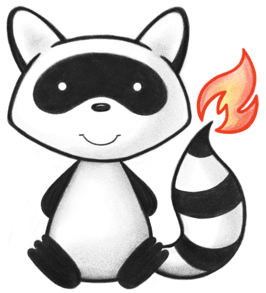
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.instance.model.api.IBaseConformance; 040import org.hl7.fhir.r4.model.Enumerations.FHIRVersion; 041import org.hl7.fhir.r4.model.Enumerations.FHIRVersionEnumFactory; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 043import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 044import org.hl7.fhir.r4.model.Enumerations.SearchParamType; 045import org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory; 046import org.hl7.fhir.utilities.Utilities; 047 048import ca.uhn.fhir.model.api.annotation.Block; 049import ca.uhn.fhir.model.api.annotation.Child; 050import ca.uhn.fhir.model.api.annotation.ChildOrder; 051import ca.uhn.fhir.model.api.annotation.Description; 052import ca.uhn.fhir.model.api.annotation.ResourceDef; 053import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 054 055/** 056 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 057 * Server for a particular version of FHIR that may be used as a statement of 058 * actual server functionality or a statement of required or desired server 059 * implementation. 060 */ 061@ResourceDef(name = "CapabilityStatement", profile = "http://hl7.org/fhir/StructureDefinition/CapabilityStatement") 062@ChildOrder(names = { "url", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", 063 "description", "useContext", "jurisdiction", "purpose", "copyright", "kind", "instantiates", "imports", "software", 064 "implementation", "fhirVersion", "format", "patchFormat", "implementationGuide", "rest", "messaging", "document" }) 065public class CapabilityStatement extends MetadataResource implements IBaseConformance { 066 067 public enum CapabilityStatementKind { 068 /** 069 * The CapabilityStatement instance represents the present capabilities of a 070 * specific system instance. This is the kind returned by /metadata for a FHIR 071 * server end-point. 072 */ 073 INSTANCE, 074 /** 075 * The CapabilityStatement instance represents the capabilities of a system or 076 * piece of software, independent of a particular installation. 077 */ 078 CAPABILITY, 079 /** 080 * The CapabilityStatement instance represents a set of requirements for other 081 * systems to meet; e.g. as part of an implementation guide or 'request for 082 * proposal'. 083 */ 084 REQUIREMENTS, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 090 public static CapabilityStatementKind fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("instance".equals(codeString)) 094 return INSTANCE; 095 if ("capability".equals(codeString)) 096 return CAPABILITY; 097 if ("requirements".equals(codeString)) 098 return REQUIREMENTS; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown CapabilityStatementKind code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case INSTANCE: 108 return "instance"; 109 case CAPABILITY: 110 return "capability"; 111 case REQUIREMENTS: 112 return "requirements"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case INSTANCE: 123 return "http://hl7.org/fhir/capability-statement-kind"; 124 case CAPABILITY: 125 return "http://hl7.org/fhir/capability-statement-kind"; 126 case REQUIREMENTS: 127 return "http://hl7.org/fhir/capability-statement-kind"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case INSTANCE: 138 return "The CapabilityStatement instance represents the present capabilities of a specific system instance. This is the kind returned by /metadata for a FHIR server end-point."; 139 case CAPABILITY: 140 return "The CapabilityStatement instance represents the capabilities of a system or piece of software, independent of a particular installation."; 141 case REQUIREMENTS: 142 return "The CapabilityStatement instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'."; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getDisplay() { 