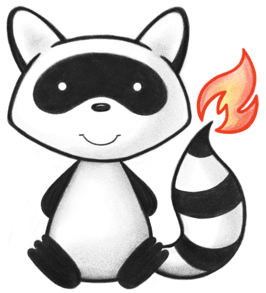
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A booking of a healthcare event among patient(s), practitioner(s), related 049 * person(s) and/or device(s) for a specific date/time. This may result in one 050 * or more Encounter(s). 051 */ 052@ResourceDef(name = "Appointment", profile = "http://hl7.org/fhir/StructureDefinition/Appointment") 053public class Appointment extends DomainResource { 054 055 public enum AppointmentStatus { 056 /** 057 * None of the participant(s) have finalized their acceptance of the appointment 058 * request, and the start/end time might not be set yet. 059 */ 060 PROPOSED, 061 /** 062 * Some or all of the participant(s) have not finalized their acceptance of the 063 * appointment request. 064 */ 065 PENDING, 066 /** 067 * All participant(s) have been considered and the appointment is confirmed to 068 * go ahead at the date/times specified. 069 */ 070 BOOKED, 071 /** 072 * The patient/patients has/have arrived and is/are waiting to be seen. 073 */ 074 ARRIVED, 075 /** 076 * The planning stages of the appointment are now complete, the encounter 077 * resource will exist and will track further status changes. Note that an 078 * encounter may exist before the appointment status is fulfilled for many 079 * reasons. 080 */ 081 FULFILLED, 082 /** 083 * The appointment has been cancelled. 084 */ 085 CANCELLED, 086 /** 087 * Some or all of the participant(s) have not/did not appear for the appointment 088 * (usually the patient). 089 */ 090 NOSHOW, 091 /** 092 * This instance should not have been part of this patient's medical record. 093 */ 094 ENTEREDINERROR, 095 /** 096 * When checked in, all pre-encounter administrative work is complete, and the 097 * encounter may begin. (where multiple patients are involved, they are all 098 * present). 099 */ 100 CHECKEDIN, 101 /** 102 * The appointment has been placed on a waitlist, to be scheduled/confirmed in 103 * the future when a slot/service is available. A specific time might or might 104 * not be pre-allocated. 105 */ 106 WAITLIST, 107 /** 108 * added to help the parsers with the generic types 109 */ 110 NULL; 111 112 public static AppointmentStatus fromCode(String codeString) throws FHIRException { 113 if (codeString == null || "".equals(codeString)) 114 return null; 115 if ("proposed".equals(codeString)) 116 return PROPOSED; 117 if ("pending".equals(codeString)) 118 return PENDING; 119 if ("booked".equals(codeString)) 120 return BOOKED; 121 if ("arrived".equals(codeString)) 122 return ARRIVED; 123 if ("fulfilled".equals(codeString)) 124 return FULFILLED; 125 if ("cancelled".equals(codeString)) 126 return CANCELLED; 127 if ("noshow".equals(codeString)) 128 return NOSHOW; 129 if ("entered-in-error".equals(codeString)) 130 return ENTEREDINERROR; 131 if ("checked-in".equals(codeString)) 132 return CHECKEDIN; 133 if ("waitlist".equals(codeString)) 134 return WAITLIST; 135 if (Configuration.isAcceptInvalidEnums()) 136 return null; 137 else 138 throw new FHIRException("Unknown AppointmentStatus code '" + codeString + "'"); 139 } 140 141 public String toCode() { 142 switch (this) { 143 case PROPOSED: 144 return "proposed"; 145 case PENDING: 146 return "pending"; 147 case BOOKED: 148 return "booked"; 149 case ARRIVED: 150 return "arrived"; 151 case FULFILLED: 152 return "fulfilled"; 153 case CANCELLED: 154 return "cancelled"; 155 case NOSHOW: 156 return "noshow"; 157 case ENTEREDINERROR: 158 return "entered-in-error"; 159 case CHECKEDIN: 160 return "checked-in"; 161 case WAITLIST: 162 return "waitlist"; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getSystem() { 171 switch (this) { 172 case PROPOSED: 173 return "http://hl7.org/fhir/appointmentstatus"; 174 case PENDING: 175 return "http://hl7.org/fhir/appointmentstatus"; 176 case BOOKED: 177 return "http://hl7.org/fhir/appointmentstatus"; 178 case ARRIVED: 179 return "http://hl7.org/fhir/appointmentstatus"; 180 case FULFILLED: 181 return "http://hl7.org/fhir/appointmentstatus"; 182 case CANCELLED: 183 return "http://hl7.org/fhir/appointmentstatus"; 184 case NOSHOW: 185 return "http://hl7.org/fhir/appointmentstatus"; 186 case ENTEREDINERROR: 187 return "http://hl7.org/fhir/appointmentstatus"; 188 case CHECKEDIN: 189 return "http://hl7.org/fhir/appointmentstatus"; 190 case WAITLIST: 191 return "http://hl7.org/fhir/appointmentstatus"; 192 case NULL: 193 return null; 194 default: 195 return "?"; 196 } 197 } 198 199 public String getDefinition() { 200 switch (this) { 201 case PROPOSED: 202 return "None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time might not be set yet."; 203 case PENDING: 204 return "Some or all of the participant(s) have not finalized their acceptance of the appointment request."; 205 case BOOKED: 206 return "All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified."; 207 case ARRIVED: 208 return "The patient/patients has/have arrived and is/are waiting to be seen."; 209 case FULFILLED: 210 return "The planning stages of the appointment are now complete, the encounter resource will exist and will track further status changes. Note that an encounter may exist before the appointment status is fulfilled for many reasons."; 211 case CANCELLED: 212 return "The appointment has been cancelled."; 213 case NOSHOW: 214 return "Some or all of the participant(s) have not/did not appear for the appointment (usually the patient)."; 215 case ENTEREDINERROR: 216 return "This instance should not have been part of this patient's medical record."; 217 case CHECKEDIN: 218 return "When checked in, all pre-encounter administrative work is complete, and the encounter may begin. (where multiple patients are involved, they are all present)."; 219 case WAITLIST: 220 return "The appointment has been placed on a waitlist, to be scheduled/confirmed in the future when a slot/service is available.\nA specific time might or might not be pre-allocated."; 221 case NULL: 222 return null; 223 default: 224 return "?"; 225 } 226 } 227 228 public String getDisplay() { 229 switch (this) { 230 case PROPOSED: 231 return "Proposed"; 232 case PENDING: 233 return "Pending"; 234 case BOOKED: 235 return "Booked"; 236 case ARRIVED: 237 return "Arrived"; 238 case FULFILLED: 239 return "Fulfilled"; 240 case CANCELLED: 241 return "Cancelled"; 242 case NOSHOW: 243 return "No Show"; 244 case ENTEREDINERROR: 245 return "Entered in error"; 246 case CHECKEDIN: 247 return "Checked In"; 248 case WAITLIST: 249 return "Waitlisted"; 250 case NULL: 251 return null; 252 default: 253 return "?"; 254 } 255 } 256 } 257 258 public static class AppointmentStatusEnumFactory implements EnumFactory<AppointmentStatus> { 259 public AppointmentStatus fromCode(String codeString) throws IllegalArgumentException { 260 if (codeString == null || "".equals(codeString)) 261 if (codeString == null || "".equals(codeString)) 262 return null; 263 if ("proposed".equals(codeString)) 264 return AppointmentStatus.PROPOSED; 265 if ("pending".equals(codeString)) 266 return AppointmentStatus.PENDING; 267 if ("booked".equals(codeString)) 268 return AppointmentStatus.BOOKED; 269 if ("arrived".equals(codeString)) 270 return AppointmentStatus.ARRIVED; 271 if ("fulfilled".equals(codeString)) 272 return AppointmentStatus.FULFILLED; 273 if ("cancelled".equals(codeString)) 274 return AppointmentStatus.CANCELLED; 275 if ("noshow".equals(codeString)) 276 return AppointmentStatus.NOSHOW; 277 if ("entered-in-error".equals(codeString)) 278 return AppointmentStatus.ENTEREDINERROR; 279 if ("checked-in".equals(codeString)) 280 return AppointmentStatus.CHECKEDIN; 281 if ("waitlist".equals(codeString)) 282 return AppointmentStatus.WAITLIST; 283 throw new IllegalArgumentException("Unknown AppointmentStatus code '" + codeString + "'"); 284 } 285 286 public Enumeration<AppointmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 287 if (code == null) 288 return null; 289 if (code.isEmpty()) 290 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NULL, code); 291 String codeString = code.asStringValue(); 292 if (codeString == null || "".equals(codeString)) 293 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NULL, code); 294 if ("proposed".equals(codeString)) 295 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PROPOSED, code); 296 if ("pending".equals(codeString)) 297 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PENDING, code); 298 if ("booked".equals(codeString)) 299 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.BOOKED, code); 300 if ("arrived".equals(codeString)) 301 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ARRIVED, code); 302 if ("fulfilled".equals(codeString)) 303 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.FULFILLED, code); 304 if ("cancelled".equals(codeString)) 305 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CANCELLED, code); 306 if ("noshow".equals(codeString)) 307 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NOSHOW, code); 308 if ("entered-in-error".equals(codeString)) 309 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ENTEREDINERROR, code); 310 if ("checked-in".equals(codeString)) 311 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CHECKEDIN, code); 312 if ("waitlist".equals(codeString)) 313 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.WAITLIST, code); 314 throw new FHIRException("Unknown AppointmentStatus code '" + codeString + "'"); 315 } 316 317 public String toCode(AppointmentStatus code) { 318 if (code == AppointmentStatus.NULL) 319 return null; 320 if (code == AppointmentStatus.PROPOSED) 321 return "proposed"; 322 if (code == AppointmentStatus.PENDING) 323 return "pending"; 324 if (code == AppointmentStatus.BOOKED) 325 return "booked"; 326 if (code == AppointmentStatus.ARRIVED) 327 return "arrived"; 328 if (code == AppointmentStatus.FULFILLED) 329 return "fulfilled"; 330 if (code == AppointmentStatus.CANCELLED) 331 return "cancelled"; 332 if (code == AppointmentStatus.NOSHOW) 333 return "noshow"; 334 if (code == AppointmentStatus.ENTEREDINERROR) 335 return "entered-in-error"; 336 if (code == AppointmentStatus.CHECKEDIN) 337 return "checked-in"; 338 if (code == AppointmentStatus.WAITLIST) 339 return "waitlist"; 340 return "?"; 341 } 342 343 public String toSystem(AppointmentStatus code) { 344 return code.getSystem(); 345 } 346 } 347 348 public enum ParticipantRequired { 349 /** 350 * The participant is required to attend the appointment. 351 */ 352 REQUIRED, 353 /** 354 * The participant may optionally attend the appointment. 355 */ 356 OPTIONAL, 357 /** 358 * The participant is excluded from the appointment, and might not be informed 359 * of the appointment taking place. (Appointment is about them, not for them - 360 * such as 2 doctors discussing results about a patient's test). 361 */ 362 INFORMATIONONLY, 363 /** 364 * added to help the parsers with the generic types 365 */ 366 NULL; 367 368 public static ParticipantRequired fromCode(String codeString) throws FHIRException { 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("required".equals(codeString)) 372 return REQUIRED; 373 if ("optional".equals(codeString)) 374 return OPTIONAL; 375 if ("information-only".equals(codeString)) 376 return INFORMATIONONLY; 377 if (Configuration.isAcceptInvalidEnums()) 378 return null; 379 else 380 throw new FHIRException("Unknown ParticipantRequired code '" + codeString + "'"); 381 } 382 383 public String toCode() { 384 switch (this) { 385 case REQUIRED: 386 return "required"; 387 case OPTIONAL: 388 return "optional"; 389 case INFORMATIONONLY: 390 return "information-only"; 391 case NULL: 392 return null; 393 default: 394 return "?"; 395 } 396 } 397 398 public String getSystem() { 399 switch (this) { 400 case REQUIRED: 401 return "http://hl7.org/fhir/participantrequired"; 402 case OPTIONAL: 403 return "http://hl7.org/fhir/participantrequired"; 404 case INFORMATIONONLY: 405 return "http://hl7.org/fhir/participantrequired"; 406 case NULL: 407 return null; 408 default: 409 return "?"; 410 } 411 } 412 413 public String getDefinition() { 414 switch (this) { 415 case REQUIRED: 416 return "The participant is required to attend the appointment."; 417 case OPTIONAL: 418 return "The participant may optionally attend the appointment."; 419 case INFORMATIONONLY: 420 return "The participant is excluded from the appointment, and might not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test)."; 421 case NULL: 422 return null; 423 default: 424 return "?"; 425 } 426 } 427 428 public String getDisplay() { 429 switch (this) { 430 case REQUIRED: 431 return "Required"; 432 case OPTIONAL: 433 return "Optional"; 434 case INFORMATIONONLY: 435 return "Information Only"; 436 case NULL: 437 return null; 438 default: 439 return "?"; 440 } 441 } 442 } 443 444 public static class ParticipantRequiredEnumFactory implements EnumFactory<ParticipantRequired> { 445 public ParticipantRequired fromCode(String codeString) throws IllegalArgumentException { 446 if (codeString == null || "".equals(codeString)) 447 if (codeString == null || "".equals(codeString)) 448 return null; 449 if ("required".equals(codeString)) 450 return ParticipantRequired.REQUIRED; 451 if ("optional".equals(codeString)) 452 return ParticipantRequired.OPTIONAL; 453 if ("information-only".equals(codeString)) 454 return ParticipantRequired.INFORMATIONONLY; 455 throw new IllegalArgumentException("Unknown ParticipantRequired code '" + codeString + "'"); 456 } 457 458 public Enumeration<ParticipantRequired> fromType(PrimitiveType<?> code) throws FHIRException { 459 if (code == null) 460 return null; 461 if (code.isEmpty()) 462 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.NULL, code); 463 String codeString = code.asStringValue(); 464 if (codeString == null || "".equals(codeString)) 465 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.NULL, code); 466 if ("required".equals(codeString)) 467 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.REQUIRED, code); 468 if ("optional".equals(codeString)) 469 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.OPTIONAL, code); 470 if ("information-only".equals(codeString)) 471 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.INFORMATIONONLY, code); 472 throw new FHIRException("Unknown ParticipantRequired code '" + codeString + "'"); 473 } 474 475 public String toCode(ParticipantRequired code) { 476 if (code == ParticipantRequired.NULL) 477 return null; 478 if (code == ParticipantRequired.REQUIRED) 479 return "required"; 480 if (code == ParticipantRequired.OPTIONAL) 481 return "optional"; 482 if (code == ParticipantRequired.INFORMATIONONLY) 483 return "information-only"; 484 return "?"; 485 } 486 487 public String toSystem(ParticipantRequired code) { 488 return code.getSystem(); 489 } 490 } 491 492 public enum ParticipationStatus { 493 /** 494 * The participant has accepted the appointment. 495 */ 496 ACCEPTED, 497 /** 498 * The participant has declined the appointment and will not participate in the 499 * appointment. 500 */ 501 DECLINED, 502 /** 503 * The participant has tentatively accepted the appointment. This could be 504 * automatically created by a system and requires further processing before it 505 * can be accepted. There is no commitment that attendance will occur. 506 */ 507 TENTATIVE, 508 /** 509 * The participant needs to indicate if they accept the appointment by changing 510 * this status to one of the other statuses. 511 */ 512 NEEDSACTION, 513 /** 514 * added to help the parsers with the generic types 515 */ 516 NULL; 517 518 public static ParticipationStatus fromCode(String codeString) throws FHIRException { 519 if (codeString == null || "".equals(codeString)) 520 return null; 521 if ("accepted".equals(codeString)) 522 return ACCEPTED; 523 if ("declined".equals(codeString)) 524 return DECLINED; 525 if ("tentative".equals(codeString)) 526 return TENTATIVE; 527 if ("needs-action".equals(codeString)) 528 return NEEDSACTION; 529 if (Configuration.isAcceptInvalidEnums()) 530 return null; 531 else 532 throw new FHIRException("Unknown ParticipationStatus code '" + codeString + "'"); 533 } 534 535 public String toCode() { 536 switch (this) { 537 case ACCEPTED: 538 return "accepted"; 539 case DECLINED: 540 return "declined"; 541 case TENTATIVE: 542 return "tentative"; 543 case NEEDSACTION: 544 return "needs-action"; 545 case NULL: 546 return null; 547 default: 548 return "?"; 549 } 550 } 551 552 public String getSystem() { 553 switch (this) { 554 case ACCEPTED: 555 return "http://hl7.org/fhir/participationstatus"; 556 case DECLINED: 557 return "http://hl7.org/fhir/participationstatus"; 558 case TENTATIVE: 559 return "http://hl7.org/fhir/participationstatus"; 560 case NEEDSACTION: 561 return "http://hl7.org/fhir/participationstatus"; 562 case NULL: 563 return null; 564 default: 565 return "?"; 566 } 567 } 568 569 public String getDefinition() { 570 switch (this) { 571 case ACCEPTED: 572 return "The participant has accepted the appointment."; 573 case DECLINED: 574 return "The participant has declined the appointment and will not participate in the appointment."; 575 case TENTATIVE: 576 return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 577 case NEEDSACTION: 578 return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 579 case NULL: 580 return null; 581 default: 582 return "?"; 583 } 584 } 585 586 public String getDisplay() { 587 switch (this) { 588 case ACCEPTED: 589 return "Accepted"; 590 case DECLINED: 591 return "Declined"; 592 case TENTATIVE: 593 return "Tentative"; 594 case NEEDSACTION: 595 return "Needs Action"; 596 case NULL: 597 return null; 598 default: 599 return "?"; 600 } 601 } 602 } 603 604 public static class ParticipationStatusEnumFactory implements EnumFactory<ParticipationStatus> { 605 public ParticipationStatus fromCode(String codeString) throws IllegalArgumentException { 606 if (codeString == null || "".equals(codeString)) 607 if (codeString == null || "".equals(codeString)) 608 return null; 609 if ("accepted".equals(codeString)) 610 return ParticipationStatus.ACCEPTED; 611 if ("declined".equals(codeString)) 612 return ParticipationStatus.DECLINED; 613 if ("tentative".equals(codeString)) 614 return ParticipationStatus.TENTATIVE; 615 if ("needs-action".equals(codeString)) 616 return ParticipationStatus.NEEDSACTION; 617 throw new IllegalArgumentException("Unknown ParticipationStatus code '" + codeString + "'"); 618 } 619 620 public Enumeration<ParticipationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 621 if (code == null) 622 return null; 623 if (code.isEmpty()) 624 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NULL, code); 625 String codeString = code.asStringValue(); 626 if (codeString == null || "".equals(codeString)) 627 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NULL, code); 628 if ("accepted".equals(codeString)) 629 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.ACCEPTED, code); 630 if ("declined".equals(codeString)) 631 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.DECLINED, code); 632 if ("tentative".equals(codeString)) 633 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.TENTATIVE, code); 634 if ("needs-action".equals(codeString)) 635 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NEEDSACTION, code); 636 throw new FHIRException("Unknown ParticipationStatus code '" + codeString + "'"); 637 } 638 639 public String toCode(ParticipationStatus code) { 640 if (code == ParticipationStatus.NULL) 641 return null; 642 if (code == ParticipationStatus.ACCEPTED) 643 return "accepted"; 644 if (code == ParticipationStatus.DECLINED) 645 return "declined"; 646 if (code == ParticipationStatus.TENTATIVE) 647 return "tentative"; 648 if (code == ParticipationStatus.NEEDSACTION) 649 return "needs-action"; 650 return "?"; 651 } 652 653 public String toSystem(ParticipationStatus code) { 654 return code.getSystem(); 655 } 656 } 657 658 @Block() 659 public static class AppointmentParticipantComponent extends BackboneElement implements IBaseBackboneElement { 660 /** 661 * Role of participant in the appointment. 