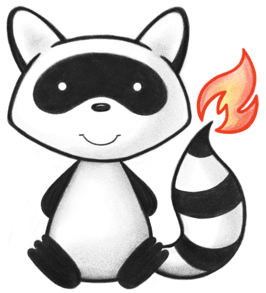
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046 047/** 048 * A material substance originating from a biological entity intended to be 049 * transplanted or infused into another (possibly the same) biological entity. 050 */ 051@ResourceDef(name = "BiologicallyDerivedProduct", profile = "http://hl7.org/fhir/StructureDefinition/BiologicallyDerivedProduct") 052public class BiologicallyDerivedProduct extends DomainResource { 053 054 public enum BiologicallyDerivedProductCategory { 055 /** 056 * A collection of tissues joined in a structural unit to serve a common 057 * function. 058 */ 059 ORGAN, 060 /** 061 * An ensemble of similar cells and their extracellular matrix from the same 062 * origin that together carry out a specific function. 063 */ 064 TISSUE, 065 /** 066 * Body fluid. 067 */ 068 FLUID, 069 /** 070 * Collection of cells. 071 */ 072 CELLS, 073 /** 074 * Biological agent of unspecified type. 075 */ 076 BIOLOGICALAGENT, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static BiologicallyDerivedProductCategory fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("organ".equals(codeString)) 086 return ORGAN; 087 if ("tissue".equals(codeString)) 088 return TISSUE; 089 if ("fluid".equals(codeString)) 090 return FLUID; 091 if ("cells".equals(codeString)) 092 return CELLS; 093 if ("biologicalAgent".equals(codeString)) 094 return BIOLOGICALAGENT; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown BiologicallyDerivedProductCategory code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case ORGAN: 104 return "organ"; 105 case TISSUE: 106 return "tissue"; 107 case FLUID: 108 return "fluid"; 109 case CELLS: 110 return "cells"; 111 case BIOLOGICALAGENT: 112 return "biologicalAgent"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case ORGAN: 123 return "http://hl7.org/fhir/product-category"; 124 case TISSUE: 125 return "http://hl7.org/fhir/product-category"; 126 case FLUID: 127 return "http://hl7.org/fhir/product-category"; 128 case CELLS: 129 return "http://hl7.org/fhir/product-category"; 130 case BIOLOGICALAGENT: 131 return "http://hl7.org/fhir/product-category"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case ORGAN: 142 return "A collection of tissues joined in a structural unit to serve a common function."; 143 case TISSUE: 144 return "An ensemble of similar cells and their extracellular matrix from the same origin that together carry out a specific function."; 145 case FLUID: 146 return "Body fluid."; 147 case CELLS: 148 return "Collection of cells."; 149 case BIOLOGICALAGENT: 150 return "Biological agent of unspecified type."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case ORGAN: 161 return "Organ"; 162 case TISSUE: 163 return "Tissue"; 164 case FLUID: 165 return "Fluid"; 166 case CELLS: 167 return "Cells"; 168 case BIOLOGICALAGENT: 169 return "BiologicalAgent"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class BiologicallyDerivedProductCategoryEnumFactory 179 implements EnumFactory<BiologicallyDerivedProductCategory> { 180 public BiologicallyDerivedProductCategory fromCode(String codeString) throws IllegalArgumentException { 181 if (codeString == null || "".equals(codeString)) 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("organ".equals(codeString)) 185 return BiologicallyDerivedProductCategory.ORGAN; 186 if ("tissue".equals(codeString)) 187 return BiologicallyDerivedProductCategory.TISSUE; 188 if ("fluid".equals(codeString)) 189 return BiologicallyDerivedProductCategory.FLUID; 190 if ("cells".equals(codeString)) 191 return BiologicallyDerivedProductCategory.CELLS; 192 if ("biologicalAgent".equals(codeString)) 193 return BiologicallyDerivedProductCategory.BIOLOGICALAGENT; 194 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductCategory code '" + codeString + "'"); 195 } 196 197 public Enumeration<BiologicallyDerivedProductCategory> fromType(PrimitiveType<?> code) throws FHIRException { 198 if (code == null) 199 return null; 200 if (code.isEmpty()) 201 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.NULL, code); 202 String codeString = code.asStringValue(); 203 if (codeString == null || "".equals(codeString)) 204 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.NULL, code); 205 if ("organ".equals(codeString)) 206 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.ORGAN, 207 code); 208 if ("tissue".equals(codeString)) 209 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.TISSUE, 210 code); 211 if ("fluid".equals(codeString)) 212 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.FLUID, 213 code); 214 if ("cells".equals(codeString)) 215 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.CELLS, 216 code); 217 if ("biologicalAgent".equals(codeString)) 218 return new Enumeration<BiologicallyDerivedProductCategory>(this, 219 BiologicallyDerivedProductCategory.BIOLOGICALAGENT, code); 220 throw new FHIRException("Unknown BiologicallyDerivedProductCategory code '" + codeString + "'"); 221 } 222 223 public String toCode(BiologicallyDerivedProductCategory code) { 224 if (code == BiologicallyDerivedProductCategory.ORGAN) 225 return "organ"; 226 if (code == BiologicallyDerivedProductCategory.TISSUE) 227 return "tissue"; 228 if (code == BiologicallyDerivedProductCategory.FLUID) 229 return "fluid"; 230 if (code == BiologicallyDerivedProductCategory.CELLS) 231 return "cells"; 232 if (code == BiologicallyDerivedProductCategory.BIOLOGICALAGENT) 233 return "biologicalAgent"; 234 return "?"; 235 } 236 237 public String toSystem(BiologicallyDerivedProductCategory code) { 238 return code.getSystem(); 239 } 240 } 241 242 public enum BiologicallyDerivedProductStatus { 243 /** 244 * Product is currently available for use. 245 */ 246 AVAILABLE, 247 /** 248 * Product is not currently available for use. 249 */ 250 UNAVAILABLE, 251 /** 252 * added to help the parsers with the generic types 253 */ 254 NULL; 255 256 public static BiologicallyDerivedProductStatus fromCode(String codeString) throws FHIRException { 257 if (codeString == null || "".equals(codeString)) 258 return null; 259 if ("available".equals(codeString)) 260 return AVAILABLE; 261 if ("unavailable".equals(codeString)) 262 return UNAVAILABLE; 263 if (Configuration.isAcceptInvalidEnums()) 264 return null; 265 else 266 throw new FHIRException("Unknown BiologicallyDerivedProductStatus code '" + codeString + "'"); 267 } 268 269 public String toCode() { 270 switch (this) { 271 case AVAILABLE: 272 return "available"; 273 case UNAVAILABLE: 274 return "unavailable"; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getSystem() { 283 switch (this) { 284 case AVAILABLE: 285 return "http://hl7.org/fhir/product-status"; 286 case UNAVAILABLE: 287 return "http://hl7.org/fhir/product-status"; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 295 public String getDefinition() { 296 switch (this) { 297 case AVAILABLE: 298 return "Product is currently available for use."; 299 case UNAVAILABLE: 300 return "Product is not currently available for use."; 301 case NULL: 302 return null; 303 default: 304 return "?"; 305 } 306 } 307 308 public String getDisplay() { 309 switch (this) { 310 case AVAILABLE: 311 return "Available"; 312 case UNAVAILABLE: 313 return "Unavailable"; 314 case NULL: 315 return null; 316 default: 317 return "?"; 318 } 319 } 320 } 321 322 public static class BiologicallyDerivedProductStatusEnumFactory 323 implements EnumFactory<BiologicallyDerivedProductStatus> { 324 public BiologicallyDerivedProductStatus fromCode(String codeString) throws IllegalArgumentException { 325 if (codeString == null || "".equals(codeString)) 326 if (codeString == null || "".equals(codeString)) 327 return null; 328 if ("available".equals(codeString)) 329 return BiologicallyDerivedProductStatus.AVAILABLE; 330 if ("unavailable".equals(codeString)) 331 return BiologicallyDerivedProductStatus.UNAVAILABLE; 332 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductStatus code '" + codeString + "'"); 333 } 334 335 public Enumeration<BiologicallyDerivedProductStatus> fromType(PrimitiveType<?> code) throws FHIRException { 336 if (code == null) 337 return null; 338 if (code.isEmpty()) 339 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.NULL, code); 340 String codeString = code.asStringValue(); 341 if (codeString == null || "".equals(codeString)) 342 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.NULL, code); 343 if ("available".equals(codeString)) 344 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.AVAILABLE, 345 code); 346 if ("unavailable".equals(codeString)) 347 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.UNAVAILABLE, 348 code); 349 throw new FHIRException("Unknown BiologicallyDerivedProductStatus code '" + codeString + "'"); 350 } 351 352 public String toCode(BiologicallyDerivedProductStatus code) { 353 if (code == BiologicallyDerivedProductStatus.AVAILABLE) 354 return "available"; 355 if (code == BiologicallyDerivedProductStatus.UNAVAILABLE) 356 return "unavailable"; 357 return "?"; 358 } 359 360 public String toSystem(BiologicallyDerivedProductStatus code) { 361 return code.getSystem(); 362 } 363 } 364 365 public enum BiologicallyDerivedProductStorageScale { 366 /** 367 * Fahrenheit temperature scale. 368 */ 369 FARENHEIT, 370 /** 371 * Celsius or centigrade temperature scale. 372 */ 373 CELSIUS, 374 /** 375 * Kelvin absolute thermodynamic temperature scale. 376 */ 377 KELVIN, 378 /** 379 * added to help the parsers with the generic types 380 */ 381 NULL; 382 383 public static BiologicallyDerivedProductStorageScale fromCode(String codeString) throws FHIRException { 384 if (codeString == null || "".equals(codeString)) 385 return null; 386 if ("farenheit".equals(codeString)) 387 return FARENHEIT; 388 if ("celsius".equals(codeString)) 389 return CELSIUS; 390 if ("kelvin".equals(codeString)) 391 return KELVIN; 392 if (Configuration.isAcceptInvalidEnums()) 393 return null; 394 else 395 throw new FHIRException("Unknown BiologicallyDerivedProductStorageScale code '" + codeString + "'"); 396 } 397 398 public String toCode() { 399 switch (this) { 400 case FARENHEIT: 401 return "farenheit"; 402 case CELSIUS: 403 return "celsius"; 404 case KELVIN: 405 return "kelvin"; 406 case NULL: 407 return null; 408 default: 409 return "?"; 410 } 411 } 412 413 public String getSystem() { 414 switch (this) { 415 case FARENHEIT: 416 return "http://hl7.org/fhir/product-storage-scale"; 417 case CELSIUS: 418 return "http://hl7.org/fhir/product-storage-scale"; 419 case KELVIN: 420 return "http://hl7.org/fhir/product-storage-scale"; 421 case NULL: 422 return null; 423 default: 424 return "?"; 425 } 426 } 427 428 public String getDefinition() { 429 switch (this) { 430 case FARENHEIT: 431 return "Fahrenheit temperature scale."; 432 case CELSIUS: 433 return "Celsius or centigrade temperature scale."; 434 case KELVIN: 435 return "Kelvin absolute thermodynamic temperature scale."; 436 case NULL: 437 return null; 438 default: 439 return "?"; 440 } 441 } 442 443 public String getDisplay() { 444 switch (this) { 445 case FARENHEIT: 446 return "Fahrenheit"; 447 case CELSIUS: 448 return "Celsius"; 449 case KELVIN: 450 return "Kelvin"; 451 case NULL: 452 return null; 453 default: 454 return "?"; 455 } 456 } 457 } 458 459 public static class BiologicallyDerivedProductStorageScaleEnumFactory 460 implements EnumFactory<BiologicallyDerivedProductStorageScale> { 461 public BiologicallyDerivedProductStorageScale fromCode(String codeString) throws IllegalArgumentException { 462 if (codeString == null || "".equals(codeString)) 463 if (codeString == null || "".equals(codeString)) 464 return null; 465 if ("farenheit".equals(codeString)) 466 return BiologicallyDerivedProductStorageScale.FARENHEIT; 467 if ("celsius".equals(codeString)) 468 return BiologicallyDerivedProductStorageScale.CELSIUS; 469 if ("kelvin".equals(codeString)) 470 return BiologicallyDerivedProductStorageScale.KELVIN; 471 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductStorageScale code '" + codeString + "'"); 472 } 473 474 public Enumeration<BiologicallyDerivedProductStorageScale> fromType(PrimitiveType<?> code) throws FHIRException { 475 if (code == null) 476 return null; 477 if (code.isEmpty()) 478 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 479 BiologicallyDerivedProductStorageScale.NULL, code); 480 String codeString = code.asStringValue(); 481 if (codeString == null || "".equals(codeString)) 482 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 483 BiologicallyDerivedProductStorageScale.NULL, code); 484 if ("farenheit".equals(codeString)) 485 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 486 BiologicallyDerivedProductStorageScale.FARENHEIT, code); 487 if ("celsius".equals(codeString)) 488 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 489 BiologicallyDerivedProductStorageScale.CELSIUS, code); 490 if ("kelvin".equals(codeString)) 491 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 492 BiologicallyDerivedProductStorageScale.KELVIN, code); 493 throw new FHIRException("Unknown BiologicallyDerivedProductStorageScale code '" + codeString + "'"); 494 } 495 496 public String toCode(BiologicallyDerivedProductStorageScale code) { 497 if (code == BiologicallyDerivedProductStorageScale.FARENHEIT) 498 return "farenheit"; 499 if (code == BiologicallyDerivedProductStorageScale.CELSIUS) 500 return "celsius"; 501 if (code == BiologicallyDerivedProductStorageScale.KELVIN) 502 return "kelvin"; 503 return "?"; 504 } 505 506 public String toSystem(BiologicallyDerivedProductStorageScale code) { 507 return code.getSystem(); 508 } 509 } 510 511 @Block() 512 public static class BiologicallyDerivedProductCollectionComponent extends BackboneElement 513 implements IBaseBackboneElement { 514 /** 515 * Healthcare professional who is performing the collection. 