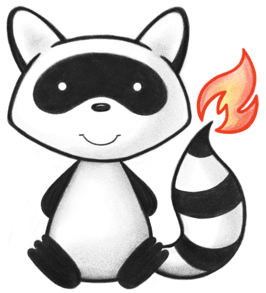
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A record of an event made for purposes of maintaining a security log. Typical 049 * uses include detection of intrusion attempts and monitoring for inappropriate 050 * usage. 051 */ 052@ResourceDef(name = "AuditEvent", profile = "http://hl7.org/fhir/StructureDefinition/AuditEvent") 053public class AuditEvent extends DomainResource { 054 055 public enum AuditEventAction { 056 /** 057 * Create a new database object, such as placing an order. 058 */ 059 C, 060 /** 061 * Display or print data, such as a doctor census. 062 */ 063 R, 064 /** 065 * Update data, such as revise patient information. 066 */ 067 U, 068 /** 069 * Delete items, such as a doctor master file record. 070 */ 071 D, 072 /** 073 * Perform a system or application function such as log-on, program execution or 074 * use of an object's method, or perform a query/search operation. 075 */ 076 E, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static AuditEventAction fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("C".equals(codeString)) 086 return C; 087 if ("R".equals(codeString)) 088 return R; 089 if ("U".equals(codeString)) 090 return U; 091 if ("D".equals(codeString)) 092 return D; 093 if ("E".equals(codeString)) 094 return E; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown AuditEventAction code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case C: 104 return "C"; 105 case R: 106 return "R"; 107 case U: 108 return "U"; 109 case D: 110 return "D"; 111 case E: 112 return "E"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case C: 123 return "http://hl7.org/fhir/audit-event-action"; 124 case R: 125 return "http://hl7.org/fhir/audit-event-action"; 126 case U: 127 return "http://hl7.org/fhir/audit-event-action"; 128 case D: 129 return "http://hl7.org/fhir/audit-event-action"; 130 case E: 131 return "http://hl7.org/fhir/audit-event-action"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case C: 142 return "Create a new database object, such as placing an order."; 143 case R: 144 return "Display or print data, such as a doctor census."; 145 case U: 146 return "Update data, such as revise patient information."; 147 case D: 148 return "Delete items, such as a doctor master file record."; 149 case E: 150 return "Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case C: 161 return "Create"; 162 case R: 163 return "Read/View/Print"; 164 case U: 165 return "Update"; 166 case D: 167 return "Delete"; 168 case E: 169 return "Execute"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class AuditEventActionEnumFactory implements EnumFactory<AuditEventAction> { 179 public AuditEventAction fromCode(String codeString) throws IllegalArgumentException { 180 if (codeString == null || "".equals(codeString)) 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("C".equals(codeString)) 184 return AuditEventAction.C; 185 if ("R".equals(codeString)) 186 return AuditEventAction.R; 187 if ("U".equals(codeString)) 188 return AuditEventAction.U; 189 if ("D".equals(codeString)) 190 return AuditEventAction.D; 191 if ("E".equals(codeString)) 192 return AuditEventAction.E; 193 throw new IllegalArgumentException("Unknown AuditEventAction code '" + codeString + "'"); 194 } 195 196 public Enumeration<AuditEventAction> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<AuditEventAction>(this, AuditEventAction.NULL, code); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<AuditEventAction>(this, AuditEventAction.NULL, code); 204 if ("C".equals(codeString)) 205 return new Enumeration<AuditEventAction>(this, AuditEventAction.C, code); 206 if ("R".equals(codeString)) 207 return new Enumeration<AuditEventAction>(this, AuditEventAction.R, code); 208 if ("U".equals(codeString)) 209 return new Enumeration<AuditEventAction>(this, AuditEventAction.U, code); 210 if ("D".equals(codeString)) 211 return new Enumeration<AuditEventAction>(this, AuditEventAction.D, code); 212 if ("E".equals(codeString)) 213 return new Enumeration<AuditEventAction>(this, AuditEventAction.E, code); 214 throw new FHIRException("Unknown AuditEventAction code '" + codeString + "'"); 215 } 216 217 public String toCode(AuditEventAction code) { 218 if (code == AuditEventAction.NULL) 219 return null; 220 if (code == AuditEventAction.C) 221 return "C"; 222 if (code == AuditEventAction.R) 223 return "R"; 224 if (code == AuditEventAction.U) 225 return "U"; 226 if (code == AuditEventAction.D) 227 return "D"; 228 if (code == AuditEventAction.E) 229 return "E"; 230 return "?"; 231 } 232 233 public String toSystem(AuditEventAction code) { 234 return code.getSystem(); 235 } 236 } 237 238 public enum AuditEventOutcome { 239 /** 240 * The operation completed successfully (whether with warnings or not). 241 */ 242 _0, 243 /** 244 * The action was not successful due to some kind of minor failure (often 245 * equivalent to an HTTP 400 response). 246 */ 247 _4, 248 /** 249 * The action was not successful due to some kind of unexpected error (often 250 * equivalent to an HTTP 500 response). 251 */ 252 _8, 253 /** 254 * An error of such magnitude occurred that the system is no longer available 255 * for use (i.e. the system died). 256 */ 257 _12, 258 /** 259 * added to help the parsers with the generic types 260 */ 261 NULL; 262 263 public static AuditEventOutcome fromCode(String codeString) throws FHIRException { 264 if (codeString == null || "".equals(codeString)) 265 return null; 266 if ("0".equals(codeString)) 267 return _0; 268 if ("4".equals(codeString)) 269 return _4; 270 if ("8".equals(codeString)) 271 return _8; 272 if ("12".equals(codeString)) 273 return _12; 274 if (Configuration.isAcceptInvalidEnums()) 275 return null; 276 else 277 throw new FHIRException("Unknown AuditEventOutcome code '" + codeString + "'"); 278 } 279 280 public String toCode() { 281 switch (this) { 282 case _0: 283 return "0"; 284 case _4: 285 return "4"; 286 case _8: 287 return "8"; 288 case _12: 289 return "12"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 297 public String getSystem() { 298 switch (this) { 299 case _0: 300 return "http://hl7.org/fhir/audit-event-outcome"; 301 case _4: 302 return "http://hl7.org/fhir/audit-event-outcome"; 303 case _8: 304 return "http://hl7.org/fhir/audit-event-outcome"; 305 case _12: 306 return "http://hl7.org/fhir/audit-event-outcome"; 307 case NULL: 308 return null; 309 default: 310 return "?"; 311 } 312 } 313 314 public String getDefinition() { 315 switch (this) { 316 case _0: 317 return "The operation completed successfully (whether with warnings or not)."; 318 case _4: 319 return "The action was not successful due to some kind of minor failure (often equivalent to an HTTP 400 response)."; 320 case _8: 321 return "The action was not successful due to some kind of unexpected error (often equivalent to an HTTP 500 response)."; 322 case _12: 323 return "An error of such magnitude occurred that the system is no longer available for use (i.e. the system died)."; 324 case NULL: 325 return null; 326 default: 327 return "?"; 328 } 329 } 330 331 public String getDisplay() { 332 switch (this) { 333 case _0: 334 return "Success"; 335 case _4: 336 return "Minor failure"; 337 case _8: 338 return "Serious failure"; 339 case _12: 340 return "Major failure"; 341 case NULL: 342 return null; 343 default: 344 return "?"; 345 } 346 } 347 } 348 349 public static class AuditEventOutcomeEnumFactory implements EnumFactory<AuditEventOutcome> { 350 public AuditEventOutcome fromCode(String codeString) throws IllegalArgumentException { 351 if (codeString == null || "".equals(codeString)) 352 if (codeString == null || "".equals(codeString)) 353 return null; 354 if ("0".equals(codeString)) 355 return AuditEventOutcome._0; 356 if ("4".equals(codeString)) 357 return AuditEventOutcome._4; 358 if ("8".equals(codeString)) 359 return AuditEventOutcome._8; 360 if ("12".equals(codeString)) 361 return AuditEventOutcome._12; 362 throw new IllegalArgumentException("Unknown AuditEventOutcome code '" + codeString + "'"); 363 } 364 365 public Enumeration<AuditEventOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 366 if (code == null) 367 return null; 368 if (code.isEmpty()) 369 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome.NULL, code); 370 String codeString = code.asStringValue(); 371 if (codeString == null || "".equals(codeString)) 372 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome.NULL, code); 373 if ("0".equals(codeString)) 374 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._0, code); 375 if ("4".equals(codeString)) 376 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._4, code); 377 if ("8".equals(codeString)) 378 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._8, code); 379 if ("12".equals(codeString)) 380 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._12, code); 381 throw new FHIRException("Unknown AuditEventOutcome code '" + codeString + "'"); 382 } 383 384 public String toCode(AuditEventOutcome code) { 385 if (code == AuditEventOutcome.NULL) 386 return null; 387 if (code == AuditEventOutcome._0) 388 return "0"; 389 if (code == AuditEventOutcome._4) 390 return "4"; 391 if (code == AuditEventOutcome._8) 392 return "8"; 393 if (code == AuditEventOutcome._12) 394 return "12"; 395 return "?"; 396 } 397 398 public String toSystem(AuditEventOutcome code) { 399 return code.getSystem(); 400 } 401 } 402 403 public enum AuditEventAgentNetworkType { 404 /** 405 * The machine name, including DNS name. 406 */ 407 _1, 408 /** 409 * The assigned Internet Protocol (IP) address. 410 */ 411 _2, 412 /** 413 * The assigned telephone number. 414 */ 415 _3, 416 /** 417 * The assigned email address. 418 */ 419 _4, 420 /** 421 * URI (User directory, HTTP-PUT, ftp, etc.). 422 */ 423 _5, 424 /** 425 * added to help the parsers with the generic types 426 */ 427 NULL; 428 429 public static AuditEventAgentNetworkType fromCode(String codeString) throws FHIRException { 430 if (codeString == null || "".equals(codeString)) 431 return null; 432 if ("1".equals(codeString)) 433 return _1; 434 if ("2".equals(codeString)) 435 return _2; 436 if ("3".equals(codeString)) 437 return _3; 438 if ("4".equals(codeString)) 439 return _4; 440 if ("5".equals(codeString)) 441 return _5; 442 if (Configuration.isAcceptInvalidEnums()) 443 return null; 444 else 445 throw new FHIRException("Unknown AuditEventAgentNetworkType code '" + codeString + "'"); 446 } 447 448 public String toCode() { 449 switch (this) { 450 case _1: 451 return "1"; 452 case _2: 453 return "2"; 454 case _3: 455 return "3"; 456 case _4: 457 return "4"; 458 case _5: 459 return "5"; 460 case NULL: 461 return null; 462 default: 463 return "?"; 464 } 465 } 466 467 public String getSystem() { 468 switch (this) { 469 case _1: 470 return "http://hl7.org/fhir/network-type"; 471 case _2: 472 return "http://hl7.org/fhir/network-type"; 473 case _3: 474 return "http://hl7.org/fhir/network-type"; 475 case _4: 476 return "http://hl7.org/fhir/network-type"; 477 case _5: 478 return "http://hl7.org/fhir/network-type"; 479 case NULL: 480 return null; 481 default: 482 return "?"; 483 } 484 } 485 486 public String getDefinition() { 487 switch (this) { 488 case _1: 489 return "The machine name, including DNS name."; 490 case _2: 491 return "The assigned Internet Protocol (IP) address."; 492 case _3: 493 return "The assigned telephone number."; 494 case _4: 495 return "The assigned email address."; 496 case _5: 497 return "URI (User directory, HTTP-PUT, ftp, etc.)."; 498 case NULL: 499 return null; 500 default: 501 return "?"; 502 } 503 } 504 505 public String getDisplay() { 506 switch (this) { 507 case _1: 508 return "Machine Name"; 509 case _2: 510 return "IP Address"; 511 case _3: 512 return "Telephone Number"; 513 case _4: 514 return "Email address"; 515 case _5: 516 return "URI"; 517 case NULL: 518 return null; 519 default: 520 return "?"; 521 } 522 } 523 } 524 525 public static class AuditEventAgentNetworkTypeEnumFactory implements EnumFactory<AuditEventAgentNetworkType> { 526 public AuditEventAgentNetworkType fromCode(String codeString) throws IllegalArgumentException { 527 if (codeString == null || "".equals(codeString)) 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("1".equals(codeString)) 531 return AuditEventAgentNetworkType._1; 532 if ("2".equals(codeString)) 533 return AuditEventAgentNetworkType._2; 534 if ("3".equals(codeString)) 535 return AuditEventAgentNetworkType._3; 536 if ("4".equals(codeString)) 537 return AuditEventAgentNetworkType._4; 538 if ("5".equals(codeString)) 539 return AuditEventAgentNetworkType._5; 540 throw new IllegalArgumentException("Unknown AuditEventAgentNetworkType code '" + codeString + "'"); 541 } 542 543 public Enumeration<AuditEventAgentNetworkType> fromType(PrimitiveType<?> code) throws FHIRException { 544 if (code == null) 545 return null; 546 if (code.isEmpty()) 547 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType.NULL, code); 548 String codeString = code.asStringValue(); 549 if (codeString == null || "".equals(codeString)) 550 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType.NULL, code); 551 if ("1".equals(codeString)) 552 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._1, code); 553 if ("2".equals(codeString)) 554 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._2, code); 555 if ("3".equals(codeString)) 556 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._3, code); 557 if ("4".equals(codeString)) 558 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._4, code); 559 if ("5".equals(codeString)) 560 return new Enumeration<AuditEventAgentNetworkType>(this, AuditEventAgentNetworkType._5, code); 561 throw new FHIRException("Unknown AuditEventAgentNetworkType code '" + codeString + "'"); 562 } 563 564 public String toCode(AuditEventAgentNetworkType code) { 565 if (code == AuditEventAgentNetworkType.NULL) 566 return null; 567 if (code == AuditEventAgentNetworkType._1) 568 return "1"; 569 if (code == AuditEventAgentNetworkType._2) 570 return "2"; 571 if (code == AuditEventAgentNetworkType._3) 572 return "3"; 573 if (code == AuditEventAgentNetworkType._4) 574 return "4"; 575 if (code == AuditEventAgentNetworkType._5) 576 return "5"; 577 return "?"; 578 } 579 580 public String toSystem(AuditEventAgentNetworkType code) { 581 return code.getSystem(); 582 } 583 } 584 585 @Block() 586 public static class AuditEventAgentComponent extends BackboneElement implements IBaseBackboneElement { 587 /** 588 * Specification of the participation type the user plays when performing the 589 * event. 590 */ 591 @Child(name = "type", type = { 592 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 593 @Description(shortDefinition = "How agent participated", formalDefinition = "Specification of the participation type the user plays when performing the event.") 594 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participation-role-type") 595 protected CodeableConcept type; 596 597 /** 598 * The security role that the user was acting under, that come from local codes 599 * defined by the access control security system (e.g. RBAC, ABAC) used in the 600 * local context. 601 */ 602 @Child(name = "role", type = { 603 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 604 @Description(shortDefinition = "Agent role in the event", formalDefinition = "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.") 605 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-role-type") 606 protected List<CodeableConcept> role; 607 608 /** 609 * Reference to who this agent is that was involved in the event. 610 */ 611 @Child(name = "who", type = { PractitionerRole.class, Practitioner.class, Organization.class, Device.class, 612 Patient.class, RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 613 @Description(shortDefinition = "Identifier of who", formalDefinition = "Reference to who this agent is that was involved in the event.") 614 protected Reference who; 615 616 /** 617 * The actual object that is the target of the reference (Reference to who this 618 * agent is that was involved in the event.) 619 */ 620 protected Resource whoTarget; 621 622 /** 623 * Alternative agent Identifier. For a human, this should be a user identifier 624 * text string from authentication system. This identifier would be one known to 625 * a common authentication system (e.g. single sign-on), if available. 626 */ 627 @Child(name = "altId", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 628 @Description(shortDefinition = "Alternative User identity", formalDefinition = "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.") 629 protected StringType altId; 630 631 /** 632 * Human-meaningful name for the agent. 633 */ 634 @Child(name = "name", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 635 @Description(shortDefinition = "Human friendly name for the agent", formalDefinition = "Human-meaningful name for the agent.") 636 protected StringType name; 637 638 /** 639 * Indicator that the user is or is not the requestor, or initiator, for the 640 * event being audited. 641 */ 642 @Child(name = "requestor", type = { 643 BooleanType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 644 @Description(shortDefinition = "Whether user is initiator", formalDefinition = "Indicator that the user is or is not the requestor, or initiator, for the event being audited.") 645 protected BooleanType requestor; 646 647 /** 648 * Where the event occurred. 649 */ 650 @Child(name = "location", type = { Location.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 651 @Description(shortDefinition = "Where", formalDefinition = "Where the event occurred.") 652 protected Reference location; 653 654 /** 655 * The actual object that is the target of the reference (Where the event 656 * occurred.) 657 */ 658 protected Location locationTarget; 659 660 /** 661 * The policy or plan that authorized the activity being recorded. Typically, a 662 * single activity may have multiple applicable policies, such as patient 663 * consent, guarantor funding, etc. The policy would also indicate the security 664 * token used. 665 */ 666 @Child(name = "policy", type = { 667 UriType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 668 @Description(shortDefinition = "Policy that authorized event", formalDefinition = "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.") 669 protected List<UriType> policy; 670 671 /** 672 * Type of media involved. Used when the event is about exporting/importing onto 673 * media. 674 */ 675 @Child(name = "media", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 676 @Description(shortDefinition = "Type of media", formalDefinition = "Type of media involved. Used when the event is about exporting/importing onto media.") 677 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/dicm-405-mediatype") 678 protected Coding media; 679 680 /** 681 * Logical network location for application activity, if the activity has a 682 * network location. 683 */ 684 @Child(name = "network", type = {}, order = 10, min = 0, max = 1, modifier = false, summary = false) 685 @Description(shortDefinition = "Logical network location for application activity", formalDefinition = "Logical network location for application activity, if the activity has a network location.") 686 protected AuditEventAgentNetworkComponent network; 687 688 /** 689 * The reason (purpose of use), specific to this agent, that was used during the 690 * event being recorded. 691 */ 692 @Child(name = "purposeOfUse", type = { 693 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 694 @Description(shortDefinition = "Reason given for this user", formalDefinition = "The reason (purpose of use), specific to this agent, that was used during the event being recorded.") 695 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 696 protected List<CodeableConcept> purposeOfUse; 697 698 private static final long serialVersionUID = -957410638L; 699 700 /** 701 * Constructor 702 */ 703 public AuditEventAgentComponent() { 704 super(); 705 } 706 707 /** 708 * Constructor 709 */ 710 public AuditEventAgentComponent(BooleanType requestor) { 711 super(); 712 this.requestor = requestor; 713 } 714 715 /** 716 * @return {@link #type} (Specification of the participation type the user plays 717 * when performing the event.) 