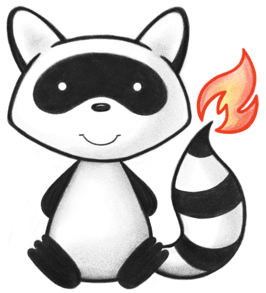
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IBaseBinary; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041 042/** 043 * A resource that represents the data of a single raw artifact as digital 044 * content accessible in its native format. A Binary resource can contain any 045 * content, whether text, image, pdf, zip archive, etc. 046 */ 047@ResourceDef(name = "Binary", profile = "http://hl7.org/fhir/StructureDefinition/Binary") 048public class Binary extends BaseBinary implements IBaseBinary { 049 050 /** 051 * MimeType of the binary content represented as a standard MimeType (BCP 13). 052 */ 053 @Child(name = "contentType", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "MimeType of the binary content", formalDefinition = "MimeType of the binary content represented as a standard MimeType (BCP 13).") 055 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 056 protected CodeType contentType; 057 058 /** 059 * This element identifies another resource that can be used as a proxy of the 060 * security sensitivity to use when deciding and enforcing access control rules 061 * for the Binary resource. Given that the Binary resource contains very few 062 * elements that can be used to determine the sensitivity of the data and 063 * relationships to individuals, the referenced resource stands in as a proxy 064 * equivalent for this purpose. This referenced resource may be related to the 065 * Binary (e.g. Media, DocumentReference), or may be some non-related Resource 066 * purely as a security proxy. E.g. to identify that the binary resource relates 067 * to a patient, and access should only be granted to applications that have 068 * access to the patient. 069 */ 070 @Child(name = "securityContext", type = { 071 Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Identifies another resource to use as proxy when enforcing access control", formalDefinition = "This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. Media, DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.") 073 protected Reference securityContext; 074 075 /** 076 * The actual object that is the target of the reference (This element 077 * identifies another resource that can be used as a proxy of the security 078 * sensitivity to use when deciding and enforcing access control rules for the 079 * Binary resource. Given that the Binary resource contains very few elements 080 * that can be used to determine the sensitivity of the data and relationships 081 * to individuals, the referenced resource stands in as a proxy equivalent for 082 * this purpose. This referenced resource may be related to the Binary (e.g. 083 * Media, DocumentReference), or may be some non-related Resource purely as a 084 * security proxy. E.g. to identify that the binary resource relates to a 085 * patient, and access should only be granted to applications that have access 086 * to the patient.) 087 */ 088 protected Resource securityContextTarget; 089 090 /** 091 * The actual content, base64 encoded. 092 */ 093 @Child(name = "data", type = { 094 Base64BinaryType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 095 @Description(shortDefinition = "The actual content", formalDefinition = "The actual content, base64 encoded.") 096 protected Base64BinaryType data; 097 098 private static final long serialVersionUID = 1353224198L; 099 100 /** 101 * Constructor 102 */ 103 public Binary() { 104 super(); 105 } 106 107 /** 108 * Constructor 109 */ 110 public Binary(CodeType contentType) { 111 super(); 112 this.contentType = contentType; 113 } 114 115 /** 116 * @return {@link #contentType} (MimeType of the binary content represented as a 117 * standard MimeType (BCP 13).). This is the underlying object with id, 118 * value and extensions. The accessor "getContentType" gives direct 119 * access to the value 120 */ 121 public CodeType getContentTypeElement() { 122 if (this.contentType == null) 123 if (Configuration.errorOnAutoCreate()) 124 throw new Error("Attempt to auto-create Binary.contentType"); 125 else if (Configuration.doAutoCreate()) 126 this.contentType = new CodeType(); // bb 127 return this.contentType; 128 } 129 130 public boolean hasContentTypeElement() { 131 return this.contentType != null && !this.contentType.isEmpty(); 132 } 133 134 public boolean hasContentType() { 135 return this.contentType != null && !this.contentType.isEmpty(); 136 } 137 138 /** 139 * @param value {@link #contentType} (MimeType of the binary content represented 140 * as a standard MimeType (BCP 13).). This is the underlying object 141 * with id, value and extensions. The accessor "getContentType" 142 * gives direct access to the value 143 */ 144 public Binary setContentTypeElement(CodeType value) { 145 this.contentType = value; 146 return this; 147 } 148 149 /** 150 * @return MimeType of the binary content represented as a standard MimeType 151 * (BCP 13). 