516 */ 517 @Child(name = "collector", type = { Practitioner.class, 518 PractitionerRole.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 519 @Description(shortDefinition = "Individual performing collection", formalDefinition = "Healthcare professional who is performing the collection.") 520 protected Reference collector; 521 522 /** 523 * The actual object that is the target of the reference (Healthcare 524 * professional who is performing the collection.) 525 */ 526 protected Resource collectorTarget; 527 528 /** 529 * The patient or entity, such as a hospital or vendor in the case of a 530 * processed/manipulated/manufactured product, providing the product. 531 */ 532 @Child(name = "source", type = { Patient.class, 533 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 534 @Description(shortDefinition = "Who is product from", formalDefinition = "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.") 535 protected Reference source; 536 537 /** 538 * The actual object that is the target of the reference (The patient or entity, 539 * such as a hospital or vendor in the case of a 540 * processed/manipulated/manufactured product, providing the product.) 541 */ 542 protected Resource sourceTarget; 543 544 /** 545 * Time of product collection. 546 */ 547 @Child(name = "collected", type = { DateTimeType.class, 548 Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 549 @Description(shortDefinition = "Time of product collection", formalDefinition = "Time of product collection.") 550 protected Type collected; 551 552 private static final long serialVersionUID = 892130089L; 553 554 /** 555 * Constructor 556 */ 557 public BiologicallyDerivedProductCollectionComponent() { 558 super(); 559 } 560 561 /** 562 * @return {@link #collector} (Healthcare professional who is performing the 563 * collection.) 564 */ 565 public Reference getCollector() { 566 if (this.collector == null) 567 if (Configuration.errorOnAutoCreate()) 568 throw new Error("Attempt to auto-create BiologicallyDerivedProductCollectionComponent.collector"); 569 else if (Configuration.doAutoCreate()) 570 this.collector = new Reference(); // cc 571 return this.collector; 572 } 573 574 public boolean hasCollector() { 575 return this.collector != null && !this.collector.isEmpty(); 576 } 577 578 /** 579 * @param value {@link #collector} (Healthcare professional who is performing 580 * the collection.) 581 */ 582 public BiologicallyDerivedProductCollectionComponent setCollector(Reference value) { 583 this.collector = value; 584 return this; 585 } 586 587 /** 588 * @return {@link #collector} The actual object that is the target of the 589 * reference. The reference library doesn't populate this, but you can 590 * use it to hold the resource if you resolve it. (Healthcare 591 * professional who is performing the collection.) 592 */ 593 public Resource getCollectorTarget() { 594 return this.collectorTarget; 595 } 596 597 /** 598 * @param value {@link #collector} The actual object that is the target of the 599 * reference. The reference library doesn't use these, but you can 600 * use it to hold the resource if you resolve it. (Healthcare 601 * professional who is performing the collection.) 602 */ 603 public BiologicallyDerivedProductCollectionComponent setCollectorTarget(Resource value) { 604 this.collectorTarget = value; 605 return this; 606 } 607 608 /** 609 * @return {@link #source} (The patient or entity, such as a hospital or vendor 610 * in the case of a processed/manipulated/manufactured product, 611 * providing the product.) 612 */ 613 public Reference getSource() { 614 if (this.source == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create BiologicallyDerivedProductCollectionComponent.source"); 617 else if (Configuration.doAutoCreate()) 618 this.source = new Reference(); // cc 619 return this.source; 620 } 621 622 public boolean hasSource() { 623 return this.source != null && !this.source.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #source} (The patient or entity, such as a hospital or 628 * vendor in the case of a processed/manipulated/manufactured 629 * product, providing the product.) 630 */ 631 public BiologicallyDerivedProductCollectionComponent setSource(Reference value) { 632 this.source = value; 633 return this; 634 } 635 636 /** 637 * @return {@link #source} The actual object that is the target of the 638 * reference. The reference library doesn't populate this, but you can 639 * use it to hold the resource if you resolve it. (The patient or 640 * entity, such as a hospital or vendor in the case of a 641 * processed/manipulated/manufactured product, providing the product.) 642 */ 643 public Resource getSourceTarget() { 644 return this.sourceTarget; 645 } 646 647 /** 648 * @param value {@link #source} The actual object that is the target of the 649 * reference. The reference library doesn't use these, but you can 650 * use it to hold the resource if you resolve it. (The patient or 651 * entity, such as a hospital or vendor in the case of a 652 * processed/manipulated/manufactured product, providing the 653 * product.) 654 */ 655 public BiologicallyDerivedProductCollectionComponent setSourceTarget(Resource value) { 656 this.sourceTarget = value; 657 return this; 658 } 659 660 /** 661 * @return {@link #collected} (Time of product collection.) 662 */ 663 public Type getCollected() { 664 return this.collected; 665 } 666 667 /** 668 * @return {@link #collected} (Time of product collection.) 669 */ 670 public DateTimeType getCollectedDateTimeType() throws FHIRException { 671 if (this.collected == null) 672 this.collected = new DateTimeType(); 673 if (!(this.collected instanceof DateTimeType)) 674 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 675 + this.collected.getClass().getName() + " was encountered"); 676 return (DateTimeType) this.collected; 677 } 678 679 public boolean hasCollectedDateTimeType() { 680 return this != null && this.collected instanceof DateTimeType; 681 } 682 683 /** 684 * @return {@link #collected} (Time of product collection.) 685 */ 686 public Period getCollectedPeriod() throws FHIRException { 687 if (this.collected == null) 688 this.collected = new Period(); 689 if (!(this.collected instanceof Period)) 690 throw new FHIRException("Type mismatch: the type Period was expected, but " 691 + this.collected.getClass().getName() + " was encountered"); 692 return (Period) this.collected; 693 } 694 695 public boolean hasCollectedPeriod() { 696 return this != null && this.collected instanceof Period; 697 } 698 699 public boolean hasCollected() { 700 return this.collected != null && !this.collected.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #collected} (Time of product collection.) 705 */ 706 public BiologicallyDerivedProductCollectionComponent setCollected(Type value) { 707 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 708 throw new Error( 709 "Not the right type for BiologicallyDerivedProduct.collection.collected[x]: " + value.fhirType()); 710 this.collected = value; 711 return this; 712 } 713 714 protected void listChildren(List<Property> children) { 715 super.listChildren(children); 716 children.add(new Property("collector", "Reference(Practitioner|PractitionerRole)", 717 "Healthcare professional who is performing the collection.", 0, 1, collector)); 718 children.add(new Property("source", "Reference(Patient|Organization)", 719 "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.", 720 0, 1, source)); 721 children.add(new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, collected)); 722 } 723 724 @Override 725 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 726 switch (_hash) { 727 case 1883491469: 728 /* collector */ return new Property("collector", "Reference(Practitioner|PractitionerRole)", 729 "Healthcare professional who is performing the collection.", 0, 1, collector); 730 case -896505829: 731 /* source */ return new Property("source", "Reference(Patient|Organization)", 732 "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.", 733 0, 1, source); 734 case 1632037015: 735 /* collected[x] */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, 736 collected); 737 case 1883491145: 738 /* collected */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, 739 collected); 740 case 2005009924: 741 /* collectedDateTime */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 742 1, collected); 743 case 653185642: 744 /* collectedPeriod */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 745 1, collected); 746 default: 747 return super.getNamedProperty(_hash, _name, _checkValid); 748 } 749 750 } 751 752 @Override 753 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 754 switch (hash) { 755 case 1883491469: 756 /* collector */ return this.collector == null ? new Base[0] : new Base[] { this.collector }; // Reference 757 case -896505829: 758 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 759 case 1883491145: 760 /* collected */ return this.collected == null ? new Base[0] : new Base[] { this.collected }; // Type 761 default: 762 return super.getProperty(hash, name, checkValid); 763 } 764 765 } 766 767 @Override 768 public Base setProperty(int hash, String name, Base value) throws FHIRException { 769 switch (hash) { 770 case 1883491469: // collector 771 this.collector = castToReference(value); // Reference 772 return value; 773 case -896505829: // source 774 this.source = castToReference(value); // Reference 775 return value; 776 case 1883491145: // collected 777 this.collected = castToType(value); // Type 778 return value; 779 default: 780 return super.setProperty(hash, name, value); 781 } 782 783 } 784 785 @Override 786 public Base setProperty(String name, Base value) throws FHIRException { 787 if (name.equals("collector")) { 788 this.collector = castToReference(value); // Reference 789 } else if (name.equals("source")) { 790 this.source = castToReference(value); // Reference 791 } else if (name.equals("collected[x]")) { 792 this.collected = castToType(value); // Type 793 } else 794 return super.setProperty(name, value); 795 return value; 796 } 797 798 @Override 799 public void removeChild(String name, Base value) throws FHIRException { 800 if (name.equals("collector")) { 801 this.collector = null; 802 } else if (name.equals("source")) { 803 this.source = null; 804 } else if (name.equals("collected[x]")) { 805 this.collected = null; 806 } else 807 super.removeChild(name, value); 808 809 } 810 811 @Override 812 public Base makeProperty(int hash, String name) throws FHIRException { 813 switch (hash) { 814 case 1883491469: 815 return getCollector(); 816 case -896505829: 817 return getSource(); 818 case 1632037015: 819 return getCollected(); 820 case 1883491145: 821 return getCollected(); 822 default: 823 return super.makeProperty(hash, name); 824 } 825 826 } 827 828 @Override 829 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 830 switch (hash) { 831 case 1883491469: 832 /* collector */ return new String[] { "Reference" }; 833 case -896505829: 834 /* source */ return new String[] { "Reference" }; 835 case 1883491145: 836 /* collected */ return new String[] { "dateTime", "Period" }; 837 default: 838 return super.getTypesForProperty(hash, name); 839 } 840 841 } 842 843 @Override 844 public Base addChild(String name) throws FHIRException { 845 if (name.equals("collector")) { 846 this.collector = new Reference(); 847 return this.collector; 848 } else if (name.equals("source")) { 849 this.source = new Reference(); 850 return this.source; 851 } else if (name.equals("collectedDateTime")) { 852 this.collected = new DateTimeType(); 853 return this.collected; 854 } else if (name.equals("collectedPeriod")) { 855 this.collected = new Period(); 856 return this.collected; 857 } else 858 return super.addChild(name); 859 } 860 861 public BiologicallyDerivedProductCollectionComponent copy() { 862 BiologicallyDerivedProductCollectionComponent dst = new BiologicallyDerivedProductCollectionComponent(); 863 copyValues(dst); 864 return dst; 865 } 866 867 public void copyValues(BiologicallyDerivedProductCollectionComponent dst) { 868 super.copyValues(dst); 869 dst.collector = collector == null ? null : collector.copy(); 870 dst.source = source == null ? null : source.copy(); 871 dst.collected = collected == null ? null : collected.copy(); 872 } 873 874 @Override 875 public boolean equalsDeep(Base other_) { 876 if (!super.equalsDeep(other_)) 877 return false; 878 if (!(other_ instanceof BiologicallyDerivedProductCollectionComponent)) 879 return false; 880 BiologicallyDerivedProductCollectionComponent o = (BiologicallyDerivedProductCollectionComponent) other_; 881 return compareDeep(collector, o.collector, true) && compareDeep(source, o.source, true) 882 && compareDeep(collected, o.collected, true); 883 } 884 885 @Override 886 public boolean equalsShallow(Base other_) { 887 if (!super.equalsShallow(other_)) 888 return false; 889 if (!(other_ instanceof BiologicallyDerivedProductCollectionComponent)) 890 return false; 891 BiologicallyDerivedProductCollectionComponent o = (BiologicallyDerivedProductCollectionComponent) other_; 892 return true; 893 } 894 895 public boolean isEmpty() { 896 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, source, collected); 897 } 898 899 public String fhirType() { 900 return "BiologicallyDerivedProduct.collection"; 901 902 } 903 904 } 905 906 @Block() 907 public static class BiologicallyDerivedProductProcessingComponent extends BackboneElement 908 implements IBaseBackboneElement { 909 /** 910 * Description of of processing. 