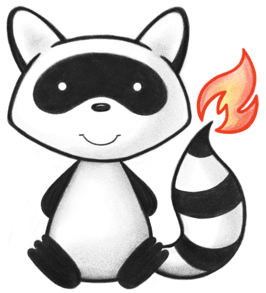
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A record of an event made for purposes of maintaining a security log. Typical 049 * uses include detection of intrusion attempts and monitoring for inappropriate 050 * usage. 051 */ 052@ResourceDef(name = "AuditEvent", profile = "http://hl7.org/fhir/StructureDefinition/AuditEvent") 053public class AuditEvent extends DomainResource { 054 055 public enum AuditEventAction { 056 /** 057 * Create a new database object, such as placing an order. 058 */ 059 C, 060 /** 061 * Display or print data, such as a doctor census. 062 */ 063 R, 064 /** 065 * Update data, such as revise patient information. 066 */ 067 U, 068 /** 069 * Delete items, such as a doctor master file record. 070 */ 071 D, 072 /** 073 * Perform a system or application function such as log-on, program execution or 074 * use of an object's method, or perform a query/search operation. 075 */ 076 E, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static AuditEventAction fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("C".equals(codeString)) 086 return C; 087 if ("R".equals(codeString)) 088 return R; 089 if ("U".equals(codeString)) 090 return U; 091 if ("D".equals(codeString)) 092 return D; 093 if ("E".equals(codeString)) 094 return E; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown AuditEventAction code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case C: 104 return "C"; 105 case R: 106 return "R"; 107 case U: 108 return "U"; 109 case D: 110 return "D"; 111 case E: 112 return "E"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case C: 123 return "http://hl7.org/fhir/audit-event-action"; 124 case R: 125 return "http://hl7.org/fhir/audit-event-action"; 126 case U: 127 return "http://hl7.org/fhir/audit-event-action"; 128 case D: 129 return "http://hl7.org/fhir/audit-event-action"; 130 case E: 131 return "http://hl7.org/fhir/audit-event-action"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case C: 142 return "Create a new database object, such as placing an order."; 143 case R: 144 return "Display or print data, such as a doctor census."; 145 case U: 146 return "Update data, such as revise patient information."; 147 case D: 148 return "Delete items, such as a doctor master file record."; 149 case E: 150 return "Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case C: 161 return "Create"; 162 case R: 163 return "Read/View/Print"; 164 case U: 165 return "Update"; 166 case D: 167 return "Delete"; 168 case E: 169 return "Execute"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class AuditEventActionEnumFactory implements EnumFactory<AuditEventAction> { 179 public AuditEventAction fromCode(String codeString) throws IllegalArgumentException { 180 if (codeString == null || "".equals(codeString)) 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("C".equals(codeString)) 184 return AuditEventAction.C; 185 if ("R".equals(codeString)) 186 return AuditEventAction.R; 187 if ("U".equals(codeString)) 188 return AuditEventAction.U; 189 if ("D".equals(codeString)) 190 return AuditEventAction.D; 191 if ("E".equals(codeString)) 192 return AuditEventAction.E; 193 throw new IllegalArgumentException("Unknown AuditEventAction code '" + codeString + "'"); 194 } 195 196 public Enumeration<AuditEventAction> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<AuditEventAction>(this, AuditEventAction.NULL, code); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<AuditEventAction>(this, AuditEventAction.NULL, code); 204 if ("C".equals(codeString)) 205 return new Enumeration<AuditEventAction>(this, AuditEventAction.C, code); 206 if ("R".equals(codeString)) 207 return new Enumeration<AuditEventAction>(this, AuditEventAction.R, code); 208 if ("U".equals(codeString)) 209 return new Enumeration<AuditEventAction>(this, AuditEventAction.U, code); 210 if ("D".equals(codeString)) 211 return new Enumeration<AuditEventAction>(this, AuditEventAction.D, code); 212 if ("E".equals(codeString)) 213 return new Enumeration<AuditEventAction>(this, AuditEventAction.E, code); 214 throw new FHIRException("Unknown AuditEventAction code '" + codeString + "'"); 215 } 216 217 public String toCode(AuditEventAction code) { 218 if (code == AuditEventAction.C) 219 return "C"; 220 if (code == AuditEventAction.R) 221 return "R"; 222 if (code == AuditEventAction.U) 223 return "U"; 224 if (code == AuditEventAction.D) 225 return "D"; 226 if (code == AuditEventAction.E) 227 return "E"; 228 return "?"; 229 } 230 231 public String toSystem(AuditEventAction code) { 232 return code.getSystem(); 233 } 234 } 235 236 public enum AuditEventOutcome { 237 /** 238 * The operation completed successfully (whether with warnings or not). 239 */ 240 _0, 241 /** 242 * The action was not successful due to some kind of minor failure (often 243 * equivalent to an HTTP 400 response). 244 */ 245 _4, 246 /** 247 * The action was not successful due to some kind of unexpected error (often 248 * equivalent to an HTTP 500 response). 249 */ 250 _8, 251 /** 252 * An error of such magnitude occurred that the system is no longer available 253 * for use (i.e. the system died). 254 */ 255 _12, 256 /** 257 * added to help the parsers with the generic types 258 */ 259 NULL; 260 261 public static AuditEventOutcome fromCode(String codeString) throws FHIRException { 262 if (codeString == null || "".equals(codeString)) 263 return null; 264 if ("0".equals(codeString)) 265 return _0; 266 if ("4".equals(codeString)) 267 return _4; 268 if ("8".equals(codeString)) 269 return _8; 270 if ("12".equals(codeString)) 271 return _12; 272 if (Configuration.isAcceptInvalidEnums()) 273 return null; 274 else 275 throw new FHIRException("Unknown AuditEventOutcome code '" + codeString + "'"); 276 } 277 278 public String toCode() { 279 switch (this) { 280 case _0: 281 return "0"; 282 case _4: 283 return "4"; 284 case _8: 285 return "8"; 286 case _12: 287 return "12"; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 295 public String getSystem() { 296 switch (this) { 297 case _0: 298 return "http://hl7.org/fhir/audit-event-outcome"; 299 case _4: 300 return "http://hl7.org/fhir/audit-event-outcome"; 301 case _8: 302 return "http://hl7.org/fhir/audit-event-outcome"; 303 case _12: 304 return "http://hl7.org/fhir/audit-event-outcome"; 305 case NULL: 306 return null; 307 default: 308 return "?"; 309 } 310 } 311 312 public String getDefinition() { 313 switch (this) { 314 case _0: 315 return "The operation completed successfully (whether with warnings or not)."; 316 case _4: 317 return "The action was not successful due to some kind of minor failure (often equivalent to an HTTP 400 response)."; 318 case _8: 319 return "The action was not successful due to some kind of unexpected error (often equivalent to an HTTP 500 response)."; 320 case _12: 321 return "An error of such magnitude occurred that the system is no longer available for use (i.e. the system died)."; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 329 public String getDisplay() { 330 switch (this) { 331 case _0: 332 return "Success"; 333 case _4: 334 return "Minor failure"; 335 case _8: 336 return "Serious failure"; 337 case _12: 338 return "Major failure"; 339 case NULL: 340 return null; 341 default: 342 return "?"; 343 } 344 } 345 } 346 347 public static class AuditEventOutcomeEnumFactory implements EnumFactory<AuditEventOutcome> { 348 public AuditEventOutcome fromCode(String codeString) throws IllegalArgumentException { 349 if (codeString == null || "".equals(codeString)) 350 if (codeString == null || "".equals(codeString)) 351 return null; 352 if ("0".equals(codeString)) 353 return AuditEventOutcome._0; 354 if ("4".equals(codeString)) 355 return AuditEventOutcome._4; 356 if ("8".equals(codeString)) 357 return AuditEventOutcome._8; 358 if ("12".equals(codeString)) 359 return AuditEventOutcome._12; 360 throw new IllegalArgumentException("Unknown AuditEventOutcome code '" + codeString + "'"); 361 } 362 363 public Enumeration<AuditEventOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 364 if (code == null) 365 return null; 366 if (code.isEmpty()) 367 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome.NULL, code); 368 String codeString = code.asStringValue(); 369 if (codeString == null || "".equals(codeString)) 370 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome.NULL, code); 371 if ("0".equals(codeString)) 372 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._0, code); 373 if ("4".equals(codeString)) 374 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._4, code); 375 if ("8".equals(codeString)) 376 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._8, code); 377 if ("12".equals(codeString)) 378 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._12, code); 379 throw new FHIRException("Unknown AuditEventOutcome code '" + codeString + "'"); 380 } 381 382 public String toCode(AuditEventOutcome code) { 383 if (code == AuditEventOutcome._0) 384 return "0"; 385 if (code == AuditEventOutcome._4) 386 return "4"; 387 if (code == AuditEventOutcome._8) 388 return "8"; 389 if (code == AuditEventOutcome._12) 390 return "12"; 391 return "?"; 392 } 393 394 public String toSystem(AuditEventOutcome code) { 395 return code.getSystem(); 396 } 397 } 398 399 public enum AuditEventAgentNetworkType { 400 /** 401 * The machine name, including DNS name. 402 */ 403 _1, 404 /** 405 * The assigned Internet Protocol (IP) address. 406 */ 407 _2, 408 /** 409 * The assigned telephone number. 410 */ 411 _3, 412 /** 413 * The assigned email address. 414 */ 415 _4, 416 /** 417 * URI (User directory, HTTP-PUT, ftp, etc.). 418 */ 419 _5, 420 /** 421 * added to help the parsers with the generic types 422 */ 423 NULL; 424 425 public static AuditEventAgentNetworkType fromCode(String codeString) throws FHIRException { 426 if (codeString == null || "".equals(codeString)) 427 return null; 428 if ("1".equals(codeString)) 429 return _1; 430 if ("2".equals(codeString)) 431 return _2; 432 if ("3".equals(codeString)) 433 return _3; 434 if ("4".equals(codeString)) 435 return _4; 436 if ("5".equals(codeString)) 437 return _5; 438 if (Configuration.isAcceptInvalidEnums()) 439 return null; 440 else 441 throw new FHIRException("Unknown AuditEventAgentNetworkType code '" + codeString + "'"); 442 } 443 444 public String toCode() { 445 switch (this) { 446 case _1: 447 return "1"; 448 case _2: 449 return "2"; 450 case _3: 451 return "3"; 452 case _4: 453 return "4"; 454 case _5: 455 return "5"; 456 case NULL: 457 return null; 458 default: 459 return "?"; 460 } 461 } 462 463 public String getSystem() { 464 switch (this) { 465 case _1: 466 return "http://hl7.org/fhir/network-type"; 467 case _2: 468 return "http://hl7.org/fhir/network-type"; 469 case _3: 470 return "http://hl7.org/fhir/network-type"; 471 case _4: 472 return "http://hl7.org/fhir/network-type"; 473 case _5: 474 return "http://hl7.org/fhir/network-type"; 475 case NULL: 476 return null; 477 default: 478 return "?"; 479 } 480 } 481 482 public String getDefinition() { 483 switch (this) { 484 case _1: 485 return "The machine name, including DNS name."; 486 case _2: 487 return "The assigned Internet Protocol (IP) address."; 488 case _3: 489 return "The assigned telephone number."; 490 case _4: 491 return "The assigned email address."; 492 case _5: 493 return "URI (User directory, HTTP-PUT, ftp, etc.)."; 494 case NULL: 495 return null; 496 default: 497 return "?"; 498 } 499 } 500 501 public String getDisplay() { 502 switch (this) { 503 case _1: 504 return "Machine Name"; 505 case _2: 506 return "IP Address"; 507 case _3: 508 return "Telephone Number"; 509 case _4: 510 return "Email address"; 511 case _5: 512 return "URI"; 513 case NULL: 514 return null; 515 default: 516 return "?"; 517 } 518 } 519 } 520 521 public static class AuditEventAgentNetworkTypeEnumFactory implements EnumFactory<AuditEventAgentNetworkType> { 522 public AuditEventAgentNetworkType fromCode(String codeString) throws IllegalArgumentException { 523 if (codeString == null || "".equals(codeString)) 524 if (codeString == null || "".equals(codeString)) 525 return null; 526 if ("1".equals(codeString)) 527 return AuditEventAgentNetworkType._1; 528 if ("2".equals(codeString)) 529 return AuditEventAgentNetworkType._2; 530 if ("3".equals(codeString)) 531 return AuditEventAgentNetworkType._3; 532 if ("4".equals(codeString)) 533 return AuditEventAgentNetworkType._4; 534 if ("5".equals(codeString)) 535 return AuditEventAgentNetworkType._5; 536 throw new IllegalArgumentException("Unknown AuditEventAgentNetworkType code '" + codeString + "'"); 537 } 538 539 public Enumeration<AuditEventAgentNetworkType> fromType(PrimitiveType<?> code) throws FHIRException { 540 if (code == null) 541 return null; 542 if (code.isEmpty()) 543 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType.NULL, code); 544 String codeString = code.asStringValue(); 545 if (codeString == null || "".equals(codeString)) 546 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType.NULL, code); 547 if ("1".equals(codeString)) 548 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._1, code); 549 if ("2".equals(codeString)) 550 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._2, code); 551 if ("3".equals(codeString)) 552 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._3, code); 553 if ("4".equals(codeString)) 554 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._4, code); 555 if ("5".equals(codeString)) 556 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._5, code); 557 throw new FHIRException("Unknown AuditEventAgentNetworkType code '" + codeString + "'"); 558 } 559 560 public String toCode(AuditEventAgentNetworkType code) { 561 if (code == AuditEventAgentNetworkType._1) 562 return "1"; 563 if (code == AuditEventAgentNetworkType._2) 564 return "2"; 565 if (code == AuditEventAgentNetworkType._3) 566 return "3"; 567 if (code == AuditEventAgentNetworkType._4) 568 return "4"; 569 if (code == AuditEventAgentNetworkType._5) 570 return "5"; 571 return "?"; 572 } 573 574 public String toSystem(AuditEventAgentNetworkType code) { 575 return code.getSystem(); 576 } 577 } 578 579 @Block() 580 public static class AuditEventAgentComponent extends BackboneElement implements IBaseBackboneElement { 581 /** 582 * Specification of the participation type the user plays when performing the 583 * event. 584 */ 585 @Child(name = "type", type = { 586 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 587 @Description(shortDefinition = "How agent participated", formalDefinition = "Specification of the participation type the user plays when performing the event.") 588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participation-role-type") 589 protected CodeableConcept type; 590 591 /** 592 * The security role that the user was acting under, that come from local codes 593 * defined by the access control security system (e.g. RBAC, ABAC) used in the 594 * local context. 595 */ 596 @Child(name = "role", type = { 597 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 598 @Description(shortDefinition = "Agent role in the event", formalDefinition = "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.") 599 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-role-type") 600 protected List<CodeableConcept> role; 601 602 /** 603 * Reference to who this agent is that was involved in the event. 604 */ 605 @Child(name = "who", type = { PractitionerRole.class, Practitioner.class, Organization.class, Device.class, 606 Patient.class, RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 607 @Description(shortDefinition = "Identifier of who", formalDefinition = "Reference to who this agent is that was involved in the event.") 608 protected Reference who; 609 610 /** 611 * The actual object that is the target of the reference (Reference to who this 612 * agent is that was involved in the event.) 613 */ 614 protected Resource whoTarget; 615 616 /** 617 * Alternative agent Identifier. For a human, this should be a user identifier 618 * text string from authentication system. This identifier would be one known to 619 * a common authentication system (e.g. single sign-on), if available. 620 */ 621 @Child(name = "altId", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 622 @Description(shortDefinition = "Alternative User identity", formalDefinition = "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.") 623 protected StringType altId; 624 625 /** 626 * Human-meaningful name for the agent. 627 */ 628 @Child(name = "name", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 629 @Description(shortDefinition = "Human friendly name for the agent", formalDefinition = "Human-meaningful name for the agent.") 630 protected StringType name; 631 632 /** 633 * Indicator that the user is or is not the requestor, or initiator, for the 634 * event being audited. 635 */ 636 @Child(name = "requestor", type = { 637 BooleanType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 638 @Description(shortDefinition = "Whether user is initiator", formalDefinition = "Indicator that the user is or is not the requestor, or initiator, for the event being audited.") 639 protected BooleanType requestor; 640 641 /** 642 * Where the event occurred. 643 */ 644 @Child(name = "location", type = { Location.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 645 @Description(shortDefinition = "Where", formalDefinition = "Where the event occurred.") 646 protected Reference location; 647 648 /** 649 * The actual object that is the target of the reference (Where the event 650 * occurred.) 651 */ 652 protected Location locationTarget; 653 654 /** 655 * The policy or plan that authorized the activity being recorded. Typically, a 656 * single activity may have multiple applicable policies, such as patient 657 * consent, guarantor funding, etc. The policy would also indicate the security 658 * token used. 659 */ 660 @Child(name = "policy", type = { 661 UriType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 662 @Description(shortDefinition = "Policy that authorized event", formalDefinition = "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.") 663 protected List<UriType> policy; 664 665 /** 666 * Type of media involved. Used when the event is about exporting/importing onto 667 * media. 668 */ 669 @Child(name = "media", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 670 @Description(shortDefinition = "Type of media", formalDefinition = "Type of media involved. Used when the event is about exporting/importing onto media.") 671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/dicm-405-mediatype") 672 protected Coding media; 673 674 /** 675 * Logical network location for application activity, if the activity has a 676 * network location. 677 */ 678 @Child(name = "network", type = {}, order = 10, min = 0, max = 1, modifier = false, summary = false) 679 @Description(shortDefinition = "Logical network location for application activity", formalDefinition = "Logical network location for application activity, if the activity has a network location.") 680 protected AuditEventAgentNetworkComponent network; 681 682 /** 683 * The reason (purpose of use), specific to this agent, that was used during the 684 * event being recorded. 685 */ 686 @Child(name = "purposeOfUse", type = { 687 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 688 @Description(shortDefinition = "Reason given for this user", formalDefinition = "The reason (purpose of use), specific to this agent, that was used during the event being recorded.") 689 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 690 protected List<CodeableConcept> purposeOfUse; 691 692 private static final long serialVersionUID = -957410638L; 693 694 /** 695 * Constructor 696 */ 697 public AuditEventAgentComponent() { 698 super(); 699 } 700 701 /** 702 * Constructor 703 */ 704 public AuditEventAgentComponent(BooleanType requestor) { 705 super(); 706 this.requestor = requestor; 707 } 708 709 /** 710 * @return {@link #type} (Specification of the participation type the user plays 711 * when performing the event.) 712 */ 713 public CodeableConcept getType() { 714 if (this.type == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create AuditEventAgentComponent.type"); 717 else if (Configuration.doAutoCreate()) 718 this.type = new CodeableConcept(); // cc 719 return this.type; 720 } 721 722 public boolean hasType() { 723 return this.type != null && !this.type.