151 switch (this) { 152 case INSTANCE: 153 return "Instance"; 154 case CAPABILITY: 155 return "Capability"; 156 case REQUIREMENTS: 157 return "Requirements"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 } 165 166 public static class CapabilityStatementKindEnumFactory implements EnumFactory<CapabilityStatementKind> { 167 public CapabilityStatementKind fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("instance".equals(codeString)) 172 return CapabilityStatementKind.INSTANCE; 173 if ("capability".equals(codeString)) 174 return CapabilityStatementKind.CAPABILITY; 175 if ("requirements".equals(codeString)) 176 return CapabilityStatementKind.REQUIREMENTS; 177 throw new IllegalArgumentException("Unknown CapabilityStatementKind code '" + codeString + "'"); 178 } 179 180 public Enumeration<CapabilityStatementKind> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.NULL, code); 188 if ("instance".equals(codeString)) 189 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.INSTANCE, code); 190 if ("capability".equals(codeString)) 191 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.CAPABILITY, code); 192 if ("requirements".equals(codeString)) 193 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.REQUIREMENTS, code); 194 throw new FHIRException("Unknown CapabilityStatementKind code '" + codeString + "'"); 195 } 196 197 public String toCode(CapabilityStatementKind code) { 198 if (code == CapabilityStatementKind.NULL) 199 return null; 200 if (code == CapabilityStatementKind.INSTANCE) 201 return "instance"; 202 if (code == CapabilityStatementKind.CAPABILITY) 203 return "capability"; 204 if (code == CapabilityStatementKind.REQUIREMENTS) 205 return "requirements"; 206 return "?"; 207 } 208 209 public String toSystem(CapabilityStatementKind code) { 210 return code.getSystem(); 211 } 212 } 213 214 public enum RestfulCapabilityMode { 215 /** 216 * The application acts as a client for this resource. 217 */ 218 CLIENT, 219 /** 220 * The application acts as a server for this resource. 221 */ 222 SERVER, 223 /** 224 * added to help the parsers with the generic types 225 */ 226 NULL; 227 228 public static RestfulCapabilityMode fromCode(String codeString) throws FHIRException { 229 if (codeString == null || "".equals(codeString)) 230 return null; 231 if ("client".equals(codeString)) 232 return CLIENT; 233 if ("server".equals(codeString)) 234 return SERVER; 235 if (Configuration.isAcceptInvalidEnums()) 236 return null; 237 else 238 throw new FHIRException("Unknown RestfulCapabilityMode code '" + codeString + "'"); 239 } 240 241 public String toCode() { 242 switch (this) { 243 case CLIENT: 244 return "client"; 245 case SERVER: 246 return "server"; 247 case NULL: 248 return null; 249 default: 250 return "?"; 251 } 252 } 253 254 public String getSystem() { 255 switch (this) { 256 case CLIENT: 257 return "http://hl7.org/fhir/restful-capability-mode"; 258 case SERVER: 259 return "http://hl7.org/fhir/restful-capability-mode"; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getDefinition() { 268 switch (this) { 269 case CLIENT: 270 return "The application acts as a client for this resource."; 271 case SERVER: 272 return "The application acts as a server for this resource."; 273 case NULL: 274 return null; 275 default: 276 return "?"; 277 } 278 } 279 280 public String getDisplay() { 281 switch (this) { 282 case CLIENT: 283 return "Client"; 284 case SERVER: 285 return "Server"; 286 case NULL: 287 return null; 288 default: 289 return "?"; 290 } 291 } 292 } 293 294 public static class RestfulCapabilityModeEnumFactory implements EnumFactory<RestfulCapabilityMode> { 295 public RestfulCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 296 if (codeString == null || "".equals(codeString)) 