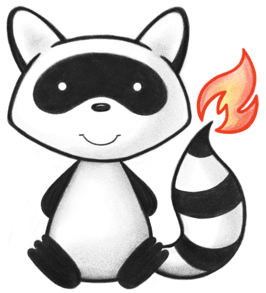
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046 047/** 048 * A material substance originating from a biological entity intended to be 049 * transplanted or infused into another (possibly the same) biological entity. 050 */ 051@ResourceDef(name = "BiologicallyDerivedProduct", profile = "http://hl7.org/fhir/StructureDefinition/BiologicallyDerivedProduct") 052public class BiologicallyDerivedProduct extends DomainResource { 053 054 public enum BiologicallyDerivedProductCategory { 055 /** 056 * A collection of tissues joined in a structural unit to serve a common 057 * function. 058 */ 059 ORGAN, 060 /** 061 * An ensemble of similar cells and their extracellular matrix from the same 062 * origin that together carry out a specific function. 063 */ 064 TISSUE, 065 /** 066 * Body fluid. 067 */ 068 FLUID, 069 /** 070 * Collection of cells. 071 */ 072 CELLS, 073 /** 074 * Biological agent of unspecified type. 075 */ 076 BIOLOGICALAGENT, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static BiologicallyDerivedProductCategory fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("organ".equals(codeString)) 086 return ORGAN; 087 if ("tissue".equals(codeString)) 088 return TISSUE; 089 if ("fluid".equals(codeString)) 090 return FLUID; 091 if ("cells".equals(codeString)) 092 return CELLS; 093 if ("biologicalAgent".equals(codeString)) 094 return BIOLOGICALAGENT; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown BiologicallyDerivedProductCategory code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case ORGAN: 104 return "organ"; 105 case TISSUE: 106 return "tissue"; 107 case FLUID: 108 return "fluid"; 109 case CELLS: 110 return "cells"; 111 case BIOLOGICALAGENT: 112 return "biologicalAgent"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case ORGAN: 123 return "http://hl7.org/fhir/product-category"; 124 case TISSUE: 125 return "http://hl7.org/fhir/product-category"; 126 case FLUID: 127 return "http://hl7.org/fhir/product-category"; 128 case CELLS: 129 return "http://hl7.org/fhir/product-category"; 130 case BIOLOGICALAGENT: 131 return "http://hl7.org/fhir/product-category"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case ORGAN: 142 return "A collection of tissues joined in a structural unit to serve a common function."; 143 case TISSUE: 144 return "An ensemble of similar cells and their extracellular matrix from the same origin that together carry out a specific function."; 145 case FLUID: 146 return "Body fluid."; 147 case CELLS: 148 return "Collection of cells."; 149 case BIOLOGICALAGENT: 150 return "Biological agent of unspecified type."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case ORGAN: 161 return "Organ"; 162 case TISSUE: 163 return "Tissue"; 164 case FLUID: 165 return "Fluid"; 166 case CELLS: 167 return "Cells"; 168 case BIOLOGICALAGENT: 169 return "BiologicalAgent"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class BiologicallyDerivedProductCategoryEnumFactory 179 implements EnumFactory<BiologicallyDerivedProductCategory> { 180 public BiologicallyDerivedProductCategory fromCode(String codeString) throws IllegalArgumentException { 181 if (codeString == null || "".equals(codeString)) 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("organ".equals(codeString)) 185 return BiologicallyDerivedProductCategory.ORGAN; 186 if ("tissue".equals(codeString)) 187 return BiologicallyDerivedProductCategory.TISSUE; 188 if ("fluid".equals(codeString)) 189 return BiologicallyDerivedProductCategory.FLUID; 190 if ("cells".equals(codeString)) 191 return BiologicallyDerivedProductCategory.CELLS; 192 if ("biologicalAgent".equals(codeString)) 193 return BiologicallyDerivedProductCategory.BIOLOGICALAGENT; 194 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductCategory code '" + codeString + "'"); 195 } 196 197 public Enumeration<BiologicallyDerivedProductCategory> fromType(PrimitiveType<?> code) throws FHIRException { 198 if (code == null) 199 return null; 200 if (code.isEmpty()) 201 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.NULL, code); 202 String codeString = code.asStringValue(); 203 if (codeString == null || "".equals(codeString)) 204 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.NULL, code); 205 if ("organ".equals(codeString)) 206 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.ORGAN, 207 code); 208 if ("tissue".equals(codeString)) 209 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.TISSUE, 210 code); 211 if ("fluid".equals(codeString)) 212 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.FLUID, 213 code); 214 if ("cells".equals(codeString)) 215 return new Enumeration<BiologicallyDerivedProductCategory>(this, BiologicallyDerivedProductCategory.CELLS, 216 code); 217 if ("biologicalAgent".equals(codeString)) 218 return new Enumeration<BiologicallyDerivedProductCategory>(this, 219 BiologicallyDerivedProductCategory.BIOLOGICALAGENT, code); 220 throw new FHIRException("Unknown BiologicallyDerivedProductCategory code '" + codeString + "'"); 221 } 222 223 public String toCode(BiologicallyDerivedProductCategory code) { 224 if (code == BiologicallyDerivedProductCategory.NULL) 225 return null; 226 if (code == BiologicallyDerivedProductCategory.ORGAN) 227 return "organ"; 228 if (code == BiologicallyDerivedProductCategory.TISSUE) 229 return "tissue"; 230 if (code == BiologicallyDerivedProductCategory.FLUID) 231 return "fluid"; 232 if (code == BiologicallyDerivedProductCategory.CELLS) 233 return "cells"; 234 if (code == BiologicallyDerivedProductCategory.BIOLOGICALAGENT) 235 return "biologicalAgent"; 236 return "?"; 237 } 238 239 public String toSystem(BiologicallyDerivedProductCategory code) { 240 return code.getSystem(); 241 } 242 } 243 244 public enum BiologicallyDerivedProductStatus { 245 /** 246 * Product is currently available for use. 247 */ 248 AVAILABLE, 249 /** 250 * Product is not currently available for use. 251 */ 252 UNAVAILABLE, 253 /** 254 * added to help the parsers with the generic types 255 */ 256 NULL; 257 258 public static BiologicallyDerivedProductStatus fromCode(String codeString) throws FHIRException { 259 if (codeString == null || "".equals(codeString)) 260 return null; 261 if ("available".equals(codeString)) 262 return AVAILABLE; 263 if ("unavailable".equals(codeString)) 264 return UNAVAILABLE; 265 if (Configuration.isAcceptInvalidEnums()) 266 return null; 267 else 268 throw new FHIRException("Unknown BiologicallyDerivedProductStatus code '" + codeString + "'"); 269 } 270 271 public String toCode() { 272 switch (this) { 273 case AVAILABLE: 274 return "available"; 275 case UNAVAILABLE: 276 return "unavailable"; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getSystem() { 285 switch (this) { 286 case AVAILABLE: 287 return "http://hl7.org/fhir/product-status"; 288 case UNAVAILABLE: 289 return "http://hl7.org/fhir/product-status"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 297 public String getDefinition() { 298 switch (this) { 299 case AVAILABLE: 300 return "Product is currently available for use."; 301 case UNAVAILABLE: 302 return "Product is not currently available for use."; 303 case NULL: 304 return null; 305 default: 306 return "?"; 307 } 308 } 309 310 public String getDisplay() { 311 switch (this) { 312 case AVAILABLE: 313 return "Available"; 314 case UNAVAILABLE: 315 return "Unavailable"; 316 case NULL: 317 return null; 318 default: 319 return "?"; 320 } 321 } 322 } 323 324 public static class BiologicallyDerivedProductStatusEnumFactory 325 implements EnumFactory<BiologicallyDerivedProductStatus> { 326 public BiologicallyDerivedProductStatus fromCode(String codeString) throws IllegalArgumentException { 327 if (codeString == null || "".equals(codeString)) 328 if (codeString == null || "".equals(codeString)) 329 return null; 330 if ("available".equals(codeString)) 331 return BiologicallyDerivedProductStatus.AVAILABLE; 332 if ("unavailable".equals(codeString)) 333 return BiologicallyDerivedProductStatus.UNAVAILABLE; 334 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductStatus code '" + codeString + "'"); 335 } 336 337 public Enumeration<BiologicallyDerivedProductStatus> fromType(PrimitiveType<?> code) throws FHIRException { 338 if (code == null) 339 return null; 340 if (code.isEmpty()) 341 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.NULL, code); 342 String codeString = code.asStringValue(); 343 if (codeString == null || "".equals(codeString)) 344 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.NULL, code); 345 if ("available".equals(codeString)) 346 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.AVAILABLE, 347 code); 348 if ("unavailable".equals(codeString)) 349 return new Enumeration<BiologicallyDerivedProductStatus>(this, BiologicallyDerivedProductStatus.UNAVAILABLE, 350 code); 351 throw new FHIRException("Unknown BiologicallyDerivedProductStatus code '" + codeString + "'"); 352 } 353 354 public String toCode(BiologicallyDerivedProductStatus code) { 355 if (code == BiologicallyDerivedProductStatus.NULL) 356 return null; 357 if (code == BiologicallyDerivedProductStatus.AVAILABLE) 358 return "available"; 359 if (code == BiologicallyDerivedProductStatus.UNAVAILABLE) 360 return "unavailable"; 361 return "?"; 362 } 363 364 public String toSystem(BiologicallyDerivedProductStatus code) { 365 return code.getSystem(); 366 } 367 } 368 369 public enum BiologicallyDerivedProductStorageScale { 370 /** 371 * Fahrenheit temperature scale. 372 */ 373 FARENHEIT, 374 /** 375 * Celsius or centigrade temperature scale. 376 */ 377 CELSIUS, 378 /** 379 * Kelvin absolute thermodynamic temperature scale. 380 */ 381 KELVIN, 382 /** 383 * added to help the parsers with the generic types 384 */ 385 NULL; 386 387 public static BiologicallyDerivedProductStorageScale fromCode(String codeString) throws FHIRException { 388 if (codeString == null || "".equals(codeString)) 389 return null; 390 if ("farenheit".equals(codeString)) 391 return FARENHEIT; 392 if ("celsius".equals(codeString)) 393 return CELSIUS; 394 if ("kelvin".equals(codeString)) 395 return KELVIN; 396 if (Configuration.isAcceptInvalidEnums()) 397 return null; 398 else 399 throw new FHIRException("Unknown BiologicallyDerivedProductStorageScale code '" + codeString + "'"); 400 } 401 402 public String toCode() { 403 switch (this) { 404 case FARENHEIT: 405 return "farenheit"; 406 case CELSIUS: 407 return "celsius"; 408 case KELVIN: 409 return "kelvin"; 410 case NULL: 411 return null; 412 default: 413 return "?"; 414 } 415 } 416 417 public String getSystem() { 418 switch (this) { 419 case FARENHEIT: 420 return "http://hl7.org/fhir/product-storage-scale"; 421 case CELSIUS: 422 return "http://hl7.org/fhir/product-storage-scale"; 423 case KELVIN: 424 return "http://hl7.org/fhir/product-storage-scale"; 425 case NULL: 426 return null; 427 default: 428 return "?"; 429 } 430 } 431 432 public String getDefinition() { 433 switch (this) { 434 case FARENHEIT: 435 return "Fahrenheit temperature scale."; 436 case CELSIUS: 437 return "Celsius or centigrade temperature scale."; 438 case KELVIN: 439 return "Kelvin absolute thermodynamic temperature scale."; 440 case NULL: 441 return null; 442 default: 443 return "?"; 444 } 445 } 446 447 public String getDisplay() { 448 switch (this) { 449 case FARENHEIT: 450 return "Fahrenheit"; 451 case CELSIUS: 452 return "Celsius"; 453 case KELVIN: 454 return "Kelvin"; 455 case NULL: 456 return null; 457 default: 458 return "?"; 459 } 460 } 461 } 462 463 public static class BiologicallyDerivedProductStorageScaleEnumFactory 464 implements EnumFactory<BiologicallyDerivedProductStorageScale> { 465 public BiologicallyDerivedProductStorageScale fromCode(String codeString) throws IllegalArgumentException { 466 if (codeString == null || "".equals(codeString)) 467 if (codeString == null || "".equals(codeString)) 468 return null; 469 if ("farenheit".equals(codeString)) 470 return BiologicallyDerivedProductStorageScale.FARENHEIT; 471 if ("celsius".equals(codeString)) 472 return BiologicallyDerivedProductStorageScale.CELSIUS; 473 if ("kelvin".equals(codeString)) 474 return BiologicallyDerivedProductStorageScale.KELVIN; 475 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductStorageScale code '" + codeString + "'"); 476 } 477 478 public Enumeration<BiologicallyDerivedProductStorageScale> fromType(PrimitiveType<?> code) throws FHIRException { 479 if (code == null) 480 return null; 481 if (code.isEmpty()) 482 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 483 BiologicallyDerivedProductStorageScale.NULL, code); 484 String codeString = code.asStringValue(); 485 if (codeString == null || "".equals(codeString)) 486 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 487 BiologicallyDerivedProductStorageScale.NULL, code); 488 if ("farenheit".equals(codeString)) 489 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 490 BiologicallyDerivedProductStorageScale.FARENHEIT, code); 491 if ("celsius".equals(codeString)) 492 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 493 BiologicallyDerivedProductStorageScale.CELSIUS, code); 494 if ("kelvin".equals(codeString)) 495 return new Enumeration<BiologicallyDerivedProductStorageScale>(this, 496 BiologicallyDerivedProductStorageScale.KELVIN, code); 497 throw new FHIRException("Unknown BiologicallyDerivedProductStorageScale code '" + codeString + "'"); 498 } 499 500 public String toCode(BiologicallyDerivedProductStorageScale code) { 501 if (code == BiologicallyDerivedProductStorageScale.NULL) 502 return null; 503 if (code == BiologicallyDerivedProductStorageScale.FARENHEIT) 504 return "farenheit"; 505 if (code == BiologicallyDerivedProductStorageScale.CELSIUS) 506 return "celsius"; 507 if (code == BiologicallyDerivedProductStorageScale.KELVIN) 508 return "kelvin"; 509 return "?"; 510 } 511 512 public String toSystem(BiologicallyDerivedProductStorageScale code) { 513 return code.getSystem(); 514 } 515 } 516 517 @Block() 518 public static class BiologicallyDerivedProductCollectionComponent extends BackboneElement 519 implements IBaseBackboneElement { 520 /** 521 * Healthcare professional who is performing the collection. 