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #type} (Specification of the participation type the user 728 * plays when performing the event.) 729 */ 730 public AuditEventAgentComponent setType(CodeableConcept value) { 731 this.type = value; 732 return this; 733 } 734 735 /** 736 * @return {@link #role} (The security role that the user was acting under, that 737 * come from local codes defined by the access control security system 738 * (e.g. RBAC, ABAC) used in the local context.) 739 */ 740 public List<CodeableConcept> getRole() { 741 if (this.role == null) 742 this.role = new ArrayList<CodeableConcept>(); 743 return this.role; 744 } 745 746 /** 747 * @return Returns a reference to <code>this</code> for easy method chaining 748 */ 749 public AuditEventAgentComponent setRole(List<CodeableConcept> theRole) { 750 this.role = theRole; 751 return this; 752 } 753 754 public boolean hasRole() { 755 if (this.role == null) 756 return false; 757 for (CodeableConcept item : this.role) 758 if (!item.isEmpty()) 759 return true; 760 return false; 761 } 762 763 public CodeableConcept addRole() { // 3 764 CodeableConcept t = new CodeableConcept(); 765 if (this.role == null) 766 this.role = new ArrayList<CodeableConcept>(); 767 this.role.add(t); 768 return t; 769 } 770 771 public AuditEventAgentComponent addRole(CodeableConcept t) { // 3 772 if (t == null) 773 return this; 774 if (this.role == null) 775 this.role = new ArrayList<CodeableConcept>(); 776 this.role.add(t); 777 return this; 778 } 779 780 /** 781 * @return The first repetition of repeating field {@link #role}, creating it if 782 * it does not already exist 783 */ 784 public CodeableConcept getRoleFirstRep() { 785 if (getRole().isEmpty()) { 786 addRole(); 787 } 788 return getRole().get(0); 789 } 790 791 /** 792 * @return {@link #who} (Reference to who this agent is that was involved in the 793 * event.) 794 */ 795 public Reference getWho() { 796 if (this.who == null) 797 if (Configuration.errorOnAutoCreate()) 798 throw new Error("Attempt to auto-create AuditEventAgentComponent.who"); 799 else if (Configuration.doAutoCreate()) 800 this.who = new Reference(); // cc 801 return this.who; 802 } 803 804 public boolean hasWho() { 805 return this.who != null && !this.who.isEmpty(); 806 } 807 808 /** 809 * @param value {@link #who} (Reference to who this agent is that was involved 810 * in the event.) 811 */ 812 public AuditEventAgentComponent setWho(Reference value) { 813 this.who = value; 814 return this; 815 } 816 817 /** 818 * @return {@link #who} The actual object that is the target of the reference. 819 * The reference library doesn't populate this, but you can use it to 820 * hold the resource if you resolve it. (Reference to who this agent is 821 * that was involved in the event.) 822 */ 823 public Resource getWhoTarget() { 824 return this.whoTarget; 825 } 826 827 /** 828 * @param value {@link #who} The actual object that is the target of the 829 * reference. The reference library doesn't use these, but you can 830 * use it to hold the resource if you resolve it. (Reference to who 831 * this agent is that was involved in the event.) 832 */ 833 public AuditEventAgentComponent setWhoTarget(Resource value) { 834 this.whoTarget = value; 835 return this; 836 } 837 838 /** 839 * @return {@link #altId} (Alternative agent Identifier. For a human, this 840 * should be a user identifier text string from authentication system. 841 * This identifier would be one known to a common authentication system 842 * (e.g. single sign-on), if available.). This is the underlying object 843 * with id, value and extensions. The accessor "getAltId" gives direct 844 * access to the value 845 */ 846 public StringType getAltIdElement() { 847 if (this.altId == null) 848 if (Configuration.errorOnAutoCreate()) 849 throw new Error("Attempt to auto-create AuditEventAgentComponent.altId"); 850 else if (Configuration.doAutoCreate()) 851 this.altId = new StringType(); // bb 852 return this.altId; 853 } 854 855 public boolean hasAltIdElement() { 856 return this.altId != null && !this.altId.isEmpty(); 857 } 858 859 public boolean hasAltId() { 860 return this.altId != null && !this.altId.isEmpty(); 861 } 862 863 /** 864 * @param value {@link #altId} (Alternative agent Identifier. For a human, this 865 * should be a user identifier text string from authentication 866 * system. This identifier would be one known to a common 867 * authentication system (e.g. single sign-on), if available.). 868 * This is the underlying object with id, value and extensions. The 869 * accessor "getAltId" gives direct access to the value 870 */ 871 public AuditEventAgentComponent setAltIdElement(StringType value) { 872 this.altId = value; 873 return this; 874 } 875 876 /** 877 * @return Alternative agent Identifier. For a human, this should be a user 878 * identifier text string from authentication system. This identifier 879 * would be one known to a common authentication system (e.g. single 880 * sign-on), if available. 881 */ 882 public String getAltId() { 883 return this.altId == null ? null : this.altId.getValue(); 884 } 885 886 /** 887 * @param value Alternative agent Identifier. For a human, this should be a user 888 * identifier text string from authentication system. This 889 * identifier would be one known to a common authentication system 890 * (e.g. single sign-on), if available. 891 */ 892 public AuditEventAgentComponent setAltId(String value) { 893 if (Utilities.noString(value)) 894 this.altId = null; 895 else { 896 if (this.altId == null) 897 this.altId = new StringType(); 898 this.altId.setValue(value); 899 } 900 return this; 901 } 902 903 /** 904 * @return {@link #name} (Human-meaningful name for the agent.). This is the 905 * underlying object with id, value and extensions. The accessor 906 * "getName" gives direct access to the value 907 */ 908 public StringType getNameElement() { 909 if (this.name == null) 910 if (Configuration.errorOnAutoCreate()) 911 throw new Error("Attempt to auto-create AuditEventAgentComponent.name"); 912 else if (Configuration.doAutoCreate()) 913 this.name = new StringType(); // bb 914 return this.name; 915 } 916 917 public boolean hasNameElement() { 918 return this.name != null && !this.name.isEmpty(); 919 } 920 921 public boolean hasName() { 922 return this.name != null && !this.name.isEmpty(); 923 } 924 925 /** 926 * @param value {@link #name} (Human-meaningful name for the agent.). This is 927 * the underlying object with id, value and extensions. The 928 * accessor "getName" gives direct access to the value 929 */ 930 public AuditEventAgentComponent setNameElement(StringType value) { 931 this.name = value; 932 return this; 933 } 934 935 /** 936 * @return Human-meaningful name for the agent. 937 */ 938 public String getName() { 939 return this.name == null ? null : this.name.getValue(); 940 } 941 942 /** 943 * @param value Human-meaningful name for the agent. 944 */ 945 public AuditEventAgentComponent setName(String value) { 946 if (Utilities.noString(value)) 947 this.name = null; 948 else { 949 if (this.name == null) 950 this.name = new StringType(); 951 this.name.setValue(value); 952 } 953 return this; 954 } 955 956 /** 957 * @return {@link #requestor} (Indicator that the user is or is not the 958 * requestor, or initiator, for the event being audited.). This is the 959 * underlying object with id, value and extensions. The accessor 960 * "getRequestor" gives direct access to the value 961 */ 962 public BooleanType getRequestorElement() { 963 if (this.requestor == null) 964 if (Configuration.errorOnAutoCreate()) 965 throw new Error("Attempt to auto-create AuditEventAgentComponent.requestor"); 966 else if (Configuration.doAutoCreate()) 967 this.requestor = new BooleanType(); // bb 968 return this.requestor; 969 } 970 971 public boolean hasRequestorElement() { 972 return this.requestor != null && !this.requestor.isEmpty(); 973 } 974 975 public boolean hasRequestor() { 976 return this.requestor != null && !this.requestor.isEmpty(); 977 } 978 979 /** 980 * @param value {@link #requestor} (Indicator that the user is or is not the 981 * requestor, or initiator, for the event being audited.). This is 982 * the underlying object with id, value and extensions. The 983 * accessor "getRequestor" gives direct access to the value 984 */ 985 public AuditEventAgentComponent setRequestorElement(BooleanType value) { 986 this.requestor = value; 987 return this; 988 } 989 990 /** 991 * @return Indicator that the user is or is not the requestor, or initiator, for 992 * the event being audited. 993 */ 994 public boolean getRequestor() { 995 return this.requestor == null || this.requestor.isEmpty() ? false : this.requestor.getValue(); 996 } 997 998 /** 999 * @param value Indicator that the user is or is not the requestor, or 1000 * initiator, for the event being audited. 1001 */ 1002 public AuditEventAgentComponent setRequestor(boolean value) { 1003 if (this.requestor == null) 1004 this.requestor = new BooleanType(); 1005 this.requestor.setValue(value); 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #location} (Where the event occurred.) 1011 */ 1012 public Reference getLocation() { 1013 if (this.location == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 1016 else if (Configuration.doAutoCreate()) 1017 this.location = new Reference(); // cc 1018 return this.location; 1019 } 1020 1021 public boolean hasLocation() { 1022 return this.location != null && !this.location.isEmpty(); 1023 } 1024 1025 /** 1026 * @param value {@link #location} (Where the event occurred.) 1027 */ 1028 public AuditEventAgentComponent setLocation(Reference value) { 1029 this.location = value; 1030 return this; 1031 } 1032 1033 /** 1034 * @return {@link #location} The actual object that is the target of the 1035 * reference. The reference library doesn't populate this, but you can 1036 * use it to hold the resource if you resolve it. (Where the event 1037 * occurred.) 1038 */ 1039 public Location getLocationTarget() { 1040 if (this.locationTarget == null) 1041 if (Configuration.errorOnAutoCreate()) 1042 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 1043 else if (Configuration.doAutoCreate()) 1044 this.locationTarget = new Location(); // aa 1045 return this.locationTarget; 1046 } 1047 1048 /** 1049 * @param value {@link #location} The actual object that is the target of the 1050 * reference. The reference library doesn't use these, but you can 1051 * use it to hold the resource if you resolve it. (Where the event 1052 * occurred.) 1053 */ 1054 public AuditEventAgentComponent setLocationTarget(Location value) { 1055 this.locationTarget = value; 1056 return this; 1057 } 1058 1059 /** 1060 * @return {@link #policy} (The policy or plan that authorized the activity 1061 * being recorded. Typically, a single activity may have multiple 1062 * applicable policies, such as patient consent, guarantor funding, etc. 1063 * The policy would also indicate the security token used.) 1064 */ 1065 public List<UriType> getPolicy() { 1066 if (this.policy == null) 1067 this.policy = new ArrayList<UriType>(); 1068 return this.policy; 1069 } 1070 1071 /** 1072 * @return Returns a reference to <code>this</code> for easy method chaining 1073 */ 1074 public AuditEventAgentComponent setPolicy(List<UriType> thePolicy) { 1075 this.policy = thePolicy; 1076 return this; 1077 } 1078 1079 public boolean hasPolicy() { 1080 if (this.policy == null) 1081 return false; 1082 for (UriType item : this.policy) 1083 if (!item.isEmpty()) 1084 return true; 1085 return false; 1086 } 1087 1088 /** 1089 * @return {@link #policy} (The policy or plan that authorized the activity 1090 * being recorded. Typically, a single activity may have multiple 1091 * applicable policies, such as patient consent, guarantor funding, etc. 1092 * The policy would also indicate the security token used.) 1093 */ 1094 public UriType addPolicyElement() {// 2 1095 UriType t = new UriType(); 1096 if (this.policy == null) 1097 this.policy = new ArrayList<UriType>(); 1098 this.policy.add(t); 1099 return t; 1100 } 1101 1102 /** 1103 * @param value {@link #policy} (The policy or plan that authorized the activity 1104 * being recorded. Typically, a single activity may have multiple 1105 * applicable policies, such as patient consent, guarantor funding, 1106 * etc. The policy would also indicate the security token used.) 1107 */ 1108 public AuditEventAgentComponent addPolicy(String value) { // 1 1109 UriType t = new UriType(); 1110 t.setValue(value); 1111 if (this.policy == null) 1112 this.policy = new ArrayList<UriType>(); 1113 this.policy.add(t); 1114 return this; 1115 } 1116 1117 /** 1118 * @param value {@link #policy} (The policy or plan that authorized the activity 1119 * being recorded. Typically, a single activity may have multiple 1120 * applicable policies, such as patient consent, guarantor funding, 1121 * etc. The policy would also indicate the security token used.) 1122 */ 1123 public boolean hasPolicy(String value) { 1124 if (this.policy == null) 1125 return false; 1126 for (UriType v : this.policy) 1127 if (v.getValue().equals(value)) // uri 1128 return true; 1129 return false; 1130 } 1131 1132 /** 1133 * @return {@link #media} (Type of media involved. Used when the event is about 1134 * exporting/importing onto media.) 1135 */ 1136 public Coding getMedia() { 1137 if (this.media == null) 1138 if (Configuration.errorOnAutoCreate()) 1139 throw new Error("Attempt to auto-create AuditEventAgentComponent.media"); 1140 else if (Configuration.doAutoCreate()) 1141 this.media = new Coding(); // cc 1142 return this.media; 1143 } 1144 1145 public boolean hasMedia() { 1146 return this.media != null && !this.media.isEmpty(); 1147 } 1148 1149 /** 1150 * @param value {@link #media} (Type of media involved. Used when the event is 1151 * about exporting/importing onto media.) 1152 */ 1153 public AuditEventAgentComponent setMedia(Coding value) { 1154 this.media = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return {@link #network} (Logical network location for application activity, 1160 * if the activity has a network location.) 1161 */ 1162 public AuditEventAgentNetworkComponent getNetwork() { 1163 if (this.network == null) 1164 if (Configuration.errorOnAutoCreate()) 1165 throw new Error("Attempt to auto-create AuditEventAgentComponent.network"); 1166 else if (Configuration.doAutoCreate()) 1167 this.network = new AuditEventAgentNetworkComponent(); // cc 1168 return this.network; 1169 } 1170 1171 public boolean hasNetwork() { 1172 return this.network != null && !this.network.isEmpty(); 1173 } 1174 1175 /** 1176 * @param value {@link #network} (Logical network location for application 1177 * activity, if the activity has a network location.) 1178 */ 1179 public AuditEventAgentComponent setNetwork(AuditEventAgentNetworkComponent value) { 1180 this.network = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #purposeOfUse} (The reason (purpose of use), specific to this 1186 * agent, that was used during the event being recorded.) 1187 */ 1188 public List<CodeableConcept> getPurposeOfUse() { 1189 if (this.purposeOfUse == null) 1190 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1191 return this.purposeOfUse; 1192 } 1193 1194 /** 1195 * @return Returns a reference to <code>this</code> for easy method chaining 1196 */ 1197 public AuditEventAgentComponent setPurposeOfUse(List<CodeableConcept> thePurposeOfUse) { 1198 this.purposeOfUse = thePurposeOfUse; 1199 return this; 1200 } 1201 1202 public boolean hasPurposeOfUse() { 1203 if (this.purposeOfUse == null) 1204 return false; 1205 for (CodeableConcept item : this.purposeOfUse) 1206 if (!item.isEmpty()) 1207 return true; 1208 return false; 1209 } 1210 1211 public CodeableConcept addPurposeOfUse() { // 3 1212 CodeableConcept t = new CodeableConcept(); 1213 if (this.purposeOfUse == null) 1214 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1215 this.purposeOfUse.add(t); 1216 return t; 1217 } 1218 1219 public AuditEventAgentComponent addPurposeOfUse(CodeableConcept t) { // 3 1220 if (t == null) 1221 return this; 1222 if (this.purposeOfUse == null) 1223 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1224 this.purposeOfUse.add(t); 1225 return this; 1226 } 1227 1228 /** 1229 * @return The first repetition of repeating field {@link #purposeOfUse}, 1230 * creating it if it does not already exist 1231 */ 1232 public CodeableConcept getPurposeOfUseFirstRep() { 1233 if (getPurposeOfUse().isEmpty()) { 1234 addPurposeOfUse(); 1235 } 1236 return getPurposeOfUse().get(0); 1237 } 1238 1239 protected void listChildren(List<Property> children) { 1240 super.listChildren(children); 1241 children.add(new Property("type", "CodeableConcept", 1242 "Specification of the participation type the user plays when performing the event.", 0, 1, type)); 1243 children.add(new Property("role", "CodeableConcept", 1244 "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.", 1245 0, java.lang.Integer.MAX_VALUE, role)); 1246 children 1247 .add(new Property("who", "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 1248 "Reference to who this agent is that was involved in the event.", 0, 1, who)); 1249 children.add(new Property("altId", "string", 1250 "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 1251 0, 1, altId)); 1252 children.add(new Property("name", "string", "Human-meaningful name for the agent.", 0, 1, name)); 1253 children.add(new Property("requestor", "boolean", 1254 "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, 1255 requestor)); 1256 children.add(new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1, location)); 1257 children.add(new Property("policy", "uri", 1258 "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 1259 0, java.lang.Integer.MAX_VALUE, policy)); 1260 children.add(new Property("media", "Coding", 1261 "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1, media)); 1262 children.add(new Property("network", "", 1263 "Logical network location for application activity, if the activity has a network location.", 0, 1, network)); 1264 children.add(new Property("purposeOfUse", "CodeableConcept", 1265 "The reason (purpose of use), specific to this agent, that was used during the event being recorded.", 0, 1266 java.lang.Integer.MAX_VALUE, purposeOfUse)); 1267 } 1268 1269 @Override 1270 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1271 switch (_hash) { 1272 case 3575610: 1273 /* type */ return new Property("type", "CodeableConcept", 1274 "Specification of the participation type the user plays when performing the event.", 0, 1, type); 1275 case 3506294: 1276 /* role */ return new Property("role", "CodeableConcept", 1277 "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.", 1278 0, java.lang.Integer.MAX_VALUE, role); 1279 case 117694: 1280 /* who */ return new Property("who", 1281 "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 1282 "Reference to who this agent is that was involved in the event.", 0, 1, who); 1283 case 92912804: 1284 /* altId */ return new Property("altId", "string", 1285 "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 1286 0, 1, altId); 1287 case 3373707: 1288 /* name */ return new Property("name", "string", "Human-meaningful name for the agent.", 0, 1, name); 1289 case 693934258: 1290 /* requestor */ return new Property("requestor", "boolean", 1291 "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, 1292 requestor); 1293 case 1901043637: 1294 /* location */ return new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1, 1295 location); 1296 case -982670030: 1297 /* policy */ return new Property("policy", "uri", 1298 "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 1299 0, java.lang.Integer.MAX_VALUE, policy); 1300 case 103772132: 1301 /* media */ return new Property("media", "Coding", 1302 "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1, media); 1303 case 1843485230: 1304 /* network */ return new Property("network", "", 1305 "Logical network location for application activity, if the activity has a network location.", 0, 1, 1306 network); 1307 case -1881902670: 1308 /* purposeOfUse */ return new Property("purposeOfUse", "CodeableConcept", 1309 "The reason (purpose of use), specific to this agent, that was used during the event being recorded.", 0, 1310 java.lang.Integer.MAX_VALUE, purposeOfUse); 