662 */ 663 @Child(name = "type", type = { 664 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 665 @Description(shortDefinition = "Role of participant in the appointment", formalDefinition = "Role of participant in the appointment.") 666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-participant-type") 667 protected List<CodeableConcept> type; 668 669 /** 670 * A Person, Location/HealthcareService or Device that is participating in the 671 * appointment. 672 */ 673 @Child(name = "actor", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 674 Device.class, HealthcareService.class, 675 Location.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 676 @Description(shortDefinition = "Person, Location/HealthcareService or Device", formalDefinition = "A Person, Location/HealthcareService or Device that is participating in the appointment.") 677 protected Reference actor; 678 679 /** 680 * The actual object that is the target of the reference (A Person, 681 * Location/HealthcareService or Device that is participating in the 682 * appointment.) 683 */ 684 protected Resource actorTarget; 685 686 /** 687 * Whether this participant is required to be present at the meeting. This 688 * covers a use-case where two doctors need to meet to discuss the results for a 689 * specific patient, and the patient is not required to be present. 690 */ 691 @Child(name = "required", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 692 @Description(shortDefinition = "required | optional | information-only", formalDefinition = "Whether this participant is required to be present at the meeting. This covers a use-case where two doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.") 693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participantrequired") 694 protected Enumeration<ParticipantRequired> required; 695 696 /** 697 * Participation status of the actor. 698 */ 699 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 700 @Description(shortDefinition = "accepted | declined | tentative | needs-action", formalDefinition = "Participation status of the actor.") 701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participationstatus") 702 protected Enumeration<ParticipationStatus> status; 703 704 /** 705 * Participation period of the actor. 706 */ 707 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 708 @Description(shortDefinition = "Participation period of the actor", formalDefinition = "Participation period of the actor.") 709 protected Period period; 710 711 private static final long serialVersionUID = -1939292177L; 712 713 /** 714 * Constructor 715 */ 716 public AppointmentParticipantComponent() { 717 super(); 718 } 719 720 /** 721 * Constructor 722 */ 723 public AppointmentParticipantComponent(Enumeration<ParticipationStatus> status) { 724 super(); 725 this.status = status; 726 } 727 728 /** 729 * @return {@link #type} (Role of participant in the appointment.) 730 */ 731 public List<CodeableConcept> getType() { 732 if (this.type == null) 733 this.type = new ArrayList<CodeableConcept>(); 734 return this.type; 735 } 736 737 /** 738 * @return Returns a reference to <code>this</code> for easy method chaining 739 */ 740 public AppointmentParticipantComponent setType(List<CodeableConcept> theType) { 741 this.type = theType; 742 return this; 743 } 744 745 public boolean hasType() { 746 if (this.type == null) 747 return false; 748 for (CodeableConcept item : this.type) 749 if (!item.isEmpty()) 750 return true; 751 return false; 752 } 753 754 public CodeableConcept addType() { // 3 755 CodeableConcept t = new CodeableConcept(); 756 if (this.type == null) 757 this.type = new ArrayList<CodeableConcept>(); 758 this.type.add(t); 759 return t; 760 } 761 762 public AppointmentParticipantComponent addType(CodeableConcept t) { // 3 763 if (t == null) 764 return this; 765 if (this.type == null) 766 this.type = new ArrayList<CodeableConcept>(); 767 this.type.add(t); 768 return this; 769 } 770 771 /** 772 * @return The first repetition of repeating field {@link #type}, creating it if 773 * it does not already exist 774 */ 775 public CodeableConcept getTypeFirstRep() { 776 if (getType().isEmpty()) { 777 addType(); 778 } 779 return getType().get(0); 780 } 781 782 /** 783 * @return {@link #actor} (A Person, Location/HealthcareService or Device that 784 * is participating in the appointment.) 785 */ 786 public Reference getActor() { 787 if (this.actor == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create AppointmentParticipantComponent.actor"); 790 else if (Configuration.doAutoCreate()) 791 this.actor = new Reference(); // cc 792 return this.actor; 793 } 794 795 public boolean hasActor() { 796 return this.actor != null && !this.actor.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #actor} (A Person, Location/HealthcareService or Device 801 * that is participating in the appointment.) 802 */ 803 public AppointmentParticipantComponent setActor(Reference value) { 804 this.actor = value; 805 return this; 806 } 807 808 /** 809 * @return {@link #actor} The actual object that is the target of the reference. 810 * The reference library doesn't populate this, but you can use it to 811 * hold the resource if you resolve it. (A Person, 812 * Location/HealthcareService or Device that is participating in the 813 * appointment.) 814 */ 815 public Resource getActorTarget() { 816 return this.actorTarget; 817 } 818 819 /** 820 * @param value {@link #actor} The actual object that is the target of the 821 * reference. The reference library doesn't use these, but you can 822 * use it to hold the resource if you resolve it. (A Person, 823 * Location/HealthcareService or Device that is participating in 824 * the appointment.) 825 */ 826 public AppointmentParticipantComponent setActorTarget(Resource value) { 827 this.actorTarget = value; 828 return this; 829 } 830 831 /** 832 * @return {@link #required} (Whether this participant is required to be present 833 * at the meeting. This covers a use-case where two doctors need to meet 834 * to discuss the results for a specific patient, and the patient is not 835 * required to be present.). This is the underlying object with id, 836 * value and extensions. The accessor "getRequired" gives direct access 837 * to the value 838 */ 839 public Enumeration<ParticipantRequired> getRequiredElement() { 840 if (this.required == null) 841 if (Configuration.errorOnAutoCreate()) 842 throw new Error("Attempt to auto-create AppointmentParticipantComponent.required"); 843 else if (Configuration.doAutoCreate()) 844 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); // bb 845 return this.required; 846 } 847 848 public boolean hasRequiredElement() { 849 return this.required != null && !this.required.isEmpty(); 850 } 851 852 public boolean hasRequired() { 853 return this.required != null && !this.required.isEmpty(); 854 } 855 856 /** 857 * @param value {@link #required} (Whether this participant is required to be 858 * present at the meeting. This covers a use-case where two doctors 859 * need to meet to discuss the results for a specific patient, and 860 * the patient is not required to be present.). This is the 861 * underlying object with id, value and extensions. The accessor 862 * "getRequired" gives direct access to the value 863 */ 864 public AppointmentParticipantComponent setRequiredElement(Enumeration<ParticipantRequired> value) { 865 this.required = value; 866 return this; 867 } 868 869 /** 870 * @return Whether this participant is required to be present at the meeting. 871 * This covers a use-case where two doctors need to meet to discuss the 872 * results for a specific patient, and the patient is not required to be 873 * present. 874 */ 875 public ParticipantRequired getRequired() { 876 return this.required == null ? null : this.required.getValue(); 877 } 878 879 /** 880 * @param value Whether this participant is required to be present at the 881 * meeting. This covers a use-case where two doctors need to meet 882 * to discuss the results for a specific patient, and the patient 883 * is not required to be present. 884 */ 885 public AppointmentParticipantComponent setRequired(ParticipantRequired value) { 886 if (value == null) 887 this.required = null; 888 else { 889 if (this.required == null) 890 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); 891 this.required.setValue(value); 892 } 893 return this; 894 } 895 896 /** 897 * @return {@link #status} (Participation status of the actor.). This is the 898 * underlying object with id, value and extensions. The accessor 899 * "getStatus" gives direct access to the value 900 */ 901 public Enumeration<ParticipationStatus> getStatusElement() { 902 if (this.status == null) 903 if (Configuration.errorOnAutoCreate()) 904 throw new Error("Attempt to auto-create AppointmentParticipantComponent.status"); 905 else if (Configuration.doAutoCreate()) 906 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); // bb 907 return this.status; 908 } 909 910 public boolean hasStatusElement() { 911 return this.status != null && !this.status.isEmpty(); 912 } 913 914 public boolean hasStatus() { 915 return this.status != null && !this.status.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #status} (Participation status of the actor.). This is 920 * the underlying object with id, value and extensions. The 921 * accessor "getStatus" gives direct access to the value 922 */ 923 public AppointmentParticipantComponent setStatusElement(Enumeration<ParticipationStatus> value) { 924 this.status = value; 925 return this; 926 } 927 928 /** 929 * @return Participation status of the actor. 930 */ 931 public ParticipationStatus getStatus() { 932 return this.status == null ? null : this.status.getValue(); 933 } 934 935 /** 936 * @param value Participation status of the actor. 937 */ 938 public AppointmentParticipantComponent setStatus(ParticipationStatus value) { 939 if (this.status == null) 940 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); 941 this.status.setValue(value); 942 return this; 943 } 944 945 /** 946 * @return {@link #period} (Participation period of the actor.) 947 */ 948 public Period getPeriod() { 949 if (this.period == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create AppointmentParticipantComponent.period"); 952 else if (Configuration.doAutoCreate()) 953 this.period = new Period(); // cc 954 return this.period; 955 } 956 957 public boolean hasPeriod() { 958 return this.period != null && !this.period.isEmpty(); 959 } 960 961 /** 962 * @param value {@link #period} (Participation period of the actor.) 963 */ 964 public AppointmentParticipantComponent setPeriod(Period value) { 965 this.period = value; 966 return this; 967 } 968 969 protected void listChildren(List<Property> children) { 970 super.listChildren(children); 971 children.add(new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, 972 java.lang.Integer.MAX_VALUE, type)); 973 children.add(new Property("actor", 974 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 975 "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor)); 976 children.add(new Property("required", "code", 977 "Whether this participant is required to be present at the meeting. This covers a use-case where two doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 978 0, 1, required)); 979 children.add(new Property("status", "code", "Participation status of the actor.", 0, 1, status)); 980 children.add(new Property("period", "Period", "Participation period of the actor.", 0, 1, period)); 981 } 982 983 @Override 984 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 985 switch (_hash) { 986 case 3575610: 987 /* type */ return new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, 988 java.lang.Integer.MAX_VALUE, type); 989 case 92645877: 990 /* actor */ return new Property("actor", 991 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 992 "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 1, actor); 993 case -393139297: 994 /* required */ return new Property("required", "code", 995 "Whether this participant is required to be present at the meeting. This covers a use-case where two doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 996 0, 1, required); 997 case -892481550: 998 /* status */ return new Property("status", "code", "Participation status of the actor.", 0, 1, status); 999 case -991726143: 1000 /* period */ return new Property("period", "Period", "Participation period of the actor.", 0, 1, period); 1001 default: 1002 return super.getNamedProperty(_hash, _name, _checkValid); 1003 } 1004 1005 } 1006 1007 @Override 1008 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1009 switch (hash) { 1010 case 3575610: 1011 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1012 case 92645877: 1013 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 1014 case -393139297: 1015 /* required */ return this.required == null ? new Base[0] : new Base[] { this.required }; // Enumeration<ParticipantRequired> 1016 case -892481550: 1017 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ParticipationStatus> 1018 case -991726143: 1019 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1020 default: 1021 return super.getProperty(hash, name, checkValid); 1022 } 1023 1024 } 1025 1026 @Override 1027 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1028 switch (hash) { 1029 case 3575610: // type 1030 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1031 return value; 1032 case 92645877: // actor 1033 this.actor = castToReference(value); // Reference 1034 return value; 1035 case -393139297: // required 1036 value = new ParticipantRequiredEnumFactory().fromType(castToCode(value)); 1037 this.required = (Enumeration) value; // Enumeration<ParticipantRequired> 1038 return value; 1039 case -892481550: // status 1040 value = new ParticipationStatusEnumFactory().fromType(castToCode(value)); 1041 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 1042 return value; 1043 case -991726143: // period 1044 this.period = castToPeriod(value); // Period 1045 return value; 1046 default: 1047 return super.setProperty(hash, name, value); 1048 } 1049 1050 } 1051 1052 @Override 1053 public Base setProperty(String name, Base value) throws FHIRException { 1054 if (name.equals("type")) { 1055 this.getType().add(castToCodeableConcept(value)); 1056 } else if (name.equals("actor")) { 1057 this.actor = castToReference(value); // Reference 1058 } else if (name.equals("required")) { 1059 value = new ParticipantRequiredEnumFactory().fromType(castToCode(value)); 1060 this.required = (Enumeration) value; // Enumeration<ParticipantRequired> 1061 } else if (name.equals("status")) { 1062 value = new ParticipationStatusEnumFactory().fromType(castToCode(value)); 1063 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 1064 } else if (name.equals("period")) { 1065 this.period = castToPeriod(value); // Period 1066 } else 1067 return super.setProperty(name, value); 1068 return value; 1069 } 1070 1071 @Override 1072 public void removeChild(String name, Base value) throws FHIRException { 1073 if (name.equals("type")) { 1074 this.getType().remove(castToCodeableConcept(value)); 1075 } else if (name.equals("actor")) { 1076 this.actor = null; 1077 } else if (name.equals("required")) { 1078 this.required = null; 1079 } else if (name.equals("status")) { 1080 this.status = null; 1081 } else if (name.equals("period")) { 1082 this.period = null; 1083 } else 1084 super.removeChild(name, value); 1085 1086 } 1087 1088 @Override 1089 public Base makeProperty(int hash, String name) throws FHIRException { 1090 switch (hash) { 1091 case 3575610: 1092 return addType(); 1093 case 92645877: 1094 return getActor(); 1095 case -393139297: 1096 return getRequiredElement(); 1097 case -892481550: 1098 return getStatusElement(); 1099 case -991726143: 1100 return getPeriod(); 1101 default: 1102 return super.makeProperty(hash, name); 1103 } 1104 1105 } 1106 1107 @Override 1108 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1109 switch (hash) { 1110 case 3575610: 1111 /* type */ return new String[] { "CodeableConcept" }; 1112 case 92645877: 1113 /* actor */ return new String[] { "Reference" }; 1114 case -393139297: 1115 /* required */ return new String[] { "code" }; 1116 case -892481550: 1117 /* status */ return new String[] { "code" }; 1118 case -991726143: 1119 /* period */ return new String[] { "Period" }; 1120 default: 1121 return super.getTypesForProperty(hash, name); 1122 } 1123 1124 } 1125 1126 @Override 1127 public Base addChild(String name) throws FHIRException { 1128 if (name.equals("type")) { 1129 return addType(); 1130 } else if (name.equals("actor")) { 1131 this.actor = new Reference(); 1132 return this.actor; 1133 } else if (name.equals("required")) { 1134 throw new FHIRException("Cannot call addChild on a singleton property Appointment.required"); 1135 } else if (name.equals("status")) { 1136 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 1137 } else if (name.equals("period")) { 1138 this.period = new Period(); 1139 return this.period; 1140 } else 1141 return super.addChild(name); 1142 } 1143 1144 public AppointmentParticipantComponent copy() { 1145 AppointmentParticipantComponent dst = new AppointmentParticipantComponent(); 1146 copyValues(dst); 1147 return dst; 1148 } 1149 1150 public void copyValues(AppointmentParticipantComponent dst) { 1151 super.copyValues(dst); 1152 if (type != null) { 1153 dst.type = new ArrayList<CodeableConcept>(); 1154 for (CodeableConcept i : type) 1155 dst.type.add(i.copy()); 1156 } 1157 ; 1158 dst.actor = actor == null ? null : actor.copy(); 1159 dst.required = required == null ? null : required.copy(); 1160 dst.status = status == null ? null : status.copy(); 1161 dst.period = period == null ? null : period.copy(); 1162 } 1163 1164 @Override 1165 public boolean equalsDeep(Base other_) { 1166 if (!super.equalsDeep(other_)) 1167 return false; 1168 if (!