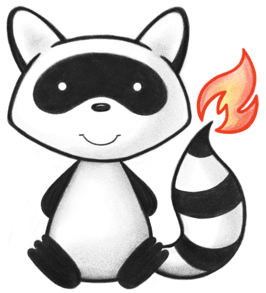
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.instance.model.api.IBaseBundle; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A container for a collection of resources. 052 */ 053@ResourceDef(name = "Bundle", profile = "http://hl7.org/fhir/StructureDefinition/Bundle") 054public class Bundle extends Resource implements IBaseBundle { 055 056 public enum BundleType { 057 /** 058 * The bundle is a document. The first resource is a Composition. 059 */ 060 DOCUMENT, 061 /** 062 * The bundle is a message. The first resource is a MessageHeader. 063 */ 064 MESSAGE, 065 /** 066 * The bundle is a transaction - intended to be processed by a server as an 067 * atomic commit. 068 */ 069 TRANSACTION, 070 /** 071 * The bundle is a transaction response. Because the response is a transaction 072 * response, the transaction has succeeded, and all responses are error free. 073 */ 074 TRANSACTIONRESPONSE, 075 /** 076 * The bundle is a set of actions - intended to be processed by a server as a 077 * group of independent actions. 078 */ 079 BATCH, 080 /** 081 * The bundle is a batch response. Note that as a batch, some responses may 082 * indicate failure and others success. 083 */ 084 BATCHRESPONSE, 085 /** 086 * The bundle is a list of resources from a history interaction on a server. 087 */ 088 HISTORY, 089 /** 090 * The bundle is a list of resources returned as a result of a search/query 091 * interaction, operation, or message. 092 */ 093 SEARCHSET, 094 /** 095 * The bundle is a set of resources collected into a single package for ease of 096 * distribution that imposes no processing obligations or behavioral rules 097 * beyond persistence. 098 */ 099 COLLECTION, 100 /** 101 * added to help the parsers with the generic types 102 */ 103 NULL; 104 105 public static BundleType fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("document".equals(codeString)) 109 return DOCUMENT; 110 if ("message".equals(codeString)) 111 return MESSAGE; 112 if ("transaction".equals(codeString)) 113 return TRANSACTION; 114 if ("transaction-response".equals(codeString)) 115 return TRANSACTIONRESPONSE; 116 if ("batch".equals(codeString)) 117 return BATCH; 118 if ("batch-response".equals(codeString)) 119 return BATCHRESPONSE; 120 if ("history".equals(codeString)) 121 return HISTORY; 122 if ("searchset".equals(codeString)) 123 return SEARCHSET; 124 if ("collection".equals(codeString)) 125 return COLLECTION; 126 if (Configuration.isAcceptInvalidEnums()) 127 return null; 128 else 129 throw new FHIRException("Unknown BundleType code '" + codeString + "'"); 130 } 131 132 public String toCode() { 133 switch (this) { 134 case DOCUMENT: 135 return "document"; 136 case MESSAGE: 137 return "message"; 138 case TRANSACTION: 139 return "transaction"; 140 case TRANSACTIONRESPONSE: 141 return "transaction-response"; 142 case BATCH: 143 return "batch"; 144 case BATCHRESPONSE: 145 return "batch-response"; 146 case HISTORY: 147 return "history"; 148 case SEARCHSET: 149 return "searchset"; 150 case COLLECTION: 151 return "collection"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getSystem() { 160 switch (this) { 161 case DOCUMENT: 162 return "http://hl7.org/fhir/bundle-type"; 163 case MESSAGE: 164 return "http://hl7.org/fhir/bundle-type"; 165 case TRANSACTION: 166 return "http://hl7.org/fhir/bundle-type"; 167 case TRANSACTIONRESPONSE: 168 return "http://hl7.org/fhir/bundle-type"; 169 case BATCH: 170 return "http://hl7.org/fhir/bundle-type"; 171 case BATCHRESPONSE: 172 return "http://hl7.org/fhir/bundle-type"; 173 case HISTORY: 174 return "http://hl7.org/fhir/bundle-type"; 175 case SEARCHSET: 176 return "http://hl7.org/fhir/bundle-type"; 177 case COLLECTION: 178 return "http://hl7.org/fhir/bundle-type"; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 186 public String getDefinition() { 187 switch (this) { 188 case DOCUMENT: 189 return "The bundle is a document. The first resource is a Composition."; 190 case MESSAGE: 191 return "The bundle is a message. The first resource is a MessageHeader."; 192 case TRANSACTION: 193 return "The bundle is a transaction - intended to be processed by a server as an atomic commit."; 194 case TRANSACTIONRESPONSE: 195 return "The bundle is a transaction response. Because the response is a transaction response, the transaction has succeeded, and all responses are error free."; 196 case BATCH: 197 return "The bundle is a set of actions - intended to be processed by a server as a group of independent actions."; 198 case BATCHRESPONSE: 199 return "The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success."; 200 case HISTORY: 201 return "The bundle is a list of resources from a history interaction on a server."; 202 case SEARCHSET: 203 return "The bundle is a list of resources returned as a result of a search/query interaction, operation, or message."; 204 case COLLECTION: 205 return "The bundle is a set of resources collected into a single package for ease of distribution that imposes no processing obligations or behavioral rules beyond persistence."; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 213 public String getDisplay() { 214 switch (this) { 215 case DOCUMENT: 216 return "Document"; 217 case MESSAGE: 218 return "Message"; 219 case TRANSACTION: 220 return "Transaction"; 221 case TRANSACTIONRESPONSE: 222 return "Transaction Response"; 223 case BATCH: 224 return "Batch"; 225 case BATCHRESPONSE: 226 return "Batch Response"; 227 case HISTORY: 228 return "History List"; 229 case SEARCHSET: 230 return "Search Results"; 231 case COLLECTION: 232 return "Collection"; 233 case NULL: 234 return null; 235 default: 236 return "?"; 237 } 238 } 239 } 240 241 public static class BundleTypeEnumFactory implements EnumFactory<BundleType> { 242 public BundleType fromCode(String codeString) throws IllegalArgumentException { 243 if (codeString == null || "".equals(codeString)) 244 if (codeString == null || "".equals(codeString)) 245 return null; 246 if ("document".equals(codeString)) 247 return BundleType.DOCUMENT; 248 if ("message".equals(codeString)) 249 return BundleType.MESSAGE; 250 if ("transaction".equals(codeString)) 251 return BundleType.TRANSACTION; 252 if ("transaction-response".equals(codeString)) 253 return BundleType.TRANSACTIONRESPONSE; 254 if ("batch".equals(codeString)) 255 return BundleType.BATCH; 256 if ("batch-response".equals(codeString)) 257 return BundleType.BATCHRESPONSE; 258 if ("history".equals(codeString)) 259 return BundleType.HISTORY; 260 if ("searchset".equals(codeString)) 261 return BundleType.SEARCHSET; 262 if ("collection".equals(codeString)) 263 return BundleType.COLLECTION; 264 throw new IllegalArgumentException("Unknown BundleType code '" + codeString + "'"); 265 } 266 267 public Enumeration<BundleType> fromType(PrimitiveType<?> code) throws FHIRException { 268 if (code == null) 269 return null; 270 if (code.isEmpty()) 271 return new Enumeration<BundleType>(this, BundleType.NULL, code); 272 String codeString = code.asStringValue(); 273 if (codeString == null || "".equals(codeString)) 274 return new Enumeration<BundleType>(this, BundleType.NULL, code); 275 if ("document".equals(codeString)) 276 return new Enumeration<BundleType>(this, BundleType.DOCUMENT, code); 277 if ("message".equals(codeString)) 278 return new Enumeration<BundleType>(this, BundleType.MESSAGE, code); 279 if ("transaction".equals(codeString)) 280 return new Enumeration<BundleType>(this, BundleType.TRANSACTION, code); 281 if ("transaction-response".equals(codeString)) 282 return new Enumeration<BundleType>(this, BundleType.TRANSACTIONRESPONSE, code); 283 if ("batch".equals(codeString)) 284 return new Enumeration<BundleType>(this, BundleType.BATCH, code); 285 if ("batch-response".equals(codeString)) 286 return new Enumeration<BundleType>(this, BundleType.BATCHRESPONSE, code); 287 if ("history".equals(codeString)) 288 return new Enumeration<BundleType>(this, BundleType.HISTORY, code); 289 if ("searchset".equals(codeString)) 290 return new Enumeration<BundleType>(this, BundleType.SEARCHSET, code); 291 if ("collection".equals(codeString)) 292 return new Enumeration<BundleType>(this, BundleType.COLLECTION, code); 293 throw new FHIRException("Unknown BundleType code '" + codeString + "'"); 294 } 295 296 public String toCode(BundleType code) { 297 if (code == BundleType.NULL) 298 return null; 299 if (code == BundleType.DOCUMENT) 300 return "document"; 301 if (code == BundleType.MESSAGE) 302 return "message"; 303 if (code == BundleType.TRANSACTION) 304 return "transaction"; 305 if (code == BundleType.TRANSACTIONRESPONSE) 306 return "transaction-response"; 307 if (code == BundleType.BATCH) 308 return "batch"; 309 if (code == BundleType.BATCHRESPONSE) 310 return "batch-response"; 311 if (code == BundleType.HISTORY) 312 return "history"; 313 if (code == BundleType.SEARCHSET) 314 return "searchset"; 315 if (code == BundleType.COLLECTION) 316 return "collection"; 317 return "?"; 318 } 319 320 public String toSystem(BundleType code) { 321 return code.getSystem(); 322 } 323 } 324 325 public enum SearchEntryMode { 326 /** 327 * This resource matched the search specification. 328 */ 329 MATCH, 330 /** 331 * This resource is returned because it is referred to from another resource in 332 * the search set. 333 */ 334 INCLUDE, 335 /** 336 * An OperationOutcome that provides additional information about the processing 337 * of a search. 338 */ 339 OUTCOME, 340 /** 341 * added to help the parsers with the generic types 342 */ 343 NULL; 344 345 public static SearchEntryMode fromCode(String codeString) throws FHIRException { 346 if (codeString == null || "".equals(codeString)) 347 return null; 348 if ("match".equals(codeString)) 349 return MATCH; 350 if ("include".equals(codeString)) 351 return INCLUDE; 352 if ("outcome".equals(codeString)) 353 return OUTCOME; 354 if (Configuration.isAcceptInvalidEnums()) 355 return null; 356 else 357 throw new FHIRException("Unknown SearchEntryMode code '" + codeString + "'"); 358 } 359 360 public String toCode() { 361 switch (this) { 362 case MATCH: 363 return "match"; 364 case INCLUDE: 365 return "include"; 366 case OUTCOME: 367 return "outcome"; 368 case NULL: 369 return null; 370 default: 371 return "?"; 372 } 373 } 374 375 public String getSystem() { 376 switch (this) { 377 case MATCH: 378 return "http://hl7.org/fhir/search-entry-mode"; 379 case INCLUDE: 380 return "http://hl7.org/fhir/search-entry-mode"; 381 case OUTCOME: 382 return "http://hl7.org/fhir/search-entry-mode"; 383 case NULL: 384 return null; 385 default: 386 return "?"; 387 } 388 } 389 390 public String getDefinition() { 391 switch (this) { 392 case MATCH: 393 return "This resource matched the search specification."; 394 case INCLUDE: 395 return "This resource is returned because it is referred to from another resource in the search set."; 396 case OUTCOME: 397 return "An OperationOutcome that provides additional information about the processing of a search."; 398 case NULL: 399 return null; 400 default: 401 return "?"; 402 } 403 } 404 405 public String getDisplay() { 406 switch (this) { 407 case MATCH: 408 return "Match"; 409 case INCLUDE: 410 return "Include"; 411 case OUTCOME: 412 return "Outcome"; 413 case NULL: 414 return null; 415 default: 416 return "?"; 417 } 418 } 419 } 420 421 public static class SearchEntryModeEnumFactory implements EnumFactory<SearchEntryMode> { 422 public SearchEntryMode fromCode(String codeString) throws IllegalArgumentException { 423 if (codeString == null || "".equals(codeString)) 424 if (codeString == null || "".equals(codeString)) 425 return null; 426 if ("match".equals(codeString)) 427 return SearchEntryMode.MATCH; 428 if ("include".equals(codeString)) 429 return SearchEntryMode.INCLUDE; 430 if ("outcome".equals(codeString)) 431 return SearchEntryMode.OUTCOME; 432 throw new IllegalArgumentException("Unknown SearchEntryMode code '" + codeString + "'"); 433 } 434 435 public Enumeration<SearchEntryMode> fromType(PrimitiveType<?> code) throws FHIRException { 436 if (code == null) 437 return null; 438 if (code.isEmpty()) 439 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.NULL, code); 440 String codeString = code.asStringValue(); 441 if (codeString == null || "".equals(codeString)) 442 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.NULL, code); 443 if ("match".equals(codeString)) 444 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.MATCH, code); 445 if ("include".equals(codeString)) 446 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.INCLUDE, code); 447 if ("outcome".equals(codeString)) 448 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.OUTCOME, code); 449 throw new FHIRException("Unknown SearchEntryMode code '" + codeString + "'"); 450 } 451 452 public String toCode(SearchEntryMode code) { 453 if (code == SearchEntryMode.NULL) 454 return null; 455 if (code == SearchEntryMode.MATCH) 456 return "match"; 457 if (code == SearchEntryMode.INCLUDE) 458 return "include"; 459 if (code == SearchEntryMode.OUTCOME) 460 return "outcome"; 461 return "?"; 462 } 463 464 public String toSystem(SearchEntryMode code) { 465 return code.getSystem(); 466 } 467 } 468 469 public enum HTTPVerb { 470 /** 471 * HTTP GET Command. 472 */ 473 GET, 474 /** 475 * HTTP HEAD Command. 476 */ 477 HEAD, 478 /** 479 * HTTP POST Command. 480 */ 481 POST, 482 /** 483 * HTTP PUT Command. 484 */ 485 PUT, 486 /** 487 * HTTP DELETE Command. 488 */ 489 DELETE, 490 /** 491 * HTTP PATCH Command. 492 */ 493 PATCH, 494 /** 495 * added to help the parsers with the generic types 496 */ 497 NULL; 498 499 public static HTTPVerb fromCode(String codeString) throws FHIRException { 500 if (codeString == null || "".equals(codeString)) 501 return null; 502 if ("GET".equals(codeString)) 503 return GET; 504 if ("HEAD".equals(codeString)) 505 return HEAD; 506 if ("POST".equals(codeString)) 507 return POST; 508 if ("PUT".equals(codeString)) 509 return PUT; 510 if ("DELETE".equals(codeString)) 511 return DELETE; 512 if ("PATCH".equals(codeString)) 513 return PATCH; 514 if (Configuration.isAcceptInvalidEnums()) 515 return null; 516 else 517 throw new FHIRException("Unknown HTTPVerb code '" + codeString + "'"); 518 } 519 520 public String toCode() { 521 switch (this) { 522 case GET: 523 return "GET"; 524 case HEAD: 525 return "HEAD"; 526 case POST: 527 return "POST"; 528 case PUT: 529 return "PUT"; 530 case DELETE: 531 return "DELETE"; 532 case PATCH: 533 return "PATCH"; 534 case NULL: 535 return null; 536 default: 537 return "?"; 538 } 539 } 540 541 public String getSystem() { 542 switch (this) { 543 case GET: 544 return "http://hl7.org/fhir/http-verb"; 545 case HEAD: 546 return "http://hl7.org/fhir/http-verb"; 547 case POST: 548 return "http://hl7.org/fhir/http-verb"; 549 case PUT: 550 return "http://hl7.org/fhir/http-verb"; 551 case DELETE: 552 return "http://hl7.org/fhir/http-verb"; 553 case PATCH: 554 return "http://hl7.org/fhir/http-verb"; 555 case NULL: 556 return null; 557 default: 558 return "?"; 559 } 560 } 561 562 public String getDefinition() { 563 switch (this) { 564 case GET: 565 return "HTTP GET Command."; 566 case HEAD: 567 return "HTTP HEAD Command."; 568 case POST: 569 return "HTTP POST Command."; 570 case PUT: 571 return "HTTP PUT Command."; 572 case DELETE: 573 return "HTTP DELETE Command."; 574 case PATCH: 575 return "HTTP PATCH Command."; 576 case NULL: 577 return null; 578 default: 579 return "?"; 580 } 581 } 582 583 public String getDisplay() { 584 switch (this) { 585 case GET: 586 return "GET"; 587 case HEAD: 588 return "HEAD"; 589 case POST: 590 return "POST"; 591 case PUT: 592 return "PUT"; 593 case DELETE: 594 return "DELETE"; 595 case PATCH: 596 return "PATCH"; 597 case NULL: 598 return null; 599 default: 600 return "?"; 601 } 602 } 603 } 604 605 public static class HTTPVerbEnumFactory implements EnumFactory<HTTPVerb> { 606 public HTTPVerb fromCode(String codeString) throws IllegalArgumentException { 607 if (codeString == null || "".equals(codeString)) 608 if (codeString == null || "".equals(codeString)) 609 return null; 610 if ("GET".equals(codeString)) 611 return HTTPVerb.GET; 612 if ("HEAD".equals(codeString)) 613 return HTTPVerb.HEAD; 614 if ("POST".equals(codeString)) 615 return HTTPVerb.POST; 616 if ("PUT".equals(codeString)) 617 return HTTPVerb.PUT; 618 if ("DELETE".equals(codeString)) 619 return HTTPVerb.DELETE; 620 if ("PATCH".equals(codeString)) 621 return HTTPVerb.PATCH; 622 throw new IllegalArgumentException("Unknown HTTPVerb code '" + codeString + "'"); 623 } 624 625 public Enumeration<HTTPVerb> fromType(PrimitiveType<?