1311 default: 1312 return super.getNamedProperty(_hash, _name, _checkValid); 1313 } 1314 1315 } 1316 1317 @Override 1318 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1319 switch (hash) { 1320 case 3575610: 1321 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1322 case 3506294: 1323 /* role */ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 1324 case 117694: 1325 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 1326 case 92912804: 1327 /* altId */ return this.altId == null ? new Base[0] : new Base[] { this.altId }; // StringType 1328 case 3373707: 1329 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1330 case 693934258: 1331 /* requestor */ return this.requestor == null ? new Base[0] : new Base[] { this.requestor }; // BooleanType 1332 case 1901043637: 1333 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 1334 case -982670030: 1335 /* policy */ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1336 case 103772132: 1337 /* media */ return this.media == null ? new Base[0] : new Base[] { this.media }; // Coding 1338 case 1843485230: 1339 /* network */ return this.network == null ? new Base[0] : new Base[] { this.network }; // AuditEventAgentNetworkComponent 1340 case -1881902670: 1341 /* purposeOfUse */ return this.purposeOfUse == null ? new Base[0] 1342 : this.purposeOfUse.toArray(new Base[this.purposeOfUse.size()]); // CodeableConcept 1343 default: 1344 return super.getProperty(hash, name, checkValid); 1345 } 1346 1347 } 1348 1349 @Override 1350 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1351 switch (hash) { 1352 case 3575610: // type 1353 this.type = castToCodeableConcept(value); // CodeableConcept 1354 return value; 1355 case 3506294: // role 1356 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 1357 return value; 1358 case 117694: // who 1359 this.who = castToReference(value); // Reference 1360 return value; 1361 case 92912804: // altId 1362 this.altId = castToString(value); // StringType 1363 return value; 1364 case 3373707: // name 1365 this.name = castToString(value); // StringType 1366 return value; 1367 case 693934258: // requestor 1368 this.requestor = castToBoolean(value); // BooleanType 1369 return value; 1370 case 1901043637: // location 1371 this.location = castToReference(value); // Reference 1372 return value; 1373 case -982670030: // policy 1374 this.getPolicy().add(castToUri(value)); // UriType 1375 return value; 1376 case 103772132: // media 1377 this.media = castToCoding(value); // Coding 1378 return value; 1379 case 1843485230: // network 1380 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1381 return value; 1382 case -1881902670: // purposeOfUse 1383 this.getPurposeOfUse().add(castToCodeableConcept(value)); // CodeableConcept 1384 return value; 1385 default: 1386 return super.setProperty(hash, name, value); 1387 } 1388 1389 } 1390 1391 @Override 1392 public Base setProperty(String name, Base value) throws FHIRException { 1393 if (name.equals("type")) { 1394 this.type = castToCodeableConcept(value); // CodeableConcept 1395 } else if (name.equals("role")) { 1396 this.getRole().add(castToCodeableConcept(value)); 1397 } else if (name.equals("who")) { 1398 this.who = castToReference(value); // Reference 1399 } else if (name.equals("altId")) { 1400 this.altId = castToString(value); // StringType 1401 } else if (name.equals("name")) { 1402 this.name = castToString(value); // StringType 1403 } else if (name.equals("requestor")) { 1404 this.requestor = castToBoolean(value); // BooleanType 1405 } else if (name.equals("location")) { 1406 this.location = castToReference(value); // Reference 1407 } else if (name.equals("policy")) { 1408 this.getPolicy().add(castToUri(value)); 1409 } else if (name.equals("media")) { 1410 this.media = castToCoding(value); // Coding 1411 } else if (name.equals("network")) { 1412 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1413 } else if (name.equals("purposeOfUse")) { 1414 this.getPurposeOfUse().add(castToCodeableConcept(value)); 1415 } else 1416 return super.setProperty(name, value); 1417 return value; 1418 } 1419 1420 @Override 1421 public void removeChild(String name, Base value) throws FHIRException { 1422 if (name.equals("type")) { 1423 this.type = null; 1424 } else if (name.equals("role")) { 1425 this.getRole().remove(castToCodeableConcept(value)); 1426 } else if (name.equals("who")) { 1427 this.who = null; 1428 } else if (name.equals("altId")) { 1429 this.altId = null; 1430 } else if (name.equals("name")) { 1431 this.name = null; 1432 } else if (name.equals("requestor")) { 1433 this.requestor = null; 1434 } else if (name.equals("location")) { 1435 this.location = null; 1436 } else if (name.equals("policy")) { 1437 this.getPolicy().remove(castToUri(value)); 1438 } else if (name.equals("media")) { 1439 this.media = null; 1440 } else if (name.equals("network")) { 1441 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1442 } else if (name.equals("purposeOfUse")) { 1443 this.getPurposeOfUse().remove(castToCodeableConcept(value)); 1444 } else 1445 super.removeChild(name, value); 1446 1447 } 1448 1449 @Override 1450 public Base makeProperty(int hash, String name) throws FHIRException { 1451 switch (hash) { 1452 case 3575610: 1453 return getType(); 1454 case 3506294: 1455 return addRole(); 1456 case 117694: 1457 return getWho(); 1458 case 92912804: 1459 return getAltIdElement(); 1460 case 3373707: 1461 return getNameElement(); 1462 case 693934258: 1463 return getRequestorElement(); 1464 case 1901043637: 1465 return getLocation(); 1466 case -982670030: 1467 return addPolicyElement(); 1468 case 103772132: 1469 return getMedia(); 1470 case 1843485230: 1471 return getNetwork(); 1472 case -1881902670: 1473 return addPurposeOfUse(); 1474 default: 1475 return super.makeProperty(hash, name); 1476 } 1477 1478 } 1479 1480 @Override 1481 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case 3575610: 1484 /* type */ return new String[] { "CodeableConcept" }; 1485 case 3506294: 1486 /* role */ return new String[] { "CodeableConcept" }; 1487 case 117694: 1488 /* who */ return new String[] { "Reference" }; 1489 case 92912804: 1490 /* altId */ return new String[] { "string" }; 1491 case 3373707: 1492 /* name */ return new String[] { "string" }; 1493 case 693934258: 1494 /* requestor */ return new String[] { "boolean" }; 1495 case 1901043637: 1496 /* location */ return new String[] { "Reference" }; 1497 case -982670030: 1498 /* policy */ return new String[] { "uri" }; 1499 case 103772132: 1500 /* media */ return new String[] { "Coding" }; 1501 case 1843485230: 1502 /* network */ return new String[] {}; 1503 case -1881902670: 1504 /* purposeOfUse */ return new String[] { "CodeableConcept" }; 1505 default: 1506 return super.getTypesForProperty(hash, name); 1507 } 1508 1509 } 1510 1511 @Override 1512 public Base addChild(String name) throws FHIRException { 1513 if (name.equals("type")) { 1514 this.type = new CodeableConcept(); 1515 return this.type; 1516 } else if (name.equals("role")) { 1517 return addRole(); 1518 } else if (name.equals("who")) { 1519 this.who = new Reference(); 1520 return this.who; 1521 } else if (name.equals("altId")) { 1522 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.altId"); 1523 } else if (name.equals("name")) { 1524 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 1525 } else if (name.equals("requestor")) { 1526 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.requestor"); 1527 } else if (name.equals("location")) { 1528 this.location = new Reference(); 1529 return this.location; 1530 } else if (name.equals("policy")) { 1531 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.policy"); 1532 } else if (name.equals("media")) { 1533 this.media = new Coding(); 1534 return this.media; 1535 } else if (name.equals("network")) { 1536 this.network = new AuditEventAgentNetworkComponent(); 1537 return this.network; 1538 } else if (name.equals("purposeOfUse")) { 1539 return addPurposeOfUse(); 1540 } else 1541 return super.addChild(name); 1542 } 1543 1544 public AuditEventAgentComponent copy() { 1545 AuditEventAgentComponent dst = new AuditEventAgentComponent(); 1546 copyValues(dst); 1547 return dst; 1548 } 1549 1550 public void copyValues(AuditEventAgentComponent dst) { 1551 super.copyValues(dst); 1552 dst.type = type == null ? null : type.copy(); 1553 if (role != null) { 1554 dst.role = new ArrayList<CodeableConcept>(); 1555 for (CodeableConcept i : role) 1556 dst.role.add(i.copy()); 1557 } 1558 ; 1559 dst.who = who == null ? null : who.copy(); 1560 dst.altId = altId == null ? null : altId.copy(); 1561 dst.name = name == null ? null : name.copy(); 1562 dst.requestor = requestor == null ? null : requestor.copy(); 1563 dst.location = location == null ? null : location.copy(); 1564 if (policy != null) { 1565 dst.policy = new ArrayList<UriType>(); 1566 for (UriType i : policy) 1567 dst.policy.add(i.copy()); 1568 } 1569 ; 1570 dst.media = media == null ? null : media.copy(); 1571 dst.network = network == null ? null : network.copy(); 1572 if (purposeOfUse != null) { 1573 dst.purposeOfUse = new ArrayList<CodeableConcept>(); 1574 for (CodeableConcept i : purposeOfUse) 1575 dst.purposeOfUse.add(i.copy()); 1576 } 1577 ; 1578 } 1579 1580 @Override 1581 public boolean equalsDeep(Base other_) { 1582 if (!super.equalsDeep(other_)) 1583 return false; 1584 if (!(other_ instanceof AuditEventAgentComponent)) 1585 return false; 1586 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1587 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true) && compareDeep(who, o.who, true) 1588 && compareDeep(altId, o.altId, true) && compareDeep(name, o.name, true) 1589 && compareDeep(requestor, o.requestor, true) && compareDeep(location, o.location, true) 1590 && compareDeep(policy, o.policy, true) && compareDeep(media, o.media, true) 1591 && compareDeep(network, o.network, true) && compareDeep(purposeOfUse, o.purposeOfUse, true); 1592 } 1593 1594 @Override 1595 public boolean equalsShallow(Base other_) { 1596 if (!super.equalsShallow(other_)) 1597 return false; 1598 if (!(other_ instanceof AuditEventAgentComponent)) 1599 return false; 1600 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1601 return compareValues(altId, o.altId, true) && compareValues(name, o.name, true) 1602 && compareValues(requestor, o.requestor, true) && compareValues(policy, o.policy, true); 1603 } 1604 1605 public boolean isEmpty() { 1606 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role, who, altId, name, requestor, location, 1607 policy, media, network, purposeOfUse); 1608 } 1609 1610 public String fhirType() { 1611 return "AuditEvent.agent"; 1612 1613 } 1614 1615 } 1616 1617 @Block() 1618 public static class AuditEventAgentNetworkComponent extends BackboneElement implements IBaseBackboneElement { 1619 /** 1620 * An identifier for the network access point of the user device for the audit 1621 * event. 1622 */ 1623 @Child(name = "address", type = { 1624 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1625 @Description(shortDefinition = "Identifier for the network access point of the user device", formalDefinition = "An identifier for the network access point of the user device for the audit event.") 1626 protected StringType address; 1627 1628 /** 1629 * An identifier for the type of network access point that originated the audit 1630 * event. 1631 */ 1632 @Child(name = "type", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1633 @Description(shortDefinition = "The type of network access point", formalDefinition = "An identifier for the type of network access point that originated the audit event.") 1634 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/network-type") 1635 protected Enumeration<AuditEventAgentNetworkType> type; 1636 1637 private static final long serialVersionUID = -160715924L; 1638 1639 /** 1640 * Constructor 1641 */ 1642 public AuditEventAgentNetworkComponent() { 1643 super(); 1644 } 1645 1646 /** 1647 * @return {@link #address} (An identifier for the network access point of the 1648 * user device for the audit event.). This is the underlying object with 1649 * id, value and extensions. The accessor "getAddress" gives direct 1650 * access to the value 1651 */ 1652 public StringType getAddressElement() { 1653 if (this.address == null) 1654 if (Configuration.errorOnAutoCreate()) 1655 throw new Error("Attempt to auto-create AuditEventAgentNetworkComponent.address"); 1656 else if (Configuration.doAutoCreate()) 1657 this.address = new StringType(); // bb 1658 return this.address; 1659 } 1660 1661 public boolean hasAddressElement() { 1662 return this.address != null && !this.address.isEmpty(); 1663 } 1664 1665 public boolean hasAddress() { 1666 return this.address != null && !this.address.isEmpty(); 1667 } 1668 1669 /** 1670 * @param value {@link #address} (An identifier for the network access point of 1671 * the user device for the audit event.). This is the underlying 1672 * object with id, value and extensions. The accessor "getAddress" 1673 * gives direct access to the value 1674 */ 1675 public AuditEventAgentNetworkComponent setAddressElement(StringType value) { 1676 this.address = value; 1677 return this; 1678 } 1679 1680 /** 1681 * @return An identifier for the network access point of the user device for the 1682 * audit event. 1683 */ 1684 public String getAddress() { 1685 return this.address == null ? null : this.address.getValue(); 1686 } 1687 1688 /** 1689 * @param value An identifier for the network access point of the user device 1690 * for the audit event. 1691 */ 1692 public AuditEventAgentNetworkComponent setAddress(String value) { 1693 if (Utilities.noString(value)) 1694 this.address = null; 1695 else { 1696 if (this.address == null) 1697 this.address = new StringType(); 1698 this.address.setValue(value); 1699 } 1700 return this; 1701 } 1702 1703 /** 1704 * @return {@link #type} (An identifier for the type of network access point 1705 * that originated the audit event.). This is the underlying object with 1706 * id, value and extensions. The accessor "getType" gives direct access 1707 * to the value 1708 */ 1709 public Enumeration<AuditEventAgentNetworkType> getTypeElement() { 1710 if (this.type == null) 1711 if (Configuration.errorOnAutoCreate()) 1712 throw new Error("Attempt to auto-create AuditEventAgentNetworkComponent.type"); 1713 else if (Configuration.doAutoCreate()) 1714 this.type = new Enumeration<AuditEventAgentNetworkType>(new AuditEventAgentNetworkTypeEnumFactory()); // bb 1715 return this.type; 1716 } 1717 1718 public boolean hasTypeElement() { 1719 return this.type != null && !this.type.isEmpty(); 1720 } 1721 1722 public boolean hasType() { 1723 return this.type != null && !this.type.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #type} (An identifier for the type of network access 1728 * point that originated the audit event.). This is the underlying 1729 * object with id, value and extensions. The accessor "getType" 1730 * gives direct access to the value 1731 */ 1732 public AuditEventAgentNetworkComponent setTypeElement(Enumeration<AuditEventAgentNetworkType> value) { 1733 this.type = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return An identifier for the type of network access point that originated 1739 * the audit event. 1740 */ 1741 public AuditEventAgentNetworkType getType() { 1742 return this.type == null ? null : this.type.getValue(); 1743 } 1744 1745 /** 1746 * @param value An identifier for the type of network access point that 1747 * originated the audit event. 1748 */ 1749 public AuditEventAgentNetworkComponent setType(AuditEventAgentNetworkType value) { 1750 if (value == null) 1751 this.type = null; 1752 else { 1753 if (this.type == null) 1754 this.type = new Enumeration<AuditEventAgentNetworkType>(new AuditEventAgentNetworkTypeEnumFactory()); 1755 this.type.setValue(value); 1756 } 1757 return this; 1758 } 1759 1760 protected void listChildren(List<Property> children) { 1761 super.listChildren(children); 1762 children.add(new Property("address", "string", 1763 "An identifier for the network access point of the user device for the audit event.", 0, 1, address)); 1764 children.add(new Property("type", "code", 1765 "An identifier for the type of network access point that originated the audit event.", 0, 1, type)); 1766 } 1767 1768 @Override 1769 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1770 switch (_hash) { 1771 case -1147692044: 1772 /* address */ return new Property("address", "string", 1773 "An identifier for the network access point of the user device for the audit event.", 0, 1, address); 1774 case 3575610: 1775 /* type */ return new Property("type", "code", 1776 "An identifier for the type of network access point that originated the audit event.", 0, 1, type); 1777 default: 1778 return super.getNamedProperty(_hash, _name, _checkValid); 1779 } 1780 1781 } 1782 1783 @Override 1784 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1785 switch (hash) { 1786 case -1147692044: 1787 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // StringType 1788 case 3575610: 1789 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<AuditEventAgentNetworkType> 1790 default: 1791 return super.getProperty(hash, name, checkValid); 1792 } 1793 1794 } 1795 1796 @Override 1797 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1798 switch (hash) { 1799 case -1147692044: // address 1800 this.address = castToString(value); // StringType 1801 return value; 1802 case 3575610: // type 1803 value = new AuditEventAgentNetworkTypeEnumFactory().fromType(castToCode(value)); 1804 this.type = (Enumeration) value; // Enumeration<AuditEventAgentNetworkType> 1805 return value; 1806 default: 1807 return super.setProperty(hash, name, value); 1808 } 1809 1810 } 1811 1812 @Override 1813 public Base setProperty(String name, Base value) throws FHIRException { 1814 if (name.equals("address")) { 1815 this.address = castToString(value); // StringType 1816 } else if (name.equals("type")) { 1817 value = new AuditEventAgentNetworkTypeEnumFactory().fromType(castToCode(value)); 1818 this.type = (Enumeration) value; // Enumeration<AuditEventAgentNetworkType> 1819 } else 1820 return super.setProperty(name, value); 1821 return value; 1822 } 1823 1824 @Override 1825 public void removeChild(String name, Base value) throws FHIRException { 1826 if (name.equals("address")) { 1827 this.address = null; 1828 } else if (name.equals("type")) { 1829 this.type = null; 1830 } else 1831 super.removeChild(name, value); 1832 1833 } 1834 1835 @Override 1836 public Base makeProperty(int hash, String name) throws FHIRException { 1837 switch (hash) { 1838 case -1147692044: 1839 return getAddressElement(); 1840 case 3575610: 1841 return getTypeElement(); 1842 default: 1843 return super.makeProperty(hash, name); 1844 } 1845 1846 } 1847 1848 @Override 1849 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1850 switch (hash) { 1851 case -1147692044: 1852 /* address */ return new String[] { "string" }; 1853 case 3575610: 1854 /* type */ return new String[] { "code" }; 1855 default: 1856 return super.getTypesForProperty(hash, name); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base addChild(String name) throws FHIRException { 1863 if (name.equals("address")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.address"); 1865 } else if (name.equals("type")) { 1866 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 1867 } else 1868 return super.addChild(name); 1869 } 1870 1871 public AuditEventAgentNetworkComponent copy() { 1872 AuditEventAgentNetworkComponent dst = new AuditEventAgentNetworkComponent(); 1873 copyValues(dst); 1874 return dst; 1875 } 1876 1877 public void copyValues(AuditEventAgentNetworkComponent dst) { 1878 super.copyValues(dst); 1879 dst.address = address == null ? null : address.copy(); 1880 dst.type = type == null ? null : type.copy(); 1881 } 1882 1883 @Override 1884 public boolean equalsDeep(Base other_) { 1885 if (!super.equalsDeep(other_)) 1886 return false; 1887 if (!(other_ instanceof AuditEventAgentNetworkComponent)) 1888 return false; 1889 AuditEventAgentNetworkComponent o = (AuditEventAgentNetworkComponent) other_; 1890 return compareDeep(address, o.address, true) && compareDeep(type, o.type, true); 1891 } 1892 1893 @Override 1894 public boolean equalsShallow(Base other_) { 1895 if (!super.equalsShallow(other_)) 1896 return false; 1897 if (!