718 */ 719 public CodeableConcept getType() { 720 if (this.type == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create AuditEventAgentComponent.type"); 723 else if (Configuration.doAutoCreate()) 724 this.type = new CodeableConcept(); // cc 725 return this.type; 726 } 727 728 public boolean hasType() { 729 return this.type != null && !this.type.isEmpty(); 730 } 731 732 /** 733 * @param value {@link #type} (Specification of the participation type the user 734 * plays when performing the event.) 735 */ 736 public AuditEventAgentComponent setType(CodeableConcept value) { 737 this.type = value; 738 return this; 739 } 740 741 /** 742 * @return {@link #role} (The security role that the user was acting under, that 743 * come from local codes defined by the access control security system 744 * (e.g. RBAC, ABAC) used in the local context.) 745 */ 746 public List<CodeableConcept> getRole() { 747 if (this.role == null) 748 this.role = new ArrayList<CodeableConcept>(); 749 return this.role; 750 } 751 752 /** 753 * @return Returns a reference to <code>this</code> for easy method chaining 754 */ 755 public AuditEventAgentComponent setRole(List<CodeableConcept> theRole) { 756 this.role = theRole; 757 return this; 758 } 759 760 public boolean hasRole() { 761 if (this.role == null) 762 return false; 763 for (CodeableConcept item : this.role) 764 if (!item.isEmpty()) 765 return true; 766 return false; 767 } 768 769 public CodeableConcept addRole() { // 3 770 CodeableConcept t = new CodeableConcept(); 771 if (this.role == null) 772 this.role = new ArrayList<CodeableConcept>(); 773 this.role.add(t); 774 return t; 775 } 776 777 public AuditEventAgentComponent addRole(CodeableConcept t) { // 3 778 if (t == null) 779 return this; 780 if (this.role == null) 781 this.role = new ArrayList<CodeableConcept>(); 782 this.role.add(t); 783 return this; 784 } 785 786 /** 787 * @return The first repetition of repeating field {@link #role}, creating it if 788 * it does not already exist 789 */ 790 public CodeableConcept getRoleFirstRep() { 791 if (getRole().isEmpty()) { 792 addRole(); 793 } 794 return getRole().get(0); 795 } 796 797 /** 798 * @return {@link #who} (Reference to who this agent is that was involved in the 799 * event.) 800 */ 801 public Reference getWho() { 802 if (this.who == null) 803 if (Configuration.errorOnAutoCreate()) 804 throw new Error("Attempt to auto-create AuditEventAgentComponent.who"); 805 else if (Configuration.doAutoCreate()) 806 this.who = new Reference(); // cc 807 return this.who; 808 } 809 810 public boolean hasWho() { 811 return this.who != null && !this.who.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #who} (Reference to who this agent is that was involved 816 * in the event.) 817 */ 818 public AuditEventAgentComponent setWho(Reference value) { 819 this.who = value; 820 return this; 821 } 822 823 /** 824 * @return {@link #who} The actual object that is the target of the reference. 825 * The reference library doesn't populate this, but you can use it to 826 * hold the resource if you resolve it. (Reference to who this agent is 827 * that was involved in the event.) 828 */ 829 public Resource getWhoTarget() { 830 return this.whoTarget; 831 } 832 833 /** 834 * @param value {@link #who} The actual object that is the target of the 835 * reference. The reference library doesn't use these, but you can 836 * use it to hold the resource if you resolve it. (Reference to who 837 * this agent is that was involved in the event.) 838 */ 839 public AuditEventAgentComponent setWhoTarget(Resource value) { 840 this.whoTarget = value; 841 return this; 842 } 843 844 /** 845 * @return {@link #altId} (Alternative agent Identifier. For a human, this 846 * should be a user identifier text string from authentication system. 847 * This identifier would be one known to a common authentication system 848 * (e.g. single sign-on), if available.). This is the underlying object 849 * with id, value and extensions. The accessor "getAltId" gives direct 850 * access to the value 851 */ 852 public StringType getAltIdElement() { 853 if (this.altId == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create AuditEventAgentComponent.altId"); 856 else if (Configuration.doAutoCreate()) 857 this.altId = new StringType(); // bb 858 return this.altId; 859 } 860 861 public boolean hasAltIdElement() { 862 return this.altId != null && !this.altId.isEmpty(); 863 } 864 865 public boolean hasAltId() { 866 return this.altId != null && !this.altId.isEmpty(); 867 } 868 869 /** 870 * @param value {@link #altId} (Alternative agent Identifier. For a human, this 871 * should be a user identifier text string from authentication 872 * system. This identifier would be one known to a common 873 * authentication system (e.g. single sign-on), if available.). 874 * This is the underlying object with id, value and extensions. The 875 * accessor "getAltId" gives direct access to the value 876 */ 877 public AuditEventAgentComponent setAltIdElement(StringType value) { 878 this.altId = value; 879 return this; 880 } 881 882 /** 883 * @return Alternative agent Identifier. For a human, this should be a user 884 * identifier text string from authentication system. This identifier 885 * would be one known to a common authentication system (e.g. single 886 * sign-on), if available. 887 */ 888 public String getAltId() { 889 return this.altId == null ? null : this.altId.getValue(); 890 } 891 892 /** 893 * @param value Alternative agent Identifier. For a human, this should be a user 894 * identifier text string from authentication system. This 895 * identifier would be one known to a common authentication system 896 * (e.g. single sign-on), if available. 897 */ 898 public AuditEventAgentComponent setAltId(String value) { 899 if (Utilities.noString(value)) 900 this.altId = null; 901 else { 902 if (this.altId == null) 903 this.altId = new StringType(); 904 this.altId.setValue(value); 905 } 906 return this; 907 } 908 909 /** 910 * @return {@link #name} (Human-meaningful name for the agent.). This is the 911 * underlying object with id, value and extensions. The accessor 912 * "getName" gives direct access to the value 913 */ 914 public StringType getNameElement() { 915 if (this.name == null) 916 if (Configuration.errorOnAutoCreate()) 917 throw new Error("Attempt to auto-create AuditEventAgentComponent.name"); 918 else if (Configuration.doAutoCreate()) 919 this.name = new StringType(); // bb 920 return this.name; 921 } 922 923 public boolean hasNameElement() { 924 return this.name != null && !this.name.isEmpty(); 925 } 926 927 public boolean hasName() { 928 return this.name != null && !this.name.isEmpty(); 929 } 930 931 /** 932 * @param value {@link #name} (Human-meaningful name for the agent.). This is 933 * the underlying object with id, value and extensions. The 934 * accessor "getName" gives direct access to the value 935 */ 936 public AuditEventAgentComponent setNameElement(StringType value) { 937 this.name = value; 938 return this; 939 } 940 941 /** 942 * @return Human-meaningful name for the agent. 943 */ 944 public String getName() { 945 return this.name == null ? null : this.name.getValue(); 946 } 947 948 /** 949 * @param value Human-meaningful name for the agent. 950 */ 951 public AuditEventAgentComponent setName(String value) { 952 if (Utilities.noString(value)) 953 this.name = null; 954 else { 955 if (this.name == null) 956 this.name = new StringType(); 957 this.name.setValue(value); 958 } 959 return this; 960 } 961 962 /** 963 * @return {@link #requestor} (Indicator that the user is or is not the 964 * requestor, or initiator, for the event being audited.). This is the 965 * underlying object with id, value and extensions. The accessor 966 * "getRequestor" gives direct access to the value 967 */ 968 public BooleanType getRequestorElement() { 969 if (this.requestor == null) 970 if (Configuration.errorOnAutoCreate()) 971 throw new Error("Attempt to auto-create AuditEventAgentComponent.requestor"); 972 else if (Configuration.doAutoCreate()) 973 this.requestor = new BooleanType(); // bb 974 return this.requestor; 975 } 976 977 public boolean hasRequestorElement() { 978 return this.requestor != null && !this.requestor.isEmpty(); 979 } 980 981 public boolean hasRequestor() { 982 return this.requestor != null && !this.requestor.isEmpty(); 983 } 984 985 /** 986 * @param value {@link #requestor} (Indicator that the user is or is not the 987 * requestor, or initiator, for the event being audited.). This is 988 * the underlying object with id, value and extensions. The 989 * accessor "getRequestor" gives direct access to the value 990 */ 991 public AuditEventAgentComponent setRequestorElement(BooleanType value) { 992 this.requestor = value; 993 return this; 994 } 995 996 /** 997 * @return Indicator that the user is or is not the requestor, or initiator, for 998 * the event being audited. 999 */ 1000 public boolean getRequestor() { 1001 return this.requestor == null || this.requestor.isEmpty() ? false : this.requestor.getValue(); 1002 } 1003 1004 /** 1005 * @param value Indicator that the user is or is not the requestor, or 1006 * initiator, for the event being audited. 1007 */ 1008 public AuditEventAgentComponent setRequestor(boolean value) { 1009 if (this.requestor == null) 1010 this.requestor = new BooleanType(); 1011 this.requestor.setValue(value); 1012 return this; 1013 } 1014 1015 /** 1016 * @return {@link #location} (Where the event occurred.) 1017 */ 1018 public Reference getLocation() { 1019 if (this.location == null) 1020 if (Configuration.errorOnAutoCreate()) 1021 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 1022 else if (Configuration.doAutoCreate()) 1023 this.location = new Reference(); // cc 1024 return this.location; 1025 } 1026 1027 public boolean hasLocation() { 1028 return this.location != null && !this.location.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #location} (Where the event occurred.) 1033 */ 1034 public AuditEventAgentComponent setLocation(Reference value) { 1035 this.location = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return {@link #location} The actual object that is the target of the 1041 * reference. The reference library doesn't populate this, but you can 1042 * use it to hold the resource if you resolve it. (Where the event 1043 * occurred.) 1044 */ 1045 public Location getLocationTarget() { 1046 if (this.locationTarget == null) 1047 if (Configuration.errorOnAutoCreate()) 1048 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 1049 else if (Configuration.doAutoCreate()) 1050 this.locationTarget = new Location(); // aa 1051 return this.locationTarget; 1052 } 1053 1054 /** 1055 * @param value {@link #location} The actual object that is the target of the 1056 * reference. The reference library doesn't use these, but you can 1057 * use it to hold the resource if you resolve it. (Where the event 1058 * occurred.) 1059 */ 1060 public AuditEventAgentComponent setLocationTarget(Location value) { 1061 this.locationTarget = value; 1062 return this; 1063 } 1064 1065 /** 1066 * @return {@link #policy} (The policy or plan that authorized the activity 1067 * being recorded. Typically, a single activity may have multiple 1068 * applicable policies, such as patient consent, guarantor funding, etc. 1069 * The policy would also indicate the security token used.) 1070 */ 1071 public List<UriType> getPolicy() { 1072 if (this.policy == null) 1073 this.policy = new ArrayList<UriType>(); 1074 return this.policy; 1075 } 1076 1077 /** 1078 * @return Returns a reference to <code>this</code> for easy method chaining 1079 */ 1080 public AuditEventAgentComponent setPolicy(List<UriType> thePolicy) { 1081 this.policy = thePolicy; 1082 return this; 1083 } 1084 1085 public boolean hasPolicy() { 1086 if (this.policy == null) 1087 return false; 1088 for (UriType item : this.policy) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 /** 1095 * @return {@link #policy} (The policy or plan that authorized the activity 1096 * being recorded. Typically, a single activity may have multiple 1097 * applicable policies, such as patient consent, guarantor funding, etc. 1098 * The policy would also indicate the security token used.) 1099 */ 1100 public UriType addPolicyElement() {// 2 1101 UriType t = new UriType(); 1102 if (this.policy == null) 1103 this.policy = new ArrayList<UriType>(); 1104 this.policy.add(t); 1105 return t; 1106 } 1107 1108 /** 1109 * @param value {@link #policy} (The policy or plan that authorized the activity 1110 * being recorded. Typically, a single activity may have multiple 1111 * applicable policies, such as patient consent, guarantor funding, 1112 * etc. The policy would also indicate the security token used.) 1113 */ 1114 public AuditEventAgentComponent addPolicy(String value) { // 1 1115 UriType t = new UriType(); 1116 t.setValue(value); 1117 if (this.policy == null) 1118 this.policy = new ArrayList<UriType>(); 1119 this.policy.add(t); 1120 return this; 1121 } 1122 1123 /** 1124 * @param value {@link #policy} (The policy or plan that authorized the activity 1125 * being recorded. Typically, a single activity may have multiple 1126 * applicable policies, such as patient consent, guarantor funding, 1127 * etc. The policy would also indicate the security token used.) 1128 */ 1129 public boolean hasPolicy(String value) { 1130 if (this.policy == null) 1131 return false; 1132 for (UriType v : this.policy) 1133 if (v.getValue().equals(value)) // uri 1134 return true; 1135 return false; 1136 } 1137 1138 /** 1139 * @return {@link #media} (Type of media involved. Used when the event is about 1140 * exporting/importing onto media.) 1141 */ 1142 public Coding getMedia() { 1143 if (this.media == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create AuditEventAgentComponent.media"); 1146 else if (Configuration.doAutoCreate()) 1147 this.media = new Coding(); // cc 1148 return this.media; 1149 } 1150 1151 public boolean hasMedia() { 1152 return this.media != null && !this.media.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #media} (Type of media involved. Used when the event is 1157 * about exporting/importing onto media.) 1158 */ 1159 public AuditEventAgentComponent setMedia(Coding value) { 1160 this.media = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return {@link #network} (Logical network location for application activity, 1166 * if the activity has a network location.) 1167 */ 1168 public AuditEventAgentNetworkComponent getNetwork() { 1169 if (this.network == null) 1170 if (Configuration.errorOnAutoCreate()) 1171 throw new Error("Attempt to auto-create AuditEventAgentComponent.network"); 1172 else if (Configuration.doAutoCreate()) 1173 this.network = new AuditEventAgentNetworkComponent(); // cc 1174 return this.network; 1175 } 1176 1177 public boolean hasNetwork() { 1178 return this.network != null && !this.network.isEmpty(); 1179 } 1180 1181 /** 1182 * @param value {@link #network} (Logical network location for application 1183 * activity, if the activity has a network location.) 1184 */ 1185 public AuditEventAgentComponent setNetwork(AuditEventAgentNetworkComponent value) { 1186 this.network = value; 1187 return this; 1188 } 1189 1190 /** 1191 * @return {@link #purposeOfUse} (The reason (purpose of use), specific to this 1192 * agent, that was used during the event being recorded.) 1193 */ 1194 public List<CodeableConcept> getPurposeOfUse() { 1195 if (this.purposeOfUse == null) 1196 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1197 return this.purposeOfUse; 1198 } 1199 1200 /** 1201 * @return Returns a reference to <code>this</code> for easy method chaining 1202 */ 1203 public AuditEventAgentComponent setPurposeOfUse(List<CodeableConcept> thePurposeOfUse) { 1204 this.purposeOfUse = thePurposeOfUse; 1205 return this; 1206 } 1207 1208 public boolean hasPurposeOfUse() { 1209 if (this.purposeOfUse == null) 1210 return false; 1211 for (CodeableConcept item : this.purposeOfUse) 1212 if (!item.isEmpty()) 1213 return true; 1214 return false; 1215 } 1216 1217 public CodeableConcept addPurposeOfUse() { // 3 1218 CodeableConcept t = new CodeableConcept(); 1219 if (this.purposeOfUse == null) 1220 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1221 this.purposeOfUse.add(t); 1222 return t; 1223 } 1224 1225 public AuditEventAgentComponent addPurposeOfUse(CodeableConcept t) { // 3 1226 if (t == null) 1227 return this; 1228 if (this.purposeOfUse == null) 1229 this.purposeOfUse = new ArrayList<CodeableConcept>(); 1230 this.purposeOfUse.add(t); 1231 return this; 1232 } 1233 1234 /** 1235 * @return The first repetition of repeating field {@link #purposeOfUse}, 1236 * creating it if it does not already exist 1237 */ 1238 public CodeableConcept getPurposeOfUseFirstRep() { 1239 if (getPurposeOfUse().isEmpty()) { 1240 addPurposeOfUse(); 1241 } 1242 return getPurposeOfUse().get(0); 1243 } 1244 1245 protected void listChildren(List<Property> children) { 1246 super.listChildren(children); 1247 children.add(new Property("type", "CodeableConcept", 1248 "Specification of the participation type the user plays when performing the event.", 0, 1, type)); 1249 children.add(new Property("role", "CodeableConcept", 1250 "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.", 1251 0, java.lang.Integer.MAX_VALUE, role)); 1252 children 1253 .add(new Property("who", "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 1254 "Reference to who this agent is that was involved in the event.", 0, 1, who)); 1255 children.add(new Property("altId", "string", 1256 "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 1257 0, 1, altId)); 1258 children.add(new Property("name", "string", "Human-meaningful name for the agent.", 0, 1, name)); 1259 children.add(new Property("requestor", "boolean", 1260 "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, 1261 requestor)); 1262 children.add(new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1, location)); 1263 children.add(new Property("policy", "uri", 1264 "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 1265 0, java.lang.Integer.MAX_VALUE, policy)); 1266 children.add(new Property("media", "Coding", 1267 "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1, media)); 1268 children.add(new Property("network", "", 1269 "Logical network location for application activity, if the activity has a network location.", 0, 1, network)); 1270 children.add(new Property("purposeOfUse", "CodeableConcept", 1271 "The reason (purpose of use), specific to this agent, that was used during the event being recorded.", 0, 1272 java.lang.Integer.MAX_VALUE, purposeOfUse)); 1273 } 1274 1275 @Override 1276 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1277 switch (_hash) { 1278 case 3575610: 1279 /* type */ return new Property("type", "CodeableConcept", 1280 "Specification of the participation type the user plays when performing the event.", 0, 1, type); 1281 case 3506294: 1282 /* role */ return new Property("role", "CodeableConcept", 1283 "The security role that the user was acting under, that come from local codes defined by the access control security system (e.g. RBAC, ABAC) used in the local context.", 1284 0, java.lang.Integer.MAX_VALUE, role); 1285 case 117694: 1286 /* who */ return new Property("who", 1287 "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 1288 "Reference to who this agent is that was involved in the event.", 0, 1, who); 1289 case 92912804: 1290 /* altId */ return new Property("altId", "string", 1291 "Alternative agent Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 1292 0, 1, altId); 1293 case 3373707: 1294 /* name */ return new Property("name", "string", "Human-meaningful name for the agent.", 0, 1, name); 1295 case 693934258: 1296 /* requestor */ return new Property("requestor", "boolean", 1297 "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, 1298 requestor); 1299 case 1901043637: 1300 /* location */ return new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1, 1301 location); 1302 case -982670030: 1303 /* policy */ return new Property("policy", "uri", 1304 "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 1305 0, java.lang.Integer.MAX_VALUE, policy); 1306 case 103772132: 1307 /* media */ return new Property("media", "Coding", 1308 "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1, media); 1309 case 1843485230: 1310 /* network */ return new Property("network", "", 1311 "Logical network location for application activity, if the activity has a network location.", 0, 1, 1312 network); 1313 case -1881902670: 1314 /* purposeOfUse */ return new Property("purposeOfUse", "CodeableConcept", 1315 "The reason (purpose of use), specific to this agent, that was used during the event being recorded.", 0, 1316 java.lang.Integer.MAX_VALUE, purposeOfUse); 1317 default: 1318 return super.getNamedProperty(_hash, _name, _checkValid); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1325 switch (hash) { 1326 case 3575610: 1327 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1328 case 3506294: 1329 /* role */ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 1330 case 117694: 1331 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 1332 case 92912804: 1333 /* altId */ return this.altId == null ? new Base[0] : new Base[] { this.altId }; // StringType 1334 case 3373707: 1335 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1336 case 693934258: 1337 /* requestor */ return this.requestor == null ? new Base[0] : new Base[] { this.requestor }; // BooleanType 1338 case 1901043637: 1339 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 1340 case -982670030: 1341 /* policy */ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1342 case 103772132: 1343 /* media */ return this.media == null ? new Base[0] : new Base[] { this.media }; // Coding 1344 case 1843485230: 1345 /* network */ return this.network == null ? new Base[0] : new Base[] { this.network }; // AuditEventAgentNetworkComponent 1346 case -1881902670: 1347 /* purposeOfUse */ return this.purposeOfUse == null ? new Base[0] 1348 : this.purposeOfUse.toArray(new Base[this.purposeOfUse.size()]); // CodeableConcept 1349 default: 1350 return super.getProperty(hash, name, checkValid); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1357 switch (hash) { 1358 case 3575610: // type 1359 this.type = castToCodeableConcept(value); // CodeableConcept 1360 return value; 1361 case 3506294: // role 1362 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 1363 return value; 1364 case 117694: // who 1365 this.who = castToReference(value); // Reference 1366 return value; 1367 case 92912804: // altId 1368 this.altId = castToString(value); // StringType 1369 return value; 1370 case 3373707: // name 1371 this.name = castToString(value); // StringType 1372 return value; 1373 case 693934258: // requestor 1374 this.requestor = castToBoolean(value); // BooleanType 1375 return value; 1376 case 1901043637: // location 1377 this.location = castToReference(value); // Reference 1378 return value; 1379 case -982670030: // policy 1380 this.getPolicy().add(castToUri(value)); // UriType 1381 return value; 1382 case 103772132: // media 1383 this.media = castToCoding(value); // Coding 1384 return value; 1385 case 1843485230: // network 1386 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1387 return value; 1388 case -1881902670: // purposeOfUse 1389 this.getPurposeOfUse().add(castToCodeableConcept(value)); // CodeableConcept 1390 return value; 1391 default: 1392 return super.setProperty(hash, name, value); 1393 } 1394 1395 } 1396 1397 @Override 1398 public Base setProperty(String name, Base value) throws FHIRException { 1399 if (name.equals("type")) { 1400 this.type = castToCodeableConcept(value); // CodeableConcept 1401 } else if (name.equals("role")) { 1402 this.getRole().add(castToCodeableConcept(value)); 1403 } else if (name.equals("who")) { 1404 this.who = castToReference(value); // Reference 1405 } else if (name.equals("altId")) { 1406 this.altId = castToString(value); // StringType 1407 } else if (name.equals("name")) { 1408 this.name = castToString(value); // StringType 1409 } else if (name.equals("requestor")) { 1410 this.requestor = castToBoolean(value); // BooleanType 1411 } else if (name.equals("location")) { 1412 this.location = castToReference(value); // Reference 1413 } else if (name.equals("policy")) { 1414 this.getPolicy().add(castToUri(value)); 1415 } else if (name.equals("media")) { 1416 this.media = castToCoding(value); // Coding 1417 } else if (name.equals("network")) { 1418 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1419 } else if (name.equals("purposeOfUse")) { 1420 this.getPurposeOfUse().add(castToCodeableConcept(value)); 1421 } else 1422 return super.setProperty(name, value); 1423 return value; 1424 } 1425 1426 @Override 1427 public void removeChild(String name, Base value) throws FHIRException { 1428 if (name.equals("type")) { 1429 this.type = null; 1430 } else if (name.equals("role")) { 1431 this.getRole().remove(castToCodeableConcept(value)); 1432 } else if (name.equals("who")) { 1433 this.who = null; 1434 } else if (name.equals("altId")) { 1435 this.altId = null; 1436 } else if (name.equals("name")) { 1437 this.name = null; 1438 } else if (name.equals("requestor")) { 1439 this.requestor = null; 1440 } else if (name.equals("location")) { 1441 this.location = null; 1442 } else if (name.equals("policy")) { 1443 this.getPolicy().remove(castToUri(value)); 1444 } else if (name.equals("media")) { 1445 this.media = null; 1446 } else if (name.equals("network")) { 1447 this.network = (AuditEventAgentNetworkComponent) value; // AuditEventAgentNetworkComponent 1448 } else if (name.equals("purposeOfUse")) { 1449 this.getPurposeOfUse().remove(castToCodeableConcept(value)); 1450 } else 1451 super.removeChild(name, value); 1452 1453 } 1454 1455 @Override 1456 public Base makeProperty(int hash, String name) throws FHIRException { 1457 switch (hash) { 1458 case 3575610: 1459 return getType(); 1460 case 3506294: 1461 return addRole(); 1462 case 117694: 1463 return getWho(); 1464 case 92912804: 1465 return getAltIdElement(); 1466 case 3373707: 1467 return getNameElement(); 1468 case 693934258: 1469 return getRequestorElement(); 1470 case 1901043637: 1471 return getLocation(); 1472 case -982670030: 1473 return addPolicyElement(); 1474 case 103772132: 1475 return getMedia(); 1476 case 1843485230: 1477 return getNetwork(); 1478 case -1881902670: 1479 return addPurposeOfUse(); 1480 default: 1481 return super.makeProperty(hash, name); 1482 } 1483 1484 } 1485 1486 @Override 1487 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1488 switch (hash) { 1489 case 3575610: 1490 /* type */ return new String[] { "CodeableConcept" }; 1491 case 3506294: 1492 /* role */ return new String[] { "CodeableConcept" }; 1493 case 117694: 1494 /* who */ return new String[] { "Reference" }; 1495 case 92912804: 1496 /* altId */ return new String[] { "string" }; 1497 case 3373707: 1498 /* name */ return new String[] { "string" }; 1499 case 693934258: 1500 /* requestor */ return new String[] { "boolean" }; 1501 case 1901043637: 1502 /* location */ return new String[] { "Reference" }; 1503 case -982670030: 1504 /* policy */ return new String[] { "uri" }; 1505 case 103772132: 1506 /* media */ return new String[] { "Coding" }; 1507 case 1843485230: 1508 /* network */ return new String[] {}; 1509 case -1881902670: 1510 /* purposeOfUse */ return new String[] { "CodeableConcept" }; 1511 default: 1512 return super.getTypesForProperty(hash, name); 1513 } 1514 1515 } 1516 1517 @Override 1518 public Base addChild(String name) throws FHIRException { 1519 if (name.equals("type")) { 1520 this.type = new CodeableConcept(); 1521 return this.type; 1522 } else if (name.equals("role")) { 1523 return addRole(); 1524 } else if (name.equals("who")) { 1525 this.who = new Reference(); 1526 return this.who; 1527 } else if (name.equals("altId")) { 1528 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.altId"); 1529 } else if (name.equals("name")) { 1530 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 1531 } else if (name.equals("requestor")) { 1532 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.requestor"); 1533 } else if (name.equals("location")) { 1534 this.location = new Reference(); 1535 return this.location; 1536 } else if (name.equals("policy")) { 1537 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.policy"); 1538 } else if (name.equals("media")) { 1539 this.media = new Coding(); 1540 return this.media; 1541 } else if (name.equals("network")) { 1542 this.network = new AuditEventAgentNetworkComponent(); 1543 return this.network; 1544 } else if (name.equals("purposeOfUse")) { 1545 return addPurposeOfUse(); 1546 } else 1547 return super.addChild(name); 1548 } 1549 1550 public AuditEventAgentComponent copy() { 1551 AuditEventAgentComponent dst = new AuditEventAgentComponent(); 1552 copyValues(dst); 1553 return dst; 1554 } 1555 1556 public void copyValues(AuditEventAgentComponent dst) { 1557 super.copyValues(dst); 1558 dst.type = type == null ? null : type.copy(); 1559 if (role != null) { 1560 dst.role = new ArrayList<CodeableConcept>(); 1561 for (CodeableConcept i : role) 1562 dst.role.add(i.copy()); 1563 } 1564 ; 1565 dst.who = who == null ? null : who.copy(); 1566 dst.altId = altId == null ? null : altId.copy(); 1567 dst.name = name == null ? null : name.copy(); 1568 dst.requestor = requestor == null ? null : requestor.copy(); 1569 dst.location = location == null ? null : location.copy(); 1570 if (policy != null) { 1571 dst.policy = new ArrayList<UriType>(); 1572 for (UriType i : policy) 1573 dst.policy.add(i.copy()); 1574 } 1575 ; 1576 dst.media = media == null ? null : media.copy(); 1577 dst.network = network == null ? null : network.copy(); 1578 if (purposeOfUse != null) { 1579 dst.purposeOfUse = new ArrayList<CodeableConcept>(); 1580 for (CodeableConcept i : purposeOfUse) 1581 dst.purposeOfUse.add(i.copy()); 1582 } 1583 ; 1584 } 1585 1586 @Override 1587 public boolean equalsDeep(Base other_) { 1588 if (!super.equalsDeep(other_)) 1589 return false; 1590 if (!(other_ instanceof AuditEventAgentComponent)) 1591 return false; 1592 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1593 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true) && compareDeep(who, o.who, true) 1594 && compareDeep(altId, o.altId, true) && compareDeep(name, o.name, true) 1595 && compareDeep(requestor, o.requestor, true) && compareDeep(location, o.location, true) 1596 && compareDeep(policy, o.policy, true) && compareDeep(media, o.media, true) 1597 && compareDeep(network, o.network, true) && compareDeep(purposeOfUse, o.purposeOfUse, true); 1598 } 1599 1600 @Override 1601 public boolean equalsShallow(Base other_) { 1602 if (!super.equalsShallow(other_)) 1603 return false; 1604 if (!(other_ instanceof AuditEventAgentComponent)) 1605 return false; 1606 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1607 return compareValues(altId, o.altId, true) && compareValues(name, o.name, true) 1608 && compareValues(requestor, o.requestor, true) && compareValues(policy, o.policy, true); 1609 } 1610 1611 public boolean isEmpty() { 1612 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role, who, altId, name, requestor, location, 1613 policy, media, network, purposeOfUse); 1614 } 1615 1616 public String fhirType() { 1617 return "AuditEvent.agent"; 1618 1619 } 1620 1621 } 1622 1623 @Block() 1624 public static class AuditEventAgentNetworkComponent extends BackboneElement implements IBaseBackboneElement { 1625 /** 1626 * An identifier for the network access point of the user device for the audit 1627 * event. 1628 */ 1629 @Child(name = "address", type = { 1630 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1631 @Description(shortDefinition = "Identifier for the network access point of the user device", formalDefinition = "An identifier for the network access point of the user device for the audit event.") 1632 protected StringType address; 1633 1634 /** 1635 * An identifier for the type of network access point that originated the audit 1636 * event. 1637 */ 1638 @Child(name = "type", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1639 @Description(shortDefinition = "The type of network access point", formalDefinition = "An identifier for the type of network access point that originated the audit event.") 1640 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/network-type") 1641 protected Enumeration<AuditEventAgentNetworkType> type; 1642 1643 private static final long serialVersionUID = -160715924L; 1644 1645 /** 1646 * Constructor 1647 */ 1648 public AuditEventAgentNetworkComponent() { 1649 super(); 1650 } 1651 1652 /** 1653 * @return {@link #address} (An identifier for the network access point of the 1654 * user device for the audit event.). This is the underlying object with 1655 * id, value and extensions. The accessor "getAddress" gives direct 1656 * access to the value 1657 */ 1658 public StringType getAddressElement() { 1659 if (this.address == null) 1660 if (Configuration.errorOnAutoCreate()) 1661 throw new Error("Attempt to auto-create AuditEventAgentNetworkComponent.address"); 1662 else if (Configuration.doAutoCreate()) 1663 this.address = new StringType(); // bb 1664 return this.address; 1665 } 1666 1667 public boolean hasAddressElement() { 1668 return this.address != null && !this.address.isEmpty(); 1669 } 1670 1671 public boolean hasAddress() { 1672 return this.address != null && !this.address.isEmpty(); 1673 } 1674 1675 /** 1676 * @param value {@link #address} (An identifier for the network access point of 1677 * the user device for the audit event.). This is the underlying 1678 * object with id, value and extensions. The accessor "getAddress" 1679 * gives direct access to the value 1680 */ 1681 public AuditEventAgentNetworkComponent setAddressElement(StringType value) { 1682 this.address = value; 1683 return this; 1684 } 1685 1686 /** 1687 * @return An identifier for the network access point of the user device for the 1688 * audit event. 1689 */ 1690 public String getAddress() { 1691 return this.address == null ? null : this.address.getValue(); 1692 } 1693 1694 /** 1695 * @param value An identifier for the network access point of the user device 1696 * for the audit event. 1697 */ 1698 public AuditEventAgentNetworkComponent setAddress(String value) { 1699 if (Utilities.noString(value)) 1700 this.address = null; 1701 else { 1702 if (this.address == null) 1703 this.address = new StringType(); 1704 this.address.setValue(value); 1705 } 1706 return this; 1707 } 1708 1709 /** 1710 * @return {@link #type} (An identifier for the type of network access point 1711 * that originated the audit event.). This is the underlying object with 1712 * id, value and extensions. The accessor "getType" gives direct access 1713 * to the value 1714 */ 1715 public Enumeration<AuditEventAgentNetworkType> getTypeElement() { 1716 if (this.type == null) 1717 if (Configuration.errorOnAutoCreate()) 1718 throw new Error("Attempt to auto-create AuditEventAgentNetworkComponent.type"); 1719 else if (Configuration.doAutoCreate()) 1720 this.type = new Enumeration<AuditEventAgentNetworkType>(new AuditEventAgentNetworkTypeEnumFactory()); // bb 1721 return this.type; 1722 } 1723 1724 public boolean hasTypeElement() { 1725 return this.type != null && !this.type.isEmpty(); 1726 } 1727 1728 public boolean hasType() { 1729 return this.type != null && !this.type.isEmpty(); 1730 } 1731 1732 /** 1733 * @param value {@link #type} (An identifier for the type of network access 1734 * point that originated the audit event.). This is the underlying 1735 * object with id, value and extensions. The accessor "getType" 1736 * gives direct access to the value 1737 */ 1738 public AuditEventAgentNetworkComponent setTypeElement(Enumeration<AuditEventAgentNetworkType> value) { 1739 this.type = value; 1740 return this; 1741 } 1742 1743 /** 1744 * @return An identifier for the type of network access point that originated 1745 * the audit event. 1746 */ 1747 public AuditEventAgentNetworkType getType() { 1748 return this.type == null ? null : this.type.getValue(); 1749 } 1750 1751 /** 1752 * @param value An identifier for the type of network access point that 1753 * originated the audit event. 1754 */ 1755 public AuditEventAgentNetworkComponent setType(AuditEventAgentNetworkType value) { 1756 if (value == null) 1757 this.type = null; 1758 else { 1759 if (this.type == null) 1760 this.type = new Enumeration<AuditEventAgentNetworkType>(new AuditEventAgentNetworkTypeEnumFactory()); 1761 this.type.setValue(value); 1762 } 1763 return this; 1764 } 1765 1766 protected void listChildren(List<Property> children) { 1767 super.listChildren(children); 1768 children.add(new Property("address", "string", 1769 "An identifier for the network access point of the user device for the audit event.", 0, 1, address)); 1770 children.add(new Property("type", "code", 1771 "An identifier for the type of network access point that originated the audit event.", 0, 1, type)); 1772 } 1773 1774 @Override 1775 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1776 switch (_hash) { 1777 case -1147692044: 1778 /* address */ return new Property("address", "string", 1779 "An identifier for the network access point of the user device for the audit event.", 0, 1, address); 1780 case 3575610: 1781 /* type */ return new Property("type", "code", 1782 "An identifier for the type of network access point that originated the audit event.", 0, 1, type); 1783 default: 1784 return super.getNamedProperty(_hash, _name, _checkValid); 1785 } 1786 1787 } 1788 1789 @Override 1790 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1791 switch (hash) { 1792 case -1147692044: 1793 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // StringType 1794 case 3575610: 1795 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<AuditEventAgentNetworkType> 1796 default: 1797 return super.getProperty(hash, name, checkValid); 1798 } 1799 1800 } 1801 1802 @Override 1803 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1804 switch (hash) { 1805 case -1147692044: // address 1806 this.address = castToString(value); // StringType 1807 return value; 1808 case 3575610: // type 1809 value = new AuditEventAgentNetworkTypeEnumFactory().fromType(castToCode(value)); 1810 this.type = (Enumeration) value; // Enumeration<AuditEventAgentNetworkType> 1811 return value; 1812 default: 1813 return super.setProperty(hash, name, value); 1814 } 1815 1816 } 1817 1818 @Override 1819 public Base setProperty(String name, Base value) throws FHIRException { 1820 if (name.equals("address")) { 1821 this.address = castToString(value); // StringType 1822 } else if (name.equals("type")) { 1823 value = new AuditEventAgentNetworkTypeEnumFactory().fromType(castToCode(value)); 1824 this.type = (Enumeration) value; // Enumeration<AuditEventAgentNetworkType> 1825 } else 1826 return super.setProperty(name, value); 1827 return value; 1828 } 1829 1830 @Override 1831 public void removeChild(String name, Base value) throws FHIRException { 1832 if (name.equals("address")) { 1833 this.address = null; 1834 } else if (name.equals("type")) { 1835 this.type = null; 1836 } else 1837 super.removeChild(name, value); 1838 1839 } 1840 1841 @Override 1842 public Base makeProperty(int hash, String name) throws FHIRException { 1843 switch (hash) { 1844 case -1147692044: 1845 return getAddressElement(); 1846 case 3575610: 1847 return getTypeElement(); 1848 default: 1849 return super.makeProperty(hash, name); 1850 } 1851 1852 } 1853 1854 @Override 1855 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1856 switch (hash) { 1857 case -1147692044: 1858 /* address */ return new String[] { "string" }; 1859 case 3575610: 1860 /* type */ return new String[] { "code" }; 1861 default: 1862 return super.getTypesForProperty(hash, name); 1863 } 1864 1865 } 1866 1867 @Override 1868 public Base addChild(String name) throws FHIRException { 1869 if (name.equals("address")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.address"); 1871 } else if (name.equals("type")) { 1872 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 1873 } else 1874 return super.addChild(name); 1875 } 1876 1877 public AuditEventAgentNetworkComponent copy() { 1878 AuditEventAgentNetworkComponent dst = new AuditEventAgentNetworkComponent(); 1879 copyValues(dst); 1880 return dst; 1881 } 1882 1883 public void copyValues(AuditEventAgentNetworkComponent dst) { 1884 super.copyValues(dst); 1885 dst.address = address == null ? null : address.copy(); 1886 dst.type = type == null ? null : type.copy(); 1887 } 1888 1889 @Override 1890 public boolean equalsDeep(Base other_) { 1891 if (!super.equalsDeep(other_)) 1892 return false; 1893 if (!(other_ instanceof AuditEventAgentNetworkComponent)) 1894 return false; 1895 AuditEventAgentNetworkComponent o = (AuditEventAgentNetworkComponent) other_; 1896 return compareDeep(address, o.address, true) && compareDeep(type, o.type, true); 1897 } 1898 1899 @Override 1900 public boolean equalsShallow(Base other_) { 1901 if (!super.equalsShallow(other_)) 1902 return false; 1903 if (!