152 */ 153 public String getContentType() { 154 return this.contentType == null ? null : this.contentType.getValue(); 155 } 156 157 /** 158 * @param value MimeType of the binary content represented as a standard 159 * MimeType (BCP 13). 160 */ 161 public Binary setContentType(String value) { 162 if (this.contentType == null) 163 this.contentType = new CodeType(); 164 this.contentType.setValue(value); 165 return this; 166 } 167 168 /** 169 * @return {@link #securityContext} (This element identifies another resource 170 * that can be used as a proxy of the security sensitivity to use when 171 * deciding and enforcing access control rules for the Binary resource. 172 * Given that the Binary resource contains very few elements that can be 173 * used to determine the sensitivity of the data and relationships to 174 * individuals, the referenced resource stands in as a proxy equivalent 175 * for this purpose. This referenced resource may be related to the 176 * Binary (e.g. Media, DocumentReference), or may be some non-related 177 * Resource purely as a security proxy. E.g. to identify that the binary 178 * resource relates to a patient, and access should only be granted to 179 * applications that have access to the patient.) 180 */ 181 public Reference getSecurityContext() { 182 if (this.securityContext == null) 183 if (Configuration.errorOnAutoCreate()) 184 throw new Error("Attempt to auto-create Binary.securityContext"); 185 else if (Configuration.doAutoCreate()) 186 this.securityContext = new Reference(); // cc 187 return this.securityContext; 188 } 189 190 public boolean hasSecurityContext() { 191 return this.securityContext != null && !this.securityContext.isEmpty(); 192 } 193 194 /** 195 * @param value {@link #securityContext} (This element identifies another 196 * resource that can be used as a proxy of the security sensitivity 197 * to use when deciding and enforcing access control rules for the 198 * Binary resource. Given that the Binary resource contains very 199 * few elements that can be used to determine the sensitivity of 200 * the data and relationships to individuals, the referenced 201 * resource stands in as a proxy equivalent for this purpose. This 202 * referenced resource may be related to the Binary (e.g. Media, 203 * DocumentReference), or may be some non-related Resource purely 204 * as a security proxy. E.g. to identify that the binary resource 205 * relates to a patient, and access should only be granted to 206 * applications that have access to the patient.) 207 */ 208 public Binary setSecurityContext(Reference value) { 209 this.securityContext = value; 210 return this; 211 } 212 213 /** 214 * @return {@link #securityContext} The actual object that is the target of the 215 * reference. The reference library doesn't populate this, but you can 216 * use it to hold the resource if you resolve it. (This element 217 * identifies another resource that can be used as a proxy of the 218 * security sensitivity to use when deciding and enforcing access 219 * control rules for the Binary resource. Given that the Binary resource 220 * contains very few elements that can be used to determine the 221 * sensitivity of the data and relationships to individuals, the 222 * referenced resource stands in as a proxy equivalent for this purpose. 223 * This referenced resource may be related to the Binary (e.g. Media, 224 * DocumentReference), or may be some non-related Resource purely as a 225 * security proxy. E.g. to identify that the binary resource relates to 226 * a patient, and access should only be granted to applications that 227 * have access to the patient.) 