522 */ 523 @Child(name = "collector", type = { Practitioner.class, 524 PractitionerRole.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 525 @Description(shortDefinition = "Individual performing collection", formalDefinition = "Healthcare professional who is performing the collection.") 526 protected Reference collector; 527 528 /** 529 * The actual object that is the target of the reference (Healthcare 530 * professional who is performing the collection.) 531 */ 532 protected Resource collectorTarget; 533 534 /** 535 * The patient or entity, such as a hospital or vendor in the case of a 536 * processed/manipulated/manufactured product, providing the product. 537 */ 538 @Child(name = "source", type = { Patient.class, 539 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 540 @Description(shortDefinition = "Who is product from", formalDefinition = "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.") 541 protected Reference source; 542 543 /** 544 * The actual object that is the target of the reference (The patient or entity, 545 * such as a hospital or vendor in the case of a 546 * processed/manipulated/manufactured product, providing the product.) 547 */ 548 protected Resource sourceTarget; 549 550 /** 551 * Time of product collection. 552 */ 553 @Child(name = "collected", type = { DateTimeType.class, 554 Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 555 @Description(shortDefinition = "Time of product collection", formalDefinition = "Time of product collection.") 556 protected Type collected; 557 558 private static final long serialVersionUID = 892130089L; 559 560 /** 561 * Constructor 562 */ 563 public BiologicallyDerivedProductCollectionComponent() { 564 super(); 565 } 566 567 /** 568 * @return {@link #collector} (Healthcare professional who is performing the 569 * collection.) 570 */ 571 public Reference getCollector() { 572 if (this.collector == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create BiologicallyDerivedProductCollectionComponent.collector"); 575 else if (Configuration.doAutoCreate()) 576 this.collector = new Reference(); // cc 577 return this.collector; 578 } 579 580 public boolean hasCollector() { 581 return this.collector != null && !this.collector.isEmpty(); 582 } 583 584 /** 585 * @param value {@link #collector} (Healthcare professional who is performing 586 * the collection.) 587 */ 588 public BiologicallyDerivedProductCollectionComponent setCollector(Reference value) { 589 this.collector = value; 590 return this; 591 } 592 593 /** 594 * @return {@link #collector} The actual object that is the target of the 595 * reference. The reference library doesn't populate this, but you can 596 * use it to hold the resource if you resolve it. (Healthcare 597 * professional who is performing the collection.) 598 */ 599 public Resource getCollectorTarget() { 600 return this.collectorTarget; 601 } 602 603 /** 604 * @param value {@link #collector} The actual object that is the target of the 605 * reference. The reference library doesn't use these, but you can 606 * use it to hold the resource if you resolve it. (Healthcare 607 * professional who is performing the collection.) 608 */ 609 public BiologicallyDerivedProductCollectionComponent setCollectorTarget(Resource value) { 610 this.collectorTarget = value; 611 return this; 612 } 613 614 /** 615 * @return {@link #source} (The patient or entity, such as a hospital or vendor 616 * in the case of a processed/manipulated/manufactured product, 617 * providing the product.) 618 */ 619 public Reference getSource() { 620 if (this.source == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create BiologicallyDerivedProductCollectionComponent.source"); 623 else if (Configuration.doAutoCreate()) 624 this.source = new Reference(); // cc 625 return this.source; 626 } 627 628 public boolean hasSource() { 629 return this.source != null && !this.source.isEmpty(); 630 } 631 632 /** 633 * @param value {@link #source} (The patient or entity, such as a hospital or 634 * vendor in the case of a processed/manipulated/manufactured 635 * product, providing the product.) 636 */ 637 public BiologicallyDerivedProductCollectionComponent setSource(Reference value) { 638 this.source = value; 639 return this; 640 } 641 642 /** 643 * @return {@link #source} The actual object that is the target of the 644 * reference. The reference library doesn't populate this, but you can 645 * use it to hold the resource if you resolve it. (The patient or 646 * entity, such as a hospital or vendor in the case of a 647 * processed/manipulated/manufactured product, providing the product.) 648 */ 649 public Resource getSourceTarget() { 650 return this.sourceTarget; 651 } 652 653 /** 654 * @param value {@link #source} The actual object that is the target of the 655 * reference. The reference library doesn't use these, but you can 656 * use it to hold the resource if you resolve it. (The patient or 657 * entity, such as a hospital or vendor in the case of a 658 * processed/manipulated/manufactured product, providing the 659 * product.) 660 */ 661 public BiologicallyDerivedProductCollectionComponent setSourceTarget(Resource value) { 662 this.sourceTarget = value; 663 return this; 664 } 665 666 /** 667 * @return {@link #collected} (Time of product collection.) 668 */ 669 public Type getCollected() { 670 return this.collected; 671 } 672 673 /** 674 * @return {@link #collected} (Time of product collection.) 675 */ 676 public DateTimeType getCollectedDateTimeType() throws FHIRException { 677 if (this.collected == null) 678 this.collected = new DateTimeType(); 679 if (!(this.collected instanceof DateTimeType)) 680 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 681 + this.collected.getClass().getName() + " was encountered"); 682 return (DateTimeType) this.collected; 683 } 684 685 public boolean hasCollectedDateTimeType() { 686 return this.collected instanceof DateTimeType; 687 } 688 689 /** 690 * @return {@link #collected} (Time of product collection.) 691 */ 692 public Period getCollectedPeriod() throws FHIRException { 693 if (this.collected == null) 694 this.collected = new Period(); 695 if (!(this.collected instanceof Period)) 696 throw new FHIRException("Type mismatch: the type Period was expected, but " 697 + this.collected.getClass().getName() + " was encountered"); 698 return (Period) this.collected; 699 } 700 701 public boolean hasCollectedPeriod() { 702 return this.collected instanceof Period; 703 } 704 705 public boolean hasCollected() { 706 return this.collected != null && !this.collected.isEmpty(); 707 } 708 709 /** 710 * @param value {@link #collected} (Time of product collection.) 711 */ 712 public BiologicallyDerivedProductCollectionComponent setCollected(Type value) { 713 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 714 throw new Error( 715 "Not the right type for BiologicallyDerivedProduct.collection.collected[x]: " + value.fhirType()); 716 this.collected = value; 717 return this; 718 } 719 720 protected void listChildren(List<Property> children) { 721 super.listChildren(children); 722 children.add(new Property("collector", "Reference(Practitioner|PractitionerRole)", 723 "Healthcare professional who is performing the collection.", 0, 1, collector)); 724 children.add(new Property("source", "Reference(Patient|Organization)", 725 "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.", 726 0, 1, source)); 727 children.add(new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, collected)); 728 } 729 730 @Override 731 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 732 switch (_hash) { 733 case 1883491469: 734 /* collector */ return new Property("collector", "Reference(Practitioner|PractitionerRole)", 735 "Healthcare professional who is performing the collection.", 0, 1, collector); 736 case -896505829: 737 /* source */ return new Property("source", "Reference(Patient|Organization)", 738 "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.", 739 0, 1, source); 740 case 1632037015: 741 /* collected[x] */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, 742 collected); 743 case 1883491145: 744 /* collected */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, 745 collected); 746 case 2005009924: 747 /* collectedDateTime */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 748 1, collected); 749 case 653185642: 750 /* collectedPeriod */ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 751 1, collected); 752 default: 753 return super.getNamedProperty(_hash, _name, _checkValid); 754 } 755 756 } 757 758 @Override 759 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 760 switch (hash) { 761 case 1883491469: 762 /* collector */ return this.collector == null ? new Base[0] : new Base[] { this.collector }; // Reference 763 case -896505829: 764 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 765 case 1883491145: 766 /* collected */ return this.collected == null ? new Base[0] : new Base[] { this.collected }; // Type 767 default: 768 return super.getProperty(hash, name, checkValid); 769 } 770 771 } 772 773 @Override 774 public Base setProperty(int hash, String name, Base value) throws FHIRException { 775 switch (hash) { 776 case 1883491469: // collector 777 this.collector = castToReference(value); // Reference 778 return value; 779 case -896505829: // source 780 this.source = castToReference(value); // Reference 781 return value; 782 case 1883491145: // collected 783 this.collected = castToType(value); // Type 784 return value; 785 default: 786 return super.setProperty(hash, name, value); 787 } 788 789 } 790 791 @Override 792 public Base setProperty(String name, Base value) throws FHIRException { 793 if (name.equals("collector")) { 794 this.collector = castToReference(value); // Reference 795 } else if (name.equals("source")) { 796 this.source = castToReference(value); // Reference 797 } else if (name.equals("collected[x]")) { 798 this.collected = castToType(value); // Type 799 } else 800 return super.setProperty(name, value); 801 return value; 802 } 803 804 @Override 805 public void removeChild(String name, Base value) throws FHIRException { 806 if (name.equals("collector")) { 807 this.collector = null; 808 } else if (name.equals("source")) { 809 this.source = null; 810 } else if (name.equals("collected[x]")) { 811 this.collected = null; 812 } else 813 super.removeChild(name, value); 814 815 } 816 817 @Override 818 public Base makeProperty(int hash, String name) throws FHIRException { 819 switch (hash) { 820 case 1883491469: 821 return getCollector(); 822 case -896505829: 823 return getSource(); 824 case 1632037015: 825 return getCollected(); 826 case 1883491145: 827 return getCollected(); 828 default: 829 return super.makeProperty(hash, name); 830 } 831 832 } 833 834 @Override 835 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 836 switch (hash) { 837 case 1883491469: 838 /* collector */ return new String[] { "Reference" }; 839 case -896505829: 840 /* source */ return new String[] { "Reference" }; 841 case 1883491145: 842 /* collected */ return new String[] { "dateTime", "Period" }; 843 default: 844 return super.getTypesForProperty(hash, name); 845 } 846 847 } 848 849 @Override 850 public Base addChild(String name) throws FHIRException { 851 if (name.equals("collector")) { 852 this.collector = new Reference(); 853 return this.collector; 854 } else if (name.equals("source")) { 855 this.source = new Reference(); 856 return this.source; 857 } else if (name.equals("collectedDateTime")) { 858 this.collected = new DateTimeType(); 859 return this.collected; 860 } else if (name.equals("collectedPeriod")) { 861 this.collected = new Period(); 862 return this.collected; 863 } else 864 return super.addChild(name); 865 } 866 867 public BiologicallyDerivedProductCollectionComponent copy() { 868 BiologicallyDerivedProductCollectionComponent dst = new BiologicallyDerivedProductCollectionComponent(); 869 copyValues(dst); 870 return dst; 871 } 872 873 public void copyValues(BiologicallyDerivedProductCollectionComponent dst) { 874 super.copyValues(dst); 875 dst.collector = collector == null ? null : collector.copy(); 876 dst.source = source == null ? null : source.copy(); 877 dst.collected = collected == null ? null : collected.copy(); 878 } 879 880 @Override 881 public boolean equalsDeep(Base other_) { 882 if (!super.equalsDeep(other_)) 883 return false; 884 if (!(other_ instanceof BiologicallyDerivedProductCollectionComponent)) 885 return false; 886 BiologicallyDerivedProductCollectionComponent o = (BiologicallyDerivedProductCollectionComponent) other_; 887 return compareDeep(collector, o.collector, true) && compareDeep(source, o.source, true) 888 && compareDeep(collected, o.collected, true); 889 } 890 891 @Override 892 public boolean equalsShallow(Base other_) { 893 if (!super.equalsShallow(other_)) 894 return false; 895 if (!