911 */ 912 @Child(name = "description", type = { 913 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 914 @Description(shortDefinition = "Description of of processing", formalDefinition = "Description of of processing.") 915 protected StringType description; 916 917 /** 918 * Procesing code. 919 */ 920 @Child(name = "procedure", type = { 921 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 922 @Description(shortDefinition = "Procesing code", formalDefinition = "Procesing code.") 923 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 924 protected CodeableConcept procedure; 925 926 /** 927 * Substance added during processing. 928 */ 929 @Child(name = "additive", type = { 930 Substance.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 931 @Description(shortDefinition = "Substance added during processing", formalDefinition = "Substance added during processing.") 932 protected Reference additive; 933 934 /** 935 * The actual object that is the target of the reference (Substance added during 936 * processing.) 937 */ 938 protected Substance additiveTarget; 939 940 /** 941 * Time of processing. 942 */ 943 @Child(name = "time", type = { DateTimeType.class, 944 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 945 @Description(shortDefinition = "Time of processing", formalDefinition = "Time of processing.") 946 protected Type time; 947 948 private static final long serialVersionUID = -1007041216L; 949 950 /** 951 * Constructor 952 */ 953 public BiologicallyDerivedProductProcessingComponent() { 954 super(); 955 } 956 957 /** 958 * @return {@link #description} (Description of of processing.). This is the 959 * underlying object with id, value and extensions. The accessor 960 * "getDescription" gives direct access to the value 961 */ 962 public StringType getDescriptionElement() { 963 if (this.description == null) 964 if (Configuration.errorOnAutoCreate()) 965 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.description"); 966 else if (Configuration.doAutoCreate()) 967 this.description = new StringType(); // bb 968 return this.description; 969 } 970 971 public boolean hasDescriptionElement() { 972 return this.description != null && !this.description.isEmpty(); 973 } 974 975 public boolean hasDescription() { 976 return this.description != null && !this.description.isEmpty(); 977 } 978 979 /** 980 * @param value {@link #description} (Description of of processing.). This is 981 * the underlying object with id, value and extensions. The 982 * accessor "getDescription" gives direct access to the value 983 */ 984 public BiologicallyDerivedProductProcessingComponent setDescriptionElement(StringType value) { 985 this.description = value; 986 return this; 987 } 988 989 /** 990 * @return Description of of processing. 991 */ 992 public String getDescription() { 993 return this.description == null ? null : this.description.getValue(); 994 } 995 996 /** 997 * @param value Description of of processing. 998 */ 999 public BiologicallyDerivedProductProcessingComponent setDescription(String value) { 1000 if (Utilities.noString(value)) 1001 this.description = null; 1002 else { 1003 if (this.description == null) 1004 this.description = new StringType(); 1005 this.description.setValue(value); 1006 } 1007 return this; 1008 } 1009 1010 /** 1011 * @return {@link #procedure} (Procesing code.) 1012 */ 1013 public CodeableConcept getProcedure() { 1014 if (this.procedure == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.procedure"); 1017 else if (Configuration.doAutoCreate()) 1018 this.procedure = new CodeableConcept(); // cc 1019 return this.procedure; 1020 } 1021 1022 public boolean hasProcedure() { 1023 return this.procedure != null && !this.procedure.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #procedure} (Procesing code.) 1028 */ 1029 public BiologicallyDerivedProductProcessingComponent setProcedure(CodeableConcept value) { 1030 this.procedure = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #additive} (Substance added during processing.) 1036 */ 1037 public Reference getAdditive() { 1038 if (this.additive == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.additive"); 1041 else if (Configuration.doAutoCreate()) 1042 this.additive = new Reference(); // cc 1043 return this.additive; 1044 } 1045 1046 public boolean hasAdditive() { 1047 return this.additive != null && !this.additive.isEmpty(); 1048 } 1049 1050 /** 1051 * @param value {@link #additive} (Substance added during processing.) 1052 */ 1053 public BiologicallyDerivedProductProcessingComponent setAdditive(Reference value) { 1054 this.additive = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return {@link #additive} The actual object that is the target of the 1060 * reference. The reference library doesn't populate this, but you can 1061 * use it to hold the resource if you resolve it. (Substance added 1062 * during processing.) 1063 */ 1064 public Substance getAdditiveTarget() { 1065 if (this.additiveTarget == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.additive"); 1068 else if (Configuration.doAutoCreate()) 1069 this.additiveTarget = new Substance(); // aa 1070 return this.additiveTarget; 1071 } 1072 1073 /** 1074 * @param value {@link #additive} The actual object that is the target of the 1075 * reference. The reference library doesn't use these, but you can 1076 * use it to hold the resource if you resolve it. (Substance added 1077 * during processing.) 1078 */ 1079 public BiologicallyDerivedProductProcessingComponent setAdditiveTarget(Substance value) { 1080 this.additiveTarget = value; 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #time} (Time of processing.) 1086 */ 1087 public Type getTime() { 1088 return this.time; 1089 } 1090 1091 /** 1092 * @return {@link #time} (Time of processing.) 1093 */ 1094 public DateTimeType getTimeDateTimeType() throws FHIRException { 1095 if (this.time == null) 1096 this.time = new DateTimeType(); 1097 if (!(this.time instanceof DateTimeType)) 1098 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1099 + this.time.getClass().getName() + " was encountered"); 1100 return (DateTimeType) this.time; 1101 } 1102 1103 public boolean hasTimeDateTimeType() { 1104 return this != null && this.time instanceof DateTimeType; 1105 } 1106 1107 /** 1108 * @return {@link #time} (Time of processing.) 1109 */ 1110 public Period getTimePeriod() throws FHIRException { 1111 if (this.time == null) 1112 this.time = new Period(); 1113 if (!(this.time instanceof Period)) 1114 throw new FHIRException( 1115 "Type mismatch: the type Period was expected, but " + this.time.getClass().getName() + " was encountered"); 1116 return (Period) this.time; 1117 } 1118 1119 public boolean hasTimePeriod() { 1120 return this != null && this.time instanceof Period; 1121 } 1122 1123 public boolean hasTime() { 1124 return this.time != null && !this.time.isEmpty(); 1125 } 1126 1127 /** 1128 * @param value {@link #time} (Time of processing.) 1129 */ 1130 public BiologicallyDerivedProductProcessingComponent setTime(Type value) { 1131 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1132 throw new Error("Not the right type for BiologicallyDerivedProduct.processing.time[x]: " + value.fhirType()); 1133 this.time = value; 1134 return this; 1135 } 1136 1137 protected void listChildren(List<Property> children) { 1138 super.listChildren(children); 1139 children.add(new Property("description", "string", "Description of of processing.", 0, 1, description)); 1140 children.add(new Property("procedure", "CodeableConcept", "Procesing code.", 0, 1, procedure)); 1141 children 1142 .add(new Property("additive", "Reference(Substance)", "Substance added during processing.", 0, 1, additive)); 1143 children.add(new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time)); 1144 } 1145 1146 @Override 1147 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1148 switch (_hash) { 1149 case -1724546052: 1150 /* description */ return new Property("description", "string", "Description of of processing.", 0, 1, 1151 description); 1152 case -1095204141: 1153 /* procedure */ return new Property("procedure", "CodeableConcept", "Procesing code.", 0, 1, procedure); 1154 case -1226589236: 1155 /* additive */ return new Property("additive", "Reference(Substance)", "Substance added during processing.", 0, 1156 1, additive); 1157 case -1313930605: 1158 /* time[x] */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1159 case 3560141: 1160 /* time */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1161 case 2135345544: 1162 /* timeDateTime */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1163 case 693544686: 1164 /* timePeriod */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1165 default: 1166 return super.getNamedProperty(_hash, _name, _checkValid); 1167 } 1168 1169 } 1170 1171 @Override 1172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1173 switch (hash) { 1174 case -1724546052: 1175 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1176 case -1095204141: 1177 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // CodeableConcept 1178 case -1226589236: 1179 /* additive */ return this.additive == null ? new Base[0] : new Base[] { this.additive }; // Reference 1180 case 3560141: 1181 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // Type 1182 default: 1183 return super.getProperty(hash, name, checkValid); 1184 } 1185 1186 } 1187 1188 @Override 1189 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1190 switch (hash) { 1191 case -1724546052: // description 1192 this.description = castToString(value); // StringType 1193 return value; 1194 case -1095204141: // procedure 1195 this.procedure = castToCodeableConcept(value); // CodeableConcept 1196 return value; 1197 case -1226589236: // additive 1198 this.additive = castToReference(value); // Reference 1199 return value; 1200 case 3560141: // time 1201 this.time = castToType(value); // Type 1202 return value; 1203 default: 1204 return super.setProperty(hash, name, value); 1205 } 1206 1207 } 1208 1209 @Override 1210 public Base setProperty(String name, Base value) throws FHIRException { 1211 if (name.equals("description")) { 1212 this.description = castToString(value); // StringType 1213 } else if (name.equals("procedure")) { 1214 this.procedure = castToCodeableConcept(value); // CodeableConcept 1215 } else if (name.equals("additive")) { 1216 this.additive = castToReference(value); // Reference 1217 } else if (name.equals("time[x]")) { 1218 this.time = castToType(value); // Type 1219 } else 1220 return super.setProperty(name, value); 1221 return value; 1222 } 1223 1224 @Override 1225 public void removeChild(String name, Base value) throws FHIRException { 1226 if (name.equals("description")) { 1227 this.description = null; 1228 } else if (name.equals("procedure")) { 1229 this.procedure = null; 1230 } else if (name.equals("additive")) { 1231 this.additive = null; 1232 } else if (name.equals("time[x]")) { 1233 this.time = null; 1234 } else 1235 super.removeChild(name, value); 1236 1237 } 1238 1239 @Override 1240 public Base makeProperty(int hash, String name) throws FHIRException { 1241 switch (hash) { 1242 case -1724546052: 1243 return getDescriptionElement(); 1244 case -1095204141: 1245 return getProcedure(); 1246 case -1226589236: 1247 return getAdditive(); 1248 case -1313930605: 1249 return getTime(); 1250 case 3560141: 1251 return getTime(); 1252 default: 1253 return super.makeProperty(hash, name); 1254 } 1255 1256 } 1257 1258 @Override 1259 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1260 switch (hash) { 1261 case -1724546052: 1262 /* description */ return new String[] { "string" }; 1263 case -1095204141: 1264 /* procedure */ return new String[] { "CodeableConcept" }; 1265 case -1226589236: 1266 /* additive */ return new String[] { "Reference" }; 1267 case 3560141: 1268 /* time */ return new String[] { "dateTime", "Period" }; 1269 default: 1270 return super.getTypesForProperty(hash, name); 1271 } 1272 1273 } 1274 1275 @Override 1276 public Base addChild(String name) throws FHIRException { 1277 if (name.equals("description")) { 1278 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.description"); 1279 } else if (name.equals("procedure")) { 1280 this.procedure = new CodeableConcept(); 1281 return this.procedure; 1282 } else if (name.equals("additive")) { 1283 this.additive = new Reference(); 1284 return this.additive; 1285 } else if (name.equals("timeDateTime")) { 1286 this.time = new DateTimeType(); 1287 return this.time; 1288 } else if (name.equals("timePeriod")) { 1289 this.time = new Period(); 1290 return this.time; 1291 } else 1292 return super.addChild(name); 1293 } 1294 1295 public BiologicallyDerivedProductProcessingComponent copy() { 1296 BiologicallyDerivedProductProcessingComponent dst = new BiologicallyDerivedProductProcessingComponent(); 1297 copyValues(dst); 1298 return dst; 1299 } 1300 1301 public void copyValues(BiologicallyDerivedProductProcessingComponent dst) { 1302 super.copyValues(dst); 1303 dst.description = description == null ? null : description.copy(); 1304 dst.procedure = procedure == null ? null : procedure.copy(); 1305 dst.additive = additive == null ? null : additive.copy(); 1306 dst.time = time == null ? null : time.copy(); 1307 } 1308 1309 @Override 1310 public boolean equalsDeep(Base other_) { 1311 if (!super.equalsDeep(other_)) 1312 return false; 1313 if (!