> code) throws FHIRException { 626 if (code == null) 627 return null; 628 if (code.isEmpty()) 629 return new Enumeration<HTTPVerb>(this, HTTPVerb.NULL, code); 630 String codeString = code.asStringValue(); 631 if (codeString == null || "".equals(codeString)) 632 return new Enumeration<HTTPVerb>(this, HTTPVerb.NULL, code); 633 if ("GET".equals(codeString)) 634 return new Enumeration<HTTPVerb>(this, HTTPVerb.GET, code); 635 if ("HEAD".equals(codeString)) 636 return new Enumeration<HTTPVerb>(this, HTTPVerb.HEAD, code); 637 if ("POST".equals(codeString)) 638 return new Enumeration<HTTPVerb>(this, HTTPVerb.POST, code); 639 if ("PUT".equals(codeString)) 640 return new Enumeration<HTTPVerb>(this, HTTPVerb.PUT, code); 641 if ("DELETE".equals(codeString)) 642 return new Enumeration<HTTPVerb>(this, HTTPVerb.DELETE, code); 643 if ("PATCH".equals(codeString)) 644 return new Enumeration<HTTPVerb>(this, HTTPVerb.PATCH, code); 645 throw new FHIRException("Unknown HTTPVerb code '" + codeString + "'"); 646 } 647 648 public String toCode(HTTPVerb code) { 649 if (code == HTTPVerb.NULL) 650 return null; 651 if (code == HTTPVerb.GET) 652 return "GET"; 653 if (code == HTTPVerb.HEAD) 654 return "HEAD"; 655 if (code == HTTPVerb.POST) 656 return "POST"; 657 if (code == HTTPVerb.PUT) 658 return "PUT"; 659 if (code == HTTPVerb.DELETE) 660 return "DELETE"; 661 if (code == HTTPVerb.PATCH) 662 return "PATCH"; 663 return "?"; 664 } 665 666 public String toSystem(HTTPVerb code) { 667 return code.getSystem(); 668 } 669 } 670 671 @Block() 672 public static class BundleLinkComponent extends BackboneElement implements IBaseBackboneElement { 673 /** 674 * A name which details the functional use for this link - see 675 * [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 676 */ 677 @Child(name = "relation", type = { 678 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 679 @Description(shortDefinition = "See http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1", formalDefinition = "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).") 680 protected StringType relation; 681 682 /** 683 * The reference details for the link. 684 */ 685 @Child(name = "url", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 686 @Description(shortDefinition = "Reference details for the link", formalDefinition = "The reference details for the link.") 687 protected UriType url; 688 689 private static final long serialVersionUID = -1010386066L; 690 691 /** 692 * Constructor 693 */ 694 public BundleLinkComponent() { 695 super(); 696 } 697 698 /** 699 * Constructor 700 */ 701 public BundleLinkComponent(StringType relation, UriType url) { 702 super(); 703 this.relation = relation; 704 this.url = url; 705 } 706 707 /** 708 * @return {@link #relation} (A name which details the functional use for this 709 * link - see 710 * [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).). 711 * This is the underlying object with id, value and extensions. The 712 * accessor "getRelation" gives direct access to the value 713 */ 714 public StringType getRelationElement() { 715 if (this.relation == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create BundleLinkComponent.relation"); 718 else if (Configuration.doAutoCreate()) 719 this.relation = new StringType(); // bb 720 return this.relation; 721 } 722 723 public boolean hasRelationElement() { 724 return this.relation != null && !this.relation.isEmpty(); 725 } 726 727 public boolean hasRelation() { 728 return this.relation != null && !this.relation.isEmpty(); 729 } 730 731 /** 732 * @param value {@link #relation} (A name which details the functional use for 733 * this link - see 734 * [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).). 735 * This is the underlying object with id, value and extensions. The 736 * accessor "getRelation" gives direct access to the value 737 */ 738 public BundleLinkComponent setRelationElement(StringType value) { 739 this.relation = value; 740 return this; 741 } 742 743 /** 744 * @return A name which details the functional use for this link - see 745 * [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 746 */ 747 public String getRelation() { 748 return this.relation == null ? null : this.relation.getValue(); 749 } 750 751 /** 752 * @param value A name which details the functional use for this link - see 753 * [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 754 */ 755 public BundleLinkComponent setRelation(String value) { 756 if (this.relation == null) 757 this.relation = new StringType(); 758 this.relation.setValue(value); 759 return this; 760 } 761 762 /** 763 * @return {@link #url} (The reference details for the link.). This is the 764 * underlying object with id, value and extensions. The accessor 765 * "getUrl" gives direct access to the value 766 */ 767 public UriType getUrlElement() { 768 if (this.url == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create BundleLinkComponent.url"); 771 else if (Configuration.doAutoCreate()) 772 this.url = new UriType(); // bb 773 return this.url; 774 } 775 776 public boolean hasUrlElement() { 777 return this.url != null && !this.url.isEmpty(); 778 } 779 780 public boolean hasUrl() { 781 return this.url != null && !this.url.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #url} (The reference details for the link.). This is the 786 * underlying object with id, value and extensions. The accessor 787 * "getUrl" gives direct access to the value 788 */ 789 public BundleLinkComponent setUrlElement(UriType value) { 790 this.url = value; 791 return this; 792 } 793 794 /** 795 * @return The reference details for the link. 796 */ 797 public String getUrl() { 798 return this.url == null ? null : this.url.getValue(); 799 } 800 801 /** 802 * @param value The reference details for the link. 803 */ 804 public BundleLinkComponent setUrl(String value) { 805 if (this.url == null) 806 this.url = new UriType(); 807 this.url.setValue(value); 808 return this; 809 } 810 811 protected void listChildren(List<Property> children) { 812 super.listChildren(children); 813 children.add(new Property("relation", "string", 814 "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).", 815 0, 1, relation)); 816 children.add(new Property("url", "uri", "The reference details for the link.", 0, 1, url)); 817 } 818 819 @Override 820 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 821 switch (_hash) { 822 case -554436100: 823 /* relation */ return new Property("relation", "string", 824 "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).", 825 0, 1, relation); 826 case 116079: 827 /* url */ return new Property("url", "uri", "The reference details for the link.", 0, 1, url); 828 default: 829 return super.getNamedProperty(_hash, _name, _checkValid); 830 } 831 832 } 833 834 @Override 835 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 836 switch (hash) { 837 case -554436100: 838 /* relation */ return this.relation == null ? new Base[0] : new Base[] { this.relation }; // StringType 839 case 116079: 840 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 841 default: 842 return super.getProperty(hash, name, checkValid); 843 } 844 845 } 846 847 @Override 848 public Base setProperty(int hash, String name, Base value) throws FHIRException { 849 switch (hash) { 850 case -554436100: // relation 851 this.relation = castToString(value); // StringType 852 return value; 853 case 116079: // url 854 this.url = castToUri(value); // UriType 855 return value; 856 default: 857 return super.setProperty(hash, name, value); 858 } 859 860 } 861 862 @Override 863 public Base setProperty(String name, Base value) throws FHIRException { 864 if (name.equals("relation")) { 865 this.relation = castToString(value); // StringType 866 } else if (name.equals("url")) { 867 this.url = castToUri(value); // UriType 868 } else 869 return super.setProperty(name, value); 870 return value; 871 } 872 873 @Override 874 public void removeChild(String name, Base value) throws FHIRException { 875 if (name.equals("relation")) { 876 this.relation = null; 877 } else if (name.equals("url")) { 878 this.url = null; 879 } else 880 super.removeChild(name, value); 881 882 } 883 884 @Override 885 public Base makeProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case -554436100: 888 return getRelationElement(); 889 case 116079: 890 return getUrlElement(); 891 default: 892 return super.makeProperty(hash, name); 893 } 894 895 } 896 897 @Override 898 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 899 switch (hash) { 900 case -554436100: 901 /* relation */ return new String[] { "string" }; 902 case 116079: 903 /* url */ return new String[] { "uri" }; 904 default: 905 return super.getTypesForProperty(hash, name); 906 } 907 908 } 909 910 @Override 911 public Base addChild(String name) throws FHIRException { 912 if (name.equals("relation")) { 913 throw new FHIRException("Cannot call addChild on a singleton property Bundle.relation"); 914 } else if (name.equals("url")) { 915 throw new FHIRException("Cannot call addChild on a singleton property Bundle.url"); 916 } else 917 return super.addChild(name); 918 } 919 920 public BundleLinkComponent copy() { 921 BundleLinkComponent dst = new BundleLinkComponent(); 922 copyValues(dst); 923 return dst; 924 } 925 926 public void copyValues(BundleLinkComponent dst) { 927 super.copyValues(dst); 928 dst.relation = relation == null ? null : relation.copy(); 929 dst.url = url == null ? null : url.copy(); 930 } 931 932 @Override 933 public boolean equalsDeep(Base other_) { 934 if (!super.equalsDeep(other_)) 935 return false; 936 if (!(other_ instanceof BundleLinkComponent)) 937 return false; 938 BundleLinkComponent o = (BundleLinkComponent) other_; 939 return compareDeep(relation, o.relation, true) && compareDeep(url, o.url, true); 940 } 941 942 @Override 943 public boolean equalsShallow(Base other_) { 944 if (!super.equalsShallow(other_)) 945 return false; 946 if (!(other_ instanceof BundleLinkComponent)) 947 return false; 948 BundleLinkComponent o = (BundleLinkComponent) other_; 949 return compareValues(relation, o.relation, true) && compareValues(url, o.url, true); 950 } 951 952 public boolean isEmpty() { 953 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relation, url); 954 } 955 956 public String fhirType() { 957 return "Bundle.link"; 958 959 } 960 961 } 962 963 @Block() 964 public static class BundleEntryComponent extends BackboneElement implements IBaseBackboneElement { 965 /** 966 * A series of links that provide context to this entry. 967 */ 968 @Child(name = "link", type = { 969 BundleLinkComponent.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 970 @Description(shortDefinition = "Links related to this entry", formalDefinition = "A series of links that provide context to this entry.") 971 protected List<BundleLinkComponent> link; 972 973 /** 974 * The Absolute URL for the resource. The fullUrl SHALL NOT disagree with the id 975 * in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be 976 * version-independent URL consistent with the Resource.id. The fullUrl is a 977 * version independent reference to the resource. The fullUrl element SHALL have 978 * a value except that: fullUrl can be empty on a POST (although it does not 979 * need to when specifying a temporary id for reference in the bundle) Results 980 * from operations might involve resources that are not identified. 981 */ 982 @Child(name = "fullUrl", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 983 @Description(shortDefinition = "URI for resource (Absolute URL server address or URI for UUID/OID)", formalDefinition = "The Absolute URL for the resource. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: \n* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle)\n* Results from operations might involve resources that are not identified.") 984 protected UriType fullUrl; 985 986 /** 987 * The Resource for the entry. The purpose/meaning of the resource is determined 988 * by the Bundle.type. 989 */ 990 @Child(name = "resource", type = { Resource.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 991 @Description(shortDefinition = "A resource in the bundle", formalDefinition = "The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type.") 992 protected Resource resource; 993 994 /** 995 * Information about the search process that lead to the creation of this entry. 996 */ 997 @Child(name = "search", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = true) 998 @Description(shortDefinition = "Search related information", formalDefinition = "Information about the search process that lead to the creation of this entry.") 999 protected BundleEntrySearchComponent search; 1000 1001 /** 1002 * Additional information about how this entry should be processed as part of a 1003 * transaction or batch. For history, it shows how the entry was processed to 1004 * create the version contained in the entry. 1005 */ 1006 @Child(name = "request", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 1007 @Description(shortDefinition = "Additional execution information (transaction/batch/history)", formalDefinition = "Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.") 1008 protected BundleEntryRequestComponent request; 1009 1010 /** 1011 * Indicates the results of processing the corresponding 'request' entry in the 1012 * batch or transaction being responded to or what the results of an operation 1013 * where when returning history. 1014 */ 1015 @Child(name = "response", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 1016 @Description(shortDefinition = "Results of execution (transaction/batch/history)", formalDefinition = "Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.") 1017 protected BundleEntryResponseComponent response; 1018 1019 private static final long serialVersionUID = 517783054L; 1020 1021 /** 1022 * Constructor 1023 */ 1024 public BundleEntryComponent() { 1025 super(); 1026 } 1027 1028 /** 1029 * @return {@link #link} (A series of links that provide context to this entry.) 1030 */ 1031 public List<BundleLinkComponent> getLink() { 1032 if (this.link == null) 1033 this.link = new ArrayList<BundleLinkComponent>(); 1034 return this.link; 1035 } 1036 1037 /** 1038 * @return Returns a reference to <code>this</code> for easy method chaining 1039 */ 1040 public BundleEntryComponent setLink(List<BundleLinkComponent> theLink) { 1041 this.link = theLink; 1042 return this; 1043 } 1044 1045 public boolean hasLink() { 1046 if (this.link == null) 1047 return false; 1048 for (BundleLinkComponent item : this.link) 1049 if (!item.isEmpty()) 1050 return true; 1051 return false; 1052 } 1053 1054 public BundleLinkComponent addLink() { // 3 1055 BundleLinkComponent t = new BundleLinkComponent(); 1056 if (this.link == null) 1057 this.link = new ArrayList<BundleLinkComponent>(); 1058 this.link.add(t); 1059 return t; 1060 } 1061 1062 public BundleEntryComponent addLink(BundleLinkComponent t) { // 3 1063 if (t == null) 1064 return this; 1065 if (this.link == null) 1066 this.link = new ArrayList<BundleLinkComponent>(); 1067 this.link.add(t); 1068 return this; 1069 } 1070 1071 /** 1072 * @return The first repetition of repeating field {@link #link}, creating it if 1073 * it does not already exist 1074 */ 1075 public BundleLinkComponent getLinkFirstRep() { 1076 if (getLink().isEmpty()) { 1077 addLink(); 1078 } 1079 return getLink().get(0); 1080 } 1081 1082 /** 1083 * @return {@link #fullUrl} (The Absolute URL for the resource. The fullUrl 1084 * SHALL NOT disagree with the id in the resource - i.e. if the fullUrl 1085 * is not a urn:uuid, the URL shall be version-independent URL 1086 * consistent with the Resource.id. The fullUrl is a version independent 1087 * reference to the resource. The fullUrl element SHALL have a value 1088 * except that: fullUrl can be empty on a POST (although it does not 1089 * need to when specifying a temporary id for reference in the bundle) 1090 * Results from operations might involve resources that are not 1091 * identified.). This is the underlying object with id, value and 1092 * extensions. The accessor "getFullUrl" gives direct access to the 1093 * value 1094 */ 1095 public UriType getFullUrlElement() { 1096 if (this.fullUrl == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create BundleEntryComponent.fullUrl"); 1099 else if (Configuration.doAutoCreate()) 1100 this.fullUrl = new UriType(); // bb 1101 return this.fullUrl; 1102 } 1103 1104 public boolean hasFullUrlElement() { 1105 return this.fullUrl != null && !this.fullUrl.isEmpty(); 1106 } 1107 1108 public boolean hasFullUrl() { 1109 return this.fullUrl != null && !this.fullUrl.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #fullUrl} (The Absolute URL for the resource. The fullUrl 1114 * SHALL NOT disagree with the id in the resource - i.e. if the 1115 * fullUrl is not a urn:uuid, the URL shall be version-independent 1116 * URL consistent with the Resource.id. The fullUrl is a version 1117 * independent reference to the resource. The fullUrl element SHALL 1118 * have a value except that: fullUrl can be empty on a POST 1119 * (although it does not need to when specifying a temporary id for 1120 * reference in the bundle) Results from operations might involve 1121 * resources that are not identified.). This is the underlying 1122 * object with id, value and extensions. The accessor "getFullUrl" 1123 * gives direct access to the value 1124 */ 1125 public BundleEntryComponent setFullUrlElement(UriType value) { 1126 this.fullUrl = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return The Absolute URL for the resource. The fullUrl SHALL NOT disagree 1132 * with the id in the resource - i.e. if the fullUrl is not a urn:uuid, 1133 * the URL shall be version-independent URL consistent with the 1134 * Resource.id. The fullUrl is a version independent reference to the 1135 * resource. The fullUrl element SHALL have a value except that: fullUrl 1136 * can be empty on a POST (although it does not need to when specifying 1137 * a temporary id for reference in the bundle) Results from operations 1138 * might involve resources that are not identified. 1139 */ 1140 public String getFullUrl() { 1141 return this.fullUrl == null ? null : this.fullUrl.getValue(); 1142 } 1143 1144 /** 1145 * @param value The Absolute URL for the resource. The fullUrl SHALL NOT 1146 * disagree with the id in the resource - i.e. if the fullUrl is 1147 * not a urn:uuid, the URL shall be version-independent URL 1148 * consistent with the Resource.id. The fullUrl is a version 1149 * independent reference to the resource. The fullUrl element SHALL 1150 * have a value except that: fullUrl can be empty on a POST 1151 * (although it does not need to when specifying a temporary id for 1152 * reference in the bundle) Results from operations might involve 1153 * resources that are not identified. 