(other_ instanceof AuditEventAgentNetworkComponent)) 1904 return false; 1905 AuditEventAgentNetworkComponent o = (AuditEventAgentNetworkComponent) other_; 1906 return compareValues(address, o.address, true) && compareValues(type, o.type, true); 1907 } 1908 1909 public boolean isEmpty() { 1910 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(address, type); 1911 } 1912 1913 public String fhirType() { 1914 return "AuditEvent.agent.network"; 1915 1916 } 1917 1918 } 1919 1920 @Block() 1921 public static class AuditEventSourceComponent extends BackboneElement implements IBaseBackboneElement { 1922 /** 1923 * Logical source location within the healthcare enterprise network. For 1924 * example, a hospital or other provider location within a multi-entity provider 1925 * group. 1926 */ 1927 @Child(name = "site", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1928 @Description(shortDefinition = "Logical source location within the enterprise", formalDefinition = "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.") 1929 protected StringType site; 1930 1931 /** 1932 * Identifier of the source where the event was detected. 1933 */ 1934 @Child(name = "observer", type = { PractitionerRole.class, Practitioner.class, Organization.class, Device.class, 1935 Patient.class, RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1936 @Description(shortDefinition = "The identity of source detecting the event", formalDefinition = "Identifier of the source where the event was detected.") 1937 protected Reference observer; 1938 1939 /** 1940 * The actual object that is the target of the reference (Identifier of the 1941 * source where the event was detected.) 1942 */ 1943 protected Resource observerTarget; 1944 1945 /** 1946 * Code specifying the type of source where event originated. 1947 */ 1948 @Child(name = "type", type = { 1949 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1950 @Description(shortDefinition = "The type of source where event originated", formalDefinition = "Code specifying the type of source where event originated.") 1951 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-source-type") 1952 protected List<Coding> type; 1953 1954 private static final long serialVersionUID = 2133038564L; 1955 1956 /** 1957 * Constructor 1958 */ 1959 public AuditEventSourceComponent() { 1960 super(); 1961 } 1962 1963 /** 1964 * Constructor 1965 */ 1966 public AuditEventSourceComponent(Reference observer) { 1967 super(); 1968 this.observer = observer; 1969 } 1970 1971 /** 1972 * @return {@link #site} (Logical source location within the healthcare 1973 * enterprise network. For example, a hospital or other provider 1974 * location within a multi-entity provider group.). This is the 1975 * underlying object with id, value and extensions. The accessor 1976 * "getSite" gives direct access to the value 1977 */ 1978 public StringType getSiteElement() { 1979 if (this.site == null) 1980 if (Configuration.errorOnAutoCreate()) 1981 throw new Error("Attempt to auto-create AuditEventSourceComponent.site"); 1982 else if (Configuration.doAutoCreate()) 1983 this.site = new StringType(); // bb 1984 return this.site; 1985 } 1986 1987 public boolean hasSiteElement() { 1988 return this.site != null && !this.site.isEmpty(); 1989 } 1990 1991 public boolean hasSite() { 1992 return this.site != null && !this.site.isEmpty(); 1993 } 1994 1995 /** 1996 * @param value {@link #site} (Logical source location within the healthcare 1997 * enterprise network. For example, a hospital or other provider 1998 * location within a multi-entity provider group.). This is the 1999 * underlying object with id, value and extensions. The accessor 2000 * "getSite" gives direct access to the value 2001 */ 2002 public AuditEventSourceComponent setSiteElement(StringType value) { 2003 this.site = value; 2004 return this; 2005 } 2006 2007 /** 2008 * @return Logical source location within the healthcare enterprise network. For 2009 * example, a hospital or other provider location within a multi-entity 2010 * provider group. 2011 */ 2012 public String getSite() { 2013 return this.site == null ? null : this.site.getValue(); 2014 } 2015 2016 /** 2017 * @param value Logical source location within the healthcare enterprise 2018 * network. For example, a hospital or other provider location 2019 * within a multi-entity provider group. 2020 */ 2021 public AuditEventSourceComponent setSite(String value) { 2022 if (Utilities.noString(value)) 2023 this.site = null; 2024 else { 2025 if (this.site == null) 2026 this.site = new StringType(); 2027 this.site.setValue(value); 2028 } 2029 return this; 2030 } 2031 2032 /** 2033 * @return {@link #observer} (Identifier of the source where the event was 2034 * detected.) 2035 */ 2036 public Reference getObserver() { 2037 if (this.observer == null) 2038 if (Configuration.errorOnAutoCreate()) 2039 throw new Error("Attempt to auto-create AuditEventSourceComponent.observer"); 2040 else if (Configuration.doAutoCreate()) 2041 this.observer = new Reference(); // cc 2042 return this.observer; 2043 } 2044 2045 public boolean hasObserver() { 2046 return this.observer != null && !this.observer.isEmpty(); 2047 } 2048 2049 /** 2050 * @param value {@link #observer} (Identifier of the source where the event was 2051 * detected.) 2052 */ 2053 public AuditEventSourceComponent setObserver(Reference value) { 2054 this.observer = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #observer} The actual object that is the target of the 2060 * reference. The reference library doesn't populate this, but you can 2061 * use it to hold the resource if you resolve it. (Identifier of the 2062 * source where the event was detected.) 2063 */ 2064 public Resource getObserverTarget() { 2065 return this.observerTarget; 2066 } 2067 2068 /** 2069 * @param value {@link #observer} The actual object that is the target of the 2070 * reference. The reference library doesn't use these, but you can 2071 * use it to hold the resource if you resolve it. (Identifier of 2072 * the source where the event was detected.) 2073 */ 2074 public AuditEventSourceComponent setObserverTarget(Resource value) { 2075 this.observerTarget = value; 2076 return this; 2077 } 2078 2079 /** 2080 * @return {@link #type} (Code specifying the type of source where event 2081 * originated.) 2082 */ 2083 public List<Coding> getType() { 2084 if (this.type == null) 2085 this.type = new ArrayList<Coding>(); 2086 return this.type; 2087 } 2088 2089 /** 2090 * @return Returns a reference to <code>this</code> for easy method chaining 2091 */ 2092 public AuditEventSourceComponent setType(List<Coding> theType) { 2093 this.type = theType; 2094 return this; 2095 } 2096 2097 public boolean hasType() { 2098 if (this.type == null) 2099 return false; 2100 for (Coding item : this.type) 2101 if (!item.isEmpty()) 2102 return true; 2103 return false; 2104 } 2105 2106 public Coding addType() { // 3 2107 Coding t = new Coding(); 2108 if (this.type == null) 2109 this.type = new ArrayList<Coding>(); 2110 this.type.add(t); 2111 return t; 2112 } 2113 2114 public AuditEventSourceComponent addType(Coding t) { // 3 2115 if (t == null) 2116 return this; 2117 if (this.type == null) 2118 this.type = new ArrayList<Coding>(); 2119 this.type.add(t); 2120 return this; 2121 } 2122 2123 /** 2124 * @return The first repetition of repeating field {@link #type}, creating it if 2125 * it does not already exist 2126 */ 2127 public Coding getTypeFirstRep() { 2128 if (getType().isEmpty()) { 2129 addType(); 2130 } 2131 return getType().get(0); 2132 } 2133 2134 protected void listChildren(List<Property> children) { 2135 super.listChildren(children); 2136 children.add(new Property("site", "string", 2137 "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 2138 0, 1, site)); 2139 children.add( 2140 new Property("observer", "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 2141 "Identifier of the source where the event was detected.", 0, 1, observer)); 2142 children.add(new Property("type", "Coding", "Code specifying the type of source where event originated.", 0, 2143 java.lang.Integer.MAX_VALUE, type)); 2144 } 2145 2146 @Override 2147 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2148 switch (_hash) { 2149 case 3530567: 2150 /* site */ return new Property("site", "string", 2151 "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 2152 0, 1, site); 2153 case 348607190: 2154 /* observer */ return new Property("observer", 2155 "Reference(PractitionerRole|Practitioner|Organization|Device|Patient|RelatedPerson)", 2156 "Identifier of the source where the event was detected.", 0, 1, observer); 2157 case 3575610: 2158 /* type */ return new Property("type", "Coding", "Code specifying the type of source where event originated.", 2159 0, java.lang.Integer.MAX_VALUE, type); 2160 default: 2161 return super.getNamedProperty(_hash, _name, _checkValid); 2162 } 2163 2164 } 2165 2166 @Override 2167 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2168 switch (hash) { 2169 case 3530567: 2170 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // StringType 2171 case 348607190: 2172 /* observer */ return this.observer == null ? new Base[0] : new Base[] { this.observer }; // Reference 2173 case 3575610: 2174 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // Coding 2175 default: 2176 return super.getProperty(hash, name, checkValid); 2177 } 2178 2179 } 2180 2181 @Override 2182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2183 switch (hash) { 2184 case 3530567: // site 2185 this.site = castToString(value); // StringType 2186 return value; 2187 case 348607190: // observer 2188 this.observer = castToReference(value); // Reference 2189 return value; 2190 case 3575610: // type 2191 this.getType().add(castToCoding(value)); // Coding 2192 return value; 2193 default: 2194 return super.setProperty(hash, name, value); 2195 } 2196 2197 } 2198 2199 @Override 2200 public Base setProperty(String name, Base value) throws FHIRException { 2201 if (name.equals("site")) { 2202 this.site = castToString(value); // StringType 2203 } else if (name.equals("observer")) { 2204 this.observer = castToReference(value); // Reference 2205 } else if (name.equals("type")) { 2206 this.getType().add(castToCoding(value)); 2207 } else 2208 return super.setProperty(name, value); 2209 return value; 2210 } 2211 2212 @Override 2213 public void removeChild(String name, Base value) throws FHIRException { 2214 if (name.equals("site")) { 2215 this.site = null; 2216 } else if (name.equals("observer")) { 2217 this.observer = null; 2218 } else if (name.equals("type")) { 2219 this.getType().remove(castToCoding(value)); 2220 } else 2221 super.removeChild(name, value); 2222 2223 } 2224 2225 @Override 2226 public Base makeProperty(int hash, String name) throws FHIRException { 2227 switch (hash) { 2228 case 3530567: 2229 return getSiteElement(); 2230 case 348607190: 2231 return getObserver(); 2232 case 3575610: 2233 return addType(); 2234 default: 2235 return super.makeProperty(hash, name); 2236 } 2237 2238 } 2239 2240 @Override 2241 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2242 switch (hash) { 2243 case 3530567: 2244 /* site */ return new String[] { "string" }; 2245 case 348607190: 2246 /* observer */ return new String[] { "Reference" }; 2247 case 3575610: 2248 /* type */ return new String[] { "Coding" }; 2249 default: 2250 return super.getTypesForProperty(hash, name); 2251 } 2252 2253 } 2254 2255 @Override 2256 public Base addChild(String name) throws FHIRException { 2257 if (name.equals("site")) { 2258 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.site"); 2259 } else if (name.equals("observer")) { 2260 this.observer = new Reference(); 2261 return this.observer; 2262 } else if (name.equals("type")) { 2263 return addType(); 2264 } else 2265 return super.addChild(name); 2266 } 2267 2268 public AuditEventSourceComponent copy() { 2269 AuditEventSourceComponent dst = new AuditEventSourceComponent(); 2270 copyValues(dst); 2271 return dst; 2272 } 2273 2274 public void copyValues(AuditEventSourceComponent dst) { 2275 super.copyValues(dst); 2276 dst.site = site == null ? null : site.copy(); 2277 dst.observer = observer == null ? null : observer.copy(); 2278 if (type != null) { 2279 dst.type = new ArrayList<Coding>(); 2280 for (Coding i : type) 2281 dst.type.add(i.copy()); 2282 } 2283 ; 2284 } 2285 2286 @Override 2287 public boolean equalsDeep(Base other_) { 2288 if (!super.equalsDeep(other_)) 2289 return false; 2290 if (!(other_ instanceof AuditEventSourceComponent)) 2291 return false; 2292 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 2293 return compareDeep(site, o.site, true) && compareDeep(observer, o.observer, true) 2294 && compareDeep(type, o.type, true); 2295 } 2296 2297 @Override 2298 public boolean equalsShallow(Base other_) { 2299 if (!super.equalsShallow(other_)) 2300 return false; 2301 if (!(other_ instanceof AuditEventSourceComponent)) 2302 return false; 2303 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 2304 return compareValues(site, o.site, true); 2305 } 2306 2307 public boolean isEmpty() { 2308 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, observer, type); 2309 } 2310 2311 public String fhirType() { 2312 return "AuditEvent.source"; 2313 2314 } 2315 2316 } 2317 2318 @Block() 2319 public static class AuditEventEntityComponent extends BackboneElement implements IBaseBackboneElement { 2320 /** 2321 * Identifies a specific instance of the entity. The reference should be version 2322 * specific. 2323 */ 2324 @Child(name = "what", type = { Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2325 @Description(shortDefinition = "Specific instance of resource", formalDefinition = "Identifies a specific instance of the entity. The reference should be version specific.") 2326 protected Reference what; 2327 2328 /** 2329 * The actual object that is the target of the reference (Identifies a specific 2330 * instance of the entity. The reference should be version specific.) 2331 */ 2332 protected Resource whatTarget; 2333 2334 /** 2335 * The type of the object that was involved in this audit event. 2336 */ 2337 @Child(name = "type", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2338 @Description(shortDefinition = "Type of entity involved", formalDefinition = "The type of the object that was involved in this audit event.") 2339 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-entity-type") 2340 protected Coding type; 2341 2342 /** 2343 * Code representing the role the entity played in the event being audited. 2344 */ 2345 @Child(name = "role", type = { Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2346 @Description(shortDefinition = "What role the entity played", formalDefinition = "Code representing the role the entity played in the event being audited.") 2347 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/object-role") 2348 protected Coding role; 2349 2350 /** 2351 * Identifier for the data life-cycle stage for the entity. 2352 */ 2353 @Child(name = "lifecycle", type = { Coding.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2354 @Description(shortDefinition = "Life-cycle stage for the entity", formalDefinition = "Identifier for the data life-cycle stage for the entity.") 2355 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/object-lifecycle-events") 2356 protected Coding lifecycle; 2357 2358 /** 2359 * Security labels for the identified entity. 2360 */ 2361 @Child(name = "securityLabel", type = { 2362 Coding.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2363 @Description(shortDefinition = "Security labels on the entity", formalDefinition = "Security labels for the identified entity.") 2364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 2365 protected List<Coding> securityLabel; 2366 2367 /** 2368 * A name of the entity in the audit event. 2369 */ 2370 @Child(name = "name", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2371 @Description(shortDefinition = "Descriptor for entity", formalDefinition = "A name of the entity in the audit event.") 2372 protected StringType name; 2373 2374 /** 2375 * Text that describes the entity in more detail. 2376 */ 2377 @Child(name = "description", type = { 2378 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2379 @Description(shortDefinition = "Descriptive text", formalDefinition = "Text that describes the entity in more detail.") 2380 protected StringType description; 2381 2382 /** 2383 * The query parameters for a query-type entities. 2384 */ 2385 @Child(name = "query", type = { 2386 Base64BinaryType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2387 @Description(shortDefinition = "Query parameters", formalDefinition = "The query parameters for a query-type entities.") 2388 protected Base64BinaryType query; 2389 2390 /** 2391 * Tagged value pairs for conveying additional information about the entity. 2392 */ 2393 @Child(name = "detail", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2394 @Description(shortDefinition = "Additional Information about the entity", formalDefinition = "Tagged value pairs for conveying additional information about the entity.") 2395 protected List<AuditEventEntityDetailComponent> detail; 2396 2397 private static final long serialVersionUID = 334545686L; 2398 2399 /** 2400 * Constructor 2401 */ 2402 public AuditEventEntityComponent() { 2403 super(); 2404 } 2405 2406 /** 2407 * @return {@link #what} (Identifies a specific instance of the entity. The 2408 * reference should be version specific.) 2409 */ 2410 public Reference getWhat() { 2411 if (this.what == null) 2412 if (Configuration.errorOnAutoCreate()) 2413 throw new Error("Attempt to auto-create AuditEventEntityComponent.what"); 2414 else if (Configuration.doAutoCreate()) 2415 this.what = new Reference(); // cc 2416 return this.what; 2417 } 2418 2419 public boolean hasWhat() { 2420 return this.what != null && !this.what.isEmpty(); 2421 } 2422 2423 /** 2424 * @param value {@link #what} (Identifies a specific instance of the entity. The 2425 * reference should be version specific.) 2426 */ 2427 public AuditEventEntityComponent setWhat(Reference value) { 2428 this.what = value; 2429 return this; 2430 } 2431 2432 /** 2433 * @return {@link #what} The actual object that is the target of the reference. 2434 * The reference library doesn't populate this, but you can use it to 2435 * hold the resource if you resolve it. (Identifies a specific instance 2436 * of the entity. The reference should be version specific.) 2437 */ 2438 public Resource getWhatTarget() { 2439 return this.whatTarget; 2440 } 2441 2442 /** 2443 * @param value {@link #what} The actual object that is the target of the 2444 * reference. The reference library doesn't use these, but you can 2445 * use it to hold the resource if you resolve it. (Identifies a 2446 * specific instance of the entity. The reference should be version 2447 * specific.) 2448 */ 2449 public AuditEventEntityComponent setWhatTarget(Resource value) { 2450 this.whatTarget = value; 2451 return this; 2452 } 2453 2454 /** 2455 * @return {@link #type} (The type of the object that was involved in this audit 2456 * event.) 2457 */ 2458 public Coding getType() { 2459 if (this.type == null) 2460 if (Configuration.errorOnAutoCreate()) 2461 throw new Error("Attempt to auto-create AuditEventEntityComponent.type"); 2462 else if (Configuration.doAutoCreate()) 2463 this.type = new Coding(); // cc 2464 return this.type; 2465 } 2466 2467 public boolean hasType() { 2468 return this.type != null && !this.type.isEmpty(); 2469 } 2470 2471 /** 2472 * @param value {@link #type} (The type of the object that was involved in this 2473 * audit event.) 2474 */ 2475 public AuditEventEntityComponent setType(Coding value) { 2476 this.type = value; 2477 return this; 2478 } 2479 2480 /** 2481 * @return {@link #role} (Code representing the role the entity played in the 2482 * event being audited.) 2483 */ 2484 public Coding getRole() { 2485 if (this.role == null) 2486 if (Configuration.errorOnAutoCreate()) 2487 throw new Error("Attempt to auto-create AuditEventEntityComponent.role"); 2488 else if (Configuration.doAutoCreate()) 2489 this.role = new Coding(); // cc 2490 return this.role; 2491 } 2492 2493 public boolean hasRole() { 2494 return this.role != null && !this.role.isEmpty(); 2495 } 2496 2497 /** 2498 * @param value {@link #role} (Code representing the role the entity played in 2499 * the event being audited.) 