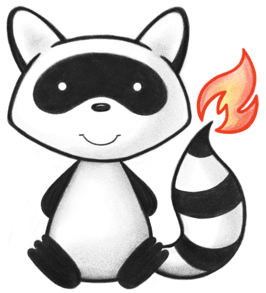
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.Date; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * For referring to data content defined in other formats. 046 */ 047@DatatypeDef(name = "Attachment") 048public class Attachment extends Type implements ICompositeType { 049 050 /** 051 * Identifies the type of the data in the attachment and allows a method to be 052 * chosen to interpret or render the data. Includes mime type parameters such as 053 * charset where appropriate. 054 */ 055 @Child(name = "contentType", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 056 @Description(shortDefinition = "Mime type of the content, with charset etc.", formalDefinition = "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.") 057 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 058 protected CodeType contentType; 059 060 /** 061 * The human language of the content. The value can be any valid value according 062 * to BCP 47. 063 */ 064 @Child(name = "language", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 065 @Description(shortDefinition = "Human language of the content (BCP-47)", formalDefinition = "The human language of the content. The value can be any valid value according to BCP 47.") 066 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 067 protected CodeType language; 068 069 /** 070 * The actual data of the attachment - a sequence of bytes, base64 encoded. 071 */ 072 @Child(name = "data", type = { 073 Base64BinaryType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 074 @Description(shortDefinition = "Data inline, base64ed", formalDefinition = "The actual data of the attachment - a sequence of bytes, base64 encoded.") 075 protected Base64BinaryType data; 076 077 /** 078 * A location where the data can be accessed. 079 */ 080 @Child(name = "url", type = { UrlType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 081 @Description(shortDefinition = "Uri where the data can be found", formalDefinition = "A location where the data can be accessed.") 082 protected UrlType url; 083 084 /** 085 * The number of bytes of data that make up this attachment (before base64 086 * encoding, if that is done). 087 */ 088 @Child(name = "size", type = { UnsignedIntType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 089 @Description(shortDefinition = "Number of bytes of content (if url provided)", formalDefinition = "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).") 090 protected UnsignedIntType size; 091 092 /** 093 * The calculated hash of the data using SHA-1. Represented using base64. 094 */ 095 @Child(name = "hash", type = { 096 Base64BinaryType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 097 @Description(shortDefinition = "Hash of the data (sha-1, base64ed)", formalDefinition = "The calculated hash of the data using SHA-1. Represented using base64.") 098 protected Base64BinaryType hash; 099 100 /** 101 * A label or set of text to display in place of the data. 102 */ 103 @Child(name = "title", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 104 @Description(shortDefinition = "Label to display in place of the data", formalDefinition = "A label or set of text to display in place of the data.") 105 protected StringType title; 106 107 /** 108 * The date that the attachment was first created. 109 */ 110 @Child(name = "creation", type = { 111 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 112 @Description(shortDefinition = "Date attachment was first created", formalDefinition = "The date that the attachment was first created.") 113 protected DateTimeType creation; 114 115 private static final long serialVersionUID = -564352571L; 116 117 /** 118 * Constructor 119 */ 120 public Attachment() { 121 super(); 122 } 123 124 /** 125 * @return {@link #contentType} (Identifies the type of the data in the 126 * attachment and allows a method to be chosen to interpret or render 127 * the data. Includes mime type parameters such as charset where 128 * appropriate.). This is the underlying object with id, value and 129 * extensions. The accessor "getContentType" gives direct access to the 130 * value 131 */ 132 public CodeType getContentTypeElement() { 133 if (this.contentType == null) 134 if (Configuration.errorOnAutoCreate()) 135 throw new Error("Attempt to auto-create Attachment.contentType"); 136 else if (Configuration.doAutoCreate()) 137 this.contentType = new CodeType(); // bb 138 return this.contentType; 139 } 140 141 public boolean hasContentTypeElement() { 142 return this.contentType != null && !this.contentType.isEmpty(); 143 } 144 145 public boolean hasContentType() { 146 return this.contentType != null && !this.contentType.isEmpty(); 147 } 148 149 /** 150 * @param value {@link #contentType} (Identifies the type of the data in the 151 * attachment and allows a method to be chosen to interpret or 152 * render the data. Includes mime type parameters such as charset 153 * where appropriate.). This is the underlying object with id, 154 * value and extensions. The accessor "getContentType" gives direct 155 * access to the value 156 */ 157 public Attachment setContentTypeElement(CodeType value) { 158 this.contentType = value; 159 return this; 160 } 161 162 /** 163 * @return Identifies the type of the data in the attachment and allows a method 164 * to be chosen to interpret or render the data. Includes mime type 165 * parameters such as charset where appropriate. 