); 1500 return this; 1501 } 1502 1503 /** 1504 * @return The first repetition of repeating field {@link #identifier}, creating 1505 * it if it does not already exist 1506 */ 1507 public Identifier getIdentifierFirstRep() { 1508 if (getIdentifier().isEmpty()) { 1509 addIdentifier(); 1510 } 1511 return getIdentifier().get(0); 1512 } 1513 1514 /** 1515 * @return {@link #status} (The overall status of the Appointment. Each of the 1516 * participants has their own participation status which indicates their 1517 * involvement in the process, however this status indicates the shared 1518 * status.). This is the underlying object with id, value and 1519 * extensions. The accessor "getStatus" gives direct access to the value 1520 */ 1521 public Enumeration<AppointmentStatus> getStatusElement() { 1522 if (this.status == null) 1523 if (Configuration.errorOnAutoCreate()) 1524 throw new Error("Attempt to auto-create Appointment.status"); 1525 else if (Configuration.doAutoCreate()) 1526 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); // bb 1527 return this.status; 1528 } 1529 1530 public boolean hasStatusElement() { 1531 return this.status != null && !this.status.isEmpty(); 1532 } 1533 1534 public boolean hasStatus() { 1535 return this.status != null && !this.status.isEmpty(); 1536 } 1537 1538 /** 1539 * @param value {@link #status} (The overall status of the Appointment. Each of 1540 * the participants has their own participation status which 1541 * indicates their involvement in the process, however this status 1542 * indicates the shared status.). This is the underlying object 1543 * with id, value and extensions. The accessor "getStatus" gives 1544 * direct access to the value 1545 */ 1546 public Appointment setStatusElement(Enumeration<AppointmentStatus> value) { 1547 this.status = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return The overall status of the Appointment. Each of the participants has 1553 * their own participation status which indicates their involvement in 1554 * the process, however this status indicates the shared status. 1555 */ 1556 public AppointmentStatus getStatus() { 1557 return this.status == null ? null : this.status.getValue(); 1558 } 1559 1560 /** 1561 * @param value The overall status of the Appointment. Each of the participants 1562 * has their own participation status which indicates their 1563 * involvement in the process, however this status indicates the 1564 * shared status. 1565 */ 1566 public Appointment setStatus(AppointmentStatus value) { 1567 if (this.status == null) 1568 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); 1569 this.status.setValue(value); 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #cancelationReason} (The coded reason for the appointment 1575 * being cancelled. This is often used in reporting/billing/futher 1576 * processing to determine if further actions are required, or specific 1577 * fees apply.) 1578 */ 1579 public CodeableConcept getCancelationReason() { 1580 if (this.cancelationReason == null) 1581 if (Configuration.errorOnAutoCreate()) 1582 throw new Error("Attempt to auto-create Appointment.cancelationReason"); 1583 else if (Configuration.doAutoCreate()) 1584 this.cancelationReason = new CodeableConcept(); // cc 1585 return this.cancelationReason; 1586 } 1587 1588 public boolean hasCancelationReason() { 1589 return this.cancelationReason != null && !this.cancelationReason.isEmpty(); 1590 } 1591 1592 /** 1593 * @param value {@link #cancelationReason} (The coded reason for the appointment 1594 * being cancelled. This is often used in reporting/billing/futher 1595 * processing to determine if further actions are required, or 1596 * specific fees apply.) 1597 */ 1598 public Appointment setCancelationReason(CodeableConcept value) { 1599 this.cancelationReason = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return {@link #serviceCategory} (A broad categorization of the service that 1605 * is to be performed during this appointment.) 1606 */ 1607 public List<CodeableConcept> getServiceCategory() { 1608 if (this.serviceCategory == null) 1609 this.serviceCategory = new ArrayList<CodeableConcept>(); 1610 return this.serviceCategory; 1611 } 1612 1613 /** 1614 * @return Returns a reference to <code>this</code> for easy method chaining 1615 */ 1616 public Appointment setServiceCategory(List<CodeableConcept> theServiceCategory) { 1617 this.serviceCategory = theServiceCategory; 1618 return this; 1619 } 1620 1621 public boolean hasServiceCategory() { 1622 if (this.serviceCategory == null) 1623 return false; 1624 for (CodeableConcept item : this.serviceCategory) 1625 if (!item.isEmpty()) 1626 return true; 1627 return false; 1628 } 1629 1630 public CodeableConcept addServiceCategory() { // 3 1631 CodeableConcept t = new CodeableConcept(); 1632 if (this.serviceCategory == null) 1633 this.serviceCategory = new ArrayList<CodeableConcept>(); 1634 this.serviceCategory.add(t); 1635 return t; 1636 } 1637 1638 public Appointment addServiceCategory(CodeableConcept t) { // 3 1639 if (t == null) 1640 return this; 1641 if (this.serviceCategory == null) 1642 this.serviceCategory = new ArrayList<CodeableConcept>(); 1643 this.serviceCategory.add(t); 1644 return this; 1645 } 1646 1647 /** 1648 * @return The first repetition of repeating field {@link #serviceCategory}, 1649 * creating it if it does not already exist 1650 */ 1651 public CodeableConcept getServiceCategoryFirstRep() { 1652 if (getServiceCategory().isEmpty()) { 1653 addServiceCategory(); 1654 } 1655 return getServiceCategory().get(0); 1656 } 1657 1658 /** 1659 * @return {@link #serviceType} (The specific service that is to be performed 1660 * during this appointment.) 1661 */ 1662 public List<CodeableConcept> getServiceType() { 1663 if (this.serviceType == null) 1664 this.serviceType = new ArrayList<CodeableConcept>(); 1665 return this.serviceType; 1666 } 1667 1668 /** 1669 * @return Returns a reference to <code>this</code> for easy method chaining 1670 */ 1671 public Appointment setServiceType(List<CodeableConcept> theServiceType) { 1672 this.serviceType = theServiceType; 1673 return this; 1674 } 1675 1676 public boolean hasServiceType() { 1677 if (this.serviceType == null) 1678 return false; 1679 for (CodeableConcept item : this.serviceType) 1680 if (!item.isEmpty()) 1681 return true; 1682 return false; 1683 } 1684 1685 public CodeableConcept addServiceType() { // 3 1686 CodeableConcept t = new CodeableConcept(); 1687 if (this.serviceType == null) 1688 this.serviceType = new ArrayList<CodeableConcept>(); 1689 this.serviceType.add(t); 1690 return t; 1691 } 1692 1693 public Appointment addServiceType(CodeableConcept t) { // 3 1694 if (t == null) 1695 return this; 1696 if (this.serviceType == null) 1697 this.serviceType = new ArrayList<CodeableConcept>(); 1698 this.serviceType.add(t); 1699 return this; 1700 } 1701 1702 /** 1703 * @return The first repetition of repeating field {@link #serviceType}, 1704 * creating it if it does not already exist 1705 */ 1706 public CodeableConcept getServiceTypeFirstRep() { 1707 if (getServiceType().isEmpty()) { 1708 addServiceType(); 1709 } 1710 return getServiceType().get(0); 1711 } 1712 1713 /** 1714 * @return {@link #specialty} (The specialty of a practitioner that would be 1715 * required to perform the service requested in this appointment.) 1716 */ 1717 public List<CodeableConcept> getSpecialty() { 1718 if (this.specialty == null) 1719 this.specialty = new ArrayList<CodeableConcept>(); 1720 return this.specialty; 1721 } 1722 1723 /** 1724 * @return Returns a reference to <code>this</code> for easy method chaining 1725 */ 1726 public Appointment setSpecialty(List<CodeableConcept> theSpecialty) { 1727 this.specialty = theSpecialty; 1728 return this; 1729 } 1730 1731 public boolean hasSpecialty() { 1732 if (this.specialty == null) 1733 return false; 1734 for (CodeableConcept item : this.specialty) 1735 if (!item.isEmpty()) 1736 return true; 1737 return false; 1738 } 1739 1740 public CodeableConcept addSpecialty() { // 3 1741 CodeableConcept t = new CodeableConcept(); 1742 if (this.specialty == null) 1743 this.specialty = new ArrayList<CodeableConcept>(); 1744 this.specialty.add(t); 1745 return t; 1746 } 1747 1748 public Appointment addSpecialty(CodeableConcept t) { // 3 1749 if (t == null) 1750 return this; 1751 if (this.specialty == null) 1752 this.specialty = new ArrayList<CodeableConcept>(); 1753 this.specialty.add(t); 1754 return this; 1755 } 1756 1757 /** 1758 * @return The first repetition of repeating field {@link #specialty}, creating 1759 * it if it does not already exist 1760 */ 1761 public CodeableConcept getSpecialtyFirstRep() { 1762 if (getSpecialty().isEmpty()) { 1763 addSpecialty(); 1764 } 1765 return getSpecialty().get(0); 1766 } 1767 1768 /** 1769 * @return {@link #appointmentType} (The style of appointment or patient that 1770 * has been booked in the slot (not service type).) 1771 */ 1772 public CodeableConcept getAppointmentType() { 1773 if (this.appointmentType == null) 1774 if (Configuration.errorOnAutoCreate()) 1775 throw new Error("Attempt to auto-create Appointment.appointmentType"); 1776 else if (Configuration.doAutoCreate()) 1777 this.appointmentType = new CodeableConcept(); // cc 1778 return this.appointmentType; 1779 } 1780 1781 public boolean hasAppointmentType() { 1782 return this.appointmentType != null && !this.appointmentType.isEmpty(); 1783 } 1784 1785 /** 1786 * @param value {@link #appointmentType} (The style of appointment or patient 1787 * that has been booked in the slot (not service type).) 1788 */ 1789 public Appointment setAppointmentType(CodeableConcept value) { 1790 this.appointmentType = value; 1791 return this; 1792 } 1793 1794 /** 1795 * @return {@link #reasonCode} (The coded reason that this appointment is being 1796 * scheduled. This is more clinical than administrative.) 1797 */ 1798 public List<CodeableConcept> getReasonCode() { 1799 if (this.reasonCode == null) 1800 this.reasonCode = new ArrayList<CodeableConcept>(); 1801 return this.reasonCode; 1802 } 1803 1804 /** 1805 * @return Returns a reference to <code>this</code> for easy method chaining 1806 */ 1807 public Appointment setReasonCode(List<CodeableConcept> theReasonCode) { 1808 this.reasonCode = theReasonCode; 1809 return this; 1810 } 1811 1812 public boolean hasReasonCode() { 1813 if (this.reasonCode == null) 1814 return false; 1815 for (CodeableConcept item : this.reasonCode) 1816 if (!item.isEmpty()) 1817 return true; 1818 return false; 1819 } 1820 1821 public CodeableConcept addReasonCode() { // 3 1822 CodeableConcept t = new CodeableConcept(); 1823 if (this.reasonCode == null) 1824 this.reasonCode = new ArrayList<CodeableConcept>(); 1825 this.reasonCode.add(t); 1826 return t; 1827 } 1828 1829 public Appointment addReasonCode(CodeableConcept t) { // 3 1830 if (t == null) 1831 return this; 1832 if (this.reasonCode == null) 1833 this.reasonCode = new ArrayList<CodeableConcept>(); 1834 this.reasonCode.add(t); 1835 return this; 1836 } 1837 1838 /** 1839 * @return The first repetition of repeating field {@link #reasonCode}, creating 1840 * it if it does not already exist 1841 */ 1842 public CodeableConcept getReasonCodeFirstRep() { 1843 if (getReasonCode().isEmpty()) { 1844 addReasonCode(); 1845 } 1846 return getReasonCode().get(0); 1847 } 1848 1849 /** 1850 * @return {@link #reasonReference} (Reason the appointment has been scheduled 1851 * to take place, as specified using information from another resource. 