228 */ 229 public Resource getSecurityContextTarget() { 230 return this.securityContextTarget; 231 } 232 233 /** 234 * @param value {@link #securityContext} The actual object that is the target of 235 * the reference. The reference library doesn't use these, but you 236 * can use it to hold the resource if you resolve it. (This element 237 * identifies another resource that can be used as a proxy of the 238 * security sensitivity to use when deciding and enforcing access 239 * control rules for the Binary resource. Given that the Binary 240 * resource contains very few elements that can be used to 241 * determine the sensitivity of the data and relationships to 242 * individuals, the referenced resource stands in as a proxy 243 * equivalent for this purpose. This referenced resource may be 244 * related to the Binary (e.g. Media, DocumentReference), or may be 245 * some non-related Resource purely as a security proxy. E.g. to 246 * identify that the binary resource relates to a patient, and 247 * access should only be granted to applications that have access 248 * to the patient.) 249 */ 250 public Binary setSecurityContextTarget(Resource value) { 251 this.securityContextTarget = value; 252 return this; 253 } 254 255 /** 256 * @return {@link #data} (The actual content, base64 encoded.). This is the 257 * underlying object with id, value and extensions. The accessor 258 * "getData" gives direct access to the value 259 */ 260 public Base64BinaryType getDataElement() { 261 if (this.data == null) 262 if (Configuration.errorOnAutoCreate()) 263 throw new Error("Attempt to auto-create Binary.data"); 264 else if (Configuration.doAutoCreate()) 265 this.data = new Base64BinaryType(); // bb 266 return this.data; 267 } 268 269 public boolean hasDataElement() { 270 return this.data != null && !this.data.isEmpty(); 271 } 272 273 public boolean hasData() { 274 return this.data != null && !this.data.isEmpty(); 275 } 276 277 /** 278 * @param value {@link #data} (The actual content, base64 encoded.). This is the 279 * underlying object with id, value and extensions. The accessor 280 * "getData" gives direct access to the value 281 */ 282 public Binary setDataElement(Base64BinaryType value) { 283 this.data = value; 284 return this; 285 } 286 287 /** 288 * @return The actual content, base64 encoded. 289 */ 290 public byte[] getData() { 291 return this.data == null ? null : this.data.getValue(); 292 } 293 294 /** 295 * @param value The actual content, base64 encoded. 296 */ 297 public Binary setData(byte[] value) { 298 if (value == null) 299 this.data = null; 300 else { 301 if (this.data == null) 302 this.data = new Base64BinaryType(); 303 this.data.setValue(value); 304 } 305 return this; 306 } 307 308 protected void listChildren(List<Property> children) { 309 super.listChildren(children); 310 children.add(new Property("contentType", "code", 311 "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, 1, contentType)); 312 children.add(new Property("securityContext", "Reference(Any)", 313 "This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. Media, DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.", 314 0, 1, securityContext)); 315 children.add(new Property("data", "base64Binary", "The actual content, base64 encoded.", 0, 1, data)); 316 } 317 318 @Override 319 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 320 switch (_hash) { 321 case -389131437: 322 /* contentType */ return new Property("contentType", "code", 323 "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, 1, contentType); 324 case -1622888881: 325 /* securityContext */ return new Property("securityContext", "Reference(Any)", 326 "This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. Media, DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.", 327 0, 1, securityContext); 328 case 3076010: 329 /* data */ return new Property("data", "base64Binary", "The actual content, base64 encoded.", 0, 1, data); 330 default: 331 return super.getNamedProperty(_hash, _name, _checkValid); 332 } 333 334 } 335 336 @Override 337 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 338 switch (hash) { 339 case -389131437: 340 /* contentType */ return this.contentType == null ? new Base[0] : new Base[] { this.contentType }; // CodeType 341 case -1622888881: 342 /* securityContext */ return this.securityContext == null ? new Base[0] : new Base[] { this.securityContext }; // Reference 343 case 3076010: 344 /* data */ return this.data == null ? new Base[0] : new Base[] { this.data }; // Base64BinaryType 345 default: 346 return super.getProperty(hash, name, checkValid); 347 } 348 349 } 350 