297 if (codeString == null || "".equals(codeString)) 298 return null; 299 if ("client".equals(codeString)) 300 return RestfulCapabilityMode.CLIENT; 301 if ("server".equals(codeString)) 302 return RestfulCapabilityMode.SERVER; 303 throw new IllegalArgumentException("Unknown RestfulCapabilityMode code '" + codeString + "'"); 304 } 305 306 public Enumeration<RestfulCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 307 if (code == null) 308 return null; 309 if (code.isEmpty()) 310 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.NULL, code); 311 String codeString = code.asStringValue(); 312 if (codeString == null || "".equals(codeString)) 313 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.NULL, code); 314 if ("client".equals(codeString)) 315 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.CLIENT, code); 316 if ("server".equals(codeString)) 317 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.SERVER, code); 318 throw new FHIRException("Unknown RestfulCapabilityMode code '" + codeString + "'"); 319 } 320 321 public String toCode(RestfulCapabilityMode code) { 322 if (code == RestfulCapabilityMode.NULL) 323 return null; 324 if (code == RestfulCapabilityMode.CLIENT) 325 return "client"; 326 if (code == RestfulCapabilityMode.SERVER) 327 return "server"; 328 return "?"; 329 } 330 331 public String toSystem(RestfulCapabilityMode code) { 332 return code.getSystem(); 333 } 334 } 335 336 public enum TypeRestfulInteraction { 337 /** 338 * null 339 */ 340 READ, 341 /** 342 * null 343 */ 344 VREAD, 345 /** 346 * null 347 */ 348 UPDATE, 349 /** 350 * null 351 */ 352 PATCH, 353 /** 354 * null 355 */ 356 DELETE, 357 /** 358 * null 359 */ 360 HISTORYINSTANCE, 361 /** 362 * null 363 */ 364 HISTORYTYPE, 365 /** 366 * null 367 */ 368 CREATE, 369 /** 370 * null 371 */ 372 SEARCHTYPE, 373 /** 374 * added to help the parsers with the generic types 375 */ 376 NULL; 377 378 public static TypeRestfulInteraction fromCode(String codeString) throws FHIRException { 379 if (codeString == null || "".equals(codeString)) 380 return null; 381 if ("read".equals(codeString)) 382 return READ; 383 if ("vread".equals(codeString)) 384 return VREAD; 385 if ("update".equals(codeString)) 386 return UPDATE; 387 if ("patch".equals(codeString)) 388 return PATCH; 389 if ("delete".equals(codeString)) 390 return DELETE; 391 if ("history-instance".equals(codeString)) 392 return HISTORYINSTANCE; 393 if ("history-type".equals(codeString)) 394 return HISTORYTYPE; 395 if ("create".equals(codeString)) 396 return CREATE; 397 if ("search-type".equals(codeString)) 398 return SEARCHTYPE; 399 if (Configuration.isAcceptInvalidEnums()) 400 return null; 401 else 402 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 403 } 404 405 public String toCode() { 406 switch (this) { 407 case READ: 408 return "read"; 409 case VREAD: 410 return "vread"; 411 case UPDATE: 412 return "update"; 413 case PATCH: 414 return "patch"; 415 case DELETE: 416 return "delete"; 417 case HISTORYINSTANCE: 418 return "history-instance"; 419 case HISTORYTYPE: 420 return "history-type"; 421 case CREATE: 422 return "create"; 423 case SEARCHTYPE: 424 return "search-type"; 425 case NULL: 426 return null; 427 default: 428 return "?"; 429 } 430 } 431 432 public String getSystem() { 433 switch (this) { 434 case READ: 435 return "http://hl7.org/fhir/restful-interaction"; 436 case VREAD: 437 return "http://hl7.org/fhir/restful-interaction"; 438 case UPDATE: 439 return "http://hl7.org/fhir/restful-interaction"; 440 case PATCH: 441 return "http://hl7.org/fhir/restful-interaction"; 442 case DELETE: 443 return "http://hl7.org/fhir/restful-interaction"; 444 case HISTORYINSTANCE: 445 return "http://hl7.org/fhir/restful-interaction"; 446 case HISTORYTYPE: 447 return "http://hl7.org/fhir/restful-interaction"; 