(other_ instanceof AppointmentParticipantComponent)) 1169 return false; 1170 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 1171 return compareDeep(type, o.type, true) && compareDeep(actor, o.actor, true) 1172 && compareDeep(required, o.required, true) && compareDeep(status, o.status, true) 1173 && compareDeep(period, o.period, true); 1174 } 1175 1176 @Override 1177 public boolean equalsShallow(Base other_) { 1178 if (!super.equalsShallow(other_)) 1179 return false; 1180 if (!(other_ instanceof AppointmentParticipantComponent)) 1181 return false; 1182 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 1183 return compareValues(required, o.required, true) && compareValues(status, o.status, true); 1184 } 1185 1186 public boolean isEmpty() { 1187 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, actor, required, status, period); 1188 } 1189 1190 public String fhirType() { 1191 return "Appointment.participant"; 1192 1193 } 1194 1195 } 1196 1197 /** 1198 * This records identifiers associated with this appointment concern that are 1199 * defined by business processes and/or used to refer to it when a direct URL 1200 * reference to the resource itself is not appropriate (e.g. in CDA documents, 1201 * or in written / printed documentation). 1202 */ 1203 @Child(name = "identifier", type = { 1204 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1205 @Description(shortDefinition = "External Ids for this item", formalDefinition = "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 1206 protected List<Identifier> identifier; 1207 1208 /** 1209 * The overall status of the Appointment. Each of the participants has their own 1210 * participation status which indicates their involvement in the process, 1211 * however this status indicates the shared status. 1212 */ 1213 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1214 @Description(shortDefinition = "proposed | pending | booked | arrived | fulfilled | cancelled | noshow | entered-in-error | checked-in | waitlist", formalDefinition = "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.") 1215 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/appointmentstatus") 1216 protected Enumeration<AppointmentStatus> status; 1217 1218 /** 1219 * The coded reason for the appointment being cancelled. This is often used in 1220 * reporting/billing/futher processing to determine if further actions are 1221 * required, or specific fees apply. 1222 */ 1223 @Child(name = "cancelationReason", type = { 1224 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1225 @Description(shortDefinition = "The coded reason for the appointment being cancelled", formalDefinition = "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.") 1226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/appointment-cancellation-reason") 1227 protected CodeableConcept cancelationReason; 1228 1229 /** 1230 * A broad categorization of the service that is to be performed during this 1231 * appointment. 1232 */ 1233 @Child(name = "serviceCategory", type = { 1234 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1235 @Description(shortDefinition = "A broad categorization of the service that is to be performed during this appointment", formalDefinition = "A broad categorization of the service that is to be performed during this appointment.") 1236 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 1237 protected List<CodeableConcept> serviceCategory; 1238 1239 /** 1240 * The specific service that is to be performed during this appointment. 1241 */ 1242 @Child(name = "serviceType", type = { 1243 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1244 @Description(shortDefinition = "The specific service that is to be performed during this appointment", formalDefinition = "The specific service that is to be performed during this appointment.") 1245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 1246 protected List<CodeableConcept> serviceType; 1247 1248 /** 1249 * The specialty of a practitioner that would be required to perform the service 1250 * requested in this appointment. 1251 */ 1252 @Child(name = "specialty", type = { 1253 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1254 @Description(shortDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment.") 1255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 1256 protected List<CodeableConcept> specialty; 1257 1258 /** 1259 * The style of appointment or patient that has been booked in the slot (not 1260 * service type). 1261 */ 1262 @Child(name = "appointmentType", type = { 1263 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1264 @Description(shortDefinition = "The style of appointment or patient that has been booked in the slot (not service type)", formalDefinition = "The style of appointment or patient that has been booked in the slot (not service type).") 1265 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0276") 1266 protected CodeableConcept appointmentType; 1267 1268 /** 1269 * The coded reason that this appointment is being scheduled. This is more 1270 * clinical than administrative. 1271 */ 1272 @Child(name = "reasonCode", type = { 1273 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1274 @Description(shortDefinition = "Coded reason this appointment is scheduled", formalDefinition = "The coded reason that this appointment is being scheduled. This is more clinical than administrative.") 1275 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-reason") 1276 protected List<CodeableConcept> reasonCode; 1277 1278 /** 1279 * Reason the appointment has been scheduled to take place, as specified using 1280 * information from another resource. When the patient arrives and the encounter 1281 * begins it may be used as the admission diagnosis. The indication will 1282 * typically be a Condition (with other resources referenced in the 1283 * evidence.detail), or a Procedure. 1284 */ 1285 @Child(name = "reasonReference", type = { Condition.class, Procedure.class, Observation.class, 1286 ImmunizationRecommendation.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1287 @Description(shortDefinition = "Reason the appointment is to take place (resource)", formalDefinition = "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.") 1288 protected List<Reference> reasonReference; 1289 /** 1290 * The actual objects that are the target of the reference (Reason the 1291 * appointment has been scheduled to take place, as specified using information 1292 * from another resource. When the patient arrives and the encounter begins it 1293 * may be used as the admission diagnosis. The indication will typically be a 1294 * Condition (with other resources referenced in the evidence.detail), or a 1295 * Procedure.) 1296 */ 1297 protected List<Resource> reasonReferenceTarget; 1298 1299 /** 1300 * The priority of the appointment. Can be used to make informed decisions if 1301 * needing to re-prioritize appointments. (The iCal Standard specifies 0 as 1302 * undefined, 1 as highest, 9 as lowest priority). 1303 */ 1304 @Child(name = "priority", type = { 1305 UnsignedIntType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1306 @Description(shortDefinition = "Used to make informed decisions if needing to re-prioritize", formalDefinition = "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).") 1307 protected UnsignedIntType priority; 1308 1309 /** 1310 * The brief description of the appointment as would be shown on a subject line 1311 * in a meeting request, or appointment list. Detailed or expanded information 1312 * should be put in the comment field. 1313 */ 1314 @Child(name = "description", type = { 1315 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1316 @Description(shortDefinition = "Shown on a subject line in a meeting request, or appointment list", formalDefinition = "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.") 1317 protected StringType description; 1318 1319 /** 1320 * Additional information to support the appointment provided when making the 1321 * appointment. 1322 */ 1323 @Child(name = "supportingInformation", type = { 1324 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1325 @Description(shortDefinition = "Additional information to support the appointment", formalDefinition = "Additional information to support the appointment provided when making the appointment.") 1326 protected List<Reference> supportingInformation; 1327 /** 1328 * The actual objects that are the target of the reference (Additional 1329 * information to support the appointment provided when making the appointment.) 1330 */ 1331 protected List<Resource> supportingInformationTarget; 1332 1333 /** 1334 * Date/Time that the appointment is to take place. 1335 */ 1336 @Child(name = "start", type = { InstantType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1337 @Description(shortDefinition = "When appointment is to take place", formalDefinition = "Date/Time that the appointment is to take place.") 1338 protected InstantType start; 1339 1340 /** 1341 * Date/Time that the appointment is to conclude. 1342 */ 1343 @Child(name = "end", type = { InstantType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1344 @Description(shortDefinition = "When appointment is to conclude", formalDefinition = "Date/Time that the appointment is to conclude.") 1345 protected InstantType end; 1346 1347 /** 1348 * Number of minutes that the appointment is to take. This can be less than the 1349 * duration between the start and end times. For example, where the actual time 1350 * of appointment is only an estimate or if a 30 minute appointment is being 1351 * requested, but any time would work. Also, if there is, for example, a planned 1352 * 15 minute break in the middle of a long appointment, the duration may be 15 1353 * minutes less than the difference between the start and end. 1354 */ 1355 @Child(name = "minutesDuration", type = { 1356 PositiveIntType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1357 @Description(shortDefinition = "Can be less than start/end (e.g. estimate)", formalDefinition = "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.") 1358 protected PositiveIntType minutesDuration; 1359 1360 /** 1361 * The slots from the participants' schedules that will be filled by the 1362 * appointment. 1363 */ 1364 @Child(name = "slot", type = { 1365 Slot.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1366 @Description(shortDefinition = "The slots that this appointment is filling", formalDefinition = "The slots from the participants' schedules that will be filled by the appointment.") 1367 protected List<Reference> slot; 1368 /** 1369 * The actual objects that are the target of the reference (The slots from the 1370 * participants' schedules that will be filled by the appointment.) 1371 */ 1372 protected List<Slot> slotTarget; 1373 1374 /** 1375 * The date that this appointment was initially created. This could be different 1376 * to the meta.lastModified value on the initial entry, as this could have been 1377 * before the resource was created on the FHIR server, and should remain 1378 * unchanged over the lifespan of the appointment. 1379 */ 1380 @Child(name = "created", type = { 1381 DateTimeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1382 @Description(shortDefinition = "The date that this appointment was initially created", formalDefinition = "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.") 1383 protected DateTimeType created; 1384 1385 /** 1386 * Additional comments about the appointment. 1387 */ 1388 @Child(name = "comment", type = { StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1389 @Description(shortDefinition = "Additional comments", formalDefinition = "Additional comments about the appointment.") 1390 protected StringType comment; 1391 1392 /** 1393 * While Appointment.comment contains information for internal use, 1394 * Appointment.patientInstructions is used to capture patient facing information 1395 * about the Appointment (e.g. please bring your referral or fast from 8pm night 1396 * before). 1397 */ 1398 @Child(name = "patientInstruction", type = { 1399 StringType.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 1400 @Description(shortDefinition = "Detailed information and instructions for the patient", formalDefinition = "While Appointment.comment contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).") 1401 protected StringType patientInstruction; 1402 1403 /** 1404 * The service request this appointment is allocated to assess (e.g. incoming 1405 * referral or procedure request). 1406 */ 1407 @Child(name = "basedOn", type = { 1408 ServiceRequest.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1409 @Description(shortDefinition = "The service request this appointment is allocated to assess", formalDefinition = "The service request this appointment is allocated to assess (e.g. incoming referral or procedure request).") 1410 protected List<Reference> basedOn; 1411 /** 1412 * The actual objects that are the target of the reference (The service request 1413 * this appointment is allocated to assess (e.g. incoming referral or procedure 1414 * request).) 1415 */ 1416 protected List<ServiceRequest> basedOnTarget; 1417 1418 /** 1419 * List of participants involved in the appointment. 1420 */ 1421 @Child(name = "participant", type = {}, order = 20, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1422 @Description(shortDefinition = "Participants involved in appointment", formalDefinition = "List of participants involved in the appointment.") 1423 protected List<AppointmentParticipantComponent> participant; 1424 1425 /** 1426 * A set of date ranges (potentially including times) that the appointment is 1427 * preferred to be scheduled within. 1428 * 1429 * The duration (usually in minutes) could also be provided to indicate the 1430 * length of the appointment to fill and populate the start/end times for the 1431 * actual allocated time. However, in other situations the duration may be 1432 * calculated by the scheduling system. 1433 */ 1434 @Child(name = "requestedPeriod", type = { 1435 Period.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1436 @Description(shortDefinition = "Potential date/time interval(s) requested to allocate the appointment within", formalDefinition = "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.") 1437 protected List<Period> requestedPeriod; 1438 1439 private static final long serialVersionUID = -1096822339L; 1440 1441 /** 1442 * Constructor 1443 */ 1444 public Appointment() { 1445 super(); 1446 } 1447 1448 /** 1449 * Constructor 1450 */ 1451 public Appointment(Enumeration<AppointmentStatus> status) { 1452 super(); 1453 this.status = status; 1454 } 1455 1456 /** 1457 * @return {@link #identifier} (This records identifiers associated with this 1458 * appointment concern that are defined by business processes and/or 1459 * used to refer to it when a direct URL reference to the resource 1460 * itself is not appropriate (e.g. in CDA documents, or in written / 1461 * printed documentation).) 