2500 */ 2501 public AuditEventEntityComponent setRole(Coding value) { 2502 this.role = value; 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #lifecycle} (Identifier for the data life-cycle stage for the 2508 * entity.) 2509 */ 2510 public Coding getLifecycle() { 2511 if (this.lifecycle == null) 2512 if (Configuration.errorOnAutoCreate()) 2513 throw new Error("Attempt to auto-create AuditEventEntityComponent.lifecycle"); 2514 else if (Configuration.doAutoCreate()) 2515 this.lifecycle = new Coding(); // cc 2516 return this.lifecycle; 2517 } 2518 2519 public boolean hasLifecycle() { 2520 return this.lifecycle != null && !this.lifecycle.isEmpty(); 2521 } 2522 2523 /** 2524 * @param value {@link #lifecycle} (Identifier for the data life-cycle stage for 2525 * the entity.) 2526 */ 2527 public AuditEventEntityComponent setLifecycle(Coding value) { 2528 this.lifecycle = value; 2529 return this; 2530 } 2531 2532 /** 2533 * @return {@link #securityLabel} (Security labels for the identified entity.) 2534 */ 2535 public List<Coding> getSecurityLabel() { 2536 if (this.securityLabel == null) 2537 this.securityLabel = new ArrayList<Coding>(); 2538 return this.securityLabel; 2539 } 2540 2541 /** 2542 * @return Returns a reference to <code>this</code> for easy method chaining 2543 */ 2544 public AuditEventEntityComponent setSecurityLabel(List<Coding> theSecurityLabel) { 2545 this.securityLabel = theSecurityLabel; 2546 return this; 2547 } 2548 2549 public boolean hasSecurityLabel() { 2550 if (this.securityLabel == null) 2551 return false; 2552 for (Coding item : this.securityLabel) 2553 if (!item.isEmpty()) 2554 return true; 2555 return false; 2556 } 2557 2558 public Coding addSecurityLabel() { // 3 2559 Coding t = new Coding(); 2560 if (this.securityLabel == null) 2561 this.securityLabel = new ArrayList<Coding>(); 2562 this.securityLabel.add(t); 2563 return t; 2564 } 2565 2566 public AuditEventEntityComponent addSecurityLabel(Coding t) { // 3 2567 if (t == null) 2568 return this; 2569 if (this.securityLabel == null) 2570 this.securityLabel = new ArrayList<Coding>(); 2571 this.securityLabel.add(t); 2572 return this; 2573 } 2574 2575 /** 2576 * @return The first repetition of repeating field {@link #securityLabel}, 2577 * creating it if it does not already exist 2578 */ 2579 public Coding getSecurityLabelFirstRep() { 2580 if (getSecurityLabel().isEmpty()) { 2581 addSecurityLabel(); 2582 } 2583 return getSecurityLabel().get(0); 2584 } 2585 2586 /** 2587 * @return {@link #name} (A name of the entity in the audit event.). This is the 2588 * underlying object with id, value and extensions. The accessor 2589 * "getName" gives direct access to the value 2590 */ 2591 public StringType getNameElement() { 2592 if (this.name == null) 2593 if (Configuration.errorOnAutoCreate()) 2594 throw new Error("Attempt to auto-create AuditEventEntityComponent.name"); 2595 else if (Configuration.doAutoCreate()) 2596 this.name = new StringType(); // bb 2597 return this.name; 2598 } 2599 2600 public boolean hasNameElement() { 2601 return this.name != null && !this.name.isEmpty(); 2602 } 2603 2604 public boolean hasName() { 2605 return this.name != null && !this.name.isEmpty(); 2606 } 2607 2608 /** 2609 * @param value {@link #name} (A name of the entity in the audit event.). This 2610 * is the underlying object with id, value and extensions. The 2611 * accessor "getName" gives direct access to the value 2612 */ 2613 public AuditEventEntityComponent setNameElement(StringType value) { 2614 this.name = value; 2615 return this; 2616 } 2617 2618 /** 2619 * @return A name of the entity in the audit event. 2620 */ 2621 public String getName() { 2622 return this.name == null ? null : this.name.getValue(); 2623 } 2624 2625 /** 2626 * @param value A name of the entity in the audit event. 2627 */ 2628 public AuditEventEntityComponent setName(String value) { 2629 if (Utilities.noString(value)) 2630 this.name = null; 2631 else { 2632 if (this.name == null) 2633 this.name = new StringType(); 2634 this.name.setValue(value); 2635 } 2636 return this; 2637 } 2638 2639 /** 2640 * @return {@link #description} (Text that describes the entity in more 2641 * detail.). This is the underlying object with id, value and 2642 * extensions. The accessor "getDescription" gives direct access to the 2643 * value 2644 */ 2645 public StringType getDescriptionElement() { 2646 if (this.description == null) 2647 if (Configuration.errorOnAutoCreate()) 2648 throw new Error("Attempt to auto-create AuditEventEntityComponent.description"); 2649 else if (Configuration.doAutoCreate()) 2650 this.description = new StringType(); // bb 2651 return this.description; 2652 } 2653 2654 public boolean hasDescriptionElement() { 2655 return this.description != null && !this.description.isEmpty(); 2656 } 2657 2658 public boolean hasDescription() { 2659 return this.description != null && !this.description.isEmpty(); 2660 } 2661 2662 /** 2663 * @param value {@link #description} (Text that describes the entity in more 2664 * detail.). This is the underlying object with id, value and 2665 * extensions. The accessor "getDescription" gives direct access to 2666 * the value 2667 */ 2668 public AuditEventEntityComponent setDescriptionElement(StringType value) { 2669 this.description = value; 2670 return this; 2671 } 2672 2673 /** 2674 * @return Text that describes the entity in more detail. 2675 */ 2676 public String getDescription() { 2677 return this.description == null ? null : this.description.getValue(); 2678 } 2679 2680 /** 2681 * @param value Text that describes the entity in more detail. 2682 */ 2683 public AuditEventEntityComponent setDescription(String value) { 2684 if (Utilities.noString(value)) 2685 this.description = null; 2686 else { 2687 if (this.description == null) 2688 this.description = new StringType(); 2689 this.description.setValue(value); 2690 } 2691 return this; 2692 } 2693 2694 /** 2695 * @return {@link #query} (The query parameters for a query-type entities.). 2696 * This is the underlying object with id, value and extensions. The 2697 * accessor "getQuery" gives direct access to the value 2698 */ 2699 public Base64BinaryType getQueryElement() { 2700 if (this.query == null) 2701 if (Configuration.errorOnAutoCreate()) 2702 throw new Error("Attempt to auto-create AuditEventEntityComponent.query"); 2703 else if (Configuration.doAutoCreate()) 2704 this.query = new Base64BinaryType(); // bb 2705 return this.query; 2706 } 2707 2708 public boolean hasQueryElement() { 2709 return this.query != null && !this.query.isEmpty(); 2710 } 2711 2712 public boolean hasQuery() { 2713 return this.query != null && !this.query.isEmpty(); 2714 } 2715 2716 /** 2717 * @param value {@link #query} (The query parameters for a query-type 2718 * entities.). This is the underlying object with id, value and 2719 * extensions. The accessor "getQuery" gives direct access to the 2720 * value 2721 */ 2722 public AuditEventEntityComponent setQueryElement(Base64BinaryType value) { 2723 this.query = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return The query parameters for a query-type entities. 2729 */ 2730 public byte[] getQuery() { 2731 return this.query == null ? null : this.query.getValue(); 2732 } 2733 2734 /** 2735 * @param value The query parameters for a query-type entities. 2736 */ 2737 public AuditEventEntityComponent setQuery(byte[] value) { 2738 if (value == null) 2739 this.query = null; 2740 else { 2741 if (this.query == null) 2742 this.query = new Base64BinaryType(); 2743 this.query.setValue(value); 2744 } 2745 return this; 2746 } 2747 2748 /** 2749 * @return {@link #detail} (Tagged value pairs for conveying additional 2750 * information about the entity.) 2751 */ 2752 public List<AuditEventEntityDetailComponent> getDetail() { 2753 if (this.detail == null) 2754 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2755 return this.detail; 2756 } 2757 2758 /** 2759 * @return Returns a reference to <code>this</code> for easy method chaining 2760 */ 2761 public AuditEventEntityComponent setDetail(List<AuditEventEntityDetailComponent> theDetail) { 2762 this.detail = theDetail; 2763 return this; 2764 } 2765 2766 public boolean hasDetail() { 2767 if (this.detail == null) 2768 return false; 2769 for (AuditEventEntityDetailComponent item : this.detail) 2770 if (!item.isEmpty()) 2771 return true; 2772 return false; 2773 } 2774 2775 public AuditEventEntityDetailComponent addDetail() { // 3 2776 AuditEventEntityDetailComponent t = new AuditEventEntityDetailComponent(); 2777 if (this.detail == null) 2778 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2779 this.detail.add(t); 2780 return t; 2781 } 2782 2783 public AuditEventEntityComponent addDetail(AuditEventEntityDetailComponent t) { // 3 2784 if (t == null) 2785 return this; 2786 if (this.detail == null) 2787 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2788 this.detail.add(t); 2789 return this; 2790 } 2791 2792 /** 2793 * @return The first repetition of repeating field {@link #detail}, creating it 2794 * if it does not already exist 2795 */ 2796 public AuditEventEntityDetailComponent getDetailFirstRep() { 2797 if (getDetail().isEmpty()) { 2798 addDetail(); 2799 } 2800 return getDetail().get(0); 2801 } 2802 2803 protected void listChildren(List<Property> children) { 2804 super.listChildren(children); 2805 children.add(new Property("what", "Reference(Any)", 2806 "Identifies a specific instance of the entity. The reference should be version specific.", 0, 1, what)); 2807 children.add( 2808 new Property("type", "Coding", "The type of the object that was involved in this audit event.", 0, 1, type)); 2809 children.add(new Property("role", "Coding", 2810 "Code representing the role the entity played in the event being audited.", 0, 1, role)); 2811 children.add(new Property("lifecycle", "Coding", "Identifier for the data life-cycle stage for the entity.", 0, 1, 2812 lifecycle)); 2813 children.add(new Property("securityLabel", "Coding", "Security labels for the identified entity.", 0, 2814 java.lang.Integer.MAX_VALUE, securityLabel)); 2815 children.add(new Property("name", "string", "A name of the entity in the audit event.", 0, 1, name)); 2816 children.add( 2817 new Property("description", "string", "Text that describes the entity in more detail.", 0, 1, description)); 2818 children 2819 .add(new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 1, query)); 2820 children 2821 .add(new Property("detail", "", "Tagged value pairs for conveying additional information about the entity.", 2822 0, java.lang.Integer.MAX_VALUE, detail)); 2823 } 2824 2825 @Override 2826 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2827 switch (_hash) { 2828 case 3648196: 2829 /* what */ return new Property("what", "Reference(Any)", 2830 "Identifies a specific instance of the entity. The reference should be version specific.", 0, 1, what); 2831 case 3575610: 2832 /* type */ return new Property("type", "Coding", 2833 "The type of the object that was involved in this audit event.", 0, 1, type); 2834 case 3506294: 2835 /* role */ return new Property("role", "Coding", 2836 "Code representing the role the entity played in the event being audited.", 0, 1, role); 2837 case -302323862: 2838 /* lifecycle */ return new Property("lifecycle", "Coding", 2839 "Identifier for the data life-cycle stage for the entity.", 0, 1, lifecycle); 2840 case -722296940: 2841 /* securityLabel */ return new Property("securityLabel", "Coding", "Security labels for the identified entity.", 2842 0, java.lang.Integer.MAX_VALUE, securityLabel); 2843 case 3373707: 2844 /* name */ return new Property("name", "string", "A name of the entity in the audit event.", 0, 1, name); 2845 case -1724546052: 2846 /* description */ return new Property("description", "string", "Text that describes the entity in more detail.", 2847 0, 1, description); 2848 case 107944136: 2849 /* query */ return new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 2850 1, query); 2851 case -1335224239: 2852 /* detail */ return new Property("detail", "", 2853 "Tagged value pairs for conveying additional information about the entity.", 0, java.lang.Integer.MAX_VALUE, 2854 detail); 2855 default: 2856 return super.getNamedProperty(_hash, _name, _checkValid); 2857 } 2858 2859 } 2860 2861 @Override 2862 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2863 switch (hash) { 2864 case 3648196: 2865 /* what */ return this.what == null ? new Base[0] : new Base[] { this.what }; // Reference 2866 case 3575610: 2867 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 2868 case 3506294: 2869 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // Coding 2870 case -302323862: 2871 /* lifecycle */ return this.lifecycle == null ? new Base[0] : new Base[] { this.lifecycle }; // Coding 2872 case -722296940: 2873 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2874 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 2875 case 3373707: 2876 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2877 case -1724546052: 2878 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2879 case 107944136: 2880 /* query */ return this.query == null ? new Base[0] : new Base[] { this.query }; // Base64BinaryType 2881 case -1335224239: 2882 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AuditEventEntityDetailComponent 2883 default: 2884 return super.getProperty(hash, name, checkValid); 2885 } 2886 2887 } 2888 2889 @Override 2890 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2891 switch (hash) { 2892 case 3648196: // what 2893 this.what = castToReference(value); // Reference 2894 return value; 2895 case 3575610: // type 2896 this.type = castToCoding(value); // Coding 2897 return value; 2898 case 3506294: // role 2899 this.role = castToCoding(value); // Coding 2900 return value; 2901 case -302323862: // lifecycle 2902 this.lifecycle = castToCoding(value); // Coding 2903 return value; 2904 case -722296940: // securityLabel 2905 this.getSecurityLabel().add(castToCoding(value)); // Coding 2906 return value; 2907 case 3373707: // name 2908 this.name = castToString(value); // StringType 2909 return value; 2910 case -1724546052: // description 2911 this.description = castToString(value); // StringType 2912 return value; 2913 case 107944136: // query 2914 this.query = castToBase64Binary(value); // Base64BinaryType 2915 return value; 2916 case -1335224239: // detail 2917 this.getDetail().add((AuditEventEntityDetailComponent) value); // AuditEventEntityDetailComponent 2918 return value; 2919 default: 2920 return super.setProperty(hash, name, value); 2921 } 2922 2923 } 2924 2925 @Override 2926 public Base setProperty(String name, Base value) throws FHIRException { 2927 if (name.equals("what")) { 2928 this.what = castToReference(value); // Reference 2929 } else if (name.equals("type")) { 2930 this.type = castToCoding(value); // Coding 2931 } else if (name.equals("role")) { 2932 this.role = castToCoding(value); // Coding 2933 } else if (name.equals("lifecycle")) { 2934 this.lifecycle = castToCoding(value); // Coding 2935 } else if (name.equals("securityLabel")) { 2936 this.getSecurityLabel().add(castToCoding(value)); 2937 } else if (name.equals("name")) { 2938 this.name = castToString(value); // StringType 2939 } else if (name.equals("description")) { 2940 this.description = castToString(value); // StringType 2941 } else if (name.equals("query")) { 2942 this.query = castToBase64Binary(value); // Base64BinaryType 2943 } else if (name.equals("detail")) { 2944 this.getDetail().add((AuditEventEntityDetailComponent) value); 2945 } else 2946 return super.setProperty(name, value); 2947 return value; 2948 } 2949 2950 @Override 2951 public void removeChild(String name, Base value) throws FHIRException { 2952 if (name.equals("what")) { 2953 this.what = null; 2954 } else if (name.equals("type")) { 2955 this.type = null; 2956 } else if (name.equals("role")) { 2957 this.role = null; 2958 } else if (name.equals("lifecycle")) { 2959 this.lifecycle = null; 2960 } else if (name.equals("securityLabel")) { 2961 this.getSecurityLabel().remove(castToCoding(value)); 2962 } else if (name.equals("name")) { 2963 this.name = null; 2964 } else if (name.equals("description")) { 2965 this.description = null; 2966 } else if (name.equals("query")) { 2967 this.query = null; 2968 } else if (name.equals("detail")) { 2969 this.getDetail().remove((AuditEventEntityDetailComponent) value); 2970 } else 2971 super.removeChild(name, value); 2972 2973 } 2974 2975 @Override 2976 public Base makeProperty(int hash, String name) throws FHIRException { 2977 switch (hash) { 2978 case 3648196: 2979 return getWhat(); 2980 case 3575610: 2981 return getType(); 2982 case 3506294: 2983 return getRole(); 2984 case -302323862: 2985 return getLifecycle(); 2986 case -722296940: 2987 return addSecurityLabel(); 2988 case 3373707: 2989 return getNameElement(); 2990 case -1724546052: 2991 return getDescriptionElement(); 2992 case 107944136: 2993 return getQueryElement(); 2994 case -1335224239: 2995 return addDetail(); 2996 default: 2997 return super.makeProperty(hash, name); 2998 } 2999 3000 } 3001 3002 @Override 3003 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3004 switch (hash) { 3005 case 3648196: 3006 /* what */ return new String[] { "Reference" }; 3007 case 3575610: 3008 /* type */ return new String[] { "Coding" }; 3009 case 3506294: 3010 /* role */ return new String[] { "Coding" }; 3011 case -302323862: 3012 /* lifecycle */ return new String[] { "Coding" }; 3013 case -722296940: 3014 /* securityLabel */ return new String[] { "Coding" }; 3015 case 3373707: 3016 /* name */ return new String[] { "string" }; 3017 case -1724546052: 3018 /* description */ return new String[] { "string" }; 3019 case 107944136: 3020 /* query */ return new String[] { "base64Binary" }; 3021 case -1335224239: 3022 /* detail */ return new String[] {}; 3023 default: 3024 return super.getTypesForProperty(hash, name); 3025 } 3026 3027 } 3028 3029 @Override 3030 public Base addChild(String name) throws FHIRException { 3031 if (name.equals("what")) { 3032 this.what = new Reference(); 3033 return this.what; 3034 } else if (name.equals("type")) { 3035 this.type = new Coding(); 3036 return this.type; 3037 } else if (name.equals("role")) { 3038 this.role = new Coding(); 3039 return this.role; 3040 } else if (name.equals("lifecycle")) { 3041 this.lifecycle = new Coding(); 3042 return this.lifecycle; 3043 } else if (name.equals("securityLabel")) { 3044 return addSecurityLabel(); 3045 } else if (name.equals("name")) { 3046 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 3047 } else if (name.equals("description")) { 3048 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.description"); 3049 } else if (name.equals("query")) { 3050 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.query"); 3051 } else if (name.equals("detail")) { 3052 return addDetail(); 3053 } else 3054 return super.addChild(name); 3055 } 3056 3057 public AuditEventEntityComponent copy() { 3058 AuditEventEntityComponent dst = new AuditEventEntityComponent(); 3059 copyValues(dst); 3060 return dst; 3061 } 3062 3063 public void copyValues(AuditEventEntityComponent dst) { 3064 super.copyValues(dst); 3065 dst.what = what == null ? null : what.copy(); 3066 dst.type = type == null ? null : type.copy(); 3067 dst.role = role == null ? null : role.copy(); 3068 dst.lifecycle = lifecycle == null ? null : lifecycle.copy(); 3069 if (securityLabel != null) { 3070 dst.securityLabel = new ArrayList<Coding>(); 3071 for (Coding i : securityLabel) 3072 dst.securityLabel.add(i.copy()); 3073 } 3074 ; 3075 dst.name = name == null ? null : name.copy(); 3076 dst.description = description == null ? null : description.copy(); 3077 dst.query = query == null ? null : query.copy(); 3078 if (detail != null) { 3079 dst.detail = new ArrayList<AuditEventEntityDetailComponent>(); 3080 for (AuditEventEntityDetailComponent i : detail) 3081 dst.detail.add(i.copy()); 3082 } 3083 ; 3084 } 3085 3086 @Override 3087 public boolean equalsDeep(Base other_) { 3088 if (!super.equalsDeep(other_)) 3089 return false; 3090 if (!