166 */ 167 public String getContentType() { 168 return this.contentType == null ? null : this.contentType.getValue(); 169 } 170 171 /** 172 * @param value Identifies the type of the data in the attachment and allows a 173 * method to be chosen to interpret or render the data. Includes 174 * mime type parameters such as charset where appropriate. 175 */ 176 public Attachment setContentType(String value) { 177 if (Utilities.noString(value)) 178 this.contentType = null; 179 else { 180 if (this.contentType == null) 181 this.contentType = new CodeType(); 182 this.contentType.setValue(value); 183 } 184 return this; 185 } 186 187 /** 188 * @return {@link #language} (The human language of the content. The value can 189 * be any valid value according to BCP 47.). This is the underlying 190 * object with id, value and extensions. The accessor "getLanguage" 191 * gives direct access to the value 192 */ 193 public CodeType getLanguageElement() { 194 if (this.language == null) 195 if (Configuration.errorOnAutoCreate()) 196 throw new Error("Attempt to auto-create Attachment.language"); 197 else if (Configuration.doAutoCreate()) 198 this.language = new CodeType(); // bb 199 return this.language; 200 } 201 202 public boolean hasLanguageElement() { 203 return this.language != null && !this.language.isEmpty(); 204 } 205 206 public boolean hasLanguage() { 207 return this.language != null && !this.language.isEmpty(); 208 } 209 210 /** 211 * @param value {@link #language} (The human language of the content. The value 212 * can be any valid value according to BCP 47.). This is the 213 * underlying object with id, value and extensions. The accessor 214 * "getLanguage" gives direct access to the value 215 */ 216 public Attachment setLanguageElement(CodeType value) { 217 this.language = value; 218 return this; 219 } 220 221 /** 222 * @return The human language of the content. The value can be any valid value 223 * according to BCP 47. 224 */ 225 public String getLanguage() { 226 return this.language == null ? null : this.language.getValue(); 227 } 228 229 /** 230 * @param value The human language of the content. The value can be any valid 231 * value according to BCP 47. 232 */ 233 public Attachment setLanguage(String value) { 234 if (Utilities.noString(value)) 235 this.language = null; 236 else { 237 if (this.language == null) 238 this.language = new CodeType(); 239 this.language.setValue(value); 240 } 241 return this; 242 } 243 244 /** 245 * @return {@link #data} (The actual data of the attachment - a sequence of 246 * bytes, base64 encoded.). This is the underlying object with id, value 247 * and extensions. The accessor "getData" gives direct access to the 248 * value 249 */ 250 public Base64BinaryType getDataElement() { 251 if (this.data == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create Attachment.data"); 254 else if (Configuration.doAutoCreate()) 255 this.data = new Base64BinaryType(); // bb 256 return this.data; 257 } 258 259 public boolean hasDataElement() { 260 return this.data != null && !this.data.isEmpty(); 261 } 262 263 public boolean hasData() { 264 return this.data != null && !this.data.isEmpty(); 265 } 266 267 /** 268 * @param value {@link #data} (The actual data of the attachment - a sequence of 269 * bytes, base64 encoded.). This is the underlying object with id, 270 * value and extensions. The accessor "getData" gives direct access 271 * to the value 272 */ 273 public Attachment setDataElement(Base64BinaryType value) { 274 this.data = value; 275 return this; 276 } 277 278 /** 279 * @return The actual data of the attachment - a sequence of bytes, base64 280 * encoded. 281 */ 282 public byte[] getData() { 283 return this.data == null ? null : this.data.getValue(); 284 } 285 286 /** 287 * @param value The actual data of the attachment - a sequence of bytes, base64 288 * encoded. 