1154 */ 1155 public BundleEntryComponent setFullUrl(String value) { 1156 if (Utilities.noString(value)) 1157 this.fullUrl = null; 1158 else { 1159 if (this.fullUrl == null) 1160 this.fullUrl = new UriType(); 1161 this.fullUrl.setValue(value); 1162 } 1163 return this; 1164 } 1165 1166 /** 1167 * @return {@link #resource} (The Resource for the entry. The purpose/meaning of 1168 * the resource is determined by the Bundle.type.) 1169 */ 1170 public Resource getResource() { 1171 return this.resource; 1172 } 1173 1174 public boolean hasResource() { 1175 return this.resource != null && !this.resource.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #resource} (The Resource for the entry. The 1180 * purpose/meaning of the resource is determined by the 1181 * Bundle.type.) 1182 */ 1183 public BundleEntryComponent setResource(Resource value) { 1184 this.resource = value; 1185 return this; 1186 } 1187 1188 /** 1189 * @return {@link #search} (Information about the search process that lead to 1190 * the creation of this entry.) 1191 */ 1192 public BundleEntrySearchComponent getSearch() { 1193 if (this.search == null) 1194 if (Configuration.errorOnAutoCreate()) 1195 throw new Error("Attempt to auto-create BundleEntryComponent.search"); 1196 else if (Configuration.doAutoCreate()) 1197 this.search = new BundleEntrySearchComponent(); // cc 1198 return this.search; 1199 } 1200 1201 public boolean hasSearch() { 1202 return this.search != null && !this.search.isEmpty(); 1203 } 1204 1205 /** 1206 * @param value {@link #search} (Information about the search process that lead 1207 * to the creation of this entry.) 1208 */ 1209 public BundleEntryComponent setSearch(BundleEntrySearchComponent value) { 1210 this.search = value; 1211 return this; 1212 } 1213 1214 /** 1215 * @return {@link #request} (Additional information about how this entry should 1216 * be processed as part of a transaction or batch. For history, it shows 1217 * how the entry was processed to create the version contained in the 1218 * entry.) 1219 */ 1220 public BundleEntryRequestComponent getRequest() { 1221 if (this.request == null) 1222 if (Configuration.errorOnAutoCreate()) 1223 throw new Error("Attempt to auto-create BundleEntryComponent.request"); 1224 else if (Configuration.doAutoCreate()) 1225 this.request = new BundleEntryRequestComponent(); // cc 1226 return this.request; 1227 } 1228 1229 public boolean hasRequest() { 1230 return this.request != null && !this.request.isEmpty(); 1231 } 1232 1233 /** 1234 * @param value {@link #request} (Additional information about how this entry 1235 * should be processed as part of a transaction or batch. For 1236 * history, it shows how the entry was processed to create the 1237 * version contained in the entry.) 1238 */ 1239 public BundleEntryComponent setRequest(BundleEntryRequestComponent value) { 1240 this.request = value; 1241 return this; 1242 } 1243 1244 /** 1245 * @return {@link #response} (Indicates the results of processing the 1246 * corresponding 'request' entry in the batch or transaction being 1247 * responded to or what the results of an operation where when returning 1248 * history.) 1249 */ 1250 public BundleEntryResponseComponent getResponse() { 1251 if (this.response == null) 1252 if (Configuration.errorOnAutoCreate()) 1253 throw new Error("Attempt to auto-create BundleEntryComponent.response"); 1254 else if (Configuration.doAutoCreate()) 1255 this.response = new BundleEntryResponseComponent(); // cc 1256 return this.response; 1257 } 1258 1259 public boolean hasResponse() { 1260 return this.response != null && !this.response.isEmpty(); 1261 } 1262 1263 /** 1264 * @param value {@link #response} (Indicates the results of processing the 1265 * corresponding 'request' entry in the batch or transaction being 1266 * responded to or what the results of an operation where when 1267 * returning history.) 1268 */ 1269 public BundleEntryComponent setResponse(BundleEntryResponseComponent value) { 1270 this.response = value; 1271 return this; 1272 } 1273 1274 /** 1275 * Returns the {@link #getLink() link} which matches a given 1276 * {@link BundleLinkComponent#getRelation() relation}. If no link is found which 1277 * matches the given relation, returns <code>null</code>. If more than one link 1278 * is found which matches the given relation, returns the first matching 1279 * BundleLinkComponent. 1280 * 1281 * @param theRelation The relation, such as "next", or "self. See the constants 1282 * such as {@link IBaseBundle#LINK_SELF} and 1283 * {@link IBaseBundle#LINK_NEXT}. 1284 * @return Returns a matching BundleLinkComponent, or <code>null</code> 1285 * @see IBaseBundle#LINK_NEXT 1286 * @see IBaseBundle#LINK_PREV 1287 * @see IBaseBundle#LINK_SELF 1288 */ 1289 public BundleLinkComponent getLink(String theRelation) { 1290 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 1291 for (BundleLinkComponent next : getLink()) { 1292 if (theRelation.equals(next.getRelation())) { 1293 return next; 1294 } 1295 } 1296 return null; 1297 } 1298 1299 /** 1300 * Returns the {@link #getLink() link} which matches a given 1301 * {@link BundleLinkComponent#getRelation() relation}. If no link is found which 1302 * matches the given relation, creates a new BundleLinkComponent with the given 1303 * relation and adds it to this Bundle. If more than one link is found which 1304 * matches the given relation, returns the first matching BundleLinkComponent. 1305 * 1306 * @param theRelation The relation, such as "next", or "self. See the constants 1307 * such as {@link IBaseBundle#LINK_SELF} and 1308 * {@link IBaseBundle#LINK_NEXT}. 1309 * @return Returns a matching BundleLinkComponent, or <code>null</code> 1310 * @see IBaseBundle#LINK_NEXT 1311 * @see IBaseBundle#LINK_PREV 1312 * @see IBaseBundle#LINK_SELF 1313 */ 1314 public BundleLinkComponent getLinkOrCreate(String theRelation) { 1315 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 1316 for (BundleLinkComponent next : getLink()) { 1317 if (theRelation.equals(next.getRelation())) { 1318 return next; 1319 } 1320 } 1321 BundleLinkComponent retVal = new BundleLinkComponent(); 1322 retVal.setRelation(theRelation); 1323 getLink().add(retVal); 1324 return retVal; 1325 } 1326 1327 protected void listChildren(List<Property> children) { 1328 super.listChildren(children); 1329 children.add(new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 0, 1330 java.lang.Integer.MAX_VALUE, link)); 1331 children.add(new Property("fullUrl", "uri", 1332 "The Absolute URL for the resource. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: \n* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle)\n* Results from operations might involve resources that are not identified.", 1333 0, 1, fullUrl)); 1334 children.add(new Property("resource", "Resource", 1335 "The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type.", 0, 1, 1336 resource)); 1337 children.add(new Property("search", "", 1338 "Information about the search process that lead to the creation of this entry.", 0, 1, search)); 1339 children.add(new Property("request", "", 1340 "Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.", 1341 0, 1, request)); 1342 children.add(new Property("response", "", 1343 "Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.", 1344 0, 1, response)); 1345 } 1346 1347 @Override 1348 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1349 switch (_hash) { 1350 case 3321850: 1351 /* link */ return new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 1352 0, java.lang.Integer.MAX_VALUE, link); 1353 case -511251360: 1354 /* fullUrl */ return new Property("fullUrl", "uri", 1355 "The Absolute URL for the resource. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. The fullUrl element SHALL have a value except that: \n* fullUrl can be empty on a POST (although it does not need to when specifying a temporary id for reference in the bundle)\n* Results from operations might involve resources that are not identified.", 1356 0, 1, fullUrl); 1357 case -341064690: 1358 /* resource */ return new Property("resource", "Resource", 1359 "The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type.", 0, 1, 1360 resource); 1361 case -906336856: 1362 /* search */ return new Property("search", "", 1363 "Information about the search process that lead to the creation of this entry.", 0, 1, search); 1364 case 1095692943: 1365 /* request */ return new Property("request", "", 1366 "Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.", 1367 0, 1, request); 1368 case -340323263: 1369 /* response */ return new Property("response", "", 1370 "Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.", 1371 0, 1, response); 1372 default: 1373 return super.getNamedProperty(_hash, _name, _checkValid); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1380 switch (hash) { 1381 case 3321850: 1382 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // BundleLinkComponent 1383 case -511251360: 1384 /* fullUrl */ return this.fullUrl == null ? new Base[0] : new Base[] { this.fullUrl }; // UriType 1385 case -341064690: 1386 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // Resource 1387 case -906336856: 1388 /* search */ return this.search == null ? new Base[0] : new Base[] { this.search }; // BundleEntrySearchComponent 1389 case 1095692943: 1390 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // BundleEntryRequestComponent 1391 case -340323263: 1392 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // BundleEntryResponseComponent 1393 default: 1394 return super.getProperty(hash, name, checkValid); 1395 } 1396 1397 } 1398 1399 @Override 1400 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1401 switch (hash) { 1402 case 3321850: // link 1403 this.getLink().add((BundleLinkComponent) value); // BundleLinkComponent 1404 return value; 1405 case -511251360: // fullUrl 1406 this.fullUrl = castToUri(value); // UriType 1407 return value; 1408 case -341064690: // resource 1409 this.resource = castToResource(value); // Resource 1410 return value; 1411 case -906336856: // search 1412 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 1413 return value; 1414 case 1095692943: // request 1415 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 1416 return value; 1417 case -340323263: // response 1418 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 1419 return value; 1420 default: 1421 return super.setProperty(hash, name, value); 1422 } 1423 1424 } 1425 1426 @Override 1427 public Base setProperty(String name, Base value) throws FHIRException { 1428 if (name.equals("link")) { 1429 this.getLink().add((BundleLinkComponent) value); 1430 } else if (name.equals("fullUrl")) { 1431 this.fullUrl = castToUri(value); // UriType 1432 } else if (name.equals("resource")) { 1433 this.resource = castToResource(value); // Resource 1434 } else if (name.equals("search")) { 1435 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 1436 } else if (name.equals("request")) { 1437 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 1438 } else if (name.equals("response")) { 1439 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 1440 } else 1441 return super.setProperty(name, value); 1442 return value; 1443 } 1444 1445 @Override 1446 public void removeChild(String name, Base value) throws FHIRException { 1447 if (name.equals("link")) { 1448 this.getLink().remove((BundleLinkComponent) value); 1449 } else if (name.equals("fullUrl")) { 1450 this.fullUrl = null; 1451 } else if (name.equals("resource")) { 1452 this.resource = null; 1453 } else if (name.equals("search")) { 1454 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 1455 } else if (name.equals("request")) { 1456 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 1457 } else if (name.equals("response")) { 1458 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 1459 } else 1460 super.removeChild(name, value); 1461 1462 } 1463 1464 @Override 1465 public Base makeProperty(int hash, String name) throws FHIRException { 1466 switch (hash) { 1467 case 3321850: 1468 return addLink(); 1469 case -511251360: 1470 return getFullUrlElement(); 1471 case -341064690: 1472 throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 1473 case -906336856: 1474 return getSearch(); 1475 case 1095692943: 1476 return getRequest(); 1477 case -340323263: 1478 return getResponse(); 1479 default: 1480 return super.makeProperty(hash, name); 1481 } 1482 1483 } 1484 1485 @Override 1486 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1487 switch (hash) { 1488 case 3321850: 1489 /* link */ return new String[] { "@Bundle.link" }; 1490 case -511251360: 1491 /* fullUrl */ return new String[] { "uri" }; 1492 case -341064690: 1493 /* resource */ return new String[] { "Resource" }; 1494 case -906336856: 1495 /* search */ return new String[] {}; 1496 case 1095692943: 1497 /* request */ return new String[] {}; 1498 case -340323263: 1499 /* response */ return new String[] {}; 1500 default: 1501 return super.getTypesForProperty(hash, name); 1502 } 1503 1504 } 1505 1506 @Override 1507 public Base addChild(String name) throws FHIRException { 1508 if (name.equals("link")) { 1509 return addLink(); 1510 } else if (name.equals("fullUrl")) { 1511 throw new FHIRException("Cannot call addChild on a singleton property Bundle.fullUrl"); 1512 } else if (name.equals("resource")) { 1513 throw new FHIRException("Cannot call addChild on an abstract type Bundle.resource"); 1514 } else if (name.equals("search")) { 1515 this.search = new BundleEntrySearchComponent(); 1516 return this.search; 1517 } else if (name.equals("request")) { 1518 this.request = new BundleEntryRequestComponent(); 1519 return this.request; 1520 } else if (name.equals("response")) { 1521 this.response = new BundleEntryResponseComponent(); 1522 return this.response; 1523 } else 1524 return super.addChild(name); 1525 } 1526 1527 public BundleEntryComponent copy() { 1528 BundleEntryComponent dst = new BundleEntryComponent(); 1529 copyValues(dst); 1530 return dst; 1531 } 1532 1533 public void copyValues(BundleEntryComponent dst) { 1534 super.copyValues(dst); 1535 if (link != null) { 1536 dst.link = new ArrayList<BundleLinkComponent>(); 1537 for (BundleLinkComponent i : link) 1538 dst.link.add(i.copy()); 1539 } 1540 ; 1541 dst.fullUrl = fullUrl == null ? null : fullUrl.copy(); 1542 dst.resource = resource == null ? null : resource.copy(); 1543 dst.search = search == null ? null : search.copy(); 1544 dst.request = request == null ? null : request.copy(); 1545 dst.response = response == null ? null : response.copy(); 1546 } 1547 1548 @Override 1549 public boolean equalsDeep(Base other_) { 1550 if (!super.equalsDeep(other_)) 1551 return false; 1552 if (!(other_ instanceof BundleEntryComponent)) 1553 return false; 1554 BundleEntryComponent o = (BundleEntryComponent) other_; 1555 return compareDeep(link, o.link, true) && compareDeep(fullUrl, o.fullUrl, true) 1556 && compareDeep(resource, o.resource, true) && compareDeep(search, o.search, true) 1557 && compareDeep(request, o.request, true) && compareDeep(response, o.response, true); 1558 } 1559 1560 @Override 1561 public boolean equalsShallow(Base other_) { 1562 if (!super.equalsShallow(other_)) 1563 return false; 1564 if (!(other_ instanceof BundleEntryComponent)) 1565 return false; 1566 BundleEntryComponent o = (BundleEntryComponent) other_; 1567 return compareValues(fullUrl, o.fullUrl, true); 1568 } 1569 1570 public boolean isEmpty() { 1571 return super.isEmpty() 1572 && ca.uhn.fhir.util.ElementUtil.isEmpty(link, fullUrl, resource, search, request, response); 1573 } 1574 1575 public String fhirType() { 1576 return "Bundle.entry"; 1577 1578 } 1579 1580 } 1581 1582 @Block() 1583 public static class BundleEntrySearchComponent extends BackboneElement implements IBaseBackboneElement { 1584 /** 1585 * Why this entry is in the result set - whether it's included as a match or 1586 * because of an _include requirement, or to convey information or warning 1587 * information about the search process. 1588 */ 1589 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1590 @Description(shortDefinition = "match | include | outcome - why this is in the result set", formalDefinition = "Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.") 1591 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-entry-mode") 1592 protected Enumeration<SearchEntryMode> mode; 1593 1594 /** 1595 * When searching, the server's search ranking score for the entry. 1596 */ 1597 @Child(name = "score", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1598 @Description(shortDefinition = "Search ranking (between 0 and 1)", formalDefinition = "When searching, the server's search ranking score for the entry.") 1599 protected DecimalType score; 1600 1601 private static final long serialVersionUID = 837739866L; 1602 1603 /** 1604 * Constructor 1605 */ 1606 public BundleEntrySearchComponent() { 1607 super(); 1608 } 1609 1610 /** 1611 * @return {@link #mode} (Why this entry is in the result set - whether it's 1612 * included as a match or because of an _include requirement, or to 1613 * convey information or warning information about the search process.). 