(other_ instanceof AuditEventAgentNetworkComponent)) 1898 return false; 1899 AuditEventAgentNetworkComponent o = (AuditEventAgentNetworkComponent) other_; 1900 return compareValues(address, o.address, true) && compareValues(type, o.type, true); 1901 } 1902 1903 public boolean isEmpty() { 1904 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(address, type); 1905 } 1906 1907 public String fhirType() { 1908 return "AuditEvent.agent.network"; 1909 1910 } 1911 1912 } 1913 1914 @Block() 1915 public static class AuditEventSourceComponent extends BackboneElement implements IBaseBackboneElement { 1916 /** 1917 * Logical source location within the healthcare enterprise network. For 1918 * example, a hospital or other provider location within a multi-entity provider 1919 * group. 1920 */ 1921 @Child(name = "site", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1922 @Description(shortDefinition = "Logical source location within the enterprise", formalDefinition = "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.") 1923 protected StringType site; 1924 1925 /** 1926 * Identifier of the source where the event was detected. 1927 */ 1928 @Child(name = "observer", type = { PractitionerRole.class, Practitioner.class, Organization.class, Device.class, 1929 Patient.class, RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1930 @Description(shortDefinition = "The identity of source detecting the event", formalDefinition = "Identifier of the source where the event was detected.") 1931 protected Reference observer; 1932 1933 /** 1934 * The actual object that is the target of the reference (Identifier of the 1935 * source where the event was detected.) 1936 */ 1937 protected Resource observerTarget; 1938 1939 /** 1940 * Code specifying the type of source where event originated. 1941 */ 1942 @Child(name = "type", type = { 1943 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1944 @Description(shortDefinition = "The type of source where event originated", formalDefinition = "Code specifying the type of source where event originated.") 1945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-source-type") 1946 protected List<Coding> type; 1947 1948 private static final long serialVersionUID = 2133038564L; 1949 1950 /** 1951 * Constructor 1952 */ 1953 public AuditEventSourceComponent() { 1954 super(); 1955 } 1956 1957 /** 1958 * Constructor 1959 */ 1960 public AuditEventSourceComponent(Reference observer) { 1961 super(); 1962 this.observer = observer; 1963 } 1964 1965 /** 1966 * @return {@link #site} (Logical source location within the healthcare 1967 * enterprise network. For example, a hospital or other provider 1968 * location within a multi-entity provider group.). This is the 1969 * underlying object with id, value and extensions. The accessor 1970 * "getSite" gives direct access to the value 1971 */ 1972 public StringType getSiteElement() { 1973 if (this.site == null) 1974 if (Configuration.errorOnAutoCreate()) 1975 throw new Error("Attempt to auto-create AuditEventSourceComponent.site"); 1976 else if (Configuration.doAutoCreate()) 1977 this.site = new StringType(); // bb 1978 return this.site; 1979 } 1980 1981 public boolean hasSiteElement() { 1982 return this.site != null && !this.site.isEmpty(); 1983 } 1984 1985 public boolean hasSite() { 1986 return this.site != null && !this.site.isEmpty(); 1987 } 1988 1989 /** 1990 * @param value {@link #site} (Logical source location within the healthcare 1991 * enterprise network. For example, a hospital or other provider 1992 * location within a multi-entity provider group.). This is the 1993 * underlying object with id, value and extensions. The accessor 1994 * "getSite" gives direct access to the value 1995 */ 1996 public AuditEventSourceComponent setSiteElement(StringType value) { 1997 this.site = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return Logical source location within the healthcare enterprise network. For 2003 * example, a hospital or other provider location within a multi-entity 2004 * provider group. 2005 */ 2006 public String getSite() { 2007 return this.site == null ? null : this.site.getValue(); 2008 } 2009 2010 /** 2011 * @param value Logical source location within the healthcare enterprise 2012 * network. For example, a hospital or other provider location 2013 * within a multi-entity provider group. 2014 */ 2015 public AuditEventSourceComponent setSite(String value) { 2016 if (Utilities.noString(value)) 2017 this.site = null; 2018 else { 2019 if (this.site == null) 2020 this.site = new StringType(); 2021 this.site.setValue(value); 2022 } 2023 return this; 2024 } 2025 2026 /** 2027 * @return {@link #observer} (Identifier of the source where the event was 2028 * detected.) 2029 */ 2030 public Reference getObserver() { 2031 if (this.observer == null) 2032 if (Configuration.errorOnAutoCreate()) 2033 throw new Error("Attempt to auto-create AuditEventSourceComponent.observer"); 2034 else if (Configuration.doAutoCreate()) 2035 this.observer = new Reference(); // cc 2036 return this.observer; 2037 } 2038 2039 public boolean hasObserver() { 2040 return this.observer != null && !this.observer.isEmpty(); 2041 } 2042 2043 /** 2044 * @param value {@link #observer} (Identifier of the source where the event was 2045 * detected.) 2046 */ 2047 public AuditEventSourceComponent setObserver(Reference value) { 2048 this.observer = value; 2049 return this; 2050 } 2051 2052 /** 2053 * @return {@link #observer} The actual object that is the target of the 2054 * reference. The reference library doesn't populate this, but you can 2055 * use it to hold the resource if you resolve it. (Identifier of the 2056 * source where the event was detected.) 2057 */ 2058 public Resource getObserverTarget() { 2059 return this.observerTarget; 2060 } 2061 2062 /** 2063 * @param value {@link #observer} The actual object that is the target of the 2064 * reference. The reference library doesn't use these, but you can 2065 * use it to hold the resource if you resolve it. (Identifier of 2066 * the source where the event was detected.) 2067 */ 2068 public AuditEventSourceComponent setObserverTarget(Resource value) { 2069 this.observerTarget = value; 2070 return this; 2071 } 2072 2073 /** 2074 * @return {@link #type} (Code specifying the type of source where event 2075 * originated.) 2076 */ 2077 public List<Coding> getType() { 2078 if (this.type == null) 2079 this.type = new ArrayList<Coding>(); 2080 return this.type; 2081 } 2082 2083 /** 2084 * @return Returns a reference to <code>this</code> for easy method chaining 2085 */ 2086 public AuditEventSourceComponent setType(List<Coding> theType) { 2087 this.type = theType; 2088 return this; 2089 } 2090 2091 public boolean hasType() { 2092 if (this.type == null) 2093 return false; 2094 for (Coding item : this.type) 2095 if (!item.isEmpty()) 2096 return true; 2097 return false; 2098 } 2099 2100 public Coding addType() { // 3 2101 Coding t = new Coding(); 2102 if (this.type == null) 2103 this.type = new ArrayList<Coding>(); 2104 this.type.add(t); 2105 return t; 2106 } 2107 2108 public AuditEventSourceComponent addType(Coding t) { // 3 2109 if (t == null) 2110 return this; 2111 if (this.type == null) 2112 this.type = new ArrayList<Coding>(); 2113 this.type.add(t); 2114 return this; 2115 } 2116 2117 /** 2118 * @return The first repetition of repeating field {@link #type}, creating it if 2119 * it does not already exist 2120 */ 2121 public Coding getTypeFirstRep() { 2122 if (getType().isEmpty()) { 2123 addType(); 2124 } 2125 return getType().get(0); 2126 } 2127 2128 protected void listChildren(List<Property> children) { 2129 super.listChildren(children); 2130 children.add(new Property("site", "string", 2131 "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 2132 0, 1, site)); 2133 children.add( 2134 new Property("observer", "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 2135 "Identifier of the source where the event was detected.", 0, 1, observer)); 2136 children.add(new Property("type", "Coding", "Code specifying the type of source where event originated.", 0, 2137 java.lang.Integer.MAX_VALUE, type)); 2138 } 2139 2140 @Override 2141 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2142 switch (_hash) { 2143 case 3530567: 2144 /* site */ return new Property("site", "string", 2145 "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 2146 0, 1, site); 2147 case 348607190: 2148 /* observer */ return new Property("observer", 2149 "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 2150 "Identifier of the source where the event was detected.", 0, 1, observer); 2151 case 3575610: 2152 /* type */ return new Property("type", "Coding", "Code specifying the type of source where event originated.", 2153 0, java.lang.Integer.MAX_VALUE, type); 2154 default: 2155 return super.getNamedProperty(_hash, _name, _checkValid); 2156 } 2157 2158 } 2159 2160 @Override 2161 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2162 switch (hash) { 2163 case 3530567: 2164 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // StringType 2165 case 348607190: 2166 /* observer */ return this.observer == null ? new Base[0] : new Base[] { this.observer }; // Reference 2167 case 3575610: 2168 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // Coding 2169 default: 2170 return super.getProperty(hash, name, checkValid); 2171 } 2172 2173 } 2174 2175 @Override 2176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2177 switch (hash) { 2178 case 3530567: // site 2179 this.site = castToString(value); // StringType 2180 return value; 2181 case 348607190: // observer 2182 this.observer = castToReference(value); // Reference 2183 return value; 2184 case 3575610: // type 2185 this.getType().add(castToCoding(value)); // Coding 2186 return value; 2187 default: 2188 return super.setProperty(hash, name, value); 2189 } 2190 2191 } 2192 2193 @Override 2194 public Base setProperty(String name, Base value) throws FHIRException { 2195 if (name.equals("site")) { 2196 this.site = castToString(value); // StringType 2197 } else if (name.equals("observer")) { 2198 this.observer = castToReference(value); // Reference 2199 } else if (name.equals("type")) { 2200 this.getType().add(castToCoding(value)); 2201 } else 2202 return super.setProperty(name, value); 2203 return value; 2204 } 2205 2206 @Override 2207 public void removeChild(String name, Base value) throws FHIRException { 2208 if (name.equals("site")) { 2209 this.site = null; 2210 } else if (name.equals("observer")) { 2211 this.observer = null; 2212 } else if (name.equals("type")) { 2213 this.getType().remove(castToCoding(value)); 2214 } else 2215 super.removeChild(name, value); 2216 2217 } 2218 2219 @Override 2220 public Base makeProperty(int hash, String name) throws FHIRException { 2221 switch (hash) { 2222 case 3530567: 2223 return getSiteElement(); 2224 case 348607190: 2225 return getObserver(); 2226 case 3575610: 2227 return addType(); 2228 default: 2229 return super.makeProperty(hash, name); 2230 } 2231 2232 } 2233 2234 @Override 2235 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2236 switch (hash) { 2237 case 3530567: 2238 /* site */ return new String[] { "string" }; 2239 case 348607190: 2240 /* observer */ return new String[] { "Reference" }; 2241 case 3575610: 2242 /* type */ return new String[] { "Coding" }; 2243 default: 2244 return super.getTypesForProperty(hash, name); 2245 } 2246 2247 } 2248 2249 @Override 2250 public Base addChild(String name) throws FHIRException { 2251 if (name.equals("site")) { 2252 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.site"); 2253 } else if (name.equals("observer")) { 2254 this.observer = new Reference(); 2255 return this.observer; 2256 } else if (name.equals("type")) { 2257 return addType(); 2258 } else 2259 return super.addChild(name); 2260 } 2261 2262 public AuditEventSourceComponent copy() { 2263 AuditEventSourceComponent dst = new AuditEventSourceComponent(); 2264 copyValues(dst); 2265 return dst; 2266 } 2267 2268 public void copyValues(AuditEventSourceComponent dst) { 2269 super.copyValues(dst); 2270 dst.site = site == null ? null : site.copy(); 2271 dst.observer = observer == null ? null : observer.copy(); 2272 if (type != null) { 2273 dst.type = new ArrayList<Coding>(); 2274 for (Coding i : type) 2275 dst.type.add(i.copy()); 2276 } 2277 ; 2278 } 2279 2280 @Override 2281 public boolean equalsDeep(Base other_) { 2282 if (!super.equalsDeep(other_)) 2283 return false; 2284 if (!(other_ instanceof AuditEventSourceComponent)) 2285 return false; 2286 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 2287 return compareDeep(site, o.site, true) && compareDeep(observer, o.observer, true) 2288 && compareDeep(type, o.type, true); 2289 } 2290 2291 @Override 2292 public boolean equalsShallow(Base other_) { 2293 if (!super.equalsShallow(other_)) 2294 return false; 2295 if (!(other_ instanceof AuditEventSourceComponent)) 2296 return false; 2297 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 2298 return compareValues(site, o.site, true); 2299 } 2300 2301 public boolean isEmpty() { 2302 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, observer, type); 2303 } 2304 2305 public String fhirType() { 2306 return "AuditEvent.source"; 2307 2308 } 2309 2310 } 2311 2312 @Block() 2313 public static class AuditEventEntityComponent extends BackboneElement implements IBaseBackboneElement { 2314 /** 2315 * Identifies a specific instance of the entity. The reference should be version 2316 * specific. 2317 */ 2318 @Child(name = "what", type = { Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2319 @Description(shortDefinition = "Specific instance of resource", formalDefinition = "Identifies a specific instance of the entity. The reference should be version specific.") 2320 protected Reference what; 2321 2322 /** 2323 * The actual object that is the target of the reference (Identifies a specific 2324 * instance of the entity. The reference should be version specific.) 2325 */ 2326 protected Resource whatTarget; 2327 2328 /** 2329 * The type of the object that was involved in this audit event. 2330 */ 2331 @Child(name = "type", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2332 @Description(shortDefinition = "Type of entity involved", formalDefinition = "The type of the object that was involved in this audit event.") 2333 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-entity-type") 2334 protected Coding type; 2335 2336 /** 2337 * Code representing the role the entity played in the event being audited. 2338 */ 2339 @Child(name = "role", type = { Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2340 @Description(shortDefinition = "What role the entity played", formalDefinition = "Code representing the role the entity played in the event being audited.") 2341 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/object-role") 2342 protected Coding role; 2343 2344 /** 2345 * Identifier for the data life-cycle stage for the entity. 2346 */ 2347 @Child(name = "lifecycle", type = { Coding.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2348 @Description(shortDefinition = "Life-cycle stage for the entity", formalDefinition = "Identifier for the data life-cycle stage for the entity.") 2349 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/object-lifecycle-events") 2350 protected Coding lifecycle; 2351 2352 /** 2353 * Security labels for the identified entity. 2354 */ 2355 @Child(name = "securityLabel", type = { 2356 Coding.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2357 @Description(shortDefinition = "Security labels on the entity", formalDefinition = "Security labels for the identified entity.") 2358 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 2359 protected List<Coding> securityLabel; 2360 2361 /** 2362 * A name of the entity in the audit event. 2363 */ 2364 @Child(name = "name", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2365 @Description(shortDefinition = "Descriptor for entity", formalDefinition = "A name of the entity in the audit event.") 2366 protected StringType name; 2367 2368 /** 2369 * Text that describes the entity in more detail. 2370 */ 2371 @Child(name = "description", type = { 2372 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2373 @Description(shortDefinition = "Descriptive text", formalDefinition = "Text that describes the entity in more detail.") 2374 protected StringType description; 2375 2376 /** 2377 * The query parameters for a query-type entities. 2378 */ 2379 @Child(name = "query", type = { 2380 Base64BinaryType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2381 @Description(shortDefinition = "Query parameters", formalDefinition = "The query parameters for a query-type entities.") 2382 protected Base64BinaryType query; 2383 2384 /** 2385 * Tagged value pairs for conveying additional information about the entity. 2386 */ 2387 @Child(name = "detail", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2388 @Description(shortDefinition = "Additional Information about the entity", formalDefinition = "Tagged value pairs for conveying additional information about the entity.") 2389 protected List<AuditEventEntityDetailComponent> detail; 2390 2391 private static final long serialVersionUID = 334545686L; 2392 2393 /** 2394 * Constructor 2395 */ 2396 public AuditEventEntityComponent() { 2397 super(); 2398 } 2399 2400 /** 2401 * @return {@link #what} (Identifies a specific instance of the entity. The 2402 * reference should be version specific.) 2403 */ 2404 public Reference getWhat() { 2405 if (this.what == null) 2406 if (Configuration.errorOnAutoCreate()) 2407 throw new Error("Attempt to auto-create AuditEventEntityComponent.what"); 2408 else if (Configuration.doAutoCreate()) 2409 this.what = new Reference(); // cc 2410 return this.what; 2411 } 2412 2413 public boolean hasWhat() { 2414 return this.what != null && !this.what.isEmpty(); 2415 } 2416 2417 /** 2418 * @param value {@link #what} (Identifies a specific instance of the entity. The 2419 * reference should be version specific.) 2420 */ 2421 public AuditEventEntityComponent setWhat(Reference value) { 2422 this.what = value; 2423 return this; 2424 } 2425 2426 /** 2427 * @return {@link #what} The actual object that is the target of the reference. 2428 * The reference library doesn't populate this, but you can use it to 2429 * hold the resource if you resolve it. (Identifies a specific instance 2430 * of the entity. The reference should be version specific.) 2431 */ 2432 public Resource getWhatTarget() { 2433 return this.whatTarget; 2434 } 2435 2436 /** 2437 * @param value {@link #what} The actual object that is the target of the 2438 * reference. The reference library doesn't use these, but you can 2439 * use it to hold the resource if you resolve it. (Identifies a 2440 * specific instance of the entity. The reference should be version 2441 * specific.) 2442 */ 2443 public AuditEventEntityComponent setWhatTarget(Resource value) { 2444 this.whatTarget = value; 2445 return this; 2446 } 2447 2448 /** 2449 * @return {@link #type} (The type of the object that was involved in this audit 2450 * event.) 2451 */ 2452 public Coding getType() { 2453 if (this.type == null) 2454 if (Configuration.errorOnAutoCreate()) 2455 throw new Error("Attempt to auto-create AuditEventEntityComponent.type"); 2456 else if (Configuration.doAutoCreate()) 2457 this.type = new Coding(); // cc 2458 return this.type; 2459 } 2460 2461 public boolean hasType() { 2462 return this.type != null && !this.type.isEmpty(); 2463 } 2464 2465 /** 2466 * @param value {@link #type} (The type of the object that was involved in this 2467 * audit event.) 2468 */ 2469 public AuditEventEntityComponent setType(Coding value) { 2470 this.type = value; 2471 return this; 2472 } 2473 2474 /** 2475 * @return {@link #role} (Code representing the role the entity played in the 2476 * event being audited.) 2477 */ 2478 public Coding getRole() { 2479 if (this.role == null) 2480 if (Configuration.errorOnAutoCreate()) 2481 throw new Error("Attempt to auto-create AuditEventEntityComponent.role"); 2482 else if (Configuration.doAutoCreate()) 2483 this.role = new Coding(); // cc 2484 return this.role; 2485 } 2486 2487 public boolean hasRole() { 2488 return this.role != null && !this.role.isEmpty(); 2489 } 2490 2491 /** 2492 * @param value {@link #role} (Code representing the role the entity played in 2493 * the event being audited.) 