(other_ instanceof BiologicallyDerivedProductProcessingComponent)) 1314 return false; 1315 BiologicallyDerivedProductProcessingComponent o = (BiologicallyDerivedProductProcessingComponent) other_; 1316 return compareDeep(description, o.description, true) && compareDeep(procedure, o.procedure, true) 1317 && compareDeep(additive, o.additive, true) && compareDeep(time, o.time, true); 1318 } 1319 1320 @Override 1321 public boolean equalsShallow(Base other_) { 1322 if (!super.equalsShallow(other_)) 1323 return false; 1324 if (!(other_ instanceof BiologicallyDerivedProductProcessingComponent)) 1325 return false; 1326 BiologicallyDerivedProductProcessingComponent o = (BiologicallyDerivedProductProcessingComponent) other_; 1327 return compareValues(description, o.description, true); 1328 } 1329 1330 public boolean isEmpty() { 1331 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, procedure, additive, time); 1332 } 1333 1334 public String fhirType() { 1335 return "BiologicallyDerivedProduct.processing"; 1336 1337 } 1338 1339 } 1340 1341 @Block() 1342 public static class BiologicallyDerivedProductManipulationComponent extends BackboneElement 1343 implements IBaseBackboneElement { 1344 /** 1345 * Description of manipulation. 1346 */ 1347 @Child(name = "description", type = { 1348 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1349 @Description(shortDefinition = "Description of manipulation", formalDefinition = "Description of manipulation.") 1350 protected StringType description; 1351 1352 /** 1353 * Time of manipulation. 1354 */ 1355 @Child(name = "time", type = { DateTimeType.class, 1356 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1357 @Description(shortDefinition = "Time of manipulation", formalDefinition = "Time of manipulation.") 1358 protected Type time; 1359 1360 private static final long serialVersionUID = 717201078L; 1361 1362 /** 1363 * Constructor 1364 */ 1365 public BiologicallyDerivedProductManipulationComponent() { 1366 super(); 1367 } 1368 1369 /** 1370 * @return {@link #description} (Description of manipulation.). This is the 1371 * underlying object with id, value and extensions. The accessor 1372 * "getDescription" gives direct access to the value 1373 */ 1374 public StringType getDescriptionElement() { 1375 if (this.description == null) 1376 if (Configuration.errorOnAutoCreate()) 1377 throw new Error("Attempt to auto-create BiologicallyDerivedProductManipulationComponent.description"); 1378 else if (Configuration.doAutoCreate()) 1379 this.description = new StringType(); // bb 1380 return this.description; 1381 } 1382 1383 public boolean hasDescriptionElement() { 1384 return this.description != null && !this.description.isEmpty(); 1385 } 1386 1387 public boolean hasDescription() { 1388 return this.description != null && !this.description.isEmpty(); 1389 } 1390 1391 /** 1392 * @param value {@link #description} (Description of manipulation.). This is the 1393 * underlying object with id, value and extensions. The accessor 1394 * "getDescription" gives direct access to the value 1395 */ 1396 public BiologicallyDerivedProductManipulationComponent setDescriptionElement(StringType value) { 1397 this.description = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return Description of manipulation. 1403 */ 1404 public String getDescription() { 1405 return this.description == null ? null : this.description.getValue(); 1406 } 1407 1408 /** 1409 * @param value Description of manipulation. 1410 */ 1411 public BiologicallyDerivedProductManipulationComponent setDescription(String value) { 1412 if (Utilities.noString(value)) 1413 this.description = null; 1414 else { 1415 if (this.description == null) 1416 this.description = new StringType(); 1417 this.description.setValue(value); 1418 } 1419 return this; 1420 } 1421 1422 /** 1423 * @return {@link #time} (Time of manipulation.) 1424 */ 1425 public Type getTime() { 1426 return this.time; 1427 } 1428 1429 /** 1430 * @return {@link #time} (Time of manipulation.) 1431 */ 1432 public DateTimeType getTimeDateTimeType() throws FHIRException { 1433 if (this.time == null) 1434 this.time = new DateTimeType(); 1435 if (!(this.time instanceof DateTimeType)) 1436 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1437 + this.time.getClass().getName() + " was encountered"); 1438 return (DateTimeType) this.time; 1439 } 1440 1441 public boolean hasTimeDateTimeType() { 1442 return this != null && this.time instanceof DateTimeType; 1443 } 1444 1445 /** 1446 * @return {@link #time} (Time of manipulation.) 1447 */ 1448 public Period getTimePeriod() throws FHIRException { 1449 if (this.time == null) 1450 this.time = new Period(); 1451 if (!(this.time instanceof Period)) 1452 throw new FHIRException( 1453 "Type mismatch: the type Period was expected, but " + this.time.getClass().getName() + " was encountered"); 1454 return (Period) this.time; 1455 } 1456 1457 public boolean hasTimePeriod() { 1458 return this != null && this.time instanceof Period; 1459 } 1460 1461 public boolean hasTime() { 1462 return this.time != null && !this.time.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #time} (Time of manipulation.) 1467 */ 1468 public BiologicallyDerivedProductManipulationComponent setTime(Type value) { 1469 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1470 throw new Error("Not the right type for BiologicallyDerivedProduct.manipulation.time[x]: " + value.fhirType()); 1471 this.time = value; 1472 return this; 1473 } 1474 1475 protected void listChildren(List<Property> children) { 1476 super.listChildren(children); 1477 children.add(new Property("description", "string", "Description of manipulation.", 0, 1, description)); 1478 children.add(new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time)); 1479 } 1480 1481 @Override 1482 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1483 switch (_hash) { 1484 case -1724546052: 1485 /* description */ return new Property("description", "string", "Description of manipulation.", 0, 1, 1486 description); 1487 case -1313930605: 1488 /* time[x] */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1489 case 3560141: 1490 /* time */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1491 case 2135345544: 1492 /* timeDateTime */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1493 case 693544686: 1494 /* timePeriod */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1495 default: 1496 return super.getNamedProperty(_hash, _name, _checkValid); 1497 } 1498 1499 } 1500 1501 @Override 1502 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1503 switch (hash) { 1504 case -1724546052: 1505 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1506 case 3560141: 1507 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // Type 1508 default: 1509 return super.getProperty(hash, name, checkValid); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1516 switch (hash) { 1517 case -1724546052: // description 1518 this.description = castToString(value); // StringType 1519 return value; 1520 case 3560141: // time 1521 this.time = castToType(value); // Type 1522 return value; 1523 default: 1524 return super.setProperty(hash, name, value); 1525 } 1526 1527 } 1528 1529 @Override 1530 public Base setProperty(String name, Base value) throws FHIRException { 1531 if (name.equals("description")) { 1532 this.description = castToString(value); // StringType 1533 } else if (name.equals("time[x]")) { 1534 this.time = castToType(value); // Type 1535 } else 1536 return super.setProperty(name, value); 1537 return value; 1538 } 1539 1540 @Override 1541 public void removeChild(String name, Base value) throws FHIRException { 1542 if (name.equals("description")) { 1543 this.description = null; 1544 } else if (name.equals("time[x]")) { 1545 this.time = null; 1546 } else 1547 super.removeChild(name, value); 1548 1549 } 1550 1551 @Override 1552 public Base makeProperty(int hash, String name) throws FHIRException { 1553 switch (hash) { 1554 case -1724546052: 1555 return getDescriptionElement(); 1556 case -1313930605: 1557 return getTime(); 1558 case 3560141: 1559 return getTime(); 1560 default: 1561 return super.makeProperty(hash, name); 1562 } 1563 1564 } 1565 1566 @Override 1567 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1568 switch (hash) { 1569 case -1724546052: 1570 /* description */ return new String[] { "string" }; 1571 case 3560141: 1572 /* time */ return new String[] { "dateTime", "Period" }; 1573 default: 1574 return super.getTypesForProperty(hash, name); 1575 } 1576 1577 } 1578 1579 @Override 1580 public Base addChild(String name) throws FHIRException { 1581 if (name.equals("description")) { 1582 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.description"); 1583 } else if (name.equals("timeDateTime")) { 1584 this.time = new DateTimeType(); 1585 return this.time; 1586 } else if (name.equals("timePeriod")) { 1587 this.time = new Period(); 1588 return this.time; 1589 } else 1590 return super.addChild(name); 1591 } 1592 1593 public BiologicallyDerivedProductManipulationComponent copy() { 1594 BiologicallyDerivedProductManipulationComponent dst = new BiologicallyDerivedProductManipulationComponent(); 1595 copyValues(dst); 1596 return dst; 1597 } 1598 1599 public void copyValues(BiologicallyDerivedProductManipulationComponent dst) { 1600 super.copyValues(dst); 1601 dst.description = description == null ? null : description.copy(); 1602 dst.time = time == null ? null : time.copy(); 1603 } 1604 1605 @Override 1606 public boolean equalsDeep(Base other_) { 1607 if (!super.equalsDeep(other_)) 1608 return false; 1609 if (!(other_ instanceof BiologicallyDerivedProductManipulationComponent)) 1610 return false; 1611 BiologicallyDerivedProductManipulationComponent o = (BiologicallyDerivedProductManipulationComponent) other_; 1612 return compareDeep(description, o.description, true) && compareDeep(time, o.time, true); 1613 } 1614 1615 @Override 1616 public boolean equalsShallow(Base other_) { 1617 if (!super.equalsShallow(other_)) 1618 return false; 1619 if (!(other_ instanceof BiologicallyDerivedProductManipulationComponent)) 1620 return false; 1621 BiologicallyDerivedProductManipulationComponent o = (BiologicallyDerivedProductManipulationComponent) other_; 1622 return compareValues(description, o.description, true); 1623 } 1624 1625 public boolean isEmpty() { 1626 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, time); 1627 } 1628 1629 public String fhirType() { 1630 return "BiologicallyDerivedProduct.manipulation"; 1631 1632 } 1633 1634 } 1635 1636 @Block() 1637 public static class BiologicallyDerivedProductStorageComponent extends BackboneElement 1638 implements IBaseBackboneElement { 1639 /** 1640 * Description of storage. 1641 */ 1642 @Child(name = "description", type = { 1643 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1644 @Description(shortDefinition = "Description of storage", formalDefinition = "Description of storage.") 1645 protected StringType description; 1646 1647 /** 1648 * Storage temperature. 1649 */ 1650 @Child(name = "temperature", type = { 1651 DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1652 @Description(shortDefinition = "Storage temperature", formalDefinition = "Storage temperature.") 1653 protected DecimalType temperature; 1654 1655 /** 1656 * Temperature scale used. 1657 */ 1658 @Child(name = "scale", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1659 @Description(shortDefinition = "farenheit | celsius | kelvin", formalDefinition = "Temperature scale used.") 1660 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/product-storage-scale") 1661 protected Enumeration<BiologicallyDerivedProductStorageScale> scale; 1662 1663 /** 1664 * Storage timeperiod. 1665 */ 1666 @Child(name = "duration", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1667 @Description(shortDefinition = "Storage timeperiod", formalDefinition = "Storage timeperiod.") 1668 protected Period duration; 1669 1670 private static final long serialVersionUID = 1509141319L; 1671 1672 /** 1673 * Constructor 1674 */ 1675 public BiologicallyDerivedProductStorageComponent() { 1676 super(); 1677 } 1678 1679 /** 1680 * @return {@link #description} (Description of storage.). This is the 1681 * underlying object with id, value and extensions. The accessor 1682 * "getDescription" gives direct access to the value 1683 */ 1684 public StringType getDescriptionElement() { 1685 if (this.description == null) 1686 if (Configuration.errorOnAutoCreate()) 1687 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.description"); 1688 else if (Configuration.doAutoCreate()) 1689 this.description = new StringType(); // bb 1690 return this.description; 1691 } 1692 1693 public boolean hasDescriptionElement() { 1694 return this.description != null && !this.description.isEmpty(); 1695 } 1696 1697 public boolean hasDescription() { 1698 return this.description != null && !this.description.isEmpty(); 1699 } 1700 1701 /** 1702 * @param value {@link #description} (Description of storage.). This is the 1703 * underlying object with id, value and extensions. The accessor 1704 * "getDescription" gives direct access to the value 1705 */ 1706 public BiologicallyDerivedProductStorageComponent setDescriptionElement(StringType value) { 1707 this.description = value; 1708 return this; 1709 } 1710 1711 /** 1712 * @return Description of storage. 