1852 * When the patient arrives and the encounter begins it may be used as 1853 * the admission diagnosis. The indication will typically be a Condition 1854 * (with other resources referenced in the evidence.detail), or a 1855 * Procedure.) 1856 */ 1857 public List<Reference> getReasonReference() { 1858 if (this.reasonReference == null) 1859 this.reasonReference = new ArrayList<Reference>(); 1860 return this.reasonReference; 1861 } 1862 1863 /** 1864 * @return Returns a reference to <code>this</code> for easy method chaining 1865 */ 1866 public Appointment setReasonReference(List<Reference> theReasonReference) { 1867 this.reasonReference = theReasonReference; 1868 return this; 1869 } 1870 1871 public boolean hasReasonReference() { 1872 if (this.reasonReference == null) 1873 return false; 1874 for (Reference item : this.reasonReference) 1875 if (!item.isEmpty()) 1876 return true; 1877 return false; 1878 } 1879 1880 public Reference addReasonReference() { // 3 1881 Reference t = new Reference(); 1882 if (this.reasonReference == null) 1883 this.reasonReference = new ArrayList<Reference>(); 1884 this.reasonReference.add(t); 1885 return t; 1886 } 1887 1888 public Appointment addReasonReference(Reference t) { // 3 1889 if (t == null) 1890 return this; 1891 if (this.reasonReference == null) 1892 this.reasonReference = new ArrayList<Reference>(); 1893 this.reasonReference.add(t); 1894 return this; 1895 } 1896 1897 /** 1898 * @return The first repetition of repeating field {@link #reasonReference}, 1899 * creating it if it does not already exist 1900 */ 1901 public Reference getReasonReferenceFirstRep() { 1902 if (getReasonReference().isEmpty()) { 1903 addReasonReference(); 1904 } 1905 return getReasonReference().get(0); 1906 } 1907 1908 /** 1909 * @deprecated Use Reference#setResource(IBaseResource) instead 1910 */ 1911 @Deprecated 1912 public List<Resource> getReasonReferenceTarget() { 1913 if (this.reasonReferenceTarget == null) 1914 this.reasonReferenceTarget = new ArrayList<Resource>(); 1915 return this.reasonReferenceTarget; 1916 } 1917 1918 /** 1919 * @return {@link #priority} (The priority of the appointment. Can be used to 1920 * make informed decisions if needing to re-prioritize appointments. 1921 * (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as 1922 * lowest priority).). This is the underlying object with id, value and 1923 * extensions. The accessor "getPriority" gives direct access to the 1924 * value 1925 */ 1926 public UnsignedIntType getPriorityElement() { 1927 if (this.priority == null) 1928 if (Configuration.errorOnAutoCreate()) 1929 throw new Error("Attempt to auto-create Appointment.priority"); 1930 else if (Configuration.doAutoCreate()) 1931 this.priority = new UnsignedIntType(); // bb 1932 return this.priority; 1933 } 1934 1935 public boolean hasPriorityElement() { 1936 return this.priority != null && !this.priority.isEmpty(); 1937 } 1938 1939 public boolean hasPriority() { 1940 return this.priority != null && !this.priority.isEmpty(); 1941 } 1942 1943 /** 1944 * @param value {@link #priority} (The priority of the appointment. Can be used 1945 * to make informed decisions if needing to re-prioritize 1946 * appointments. (The iCal Standard specifies 0 as undefined, 1 as 1947 * highest, 9 as lowest priority).). This is the underlying object 1948 * with id, value and extensions. The accessor "getPriority" gives 1949 * direct access to the value 1950 */ 1951 public Appointment setPriorityElement(UnsignedIntType value) { 1952 this.priority = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return The priority of the appointment. Can be used to make informed 1958 * decisions if needing to re-prioritize appointments. (The iCal 1959 * Standard specifies 0 as undefined, 1 as highest, 9 as lowest 1960 * priority). 1961 */ 1962 public int getPriority() { 1963 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 1964 } 1965 1966 /** 1967 * @param value The priority of the appointment. Can be used to make informed 1968 * decisions if needing to re-prioritize appointments. (The iCal 1969 * Standard specifies 0 as undefined, 1 as highest, 9 as lowest 1970 * priority). 1971 */ 1972 public Appointment setPriority(int value) { 1973 if (this.priority == null) 1974 this.priority = new UnsignedIntType(); 1975 this.priority.setValue(value); 1976 return this; 1977 } 1978 1979 /** 1980 * @return {@link #description} (The brief description of the appointment as 1981 * would be shown on a subject line in a meeting request, or appointment 1982 * list. Detailed or expanded information should be put in the comment 1983 * field.). This is the underlying object with id, value and extensions. 1984 * The accessor "getDescription" gives direct access to the value 1985 */ 1986 public StringType getDescriptionElement() { 1987 if (this.description == null) 1988 if (Configuration.errorOnAutoCreate()) 1989 throw new Error("Attempt to auto-create Appointment.description"); 1990 else if (Configuration.doAutoCreate()) 1991 this.description = new StringType(); // bb 1992 return this.description; 1993 } 1994 1995 public boolean hasDescriptionElement() { 1996 return this.description != null && !this.description.isEmpty(); 1997 } 1998 1999 public boolean hasDescription() { 2000 return this.description != null && !this.description.isEmpty(); 2001 } 2002 2003 /** 2004 * @param value {@link #description} (The brief description of the appointment 2005 * as would be shown on a subject line in a meeting request, or 2006 * appointment list. Detailed or expanded information should be put 2007 * in the comment field.). This is the underlying object with id, 2008 * value and extensions. The accessor "getDescription" gives direct 2009 * access to the value 2010 */ 2011 public Appointment setDescriptionElement(StringType value) { 2012 this.description = value; 2013 return this; 2014 } 2015 2016 /** 2017 * @return The brief description of the appointment as would be shown on a 2018 * subject line in a meeting request, or appointment list. Detailed or 2019 * expanded information should be put in the comment field. 2020 */ 2021 public String getDescription() { 2022 return this.description == null ? null : this.description.getValue(); 2023 } 2024 2025 /** 2026 * @param value The brief description of the appointment as would be shown on a 2027 * subject line in a meeting request, or appointment list. Detailed 2028 * or expanded information should be put in the comment field. 2029 */ 2030 public Appointment setDescription(String value) { 2031 if (Utilities.noString(value)) 2032 this.description = null; 2033 else { 2034 if (this.description == null) 2035 this.description = new StringType(); 2036 this.description.setValue(value); 2037 } 2038 return this; 2039 } 2040 2041 /** 2042 * @return {@link #supportingInformation} (Additional information to support the 2043 * appointment provided when making the appointment.) 2044 */ 2045 public List<Reference> getSupportingInformation() { 2046 if (this.supportingInformation == null) 2047 this.supportingInformation = new ArrayList<Reference>(); 2048 return this.supportingInformation; 2049 } 2050 2051 /** 2052 * @return Returns a reference to <code>this</code> for easy method chaining 2053 */ 2054 public Appointment setSupportingInformation(List<Reference> theSupportingInformation) { 2055 this.supportingInformation = theSupportingInformation; 2056 return this; 2057 } 2058 2059 public boolean hasSupportingInformation() { 2060 if (this.supportingInformation == null) 2061 return false; 2062 for (Reference item : this.supportingInformation) 2063 if (!item.isEmpty()) 2064 return true; 2065 return false; 2066 } 2067 2068 public Reference addSupportingInformation() { // 3 2069 Reference t = new Reference(); 2070 if (this.supportingInformation == null) 2071 this.supportingInformation = new ArrayList<Reference>(); 2072 this.supportingInformation.add(t); 2073 return t; 2074 } 2075 2076 public Appointment addSupportingInformation(Reference t) { // 3 2077 if (t == null) 2078 return this; 2079 if (this.supportingInformation == null) 2080 this.supportingInformation = new ArrayList<Reference>(); 2081 this.supportingInformation.add(t); 2082 return this; 2083 } 2084 2085 /** 2086 * @return The first repetition of repeating field 2087 * {@link #supportingInformation}, creating it if it does not already 2088 * exist 2089 */ 2090 public Reference getSupportingInformationFirstRep() { 2091 if (getSupportingInformation().isEmpty()) { 2092 addSupportingInformation(); 2093 } 2094 return getSupportingInformation().get(0); 2095 } 2096 2097 /** 2098 * @deprecated Use Reference#setResource(IBaseResource) instead 2099 */ 2100 @Deprecated 2101 public List<Resource> getSupportingInformationTarget() { 2102 if (this.supportingInformationTarget == null) 2103 this.supportingInformationTarget = new ArrayList<Resource>(); 2104 return this.supportingInformationTarget; 2105 } 2106 2107 /** 2108 * @return {@link #start} (Date/Time that the appointment is to take place.). 2109 * This is the underlying object with id, value and extensions. The 2110 * accessor "getStart" gives direct access to the value 2111 */ 2112 public InstantType getStartElement() { 2113 if (this.start == null) 2114 if (Configuration.errorOnAutoCreate()) 2115 throw new Error("Attempt to auto-create Appointment.start"); 2116 else if (Configuration.doAutoCreate()) 2117 this.start = new InstantType(); // bb 2118 return this.start; 2119 } 2120 2121 public boolean hasStartElement() { 2122 return this.start != null && !this.start.isEmpty(); 2123 } 2124 2125 public boolean hasStart() { 2126 return this.start != null && !this.start.isEmpty(); 2127 } 2128 2129 /** 2130 * @param value {@link #start} (Date/Time that the appointment is to take 2131 * place.). This is the underlying object with id, value and 2132 * extensions. The accessor "getStart" gives direct access to the 2133 * value 2134 */ 2135 public Appointment setStartElement(InstantType value) { 2136 this.start = value; 2137 return this; 2138 } 2139 2140 /** 2141 * @return Date/Time that the appointment is to take place. 2142 */ 2143 public Date getStart() { 2144 return this.start == null ? null : this.start.getValue(); 2145 } 2146 2147 /** 2148 * @param value Date/Time that the appointment is to take place. 2149 */ 2150 public Appointment setStart(Date value) { 2151 if (value == null) 2152 this.start = null; 2153 else { 2154 if (this.start == null) 2155 this.start = new InstantType(); 2156 this.start.setValue(value); 2157 } 2158 return this; 2159 } 2160 2161 /** 2162 * @return {@link #end} (Date/Time that the appointment is to conclude.). This 2163 * is the underlying object with id, value and extensions. The accessor 2164 * "getEnd" gives direct access to the value 2165 */ 2166 public InstantType getEndElement() { 2167 if (this.end == null) 2168 if (Configuration.errorOnAutoCreate()) 2169 throw new Error("Attempt to auto-create Appointment.end"); 2170 else if (Configuration.doAutoCreate()) 2171 this.end = new InstantType(); // bb 2172 return this.end; 2173 } 2174 2175 public boolean hasEndElement() { 2176 return this.end != null && !this.end.isEmpty(); 2177 } 2178 2179 public boolean hasEnd() { 2180 return this.end != null && !this.end.isEmpty(); 2181 } 2182 2183 /** 2184 * @param value {@link #end} (Date/Time that the appointment is to conclude.). 