448 case CREATE: 449 return "http://hl7.org/fhir/restful-interaction"; 450 case SEARCHTYPE: 451 return "http://hl7.org/fhir/restful-interaction"; 452 case NULL: 453 return null; 454 default: 455 return "?"; 456 } 457 } 458 459 public String getDefinition() { 460 switch (this) { 461 case READ: 462 return ""; 463 case VREAD: 464 return ""; 465 case UPDATE: 466 return ""; 467 case PATCH: 468 return ""; 469 case DELETE: 470 return ""; 471 case HISTORYINSTANCE: 472 return ""; 473 case HISTORYTYPE: 474 return ""; 475 case CREATE: 476 return ""; 477 case SEARCHTYPE: 478 return ""; 479 case NULL: 480 return null; 481 default: 482 return "?"; 483 } 484 } 485 486 public String getDisplay() { 487 switch (this) { 488 case READ: 489 return "read"; 490 case VREAD: 491 return "vread"; 492 case UPDATE: 493 return "update"; 494 case PATCH: 495 return "patch"; 496 case DELETE: 497 return "delete"; 498 case HISTORYINSTANCE: 499 return "history-instance"; 500 case HISTORYTYPE: 501 return "history-type"; 502 case CREATE: 503 return "create"; 504 case SEARCHTYPE: 505 return "search-type"; 506 case NULL: 507 return null; 508 default: 509 return "?"; 510 } 511 } 512 } 513 514 public static class TypeRestfulInteractionEnumFactory implements EnumFactory<TypeRestfulInteraction> { 515 public TypeRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 516 if (codeString == null || "".equals(codeString)) 517 if (codeString == null || "".equals(codeString)) 518 return null; 519 if ("read".equals(codeString)) 520 return TypeRestfulInteraction.READ; 521 if ("vread".equals(codeString)) 522 return TypeRestfulInteraction.VREAD; 523 if ("update".equals(codeString)) 524 return TypeRestfulInteraction.UPDATE; 525 if ("patch".equals(codeString)) 526 return TypeRestfulInteraction.PATCH; 527 if ("delete".equals(codeString)) 528 return TypeRestfulInteraction.DELETE; 529 if ("history-instance".equals(codeString)) 530 return TypeRestfulInteraction.HISTORYINSTANCE; 531 if ("history-type".equals(codeString)) 532 return TypeRestfulInteraction.HISTORYTYPE; 533 if ("create".equals(codeString)) 534 return TypeRestfulInteraction.CREATE; 535 if ("search-type".equals(codeString)) 536 return TypeRestfulInteraction.SEARCHTYPE; 537 throw new IllegalArgumentException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 538 } 539 540 public Enumeration<TypeRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 541 if (code == null) 542 return null; 543 if (code.isEmpty()) 544 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.NULL, code); 545 String codeString = code.asStringValue(); 546 if (codeString == null || "".equals(codeString)) 547 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.NULL, code); 548 if ("read".equals(codeString)) 549 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.READ, code); 550 if ("vread".equals(codeString)) 551 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VREAD, code); 552 if ("update".equals(codeString)) 553 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.UPDATE, code); 554 if ("patch".equals(codeString)) 555 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.PATCH, code); 556 if ("delete".equals(codeString)) 557 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.DELETE, code); 558 if ("history-instance".equals(codeString)) 559 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYINSTANCE, code); 560 if ("history-type".equals(codeString)) 561 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYTYPE, code); 562 if ("create".equals(codeString)) 563 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.CREATE, code); 564 if ("search-type".equals(codeString)) 565 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.SEARCHTYPE, code); 566 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 567 } 568 