(other_ instanceof AuditEventEntityComponent)) 3091 return false; 3092 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 3093 return compareDeep(what, o.what, true) && compareDeep(type, o.type, true) && compareDeep(role, o.role, true) 3094 && compareDeep(lifecycle, o.lifecycle, true) && compareDeep(securityLabel, o.securityLabel, true) 3095 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 3096 && compareDeep(query, o.query, true) && compareDeep(detail, o.detail, true); 3097 } 3098 3099 @Override 3100 public boolean equalsShallow(Base other_) { 3101 if (!super.equalsShallow(other_)) 3102 return false; 3103 if (!(other_ instanceof AuditEventEntityComponent)) 3104 return false; 3105 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 3106 return compareValues(name, o.name, true) && compareValues(description, o.description, true) 3107 && compareValues(query, o.query, true); 3108 } 3109 3110 public boolean isEmpty() { 3111 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(what, type, role, lifecycle, securityLabel, name, 3112 description, query, detail); 3113 } 3114 3115 public String fhirType() { 3116 return "AuditEvent.entity"; 3117 3118 } 3119 3120 } 3121 3122 @Block() 3123 public static class AuditEventEntityDetailComponent extends BackboneElement implements IBaseBackboneElement { 3124 /** 3125 * The type of extra detail provided in the value. 3126 */ 3127 @Child(name = "type", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3128 @Description(shortDefinition = "Name of the property", formalDefinition = "The type of extra detail provided in the value.") 3129 protected StringType type; 3130 3131 /** 3132 * The value of the extra detail. 3133 */ 3134 @Child(name = "value", type = { StringType.class, 3135 Base64BinaryType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3136 @Description(shortDefinition = "Property value", formalDefinition = "The value of the extra detail.") 3137 protected Type value; 3138 3139 private static final long serialVersionUID = -1035059584L; 3140 3141 /** 3142 * Constructor 3143 */ 3144 public AuditEventEntityDetailComponent() { 3145 super(); 3146 } 3147 3148 /** 3149 * Constructor 3150 */ 3151 public AuditEventEntityDetailComponent(StringType type, Type value) { 3152 super(); 3153 this.type = type; 3154 this.value = value; 3155 } 3156 3157 /** 3158 * @return {@link #type} (The type of extra detail provided in the value.). This 3159 * is the underlying object with id, value and extensions. The accessor 3160 * "getType" gives direct access to the value 3161 */ 3162 public StringType getTypeElement() { 3163 if (this.type == null) 3164 if (Configuration.errorOnAutoCreate()) 3165 throw new Error("Attempt to auto-create AuditEventEntityDetailComponent.type"); 3166 else if (Configuration.doAutoCreate()) 3167 this.type = new StringType(); // bb 3168 return this.type; 3169 } 3170 3171 public boolean hasTypeElement() { 3172 return this.type != null && !this.type.isEmpty(); 3173 } 3174 3175 public boolean hasType() { 3176 return this.type != null && !this.type.isEmpty(); 3177 } 3178 3179 /** 3180 * @param value {@link #type} (The type of extra detail provided in the value.). 3181 * This is the underlying object with id, value and extensions. The 3182 * accessor "getType" gives direct access to the value 3183 */ 3184 public AuditEventEntityDetailComponent setTypeElement(StringType value) { 3185 this.type = value; 3186 return this; 3187 } 3188 3189 /** 3190 * @return The type of extra detail provided in the value. 3191 */ 3192 public String getType() { 3193 return this.type == null ? null : this.type.getValue(); 3194 } 3195 3196 /** 3197 * @param value The type of extra detail provided in the value. 3198 */ 3199 public AuditEventEntityDetailComponent setType(String value) { 3200 if (this.type == null) 3201 this.type = new StringType(); 3202 this.type.setValue(value); 3203 return this; 3204 } 3205 3206 /** 3207 * @return {@link #value} (The value of the extra detail.) 3208 */ 3209 public Type getValue() { 3210 return this.value; 3211 } 3212 3213 /** 3214 * @return {@link #value} (The value of the extra detail.) 3215 */ 3216 public StringType getValueStringType() throws FHIRException { 3217 if (this.value == null) 3218 this.value = new StringType(); 3219 if (!(this.value instanceof StringType)) 3220 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3221 + this.value.getClass().getName() + " was encountered"); 3222 return (StringType) this.value; 3223 } 3224 3225 public boolean hasValueStringType() { 3226 return this.value instanceof StringType; 3227 } 3228 3229 /** 3230 * @return {@link #value} (The value of the extra detail.) 3231 */ 3232 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 3233 if (this.value == null) 3234 this.value = new Base64BinaryType(); 3235 if (!(this.value instanceof Base64BinaryType)) 3236 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but " 3237 + this.value.getClass().getName() + " was encountered"); 3238 return (Base64BinaryType) this.value; 3239 } 3240 3241 public boolean hasValueBase64BinaryType() { 3242 return this.value instanceof Base64BinaryType; 3243 } 3244 3245 public boolean hasValue() { 3246 return this.value != null && !this.value.isEmpty(); 3247 } 3248 3249 /** 3250 * @param value {@link #value} (The value of the extra detail.) 3251 */ 3252 public AuditEventEntityDetailComponent setValue(Type value) { 3253 if (value != null && !(value instanceof StringType || value instanceof Base64BinaryType)) 3254 throw new Error("Not the right type for AuditEvent.entity.detail.value[x]: " + value.fhirType()); 3255 this.value = value; 3256 return this; 3257 } 3258 3259 protected void listChildren(List<Property> children) { 3260 super.listChildren(children); 3261 children.add(new Property("type", "string", "The type of extra detail provided in the value.", 0, 1, type)); 3262 children.add(new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 1, value)); 3263 } 3264 3265 @Override 3266 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3267 switch (_hash) { 3268 case 3575610: 3269 /* type */ return new Property("type", "string", "The type of extra detail provided in the value.", 0, 1, type); 3270 case -1410166417: 3271 /* value[x] */ return new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 1, 3272 value); 3273 case 111972721: 3274 /* value */ return new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 1, 3275 value); 3276 case -1424603934: 3277 /* valueString */ return new Property("value[x]", "string|base64Binary", "The value of the extra detail.", 0, 3278 1, value); 3279 case -1535024575: 3280 /* valueBase64Binary */ return new Property("value[x]", "string|base64Binary", 3281 "The value of the extra detail.", 0, 1, value); 3282 default: 3283 return super.getNamedProperty(_hash, _name, _checkValid); 3284 } 3285 3286 } 3287 3288 @Override 3289 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3290 switch (hash) { 3291 case 3575610: 3292 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // StringType 3293 case 111972721: 3294 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3295 default: 3296 return super.getProperty(hash, name, checkValid); 3297 } 3298 3299 } 3300 3301 @Override 3302 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3303 switch (hash) { 3304 case 3575610: // type 3305 this.type = castToString(value); // StringType 3306 return value; 3307 case 111972721: // value 3308 this.value = castToType(value); // Type 3309 return value; 3310 default: 3311 return super.setProperty(hash, name, value); 3312 } 3313 3314 } 3315 3316 @Override 3317 public Base setProperty(String name, Base value) throws FHIRException { 3318 if (name.equals("type")) { 3319 this.type = castToString(value); // StringType 3320 } else if (name.equals("value[x]")) { 3321 this.value = castToType(value); // Type 3322 } else 3323 return super.setProperty(name, value); 3324 return value; 3325 } 3326 3327 @Override 3328 public void removeChild(String name, Base value) throws FHIRException { 3329 if (name.equals("type")) { 3330 this.type = null; 3331 } else if (name.equals("value[x]")) { 3332 this.value = null; 3333 } else 3334 super.removeChild(name, value); 3335 3336 } 3337 3338 @Override 3339 public Base makeProperty(int hash, String name) throws FHIRException { 3340 switch (hash) { 3341 case 3575610: 3342 return getTypeElement(); 3343 case -1410166417: 3344 return getValue(); 3345 case 111972721: 3346 return getValue(); 3347 default: 3348 return super.makeProperty(hash, name); 3349 } 3350 3351 } 3352 3353 @Override 3354 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3355 switch (hash) { 3356 case 3575610: 3357 /* type */ return new String[] { "string" }; 3358 case 111972721: 3359 /* value */ return new String[] { "string", "base64Binary" }; 3360 default: 3361 return super.getTypesForProperty(hash, name); 3362 } 3363 3364 } 3365 3366 @Override 3367 public Base addChild(String name) throws FHIRException { 3368 if (name.equals("type")) { 3369 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 3370 } else if (name.equals("valueString")) { 3371 this.value = new StringType(); 3372 return this.value; 3373 } else if (name.equals("valueBase64Binary")) { 3374 this.value = new Base64BinaryType(); 3375 return this.value; 3376 } else 3377 return super.addChild(name); 3378 } 3379 3380 public AuditEventEntityDetailComponent copy() { 3381 AuditEventEntityDetailComponent dst = new AuditEventEntityDetailComponent(); 3382 copyValues(dst); 3383 return dst; 3384 } 3385 3386 public void copyValues(AuditEventEntityDetailComponent dst) { 3387 super.copyValues(dst); 3388 dst.type = type == null ? null : type.copy(); 3389 dst.value = value == null ? null : value.copy(); 3390 } 3391 3392 @Override 3393 public boolean equalsDeep(Base other_) { 3394 if (!super.equalsDeep(other_)) 3395 return false; 3396 if (!(other_ instanceof AuditEventEntityDetailComponent)) 3397 return false; 3398 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 3399 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3400 } 3401 3402 @Override 3403 public boolean equalsShallow(Base other_) { 3404 if (!super.equalsShallow(other_)) 3405 return false; 3406 if (!(other_ instanceof AuditEventEntityDetailComponent)) 3407 return false; 3408 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 3409 return compareValues(type, o.type, true); 3410 } 3411 3412 public boolean isEmpty() { 3413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3414 } 3415 3416 public String fhirType() { 3417 return "AuditEvent.entity.detail"; 3418 3419 } 3420 3421 } 3422 3423 /** 3424 * Identifier for a family of the event. For example, a menu item, program, 3425 * rule, policy, function code, application name or URL. It identifies the 3426 * performed function. 3427 */ 3428 @Child(name = "type", type = { Coding.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 3429 @Description(shortDefinition = "Type/identifier of event", formalDefinition = "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.") 3430 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-type") 3431 protected Coding type; 3432 3433 /** 3434 * Identifier for the category of event. 3435 */ 3436 @Child(name = "subtype", type = { 3437 Coding.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3438 @Description(shortDefinition = "More specific type/id for the event", formalDefinition = "Identifier for the category of event.") 3439 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-sub-type") 3440 protected List<Coding> subtype; 3441 3442 /** 3443 * Indicator for type of action performed during the event that generated the 3444 * audit. 3445 */ 3446 @Child(name = "action", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3447 @Description(shortDefinition = "Type of action performed during the event", formalDefinition = "Indicator for type of action performed during the event that generated the audit.") 3448 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-action") 3449 protected Enumeration<AuditEventAction> action; 3450 3451 /** 3452 * The period during which the activity occurred. 3453 */ 3454 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3455 @Description(shortDefinition = "When the activity occurred", formalDefinition = "The period during which the activity occurred.") 3456 protected Period period; 3457 3458 /** 3459 * The time when the event was recorded. 3460 */ 3461 @Child(name = "recorded", type = { InstantType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3462 @Description(shortDefinition = "Time when the event was recorded", formalDefinition = "The time when the event was recorded.") 3463 protected InstantType recorded; 3464 3465 /** 3466 * Indicates whether the event succeeded or failed. 3467 */ 3468 @Child(name = "outcome", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3469 @Description(shortDefinition = "Whether the event succeeded or failed", formalDefinition = "Indicates whether the event succeeded or failed.") 3470 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/audit-event-outcome") 3471 protected Enumeration<AuditEventOutcome> outcome; 3472 3473 /** 3474 * A free text description of the outcome of the event. 3475 */ 3476 @Child(name = "outcomeDesc", type = { 3477 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3478 @Description(shortDefinition = "Description of the event outcome", formalDefinition = "A free text description of the outcome of the event.") 3479 protected StringType outcomeDesc; 3480 3481 /** 3482 * The purposeOfUse (reason) that was used during the event being recorded. 3483 */ 3484 @Child(name = "purposeOfEvent", type = { 3485 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3486 @Description(shortDefinition = "The purposeOfUse of the event", formalDefinition = "The purposeOfUse (reason) that was used during the event being recorded.") 3487 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 3488 protected List<CodeableConcept> purposeOfEvent; 3489 3490 /** 3491 * An actor taking an active role in the event or activity that is logged. 3492 */ 3493 @Child(name = "agent", type = {}, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3494 @Description(shortDefinition = "Actor involved in the event", formalDefinition = "An actor taking an active role in the event or activity that is logged.") 3495 protected List<AuditEventAgentComponent> agent; 3496 3497 /** 3498 * The system that is reporting the event. 3499 */ 3500 @Child(name = "source", type = {}, order = 9, min = 1, max = 1, modifier = false, summary = false) 3501 @Description(shortDefinition = "Audit Event Reporter", formalDefinition = "The system that is reporting the event.") 3502 protected AuditEventSourceComponent source; 3503 3504 /** 3505 * Specific instances of data or objects that have been accessed. 3506 */ 3507 @Child(name = "entity", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3508 @Description(shortDefinition = "Data or objects used", formalDefinition = "Specific instances of data or objects that have been accessed.") 3509 protected List<AuditEventEntityComponent> entity; 3510 3511 private static final long serialVersionUID = 106433685L; 3512 3513 /** 3514 * Constructor 3515 */ 3516 public AuditEvent() { 3517 super(); 3518 } 3519 3520 /** 3521 * Constructor 3522 */ 3523 public AuditEvent(Coding type, InstantType recorded, AuditEventSourceComponent source) { 3524 super(); 3525 this.type = type; 3526 this.recorded = recorded; 3527 this.source = source; 3528 } 3529 3530 /** 3531 * @return {@link #type} (Identifier for a family of the event. For example, a 3532 * menu item, program, rule, policy, function code, application name or 3533 * URL. It identifies the performed function.) 3534 */ 3535 public Coding getType() { 3536 if (this.type == null) 3537 if (Configuration.errorOnAutoCreate()) 3538 throw new Error("Attempt to auto-create AuditEvent.type"); 3539 else if (Configuration.doAutoCreate()) 3540 this.type = new Coding(); // cc 3541 return this.type; 3542 } 3543 3544 public boolean hasType() { 3545 return this.type != null && !this.type.isEmpty(); 3546 } 3547 3548 /** 3549 * @param value {@link #type} (Identifier for a family of the event. For 3550 * example, a menu item, program, rule, policy, function code, 3551 * application name or URL. It identifies the performed function.) 3552 */ 3553 public AuditEvent setType(Coding value) { 3554 this.type = value; 3555 return this; 3556 } 3557 3558 /** 3559 * @return {@link #subtype} (Identifier for the category of event.) 3560 */ 3561 public List<Coding> getSubtype() { 3562 if (this.subtype == null) 3563 this.subtype = new ArrayList<Coding>(); 3564 return this.subtype; 3565 } 3566 3567 /** 3568 * @return Returns a reference to <code>this</code> for easy method chaining 3569 */ 3570 public AuditEvent setSubtype(List<Coding> theSubtype) { 3571 this.subtype = theSubtype; 3572 return this; 3573 } 3574 3575 public boolean hasSubtype() { 3576 if (this.subtype == null) 3577 return false; 3578 for (Coding item : this.subtype) 3579 if (!item.isEmpty()) 3580 return true; 3581 return false; 3582 } 3583 3584 public Coding addSubtype() { // 3 3585 Coding t = new Coding(); 3586 if (this.subtype == null) 3587 this.subtype = new ArrayList<Coding>(); 3588 this.subtype.add(t); 3589 return t; 3590 } 3591 3592 public AuditEvent addSubtype(Coding t) { // 3 3593 if (t == null) 3594 return this; 3595 if (this.subtype == null) 3596 this.subtype = new ArrayList<Coding>(); 3597 this.subtype.add(t); 3598 return this; 3599 } 3600 3601 /** 3602 * @return The first repetition of repeating field {@link #subtype}, creating it 3603 * if it does not already exist 3604 */ 3605 public Coding getSubtypeFirstRep() { 3606 if (getSubtype().isEmpty()) { 3607 addSubtype(); 3608 } 3609 return getSubtype().get(0); 3610 } 3611 3612 /** 3613 * @return {@link #action} (Indicator for type of action performed during the 3614 * event that generated the audit.). This is the underlying object with 3615 * id, value and extensions. The accessor "getAction" gives direct 3616 * access to the value 3617 */ 3618 public Enumeration<AuditEventAction> getActionElement() { 3619 if (this.action == null) 3620 if (Configuration.errorOnAutoCreate()) 3621 throw new Error("Attempt to auto-create AuditEvent.action"); 3622 else if (Configuration.doAutoCreate()) 3623 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); // bb 3624 return this.action; 3625 } 3626 3627 public boolean hasActionElement() { 3628 return this.action != null && !this.action.isEmpty(); 3629 } 3630 3631 public boolean hasAction() { 3632 return this.action != null && !this.action.isEmpty(); 3633 } 3634 3635 /** 3636 * @param value {@link #action} (Indicator for type of action performed during 3637 * the event that generated the audit.). This is the underlying 3638 * object with id, value and extensions. The accessor "getAction" 3639 * gives direct access to the value 3640 */ 3641 public AuditEvent setActionElement(Enumeration<AuditEventAction> value) { 3642 this.action = value; 3643 return this; 3644 } 3645 3646 /** 3647 * @return Indicator for type of action performed during the event that 3648 * generated the audit. 3649 */ 3650 public AuditEventAction getAction() { 3651 return this.action == null ? null : this.action.getValue(); 3652 } 3653 3654 /** 3655 * @param value Indicator for type of action performed during the event that 3656 * generated the audit. 3657 */ 3658 public AuditEvent setAction(AuditEventAction value) { 3659 if (value == null) 3660 this.action = null; 3661 else { 3662 if (this.action == null) 3663 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); 3664 this.action.setValue(value); 3665 } 3666 return this; 3667 } 3668 3669 /** 3670 * @return {@link #period} (The period during which the activity occurred.) 3671 */ 3672 public Period getPeriod() { 3673 if (this.period == null) 3674 if (Configuration.errorOnAutoCreate()) 3675 throw new Error("Attempt to auto-create AuditEvent.period"); 3676 else if (Configuration.doAutoCreate()) 3677 this.period = new Period(); // cc 3678 return this.period; 3679 } 3680 3681 public boolean hasPeriod() { 3682 return this.period != null && !this.period.isEmpty(); 3683 } 3684 3685 /** 3686 * @param value {@link #period} (The period during which the activity occurred.) 