(other_ instanceof BiologicallyDerivedProductCollectionComponent)) 896 return false; 897 BiologicallyDerivedProductCollectionComponent o = (BiologicallyDerivedProductCollectionComponent) other_; 898 return true; 899 } 900 901 public boolean isEmpty() { 902 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, source, collected); 903 } 904 905 public String fhirType() { 906 return "BiologicallyDerivedProduct.collection"; 907 908 } 909 910 } 911 912 @Block() 913 public static class BiologicallyDerivedProductProcessingComponent extends BackboneElement 914 implements IBaseBackboneElement { 915 /** 916 * Description of of processing. 917 */ 918 @Child(name = "description", type = { 919 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 920 @Description(shortDefinition = "Description of of processing", formalDefinition = "Description of of processing.") 921 protected StringType description; 922 923 /** 924 * Procesing code. 925 */ 926 @Child(name = "procedure", type = { 927 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 928 @Description(shortDefinition = "Procesing code", formalDefinition = "Procesing code.") 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 930 protected CodeableConcept procedure; 931 932 /** 933 * Substance added during processing. 934 */ 935 @Child(name = "additive", type = { 936 Substance.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 937 @Description(shortDefinition = "Substance added during processing", formalDefinition = "Substance added during processing.") 938 protected Reference additive; 939 940 /** 941 * The actual object that is the target of the reference (Substance added during 942 * processing.) 943 */ 944 protected Substance additiveTarget; 945 946 /** 947 * Time of processing. 948 */ 949 @Child(name = "time", type = { DateTimeType.class, 950 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 951 @Description(shortDefinition = "Time of processing", formalDefinition = "Time of processing.") 952 protected Type time; 953 954 private static final long serialVersionUID = -1007041216L; 955 956 /** 957 * Constructor 958 */ 959 public BiologicallyDerivedProductProcessingComponent() { 960 super(); 961 } 962 963 /** 964 * @return {@link #description} (Description of of processing.). This is the 965 * underlying object with id, value and extensions. The accessor 966 * "getDescription" gives direct access to the value 967 */ 968 public StringType getDescriptionElement() { 969 if (this.description == null) 970 if (Configuration.errorOnAutoCreate()) 971 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.description"); 972 else if (Configuration.doAutoCreate()) 973 this.description = new StringType(); // bb 974 return this.description; 975 } 976 977 public boolean hasDescriptionElement() { 978 return this.description != null && !this.description.isEmpty(); 979 } 980 981 public boolean hasDescription() { 982 return this.description != null && !this.description.isEmpty(); 983 } 984 985 /** 986 * @param value {@link #description} (Description of of processing.). This is 987 * the underlying object with id, value and extensions. The 988 * accessor "getDescription" gives direct access to the value 989 */ 990 public BiologicallyDerivedProductProcessingComponent setDescriptionElement(StringType value) { 991 this.description = value; 992 return this; 993 } 994 995 /** 996 * @return Description of of processing. 997 */ 998 public String getDescription() { 999 return this.description == null ? null : this.description.getValue(); 1000 } 1001 1002 /** 1003 * @param value Description of of processing. 1004 */ 1005 public BiologicallyDerivedProductProcessingComponent setDescription(String value) { 1006 if (Utilities.noString(value)) 1007 this.description = null; 1008 else { 1009 if (this.description == null) 1010 this.description = new StringType(); 1011 this.description.setValue(value); 1012 } 1013 return this; 1014 } 1015 1016 /** 1017 * @return {@link #procedure} (Procesing code.) 1018 */ 1019 public CodeableConcept getProcedure() { 1020 if (this.procedure == null) 1021 if (Configuration.errorOnAutoCreate()) 1022 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.procedure"); 1023 else if (Configuration.doAutoCreate()) 1024 this.procedure = new CodeableConcept(); // cc 1025 return this.procedure; 1026 } 1027 1028 public boolean hasProcedure() { 1029 return this.procedure != null && !this.procedure.isEmpty(); 1030 } 1031 1032 /** 1033 * @param value {@link #procedure} (Procesing code.) 1034 */ 1035 public BiologicallyDerivedProductProcessingComponent setProcedure(CodeableConcept value) { 1036 this.procedure = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return {@link #additive} (Substance added during processing.) 1042 */ 1043 public Reference getAdditive() { 1044 if (this.additive == null) 1045 if (Configuration.errorOnAutoCreate()) 1046 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.additive"); 1047 else if (Configuration.doAutoCreate()) 1048 this.additive = new Reference(); // cc 1049 return this.additive; 1050 } 1051 1052 public boolean hasAdditive() { 1053 return this.additive != null && !this.additive.isEmpty(); 1054 } 1055 1056 /** 1057 * @param value {@link #additive} (Substance added during processing.) 1058 */ 1059 public BiologicallyDerivedProductProcessingComponent setAdditive(Reference value) { 1060 this.additive = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #additive} The actual object that is the target of the 1066 * reference. The reference library doesn't populate this, but you can 1067 * use it to hold the resource if you resolve it. (Substance added 1068 * during processing.) 1069 */ 1070 public Substance getAdditiveTarget() { 1071 if (this.additiveTarget == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create BiologicallyDerivedProductProcessingComponent.additive"); 1074 else if (Configuration.doAutoCreate()) 1075 this.additiveTarget = new Substance(); // aa 1076 return this.additiveTarget; 1077 } 1078 1079 /** 1080 * @param value {@link #additive} The actual object that is the target of the 1081 * reference. The reference library doesn't use these, but you can 1082 * use it to hold the resource if you resolve it. (Substance added 1083 * during processing.) 1084 */ 1085 public BiologicallyDerivedProductProcessingComponent setAdditiveTarget(Substance value) { 1086 this.additiveTarget = value; 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #time} (Time of processing.) 1092 */ 1093 public Type getTime() { 1094 return this.time; 1095 } 1096 1097 /** 1098 * @return {@link #time} (Time of processing.) 1099 */ 1100 public DateTimeType getTimeDateTimeType() throws FHIRException { 1101 if (this.time == null) 1102 this.time = new DateTimeType(); 1103 if (!(this.time instanceof DateTimeType)) 1104 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1105 + this.time.getClass().getName() + " was encountered"); 1106 return (DateTimeType) this.time; 1107 } 1108 1109 public boolean hasTimeDateTimeType() { 1110 return this.time instanceof DateTimeType; 1111 } 1112 1113 /** 1114 * @return {@link #time} (Time of processing.) 1115 */ 1116 public Period getTimePeriod() throws FHIRException { 1117 if (this.time == null) 1118 this.time = new Period(); 1119 if (!(this.time instanceof Period)) 1120 throw new FHIRException( 1121 "Type mismatch: the type Period was expected, but " + this.time.getClass().getName() + " was encountered"); 1122 return (Period) this.time; 1123 } 1124 1125 public boolean hasTimePeriod() { 1126 return this.time instanceof Period; 1127 } 1128 1129 public boolean hasTime() { 1130 return this.time != null && !this.time.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #time} (Time of processing.) 1135 */ 1136 public BiologicallyDerivedProductProcessingComponent setTime(Type value) { 1137 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1138 throw new Error("Not the right type for BiologicallyDerivedProduct.processing.time[x]: " + value.fhirType()); 1139 this.time = value; 1140 return this; 1141 } 1142 1143 protected void listChildren(List<Property> children) { 1144 super.listChildren(children); 1145 children.add(new Property("description", "string", "Description of of processing.", 0, 1, description)); 1146 children.add(new Property("procedure", "CodeableConcept", "Procesing code.", 0, 1, procedure)); 1147 children 1148 .add(new Property("additive", "Reference(Substance)", "Substance added during processing.", 0, 1, additive)); 1149 children.add(new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time)); 1150 } 1151 1152 @Override 1153 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1154 switch (_hash) { 1155 case -1724546052: 1156 /* description */ return new Property("description", "string", "Description of of processing.", 0, 1, 1157 description); 1158 case -1095204141: 1159 /* procedure */ return new Property("procedure", "CodeableConcept", "Procesing code.", 0, 1, procedure); 1160 case -1226589236: 1161 /* additive */ return new Property("additive", "Reference(Substance)", "Substance added during processing.", 0, 1162 1, additive); 1163 case -1313930605: 1164 /* time[x] */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1165 case 3560141: 1166 /* time */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1167 case 2135345544: 1168 /* timeDateTime */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1169 case 693544686: 1170 /* timePeriod */ return new Property("time[x]", "dateTime|Period", "Time of processing.", 0, 1, time); 1171 default: 1172 return super.getNamedProperty(_hash, _name, _checkValid); 1173 } 1174 1175 } 1176 1177 @Override 1178 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1179 switch (hash) { 1180 case -1724546052: 1181 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1182 case -1095204141: 1183 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // CodeableConcept 1184 case -1226589236: 1185 /* additive */ return this.additive == null ? new Base[0] : new Base[] { this.additive }; // Reference 1186 case 3560141: 1187 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // Type 1188 default: 1189 return super.getProperty(hash, name, checkValid); 1190 } 1191 1192 } 1193 1194 @Override 1195 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1196 switch (hash) { 1197 case -1724546052: // description 1198 this.description = castToString(value); // StringType 1199 return value; 1200 case -1095204141: // procedure 1201 this.procedure = castToCodeableConcept(value); // CodeableConcept 1202 return value; 1203 case -1226589236: // additive 1204 this.additive = castToReference(value); // Reference 1205 return value; 1206 case 3560141: // time 1207 this.time = castToType(value); // Type 1208 return value; 1209 default: 1210 return super.setProperty(hash, name, value); 1211 } 1212 1213 } 1214 1215 @Override 1216 public Base setProperty(String name, Base value) throws FHIRException { 1217 if (name.equals("description")) { 1218 this.description = castToString(value); // StringType 1219 } else if (name.equals("procedure")) { 1220 this.procedure = castToCodeableConcept(value); // CodeableConcept 1221 } else if (name.equals("additive")) { 1222 this.additive = castToReference(value); // Reference 1223 } else if (name.equals("time[x]")) { 1224 this.time = castToType(value); // Type 1225 } else 1226 return super.setProperty(name, value); 1227 return value; 1228 } 1229 1230 @Override 1231 public void removeChild(String name, Base value) throws FHIRException { 1232 if (name.equals("description")) { 1233 this.description = null; 1234 } else if (name.equals("procedure")) { 1235 this.procedure = null; 1236 } else if (name.equals("additive")) { 1237 this.additive = null; 1238 } else if (name.equals("time[x]")) { 1239 this.time = null; 1240 } else 1241 super.removeChild(name, value); 1242 1243 } 1244 1245 @Override 1246 public Base makeProperty(int hash, String name) throws FHIRException { 1247 switch (hash) { 1248 case -1724546052: 1249 return getDescriptionElement(); 1250 case -1095204141: 1251 return getProcedure(); 1252 case -1226589236: 1253 return getAdditive(); 1254 case -1313930605: 1255 return getTime(); 1256 case 3560141: 1257 return getTime(); 1258 default: 1259 return super.makeProperty(hash, name); 1260 } 1261 1262 } 1263 1264 @Override 1265 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1266 switch (hash) { 1267 case -1724546052: 1268 /* description */ return new String[] { "string" }; 1269 case -1095204141: 1270 /* procedure */ return new String[] { "CodeableConcept" }; 1271 case -1226589236: 1272 /* additive */ return new String[] { "Reference" }; 1273 case 3560141: 1274 /* time */ return new String[] { "dateTime", "Period" }; 1275 default: 1276 return super.getTypesForProperty(hash, name); 1277 } 1278 1279 } 1280 1281 @Override 1282 public Base addChild(String name) throws FHIRException { 1283 if (name.equals("description")) { 1284 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.description"); 1285 } else if (name.equals("procedure")) { 1286 this.procedure = new CodeableConcept(); 1287 return this.procedure; 1288 } else if (name.equals("additive")) { 1289 this.additive = new Reference(); 1290 return this.additive; 1291 } else if (name.equals("timeDateTime")) { 1292 this.time = new DateTimeType(); 1293 return this.time; 1294 } else if (name.equals("timePeriod")) { 1295 this.time = new Period(); 1296 return this.time; 1297 } else 1298 return super.addChild(name); 1299 } 1300 1301 public BiologicallyDerivedProductProcessingComponent copy() { 1302 BiologicallyDerivedProductProcessingComponent dst = new BiologicallyDerivedProductProcessingComponent(); 1303 copyValues(dst); 1304 return dst; 1305 } 1306 1307 public void copyValues(BiologicallyDerivedProductProcessingComponent dst) { 1308 super.copyValues(dst); 1309 dst.description = description == null ? null : description.copy(); 1310 dst.procedure = procedure == null ? null : procedure.copy(); 1311 dst.additive = additive == null ? null : additive.copy(); 1312 dst.time = time == null ? null : time.copy(); 1313 } 1314 1315 @Override 1316 public boolean equalsDeep(Base other_) { 1317 if (!super.equalsDeep(other_)) 1318 return false; 1319 if (!