569 public String toCode(TypeRestfulInteraction code) { 570 if (code == TypeRestfulInteraction.NULL) 571 return null; 572 if (code == TypeRestfulInteraction.READ) 573 return "read"; 574 if (code == TypeRestfulInteraction.VREAD) 575 return "vread"; 576 if (code == TypeRestfulInteraction.UPDATE) 577 return "update"; 578 if (code == TypeRestfulInteraction.PATCH) 579 return "patch"; 580 if (code == TypeRestfulInteraction.DELETE) 581 return "delete"; 582 if (code == TypeRestfulInteraction.HISTORYINSTANCE) 583 return "history-instance"; 584 if (code == TypeRestfulInteraction.HISTORYTYPE) 585 return "history-type"; 586 if (code == TypeRestfulInteraction.CREATE) 587 return "create"; 588 if (code == TypeRestfulInteraction.SEARCHTYPE) 589 return "search-type"; 590 return "?"; 591 } 592 593 public String toSystem(TypeRestfulInteraction code) { 594 return code.getSystem(); 595 } 596 } 597 598 public enum ResourceVersionPolicy { 599 /** 600 * VersionId meta-property is not supported (server) or used (client). 601 */ 602 NOVERSION, 603 /** 604 * VersionId meta-property is supported (server) or used (client). 605 */ 606 VERSIONED, 607 /** 608 * VersionId must be correct for updates (server) or will be specified (If-match 609 * header) for updates (client). 610 */ 611 VERSIONEDUPDATE, 612 /** 613 * added to help the parsers with the generic types 614 */ 615 NULL; 616 617 public static ResourceVersionPolicy fromCode(String codeString) throws FHIRException { 618 if (codeString == null || "".equals(codeString)) 619 return null; 620 if ("no-version".equals(codeString)) 621 return NOVERSION; 622 if ("versioned".equals(codeString)) 623 return VERSIONED; 624 if ("versioned-update".equals(codeString)) 625 return VERSIONEDUPDATE; 626 if (Configuration.isAcceptInvalidEnums()) 627 return null; 628 else 629 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 630 } 631 632 public String toCode() { 633 switch (this) { 634 case NOVERSION: 635 return "no-version"; 636 case VERSIONED: 637 return "versioned"; 638 case VERSIONEDUPDATE: 639 return "versioned-update"; 640 case NULL: 641 return null; 642 default: 643 return "?"; 644 } 645 } 646 647 public String getSystem() { 648 switch (this) { 649 case NOVERSION: 650 return "http://hl7.org/fhir/versioning-policy"; 651 case VERSIONED: 652 return "http://hl7.org/fhir/versioning-policy"; 653 case VERSIONEDUPDATE: 654 return "http://hl7.org/fhir/versioning-policy"; 655 case NULL: 656 return null; 657 default: 658 return "?"; 659 } 660 } 661 662 public String getDefinition() { 663 switch (this) { 664 case NOVERSION: 665 return "VersionId meta-property is not supported (server) or used (client)."; 666 case VERSIONED: 667 return "VersionId meta-property is supported (server) or used (client)."; 668 case VERSIONEDUPDATE: 669 return "VersionId must be correct for updates (server) or will be specified (If-match header) for updates (client)."; 670 case NULL: 671 return null; 672 default: 673 return "?"; 674 } 675 } 676 677 public String getDisplay() { 678 switch (this) { 679 case NOVERSION: 680 return "No VersionId Support"; 681 case VERSIONED: 682 return "Versioned"; 683 case VERSIONEDUPDATE: 684 return "VersionId tracked fully"; 685 case NULL: 686 return null; 687 default: 688 return "?"; 689 } 690 } 691 } 692 693 public static class ResourceVersionPolicyEnumFactory implements EnumFactory<ResourceVersionPolicy> { 694 public ResourceVersionPolicy fromCode(String codeString) throws IllegalArgumentException { 695 if (codeString == null || "".equals(codeString)) 696 if (codeString == null || "".equals(codeString)) 697 return null; 698 if ("no-version".equals(codeString)) 699 return ResourceVersionPolicy.NOVERSION; 700 if ("versioned".equals(codeString)) 701 return ResourceVersionPolicy.VERSIONED; 702 if ("versioned-update".equals(codeString)) 703 return ResourceVersionPolicy.VERSIONEDUPDATE; 704 throw new IllegalArgumentException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 705 } 706 707 public Enumeration<ResourceVersionPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 708 if (code == null) 709 return null; 710 if (code.isEmpty()) 711 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NULL, code); 712 String codeString = code.asStringValue(); 713 if (codeString == null || "".equals(codeString)) 714 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NULL, code); 715 if ("no-version".equals(codeString)) 716 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NOVERSION, code); 717 if ("versioned".equals(codeString)) 718 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONED, code); 719 if ("versioned-update".equals(codeString)) 720 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONEDUPDATE, code); 721 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 722 } 723 724 public String toCode(ResourceVersionPolicy code) { 725 if (code == ResourceVersionPolicy.NULL) 726 return null; 727 if (code == ResourceVersionPolicy.NOVERSION) 728 return "no-version"; 729 if (code == ResourceVersionPolicy.VERSIONED) 730 return "versioned"; 731 if (code == ResourceVersionPolicy.VERSIONEDUPDATE) 732 return "versioned-update"; 733 return "?"; 734 } 735 736 public String toSystem(ResourceVersionPolicy code) { 737 return code.getSystem(); 738 } 739 } 740 741 public enum ConditionalReadStatus { 742 /** 743 * No support for conditional reads. 744 */ 745 NOTSUPPORTED, 746 /** 747 * Conditional reads are supported, but only with the If-Modified-Since HTTP 748 * Header. 749 */ 750 MODIFIEDSINCE, 751 /** 752 * Conditional reads are supported, but only with the If-None-Match HTTP Header. 753 */ 754 NOTMATCH, 755 /** 756 * Conditional reads are supported, with both If-Modified-Since and 757 * If-None-Match HTTP Headers. 758 */ 759 FULLSUPPORT, 760 /** 761 * added to help the parsers with the generic types 762 */ 763 NULL; 764 765 public static ConditionalReadStatus fromCode(String codeString) throws FHIRException { 766 if (codeString == null || "".equals(codeString)) 767 return null; 768 if ("not-supported".equals(codeString)) 769 return NOTSUPPORTED; 770 if ("modified-since".equals(codeString)) 771 return MODIFIEDSINCE; 772 if ("not-match".equals(codeString)) 773 return NOTMATCH; 774 if ("full-support".equals(codeString)) 775 return FULLSUPPORT; 776 if (Configuration.isAcceptInvalidEnums()) 777 return null; 778 else 779 throw new FHIRException("Unknown ConditionalReadStatus code '" + codeString + "'"); 780 } 781 782 public String toCode() { 783 switch (this) { 784 case NOTSUPPORTED: 785 return "not-supported"; 786 case MODIFIEDSINCE: 787 return "modified-since"; 788 case NOTMATCH: 789 return "not-match"; 790 case FULLSUPPORT: 791 return "full-support"; 792 case NULL: 793 return null; 794 default: 795 return "?"; 796 } 797 } 798 799 public String getSystem() { 800 switch (this) { 801 case NOTSUPPORTED: 802 return "http://hl7.org/fhir/conditional-read-status"; 803 case MODIFIEDSINCE: 804 return "http://hl7.org/fhir/conditional-read-status"; 805 case NOTMATCH: 806 return "http://hl7.org/fhir/conditional-read-status"; 807 case FULLSUPPORT: 808 return "http://hl7.org/fhir/conditional-read-status"; 809 case NULL: 810 return null; 811 default: 812 return "?"; 813 } 814 } 815 816 public String getDefinition() { 817 switch (this) { 818 case NOTSUPPORTED: 819 return "No support for conditional reads."; 820 case MODIFIEDSINCE: 821 return "Conditional reads are supported, but only with the If-Modified-Since HTTP Header."; 822 case NOTMATCH: 823 return "Conditional reads are supported, but only with the If-None-Match HTTP Header."; 824 case