2494 */ 2495 public AuditEventEntityComponent setRole(Coding value) { 2496 this.role = value; 2497 return this; 2498 } 2499 2500 /** 2501 * @return {@link #lifecycle} (Identifier for the data life-cycle stage for the 2502 * entity.) 2503 */ 2504 public Coding getLifecycle() { 2505 if (this.lifecycle == null) 2506 if (Configuration.errorOnAutoCreate()) 2507 throw new Error("Attempt to auto-create AuditEventEntityComponent.lifecycle"); 2508 else if (Configuration.doAutoCreate()) 2509 this.lifecycle = new Coding(); // cc 2510 return this.lifecycle; 2511 } 2512 2513 public boolean hasLifecycle() { 2514 return this.lifecycle != null && !this.lifecycle.isEmpty(); 2515 } 2516 2517 /** 2518 * @param value {@link #lifecycle} (Identifier for the data life-cycle stage for 2519 * the entity.) 2520 */ 2521 public AuditEventEntityComponent setLifecycle(Coding value) { 2522 this.lifecycle = value; 2523 return this; 2524 } 2525 2526 /** 2527 * @return {@link #securityLabel} (Security labels for the identified entity.) 2528 */ 2529 public List<Coding> getSecurityLabel() { 2530 if (this.securityLabel == null) 2531 this.securityLabel = new ArrayList<Coding>(); 2532 return this.securityLabel; 2533 } 2534 2535 /** 2536 * @return Returns a reference to <code>this</code> for easy method chaining 2537 */ 2538 public AuditEventEntityComponent setSecurityLabel(List<Coding> theSecurityLabel) { 2539 this.securityLabel = theSecurityLabel; 2540 return this; 2541 } 2542 2543 public boolean hasSecurityLabel() { 2544 if (this.securityLabel == null) 2545 return false; 2546 for (Coding item : this.securityLabel) 2547 if (!item.isEmpty()) 2548 return true; 2549 return false; 2550 } 2551 2552 public Coding addSecurityLabel() { // 3 2553 Coding t = new Coding(); 2554 if (this.securityLabel == null) 2555 this.securityLabel = new ArrayList<Coding>(); 2556 this.securityLabel.add(t); 2557 return t; 2558 } 2559 2560 public AuditEventEntityComponent addSecurityLabel(Coding t) { // 3 2561 if (t == null) 2562 return this; 2563 if (this.securityLabel == null) 2564 this.securityLabel = new ArrayList<Coding>(); 2565 this.securityLabel.add(t); 2566 return this; 2567 } 2568 2569 /** 2570 * @return The first repetition of repeating field {@link #securityLabel}, 2571 * creating it if it does not already exist 2572 */ 2573 public Coding getSecurityLabelFirstRep() { 2574 if (getSecurityLabel().isEmpty()) { 2575 addSecurityLabel(); 2576 } 2577 return getSecurityLabel().get(0); 2578 } 2579 2580 /** 2581 * @return {@link #name} (A name of the entity in the audit event.). This is the 2582 * underlying object with id, value and extensions. The accessor 2583 * "getName" gives direct access to the value 2584 */ 2585 public StringType getNameElement() { 2586 if (this.name == null) 2587 if (Configuration.errorOnAutoCreate()) 2588 throw new Error("Attempt to auto-create AuditEventEntityComponent.name"); 2589 else if (Configuration.doAutoCreate()) 2590 this.name = new StringType(); // bb 2591 return this.name; 2592 } 2593 2594 public boolean hasNameElement() { 2595 return this.name != null && !this.name.isEmpty(); 2596 } 2597 2598 public boolean hasName() { 2599 return this.name != null && !this.name.isEmpty(); 2600 } 2601 2602 /** 2603 * @param value {@link #name} (A name of the entity in the audit event.). This 2604 * is the underlying object with id, value and extensions. The 2605 * accessor "getName" gives direct access to the value 2606 */ 2607 public AuditEventEntityComponent setNameElement(StringType value) { 2608 this.name = value; 2609 return this; 2610 } 2611 2612 /** 2613 * @return A name of the entity in the audit event. 2614 */ 2615 public String getName() { 2616 return this.name == null ? null : this.name.getValue(); 2617 } 2618 2619 /** 2620 * @param value A name of the entity in the audit event. 2621 */ 2622 public AuditEventEntityComponent setName(String value) { 2623 if (Utilities.noString(value)) 2624 this.name = null; 2625 else { 2626 if (this.name == null) 2627 this.name = new StringType(); 2628 this.name.setValue(value); 2629 } 2630 return this; 2631 } 2632 2633 /** 2634 * @return {@link #description} (Text that describes the entity in more 2635 * detail.). This is the underlying object with id, value and 2636 * extensions. The accessor "getDescription" gives direct access to the 2637 * value 2638 */ 2639 public StringType getDescriptionElement() { 2640 if (this.description == null) 2641 if (Configuration.errorOnAutoCreate()) 2642 throw new Error("Attempt to auto-create AuditEventEntityComponent.description"); 2643 else if (Configuration.doAutoCreate()) 2644 this.description = new StringType(); // bb 2645 return this.description; 2646 } 2647 2648 public boolean hasDescriptionElement() { 2649 return this.description != null && !this.description.isEmpty(); 2650 } 2651 2652 public boolean hasDescription() { 2653 return this.description != null && !this.description.isEmpty(); 2654 } 2655 2656 /** 2657 * @param value {@link #description} (Text that describes the entity in more 2658 * detail.). This is the underlying object with id, value and 2659 * extensions. The accessor "getDescription" gives direct access to 2660 * the value 2661 */ 2662 public AuditEventEntityComponent setDescriptionElement(StringType value) { 2663 this.description = value; 2664 return this; 2665 } 2666 2667 /** 2668 * @return Text that describes the entity in more detail. 2669 */ 2670 public String getDescription() { 2671 return this.description == null ? null : this.description.getValue(); 2672 } 2673 2674 /** 2675 * @param value Text that describes the entity in more detail. 2676 */ 2677 public AuditEventEntityComponent setDescription(String value) { 2678 if (Utilities.noString(value)) 2679 this.description = null; 2680 else { 2681 if (this.description == null) 2682 this.description = new StringType(); 2683 this.description.setValue(value); 2684 } 2685 return this; 2686 } 2687 2688 /** 2689 * @return {@link #query} (The query parameters for a query-type entities.). 2690 * This is the underlying object with id, value and extensions. The 2691 * accessor "getQuery" gives direct access to the value 2692 */ 2693 public Base64BinaryType getQueryElement() { 2694 if (this.query == null) 2695 if (Configuration.errorOnAutoCreate()) 2696 throw new Error("Attempt to auto-create AuditEventEntityComponent.query"); 2697 else if (Configuration.doAutoCreate()) 2698 this.query = new Base64BinaryType(); // bb 2699 return this.query; 2700 } 2701 2702 public boolean hasQueryElement() { 2703 return this.query != null && !this.query.isEmpty(); 2704 } 2705 2706 public boolean hasQuery() { 2707 return this.query != null && !this.query.isEmpty(); 2708 } 2709 2710 /** 2711 * @param value {@link #query} (The query parameters for a query-type 2712 * entities.). This is the underlying object with id, value and 2713 * extensions. The accessor "getQuery" gives direct access to the 2714 * value 2715 */ 2716 public AuditEventEntityComponent setQueryElement(Base64BinaryType value) { 2717 this.query = value; 2718 return this; 2719 } 2720 2721 /** 2722 * @return The query parameters for a query-type entities. 2723 */ 2724 public byte[] getQuery() { 2725 return this.query == null ? null : this.query.getValue(); 2726 } 2727 2728 /** 2729 * @param value The query parameters for a query-type entities. 2730 */ 2731 public AuditEventEntityComponent setQuery(byte[] value) { 2732 if (value == null) 2733 this.query = null; 2734 else { 2735 if (this.query == null) 2736 this.query = new Base64BinaryType(); 2737 this.query.setValue(value); 2738 } 2739 return this; 2740 } 2741 2742 /** 2743 * @return {@link #detail} (Tagged value pairs for conveying additional 2744 * information about the entity.) 2745 */ 2746 public List<AuditEventEntityDetailComponent> getDetail() { 2747 if (this.detail == null) 2748 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2749 return this.detail; 2750 } 2751 2752 /** 2753 * @return Returns a reference to <code>this</code> for easy method chaining 2754 */ 2755 public AuditEventEntityComponent setDetail(List<AuditEventEntityDetailComponent> theDetail) { 2756 this.detail = theDetail; 2757 return this; 2758 } 2759 2760 public boolean hasDetail() { 2761 if (this.detail == null) 2762 return false; 2763 for (AuditEventEntityDetailComponent item : this.detail) 2764 if (!item.isEmpty()) 2765 return true; 2766 return false; 2767 } 2768 2769 public AuditEventEntityDetailComponent addDetail() { // 3 2770 AuditEventEntityDetailComponent t = new AuditEventEntityDetailComponent(); 2771 if (this.detail == null) 2772 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2773 this.detail.add(t); 2774 return t; 2775 } 2776 2777 public AuditEventEntityComponent addDetail(AuditEventEntityDetailComponent t) { // 3 2778 if (t == null) 2779 return this; 2780 if (this.detail == null) 2781 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2782 this.detail.add(t); 2783 return this; 2784 } 2785 2786 /** 2787 * @return The first repetition of repeating field {@link #detail}, creating it 2788 * if it does not already exist 2789 */ 2790 public AuditEventEntityDetailComponent getDetailFirstRep() { 2791 if (getDetail().isEmpty()) { 2792 addDetail(); 2793 } 2794 return getDetail().get(0); 2795 } 2796 2797 protected void listChildren(List<Property> children) { 2798 super.listChildren(children); 2799 children.add(new Property("what", "Reference(Any)", 2800 "Identifies a specific instance of the entity. The reference should be version specific.", 0, 1, what)); 2801 children.add( 2802 new Property("type", "Coding", "The type of the object that was involved in this audit event.", 0, 1, type)); 2803 children.add(new Property("role", "Coding", 2804 "Code representing the role the entity played in the event being audited.", 0, 1, role)); 2805 children.add(new Property("lifecycle", "Coding", "Identifier for the data life-cycle stage for the entity.", 0, 1, 2806 lifecycle)); 2807 children.add(new Property("securityLabel", "Coding", "Security labels for the identified entity.", 0, 2808 java.lang.Integer.MAX_VALUE, securityLabel)); 2809 children.add(new Property("name", "string", "A name of the entity in the audit event.", 0, 1, name)); 2810 children.add( 2811 new Property("description", "string", "Text that describes the entity in more detail.", 0, 1, description)); 2812 children 2813 .add(new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 1, query)); 2814 children 2815 .add(new Property("detail", "", "Tagged value pairs for conveying additional information about the entity.", 2816 0, java.lang.Integer.MAX_VALUE, detail)); 2817 } 2818 2819 @Override 2820 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2821 switch (_hash) { 2822 case 3648196: 2823 /* what */ return new Property("what", "Reference(Any)", 2824 "Identifies a specific instance of the entity. The reference should be version specific.", 0, 1, what); 2825 case 3575610: 2826 /* type */ return new Property("type", "Coding", 2827 "The type of the object that was involved in this audit event.", 0, 1, type); 2828 case 3506294: 2829 /* role */ return new Property("role", "Coding", 2830 "Code representing the role the entity played in the event being audited.", 0, 1, role); 2831 case -302323862: 2832 /* lifecycle */ return new Property("lifecycle", "Coding", 2833 "Identifier for the data life-cycle stage for the entity.", 0, 1, lifecycle); 2834 case -722296940: 2835 /* securityLabel */ return new Property("securityLabel", "Coding", "Security labels for the identified entity.", 2836 0, java.lang.Integer.MAX_VALUE, securityLabel); 2837 case 3373707: 2838 /* name */ return new Property("name", "string", "A name of the entity in the audit event.", 0, 1, name); 2839 case -1724546052: 2840 /* description */ return new Property("description", "string", "Text that describes the entity in more detail.", 2841 0, 1, description); 2842 case 107944136: 2843 /* query */ return new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 2844 1, query); 2845 case -1335224239: 2846 /* detail */ return new Property("detail", "", 2847 "Tagged value pairs for conveying additional information about the entity.", 0, java.lang.Integer.MAX_VALUE, 2848 detail); 2849 default: 2850 return super.getNamedProperty(_hash, _name, _checkValid); 2851 } 2852 2853 } 2854 2855 @Override 2856 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2857 switch (hash) { 2858 case 3648196: 2859 /* what */ return this.what == null ? new Base[0] : new Base[] { this.what }; // Reference 2860 case 3575610: 2861 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 2862 case 3506294: 2863 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // Coding 2864 case -302323862: 2865 /* lifecycle */ return this.lifecycle == null ? new Base[0] : new Base[] { this.lifecycle }; // Coding 2866 case -722296940: 2867 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2868 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 2869 case 3373707: 2870 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2871 case -1724546052: 2872 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2873 case 107944136: 2874 /* query */ return this.query == null ? new Base[0] : new Base[] { this.query }; // Base64BinaryType 2875 case -1335224239: 2876 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AuditEventEntityDetailComponent 2877 default: 2878 return super.getProperty(hash, name, checkValid); 2879 } 2880 2881 } 2882 2883 @Override 2884 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2885 switch (hash) { 2886 case 3648196: // what 2887 this.what = castToReference(value); // Reference 2888 return value; 2889 case 3575610: // type 2890 this.type = castToCoding(value); // Coding 2891 return value; 2892 case 3506294: // role 2893 this.role = castToCoding(value); // Coding 2894 return value; 2895 case -302323862: // lifecycle 2896 this.lifecycle = castToCoding(value); // Coding 2897 return value; 2898 case -722296940: // securityLabel 2899 this.getSecurityLabel().add(castToCoding(value)); // Coding 2900 return value; 2901 case 3373707: // name 2902 this.name = castToString(value); // StringType 2903 return value; 2904 case -1724546052: // description 2905 this.description = castToString(value); // StringType 2906 return value; 2907 case 107944136: // query 2908 this.query = castToBase64Binary(value); // Base64BinaryType 2909 return value; 2910 case -1335224239: // detail 2911 this.getDetail().add((AuditEventEntityDetailComponent) value); // AuditEventEntityDetailComponent 2912 return value; 2913 default: 2914 return super.setProperty(hash, name, value); 2915 } 2916 2917 } 2918 2919 @Override 2920 public Base setProperty(String name, Base value) throws FHIRException { 2921 if (name.equals("what")) { 2922 this.what = castToReference(value); // Reference 2923 } else if (name.equals("type")) { 2924 this.type = castToCoding(value); // Coding 2925 } else if (name.equals("role")) { 2926 this.role = castToCoding(value); // Coding 2927 } else if (name.equals("lifecycle")) { 2928 this.lifecycle = castToCoding(value); // Coding 2929 } else if (name.equals("securityLabel")) { 2930 this.getSecurityLabel().add(castToCoding(value)); 2931 } else if (name.equals("name")) { 2932 this.name = castToString(value); // StringType 2933 } else if (name.equals("description")) { 2934 this.description = castToString(value); // StringType 2935 } else if (name.equals("query")) { 2936 this.query = castToBase64Binary(value); // Base64BinaryType 2937 } else if (name.equals("detail")) { 2938 this.getDetail().add((AuditEventEntityDetailComponent) value); 2939 } else 2940 return super.setProperty(name, value); 2941 return value; 2942 } 2943 2944 @Override 2945 public void removeChild(String name, Base value) throws FHIRException { 2946 if (name.equals("what")) { 2947 this.what = null; 2948 } else if (name.equals("type")) { 2949 this.type = null; 2950 } else if (name.equals("role")) { 2951 this.role = null; 2952 } else if (name.equals("lifecycle")) { 2953 this.lifecycle = null; 2954 } else if (name.equals("securityLabel")) { 2955 this.getSecurityLabel().remove(castToCoding(value)); 2956 } else if (name.equals("name")) { 2957 this.name = null; 2958 } else if (name.equals("description")) { 2959 this.description = null; 2960 } else if (name.equals("query")) { 2961 this.query = null; 2962 } else if (name.equals("detail")) { 2963 this.getDetail().remove((AuditEventEntityDetailComponent) value); 2964 } else 2965 super.removeChild(name, value); 2966 2967 } 2968 2969 @Override 2970 public Base makeProperty(int hash, String name) throws FHIRException { 2971 switch (hash) { 2972 case 3648196: 2973 return getWhat(); 2974 case 3575610: 2975 return getType(); 2976 case 3506294: 2977 return getRole(); 2978 case -302323862: 2979 return getLifecycle(); 2980 case -722296940: 2981 return addSecurityLabel(); 2982 case 3373707: 2983 return getNameElement(); 2984 case -1724546052: 2985 return getDescriptionElement(); 2986 case 107944136: 2987 return getQueryElement(); 2988 case -1335224239: 2989 return addDetail(); 2990 default: 2991 return super.makeProperty(hash, name); 2992 } 2993 2994 } 2995 2996 @Override 2997 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2998 switch (hash) { 2999 case 3648196: 3000 /* what */ return new String[] { "Reference" }; 3001 case 3575610: 3002 /* type */ return new String[] { "Coding" }; 3003 case 3506294: 3004 /* role */ return new String[] { "Coding" }; 3005 case -302323862: 3006 /* lifecycle */ return new String[] { "Coding" }; 3007 case -722296940: 3008 /* securityLabel */ return new String[] { "Coding" }; 3009 case 3373707: 3010 /* name */ return new String[] { "string" }; 3011 case -1724546052: 3012 /* description */ return new String[] { "string" }; 3013 case 107944136: 3014 /* query */ return new String[] { "base64Binary" }; 3015 case -1335224239: 3016 /* detail */ return new String[] {}; 3017 default: 3018 return super.getTypesForProperty(hash, name); 3019 } 3020 3021 } 3022 3023 @Override 3024 public Base addChild(String name) throws FHIRException { 3025 if (name.equals("what")) { 3026 this.what = new Reference(); 3027 return this.what; 3028 } else if (name.equals("type")) { 3029 this.type = new Coding(); 3030 return this.type; 3031 } else if (name.equals("role")) { 3032 this.role = new Coding(); 3033 return this.role; 3034 } else if (name.equals("lifecycle")) { 3035 this.lifecycle = new Coding(); 3036 return this.lifecycle; 3037 } else if (name.equals("securityLabel")) { 3038 return addSecurityLabel(); 3039 } else if (name.equals("name")) { 3040 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 3041 } else if (name.equals("description")) { 3042 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.description"); 3043 } else if (name.equals("query")) { 3044 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.query"); 3045 } else if (name.equals("detail")) { 3046 return addDetail(); 3047 } else 3048 return super.addChild(name); 3049 } 3050 3051 public AuditEventEntityComponent copy() { 3052 AuditEventEntityComponent dst = new AuditEventEntityComponent(); 3053 copyValues(dst); 3054 return dst; 3055 } 3056 3057 public void copyValues(AuditEventEntityComponent dst) { 3058 super.copyValues(dst); 3059 dst.what = what == null ? null : what.copy(); 3060 dst.type = type == null ? null : type.copy(); 3061 dst.role = role == null ? null : role.copy(); 3062 dst.lifecycle = lifecycle == null ? null : lifecycle.copy(); 3063 if (securityLabel != null) { 3064 dst.securityLabel = new ArrayList<Coding>(); 3065 for (Coding i : securityLabel) 3066 dst.securityLabel.add(i.copy()); 3067 } 3068 ; 3069 dst.name = name == null ? null : name.copy(); 3070 dst.description = description == null ? null : description.copy(); 3071 dst.query = query == null ? null : query.copy(); 3072 if (detail != null) { 3073 dst.detail = new ArrayList<AuditEventEntityDetailComponent>(); 3074 for (AuditEventEntityDetailComponent i : detail) 3075 dst.detail.add(i.copy()); 3076 } 3077 ; 3078 } 3079 3080 @Override 3081 public boolean equalsDeep(Base other_) { 3082 if (!super.equalsDeep(other_)) 3083 return false; 3084 if (!