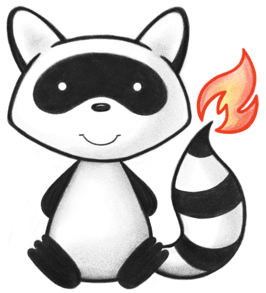
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * A reply to an appointment request for a patient and/or practitioner(s), such 047 * as a confirmation or rejection. 048 */ 049@ResourceDef(name = "AppointmentResponse", profile = "http://hl7.org/fhir/StructureDefinition/AppointmentResponse") 050public class AppointmentResponse extends DomainResource { 051 052 public enum ParticipantStatus { 053 /** 054 * The participant has accepted the appointment. 055 */ 056 ACCEPTED, 057 /** 058 * The participant has declined the appointment and will not participate in the 059 * appointment. 060 */ 061 DECLINED, 062 /** 063 * The participant has tentatively accepted the appointment. This could be 064 * automatically created by a system and requires further processing before it 065 * can be accepted. There is no commitment that attendance will occur. 066 */ 067 TENTATIVE, 068 /** 069 * The participant needs to indicate if they accept the appointment by changing 070 * this status to one of the other statuses. 071 */ 072 NEEDSACTION, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static ParticipantStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("accepted".equals(codeString)) 082 return ACCEPTED; 083 if ("declined".equals(codeString)) 084 return DECLINED; 085 if ("tentative".equals(codeString)) 086 return TENTATIVE; 087 if ("needs-action".equals(codeString)) 088 return NEEDSACTION; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown ParticipantStatus code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case ACCEPTED: 098 return "accepted"; 099 case DECLINED: 100 return "declined"; 101 case TENTATIVE: 102 return "tentative"; 103 case NEEDSACTION: 104 return "needs-action"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case ACCEPTED: 115 return "http://hl7.org/fhir/participationstatus"; 116 case DECLINED: 117 return "http://hl7.org/fhir/participationstatus"; 118 case TENTATIVE: 119 return "http://hl7.org/fhir/participationstatus"; 120 case NEEDSACTION: 121 return "http://hl7.org/fhir/participationstatus"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case ACCEPTED: 132 return "The participant has accepted the appointment."; 133 case DECLINED: 134 return "The participant has declined the appointment and will not participate in the appointment."; 135 case TENTATIVE: 136 return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 137 case NEEDSACTION: 138 return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDisplay() { 147 switch (this) { 148 case ACCEPTED: 149 return "Accepted"; 150 case DECLINED: 151 return "Declined"; 152 case TENTATIVE: 153 return "Tentative"; 154 case NEEDSACTION: 155 return "Needs Action"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 } 163 164 public static class ParticipantStatusEnumFactory implements EnumFactory<ParticipantStatus> { 165 public ParticipantStatus fromCode(String codeString) throws IllegalArgumentException { 166 if (codeString == null || "".equals(codeString)) 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("accepted".equals(codeString)) 170 return ParticipantStatus.ACCEPTED; 171 if ("declined".equals(codeString)) 172 return ParticipantStatus.DECLINED; 173 if ("tentative".equals(codeString)) 174 return ParticipantStatus.TENTATIVE; 175 if ("needs-action".equals(codeString)) 176 return ParticipantStatus.NEEDSACTION; 177 throw new IllegalArgumentException("Unknown ParticipantStatus code '" + codeString + "'"); 178 } 179 180 public Enumeration<ParticipantStatus> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.NULL, code); 188 if ("accepted".equals(codeString)) 189 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.ACCEPTED, code); 190 if ("declined".equals(codeString)) 191 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.DECLINED, code); 192 if ("tentative".equals(codeString)) 193 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.TENTATIVE, code); 194 if ("needs-action".equals(codeString)) 195 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.NEEDSACTION, code); 196 throw new FHIRException("Unknown ParticipantStatus code '" + codeString + "'"); 197 } 198 199 public String toCode(ParticipantStatus code) { 200 if (code == ParticipantStatus.NULL) 201 return null; 202 if (code == ParticipantStatus.ACCEPTED) 203 return "accepted"; 204 if (code == ParticipantStatus.DECLINED) 205 return "declined"; 206 if (code == ParticipantStatus.TENTATIVE) 207 return "tentative"; 208 if (code == ParticipantStatus.NEEDSACTION) 209 return "needs-action"; 210 return "?"; 211 } 212 213 public String toSystem(ParticipantStatus code) { 214 return code.getSystem(); 215 } 216 } 217 218 /** 219 * This records identifiers associated with this appointment response concern 220 * that are defined by business processes and/ or used to refer to it when a 221 * direct URL reference to the resource itself is not appropriate. 222 */ 223 @Child(name = "identifier", type = { 224 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 225 @Description(shortDefinition = "External Ids for this item", formalDefinition = "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.") 