1713 */ 1714 public String getDescription() { 1715 return this.description == null ? null : this.description.getValue(); 1716 } 1717 1718 /** 1719 * @param value Description of storage. 1720 */ 1721 public BiologicallyDerivedProductStorageComponent setDescription(String value) { 1722 if (Utilities.noString(value)) 1723 this.description = null; 1724 else { 1725 if (this.description == null) 1726 this.description = new StringType(); 1727 this.description.setValue(value); 1728 } 1729 return this; 1730 } 1731 1732 /** 1733 * @return {@link #temperature} (Storage temperature.). This is the underlying 1734 * object with id, value and extensions. The accessor "getTemperature" 1735 * gives direct access to the value 1736 */ 1737 public DecimalType getTemperatureElement() { 1738 if (this.temperature == null) 1739 if (Configuration.errorOnAutoCreate()) 1740 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.temperature"); 1741 else if (Configuration.doAutoCreate()) 1742 this.temperature = new DecimalType(); // bb 1743 return this.temperature; 1744 } 1745 1746 public boolean hasTemperatureElement() { 1747 return this.temperature != null && !this.temperature.isEmpty(); 1748 } 1749 1750 public boolean hasTemperature() { 1751 return this.temperature != null && !this.temperature.isEmpty(); 1752 } 1753 1754 /** 1755 * @param value {@link #temperature} (Storage temperature.). This is the 1756 * underlying object with id, value and extensions. The accessor 1757 * "getTemperature" gives direct access to the value 1758 */ 1759 public BiologicallyDerivedProductStorageComponent setTemperatureElement(DecimalType value) { 1760 this.temperature = value; 1761 return this; 1762 } 1763 1764 /** 1765 * @return Storage temperature. 1766 */ 1767 public BigDecimal getTemperature() { 1768 return this.temperature == null ? null : this.temperature.getValue(); 1769 } 1770 1771 /** 1772 * @param value Storage temperature. 1773 */ 1774 public BiologicallyDerivedProductStorageComponent setTemperature(BigDecimal value) { 1775 if (value == null) 1776 this.temperature = null; 1777 else { 1778 if (this.temperature == null) 1779 this.temperature = new DecimalType(); 1780 this.temperature.setValue(value); 1781 } 1782 return this; 1783 } 1784 1785 /** 1786 * @param value Storage temperature. 1787 */ 1788 public BiologicallyDerivedProductStorageComponent setTemperature(long value) { 1789 this.temperature = new DecimalType(); 1790 this.temperature.setValue(value); 1791 return this; 1792 } 1793 1794 /** 1795 * @param value Storage temperature. 1796 */ 1797 public BiologicallyDerivedProductStorageComponent setTemperature(double value) { 1798 this.temperature = new DecimalType(); 1799 this.temperature.setValue(value); 1800 return this; 1801 } 1802 1803 /** 1804 * @return {@link #scale} (Temperature scale used.). This is the underlying 1805 * object with id, value and extensions. The accessor "getScale" gives 1806 * direct access to the value 1807 */ 1808 public Enumeration<BiologicallyDerivedProductStorageScale> getScaleElement() { 1809 if (this.scale == null) 1810 if (Configuration.errorOnAutoCreate()) 1811 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.scale"); 1812 else if (Configuration.doAutoCreate()) 1813 this.scale = new Enumeration<BiologicallyDerivedProductStorageScale>( 1814 new BiologicallyDerivedProductStorageScaleEnumFactory()); // bb 1815 return this.scale; 1816 } 1817 1818 public boolean hasScaleElement() { 1819 return this.scale != null && !this.scale.isEmpty(); 1820 } 1821 1822 public boolean hasScale() { 1823 return this.scale != null && !this.scale.isEmpty(); 1824 } 1825 1826 /** 1827 * @param value {@link #scale} (Temperature scale used.). This is the underlying 1828 * object with id, value and extensions. The accessor "getScale" 1829 * gives direct access to the value 1830 */ 1831 public BiologicallyDerivedProductStorageComponent setScaleElement( 1832 Enumeration<BiologicallyDerivedProductStorageScale> value) { 1833 this.scale = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return Temperature scale used. 1839 */ 1840 public BiologicallyDerivedProductStorageScale getScale() { 1841 return this.scale == null ? null : this.scale.getValue(); 1842 } 1843 1844 /** 1845 * @param value Temperature scale used. 1846 */ 1847 public BiologicallyDerivedProductStorageComponent setScale(BiologicallyDerivedProductStorageScale value) { 1848 if (value == null) 1849 this.scale = null; 1850 else { 1851 if (this.scale == null) 1852 this.scale = new Enumeration<BiologicallyDerivedProductStorageScale>( 1853 new BiologicallyDerivedProductStorageScaleEnumFactory()); 1854 this.scale.setValue(value); 1855 } 1856 return this; 1857 } 1858 1859 /** 1860 * @return {@link #duration} (Storage timeperiod.) 1861 */ 1862 public Period getDuration() { 1863 if (this.duration == null) 1864 if (Configuration.errorOnAutoCreate()) 1865 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.duration"); 1866 else if (Configuration.doAutoCreate()) 1867 this.duration = new Period(); // cc 1868 return this.duration; 1869 } 1870 1871 public boolean hasDuration() { 1872 return this.duration != null && !this.duration.isEmpty(); 1873 } 1874 1875 /** 1876 * @param value {@link #duration} (Storage timeperiod.) 1877 */ 1878 public BiologicallyDerivedProductStorageComponent setDuration(Period value) { 1879 this.duration = value; 1880 return this; 1881 } 1882 1883 protected void listChildren(List<Property> children) { 1884 super.listChildren(children); 1885 children.add(new Property("description", "string", "Description of storage.", 0, 1, description)); 1886 children.add(new Property("temperature", "decimal", "Storage temperature.", 0, 1, temperature)); 1887 children.add(new Property("scale", "code", "Temperature scale used.", 0, 1, scale)); 1888 children.add(new Property("duration", "Period", "Storage timeperiod.", 0, 1, duration)); 1889 } 1890 1891 @Override 1892 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1893 switch (_hash) { 1894 case -1724546052: 1895 /* description */ return new Property("description", "string", "Description of storage.", 0, 1, description); 1896 case 321701236: 1897 /* temperature */ return new Property("temperature", "decimal", "Storage temperature.", 0, 1, temperature); 1898 case 109250890: 1899 /* scale */ return new Property("scale", "code", "Temperature scale used.", 0, 1, scale); 1900 case -1992012396: 1901 /* duration */ return new Property("duration", "Period", "Storage timeperiod.", 0, 1, duration); 1902 default: 1903 return super.getNamedProperty(_hash, _name, _checkValid); 1904 } 1905 1906 } 1907 1908 @Override 1909 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1910 switch (hash) { 1911 case -1724546052: 1912 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1913 case 321701236: 1914 /* temperature */ return this.temperature == null ? new Base[0] : new Base[] { this.temperature }; // DecimalType 1915 case 109250890: 1916 /* scale */ return this.scale == null ? new Base[0] : new Base[] { this.scale }; // Enumeration<BiologicallyDerivedProductStorageScale> 1917 case -1992012396: 1918 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Period 1919 default: 1920 return super.getProperty(hash, name, checkValid); 1921 } 1922 1923 } 1924 1925 @Override 1926 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1927 switch (hash) { 1928 case -1724546052: // description 1929 this.description = castToString(value); // StringType 1930 return value; 1931 case 321701236: // temperature 1932 this.temperature = castToDecimal(value); // DecimalType 1933 return value; 1934 case 109250890: // scale 1935 value = new BiologicallyDerivedProductStorageScaleEnumFactory().fromType(castToCode(value)); 1936 this.scale = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStorageScale> 1937 return value; 1938 case -1992012396: // duration 1939 this.duration = castToPeriod(value); // Period 1940 return value; 1941 default: 1942 return super.setProperty(hash, name, value); 1943 } 1944 1945 } 1946 1947 @Override 1948 public Base setProperty(String name, Base value) throws FHIRException { 1949 if (name.equals("description")) { 1950 this.description = castToString(value); // StringType 1951 } else if (name.equals("temperature")) { 1952 this.temperature = castToDecimal(value); // DecimalType 1953 } else if (name.equals("scale")) { 1954 value = new BiologicallyDerivedProductStorageScaleEnumFactory().fromType(castToCode(value)); 1955 this.scale = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStorageScale> 1956 } else if (name.equals("duration")) { 1957 this.duration = castToPeriod(value); // Period 1958 } else 1959 return super.setProperty(name, value); 1960 return value; 1961 } 1962 1963 @Override 1964 public void removeChild(String name, Base value) throws FHIRException { 1965 if (name.equals("description")) { 1966 this.description = null; 1967 } else if (name.equals("temperature")) { 1968 this.temperature = null; 1969 } else if (name.equals("scale")) { 1970 this.scale = null; 1971 } else if (name.equals("duration")) { 1972 this.duration = null; 1973 } else 1974 super.removeChild(name, value); 1975 1976 } 1977 1978 @Override 1979 public Base makeProperty(int hash, String name) throws FHIRException { 1980 switch (hash) { 1981 case -1724546052: 1982 return getDescriptionElement(); 1983 case 321701236: 1984 return getTemperatureElement(); 1985 case 109250890: 1986 return getScaleElement(); 1987 case -1992012396: 1988 return getDuration(); 1989 default: 1990 return super.makeProperty(hash, name); 1991 } 1992 1993 } 1994 1995 @Override 1996 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1997 switch (hash) { 1998 case -1724546052: 1999 /* description */ return new String[] { "string" }; 2000 case 321701236: 2001 /* temperature */ return new String[] { "decimal" }; 2002 case 109250890: 2003 /* scale */ return new String[] { "code" }; 2004 case -1992012396: 2005 /* duration */ return new String[] { "Period" }; 2006 default: 2007 return super.getTypesForProperty(hash, name); 2008 } 2009 2010 } 2011 2012 @Override 2013 public Base addChild(String name) throws FHIRException { 2014 if (name.equals("description")) { 2015 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.description"); 2016 } else if (name.equals("temperature")) { 2017 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.temperature"); 2018 } else if (name.equals("scale")) { 2019 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.scale"); 2020 } else if (name.equals("duration")) { 2021 this.duration = new Period(); 2022 return this.duration; 2023 } else 2024 return super.addChild(name); 2025 } 2026 2027 public BiologicallyDerivedProductStorageComponent copy() { 2028 BiologicallyDerivedProductStorageComponent dst = new BiologicallyDerivedProductStorageComponent(); 2029 copyValues(dst); 2030 return dst; 2031 } 2032 2033 public void copyValues(BiologicallyDerivedProductStorageComponent dst) { 2034 super.copyValues(dst); 2035 dst.description = description == null ? null : description.copy(); 2036 dst.temperature = temperature == null ? null : temperature.copy(); 2037 dst.scale = scale == null ? null : scale.copy(); 2038 dst.duration = duration == null ? null : duration.copy(); 2039 } 2040 2041 @Override 2042 public boolean equalsDeep(Base other_) { 2043 if (!super.equalsDeep(other_)) 2044 return false; 2045 if (!(other_ instanceof BiologicallyDerivedProductStorageComponent)) 2046 return false; 2047 BiologicallyDerivedProductStorageComponent o = (BiologicallyDerivedProductStorageComponent) other_; 2048 return compareDeep(description, o.description, true) && compareDeep(temperature, o.temperature, true) 2049 && compareDeep(scale, o.scale, true) && compareDeep(duration, o.duration, true); 2050 } 2051 2052 @Override 2053 public boolean equalsShallow(Base other_) { 2054 if (!super.equalsShallow(other_)) 2055 return false; 2056 if (!(other_ instanceof BiologicallyDerivedProductStorageComponent)) 2057 return false; 2058 BiologicallyDerivedProductStorageComponent o = (BiologicallyDerivedProductStorageComponent) other_; 2059 return compareValues(description, o.description, true) && compareValues(temperature, o.temperature, true) 2060 && compareValues(scale, o.scale, true); 2061 } 2062 2063 public boolean isEmpty() { 2064 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, temperature, scale, duration); 2065 } 2066 2067 public String fhirType() { 2068 return "BiologicallyDerivedProduct.storage"; 2069 2070 } 2071 2072 } 2073 2074 /** 2075 * This records identifiers associated with this biologically derived product 2076 * instance that are defined by business processes and/or used to refer to it 2077 * when a direct URL reference to the resource itself is not appropriate (e.g. 2078 * in CDA documents, or in written / printed documentation). 2079 */ 2080 @Child(name = "identifier", type = { 2081 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2082 @Description(shortDefinition = "External ids for this item", formalDefinition = "This records identifiers associated with this biologically derived product instance that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 2083 protected List<Identifier> identifier; 2084 2085 /** 2086 * Broad category of this product. 2087 */ 2088 @Child(name = "productCategory", type = { 2089 CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2090 @Description(shortDefinition = "organ | tissue | fluid | cells | biologicalAgent", formalDefinition = "Broad category of this product.") 