(other_ instanceof BiologicallyDerivedProductProcessingComponent)) 1320 return false; 1321 BiologicallyDerivedProductProcessingComponent o = (BiologicallyDerivedProductProcessingComponent) other_; 1322 return compareDeep(description, o.description, true) && compareDeep(procedure, o.procedure, true) 1323 && compareDeep(additive, o.additive, true) && compareDeep(time, o.time, true); 1324 } 1325 1326 @Override 1327 public boolean equalsShallow(Base other_) { 1328 if (!super.equalsShallow(other_)) 1329 return false; 1330 if (!(other_ instanceof BiologicallyDerivedProductProcessingComponent)) 1331 return false; 1332 BiologicallyDerivedProductProcessingComponent o = (BiologicallyDerivedProductProcessingComponent) other_; 1333 return compareValues(description, o.description, true); 1334 } 1335 1336 public boolean isEmpty() { 1337 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, procedure, additive, time); 1338 } 1339 1340 public String fhirType() { 1341 return "BiologicallyDerivedProduct.processing"; 1342 1343 } 1344 1345 } 1346 1347 @Block() 1348 public static class BiologicallyDerivedProductManipulationComponent extends BackboneElement 1349 implements IBaseBackboneElement { 1350 /** 1351 * Description of manipulation. 1352 */ 1353 @Child(name = "description", type = { 1354 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1355 @Description(shortDefinition = "Description of manipulation", formalDefinition = "Description of manipulation.") 1356 protected StringType description; 1357 1358 /** 1359 * Time of manipulation. 1360 */ 1361 @Child(name = "time", type = { DateTimeType.class, 1362 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1363 @Description(shortDefinition = "Time of manipulation", formalDefinition = "Time of manipulation.") 1364 protected Type time; 1365 1366 private static final long serialVersionUID = 717201078L; 1367 1368 /** 1369 * Constructor 1370 */ 1371 public BiologicallyDerivedProductManipulationComponent() { 1372 super(); 1373 } 1374 1375 /** 1376 * @return {@link #description} (Description of manipulation.). This is the 1377 * underlying object with id, value and extensions. The accessor 1378 * "getDescription" gives direct access to the value 1379 */ 1380 public StringType getDescriptionElement() { 1381 if (this.description == null) 1382 if (Configuration.errorOnAutoCreate()) 1383 throw new Error("Attempt to auto-create BiologicallyDerivedProductManipulationComponent.description"); 1384 else if (Configuration.doAutoCreate()) 1385 this.description = new StringType(); // bb 1386 return this.description; 1387 } 1388 1389 public boolean hasDescriptionElement() { 1390 return this.description != null && !this.description.isEmpty(); 1391 } 1392 1393 public boolean hasDescription() { 1394 return this.description != null && !this.description.isEmpty(); 1395 } 1396 1397 /** 1398 * @param value {@link #description} (Description of manipulation.). This is the 1399 * underlying object with id, value and extensions. The accessor 1400 * "getDescription" gives direct access to the value 1401 */ 1402 public BiologicallyDerivedProductManipulationComponent setDescriptionElement(StringType value) { 1403 this.description = value; 1404 return this; 1405 } 1406 1407 /** 1408 * @return Description of manipulation. 1409 */ 1410 public String getDescription() { 1411 return this.description == null ? null : this.description.getValue(); 1412 } 1413 1414 /** 1415 * @param value Description of manipulation. 1416 */ 1417 public BiologicallyDerivedProductManipulationComponent setDescription(String value) { 1418 if (Utilities.noString(value)) 1419 this.description = null; 1420 else { 1421 if (this.description == null) 1422 this.description = new StringType(); 1423 this.description.setValue(value); 1424 } 1425 return this; 1426 } 1427 1428 /** 1429 * @return {@link #time} (Time of manipulation.) 1430 */ 1431 public Type getTime() { 1432 return this.time; 1433 } 1434 1435 /** 1436 * @return {@link #time} (Time of manipulation.) 1437 */ 1438 public DateTimeType getTimeDateTimeType() throws FHIRException { 1439 if (this.time == null) 1440 this.time = new DateTimeType(); 1441 if (!(this.time instanceof DateTimeType)) 1442 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1443 + this.time.getClass().getName() + " was encountered"); 1444 return (DateTimeType) this.time; 1445 } 1446 1447 public boolean hasTimeDateTimeType() { 1448 return this.time instanceof DateTimeType; 1449 } 1450 1451 /** 1452 * @return {@link #time} (Time of manipulation.) 1453 */ 1454 public Period getTimePeriod() throws FHIRException { 1455 if (this.time == null) 1456 this.time = new Period(); 1457 if (!(this.time instanceof Period)) 1458 throw new FHIRException( 1459 "Type mismatch: the type Period was expected, but " + this.time.getClass().getName() + " was encountered"); 1460 return (Period) this.time; 1461 } 1462 1463 public boolean hasTimePeriod() { 1464 return this.time instanceof Period; 1465 } 1466 1467 public boolean hasTime() { 1468 return this.time != null && !this.time.isEmpty(); 1469 } 1470 1471 /** 1472 * @param value {@link #time} (Time of manipulation.) 1473 */ 1474 public BiologicallyDerivedProductManipulationComponent setTime(Type value) { 1475 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1476 throw new Error("Not the right type for BiologicallyDerivedProduct.manipulation.time[x]: " + value.fhirType()); 1477 this.time = value; 1478 return this; 1479 } 1480 1481 protected void listChildren(List<Property> children) { 1482 super.listChildren(children); 1483 children.add(new Property("description", "string", "Description of manipulation.", 0, 1, description)); 1484 children.add(new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time)); 1485 } 1486 1487 @Override 1488 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1489 switch (_hash) { 1490 case -1724546052: 1491 /* description */ return new Property("description", "string", "Description of manipulation.", 0, 1, 1492 description); 1493 case -1313930605: 1494 /* time[x] */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1495 case 3560141: 1496 /* time */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1497 case 2135345544: 1498 /* timeDateTime */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1499 case 693544686: 1500 /* timePeriod */ return new Property("time[x]", "dateTime|Period", "Time of manipulation.", 0, 1, time); 1501 default: 1502 return super.getNamedProperty(_hash, _name, _checkValid); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1509 switch (hash) { 1510 case -1724546052: 1511 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1512 case 3560141: 1513 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // Type 1514 default: 1515 return super.getProperty(hash, name, checkValid); 1516 } 1517 1518 } 1519 1520 @Override 1521 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1522 switch (hash) { 1523 case -1724546052: // description 1524 this.description = castToString(value); // StringType 1525 return value; 1526 case 3560141: // time 1527 this.time = castToType(value); // Type 1528 return value; 1529 default: 1530 return super.setProperty(hash, name, value); 1531 } 1532 1533 } 1534 1535 @Override 1536 public Base setProperty(String name, Base value) throws FHIRException { 1537 if (name.equals("description")) { 1538 this.description = castToString(value); // StringType 1539 } else if (name.equals("time[x]")) { 1540 this.time = castToType(value); // Type 1541 } else 1542 return super.setProperty(name, value); 1543 return value; 1544 } 1545 1546 @Override 1547 public void removeChild(String name, Base value) throws FHIRException { 1548 if (name.equals("description")) { 1549 this.description = null; 1550 } else if (name.equals("time[x]")) { 1551 this.time = null; 1552 } else 1553 super.removeChild(name, value); 1554 1555 } 1556 1557 @Override 1558 public Base makeProperty(int hash, String name) throws FHIRException { 1559 switch (hash) { 1560 case -1724546052: 1561 return getDescriptionElement(); 1562 case -1313930605: 1563 return getTime(); 1564 case 3560141: 1565 return getTime(); 1566 default: 1567 return super.makeProperty(hash, name); 1568 } 1569 1570 } 1571 1572 @Override 1573 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1574 switch (hash) { 1575 case -1724546052: 1576 /* description */ return new String[] { "string" }; 1577 case 3560141: 1578 /* time */ return new String[] { "dateTime", "Period" }; 1579 default: 1580 return super.getTypesForProperty(hash, name); 1581 } 1582 1583 } 1584 1585 @Override 1586 public Base addChild(String name) throws FHIRException { 1587 if (name.equals("description")) { 1588 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.description"); 1589 } else if (name.equals("timeDateTime")) { 1590 this.time = new DateTimeType(); 1591 return this.time; 1592 } else if (name.equals("timePeriod")) { 1593 this.time = new Period(); 1594 return this.time; 1595 } else 1596 return super.addChild(name); 1597 } 1598 1599 public BiologicallyDerivedProductManipulationComponent copy() { 1600 BiologicallyDerivedProductManipulationComponent dst = new BiologicallyDerivedProductManipulationComponent(); 1601 copyValues(dst); 1602 return dst; 1603 } 1604 1605 public void copyValues(BiologicallyDerivedProductManipulationComponent dst) { 1606 super.copyValues(dst); 1607 dst.description = description == null ? null : description.copy(); 1608 dst.time = time == null ? null : time.copy(); 1609 } 1610 1611 @Override 1612 public boolean equalsDeep(Base other_) { 1613 if (!super.equalsDeep(other_)) 1614 return false; 1615 if (!(other_ instanceof BiologicallyDerivedProductManipulationComponent)) 1616 return false; 1617 BiologicallyDerivedProductManipulationComponent o = (BiologicallyDerivedProductManipulationComponent) other_; 1618 return compareDeep(description, o.description, true) && compareDeep(time, o.time, true); 1619 } 1620 1621 @Override 1622 public boolean equalsShallow(Base other_) { 1623 if (!super.equalsShallow(other_)) 1624 return false; 1625 if (!(other_ instanceof BiologicallyDerivedProductManipulationComponent)) 1626 return false; 1627 BiologicallyDerivedProductManipulationComponent o = (BiologicallyDerivedProductManipulationComponent) other_; 1628 return compareValues(description, o.description, true); 1629 } 1630 1631 public boolean isEmpty() { 1632 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, time); 1633 } 1634 1635 public String fhirType() { 1636 return "BiologicallyDerivedProduct.manipulation"; 1637 1638 } 1639 1640 } 1641 1642 @Block() 1643 public static class BiologicallyDerivedProductStorageComponent extends BackboneElement 1644 implements IBaseBackboneElement { 1645 /** 1646 * Description of storage. 1647 */ 1648 @Child(name = "description", type = { 1649 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1650 @Description(shortDefinition = "Description of storage", formalDefinition = "Description of storage.") 1651 protected StringType description; 1652 1653 /** 1654 * Storage temperature. 1655 */ 1656 @Child(name = "temperature", type = { 1657 DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1658 @Description(shortDefinition = "Storage temperature", formalDefinition = "Storage temperature.") 1659 protected DecimalType temperature; 1660 1661 /** 1662 * Temperature scale used. 1663 */ 1664 @Child(name = "scale", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1665 @Description(shortDefinition = "farenheit | celsius | kelvin", formalDefinition = "Temperature scale used.") 1666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/product-storage-scale") 1667 protected Enumeration<BiologicallyDerivedProductStorageScale> scale; 1668 1669 /** 1670 * Storage timeperiod. 1671 */ 1672 @Child(name = "duration", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1673 @Description(shortDefinition = "Storage timeperiod", formalDefinition = "Storage timeperiod.") 