226 protected List<Identifier> identifier; 227 228 /** 229 * Appointment that this response is replying to. 230 */ 231 @Child(name = "appointment", type = { 232 Appointment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 233 @Description(shortDefinition = "Appointment this response relates to", formalDefinition = "Appointment that this response is replying to.") 234 protected Reference appointment; 235 236 /** 237 * The actual object that is the target of the reference (Appointment that this 238 * response is replying to.) 239 */ 240 protected Appointment appointmentTarget; 241 242 /** 243 * Date/Time that the appointment is to take place, or requested new start time. 244 */ 245 @Child(name = "start", type = { InstantType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 246 @Description(shortDefinition = "Time from appointment, or requested new start time", formalDefinition = "Date/Time that the appointment is to take place, or requested new start time.") 247 protected InstantType start; 248 249 /** 250 * This may be either the same as the appointment request to confirm the details 251 * of the appointment, or alternately a new time to request a re-negotiation of 252 * the end time. 253 */ 254 @Child(name = "end", type = { InstantType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 255 @Description(shortDefinition = "Time from appointment, or requested new end time", formalDefinition = "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.") 256 protected InstantType end; 257 258 /** 259 * Role of participant in the appointment. 260 */ 261 @Child(name = "participantType", type = { 262 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 263 @Description(shortDefinition = "Role of participant in the appointment", formalDefinition = "Role of participant in the appointment.") 264 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-participant-type") 265 protected List<CodeableConcept> participantType; 266 267 /** 268 * A Person, Location, HealthcareService, or Device that is participating in the 269 * appointment. 270 */ 271 @Child(name = "actor", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 272 Device.class, HealthcareService.class, 273 Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 274 @Description(shortDefinition = "Person, Location, HealthcareService, or Device", formalDefinition = "A Person, Location, HealthcareService, or Device that is participating in the appointment.") 275 protected Reference actor; 276 277 /** 278 * The actual object that is the target of the reference (A Person, Location, 279 * HealthcareService, or Device that is participating in the appointment.) 280 */ 281 protected Resource actorTarget; 282 283 /** 284 * Participation status of the participant. When the status is declined or 285 * tentative if the start/end times are different to the appointment, then these 286 * times should be interpreted as a requested time change. When the status is 287 * accepted, the times can either be the time of the appointment (as a 288 * confirmation of the time) or can be empty. 289 */ 290 @Child(name = "participantStatus", type = { 291 CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 292 @Description(shortDefinition = "accepted | declined | tentative | needs-action", formalDefinition = "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.") 293 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participationstatus") 294 protected Enumeration<ParticipantStatus> participantStatus; 295 296 /** 297 * Additional comments about the appointment. 298 */ 299 @Child(name = "comment", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 300 @Description(shortDefinition = "Additional comments", formalDefinition = "Additional comments about the appointment.") 301 protected StringType comment; 302 303 private static final long serialVersionUID = 248548635L; 304 305 /** 306 * Constructor 307 */ 308 public AppointmentResponse() { 309 super(); 310 } 311 312 /** 313 * Constructor 314 */ 315 public AppointmentResponse(Reference appointment, Enumeration<ParticipantStatus> participantStatus) { 316 super(); 317 this.appointment = appointment; 318 this.participantStatus = participantStatus; 319 } 320 321 /** 322 * @return {@link #identifier} (This records identifiers associated with this 323 * appointment response concern that are defined by business processes 324 * and/ or used to refer to it when a direct URL reference to the 325 * resource itself is not appropriate.) 326 */ 327 public List<Identifier> getIdentifier() { 328 if (this.identifier == null) 329 this.identifier = new ArrayList<Identifier>(); 330 return this.identifier; 331 } 332 333 /** 334 * @return Returns a reference to <code>this</code> for easy method chaining 335 */ 336 public AppointmentResponse setIdentifier(List<Identifier> theIdentifier) { 337 this.identifier = theIdentifier; 338 return this; 339 } 340 341 public boolean hasIdentifier() { 342 if (this.identifier == null) 343 return false; 344 for (Identifier item : this.identifier) 345 if (!item.isEmpty()) 346 return true; 347 return false; 348 } 349 350 public Identifier addIdentifier() { // 3 351 Identifier t = new Identifier(); 352 if (this.identifier == null) 353 this.identifier = new ArrayList<Identifier>(); 354 this.identifier.add(t); 355 return t; 356 } 357 358 public AppointmentResponse addIdentifier(Identifier t) { // 3 359 if (t == null) 360 return this; 361 if (this.identifier == null) 362 this.identifier = new ArrayList<Identifier>(); 363 this.identifier.add(t); 364 return this; 365 } 366 367 /** 368 * @return The first repetition of repeating field {@link #identifier}, creating 369 * it if it does not already exist 370 */ 371 public Identifier getIdentifierFirstRep() { 372 if (getIdentifier().isEmpty()) { 373 addIdentifier(); 374 } 375 return getIdentifier().get(0); 376 } 377 378 /** 379 * @return {@link #appointment} (Appointment that this response is replying to.) 380 */ 381 public Reference getAppointment() { 382 if (this.appointment == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 385 else if (Configuration.doAutoCreate()) 386 this.appointment = new Reference(); // cc 387 return this.appointment; 388 } 389 390 public boolean hasAppointment() { 391 return this.appointment != null && !this.appointment.