289 */ 290 public Attachment setData(byte[] value) { 291 if (value == null) 292 this.data = null; 293 else { 294 if (this.data == null) 295 this.data = new Base64BinaryType(); 296 this.data.setValue(value); 297 } 298 return this; 299 } 300 301 /** 302 * @return {@link #url} (A location where the data can be accessed.). This is 303 * the underlying object with id, value and extensions. The accessor 304 * "getUrl" gives direct access to the value 305 */ 306 public UrlType getUrlElement() { 307 if (this.url == null) 308 if (Configuration.errorOnAutoCreate()) 309 throw new Error("Attempt to auto-create Attachment.url"); 310 else if (Configuration.doAutoCreate()) 311 this.url = new UrlType(); // bb 312 return this.url; 313 } 314 315 public boolean hasUrlElement() { 316 return this.url != null && !this.url.isEmpty(); 317 } 318 319 public boolean hasUrl() { 320 return this.url != null && !this.url.isEmpty(); 321 } 322 323 /** 324 * @param value {@link #url} (A location where the data can be accessed.). This 325 * is the underlying object with id, value and extensions. The 326 * accessor "getUrl" gives direct access to the value 327 */ 328 public Attachment setUrlElement(UrlType value) { 329 this.url = value; 330 return this; 331 } 332 333 /** 334 * @return A location where the data can be accessed. 335 */ 336 public String getUrl() { 337 return this.url == null ? null : this.url.getValue(); 338 } 339 340 /** 341 * @param value A location where the data can be accessed. 342 */ 343 public Attachment setUrl(String value) { 344 if (Utilities.noString(value)) 345 this.url = null; 346 else { 347 if (this.url == null) 348 this.url = new UrlType(); 349 this.url.setValue(value); 350 } 351 return this; 352 } 353 354 /** 355 * @return {@link #size} (The number of bytes of data that make up this 356 * attachment (before base64 encoding, if that is done).). This is the 357 * underlying object with id, value and extensions. The accessor 358 * "getSize" gives direct access to the value 359 */ 360 public UnsignedIntType getSizeElement() { 361 if (this.size == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create Attachment.size"); 364 else if (Configuration.doAutoCreate()) 365 this.size = new UnsignedIntType(); // bb 366 return this.size; 367 } 368 369 public boolean hasSizeElement() { 370 return this.size != null && !this.size.isEmpty(); 371 } 372 373 public boolean hasSize() { 374 return this.size != null && !this.size.isEmpty(); 375 } 376 377 /** 378 * @param value {@link #size} (The number of bytes of data that make up this 379 * attachment (before base64 encoding, if that is done).). This is 380 * the underlying object with id, value and extensions. The 381 * accessor "getSize" gives direct access to the value 382 */ 383 public Attachment setSizeElement(UnsignedIntType value) { 384 this.size = value; 385 return this; 386 } 387 388 /** 389 * @return The number of bytes of data that make up this attachment (before 390 * base64 encoding, if that is done). 391 */ 392 public int getSize() { 393 return this.size == null || this.size.isEmpty() ? 0 : this.size.getValue(); 394 } 395 396 /** 397 * @param value The number of bytes of data that make up this attachment (before 398 * base64 encoding, if that is done). 399 */ 400 public Attachment setSize(int value) { 401 if (this.size == null) 402 this.size = new UnsignedIntType(); 403 this.size.setValue(value); 404 return this; 405 } 406 407 /** 408 * @return {@link #hash} (The calculated hash of the data using SHA-1. 409 * Represented using base64.). This is the underlying object with id, 410 * value and extensions. The accessor "getHash" gives direct access to 411 * the value 412 */ 413 public Base64BinaryType getHashElement() { 414 if (this.hash == null) 415 if (Configuration.errorOnAutoCreate()) 416 throw new Error("Attempt to auto-create Attachment.hash"); 417 else if (Configuration.doAutoCreate()) 418 this.hash = new Base64BinaryType(); // bb 419 return this.hash; 420 } 421 422 public boolean hasHashElement() { 423 return this.hash != null && !this.hash.isEmpty(); 424 } 425 426 public boolean hasHash() { 427 return this.hash != null && !this.hash.isEmpty(); 428 } 429 430 /** 431 * @param value {@link #hash} (The calculated hash of the data using SHA-1. 432 * Represented using base64.). This is the underlying object with 433 * id, value and extensions. The accessor "getHash" gives direct 434 * access to the value 435 */ 436 public Attachment setHashElement(Base64BinaryType value) { 437 this.hash = value; 438 return this; 439 } 440 441 /** 442 * @return The calculated hash of the data using SHA-1. Represented using 443 * base64. 444 */ 445 public byte[] getHash() { 446 return this.hash == null ? null : this.hash.getValue(); 447 } 448 449 /** 450 * @param value The calculated hash of the data using SHA-1. Represented using 451 * base64. 