2091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/product-category") 2092 protected Enumeration<BiologicallyDerivedProductCategory> productCategory; 2093 2094 /** 2095 * A code that identifies the kind of this biologically derived product (SNOMED 2096 * Ctcode). 2097 */ 2098 @Child(name = "productCode", type = { 2099 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2100 @Description(shortDefinition = "What this biologically derived product is", formalDefinition = "A code that identifies the kind of this biologically derived product (SNOMED Ctcode).") 2101 protected CodeableConcept productCode; 2102 2103 /** 2104 * Whether the product is currently available. 2105 */ 2106 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2107 @Description(shortDefinition = "available | unavailable", formalDefinition = "Whether the product is currently available.") 2108 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/product-status") 2109 protected Enumeration<BiologicallyDerivedProductStatus> status; 2110 2111 /** 2112 * Procedure request to obtain this biologically derived product. 2113 */ 2114 @Child(name = "request", type = { 2115 ServiceRequest.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2116 @Description(shortDefinition = "Procedure request", formalDefinition = "Procedure request to obtain this biologically derived product.") 2117 protected List<Reference> request; 2118 /** 2119 * The actual objects that are the target of the reference (Procedure request to 2120 * obtain this biologically derived product.) 2121 */ 2122 protected List<ServiceRequest> requestTarget; 2123 2124 /** 2125 * Number of discrete units within this product. 2126 */ 2127 @Child(name = "quantity", type = { 2128 IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2129 @Description(shortDefinition = "The amount of this biologically derived product", formalDefinition = "Number of discrete units within this product.") 2130 protected IntegerType quantity; 2131 2132 /** 2133 * Parent product (if any). 2134 */ 2135 @Child(name = "parent", type = { 2136 BiologicallyDerivedProduct.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2137 @Description(shortDefinition = "BiologicallyDerivedProduct parent", formalDefinition = "Parent product (if any).") 2138 protected List<Reference> parent; 2139 /** 2140 * The actual objects that are the target of the reference (Parent product (if 2141 * any).) 2142 */ 2143 protected List<BiologicallyDerivedProduct> parentTarget; 2144 2145 /** 2146 * How this product was collected. 2147 */ 2148 @Child(name = "collection", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 2149 @Description(shortDefinition = "How this product was collected", formalDefinition = "How this product was collected.") 2150 protected BiologicallyDerivedProductCollectionComponent collection; 2151 2152 /** 2153 * Any processing of the product during collection that does not change the 2154 * fundamental nature of the product. For example adding anti-coagulants during 2155 * the collection of Peripheral Blood Stem Cells. 2156 */ 2157 @Child(name = "processing", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2158 @Description(shortDefinition = "Any processing of the product during collection", formalDefinition = "Any processing of the product during collection that does not change the fundamental nature of the product. For example adding anti-coagulants during the collection of Peripheral Blood Stem Cells.") 2159 protected List<BiologicallyDerivedProductProcessingComponent> processing; 2160 2161 /** 2162 * Any manipulation of product post-collection that is intended to alter the 2163 * product. For example a buffy-coat enrichment or CD8 reduction of Peripheral 2164 * Blood Stem Cells to make it more suitable for infusion. 2165 */ 2166 @Child(name = "manipulation", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 2167 @Description(shortDefinition = "Any manipulation of product post-collection", formalDefinition = "Any manipulation of product post-collection that is intended to alter the product. For example a buffy-coat enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it more suitable for infusion.") 2168 protected BiologicallyDerivedProductManipulationComponent manipulation; 2169 2170 /** 2171 * Product storage. 2172 */ 2173 @Child(name = "storage", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2174 @Description(shortDefinition = "Product storage", formalDefinition = "Product storage.") 2175 protected List<BiologicallyDerivedProductStorageComponent> storage; 2176 2177 private static final long serialVersionUID = -1367034547L; 2178 2179 /** 2180 * Constructor 2181 */ 2182 public BiologicallyDerivedProduct() { 2183 super(); 2184 } 2185 2186 /** 2187 * @return {@link #identifier} (This records identifiers associated with this 2188 * biologically derived product instance that are defined by business 2189 * processes and/or used to refer to it when a direct URL reference to 2190 * the resource itself is not appropriate (e.g. in CDA documents, or in 2191 * written / printed documentation).) 2192 */ 2193 public List<Identifier> getIdentifier() { 2194 if (this.identifier == null) 2195 this.identifier = new ArrayList<Identifier>(); 2196 return this.identifier; 2197 } 2198 2199 /** 2200 * @return Returns a reference to <code>this</code> for easy method chaining 2201 */ 2202 public BiologicallyDerivedProduct setIdentifier(List<Identifier> theIdentifier) { 2203 this.identifier = theIdentifier; 2204 return this; 2205 } 2206 2207 public boolean hasIdentifier() { 2208 if (this.identifier == null) 2209 return false; 2210 for (Identifier item : this.identifier) 2211 if (!item.isEmpty()) 2212 return true; 2213 return false; 2214 } 2215 2216 public Identifier addIdentifier() { // 3 2217 Identifier t = new Identifier(); 2218 if (this.identifier == null) 2219 this.identifier = new ArrayList<Identifier>(); 2220 this.identifier.add(t); 2221 return t; 2222 } 2223 2224 public BiologicallyDerivedProduct addIdentifier(Identifier t) { // 3 2225 if (t == null) 2226 return this; 2227 if (this.identifier == null) 2228 this.identifier = new ArrayList<Identifier>(); 2229 this.identifier.add(t); 2230 return this; 2231 } 2232 2233 /** 2234 * @return The first repetition of repeating field {@link #identifier}, creating 2235 * it if it does not already exist 2236 */ 2237 public Identifier getIdentifierFirstRep() { 2238 if (getIdentifier().isEmpty()) { 2239 addIdentifier(); 2240 } 2241 return getIdentifier().get(0); 2242 } 2243 2244 /** 2245 * @return {@link #productCategory} (Broad category of this product.). This is 2246 * the underlying object with id, value and extensions. The accessor 2247 * "getProductCategory" gives direct access to the value 2248 */ 2249 public Enumeration<BiologicallyDerivedProductCategory> getProductCategoryElement() { 2250 if (this.productCategory == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productCategory"); 2253 else if (Configuration.doAutoCreate()) 2254 this.productCategory = new Enumeration<BiologicallyDerivedProductCategory>( 2255 new BiologicallyDerivedProductCategoryEnumFactory()); // bb 2256 return this.productCategory; 2257 } 2258 2259 public boolean hasProductCategoryElement() { 2260 return this.productCategory != null && !this.productCategory.isEmpty(); 2261 } 2262 2263 public boolean hasProductCategory() { 2264 return this.productCategory != null && !this.productCategory.isEmpty(); 2265 } 2266 2267 /** 2268 * @param value {@link #productCategory} (Broad category of this product.). This 2269 * is the underlying object with id, value and extensions. The 2270 * accessor "getProductCategory" gives direct access to the value 2271 */ 2272 public BiologicallyDerivedProduct setProductCategoryElement(Enumeration<BiologicallyDerivedProductCategory> value) { 2273 this.productCategory = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return Broad category of this product. 2279 */ 2280 public BiologicallyDerivedProductCategory getProductCategory() { 2281 return this.productCategory == null ? null : this.productCategory.getValue(); 2282 } 2283 2284 /** 2285 * @param value Broad category of this product. 2286 */ 2287 public BiologicallyDerivedProduct setProductCategory(BiologicallyDerivedProductCategory value) { 2288 if (value == null) 2289 this.productCategory = null; 2290 else { 2291 if (this.productCategory == null) 2292 this.productCategory = new Enumeration<BiologicallyDerivedProductCategory>( 2293 new BiologicallyDerivedProductCategoryEnumFactory()); 2294 this.productCategory.setValue(value); 2295 } 2296 return this; 2297 } 2298 2299 /** 2300 * @return {@link #productCode} (A code that identifies the kind of this 2301 * biologically derived product (SNOMED Ctcode).) 2302 */ 2303 public CodeableConcept getProductCode() { 2304 if (this.productCode == null) 2305 if (Configuration.errorOnAutoCreate()) 2306 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productCode"); 2307 else if (Configuration.doAutoCreate()) 2308 this.productCode = new CodeableConcept(); // cc 2309 return this.productCode; 2310 } 2311 2312 public boolean hasProductCode() { 2313 return this.productCode != null && !this.productCode.isEmpty(); 2314 } 2315 2316 /** 2317 * @param value {@link #productCode} (A code that identifies the kind of this 2318 * biologically derived product (SNOMED Ctcode).) 2319 */ 2320 public BiologicallyDerivedProduct setProductCode(CodeableConcept value) { 2321 this.productCode = value; 2322 return this; 2323 } 2324 2325 /** 2326 * @return {@link #status} (Whether the product is currently available.). This 2327 * is the underlying object with id, value and extensions. The accessor 2328 * "getStatus" gives direct access to the value 2329 */ 2330 public Enumeration<BiologicallyDerivedProductStatus> getStatusElement() { 2331 if (this.status == null) 2332 if (Configuration.errorOnAutoCreate()) 2333 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.status"); 2334 else if (Configuration.doAutoCreate()) 2335 this.status = new Enumeration<BiologicallyDerivedProductStatus>( 2336 new BiologicallyDerivedProductStatusEnumFactory()); // bb 2337 return this.status; 2338 } 2339 2340 public boolean hasStatusElement() { 2341 return this.status != null && !this.status.isEmpty(); 2342 } 2343 2344 public boolean hasStatus() { 2345 return this.status != null && !this.status.isEmpty(); 2346 } 2347 2348 /** 2349 * @param value {@link #status} (Whether the product is currently available.). 2350 * This is the underlying object with id, value and extensions. The 2351 * accessor "getStatus" gives direct access to the value 2352 */ 2353 public BiologicallyDerivedProduct setStatusElement(Enumeration<BiologicallyDerivedProductStatus> value) { 2354 this.status = value; 2355 return this; 2356 } 2357 2358 /** 2359 * @return Whether the product is currently available. 2360 */ 2361 public BiologicallyDerivedProductStatus getStatus() { 2362 return this.status == null ? null : this.status.getValue(); 2363 } 2364 2365 /** 2366 * @param value Whether the product is currently available. 2367 */ 2368 public BiologicallyDerivedProduct setStatus(BiologicallyDerivedProductStatus value) { 2369 if (value == null) 2370 this.status = null; 2371 else { 2372 if (this.status == null) 2373 this.status = new Enumeration<BiologicallyDerivedProductStatus>( 2374 new BiologicallyDerivedProductStatusEnumFactory()); 2375 this.status.setValue(value); 2376 } 2377 return this; 2378 } 2379 2380 /** 2381 * @return {@link #request} (Procedure request to obtain this biologically 2382 * derived product.) 2383 */ 2384 public List<Reference> getRequest() { 2385 if (this.request == null) 2386 this.request = new ArrayList<Reference>(); 2387 return this.request; 2388 } 2389 2390 /** 2391 * @return Returns a reference to <code>this</code> for easy method chaining 2392 */ 2393 public BiologicallyDerivedProduct setRequest(List<Reference> theRequest) { 2394 this.request = theRequest; 2395 return this; 2396 } 2397 2398 public boolean hasRequest() { 2399 if (this.request == null) 2400 return false; 2401 for (Reference item : this.request) 2402 if (!item.isEmpty()) 2403 return true; 2404 return false; 2405 } 2406 2407 public Reference addRequest() { // 3 2408 Reference t = new Reference(); 2409 if (this.request == null) 2410 this.request = new ArrayList<Reference>(); 2411 this.request.add(t); 2412 return t; 2413 } 2414 2415 public BiologicallyDerivedProduct addRequest(Reference t) { // 3 2416 if (t == null) 2417 return this; 2418 if (this.request == null) 2419 this.request = new ArrayList<Reference>(); 2420 this.request.add(t); 2421 return this; 2422 } 2423 2424 /** 2425 * @return The first repetition of repeating field {@link #request}, creating it 2426 * if it does not already exist 2427 */ 2428 public Reference getRequestFirstRep() { 2429 if (getRequest().isEmpty()) { 2430 addRequest(); 2431 } 2432 return getRequest().get(0); 2433 } 2434 2435 /** 2436 * @deprecated Use Reference#setResource(IBaseResource) instead 2437 */ 2438 @Deprecated 2439 public List<ServiceRequest> getRequestTarget() { 2440 if (this.requestTarget == null) 2441 this.requestTarget = new ArrayList<ServiceRequest>(); 2442 return this.requestTarget; 2443 } 2444 2445 /** 2446 * @deprecated Use Reference#setResource(IBaseResource) instead 2447 */ 2448 @Deprecated 2449 public ServiceRequest addRequestTarget() { 2450 ServiceRequest r = new ServiceRequest(); 2451 if (this.requestTarget == null) 2452 this.requestTarget = new ArrayList<ServiceRequest>(); 2453 this.requestTarget.add(r); 2454 return r; 2455 } 2456 2457 /** 2458 * @return {@link #quantity} (Number of discrete units within this product.). 