1462 */ 1463 public List<Identifier> getIdentifier() { 1464 if (this.identifier == null) 1465 this.identifier = new ArrayList<Identifier>(); 1466 return this.identifier; 1467 } 1468 1469 /** 1470 * @return Returns a reference to <code>this</code> for easy method chaining 1471 */ 1472 public Appointment setIdentifier(List<Identifier> theIdentifier) { 1473 this.identifier = theIdentifier; 1474 return this; 1475 } 1476 1477 public boolean hasIdentifier() { 1478 if (this.identifier == null) 1479 return false; 1480 for (Identifier item : this.identifier) 1481 if (!item.isEmpty()) 1482 return true; 1483 return false; 1484 } 1485 1486 public Identifier addIdentifier() { // 3 1487 Identifier t = new Identifier(); 1488 if (this.identifier == null) 1489 this.identifier = new ArrayList<Identifier>(); 1490 this.identifier.add(t); 1491 return t; 1492 } 1493 1494 public Appointment addIdentifier(Identifier t) { // 3 1495 if (t == null) 1496 return this; 1497 if (this.identifier == null) 1498 this.identifier = new ArrayList<Identifier>(); 1499 this.identifier.add(t); 1500 return this; 1501 } 1502 1503 /** 1504 * @return The first repetition of repeating field {@link #identifier}, creating 1505 * it if it does not already exist 1506 */ 1507 public Identifier getIdentifierFirstRep() { 1508 if (getIdentifier().isEmpty()) { 1509 addIdentifier(); 1510 } 1511 return getIdentifier().get(0); 1512 } 1513 1514 /** 1515 * @return {@link #status} (The overall status of the Appointment. Each of the 1516 * participants has their own participation status which indicates their 1517 * involvement in the process, however this status indicates the shared 1518 * status.). This is the underlying object with id, value and 1519 * extensions. The accessor "getStatus" gives direct access to the value 1520 */ 1521 public Enumeration<AppointmentStatus> getStatusElement() { 1522 if (this.status == null) 1523 if (Configuration.errorOnAutoCreate()) 1524 throw new Error("Attempt to auto-create Appointment.status"); 1525 else if (Configuration.doAutoCreate()) 1526 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); // bb 1527 return this.status; 1528 } 1529 1530 public boolean hasStatusElement() { 1531 return this.status != null && !this.status.isEmpty(); 1532 } 1533 1534 public boolean hasStatus() { 1535 return this.status != null && !this.status.isEmpty(); 1536 } 1537 1538 /** 1539 * @param value {@link #status} (The overall status of the Appointment. Each of 1540 * the participants has their own participation status which 1541 * indicates their involvement in the process, however this status 1542 * indicates the shared status.). This is the underlying object 1543 * with id, value and extensions. The accessor "getStatus" gives 1544 * direct access to the value 1545 */ 1546 public Appointment setStatusElement(Enumeration<AppointmentStatus> value) { 1547 this.status = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return The overall status of the Appointment. Each of the participants has 1553 * their own participation status which indicates their involvement in 1554 * the process, however this status indicates the shared status. 1555 */ 1556 public AppointmentStatus getStatus() { 1557 return this.status == null ? null : this.status.getValue(); 1558 } 1559 1560 /** 1561 * @param value The overall status of the Appointment. Each of the participants 1562 * has their own participation status which indicates their 1563 * involvement in the process, however this status indicates the 1564 * shared status. 1565 */ 1566 public Appointment setStatus(AppointmentStatus value) { 1567 if (this.status == null) 1568 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); 1569 this.status.setValue(value); 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #cancelationReason} (The coded reason for the appointment 1575 * being cancelled. This is often used in reporting/billing/futher 1576 * processing to determine if further actions are required, or specific 1577 * fees apply.) 1578 */ 1579 public CodeableConcept getCancelationReason() { 1580 if (this.cancelationReason == null) 1581 if (Configuration.errorOnAutoCreate()) 1582 throw new Error("Attempt to auto-create Appointment.cancelationReason"); 1583 else if (Configuration.doAutoCreate()) 1584 this.cancelationReason = new CodeableConcept(); // cc 1585 return this.cancelationReason; 1586 } 1587 1588 public boolean hasCancelationReason() { 1589 return this.cancelationReason != null && !this.cancelationReason.isEmpty(); 1590 } 1591 1592 /** 1593 * @param value {@link #cancelationReason} (The coded reason for the appointment 1594 * being cancelled. This is often used in reporting/billing/futher 1595 * processing to determine if further actions are required, or 1596 * specific fees apply.) 1597 */ 1598 public Appointment setCancelationReason(CodeableConcept value) { 1599 this.cancelationReason = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return {@link #serviceCategory} (A broad categorization of the service that 1605 * is to be performed during this appointment.) 1606 */ 1607 public List<CodeableConcept> getServiceCategory() { 1608 if (this.serviceCategory == null) 1609 this.serviceCategory = new ArrayList<CodeableConcept>(); 1610 return this.serviceCategory; 1611 } 1612 1613 /** 1614 * @return Returns a reference to <code>this</code> for easy method chaining 1615 */ 1616 public Appointment setServiceCategory(List<CodeableConcept> theServiceCategory) { 1617 this.serviceCategory = theServiceCategory; 1618 return this; 1619 } 1620 1621 public boolean hasServiceCategory() { 1622 if (this.serviceCategory == null) 1623 return false; 1624 for (CodeableConcept item : this.serviceCategory) 1625 if (!item.isEmpty()) 1626 return true; 1627 return false; 1628 } 1629 1630 public CodeableConcept addServiceCategory() { // 3 1631 CodeableConcept t = new CodeableConcept(); 1632 if (this.serviceCategory == null) 1633 this.serviceCategory = new ArrayList<CodeableConcept>(); 1634 this.serviceCategory.add(t); 1635 return t; 1636 } 1637 1638 public Appointment addServiceCategory(CodeableConcept t) { // 3 1639 if (t == null) 1640 return this; 1641 if (this.serviceCategory == null) 1642 this.serviceCategory = new ArrayList<CodeableConcept>(); 1643 this.serviceCategory.add(t); 1644 return this; 1645 } 1646 1647 /** 1648 * @return The first repetition of repeating field {@link #serviceCategory}, 1649 * creating it if it does not already exist 1650 */ 1651 public CodeableConcept getServiceCategoryFirstRep() { 1652 if (getServiceCategory().isEmpty()) { 1653 addServiceCategory(); 1654 } 1655 return getServiceCategory().get(0); 1656 } 1657 1658 /** 1659 * @return {@link #serviceType} (The specific service that is to be performed 1660 * during this appointment.) 1661 */ 1662 public List<CodeableConcept> getServiceType() { 1663 if (this.serviceType == null) 1664 this.serviceType = new ArrayList<CodeableConcept>(); 1665 return this.serviceType; 1666 } 1667 1668 /** 1669 * @return Returns a reference to <code>this</code> for easy method chaining 1670 */ 1671 public Appointment setServiceType(List<CodeableConcept> theServiceType) { 1672 this.serviceType = theServiceType; 1673 return this; 1674 } 1675 1676 public boolean hasServiceType() { 1677 if (this.serviceType == null) 1678 return false; 1679 for (CodeableConcept item : this.serviceType) 1680 if (!item.isEmpty()) 1681 return true; 1682 return false; 1683 } 1684 1685 public CodeableConcept addServiceType() { // 3 1686 CodeableConcept t = new CodeableConcept(); 1687 if (this.serviceType == null) 1688 this.serviceType = new ArrayList<CodeableConcept>(); 1689 this.serviceType.add(t); 1690 return t; 1691 } 1692 1693 public Appointment addServiceType(CodeableConcept t) { // 3 1694 if (t == null) 1695 return this; 1696 if (this.serviceType == null) 1697 this.serviceType = new ArrayList<CodeableConcept>(); 1698 this.serviceType.add(t); 1699 return this; 1700 } 1701 1702 /** 1703 * @return The first repetition of repeating field {@link #serviceType}, 1704 * creating it if it does not already exist 1705 */ 1706 public CodeableConcept getServiceTypeFirstRep() { 1707 if (getServiceType().isEmpty()) { 1708 addServiceType(); 1709 } 1710 return getServiceType().get(0); 1711 } 1712 1713 /** 1714 * @return {@link #specialty} (The specialty of a practitioner that would be 1715 * required to perform the service requested in this appointment.) 1716 */ 1717 public List<CodeableConcept> getSpecialty() { 1718 if (this.specialty == null) 1719 this.specialty = new ArrayList<CodeableConcept>(); 1720 return this.specialty; 1721 } 1722 1723 /** 1724 * @return Returns a reference to <code>this</code> for easy method chaining 1725 */ 1726 public Appointment setSpecialty(List<CodeableConcept> theSpecialty) { 1727 this.specialty = theSpecialty; 1728 return this; 1729 } 1730 1731 public boolean hasSpecialty() { 1732 if (this.specialty == null) 1733 return false; 1734 for (CodeableConcept item : this.specialty) 1735 if (!item.isEmpty()) 1736 return true; 1737 return false; 1738 } 1739 1740 public CodeableConcept addSpecialty() { // 3 1741 CodeableConcept t = new CodeableConcept(); 1742 if (this.specialty == null) 1743 this.specialty = new ArrayList<CodeableConcept>(); 1744 this.specialty.add(t); 1745 return t; 1746 } 1747 1748 public Appointment addSpecialty(CodeableConcept t) { // 3 1749 if (t == null) 1750 return this; 1751 if (this.specialty == null) 1752 this.specialty = new ArrayList<CodeableConcept>(); 1753 this.specialty.add(t); 1754 return this; 1755 } 1756 1757 /** 1758 * @return The first repetition of repeating field {@link #specialty}, creating 1759 * it if it does not already exist 1760 */ 1761 public CodeableConcept getSpecialtyFirstRep() { 1762 if (getSpecialty().isEmpty()) { 1763 addSpecialty(); 1764 } 1765 return getSpecialty().get(0); 1766 } 1767 1768 /** 1769 * @return {@link #appointmentType} (The style of appointment or patient that 1770 * has been booked in the slot (not service type).) 1771 */ 1772 public CodeableConcept getAppointmentType() { 1773 if (this.appointmentType == null) 1774 if (Configuration.errorOnAutoCreate()) 1775 throw new Error("Attempt to auto-create Appointment.appointmentType"); 1776 else if (Configuration.doAutoCreate()) 1777 this.appointmentType = new CodeableConcept(); // cc 1778 return this.appointmentType; 1779 } 1780 1781 public boolean hasAppointmentType() { 1782 return this.appointmentType != null && !this.appointmentType.isEmpty(); 1783 } 1784 1785 /** 1786 * @param value {@link #appointmentType} (The style of appointment or patient 1787 * that has been booked in the slot (not service type).) 1788 */ 1789 public Appointment setAppointmentType(CodeableConcept value) { 1790 this.appointmentType = value; 1791 return this; 1792 } 1793 1794 /** 1795 * @return {@link #reasonCode} (The coded reason that this appointment is being 1796 * scheduled. This is more clinical than administrative.) 1797 */ 1798 public List<CodeableConcept> getReasonCode() { 1799 if (this.reasonCode == null) 1800 this.reasonCode = new ArrayList<CodeableConcept>(); 1801 return this.reasonCode; 1802 } 1803 1804 /** 1805 * @return Returns a reference to <code>this</code> for easy method chaining 1806 */ 1807 public Appointment setReasonCode(List<CodeableConcept> theReasonCode) { 1808 this.reasonCode = theReasonCode; 1809 return this; 1810 } 1811 1812 public boolean hasReasonCode() { 1813 if (this.reasonCode == null) 1814 return false; 1815 for (CodeableConcept item : this.reasonCode) 1816 if (!item.isEmpty()) 1817 return true; 1818 return false; 1819 } 1820 1821 public CodeableConcept addReasonCode() { // 3 1822 CodeableConcept t = new CodeableConcept(); 1823 if (this.reasonCode == null) 1824 this.reasonCode = new ArrayList<CodeableConcept>(); 1825 this.reasonCode.add(t); 1826 return t; 1827 } 1828 1829 public Appointment addReasonCode(CodeableConcept t) { // 3 1830 if (t == null) 1831 return this; 1832 if (this.reasonCode == null) 1833 this.reasonCode = new ArrayList<CodeableConcept>(); 1834 this.reasonCode.add(t); 1835 return this; 1836 } 1837 1838 /** 1839 * @return The first repetition of repeating field {@link #reasonCode}, creating 1840 * it if it does not already exist 1841 */ 1842 public CodeableConcept getReasonCodeFirstRep() { 1843 if (getReasonCode().isEmpty()) { 1844 addReasonCode(); 1845 } 1846 return getReasonCode().get(0); 1847 } 1848 1849 /** 1850 * @return {@link #reasonReference} (Reason the appointment has been scheduled 1851 * to take place, as specified using information from another resource. 1852 * When the patient arrives and the encounter begins it may be used as 1853 * the admission diagnosis. The indication will typically be a Condition 1854 * (with other resources referenced in the evidence.detail), or a 1855 * Procedure.) 1856 */ 1857 public List<Reference> getReasonReference() { 1858 if (this.reasonReference == null) 1859 this.reasonReference = new ArrayList<Reference>(); 1860 return this.reasonReference; 1861 } 1862 1863 /** 1864 * @return Returns a reference to <code>this</code> for easy method chaining 1865 */ 1866 public Appointment setReasonReference(List<Reference> theReasonReference) { 1867 this.reasonReference = theReasonReference; 1868 return this; 1869 } 1870 1871 public boolean hasReasonReference() { 1872 if (this.reasonReference == null) 1873 return false; 1874 for (Reference item : this.reasonReference) 1875 if (!item.isEmpty()) 1876 return true; 1877 return false; 1878 } 1879 1880 public Reference addReasonReference() { // 3 1881 Reference t = new Reference(); 1882 if (this.reasonReference == null) 1883 this.reasonReference = new ArrayList<Reference>(); 1884 this.reasonReference.add(t); 1885 return t; 1886 } 1887 1888 public Appointment addReasonReference(Reference t) { // 3 1889 if (t == null) 1890 return this; 1891 if (this.reasonReference == null) 1892 this.reasonReference = new ArrayList<Reference>(); 1893 this.reasonReference.add(t); 1894 return this; 1895 } 1896 1897 /** 1898 * @return The first repetition of repeating field {@link #reasonReference}, 1899 * creating it if it does not already exist 1900 */ 1901 public Reference getReasonReferenceFirstRep() { 1902 if (getReasonReference().isEmpty()) { 1903 addReasonReference(); 1904 } 1905 return getReasonReference().get(0); 1906 } 1907 1908 /** 1909 * @return {@link #priority} (The priority of the appointment. Can be used to 1910 * make informed decisions if needing to re-prioritize appointments. 1911 * (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as 1912 * lowest priority).). This is the underlying object with id, value and 1913 * extensions. The accessor "getPriority" gives direct access to the 1914 * value 1915 */ 1916 public UnsignedIntType getPriorityElement() { 1917 if (this.priority == null) 1918 if (Configuration.errorOnAutoCreate()) 1919 throw new Error("Attempt to auto-create Appointment.priority"); 1920 else if (Configuration.doAutoCreate()) 1921 this.priority = new UnsignedIntType(); // bb 1922 return this.priority; 1923 } 1924 1925 public boolean hasPriorityElement() { 1926 return this.priority != null && !this.priority.isEmpty(); 1927 } 1928 1929 public boolean hasPriority() { 1930 return this.priority != null && !this.priority.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #priority} (The priority of the appointment. Can be used 1935 * to make informed decisions if needing to re-prioritize 1936 * appointments. (The iCal Standard specifies 0 as undefined, 1 as 1937 * highest, 9 as lowest priority).). This is the underlying object 1938 * with id, value and extensions. The accessor "getPriority" gives 1939 * direct access to the value 1940 */ 1941 public Appointment setPriorityElement(UnsignedIntType value) { 1942 this.priority = value; 1943 return this; 1944 } 1945 1946 /** 1947 * @return The priority of the appointment. Can be used to make informed 1948 * decisions if needing to re-prioritize appointments. (The iCal 1949 * Standard specifies 0 as undefined, 1 as highest, 9 as lowest 1950 * priority). 1951 */ 1952 public int getPriority() { 1953 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 1954 } 1955 1956 /** 1957 * @param value The priority of the appointment. Can be used to make informed 1958 * decisions if needing to re-prioritize appointments. (The iCal 1959 * Standard specifies 0 as undefined, 1 as highest, 9 as lowest 1960 * priority). 1961 */ 1962 public Appointment setPriority(int value) { 1963 if (this.priority == null) 1964 this.priority = new UnsignedIntType(); 1965 this.priority.setValue(value); 1966 return this; 1967 } 1968 1969 /** 1970 * @return {@link #description} (The brief description of the appointment as 1971 * would be shown on a subject line in a meeting request, or appointment 1972 * list. Detailed or expanded information should be put in the comment 1973 * field.). This is the underlying object with id, value and extensions. 1974 * The accessor "getDescription" gives direct access to the value 1975 */ 1976 public StringType getDescriptionElement() { 1977 if (this.description == null) 1978 if (Configuration.errorOnAutoCreate()) 1979 throw new Error("Attempt to auto-create Appointment.description"); 1980 else if (Configuration.doAutoCreate()) 1981 this.description = new StringType(); // bb 1982 return this.description; 1983 } 1984 1985 public boolean hasDescriptionElement() { 1986 return this.description != null && !this.description.isEmpty(); 1987 } 1988 1989 public boolean hasDescription() { 1990 return this.description != null && !this.description.isEmpty(); 1991 } 1992 1993 /** 1994 * @param value {@link #description} (The brief description of the appointment 1995 * as would be shown on a subject line in a meeting request, or 1996 * appointment list. Detailed or expanded information should be put 1997 * in the comment field.). This is the underlying object with id, 1998 * value and extensions. The accessor "getDescription" gives direct 1999 * access to the value 2000 */ 2001 public Appointment setDescriptionElement(StringType value) { 2002 this.description = value; 2003 return this; 2004 } 2005 2006 /** 2007 * @return The brief description of the appointment as would be shown on a 2008 * subject line in a meeting request, or appointment list. Detailed or 2009 * expanded information should be put in the comment field. 