2185 * This is the underlying object with id, value and extensions. The 2186 * accessor "getEnd" gives direct access to the value 2187 */ 2188 public Appointment setEndElement(InstantType value) { 2189 this.end = value; 2190 return this; 2191 } 2192 2193 /** 2194 * @return Date/Time that the appointment is to conclude. 2195 */ 2196 public Date getEnd() { 2197 return this.end == null ? null : this.end.getValue(); 2198 } 2199 2200 /** 2201 * @param value Date/Time that the appointment is to conclude. 2202 */ 2203 public Appointment setEnd(Date value) { 2204 if (value == null) 2205 this.end = null; 2206 else { 2207 if (this.end == null) 2208 this.end = new InstantType(); 2209 this.end.setValue(value); 2210 } 2211 return this; 2212 } 2213 2214 /** 2215 * @return {@link #minutesDuration} (Number of minutes that the appointment is 2216 * to take. This can be less than the duration between the start and end 2217 * times. For example, where the actual time of appointment is only an 2218 * estimate or if a 30 minute appointment is being requested, but any 2219 * time would work. Also, if there is, for example, a planned 15 minute 2220 * break in the middle of a long appointment, the duration may be 15 2221 * minutes less than the difference between the start and end.). This is 2222 * the underlying object with id, value and extensions. The accessor 2223 * "getMinutesDuration" gives direct access to the value 2224 */ 2225 public PositiveIntType getMinutesDurationElement() { 2226 if (this.minutesDuration == null) 2227 if (Configuration.errorOnAutoCreate()) 2228 throw new Error("Attempt to auto-create Appointment.minutesDuration"); 2229 else if (Configuration.doAutoCreate()) 2230 this.minutesDuration = new PositiveIntType(); // bb 2231 return this.minutesDuration; 2232 } 2233 2234 public boolean hasMinutesDurationElement() { 2235 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 2236 } 2237 2238 public boolean hasMinutesDuration() { 2239 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 2240 } 2241 2242 /** 2243 * @param value {@link #minutesDuration} (Number of minutes that the appointment 2244 * is to take. This can be less than the duration between the start 2245 * and end times. For example, where the actual time of appointment 2246 * is only an estimate or if a 30 minute appointment is being 2247 * requested, but any time would work. Also, if there is, for 2248 * example, a planned 15 minute break in the middle of a long 2249 * appointment, the duration may be 15 minutes less than the 2250 * difference between the start and end.). This is the underlying 2251 * object with id, value and extensions. The accessor 2252 * "getMinutesDuration" gives direct access to the value 2253 */ 2254 public Appointment setMinutesDurationElement(PositiveIntType value) { 2255 this.minutesDuration = value; 2256 return this; 2257 } 2258 2259 /** 2260 * @return Number of minutes that the appointment is to take. This can be less 2261 * than the duration between the start and end times. For example, where 2262 * the actual time of appointment is only an estimate or if a 30 minute 2263 * appointment is being requested, but any time would work. Also, if 2264 * there is, for example, a planned 15 minute break in the middle of a 2265 * long appointment, the duration may be 15 minutes less than the 2266 * difference between the start and end. 2267 */ 2268 public int getMinutesDuration() { 2269 return this.minutesDuration == null || this.minutesDuration.isEmpty() ? 0 : this.minutesDuration.getValue(); 2270 } 2271 2272 /** 2273 * @param value Number of minutes that the appointment is to take. This can be 2274 * less than the duration between the start and end times. For 2275 * example, where the actual time of appointment is only an 2276 * estimate or if a 30 minute appointment is being requested, but 2277 * any time would work. Also, if there is, for example, a planned 2278 * 15 minute break in the middle of a long appointment, the 2279 * duration may be 15 minutes less than the difference between the 2280 * start and end. 2281 */ 2282 public Appointment setMinutesDuration(int value) { 2283 if (this.minutesDuration == null) 2284 this.minutesDuration = new PositiveIntType(); 2285 this.minutesDuration.setValue(value); 2286 return this; 2287 } 2288 2289 /** 2290 * @return {@link #slot} (The slots from the participants' schedules that will 2291 * be filled by the appointment.) 2292 */ 2293 public List<Reference> getSlot() { 2294 if (this.slot == null) 2295 this.slot = new ArrayList<Reference>(); 2296 return this.slot; 2297 } 2298 2299 /** 2300 * @return Returns a reference to <code>this</code> for easy method chaining 2301 */ 2302 public Appointment setSlot(List<Reference> theSlot) { 2303 this.slot = theSlot; 2304 return this; 2305 } 2306 2307 public boolean hasSlot() { 2308 if (this.slot == null) 2309 return false; 2310 for (Reference item : this.slot) 2311 if (!item.isEmpty()) 2312 return true; 2313 return false; 2314 } 2315 2316 public Reference addSlot() { // 3 2317 Reference t = new Reference(); 2318 if (this.slot == null) 2319 this.slot = new ArrayList<Reference>(); 2320 this.slot.add(t); 2321 return t; 2322 } 2323 2324 public Appointment addSlot(Reference t) { // 3 2325 if (t == null) 2326 return this; 2327 if (this.slot == null) 2328 this.slot = new ArrayList<Reference>(); 2329 this.slot.add(t); 2330 return this; 2331 } 2332 2333 /** 2334 * @return The first repetition of repeating field {@link #slot}, creating it if 2335 * it does not already exist 2336 */ 2337 public Reference getSlotFirstRep() { 2338 if (getSlot().isEmpty()) { 2339 addSlot(); 2340 } 2341 return getSlot().get(0); 2342 } 2343 2344 /** 2345 * @deprecated Use Reference#setResource(IBaseResource) instead 2346 */ 2347 @Deprecated 2348 public List<Slot> getSlotTarget() { 2349 if (this.slotTarget == null) 2350 this.slotTarget = new ArrayList<Slot>(); 2351 return this.slotTarget; 2352 } 2353 2354 /** 2355 * @deprecated Use Reference#setResource(IBaseResource) instead 2356 */ 2357 @Deprecated 2358 public Slot addSlotTarget() { 2359 Slot r = new Slot(); 2360 if (this.slotTarget == null) 2361 this.slotTarget = new ArrayList<Slot>(); 2362 this.slotTarget.add(r); 2363 return r; 2364 } 2365 2366 /** 2367 * @return {@link #created} (The date that this appointment was initially 2368 * created. This could be different to the meta.lastModified value on 2369 * the initial entry, as this could have been before the resource was 2370 * created on the FHIR server, and should remain unchanged over the 2371 * lifespan of the appointment.). This is the underlying object with id, 2372 * value and extensions. The accessor "getCreated" gives direct access 2373 * to the value 2374 */ 2375 public DateTimeType getCreatedElement() { 2376 if (this.created == null) 2377 if (Configuration.errorOnAutoCreate()) 2378 throw new Error("Attempt to auto-create Appointment.created"); 2379 else if (Configuration.doAutoCreate()) 2380 this.created = new DateTimeType(); // bb 2381 return this.created; 2382 } 2383 2384 public boolean hasCreatedElement() { 2385 return this.created != null && !this.created.isEmpty(); 2386 } 2387 2388 public boolean hasCreated() { 2389 return this.created != null && !this.created.isEmpty(); 2390 } 2391 2392 /** 2393 * @param value {@link #created} (The date that this appointment was initially 2394 * created. This could be different to the meta.lastModified value 2395 * on the initial entry, as this could have been before the 2396 * resource was created on the FHIR server, and should remain 2397 * unchanged over the lifespan of the appointment.). This is the 2398 * underlying object with id, value and extensions. The accessor 2399 * "getCreated" gives direct access to the value 2400 */ 2401 public Appointment setCreatedElement(DateTimeType value) { 2402 this.created = value; 2403 return this; 2404 } 2405 2406 /** 2407 * @return The date that this appointment was initially created. This could be 2408 * different to the meta.lastModified value on the initial entry, as 2409 * this could have been before the resource was created on the FHIR 2410 * server, and should remain unchanged over the lifespan of the 2411 * appointment. 2412 */ 2413 public Date getCreated() { 2414 return this.created == null ? null : this.created.getValue(); 2415 } 2416 2417 /** 2418 * @param value The date that this appointment was initially created. This could 2419 * be different to the meta.lastModified value on the initial 2420 * entry, as this could have been before the resource was created 2421 * on the FHIR server, and should remain unchanged over the 2422 * lifespan of the appointment. 2423 */ 2424 public Appointment setCreated(Date value) { 2425 if (value == null) 2426 this.created = null; 2427 else { 2428 if (this.created == null) 2429 this.created = new DateTimeType(); 2430 this.created.setValue(value); 2431 } 2432 return this; 2433 } 2434 2435 /** 2436 * @return {@link #comment} (Additional comments about the appointment.). This 2437 * is the underlying object with id, value and extensions. The accessor 2438 * "getComment" gives direct access to the value 2439 */ 2440 public StringType getCommentElement() { 2441 if (this.comment == null) 2442 if (Configuration.errorOnAutoCreate()) 2443 throw new Error("Attempt to auto-create Appointment.comment"); 2444 else if (Configuration.doAutoCreate()) 2445 this.comment = new StringType(); // bb 2446 return this.comment; 2447 } 2448 2449 public boolean hasCommentElement() { 2450 return this.comment != null && !this.comment.isEmpty(); 2451 } 2452 2453 public boolean hasComment() { 2454 return this.comment != null && !this.comment.isEmpty(); 2455 } 2456 2457 /** 2458 * @param value {@link #comment} (Additional comments about the appointment.). 2459 * This is the underlying object with id, value and extensions. The 2460 * accessor "getComment" gives direct access to the value 2461 */ 2462 public Appointment setCommentElement(StringType value) { 2463 this.comment = value; 2464 return this; 2465 } 2466 2467 /** 2468 * @return Additional comments about the appointment. 2469 */ 2470 public String getComment() { 2471 return this.comment == null ? null : this.comment.getValue(); 2472 } 2473 2474 /** 2475 * @param value Additional comments about the appointment. 