(other_ instanceof AuditEventEntityComponent)) 3085 return false; 3086 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 3087 return compareDeep(what, o.what, true) && compareDeep(type, o.type, true) && compareDeep(role, o.role, true) 3088 && compareDeep(lifecycle, o.lifecycle, true) && compareDeep(securityLabel, o.securityLabel, true) 3089 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 3090 && compareDeep(query, o.query, true) && compareDeep(detail, o.detail, true); 3091 } 3092 3093 @Override 3094 public boolean equalsShallow(Base other_) { 3095 if (!super.equalsShallow(other_)) 3096 return false; 3097 if (!(other_ instanceof AuditEventEntityComponent)) 3098 return false; 3099 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 3100 return compareValues(name, o.name, true) && compareValues(description, o.description, true) 3101 && compareValues(query, o.query, true); 3102 } 3103 3104 public boolean isEmpty() { 3105 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(what, type, role, lifecycle, securityLabel, name, 3106 description, query, detail); 3107 } 3108 3109 public String fhirType() { 3110 return "AuditEvent.entity"; 3111 3112 } 3113 3114 } 3115 3116 @Block() 3117 public static class AuditEventEntityDetailComponent extends BackboneElement implements IBaseBackboneElement { 3118 /** 3119 * The type of extra detail provided in the value. 3120 */ 3121 @Child(name = "type", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3122 @Description(shortDefinition = "Name of the property", formalDefinition = "The type of extra detail provided in the value.") 3123 protected StringType type; 3124 3125 /** 3126 * The value of the extra detail. 3127 */ 3128 @Child(name = "value", type = { StringType.class, 3129 Base64BinaryType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3130 @Description(shortDefinition = "Property value", formalDefinition = "The value of the extra detail.") 3131 protected Type value; 3132 3133 private static final long serialVersionUID = -1035059584L; 3134 3135 /** 3136 * Constructor 3137 */ 3138 public AuditEventEntityDetailComponent() { 3139 super(); 3140 } 3141 3142 /** 3143 * Constructor 3144 */ 3145 public AuditEventEntityDetailComponent(StringType type, Type value) { 3146 super(); 3147 this.type = type; 3148 this.value = value; 3149 } 3150 3151 /** 3152 * @return {@link #type} (The type of extra detail provided in the value.). This 3153 * is the underlying object with id, value and extensions. The accessor 3154 * "getType" gives direct access to the value 3155 */ 3156 public StringType getTypeElement() { 3157 if (this.type == null) 3158 if (Configuration.errorOnAutoCreate()) 3159 throw new Error("Attempt to auto-create AuditEventEntityDetailComponent.type"); 3160 else if (Configuration.doAutoCreate()) 3161 this.type = new StringType(); // bb 3162 return this.type; 3163 } 3164 3165 public boolean hasTypeElement() { 3166 return this.type != null && !this.type.isEmpty(); 3167 } 3168 3169 public boolean hasType() { 3170 return this.type != null && !this.type.isEmpty(); 3171 } 3172 3173 /** 3174 * @param value {@link #type} (The type of extra detail provided in the value.). 3175 * This is the underlying object with id, value and extensions. The 3176 * accessor "getType" gives direct access to the value 3177 */ 3178 public AuditEventEntityDetailComponent setTypeElement(StringType value) { 3179 this.type = value; 3180 return this; 3181 } 3182 3183 /** 3184 * @return The type of extra detail provided in the value. 3185 */ 3186 public String getType() { 3187 return this.type == null ? null : this.type.getValue(); 3188 } 3189 3190 /** 3191 * @param value The type of extra detail provided in the value. 3192 */ 3193 public AuditEventEntityDetailComponent setType(String value) { 3194 if (this.type == null) 3195 this.type = new StringType(); 3196 this.type.setValue(value); 3197 return this; 3198 } 3199 3200 /** 3201 * @return {@link #value} (The value of the extra detail.) 3202 */ 3203 public Type getValue() { 3204 return this.value; 3205 } 3206 3207 /** 3208 * @return {@link #value} (The value of the extra detail.) 3209 */ 3210 public StringType getValueStringType() throws FHIRException { 3211 if (this.value == null) 3212 this.value = new StringType(); 3213 if (!(this.value instanceof StringType)) 3214 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3215 + this.value.getClass().getName() + " was encountered"); 3216 return (StringType) this.value; 3217 } 3218 3219 public boolean hasValueStringType() { 3220 return this != null && this.value instanceof StringType; 3221 } 3222 3223 /** 3224 * @return {@link #value} (The value of the extra detail.) 3225 */ 3226 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 3227 if (this.value == null) 3228 this.value = new Base64BinaryType(); 3229 if (!(this.value instanceof Base64BinaryType)) 3230 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but " 3231 + this.value.getClass().getName() + " was encountered"); 3232 return (Base64BinaryType) this.value; 3233 } 3234 3235 public boolean hasValueBase64BinaryType() { 3236 return this != null && this.value instanceof Base64BinaryType; 3237 } 3238 3239 public boolean hasValue() { 3240 return this.value != null && !this.value.isEmpty(); 3241 } 3242 3243 /** 3244 * @param value {@link #value} (The value of the extra detail.) 3245 */ 3246 public AuditEventEntityDetailComponent setValue(Type value) { 3247 if (value != null && !(value instanceof StringType || value instanceof Base64BinaryType)) 3248 throw new Error("Not the right type for AuditEvent.entity.detail.value[x]: " + value.fhirType()); 3249 this.value = value; 3250 return this; 3251 } 3252 3253 protected void listChildren(List<Property> children) { 3254 super.listChildren(children); 3255 children.add(new Property("type", "string", "The type of extra detail provided in the value.", 0, 1, type)); 3256 children.add(new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 1, value)); 3257 } 3258 3259 @Override 3260 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3261 switch (_hash) { 3262 case 3575610: 3263 /* type */ return new Property("type", "string", "The type of extra detail provided in the value.", 0, 1, type); 3264 case -1410166417: 3265 /* value[x] */ return new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 1, 3266 value); 3267 case 111972721: 3268 /* value */ return new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 1, 3269 value); 3270 case -1424603934: 3271 /* valueString */ return new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 3272 1, value); 3273 case -1535024575: 3274 /* valueBase64Binary */ return new Property("value[x]", "string|base64Binary", 3275 "The value of the extra detail.", 0, 1, value); 3276 default: 3277 return super.getNamedProperty(_hash, _name, _checkValid); 3278 } 3279 3280 } 3281 3282 @Override 3283 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3284 switch (hash) { 3285 case 3575610: 3286 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // StringType 3287 case 111972721: 3288 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3289 default: 3290 return super.getProperty(hash, name, checkValid); 3291 } 3292 3293 } 3294 3295 @Override 3296 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3297 switch (hash) { 3298 case 3575610: // type 3299 this.type = castToString(value); // StringType 3300 return value; 3301 case 111972721: // value 3302 this.value = castToType(value); // Type 3303 return value; 3304 default: 3305 return super.setProperty(hash, name, value); 3306 } 3307 3308 } 3309 3310 @Override 3311 public Base setProperty(String name, Base value) throws FHIRException { 3312 if (name.equals("type")) { 3313 this.type = castToString(value); // StringType 3314 } else if (name.equals("value[x]")) { 3315 this.value = castToType(value); // Type 3316 } else 3317 return super.setProperty(name, value); 3318 return value; 3319 } 3320 3321 @Override 3322 public void removeChild(String name, Base value) throws FHIRException { 3323 if (name.equals("type")) { 3324 this.type = null; 3325 } else if (name.equals("value[x]")) { 3326 this.value = null; 3327 } else 3328 super.removeChild(name, value); 3329 3330 } 3331 3332 @Override 3333 public Base makeProperty(int hash, String name) throws FHIRException { 3334 switch (hash) { 3335 case 3575610: 3336 return getTypeElement(); 3337 case -1410166417: 3338 return getValue(); 3339 case 111972721: 3340 return getValue(); 3341 default: 3342 return super.makeProperty(hash, name); 3343 } 3344 3345 } 3346 3347 @Override 3348 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3349 switch (hash) { 3350 case 3575610: 3351 /* type */ return new String[] { "string" }; 3352 case 111972721: 3353 /* value */ return new String[] { "string", "base64Binary" }; 3354 default: 3355 return super.getTypesForProperty(hash, name); 3356 } 3357 3358 } 3359 3360 @Override 3361 public Base addChild(String name) throws FHIRException { 3362 if (name.equals("type")) { 3363 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 3364 } else if (name.equals("valueString")) { 3365 this.value = new StringType(); 3366 return this.value; 3367 } else if (name.equals("valueBase64Binary")) { 3368 this.value = new Base64BinaryType(); 3369 return this.value; 3370 } else 3371 return super.addChild(name); 3372 } 3373 3374 public AuditEventEntityDetailComponent copy() { 3375 AuditEventEntityDetailComponent dst = new AuditEventEntityDetailComponent(); 3376 copyValues(dst); 3377 return dst; 3378 } 3379 3380 public void copyValues(AuditEventEntityDetailComponent dst) { 3381 super.copyValues(dst); 3382 dst.type = type == null ? null : type.copy(); 3383 dst.value = value == null ? null : value.copy(); 3384 } 3385 3386 @Override 3387 public boolean equalsDeep(Base other_) { 3388 if (!super.equalsDeep(other_)) 3389 return false; 3390 if (!(other_ instanceof AuditEventEntityDetailComponent)) 3391 return false; 3392 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 3393 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3394 } 3395 3396 @Override 3397 public boolean equalsShallow(Base other_) { 3398 if (!super.equalsShallow(other_)) 3399 return false; 3400 if (!(other_ instanceof AuditEventEntityDetailComponent)) 3401 return false; 3402 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 3403 return compareValues(type, o.type, true); 3404 } 3405 3406 public boolean isEmpty() { 3407 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3408 } 3409 3410 public String fhirType() { 3411 return "AuditEvent.entity.detail"; 3412 3413 } 3414 3415 } 3416 3417 /** 3418 * Identifier for a family of the event. For example, a menu item, program, 3419 * rule, policy, function code, application name or URL. It identifies the 3420 * performed function. 3421 */ 3422 @Child(name = "type", type = { Coding.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 3423 @Description(shortDefinition = "Type/identifier of event", formalDefinition = "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.") 3424 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-type") 3425 protected Coding type; 3426 3427 /** 3428 * Identifier for the category of event. 3429 */ 3430 @Child(name = "subtype", type = { 3431 Coding.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3432 @Description(shortDefinition = "More specific type/id for the event", formalDefinition = "Identifier for the category of event.") 3433 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-sub-type") 3434 protected List<Coding> subtype; 3435 3436 /** 3437 * Indicator for type of action performed during the event that generated the 3438 * audit. 3439 */ 3440 @Child(name = "action", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3441 @Description(shortDefinition = "Type of action performed during the event", formalDefinition = "Indicator for type of action performed during the event that generated the audit.") 3442 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-action") 3443 protected Enumeration<AuditEventAction> action; 3444 3445 /** 3446 * The period during which the activity occurred. 3447 */ 3448 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3449 @Description(shortDefinition = "When the activity occurred", formalDefinition = "The period during which the activity occurred.") 3450 protected Period period; 3451 3452 /** 3453 * The time when the event was recorded. 3454 */ 3455 @Child(name = "recorded", type = { InstantType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3456 @Description(shortDefinition = "Time when the event was recorded", formalDefinition = "The time when the event was recorded.") 3457 protected InstantType recorded; 3458 3459 /** 3460 * Indicates whether the event succeeded or failed. 3461 */ 3462 @Child(name = "outcome", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3463 @Description(shortDefinition = "Whether the event succeeded or failed", formalDefinition = "Indicates whether the event succeeded or failed.") 3464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-outcome") 3465 protected Enumeration<AuditEventOutcome> outcome; 3466 3467 /** 3468 * A free text description of the outcome of the event. 3469 */ 3470 @Child(name = "outcomeDesc", type = { 3471 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3472 @Description(shortDefinition = "Description of the event outcome", formalDefinition = "A free text description of the outcome of the event.") 3473 protected StringType outcomeDesc; 3474 3475 /** 3476 * The purposeOfUse (reason) that was used during the event being recorded. 3477 */ 3478 @Child(name = "purposeOfEvent", type = { 3479 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3480 @Description(shortDefinition = "The purposeOfUse of the event", formalDefinition = "The purposeOfUse (reason) that was used during the event being recorded.") 3481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 3482 protected List<CodeableConcept> purposeOfEvent; 3483 3484 /** 3485 * An actor taking an active role in the event or activity that is logged. 3486 */ 3487 @Child(name = "agent", type = {}, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3488 @Description(shortDefinition = "Actor involved in the event", formalDefinition = "An actor taking an active role in the event or activity that is logged.") 3489 protected List<AuditEventAgentComponent> agent; 3490 3491 /** 3492 * The system that is reporting the event. 3493 */ 3494 @Child(name = "source", type = {}, order = 9, min = 1, max = 1, modifier = false, summary = false) 3495 @Description(shortDefinition = "Audit Event Reporter", formalDefinition = "The system that is reporting the event.") 3496 protected AuditEventSourceComponent source; 3497 3498 /** 3499 * Specific instances of data or objects that have been accessed. 3500 */ 3501 @Child(name = "entity", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3502 @Description(shortDefinition = "Data or objects used", formalDefinition = "Specific instances of data or objects that have been accessed.") 3503 protected List<AuditEventEntityComponent> entity; 3504 3505 private static final long serialVersionUID = 106433685L; 3506 3507 /** 3508 * Constructor 3509 */ 3510 public AuditEvent() { 3511 super(); 3512 } 3513 3514 /** 3515 * Constructor 3516 */ 3517 public AuditEvent(Coding type, InstantType recorded, AuditEventSourceComponent source) { 3518 super(); 3519 this.type = type; 3520 this.recorded = recorded; 3521 this.source = source; 3522 } 3523 3524 /** 3525 * @return {@link #type} (Identifier for a family of the event. For example, a 3526 * menu item, program, rule, policy, function code, application name or 3527 * URL. It identifies the performed function.) 3528 */ 3529 public Coding getType() { 3530 if (this.type == null) 3531 if (Configuration.errorOnAutoCreate()) 3532 throw new Error("Attempt to auto-create AuditEvent.type"); 3533 else if (Configuration.doAutoCreate()) 3534 this.type = new Coding(); // cc 3535 return this.type; 3536 } 3537 3538 public boolean hasType() { 3539 return this.type != null && !this.type.isEmpty(); 3540 } 3541 3542 /** 3543 * @param value {@link #type} (Identifier for a family of the event. For 3544 * example, a menu item, program, rule, policy, function code, 3545 * application name or URL. It identifies the performed function.) 3546 */ 3547 public AuditEvent setType(Coding value) { 3548 this.type = value; 3549 return this; 3550 } 3551 3552 /** 3553 * @return {@link #subtype} (Identifier for the category of event.) 3554 */ 3555 public List<Coding> getSubtype() { 3556 if (this.subtype == null) 3557 this.subtype = new ArrayList<Coding>(); 3558 return this.subtype; 3559 } 3560 3561 /** 3562 * @return Returns a reference to <code>this</code> for easy method chaining 3563 */ 3564 public AuditEvent setSubtype(List<Coding> theSubtype) { 3565 this.subtype = theSubtype; 3566 return this; 3567 } 3568 3569 public boolean hasSubtype() { 3570 if (this.subtype == null) 3571 return false; 3572 for (Coding item : this.subtype) 3573 if (!item.isEmpty()) 3574 return true; 3575 return false; 3576 } 3577 3578 public Coding addSubtype() { // 3 3579 Coding t = new Coding(); 3580 if (this.subtype == null) 3581 this.subtype = new ArrayList<Coding>(); 3582 this.subtype.add(t); 3583 return t; 3584 } 3585 3586 public AuditEvent addSubtype(Coding t) { // 3 3587 if (t == null) 3588 return this; 3589 if (this.subtype == null) 3590 this.subtype = new ArrayList<Coding>(); 3591 this.subtype.add(t); 3592 return this; 3593 } 3594 3595 /** 3596 * @return The first repetition of repeating field {@link #subtype}, creating it 3597 * if it does not already exist 3598 */ 3599 public Coding getSubtypeFirstRep() { 3600 if (getSubtype().isEmpty()) { 3601 addSubtype(); 3602 } 3603 return getSubtype().get(0); 3604 } 3605 3606 /** 3607 * @return {@link #action} (Indicator for type of action performed during the 3608 * event that generated the audit.). This is the underlying object with 3609 * id, value and extensions. The accessor "getAction" gives direct 3610 * access to the value 3611 */ 3612 public Enumeration<AuditEventAction> getActionElement() { 3613 if (this.action == null) 3614 if (Configuration.errorOnAutoCreate()) 3615 throw new Error("Attempt to auto-create AuditEvent.action"); 3616 else if (Configuration.doAutoCreate()) 3617 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); // bb 3618 return this.action; 3619 } 3620 3621 public boolean hasActionElement() { 3622 return this.action != null && !this.action.isEmpty(); 3623 } 3624 3625 public boolean hasAction() { 3626 return this.action != null && !this.action.isEmpty(); 3627 } 3628 3629 /** 3630 * @param value {@link #action} (Indicator for type of action performed during 3631 * the event that generated the audit.). This is the underlying 3632 * object with id, value and extensions. The accessor "getAction" 3633 * gives direct access to the value 3634 */ 3635 public AuditEvent setActionElement(Enumeration<AuditEventAction> value) { 3636 this.action = value; 3637 return this; 3638 } 3639 3640 /** 3641 * @return Indicator for type of action performed during the event that 3642 * generated the audit. 3643 */ 3644 public AuditEventAction getAction() { 3645 return this.action == null ? null : this.action.getValue(); 3646 } 3647 3648 /** 3649 * @param value Indicator for type of action performed during the event that 3650 * generated the audit. 3651 */ 3652 public AuditEvent setAction(AuditEventAction value) { 3653 if (value == null) 3654 this.action = null; 3655 else { 3656 if (this.action == null) 3657 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); 3658 this.action.setValue(value); 3659 } 3660 return this; 3661 } 3662 3663 /** 3664 * @return {@link #period} (The period during which the activity occurred.) 3665 */ 3666 public Period getPeriod() { 3667 if (this.period == null) 3668 if (Configuration.errorOnAutoCreate()) 3669 throw new Error("Attempt to auto-create AuditEvent.period"); 3670 else if (Configuration.doAutoCreate()) 3671 this.period = new Period(); // cc 3672 return this.period; 3673 } 3674 3675 public boolean hasPeriod() { 3676 return this.period != null && !this.period.isEmpty(); 3677 } 3678 3679 /** 3680 * @param value {@link #period} (The period during which the activity occurred.) 