452 */ 453 public Attachment setHash(byte[] value) { 454 if (value == null) 455 this.hash = null; 456 else { 457 if (this.hash == null) 458 this.hash = new Base64BinaryType(); 459 this.hash.setValue(value); 460 } 461 return this; 462 } 463 464 /** 465 * @return {@link #title} (A label or set of text to display in place of the 466 * data.). This is the underlying object with id, value and extensions. 467 * The accessor "getTitle" gives direct access to the value 468 */ 469 public StringType getTitleElement() { 470 if (this.title == null) 471 if (Configuration.errorOnAutoCreate()) 472 throw new Error("Attempt to auto-create Attachment.title"); 473 else if (Configuration.doAutoCreate()) 474 this.title = new StringType(); // bb 475 return this.title; 476 } 477 478 public boolean hasTitleElement() { 479 return this.title != null && !this.title.isEmpty(); 480 } 481 482 public boolean hasTitle() { 483 return this.title != null && !this.title.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #title} (A label or set of text to display in place of 488 * the data.). This is the underlying object with id, value and 489 * extensions. The accessor "getTitle" gives direct access to the 490 * value 491 */ 492 public Attachment setTitleElement(StringType value) { 493 this.title = value; 494 return this; 495 } 496 497 /** 498 * @return A label or set of text to display in place of the data. 499 */ 500 public String getTitle() { 501 return this.title == null ? null : this.title.getValue(); 502 } 503 504 /** 505 * @param value A label or set of text to display in place of the data. 506 */ 507 public Attachment setTitle(String value) { 508 if (Utilities.noString(value)) 509 this.title = null; 510 else { 511 if (this.title == null) 512 this.title = new StringType(); 513 this.title.setValue(value); 514 } 515 return this; 516 } 517 518 /** 519 * @return {@link #creation} (The date that the attachment was first created.). 520 * This is the underlying object with id, value and extensions. The 521 * accessor "getCreation" gives direct access to the value 522 */ 523 public DateTimeType getCreationElement() { 524 if (this.creation == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create Attachment.creation"); 527 else if (Configuration.doAutoCreate()) 528 this.creation = new DateTimeType(); // bb 529 return this.creation; 530 } 531 532 public boolean hasCreationElement() { 533 return this.creation != null && !this.creation.isEmpty(); 534 } 535 536 public boolean hasCreation() { 537 return this.creation != null && !this.creation.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #creation} (The date that the attachment was first 542 * created.). This is the underlying object with id, value and 543 * extensions. The accessor "getCreation" gives direct access to 544 * the value 545 */ 546 public Attachment setCreationElement(DateTimeType value) { 547 this.creation = value; 548 return this; 549 } 550 551 /** 552 * @return The date that the attachment was first created. 553 */ 554 public Date getCreation() { 555 return this.creation == null ? null : this.creation.getValue(); 556 } 557 558 /** 559 * @param value The date that the attachment was first created. 560 */ 561 public Attachment setCreation(Date value) { 562 if (value == null) 563 this.creation = null; 564 else { 565 if (this.creation == null) 566 this.creation = new DateTimeType(); 567 this.creation.setValue(value); 568 } 569 return this; 570 } 571 572 protected void listChildren(List<Property> children) { 573 super.listChildren(children); 574 children.add(new Property("contentType", "code", 575 "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 576 0, 1, contentType)); 577 children.add(new Property("language", "code", 578 "The human language of the content. The value can be any valid value according to BCP 47.", 0, 1, language)); 579 children.add(new Property("data", "base64Binary", 580 "The actual data of the attachment - a sequence of bytes, base64 encoded.", 0, 1, data)); 581 children.add(new Property("url", "url", "A location where the data can be accessed.", 0, 1, url)); 582 children.add(new Property("size", "unsignedInt", 583 "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).", 0, 1, 584 size)); 585 children.add(new Property("hash", "base64Binary", 586 "The calculated hash of the data using SHA-1. Represented using base64.", 0, 1, hash)); 587 children 588 .add(new Property("title", "string", "A label or set of text to display in place of the data.", 0, 1, title)); 589 children 590 .add(new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 1, creation)); 591 } 592 593 @Override 594 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 595 switch (_hash) { 596 case -389131437: 597 /* contentType */ return new Property("contentType", "code", 598 "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 