351 @Override 352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 353 switch (hash) { 354 case -389131437: // contentType 355 this.contentType = castToCode(value); // CodeType 356 return value; 357 case -1622888881: // securityContext 358 this.securityContext = castToReference(value); // Reference 359 return value; 360 case 3076010: // data 361 this.data = castToBase64Binary(value); // Base64BinaryType 362 return value; 363 default: 364 return super.setProperty(hash, name, value); 365 } 366 367 } 368 369 @Override 370 public Base setProperty(String name, Base value) throws FHIRException { 371 if (name.equals("contentType")) { 372 this.contentType = castToCode(value); // CodeType 373 } else if (name.equals("securityContext")) { 374 this.securityContext = castToReference(value); // Reference 375 } else if (name.equals("data")) { 376 this.data = castToBase64Binary(value); // Base64BinaryType 377 } else 378 return super.setProperty(name, value); 379 return value; 380 } 381 382 @Override 383 public void removeChild(String name, Base value) throws FHIRException { 384 if (name.equals("contentType")) { 385 this.contentType = null; 386 } else if (name.equals("securityContext")) { 387 this.securityContext = null; 388 } else if (name.equals("data")) { 389 this.data = null; 390 } else 391 super.removeChild(name, value); 392 393 } 394 395 @Override 396 public Base makeProperty(int hash, String name) throws FHIRException { 397 switch (hash) { 398 case -389131437: 399 return getContentTypeElement(); 400 case -1622888881: 401 return getSecurityContext(); 402 case 3076010: 403 return getDataElement(); 404 default: 405 return super.makeProperty(hash, name); 406 } 407 408 } 409 410 @Override 411 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 412 switch (hash) { 413 case -389131437: 414 /* contentType */ return new String[] { "code" }; 415 case -1622888881: 416 /* securityContext */ return new String[] { "Reference" }; 417 case 3076010: 418 /* data */ return new String[] { "base64Binary" }; 419 default: 420 return super.getTypesForProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public Base addChild(String name) throws FHIRException { 427 if (name.equals("contentType")) { 428 throw new FHIRException("Cannot call addChild on a singleton property Binary.contentType"); 429 } else if (name.equals("securityContext")) { 430 this.securityContext = new Reference(); 431 return this.securityContext; 432 } else if (name.equals("data")) { 433 throw new FHIRException("Cannot call addChild on a singleton property Binary.data"); 434 } else 435 return super.addChild(name); 436 } 437 438 public String fhirType() { 439 return "Binary"; 440 441 } 442 443 public Binary copy() { 444 Binary dst = new Binary(); 445 copyValues(dst); 446 return dst; 447 } 448 449 public void copyValues(Binary dst) { 450 super.copyValues(dst); 451 dst.contentType = contentType == null ? null : contentType.copy(); 452 dst.securityContext = securityContext == null ? null : securityContext.copy(); 453 dst.data = data == null ? null : data.copy(); 454 } 455 456 protected Binary typedCopy() { 457 return copy(); 458 } 459 460 @Override 461 public boolean equalsDeep(Base other_) { 462 if (!super.equalsDeep(other_)) 463 return false; 464 if (!(other_ instanceof Binary)) 465 return false; 466 Binary o = (Binary) other_; 467 return compareDeep(contentType, o.contentType, true) && compareDeep(securityContext, o.securityContext, true) 468 && compareDeep(data, o.data, true); 469 } 470 471 @Override 472 public boolean equalsShallow(Base other_) { 473 if (!super.equalsShallow(other_)) 474 return false; 475 if (!(other_ instanceof Binary)) 476 return false; 477 Binary o = (Binary) other_; 478 return compareValues(contentType, o.contentType, true) && compareValues(data, o.data, true); 479 } 480 481 public boolean isEmpty() { 482 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(contentType, securityContext, data); 483 } 484 485 @Override 486 public ResourceType getResourceType() { 487 return ResourceType.Binary; 488 } 489 490// added from java-adornments.txt: 491 492 @Override 493 public byte[] getContent() { 494 return getData(); 495 } 496 497 @Override 498 public IBaseBinary setContent(byte[] arg0) { 499 return setData(arg0); 500 } 501 502 @Override 503 public Base64BinaryType getContentElement() { 504 return getDataElement(); 505 } 506 507// end addition 508 509}