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #appointment} (Appointment that this response is replying 396 * to.) 397 */ 398 public AppointmentResponse setAppointment(Reference value) { 399 this.appointment = value; 400 return this; 401 } 402 403 /** 404 * @return {@link #appointment} The actual object that is the target of the 405 * reference. The reference library doesn't populate this, but you can 406 * use it to hold the resource if you resolve it. (Appointment that this 407 * response is replying to.) 408 */ 409 public Appointment getAppointmentTarget() { 410 if (this.appointmentTarget == null) 411 if (Configuration.errorOnAutoCreate()) 412 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 413 else if (Configuration.doAutoCreate()) 414 this.appointmentTarget = new Appointment(); // aa 415 return this.appointmentTarget; 416 } 417 418 /** 419 * @param value {@link #appointment} The actual object that is the target of the 420 * reference. The reference library doesn't use these, but you can 421 * use it to hold the resource if you resolve it. (Appointment that 422 * this response is replying to.) 423 */ 424 public AppointmentResponse setAppointmentTarget(Appointment value) { 425 this.appointmentTarget = value; 426 return this; 427 } 428 429 /** 430 * @return {@link #start} (Date/Time that the appointment is to take place, or 431 * requested new start time.). This is the underlying object with id, 432 * value and extensions. The accessor "getStart" gives direct access to 433 * the value 434 */ 435 public InstantType getStartElement() { 436 if (this.start == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create AppointmentResponse.start"); 439 else if (Configuration.doAutoCreate()) 440 this.start = new InstantType(); // bb 441 return this.start; 442 } 443 444 public boolean hasStartElement() { 445 return this.start != null && !this.start.isEmpty(); 446 } 447 448 public boolean hasStart() { 449 return this.start != null && !this.start.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #start} (Date/Time that the appointment is to take place, 454 * or requested new start time.). This is the underlying object 455 * with id, value and extensions. The accessor "getStart" gives 456 * direct access to the value 457 */ 458 public AppointmentResponse setStartElement(InstantType value) { 459 this.start = value; 460 return this; 461 } 462 463 /** 464 * @return Date/Time that the appointment is to take place, or requested new 465 * start time. 466 */ 467 public Date getStart() { 468 return this.start == null ? null : this.start.getValue(); 469 } 470 471 /** 472 * @param value Date/Time that the appointment is to take place, or requested 473 * new start time. 474 */ 475 public AppointmentResponse setStart(Date value) { 476 if (value == null) 477 this.start = null; 478 else { 479 if (this.start == null) 480 this.start = new InstantType(); 481 this.start.setValue(value); 482 } 483 return this; 484 } 485 486 /** 487 * @return {@link #end} (This may be either the same as the appointment request 488 * to confirm the details of the appointment, or alternately a new time 489 * to request a re-negotiation of the end time.). This is the underlying 490 * object with id, value and extensions. The accessor "getEnd" gives 491 * direct access to the value 492 */ 493 public InstantType getEndElement() { 494 if (this.end == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create AppointmentResponse.end"); 497 else if (Configuration.doAutoCreate()) 498 this.end = new InstantType(); // bb 499 return this.end; 500 } 501 502 public boolean hasEndElement() { 503 return this.end != null && !this.end.isEmpty(); 504 } 505 506 public boolean hasEnd() { 507 return this.end != null && !this.end.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #end} (This may be either the same as the appointment 512 * request to confirm the details of the appointment, or 513 * alternately a new time to request a re-negotiation of the end 514 * time.). This is the underlying object with id, value and 515 * extensions. The accessor "getEnd" gives direct access to the 516 * value 517 */ 518 public AppointmentResponse setEndElement(InstantType value) { 519 this.end = value; 520 return this; 521 } 522 523 /** 524 * @return This may be either the same as the appointment request to confirm the 525 * details of the appointment, or alternately a new time to request a 526 * re-negotiation of the end time. 527 */ 528 public Date getEnd() { 529 return this.end == null ? null : this.end.getValue(); 530 } 531 532 /** 533 * @param value This may be either the same as the appointment request to 534 * confirm the details of the appointment, or alternately a new 535 * time to request a re-negotiation of the end time. 536 */ 537 public AppointmentResponse setEnd(Date value) { 538 if (value == null) 539 this.end = null; 540 else { 541 if (this.end == null) 542 this.end = new InstantType(); 543 this.end.setValue(value); 544 } 545 return this; 546 } 547 548 /** 549 * @return {@link #participantType} (Role of participant in the appointment.) 