2010 */ 2011 public String getDescription() { 2012 return this.description == null ? null : this.description.getValue(); 2013 } 2014 2015 /** 2016 * @param value The brief description of the appointment as would be shown on a 2017 * subject line in a meeting request, or appointment list. Detailed 2018 * or expanded information should be put in the comment field. 2019 */ 2020 public Appointment setDescription(String value) { 2021 if (Utilities.noString(value)) 2022 this.description = null; 2023 else { 2024 if (this.description == null) 2025 this.description = new StringType(); 2026 this.description.setValue(value); 2027 } 2028 return this; 2029 } 2030 2031 /** 2032 * @return {@link #supportingInformation} (Additional information to support the 2033 * appointment provided when making the appointment.) 2034 */ 2035 public List<Reference> getSupportingInformation() { 2036 if (this.supportingInformation == null) 2037 this.supportingInformation = new ArrayList<Reference>(); 2038 return this.supportingInformation; 2039 } 2040 2041 /** 2042 * @return Returns a reference to <code>this</code> for easy method chaining 2043 */ 2044 public Appointment setSupportingInformation(List<Reference> theSupportingInformation) { 2045 this.supportingInformation = theSupportingInformation; 2046 return this; 2047 } 2048 2049 public boolean hasSupportingInformation() { 2050 if (this.supportingInformation == null) 2051 return false; 2052 for (Reference item : this.supportingInformation) 2053 if (!item.isEmpty()) 2054 return true; 2055 return false; 2056 } 2057 2058 public Reference addSupportingInformation() { // 3 2059 Reference t = new Reference(); 2060 if (this.supportingInformation == null) 2061 this.supportingInformation = new ArrayList<Reference>(); 2062 this.supportingInformation.add(t); 2063 return t; 2064 } 2065 2066 public Appointment addSupportingInformation(Reference t) { // 3 2067 if (t == null) 2068 return this; 2069 if (this.supportingInformation == null) 2070 this.supportingInformation = new ArrayList<Reference>(); 2071 this.supportingInformation.add(t); 2072 return this; 2073 } 2074 2075 /** 2076 * @return The first repetition of repeating field 2077 * {@link #supportingInformation}, creating it if it does not already 2078 * exist 2079 */ 2080 public Reference getSupportingInformationFirstRep() { 2081 if (getSupportingInformation().isEmpty()) { 2082 addSupportingInformation(); 2083 } 2084 return getSupportingInformation().get(0); 2085 } 2086 2087 /** 2088 * @return {@link #start} (Date/Time that the appointment is to take place.). 2089 * This is the underlying object with id, value and extensions. The 2090 * accessor "getStart" gives direct access to the value 2091 */ 2092 public InstantType getStartElement() { 2093 if (this.start == null) 2094 if (Configuration.errorOnAutoCreate()) 2095 throw new Error("Attempt to auto-create Appointment.start"); 2096 else if (Configuration.doAutoCreate()) 2097 this.start = new InstantType(); // bb 2098 return this.start; 2099 } 2100 2101 public boolean hasStartElement() { 2102 return this.start != null && !this.start.isEmpty(); 2103 } 2104 2105 public boolean hasStart() { 2106 return this.start != null && !this.start.isEmpty(); 2107 } 2108 2109 /** 2110 * @param value {@link #start} (Date/Time that the appointment is to take 2111 * place.). This is the underlying object with id, value and 2112 * extensions. The accessor "getStart" gives direct access to the 2113 * value 2114 */ 2115 public Appointment setStartElement(InstantType value) { 2116 this.start = value; 2117 return this; 2118 } 2119 2120 /** 2121 * @return Date/Time that the appointment is to take place. 2122 */ 2123 public Date getStart() { 2124 return this.start == null ? null : this.start.getValue(); 2125 } 2126 2127 /** 2128 * @param value Date/Time that the appointment is to take place. 2129 */ 2130 public Appointment setStart(Date value) { 2131 if (value == null) 2132 this.start = null; 2133 else { 2134 if (this.start == null) 2135 this.start = new InstantType(); 2136 this.start.setValue(value); 2137 } 2138 return this; 2139 } 2140 2141 /** 2142 * @return {@link #end} (Date/Time that the appointment is to conclude.). This 2143 * is the underlying object with id, value and extensions. The accessor 2144 * "getEnd" gives direct access to the value 2145 */ 2146 public InstantType getEndElement() { 2147 if (this.end == null) 2148 if (Configuration.errorOnAutoCreate()) 2149 throw new Error("Attempt to auto-create Appointment.end"); 2150 else if (Configuration.doAutoCreate()) 2151 this.end = new InstantType(); // bb 2152 return this.end; 2153 } 2154 2155 public boolean hasEndElement() { 2156 return this.end != null && !this.end.isEmpty(); 2157 } 2158 2159 public boolean hasEnd() { 2160 return this.end != null && !this.end.isEmpty(); 2161 } 2162 2163 /** 2164 * @param value {@link #end} (Date/Time that the appointment is to conclude.). 2165 * This is the underlying object with id, value and extensions. The 2166 * accessor "getEnd" gives direct access to the value 2167 */ 2168 public Appointment setEndElement(InstantType value) { 2169 this.end = value; 2170 return this; 2171 } 2172 2173 /** 2174 * @return Date/Time that the appointment is to conclude. 2175 */ 2176 public Date getEnd() { 2177 return this.end == null ? null : this.end.getValue(); 2178 } 2179 2180 /** 2181 * @param value Date/Time that the appointment is to conclude. 2182 */ 2183 public Appointment setEnd(Date value) { 2184 if (value == null) 2185 this.end = null; 2186 else { 2187 if (this.end == null) 2188 this.end = new InstantType(); 2189 this.end.setValue(value); 2190 } 2191 return this; 2192 } 2193 2194 /** 2195 * @return {@link #minutesDuration} (Number of minutes that the appointment is 2196 * to take. This can be less than the duration between the start and end 2197 * times. For example, where the actual time of appointment is only an 2198 * estimate or if a 30 minute appointment is being requested, but any 2199 * time would work. Also, if there is, for example, a planned 15 minute 2200 * break in the middle of a long appointment, the duration may be 15 2201 * minutes less than the difference between the start and end.). This is 2202 * the underlying object with id, value and extensions. The accessor 2203 * "getMinutesDuration" gives direct access to the value 2204 */ 2205 public PositiveIntType getMinutesDurationElement() { 2206 if (this.minutesDuration == null) 2207 if (Configuration.errorOnAutoCreate()) 2208 throw new Error("Attempt to auto-create Appointment.minutesDuration"); 2209 else if (Configuration.doAutoCreate()) 2210 this.minutesDuration = new PositiveIntType(); // bb 2211 return this.minutesDuration; 2212 } 2213 2214 public boolean hasMinutesDurationElement() { 2215 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 2216 } 2217 2218 public boolean hasMinutesDuration() { 2219 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 2220 } 2221 2222 /** 2223 * @param value {@link #minutesDuration} (Number of minutes that the appointment 2224 * is to take. This can be less than the duration between the start 2225 * and end times. For example, where the actual time of appointment 2226 * is only an estimate or if a 30 minute appointment is being 2227 * requested, but any time would work. Also, if there is, for 2228 * example, a planned 15 minute break in the middle of a long 2229 * appointment, the duration may be 15 minutes less than the 2230 * difference between the start and end.). This is the underlying 2231 * object with id, value and extensions. The accessor 2232 * "getMinutesDuration" gives direct access to the value 2233 */ 2234 public Appointment setMinutesDurationElement(PositiveIntType value) { 2235 this.minutesDuration = value; 2236 return this; 2237 } 2238 2239 /** 2240 * @return Number of minutes that the appointment is to take. This can be less 2241 * than the duration between the start and end times. For example, where 2242 * the actual time of appointment is only an estimate or if a 30 minute 2243 * appointment is being requested, but any time would work. Also, if 2244 * there is, for example, a planned 15 minute break in the middle of a 2245 * long appointment, the duration may be 15 minutes less than the 2246 * difference between the start and end. 2247 */ 2248 public int getMinutesDuration() { 2249 return this.minutesDuration == null || this.minutesDuration.isEmpty() ? 0 : this.minutesDuration.getValue(); 2250 } 2251 2252 /** 2253 * @param value Number of minutes that the appointment is to take. This can be 2254 * less than the duration between the start and end times. For 2255 * example, where the actual time of appointment is only an 2256 * estimate or if a 30 minute appointment is being requested, but 2257 * any time would work. Also, if there is, for example, a planned 2258 * 15 minute break in the middle of a long appointment, the 2259 * duration may be 15 minutes less than the difference between the 2260 * start and end. 2261 */ 2262 public Appointment setMinutesDuration(int value) { 2263 if (this.minutesDuration == null) 2264 this.minutesDuration = new PositiveIntType(); 2265 this.minutesDuration.setValue(value); 2266 return this; 2267 } 2268 2269 /** 2270 * @return {@link #slot} (The slots from the participants' schedules that will 2271 * be filled by the appointment.) 2272 */ 2273 public List<Reference> getSlot() { 2274 if (this.slot == null) 2275 this.slot = new ArrayList<Reference>(); 2276 return this.slot; 2277 } 2278 2279 /** 2280 * @return Returns a reference to <code>this</code> for easy method chaining 2281 */ 2282 public Appointment setSlot(List<Reference> theSlot) { 2283 this.slot = theSlot; 2284 return this; 2285 } 2286 2287 public boolean hasSlot() { 2288 if (this.slot == null) 2289 return false; 2290 for (Reference item : this.slot) 2291 if (!item.isEmpty()) 2292 return true; 2293 return false; 2294 } 2295 2296 public Reference addSlot() { // 3 2297 Reference t = new Reference(); 2298 if (this.slot == null) 2299 this.slot = new ArrayList<Reference>(); 2300 this.slot.add(t); 2301 return t; 2302 } 2303 2304 public Appointment addSlot(Reference t) { // 3 2305 if (t == null) 2306 return this; 2307 if (this.slot == null) 2308 this.slot = new ArrayList<Reference>(); 2309 this.slot.add(t); 2310 return this; 2311 } 2312 2313 /** 2314 * @return The first repetition of repeating field {@link #slot}, creating it if 2315 * it does not already exist 2316 */ 2317 public Reference getSlotFirstRep() { 2318 if (getSlot().isEmpty()) { 2319 addSlot(); 2320 } 2321 return getSlot().get(0); 2322 } 2323 2324 /** 2325 * @return {@link #created} (The date that this appointment was initially 2326 * created. This could be different to the meta.lastModified value on 2327 * the initial entry, as this could have been before the resource was 2328 * created on the FHIR server, and should remain unchanged over the 2329 * lifespan of the appointment.). This is the underlying object with id, 2330 * value and extensions. The accessor "getCreated" gives direct access 2331 * to the value 2332 */ 2333 public DateTimeType getCreatedElement() { 2334 if (this.created == null) 2335 if (Configuration.errorOnAutoCreate()) 2336 throw new Error("Attempt to auto-create Appointment.created"); 2337 else if (Configuration.doAutoCreate()) 2338 this.created = new DateTimeType(); // bb 2339 return this.created; 2340 } 2341 2342 public boolean hasCreatedElement() { 2343 return this.created != null && !this.created.isEmpty(); 2344 } 2345 2346 public boolean hasCreated() { 2347 return this.created != null && !this.created.isEmpty(); 2348 } 2349 2350 /** 2351 * @param value {@link #created} (The date that this appointment was initially 2352 * created. This could be different to the meta.lastModified value 2353 * on the initial entry, as this could have been before the 2354 * resource was created on the FHIR server, and should remain 2355 * unchanged over the lifespan of the appointment.). This is the 2356 * underlying object with id, value and extensions. The accessor 2357 * "getCreated" gives direct access to the value 2358 */ 2359 public Appointment setCreatedElement(DateTimeType value) { 2360 this.created = value; 2361 return this; 2362 } 2363 2364 /** 2365 * @return The date that this appointment was initially created. This could be 2366 * different to the meta.lastModified value on the initial entry, as 2367 * this could have been before the resource was created on the FHIR 2368 * server, and should remain unchanged over the lifespan of the 2369 * appointment. 2370 */ 2371 public Date getCreated() { 2372 return this.created == null ? null : this.created.getValue(); 2373 } 2374 2375 /** 2376 * @param value The date that this appointment was initially created. This could 2377 * be different to the meta.lastModified value on the initial 2378 * entry, as this could have been before the resource was created 2379 * on the FHIR server, and should remain unchanged over the 2380 * lifespan of the appointment. 2381 */ 2382 public Appointment setCreated(Date value) { 2383 if (value == null) 2384 this.created = null; 2385 else { 2386 if (this.created == null) 2387 this.created = new DateTimeType(); 2388 this.created.setValue(value); 2389 } 2390 return this; 2391 } 2392 2393 /** 2394 * @return {@link #comment} (Additional comments about the appointment.). This 2395 * is the underlying object with id, value and extensions. The accessor 2396 * "getComment" gives direct access to the value 2397 */ 2398 public StringType getCommentElement() { 2399 if (this.comment == null) 2400 if (Configuration.errorOnAutoCreate()) 2401 throw new Error("Attempt to auto-create Appointment.comment"); 2402 else if (Configuration.doAutoCreate()) 2403 this.comment = new StringType(); // bb 2404 return this.comment; 2405 } 2406 2407 public boolean hasCommentElement() { 2408 return this.comment != null && !this.comment.isEmpty(); 2409 } 2410 2411 public boolean hasComment() { 2412 return this.comment != null && !this.comment.isEmpty(); 2413 } 2414 2415 /** 2416 * @param value {@link #comment} (Additional comments about the appointment.). 2417 * This is the underlying object with id, value and extensions. The 2418 * accessor "getComment" gives direct access to the value 2419 */ 2420 public Appointment setCommentElement(StringType value) { 2421 this.comment = value; 2422 return this; 2423 } 2424 2425 /** 2426 * @return Additional comments about the appointment. 2427 */ 2428 public String getComment() { 2429 return this.comment == null ? null : this.comment.getValue(); 2430 } 2431 2432 /** 2433 * @param value Additional comments about the appointment. 2434 */ 2435 public Appointment setComment(String value) { 2436 if (Utilities.noString(value)) 2437 this.comment = null; 2438 else { 2439 if (this.comment == null) 2440 this.comment = new StringType(); 2441 this.comment.setValue(value); 2442 } 2443 return this; 2444 } 2445 2446 /** 2447 * @return {@link #patientInstruction} (While Appointment.comment contains 2448 * information for internal use, Appointment.patientInstructions is used 2449 * to capture patient facing information about the Appointment (e.g. 2450 * please bring your referral or fast from 8pm night before).). This is 2451 * the underlying object with id, value and extensions. The accessor 2452 * "getPatientInstruction" gives direct access to the value 2453 */ 2454 public StringType getPatientInstructionElement() { 2455 if (this.patientInstruction == null) 2456 if (Configuration.errorOnAutoCreate()) 2457 throw new Error("Attempt to auto-create Appointment.patientInstruction"); 2458 else if (Configuration.doAutoCreate()) 2459 this.patientInstruction = new StringType(); // bb 2460 return this.patientInstruction; 2461 } 2462 2463 public boolean hasPatientInstructionElement() { 2464 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 2465 } 2466 2467 public boolean hasPatientInstruction() { 2468 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 2469 } 2470 2471 /** 2472 * @param value {@link #patientInstruction} (While Appointment.comment contains 2473 * information for internal use, Appointment.patientInstructions is 2474 * used to capture patient facing information about the Appointment 2475 * (e.g. please bring your referral or fast from 8pm night 2476 * before).). This is the underlying object with id, value and 2477 * extensions. The accessor "getPatientInstruction" gives direct 2478 * access to the value 2479 */ 2480 public Appointment setPatientInstructionElement(StringType value) { 2481 this.patientInstruction = value; 2482 return this; 2483 } 2484 2485 /** 2486 * @return While Appointment.comment contains information for internal use, 2487 * Appointment.patientInstructions is used to capture patient facing 2488 * information about the Appointment (e.g. please bring your referral or 2489 * fast from 8pm night before). 2490 */ 2491 public String getPatientInstruction() { 2492 return this.patientInstruction == null ? null : this.patientInstruction.getValue(); 2493 } 2494 2495 /** 2496 * @param value While Appointment.comment contains information for internal use, 2497 * Appointment.patientInstructions is used to capture patient 2498 * facing information about the Appointment (e.g. please bring your 2499 * referral or fast from 8pm night before). 2500 */ 2501 public Appointment setPatientInstruction(String value) { 2502 if (Utilities.noString(value)) 2503 this.patientInstruction = null; 2504 else { 2505 if (this.patientInstruction == null) 2506 this.patientInstruction = new StringType(); 2507 this.patientInstruction.setValue(value); 2508 } 2509 return this; 2510 } 2511 2512 /** 2513 * @return {@link #basedOn} (The service request this appointment is allocated 2514 * to assess (e.g. incoming referral or procedure request).) 