2459 * This is the underlying object with id, value and extensions. The 2460 * accessor "getQuantity" gives direct access to the value 2461 */ 2462 public IntegerType getQuantityElement() { 2463 if (this.quantity == null) 2464 if (Configuration.errorOnAutoCreate()) 2465 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.quantity"); 2466 else if (Configuration.doAutoCreate()) 2467 this.quantity = new IntegerType(); // bb 2468 return this.quantity; 2469 } 2470 2471 public boolean hasQuantityElement() { 2472 return this.quantity != null && !this.quantity.isEmpty(); 2473 } 2474 2475 public boolean hasQuantity() { 2476 return this.quantity != null && !this.quantity.isEmpty(); 2477 } 2478 2479 /** 2480 * @param value {@link #quantity} (Number of discrete units within this 2481 * product.). This is the underlying object with id, value and 2482 * extensions. The accessor "getQuantity" gives direct access to 2483 * the value 2484 */ 2485 public BiologicallyDerivedProduct setQuantityElement(IntegerType value) { 2486 this.quantity = value; 2487 return this; 2488 } 2489 2490 /** 2491 * @return Number of discrete units within this product. 2492 */ 2493 public int getQuantity() { 2494 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 2495 } 2496 2497 /** 2498 * @param value Number of discrete units within this product. 2499 */ 2500 public BiologicallyDerivedProduct setQuantity(int value) { 2501 if (this.quantity == null) 2502 this.quantity = new IntegerType(); 2503 this.quantity.setValue(value); 2504 return this; 2505 } 2506 2507 /** 2508 * @return {@link #parent} (Parent product (if any).) 2509 */ 2510 public List<Reference> getParent() { 2511 if (this.parent == null) 2512 this.parent = new ArrayList<Reference>(); 2513 return this.parent; 2514 } 2515 2516 /** 2517 * @return Returns a reference to <code>this</code> for easy method chaining 2518 */ 2519 public BiologicallyDerivedProduct setParent(List<Reference> theParent) { 2520 this.parent = theParent; 2521 return this; 2522 } 2523 2524 public boolean hasParent() { 2525 if (this.parent == null) 2526 return false; 2527 for (Reference item : this.parent) 2528 if (!item.isEmpty()) 2529 return true; 2530 return false; 2531 } 2532 2533 public Reference addParent() { // 3 2534 Reference t = new Reference(); 2535 if (this.parent == null) 2536 this.parent = new ArrayList<Reference>(); 2537 this.parent.add(t); 2538 return t; 2539 } 2540 2541 public BiologicallyDerivedProduct addParent(Reference t) { // 3 2542 if (t == null) 2543 return this; 2544 if (this.parent == null) 2545 this.parent = new ArrayList<Reference>(); 2546 this.parent.add(t); 2547 return this; 2548 } 2549 2550 /** 2551 * @return The first repetition of repeating field {@link #parent}, creating it 2552 * if it does not already exist 2553 */ 2554 public Reference getParentFirstRep() { 2555 if (getParent().isEmpty()) { 2556 addParent(); 2557 } 2558 return getParent().get(0); 2559 } 2560 2561 /** 2562 * @deprecated Use Reference#setResource(IBaseResource) instead 2563 */ 2564 @Deprecated 2565 public List<BiologicallyDerivedProduct> getParentTarget() { 2566 if (this.parentTarget == null) 2567 this.parentTarget = new ArrayList<BiologicallyDerivedProduct>(); 2568 return this.parentTarget; 2569 } 2570 2571 /** 2572 * @deprecated Use Reference#setResource(IBaseResource) instead 2573 */ 2574 @Deprecated 2575 public BiologicallyDerivedProduct addParentTarget() { 2576 BiologicallyDerivedProduct r = new BiologicallyDerivedProduct(); 2577 if (this.parentTarget == null) 2578 this.parentTarget = new ArrayList<BiologicallyDerivedProduct>(); 2579 this.parentTarget.add(r); 2580 return r; 2581 } 2582 2583 /** 2584 * @return {@link #collection} (How this product was collected.) 2585 */ 2586 public BiologicallyDerivedProductCollectionComponent getCollection() { 2587 if (this.collection == null) 2588 if (Configuration.errorOnAutoCreate()) 2589 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.collection"); 2590 else if (Configuration.doAutoCreate()) 2591 this.collection = new BiologicallyDerivedProductCollectionComponent(); // cc 2592 return this.collection; 2593 } 2594 2595 public boolean hasCollection() { 2596 return this.collection != null && !this.collection.isEmpty(); 2597 } 2598 2599 /** 2600 * @param value {@link #collection} (How this product was collected.) 2601 */ 2602 public BiologicallyDerivedProduct setCollection(BiologicallyDerivedProductCollectionComponent value) { 2603 this.collection = value; 2604 return this; 2605 } 2606 2607 /** 2608 * @return {@link #processing} (Any processing of the product during collection 2609 * that does not change the fundamental nature of the product. For 2610 * example adding anti-coagulants during the collection of Peripheral 2611 * Blood Stem Cells.) 2612 */ 2613 public List<BiologicallyDerivedProductProcessingComponent> getProcessing() { 2614 if (this.processing == null) 2615 this.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 2616 return this.processing; 2617 } 2618 2619 /** 2620 * @return Returns a reference to <code>this</code> for easy method chaining 2621 */ 2622 public BiologicallyDerivedProduct setProcessing(List<BiologicallyDerivedProductProcessingComponent> theProcessing) { 2623 this.processing = theProcessing; 2624 return this; 2625 } 2626 2627 public boolean hasProcessing() { 2628 if (this.processing == null) 2629 return false; 2630 for (BiologicallyDerivedProductProcessingComponent item : this.processing) 2631 if (!item.isEmpty()) 2632 return true; 2633 return false; 2634 } 2635 2636 public BiologicallyDerivedProductProcessingComponent addProcessing() { // 3 2637 BiologicallyDerivedProductProcessingComponent t = new BiologicallyDerivedProductProcessingComponent(); 2638 if (this.processing == null) 2639 this.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 2640 this.processing.add(t); 2641 return t; 2642 } 2643 2644 public BiologicallyDerivedProduct addProcessing(BiologicallyDerivedProductProcessingComponent t) { // 3 2645 if (t == null) 2646 return this; 2647 if (this.processing == null) 2648 this.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 2649 this.processing.add(t); 2650 return this; 2651 } 2652 2653 /** 2654 * @return The first repetition of repeating field {@link #processing}, creating 2655 * it if it does not already exist 2656 */ 2657 public BiologicallyDerivedProductProcessingComponent getProcessingFirstRep() { 2658 if (getProcessing().isEmpty()) { 2659 addProcessing(); 2660 } 2661 return getProcessing().get(0); 2662 } 2663 2664 /** 2665 * @return {@link #manipulation} (Any manipulation of product post-collection 2666 * that is intended to alter the product. For example a buffy-coat 2667 * enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it 2668 * more suitable for infusion.) 2669 */ 2670 public BiologicallyDerivedProductManipulationComponent getManipulation() { 2671 if (this.manipulation == null) 2672 if (Configuration.errorOnAutoCreate()) 2673 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.manipulation"); 2674 else if (Configuration.doAutoCreate()) 2675 this.manipulation = new BiologicallyDerivedProductManipulationComponent(); // cc 2676 return this.manipulation; 2677 } 2678 2679 public boolean hasManipulation() { 2680 return this.manipulation != null && !this.manipulation.isEmpty(); 2681 } 2682 2683 /** 2684 * @param value {@link #manipulation} (Any manipulation of product 2685 * post-collection that is intended to alter the product. For 2686 * example a buffy-coat enrichment or CD8 reduction of Peripheral 2687 * Blood Stem Cells to make it more suitable for infusion.) 2688 */ 2689 public BiologicallyDerivedProduct setManipulation(BiologicallyDerivedProductManipulationComponent value) { 2690 this.manipulation = value; 2691 return this; 2692 } 2693 2694 /** 2695 * @return {@link #storage} (Product storage.) 2696 */ 2697 public List<BiologicallyDerivedProductStorageComponent> getStorage() { 2698 if (this.storage == null) 2699 this.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 2700 return this.storage; 2701 } 2702 2703 /** 2704 * @return Returns a reference to <code>this</code> for easy method chaining 2705 */ 2706 public BiologicallyDerivedProduct setStorage(List<BiologicallyDerivedProductStorageComponent> theStorage) { 2707 this.storage = theStorage; 2708 return this; 2709 } 2710 2711 public boolean hasStorage() { 2712 if (this.storage == null) 2713 return false; 2714 for (BiologicallyDerivedProductStorageComponent item : this.storage) 2715 if (!item.isEmpty()) 2716 return true; 2717 return false; 2718 } 2719 2720 public BiologicallyDerivedProductStorageComponent addStorage() { // 3 2721 BiologicallyDerivedProductStorageComponent t = new BiologicallyDerivedProductStorageComponent(); 2722 if (this.storage == null) 2723 this.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 2724 this.storage.add(t); 2725 return t; 2726 } 2727 2728 public BiologicallyDerivedProduct addStorage(BiologicallyDerivedProductStorageComponent t) { // 3 2729 if (t == null) 2730 return this; 2731 if (this.storage == null) 2732 this.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 2733 this.storage.add(t); 2734 return this; 2735 } 2736 2737 /** 2738 * @return The first repetition of repeating field {@link #storage}, creating it 2739 * if it does not already exist 2740 */ 2741 public BiologicallyDerivedProductStorageComponent getStorageFirstRep() { 2742 if (getStorage().isEmpty()) { 2743 addStorage(); 2744 } 2745 return getStorage().get(0); 2746 } 2747 2748 protected void listChildren(List<Property> children) { 2749 super.listChildren(children); 2750 children.add(new Property("identifier", "Identifier", 2751 "This records identifiers associated with this biologically derived product instance that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2752 0, java.lang.Integer.MAX_VALUE, identifier)); 2753 children.add(new Property("productCategory", "code", "Broad category of this product.", 0, 1, productCategory)); 2754 children.add(new Property("productCode", "CodeableConcept", 2755 "A code that identifies the kind of this biologically derived product (SNOMED Ctcode).", 0, 1, productCode)); 2756 children.add(new Property("status", "code", "Whether the product is currently available.", 0, 1, status)); 2757 children.add(new Property("request", "Reference(ServiceRequest)", 2758 "Procedure request to obtain this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, request)); 2759 children.add(new Property("quantity", "integer", "Number of discrete units within this product.", 0, 1, quantity)); 2760 children.add(new Property("parent", "Reference(BiologicallyDerivedProduct)", "Parent product (if any).", 0, 2761 java.lang.Integer.MAX_VALUE, parent)); 2762 children.add(new Property("collection", "", "How this product was collected.", 0, 1, collection)); 2763 children.add(new Property("processing", "", 2764 "Any processing of the product during collection that does not change the fundamental nature of the product. For example adding anti-coagulants during the collection of Peripheral Blood Stem Cells.", 2765 0, java.lang.Integer.MAX_VALUE, processing)); 2766 children.add(new Property("manipulation", "", 2767 "Any manipulation of product post-collection that is intended to alter the product. For example a buffy-coat enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it more suitable for infusion.", 2768 0, 1, manipulation)); 2769 children.add(new Property("storage", "", "Product storage.", 0, java.lang.Integer.MAX_VALUE, storage)); 2770 } 2771 2772 @Override 2773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2774 switch (_hash) { 2775 case -1618432855: 2776 /* identifier */ return new Property("identifier", "Identifier", 2777 "This records identifiers associated with this biologically derived product instance that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2778 0, java.lang.Integer.MAX_VALUE, identifier); 2779 case 197299981: 2780 /* productCategory */ return new Property("productCategory", "code", "Broad category of this product.", 0, 1, 2781 productCategory); 2782 case -1492131972: 2783 /* productCode */ return new Property("productCode", "CodeableConcept", 2784 "A code that identifies the kind of this biologically derived product (SNOMED Ctcode).", 0, 1, productCode); 2785 case -892481550: 2786 /* status */ return new Property("status", "code", "Whether the product is currently available.", 0, 1, status); 2787 case 1095692943: 2788 /* request */ return new Property("request", "Reference(ServiceRequest)", 2789 "Procedure request to obtain this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, request); 2790 case -1285004149: 2791 /* quantity */ return new Property("quantity", "integer", "Number of discrete units within this product.", 0, 1, 2792 quantity); 2793 case -995424086: 2794 /* parent */ return new Property("parent", "Reference(BiologicallyDerivedProduct)", "Parent product (if any).", 0, 2795 java.lang.Integer.MAX_VALUE, parent); 2796 case -1741312354: 2797 /* collection */ return new Property("collection", "", "How this product was collected.", 0, 1, collection); 2798 case 422194963: 2799 /* processing */ return new Property("processing", "", 2800 "Any processing of the product during collection that does not change the fundamental nature of the product. For example adding anti-coagulants during the collection of Peripheral Blood Stem Cells.", 2801 0, java.lang.Integer.MAX_VALUE, processing); 2802 case -696214627: 2803 /* manipulation */ return new Property("manipulation", "", 