1674 protected Period duration; 1675 1676 private static final long serialVersionUID = 1509141319L; 1677 1678 /** 1679 * Constructor 1680 */ 1681 public BiologicallyDerivedProductStorageComponent() { 1682 super(); 1683 } 1684 1685 /** 1686 * @return {@link #description} (Description of storage.). This is the 1687 * underlying object with id, value and extensions. The accessor 1688 * "getDescription" gives direct access to the value 1689 */ 1690 public StringType getDescriptionElement() { 1691 if (this.description == null) 1692 if (Configuration.errorOnAutoCreate()) 1693 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.description"); 1694 else if (Configuration.doAutoCreate()) 1695 this.description = new StringType(); // bb 1696 return this.description; 1697 } 1698 1699 public boolean hasDescriptionElement() { 1700 return this.description != null && !this.description.isEmpty(); 1701 } 1702 1703 public boolean hasDescription() { 1704 return this.description != null && !this.description.isEmpty(); 1705 } 1706 1707 /** 1708 * @param value {@link #description} (Description of storage.). This is the 1709 * underlying object with id, value and extensions. The accessor 1710 * "getDescription" gives direct access to the value 1711 */ 1712 public BiologicallyDerivedProductStorageComponent setDescriptionElement(StringType value) { 1713 this.description = value; 1714 return this; 1715 } 1716 1717 /** 1718 * @return Description of storage. 1719 */ 1720 public String getDescription() { 1721 return this.description == null ? null : this.description.getValue(); 1722 } 1723 1724 /** 1725 * @param value Description of storage. 1726 */ 1727 public BiologicallyDerivedProductStorageComponent setDescription(String value) { 1728 if (Utilities.noString(value)) 1729 this.description = null; 1730 else { 1731 if (this.description == null) 1732 this.description = new StringType(); 1733 this.description.setValue(value); 1734 } 1735 return this; 1736 } 1737 1738 /** 1739 * @return {@link #temperature} (Storage temperature.). This is the underlying 1740 * object with id, value and extensions. The accessor "getTemperature" 1741 * gives direct access to the value 1742 */ 1743 public DecimalType getTemperatureElement() { 1744 if (this.temperature == null) 1745 if (Configuration.errorOnAutoCreate()) 1746 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.temperature"); 1747 else if (Configuration.doAutoCreate()) 1748 this.temperature = new DecimalType(); // bb 1749 return this.temperature; 1750 } 1751 1752 public boolean hasTemperatureElement() { 1753 return this.temperature != null && !this.temperature.isEmpty(); 1754 } 1755 1756 public boolean hasTemperature() { 1757 return this.temperature != null && !this.temperature.isEmpty(); 1758 } 1759 1760 /** 1761 * @param value {@link #temperature} (Storage temperature.). This is the 1762 * underlying object with id, value and extensions. The accessor 1763 * "getTemperature" gives direct access to the value 1764 */ 1765 public BiologicallyDerivedProductStorageComponent setTemperatureElement(DecimalType value) { 1766 this.temperature = value; 1767 return this; 1768 } 1769 1770 /** 1771 * @return Storage temperature. 1772 */ 1773 public BigDecimal getTemperature() { 1774 return this.temperature == null ? null : this.temperature.getValue(); 1775 } 1776 1777 /** 1778 * @param value Storage temperature. 1779 */ 1780 public BiologicallyDerivedProductStorageComponent setTemperature(BigDecimal value) { 1781 if (value == null) 1782 this.temperature = null; 1783 else { 1784 if (this.temperature == null) 1785 this.temperature = new DecimalType(); 1786 this.temperature.setValue(value); 1787 } 1788 return this; 1789 } 1790 1791 /** 1792 * @param value Storage temperature. 1793 */ 1794 public BiologicallyDerivedProductStorageComponent setTemperature(long value) { 1795 this.temperature = new DecimalType(); 1796 this.temperature.setValue(value); 1797 return this; 1798 } 1799 1800 /** 1801 * @param value Storage temperature. 1802 */ 1803 public BiologicallyDerivedProductStorageComponent setTemperature(double value) { 1804 this.temperature = new DecimalType(); 1805 this.temperature.setValue(value); 1806 return this; 1807 } 1808 1809 /** 1810 * @return {@link #scale} (Temperature scale used.). This is the underlying 1811 * object with id, value and extensions. The accessor "getScale" gives 1812 * direct access to the value 1813 */ 1814 public Enumeration<BiologicallyDerivedProductStorageScale> getScaleElement() { 1815 if (this.scale == null) 1816 if (Configuration.errorOnAutoCreate()) 1817 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.scale"); 1818 else if (Configuration.doAutoCreate()) 1819 this.scale = new Enumeration<BiologicallyDerivedProductStorageScale>( 1820 new BiologicallyDerivedProductStorageScaleEnumFactory()); // bb 1821 return this.scale; 1822 } 1823 1824 public boolean hasScaleElement() { 1825 return this.scale != null && !this.scale.isEmpty(); 1826 } 1827 1828 public boolean hasScale() { 1829 return this.scale != null && !this.scale.isEmpty(); 1830 } 1831 1832 /** 1833 * @param value {@link #scale} (Temperature scale used.). This is the underlying 1834 * object with id, value and extensions. The accessor "getScale" 1835 * gives direct access to the value 1836 */ 1837 public BiologicallyDerivedProductStorageComponent setScaleElement( 1838 Enumeration<BiologicallyDerivedProductStorageScale> value) { 1839 this.scale = value; 1840 return this; 1841 } 1842 1843 /** 1844 * @return Temperature scale used. 1845 */ 1846 public BiologicallyDerivedProductStorageScale getScale() { 1847 return this.scale == null ? null : this.scale.getValue(); 1848 } 1849 1850 /** 1851 * @param value Temperature scale used. 1852 */ 1853 public BiologicallyDerivedProductStorageComponent setScale(BiologicallyDerivedProductStorageScale value) { 1854 if (value == null) 1855 this.scale = null; 1856 else { 1857 if (this.scale == null) 1858 this.scale = new Enumeration<BiologicallyDerivedProductStorageScale>( 1859 new BiologicallyDerivedProductStorageScaleEnumFactory()); 1860 this.scale.setValue(value); 1861 } 1862 return this; 1863 } 1864 1865 /** 1866 * @return {@link #duration} (Storage timeperiod.) 1867 */ 1868 public Period getDuration() { 1869 if (this.duration == null) 1870 if (Configuration.errorOnAutoCreate()) 1871 throw new Error("Attempt to auto-create BiologicallyDerivedProductStorageComponent.duration"); 1872 else if (Configuration.doAutoCreate()) 1873 this.duration = new Period(); // cc 1874 return this.duration; 1875 } 1876 1877 public boolean hasDuration() { 1878 return this.duration != null && !this.duration.isEmpty(); 1879 } 1880 1881 /** 1882 * @param value {@link #duration} (Storage timeperiod.) 1883 */ 1884 public BiologicallyDerivedProductStorageComponent setDuration(Period value) { 1885 this.duration = value; 1886 return this; 1887 } 1888 1889 protected void listChildren(List<Property> children) { 1890 super.listChildren(children); 1891 children.add(new Property("description", "string", "Description of storage.", 0, 1, description)); 1892 children.add(new Property("temperature", "decimal", "Storage temperature.", 0, 1, temperature)); 1893 children.add(new Property("scale", "code", "Temperature scale used.", 0, 1, scale)); 1894 children.add(new Property("duration", "Period", "Storage timeperiod.", 0, 1, duration)); 1895 } 1896 1897 @Override 1898 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1899 switch (_hash) { 1900 case -1724546052: 1901 /* description */ return new Property("description", "string", "Description of storage.", 0, 1, description); 1902 case 321701236: 1903 /* temperature */ return new Property("temperature", "decimal", "Storage temperature.", 0, 1, temperature); 1904 case 109250890: 1905 /* scale */ return new Property("scale", "code", "Temperature scale used.", 0, 1, scale); 1906 case -1992012396: 1907 /* duration */ return new Property("duration", "Period", "Storage timeperiod.", 0, 1, duration); 1908 default: 1909 return super.getNamedProperty(_hash, _name, _checkValid); 1910 } 1911 1912 } 1913 1914 @Override 1915 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1916 switch (hash) { 1917 case -1724546052: 1918 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1919 case 321701236: 1920 /* temperature */ return this.temperature == null ? new Base[0] : new Base[] { this.temperature }; // DecimalType 1921 case 109250890: 1922 /* scale */ return this.scale == null ? new Base[0] : new Base[] { this.scale }; // Enumeration<BiologicallyDerivedProductStorageScale> 1923 case -1992012396: 1924 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Period 1925 default: 1926 return super.getProperty(hash, name, checkValid); 1927 } 1928 1929 } 1930 1931 @Override 1932 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1933 switch (hash) { 1934 case -1724546052: // description 1935 this.description = castToString(value); // StringType 1936 return value; 1937 case 321701236: // temperature 1938 this.temperature = castToDecimal(value); // DecimalType 1939 return value; 1940 case 109250890: // scale 1941 value = new BiologicallyDerivedProductStorageScaleEnumFactory().fromType(castToCode(value)); 1942 this.scale = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStorageScale> 1943 return value; 1944 case -1992012396: // duration 1945 this.duration = castToPeriod(value); // Period 1946 return value; 1947 default: 1948 return super.setProperty(hash, name, value); 1949 } 1950 1951 } 1952 1953 @Override 1954 public Base setProperty(String name, Base value) throws FHIRException { 1955 if (name.equals("description")) { 1956 this.description = castToString(value); // StringType 1957 } else if (name.equals("temperature")) { 1958 this.temperature = castToDecimal(value); // DecimalType 1959 } else if (name.equals("scale")) { 1960 value = new BiologicallyDerivedProductStorageScaleEnumFactory().fromType(castToCode(value)); 1961 this.scale = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStorageScale> 1962 } else if (name.equals("duration")) { 1963 this.duration = castToPeriod(value); // Period 1964 } else 1965 return super.setProperty(name, value); 1966 return value; 1967 } 1968 1969 @Override 1970 public void removeChild(String name, Base value) throws FHIRException { 1971 if (name.equals("description")) { 1972 this.description = null; 1973 } else if (name.equals("temperature")) { 1974 this.temperature = null; 1975 } else if (name.equals("scale")) { 1976 this.scale = null; 1977 } else if (name.equals("duration")) { 1978 this.duration = null; 1979 } else 1980 super.removeChild(name, value); 1981 1982 } 1983 1984 @Override 1985 public Base makeProperty(int hash, String name) throws FHIRException { 1986 switch (hash) { 1987 case -1724546052: 1988 return getDescriptionElement(); 1989 case 321701236: 1990 return getTemperatureElement(); 1991 case 109250890: 1992 return getScaleElement(); 1993 case -1992012396: 1994 return getDuration(); 1995 default: 1996 return super.makeProperty(hash, name); 1997 } 1998 1999 } 2000 2001 @Override 2002 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2003 switch (hash) { 2004 case -1724546052: 2005 /* description */ return new String[] { "string" }; 2006 case 321701236: 2007 /* temperature */ return new String[] { "decimal" }; 2008 case 109250890: 2009 /* scale */ return new String[] { "code" }; 2010 case -1992012396: 2011 /* duration */ return new String[] { "Period" }; 2012 default: 2013 return super.getTypesForProperty(hash, name); 2014 } 2015 2016 } 2017 2018 @Override 2019 public Base addChild(String name) throws FHIRException { 2020 if (name.equals("description")) { 2021 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.description"); 2022 } else if (name.equals("temperature")) { 2023 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.temperature"); 2024 } else if (name.equals("scale")) { 2025 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.scale"); 2026 } else if (name.equals("duration")) { 2027 this.duration = new Period(); 2028 return this.duration; 2029 } else 2030 return super.addChild(name); 2031 } 2032 2033 public BiologicallyDerivedProductStorageComponent copy() { 2034 BiologicallyDerivedProductStorageComponent dst = new BiologicallyDerivedProductStorageComponent(); 2035 copyValues(dst); 2036 return dst; 2037 } 2038 2039 public void copyValues(BiologicallyDerivedProductStorageComponent dst) { 2040 super.copyValues(dst); 2041 dst.description = description == null ? null : description.copy(); 2042 dst.temperature = temperature == null ? null : temperature.copy(); 2043 dst.scale = scale == null ? null : scale.copy(); 2044 dst.duration = duration == null ? null : duration.copy(); 2045 } 2046 2047 @Override 2048 public boolean equalsDeep(Base other_) { 2049 if (!super.equalsDeep(other_)) 2050 return false; 2051 if (!(other_ instanceof BiologicallyDerivedProductStorageComponent)) 2052 return false; 2053 BiologicallyDerivedProductStorageComponent o = (BiologicallyDerivedProductStorageComponent) other_; 2054 return compareDeep(description, o.description, true) && compareDeep(temperature, o.temperature, true) 2055 && compareDeep(scale, o.scale, true) && compareDeep(duration, o.duration, true); 2056 } 2057 2058 @Override 2059 public boolean equalsShallow(Base other_) { 2060 if (!super.equalsShallow(other_)) 2061 return false; 2062 if (!(other_ instanceof BiologicallyDerivedProductStorageComponent)) 2063 return false; 2064 BiologicallyDerivedProductStorageComponent o = (BiologicallyDerivedProductStorageComponent) other_; 2065 return compareValues(description, o.description, true) && compareValues(temperature, o.temperature, true) 2066 && compareValues(scale, o.scale, true); 2067 } 2068 2069 public boolean isEmpty() { 2070 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, temperature, scale, duration); 2071 } 2072 2073 public String fhirType() { 2074 return "BiologicallyDerivedProduct.storage"; 2075 2076 } 2077 2078 } 2079 2080 /** 2081 * This records identifiers associated with this biologically derived product 2082 * instance that are defined by business processes and/or used to refer to it 2083 * when a direct URL reference to the resource itself is not appropriate (e.g. 2084 * in CDA documents, or in written / printed documentation). 