2476 */ 2477 public Appointment setComment(String value) { 2478 if (Utilities.noString(value)) 2479 this.comment = null; 2480 else { 2481 if (this.comment == null) 2482 this.comment = new StringType(); 2483 this.comment.setValue(value); 2484 } 2485 return this; 2486 } 2487 2488 /** 2489 * @return {@link #patientInstruction} (While Appointment.comment contains 2490 * information for internal use, Appointment.patientInstructions is used 2491 * to capture patient facing information about the Appointment (e.g. 2492 * please bring your referral or fast from 8pm night before).). This is 2493 * the underlying object with id, value and extensions. The accessor 2494 * "getPatientInstruction" gives direct access to the value 2495 */ 2496 public StringType getPatientInstructionElement() { 2497 if (this.patientInstruction == null) 2498 if (Configuration.errorOnAutoCreate()) 2499 throw new Error("Attempt to auto-create Appointment.patientInstruction"); 2500 else if (Configuration.doAutoCreate()) 2501 this.patientInstruction = new StringType(); // bb 2502 return this.patientInstruction; 2503 } 2504 2505 public boolean hasPatientInstructionElement() { 2506 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 2507 } 2508 2509 public boolean hasPatientInstruction() { 2510 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 2511 } 2512 2513 /** 2514 * @param value {@link #patientInstruction} (While Appointment.comment contains 2515 * information for internal use, Appointment.patientInstructions is 2516 * used to capture patient facing information about the Appointment 2517 * (e.g. please bring your referral or fast from 8pm night 2518 * before).). This is the underlying object with id, value and 2519 * extensions. The accessor "getPatientInstruction" gives direct 2520 * access to the value 2521 */ 2522 public Appointment setPatientInstructionElement(StringType value) { 2523 this.patientInstruction = value; 2524 return this; 2525 } 2526 2527 /** 2528 * @return While Appointment.comment contains information for internal use, 2529 * Appointment.patientInstructions is used to capture patient facing 2530 * information about the Appointment (e.g. please bring your referral or 2531 * fast from 8pm night before). 2532 */ 2533 public String getPatientInstruction() { 2534 return this.patientInstruction == null ? null : this.patientInstruction.getValue(); 2535 } 2536 2537 /** 2538 * @param value While Appointment.comment contains information for internal use, 2539 * Appointment.patientInstructions is used to capture patient 2540 * facing information about the Appointment (e.g. please bring your 2541 * referral or fast from 8pm night before). 2542 */ 2543 public Appointment setPatientInstruction(String value) { 2544 if (Utilities.noString(value)) 2545 this.patientInstruction = null; 2546 else { 2547 if (this.patientInstruction == null) 2548 this.patientInstruction = new StringType(); 2549 this.patientInstruction.setValue(value); 2550 } 2551 return this; 2552 } 2553 2554 /** 2555 * @return {@link #basedOn} (The service request this appointment is allocated 2556 * to assess (e.g. incoming referral or procedure request).) 2557 */ 2558 public List<Reference> getBasedOn() { 2559 if (this.basedOn == null) 2560 this.basedOn = new ArrayList<Reference>(); 2561 return this.basedOn; 2562 } 2563 2564 /** 2565 * @return Returns a reference to <code>this</code> for easy method chaining 2566 */ 2567 public Appointment setBasedOn(List<Reference> theBasedOn) { 2568 this.basedOn = theBasedOn; 2569 return this; 2570 } 2571 2572 public boolean hasBasedOn() { 2573 if (this.basedOn == null) 2574 return false; 2575 for (Reference item : this.basedOn) 2576 if (!item.isEmpty()) 2577 return true; 2578 return false; 2579 } 2580 2581 public Reference addBasedOn() { // 3 2582 Reference t = new Reference(); 2583 if (this.basedOn == null) 2584 this.basedOn = new ArrayList<Reference>(); 2585 this.basedOn.add(t); 2586 return t; 2587 } 2588 2589 public Appointment addBasedOn(Reference t) { // 3 2590 if (t == null) 2591 return this; 2592 if (this.basedOn == null) 2593 this.basedOn = new ArrayList<Reference>(); 2594 this.basedOn.add(t); 2595 return this; 2596 } 2597 2598 /** 2599 * @return The first repetition of repeating field {@link #basedOn}, creating it 2600 * if it does not already exist 2601 */ 2602 public Reference getBasedOnFirstRep() { 2603 if (getBasedOn().isEmpty()) { 2604 addBasedOn(); 2605 } 2606 return getBasedOn().get(0); 2607 } 2608 2609 /** 2610 * @deprecated Use Reference#setResource(IBaseResource) instead 2611 */ 2612 @Deprecated 2613 public List<ServiceRequest> getBasedOnTarget() { 2614 if (this.basedOnTarget == null) 2615 this.basedOnTarget = new ArrayList<ServiceRequest>(); 2616 return this.basedOnTarget; 2617 } 2618 2619 /** 2620 * @deprecated Use Reference#setResource(IBaseResource) instead 2621 */ 2622 @Deprecated 2623 public ServiceRequest addBasedOnTarget() { 2624 ServiceRequest r = new ServiceRequest(); 2625 if (this.basedOnTarget == null) 2626 this.basedOnTarget = new ArrayList<ServiceRequest>(); 2627 this.basedOnTarget.add(r); 2628 return r; 2629 } 2630 2631 /** 2632 * @return {@link #participant} (List of participants involved in the 2633 * appointment.) 2634 */ 2635 public List<AppointmentParticipantComponent> getParticipant() { 2636 if (this.participant == null) 2637 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2638 return this.participant; 2639 } 2640 2641 /** 2642 * @return Returns a reference to <code>this</code> for easy method chaining 2643 */ 2644 public Appointment setParticipant(List<AppointmentParticipantComponent> theParticipant) { 2645 this.participant = theParticipant; 2646 return this; 2647 } 2648 2649 public boolean hasParticipant() { 2650 if (this.participant == null) 2651 return false; 2652 for (AppointmentParticipantComponent item : this.participant) 2653 if (!item.isEmpty()) 2654 return true; 2655 return false; 2656 } 2657 2658 public AppointmentParticipantComponent addParticipant() { // 3 2659 AppointmentParticipantComponent t = new AppointmentParticipantComponent(); 2660 if (this.participant == null) 2661 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2662 this.participant.add(t); 2663 return t; 2664 } 2665 2666 public Appointment addParticipant(AppointmentParticipantComponent t) { // 3 2667 if (t == null) 2668 return this; 2669 if (this.participant == null) 2670 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2671 this.participant.add(t); 2672 return this; 2673 } 2674 2675 /** 2676 * @return The first repetition of repeating field {@link #participant}, 2677 * creating it if it does not already exist 2678 */ 2679 public AppointmentParticipantComponent getParticipantFirstRep() { 2680 if (getParticipant().isEmpty()) { 2681 addParticipant(); 2682 } 2683 return getParticipant().get(0); 2684 } 2685 2686 /** 2687 * @return {@link #requestedPeriod} (A set of date ranges (potentially including 2688 * times) that the appointment is preferred to be scheduled within. 2689 * 2690 * The duration (usually in minutes) could also be provided to indicate 2691 * the length of the appointment to fill and populate the start/end 2692 * times for the actual allocated time. However, in other situations the 2693 * duration may be calculated by the scheduling system.) 2694 */ 2695 public List<Period> getRequestedPeriod() { 2696 if (this.requestedPeriod == null) 2697 this.requestedPeriod = new ArrayList<Period>(); 2698 return this.requestedPeriod; 2699 } 2700 2701 /** 2702 * @return Returns a reference to <code>this</code> for easy method chaining 2703 */ 2704 public Appointment setRequestedPeriod(List<Period> theRequestedPeriod) { 2705 this.requestedPeriod = theRequestedPeriod; 2706 return this; 2707 } 2708 2709 public boolean hasRequestedPeriod() { 2710 if (this.requestedPeriod == null) 2711 return false; 2712 for (Period item : this.requestedPeriod) 2713 if (!item.isEmpty()) 2714 return true; 2715 return false; 2716 } 2717 2718 public Period addRequestedPeriod() { // 3 2719 Period t = new Period(); 2720 if (this.requestedPeriod == null) 2721 this.requestedPeriod = new ArrayList<Period>(); 2722 this.requestedPeriod.add(t); 2723 return t; 2724 } 2725 2726 public Appointment addRequestedPeriod(Period t) { // 3 2727 if (t == null) 2728 return this; 2729 if (this.requestedPeriod == null) 2730 this.requestedPeriod = new ArrayList<Period>(); 2731 this.requestedPeriod.add(t); 2732 return this; 2733 } 2734 2735 /** 2736 * @return The first repetition of repeating field {@link #requestedPeriod}, 2737 * creating it if it does not already exist 2738 */ 2739 public Period getRequestedPeriodFirstRep() { 2740 if (getRequestedPeriod().isEmpty()) { 2741 addRequestedPeriod(); 2742 } 2743 return getRequestedPeriod().get(0); 2744 } 2745 2746 protected void listChildren(List<Property> children) { 2747 super.listChildren(children); 2748 children.add(new Property("identifier", "Identifier", 2749 "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2750 0, java.lang.Integer.MAX_VALUE, identifier)); 2751 children.add(new Property("status", "code", 2752 "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 2753 0, 1, status)); 2754 children.add(new Property("cancelationReason", "CodeableConcept", 2755 "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.", 2756 0, 1, cancelationReason)); 2757 children.add(new Property("serviceCategory", "CodeableConcept", 2758 "A broad categorization of the service that is to be performed during this appointment.", 0, 2759 java.lang.Integer.MAX_VALUE, serviceCategory)); 2760 children.add(new Property("serviceType", "CodeableConcept", 2761 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 2762 serviceType)); 2763 children.add(new Property("specialty", "CodeableConcept", 2764 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 2765 0, java.lang.Integer.MAX_VALUE, specialty)); 2766 children.add(new Property("appointmentType", "CodeableConcept", 2767 "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, 2768 appointmentType)); 2769 children.add(new Property("reasonCode", "CodeableConcept", 2770 "The coded reason that this appointment is being scheduled. This is more clinical than administrative.", 0, 2771 java.lang.Integer.MAX_VALUE, reasonCode)); 2772 children.add(new Property("reasonReference", 2773 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 2774 "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 2775 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2776 children.add(new Property("priority", "unsignedInt", 2777 "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 2778 0, 1, priority)); 2779 children.add(new Property("description", "string", 2780 "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 2781 0, 1, description)); 2782 children.add(new Property("supportingInformation", "Reference(Any)", 2783 "Additional information to support the appointment provided when making the appointment.", 0, 2784 java.lang.Integer.MAX_VALUE, supportingInformation)); 2785 children.add(new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, start)); 2786 children.add(new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end)); 2787 children.add(new Property("minutesDuration", "positiveInt", 2788 "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.", 2789 0, 1, minutesDuration)); 2790 children.add(new Property("slot", "Reference(Slot)", 2791 "The slots from the participants' schedules that will be filled by the appointment.", 0, 2792 java.lang.Integer.MAX_VALUE, slot)); 2793 children.add(new Property("created", "dateTime", 2794 "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 2795 0, 1, created)); 2796 children.