1614 * This is the underlying object with id, value and extensions. The 1615 * accessor "getMode" gives direct access to the value 1616 */ 1617 public Enumeration<SearchEntryMode> getModeElement() { 1618 if (this.mode == null) 1619 if (Configuration.errorOnAutoCreate()) 1620 throw new Error("Attempt to auto-create BundleEntrySearchComponent.mode"); 1621 else if (Configuration.doAutoCreate()) 1622 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); // bb 1623 return this.mode; 1624 } 1625 1626 public boolean hasModeElement() { 1627 return this.mode != null && !this.mode.isEmpty(); 1628 } 1629 1630 public boolean hasMode() { 1631 return this.mode != null && !this.mode.isEmpty(); 1632 } 1633 1634 /** 1635 * @param value {@link #mode} (Why this entry is in the result set - whether 1636 * it's included as a match or because of an _include requirement, 1637 * or to convey information or warning information about the search 1638 * process.). This is the underlying object with id, value and 1639 * extensions. The accessor "getMode" gives direct access to the 1640 * value 1641 */ 1642 public BundleEntrySearchComponent setModeElement(Enumeration<SearchEntryMode> value) { 1643 this.mode = value; 1644 return this; 1645 } 1646 1647 /** 1648 * @return Why this entry is in the result set - whether it's included as a 1649 * match or because of an _include requirement, or to convey information 1650 * or warning information about the search process. 1651 */ 1652 public SearchEntryMode getMode() { 1653 return this.mode == null ? null : this.mode.getValue(); 1654 } 1655 1656 /** 1657 * @param value Why this entry is in the result set - whether it's included as a 1658 * match or because of an _include requirement, or to convey 1659 * information or warning information about the search process. 1660 */ 1661 public BundleEntrySearchComponent setMode(SearchEntryMode value) { 1662 if (value == null) 1663 this.mode = null; 1664 else { 1665 if (this.mode == null) 1666 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); 1667 this.mode.setValue(value); 1668 } 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #score} (When searching, the server's search ranking score for 1674 * the entry.). This is the underlying object with id, value and 1675 * extensions. The accessor "getScore" gives direct access to the value 1676 */ 1677 public DecimalType getScoreElement() { 1678 if (this.score == null) 1679 if (Configuration.errorOnAutoCreate()) 1680 throw new Error("Attempt to auto-create BundleEntrySearchComponent.score"); 1681 else if (Configuration.doAutoCreate()) 1682 this.score = new DecimalType(); // bb 1683 return this.score; 1684 } 1685 1686 public boolean hasScoreElement() { 1687 return this.score != null && !this.score.isEmpty(); 1688 } 1689 1690 public boolean hasScore() { 1691 return this.score != null && !this.score.isEmpty(); 1692 } 1693 1694 /** 1695 * @param value {@link #score} (When searching, the server's search ranking 1696 * score for the entry.). This is the underlying object with id, 1697 * value and extensions. The accessor "getScore" gives direct 1698 * access to the value 1699 */ 1700 public BundleEntrySearchComponent setScoreElement(DecimalType value) { 1701 this.score = value; 1702 return this; 1703 } 1704 1705 /** 1706 * @return When searching, the server's search ranking score for the entry. 1707 */ 1708 public BigDecimal getScore() { 1709 return this.score == null ? null : this.score.getValue(); 1710 } 1711 1712 /** 1713 * @param value When searching, the server's search ranking score for the entry. 1714 */ 1715 public BundleEntrySearchComponent setScore(BigDecimal value) { 1716 if (value == null) 1717 this.score = null; 1718 else { 1719 if (this.score == null) 1720 this.score = new DecimalType(); 1721 this.score.setValue(value); 1722 } 1723 return this; 1724 } 1725 1726 /** 1727 * @param value When searching, the server's search ranking score for the entry. 1728 */ 1729 public BundleEntrySearchComponent setScore(long value) { 1730 this.score = new DecimalType(); 1731 this.score.setValue(value); 1732 return this; 1733 } 1734 1735 /** 1736 * @param value When searching, the server's search ranking score for the entry. 1737 */ 1738 public BundleEntrySearchComponent setScore(double value) { 1739 this.score = new DecimalType(); 1740 this.score.setValue(value); 1741 return this; 1742 } 1743 1744 protected void listChildren(List<Property> children) { 1745 super.listChildren(children); 1746 children.add(new Property("mode", "code", 1747 "Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.", 1748 0, 1, mode)); 1749 children.add(new Property("score", "decimal", "When searching, the server's search ranking score for the entry.", 1750 0, 1, score)); 1751 } 1752 1753 @Override 1754 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1755 switch (_hash) { 1756 case 3357091: 1757 /* mode */ return new Property("mode", "code", 1758 "Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.", 1759 0, 1, mode); 1760 case 109264530: 1761 /* score */ return new Property("score", "decimal", 1762 "When searching, the server's search ranking score for the entry.", 0, 1, score); 1763 default: 1764 return super.getNamedProperty(_hash, _name, _checkValid); 1765 } 1766 1767 } 1768 1769 @Override 1770 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1771 switch (hash) { 1772 case 3357091: 1773 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<SearchEntryMode> 1774 case 109264530: 1775 /* score */ return this.score == null ? new Base[0] : new Base[] { this.score }; // DecimalType 1776 default: 1777 return super.getProperty(hash, name, checkValid); 1778 } 1779 1780 } 1781 1782 @Override 1783 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1784 switch (hash) { 1785 case 3357091: // mode 1786 value = new SearchEntryModeEnumFactory().fromType(castToCode(value)); 1787 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 1788 return value; 1789 case 109264530: // score 1790 this.score = castToDecimal(value); // DecimalType 1791 return value; 1792 default: 1793 return super.setProperty(hash, name, value); 1794 } 1795 1796 } 1797 1798 @Override 1799 public Base setProperty(String name, Base value) throws FHIRException { 1800 if (name.equals("mode")) { 1801 value = new SearchEntryModeEnumFactory().fromType(castToCode(value)); 1802 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 1803 } else if (name.equals("score")) { 1804 this.score = castToDecimal(value); // DecimalType 1805 } else 1806 return super.setProperty(name, value); 1807 return value; 1808 } 1809 1810 @Override 1811 public void removeChild(String name, Base value) throws FHIRException { 1812 if (name.equals("mode")) { 1813 this.mode = null; 1814 } else if (name.equals("score")) { 1815 this.score = null; 1816 } else 1817 super.removeChild(name, value); 1818 1819 } 1820 1821 @Override 1822 public Base makeProperty(int hash, String name) throws FHIRException { 1823 switch (hash) { 1824 case 3357091: 1825 return getModeElement(); 1826 case 109264530: 1827 return getScoreElement(); 1828 default: 1829 return super.makeProperty(hash, name); 1830 } 1831 1832 } 1833 1834 @Override 1835 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1836 switch (hash) { 1837 case 3357091: 1838 /* mode */ return new String[] { "code" }; 1839 case 109264530: 1840 /* score */ return new String[] { "decimal" }; 1841 default: 1842 return super.getTypesForProperty(hash, name); 1843 } 1844 1845 } 1846 1847 @Override 1848 public Base addChild(String name) throws FHIRException { 1849 if (name.equals("mode")) { 1850 throw new FHIRException("Cannot call addChild on a singleton property Bundle.mode"); 1851 } else if (name.equals("score")) { 1852 throw new FHIRException("Cannot call addChild on a singleton property Bundle.score"); 1853 } else 1854 return super.addChild(name); 1855 } 1856 1857 public BundleEntrySearchComponent copy() { 1858 BundleEntrySearchComponent dst = new BundleEntrySearchComponent(); 1859 copyValues(dst); 1860 return dst; 1861 } 1862 1863 public void copyValues(BundleEntrySearchComponent dst) { 1864 super.copyValues(dst); 1865 dst.mode = mode == null ? null : mode.copy(); 1866 dst.score = score == null ? null : score.copy(); 1867 } 1868 1869 @Override 1870 public boolean equalsDeep(Base other_) { 1871 if (!super.equalsDeep(other_)) 1872 return false; 1873 if (!(other_ instanceof BundleEntrySearchComponent)) 1874 return false; 1875 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other_; 1876 return compareDeep(mode, o.mode, true) && compareDeep(score, o.score, true); 1877 } 1878 1879 @Override 1880 public boolean equalsShallow(Base other_) { 1881 if (!super.equalsShallow(other_)) 1882 return false; 1883 if (!(other_ instanceof BundleEntrySearchComponent)) 1884 return false; 1885 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other_; 1886 return compareValues(mode, o.mode, true) && compareValues(score, o.score, true); 1887 } 1888 1889 public boolean isEmpty() { 1890 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, score); 1891 } 1892 1893 public String fhirType() { 1894 return "Bundle.entry.search"; 1895 1896 } 1897 1898 } 1899 1900 @Block() 1901 public static class BundleEntryRequestComponent extends BackboneElement implements IBaseBackboneElement { 1902 /** 1903 * In a transaction or batch, this is the HTTP action to be executed for this 1904 * entry. In a history bundle, this indicates the HTTP action that occurred. 1905 */ 1906 @Child(name = "method", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1907 @Description(shortDefinition = "GET | HEAD | POST | PUT | DELETE | PATCH", formalDefinition = "In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.") 1908 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/http-verb") 1909 protected Enumeration<HTTPVerb> method; 1910 1911 /** 1912 * The URL for this entry, relative to the root (the address to which the 1913 * request is posted). 1914 */ 1915 @Child(name = "url", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1916 @Description(shortDefinition = "URL for HTTP equivalent of this entry", formalDefinition = "The URL for this entry, relative to the root (the address to which the request is posted).") 1917 protected UriType url; 1918 1919 /** 1920 * If the ETag values match, return a 304 Not Modified status. See the API 1921 * documentation for ["Conditional Read"](http.html#cread). 1922 */ 1923 @Child(name = "ifNoneMatch", type = { 1924 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1925 @Description(shortDefinition = "For managing cache currency", formalDefinition = "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).") 1926 protected StringType ifNoneMatch; 1927 1928 /** 1929 * Only perform the operation if the last updated date matches. See the API 1930 * documentation for ["Conditional Read"](http.html#cread). 1931 */ 1932 @Child(name = "ifModifiedSince", type = { 1933 InstantType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1934 @Description(shortDefinition = "For managing cache currency", formalDefinition = "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).") 1935 protected InstantType ifModifiedSince; 1936 1937 /** 1938 * Only perform the operation if the Etag value matches. For more information, 1939 * see the API section ["Managing Resource Contention"](http.html#concurrency). 1940 */ 1941 @Child(name = "ifMatch", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1942 @Description(shortDefinition = "For managing update contention", formalDefinition = "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).") 1943 protected StringType ifMatch; 1944 1945 /** 1946 * Instruct the server not to perform the create if a specified resource already 1947 * exists. For further information, see the API documentation for ["Conditional 1948 * Create"](http.html#ccreate). This is just the query portion of the URL - what 1949 * follows the "?" (not including the "?"). 1950 */ 1951 @Child(name = "ifNoneExist", type = { 1952 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1953 @Description(shortDefinition = "For conditional creates", formalDefinition = "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").") 1954 protected StringType ifNoneExist; 1955 1956 private static final long serialVersionUID = -1349769744L; 1957 1958 /** 1959 * Constructor 1960 */ 1961 public BundleEntryRequestComponent() { 1962 super(); 1963 } 1964 1965 /** 1966 * Constructor 1967 */ 1968 public BundleEntryRequestComponent(Enumeration<HTTPVerb> method, UriType url) { 1969 super(); 1970 this.method = method; 1971 this.url = url; 1972 } 1973 1974 /** 1975 * @return {@link #method} (In a transaction or batch, this is the HTTP action 1976 * to be executed for this entry. In a history bundle, this indicates 1977 * the HTTP action that occurred.). This is the underlying object with 1978 * id, value and extensions. The accessor "getMethod" gives direct 1979 * access to the value 1980 */ 1981 public Enumeration<HTTPVerb> getMethodElement() { 1982 if (this.method == null) 1983 if (Configuration.errorOnAutoCreate()) 1984 throw new Error("Attempt to auto-create BundleEntryRequestComponent.method"); 1985 else if (Configuration.doAutoCreate()) 1986 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); // bb 1987 return this.method; 1988 } 1989 1990 public boolean hasMethodElement() { 1991 return this.method != null && !this.method.isEmpty(); 1992 } 1993 1994 public boolean hasMethod() { 1995 return this.method != null && !this.method.isEmpty(); 1996 } 1997 1998 /** 1999 * @param value {@link #method} (In a transaction or batch, this is the HTTP 2000 * action to be executed for this entry. In a history bundle, this 2001 * indicates the HTTP action that occurred.). This is the 2002 * underlying object with id, value and extensions. The accessor 2003 * "getMethod" gives direct access to the value 2004 */ 2005 public BundleEntryRequestComponent setMethodElement(Enumeration<HTTPVerb> value) { 2006 this.method = value; 2007 return this; 2008 } 2009 2010 /** 2011 * @return In a transaction or batch, this is the HTTP action to be executed for 2012 * this entry. In a history bundle, this indicates the HTTP action that 2013 * occurred. 2014 */ 2015 public HTTPVerb getMethod() { 2016 return this.method == null ? null : this.method.getValue(); 2017 } 2018 2019 /** 2020 * @param value In a transaction or batch, this is the HTTP action to be 2021 * executed for this entry. In a history bundle, this indicates the 2022 * HTTP action that occurred. 2023 */ 2024 public BundleEntryRequestComponent setMethod(HTTPVerb value) { 2025 if (this.method == null) 2026 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); 2027 this.method.setValue(value); 2028 return this; 2029 } 2030 2031 /** 2032 * @return {@link #url} (The URL for this entry, relative to the root (the 2033 * address to which the request is posted).). This is the underlying 2034 * object with id, value and extensions. The accessor "getUrl" gives 2035 * direct access to the value 2036 */ 2037 public UriType getUrlElement() { 2038 if (this.url == null) 2039 if (Configuration.errorOnAutoCreate()) 2040 throw new Error("Attempt to auto-create BundleEntryRequestComponent.url"); 2041 else if (Configuration.doAutoCreate()) 2042 this.url = new UriType(); // bb 2043 return this.url; 2044 } 2045 2046 public boolean hasUrlElement() { 2047 return this.url != null && !this.url.isEmpty(); 2048 } 2049 2050 public boolean hasUrl() { 2051 return this.url != null && !this.url.isEmpty(); 2052 } 2053 2054 /** 2055 * @param value {@link #url} (The URL for this entry, relative to the root (the 2056 * address to which the request is posted).). This is the 2057 * underlying object with id, value and extensions. The accessor 2058 * "getUrl" gives direct access to the value 2059 */ 2060 public BundleEntryRequestComponent setUrlElement(UriType value) { 2061 this.url = value; 2062 return this; 2063 } 2064 2065 /** 2066 * @return The URL for this entry, relative to the root (the address to which 2067 * the request is posted). 2068 */ 2069 public String getUrl() { 2070 return this.url == null ? null : this.url.getValue(); 2071 } 2072 2073 /** 2074 * @param value The URL for this entry, relative to the root (the address to 2075 * which the request is posted). 2076 */ 2077 public BundleEntryRequestComponent setUrl(String value) { 2078 if (this.url == null) 2079 this.url = new UriType(); 2080 this.url.setValue(value); 2081 return this; 2082 } 2083 2084 /** 2085 * @return {@link #ifNoneMatch} (If the ETag values match, return a 304 Not 2086 * Modified status. See the API documentation for ["Conditional 2087 * Read"](http.html#cread).). This is the underlying object with id, 2088 * value and extensions. The accessor "getIfNoneMatch" gives direct 2089 * access to the value 2090 */ 2091 public StringType getIfNoneMatchElement() { 2092 if (this.ifNoneMatch == null) 2093 if (Configuration.errorOnAutoCreate()) 2094 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneMatch"); 2095 else if (Configuration.doAutoCreate()) 2096 this.ifNoneMatch = new StringType(); // bb 2097 return this.ifNoneMatch; 2098 } 2099 2100 public boolean hasIfNoneMatchElement() { 2101 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 2102 } 2103 2104 public boolean hasIfNoneMatch() { 2105 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 2106 } 2107 2108 /** 2109 * @param value {@link #ifNoneMatch} (If the ETag values match, return a 304 Not 2110 * Modified status. See the API documentation for ["Conditional 2111 * Read"](http.html#cread).). This is the underlying object with 2112 * id, value and extensions. The accessor "getIfNoneMatch" gives 2113 * direct access to the value 2114 */ 2115 public BundleEntryRequestComponent setIfNoneMatchElement(StringType value) { 2116 this.ifNoneMatch = value; 2117 return this; 2118 } 2119 2120 /** 2121 * @return If the ETag values match, return a 304 Not Modified status. See the 2122 * API documentation for ["Conditional Read"](http.html#cread). 