2085 */ 2086 @Child(name = "identifier", type = { 2087 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2088 @Description(shortDefinition = "External ids for this item", formalDefinition = "This records identifiers associated with this biologically derived product instance that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 2089 protected List<Identifier> identifier; 2090 2091 /** 2092 * Broad category of this product. 2093 */ 2094 @Child(name = "productCategory", type = { 2095 CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2096 @Description(shortDefinition = "organ | tissue | fluid | cells | biologicalAgent", formalDefinition = "Broad category of this product.") 2097 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/product-category") 2098 protected Enumeration<BiologicallyDerivedProductCategory> productCategory; 2099 2100 /** 2101 * A code that identifies the kind of this biologically derived product (SNOMED 2102 * Ctcode). 2103 */ 2104 @Child(name = "productCode", type = { 2105 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2106 @Description(shortDefinition = "What this biologically derived product is", formalDefinition = "A code that identifies the kind of this biologically derived product (SNOMED Ctcode).") 2107 protected CodeableConcept productCode; 2108 2109 /** 2110 * Whether the product is currently available. 2111 */ 2112 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2113 @Description(shortDefinition = "available | unavailable", formalDefinition = "Whether the product is currently available.") 2114 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/product-status") 2115 protected Enumeration<BiologicallyDerivedProductStatus> status; 2116 2117 /** 2118 * Procedure request to obtain this biologically derived product. 2119 */ 2120 @Child(name = "request", type = { 2121 ServiceRequest.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2122 @Description(shortDefinition = "Procedure request", formalDefinition = "Procedure request to obtain this biologically derived product.") 2123 protected List<Reference> request; 2124 /** 2125 * The actual objects that are the target of the reference (Procedure request to 2126 * obtain this biologically derived product.) 2127 */ 2128 protected List<ServiceRequest> requestTarget; 2129 2130 /** 2131 * Number of discrete units within this product. 2132 */ 2133 @Child(name = "quantity", type = { 2134 IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2135 @Description(shortDefinition = "The amount of this biologically derived product", formalDefinition = "Number of discrete units within this product.") 2136 protected IntegerType quantity; 2137 2138 /** 2139 * Parent product (if any). 2140 */ 2141 @Child(name = "parent", type = { 2142 BiologicallyDerivedProduct.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2143 @Description(shortDefinition = "BiologicallyDerivedProduct parent", formalDefinition = "Parent product (if any).") 2144 protected List<Reference> parent; 2145 /** 2146 * The actual objects that are the target of the reference (Parent product (if 2147 * any).) 2148 */ 2149 protected List<BiologicallyDerivedProduct> parentTarget; 2150 2151 /** 2152 * How this product was collected. 2153 */ 2154 @Child(name = "collection", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 2155 @Description(shortDefinition = "How this product was collected", formalDefinition = "How this product was collected.") 2156 protected BiologicallyDerivedProductCollectionComponent collection; 2157 2158 /** 2159 * Any processing of the product during collection that does not change the 2160 * fundamental nature of the product. For example adding anti-coagulants during 2161 * the collection of Peripheral Blood Stem Cells. 2162 */ 2163 @Child(name = "processing", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2164 @Description(shortDefinition = "Any processing of the product during collection", formalDefinition = "Any processing of the product during collection that does not change the fundamental nature of the product. For example adding anti-coagulants during the collection of Peripheral Blood Stem Cells.") 2165 protected List<BiologicallyDerivedProductProcessingComponent> processing; 2166 2167 /** 2168 * Any manipulation of product post-collection that is intended to alter the 2169 * product. For example a buffy-coat enrichment or CD8 reduction of Peripheral 2170 * Blood Stem Cells to make it more suitable for infusion. 2171 */ 2172 @Child(name = "manipulation", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 2173 @Description(shortDefinition = "Any manipulation of product post-collection", formalDefinition = "Any manipulation of product post-collection that is intended to alter the product. For example a buffy-coat enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it more suitable for infusion.") 2174 protected BiologicallyDerivedProductManipulationComponent manipulation; 2175 2176 /** 2177 * Product storage. 2178 */ 2179 @Child(name = "storage", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2180 @Description(shortDefinition = "Product storage", formalDefinition = "Product storage.") 2181 protected List<BiologicallyDerivedProductStorageComponent> storage; 2182 2183 private static final long serialVersionUID = -1367034547L; 2184 2185 /** 2186 * Constructor 2187 */ 2188 public BiologicallyDerivedProduct() { 2189 super(); 2190 } 2191 2192 /** 2193 * @return {@link #identifier} (This records identifiers associated with this 2194 * biologically derived product instance that are defined by business 2195 * processes and/or used to refer to it when a direct URL reference to 2196 * the resource itself is not appropriate (e.g. in CDA documents, or in 2197 * written / printed documentation).) 2198 */ 2199 public List<Identifier> getIdentifier() { 2200 if (this.identifier == null) 2201 this.identifier = new ArrayList<Identifier>(); 2202 return this.identifier; 2203 } 2204 2205 /** 2206 * @return Returns a reference to <code>this</code> for easy method chaining 2207 */ 2208 public BiologicallyDerivedProduct setIdentifier(List<Identifier> theIdentifier) { 2209 this.identifier = theIdentifier; 2210 return this; 2211 } 2212 2213 public boolean hasIdentifier() { 2214 if (this.identifier == null) 2215 return false; 2216 for (Identifier item : this.identifier) 2217 if (!item.isEmpty()) 2218 return true; 2219 return false; 2220 } 2221 2222 public Identifier addIdentifier() { // 3 2223 Identifier t = new Identifier(); 2224 if (this.identifier == null) 2225 this.identifier = new ArrayList<Identifier>(); 2226 this.identifier.add(t); 2227 return t; 2228 } 2229 2230 public BiologicallyDerivedProduct addIdentifier(Identifier t) { // 3 2231 if (t == null) 2232 return this; 2233 if (this.identifier == null) 2234 this.identifier = new ArrayList<Identifier>(); 2235 this.identifier.add(t); 2236 return this; 2237 } 2238 2239 /** 2240 * @return The first repetition of repeating field {@link #identifier}, creating 2241 * it if it does not already exist 2242 */ 2243 public Identifier getIdentifierFirstRep() { 2244 if (getIdentifier().isEmpty()) { 2245 addIdentifier(); 2246 } 2247 return getIdentifier().get(0); 2248 } 2249 2250 /** 2251 * @return {@link #productCategory} (Broad category of this product.). This is 2252 * the underlying object with id, value and extensions. The accessor 2253 * "getProductCategory" gives direct access to the value 2254 */ 2255 public Enumeration<BiologicallyDerivedProductCategory> getProductCategoryElement() { 2256 if (this.productCategory == null) 2257 if (Configuration.errorOnAutoCreate()) 2258 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productCategory"); 2259 else if (Configuration.doAutoCreate()) 2260 this.productCategory = new Enumeration<BiologicallyDerivedProductCategory>( 2261 new BiologicallyDerivedProductCategoryEnumFactory()); // bb 2262 return this.productCategory; 2263 } 2264 2265 public boolean hasProductCategoryElement() { 2266 return this.productCategory != null && !this.productCategory.isEmpty(); 2267 } 2268 2269 public boolean hasProductCategory() { 2270 return this.productCategory != null && !this.productCategory.isEmpty(); 2271 } 2272 2273 /** 2274 * @param value {@link #productCategory} (Broad category of this product.). This 2275 * is the underlying object with id, value and extensions. The 2276 * accessor "getProductCategory" gives direct access to the value 2277 */ 2278 public BiologicallyDerivedProduct setProductCategoryElement(Enumeration<BiologicallyDerivedProductCategory> value) { 2279 this.productCategory = value; 2280 return this; 2281 } 2282 2283 /** 2284 * @return Broad category of this product. 2285 */ 2286 public BiologicallyDerivedProductCategory getProductCategory() { 2287 return this.productCategory == null ? null : this.productCategory.getValue(); 2288 } 2289 2290 /** 2291 * @param value Broad category of this product. 2292 */ 2293 public BiologicallyDerivedProduct setProductCategory(BiologicallyDerivedProductCategory value) { 2294 if (value == null) 2295 this.productCategory = null; 2296 else { 2297 if (this.productCategory == null) 2298 this.productCategory = new Enumeration<BiologicallyDerivedProductCategory>( 2299 new BiologicallyDerivedProductCategoryEnumFactory()); 2300 this.productCategory.setValue(value); 2301 } 2302 return this; 2303 } 2304 2305 /** 2306 * @return {@link #productCode} (A code that identifies the kind of this 2307 * biologically derived product (SNOMED Ctcode).) 2308 */ 2309 public CodeableConcept getProductCode() { 2310 if (this.productCode == null) 2311 if (Configuration.errorOnAutoCreate()) 2312 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productCode"); 2313 else if (Configuration.doAutoCreate()) 2314 this.productCode = new CodeableConcept(); // cc 2315 return this.productCode; 2316 } 2317 2318 public boolean hasProductCode() { 2319 return this.productCode != null && !this.productCode.isEmpty(); 2320 } 2321 2322 /** 2323 * @param value {@link #productCode} (A code that identifies the kind of this 2324 * biologically derived product (SNOMED Ctcode).) 2325 */ 2326 public BiologicallyDerivedProduct setProductCode(CodeableConcept value) { 2327 this.productCode = value; 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #status} (Whether the product is currently available.). This 2333 * is the underlying object with id, value and extensions. The accessor 2334 * "getStatus" gives direct access to the value 2335 */ 2336 public Enumeration<BiologicallyDerivedProductStatus> getStatusElement() { 2337 if (this.status == null) 2338 if (Configuration.errorOnAutoCreate()) 2339 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.status"); 2340 else if (Configuration.doAutoCreate()) 2341 this.status = new Enumeration<BiologicallyDerivedProductStatus>( 2342 new BiologicallyDerivedProductStatusEnumFactory()); // bb 2343 return this.status; 2344 } 2345 2346 public boolean hasStatusElement() { 2347 return this.status != null && !this.status.isEmpty(); 2348 } 2349 2350 public boolean hasStatus() { 2351 return this.status != null && !this.status.isEmpty(); 2352 } 2353 2354 /** 2355 * @param value {@link #status} (Whether the product is currently available.). 2356 * This is the underlying object with id, value and extensions. The 2357 * accessor "getStatus" gives direct access to the value 2358 */ 2359 public BiologicallyDerivedProduct setStatusElement(Enumeration<BiologicallyDerivedProductStatus> value) { 2360 this.status = value; 2361 return this; 2362 } 2363 2364 /** 2365 * @return Whether the product is currently available. 2366 */ 2367 public BiologicallyDerivedProductStatus getStatus() { 2368 return this.status == null ? null : this.status.getValue(); 2369 } 2370 2371 /** 2372 * @param value Whether the product is currently available. 2373 */ 2374 public BiologicallyDerivedProduct setStatus(BiologicallyDerivedProductStatus value) { 2375 if (value == null) 2376 this.status = null; 2377 else { 2378 if (this.status == null) 2379 this.status = new Enumeration<BiologicallyDerivedProductStatus>( 2380 new BiologicallyDerivedProductStatusEnumFactory()); 2381 this.status.setValue(value); 2382 } 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #request} (Procedure request to obtain this biologically 2388 * derived product.) 2389 */ 2390 public List<Reference> getRequest() { 2391 if (this.request == null) 2392 this.request = new ArrayList<Reference>(); 2393 return this.request; 2394 } 2395 2396 /** 2397 * @return Returns a reference to <code>this</code> for easy method chaining 2398 */ 2399 public BiologicallyDerivedProduct setRequest(List<Reference> theRequest) { 2400 this.request = theRequest; 2401 return this; 2402 } 2403 2404 public boolean hasRequest() { 2405 if (this.request == null) 2406 return false; 2407 for (Reference item : this.request) 2408 if (!item.isEmpty()) 2409 return true; 2410 return false; 2411 } 2412 2413 public Reference addRequest() { // 3 2414 Reference t = new Reference(); 2415 if (this.request == null) 2416 this.request = new ArrayList<Reference>(); 2417 this.request.add(t); 2418 return t; 2419 } 2420 2421 public BiologicallyDerivedProduct addRequest(Reference t) { // 3 2422 if (t == null) 2423 return this; 2424 if (this.request == null) 2425 this.request = new ArrayList<Reference>(); 2426 this.request.add(t); 2427 return this; 2428 } 2429 2430 /** 2431 * @return The first repetition of repeating field {@link #request}, creating it 2432 * if it does not already exist 2433 */ 2434 public Reference getRequestFirstRep() { 2435 if (getRequest().isEmpty()) { 2436 addRequest(); 2437 } 2438 return getRequest().get(0); 2439 } 2440 2441 /** 2442 * @return {@link #quantity} (Number of discrete units within this product.). 