550 */ 551 public List<CodeableConcept> getParticipantType() { 552 if (this.participantType == null) 553 this.participantType = new ArrayList<CodeableConcept>(); 554 return this.participantType; 555 } 556 557 /** 558 * @return Returns a reference to <code>this</code> for easy method chaining 559 */ 560 public AppointmentResponse setParticipantType(List<CodeableConcept> theParticipantType) { 561 this.participantType = theParticipantType; 562 return this; 563 } 564 565 public boolean hasParticipantType() { 566 if (this.participantType == null) 567 return false; 568 for (CodeableConcept item : this.participantType) 569 if (!item.isEmpty()) 570 return true; 571 return false; 572 } 573 574 public CodeableConcept addParticipantType() { // 3 575 CodeableConcept t = new CodeableConcept(); 576 if (this.participantType == null) 577 this.participantType = new ArrayList<CodeableConcept>(); 578 this.participantType.add(t); 579 return t; 580 } 581 582 public AppointmentResponse addParticipantType(CodeableConcept t) { // 3 583 if (t == null) 584 return this; 585 if (this.participantType == null) 586 this.participantType = new ArrayList<CodeableConcept>(); 587 this.participantType.add(t); 588 return this; 589 } 590 591 /** 592 * @return The first repetition of repeating field {@link #participantType}, 593 * creating it if it does not already exist 594 */ 595 public CodeableConcept getParticipantTypeFirstRep() { 596 if (getParticipantType().isEmpty()) { 597 addParticipantType(); 598 } 599 return getParticipantType().get(0); 600 } 601 602 /** 603 * @return {@link #actor} (A Person, Location, HealthcareService, or Device that 604 * is participating in the appointment.) 605 */ 606 public Reference getActor() { 607 if (this.actor == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create AppointmentResponse.actor"); 610 else if (Configuration.doAutoCreate()) 611 this.actor = new Reference(); // cc 612 return this.actor; 613 } 614 615 public boolean hasActor() { 616 return this.actor != null && !this.actor.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #actor} (A Person, Location, HealthcareService, or Device 621 * that is participating in the appointment.) 622 */ 623 public AppointmentResponse setActor(Reference value) { 624 this.actor = value; 625 return this; 626 } 627 628 /** 629 * @return {@link #actor} The actual object that is the target of the reference. 630 * The reference library doesn't populate this, but you can use it to 631 * hold the resource if you resolve it. (A Person, Location, 632 * HealthcareService, or Device that is participating in the 633 * appointment.) 634 */ 635 public Resource getActorTarget() { 636 return this.actorTarget; 637 } 638 639 /** 640 * @param value {@link #actor} The actual object that is the target of the 641 * reference. The reference library doesn't use these, but you can 642 * use it to hold the resource if you resolve it. (A Person, 643 * Location, HealthcareService, or Device that is participating in 644 * the appointment.) 645 */ 646 public AppointmentResponse setActorTarget(Resource value) { 647 this.actorTarget = value; 648 return this; 649 } 650 651 /** 652 * @return {@link #participantStatus} (Participation status of the participant. 653 * When the status is declined or tentative if the start/end times are 654 * different to the appointment, then these times should be interpreted 655 * as a requested time change. When the status is accepted, the times 656 * can either be the time of the appointment (as a confirmation of the 657 * time) or can be empty.). This is the underlying object with id, value 658 * and extensions. The accessor "getParticipantStatus" gives direct 659 * access to the value 660 */ 661 public Enumeration<ParticipantStatus> getParticipantStatusElement() { 662 if (this.participantStatus == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create AppointmentResponse.participantStatus"); 665 else if (Configuration.doAutoCreate()) 666 this.participantStatus = new Enumeration<ParticipantStatus>(new ParticipantStatusEnumFactory()); // bb 667 return this.participantStatus; 668 } 669 670 public boolean hasParticipantStatusElement() { 671 return this.participantStatus != null && !this.participantStatus.isEmpty(); 672 } 673 674 public boolean hasParticipantStatus() { 675 return this.participantStatus != null && !this.participantStatus.isEmpty(); 676 } 677 678 /** 679 * @param value {@link #participantStatus} (Participation status of the 680 * participant. When the status is declined or tentative if the 681 * start/end times are different to the appointment, then these 682 * times should be interpreted as a requested time change. When the 683 * status is accepted, the times can either be the time of the 684 * appointment (as a confirmation of the time) or can be empty.). 685 * This is the underlying object with id, value and extensions. The 686 * accessor "getParticipantStatus" gives direct access to the value 687 */ 688 public AppointmentResponse setParticipantStatusElement(Enumeration<ParticipantStatus> value) { 689 this.participantStatus = value; 690 return this; 691 } 692 693 /** 694 * @return Participation status of the participant. When the status is declined 695 * or tentative if the start/end times are different to the appointment, 696 * then these times should be interpreted as a requested time change. 697 * When the status is accepted, the times can either be the time of the 698 * appointment (as a confirmation of the time) or can be empty. 