3681 */ 3682 public AuditEvent setPeriod(Period value) { 3683 this.period = value; 3684 return this; 3685 } 3686 3687 /** 3688 * @return {@link #recorded} (The time when the event was recorded.). This is 3689 * the underlying object with id, value and extensions. The accessor 3690 * "getRecorded" gives direct access to the value 3691 */ 3692 public InstantType getRecordedElement() { 3693 if (this.recorded == null) 3694 if (Configuration.errorOnAutoCreate()) 3695 throw new Error("Attempt to auto-create AuditEvent.recorded"); 3696 else if (Configuration.doAutoCreate()) 3697 this.recorded = new InstantType(); // bb 3698 return this.recorded; 3699 } 3700 3701 public boolean hasRecordedElement() { 3702 return this.recorded != null && !this.recorded.isEmpty(); 3703 } 3704 3705 public boolean hasRecorded() { 3706 return this.recorded != null && !this.recorded.isEmpty(); 3707 } 3708 3709 /** 3710 * @param value {@link #recorded} (The time when the event was recorded.). This 3711 * is the underlying object with id, value and extensions. The 3712 * accessor "getRecorded" gives direct access to the value 3713 */ 3714 public AuditEvent setRecordedElement(InstantType value) { 3715 this.recorded = value; 3716 return this; 3717 } 3718 3719 /** 3720 * @return The time when the event was recorded. 3721 */ 3722 public Date getRecorded() { 3723 return this.recorded == null ? null : this.recorded.getValue(); 3724 } 3725 3726 /** 3727 * @param value The time when the event was recorded. 3728 */ 3729 public AuditEvent setRecorded(Date value) { 3730 if (this.recorded == null) 3731 this.recorded = new InstantType(); 3732 this.recorded.setValue(value); 3733 return this; 3734 } 3735 3736 /** 3737 * @return {@link #outcome} (Indicates whether the event succeeded or failed.). 3738 * This is the underlying object with id, value and extensions. The 3739 * accessor "getOutcome" gives direct access to the value 3740 */ 3741 public Enumeration<AuditEventOutcome> getOutcomeElement() { 3742 if (this.outcome == null) 3743 if (Configuration.errorOnAutoCreate()) 3744 throw new Error("Attempt to auto-create AuditEvent.outcome"); 3745 else if (Configuration.doAutoCreate()) 3746 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); // bb 3747 return this.outcome; 3748 } 3749 3750 public boolean hasOutcomeElement() { 3751 return this.outcome != null && !this.outcome.isEmpty(); 3752 } 3753 3754 public boolean hasOutcome() { 3755 return this.outcome != null && !this.outcome.isEmpty(); 3756 } 3757 3758 /** 3759 * @param value {@link #outcome} (Indicates whether the event succeeded or 3760 * failed.). This is the underlying object with id, value and 3761 * extensions. The accessor "getOutcome" gives direct access to the 3762 * value 3763 */ 3764 public AuditEvent setOutcomeElement(Enumeration<AuditEventOutcome> value) { 3765 this.outcome = value; 3766 return this; 3767 } 3768 3769 /** 3770 * @return Indicates whether the event succeeded or failed. 3771 */ 3772 public AuditEventOutcome getOutcome() { 3773 return this.outcome == null ? null : this.outcome.getValue(); 3774 } 3775 3776 /** 3777 * @param value Indicates whether the event succeeded or failed. 3778 */ 3779 public AuditEvent setOutcome(AuditEventOutcome value) { 3780 if (value == null) 3781 this.outcome = null; 3782 else { 3783 if (this.outcome == null) 3784 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); 3785 this.outcome.setValue(value); 3786 } 3787 return this; 3788 } 3789 3790 /** 3791 * @return {@link #outcomeDesc} (A free text description of the outcome of the 3792 * event.). This is the underlying object with id, value and extensions. 3793 * The accessor "getOutcomeDesc" gives direct access to the value 3794 */ 3795 public StringType getOutcomeDescElement() { 3796 if (this.outcomeDesc == null) 3797 if (Configuration.errorOnAutoCreate()) 3798 throw new Error("Attempt to auto-create AuditEvent.outcomeDesc"); 3799 else if (Configuration.doAutoCreate()) 3800 this.outcomeDesc = new StringType(); // bb 3801 return this.outcomeDesc; 3802 } 3803 3804 public boolean hasOutcomeDescElement() { 3805 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 3806 } 3807 3808 public boolean hasOutcomeDesc() { 3809 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 3810 } 3811 3812 /** 3813 * @param value {@link #outcomeDesc} (A free text description of the outcome of 3814 * the event.). This is the underlying object with id, value and 3815 * extensions. The accessor "getOutcomeDesc" gives direct access to 3816 * the value 3817 */ 3818 public AuditEvent setOutcomeDescElement(StringType value) { 3819 this.outcomeDesc = value; 3820 return this; 3821 } 3822 3823 /** 3824 * @return A free text description of the outcome of the event. 3825 */ 3826 public String getOutcomeDesc() { 3827 return this.outcomeDesc == null ? null : this.outcomeDesc.getValue(); 3828 } 3829 3830 /** 3831 * @param value A free text description of the outcome of the event. 3832 */ 3833 public AuditEvent setOutcomeDesc(String value) { 3834 if (Utilities.noString(value)) 3835 this.outcomeDesc = null; 3836 else { 3837 if (this.outcomeDesc == null) 3838 this.outcomeDesc = new StringType(); 3839 this.outcomeDesc.setValue(value); 3840 } 3841 return this; 3842 } 3843 3844 /** 3845 * @return {@link #purposeOfEvent} (The purposeOfUse (reason) that was used 3846 * during the event being recorded.) 3847 */ 3848 public List<CodeableConcept> getPurposeOfEvent() { 3849 if (this.purposeOfEvent == null) 3850 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3851 return this.purposeOfEvent; 3852 } 3853 3854 /** 3855 * @return Returns a reference to <code>this</code> for easy method chaining 3856 */ 3857 public AuditEvent setPurposeOfEvent(List<CodeableConcept> thePurposeOfEvent) { 3858 this.purposeOfEvent = thePurposeOfEvent; 3859 return this; 3860 } 3861 3862 public boolean hasPurposeOfEvent() { 3863 if (this.purposeOfEvent == null) 3864 return false; 3865 for (CodeableConcept item : this.purposeOfEvent) 3866 if (!item.isEmpty()) 3867 return true; 3868 return false; 3869 } 3870 3871 public CodeableConcept addPurposeOfEvent() { // 3 3872 CodeableConcept t = new CodeableConcept(); 3873 if (this.purposeOfEvent == null) 3874 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3875 this.purposeOfEvent.add(t); 3876 return t; 3877 } 3878 3879 public AuditEvent addPurposeOfEvent(CodeableConcept t) { // 3 3880 if (t == null) 3881 return this; 3882 if (this.purposeOfEvent == null) 3883 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3884 this.purposeOfEvent.add(t); 3885 return this; 3886 } 3887 3888 /** 3889 * @return The first repetition of repeating field {@link #purposeOfEvent}, 3890 * creating it if it does not already exist 3891 */ 3892 public CodeableConcept getPurposeOfEventFirstRep() { 3893 if (getPurposeOfEvent().isEmpty()) { 3894 addPurposeOfEvent(); 3895 } 3896 return getPurposeOfEvent().get(0); 3897 } 3898 3899 /** 3900 * @return {@link #agent} (An actor taking an active role in the event or 3901 * activity that is logged.) 3902 */ 3903 public List<AuditEventAgentComponent> getAgent() { 3904 if (this.agent == null) 3905 this.agent = new ArrayList<AuditEventAgentComponent>(); 3906 return this.agent; 3907 } 3908 3909 /** 3910 * @return Returns a reference to <code>this</code> for easy method chaining 3911 */ 3912 public AuditEvent setAgent(List<AuditEventAgentComponent> theAgent) { 3913 this.agent = theAgent; 3914 return this; 3915 } 3916 3917 public boolean hasAgent() { 3918 if (this.agent == null) 3919 return false; 3920 for (AuditEventAgentComponent item : this.agent) 3921 if (!item.isEmpty()) 3922 return true; 3923 return false; 3924 } 3925 3926 public AuditEventAgentComponent addAgent() { // 3 3927 AuditEventAgentComponent t = new AuditEventAgentComponent(); 3928 if (this.agent == null) 3929 this.agent = new ArrayList<AuditEventAgentComponent>(); 3930 this.agent.add(t); 3931 return t; 3932 } 3933 3934 public AuditEvent addAgent(AuditEventAgentComponent t) { // 3 3935 if (t == null) 3936 return this; 3937 if (this.agent == null) 3938 this.agent = new ArrayList<AuditEventAgentComponent>(); 3939 this.agent.add(t); 3940 return this; 3941 } 3942 3943 /** 3944 * @return The first repetition of repeating field {@link #agent}, creating it 3945 * if it does not already exist 3946 */ 3947 public AuditEventAgentComponent getAgentFirstRep() { 3948 if (getAgent().isEmpty()) { 3949 addAgent(); 3950 } 3951 return getAgent().get(0); 3952 } 3953 3954 /** 3955 * @return {@link #source} (The system that is reporting the event.) 3956 */ 3957 public AuditEventSourceComponent getSource() { 3958 if (this.source == null) 3959 if (Configuration.errorOnAutoCreate()) 3960 throw new Error("Attempt to auto-create AuditEvent.source"); 3961 else if (Configuration.doAutoCreate()) 3962 this.source = new AuditEventSourceComponent(); // cc 3963 return this.source; 3964 } 3965 3966 public boolean hasSource() { 3967 return this.source != null && !this.source.isEmpty(); 3968 } 3969 3970 /** 3971 * @param value {@link #source} (The system that is reporting the event.) 3972 */ 3973 public AuditEvent setSource(AuditEventSourceComponent value) { 3974 this.source = value; 3975 return this; 3976 } 3977 3978 /** 3979 * @return {@link #entity} (Specific instances of data or objects that have been 3980 * accessed.) 3981 */ 3982 public List<AuditEventEntityComponent> getEntity() { 3983 if (this.entity == null) 3984 this.entity = new ArrayList<AuditEventEntityComponent>(); 3985 return this.entity; 3986 } 3987 3988 /** 3989 * @return Returns a reference to <code>this</code> for easy method chaining 3990 */ 3991 public AuditEvent setEntity(List<AuditEventEntityComponent> theEntity) { 3992 this.entity = theEntity; 3993 return this; 3994 } 3995 3996 public boolean hasEntity() { 3997 if (this.entity == null) 3998 return false; 3999 for (AuditEventEntityComponent item : this.entity) 4000 if (!item.isEmpty()) 4001 return true; 4002 return false; 4003 } 4004 4005 public AuditEventEntityComponent addEntity() { // 3 4006 AuditEventEntityComponent t = new AuditEventEntityComponent(); 4007 if (this.entity == null) 4008 this.entity = new ArrayList<AuditEventEntityComponent>(); 4009 this.entity.add(t); 4010 return t; 4011 } 4012 4013 public AuditEvent addEntity(AuditEventEntityComponent t) { // 3 4014 if (t == null) 4015 return this; 4016 if (this.entity == null) 4017 this.entity = new ArrayList<AuditEventEntityComponent>(); 4018 this.entity.add(t); 4019 return this; 4020 } 4021 4022 /** 4023 * @return The first repetition of repeating field {@link #entity}, creating it 4024 * if it does not already exist 4025 */ 4026 public AuditEventEntityComponent getEntityFirstRep() { 4027 if (getEntity().isEmpty()) { 4028 addEntity(); 4029 } 4030 return getEntity().get(0); 4031 } 4032 4033 protected void listChildren(List<Property> children) { 4034 super.listChildren(children); 4035 children.add(new Property("type", "Coding", 4036 "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 4037 0, 1, type)); 4038 children.add(new Property("subtype", "Coding", "Identifier for the category of event.", 0, 4039 java.lang.Integer.MAX_VALUE, subtype)); 4040 children.add(new Property("action", "code", 4041 "Indicator for type of action performed during the event that generated the audit.", 0, 1, action)); 4042 children.add(new Property("period", "Period", "The period during which the activity occurred.", 0, 1, period)); 4043 children.add(new Property("recorded", "instant", "The time when the event was recorded.", 0, 1, recorded)); 4044 children.add(new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 1, outcome)); 4045 children.add(new Property("outcomeDesc", "string", "A free text description of the outcome of the event.", 0, 1, 4046 outcomeDesc)); 4047 children.add(new Property("purposeOfEvent", "CodeableConcept", 4048 "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, 4049 purposeOfEvent)); 4050 children.add(new Property("agent", "", "An actor taking an active role in the event or activity that is logged.", 0, 4051 java.lang.Integer.MAX_VALUE, agent)); 4052 children.add(new Property("source", "", "The system that is reporting the event.", 0, 1, source)); 4053 children.add(new Property("entity", "", "Specific instances of data or objects that have been accessed.", 0, 4054 java.lang.Integer.MAX_VALUE, entity)); 4055 } 4056 4057 @Override 4058 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4059 switch (_hash) { 4060 case 3575610: 4061 /* type */ return new Property("type", "Coding", 4062 "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 4063 0, 1, type); 4064 case -1867567750: 4065 /* subtype */ return new Property("subtype", "Coding", "Identifier for the category of event.", 0, 4066 java.lang.Integer.MAX_VALUE, subtype); 4067 case -1422950858: 4068 /* action */ return new Property("action", "code", 4069 "Indicator for type of action performed during the event that generated the audit.", 0, 1, action); 4070 case -991726143: 4071 /* period */ return new Property("period", "Period", "The period during which the activity occurred.", 0, 1, 4072 period); 4073 case -799233872: 4074 /* recorded */ return new Property("recorded", "instant", "The time when the event was recorded.", 0, 1, 4075 recorded); 4076 case -1106507950: 4077 /* outcome */ return new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 1, 4078 outcome); 4079 case 1062502659: 4080 /* outcomeDesc */ return new Property("outcomeDesc", "string", 4081 "A free text description of the outcome of the event.", 0, 1, outcomeDesc); 4082 case -341917691: 4083 /* purposeOfEvent */ return new Property("purposeOfEvent", "CodeableConcept", 4084 "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, 4085 purposeOfEvent); 4086 case 92750597: 4087 /* agent */ return new Property("agent", "", 4088 "An actor taking an active role in the event or activity that is logged.", 0, java.lang.Integer.MAX_VALUE, 4089 agent); 4090 case -896505829: 4091 /* source */ return new Property("source", "", "The system that is reporting the event.", 0, 1, source); 4092 case -1298275357: 4093 /* entity */ return new Property("entity", "", "Specific instances of data or objects that have been accessed.", 4094 0, java.lang.Integer.MAX_VALUE, entity); 4095 default: 4096 return super.getNamedProperty(_hash, _name, _checkValid); 4097 } 4098 4099 } 4100 4101 @Override 4102 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4103 switch (hash) { 4104 case 3575610: 4105 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 4106 case -1867567750: 4107 /* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // Coding 4108 case -1422950858: 4109 /* action */ return this.action == null ? new Base[0] : new Base[] { this.action }; // Enumeration<AuditEventAction> 4110 case -991726143: 4111 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 4112 case -799233872: 4113 /* recorded */ return this.recorded == null ? new Base[0] : new Base[] { this.recorded }; // InstantType 4114 case -1106507950: 4115 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<AuditEventOutcome> 4116 case 1062502659: 4117 /* outcomeDesc */ return this.outcomeDesc == null ? new Base[0] : new Base[] { this.outcomeDesc }; // StringType 4118 case -341917691: 4119 /* purposeOfEvent */ return this.purposeOfEvent == null ? new Base[0] 4120 : this.purposeOfEvent.toArray(new Base[this.purposeOfEvent.size()]); // CodeableConcept 4121 case 92750597: 4122 /* agent */ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // AuditEventAgentComponent 4123 case -896505829: 4124 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // AuditEventSourceComponent 4125 case -1298275357: 4126 /* entity */ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // AuditEventEntityComponent 4127 default: 4128 return super.getProperty(hash, name, checkValid); 4129 } 4130 4131 } 4132 4133 @Override 4134 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4135 switch (hash) { 4136 case 3575610: // type 4137 this.type = castToCoding(value); // Coding 4138 return value; 4139 case -1867567750: // subtype 4140 this.getSubtype().add(castToCoding(value)); // Coding 4141 return value; 4142 case -1422950858: // action 4143 value = new AuditEventActionEnumFactory().fromType(castToCode(value)); 4144 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 4145 return value; 4146 case -991726143: // period 4147 this.period = castToPeriod(value); // Period 4148 return value; 4149 case -799233872: // recorded 4150 this.recorded = castToInstant(value); // InstantType 4151 return value; 4152 case -1106507950: // outcome 4153 value = new AuditEventOutcomeEnumFactory().fromType(castToCode(value)); 4154 this.outcome = (Enumeration) value; // Enumeration<AuditEventOutcome> 4155 return value; 4156 case 1062502659: // outcomeDesc 4157 this.outcomeDesc = castToString(value); // StringType 4158 return value; 4159 case -341917691: // purposeOfEvent 4160 this.getPurposeOfEvent().add(castToCodeableConcept(value)); // CodeableConcept 4161 return value; 4162 case 92750597: // agent 4163 this.getAgent().add((AuditEventAgentComponent) value); // AuditEventAgentComponent 4164 return value; 4165 case -896505829: // source 4166 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 4167 return value; 4168 case -1298275357: // entity 4169 this.getEntity().add((AuditEventEntityComponent) value); // AuditEventEntityComponent 4170 return value; 4171 default: 4172 return super.setProperty(hash, name, value); 4173 } 4174 4175 } 4176 4177 @Override 4178 public Base setProperty(String name, Base value) throws FHIRException { 4179 if (name.equals("type")) { 4180 this.type = castToCoding(value); // Coding 4181 } else if (name.equals("subtype")) { 4182 this.getSubtype().add(castToCoding(value)); 4183 } else if (name.equals("action")) { 4184 value = new AuditEventActionEnumFactory().fromType(castToCode(value)); 4185 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 4186 } else if (name.equals("period")) { 4187 this.period = castToPeriod(value); // Period 4188 } else if (name.equals("recorded")) { 4189 this.recorded = castToInstant(value); // InstantType 4190 } else if (name.equals("outcome")) { 4191 value = new AuditEventOutcomeEnumFactory().fromType(castToCode(value)); 4192 this.outcome = (Enumeration) value; // Enumeration<AuditEventOutcome> 4193 } else if (name.equals("outcomeDesc")) { 4194 this.outcomeDesc = castToString(value); // StringType 4195 } else if (name.equals("purposeOfEvent")) { 4196 this.getPurposeOfEvent().add(castToCodeableConcept(value)); 4197 } else if (name.equals("agent")) { 4198 this.getAgent().add((AuditEventAgentComponent) value); 4199 } else if (name.equals("source")) { 4200 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 4201 } else if (name.equals("entity")) { 4202 this.getEntity().add((AuditEventEntityComponent) value); 4203 } else 4204 return super.setProperty(name, value); 4205 return value; 4206 } 4207 4208 @Override 4209 public void removeChild(String name, Base value) throws FHIRException { 4210 if (name.equals("type")) { 4211 this.type = null; 4212 } else if (name.equals("subtype")) { 4213 this.getSubtype().remove(castToCoding(value)); 4214 } else if (name.equals("action")) { 4215 this.action = null; 4216 } else if (name.equals("period")) { 4217 this.period = null; 4218 } else if (name.equals("recorded")) { 4219 this.recorded = null; 4220 } else if (name.equals("outcome")) { 4221 this.outcome = null; 4222 } else if (name.equals("outcomeDesc")) { 4223 this.outcomeDesc = null; 4224 } else if (name.equals("purposeOfEvent")) { 4225 this.getPurposeOfEvent().remove(castToCodeableConcept(value)); 4226 } else if (name.equals("agent")) { 4227 this.getAgent().remove((AuditEventAgentComponent) value); 4228 } else if (name.equals("source")) { 4229 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 4230 } else if (name.equals("entity")) { 4231 this.getEntity().remove((AuditEventEntityComponent) value); 4232 } else 4233 super.removeChild(name, value); 4234 4235 } 4236 4237 @Override 4238 public Base makeProperty(int hash, String name) throws FHIRException { 4239 switch (hash) { 4240 case 3575610: 4241 return getType(); 4242 case -1867567750: 4243 return addSubtype(); 4244 case -1422950858: 4245 return getActionElement(); 4246 case -991726143: 4247 return getPeriod(); 4248 case -799233872: 4249 return getRecordedElement(); 4250 case -1106507950: 4251 return getOutcomeElement(); 4252 case 1062502659: 4253 return getOutcomeDescElement(); 4254 case -341917691: 4255 return addPurposeOfEvent(); 4256 case 92750597: 4257 return addAgent(); 4258 case -896505829: 4259 return getSource(); 4260 case -1298275357: 4261 return addEntity(); 4262 default: 4263 return super.makeProperty(hash, name); 4264 } 4265 4266 } 4267 4268 @Override 4269 