2443 * This is the underlying object with id, value and extensions. The 2444 * accessor "getQuantity" gives direct access to the value 2445 */ 2446 public IntegerType getQuantityElement() { 2447 if (this.quantity == null) 2448 if (Configuration.errorOnAutoCreate()) 2449 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.quantity"); 2450 else if (Configuration.doAutoCreate()) 2451 this.quantity = new IntegerType(); // bb 2452 return this.quantity; 2453 } 2454 2455 public boolean hasQuantityElement() { 2456 return this.quantity != null && !this.quantity.isEmpty(); 2457 } 2458 2459 public boolean hasQuantity() { 2460 return this.quantity != null && !this.quantity.isEmpty(); 2461 } 2462 2463 /** 2464 * @param value {@link #quantity} (Number of discrete units within this 2465 * product.). This is the underlying object with id, value and 2466 * extensions. The accessor "getQuantity" gives direct access to 2467 * the value 2468 */ 2469 public BiologicallyDerivedProduct setQuantityElement(IntegerType value) { 2470 this.quantity = value; 2471 return this; 2472 } 2473 2474 /** 2475 * @return Number of discrete units within this product. 2476 */ 2477 public int getQuantity() { 2478 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 2479 } 2480 2481 /** 2482 * @param value Number of discrete units within this product. 2483 */ 2484 public BiologicallyDerivedProduct setQuantity(int value) { 2485 if (this.quantity == null) 2486 this.quantity = new IntegerType(); 2487 this.quantity.setValue(value); 2488 return this; 2489 } 2490 2491 /** 2492 * @return {@link #parent} (Parent product (if any).) 2493 */ 2494 public List<Reference> getParent() { 2495 if (this.parent == null) 2496 this.parent = new ArrayList<Reference>(); 2497 return this.parent; 2498 } 2499 2500 /** 2501 * @return Returns a reference to <code>this</code> for easy method chaining 2502 */ 2503 public BiologicallyDerivedProduct setParent(List<Reference> theParent) { 2504 this.parent = theParent; 2505 return this; 2506 } 2507 2508 public boolean hasParent() { 2509 if (this.parent == null) 2510 return false; 2511 for (Reference item : this.parent) 2512 if (!item.isEmpty()) 2513 return true; 2514 return false; 2515 } 2516 2517 public Reference addParent() { // 3 2518 Reference t = new Reference(); 2519 if (this.parent == null) 2520 this.parent = new ArrayList<Reference>(); 2521 this.parent.add(t); 2522 return t; 2523 } 2524 2525 public BiologicallyDerivedProduct addParent(Reference t) { // 3 2526 if (t == null) 2527 return this; 2528 if (this.parent == null) 2529 this.parent = new ArrayList<Reference>(); 2530 this.parent.add(t); 2531 return this; 2532 } 2533 2534 /** 2535 * @return The first repetition of repeating field {@link #parent}, creating it 2536 * if it does not already exist 2537 */ 2538 public Reference getParentFirstRep() { 2539 if (getParent().isEmpty()) { 2540 addParent(); 2541 } 2542 return getParent().get(0); 2543 } 2544 2545 /** 2546 * @return {@link #collection} (How this product was collected.) 2547 */ 2548 public BiologicallyDerivedProductCollectionComponent getCollection() { 2549 if (this.collection == null) 2550 if (Configuration.errorOnAutoCreate()) 2551 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.collection"); 2552 else if (Configuration.doAutoCreate()) 2553 this.collection = new BiologicallyDerivedProductCollectionComponent(); // cc 2554 return this.collection; 2555 } 2556 2557 public boolean hasCollection() { 2558 return this.collection != null && !this.collection.isEmpty(); 2559 } 2560 2561 /** 2562 * @param value {@link #collection} (How this product was collected.) 2563 */ 2564 public BiologicallyDerivedProduct setCollection(BiologicallyDerivedProductCollectionComponent value) { 2565 this.collection = value; 2566 return this; 2567 } 2568 2569 /** 2570 * @return {@link #processing} (Any processing of the product during collection 2571 * that does not change the fundamental nature of the product. For 2572 * example adding anti-coagulants during the collection of Peripheral 2573 * Blood Stem Cells.) 2574 */ 2575 public List<BiologicallyDerivedProductProcessingComponent> getProcessing() { 2576 if (this.processing == null) 2577 this.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 2578 return this.processing; 2579 } 2580 2581 /** 2582 * @return Returns a reference to <code>this</code> for easy method chaining 2583 */ 2584 public BiologicallyDerivedProduct setProcessing(List<BiologicallyDerivedProductProcessingComponent> theProcessing) { 2585 this.processing = theProcessing; 2586 return this; 2587 } 2588 2589 public boolean hasProcessing() { 2590 if (this.processing == null) 2591 return false; 2592 for (BiologicallyDerivedProductProcessingComponent item : this.processing) 2593 if (!item.isEmpty()) 2594 return true; 2595 return false; 2596 } 2597 2598 public BiologicallyDerivedProductProcessingComponent addProcessing() { // 3 2599 BiologicallyDerivedProductProcessingComponent t = new BiologicallyDerivedProductProcessingComponent(); 2600 if (this.processing == null) 2601 this.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 2602 this.processing.add(t); 2603 return t; 2604 } 2605 2606 public BiologicallyDerivedProduct addProcessing(BiologicallyDerivedProductProcessingComponent t) { // 3 2607 if (t == null) 2608 return this; 2609 if (this.processing == null) 2610 this.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 2611 this.processing.add(t); 2612 return this; 2613 } 2614 2615 /** 2616 * @return The first repetition of repeating field {@link #processing}, creating 2617 * it if it does not already exist 2618 */ 2619 public BiologicallyDerivedProductProcessingComponent getProcessingFirstRep() { 2620 if (getProcessing().isEmpty()) { 2621 addProcessing(); 2622 } 2623 return getProcessing().get(0); 2624 } 2625 2626 /** 2627 * @return {@link #manipulation} (Any manipulation of product post-collection 2628 * that is intended to alter the product. For example a buffy-coat 2629 * enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it 2630 * more suitable for infusion.) 2631 */ 2632 public BiologicallyDerivedProductManipulationComponent getManipulation() { 2633 if (this.manipulation == null) 2634 if (Configuration.errorOnAutoCreate()) 2635 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.manipulation"); 2636 else if (Configuration.doAutoCreate()) 2637 this.manipulation = new BiologicallyDerivedProductManipulationComponent(); // cc 2638 return this.manipulation; 2639 } 2640 2641 public boolean hasManipulation() { 2642 return this.manipulation != null && !this.manipulation.isEmpty(); 2643 } 2644 2645 /** 2646 * @param value {@link #manipulation} (Any manipulation of product 2647 * post-collection that is intended to alter the product. For 2648 * example a buffy-coat enrichment or CD8 reduction of Peripheral 2649 * Blood Stem Cells to make it more suitable for infusion.) 2650 */ 2651 public BiologicallyDerivedProduct setManipulation(BiologicallyDerivedProductManipulationComponent value) { 2652 this.manipulation = value; 2653 return this; 2654 } 2655 2656 /** 2657 * @return {@link #storage} (Product storage.) 2658 */ 2659 public List<BiologicallyDerivedProductStorageComponent> getStorage() { 2660 if (this.storage == null) 2661 this.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 2662 return this.storage; 2663 } 2664 2665 /** 2666 * @return Returns a reference to <code>this</code> for easy method chaining 2667 */ 2668 public BiologicallyDerivedProduct setStorage(List<BiologicallyDerivedProductStorageComponent> theStorage) { 2669 this.storage = theStorage; 2670 return this; 2671 } 2672 2673 public boolean hasStorage() { 2674 if (this.storage == null) 2675 return false; 2676 for (BiologicallyDerivedProductStorageComponent item : this.storage) 2677 if (!item.isEmpty()) 2678 return true; 2679 return false; 2680 } 2681 2682 public BiologicallyDerivedProductStorageComponent addStorage() { // 3 2683 BiologicallyDerivedProductStorageComponent t = new BiologicallyDerivedProductStorageComponent(); 2684 if (this.storage == null) 2685 this.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 2686 this.storage.add(t); 2687 return t; 2688 } 2689 2690 public BiologicallyDerivedProduct addStorage(BiologicallyDerivedProductStorageComponent t) { // 3 2691 if (t == null) 2692 return this; 2693 if (this.storage == null) 2694 this.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 2695 this.storage.add(t); 2696 return this; 2697 } 2698 2699 /** 2700 * @return The first repetition of repeating field {@link #storage}, creating it 2701 * if it does not already exist 2702 */ 2703 public BiologicallyDerivedProductStorageComponent getStorageFirstRep() { 2704 if (getStorage().isEmpty()) { 2705 addStorage(); 2706 } 2707 return getStorage().get(0); 2708 } 2709 2710 protected void listChildren(List<Property> children) { 2711 super.listChildren(children); 2712 children.add(new Property("identifier", "Identifier", 2713 "This records identifiers associated with this biologically derived product instance that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2714 0, java.lang.Integer.MAX_VALUE, identifier)); 2715 children.add(new Property("productCategory", "code", "Broad category of this product.", 0, 1, productCategory)); 2716 children.add(new Property("productCode", "CodeableConcept", 2717 "A code that identifies the kind of this biologically derived product (SNOMED Ctcode).", 0, 1, productCode)); 2718 children.add(new Property("status", "code", "Whether the product is currently available.", 0, 1, status)); 2719 children.add(new Property("request", "Reference(ServiceRequest)", 2720 "Procedure request to obtain this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, request)); 2721 children.add(new Property("quantity", "integer", "Number of discrete units within this product.", 0, 1, quantity)); 2722 children.add(new Property("parent", "Reference(BiologicallyDerivedProduct)", "Parent product (if any).", 0, 2723 java.lang.Integer.MAX_VALUE, parent)); 2724 children.add(new Property("collection", "", "How this product was collected.", 0, 1, collection)); 2725 children.add(new Property("processing", "", 2726 "Any processing of the product during collection that does not change the fundamental nature of the product. For example adding anti-coagulants during the collection of Peripheral Blood Stem Cells.", 2727 0, java.lang.Integer.MAX_VALUE, processing)); 2728 children.add(new Property("manipulation", "", 2729 "Any manipulation of product post-collection that is intended to alter the product. For example a buffy-coat enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it more suitable for infusion.", 2730 0, 1, manipulation)); 2731 children.add(new Property("storage", "", "Product storage.", 0, java.lang.Integer.MAX_VALUE, storage)); 2732 } 2733 2734 @Override 2735 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2736 switch (_hash) { 2737 case -1618432855: 2738 /* identifier */ return new Property("identifier", "Identifier", 2739 "This records identifiers associated with this biologically derived product instance that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2740 0, java.lang.Integer.MAX_VALUE, identifier); 2741 case 197299981: 2742 /* productCategory */ return new Property("productCategory", "code", "Broad category of this product.", 0, 1, 2743 productCategory); 2744 case -1492131972: 2745 /* productCode */ return new Property("productCode", "CodeableConcept", 2746 "A code that identifies the kind of this biologically derived product (SNOMED Ctcode).", 0, 1, productCode); 2747 case -892481550: 2748 /* status */ return new Property("status", "code", "Whether the product is currently available.", 0, 1, status); 2749 case 1095692943: 2750 /* request */ return new Property("request", "Reference(ServiceRequest)", 2751 "Procedure request to obtain this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, request); 2752 case -1285004149: 2753 /* quantity */ return new Property("quantity", "integer", "Number of discrete units within this product.", 0, 1, 2754 quantity); 2755 case -995424086: 2756 /* parent */ return new Property("parent", "Reference(BiologicallyDerivedProduct)", "Parent product (if any).", 0, 2757 java.lang.Integer.MAX_VALUE, parent); 2758 case -1741312354: 2759 /* collection */ return new Property("collection", "", "How this product was collected.", 0, 1, collection); 2760 case 422194963: 2761 /* processing */ return new Property("processing", "", 2762 "Any processing of the product during collection that does not change the fundamental nature of the product. For example adding anti-coagulants during the collection of Peripheral Blood Stem Cells.", 2763 0, java.lang.Integer.MAX_VALUE, processing); 2764 case -696214627: 2765 /* manipulation */ return new Property("manipulation", "", 2766 "Any manipulation of product post-collection that is intended to alter the product. For example a buffy-coat enrichment or CD8 reduction of Peripheral