699 */ 700 public ParticipantStatus getParticipantStatus() { 701 return this.participantStatus == null ? null : this.participantStatus.getValue(); 702 } 703 704 /** 705 * @param value Participation status of the participant. When the status is 706 * declined or tentative if the start/end times are different to 707 * the appointment, then these times should be interpreted as a 708 * requested time change. When the status is accepted, the times 709 * can either be the time of the appointment (as a confirmation of 710 * the time) or can be empty. 711 */ 712 public AppointmentResponse setParticipantStatus(ParticipantStatus value) { 713 if (this.participantStatus == null) 714 this.participantStatus = new Enumeration<ParticipantStatus>(new ParticipantStatusEnumFactory()); 715 this.participantStatus.setValue(value); 716 return this; 717 } 718 719 /** 720 * @return {@link #comment} (Additional comments about the appointment.). This 721 * is the underlying object with id, value and extensions. The accessor 722 * "getComment" gives direct access to the value 723 */ 724 public StringType getCommentElement() { 725 if (this.comment == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create AppointmentResponse.comment"); 728 else if (Configuration.doAutoCreate()) 729 this.comment = new StringType(); // bb 730 return this.comment; 731 } 732 733 public boolean hasCommentElement() { 734 return this.comment != null && !this.comment.isEmpty(); 735 } 736 737 public boolean hasComment() { 738 return this.comment != null && !this.comment.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #comment} (Additional comments about the appointment.). 743 * This is the underlying object with id, value and extensions. The 744 * accessor "getComment" gives direct access to the value 745 */ 746 public AppointmentResponse setCommentElement(StringType value) { 747 this.comment = value; 748 return this; 749 } 750 751 /** 752 * @return Additional comments about the appointment. 753 */ 754 public String getComment() { 755 return this.comment == null ? null : this.comment.getValue(); 756 } 757 758 /** 759 * @param value Additional comments about the appointment. 760 */ 761 public AppointmentResponse setComment(String value) { 762 if (Utilities.noString(value)) 763 this.comment = null; 764 else { 765 if (this.comment == null) 766 this.comment = new StringType(); 767 this.comment.setValue(value); 768 } 769 return this; 770 } 771 772 protected void listChildren(List<Property> children) { 773 super.listChildren(children); 774 children.add(new Property("identifier", "Identifier", 775 "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 776 0, java.lang.Integer.MAX_VALUE, identifier)); 777 children.add(new Property("appointment", "Reference(Appointment)", "Appointment that this response is replying to.", 778 0, 1, appointment)); 779 children.add(new Property("start", "instant", 780 "Date/Time that the appointment is to take place, or requested new start time.", 0, 1, start)); 781 children.add(new Property("end", "instant", 782 "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 783 0, 1, end)); 784 children.add(new Property("participantType", "CodeableConcept", "Role of participant in the appointment.", 0, 785 java.lang.Integer.MAX_VALUE, participantType)); 786 children.add(new Property("actor", 787 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 788 "A Person, Location, HealthcareService, or Device that is participating in the appointment.", 0, 1, actor)); 789 children.add(new Property("participantStatus", "code", 790 "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 791 0, 1, participantStatus)); 792 children.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 1, comment)); 793 } 794 795 @Override 796 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 797 switch (_hash) { 798 case -1618432855: 799 /* identifier */ return new Property("identifier", "Identifier", 800 "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 801 0, java.lang.Integer.MAX_VALUE, identifier); 802 case -1474995297: 803 /* appointment */ return new Property("appointment", "Reference(Appointment)", 804 "Appointment that this response is replying to.", 0, 1, appointment); 805 case 109757538: 806 /* start */ return new Property("start", "instant", 807 "Date/Time that the appointment is to take place, or requested new start time.", 0, 1, start); 808 case 100571: 809 /* end */ return new Property("end", "instant", 810 "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 811 0, 1, end); 812 case 841294093: 813 /* participantType */ return new Property("participantType", "CodeableConcept", 814 "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, participantType); 815 case 92645877: 816 /* actor */ return new Property("actor", 817 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 818 "A Person, Location, HealthcareService, or Device that is participating in the appointment.", 0, 1, actor); 819 case 996096261: 820 /* participantStatus */ return new Property("participantStatus", "code", 821 "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 822 0, 1, participantStatus); 823 case 950398559: 824 /* comment */ return new Property("comment", "string", "Additional comments about the appointment.", 0, 1, 825 comment); 826 default: 827 return super.getNamedProperty(_hash, _name, _checkValid); 828 } 829 830 } 831 832 @Override 833 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 834 switch (hash) { 835 case -1618432855: 836 /* identifier */ return this.identifier == null ? new Base[0] 837 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 838 case -1474995297: 839 /* appointment */ return