2123 */ 2124 public String getIfNoneMatch() { 2125 return this.ifNoneMatch == null ? null : this.ifNoneMatch.getValue(); 2126 } 2127 2128 /** 2129 * @param value If the ETag values match, return a 304 Not Modified status. See 2130 * the API documentation for ["Conditional Read"](http.html#cread). 2131 */ 2132 public BundleEntryRequestComponent setIfNoneMatch(String value) { 2133 if (Utilities.noString(value)) 2134 this.ifNoneMatch = null; 2135 else { 2136 if (this.ifNoneMatch == null) 2137 this.ifNoneMatch = new StringType(); 2138 this.ifNoneMatch.setValue(value); 2139 } 2140 return this; 2141 } 2142 2143 /** 2144 * @return {@link #ifModifiedSince} (Only perform the operation if the last 2145 * updated date matches. See the API documentation for ["Conditional 2146 * Read"](http.html#cread).). This is the underlying object with id, 2147 * value and extensions. The accessor "getIfModifiedSince" gives direct 2148 * access to the value 2149 */ 2150 public InstantType getIfModifiedSinceElement() { 2151 if (this.ifModifiedSince == null) 2152 if (Configuration.errorOnAutoCreate()) 2153 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifModifiedSince"); 2154 else if (Configuration.doAutoCreate()) 2155 this.ifModifiedSince = new InstantType(); // bb 2156 return this.ifModifiedSince; 2157 } 2158 2159 public boolean hasIfModifiedSinceElement() { 2160 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 2161 } 2162 2163 public boolean hasIfModifiedSince() { 2164 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 2165 } 2166 2167 /** 2168 * @param value {@link #ifModifiedSince} (Only perform the operation if the last 2169 * updated date matches. See the API documentation for 2170 * ["Conditional Read"](http.html#cread).). This is the underlying 2171 * object with id, value and extensions. The accessor 2172 * "getIfModifiedSince" gives direct access to the value 2173 */ 2174 public BundleEntryRequestComponent setIfModifiedSinceElement(InstantType value) { 2175 this.ifModifiedSince = value; 2176 return this; 2177 } 2178 2179 /** 2180 * @return Only perform the operation if the last updated date matches. See the 2181 * API documentation for ["Conditional Read"](http.html#cread). 2182 */ 2183 public Date getIfModifiedSince() { 2184 return this.ifModifiedSince == null ? null : this.ifModifiedSince.getValue(); 2185 } 2186 2187 /** 2188 * @param value Only perform the operation if the last updated date matches. See 2189 * the API documentation for ["Conditional Read"](http.html#cread). 2190 */ 2191 public BundleEntryRequestComponent setIfModifiedSince(Date value) { 2192 if (value == null) 2193 this.ifModifiedSince = null; 2194 else { 2195 if (this.ifModifiedSince == null) 2196 this.ifModifiedSince = new InstantType(); 2197 this.ifModifiedSince.setValue(value); 2198 } 2199 return this; 2200 } 2201 2202 /** 2203 * @return {@link #ifMatch} (Only perform the operation if the Etag value 2204 * matches. For more information, see the API section ["Managing 2205 * Resource Contention"](http.html#concurrency).). This is the 2206 * underlying object with id, value and extensions. The accessor 2207 * "getIfMatch" gives direct access to the value 2208 */ 2209 public StringType getIfMatchElement() { 2210 if (this.ifMatch == null) 2211 if (Configuration.errorOnAutoCreate()) 2212 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifMatch"); 2213 else if (Configuration.doAutoCreate()) 2214 this.ifMatch = new StringType(); // bb 2215 return this.ifMatch; 2216 } 2217 2218 public boolean hasIfMatchElement() { 2219 return this.ifMatch != null && !this.ifMatch.isEmpty(); 2220 } 2221 2222 public boolean hasIfMatch() { 2223 return this.ifMatch != null && !this.ifMatch.isEmpty(); 2224 } 2225 2226 /** 2227 * @param value {@link #ifMatch} (Only perform the operation if the Etag value 2228 * matches. For more information, see the API section ["Managing 2229 * Resource Contention"](http.html#concurrency).). This is the 2230 * underlying object with id, value and extensions. The accessor 2231 * "getIfMatch" gives direct access to the value 2232 */ 2233 public BundleEntryRequestComponent setIfMatchElement(StringType value) { 2234 this.ifMatch = value; 2235 return this; 2236 } 2237 2238 /** 2239 * @return Only perform the operation if the Etag value matches. For more 2240 * information, see the API section ["Managing Resource 2241 * Contention"](http.html#concurrency). 2242 */ 2243 public String getIfMatch() { 2244 return this.ifMatch == null ? null : this.ifMatch.getValue(); 2245 } 2246 2247 /** 2248 * @param value Only perform the operation if the Etag value matches. For more 2249 * information, see the API section ["Managing Resource 2250 * Contention"](http.html#concurrency). 2251 */ 2252 public BundleEntryRequestComponent setIfMatch(String value) { 2253 if (Utilities.noString(value)) 2254 this.ifMatch = null; 2255 else { 2256 if (this.ifMatch == null) 2257 this.ifMatch = new StringType(); 2258 this.ifMatch.setValue(value); 2259 } 2260 return this; 2261 } 2262 2263 /** 2264 * @return {@link #ifNoneExist} (Instruct the server not to perform the create 2265 * if a specified resource already exists. For further information, see 2266 * the API documentation for ["Conditional Create"](http.html#ccreate). 2267 * This is just the query portion of the URL - what follows the "?" (not 2268 * including the "?").). This is the underlying object with id, value 2269 * and extensions. The accessor "getIfNoneExist" gives direct access to 2270 * the value 2271 */ 2272 public StringType getIfNoneExistElement() { 2273 if (this.ifNoneExist == null) 2274 if (Configuration.errorOnAutoCreate()) 2275 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneExist"); 2276 else if (Configuration.doAutoCreate()) 2277 this.ifNoneExist = new StringType(); // bb 2278 return this.ifNoneExist; 2279 } 2280 2281 public boolean hasIfNoneExistElement() { 2282 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 2283 } 2284 2285 public boolean hasIfNoneExist() { 2286 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 2287 } 2288 2289 /** 2290 * @param value {@link #ifNoneExist} (Instruct the server not to perform the 2291 * create if a specified resource already exists. For further 2292 * information, see the API documentation for ["Conditional 2293 * Create"](http.html#ccreate). This is just the query portion of 2294 * the URL - what follows the "?" (not including the "?").). This 2295 * is the underlying object with id, value and extensions. The 2296 * accessor "getIfNoneExist" gives direct access to the value 2297 */ 2298 public BundleEntryRequestComponent setIfNoneExistElement(StringType value) { 2299 this.ifNoneExist = value; 2300 return this; 2301 } 2302 2303 /** 2304 * @return Instruct the server not to perform the create if a specified resource 2305 * already exists. For further information, see the API documentation 2306 * for ["Conditional Create"](http.html#ccreate). This is just the query 2307 * portion of the URL - what follows the "?" (not including the "?"). 2308 */ 2309 public String getIfNoneExist() { 2310 return this.ifNoneExist == null ? null : this.ifNoneExist.getValue(); 2311 } 2312 2313 /** 2314 * @param value Instruct the server not to perform the create if a specified 2315 * resource already exists. For further information, see the API 2316 * documentation for ["Conditional Create"](http.html#ccreate). 2317 * This is just the query portion of the URL - what follows the "?" 2318 * (not including the "?"). 2319 */ 2320 public BundleEntryRequestComponent setIfNoneExist(String value) { 2321 if (Utilities.noString(value)) 2322 this.ifNoneExist = null; 2323 else { 2324 if (this.ifNoneExist == null) 2325 this.ifNoneExist = new StringType(); 2326 this.ifNoneExist.setValue(value); 2327 } 2328 return this; 2329 } 2330 2331 protected void listChildren(List<Property> children) { 2332 super.listChildren(children); 2333 children.add(new Property("method", "code", 2334 "In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.", 2335 0, 1, method)); 2336 children.add(new Property("url", "uri", 2337 "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1, url)); 2338 children.add(new Property("ifNoneMatch", "string", 2339 "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 2340 0, 1, ifNoneMatch)); 2341 children.add(new Property("ifModifiedSince", "instant", 2342 "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 2343 0, 1, ifModifiedSince)); 2344 children.add(new Property("ifMatch", "string", 2345 "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 2346 0, 1, ifMatch)); 2347 children.add(new Property("ifNoneExist", "string", 2348 "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 2349 0, 1, ifNoneExist)); 2350 } 2351 2352 @Override 2353 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2354 switch (_hash) { 2355 case -1077554975: 2356 /* method */ return new Property("method", "code", 2357 "In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.", 2358 0, 1, method); 2359 case 116079: 2360 /* url */ return new Property("url", "uri", 2361 "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1, url); 2362 case 171868368: 2363 /* ifNoneMatch */ return new Property("ifNoneMatch", "string", 2364 "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 2365 0, 1, ifNoneMatch); 2366 case -2061602860: 2367 /* ifModifiedSince */ return new Property("ifModifiedSince", "instant", 2368 "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 2369 0, 1, ifModifiedSince); 2370 case 1692894888: 2371 /* ifMatch */ return new Property("ifMatch", "string", 2372 "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 2373 0, 1, ifMatch); 2374 case 165155330: 2375 /* ifNoneExist */ return new Property("ifNoneExist", "string", 2376 "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 2377 0, 1, ifNoneExist); 2378 default: 2379 return super.getNamedProperty(_hash, _name, _checkValid); 2380 } 2381 2382 } 2383 2384 @Override 2385 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2386 switch (hash) { 2387 case -1077554975: 2388 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // Enumeration<HTTPVerb> 2389 case 116079: 2390 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2391 case 171868368: 2392 /* ifNoneMatch */ return this.ifNoneMatch == null ? new Base[0] : new Base[] { this.ifNoneMatch }; // StringType 2393 case -2061602860: 2394 /* ifModifiedSince */ return this.ifModifiedSince == null ? new Base[0] : new Base[] { this.ifModifiedSince }; // InstantType 2395 case 1692894888: 2396 /* ifMatch */ return this.ifMatch == null ? new Base[0] : new Base[] { this.ifMatch }; // StringType 2397 case 165155330: 2398 /* ifNoneExist */ return this.ifNoneExist == null ? new Base[0] : new Base[] { this.ifNoneExist }; // StringType 2399 default: 2400 return super.getProperty(hash, name, checkValid); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2407 switch (hash) { 2408 case -1077554975: // method 2409 value = new HTTPVerbEnumFactory().fromType(castToCode(value)); 2410 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 2411 return value; 2412 case 116079: // url 2413 this.url = castToUri(value); // UriType 2414 return value; 2415 case 171868368: // ifNoneMatch 2416 this.ifNoneMatch = castToString(value); // StringType 2417 return value; 2418 case -2061602860: // ifModifiedSince 2419 this.ifModifiedSince = castToInstant(value); // InstantType 2420 return value; 2421 case 1692894888: // ifMatch 2422 this.ifMatch = castToString(value); // StringType 2423 return value; 2424 case 165155330: // ifNoneExist 2425 this.ifNoneExist = castToString(value); // StringType 2426 return value; 2427 default: 2428 return super.setProperty(hash, name, value); 2429 } 2430 2431 } 2432 2433 @Override 2434 public Base setProperty(String name, Base value) throws FHIRException { 2435 if (name.equals("method")) { 2436 value = new HTTPVerbEnumFactory().fromType(castToCode(value)); 2437 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 2438 } else if (name.equals("url")) { 2439 this.url = castToUri(value); // UriType 2440 } else if (name.equals("ifNoneMatch")) { 2441 this.ifNoneMatch = castToString(value); // StringType 2442 } else if (name.equals("ifModifiedSince")) { 2443 this.ifModifiedSince = castToInstant(value); // InstantType 2444 } else if (name.equals("ifMatch")) { 2445 this.ifMatch = castToString(value); // StringType 2446 } else if (name.equals("ifNoneExist")) { 2447 this.ifNoneExist = castToString(value); // StringType 2448 } else 2449 return super.setProperty(name, value); 2450 return value; 2451 } 2452 2453 @Override 2454 public void removeChild(String name, Base value) throws FHIRException { 2455 if (name.equals("method")) { 2456 this.method = null; 2457 } else if (name.equals("url")) { 2458 this.url = null; 2459 } else if (name.equals("ifNoneMatch")) { 2460 this.ifNoneMatch = null; 2461 } else if (name.equals("ifModifiedSince")) { 2462 this.ifModifiedSince = null; 2463 } else if (name.equals("ifMatch")) { 2464 this.ifMatch = null; 2465 } else if (name.equals("ifNoneExist")) { 2466 this.ifNoneExist = null; 2467 } else 2468 super.removeChild(name, value); 2469 2470 } 2471 2472 @Override 2473 public Base makeProperty(int hash, String name) throws FHIRException { 2474 switch (hash) { 2475 case -1077554975: 2476 return getMethodElement(); 2477 case 116079: 2478 return getUrlElement(); 2479 case 171868368: 2480 return getIfNoneMatchElement(); 2481 case -2061602860: 2482 return getIfModifiedSinceElement(); 2483 case 1692894888: 2484 return getIfMatchElement(); 2485 case 165155330: 2486 return getIfNoneExistElement(); 2487 default: 2488 return super.makeProperty(hash, name); 2489 } 2490 2491 } 2492 2493 @Override 2494 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2495 switch (hash) { 2496 case -1077554975: 2497 /* method */ return new String[] { "code" }; 2498 case 116079: 2499 /* url */ return new String[] { "uri" }; 2500 case 171868368: 2501 /* ifNoneMatch */ return new String[] { "string" }; 2502 case -2061602860: 2503 /* ifModifiedSince */ return new String[] { "instant" }; 2504 case 1692894888: 2505 /* ifMatch */ return new String[] { "string" }; 2506 case 165155330: 2507 /* ifNoneExist */ return new String[] { "string" }; 2508 default: 2509 return super.getTypesForProperty(hash, name); 2510 } 2511 2512 } 2513 2514 @Override 2515 public Base addChild(String name) throws FHIRException { 2516 if (name.equals("method")) { 2517 throw new FHIRException("Cannot call addChild on a singleton property Bundle.method"); 2518 } else if (name.equals("url")) { 2519 throw new FHIRException("Cannot call addChild on a singleton property Bundle.url"); 2520 } else if (name.equals("ifNoneMatch")) { 2521 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifNoneMatch"); 2522 } else if (name.equals("ifModifiedSince")) { 2523 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifModifiedSince"); 2524 } else if (name.equals("ifMatch")) { 2525 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifMatch"); 2526 } else if (name.equals("ifNoneExist")) { 2527 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifNoneExist"); 2528 } else 2529 return super.addChild(name); 2530 } 2531 2532 public BundleEntryRequestComponent copy() { 2533 BundleEntryRequestComponent dst = new BundleEntryRequestComponent(); 2534 copyValues(dst); 2535 return dst; 2536 } 2537 2538 public void copyValues(BundleEntryRequestComponent dst) { 2539 super.copyValues(dst); 2540 dst.method = method == null ? null : method.copy(); 2541 dst.url = url == null ? null : url.copy(); 2542 dst.ifNoneMatch = ifNoneMatch == null ? null : ifNoneMatch.copy(); 2543 dst.ifModifiedSince = ifModifiedSince == null ? null : ifModifiedSince.copy(); 2544 dst.ifMatch = ifMatch == null ? null : ifMatch.copy(); 2545 dst.ifNoneExist = ifNoneExist == null ? null : ifNoneExist.copy(); 2546 } 2547 2548 @Override 2549 public boolean equalsDeep(Base other_) { 2550 if (!super.equalsDeep(other_)) 2551 return false; 2552 if (!(other_ instanceof BundleEntryRequestComponent)) 2553 return false; 2554 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other_; 2555 return compareDeep(method, o.method, true) && compareDeep(url, o.url, true) 2556 && compareDeep(ifNoneMatch, o.ifNoneMatch, true) && compareDeep(ifModifiedSince, o.ifModifiedSince, true) 2557 && compareDeep(ifMatch, o.ifMatch, true) && compareDeep(ifNoneExist, o.ifNoneExist, true); 2558 } 2559 2560 @Override 2561 public boolean equalsShallow(Base other_) { 2562 if (!super.equalsShallow(other_)) 2563 return false; 2564 if (!