Blood Stem Cells to make it more suitable for infusion.", 2767 0, 1, manipulation); 2768 case -1884274053: 2769 /* storage */ return new Property("storage", "", "Product storage.", 0, java.lang.Integer.MAX_VALUE, storage); 2770 default: 2771 return super.getNamedProperty(_hash, _name, _checkValid); 2772 } 2773 2774 } 2775 2776 @Override 2777 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2778 switch (hash) { 2779 case -1618432855: 2780 /* identifier */ return this.identifier == null ? new Base[0] 2781 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2782 case 197299981: 2783 /* productCategory */ return this.productCategory == null ? new Base[0] : new Base[] { this.productCategory }; // Enumeration<BiologicallyDerivedProductCategory> 2784 case -1492131972: 2785 /* productCode */ return this.productCode == null ? new Base[0] : new Base[] { this.productCode }; // CodeableConcept 2786 case -892481550: 2787 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<BiologicallyDerivedProductStatus> 2788 case 1095692943: 2789 /* request */ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 2790 case -1285004149: 2791 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // IntegerType 2792 case -995424086: 2793 /* parent */ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2794 case -1741312354: 2795 /* collection */ return this.collection == null ? new Base[0] : new Base[] { this.collection }; // BiologicallyDerivedProductCollectionComponent 2796 case 422194963: 2797 /* processing */ return this.processing == null ? new Base[0] 2798 : this.processing.toArray(new Base[this.processing.size()]); // BiologicallyDerivedProductProcessingComponent 2799 case -696214627: 2800 /* manipulation */ return this.manipulation == null ? new Base[0] : new Base[] { this.manipulation }; // BiologicallyDerivedProductManipulationComponent 2801 case -1884274053: 2802 /* storage */ return this.storage == null ? new Base[0] : this.storage.toArray(new Base[this.storage.size()]); // BiologicallyDerivedProductStorageComponent 2803 default: 2804 return super.getProperty(hash, name, checkValid); 2805 } 2806 2807 } 2808 2809 @Override 2810 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2811 switch (hash) { 2812 case -1618432855: // identifier 2813 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2814 return value; 2815 case 197299981: // productCategory 2816 value = new BiologicallyDerivedProductCategoryEnumFactory().fromType(castToCode(value)); 2817 this.productCategory = (Enumeration) value; // Enumeration<BiologicallyDerivedProductCategory> 2818 return value; 2819 case -1492131972: // productCode 2820 this.productCode = castToCodeableConcept(value); // CodeableConcept 2821 return value; 2822 case -892481550: // status 2823 value = new BiologicallyDerivedProductStatusEnumFactory().fromType(castToCode(value)); 2824 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStatus> 2825 return value; 2826 case 1095692943: // request 2827 this.getRequest().add(castToReference(value)); // Reference 2828 return value; 2829 case -1285004149: // quantity 2830 this.quantity = castToInteger(value); // IntegerType 2831 return value; 2832 case -995424086: // parent 2833 this.getParent().add(castToReference(value)); // Reference 2834 return value; 2835 case -1741312354: // collection 2836 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 2837 return value; 2838 case 422194963: // processing 2839 this.getProcessing().add((BiologicallyDerivedProductProcessingComponent) value); // BiologicallyDerivedProductProcessingComponent 2840 return value; 2841 case -696214627: // manipulation 2842 this.manipulation = (BiologicallyDerivedProductManipulationComponent) value; // BiologicallyDerivedProductManipulationComponent 2843 return value; 2844 case -1884274053: // storage 2845 this.getStorage().add((BiologicallyDerivedProductStorageComponent) value); // BiologicallyDerivedProductStorageComponent 2846 return value; 2847 default: 2848 return super.setProperty(hash, name, value); 2849 } 2850 2851 } 2852 2853 @Override 2854 public Base setProperty(String name, Base value) throws FHIRException { 2855 if (name.equals("identifier")) { 2856 this.getIdentifier().add(castToIdentifier(value)); 2857 } else if (name.equals("productCategory")) { 2858 value = new BiologicallyDerivedProductCategoryEnumFactory().fromType(castToCode(value)); 2859 this.productCategory = (Enumeration) value; // Enumeration<BiologicallyDerivedProductCategory> 2860 } else if (name.equals("productCode")) { 2861 this.productCode = castToCodeableConcept(value); // CodeableConcept 2862 } else if (name.equals("status")) { 2863 value = new BiologicallyDerivedProductStatusEnumFactory().fromType(castToCode(value)); 2864 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductStatus> 2865 } else if (name.equals("request")) { 2866 this.getRequest().add(castToReference(value)); 2867 } else if (name.equals("quantity")) { 2868 this.quantity = castToInteger(value); // IntegerType 2869 } else if (name.equals("parent")) { 2870 this.getParent().add(castToReference(value)); 2871 } else if (name.equals("collection")) { 2872 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 2873 } else if (name.equals("processing")) { 2874 this.getProcessing().add((BiologicallyDerivedProductProcessingComponent) value); 2875 } else if (name.equals("manipulation")) { 2876 this.manipulation = (BiologicallyDerivedProductManipulationComponent) value; // BiologicallyDerivedProductManipulationComponent 2877 } else if (name.equals("storage")) { 2878 this.getStorage().add((BiologicallyDerivedProductStorageComponent) value); 2879 } else 2880 return super.setProperty(name, value); 2881 return value; 2882 } 2883 2884 @Override 2885 public void removeChild(String name, Base value) throws FHIRException { 2886 if (name.equals("identifier")) { 2887 this.getIdentifier().remove(castToIdentifier(value)); 2888 } else if (name.equals("productCategory")) { 2889 this.productCategory = null; 2890 } else if (name.equals("productCode")) { 2891 this.productCode = null; 2892 } else if (name.equals("status")) { 2893 this.status = null; 2894 } else if (name.equals("request")) { 2895 this.getRequest().remove(castToReference(value)); 2896 } else if (name.equals("quantity")) { 2897 this.quantity = null; 2898 } else if (name.equals("parent")) { 2899 this.getParent().remove(castToReference(value)); 2900 } else if (name.equals("collection")) { 2901 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 2902 } else if (name.equals("processing")) { 2903 this.getProcessing().remove((BiologicallyDerivedProductProcessingComponent) value); 2904 } else if (name.equals("manipulation")) { 2905 this.manipulation = (BiologicallyDerivedProductManipulationComponent) value; // BiologicallyDerivedProductManipulationComponent 2906 } else if (name.equals("storage")) { 2907 this.getStorage().remove((BiologicallyDerivedProductStorageComponent) value); 2908 } else 2909 super.removeChild(name, value); 2910 2911 } 2912 2913 @Override 2914 public Base makeProperty(int hash, String name) throws FHIRException { 2915 switch (hash) { 2916 case -1618432855: 2917 return addIdentifier(); 2918 case 197299981: 2919 return getProductCategoryElement(); 2920 case -1492131972: 2921 return getProductCode(); 2922 case -892481550: 2923 return getStatusElement(); 2924 case 1095692943: 2925 return addRequest(); 2926 case -1285004149: 2927 return getQuantityElement(); 2928 case -995424086: 2929 return addParent(); 2930 case -1741312354: 2931 return getCollection(); 2932 case 422194963: 2933 return addProcessing(); 2934 case -696214627: 2935 return getManipulation(); 2936 case -1884274053: 2937 return addStorage(); 2938 default: 2939 return super.makeProperty(hash, name); 2940 } 2941 2942 } 2943 2944 @Override 2945 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2946 switch (hash) { 2947 case -1618432855: 2948 /* identifier */ return new String[] { "Identifier" }; 2949 case 197299981: 2950 /* productCategory */ return new String[] { "code" }; 2951 case -1492131972: 2952 /* productCode */ return new String[] { "CodeableConcept" }; 2953 case -892481550: 2954 /* status */ return new String[] { "code" }; 2955 case 1095692943: 2956 /* request */ return new String[] { "Reference" }; 2957 case -1285004149: 2958 /* quantity */ return new String[] { "integer" }; 2959 case -995424086: 2960 /* parent */ return new String[] { "Reference" }; 2961 case -1741312354: 2962 /* collection */ return new String[] {}; 2963 case 422194963: 2964 /* processing */ return new String[] {}; 2965 case -696214627: 2966 /* manipulation */ return new String[] {}; 2967 case -1884274053: 2968 /* storage */ return new String[] {}; 2969 default: 2970 return super.getTypesForProperty(hash, name); 2971 } 2972 2973 } 2974 2975 @Override 2976 public Base addChild(String name) throws FHIRException { 2977 if (name.equals("identifier")) { 2978 return addIdentifier(); 2979 } else if (name.equals("productCategory")) { 2980 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.productCategory"); 2981 } else if (name.equals("productCode")) { 2982 this.productCode = new CodeableConcept(); 2983 return this.productCode; 2984 } else if (name.equals("status")) { 2985 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.status"); 2986 } else if (name.equals("request")) { 2987 return addRequest(); 2988 } else if (name.equals("quantity")) { 2989 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.quantity"); 2990 } else if (name.equals("parent")) { 2991 return addParent(); 2992 } else if (name.equals("collection")) { 2993 this.collection = new BiologicallyDerivedProductCollectionComponent(); 2994 return this.collection; 2995 } else if (name.equals("processing")) { 2996 return addProcessing(); 2997 } else if (name.equals("manipulation")) { 2998 this.manipulation = new BiologicallyDerivedProductManipulationComponent(); 2999 return this.manipulation; 3000 } else if (name.equals("storage")) { 3001 return addStorage(); 3002 } else 3003 return super.addChild(name); 3004 } 3005 3006 public String fhirType() { 3007 return "BiologicallyDerivedProduct"; 3008 3009 } 3010 3011 public BiologicallyDerivedProduct copy() { 3012 BiologicallyDerivedProduct dst = new BiologicallyDerivedProduct(); 3013 copyValues(dst); 3014 return dst; 3015 } 3016 3017 public void copyValues(BiologicallyDerivedProduct dst) { 3018 super.copyValues(dst); 3019 if (identifier != null) { 3020 dst.identifier = new ArrayList<Identifier>(); 3021 for (Identifier i : identifier) 3022 dst.identifier.add(i.copy()); 3023 } 3024 ; 3025 dst.productCategory = productCategory == null ? null : productCategory.copy(); 3026 dst.productCode = productCode == null ? null : productCode.copy(); 3027 dst.status = status == null ? null : status.copy(); 3028 if (request != null) { 3029 dst.request = new ArrayList<Reference>(); 3030 for (Reference i : request) 3031 dst.request.add(i.copy()); 3032 } 3033 ; 3034 dst.quantity = quantity == null ? null : quantity.copy(); 3035 if (parent != null) { 3036 dst.parent = new ArrayList<Reference>(); 3037 for (Reference i : parent) 3038 dst.parent.add(i.copy()); 3039 } 3040 ; 3041 dst.collection = collection == null ? null : collection.copy(); 3042 if (processing != null) { 3043 dst.processing = new ArrayList<BiologicallyDerivedProductProcessingComponent>(); 3044 for (BiologicallyDerivedProductProcessingComponent i : processing) 3045 dst.processing.add(i.copy()); 3046 } 3047 ; 3048 dst.manipulation = manipulation == null ? null : manipulation.copy(); 3049 if (storage != null) { 3050 dst.storage = new ArrayList<BiologicallyDerivedProductStorageComponent>(); 3051 for (BiologicallyDerivedProductStorageComponent i : storage) 3052 dst.storage.add(i.copy()); 3053 } 3054 ; 3055 } 3056 3057 protected BiologicallyDerivedProduct typedCopy() { 3058 return copy(); 3059 } 3060 3061 @Override 3062 public boolean equalsDeep(Base other_) { 3063 if (!super.equalsDeep(other_)) 3064 return false; 3065 if (!(other_ instanceof BiologicallyDerivedProduct)) 3066 return false; 3067 BiologicallyDerivedProduct o = (BiologicallyDerivedProduct) other_; 3068 return compareDeep(identifier, o.identifier, true) && compareDeep(productCategory, o.productCategory, true) 3069 && compareDeep(productCode, o.productCode, true) && compareDeep(status, o.status, true) 3070 && compareDeep(request, o.request, true) && compareDeep(quantity, o.quantity, true) 3071 && compareDeep(parent, o.parent, true) && compareDeep(collection, o.collection, true) 3072 && compareDeep(processing, o.processing, true) && compareDeep(manipulation, o.manipulation, true) 3073 && compareDeep(storage, o.storage, true); 3074 } 3075 3076 @Override 3077 public boolean equalsShallow(Base other_) { 3078 if (!super.equalsShallow(other_)) 3079 return false; 3080 if (!(other_ instanceof BiologicallyDerivedProduct)) 3081 return false; 3082 BiologicallyDerivedProduct o = (BiologicallyDerivedProduct) other_; 3083 return compareValues(productCategory, o.productCategory, true) && compareValues(status, o.status, true) 3084 && compareValues(quantity, o.quantity, true); 3085 } 3086 3087 public boolean isEmpty() { 3088 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, productCategory, productCode, status, 3089 request, quantity, parent, collection, processing, manipulation, storage); 3090 } 3091 3092 @Override 3093 public ResourceType getResourceType() { 3094 return ResourceType.BiologicallyDerivedProduct; 3095 } 3096 3097}