599 0, 1, contentType); 600 case -1613589672: 601 /* language */ return new Property("language", "code", 602 "The human language of the content. The value can be any valid value according to BCP 47.", 0, 1, language); 603 case 3076010: 604 /* data */ return new Property("data", "base64Binary", 605 "The actual data of the attachment - a sequence of bytes, base64 encoded.", 0, 1, data); 606 case 116079: 607 /* url */ return new Property("url", "url", "A location where the data can be accessed.", 0, 1, url); 608 case 3530753: 609 /* size */ return new Property("size", "unsignedInt", 610 "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).", 0, 1, 611 size); 612 case 3195150: 613 /* hash */ return new Property("hash", "base64Binary", 614 "The calculated hash of the data using SHA-1. Represented using base64.", 0, 1, hash); 615 case 110371416: 616 /* title */ return new Property("title", "string", "A label or set of text to display in place of the data.", 0, 617 1, title); 618 case 1820421855: 619 /* creation */ return new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 620 1, creation); 621 default: 622 return super.getNamedProperty(_hash, _name, _checkValid); 623 } 624 625 } 626 627 @Override 628 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 629 switch (hash) { 630 case -389131437: 631 /* contentType */ return this.contentType == null ? new Base[0] : new Base[] { this.contentType }; // CodeType 632 case -1613589672: 633 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeType 634 case 3076010: 635 /* data */ return this.data == null ? new Base[0] : new Base[] { this.data }; // Base64BinaryType 636 case 116079: 637 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UrlType 638 case 3530753: 639 /* size */ return this.size == null ? new Base[0] : new Base[] { this.size }; // UnsignedIntType 640 case 3195150: 641 /* hash */ return this.hash == null ? new Base[0] : new Base[] { this.hash }; // Base64BinaryType 642 case 110371416: 643 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 644 case 1820421855: 645 /* creation */ return this.creation == null ? new Base[0] : new Base[] { this.creation }; // DateTimeType 646 default: 647 return super.getProperty(hash, name, checkValid); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(int hash, String name, Base value) throws FHIRException { 654 switch (hash) { 655 case -389131437: // contentType 656 this.contentType = castToCode(value); // CodeType 657 return value; 658 case -1613589672: // language 659 this.language = castToCode(value); // CodeType 660 return value; 661 case 3076010: // data 662 this.data = castToBase64Binary(value); // Base64BinaryType 663 return value; 664 case 116079: // url 665 this.url = castToUrl(value); // UrlType 666 return value; 667 case 3530753: // size 668 this.size = castToUnsignedInt(value); // UnsignedIntType 669 return value; 670 case 3195150: // hash 671 this.hash = castToBase64Binary(value); // Base64BinaryType 672 return value; 673 case 110371416: // title 674 this.title = castToString(value); // StringType 675 return value; 676 case 1820421855: // creation 677 this.creation = castToDateTime(value); // DateTimeType 678 return value; 679 default: 680 return super.setProperty(hash, name, value); 681 } 682 683 } 684 685 @Override 686 public Base setProperty(String name, Base value) throws FHIRException { 687 if (name.equals("contentType")) { 688 this.contentType = castToCode(value); // CodeType 689 } else if (name.equals("language")) { 690 this.language = castToCode(value); // CodeType 691 } else if (name.equals("data")) { 692 this.data = castToBase64Binary(value); // Base64BinaryType 693 } else if (name.equals("url")) { 694 this.url = castToUrl(value); // UrlType 695 } else if (name.equals("size")) { 696 this.size = castToUnsignedInt(value); // UnsignedIntType 697 } else if (name.equals("hash")) { 698 this.hash = castToBase64Binary(value); // Base64BinaryType 699 } else if (name.equals("title")) { 700 this.title = castToString(value); // StringType 701 } else if (name.equals("creation")) { 702 this.creation = castToDateTime(value); // DateTimeType 703 } else 704 return super.setProperty(name, value); 705 return value; 706 } 707 708 @Override 709 public void removeChild(String name, Base value) throws FHIRException { 710 if (name.equals("contentType")) { 711 this.contentType = null; 712 } else if (name.equals("language")) { 713 this.language = null; 714 } else if (name.equals("data")) { 715 this.data = null; 716 } else if (name.equals("url")) { 717 this.url = null; 718 } else if (name.equals("size")) { 719 this.size = null; 720 } else if (name.equals("hash")) { 721 this.hash = null; 722 } else if (name.equals("title")) { 723 this.title = null; 724 } else if (name.equals("creation")) { 725 this.creation = null; 726 } else 727 super.removeChild(name, value); 728 729 } 730 731 @Override 732 public Base makeProperty(int hash, String name) throws FHIRException { 733 switch (hash) { 734 case -389131437: 735 return getContentTypeElement(); 736 case -1613589672: 737 return getLanguageElement(); 738 case 3076010: 739 return getDataElement(); 740 case 116079: 741 return getUrlElement(); 742 case 3530753: 743 return getSizeElement(); 744 case 3195150: 745 return getHashElement(); 746 case 110371416: 747 return getTitleElement(); 748 case 1820421855: 749 return getCreationElement(); 750 default: 751 return super.makeProperty(hash, name); 752 } 753 754 } 755 756 @Override 757 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 758 switch (hash) { 759 case -389131437: 760 /* contentType */ return new String[] { "code" }; 761 case -1613589672: 762 /* language */ return new String[] { "code" }; 763 case 3076010: 764 /* data */ return new String[] { "base64Binary" }; 765 case 116079: 766 /* url */ return new String[] { "url" }; 767 case 3530753: 768 /* size */ return new String[] { "unsignedInt" }; 769 case 3195150: 770 /* hash */ return new String[] { "base64Binary" }; 771 case 110371416: 772 /* title */ return new String[] { "string" }; 773 case 1820421855: 774 /* creation */ return new String[] { "dateTime" }; 775 default: 776 return super.getTypesForProperty(hash, name); 777 } 778 779 } 780 781 @Override 782 public Base addChild(String name) throws FHIRException { 783 if (name.equals("contentType")) { 784 throw new FHIRException("Cannot call addChild on a singleton property Attachment.contentType"); 785 } else if (name.equals("language")) { 786 throw new FHIRException("Cannot call addChild on a singleton property Attachment.language"); 787 } else if (name.equals("data")) { 788 throw new FHIRException("Cannot call addChild on a singleton property Attachment.data"); 789 } else if (name.equals("url")) { 790 throw new FHIRException("Cannot call addChild on a singleton property Attachment.url"); 791 } else if (name.equals("size")) { 792 throw new FHIRException("Cannot call addChild on a singleton property Attachment.size"); 793 } else if (name.equals("hash")) { 794 throw new FHIRException("Cannot call addChild on a singleton property Attachment.hash"); 795 } else if (name.equals("title")) { 796 throw new FHIRException("Cannot call addChild on a singleton property Attachment.title"); 797 } else if (name.equals("creation")) { 798 throw new FHIRException("Cannot call addChild on a singleton property Attachment.creation"); 799 } else 800 return super.addChild(name); 801 } 802 803 public String fhirType() { 804 return "Attachment"; 805 806 } 807 808 public Attachment copy() { 809 Attachment dst = new Attachment(); 810 copyValues(dst); 811 return dst; 812 } 813 814 public void copyValues(Attachment dst) { 815 super.copyValues(dst); 816 dst.contentType = contentType == null ? null : contentType.copy(); 817 dst.language = language == null ? null : language.copy(); 818 dst.data = data == null ? null : data.copy(); 819 dst.url = url == null ? null : url.copy(); 820 dst.size = size == null ? null : size.copy(); 821 dst.hash = hash == null ? null : hash.copy(); 822 dst.title = title == null ? null : title.copy(); 823 dst.creation = creation == null ? null : creation.copy(); 824 } 825 826 protected Attachment typedCopy() { 827 return copy(); 828 } 829 830 @Override 831 public boolean equalsDeep(Base other_) { 832 if (!super.equalsDeep(other_)) 833 return false; 834 if (!(other_ instanceof Attachment)) 835 return false; 836 Attachment o = (Attachment) other_; 837 return compareDeep(contentType, o.contentType, true) && compareDeep(language, o.language, true) 838 && compareDeep(data, o.data, true) && compareDeep(url, o.url, true) && compareDeep(size, o.size, true) 839 && compareDeep(hash, o.hash, true) && compareDeep(title, o.title, true) 840 && compareDeep(creation, o.creation, true); 841 } 842 843 @Override 844 public boolean equalsShallow(Base other_) { 845 if (!super.equalsShallow(other_)) 846 return false; 847 if (!(other_ instanceof Attachment)) 848 return false; 849 Attachment o = (Attachment) other_; 850 return compareValues(contentType, o.contentType, true) && compareValues(language, o.language, true) 851 && compareValues(data, o.data, true) && compareValues(url, o.url, true) && compareValues(size, o.size, true) 852 && compareValues(hash, o.hash, true) && compareValues(title, o.title, true) 853 && compareValues(creation, o.creation, true); 854 } 855 856 public boolean isEmpty() { 857 return super.isEmpty() 858 && ca.uhn.fhir.util.ElementUtil.isEmpty(contentType, language, data, url, size, hash, title, creation); 859 } 860 861}