(other_ instanceof BundleEntryRequestComponent)) 2565 return false; 2566 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other_; 2567 return compareValues(method, o.method, true) && compareValues(url, o.url, true) 2568 && compareValues(ifNoneMatch, o.ifNoneMatch, true) && compareValues(ifModifiedSince, o.ifModifiedSince, true) 2569 && compareValues(ifMatch, o.ifMatch, true) && compareValues(ifNoneExist, o.ifNoneExist, true); 2570 } 2571 2572 public boolean isEmpty() { 2573 return super.isEmpty() 2574 && ca.uhn.fhir.util.ElementUtil.isEmpty(method, url, ifNoneMatch, ifModifiedSince, ifMatch, ifNoneExist); 2575 } 2576 2577 public String fhirType() { 2578 return "Bundle.entry.request"; 2579 2580 } 2581 2582 } 2583 2584 @Block() 2585 public static class BundleEntryResponseComponent extends BackboneElement implements IBaseBackboneElement { 2586 /** 2587 * The status code returned by processing this entry. The status SHALL start 2588 * with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP 2589 * description associated with the status code. 2590 */ 2591 @Child(name = "status", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2592 @Description(shortDefinition = "Status response code (text optional)", formalDefinition = "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.") 2593 protected StringType status; 2594 2595 /** 2596 * The location header created by processing this operation, populated if the 2597 * operation returns a location. 2598 */ 2599 @Child(name = "location", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2600 @Description(shortDefinition = "The location (if the operation returns a location)", formalDefinition = "The location header created by processing this operation, populated if the operation returns a location.") 2601 protected UriType location; 2602 2603 /** 2604 * The Etag for the resource, if the operation for the entry produced a 2605 * versioned resource (see [Resource Metadata and 2606 * Versioning](http.html#versioning) and [Managing Resource 2607 * Contention](http.html#concurrency)). 2608 */ 2609 @Child(name = "etag", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2610 @Description(shortDefinition = "The Etag for the resource (if relevant)", formalDefinition = "The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).") 2611 protected StringType etag; 2612 2613 /** 2614 * The date/time that the resource was modified on the server. 2615 */ 2616 @Child(name = "lastModified", type = { 2617 InstantType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2618 @Description(shortDefinition = "Server's date time modified", formalDefinition = "The date/time that the resource was modified on the server.") 2619 protected InstantType lastModified; 2620 2621 /** 2622 * An OperationOutcome containing hints and warnings produced as part of 2623 * processing this entry in a batch or transaction. 2624 */ 2625 @Child(name = "outcome", type = { Resource.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2626 @Description(shortDefinition = "OperationOutcome with hints and warnings (for batch/transaction)", formalDefinition = "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.") 2627 protected Resource outcome; 2628 2629 private static final long serialVersionUID = 923278008L; 2630 2631 /** 2632 * Constructor 2633 */ 2634 public BundleEntryResponseComponent() { 2635 super(); 2636 } 2637 2638 /** 2639 * Constructor 2640 */ 2641 public BundleEntryResponseComponent(StringType status) { 2642 super(); 2643 this.status = status; 2644 } 2645 2646 /** 2647 * @return {@link #status} (The status code returned by processing this entry. 2648 * The status SHALL start with a 3 digit HTTP code (e.g. 404) and may 2649 * contain the standard HTTP description associated with the status 2650 * code.). This is the underlying object with id, value and extensions. 2651 * The accessor "getStatus" gives direct access to the value 2652 */ 2653 public StringType getStatusElement() { 2654 if (this.status == null) 2655 if (Configuration.errorOnAutoCreate()) 2656 throw new Error("Attempt to auto-create BundleEntryResponseComponent.status"); 2657 else if (Configuration.doAutoCreate()) 2658 this.status = new StringType(); // bb 2659 return this.status; 2660 } 2661 2662 public boolean hasStatusElement() { 2663 return this.status != null && !this.status.isEmpty(); 2664 } 2665 2666 public boolean hasStatus() { 2667 return this.status != null && !this.status.isEmpty(); 2668 } 2669 2670 /** 2671 * @param value {@link #status} (The status code returned by processing this 2672 * entry. The status SHALL start with a 3 digit HTTP code (e.g. 2673 * 404) and may contain the standard HTTP description associated 2674 * with the status code.). This is the underlying object with id, 2675 * value and extensions. The accessor "getStatus" gives direct 2676 * access to the value 2677 */ 2678 public BundleEntryResponseComponent setStatusElement(StringType value) { 2679 this.status = value; 2680 return this; 2681 } 2682 2683 /** 2684 * @return The status code returned by processing this entry. The status SHALL 2685 * start with a 3 digit HTTP code (e.g. 404) and may contain the 2686 * standard HTTP description associated with the status code. 2687 */ 2688 public String getStatus() { 2689 return this.status == null ? null : this.status.getValue(); 2690 } 2691 2692 /** 2693 * @param value The status code returned by processing this entry. The status 2694 * SHALL start with a 3 digit HTTP code (e.g. 404) and may contain 2695 * the standard HTTP description associated with the status code. 2696 */ 2697 public BundleEntryResponseComponent setStatus(String value) { 2698 if (this.status == null) 2699 this.status = new StringType(); 2700 this.status.setValue(value); 2701 return this; 2702 } 2703 2704 /** 2705 * @return {@link #location} (The location header created by processing this 2706 * operation, populated if the operation returns a location.). This is 2707 * the underlying object with id, value and extensions. The accessor 2708 * "getLocation" gives direct access to the value 2709 */ 2710 public UriType getLocationElement() { 2711 if (this.location == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create BundleEntryResponseComponent.location"); 2714 else if (Configuration.doAutoCreate()) 2715 this.location = new UriType(); // bb 2716 return this.location; 2717 } 2718 2719 public boolean hasLocationElement() { 2720 return this.location != null && !this.location.isEmpty(); 2721 } 2722 2723 public boolean hasLocation() { 2724 return this.location != null && !this.location.isEmpty(); 2725 } 2726 2727 /** 2728 * @param value {@link #location} (The location header created by processing 2729 * this operation, populated if the operation returns a location.). 2730 * This is the underlying object with id, value and extensions. The 2731 * accessor "getLocation" gives direct access to the value 2732 */ 2733 public BundleEntryResponseComponent setLocationElement(UriType value) { 2734 this.location = value; 2735 return this; 2736 } 2737 2738 /** 2739 * @return The location header created by processing this operation, populated 2740 * if the operation returns a location. 2741 */ 2742 public String getLocation() { 2743 return this.location == null ? null : this.location.getValue(); 2744 } 2745 2746 /** 2747 * @param value The location header created by processing this operation, 2748 * populated if the operation returns a location. 2749 */ 2750 public BundleEntryResponseComponent setLocation(String value) { 2751 if (Utilities.noString(value)) 2752 this.location = null; 2753 else { 2754 if (this.location == null) 2755 this.location = new UriType(); 2756 this.location.setValue(value); 2757 } 2758 return this; 2759 } 2760 2761 /** 2762 * @return {@link #etag} (The Etag for the resource, if the operation for the 2763 * entry produced a versioned resource (see [Resource Metadata and 2764 * Versioning](http.html#versioning) and [Managing Resource 2765 * Contention](http.html#concurrency)).). This is the underlying object 2766 * with id, value and extensions. The accessor "getEtag" gives direct 2767 * access to the value 2768 */ 2769 public StringType getEtagElement() { 2770 if (this.etag == null) 2771 if (Configuration.errorOnAutoCreate()) 2772 throw new Error("Attempt to auto-create BundleEntryResponseComponent.etag"); 2773 else if (Configuration.doAutoCreate()) 2774 this.etag = new StringType(); // bb 2775 return this.etag; 2776 } 2777 2778 public boolean hasEtagElement() { 2779 return this.etag != null && !this.etag.isEmpty(); 2780 } 2781 2782 public boolean hasEtag() { 2783 return this.etag != null && !this.etag.isEmpty(); 2784 } 2785 2786 /** 2787 * @param value {@link #etag} (The Etag for the resource, if the operation for 2788 * the entry produced a versioned resource (see [Resource Metadata 2789 * and Versioning](http.html#versioning) and [Managing Resource 2790 * Contention](http.html#concurrency)).). This is the underlying 2791 * object with id, value and extensions. The accessor "getEtag" 2792 * gives direct access to the value 2793 */ 2794 public BundleEntryResponseComponent setEtagElement(StringType value) { 2795 this.etag = value; 2796 return this; 2797 } 2798 2799 /** 2800 * @return The Etag for the resource, if the operation for the entry produced a 2801 * versioned resource (see [Resource Metadata and 2802 * Versioning](http.html#versioning) and [Managing Resource 2803 * Contention](http.html#concurrency)). 2804 */ 2805 public String getEtag() { 2806 return this.etag == null ? null : this.etag.getValue(); 2807 } 2808 2809 /** 2810 * @param value The Etag for the resource, if the operation for the entry 2811 * produced a versioned resource (see [Resource Metadata and 2812 * Versioning](http.html#versioning) and [Managing Resource 2813 * Contention](http.html#concurrency)). 2814 */ 2815 public BundleEntryResponseComponent setEtag(String value) { 2816 if (Utilities.noString(value)) 2817 this.etag = null; 2818 else { 2819 if (this.etag == null) 2820 this.etag = new StringType(); 2821 this.etag.setValue(value); 2822 } 2823 return this; 2824 } 2825 2826 /** 2827 * @return {@link #lastModified} (The date/time that the resource was modified 2828 * on the server.). This is the underlying object with id, value and 2829 * extensions. The accessor "getLastModified" gives direct access to the 2830 * value 2831 */ 2832 public InstantType getLastModifiedElement() { 2833 if (this.lastModified == null) 2834 if (Configuration.errorOnAutoCreate()) 2835 throw new Error("Attempt to auto-create BundleEntryResponseComponent.lastModified"); 2836 else if (Configuration.doAutoCreate()) 2837 this.lastModified = new InstantType(); // bb 2838 return this.lastModified; 2839 } 2840 2841 public boolean hasLastModifiedElement() { 2842 return this.lastModified != null && !this.lastModified.isEmpty(); 2843 } 2844 2845 public boolean hasLastModified() { 2846 return this.lastModified != null && !this.lastModified.isEmpty(); 2847 } 2848 2849 /** 2850 * @param value {@link #lastModified} (The date/time that the resource was 2851 * modified on the server.). This is the underlying object with id, 2852 * value and extensions. The accessor "getLastModified" gives 2853 * direct access to the value 2854 */ 2855 public BundleEntryResponseComponent setLastModifiedElement(InstantType value) { 2856 this.lastModified = value; 2857 return this; 2858 } 2859 2860 /** 2861 * @return The date/time that the resource was modified on the server. 2862 */ 2863 public Date getLastModified() { 2864 return this.lastModified == null ? null : this.lastModified.getValue(); 2865 } 2866 2867 /** 2868 * @param value The date/time that the resource was modified on the server. 2869 */ 2870 public BundleEntryResponseComponent setLastModified(Date value) { 2871 if (value == null) 2872 this.lastModified = null; 2873 else { 2874 if (this.lastModified == null) 2875 this.lastModified = new InstantType(); 2876 this.lastModified.setValue(value); 2877 } 2878 return this; 2879 } 2880 2881 /** 2882 * @return {@link #outcome} (An OperationOutcome containing hints and warnings 2883 * produced as part of processing this entry in a batch or transaction.) 2884 */ 2885 public Resource getOutcome() { 2886 return this.outcome; 2887 } 2888 2889 public boolean hasOutcome() { 2890 return this.outcome != null && !this.outcome.isEmpty(); 2891 } 2892 2893 /** 2894 * @param value {@link #outcome} (An OperationOutcome containing hints and 2895 * warnings produced as part of processing this entry in a batch or 2896 * transaction.) 2897 */ 2898 public BundleEntryResponseComponent setOutcome(Resource value) { 2899 this.outcome = value; 2900 return this; 2901 } 2902 2903 protected void listChildren(List<Property> children) { 2904 super.listChildren(children); 2905 children.add(new Property("status", "string", 2906 "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.", 2907 0, 1, status)); 2908 children.add(new Property("location", "uri", 2909 "The location header created by processing this operation, populated if the operation returns a location.", 0, 2910 1, location)); 2911 children.add(new Property("etag", "string", 2912 "The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).", 2913 0, 1, etag)); 2914 children.add(new Property("lastModified", "instant", 2915 "The date/time that the resource was modified on the server.", 0, 1, lastModified)); 2916 children.add(new Property("outcome", "Resource", 2917 "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.", 2918 0, 1, outcome)); 2919 } 2920 2921 @Override 2922 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2923 switch (_hash) { 2924 case -892481550: 2925 /* status */ return new Property("status", "string", 2926 "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.", 2927 0, 1, status); 2928 case 1901043637: 2929 /* location */ return new Property("location", "uri", 2930 "The location header created by processing this operation, populated if the operation returns a location.", 2931 0, 1, location); 2932 case 3123477: 2933 /* etag */ return new Property("etag", "string", 2934 "The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).", 2935 0, 1, etag); 2936 case 1959003007: 2937 /* lastModified */ return new Property("lastModified", "instant", 2938 "The date/time that the resource was modified on the server.", 0, 1, lastModified); 2939 case -1106507950: 2940 /* outcome */ return new Property("outcome", "Resource", 2941 "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.", 2942 0, 1, outcome); 2943 default: 2944 return super.getNamedProperty(_hash, _name, _checkValid); 2945 } 2946 2947 } 2948 2949 @Override 2950 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2951 switch (hash) { 2952 case -892481550: 2953 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // StringType 2954 case 1901043637: 2955 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // UriType 2956 case 3123477: 2957 /* etag */ return this.etag == null ? new Base[0] : new Base[] { this.etag }; // StringType 2958 case 1959003007: 2959 /* lastModified */ return this.lastModified == null ? new Base[0] : new Base[] { this.lastModified }; // InstantType 2960 case -1106507950: 2961 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Resource 2962 default: 2963 return super.getProperty(hash, name, checkValid); 2964 } 2965 2966 } 2967 2968 @Override 2969 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2970 switch (hash) { 2971 case -892481550: // status 2972 this.status = castToString(value); // StringType 2973 return value; 2974 case 1901043637: // location 2975 this.location = castToUri(value); // UriType 2976 return value; 2977 case 3123477: // etag 2978 this.etag = castToString(value); // StringType 2979 return value; 2980 case 1959003007: // lastModified 2981 this.lastModified = castToInstant(value); // InstantType 2982 return value; 2983 case -1106507950: // outcome 2984 this.outcome = castToResource(value); // Resource 2985 return value; 2986 default: 2987 return super.setProperty(hash, name, value); 2988 } 2989 2990 } 2991 2992 @Override 2993 public Base setProperty(String name, Base value) throws FHIRException { 2994 if (name.equals("status")) { 2995 this.status = castToString(value); // StringType 2996 } else if (name.equals("location")) { 2997 this.location = castToUri(value); // UriType 2998 } else if (name.equals("etag")) { 2999 this.etag = castToString(value); // StringType 3000 } else if (name.equals("lastModified")) { 3001 this.lastModified = castToInstant(value); // InstantType 3002 } else if (name.equals("outcome")) { 3003 this.outcome = castToResource(value); // Resource 3004 } else 3005 return super.setProperty(name, value); 3006 return value; 3007 } 3008 3009 @Override 3010 public void removeChild(String name, Base value) throws FHIRException { 3011 if (name.equals("status")) { 3012 this.status = null; 3013 } else if (name.equals("location")) { 3014 this.location = null; 3015 } else if (name.equals("etag")) { 3016 this.etag = null; 3017 } else if (name.equals("lastModified")) { 3018 this.lastModified = null; 3019 } else if (name.equals("outcome")) { 3020 this.outcome = null; 3021 } else 3022 super.removeChild(name, value); 3023 3024 } 3025 3026 @Override 3027 public Base makeProperty(int hash, String name) throws FHIRException { 3028 switch (hash) { 3029 case -892481550: 3030 return getStatusElement(); 3031 case 1901043637: 3032 return getLocationElement(); 3033 case 3123477: 3034 return getEtagElement(); 3035 case 1959003007: 3036 return getLastModifiedElement(); 3037 case -1106507950: 3038 throw new FHIRException("Cannot make property outcome as it is not a complex type"); // Resource 3039 default: 3040 return super.makeProperty(hash, name); 3041 } 3042 3043 } 3044 3045 @Override 3046 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3047 switch (hash) { 3048 case -892481550: 3049 /* status */ return new String[] { "string" }; 3050 case 1901043637: 3051 /* location */ return new String[] { "uri" }; 3052 case 3123477: 3053 /* etag */ return new String[] { "string" }; 3054 case 1959003007: 3055 /* lastModified */ return new String[] { "instant" }; 3056 case -1106507950: 3057 /* outcome */ return new String[] { "Resource" }; 3058 default: 3059 return super.getTypesForProperty(hash, name); 3060 } 3061 3062 } 3063 3064 @Override 3065 public Base addChild(String name) throws FHIRException { 3066 if (name.equals("status")) { 3067 throw new FHIRException("Cannot call addChild on a singleton property Bundle.status"); 3068 } else if (name.equals("location")) { 3069 throw new FHIRException("Cannot call addChild on a singleton property Bundle.location"); 3070 } else if (name.equals("etag")) { 3071 throw new FHIRException("Cannot call addChild on a singleton property Bundle.etag"); 3072 } else if (name.equals("lastModified")) { 3073 throw new FHIRException("Cannot call addChild on a singleton property Bundle.lastModified"); 3074 } else if (name.equals("outcome")) { 3075 throw new FHIRException("Cannot call addChild on an abstract type Bundle.outcome"); 3076 } else 3077 return super.addChild(name); 3078 } 3079 3080 public BundleEntryResponseComponent copy() { 3081 BundleEntryResponseComponent dst = new BundleEntryResponseComponent(); 3082 copyValues(dst); 3083 return dst; 3084 } 3085 3086 public void copyValues(BundleEntryResponseComponent dst) { 3087 super.copyValues(dst); 3088 dst.status = status == null ? null : status.copy(); 3089 dst.location = location == null ? null : location.copy(); 3090 dst.etag = etag == null ? null : etag.copy(); 3091 dst.lastModified = lastModified == null ? null : lastModified.copy(); 3092 dst.outcome = outcome == null ? null : outcome.copy(); 3093 } 3094 3095 @Override 3096 public boolean equalsDeep(Base other_) { 3097 if (!super.equalsDeep(other_)) 3098 return false; 3099 if (!