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4270 switch (hash) { 4271 case 3575610: 4272 /* type */ return new String[] { "Coding" }; 4273 case -1867567750: 4274 /* subtype */ return new String[] { "Coding" }; 4275 case -1422950858: 4276 /* action */ return new String[] { "code" }; 4277 case -991726143: 4278 /* period */ return new String[] { "Period" }; 4279 case -799233872: 4280 /* recorded */ return new String[] { "instant" }; 4281 case -1106507950: 4282 /* outcome */ return new String[] { "code" }; 4283 case 1062502659: 4284 /* outcomeDesc */ return new String[] { "string" }; 4285 case -341917691: 4286 /* purposeOfEvent */ return new String[] { "CodeableConcept" }; 4287 case 92750597: 4288 /* agent */ return new String[] {}; 4289 case -896505829: 4290 /* source */ return new String[] {}; 4291 case -1298275357: 4292 /* entity */ return new String[] {}; 4293 default: 4294 return super.getTypesForProperty(hash, name); 4295 } 4296 4297 } 4298 4299 @Override 4300 public Base addChild(String name) throws FHIRException { 4301 if (name.equals("type")) { 4302 this.type = new Coding(); 4303 return this.type; 4304 } else if (name.equals("subtype")) { 4305 return addSubtype(); 4306 } else if (name.equals("action")) { 4307 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.action"); 4308 } else if (name.equals("period")) { 4309 this.period = new Period(); 4310 return this.period; 4311 } else if (name.equals("recorded")) { 4312 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.recorded"); 4313 } else if (name.equals("outcome")) { 4314 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcome"); 4315 } else if (name.equals("outcomeDesc")) { 4316 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcomeDesc"); 4317 } else if (name.equals("purposeOfEvent")) { 4318 return addPurposeOfEvent(); 4319 } else if (name.equals("agent")) { 4320 return addAgent(); 4321 } else if (name.equals("source")) { 4322 this.source = new AuditEventSourceComponent(); 4323 return this.source; 4324 } else if (name.equals("entity")) { 4325 return addEntity(); 4326 } else 4327 return super.addChild(name); 4328 } 4329 4330 public String fhirType() { 4331 return "AuditEvent"; 4332 4333 } 4334 4335 public AuditEvent copy() { 4336 AuditEvent dst = new AuditEvent(); 4337 copyValues(dst); 4338 return dst; 4339 } 4340 4341 public void copyValues(AuditEvent dst) { 4342 super.copyValues(dst); 4343 dst.type = type == null ? null : type.copy(); 4344 if (subtype != null) { 4345 dst.subtype = new ArrayList<Coding>(); 4346 for (Coding i : subtype) 4347 dst.subtype.add(i.copy()); 4348 } 4349 ; 4350 dst.action = action == null ? null : action.copy(); 4351 dst.period = period == null ? null : period.copy(); 4352 dst.recorded = recorded == null ? null : recorded.copy(); 4353 dst.outcome = outcome == null ? null : outcome.copy(); 4354 dst.outcomeDesc = outcomeDesc == null ? null : outcomeDesc.copy(); 4355 if (purposeOfEvent != null) { 4356 dst.purposeOfEvent = new ArrayList<CodeableConcept>(); 4357 for (CodeableConcept i : purposeOfEvent) 4358 dst.purposeOfEvent.add(i.copy()); 4359 } 4360 ; 4361 if (agent != null) { 4362 dst.agent = new ArrayList<AuditEventAgentComponent>(); 4363 for (AuditEventAgentComponent i : agent) 4364 dst.agent.add(i.copy()); 4365 } 4366 ; 4367 dst.source = source == null ? null : source.copy(); 4368 if (entity != null) { 4369 dst.entity = new ArrayList<AuditEventEntityComponent>(); 4370 for (AuditEventEntityComponent i : entity) 4371 dst.entity.add(i.copy()); 4372 } 4373 ; 4374 } 4375 4376 protected AuditEvent typedCopy() { 4377 return copy(); 4378 } 4379 4380 @Override 4381 public boolean equalsDeep(Base other_) { 4382 if (!super.equalsDeep(other_)) 4383 return false; 4384 if (!(other_ instanceof AuditEvent)) 4385 return false; 4386 AuditEvent o = (AuditEvent) other_; 4387 return compareDeep(type, o.type, true) && compareDeep(subtype, o.subtype, true) 4388 && compareDeep(action, o.action, true) && compareDeep(period, o.period, true) 4389 && compareDeep(recorded, o.recorded, true) && compareDeep(outcome, o.outcome, true) 4390 && compareDeep(outcomeDesc, o.outcomeDesc, true) && compareDeep(purposeOfEvent, o.purposeOfEvent, true) 4391 && compareDeep(agent, o.agent, true) && compareDeep(source, o.source, true) 4392 && compareDeep(entity, o.entity, true); 4393 } 4394 4395 @Override 4396 public boolean equalsShallow(Base other_) { 4397 if (!super.equalsShallow(other_)) 4398 return false; 4399 if (!(other_ instanceof AuditEvent)) 4400 return false; 4401 AuditEvent o = (AuditEvent) other_; 4402 return compareValues(action, o.action, true) && compareValues(recorded, o.recorded, true) 4403 && compareValues(outcome, o.outcome, true) && compareValues(outcomeDesc, o.outcomeDesc, true); 4404 } 4405 4406 public boolean isEmpty() { 4407 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subtype, action, period, recorded, outcome, 4408 outcomeDesc, purposeOfEvent, agent, source, entity); 4409 } 4410 4411 @Override 4412 public ResourceType getResourceType() { 4413 return ResourceType.AuditEvent; 4414 } 4415 4416 /** 4417 * Search parameter: <b>date</b> 4418 * <p> 4419 * Description: <b>Time when the event was recorded</b><br> 4420 * Type: <b>date</b><br> 4421 * Path: <b>AuditEvent.recorded</b><br> 4422 * </p> 4423 */ 4424 @SearchParamDefinition(name = "date", path = "AuditEvent.recorded", description = "Time when the event was recorded", type = "date") 4425 public static final String SP_DATE = "date"; 4426 /** 4427 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4428 * <p> 4429 * Description: <b>Time when the event was recorded</b><br> 4430 * Type: <b>date</b><br> 4431 * Path: <b>AuditEvent.recorded</b><br> 4432 * </p> 4433 */ 4434 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4435 SP_DATE); 4436 4437 /** 4438 * Search parameter: <b>entity-type</b> 4439 * <p> 4440 * Description: <b>Type of entity involved</b><br> 4441 * Type: <b>token</b><br> 4442 * Path: <b>AuditEvent.entity.type</b><br> 4443 * </p> 4444 */ 4445 @SearchParamDefinition(name = "entity-type", path = "AuditEvent.entity.type", description = "Type of entity involved", type = "token") 4446 public static final String SP_ENTITY_TYPE = "entity-type"; 4447 /** 4448 * <b>Fluent Client</b> search parameter constant for <b>entity-type</b> 4449 * <p> 4450 * Description: <b>Type of entity involved</b><br> 4451 * Type: <b>token</b><br> 4452 * Path: <b>AuditEvent.entity.type</b><br> 4453 * </p> 4454 */ 4455 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4456 SP_ENTITY_TYPE); 4457 4458 /** 4459 * Search parameter: <b>agent</b> 4460 * <p> 4461 * Description: <b>Identifier of who</b><br> 4462 * Type: <b>reference</b><br> 4463 * Path: <b>AuditEvent.agent.who</b><br> 4464 * </p> 4465 */ 4466 @SearchParamDefinition(name = "agent", path = "AuditEvent.agent.who", description = "Identifier of who", type = "reference", providesMembershipIn = { 4467 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4468 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 4469 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4470 public static final String SP_AGENT = "agent"; 4471 /** 4472 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 4473 * <p> 4474 * Description: <b>Identifier of who</b><br> 4475 * Type: <b>reference</b><br> 4476 * Path: <b>AuditEvent.agent.who</b><br> 4477 * </p> 4478 */ 4479 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4480 SP_AGENT); 4481 4482 /** 4483 * Constant for fluent queries to be used to add include statements. Specifies 4484 * the path value of "<b>AuditEvent:agent</b>". 4485 */ 4486 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include( 4487 "AuditEvent:agent").toLocked(); 4488 4489 /** 4490 * Search parameter: <b>address</b> 4491 * <p> 4492 * Description: <b>Identifier for the network access point of the user 4493 * device</b><br> 4494 * Type: <b>string</b><br> 4495 * Path: <b>AuditEvent.agent.network.address</b><br> 4496 * </p> 4497 */ 4498 @SearchParamDefinition(name = "address", path = "AuditEvent.agent.network.address", description = "Identifier for the network access point of the user device", type = "string") 4499 public static final String SP_ADDRESS = "address"; 4500 /** 4501 * <b>Fluent Client</b> search parameter constant for <b>address</b> 4502 * <p> 4503 * Description: <b>Identifier for the network access point of the user 4504 * device</b><br> 4505 * Type: <b>string</b><br> 4506 * Path: <b>AuditEvent.agent.network.address</b><br> 4507 * </p> 4508 */ 4509 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 4510 SP_ADDRESS); 4511 4512 /** 4513 * Search parameter: <b>entity-role</b> 4514 * <p> 4515 * Description: <b>What role the entity played</b><br> 4516 * Type: <b>token</b><br> 4517 * Path: <b>AuditEvent.entity.role</b><br> 4518 * </p> 4519 */ 4520 @SearchParamDefinition(name = "entity-role", path = "AuditEvent.entity.role", description = "What role the entity played", type = "token") 4521 public static final String SP_ENTITY_ROLE = "entity-role"; 4522 /** 4523 * <b>Fluent Client</b> search parameter constant for <b>entity-role</b> 4524 * <p> 4525 * Description: <b>What role the entity played</b><br> 4526 * Type: <b>token</b><br> 4527 * Path: <b>AuditEvent.entity.role</b><br> 4528 * </p> 4529 */ 4530 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4531 SP_ENTITY_ROLE); 4532 4533 /** 4534 * Search parameter: <b>source</b> 4535 * <p> 4536 * Description: <b>The identity of source detecting the event</b><br> 4537 * Type: <b>reference</b><br> 4538 * Path: <b>AuditEvent.source.observer</b><br> 4539 * </p> 4540 */ 4541 @SearchParamDefinition(name = "source", path = "AuditEvent.source.observer", description = "The identity of source detecting the event", type = "reference", target = { 4542 Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 4543 RelatedPerson.class }) 4544 public static final String SP_SOURCE = "source"; 4545 /** 4546 * <b>Fluent Client</b> search parameter constant for <b>source</b> 4547 * <p> 4548 * Description: <b>The identity of source detecting the event</b><br> 4549 * Type: <b>reference</b><br> 4550 * Path: <b>AuditEvent.source.observer</b><br> 4551 * </p> 4552 */ 4553 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4554 SP_SOURCE); 4555 4556 /** 4557 * Constant for fluent queries to be used to add include statements. Specifies 4558 * the path value of "<b>AuditEvent:source</b>". 4559 */ 4560 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 4561 "AuditEvent:source").toLocked(); 4562 4563 /** 4564 * Search parameter: <b>type</b> 4565 * <p> 4566 * Description: <b>Type/identifier of event</b><br> 4567 * Type: <b>token</b><br> 4568 * Path: <b>AuditEvent.type</b><br> 4569 * </p> 4570 */ 4571 @SearchParamDefinition(name = "type", path = "AuditEvent.type", description = "Type/identifier of event", type = "token") 4572 public static final String SP_TYPE = "type"; 4573 /** 4574 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4575 * <p> 4576 * Description: <b>Type/identifier of event</b><br> 4577 * Type: <b>token</b><br> 4578 * Path: <b>AuditEvent.type</b><br> 4579 * </p> 4580 */ 4581 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4582 SP_TYPE); 4583 4584 /** 4585 * Search parameter: <b>altid</b> 4586 * <p> 4587 * Description: <b>Alternative User identity</b><br> 4588 * Type: <b>token</b><br> 4589 * Path: <b>AuditEvent.agent.altId</b><br> 4590 * </p> 4591 */ 4592 @SearchParamDefinition(name = "altid", path = "AuditEvent.agent.altId", description = "Alternative User identity", type = "token") 4593 public static final String SP_ALTID = "altid"; 4594 /** 4595 * <b>Fluent Client</b> search parameter constant for <b>altid</b> 4596 * <p> 4597 * Description: <b>Alternative User identity</b><br> 4598 * Type: <b>token</b><br> 4599 * Path: <b>AuditEvent.agent.altId</b><br> 4600 * </p> 4601 */ 4602 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ALTID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4603 SP_ALTID); 4604 4605 /** 4606 * Search parameter: <b>site</b> 4607 * <p> 4608 * Description: <b>Logical source location within the enterprise</b><br> 4609 * Type: <b>token</b><br> 4610 * Path: <b>AuditEvent.source.site</b><br> 4611 * </p> 4612 */ 4613 @SearchParamDefinition(name = "site", path = "AuditEvent.source.site", description = "Logical source location within the enterprise", type = "token") 4614 public static final String SP_SITE = "site"; 4615 /** 4616 * <b>Fluent Client</b> search parameter constant for <b>site</b> 4617 * <p> 4618 * Description: <b>Logical source location within the enterprise</b><br> 4619 * Type: <b>token</b><br> 4620 * Path: <b>AuditEvent.source.site</b><br> 4621 * </p> 4622 */ 4623 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4624 SP_SITE); 4625 4626 /** 4627 * Search parameter: <b>agent-name</b> 4628 * <p> 4629 * Description: <b>Human friendly name for the agent</b><br> 4630 * Type: <b>string</b><br> 4631 * Path: <b>AuditEvent.agent.name</b><br> 4632 * </p> 4633 */ 4634 @SearchParamDefinition(name = "agent-name", path = "AuditEvent.agent.name", description = "Human friendly name for the agent", type = "string") 4635 public static final String SP_AGENT_NAME = "agent-name"; 4636 /** 4637 * <b>Fluent Client</b> search parameter constant for <b>agent-name</b> 4638 * <p> 4639 * Description: <b>Human friendly name for the agent</b><br> 4640 * Type: <b>string</b><br> 4641 * Path: <b>AuditEvent.agent.name</b><br> 4642 * </p> 4643 */ 4644 public static final ca.uhn.fhir.rest.gclient.StringClientParam AGENT_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4645 SP_AGENT_NAME); 4646 4647 /** 4648 * Search parameter: <b>entity-name</b> 4649 * <p> 4650 * Description: <b>Descriptor for entity</b><br> 4651 * Type: <b>string</b><br> 4652 * Path: <b>AuditEvent.entity.name</b><br> 4653 * </p> 4654 */ 4655 @SearchParamDefinition(name = "entity-name", path = "AuditEvent.entity.name", description = "Descriptor for entity", type = "string") 4656 public static final String SP_ENTITY_NAME = "entity-name"; 4657 /** 4658 * <b>Fluent Client</b> search parameter constant for <b>entity-name</b> 4659 * <p> 4660 * Description: <b>Descriptor for entity</b><br> 4661 * Type: <b>string</b><br> 4662 * Path: <b>AuditEvent.entity.name</b><br> 4663 * </p> 4664 */ 4665 public static final ca.uhn.fhir.rest.gclient.StringClientParam ENTITY_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4666 SP_ENTITY_NAME); 4667 4668 /** 4669 * Search parameter: <b>subtype</b> 4670 * <p> 4671 * Description: <b>More specific type/id for the event</b><br> 4672 * Type: <b>token</b><br> 4673 * Path: <b>AuditEvent.subtype</b><br> 4674 * </p> 4675 */ 4676 @SearchParamDefinition(name = "subtype", path = "AuditEvent.subtype", description = "More specific type/id for the event", type = "token") 4677 public static final String SP_SUBTYPE = "subtype"; 4678 /** 4679 * <b>Fluent Client</b> search parameter constant for <b>subtype</b> 4680 * <p> 4681 * Description: <b>More specific type/id for the event</b><br> 4682 * Type: <b>token</b><br> 4683 * Path: <b>AuditEvent.subtype</b><br> 4684 * </p> 4685 */ 4686 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4687 SP_SUBTYPE); 4688 4689 /** 4690 * Search parameter: <b>patient</b> 4691 * <p> 4692 * Description: <b>Identifier of who</b><br> 4693 * Type: <b>reference</b><br> 4694 * Path: <b>AuditEvent.agent.who, AuditEvent.entity.what</b><br> 4695 * </p> 4696 */ 4697 @SearchParamDefinition(name = "patient", path = "AuditEvent.agent.who.where(resolve() is Patient) | AuditEvent.entity.what.where(resolve() is Patient)", description = "Identifier of who", type = "reference", providesMembershipIn = { 4698 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4699 public static final String SP_PATIENT = "patient"; 4700 /** 4701 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4702 * <p> 4703 * Description: <b>Identifier of who</b><br> 4704 * Type: <b>reference</b><br> 4705 * Path: <b>AuditEvent.agent.who, AuditEvent.entity.what</b><br> 4706 * </p> 4707 */ 4708 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4709 SP_PATIENT); 4710 4711 /** 4712 * Constant for fluent queries to be used to add include statements. Specifies 4713 * the path value of "<b>AuditEvent:patient</b>". 4714 */ 4715 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4716 "AuditEvent:patient").toLocked(); 4717 4718 /** 4719 * Search parameter: <b>action</b> 4720 * <p> 4721 * Description: <b>Type of action performed during the event</b><br> 4722 * Type: <b>token</b><br> 4723 * Path: <b>AuditEvent.action</b><br> 4724 * </p> 4725 */ 4726 @SearchParamDefinition(name = "action", path = "AuditEvent.action", description = "Type of action performed during the event", type = "token") 4727 public static final String SP_ACTION = "action"; 4728 /** 4729 * <b>Fluent Client</b> search parameter constant for <b>action</b> 4730 * <p> 4731 * Description: <b>Type of action performed during the event</b><br> 4732 * Type: <b>token</b><br> 4733 * Path: <b>AuditEvent.action</b><br> 4734 * </p> 4735 */ 4736 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4737 SP_ACTION); 4738 4739 /** 4740 * Search parameter: <b>agent-role</b> 4741 * <p> 4742 * Description: <b>Agent role in the event</b><br> 4743 * Type: <b>token</b><br> 4744 * Path: <b>AuditEvent.agent.role</b><br> 4745 * </p> 4746 */ 4747 @SearchParamDefinition(name = "agent-role", path = "AuditEvent.agent.role", description = "Agent role in the event", type = "token") 4748 public static final String SP_AGENT_ROLE = "agent-role"; 4749 /** 4750 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 4751 * <p> 4752 * Description: <b>Agent role in the event</b><br> 4753 * Type: <b>token</b><br> 4754 * Path: <b>AuditEvent.agent.role</b><br> 4755 * </p> 4756 */ 4757 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4758 SP_AGENT_ROLE); 4759 4760 /** 4761 * Search parameter: <b>entity</b> 4762 * <p> 4763 * Description: <b>Specific instance of resource</b><br> 4764 * Type: <b>reference</b><br> 4765 * Path: <b>AuditEvent.entity.what</b><br> 4766 * </p> 4767 */ 4768 @SearchParamDefinition(name = "entity", path = "AuditEvent.entity.what", description = "Specific instance of resource", type = "reference") 4769 public static final String SP_ENTITY = "entity"; 4770 /** 4771 * <b>Fluent Client</b> search parameter constant for <b>entity</b> 4772 * <p> 4773 * Description: <b>Specific instance of resource</b><br> 4774 * Type: <b>reference</b><br> 4775 * Path: <b>AuditEvent.entity.what</b><br> 4776 * </p> 4777 */ 4778 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4779 SP_ENTITY); 4780 4781 /** 4782 * Constant for fluent queries to be used to add include statements. Specifies 4783 * the path value of "<b>AuditEvent:entity</b>". 4784 */ 4785 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY = new ca.uhn.fhir.model.api.Include( 4786 "AuditEvent:entity").toLocked(); 4787 4788 /** 4789 * Search parameter: <b>outcome</b> 4790 * <p> 4791 * Description: <b>Whether the event succeeded or failed</b><br> 4792 * Type: <b>token</b><br> 4793 * Path: <b>AuditEvent.outcome</b><br> 4794 * </p> 4795 */ 4796 @SearchParamDefinition(name = "outcome", path = "AuditEvent.outcome", description = "Whether the event succeeded or failed", type = "token") 4797 public static final String SP_OUTCOME = "outcome"; 4798 /** 4799 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 4800 * <p> 4801 * Description: <b>Whether the event succeeded or failed</b><br> 4802 * Type: <b>token</b><br> 4803 * Path: <b>AuditEvent.outcome</b><br> 4804 * </p> 4805 */ 4806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4807 SP_OUTCOME); 4808 4809 /** 4810 * Search parameter: <b>policy</b> 4811 * <p> 4812 * Description: <b>Policy that authorized event</b><br> 4813 * Type: <b>uri</b><br> 4814 * Path: <b>AuditEvent.agent.policy</b><br> 4815 * </p> 4816 */ 4817 @SearchParamDefinition(name = "policy", path = "AuditEvent.agent.policy", description = "Policy that authorized event", type = "uri") 4818 public static final String SP_POLICY = "policy"; 4819 /** 4820 * <b>Fluent Client</b> search parameter constant for <b>policy</b> 4821 * <p> 4822 * Description: <b>Policy that authorized event</b><br> 4823 * Type: <b>uri</b><br> 4824 * Path: <b>AuditEvent.agent.policy</b><br> 4825 * </p> 4826 */ 4827 public static final ca.uhn.fhir.rest.gclient.UriClientParam POLICY = new ca.uhn.fhir.rest.gclient.UriClientParam( 4828 SP_POLICY); 4829 4830}