2515 */ 2516 public List<Reference> getBasedOn() { 2517 if (this.basedOn == null) 2518 this.basedOn = new ArrayList<Reference>(); 2519 return this.basedOn; 2520 } 2521 2522 /** 2523 * @return Returns a reference to <code>this</code> for easy method chaining 2524 */ 2525 public Appointment setBasedOn(List<Reference> theBasedOn) { 2526 this.basedOn = theBasedOn; 2527 return this; 2528 } 2529 2530 public boolean hasBasedOn() { 2531 if (this.basedOn == null) 2532 return false; 2533 for (Reference item : this.basedOn) 2534 if (!item.isEmpty()) 2535 return true; 2536 return false; 2537 } 2538 2539 public Reference addBasedOn() { // 3 2540 Reference t = new Reference(); 2541 if (this.basedOn == null) 2542 this.basedOn = new ArrayList<Reference>(); 2543 this.basedOn.add(t); 2544 return t; 2545 } 2546 2547 public Appointment addBasedOn(Reference t) { // 3 2548 if (t == null) 2549 return this; 2550 if (this.basedOn == null) 2551 this.basedOn = new ArrayList<Reference>(); 2552 this.basedOn.add(t); 2553 return this; 2554 } 2555 2556 /** 2557 * @return The first repetition of repeating field {@link #basedOn}, creating it 2558 * if it does not already exist 2559 */ 2560 public Reference getBasedOnFirstRep() { 2561 if (getBasedOn().isEmpty()) { 2562 addBasedOn(); 2563 } 2564 return getBasedOn().get(0); 2565 } 2566 2567 /** 2568 * @return {@link #participant} (List of participants involved in the 2569 * appointment.) 2570 */ 2571 public List<AppointmentParticipantComponent> getParticipant() { 2572 if (this.participant == null) 2573 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2574 return this.participant; 2575 } 2576 2577 /** 2578 * @return Returns a reference to <code>this</code> for easy method chaining 2579 */ 2580 public Appointment setParticipant(List<AppointmentParticipantComponent> theParticipant) { 2581 this.participant = theParticipant; 2582 return this; 2583 } 2584 2585 public boolean hasParticipant() { 2586 if (this.participant == null) 2587 return false; 2588 for (AppointmentParticipantComponent item : this.participant) 2589 if (!item.isEmpty()) 2590 return true; 2591 return false; 2592 } 2593 2594 public AppointmentParticipantComponent addParticipant() { // 3 2595 AppointmentParticipantComponent t = new AppointmentParticipantComponent(); 2596 if (this.participant == null) 2597 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2598 this.participant.add(t); 2599 return t; 2600 } 2601 2602 public Appointment addParticipant(AppointmentParticipantComponent t) { // 3 2603 if (t == null) 2604 return this; 2605 if (this.participant == null) 2606 this.participant = new ArrayList<AppointmentParticipantComponent>(); 2607 this.participant.add(t); 2608 return this; 2609 } 2610 2611 /** 2612 * @return The first repetition of repeating field {@link #participant}, 2613 * creating it if it does not already exist 2614 */ 2615 public AppointmentParticipantComponent getParticipantFirstRep() { 2616 if (getParticipant().isEmpty()) { 2617 addParticipant(); 2618 } 2619 return getParticipant().get(0); 2620 } 2621 2622 /** 2623 * @return {@link #requestedPeriod} (A set of date ranges (potentially including 2624 * times) that the appointment is preferred to be scheduled within. 2625 * 2626 * The duration (usually in minutes) could also be provided to indicate 2627 * the length of the appointment to fill and populate the start/end 2628 * times for the actual allocated time. However, in other situations the 2629 * duration may be calculated by the scheduling system.) 2630 */ 2631 public List<Period> getRequestedPeriod() { 2632 if (this.requestedPeriod == null) 2633 this.requestedPeriod = new ArrayList<Period>(); 2634 return this.requestedPeriod; 2635 } 2636 2637 /** 2638 * @return Returns a reference to <code>this</code> for easy method chaining 2639 */ 2640 public Appointment setRequestedPeriod(List<Period> theRequestedPeriod) { 2641 this.requestedPeriod = theRequestedPeriod; 2642 return this; 2643 } 2644 2645 public boolean hasRequestedPeriod() { 2646 if (this.requestedPeriod == null) 2647 return false; 2648 for (Period item : this.requestedPeriod) 2649 if (!item.isEmpty()) 2650 return true; 2651 return false; 2652 } 2653 2654 public Period addRequestedPeriod() { // 3 2655 Period t = new Period(); 2656 if (this.requestedPeriod == null) 2657 this.requestedPeriod = new ArrayList<Period>(); 2658 this.requestedPeriod.add(t); 2659 return t; 2660 } 2661 2662 public Appointment addRequestedPeriod(Period t) { // 3 2663 if (t == null) 2664 return this; 2665 if (this.requestedPeriod == null) 2666 this.requestedPeriod = new ArrayList<Period>(); 2667 this.requestedPeriod.add(t); 2668 return this; 2669 } 2670 2671 /** 2672 * @return The first repetition of repeating field {@link #requestedPeriod}, 2673 * creating it if it does not already exist 2674 */ 2675 public Period getRequestedPeriodFirstRep() { 2676 if (getRequestedPeriod().isEmpty()) { 2677 addRequestedPeriod(); 2678 } 2679 return getRequestedPeriod().get(0); 2680 } 2681 2682 protected void listChildren(List<Property> children) { 2683 super.listChildren(children); 2684 children.add(new Property("identifier", "Identifier", 2685 "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2686 0, java.lang.Integer.MAX_VALUE, identifier)); 2687 children.add(new Property("status", "code", 2688 "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 2689 0, 1, status)); 2690 children.add(new Property("cancelationReason", "CodeableConcept", 2691 "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.", 2692 0, 1, cancelationReason)); 2693 children.add(new Property("serviceCategory", "CodeableConcept", 2694 "A broad categorization of the service that is to be performed during this appointment.", 0, 2695 java.lang.Integer.MAX_VALUE, serviceCategory)); 2696 children.add(new Property("serviceType", "CodeableConcept", 2697 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 2698 serviceType)); 2699 children.add(new Property("specialty", "CodeableConcept", 2700 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 2701 0, java.lang.Integer.MAX_VALUE, specialty)); 2702 children.add(new Property("appointmentType", "CodeableConcept", 2703 "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, 2704 appointmentType)); 2705 children.add(new Property("reasonCode", "CodeableConcept", 2706 "The coded reason that this appointment is being scheduled. This is more clinical than administrative.", 0, 2707 java.lang.Integer.MAX_VALUE, reasonCode)); 2708 children.add(new Property("reasonReference", 2709 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 2710 "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 2711 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2712 children.add(new Property("priority", "unsignedInt", 2713 "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 2714 0, 1, priority)); 2715 children.add(new Property("description", "string", 2716 "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 2717 0, 1, description)); 2718 children.add(new Property("supportingInformation", "Reference(Any)", 2719 "Additional information to support the appointment provided when making the appointment.", 0, 2720 java.lang.Integer.MAX_VALUE, supportingInformation)); 2721 children.add(new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, start)); 2722 children.add(new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end)); 2723 children.add(new Property("minutesDuration", "positiveInt", 2724 "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.", 2725 0, 1, minutesDuration)); 2726 children.add(new Property("slot", "Reference(Slot)", 2727 "The slots from the participants' schedules that will be filled by the appointment.", 0, 2728 java.lang.Integer.MAX_VALUE, slot)); 2729 children.add(new Property("created", "dateTime", 2730 "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 2731 0, 1, created)); 2732 children.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment)); 2733 children.add(new Property("patientInstruction", "string", 2734 "While Appointment.comment contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).", 2735 0, 1, patientInstruction)); 2736 children.add(new Property("basedOn", "Reference(ServiceRequest)", 2737 "The service request this appointment is allocated to assess (e.g. incoming referral or procedure request).", 0, 2738 java.lang.Integer.MAX_VALUE, basedOn)); 2739 children.add(new Property("participant", "", "List of participants involved in the appointment.", 0, 2740 java.lang.Integer.MAX_VALUE, participant)); 2741 children.add(new Property("requestedPeriod", "Period", 2742 "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.", 2743 0, java.lang.Integer.MAX_VALUE, requestedPeriod)); 2744 } 2745 2746 @Override 2747 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2748 switch (_hash) { 2749 case -1618432855: 2750 /* identifier */ return new Property("identifier", "Identifier", 2751 "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2752 0, java.lang.Integer.MAX_VALUE, identifier); 2753 case -892481550: 2754 /* status */ return new Property("status", "code", 2755 "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 2756 0, 1, status); 2757 case 987811551: 2758 /* cancelationReason */ return new Property("cancelationReason", "CodeableConcept", 2759 "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.", 2760 0, 1, cancelationReason); 2761 case 1281188563: 2762 /* serviceCategory */ return new Property("serviceCategory", "CodeableConcept", 2763 "A broad categorization of the service that is to be performed during this appointment.", 0, 2764 java.lang.Integer.MAX_VALUE, serviceCategory); 2765 case -1928370289: 2766 /* serviceType */ return new Property("serviceType", "CodeableConcept", 2767 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 2768 serviceType); 2769 case -1694759682: 2770 /* specialty */ return new Property("specialty", "CodeableConcept", 2771 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 2772 0, java.lang.Integer.MAX_VALUE, specialty); 2773 case -1596426375: 2774 /* appointmentType */ return new Property("appointmentType", "CodeableConcept", 2775 "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, 2776 appointmentType); 2777 case 722137681: 2778 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2779 "The coded reason that this appointment is being scheduled. This is more clinical than administrative.", 0, 2780 java.lang.Integer.MAX_VALUE, reasonCode); 2781 case -1146218137: 2782 /* reasonReference */ return new Property("reasonReference", 2783 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 2784 "Reason the appointment has been scheduled to take place, as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 2785 0, java.lang.Integer.MAX_VALUE, reasonReference); 2786 case -1165461084: 2787 /* priority */ return new Property("priority", "unsignedInt", 2788 "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 2789 0, 1, priority); 2790 case -1724546052: 2791 /* description */ return new Property("description", "string", 2792 "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 2793 0, 1, description); 2794 case -1248768647: 2795 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2796 "Additional information to support the appointment provided when making the appointment.", 0, 2797 java.lang.Integer.MAX_VALUE, supportingInformation); 2798 case 109757538: 2799 /* start */ return new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, 2800 start); 2801 case 100571: 2802 /* end */ return new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end); 2803 case -413630573: 2804 /* minutesDuration */ return new Property("minutesDuration", "positiveInt", 2805 "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.", 2806 0, 1, minutesDuration); 2807 case 3533310: 2808 /* slot */ return new Property("slot", "Reference(Slot)", 2809 "The slots from the participants' schedules that will be filled by the appointment.", 0, 2810 java.lang.Integer.MAX_VALUE, slot); 2811 case 1028554472: 2812 /* created */ return new Property("created", "dateTime", 2813 "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 2814 0, 1, created); 2815 case 950398559: 2816 /* comment */ return new Property("comment", "string", "Additional comments about the appointment.", 0, 1, 2817 comment); 2818 case 737543241: 2819 /* patientInstruction */ return new Property("patientInstruction", "string", 2820 "While Appointment.comment contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).", 2821 0, 1, patientInstruction); 2822 case -332612366: 2823 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest)", 2824 "The service request this appointment is allocated to assess (e.g. incoming referral or procedure request).", 2825 0, java.lang.Integer.MAX_VALUE, basedOn); 2826 case 767422259: 2827 /* participant */ return new Property("participant", "", "List of participants involved in the appointment.", 0, 2828 java.lang.Integer.MAX_VALUE, participant); 2829 case -897241393: 2830 /* requestedPeriod */ return new Property("requestedPeriod", "Period", 2831 "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.", 2832 0, java.lang.Integer.MAX_VALUE, requestedPeriod); 2833 default: 2834 return super.getNamedProperty(_hash, _name, _checkValid); 2835 } 2836 2837 } 2838 2839 @Override 2840 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2841 switch (hash) { 2842 case -1618432855: 2843 /* identifier */ return this.identifier == null ? new Base[0] 2844 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2845 case -892481550: 2846 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<AppointmentStatus> 2847 case 987811551: 2848 /* cancelationReason */ return this.cancelationReason == null ? new Base[0] 2849 : new Base[] { this.cancelationReason }; // CodeableConcept 2850 case 1281188563: 2851 /* serviceCategory */ return this.serviceCategory == null ? new Base[0] 2852 : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 2853 case -1928370289: 2854 /* serviceType */ return this.serviceType == null ? new Base[0] 2855 : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 2856 case -1694759682: 2857 /* specialty */ return this.specialty == null ? new Base[0] 2858 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 2859 case -1596426375: 2860 /* appointmentType */ return this.appointmentType == null ? new Base[0] : new Base[] { this.appointmentType }; // CodeableConcept 2861 case 722137681: 2862 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2863 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2864 case -1146218137: 2865 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2866 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2867 case -1165461084: 2868 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // UnsignedIntType 2869 case -1724546052: 2870 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2871 case -1248768647: 2872 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2873 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2874 case 109757538: 2875 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // InstantType 2876 case 100571: 2877 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // InstantType 2878 case -413630573: 2879 /* minutesDuration */ return this.minutesDuration == null ? new Base[0] : new Base[] { this.minutesDuration }; // PositiveIntType 2880 case 3533310: 2881 /* slot */ return this.slot == null ? new Base[0] : this.slot.toArray(new Base[this.slot.size()]); // Reference 2882 case 1028554472: 2883 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 2884 case 950398559: 2885 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2886 case 737543241: 2887 /* patientInstruction */ return this.patientInstruction == null ? new Base[0] 2888 : new Base[] { this.patientInstruction }; // StringType 2889 case -332612366: 2890 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2891 case 767422259: 2892 /* participant */ return this.participant == null ? new Base[0] 2893 : this.participant.toArray(new Base[this.participant.size()]); // AppointmentParticipantComponent 2894 case -897241393: 2895 /* requestedPeriod */ return this.requestedPeriod == null ? new Base[0] 2896 : this.requestedPeriod.toArray(new Base[this.requestedPeriod.size()]); // Period 2897 default: 2898 return super.getProperty(hash, name, checkValid); 2899 } 2900 2901 } 2902 2903 @Override 2904 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2905 switch (hash) { 2906 case -1618432855: // identifier 2907 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2908 return value; 2909 case -892481550: // status 2910 value = new AppointmentStatusEnumFactory().fromType(castToCode(value)); 2911 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 2912 return value; 2913 case 987811551: // cancelationReason 2914 this.cancelationReason = castToCodeableConcept(value); // CodeableConcept 2915 return value; 2916 case 1281188563: // serviceCategory 2917 this.getServiceCategory().add(castToCodeableConcept(value)); // CodeableConcept 2918 return value; 2919 case -1928370289: // serviceType 2920 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 2921 return value; 2922 case -1694759682: // specialty 2923 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 2924 return value; 2925 case -1596426375: // appointmentType 2926 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 2927 return value; 2928 case 722137681: // reasonCode 2929 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2930 return value; 2931 case -1146218137: // reasonReference 2932 this.getReasonReference().add(castToReference(value)); // Reference 2933 return value; 2934 case -1165461084: // priority 2935 this.priority = castToUnsignedInt(value); // UnsignedIntType 2936 return value; 2937 case -1724546052: // description 2938 this.description = castToString(value); // StringType 2939 return value; 2940 case -1248768647: // supportingInformation 2941 this.getSupportingInformation().add(castToReference(value)); // Reference 2942 return value; 2943 case 109757538: // start 2944 this.start = castToInstant(value); // InstantType 2945 return value; 2946 case 100571: // end 2947 this.end = castToInstant(value); // InstantType 2948 return value; 2949 case -413630573: // minutesDuration 2950 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 2951 return value; 2952 case 3533310: // slot 2953 this.getSlot().add(castToReference(value)); // Reference 2954 return value; 2955 case 1028554472: // created 2956 this.created = castToDateTime(value); // DateTimeType 2957 return value; 2958 case 950398559: // comment 2959 this.comment = castToString(value); // StringType 2960 return value; 2961 case 737543241: // patientInstruction 2962 this.patientInstruction = castToString(value); // StringType 2963 return value; 2964 case -332612366: // basedOn 2965 this.getBasedOn().add(castToReference(value)); // Reference 2966 return value; 2967 case 767422259: // participant 2968 this.getParticipant().add((AppointmentParticipantComponent) value); // AppointmentParticipantComponent 2969 return value; 2970 case -897241393: // requestedPeriod 2971 this.getRequestedPeriod().add(castToPeriod(value)); // Period 2972 return value; 2973 default: 2974 return super.setProperty(hash, name, value); 2975 } 2976 2977 } 2978 2979 @Override 2980 public Base setProperty(String name, Base value) throws FHIRException { 2981 if (name.equals("identifier")) { 2982 this.getIdentifier().add(castToIdentifier(value)); 2983 } else if (name.equals("status")) { 2984 value = new AppointmentStatusEnumFactory().fromType(castToCode(value)); 2985 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 2986 } else if (name.equals("cancelationReason")) { 2987 this.cancelationReason = castToCodeableConcept(value); // CodeableConcept 2988 } else if (name.equals("serviceCategory")) { 2989 this.getServiceCategory().add(castToCodeableConcept(value)); 2990 } else if (name.equals("serviceType")) { 2991 this.getServiceType().add(castToCodeableConcept(value)); 2992 } else if (name.equals("specialty")) { 2993 this.getSpecialty().add(castToCodeableConcept(value)); 2994 } else if (name.equals("appointmentType")) { 2995 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 2996 } else if (name.equals("reasonCode")) { 2997 this.getReasonCode().add(castToCodeableConcept(value)); 2998 } else if (name.equals("reasonReference")) { 2999 this.getReasonReference().add(castToReference(value)); 3000 } else if (name.equals("priority")) { 3001 this.priority = castToUnsignedInt(value); // UnsignedIntType 3002 } else if (name.equals("description")) { 3003 this.description = castToString(value); // StringType 3004 } else if (name.equals("supportingInformation")) { 3005 this.getSupportingInformation().add(castToReference(value)); 3006 } else if (name.equals("start")) { 3007 this.start = castToInstant(value); // InstantType 3008 } else if (name.equals("end")) { 3009 this.end = castToInstant(value); // InstantType 3010 } else if (name.equals("minutesDuration")) { 3011 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 3012 } else if (name.equals("slot")) { 3013 this.getSlot().add(castToReference(value)); 3014 } else if (name.equals("created")) { 3015 this.created = castToDateTime(value); // DateTimeType 3016 } else if (name.equals("comment")) { 3017 this.comment = castToString(value); // StringType 3018 } else if (name.equals("patientInstruction")) { 3019 this.patientInstruction = castToString(value); // StringType 3020 } else if (name.equals("basedOn")) { 3021 this.getBasedOn().add(castToReference(value)); 3022 } else if (name.equals("participant")) { 3023 this.getParticipant().add((AppointmentParticipantComponent) value); 3024 } else if (name.equals("requestedPeriod")) { 3025 this.getRequestedPeriod().add(castToPeriod(value)); 3026 } else 3027 return super.setProperty(name, value); 3028 return value; 3029 } 3030 3031 @Override 3032 public void removeChild(String name, Base value) throws FHIRException { 3033 if (name.equals("identifier")) { 3034 this.getIdentifier().remove(castToIdentifier(value)); 3035 } else if (name.equals("status")) { 3036 this.status = null; 3037 } else if (name.equals("cancelationReason")) { 3038 this.cancelationReason = null; 3039 } else if (name.equals("serviceCategory")) { 3040 this.getServiceCategory().remove(castToCodeableConcept(value)); 3041 } else if (name.equals("serviceType")) { 3042 this.getServiceType().remove(castToCodeableConcept(value)); 3043 } else if (name.equals("specialty")) { 3044 this.getSpecialty().remove(castToCodeableConcept(value)); 3045 } else if (name.equals("appointmentType")) { 3046 this.appointmentType = null; 3047 } else if (name.equals("reasonCode")) { 3048 this.getReasonCode().remove(castToCodeableConcept(value)); 3049 } else if (name.equals("reasonReference")) { 3050 this.getReasonReference().remove(castToReference(value)); 3051 } else if (name.equals("priority")) { 3052 this.priority = null; 3053 } else if (name.equals("description")) { 3054 this.description = null; 3055 } else if (name.equals("supportingInformation")) { 3056 this.getSupportingInformation().remove(castToReference(value)); 3057 } else if (name.equals("start")) { 3058 this.start = null; 3059 } else if (name.equals("end")) { 3060 this.end = null; 3061 } else if (name.equals("minutesDuration")) { 3062 this.minutesDuration = null; 3063 } else if (name.equals("slot")) { 3064 this.getSlot().remove(castToReference(value)); 3065 } else if (name.equals("created")) { 3066 this.created = null; 3067 } else if (name.equals("comment")) { 3068 this.comment = null; 3069 } else if (name.equals("patientInstruction")) { 3070 this.patientInstruction = null; 3071 } else if (name.equals("basedOn")) { 3072 this.getBasedOn().remove(castToReference(value)); 3073 } else if (name.equals("participant")) { 3074 this.getParticipant().remove((AppointmentParticipantComponent) value); 3075 } else if (name.equals("requestedPeriod")) { 3076 this.getRequestedPeriod().remove(castToPeriod(value)); 3077 } else 3078 super.removeChild(name, value); 3079 3080 } 3081 3082 @Override 3083 public Base makeProperty(int hash, String name) throws FHIRException { 3084 switch (hash) { 3085 case -1618432855: 3086 return addIdentifier(); 3087 case -892481550: 3088 return getStatusElement(); 3089 case 987811551: 3090 return getCancelationReason(); 3091 case 1281188563: 3092 return addServiceCategory(); 3093 case -1928370289: 3094 return addServiceType(); 3095 case -1694759682: 3096 return addSpecialty(); 3097 case -1596426375: 3098 return getAppointmentType(); 3099 case 722137681: 3100 return addReasonCode(); 3101 case -1146218137: 3102 return addReasonReference(); 3103 case -1165461084: 3104 return getPriorityElement(); 3105 case -1724546052: 3106 return getDescriptionElement(); 3107 case -1248768647: 3108 return addSupportingInformation(); 3109 case 109757538: 3110 return getStartElement(); 3111 case 100571: 3112 return getEndElement(); 3113 case -413630573: 3114 return getMinutesDurationElement(); 3115 case 3533310: 3116 return addSlot(); 3117 case 1028554472: 3118 return getCreatedElement(); 3119 case 950398559: 3120 return getCommentElement(); 3121 case 737543241: 3122 return getPatientInstructionElement(); 3123 case -332612366: 3124 return addBasedOn(); 3125 case 767422259: 3126 return addParticipant(); 3127 case -897241393: 3128 return addRequestedPeriod(); 3129 default: 3130 return super.makeProperty(hash, name); 3131 } 3132 3133 } 3134 3135 @Override 3136 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3137 switch (hash) { 3138 case -1618432855: 3139 /* identifier */ return new String[] { "Identifier" }; 3140 case -892481550: 3141 /* status */ return new String[] { "code" }; 3142 case 987811551: 3143 /* cancelationReason */ return new String[] { "CodeableConcept" }; 3144 case 1281188563: 3145 /* serviceCategory */ return new String[] { "CodeableConcept" }; 3146 case -1928370289: 3147 /* serviceType */ return new String[] { "CodeableConcept" }; 3148 case -1694759682: 3149 /* specialty */ return new String[] { "CodeableConcept" }; 3150 case -1596426375: 3151 /* appointmentType */ return new String[] { "CodeableConcept" }; 3152 case 722137681: 3153 /* reasonCode */ return new String[] { "CodeableConcept" }; 3154 case -1146218137: 3155 /* reasonReference */ return new String[] { "Reference" }; 3156 case -1165461084: 3157 /* priority */ return new String[] { "unsignedInt" }; 3158 case -1724546052: 3159 /* description */ return new String[] { "string" }; 3160 case -1248768647: 3161 /* supportingInformation */ return new String[] { "Reference" }; 3162 case 109757538: 3163 /* start */ return new String[] { "instant" }; 3164 case 100571: 3165 /* end */ return new String[] { "instant" }; 3166 case -413630573: 3167 /* minutesDuration */ return new String[] { "positiveInt" }; 3168 case 3533310: 3169 /* slot */ return new String[] { "Reference" }; 3170 case 1028554472: 3171 /* created */ return new String[] { "dateTime" }; 3172 case 950398559: 3173 /* comment */ return new String[] { "string" }; 3174 case 737543241: 3175 /* patientInstruction */ return new String[] { "string" }; 3176 case -332612366: 3177 /* basedOn */ return new String[] { "Reference" }; 3178 case 767422259: 3179 /* participant */ return new String[] {}; 3180 case -897241393: 3181 /* requestedPeriod */ return new String[] { "Period" }; 3182 default: 3183 return super.getTypesForProperty(hash, name); 3184 } 3185 3186 } 3187 3188 @Override 3189 public Base addChild(String name) throws FHIRException { 3190 if (name.equals("identifier")) { 3191 return addIdentifier(); 3192 } else if (name.equals("status")) { 3193 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 3194 } else if (name.equals("cancelationReason")) { 3195 this.cancelationReason = new CodeableConcept(); 3196 return this.cancelationReason; 3197 } else if (name.equals("serviceCategory")) { 3198 return addServiceCategory(); 3199 } else if (name.equals("serviceType")) { 3200 return addServiceType(); 3201 } else if (name.equals("specialty")) { 3202 return addSpecialty(); 3203 } else if (name.equals("appointmentType")) { 3204 this.appointmentType = new CodeableConcept(); 3205 return this.appointmentType; 3206 } else if (name.equals("reasonCode")) { 3207 return addReasonCode(); 3208 } else if (name.equals("reasonReference")) { 3209 return addReasonReference(); 3210 } else if (name.equals("priority")) { 3211 throw new FHIRException("Cannot call addChild on a singleton property Appointment.priority"); 3212 } else if (name.equals("description")) { 3213 throw new FHIRException("Cannot call addChild on a singleton property Appointment.description"); 3214 } else if (name.equals("supportingInformation")) { 3215 return addSupportingInformation(); 3216 } else if (name.equals("start")) { 3217 throw new FHIRException("Cannot call addChild on a singleton property Appointment.start"); 3218 } else if (name.equals("end")) { 3219 throw new FHIRException("Cannot call addChild on a singleton property Appointment.end"); 3220 } else if (name.equals("minutesDuration")) { 3221 throw new FHIRException("Cannot call addChild on a singleton property Appointment.minutesDuration"); 3222 } else if (name.equals("slot")) { 3223 return addSlot(); 3224 } else if (name.equals("created")) { 3225 throw new FHIRException("Cannot call addChild on a singleton property Appointment.created"); 3226 } else if (name.equals("comment")) { 3227 throw new FHIRException("Cannot call addChild on a singleton property Appointment.comment"); 3228 } else if (name.equals("patientInstruction")) { 3229 throw new FHIRException("Cannot call addChild on a singleton property Appointment.patientInstruction"); 3230 } else if (name.equals("basedOn")) { 3231 return addBasedOn(); 3232 } else if (name.equals("participant")) { 3233 return addParticipant(); 3234 } else if (name.equals("requestedPeriod")) { 3235 return addRequestedPeriod(); 3236 } else 3237 return super.addChild(name); 3238 } 3239 3240 public String fhirType() { 3241 return "Appointment"; 3242 3243 } 3244 3245 public Appointment copy() { 3246 Appointment dst = new Appointment(); 3247 copyValues(dst); 3248 return dst; 3249 } 3250 3251 public void copyValues(Appointment dst) { 3252 super.copyValues(dst); 3253 if (identifier != null) { 3254 dst.identifier = new ArrayList<Identifier>(); 3255 for (Identifier i : identifier) 3256 dst.identifier.add(i.copy()); 3257 } 3258 ; 3259 dst.status = status == null ? null : status.copy(); 3260 dst.cancelationReason = cancelationReason == null ? null : cancelationReason.copy(); 3261 if (serviceCategory != null) { 3262 dst.serviceCategory = new ArrayList<CodeableConcept>(); 3263 for (CodeableConcept i : serviceCategory) 3264 dst.serviceCategory.add(i.copy()); 3265 } 3266 ; 3267 if (serviceType != null) { 3268 dst.serviceType = new ArrayList<CodeableConcept>(); 3269 for (CodeableConcept i : serviceType) 3270 dst.serviceType.add(i.copy()); 3271 } 3272 ; 3273 if (specialty != null) { 3274 dst.specialty = new ArrayList<CodeableConcept>(); 3275 for (CodeableConcept i : specialty) 3276 dst.specialty.add(i.copy()); 3277 } 3278 ; 3279 dst.appointmentType = appointmentType == null ? null : appointmentType.copy(); 3280 if (reasonCode != null) { 3281 dst.reasonCode = new ArrayList<CodeableConcept>(); 3282 for (CodeableConcept i : reasonCode) 3283 dst.reasonCode.add(i.copy()); 3284 } 3285 ; 3286 if (reasonReference != null) { 3287 dst.reasonReference = new ArrayList<Reference>(); 3288 for (Reference i : reasonReference) 3289 dst.reasonReference.add(i.copy()); 3290 } 3291 ; 3292 dst.priority = priority == null ? null : priority.copy(); 3293 dst.description = description == null ? null : description.copy(); 3294 if (supportingInformation != null) { 3295 dst.supportingInformation = new ArrayList<Reference>(); 3296 for (Reference i : supportingInformation) 3297 dst.supportingInformation.add(i.copy()); 3298 } 3299 ; 3300 dst.start = start == null ? null : start.copy(); 3301 dst.end = end == null ? null : end.copy(); 3302 dst.minutesDuration = minutesDuration == null ? null : minutesDuration.copy(); 3303 if (slot != null) { 3304 dst.slot = new ArrayList<Reference>(); 3305 for (Reference i : slot) 3306 dst.slot.add(i.copy()); 3307 } 3308 ; 3309 dst.created = created == null ? null : created.copy(); 3310 dst.comment = comment == null ? null : comment.copy(); 3311 dst.patientInstruction = patientInstruction == null ? null : patientInstruction.copy(); 3312 if (basedOn != null) { 3313 dst.basedOn = new ArrayList<Reference>(); 3314 for (Reference i : basedOn) 3315 dst.basedOn.add(i.copy()); 3316 } 3317 ; 3318 if (participant != null) { 3319 dst.participant = new ArrayList<AppointmentParticipantComponent>(); 3320 for (AppointmentParticipantComponent i : participant) 3321 dst.participant.add(i.copy()); 3322 } 3323 ; 3324 if (requestedPeriod != null) { 3325 dst.requestedPeriod = new ArrayList<Period>(); 3326 for (Period i : requestedPeriod) 3327 dst.requestedPeriod.add(i.copy()); 3328 } 3329 ; 3330 } 3331 3332 protected Appointment typedCopy() { 3333 return copy(); 3334 } 3335 3336 @Override 3337 public boolean equalsDeep(Base other_) { 3338 if (!super.equalsDeep(other_)) 3339 return false; 3340 if (!(other_ instanceof Appointment)) 3341 return false; 3342 Appointment o = (Appointment) other_; 3343 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 3344 && compareDeep(cancelationReason, o.cancelationReason, true) 3345 && compareDeep(serviceCategory, o.serviceCategory, true) && compareDeep(serviceType, o.serviceType, true) 3346 && compareDeep(specialty, o.specialty, true) && compareDeep(appointmentType, o.appointmentType, true) 3347 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3348 && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) 3349 && compareDeep(supportingInformation, o.supportingInformation, true) && compareDeep(start, o.start, true) 3350 && compareDeep(end, o.end, true) && compareDeep(minutesDuration, o.minutesDuration, true) 3351 && compareDeep(slot, o.slot, true) && compareDeep(created, o.created, true) 3352 && compareDeep(comment, o.comment, true) && compareDeep(patientInstruction, o.patientInstruction, true) 3353 && compareDeep(basedOn, o.basedOn, true) && compareDeep(participant, o.participant, true) 3354 && compareDeep(requestedPeriod, o.requestedPeriod, true); 3355 } 3356 3357 @Override 3358 public boolean equalsShallow(Base other_) { 3359 if (!super.equalsShallow(other_)) 3360 return false; 3361 if (!(other_ instanceof Appointment)) 3362 return false; 3363 Appointment o = (Appointment) other_; 3364 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 3365 && compareValues(description, o.description, true) && compareValues(start, o.start, true) 3366 && compareValues(end, o.end, true) && compareValues(minutesDuration, o.minutesDuration, true) 3367 && compareValues(created, o.created, true) && compareValues(comment, o.comment, true) 3368 && compareValues(patientInstruction, o.patientInstruction, true); 3369 } 3370 3371 public boolean isEmpty() { 3372 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, cancelationReason, 3373 serviceCategory, serviceType, specialty, appointmentType, reasonCode, reasonReference, priority, description, 3374 supportingInformation, start, end, minutesDuration, slot, created, comment, patientInstruction, basedOn, 3375 participant, requestedPeriod); 3376 } 3377 3378 @Override 3379 public ResourceType getResourceType() { 3380 return ResourceType.Appointment; 3381 } 3382 3383 /** 3384 * Search parameter: <b>date</b> 3385 * <p> 3386 * Description: <b>Appointment date/time.