2804 "Any manipulation of product post-collection that is intended to alter the product. For example a buffy-coat enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it more suitable for infusion.", 2805 0, 1, manipulation); 2806 case -1884274053: 2807 /* storage */ return new Property("storage", "", "Product storage.", 0, java.lang.Integer.MAX_VALUE, storage); 2808 default: 2809 return super.getNamedProperty(_hash, _name, _checkValid); 2810 } 2811 2812 } 2813 2814 @Override 2815 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2816 switch (hash) { 2817 case -1618432855: 2818 /* identifier */ return this.identifier == null ? new Base[0] 2819 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2820 case 197299981: 2821 /* productCategory */ return this.productCategory == null ? new Base[0] : new Base[] { this.productCategory }; // Enumeration<BiologicallyDerivedProductCategory> 2822 case -1492131972: 2823 /* productCode */ return this.productCode == null ? new Base[0] : new Base[] { this.productCode }; // CodeableConcept 2824 case -892481550: 2825 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<BiologicallyDerivedProductStatus> 2826 case 1095692943: 2827 /* request */ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 2828 case -1285004149: 2829 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // IntegerType 2830 case -995424086: 2831 /* parent */ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2832 case -1741312354: 2833 /* collection */ return this.collection == null ? new Base[0] : new Base[] { this.collection }; // BiologicallyDerivedProductCollectionComponent 2834 case 422194963: 2835 /* processing */ return this.processing == null ? new Base[0] 2836 : this.processing.toArray(new Base[this.processing.size()]); // BiologicallyDerivedProductProcessingComponent 2837 case -696214627: 2838 /* manipulation */ return this.manipulation == null ? new Base[0] : new Base[] { this.manipulation }; // BiologicallyDerivedProductManipulationComponent 2839 case -1884274053: 2840 /* storage */ return this.storage == null ? new Base[0] : this.storage.toArray(new Base[this.storage.size()]); // BiologicallyDerivedProductStorageComponent 2841 default: 2842 return super.getProperty(hash, name, checkValid); 2843 } 2844 2845 } 2846 2847 @Override 2848 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2849 switch (hash) { 2850 case -1618432855: // identifier 2851 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2852 return value; 2853 case 197299981: // productCategory 2854 value = new BiologicallyDerivedProductCategoryEnumFactory().fromType(castToCode(value)); 2855 this.productCategory = (Enumeration) value; // Enumeration<BiologicallyDerivedProductCategory> 2856 return value; 2857 case -1492131972: // productCode 2858 this.productCode = castToCodeableConcept(value); // CodeableConcept 2859 return value; 2860 case -892481550: // status 2861 value = new BiologicallyDerivedProductStatusEnumFactory().fromType(castToCode(value)); 2862 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStatus> 2863 return value; 2864 case 1095692943: // request 2865 this.getRequest().add(castToReference(value)); // Reference 2866 return value; 2867 case -1285004149: // quantity 2868 this.quantity = castToInteger(value); // IntegerType 2869 return value; 2870 case -995424086: // parent 2871 this.getParent().add(castToReference(value)); // Reference 2872 return value; 2873 case -1741312354: // collection 2874 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 2875 return value; 2876 case 422194963: // processing 2877 this.getProcessing().add((BiologicallyDerivedProductProcessingComponent) value); // BiologicallyDerivedProductProcessingComponent 2878 return value; 2879 case -696214627: // manipulation 2880 this.manipulation = (BiologicallyDerivedProductManipulationComponent) value; // BiologicallyDerivedProductManipulationComponent 2881 return value; 2882 case -1884274053: // storage 2883 this.getStorage().add((BiologicallyDerivedProductStorageComponent) value); // BiologicallyDerivedProductStorageComponent 2884 return value; 2885 default: 2886 return super.setProperty(hash, name, value); 2887 } 2888 2889 } 2890 2891 @Override 2892 public Base setProperty(String name, Base value) throws FHIRException { 2893 if (name.equals("identifier")) { 2894 this.getIdentifier().add(castToIdentifier(value)); 2895 } else if (name.equals("productCategory")) { 2896 value = new BiologicallyDerivedProductCategoryEnumFactory().fromType(castToCode(value)); 2897 this.productCategory = (Enumeration) value; // Enumeration<BiologicallyDerivedProductCategory> 2898 } else if (name.equals("productCode")) { 2899 this.productCode = castToCodeableConcept(value); // CodeableConcept 2900 } else if (name.equals("status")) { 2901 value = new BiologicallyDerivedProductStatusEnumFactory().fromType(castToCode(value)); 2902 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStatus> 2903 } else if (name.equals("request")) { 2904 this.getRequest().add(castToReference(value)); 2905 } else if (name.equals("quantity")) { 2906 this.quantity = castToInteger(value); // IntegerType 2907 } else if (name.equals("parent")) { 2908 this.getParent().add(castToReference(value)); 2909 } else if (name.equals("collection")) { 2910 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 2911 } else if (name.equals("processing")) { 2912 this.getProcessing().add((BiologicallyDerivedProductProcessingComponent) value); 2913 } else if (name.equals("manipulation")) { 2914 this.manipulation = (BiologicallyDerivedProductManipulationComponent) value; // BiologicallyDerivedProductManipulationComponent 2915 } else if (name.equals("storage")) { 2916 this.getStorage().add((BiologicallyDerivedProductStorageComponent) value); 2917 } else 2918 return super.setProperty(name, value); 2919 return value; 2920 } 2921 2922 @Override 2923 public void removeChild(String name, Base value) throws FHIRException { 2924 if (name.equals("identifier")) { 2925 this.getIdentifier().remove(castToIdentifier(value)); 2926 } else if (name.equals("productCategory")) { 2927 this.productCategory = null; 2928 } else if (name.equals("productCode")) { 2929 this.productCode = null; 2930 } else if (name.equals("status")) { 2931 this.status = null; 2932 } else if (name.equals("request")) { 2933 this.getRequest().remove(castToReference(value)); 2934 } else if (name.equals("quantity")) { 2935 this.quantity = null; 2936 } else if (name.equals("parent")) { 2937 this.getParent().remove(castToReference(value)); 2938 } else if (name.equals("collection")) { 2939 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 2940 } else if (name.equals("processing")) { 2941 this.getProcessing().remove((BiologicallyDerivedProductProcessingComponent) value); 2942 } else if (name.equals("manipulation")) { 2943 this.manipulation = (BiologicallyDerivedProductManipulationComponent) value; // BiologicallyDerivedProductManipulationComponent 2944 } else if (name.equals("storage")) { 2945 this.getStorage().remove((BiologicallyDerivedProductStorageComponent) value); 2946 } else 2947 super.removeChild(name, value); 2948 2949 } 2950 2951 @Override 2952 public Base makeProperty(int hash, String name) throws FHIRException { 2953 switch (hash) { 2954 case -1618432855: 2955 return addIdentifier(); 2956 case 197299981: 2957 return getProductCategoryElement(); 2958 case -1492131972: 2959 return getProductCode(); 2960 case -892481550: 2961 return getStatusElement(); 2962 case 1095692943: 2963 return addRequest(); 2964 case -1285004149: 2965 return getQuantityElement(); 2966 case -995424086: 2967 return addParent(); 2968 case -1741312354: 2969 return getCollection(); 2970 case 422194963: 2971 return addProcessing(); 2972 case -696214627: 2973 return getManipulation(); 2974 case -1884274053: 2975 return addStorage(); 2976 default: 2977 return super.makeProperty(hash, name); 2978 } 2979 2980 } 2981 2982 @Override 2983 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2984 switch (hash) { 2985 case -1618432855: 2986 /* identifier */ return new String[] { "Identifier" }; 2987 case 197299981: 2988 /* productCategory */ return new String[] { "code" }; 2989 case -1492131972: 2990 /* productCode */ return new String[] { "CodeableConcept" }; 2991 case -892481550: 2992 /* status */ return new String[] { "code" }; 2993 case 1095692943: 2994 /* request */ return new String[] { "Reference" }; 2995 case -1285004149: 2996 /* quantity */ return new String[] { "integer" }; 2997 case -995424086: 2998 /* parent */ return new String[] { "Reference" }; 2999 case -1741312354: 3000 /* collection */ return new String[] {}; 3001 case 422194963: 3002 /* processing */ return new String[] {}; 3003 case -696214627: 3004 /* manipulation */ return new String[] {}; 3005 case -1884274053: 3006 /* storage */ return new String[] {}; 3007 default: 3008 return super.getTypesForProperty(hash, name); 3009 } 3010 3011 } 3012 3013 @Override 3014 public Base addChild(String name) throws FHIRException { 3015 if (name.equals("identifier")) { 3016 return addIdentifier(); 3017 } else if (name.equals("productCategory")) { 3018 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.productCategory"); 3019 } else if (name.equals("productCode")) { 3020 this.productCode = new CodeableConcept(); 3021 return this.productCode; 3022 } else if (name.equals("status")) { 3023 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.status"); 3024 } else if (name.equals("request")) { 3025 return addRequest(); 3026 } else if (name.equals("quantity")) { 3027 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.quantity"); 3028 } else if (name.equals("parent")) { 3029 return addParent(); 3030 } else if (name.equals("collection")) { 3031 this.collection = new BiologicallyDerivedProductCollectionComponent(); 3032 return this.collection; 3033 } else if (name.equals("processing")) { 3034 return addProcessing(); 3035 } else if (name.equals("manipulation")) { 3036 this.manipulation = new BiologicallyDerivedProductManipulationComponent(); 3037 return this.manipulation; 3038 } else if (name.equals("storage")) { 3039 return addStorage(); 3040 } else 3041 return super.addChild(name); 3042 } 3043 3044 public String fhirType() { 3045 return "BiologicallyDerivedProduct"; 3046 3047 } 3048 3049 public BiologicallyDerivedProduct copy() { 3050 BiologicallyDerivedProduct dst = new BiologicallyDerivedProduct(); 3051 copyValues(dst); 3052 return dst; 3053 } 3054 3055 public void copyValues(BiologicallyDerivedProduct dst) { 3056 super.copyValues(dst); 3057 if (identifier != null) { 3058 dst.identifier = new ArrayList<Identifier>(); 3059 for (Identifier i : identifier) 3060 dst.identifier.add(i.copy()); 3061 } 3062 ; 3063 dst.productCategory = productCategory == null ? null : productCategory.copy(); 3064 dst.productCode = productCode == null ? null : productCode.copy(); 3065 dst.status = status == null ? null : status.copy(); 3066 if (request != null) { 3067 dst.request = new ArrayList<Reference>(); 3068 for (Reference i : request) 3069 dst.request.add(i.copy()); 3070 } 3071 ; 3072 dst.quantity = quantity == null ? null : quantity.copy(); 3073 if (parent != null) { 3074 dst.parent = new ArrayList<Reference>(); 3075 for (Reference i : parent) 3076 dst.parent.add(i.copy()); 3077 } 3078 ; 3079 dst.collection = collection == null ? null : collection.copy(); 3080 if (processing != null) { 3081 dst.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 3082 for (BiologicallyDerivedProductProcessingComponent i : processing) 3083 dst.processing.add(i.copy()); 3084 } 3085 ; 3086 dst.manipulation = manipulation == null ? null : manipulation.copy(); 3087 if (storage != null) { 3088 dst.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 3089 for (BiologicallyDerivedProductStorageComponent i : storage) 3090 dst.storage.add(i.copy()); 3091 } 3092 ; 3093 } 3094 3095 protected BiologicallyDerivedProduct typedCopy() { 3096 return copy(); 3097 } 3098 3099 @Override 3100 public boolean equalsDeep(Base other_) { 3101 if (!super.equalsDeep(other_)) 3102 return false; 3103 if (!(other_ instanceof BiologicallyDerivedProduct)) 3104 return false; 3105 BiologicallyDerivedProduct o = (BiologicallyDerivedProduct) other_; 3106 return compareDeep(identifier, o.identifier, true) && compareDeep(productCategory, o.productCategory, true) 3107 && compareDeep(productCode, o.productCode, true) && compareDeep(status, o.status, true) 3108 && compareDeep(request, o.request, true) && compareDeep(quantity, o.quantity, true) 3109 && compareDeep(parent, o.parent, true) && compareDeep(collection, o.collection, true) 3110 && compareDeep(processing, o.processing, true) && compareDeep(manipulation, o.manipulation, true) 3111 && compareDeep(storage, o.storage, true); 3112 } 3113 3114 @Override 3115 public boolean equalsShallow(Base other_) { 3116 if (!super.equalsShallow(other_)) 3117 return false; 3118 if (!(other_ instanceof BiologicallyDerivedProduct)) 3119 return false; 3120 BiologicallyDerivedProduct o = (BiologicallyDerivedProduct) other_; 3121 return compareValues(productCategory, o.productCategory, true) && compareValues(status, o.status, true) 3122 && compareValues(quantity, o.quantity, true); 3123 } 3124 3125 public boolean isEmpty() { 3126 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, productCategory, productCode, status, 3127 request, quantity, parent, collection, processing, manipulation, storage); 3128 } 3129 3130 @Override 3131 public ResourceType getResourceType() { 3132 return ResourceType.BiologicallyDerivedProduct; 3133 } 3134 3135}