(other_ instanceof BundleEntryResponseComponent)) 3100 return false; 3101 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other_; 3102 return compareDeep(status, o.status, true) && compareDeep(location, o.location, true) 3103 && compareDeep(etag, o.etag, true) && compareDeep(lastModified, o.lastModified, true) 3104 && compareDeep(outcome, o.outcome, true); 3105 } 3106 3107 @Override 3108 public boolean equalsShallow(Base other_) { 3109 if (!super.equalsShallow(other_)) 3110 return false; 3111 if (!(other_ instanceof BundleEntryResponseComponent)) 3112 return false; 3113 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other_; 3114 return compareValues(status, o.status, true) && compareValues(location, o.location, true) 3115 && compareValues(etag, o.etag, true) && compareValues(lastModified, o.lastModified, true); 3116 } 3117 3118 public boolean isEmpty() { 3119 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, location, etag, lastModified, outcome); 3120 } 3121 3122 public String fhirType() { 3123 return "Bundle.entry.response"; 3124 3125 } 3126 3127 } 3128 3129 /** 3130 * A persistent identifier for the bundle that won't change as a bundle is 3131 * copied from server to server. 3132 */ 3133 @Child(name = "identifier", type = { 3134 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 3135 @Description(shortDefinition = "Persistent identifier for the bundle", formalDefinition = "A persistent identifier for the bundle that won't change as a bundle is copied from server to server.") 3136 protected Identifier identifier; 3137 3138 /** 3139 * Indicates the purpose of this bundle - how it is intended to be used. 3140 */ 3141 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3142 @Description(shortDefinition = "document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection", formalDefinition = "Indicates the purpose of this bundle - how it is intended to be used.") 3143 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/bundle-type") 3144 protected Enumeration<BundleType> type; 3145 3146 /** 3147 * The date/time that the bundle was assembled - i.e. when the resources were 3148 * placed in the bundle. 3149 */ 3150 @Child(name = "timestamp", type = { 3151 InstantType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3152 @Description(shortDefinition = "When the bundle was assembled", formalDefinition = "The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.") 3153 protected InstantType timestamp; 3154 3155 /** 3156 * If a set of search matches, this is the total number of entries of type 3157 * 'match' across all pages in the search. It does not include search.mode = 3158 * 'include' or 'outcome' entries and it does not provide a count of the number 3159 * of entries in the Bundle. 3160 */ 3161 @Child(name = "total", type = { 3162 UnsignedIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3163 @Description(shortDefinition = "If search, the total number of matches", formalDefinition = "If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.") 3164 protected UnsignedIntType total; 3165 3166 /** 3167 * A series of links that provide context to this bundle. 3168 */ 3169 @Child(name = "link", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3170 @Description(shortDefinition = "Links related to this Bundle", formalDefinition = "A series of links that provide context to this bundle.") 3171 protected List<BundleLinkComponent> link; 3172 3173 /** 3174 * An entry in a bundle resource - will either contain a resource or information 3175 * about a resource (transactions and history only). 3176 */ 3177 @Child(name = "entry", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3178 @Description(shortDefinition = "Entry in the bundle - will have a resource or information", formalDefinition = "An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).") 3179 protected List<BundleEntryComponent> entry; 3180 3181 /** 3182 * Digital Signature - base64 encoded. XML-DSig or a JWT. 3183 */ 3184 @Child(name = "signature", type = { Signature.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3185 @Description(shortDefinition = "Digital Signature", formalDefinition = "Digital Signature - base64 encoded. XML-DSig or a JWT.") 3186 protected Signature signature; 3187 3188 private static final long serialVersionUID = 1740470158L; 3189 3190 /** 3191 * Constructor 3192 */ 3193 public Bundle() { 3194 super(); 3195 } 3196 3197 /** 3198 * Constructor 3199 */ 3200 public Bundle(Enumeration<BundleType> type) { 3201 super(); 3202 this.type = type; 3203 } 3204 3205 /** 3206 * @return {@link #identifier} (A persistent identifier for the bundle that 3207 * won't change as a bundle is copied from server to server.) 3208 */ 3209 public Identifier getIdentifier() { 3210 if (this.identifier == null) 3211 if (Configuration.errorOnAutoCreate()) 3212 throw new Error("Attempt to auto-create Bundle.identifier"); 3213 else if (Configuration.doAutoCreate()) 3214 this.identifier = new Identifier(); // cc 3215 return this.identifier; 3216 } 3217 3218 public boolean hasIdentifier() { 3219 return this.identifier != null && !this.identifier.isEmpty(); 3220 } 3221 3222 /** 3223 * @param value {@link #identifier} (A persistent identifier for the bundle that 3224 * won't change as a bundle is copied from server to server.) 3225 */ 3226 public Bundle setIdentifier(Identifier value) { 3227 this.identifier = value; 3228 return this; 3229 } 3230 3231 /** 3232 * @return {@link #type} (Indicates the purpose of this bundle - how it is 3233 * intended to be used.). This is the underlying object with id, value 3234 * and extensions. The accessor "getType" gives direct access to the 3235 * value 3236 */ 3237 public Enumeration<BundleType> getTypeElement() { 3238 if (this.type == null) 3239 if (Configuration.errorOnAutoCreate()) 3240 throw new Error("Attempt to auto-create Bundle.type"); 3241 else if (Configuration.doAutoCreate()) 3242 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); // bb 3243 return this.type; 3244 } 3245 3246 public boolean hasTypeElement() { 3247 return this.type != null && !this.type.isEmpty(); 3248 } 3249 3250 public boolean hasType() { 3251 return this.type != null && !this.type.isEmpty(); 3252 } 3253 3254 /** 3255 * @param value {@link #type} (Indicates the purpose of this bundle - how it is 3256 * intended to be used.). This is the underlying object with id, 3257 * value and extensions. The accessor "getType" gives direct access 3258 * to the value 3259 */ 3260 public Bundle setTypeElement(Enumeration<BundleType> value) { 3261 this.type = value; 3262 return this; 3263 } 3264 3265 /** 3266 * @return Indicates the purpose of this bundle - how it is intended to be used. 3267 */ 3268 public BundleType getType() { 3269 return this.type == null ? null : this.type.getValue(); 3270 } 3271 3272 /** 3273 * @param value Indicates the purpose of this bundle - how it is intended to be 3274 * used. 3275 */ 3276 public Bundle setType(BundleType value) { 3277 if (this.type == null) 3278 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); 3279 this.type.setValue(value); 3280 return this; 3281 } 3282 3283 /** 3284 * @return {@link #timestamp} (The date/time that the bundle was assembled - 3285 * i.e. when the resources were placed in the bundle.). This is the 3286 * underlying object with id, value and extensions. The accessor 3287 * "getTimestamp" gives direct access to the value 3288 */ 3289 public InstantType getTimestampElement() { 3290 if (this.timestamp == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create Bundle.timestamp"); 3293 else if (Configuration.doAutoCreate()) 3294 this.timestamp = new InstantType(); // bb 3295 return this.timestamp; 3296 } 3297 3298 public boolean hasTimestampElement() { 3299 return this.timestamp != null && !this.timestamp.isEmpty(); 3300 } 3301 3302 public boolean hasTimestamp() { 3303 return this.timestamp != null && !this.timestamp.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #timestamp} (The date/time that the bundle was assembled 3308 * - i.e. when the resources were placed in the bundle.). This is 3309 * the underlying object with id, value and extensions. The 3310 * accessor "getTimestamp" gives direct access to the value 3311 */ 3312 public Bundle setTimestampElement(InstantType value) { 3313 this.timestamp = value; 3314 return this; 3315 } 3316 3317 /** 3318 * @return The date/time that the bundle was assembled - i.e. when the resources 3319 * were placed in the bundle. 3320 */ 3321 public Date getTimestamp() { 3322 return this.timestamp == null ? null : this.timestamp.getValue(); 3323 } 3324 3325 /** 3326 * @param value The date/time that the bundle was assembled - i.e. when the 3327 * resources were placed in the bundle. 3328 */ 3329 public Bundle setTimestamp(Date value) { 3330 if (value == null) 3331 this.timestamp = null; 3332 else { 3333 if (this.timestamp == null) 3334 this.timestamp = new InstantType(); 3335 this.timestamp.setValue(value); 3336 } 3337 return this; 3338 } 3339 3340 /** 3341 * @return {@link #total} (If a set of search matches, this is the total number 3342 * of entries of type 'match' across all pages in the search. It does 3343 * not include search.mode = 'include' or 'outcome' entries and it does 3344 * not provide a count of the number of entries in the Bundle.). This is 3345 * the underlying object with id, value and extensions. The accessor 3346 * "getTotal" gives direct access to the value 3347 */ 3348 public UnsignedIntType getTotalElement() { 3349 if (this.total == null) 3350 if (Configuration.errorOnAutoCreate()) 3351 throw new Error("Attempt to auto-create Bundle.total"); 3352 else if (Configuration.doAutoCreate()) 3353 this.total = new UnsignedIntType(); // bb 3354 return this.total; 3355 } 3356 3357 public boolean hasTotalElement() { 3358 return this.total != null && !this.total.isEmpty(); 3359 } 3360 3361 public boolean hasTotal() { 3362 return this.total != null && !this.total.isEmpty(); 3363 } 3364 3365 /** 3366 * @param value {@link #total} (If a set of search matches, this is the total 3367 * number of entries of type 'match' across all pages in the 3368 * search. It does not include search.mode = 'include' or 'outcome' 3369 * entries and it does not provide a count of the number of entries 3370 * in the Bundle.). This is the underlying object with id, value 3371 * and extensions. The accessor "getTotal" gives direct access to 3372 * the value 3373 */ 3374 public Bundle setTotalElement(UnsignedIntType value) { 3375 this.total = value; 3376 return this; 3377 } 3378 3379 /** 3380 * @return If a set of search matches, this is the total number of entries of 3381 * type 'match' across all pages in the search. It does not include 3382 * search.mode = 'include' or 'outcome' entries and it does not provide 3383 * a count of the number of entries in the Bundle. 3384 */ 3385 public int getTotal() { 3386 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 3387 } 3388 3389 /** 3390 * @param value If a set of search matches, this is the total number of entries 3391 * of type 'match' across all pages in the search. It does not 3392 * include search.mode = 'include' or 'outcome' entries and it does 3393 * not provide a count of the number of entries in the Bundle. 3394 */ 3395 public Bundle setTotal(int value) { 3396 if (this.total == null) 3397 this.total = new UnsignedIntType(); 3398 this.total.setValue(value); 3399 return this; 3400 } 3401 3402 /** 3403 * @return {@link #link} (A series of links that provide context to this 3404 * bundle.) 3405 */ 3406 public List<BundleLinkComponent> getLink() { 3407 if (this.link == null) 3408 this.link = new ArrayList<BundleLinkComponent>(); 3409 return this.link; 3410 } 3411 3412 /** 3413 * @return Returns a reference to <code>this</code> for easy method chaining 3414 */ 3415 public Bundle setLink(List<BundleLinkComponent> theLink) { 3416 this.link = theLink; 3417 return this; 3418 } 3419 3420 public boolean hasLink() { 3421 if (this.link == null) 3422 return false; 3423 for (BundleLinkComponent item : this.link) 3424 if (!item.isEmpty()) 3425 return true; 3426 return false; 3427 } 3428 3429 public BundleLinkComponent addLink() { // 3 3430 BundleLinkComponent t = new BundleLinkComponent(); 3431 if (this.link == null) 3432 this.link = new ArrayList<BundleLinkComponent>(); 3433 this.link.add(t); 3434 return t; 3435 } 3436 3437 public Bundle addLink(BundleLinkComponent t) { // 3 3438 if (t == null) 3439 return this; 3440 if (this.link == null) 3441 this.link = new ArrayList<BundleLinkComponent>(); 3442 this.link.add(t); 3443 return this; 3444 } 3445 3446 /** 3447 * @return The first repetition of repeating field {@link #link}, creating it if 3448 * it does not already exist 3449 */ 3450 public BundleLinkComponent getLinkFirstRep() { 3451 if (getLink().isEmpty()) { 3452 addLink(); 3453 } 3454 return getLink().get(0); 3455 } 3456 3457 /** 3458 * @return {@link #entry} (An entry in a bundle resource - will either contain a 3459 * resource or information about a resource (transactions and history 3460 * only).) 3461 */ 3462 public List<BundleEntryComponent> getEntry() { 3463 if (this.entry == null) 3464 this.entry = new ArrayList<BundleEntryComponent>(); 3465 return this.entry; 3466 } 3467 3468 /** 3469 * @return Returns a reference to <code>this</code> for easy method chaining 3470 */ 3471 public Bundle setEntry(List<BundleEntryComponent> theEntry) { 3472 this.entry = theEntry; 3473 return this; 3474 } 3475 3476 public boolean hasEntry() { 3477 if (this.entry == null) 3478 return false; 3479 for (BundleEntryComponent item : this.entry) 3480 if (!item.isEmpty()) 3481 return true; 3482 return false; 3483 } 3484 3485 public BundleEntryComponent addEntry() { // 3 3486 BundleEntryComponent t = new BundleEntryComponent(); 3487 if (this.entry == null) 3488 this.entry = new ArrayList<BundleEntryComponent>(); 3489 this.entry.add(t); 3490 return t; 3491 } 3492 3493 public Bundle addEntry(BundleEntryComponent t) { // 3 3494 if (t == null) 3495 return this; 3496 if (this.entry == null) 3497 this.entry = new ArrayList<BundleEntryComponent>(); 3498 this.entry.add(t); 3499 return this; 3500 } 3501 3502 /** 3503 * @return The first repetition of repeating field {@link #entry}, creating it 3504 * if it does not already exist 3505 */ 3506 public BundleEntryComponent getEntryFirstRep() { 3507 if (getEntry().isEmpty()) { 3508 addEntry(); 3509 } 3510 return getEntry().get(0); 3511 } 3512 3513 /** 3514 * @return {@link #signature} (Digital Signature - base64 encoded. XML-DSig or a 3515 * JWT.) 3516 */ 3517 public Signature getSignature() { 3518 if (this.signature == null) 3519 if (Configuration.errorOnAutoCreate()) 3520 throw new Error("Attempt to auto-create Bundle.signature"); 3521 else if (Configuration.doAutoCreate()) 3522 this.signature = new Signature(); // cc 3523 return this.signature; 3524 } 3525 3526 public boolean hasSignature() { 3527 return this.signature != null && !this.signature.isEmpty(); 3528 } 3529 3530 /** 3531 * @param value {@link #signature} (Digital Signature - base64 encoded. XML-DSig 3532 * or a JWT.) 3533 */ 3534 public Bundle setSignature(Signature value) { 3535 this.signature = value; 3536 return this; 3537 } 3538 3539 /** 3540 * Returns the {@link #getLink() link} which matches a given 3541 * {@link BundleLinkComponent#getRelation() relation}. If no link is found which 3542 * matches the given relation, returns <code>null</code>. If more than one link 3543 * is found which matches the given relation, returns the first matching 3544 * BundleLinkComponent. 3545 * 3546 * @param theRelation The relation, such as "next", or "self. See the constants 3547 * such as {@link IBaseBundle#LINK_SELF} and 3548 * {@link IBaseBundle#LINK_NEXT}. 