3687 */ 3688 public AuditEvent setPeriod(Period value) { 3689 this.period = value; 3690 return this; 3691 } 3692 3693 /** 3694 * @return {@link #recorded} (The time when the event was recorded.). This is 3695 * the underlying object with id, value and extensions. The accessor 3696 * "getRecorded" gives direct access to the value 3697 */ 3698 public InstantType getRecordedElement() { 3699 if (this.recorded == null) 3700 if (Configuration.errorOnAutoCreate()) 3701 throw new Error("Attempt to auto-create AuditEvent.recorded"); 3702 else if (Configuration.doAutoCreate()) 3703 this.recorded = new InstantType(); // bb 3704 return this.recorded; 3705 } 3706 3707 public boolean hasRecordedElement() { 3708 return this.recorded != null && !this.recorded.isEmpty(); 3709 } 3710 3711 public boolean hasRecorded() { 3712 return this.recorded != null && !this.recorded.isEmpty(); 3713 } 3714 3715 /** 3716 * @param value {@link #recorded} (The time when the event was recorded.). This 3717 * is the underlying object with id, value and extensions. The 3718 * accessor "getRecorded" gives direct access to the value 3719 */ 3720 public AuditEvent setRecordedElement(InstantType value) { 3721 this.recorded = value; 3722 return this; 3723 } 3724 3725 /** 3726 * @return The time when the event was recorded. 3727 */ 3728 public Date getRecorded() { 3729 return this.recorded == null ? null : this.recorded.getValue(); 3730 } 3731 3732 /** 3733 * @param value The time when the event was recorded. 3734 */ 3735 public AuditEvent setRecorded(Date value) { 3736 if (this.recorded == null) 3737 this.recorded = new InstantType(); 3738 this.recorded.setValue(value); 3739 return this; 3740 } 3741 3742 /** 3743 * @return {@link #outcome} (Indicates whether the event succeeded or failed.). 3744 * This is the underlying object with id, value and extensions. The 3745 * accessor "getOutcome" gives direct access to the value 3746 */ 3747 public Enumeration<AuditEventOutcome> getOutcomeElement() { 3748 if (this.outcome == null) 3749 if (Configuration.errorOnAutoCreate()) 3750 throw new Error("Attempt to auto-create AuditEvent.outcome"); 3751 else if (Configuration.doAutoCreate()) 3752 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); // bb 3753 return this.outcome; 3754 } 3755 3756 public boolean hasOutcomeElement() { 3757 return this.outcome != null && !this.outcome.isEmpty(); 3758 } 3759 3760 public boolean hasOutcome() { 3761 return this.outcome != null && !this.outcome.isEmpty(); 3762 } 3763 3764 /** 3765 * @param value {@link #outcome} (Indicates whether the event succeeded or 3766 * failed.). This is the underlying object with id, value and 3767 * extensions. The accessor "getOutcome" gives direct access to the 3768 * value 3769 */ 3770 public AuditEvent setOutcomeElement(Enumeration<AuditEventOutcome> value) { 3771 this.outcome = value; 3772 return this; 3773 } 3774 3775 /** 3776 * @return Indicates whether the event succeeded or failed. 3777 */ 3778 public AuditEventOutcome getOutcome() { 3779 return this.outcome == null ? null : this.outcome.getValue(); 3780 } 3781 3782 /** 3783 * @param value Indicates whether the event succeeded or failed. 3784 */ 3785 public AuditEvent setOutcome(AuditEventOutcome value) { 3786 if (value == null) 3787 this.outcome = null; 3788 else { 3789 if (this.outcome == null) 3790 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); 3791 this.outcome.setValue(value); 3792 } 3793 return this; 3794 } 3795 3796 /** 3797 * @return {@link #outcomeDesc} (A free text description of the outcome of the 3798 * event.). This is the underlying object with id, value and extensions. 3799 * The accessor "getOutcomeDesc" gives direct access to the value 3800 */ 3801 public StringType getOutcomeDescElement() { 3802 if (this.outcomeDesc == null) 3803 if (Configuration.errorOnAutoCreate()) 3804 throw new Error("Attempt to auto-create AuditEvent.outcomeDesc"); 3805 else if (Configuration.doAutoCreate()) 3806 this.outcomeDesc = new StringType(); // bb 3807 return this.outcomeDesc; 3808 } 3809 3810 public boolean hasOutcomeDescElement() { 3811 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 3812 } 3813 3814 public boolean hasOutcomeDesc() { 3815 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 3816 } 3817 3818 /** 3819 * @param value {@link #outcomeDesc} (A free text description of the outcome of 3820 * the event.). This is the underlying object with id, value and 3821 * extensions. The accessor "getOutcomeDesc" gives direct access to 3822 * the value 3823 */ 3824 public AuditEvent setOutcomeDescElement(StringType value) { 3825 this.outcomeDesc = value; 3826 return this; 3827 } 3828 3829 /** 3830 * @return A free text description of the outcome of the event. 3831 */ 3832 public String getOutcomeDesc() { 3833 return this.outcomeDesc == null ? null : this.outcomeDesc.getValue(); 3834 } 3835 3836 /** 3837 * @param value A free text description of the outcome of the event. 3838 */ 3839 public AuditEvent setOutcomeDesc(String value) { 3840 if (Utilities.noString(value)) 3841 this.outcomeDesc = null; 3842 else { 3843 if (this.outcomeDesc == null) 3844 this.outcomeDesc = new StringType(); 3845 this.outcomeDesc.setValue(value); 3846 } 3847 return this; 3848 } 3849 3850 /** 3851 * @return {@link #purposeOfEvent} (The purposeOfUse (reason) that was used 3852 * during the event being recorded.) 3853 */ 3854 public List<CodeableConcept> getPurposeOfEvent() { 3855 if (this.purposeOfEvent == null) 3856 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3857 return this.purposeOfEvent; 3858 } 3859 3860 /** 3861 * @return Returns a reference to <code>this</code> for easy method chaining 3862 */ 3863 public AuditEvent setPurposeOfEvent(List<CodeableConcept> thePurposeOfEvent) { 3864 this.purposeOfEvent = thePurposeOfEvent; 3865 return this; 3866 } 3867 3868 public boolean hasPurposeOfEvent() { 3869 if (this.purposeOfEvent == null) 3870 return false; 3871 for (CodeableConcept item : this.purposeOfEvent) 3872 if (!item.isEmpty()) 3873 return true; 3874 return false; 3875 } 3876 3877 public CodeableConcept addPurposeOfEvent() { // 3 3878 CodeableConcept t = new CodeableConcept(); 3879 if (this.purposeOfEvent == null) 3880 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3881 this.purposeOfEvent.add(t); 3882 return t; 3883 } 3884 3885 public AuditEvent addPurposeOfEvent(CodeableConcept t) { // 3 3886 if (t == null) 3887 return this; 3888 if (this.purposeOfEvent == null) 3889 this.purposeOfEvent = new ArrayList<CodeableConcept>(); 3890 this.purposeOfEvent.add(t); 3891 return this; 3892 } 3893 3894 /** 3895 * @return The first repetition of repeating field {@link #purposeOfEvent}, 3896 * creating it if it does not already exist 3897 */ 3898 public CodeableConcept getPurposeOfEventFirstRep() { 3899 if (getPurposeOfEvent().isEmpty()) { 3900 addPurposeOfEvent(); 3901 } 3902 return getPurposeOfEvent().get(0); 3903 } 3904 3905 /** 3906 * @return {@link #agent} (An actor taking an active role in the event or 3907 * activity that is logged.) 3908 */ 3909 public List<AuditEventAgentComponent> getAgent() { 3910 if (this.agent == null) 3911 this.agent = new ArrayList<AuditEventAgentComponent>(); 3912 return this.agent; 3913 } 3914 3915 /** 3916 * @return Returns a reference to <code>this</code> for easy method chaining 3917 */ 3918 public AuditEvent setAgent(List<AuditEventAgentComponent> theAgent) { 3919 this.agent = theAgent; 3920 return this; 3921 } 3922 3923 public boolean hasAgent() { 3924 if (this.agent == null) 3925 return false; 3926 for (AuditEventAgentComponent item : this.agent) 3927 if (!item.isEmpty()) 3928 return true; 3929 return false; 3930 } 3931 3932 public AuditEventAgentComponent addAgent() { // 3 3933 AuditEventAgentComponent t = new AuditEventAgentComponent(); 3934 if (this.agent == null) 3935 this.agent = new ArrayList<AuditEventAgentComponent>(); 3936 this.agent.add(t); 3937 return t; 3938 } 3939 3940 public AuditEvent addAgent(AuditEventAgentComponent t) { // 3 3941 if (t == null) 3942 return this; 3943 if (this.agent == null) 3944 this.agent = new ArrayList<AuditEventAgentComponent>(); 3945 this.agent.add(t); 3946 return this; 3947 } 3948 3949 /** 3950 * @return The first repetition of repeating field {@link #agent}, creating it 3951 * if it does not already exist 3952 */ 3953 public AuditEventAgentComponent getAgentFirstRep() { 3954 if (getAgent().isEmpty()) { 3955 addAgent(); 3956 } 3957 return getAgent().get(0); 3958 } 3959 3960 /** 3961 * @return {@link #source} (The system that is reporting the event.) 3962 */ 3963 public AuditEventSourceComponent getSource() { 3964 if (this.source == null) 3965 if (Configuration.errorOnAutoCreate()) 3966 throw new Error("Attempt to auto-create AuditEvent.source"); 3967 else if (Configuration.doAutoCreate()) 3968 this.source = new AuditEventSourceComponent(); // cc 3969 return this.source; 3970 } 3971 3972 public boolean hasSource() { 3973 return this.source != null && !this.source.isEmpty(); 3974 } 3975 3976 /** 3977 * @param value {@link #source} (The system that is reporting the event.) 3978 */ 3979 public AuditEvent setSource(AuditEventSourceComponent value) { 3980 this.source = value; 3981 return this; 3982 } 3983 3984 /** 3985 * @return {@link #entity} (Specific instances of data or objects that have been 3986 * accessed.) 3987 */ 3988 public List<AuditEventEntityComponent> getEntity() { 3989 if (this.entity == null) 3990 this.entity = new ArrayList<AuditEventEntityComponent>(); 3991 return this.entity; 3992 } 3993 3994 /** 3995 * @return Returns a reference to <code>this</code> for easy method chaining 3996 */ 3997 public AuditEvent setEntity(List<AuditEventEntityComponent> theEntity) { 3998 this.entity = theEntity; 3999 return this; 4000 } 4001 4002 public boolean hasEntity() { 4003 if (this.entity == null) 4004 return false; 4005 for (AuditEventEntityComponent item : this.entity) 4006 if (!item.isEmpty()) 4007 return true; 4008 return false; 4009 } 4010 4011 public AuditEventEntityComponent addEntity() { // 3 4012 AuditEventEntityComponent t = new AuditEventEntityComponent(); 4013 if (this.entity == null) 4014 this.entity = new ArrayList<AuditEventEntityComponent>(); 4015 this.entity.add(t); 4016 return t; 4017 } 4018 4019 public AuditEvent addEntity(AuditEventEntityComponent t) { // 3 4020 if (t == null) 4021 return this; 4022 if (this.entity == null) 4023 this.entity = new ArrayList<AuditEventEntityComponent>(); 4024 this.entity.add(t); 4025 return this; 4026 } 4027 4028 /** 4029 * @return The first repetition of repeating field {@link #entity}, creating it 4030 * if it does not already exist 4031 */ 4032 public AuditEventEntityComponent getEntityFirstRep() { 4033 if (getEntity().isEmpty()) { 4034 addEntity(); 4035 } 4036 return getEntity().get(0); 4037 } 4038 4039 protected void listChildren(List<Property> children) { 4040 super.listChildren(children); 4041 children.add(new Property("type", "Coding", 4042 "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 4043 0, 1, type)); 4044 children.add(new Property("subtype", "Coding", "Identifier for the category of event.", 0, 4045 java.lang.Integer.MAX_VALUE, subtype)); 4046 children.add(new Property("action", "code", 4047 "Indicator for type of action performed during the event that generated the audit.", 0, 1, action)); 4048 children.add(new Property("period", "Period", "The period during which the activity occurred.", 0, 1, period)); 4049 children.add(new Property("recorded", "instant", "The time when the event was recorded.", 0, 1, recorded)); 4050 children.add(new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 1, outcome)); 4051 children.add(new Property("outcomeDesc", "string", "A free text description of the outcome of the event.", 0, 1, 4052 outcomeDesc)); 4053 children.add(new Property("purposeOfEvent", "CodeableConcept", 4054 "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, 4055 purposeOfEvent)); 4056 children.add(new Property("agent", "", "An actor taking an active role in the event or activity that is logged.", 0, 4057 java.lang.Integer.MAX_VALUE, agent)); 4058 children.add(new Property("source", "", "The system that is reporting the event.", 0, 1, source)); 4059 children.add(new Property("entity", "", "Specific instances of data or objects that have been accessed.", 0, 4060 java.lang.Integer.MAX_VALUE, entity)); 4061 } 4062 4063 @Override 4064 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4065 switch (_hash) { 4066 case 3575610: 4067 /* type */ return new Property("type", "Coding", 4068 "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 4069 0, 1, type); 4070 case -1867567750: 4071 /* subtype */ return new Property("subtype", "Coding", "Identifier for the category of event.", 0, 4072 java.lang.Integer.MAX_VALUE, subtype); 4073 case -1422950858: 4074 /* action */ return new Property("action", "code", 4075 "Indicator for type of action performed during the event that generated the audit.", 0, 1, action); 4076 case -991726143: 4077 /* period */ return new Property("period", "Period", "The period during which the activity occurred.", 0, 1, 4078 period); 4079 case -799233872: 4080 /* recorded */ return new Property("recorded", "instant", "The time when the event was recorded.", 0, 1, 4081 recorded); 4082 case -1106507950: 4083 /* outcome */ return new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 1, 4084 outcome); 4085 case 1062502659: 4086 /* outcomeDesc */ return new Property("outcomeDesc", "string", 4087 "A free text description of the outcome of the event.", 0, 1, outcomeDesc); 4088 case -341917691: 4089 /* purposeOfEvent */ return new Property("purposeOfEvent", "CodeableConcept", 4090 "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, 4091 purposeOfEvent); 4092 case 92750597: 4093 /* agent */ return new Property("agent", "", 4094 "An actor taking an active role in the event or activity that is logged.", 0, java.lang.Integer.MAX_VALUE, 4095 agent); 4096 case -896505829: 4097 /* source */ return new Property("source", "", "The system that is reporting the event.", 0, 1, source); 4098 case -1298275357: 4099 /* entity */ return new Property("entity", "", "Specific instances of data or objects that have been accessed.", 4100 0, java.lang.Integer.MAX_VALUE, entity); 4101 default: 4102 return super.getNamedProperty(_hash, _name, _checkValid); 4103 } 4104 4105 } 4106 4107 @Override 4108 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4109 switch (hash) { 4110 case 3575610: 4111 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 4112 case -1867567750: 4113 /* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // Coding 4114 case -1422950858: 4115 /* action */ return this.action == null ? new Base[0] : new Base[] { this.action }; // Enumeration<AuditEventAction> 4116 case -991726143: 4117 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 4118 case -799233872: 4119 /* recorded */ return this.recorded == null ? new Base[0] : new Base[] { this.recorded }; // InstantType 4120 case -1106507950: 4121 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<AuditEventOutcome> 4122 case 1062502659: 4123 /* outcomeDesc */ return this.outcomeDesc == null ? new Base[0] : new Base[] { this.outcomeDesc }; // StringType 4124 case -341917691: 4125 /* purposeOfEvent */ return this.purposeOfEvent == null ? new Base[0] 4126 : this.purposeOfEvent.toArray(new Base[this.purposeOfEvent.size()]); // CodeableConcept 4127 case 92750597: 4128 /* agent */ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // AuditEventAgentComponent 4129 case -896505829: 4130 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // AuditEventSourceComponent 4131 case -1298275357: 4132 /* entity */ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // AuditEventEntityComponent 4133 default: 4134 return super.getProperty(hash, name, checkValid); 4135 } 4136 4137 } 4138 4139 @Override 4140 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4141 switch (hash) { 4142 case 3575610: // type 4143 this.type = castToCoding(value); // Coding 4144 return value; 4145 case -1867567750: // subtype 4146 this.getSubtype().add(castToCoding(value)); // Coding 4147 return value; 4148 case -1422950858: // action 4149 value = new AuditEventActionEnumFactory().fromType(castToCode(value)); 4150 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 4151 return value; 4152 case -991726143: // period 4153 this.period = castToPeriod(value); // Period 4154 return value; 4155 case -799233872: // recorded 4156 this.recorded = castToInstant(value); // InstantType 4157 return value; 4158 case -1106507950: // outcome 4159 value = new AuditEventOutcomeEnumFactory().fromType(castToCode(value)); 4160 this.outcome = (Enumeration) value; // Enumeration<AuditEventOutcome> 4161 return value; 4162 case 1062502659: // outcomeDesc 4163 this.outcomeDesc = castToString(value); // StringType 4164 return value; 4165 case -341917691: // purposeOfEvent 4166 this.getPurposeOfEvent().add(castToCodeableConcept(value)); // CodeableConcept 4167 return value; 4168 case 92750597: // agent 4169 this.getAgent().add((AuditEventAgentComponent) value); // AuditEventAgentComponent 4170 return value; 4171 case -896505829: // source 4172 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 4173 return value; 4174 case -1298275357: // entity 4175 this.getEntity().add((AuditEventEntityComponent) value); // AuditEventEntityComponent 4176 return value; 4177 default: 4178 return super.setProperty(hash, name, value); 4179 } 4180 4181 } 4182 4183 @Override 4184 public Base setProperty(String name, Base value) throws FHIRException { 4185 if (name.equals("type")) { 4186 this.type = castToCoding(value); // Coding 4187 } else if (name.equals("subtype")) { 4188 this.getSubtype().add(castToCoding(value)); 4189 } else if (name.equals("action")) { 4190 value = new AuditEventActionEnumFactory().fromType(castToCode(value)); 4191 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 4192 } else if (name.equals("period")) { 4193 this.period = castToPeriod(value); // Period 4194 } else if (name.equals("recorded")) { 4195 this.recorded = castToInstant(value); // InstantType 4196 } else if (name.equals("outcome")) { 4197 value = new AuditEventOutcomeEnumFactory().fromType(castToCode(value)); 4198 this.outcome = (Enumeration) value; // Enumeration<AuditEventOutcome> 4199 } else if (name.equals("outcomeDesc")) { 4200 this.outcomeDesc = castToString(value); // StringType 4201 } else if (name.equals("purposeOfEvent")) { 4202 this.getPurposeOfEvent().add(castToCodeableConcept(value)); 4203 } else if (name.equals("agent")) { 4204 this.getAgent().add((AuditEventAgentComponent) value); 4205 } else if (name.equals("source")) { 4206 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 4207 } else if (name.equals("entity")) { 4208 this.getEntity().add((AuditEventEntityComponent) value); 4209 } else 4210 return super.setProperty(name, value); 4211 return value; 4212 } 4213 4214 @Override 4215 public void removeChild(String name, Base value) throws FHIRException { 4216 if (name.equals("type")) { 4217 this.type = null; 4218 } else if (name.equals("subtype")) { 4219 this.getSubtype().remove(castToCoding(value)); 4220 } else if (name.equals("action")) { 4221 this.action = null; 4222 } else if (name.equals("period")) { 4223 this.period = null; 4224 } else if (name.equals("recorded")) { 4225 this.recorded = null; 4226 } else if (name.equals("outcome")) { 4227 this.outcome = null; 4228 } else if (name.equals("outcomeDesc")) { 4229 this.outcomeDesc = null; 4230 } else if (name.equals("purposeOfEvent")) { 4231 this.getPurposeOfEvent().remove(castToCodeableConcept(value)); 4232 } else if (name.equals("agent")) { 4233 this.getAgent().remove((AuditEventAgentComponent) value); 4234 } else if (name.equals("source")) { 4235 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 4236 } else if (name.equals("entity")) { 4237 this.getEntity().remove((AuditEventEntityComponent) value); 4238 } else 4239 super.removeChild(name, value); 4240 4241 } 4242 4243 @Override 4244 public Base makeProperty(int hash, String name) throws FHIRException { 4245 switch (hash) { 4246 case 3575610: 4247 return getType(); 4248 case -1867567750: 4249 return addSubtype(); 4250 case -1422950858: 4251 return getActionElement(); 4252 case -991726143: 4253 return getPeriod(); 4254 case -799233872: 4255 return getRecordedElement(); 4256 case -1106507950: 4257 return getOutcomeElement(); 4258 case 1062502659: 4259 return getOutcomeDescElement(); 4260 case -341917691: 4261 return addPurposeOfEvent(); 4262 case 92750597: 4263 return addAgent(); 4264 case -896505829: 4265 return getSource(); 4266 case -1298275357: 4267 return addEntity(); 4268 default: 4269 return super.makeProperty(hash, name); 4270 } 4271 4272 } 4273 