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment)); 2797 children.add(new Property("patientInstruction", "string", 2798 "While Appointment.comment contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).", 2799 0, 1, patientInstruction)); 2800 children.add(new Property("basedOn", "Reference(ServiceRequest)", 2801 "The service request this appointment is allocated to assess (e.g. incoming referral or procedure request).", 0, 2802 java.lang.Integer.MAX_VALUE, basedOn)); 2803 children.add(new Property("participant", "", "List of participants involved in the appointment.", 0, 2804 java.lang.Integer.MAX_VALUE, participant)); 2805 children.add(new Property("requestedPeriod", "Period", 2806 "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.", 2807 0, java.lang.Integer.MAX_VALUE, requestedPeriod)); 2808 } 2809 2810 @Override 2811 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2812 switch (_hash) { 2813 case -1618432855: 2814 /* identifier */ return new Property("identifier", "Identifier", 2815 "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2816 0, java.lang.Integer.MAX_VALUE, identifier); 2817 case -892481550: 2818 /* status */ return new Property("status", "code", 2819 "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 2820 0, 1, status); 2821 case 987811551: 2822 /* cancelationReason */ return new Property("cancelationReason", "CodeableConcept", 2823 "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.", 2824 0, 1, cancelationReason); 2825 case 1281188563: 2826 /* serviceCategory */ return new Property("serviceCategory", "CodeableConcept", 2827 "A broad categorization of the service that is to be performed during this appointment.", 0, 2828 java.lang.Integer.MAX_VALUE, serviceCategory); 2829 case -1928370289: 2830 /* serviceType */ return new Property("serviceType", "CodeableConcept", 2831 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 2832 serviceType); 2833 case -1694759682: 2834 /* specialty */ return new Property("specialty", "CodeableConcept", 2835 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 2836 0, java.lang.Integer.MAX_VALUE, specialty); 2837 case -1596426375: 2838 /* appointmentType */ return new Property("appointmentType", "CodeableConcept", 2839 "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, 2840 appointmentType); 2841 case 722137681: 2842 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2843 "The coded reason that this appointment is being scheduled. This is more clinical than administrative.", 0, 2844 java.lang.Integer.MAX_VALUE, reasonCode); 2845 case -1146218137: 2846 /* reasonReference */ return new Property("reasonReference", 2847 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 2848 "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 2849 0, java.lang.Integer.MAX_VALUE, reasonReference); 2850 case -1165461084: 2851 /* priority */ return new Property("priority", "unsignedInt", 2852 "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 2853 0, 1, priority); 2854 case -1724546052: 2855 /* description */ return new Property("description", "string", 2856 "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 2857 0, 1, description); 2858 case -1248768647: 2859 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2860 "Additional information to support the appointment provided when making the appointment.", 0, 2861 java.lang.Integer.MAX_VALUE, supportingInformation); 2862 case 109757538: 2863 /* start */ return new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, 2864 start); 2865 case 100571: 2866 /* end */ return new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end); 2867 case -413630573: 2868 /* minutesDuration */ return new Property("minutesDuration", "positiveInt", 2869 "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.", 2870 0, 1, minutesDuration); 2871 case 3533310: 2872 /* slot */ return new Property("slot", "Reference(Slot)", 2873 "The slots from the participants' schedules that will be filled by the appointment.", 0, 2874 java.lang.Integer.MAX_VALUE, slot); 2875 case 1028554472: 2876 /* created */ return new Property("created", "dateTime", 2877 "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 2878 0, 1, created); 2879 case 950398559: 2880 /* comment */ return new Property("comment", "string", "Additional comments about the appointment.", 0, 1, 2881 comment); 2882 case 737543241: 2883 /* patientInstruction */ return new Property("patientInstruction", "string", 2884 "While Appointment.comment contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).", 2885 0, 1, patientInstruction); 2886 case -332612366: 2887 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest)", 2888 "The service request this appointment is allocated to assess (e.g. incoming referral or procedure request).", 2889 0, java.lang.Integer.MAX_VALUE, basedOn); 2890 case 767422259: 2891 /* participant */ return new Property("participant", "", "List of participants involved in the appointment.", 0, 2892 java.lang.Integer.MAX_VALUE, participant); 2893 case -897241393: 2894 /* requestedPeriod */ return new Property("requestedPeriod", "Period", 2895 "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.", 2896 0, java.lang.Integer.MAX_VALUE, requestedPeriod); 2897 default: 2898 return super.getNamedProperty(_hash, _name, _checkValid); 2899 } 2900 2901 } 2902 2903 @Override 2904 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2905 switch (hash) { 2906 case -1618432855: 2907 /* identifier */ return this.identifier == null ? new Base[0] 2908 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2909 case -892481550: 2910 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<AppointmentStatus> 2911 case 987811551: 2912 /* cancelationReason */ return this.cancelationReason == null ? new Base[0] 2913 : new Base[] { this.cancelationReason }; // CodeableConcept 2914 case 1281188563: 2915 /* serviceCategory */ return this.serviceCategory == null ? new Base[0] 2916 : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 2917 case -1928370289: 2918 /* serviceType */ return this.serviceType == null ? new Base[0] 2919 : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 2920 case -1694759682: 2921 /* specialty */ return this.specialty == null ? new Base[0] 2922 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 2923 case -1596426375: 2924 /* appointmentType */ return this.appointmentType == null ? new Base[0] : new Base[] { this.appointmentType }; // CodeableConcept 2925 case 722137681: 2926 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2927 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2928 case -1146218137: 2929 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2930 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2931 case -1165461084: 2932 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // UnsignedIntType 2933 case -1724546052: 2934 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2935 case -1248768647: 2936 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2937 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2938 case 109757538: 2939 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // InstantType 2940 case 100571: 2941 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // InstantType 2942 case -413630573: 2943 /* minutesDuration */ return this.minutesDuration == null ? new Base[0] : new Base[] { this.minutesDuration }; // PositiveIntType 2944 case 3533310: 2945 /* slot */ return this.slot == null ? new Base[0] : this.slot.toArray(new Base[this.slot.size()]); // Reference 2946 case 1028554472: 2947 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 2948 case 950398559: 2949 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2950 case 737543241: 2951 /* patientInstruction */ return this.patientInstruction == null ? new Base[0] 2952 : new Base[] { this.patientInstruction }; // StringType 2953 case -332612366: 2954 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2955 case 767422259: 2956 /* participant */ return this.participant == null ? new Base[0] 2957 : this.participant.toArray(new Base[this.participant.size()]); // AppointmentParticipantComponent 2958 case -897241393: 2959 /* requestedPeriod */ return this.requestedPeriod == null ? new Base[0] 2960 : this.requestedPeriod.toArray(new Base[this.requestedPeriod.size()]); // Period 2961 default: 2962 return super.getProperty(hash, name, checkValid); 2963 } 2964 2965 } 2966 2967 @Override 2968 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2969 switch (hash) { 2970 case -1618432855: // identifier 2971 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2972 return value; 2973 case -892481550: // status 2974 value = new AppointmentStatusEnumFactory().fromType(castToCode(value)); 2975 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 2976 return value; 2977 case 987811551: // cancelationReason 2978 this.cancelationReason = castToCodeableConcept(value); // CodeableConcept 2979 return value; 2980 case 1281188563: // serviceCategory 2981 this.getServiceCategory().add(castToCodeableConcept(value)); // CodeableConcept 2982 return value; 2983 case -1928370289: // serviceType 2984 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 2985 return value; 2986 case -1694759682: // specialty 2987 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 2988 return value; 2989 case -1596426375: // appointmentType 2990 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 2991 return value; 2992 case 722137681: // reasonCode 2993 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2994 return value; 2995 case -1146218137: // reasonReference 2996 this.getReasonReference().add(castToReference(value)); // Reference 2997 return value; 2998 case -1165461084: // priority 2999 this.priority = castToUnsignedInt(value); // UnsignedIntType 3000 return value; 3001 case -1724546052: // description 3002 this.description = castToString(value); // StringType 3003 return value; 3004 case -1248768647: // supportingInformation 3005 this.getSupportingInformation().add(castToReference(value)); // Reference 3006 return value; 3007 case 109757538: // start 3008 this.start = castToInstant(value); // InstantType 3009 return value; 3010 case 100571: // end 3011 this.end = castToInstant(value); // InstantType 3012 return value; 3013 case -413630573: // minutesDuration 3014 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 3015 return value; 3016 case 3533310: // slot 3017 this.getSlot().add(castToReference(value)); // Reference 3018 return value; 3019 case 1028554472: // created 3020 this.created = castToDateTime(value); // DateTimeType 3021 return value; 3022 case 950398559: // comment 3023 this.comment = castToString(value); // StringType 3024 return value; 3025 case 737543241: // patientInstruction 3026 this.patientInstruction = castToString(value); // StringType 3027 return value; 3028 case -332612366: // basedOn 3029 this.getBasedOn().add(castToReference(value)); // Reference 3030 return value; 3031 case 767422259: // participant 3032 this.getParticipant().add((AppointmentParticipantComponent) value); // AppointmentParticipantComponent 3033 return value; 3034 case -897241393: // requestedPeriod 3035 this.getRequestedPeriod().add(castToPeriod(value)); // Period 3036 return value; 3037 default: 3038 return super.setProperty(hash, name, value); 3039 } 3040 3041 } 3042 3043 @Override 3044 public Base setProperty(String name, Base value) throws FHIRException { 3045 if (name.equals("identifier")) { 3046 this.getIdentifier().add(castToIdentifier(value)); 3047 } else if (name.equals("status")) { 3048 value = new