3549 * @return Returns a matching BundleLinkComponent, or <code>null</code> 3550 * @see IBaseBundle#LINK_NEXT 3551 * @see IBaseBundle#LINK_PREV 3552 * @see IBaseBundle#LINK_SELF 3553 */ 3554 public BundleLinkComponent getLink(String theRelation) { 3555 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 3556 for (BundleLinkComponent next : getLink()) { 3557 if (theRelation.equals(next.getRelation())) { 3558 return next; 3559 } 3560 } 3561 return null; 3562 } 3563 3564 /** 3565 * Returns the {@link #getLink() link} which matches a given 3566 * {@link BundleLinkComponent#getRelation() relation}. If no link is found which 3567 * matches the given relation, creates a new BundleLinkComponent with the given 3568 * relation and adds it to this Bundle. If more than one link is found which 3569 * matches the given relation, returns the first matching BundleLinkComponent. 3570 * 3571 * @param theRelation The relation, such as "next", or "self. See the constants 3572 * such as {@link IBaseBundle#LINK_SELF} and 3573 * {@link IBaseBundle#LINK_NEXT}. 3574 * @return Returns a matching BundleLinkComponent, or <code>null</code> 3575 * @see IBaseBundle#LINK_NEXT 3576 * @see IBaseBundle#LINK_PREV 3577 * @see IBaseBundle#LINK_SELF 3578 */ 3579 public BundleLinkComponent getLinkOrCreate(String theRelation) { 3580 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 3581 for (BundleLinkComponent next : getLink()) { 3582 if (theRelation.equals(next.getRelation())) { 3583 return next; 3584 } 3585 } 3586 BundleLinkComponent retVal = new BundleLinkComponent(); 3587 retVal.setRelation(theRelation); 3588 getLink().add(retVal); 3589 return retVal; 3590 } 3591 3592 protected void listChildren(List<Property> children) { 3593 super.listChildren(children); 3594 children.add(new Property("identifier", "Identifier", 3595 "A persistent identifier for the bundle that won't change as a bundle is copied from server to server.", 0, 1, 3596 identifier)); 3597 children.add(new Property("type", "code", "Indicates the purpose of this bundle - how it is intended to be used.", 3598 0, 1, type)); 3599 children.add(new Property("timestamp", "instant", 3600 "The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.", 0, 1, 3601 timestamp)); 3602 children.add(new Property("total", "unsignedInt", 3603 "If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.", 3604 0, 1, total)); 3605 children.add(new Property("link", "", "A series of links that provide context to this bundle.", 0, 3606 java.lang.Integer.MAX_VALUE, link)); 3607 children.add(new Property("entry", "", 3608 "An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).", 3609 0, java.lang.Integer.MAX_VALUE, entry)); 3610 children.add(new Property("signature", "Signature", "Digital Signature - base64 encoded. XML-DSig or a JWT.", 0, 1, 3611 signature)); 3612 } 3613 3614 @Override 3615 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3616 switch (_hash) { 3617 case -1618432855: 3618 /* identifier */ return new Property("identifier", "Identifier", 3619 "A persistent identifier for the bundle that won't change as a bundle is copied from server to server.", 0, 1, 3620 identifier); 3621 case 3575610: 3622 /* type */ return new Property("type", "code", 3623 "Indicates the purpose of this bundle - how it is intended to be used.", 0, 1, type); 3624 case 55126294: 3625 /* timestamp */ return new Property("timestamp", "instant", 3626 "The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.", 0, 1, 3627 timestamp); 3628 case 110549828: 3629 /* total */ return new Property("total", "unsignedInt", 3630 "If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.", 3631 0, 1, total); 3632 case 3321850: 3633 /* link */ return new Property("link", "", "A series of links that provide context to this bundle.", 0, 3634 java.lang.Integer.MAX_VALUE, link); 3635 case 96667762: 3636 /* entry */ return new Property("entry", "", 3637 "An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).", 3638 0, java.lang.Integer.MAX_VALUE, entry); 3639 case 1073584312: 3640 /* signature */ return new Property("signature", "Signature", 3641 "Digital Signature - base64 encoded. XML-DSig or a JWT.", 0, 1, signature); 3642 default: 3643 return super.getNamedProperty(_hash, _name, _checkValid); 3644 } 3645 3646 } 3647 3648 @Override 3649 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3650 switch (hash) { 3651 case -1618432855: 3652 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 3653 case 3575610: 3654 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<BundleType> 3655 case 55126294: 3656 /* timestamp */ return this.timestamp == null ? new Base[0] : new Base[] { this.timestamp }; // InstantType 3657 case 110549828: 3658 /* total */ return this.total == null ? new Base[0] : new Base[] { this.total }; // UnsignedIntType 3659 case 3321850: 3660 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // BundleLinkComponent 3661 case 96667762: 3662 /* entry */ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // BundleEntryComponent 3663 case 1073584312: 3664 /* signature */ return this.signature == null ? new Base[0] : new Base[] { this.signature }; // Signature 3665 default: 3666 return super.getProperty(hash, name, checkValid); 3667 } 3668 3669 } 3670 3671 @Override 3672 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3673 switch (hash) { 3674 case -1618432855: // identifier 3675 this.identifier = castToIdentifier(value); // Identifier 3676 return value; 3677 case 3575610: // type 3678 value = new BundleTypeEnumFactory().fromType(castToCode(value)); 3679 this.type = (Enumeration) value; // Enumeration<BundleType> 3680 return value; 3681 case 55126294: // timestamp 3682 this.timestamp = castToInstant(value); // InstantType 3683 return value; 3684 case 110549828: // total 3685 this.total = castToUnsignedInt(value); // UnsignedIntType 3686 return value; 3687 case 3321850: // link 3688 this.getLink().add((BundleLinkComponent) value); // BundleLinkComponent 3689 return value; 3690 case 96667762: // entry 3691 this.getEntry().add((BundleEntryComponent) value); // BundleEntryComponent 3692 return value; 3693 case 1073584312: // signature 3694 this.signature = castToSignature(value); // Signature 3695 return value; 3696 default: 3697 return super.setProperty(hash, name, value); 3698 } 3699 3700 } 3701 3702 @Override 3703 public Base setProperty(String name, Base value) throws FHIRException { 3704 if (name.equals("identifier")) { 3705 this.identifier = castToIdentifier(value); // Identifier 3706 } else if (name.equals("type")) { 3707 value = new BundleTypeEnumFactory().fromType(castToCode(value)); 3708 this.type = (Enumeration) value; // Enumeration<BundleType> 3709 } else if (name.equals("timestamp")) { 3710 this.timestamp = castToInstant(value); // InstantType 3711 } else if (name.equals("total")) { 3712 this.total = castToUnsignedInt(value); // UnsignedIntType 3713 } else if (name.equals("link")) { 3714 this.getLink().add((BundleLinkComponent) value); 3715 } else if (name.equals("entry")) { 3716 this.getEntry().add((BundleEntryComponent) value); 3717 } else if (name.equals("signature")) { 3718 this.signature = castToSignature(value); // Signature 3719 } else 3720 return super.setProperty(name, value); 3721 return value; 3722 } 3723 3724 @Override 3725 public void removeChild(String name, Base value) throws FHIRException { 3726 if (name.equals("identifier")) { 3727 this.identifier = null; 3728 } else if (name.equals("type")) { 3729 this.type = null; 3730 } else if (name.equals("timestamp")) { 3731 this.timestamp = null; 3732 } else if (name.equals("total")) { 3733 this.total = null; 3734 } else if (name.equals("link")) { 3735 this.getLink().remove((BundleLinkComponent) value); 3736 } else if (name.equals("entry")) { 3737 this.getEntry().remove((BundleEntryComponent) value); 3738 } else if (name.equals("signature")) { 3739 this.signature = null; 3740 } else 3741 super.removeChild(name, value); 3742 3743 } 3744 3745 @Override 3746 public Base makeProperty(int hash, String name) throws FHIRException { 3747 switch (hash) { 3748 case -1618432855: 3749 return getIdentifier(); 3750 case 3575610: 3751 return getTypeElement(); 3752 case 55126294: 3753 return getTimestampElement(); 3754 case 110549828: 3755 return getTotalElement(); 3756 case 3321850: 3757 return addLink(); 3758 case 96667762: 3759 return addEntry(); 3760 case 1073584312: 3761 return getSignature(); 3762 default: 3763 return super.makeProperty(hash, name); 3764 } 3765 3766 } 3767 3768 @Override 3769 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3770 switch (hash) { 3771 case -1618432855: 3772 /* identifier */ return new String[] { "Identifier" }; 3773 case 3575610: 3774 /* type */ return new String[] { "code" }; 3775 case 55126294: 3776 /* timestamp */ return new String[] { "instant" }; 3777 case 110549828: 3778 /* total */ return new String[] { "unsignedInt" }; 3779 case 3321850: 3780 /* link */ return new String[] {}; 3781 case 96667762: 3782 /* entry */ return new String[] {}; 3783 case 1073584312: 3784 /* signature */ return new String[] { "Signature" }; 3785 default: 3786 return super.getTypesForProperty(hash, name); 3787 } 3788 3789 } 3790 3791 @Override 3792 public Base addChild(String name) throws FHIRException { 3793 if (name.equals("identifier")) { 3794 this.identifier = new Identifier(); 3795 return this.identifier; 3796 } else if (name.equals("type")) { 3797 throw new FHIRException("Cannot call addChild on a singleton property Bundle.type"); 3798 } else if (name.equals("timestamp")) { 3799 throw new FHIRException("Cannot call addChild on a singleton property Bundle.timestamp"); 3800 } else if (name.equals("total")) { 3801 throw new FHIRException("Cannot call addChild on a singleton property Bundle.total"); 3802 } else if (name.equals("link")) { 3803 return addLink(); 3804 } else if (name.equals("entry")) { 3805 return addEntry(); 3806 } else if (name.equals("signature")) { 3807 this.signature = new Signature(); 3808 return this.signature; 3809 } else 3810 return super.addChild(name); 3811 } 3812 3813 public String fhirType() { 3814 return "Bundle"; 3815 3816 } 3817 3818 public Bundle copy() { 3819 Bundle dst = new Bundle(); 3820 copyValues(dst); 3821 return dst; 3822 } 3823 3824 public void copyValues(Bundle dst) { 3825 super.copyValues(dst); 3826 dst.identifier = identifier == null ? null : identifier.copy(); 3827 dst.type = type == null ? null : type.copy(); 3828 dst.timestamp = timestamp == null ? null : timestamp.copy(); 3829 dst.total = total == null ? null : total.copy(); 3830 if (link != null) { 3831 dst.link = new ArrayList<BundleLinkComponent>(); 3832 for (BundleLinkComponent i : link) 3833 dst.link.add(i.copy()); 3834 } 3835 ; 3836 if (entry != null) { 3837 dst.entry = new ArrayList<BundleEntryComponent>(); 3838 for (BundleEntryComponent i : entry) 3839 dst.entry.add(i.copy()); 3840 } 3841 ; 3842 dst.signature = signature == null ? null : signature.copy(); 3843 } 3844 3845 protected Bundle typedCopy() { 3846 return copy(); 3847 } 3848 3849 @Override 3850 public boolean equalsDeep(Base other_) { 3851 if (!super.equalsDeep(other_)) 3852 return false; 3853 if (!(other_ instanceof Bundle)) 3854 return false; 3855 Bundle o = (Bundle) other_; 3856 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 3857 && compareDeep(timestamp, o.timestamp, true) && compareDeep(total, o.total, true) 3858 && compareDeep(link, o.link, true) && compareDeep(entry, o.entry, true) 3859 && compareDeep(signature, o.signature, true); 3860 } 3861 3862 @Override 3863 public boolean equalsShallow(Base other_) { 3864 if (!super.equalsShallow(other_)) 3865 return false; 3866 if (!(other_ instanceof Bundle)) 3867 return false; 3868 Bundle o = (Bundle) other_; 3869 return compareValues(type, o.type, true) && compareValues(timestamp, o.timestamp, true) 3870 && compareValues(total, o.total, true); 3871 } 3872 3873 public boolean isEmpty() { 3874 return super.isEmpty() 3875 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, timestamp, total, link, entry, signature); 3876 } 3877 3878 @Override 3879 public ResourceType getResourceType() { 3880 return ResourceType.Bundle; 3881 } 3882 3883 /** 3884 * Search parameter: <b>identifier</b> 3885 * <p> 3886 * Description: <b>Persistent identifier for the bundle</b><br> 3887 * Type: <b>token</b><br> 3888 * Path: <b>Bundle.identifier</b><br> 3889 * </p> 3890 */ 3891 @SearchParamDefinition(name = "identifier", path = "Bundle.identifier", description = "Persistent identifier for the bundle", type = "token") 3892 public static final String SP_IDENTIFIER = "identifier"; 3893 /** 3894 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3895 * <p> 3896 * Description: <b>Persistent identifier for the bundle</b><br> 3897 * Type: <b>token</b><br> 3898 * Path: <b>Bundle.identifier</b><br> 3899 * </p> 3900 */ 3901 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3902 SP_IDENTIFIER); 3903 3904 /** 3905 * Search parameter: <b>composition</b> 3906 * <p> 3907 * Description: <b>The first resource in the bundle, if the bundle type is 3908 * "document" - this is a composition, and this parameter provides access to 3909 * search its contents</b><br> 3910 * Type: <b>reference</b><br> 3911 * Path: <b>Bundle.entry(0).resource</b><br> 3912 * </p> 3913 */ 3914 @SearchParamDefinition(name = "composition", path = "Bundle.entry[0].resource", description = "The first resource in the bundle, if the bundle type is \"document\" - this is a composition, and this parameter provides access to search its contents", type = "reference", target = { 3915 Composition.class }) 3916 public static final String SP_COMPOSITION = "composition"; 3917 /** 3918 * <b>Fluent Client</b> search parameter constant for <b>composition</b> 3919 * <p> 3920 * Description: <b>The first resource in the bundle, if the bundle type is 3921 * "document" - this is a composition, and this parameter provides access to 3922 * search its contents</b><br> 3923 * Type: <b>reference</b><br> 3924 * Path: <b>Bundle.entry(0).resource</b><br> 3925 * </p> 3926 */ 3927 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3928 SP_COMPOSITION); 3929 3930 /** 3931 * Constant for fluent queries to be used to add include statements. Specifies 3932 * the path value of "<b>Bundle:composition</b>". 3933 */ 3934 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSITION = new ca.uhn.fhir.model.api.Include( 3935 "Bundle:composition").toLocked(); 3936 3937 /** 3938 * Search parameter: <b>type</b> 3939 * <p> 3940 * Description: <b>document | message | transaction | transaction-response | 3941 * batch | batch-response | history | searchset | collection</b><br> 3942 * Type: <b>token</b><br> 3943 * Path: <b>Bundle.type</b><br> 3944 * </p> 3945 */ 3946 @SearchParamDefinition(name = "type", path = "Bundle.type", description = "document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection", type = "token") 3947 public static final String SP_TYPE = "type"; 3948 /** 3949 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3950 * <p> 3951 * Description: <b>document | message | transaction | transaction-response | 3952 * batch | batch-response | history | searchset | collection</b><br> 3953 * Type: <b>token</b><br> 3954 * Path: <b>Bundle.type</b><br> 3955 * </p> 3956 */ 3957 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3958 SP_TYPE); 3959 3960 /** 3961 * Search parameter: <b>message</b> 3962 * <p> 3963 * Description: <b>The first resource in the bundle, if the bundle type is 3964 * "message" - this is a message header, and this parameter provides access to 3965 * search its contents</b><br> 3966 * Type: <b>reference</b><br> 3967 * Path: <b>Bundle.entry(0).resource</b><br> 3968 * </p> 3969 */ 3970 @SearchParamDefinition(name = "message", path = "Bundle.entry[0].resource", description = "The first resource in the bundle, if the bundle type is \"message\" - this is a message header, and this parameter provides access to search its contents", type = "reference", target = { 3971 MessageHeader.class }) 3972 public static final String SP_MESSAGE = "message"; 3973 /** 3974 * <b>Fluent Client</b> search parameter constant for <b>message</b> 3975 * <p> 3976 * Description: <b>The first resource in the bundle, if the bundle type is 3977 * "message" - this is a message header, and this parameter provides access to 3978 * search its contents</b><br> 3979 * Type: <b>reference</b><br> 3980 * Path: <b>Bundle.entry(0).resource</b><br> 3981 * </p> 3982 */ 3983 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MESSAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3984 SP_MESSAGE); 3985 3986 /** 3987 * Constant for fluent queries to be used to add include statements. Specifies 3988 * the path value of "<b>Bundle:message</b>". 3989 */ 3990 public static final ca.uhn.fhir.model.api.Include INCLUDE_MESSAGE = new ca.uhn.fhir.model.api.Include( 3991 "Bundle:message").toLocked(); 3992 3993 /** 3994 * Search parameter: <b>timestamp</b> 3995 * <p> 3996 * Description: <b>When the bundle was assembled</b><br> 3997 * Type: <b>date</b><br> 3998 * Path: <b>Bundle.timestamp</b><br> 3999 * </p> 4000 */ 4001 @SearchParamDefinition(name = "timestamp", path = "Bundle.timestamp", description = "When the bundle was assembled", type = "date") 4002 public static final String SP_TIMESTAMP = "timestamp"; 4003 /** 4004 * <b>Fluent Client</b> search parameter constant for <b>timestamp</b> 4005 * <p> 4006 * Description: <b>When the bundle was assembled</b><br> 4007 * Type: <b>date</b><br> 4008 * Path: <b>Bundle.timestamp</b><br> 4009 * </p> 4010 */ 4011 public static final ca.uhn.fhir.rest.gclient.DateClientParam TIMESTAMP = new ca.uhn.fhir.rest.gclient.DateClientParam( 4012 SP_TIMESTAMP); 4013 4014}