this.appointment == null ? new Base[0] : new Base[] { this.appointment }; // Reference 840 case 109757538: 841 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // InstantType 842 case 100571: 843 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // InstantType 844 case 841294093: 845 /* participantType */ return this.participantType == null ? new Base[0] 846 : this.participantType.toArray(new Base[this.participantType.size()]); // CodeableConcept 847 case 92645877: 848 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 849 case 996096261: 850 /* participantStatus */ return this.participantStatus == null ? new Base[0] 851 : new Base[] { this.participantStatus }; // Enumeration<ParticipantStatus> 852 case 950398559: 853 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 854 default: 855 return super.getProperty(hash, name, checkValid); 856 } 857 858 } 859 860 @Override 861 public Base setProperty(int hash, String name, Base value) throws FHIRException { 862 switch (hash) { 863 case -1618432855: // identifier 864 this.getIdentifier().add(castToIdentifier(value)); // Identifier 865 return value; 866 case -1474995297: // appointment 867 this.appointment = castToReference(value); // Reference 868 return value; 869 case 109757538: // start 870 this.start = castToInstant(value); // InstantType 871 return value; 872 case 100571: // end 873 this.end = castToInstant(value); // InstantType 874 return value; 875 case 841294093: // participantType 876 this.getParticipantType().add(castToCodeableConcept(value)); // CodeableConcept 877 return value; 878 case 92645877: // actor 879 this.actor = castToReference(value); // Reference 880 return value; 881 case 996096261: // participantStatus 882 value = new ParticipantStatusEnumFactory().fromType(castToCode(value)); 883 this.participantStatus = (Enumeration) value; // Enumeration<ParticipantStatus> 884 return value; 885 case 950398559: // comment 886 this.comment = castToString(value); // StringType 887 return value; 888 default: 889 return super.setProperty(hash, name, value); 890 } 891 892 } 893 894 @Override 895 public Base setProperty(String name, Base value) throws FHIRException { 896 if (name.equals("identifier")) { 897 this.getIdentifier().add(castToIdentifier(value)); 898 } else if (name.equals("appointment")) { 899 this.appointment = castToReference(value); // Reference 900 } else if (name.equals("start")) { 901 this.start = castToInstant(value); // InstantType 902 } else if (name.equals("end")) { 903 this.end = castToInstant(value); // InstantType 904 } else if (name.equals("participantType")) { 905 this.getParticipantType().add(castToCodeableConcept(value)); 906 } else if (name.equals("actor")) { 907 this.actor = castToReference(value); // Reference 908 } else if (name.equals("participantStatus")) { 909 value = new ParticipantStatusEnumFactory().fromType(castToCode(value)); 910 this.participantStatus = (Enumeration) value; // Enumeration<ParticipantStatus> 911 } else if (name.equals("comment")) { 912 this.comment = castToString(value); // StringType 913 } else 914 return super.setProperty(name, value); 915 return value; 916 } 917 918 @Override 919 public void removeChild(String name, Base value) throws FHIRException { 920 if (name.equals("identifier")) { 921 this.getIdentifier().remove(castToIdentifier(value)); 922 } else if (name.equals("appointment")) { 923 this.appointment = null; 924 } else if (name.equals("start")) { 925 this.start = null; 926 } else if (name.equals("end")) { 927 this.end = null; 928 } else if (name.equals("participantType")) { 929 this.getParticipantType().remove(castToCodeableConcept(value)); 930 } else if (name.equals("actor")) { 931 this.actor = null; 932 } else if (name.equals("participantStatus")) { 933 this.participantStatus = null; 934 } else if (name.equals("comment")) { 935 this.comment = null; 936 } else 937 super.removeChild(name, value); 938 939 } 940 941 @Override 942 public Base makeProperty(int hash, String name) throws FHIRException { 943 switch (hash) { 944 case -1618432855: 945 return addIdentifier(); 946 case -1474995297: 947 return getAppointment(); 948 case 109757538: 949 return getStartElement(); 950 case 100571: 951 return getEndElement(); 952 case 841294093: 953 return addParticipantType(); 954 case 92645877: 955 return getActor(); 956 case 996096261: 957 return getParticipantStatusElement(); 958 case 950398559: 959 return getCommentElement(); 960 default: 961 return super.makeProperty(hash, name); 962 } 963 964 } 965 966 @Override 967 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 968 switch (hash) { 969 case -1618432855: 970 /* identifier */ return new String[] { "Identifier" }; 971 case -1474995297: 972 /* appointment */ return new String[] { "Reference" }; 973 case 109757538: 974 /* start */ return new String[] { "instant" }; 975 case 100571: 976 /* end */ return new String[] { "instant" }; 977 case 841294093: 978 /* participantType */ return new String[] { "CodeableConcept" }; 979 case 92645877: 980 /* actor */ return new String[] { "Reference" }; 981 case 996096261: 982 /* participantStatus */ return new String[] { "code" }; 983 case 950398559: 984 /* comment */ return new String[] { "string" }; 985 default: 986 return super.getTypesForProperty(hash, name); 987 } 988 989 } 990 991 @Override 992 public Base addChild(String name) throws FHIRException { 993 if (name.equals("identifier")) { 994 return addIdentifier(); 995 } else if (name.equals("appointment")) { 996 this.appointment = new Reference(); 997 return this.appointment; 