4274 @Override 4275 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4276 switch (hash) { 4277 case 3575610: 4278 /* type */ return new String[] { "Coding" }; 4279 case -1867567750: 4280 /* subtype */ return new String[] { "Coding" }; 4281 case -1422950858: 4282 /* action */ return new String[] { "code" }; 4283 case -991726143: 4284 /* period */ return new String[] { "Period" }; 4285 case -799233872: 4286 /* recorded */ return new String[] { "instant" }; 4287 case -1106507950: 4288 /* outcome */ return new String[] { "code" }; 4289 case 1062502659: 4290 /* outcomeDesc */ return new String[] { "string" }; 4291 case -341917691: 4292 /* purposeOfEvent */ return new String[] { "CodeableConcept" }; 4293 case 92750597: 4294 /* agent */ return new String[] {}; 4295 case -896505829: 4296 /* source */ return new String[] {}; 4297 case -1298275357: 4298 /* entity */ return new String[] {}; 4299 default: 4300 return super.getTypesForProperty(hash, name); 4301 } 4302 4303 } 4304 4305 @Override 4306 public Base addChild(String name) throws FHIRException { 4307 if (name.equals("type")) { 4308 this.type = new Coding(); 4309 return this.type; 4310 } else if (name.equals("subtype")) { 4311 return addSubtype(); 4312 } else if (name.equals("action")) { 4313 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.action"); 4314 } else if (name.equals("period")) { 4315 this.period = new Period(); 4316 return this.period; 4317 } else if (name.equals("recorded")) { 4318 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.recorded"); 4319 } else if (name.equals("outcome")) { 4320 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcome"); 4321 } else if (name.equals("outcomeDesc")) { 4322 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcomeDesc"); 4323 } else if (name.equals("purposeOfEvent")) { 4324 return addPurposeOfEvent(); 4325 } else if (name.equals("agent")) { 4326 return addAgent(); 4327 } else if (name.equals("source")) { 4328 this.source = new AuditEventSourceComponent(); 4329 return this.source; 4330 } else if (name.equals("entity")) { 4331 return addEntity(); 4332 } else 4333 return super.addChild(name); 4334 } 4335 4336 public String fhirType() { 4337 return "AuditEvent"; 4338 4339 } 4340 4341 public AuditEvent copy() { 4342 AuditEvent dst = new AuditEvent(); 4343 copyValues(dst); 4344 return dst; 4345 } 4346 4347 public void copyValues(AuditEvent dst) { 4348 super.copyValues(dst); 4349 dst.type = type == null ? null : type.copy(); 4350 if (subtype != null) { 4351 dst.subtype = new ArrayList<Coding>(); 4352 for (Coding i : subtype) 4353 dst.subtype.add(i.copy()); 4354 } 4355 ; 4356 dst.action = action == null ? null : action.copy(); 4357 dst.period = period == null ? null : period.copy(); 4358 dst.recorded = recorded == null ? null : recorded.copy(); 4359 dst.outcome = outcome == null ? null : outcome.copy(); 4360 dst.outcomeDesc = outcomeDesc == null ? null : outcomeDesc.copy(); 4361 if (purposeOfEvent != null) { 4362 dst.purposeOfEvent = new ArrayList<CodeableConcept>(); 4363 for (CodeableConcept i : purposeOfEvent) 4364 dst.purposeOfEvent.add(i.copy()); 4365 } 4366 ; 4367 if (agent != null) { 4368 dst.agent = new ArrayList<AuditEventAgentComponent>(); 4369 for (AuditEventAgentComponent i : agent) 4370 dst.agent.add(i.copy()); 4371 } 4372 ; 4373 dst.source = source == null ? null : source.copy(); 4374 if (entity != null) { 4375 dst.entity = new ArrayList<AuditEventEntityComponent>(); 4376 for (AuditEventEntityComponent i : entity) 4377 dst.entity.add(i.copy()); 4378 } 4379 ; 4380 } 4381 4382 protected AuditEvent typedCopy() { 4383 return copy(); 4384 } 4385 4386 @Override 4387 public boolean equalsDeep(Base other_) { 4388 if (!super.equalsDeep(other_)) 4389 return false; 4390 if (!(other_ instanceof AuditEvent)) 4391 return false; 4392 AuditEvent o = (AuditEvent) other_; 4393 return compareDeep(type, o.type, true) && compareDeep(subtype, o.subtype, true) 4394 && compareDeep(action, o.action, true) && compareDeep(period, o.period, true) 4395 && compareDeep(recorded, o.recorded, true) && compareDeep(outcome, o.outcome, true) 4396 && compareDeep(outcomeDesc, o.outcomeDesc, true) && compareDeep(purposeOfEvent, o.purposeOfEvent, true) 4397 && compareDeep(agent, o.agent, true) && compareDeep(source, o.source, true) 4398 && compareDeep(entity, o.entity, true); 4399 } 4400 4401 @Override 4402 public boolean equalsShallow(Base other_) { 4403 if (!super.equalsShallow(other_)) 4404 return false; 4405 if (!(other_ instanceof AuditEvent)) 4406 return false; 4407 AuditEvent o = (AuditEvent) other_; 4408 return compareValues(action, o.action, true) && compareValues(recorded, o.recorded, true) 4409 && compareValues(outcome, o.outcome, true) && compareValues(outcomeDesc, o.outcomeDesc, true); 4410 } 4411 4412 public boolean isEmpty() { 4413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subtype, action, period, recorded, outcome, 4414 outcomeDesc, purposeOfEvent, agent, source, entity); 4415 } 4416 4417 @Override 4418 public ResourceType getResourceType() { 4419 return ResourceType.AuditEvent; 4420 } 4421 4422 /** 4423 * Search parameter: <b>date</b> 4424 * <p> 4425 * Description: <b>Time when the event was recorded</b><br> 4426 * Type: <b>date</b><br> 4427 * Path: <b>AuditEvent.recorded</b><br> 4428 * </p> 4429 */ 4430 @SearchParamDefinition(name = "date", path = "AuditEvent.recorded", description = "Time when the event was recorded", type = "date") 4431 public static final String SP_DATE = "date"; 4432 /** 4433 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4434 * <p> 4435 * Description: <b>Time when the event was recorded</b><br> 4436 * Type: <b>date</b><br> 4437 * Path: <b>AuditEvent.recorded</b><br> 4438 * </p> 4439 */ 4440 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4441 SP_DATE); 4442 4443 /** 4444 * Search parameter: <b>entity-type</b> 4445 * <p> 4446 * Description: <b>Type of entity involved</b><br> 4447 * Type: <b>token</b><br> 4448 * Path: <b>AuditEvent.entity.type</b><br> 4449 * </p> 4450 */ 4451 @SearchParamDefinition(name = "entity-type", path = "AuditEvent.entity.type", description = "Type of entity involved", type = "token") 4452 public static final String SP_ENTITY_TYPE = "entity-type"; 4453 /** 4454 * <b>Fluent Client</b> search parameter constant for <b>entity-type</b> 4455 * <p> 4456 * Description: <b>Type of entity involved</b><br> 4457 * Type: <b>token</b><br> 4458 * Path: <b>AuditEvent.entity.type</b><br> 4459 * </p> 4460 */ 4461 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4462 SP_ENTITY_TYPE); 4463 4464 /** 4465 * Search parameter: <b>agent</b> 4466 * <p> 4467 * Description: <b>Identifier of who</b><br> 4468 * Type: <b>reference</b><br> 4469 * Path: <b>AuditEvent.agent.who</b><br> 4470 * </p> 4471 */ 4472 @SearchParamDefinition(name = "agent", path = "AuditEvent.agent.who", description = "Identifier of who", type = "reference", providesMembershipIn = { 4473 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4474 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 4475 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4476 public static final String SP_AGENT = "agent"; 4477 /** 4478 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 4479 * <p> 4480 * Description: <b>Identifier of who</b><br> 4481 * Type: <b>reference</b><br> 4482 * Path: <b>AuditEvent.agent.who</b><br> 4483 * </p> 4484 */ 4485 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4486 SP_AGENT); 4487 4488 /** 4489 * Constant for fluent queries to be used to add include statements. Specifies 4490 * the path value of "<b>AuditEvent:agent</b>". 4491 */ 4492 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include( 4493 "AuditEvent:agent").toLocked(); 4494 4495 /** 4496 * Search parameter: <b>address</b> 4497 * <p> 4498 * Description: <b>Identifier for the network access point of the user 4499 * device</b><br> 4500 * Type: <b>string</b><br> 4501 * Path: <b>AuditEvent.agent.network.address</b><br> 4502 * </p> 4503 */ 4504 @SearchParamDefinition(name = "address", path = "AuditEvent.agent.network.address", description = "Identifier for the network access point of the user device", type = "string") 4505 public static final String SP_ADDRESS = "address"; 4506 /** 4507 * <b>Fluent Client</b> search parameter constant for <b>address</b> 4508 * <p> 4509 * Description: <b>Identifier for the network access point of the user 4510 * device</b><br> 4511 * Type: <b>string</b><br> 4512 * Path: <b>AuditEvent.agent.network.address</b><br> 4513 * </p> 4514 */ 4515 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 4516 SP_ADDRESS); 4517 4518 /** 4519 * Search parameter: <b>entity-role</b> 4520 * <p> 4521 * Description: <b>What role the entity played</b><br> 4522 * Type: <b>token</b><br> 4523 * Path: <b>AuditEvent.entity.role</b><br> 4524 * </p> 4525 */ 4526 @SearchParamDefinition(name = "entity-role", path = "AuditEvent.entity.role", description = "What role the entity played", type = "token") 4527 public static final String SP_ENTITY_ROLE = "entity-role"; 4528 /** 4529 * <b>Fluent Client</b> search parameter constant for <b>entity-role</b> 4530 * <p> 4531 * Description: <b>What role the entity played</b><br> 4532 * Type: <b>token</b><br> 4533 * Path: <b>AuditEvent.entity.role</b><br> 4534 * </p> 4535 */ 4536 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4537 SP_ENTITY_ROLE); 4538 4539 /** 4540 * Search parameter: <b>source</b> 4541 * <p> 4542 * Description: <b>The identity of source detecting the event</b><br> 4543 * Type: <b>reference</b><br> 4544 * Path: <b>AuditEvent.source.observer</b><br> 4545 * </p> 4546 */ 4547 @SearchParamDefinition(name = "source", path = "AuditEvent.source.observer", description = "The identity of source detecting the event", type = "reference", target = { 4548 Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 4549 RelatedPerson.class }) 4550 public static final String SP_SOURCE = "source"; 4551 /** 4552 * <b>Fluent Client</b> search parameter constant for <b>source</b> 4553 * <p> 4554 * Description: <b>The identity of source detecting the event</b><br> 4555 * Type: <b>reference</b><br> 4556 * Path: <b>AuditEvent.source.observer</b><br> 4557 * </p> 4558 */ 4559 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4560 SP_SOURCE); 4561 4562 /** 4563 * Constant for fluent queries to be used to add include statements. Specifies 4564 * the path value of "<b>AuditEvent:source</b>". 4565 */ 4566 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 4567 "AuditEvent:source").toLocked(); 4568 4569 /** 4570 * Search parameter: <b>type</b> 4571 * <p> 4572 * Description: <b>Type/identifier of event</b><br> 4573 * Type: <b>token</b><br> 4574 * Path: <b>AuditEvent.type</b><br> 4575 * </p> 4576 */ 4577 @SearchParamDefinition(name = "type", path = "AuditEvent.type", description = "Type/identifier of event", type = "token") 4578 public static final String SP_TYPE = "type"; 4579 /** 4580 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4581 * <p> 4582 * Description: <b>Type/identifier of event</b><br> 4583 * Type: <b>token</b><br> 4584 * Path: <b>AuditEvent.type</b><br> 4585 * </p> 4586 */ 4587 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4588 SP_TYPE); 4589 4590 /** 4591 * Search parameter: <b>altid</b> 4592 * <p> 4593 * Description: <b>Alternative User identity</b><br> 4594 * Type: <b>token</b><br> 4595 * Path: <b>AuditEvent.agent.altId</b><br> 4596 * </p> 4597 */ 4598 @SearchParamDefinition(name = "altid", path = "AuditEvent.agent.altId", description = "Alternative User identity", type = "token") 4599 public static final String SP_ALTID = "altid"; 4600 /** 4601 * <b>Fluent Client</b> search parameter constant for <b>altid</b> 4602 * <p> 4603 * Description: <b>Alternative User identity</b><br> 4604 * Type: <b>token</b><br> 4605 * Path: <b>AuditEvent.agent.altId</b><br> 4606 * </p> 4607 */ 4608 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ALTID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4609 SP_ALTID); 4610 4611 /** 4612 * Search parameter: <b>site</b> 4613 * <p> 4614 * Description: <b>Logical source location within the enterprise</b><br> 4615 * Type: <b>token</b><br> 4616 * Path: <b>AuditEvent.source.site</b><br> 4617 * </p> 4618 */ 4619 @SearchParamDefinition(name = "site", path = "AuditEvent.source.site", description = "Logical source location within the enterprise", type = "token") 4620 public static final String SP_SITE = "site"; 4621 /** 4622 * <b>Fluent Client</b> search parameter constant for <b>site</b> 4623 * <p> 4624 * Description: <b>Logical source location within the enterprise</b><br> 4625 * Type: <b>token</b><br> 4626 * Path: <b>AuditEvent.source.site</b><br> 4627 * </p> 4628 */ 4629 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4630 SP_SITE); 4631 4632 /** 4633 * Search parameter: <b>agent-name</b> 4634 * <p> 4635 * Description: <b>Human friendly name for the agent</b><br> 4636 * Type: <b>string</b><br> 4637 * Path: <b>AuditEvent.agent.name</b><br> 4638 * </p> 4639 */ 4640 @SearchParamDefinition(name = "agent-name", path = "AuditEvent.agent.name", description = "Human friendly name for the agent", type = "string") 4641 public static final String SP_AGENT_NAME = "agent-name"; 4642 /** 4643 * <b>Fluent Client</b> search parameter constant for <b>agent-name</b> 4644 * <p> 4645 * Description: <b>Human friendly name for the agent</b><br> 4646 * Type: <b>string</b><br> 4647 * Path: <b>AuditEvent.agent.name</b><br> 4648 * </p> 4649 */ 4650 public static final ca.uhn.fhir.rest.gclient.StringClientParam AGENT_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4651 SP_AGENT_NAME); 4652 4653 /** 4654 * Search parameter: <b>entity-name</b> 4655 * <p> 4656 * Description: <b>Descriptor for entity</b><br> 4657 * Type: <b>string</b><br> 4658 * Path: <b>AuditEvent.entity.name</b><br> 4659 * </p> 4660 */ 4661 @SearchParamDefinition(name = "entity-name", path = "AuditEvent.entity.name", description = "Descriptor for entity", type = "string") 4662 public static final String SP_ENTITY_NAME = "entity-name"; 4663 /** 4664 * <b>Fluent Client</b> search parameter constant for <b>entity-name</b> 4665 * <p> 4666 * Description: <b>Descriptor for entity</b><br> 4667 * Type: <b>string</b><br> 4668 * Path: <b>AuditEvent.entity.name</b><br> 4669 * </p> 4670 */ 4671 public static final ca.uhn.fhir.rest.gclient.StringClientParam ENTITY_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4672 SP_ENTITY_NAME); 4673 4674 /** 4675 * Search parameter: <b>subtype</b> 4676 * <p> 4677 * Description: <b>More specific type/id for the event</b><br> 4678 * Type: <b>token</b><br> 4679 * Path: <b>AuditEvent.subtype</b><br> 4680 * </p> 4681 */ 4682 @SearchParamDefinition(name = "subtype", path = "AuditEvent.subtype", description = "More specific type/id for the event", type = "token") 4683 public static final String SP_SUBTYPE = "subtype"; 4684 /** 4685 * <b>Fluent Client</b> search parameter constant for <b>subtype</b> 4686 * <p> 4687 * Description: <b>More specific type/id for the event</b><br> 4688 * Type: <b>token</b><br> 4689 * Path: <b>AuditEvent.subtype</b><br> 4690 * </p> 4691 */ 4692 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4693 SP_SUBTYPE); 4694 4695 /** 4696 * Search parameter: <b>patient</b> 4697 * <p> 4698 * Description: <b>Identifier of who</b><br> 4699 * Type: <b>reference</b><br> 4700 * Path: <b>AuditEvent.agent.who, AuditEvent.entity.what</b><br> 4701 * </p> 4702 */ 4703 @SearchParamDefinition(name = "patient", path = "AuditEvent.agent.who.where(resolve() is Patient) | AuditEvent.entity.what.where(resolve() is Patient)", description = "Identifier of who", type = "reference", providesMembershipIn = { 4704 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4705 public static final String SP_PATIENT = "patient"; 4706 /** 4707 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4708 * <p> 4709 * Description: <b>Identifier of who</b><br> 4710 * Type: <b>reference</b><br> 4711 * Path: <b>AuditEvent.agent.who, AuditEvent.entity.what</b><br> 4712 * </p> 4713 */ 4714 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4715 SP_PATIENT); 4716 4717 /** 4718 * Constant for fluent queries to be used to add include statements. Specifies 4719 * the path value of "<b>AuditEvent:patient</b>". 4720 */ 4721 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4722 "AuditEvent:patient").toLocked(); 4723 4724 /** 4725 * Search parameter: <b>action</b> 4726 * <p> 4727 * Description: <b>Type of action performed during the event</b><br> 4728 * Type: <b>token</b><br> 4729 * Path: <b>AuditEvent.action</b><br> 4730 * </p> 4731 */ 4732 @SearchParamDefinition(name = "action", path = "AuditEvent.action", description = "Type of action performed during the event", type = "token") 4733 public static final String SP_ACTION = "action"; 4734 /** 4735 * <b>Fluent Client</b> search parameter constant for <b>action</b> 4736 * <p> 4737 * Description: <b>Type of action performed during the event</b><br> 4738 * Type: <b>token</b><br> 4739 * Path: <b>AuditEvent.action</b><br> 4740 * </p> 4741 */ 4742 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4743 SP_ACTION); 4744 4745 /** 4746 * Search parameter: <b>agent-role</b> 4747 * <p> 4748 * Description: <b>Agent role in the event</b><br> 4749 * Type: <b>token</b><br> 4750 * Path: <b>AuditEvent.agent.role</b><br> 4751 * </p> 4752 */ 4753 @SearchParamDefinition(name = "agent-role", path = "AuditEvent.agent.role", description = "Agent role in the event", type = "token") 4754 public static final String SP_AGENT_ROLE = "agent-role"; 4755 /** 4756 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 4757 * <p> 4758 * Description: <b>Agent role in the event</b><br> 4759 * Type: <b>token</b><br> 4760 * Path: <b>AuditEvent.agent.role</b><br> 4761 * </p> 4762 */ 4763 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4764 SP_AGENT_ROLE); 4765 4766 /** 4767 * Search parameter: <b>entity</b> 4768 * <p> 4769 * Description: <b>Specific instance of resource</b><br> 4770 * Type: <b>reference</b><br> 4771 * Path: <b>AuditEvent.entity.what</b><br> 4772 * </p> 4773 */ 4774 @SearchParamDefinition(name = "entity", path = "AuditEvent.entity.what", description = "Specific instance of resource", type = "reference") 4775 public static final String SP_ENTITY = "entity"; 4776 /** 4777 * <b>Fluent Client</b> search parameter constant for <b>entity</b> 4778 * <p> 4779 * Description: <b>Specific instance of resource</b><br> 4780 * Type: <b>reference</b><br> 4781 * Path: <b>AuditEvent.entity.what</b><br> 4782 * </p> 4783 */ 4784 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4785 SP_ENTITY); 4786 4787 /** 4788 * Constant for fluent queries to be used to add include statements. Specifies 4789 * the path value of "<b>AuditEvent:entity</b>". 4790 */ 4791 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY = new ca.uhn.fhir.model.api.Include( 4792 "AuditEvent:entity").toLocked(); 4793 4794 /** 4795 * Search parameter: <b>outcome</b> 4796 * <p> 4797 * Description: <b>Whether the event succeeded or failed</b><br> 4798 * Type: <b>token</b><br> 4799 * Path: <b>AuditEvent.outcome</b><br> 4800 * </p> 4801 */ 4802 @SearchParamDefinition(name = "outcome", path = "AuditEvent.outcome", description = "Whether the event succeeded or failed", type = "token") 4803 public static final String SP_OUTCOME = "outcome"; 4804 /** 4805 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 4806 * <p> 4807 * Description: <b>Whether the event succeeded or failed</b><br> 4808 * Type: <b>token</b><br> 4809 * Path: <b>AuditEvent.outcome</b><br> 4810 * </p> 4811 */ 4812 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4813 SP_OUTCOME); 4814 4815 /** 4816 * Search parameter: <b>policy</b> 4817 * <p> 4818 * Description: <b>Policy that authorized event</b><br> 4819 * Type: <b>uri</b><br> 4820 * Path: <b>AuditEvent.agent.policy</b><br> 4821 * </p> 4822 */ 4823 @SearchParamDefinition(name = "policy", path = "AuditEvent.agent.policy", description = "Policy that authorized event", type = "uri") 4824 public static final String SP_POLICY = "policy"; 4825 /** 4826 * <b>Fluent Client</b> search parameter constant for <b>policy</b> 4827 * <p> 4828 * Description: <b>Policy that authorized event</b><br> 4829 * Type: <b>uri</b><br> 4830 * Path: <b>AuditEvent.agent.policy</b><br> 4831 * </p> 4832 */ 4833 public static final ca.uhn.fhir.rest.gclient.UriClientParam POLICY = new ca.uhn.fhir.rest.gclient.UriClientParam( 4834 SP_POLICY); 4835 4836}