AppointmentStatusEnumFactory().fromType(castToCode(value)); 3049 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 3050 } else if (name.equals("cancelationReason")) { 3051 this.cancelationReason = castToCodeableConcept(value); // CodeableConcept 3052 } else if (name.equals("serviceCategory")) { 3053 this.getServiceCategory().add(castToCodeableConcept(value)); 3054 } else if (name.equals("serviceType")) { 3055 this.getServiceType().add(castToCodeableConcept(value)); 3056 } else if (name.equals("specialty")) { 3057 this.getSpecialty().add(castToCodeableConcept(value)); 3058 } else if (name.equals("appointmentType")) { 3059 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 3060 } else if (name.equals("reasonCode")) { 3061 this.getReasonCode().add(castToCodeableConcept(value)); 3062 } else if (name.equals("reasonReference")) { 3063 this.getReasonReference().add(castToReference(value)); 3064 } else if (name.equals("priority")) { 3065 this.priority = castToUnsignedInt(value); // UnsignedIntType 3066 } else if (name.equals("description")) { 3067 this.description = castToString(value); // StringType 3068 } else if (name.equals("supportingInformation")) { 3069 this.getSupportingInformation().add(castToReference(value)); 3070 } else if (name.equals("start")) { 3071 this.start = castToInstant(value); // InstantType 3072 } else if (name.equals("end")) { 3073 this.end = castToInstant(value); // InstantType 3074 } else if (name.equals("minutesDuration")) { 3075 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 3076 } else if (name.equals("slot")) { 3077 this.getSlot().add(castToReference(value)); 3078 } else if (name.equals("created")) { 3079 this.created = castToDateTime(value); // DateTimeType 3080 } else if (name.equals("comment")) { 3081 this.comment = castToString(value); // StringType 3082 } else if (name.equals("patientInstruction")) { 3083 this.patientInstruction = castToString(value); // StringType 3084 } else if (name.equals("basedOn")) { 3085 this.getBasedOn().add(castToReference(value)); 3086 } else if (name.equals("participant")) { 3087 this.getParticipant().add((AppointmentParticipantComponent) value); 3088 } else if (name.equals("requestedPeriod")) { 3089 this.getRequestedPeriod().add(castToPeriod(value)); 3090 } else 3091 return super.setProperty(name, value); 3092 return value; 3093 } 3094 3095 @Override 3096 public void removeChild(String name, Base value) throws FHIRException { 3097 if (name.equals("identifier")) { 3098 this.getIdentifier().remove(castToIdentifier(value)); 3099 } else if (name.equals("status")) { 3100 this.status = null; 3101 } else if (name.equals("cancelationReason")) { 3102 this.cancelationReason = null; 3103 } else if (name.equals("serviceCategory")) { 3104 this.getServiceCategory().remove(castToCodeableConcept(value)); 3105 } else if (name.equals("serviceType")) { 3106 this.getServiceType().remove(castToCodeableConcept(value)); 3107 } else if (name.equals("specialty")) { 3108 this.getSpecialty().remove(castToCodeableConcept(value)); 3109 } else if (name.equals("appointmentType")) { 3110 this.appointmentType = null; 3111 } else if (name.equals("reasonCode")) { 3112 this.getReasonCode().remove(castToCodeableConcept(value)); 3113 } else if (name.equals("reasonReference")) { 3114 this.getReasonReference().remove(castToReference(value)); 3115 } else if (name.equals("priority")) { 3116 this.priority = null; 3117 } else if (name.equals("description")) { 3118 this.description = null; 3119 } else if (name.equals("supportingInformation")) { 3120 this.getSupportingInformation().remove(castToReference(value)); 3121 } else if (name.equals("start")) { 3122 this.start = null; 3123 } else if (name.equals("end")) { 3124 this.end = null; 3125 } else if (name.equals("minutesDuration")) { 3126 this.minutesDuration = null; 3127 } else if (name.equals("slot")) { 3128 this.getSlot().remove(castToReference(value)); 3129 } else if (name.equals("created")) { 3130 this.created = null; 3131 } else if (name.equals("comment")) { 3132 this.comment = null; 3133 } else if (name.equals("patientInstruction")) { 3134 this.patientInstruction = null; 3135 } else if (name.equals("basedOn")) { 3136 this.getBasedOn().remove(castToReference(value)); 3137 } else if (name.equals("participant")) { 3138 this.getParticipant().remove((AppointmentParticipantComponent) value); 3139 } else if (name.equals("requestedPeriod")) { 3140 this.getRequestedPeriod().remove(castToPeriod(value)); 3141 } else 3142 super.removeChild(name, value); 3143 3144 } 3145 3146 @Override 3147 public Base makeProperty(int hash, String name) throws FHIRException { 3148 switch (hash) { 3149 case -1618432855: 3150 return addIdentifier(); 3151 case -892481550: 3152 return getStatusElement(); 3153 case 987811551: 3154 return getCancelationReason(); 3155 case 1281188563: 3156 return addServiceCategory(); 3157 case -1928370289: 3158 return addServiceType(); 3159 case -1694759682: 3160 return addSpecialty(); 3161 case -1596426375: 3162 return getAppointmentType(); 3163 case 722137681: 3164 return addReasonCode(); 3165 case -1146218137: 3166 return addReasonReference(); 3167 case -1165461084: 3168 return getPriorityElement(); 3169 case -1724546052: 3170 return getDescriptionElement(); 3171 case -1248768647: 3172 return addSupportingInformation(); 3173 case 109757538: 3174 return getStartElement(); 3175 case 100571: 3176 return getEndElement(); 3177 case -413630573: 3178 return getMinutesDurationElement(); 3179 case 3533310: 3180 return addSlot(); 3181 case 1028554472: 3182 return getCreatedElement(); 3183 case 950398559: 3184 return getCommentElement(); 3185 case 737543241: 3186 return getPatientInstructionElement(); 3187 case -332612366: 3188 return addBasedOn(); 3189 case 767422259: 3190 return addParticipant(); 3191 case -897241393: 3192 return addRequestedPeriod(); 3193 default: 3194 return super.makeProperty(hash, name); 3195 } 3196 3197 } 3198 3199 @Override 3200 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3201 switch (hash) { 3202 case -1618432855: 3203 /* identifier */ return new String[] { "Identifier" }; 3204 case -892481550: 3205 /* status */ return new String[] { "code" }; 3206 case 987811551: 3207 /* cancelationReason */ return new String[] { "CodeableConcept" }; 3208 case 1281188563: 3209 /* serviceCategory */ return new String[] { "CodeableConcept" }; 3210 case -1928370289: 3211 /* serviceType */ return new String[] { "CodeableConcept" }; 3212 case -1694759682: 3213 /* specialty */ return new String[] { "CodeableConcept" }; 3214 case -1596426375: 3215 /* appointmentType */ return new String[] { "CodeableConcept" }; 3216 case 722137681: 3217 /* reasonCode */ return new String[] { "CodeableConcept" }; 3218 case -1146218137: 3219 /* reasonReference */ return new String[] { "Reference" }; 3220 case -1165461084: 3221 /* priority */ return new String[] { "unsignedInt" }; 3222 case -1724546052: 3223 /* description */ return new String[] { "string" }; 3224 case -1248768647: 3225 /* supportingInformation */ return new String[] { "Reference" }; 3226 case 109757538: 3227 /* start */ return new String[] { "instant" }; 3228 case 100571: 3229 /* end */ return new String[] { "instant" }; 3230 case -413630573: 3231 /* minutesDuration */ return new String[] { "positiveInt" }; 3232 case 3533310: 3233 /* slot */ return new String[] { "Reference" }; 3234 case 1028554472: 3235 /* created */ return new String[] { "dateTime" }; 3236 case 950398559: 3237 /* comment */ return new String[] { "string" }; 3238 case 737543241: 3239 /* patientInstruction */ return new String[] { "string" }; 3240 case -332612366: 3241 /* basedOn */ return new String[] { "Reference" }; 3242 case 767422259: 3243 /* participant */ return new String[] {}; 3244 case -897241393: 3245 /* requestedPeriod */ return new String[] { "Period" }; 3246 default: 3247 return super.getTypesForProperty(hash, name); 3248 } 3249 3250 } 3251 3252 @Override 3253 public Base addChild(String name) throws FHIRException { 3254 if (name.equals("identifier")) { 3255 return addIdentifier(); 3256 } else if (name.equals("status")) { 3257 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 3258 } else if (name.equals("cancelationReason")) { 3259 this.cancelationReason = new CodeableConcept(); 3260 return this.cancelationReason; 3261 } else if (name.equals("serviceCategory")) { 3262 return addServiceCategory(); 3263 } else if (name.equals("serviceType")) { 3264 return addServiceType(); 3265 } else if (name.equals("specialty")) { 3266 return addSpecialty(); 3267 } else if (name.equals("appointmentType")) { 3268 this.appointmentType = new CodeableConcept(); 3269 return this.appointmentType; 3270 } else if (name.equals("reasonCode")) { 3271 return addReasonCode(); 3272 } else if (name.equals("reasonReference")) { 3273 return addReasonReference(); 3274 } else if (name.equals("priority")) { 3275 throw new FHIRException("Cannot call addChild on a singleton property Appointment.priority"); 3276 } else if (name.equals("description")) { 3277 throw new FHIRException("Cannot call addChild on a singleton property Appointment.description"); 3278 } else if (name.equals("supportingInformation")) { 3279 return addSupportingInformation(); 3280 } else if (name.equals("start")) { 3281 throw new FHIRException("Cannot call addChild on a singleton property Appointment.start"); 3282 } else if (name.equals("end")) { 3283 throw new FHIRException("Cannot call addChild on a singleton property Appointment.end"); 3284 } else if (name.equals("minutesDuration")) { 3285 throw new FHIRException("Cannot call addChild on a singleton property Appointment.minutesDuration"); 3286 } else if (name.equals("slot")) { 3287 return addSlot(); 3288 } else if (name.equals("created")) { 3289 throw new FHIRException("Cannot call addChild on a singleton property Appointment.created"); 3290 } else if (name.equals("comment")) { 3291 throw new FHIRException("Cannot call addChild on a singleton property Appointment.comment"); 3292 } else if (name.equals("patientInstruction")) { 3293 throw new FHIRException("Cannot call addChild on a singleton property Appointment.patientInstruction"); 3294 } else if (name.equals("basedOn")) { 3295 return addBasedOn(); 3296 } else if (name.equals("participant")) { 3297 return addParticipant(); 3298 } else if (name.equals("requestedPeriod")) { 3299 return addRequestedPeriod(); 3300 } else 3301 return super.addChild(name); 3302 } 3303 3304 public String fhirType() { 3305 return "Appointment"; 3306 3307 } 3308 3309 public Appointment copy() { 3310 Appointment dst = new Appointment(); 3311 copyValues(dst); 3312 return dst; 3313 } 3314 3315 public void copyValues(Appointment dst) { 3316 super.copyValues(dst); 3317 if (identifier != null) { 3318 dst.identifier = new ArrayList<Identifier>(); 3319 for (Identifier i : identifier) 3320 dst.identifier.add(i.copy()); 3321 } 3322 ; 3323 dst.status = status == null ? null : status.copy(); 3324 dst.cancelationReason = cancelationReason == null ? null : cancelationReason.copy(); 3325 if (serviceCategory != null) { 3326 dst.serviceCategory = new ArrayList<CodeableConcept>(); 3327 for (CodeableConcept i : serviceCategory) 3328 dst.serviceCategory.add(i.copy()); 3329 } 3330 ; 3331 if (serviceType != null) { 3332 dst.serviceType = new ArrayList<CodeableConcept>(); 3333 for (CodeableConcept i : serviceType) 3334 dst.serviceType.add(i.copy()); 3335 } 3336 ; 3337 if (specialty != null) { 3338 dst.specialty = new ArrayList<CodeableConcept>(); 3339 for (CodeableConcept i : specialty) 3340 dst.specialty.add(i.copy()); 3341 } 3342 ; 3343 dst.appointmentType = appointmentType == null ? null : appointmentType.copy(); 3344 if (reasonCode != null) { 3345 dst.reasonCode = new ArrayList<CodeableConcept>(); 3346 for (CodeableConcept i : reasonCode) 3347 dst.reasonCode.add(i.copy()); 3348 } 3349 ; 3350 if (reasonReference != null) { 3351 dst.reasonReference = new ArrayList<Reference>(); 3352 for (Reference i : reasonReference) 3353 dst.reasonReference.add(i.copy()); 3354 } 3355 ; 3356 dst.priority = priority == null ? null : priority.copy(); 3357 dst.description = description == null ? null : description.copy(); 3358 if (supportingInformation != null) { 3359 dst.supportingInformation = new ArrayList<Reference>(); 3360 for (Reference i : supportingInformation) 3361 dst.supportingInformation.add(i.copy()); 3362 } 3363 ; 3364 dst.start = start == null ? null : start.copy(); 3365 dst.end = end == null ? null : end.copy(); 3366 dst.minutesDuration = minutesDuration == null ? null : minutesDuration.copy(); 3367 if (slot != null) { 3368 dst.slot = new ArrayList<Reference>(); 3369 for (Reference i : slot) 3370 dst.slot.add(i.copy()); 3371 } 3372 ; 3373 dst.created = created == null ? null : created.copy(); 3374 dst.comment = comment == null ? null : comment.copy(); 3375 dst.patientInstruction = patientInstruction == null ? null : patientInstruction.copy(); 3376 if (basedOn != null) { 3377 dst.basedOn = new ArrayList<Reference>(); 3378 for (Reference i : basedOn) 3379 dst.basedOn.add(i.copy()); 3380 } 3381 ; 3382 if (participant != null) { 3383 dst.participant = new ArrayList<AppointmentParticipantComponent>(); 3384 for (AppointmentParticipantComponent i : participant) 3385 dst.participant.add(i.copy()); 3386 } 3387 ; 3388 if (requestedPeriod != null) { 3389 dst.requestedPeriod = new ArrayList<Period>(); 3390 for (Period i : requestedPeriod) 3391 dst.requestedPeriod.add(i.copy()); 3392 } 3393 ; 3394 } 3395 3396 protected Appointment typedCopy() { 3397 return copy(); 3398 } 3399 3400 @Override 3401 public boolean equalsDeep(Base other_) { 3402 if (!super.equalsDeep(other_)) 3403 return false; 3404 if (!(other_ instanceof Appointment)) 3405 return false; 3406 Appointment o = (Appointment) other_; 3407 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 3408 && compareDeep(cancelationReason, o.cancelationReason, true) 3409 && compareDeep(serviceCategory, o.serviceCategory, true) && compareDeep(serviceType, o.serviceType, true) 3410 && compareDeep(specialty, o.specialty, true) && compareDeep(appointmentType, o.appointmentType, true) 3411 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3412 && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) 3413 && compareDeep(supportingInformation, o.supportingInformation, true) && compareDeep(start, o.start, true) 3414 && compareDeep(end, o.end, true) && compareDeep(minutesDuration, o.minutesDuration, true) 3415 && compareDeep(slot, o.slot, true) && compareDeep(created, o.created, true) 3416 && compareDeep(comment, o.comment, true) && compareDeep(patientInstruction, o.patientInstruction, true) 3417 && compareDeep(basedOn, o.basedOn, true) && compareDeep(participant, o.participant, true) 3418 && compareDeep(requestedPeriod, o.requestedPeriod, true); 3419 } 3420 3421 @Override 3422 public boolean equalsShallow(Base other_) { 3423 if (!super.equalsShallow(other_)) 3424 return false; 3425 if (!(other_ instanceof Appointment)) 3426 return false; 3427 Appointment o = (Appointment) other_; 3428 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 3429 && compareValues(description, o.description, true) && compareValues(start, o.start, true) 3430 && compareValues(end, o.end, true) && compareValues(minutesDuration, o.minutesDuration, true) 3431 && compareValues(created, o.created, true) && compareValues(comment, o.comment, true) 3432 && compareValues(patientInstruction, o.patientInstruction, true); 3433 } 3434 3435 public boolean isEmpty() { 3436 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, cancelationReason, 3437 serviceCategory, serviceType, specialty, appointmentType, reasonCode, reasonReference, priority, description, 3438 supportingInformation, start, end, minutesDuration, slot, created, comment, patientInstruction, basedOn, 3439 participant, requestedPeriod); 3440 } 3441 3442 @Override 3443 public ResourceType getResourceType() { 3444 return ResourceType.Appointment; 3445 } 3446 3447 /** 3448 * Search parameter: <b>date</b> 3449 * <p> 3450 * Description: <b>Appointment date/time.</b><br> 3451 * Type: <b>date</b><br> 3452 * Path: <b>Appointment.start</b><br> 3453 * </p> 3454 */ 3455 @SearchParamDefinition(name = "date", path = "Appointment.start", description = "Appointment date/time.", type = "date") 3456 public static final String SP_DATE = "date"; 3457 /** 3458 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3459 * <p> 3460 * Description: <b>Appointment date/time.</b><br> 3461 * Type: <b>date</b><br> 3462 * Path: <b>Appointment.start</b><br> 3463 * </p> 3464 */ 3465 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3466 SP_DATE); 3467 3468 /** 3469 * Search parameter: <b>identifier</b> 3470 * <p> 3471 * Description: <b>An Identifier of the Appointment</b><br> 3472 * Type: <b>token</b><br> 3473 * Path: <b>Appointment.identifier</b><br> 3474 * </p> 3475 */ 3476 @SearchParamDefinition(name = "identifier", path = "Appointment.identifier", description = "An Identifier of the Appointment", type = "token") 3477 public static final String SP_IDENTIFIER = "identifier"; 3478 /** 3479 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3480 * <p> 3481 * Description: <b>An Identifier of the Appointment</b><br> 3482 * Type: <b>token</b><br> 3483 * Path: <b>Appointment.identifier</b><br> 3484 * </p> 3485 */ 3486 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3487 SP_IDENTIFIER); 3488 3489 /** 3490 * Search parameter: <b>specialty</b> 3491 * <p> 3492 * Description: <b>The specialty of a practitioner that would be required to 3493 * perform the service requested in this appointment</b><br> 3494 * Type: <b>token</b><br> 3495 * Path: <b>Appointment.specialty</b><br> 3496 * </p> 3497 */ 3498 @SearchParamDefinition(name = "specialty", path = "Appointment.specialty", description = "The specialty of a practitioner that would be required to perform the service requested in this appointment", type = "token") 3499 public static final String SP_SPECIALTY = "specialty"; 3500 /** 3501 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 3502 * <p> 3503 * Description: <b>The specialty of a practitioner that would be required to 3504 * perform the service requested in this appointment</b><br> 3505 * Type: <b>token</b><br> 3506 * Path: <b>Appointment.specialty</b><br> 3507 * </p> 3508 */ 3509 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3510 SP_SPECIALTY); 3511 3512 /** 3513 * Search parameter: <b>service-category</b> 3514 * <p> 3515 * Description: <b>A broad categorization of the service that is to be performed 3516 * during this appointment</b><br> 3517 * Type: <b>token</b><br> 3518 * Path: <b>Appointment.serviceCategory</b><br> 3519 * </p> 3520 */ 3521 @SearchParamDefinition(name = "service-category", path = "Appointment.serviceCategory", description = "A broad categorization of the service that is to be performed during this appointment", type = "token") 3522 public static final String SP_SERVICE_CATEGORY = "service-category"; 3523 /** 3524 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 3525 * <p> 3526 * Description: <b>A broad categorization of the service that is to be performed 3527 * during this appointment</b><br> 3528 * Type: <b>token</b><br> 3529 * Path: <b>Appointment.serviceCategory</b><br> 3530 * </p> 3531 */ 3532 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3533 SP_SERVICE_CATEGORY); 3534 3535 /** 3536 * Search parameter: <b>practitioner</b> 3537 * <p> 3538 * Description: <b>One of the individuals of the appointment is this 3539 * practitioner</b><br> 3540 * Type: <b>reference</b><br> 3541 * Path: <b>Appointment.participant.actor</b><br> 3542 * </p> 3543 */ 3544 @SearchParamDefinition(name = "practitioner", path = "Appointment.participant.actor.where(resolve() is Practitioner)", description = "One of the individuals of the appointment is this practitioner", type = "reference", target = { 3545 Practitioner.class }) 3546 public static final String SP_PRACTITIONER = "practitioner"; 3547 /** 3548 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 3549 * <p> 3550 * Description: <b>One of the individuals of the appointment is this 3551 * practitioner</b><br> 3552 * Type: <b>reference</b><br> 3553 * Path: <b>Appointment.participant.actor</b><br> 3554 * </p> 3555 */ 3556 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3557 SP_PRACTITIONER); 3558 3559 /** 3560 * Constant for fluent queries to be used to add include statements. Specifies 3561 * the path value of "<b>Appointment:practitioner</b>". 3562 */ 3563 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 3564 "Appointment:practitioner").toLocked(); 3565 3566 /** 3567 * Search parameter: <b>part-status</b> 3568 * <p> 3569 * Description: <b>The Participation status of the subject, or other participant 3570 * on the appointment. Can be used to locate participants that have not 3571 * responded to meeting requests.</b><br> 3572 * Type: <b>token</b><br> 3573 * Path: <b>Appointment.participant.status</b><br> 3574 * </p> 3575 */ 3576 @SearchParamDefinition(name = "part-status", path = "Appointment.participant.status", description = "The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.", type = "token") 3577 public static final String SP_PART_STATUS = "part-status"; 3578 /** 3579 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 3580 * <p> 3581 * Description: <b>The Participation status of the subject, or other participant 3582 * on the appointment. Can be used to locate participants that have not 3583 * responded to meeting requests.