998 } else if (name.equals("start")) { 999 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.start"); 1000 } else if (name.equals("end")) { 1001 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.end"); 1002 } else if (name.equals("participantType")) { 1003 return addParticipantType(); 1004 } else if (name.equals("actor")) { 1005 this.actor = new Reference(); 1006 return this.actor; 1007 } else if (name.equals("participantStatus")) { 1008 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.participantStatus"); 1009 } else if (name.equals("comment")) { 1010 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.comment"); 1011 } else 1012 return super.addChild(name); 1013 } 1014 1015 public String fhirType() { 1016 return "AppointmentResponse"; 1017 1018 } 1019 1020 public AppointmentResponse copy() { 1021 AppointmentResponse dst = new AppointmentResponse(); 1022 copyValues(dst); 1023 return dst; 1024 } 1025 1026 public void copyValues(AppointmentResponse dst) { 1027 super.copyValues(dst); 1028 if (identifier != null) { 1029 dst.identifier = new ArrayList<Identifier>(); 1030 for (Identifier i : identifier) 1031 dst.identifier.add(i.copy()); 1032 } 1033 ; 1034 dst.appointment = appointment == null ? null : appointment.copy(); 1035 dst.start = start == null ? null : start.copy(); 1036 dst.end = end == null ? null : end.copy(); 1037 if (participantType != null) { 1038 dst.participantType = new ArrayList<CodeableConcept>(); 1039 for (CodeableConcept i : participantType) 1040 dst.participantType.add(i.copy()); 1041 } 1042 ; 1043 dst.actor = actor == null ? null : actor.copy(); 1044 dst.participantStatus = participantStatus == null ? null : participantStatus.copy(); 1045 dst.comment = comment == null ? null : comment.copy(); 1046 } 1047 1048 protected AppointmentResponse typedCopy() { 1049 return copy(); 1050 } 1051 1052 @Override 1053 public boolean equalsDeep(Base other_) { 1054 if (!super.equalsDeep(other_)) 1055 return false; 1056 if (!(other_ instanceof AppointmentResponse)) 1057 return false; 1058 AppointmentResponse o = (AppointmentResponse) other_; 1059 return compareDeep(identifier, o.identifier, true) && compareDeep(appointment, o.appointment, true) 1060 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 1061 && compareDeep(participantType, o.participantType, true) && compareDeep(actor, o.actor, true) 1062 && compareDeep(participantStatus, o.participantStatus, true) && compareDeep(comment, o.comment, true); 1063 } 1064 1065 @Override 1066 public boolean equalsShallow(Base other_) { 1067 if (!super.equalsShallow(other_)) 1068 return false; 1069 if (!(other_ instanceof AppointmentResponse)) 1070 return false; 1071 AppointmentResponse o = (AppointmentResponse) other_; 1072 return compareValues(start, o.start, true) && compareValues(end, o.end, true) 1073 && compareValues(participantStatus, o.participantStatus, true) && compareValues(comment, o.comment, true); 1074 } 1075 1076 public boolean isEmpty() { 1077 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, appointment, start, end, participantType, 1078 actor, participantStatus, comment); 1079 } 1080 1081 @Override 1082 public ResourceType getResourceType() { 1083 return ResourceType.AppointmentResponse; 1084 } 1085 1086 /** 1087 * Search parameter: <b>actor</b> 1088 * <p> 1089 * Description: <b>The Person, Location/HealthcareService or Device that this 1090 * appointment response replies for</b><br> 1091 * Type: <b>reference</b><br> 1092 * Path: <b>AppointmentResponse.actor</b><br> 1093 * </p> 1094 */ 1095 @SearchParamDefinition(name = "actor", path = "AppointmentResponse.actor", description = "The Person, Location/HealthcareService or Device that this appointment response replies for", type = "reference", providesMembershipIn = { 1096 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 1097 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 1098 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 1099 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 1100 HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, 1101 RelatedPerson.class }) 1102 public static final String SP_ACTOR = "actor"; 1103 /** 1104 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 1105 * <p> 1106 * Description: <b>The Person, Location/HealthcareService or Device that this 1107 * appointment response replies for</b><br> 1108 * Type: <b>reference</b><br> 1109 * Path: <b>AppointmentResponse.actor</b><br> 1110 * </p> 1111 */ 1112 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1113 SP_ACTOR); 1114 1115 /** 1116 * Constant for fluent queries to be used to add include statements. Specifies 1117 * the path value of "<b>AppointmentResponse:actor</b>". 1118 */ 1119 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include( 1120 "AppointmentResponse:actor").toLocked(); 1121 1122 /** 1123 * Search parameter: <b>identifier</b> 1124 * <p> 1125 * Description: <b>An Identifier in this appointment response</b><br> 1126 * Type: <b>token</b><br> 1127 * Path: <b>AppointmentResponse.identifier</b><br> 1128 * </p> 1129 */ 1130 @SearchParamDefinition(name = "identifier", path = "AppointmentResponse.identifier", description = "An Identifier in this appointment response", type = "token") 1131 public static final String SP_IDENTIFIER = "identifier"; 1132 /** 1133 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1134 * <p> 1135 * Description: <b>An Identifier in this appointment response</b><br> 1136 * Type: <b>token</b><br> 1137 * Path: <b>AppointmentResponse.identifier</b><br> 1138 * </p> 1139 */ 1140 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1141 SP_IDENTIFIER); 1142 1143 /** 1144 * Search parameter: <b>practitioner</b> 1145 * <p> 1146 * Description: <b>This Response is for this Practitioner</b><br> 1147 * Type: <b>reference</b><br> 1148 * Path: <b>AppointmentResponse.actor</b><br> 1149 * </p> 1150 */ 1151 @SearchParamDefinition(name = "practitioner", path = "AppointmentResponse.actor.where(resolve() is Practitioner)", description = "This Response is for this Practitioner", type = "reference", target = { 1152 Practitioner.class }) 1153 public static final String SP_PRACTITIONER = "practitioner"; 1154 /** 1155 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 1156 * <p> 1157 * Description: <b>This Response is for this Practitioner</b><br> 1158 * Type: <b>reference</b><br> 1159 * Path: <b>AppointmentResponse.actor</b><br> 1160 * </p> 1161 */ 1162 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1163 SP_PRACTITIONER); 1164 1165 /** 1166 * Constant for fluent queries to be used to add include statements. Specifies 1167 * the path value of "<b>AppointmentResponse:practitioner</b>". 1168 */ 1169 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 1170 "AppointmentResponse:practitioner").toLocked(); 1171 1172 /** 1173 * Search parameter: <b>part-status</b> 1174 * <p> 1175 * Description: <b>The participants acceptance status for this 1176 * appointment</b><br> 1177 * Type: <b>token</b><br> 1178 * Path: <b>AppointmentResponse.participantStatus</b><br> 1179 * </p> 1180 */ 1181 @SearchParamDefinition(name = "part-status", path = "AppointmentResponse.participantStatus", description = "The participants acceptance status for this appointment", type = "token") 1182 public static final String SP_PART_STATUS = "part-status"; 1183 /** 1184 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 1185 * <p> 1186 * Description: <b>The participants acceptance status for this 1187 * appointment</b><br> 1188 * Type: <b>token</b><br> 1189 * Path: <b>AppointmentResponse.participantStatus</b><br> 1190 * </p> 1191 */ 1192 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1193 SP_PART_STATUS); 1194 1195 /** 1196 * Search parameter: <b>patient</b> 1197 * <p> 1198 * Description: <b>This Response is for this Patient</b><br> 1199 * Type: <b>reference</b><br> 1200 * Path: <b>AppointmentResponse.actor</b><br> 1201 * </p> 1202 */ 1203 @SearchParamDefinition(name = "patient", path = "AppointmentResponse.actor.where(resolve() is Patient)", description = "This Response is for this Patient", type = "reference", target = { 1204 Patient.class }) 1205 public static final String SP_PATIENT = "patient"; 1206 /** 1207 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1208 * <p> 1209 * Description: <b>This Response is for this Patient</b><br> 1210 * Type: <b>reference</b><br> 1211 * Path: <b>AppointmentResponse.actor</b><br> 1212 * </p> 1213 */ 1214 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1215 SP_PATIENT); 1216 1217 /** 1218 * Constant for fluent queries to be used to add include statements. Specifies 1219 * the path value of "<b>AppointmentResponse:patient</b>". 1220 */ 1221 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1222 "AppointmentResponse:patient").toLocked(); 1223 1224 /** 1225 * Search parameter: <b>appointment</b> 1226 * <p> 1227 * Description: <b>The appointment that the response is attached to</b><br> 1228 * Type: <b>reference</b><br> 1229 * Path: <b>AppointmentResponse.appointment</b><br> 1230 * </p> 1231 */ 1232 @SearchParamDefinition(name = "appointment", path = "AppointmentResponse.appointment", description = "The appointment that the response is attached to", type = "reference", target = { 1233 Appointment.class }) 1234 public static final String SP_APPOINTMENT = "appointment"; 1235 /** 1236 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 1237 * <p> 1238 * Description: <b>The appointment that the response is attached to</b><br> 1239 * Type: <b>reference</b><br> 1240 * Path: <b>AppointmentResponse.appointment</b><br> 1241 * </p> 1242 */ 1243 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1244 SP_APPOINTMENT); 1245 1246 /** 1247 * Constant for fluent queries to be used to add include statements. Specifies 1248 * the path value of "<b>AppointmentResponse:appointment</b>". 1249 */ 1250 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include( 1251 "AppointmentResponse:appointment").toLocked(); 1252 1253 /** 1254 * Search parameter: <b>location</b> 1255 * <p> 1256 * Description: <b>This Response is for this Location</b><br> 1257 * Type: <b>reference</b><br> 1258 * Path: <b>AppointmentResponse.actor</b><br> 1259 * </p> 1260 */ 1261 @SearchParamDefinition(name = "location", path = "AppointmentResponse.actor.where(resolve() is Location)", description = "This Response is for this Location", type = "reference", target = { 1262 Location.class }) 1263 public static final String SP_LOCATION = "location"; 1264 /** 1265 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1266 * <p> 1267 * Description: <b>This Response is for this Location</b><br> 1268 * Type: <b>reference</b><br> 1269 * Path: <b>AppointmentResponse.actor</b><br> 1270 * </p> 1271 */ 1272 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1273 SP_LOCATION); 1274 1275 /** 1276 * Constant for fluent queries to be used to add include statements. Specifies 1277 * the path value of "<b>AppointmentResponse:location</b>". 1278 */ 1279 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 1280 "AppointmentResponse:location").toLocked(); 1281 1282}