</b><br> 3387 * Type: <b>date</b><br> 3388 * Path: <b>Appointment.start</b><br> 3389 * </p> 3390 */ 3391 @SearchParamDefinition(name = "date", path = "Appointment.start", description = "Appointment date/time.", type = "date") 3392 public static final String SP_DATE = "date"; 3393 /** 3394 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3395 * <p> 3396 * Description: <b>Appointment date/time.</b><br> 3397 * Type: <b>date</b><br> 3398 * Path: <b>Appointment.start</b><br> 3399 * </p> 3400 */ 3401 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3402 SP_DATE); 3403 3404 /** 3405 * Search parameter: <b>identifier</b> 3406 * <p> 3407 * Description: <b>An Identifier of the Appointment</b><br> 3408 * Type: <b>token</b><br> 3409 * Path: <b>Appointment.identifier</b><br> 3410 * </p> 3411 */ 3412 @SearchParamDefinition(name = "identifier", path = "Appointment.identifier", description = "An Identifier of the Appointment", type = "token") 3413 public static final String SP_IDENTIFIER = "identifier"; 3414 /** 3415 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3416 * <p> 3417 * Description: <b>An Identifier of the Appointment</b><br> 3418 * Type: <b>token</b><br> 3419 * Path: <b>Appointment.identifier</b><br> 3420 * </p> 3421 */ 3422 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3423 SP_IDENTIFIER); 3424 3425 /** 3426 * Search parameter: <b>specialty</b> 3427 * <p> 3428 * Description: <b>The specialty of a practitioner that would be required to 3429 * perform the service requested in this appointment</b><br> 3430 * Type: <b>token</b><br> 3431 * Path: <b>Appointment.specialty</b><br> 3432 * </p> 3433 */ 3434 @SearchParamDefinition(name = "specialty", path = "Appointment.specialty", description = "The specialty of a practitioner that would be required to perform the service requested in this appointment", type = "token") 3435 public static final String SP_SPECIALTY = "specialty"; 3436 /** 3437 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 3438 * <p> 3439 * Description: <b>The specialty of a practitioner that would be required to 3440 * perform the service requested in this appointment</b><br> 3441 * Type: <b>token</b><br> 3442 * Path: <b>Appointment.specialty</b><br> 3443 * </p> 3444 */ 3445 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3446 SP_SPECIALTY); 3447 3448 /** 3449 * Search parameter: <b>service-category</b> 3450 * <p> 3451 * Description: <b>A broad categorization of the service that is to be performed 3452 * during this appointment</b><br> 3453 * Type: <b>token</b><br> 3454 * Path: <b>Appointment.serviceCategory</b><br> 3455 * </p> 3456 */ 3457 @SearchParamDefinition(name = "service-category", path = "Appointment.serviceCategory", description = "A broad categorization of the service that is to be performed during this appointment", type = "token") 3458 public static final String SP_SERVICE_CATEGORY = "service-category"; 3459 /** 3460 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 3461 * <p> 3462 * Description: <b>A broad categorization of the service that is to be performed 3463 * during this appointment</b><br> 3464 * Type: <b>token</b><br> 3465 * Path: <b>Appointment.serviceCategory</b><br> 3466 * </p> 3467 */ 3468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3469 SP_SERVICE_CATEGORY); 3470 3471 /** 3472 * Search parameter: <b>practitioner</b> 3473 * <p> 3474 * Description: <b>One of the individuals of the appointment is this 3475 * practitioner</b><br> 3476 * Type: <b>reference</b><br> 3477 * Path: <b>Appointment.participant.actor</b><br> 3478 * </p> 3479 */ 3480 @SearchParamDefinition(name = "practitioner", path = "Appointment.participant.actor.where(resolve() is Practitioner)", description = "One of the individuals of the appointment is this practitioner", type = "reference", target = { 3481 Practitioner.class }) 3482 public static final String SP_PRACTITIONER = "practitioner"; 3483 /** 3484 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 3485 * <p> 3486 * Description: <b>One of the individuals of the appointment is this 3487 * practitioner</b><br> 3488 * Type: <b>reference</b><br> 3489 * Path: <b>Appointment.participant.actor</b><br> 3490 * </p> 3491 */ 3492 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3493 SP_PRACTITIONER); 3494 3495 /** 3496 * Constant for fluent queries to be used to add include statements. Specifies 3497 * the path value of "<b>Appointment:practitioner</b>". 3498 */ 3499 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 3500 "Appointment:practitioner").toLocked(); 3501 3502 /** 3503 * Search parameter: <b>part-status</b> 3504 * <p> 3505 * Description: <b>The Participation status of the subject, or other participant 3506 * on the appointment. Can be used to locate participants that have not 3507 * responded to meeting requests.</b><br> 3508 * Type: <b>token</b><br> 3509 * Path: <b>Appointment.participant.status</b><br> 3510 * </p> 3511 */ 3512 @SearchParamDefinition(name = "part-status", path = "Appointment.participant.status", description = "The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.", type = "token") 3513 public static final String SP_PART_STATUS = "part-status"; 3514 /** 3515 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 3516 * <p> 3517 * Description: <b>The Participation status of the subject, or other participant 3518 * on the appointment. Can be used to locate participants that have not 3519 * responded to meeting requests.</b><br> 3520 * Type: <b>token</b><br> 3521 * Path: <b>Appointment.participant.status</b><br> 3522 * </p> 3523 */ 3524 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3525 SP_PART_STATUS); 3526 3527 /** 3528 * Search parameter: <b>appointment-type</b> 3529 * <p> 3530 * Description: <b>The style of appointment or patient that has been booked in 3531 * the slot (not service type)</b><br> 3532 * Type: <b>token</b><br> 3533 * Path: <b>Appointment.appointmentType</b><br> 3534 * </p> 3535 */ 3536 @SearchParamDefinition(name = "appointment-type", path = "Appointment.appointmentType", description = "The style of appointment or patient that has been booked in the slot (not service type)", type = "token") 3537 public static final String SP_APPOINTMENT_TYPE = "appointment-type"; 3538 /** 3539 * <b>Fluent Client</b> search parameter constant for <b>appointment-type</b> 3540 * <p> 3541 * Description: <b>The style of appointment or patient that has been booked in 3542 * the slot (not service type)</b><br> 3543 * Type: <b>token</b><br> 3544 * Path: <b>Appointment.appointmentType</b><br> 3545 * </p> 3546 */ 3547 public static final ca.uhn.fhir.rest.gclient.TokenClientParam APPOINTMENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3548 SP_APPOINTMENT_TYPE); 3549 3550 /** 3551 * Search parameter: <b>service-type</b> 3552 * <p> 3553 * Description: <b>The specific service that is to be performed during this 3554 * appointment</b><br> 3555 * Type: <b>token</b><br> 3556 * Path: <b>Appointment.serviceType</b><br> 3557 * </p> 3558 */ 3559 @SearchParamDefinition(name = "service-type", path = "Appointment.serviceType", description = "The specific service that is to be performed during this appointment", type = "token") 3560 public static final String SP_SERVICE_TYPE = "service-type"; 3561 /** 3562 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 3563 * <p> 3564 * Description: <b>The specific service that is to be performed during this 3565 * appointment</b><br> 3566 * Type: <b>token</b><br> 3567 * Path: <b>Appointment.serviceType</b><br> 3568 * </p> 3569 */ 3570 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3571 SP_SERVICE_TYPE); 3572 3573 /** 3574 * Search parameter: <b>slot</b> 3575 * <p> 3576 * Description: <b>The slots that this appointment is filling</b><br> 3577 * Type: <b>reference</b><br> 3578 * Path: <b>Appointment.slot</b><br> 3579 * </p> 3580 */ 3581 @SearchParamDefinition(name = "slot", path = "Appointment.slot", description = "The slots that this appointment is filling", type = "reference", target = { 3582 Slot.class }) 3583 public static final String SP_SLOT = "slot"; 3584 /** 3585 * <b>Fluent Client</b> search parameter constant for <b>slot</b> 3586 * <p> 3587 * Description: <b>The slots that this appointment is filling</b><br> 3588 * Type: <b>reference</b><br> 3589 * Path: <b>Appointment.slot</b><br> 3590 * </p> 3591 */ 3592 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SLOT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3593 SP_SLOT); 3594 3595 /** 3596 * Constant for fluent queries to be used to add include statements. Specifies 3597 * the path value of "<b>Appointment:slot</b>". 3598 */ 3599 public static final ca.uhn.fhir.model.api.Include INCLUDE_SLOT = new ca.uhn.fhir.model.api.Include("Appointment:slot") 3600 .toLocked(); 3601 3602 /** 3603 * Search parameter: <b>reason-code</b> 3604 * <p> 3605 * Description: <b>Coded reason this appointment is scheduled</b><br> 3606 * Type: <b>token</b><br> 3607 * Path: <b>Appointment.reasonCode</b><br> 3608 * </p> 3609 */ 3610 @SearchParamDefinition(name = "reason-code", path = "Appointment.reasonCode", description = "Coded reason this appointment is scheduled", type = "token") 3611 public static final String SP_REASON_CODE = "reason-code"; 3612 /** 3613 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 3614 * <p> 3615 * Description: <b>Coded reason this appointment is scheduled</b><br> 3616 * Type: <b>token</b><br> 3617 * Path: <b>Appointment.reasonCode</b><br> 3618 * </p> 3619 */ 3620 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3621 SP_REASON_CODE); 3622 3623 /** 3624 * Search parameter: <b>actor</b> 3625 * <p> 3626 * Description: <b>Any one of the individuals participating in the 3627 * appointment</b><br> 3628 * Type: <b>reference</b><br> 3629 * Path: <b>Appointment.participant.actor</b><br> 3630 * </p> 3631 */ 3632 @SearchParamDefinition(name = "actor", path = "Appointment.participant.actor", description = "Any one of the individuals participating in the appointment", type = "reference", providesMembershipIn = { 3633 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3634 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3635 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3636 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3637 HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, 3638 RelatedPerson.class }) 3639 public static final String SP_ACTOR = "actor"; 3640 /** 3641 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 3642 * <p> 3643 * Description: <b>Any one of the individuals participating in the 3644 * appointment</b><br> 3645 * Type: <b>reference</b><br> 3646 * Path: <b>Appointment.participant.actor</b><br> 3647 * </p> 3648 */ 3649 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3650 SP_ACTOR); 3651 3652 /** 3653 * Constant for fluent queries to be used to add include statements. Specifies 3654 * the path value of "<b>Appointment:actor</b>". 3655 */ 3656 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include( 3657 "Appointment:actor").toLocked(); 3658 3659 /** 3660 * Search parameter: <b>based-on</b> 3661 * <p> 3662 * Description: <b>The service request this appointment is allocated to 3663 * assess</b><br> 3664 * Type: <b>reference</b><br> 3665 * Path: <b>Appointment.basedOn</b><br> 3666 * </p> 3667 */ 3668 @SearchParamDefinition(name = "based-on", path = "Appointment.basedOn", description = "The service request this appointment is allocated to assess", type = "reference", target = { 3669 ServiceRequest.class }) 3670 public static final String SP_BASED_ON = "based-on"; 3671 /** 3672 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3673 * <p> 3674 * Description: <b>The service request this appointment is allocated to 3675 * assess</b><br> 3676 * Type: <b>reference</b><br> 3677 * Path: <b>Appointment.basedOn</b><br> 3678 * </p> 3679 */ 3680 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3681 SP_BASED_ON); 3682 3683 /** 3684 * Constant for fluent queries to be used to add include statements. Specifies 3685 * the path value of "<b>Appointment:based-on</b>". 3686 */ 3687 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3688 "Appointment:based-on").toLocked(); 3689 3690 /** 3691 * Search parameter: <b>patient</b> 3692 * <p> 3693 * Description: <b>One of the individuals of the appointment is this 3694 * patient</b><br> 3695 * Type: <b>reference</b><br> 3696 * Path: <b>Appointment.participant.actor</b><br> 3697 * </p> 3698 */ 3699 @SearchParamDefinition(name = "patient", path = "Appointment.participant.actor.where(resolve() is Patient)", description = "One of the individuals of the appointment is this patient", type = "reference", target = { 3700 Patient.class }) 3701 public static final String SP_PATIENT = "patient"; 3702 /** 3703 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3704 * <p> 3705 * Description: <b>One of the individuals of the appointment is this 3706 * patient</b><br> 3707 * Type: <b>reference</b><br> 3708 * Path: <b>Appointment.participant.actor</b><br> 3709 * </p> 3710 */ 3711 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3712 SP_PATIENT); 3713 3714 /** 3715 * Constant for fluent queries to be used to add include statements. Specifies 3716 * the path value of "<b>Appointment:patient</b>". 3717 */ 3718 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3719 "Appointment:patient").toLocked(); 3720 3721 /** 3722 * Search parameter: <b>reason-reference</b> 3723 * <p> 3724 * Description: <b>Reason the appointment is to take place (resource)</b><br> 3725 * Type: <b>reference</b><br> 3726 * Path: <b>Appointment.reasonReference</b><br> 3727 * </p> 3728 */ 3729 @SearchParamDefinition(name = "reason-reference", path = "Appointment.reasonReference", description = "Reason the appointment is to take place (resource)", type = "reference", target = { 3730 Condition.class, ImmunizationRecommendation.class, Observation.class, Procedure.class }) 3731 public static final String SP_REASON_REFERENCE = "reason-reference"; 3732 /** 3733 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 3734 * <p> 3735 * Description: <b>Reason the appointment is to take place (resource)</b><br> 3736 * Type: <b>reference</b><br> 3737 * Path: <b>Appointment.reasonReference</b><br> 3738 * </p> 3739 */ 3740 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3741 SP_REASON_REFERENCE); 3742 3743 /** 3744 * Constant for fluent queries to be used to add include statements. Specifies 3745 * the path value of "<b>Appointment:reason-reference</b>". 3746 */ 3747 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 3748 "Appointment:reason-reference").toLocked(); 3749 3750 /** 3751 * Search parameter: <b>supporting-info</b> 3752 * <p> 3753 * Description: <b>Additional information to support the appointment</b><br> 3754 * Type: <b>reference</b><br> 3755 * Path: <b>Appointment.supportingInformation</b><br> 3756 * </p> 3757 */ 3758 @SearchParamDefinition(name = "supporting-info", path = "Appointment.supportingInformation", description = "Additional information to support the appointment", type = "reference") 3759 public static final String SP_SUPPORTING_INFO = "supporting-info"; 3760 /** 3761 * <b>Fluent Client</b> search parameter constant for <b>supporting-info</b> 3762 * <p> 3763 * Description: <b>Additional information to support the appointment</b><br> 3764 * Type: <b>reference</b><br> 3765 * Path: <b>Appointment.supportingInformation</b><br> 3766 * </p> 3767 */ 3768 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTING_INFO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3769 SP_SUPPORTING_INFO); 3770 3771 /** 3772 * Constant for fluent queries to be used to add include statements. Specifies 3773 * the path value of "<b>Appointment:supporting-info</b>". 3774 */ 3775 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTING_INFO = new ca.uhn.fhir.model.api.Include( 3776 "Appointment:supporting-info").toLocked(); 3777 3778 /** 3779 * Search parameter: <b>location</b> 3780 * <p> 3781 * Description: <b>This location is listed in the participants of the 3782 * appointment</b><br> 3783 * Type: <b>reference</b><br> 3784 * Path: <b>Appointment.participant.actor</b><br> 3785 * </p> 3786 */ 3787 @SearchParamDefinition(name = "location", path = "Appointment.participant.actor.where(resolve() is Location)", description = "This location is listed in the participants of the appointment", type = "reference", target = { 3788 Location.class }) 3789 public static final String SP_LOCATION = "location"; 3790 /** 3791 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3792 * <p> 3793 * Description: <b>This location is listed in the participants of the 3794 * appointment</b><br> 3795 * Type: <b>reference</b><br> 3796 * Path: <b>Appointment.participant.actor</b><br> 3797 * </p> 3798 */ 3799 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3800 SP_LOCATION); 3801 3802 /** 3803 * Constant for fluent queries to be used to add include statements. Specifies 3804 * the path value of "<b>Appointment:location</b>". 3805 */ 3806 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 3807 "Appointment:location").toLocked(); 3808 3809 /** 3810 * Search parameter: <b>status</b> 3811 * <p> 3812 * Description: <b>The overall status of the appointment</b><br> 3813 * Type: <b>token</b><br> 3814 * Path: <b>Appointment.status</b><br> 3815 * </p> 3816 */ 3817 @SearchParamDefinition(name = "status", path = "Appointment.status", description = "The overall status of the appointment", type = "token") 3818 public static final String SP_STATUS = "status"; 3819 /** 3820 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3821 * <p> 3822 * Description: <b>The overall status of the appointment</b><br> 3823 * Type: <b>token</b><br> 3824 * Path: <b>Appointment.status</b><br> 3825 * </p> 3826 */ 3827 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3828 SP_STATUS); 3829 3830}