</b><br> 3584 * Type: <b>token</b><br> 3585 * Path: <b>Appointment.participant.status</b><br> 3586 * </p> 3587 */ 3588 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3589 SP_PART_STATUS); 3590 3591 /** 3592 * Search parameter: <b>appointment-type</b> 3593 * <p> 3594 * Description: <b>The style of appointment or patient that has been booked in 3595 * the slot (not service type)</b><br> 3596 * Type: <b>token</b><br> 3597 * Path: <b>Appointment.appointmentType</b><br> 3598 * </p> 3599 */ 3600 @SearchParamDefinition(name = "appointment-type", path = "Appointment.appointmentType", description = "The style of appointment or patient that has been booked in the slot (not service type)", type = "token") 3601 public static final String SP_APPOINTMENT_TYPE = "appointment-type"; 3602 /** 3603 * <b>Fluent Client</b> search parameter constant for <b>appointment-type</b> 3604 * <p> 3605 * Description: <b>The style of appointment or patient that has been booked in 3606 * the slot (not service type)</b><br> 3607 * Type: <b>token</b><br> 3608 * Path: <b>Appointment.appointmentType</b><br> 3609 * </p> 3610 */ 3611 public static final ca.uhn.fhir.rest.gclient.TokenClientParam APPOINTMENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3612 SP_APPOINTMENT_TYPE); 3613 3614 /** 3615 * Search parameter: <b>service-type</b> 3616 * <p> 3617 * Description: <b>The specific service that is to be performed during this 3618 * appointment</b><br> 3619 * Type: <b>token</b><br> 3620 * Path: <b>Appointment.serviceType</b><br> 3621 * </p> 3622 */ 3623 @SearchParamDefinition(name = "service-type", path = "Appointment.serviceType", description = "The specific service that is to be performed during this appointment", type = "token") 3624 public static final String SP_SERVICE_TYPE = "service-type"; 3625 /** 3626 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 3627 * <p> 3628 * Description: <b>The specific service that is to be performed during this 3629 * appointment</b><br> 3630 * Type: <b>token</b><br> 3631 * Path: <b>Appointment.serviceType</b><br> 3632 * </p> 3633 */ 3634 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3635 SP_SERVICE_TYPE); 3636 3637 /** 3638 * Search parameter: <b>slot</b> 3639 * <p> 3640 * Description: <b>The slots that this appointment is filling</b><br> 3641 * Type: <b>reference</b><br> 3642 * Path: <b>Appointment.slot</b><br> 3643 * </p> 3644 */ 3645 @SearchParamDefinition(name = "slot", path = "Appointment.slot", description = "The slots that this appointment is filling", type = "reference", target = { 3646 Slot.class }) 3647 public static final String SP_SLOT = "slot"; 3648 /** 3649 * <b>Fluent Client</b> search parameter constant for <b>slot</b> 3650 * <p> 3651 * Description: <b>The slots that this appointment is filling</b><br> 3652 * Type: <b>reference</b><br> 3653 * Path: <b>Appointment.slot</b><br> 3654 * </p> 3655 */ 3656 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SLOT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3657 SP_SLOT); 3658 3659 /** 3660 * Constant for fluent queries to be used to add include statements. Specifies 3661 * the path value of "<b>Appointment:slot</b>". 3662 */ 3663 public static final ca.uhn.fhir.model.api.Include INCLUDE_SLOT = new ca.uhn.fhir.model.api.Include("Appointment:slot") 3664 .toLocked(); 3665 3666 /** 3667 * Search parameter: <b>reason-code</b> 3668 * <p> 3669 * Description: <b>Coded reason this appointment is scheduled</b><br> 3670 * Type: <b>token</b><br> 3671 * Path: <b>Appointment.reasonCode</b><br> 3672 * </p> 3673 */ 3674 @SearchParamDefinition(name = "reason-code", path = "Appointment.reasonCode", description = "Coded reason this appointment is scheduled", type = "token") 3675 public static final String SP_REASON_CODE = "reason-code"; 3676 /** 3677 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 3678 * <p> 3679 * Description: <b>Coded reason this appointment is scheduled</b><br> 3680 * Type: <b>token</b><br> 3681 * Path: <b>Appointment.reasonCode</b><br> 3682 * </p> 3683 */ 3684 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3685 SP_REASON_CODE); 3686 3687 /** 3688 * Search parameter: <b>actor</b> 3689 * <p> 3690 * Description: <b>Any one of the individuals participating in the 3691 * appointment</b><br> 3692 * Type: <b>reference</b><br> 3693 * Path: <b>Appointment.participant.actor</b><br> 3694 * </p> 3695 */ 3696 @SearchParamDefinition(name = "actor", path = "Appointment.participant.actor", description = "Any one of the individuals participating in the appointment", type = "reference", providesMembershipIn = { 3697 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3698 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3699 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3700 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3701 HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, 3702 RelatedPerson.class }) 3703 public static final String SP_ACTOR = "actor"; 3704 /** 3705 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 3706 * <p> 3707 * Description: <b>Any one of the individuals participating in the 3708 * appointment</b><br> 3709 * Type: <b>reference</b><br> 3710 * Path: <b>Appointment.participant.actor</b><br> 3711 * </p> 3712 */ 3713 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3714 SP_ACTOR); 3715 3716 /** 3717 * Constant for fluent queries to be used to add include statements. Specifies 3718 * the path value of "<b>Appointment:actor</b>". 3719 */ 3720 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include( 3721 "Appointment:actor").toLocked(); 3722 3723 /** 3724 * Search parameter: <b>based-on</b> 3725 * <p> 3726 * Description: <b>The service request this appointment is allocated to 3727 * assess</b><br> 3728 * Type: <b>reference</b><br> 3729 * Path: <b>Appointment.basedOn</b><br> 3730 * </p> 3731 */ 3732 @SearchParamDefinition(name = "based-on", path = "Appointment.basedOn", description = "The service request this appointment is allocated to assess", type = "reference", target = { 3733 ServiceRequest.class }) 3734 public static final String SP_BASED_ON = "based-on"; 3735 /** 3736 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3737 * <p> 3738 * Description: <b>The service request this appointment is allocated to 3739 * assess</b><br> 3740 * Type: <b>reference</b><br> 3741 * Path: <b>Appointment.basedOn</b><br> 3742 * </p> 3743 */ 3744 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3745 SP_BASED_ON); 3746 3747 /** 3748 * Constant for fluent queries to be used to add include statements. Specifies 3749 * the path value of "<b>Appointment:based-on</b>". 3750 */ 3751 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3752 "Appointment:based-on").toLocked(); 3753 3754 /** 3755 * Search parameter: <b>patient</b> 3756 * <p> 3757 * Description: <b>One of the individuals of the appointment is this 3758 * patient</b><br> 3759 * Type: <b>reference</b><br> 3760 * Path: <b>Appointment.participant.actor</b><br> 3761 * </p> 3762 */ 3763 @SearchParamDefinition(name = "patient", path = "Appointment.participant.actor.where(resolve() is Patient)", description = "One of the individuals of the appointment is this patient", type = "reference", target = { 3764 Patient.class }) 3765 public static final String SP_PATIENT = "patient"; 3766 /** 3767 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3768 * <p> 3769 * Description: <b>One of the individuals of the appointment is this 3770 * patient</b><br> 3771 * Type: <b>reference</b><br> 3772 * Path: <b>Appointment.participant.actor</b><br> 3773 * </p> 3774 */ 3775 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3776 SP_PATIENT); 3777 3778 /** 3779 * Constant for fluent queries to be used to add include statements. Specifies 3780 * the path value of "<b>Appointment:patient</b>". 3781 */ 3782 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3783 "Appointment:patient").toLocked(); 3784 3785 /** 3786 * Search parameter: <b>reason-reference</b> 3787 * <p> 3788 * Description: <b>Reason the appointment is to take place (resource)</b><br> 3789 * Type: <b>reference</b><br> 3790 * Path: <b>Appointment.reasonReference</b><br> 3791 * </p> 3792 */ 3793 @SearchParamDefinition(name = "reason-reference", path = "Appointment.reasonReference", description = "Reason the appointment is to take place (resource)", type = "reference", target = { 3794 Condition.class, ImmunizationRecommendation.class, Observation.class, Procedure.class }) 3795 public static final String SP_REASON_REFERENCE = "reason-reference"; 3796 /** 3797 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 3798 * <p> 3799 * Description: <b>Reason the appointment is to take place (resource)</b><br> 3800 * Type: <b>reference</b><br> 3801 * Path: <b>Appointment.reasonReference</b><br> 3802 * </p> 3803 */ 3804 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3805 SP_REASON_REFERENCE); 3806 3807 /** 3808 * Constant for fluent queries to be used to add include statements. Specifies 3809 * the path value of "<b>Appointment:reason-reference</b>". 3810 */ 3811 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 3812 "Appointment:reason-reference").toLocked(); 3813 3814 /** 3815 * Search parameter: <b>supporting-info</b> 3816 * <p> 3817 * Description: <b>Additional information to support the appointment</b><br> 3818 * Type: <b>reference</b><br> 3819 * Path: <b>Appointment.supportingInformation</b><br> 3820 * </p> 3821 */ 3822 @SearchParamDefinition(name = "supporting-info", path = "Appointment.supportingInformation", description = "Additional information to support the appointment", type = "reference") 3823 public static final String SP_SUPPORTING_INFO = "supporting-info"; 3824 /** 3825 * <b>Fluent Client</b> search parameter constant for <b>supporting-info</b> 3826 * <p> 3827 * Description: <b>Additional information to support the appointment</b><br> 3828 * Type: <b>reference</b><br> 3829 * Path: <b>Appointment.supportingInformation</b><br> 3830 * </p> 3831 */ 3832 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTING_INFO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3833 SP_SUPPORTING_INFO); 3834 3835 /** 3836 * Constant for fluent queries to be used to add include statements. Specifies 3837 * the path value of "<b>Appointment:supporting-info</b>". 3838 */ 3839 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTING_INFO = new ca.uhn.fhir.model.api.Include( 3840 "Appointment:supporting-info").toLocked(); 3841 3842 /** 3843 * Search parameter: <b>location</b> 3844 * <p> 3845 * Description: <b>This location is listed in the participants of the 3846 * appointment</b><br> 3847 * Type: <b>reference</b><br> 3848 * Path: <b>Appointment.participant.actor</b><br> 3849 * </p> 3850 */ 3851 @SearchParamDefinition(name = "location", path = "Appointment.participant.actor.where(resolve() is Location)", description = "This location is listed in the participants of the appointment", type = "reference", target = { 3852 Location.class }) 3853 public static final String SP_LOCATION = "location"; 3854 /** 3855 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3856 * <p> 3857 * Description: <b>This location is listed in the participants of the 3858 * appointment</b><br> 3859 * Type: <b>reference</b><br> 3860 * Path: <b>Appointment.participant.actor</b><br> 3861 * </p> 3862 */ 3863 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3864 SP_LOCATION); 3865 3866 /** 3867 * Constant for fluent queries to be used to add include statements. Specifies 3868 * the path value of "<b>Appointment:location</b>". 3869 */ 3870 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 3871 "Appointment:location").toLocked(); 3872 3873 /** 3874 * Search parameter: <b>status</b> 3875 * <p> 3876 * Description: <b>The overall status of the appointment</b><br> 3877 * Type: <b>token</b><br> 3878 * Path: <b>Appointment.status</b><br> 3879 * </p> 3880 */ 3881 @SearchParamDefinition(name = "status", path = "Appointment.status", description = "The overall status of the appointment", type = "token") 3882 public static final String SP_STATUS = "status"; 3883 /** 3884 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3885 * <p> 3886 * Description: <b>The overall status of the appointment</b><br> 3887 * Type: <b>token</b><br> 3888 * Path: <b>Appointment.status</b><br> 3889 * </p> 3890 */ 3891 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3892 SP_STATUS); 3893 3894}