FULLSUPPORT: 825 return "Conditional reads are supported, with both If-Modified-Since and If-None-Match HTTP Headers."; 826 case NULL: 827 return null; 828 default: 829 return "?"; 830 } 831 } 832 833 public String getDisplay() { 834 switch (this) { 835 case NOTSUPPORTED: 836 return "Not Supported"; 837 case MODIFIEDSINCE: 838 return "If-Modified-Since"; 839 case NOTMATCH: 840 return "If-None-Match"; 841 case FULLSUPPORT: 842 return "Full Support"; 843 case NULL: 844 return null; 845 default: 846 return "?"; 847 } 848 } 849 } 850 851 public static class ConditionalReadStatusEnumFactory implements EnumFactory<ConditionalReadStatus> { 852 public ConditionalReadStatus fromCode(String codeString) throws IllegalArgumentException { 853 if (codeString == null || "".equals(codeString)) 854 if (codeString == null || "".equals(codeString)) 855 return null; 856 if ("not-supported".equals(codeString)) 857 return ConditionalReadStatus.NOTSUPPORTED; 858 if ("modified-since".equals(codeString)) 859 return ConditionalReadStatus.MODIFIEDSINCE; 860 if ("not-match".equals(codeString)) 861 return ConditionalReadStatus.NOTMATCH; 862 if ("full-support".equals(codeString)) 863 return ConditionalReadStatus.FULLSUPPORT; 864 throw new IllegalArgumentException("Unknown ConditionalReadStatus code '" + codeString + "'"); 865 } 866 867 public Enumeration<ConditionalReadStatus> fromType(PrimitiveType<?> code) throws FHIRException { 868 if (code == null) 869 return null; 870 if (code.isEmpty()) 871 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NULL, code); 872 String codeString = code.asStringValue(); 873 if (codeString == null || "".equals(codeString)) 874 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NULL, code); 875 if ("not-supported".equals(codeString)) 876 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTSUPPORTED, code); 877 if ("modified-since".equals(codeString)) 878 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.MODIFIEDSINCE, code); 879 if ("not-match".equals(codeString)) 880 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTMATCH, code); 881 if ("full-support".equals(codeString)) 882 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.FULLSUPPORT, code); 883 throw new FHIRException("Unknown ConditionalReadStatus code '" + codeString + "'"); 884 } 885 886 public String toCode(ConditionalReadStatus code) { 887 if (code == ConditionalReadStatus.NULL) 888 return null; 889 if (code == ConditionalReadStatus.NOTSUPPORTED) 890 return "not-supported"; 891 if (code == ConditionalReadStatus.MODIFIEDSINCE) 892 return "modified-since"; 893 if (code == ConditionalReadStatus.NOTMATCH) 894 return "not-match"; 895 if (code == ConditionalReadStatus.FULLSUPPORT) 896 return "full-support"; 897 return "?"; 898 } 899 900 public String toSystem(ConditionalReadStatus code) { 901 return code.getSystem(); 902 } 903 } 904 905 public enum ConditionalDeleteStatus { 906 /** 907 * No support for conditional deletes. 908 */ 909 NOTSUPPORTED, 910 /** 911 * Conditional deletes are supported, but only single resources at a time. 912 */ 913 SINGLE, 914 /** 915 * Conditional deletes are supported, and multiple resources can be deleted in a 916 * single interaction. 917 */ 918 MULTIPLE, 919 /** 920 * added to help the parsers with the generic types 921 */ 922 NULL; 923 924 public static ConditionalDeleteStatus fromCode(String codeString) throws FHIRException { 925 if (codeString == null || "".equals(codeString)) 926 return null; 927 if ("not-supported".equals(codeString)) 928 return NOTSUPPORTED; 929 if ("single".equals(codeString)) 930 return SINGLE; 931 if ("multiple".equals(codeString)) 932 return MULTIPLE; 933 if (Configuration.isAcceptInvalidEnums()) 934 return null; 935 else 936 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 937 } 938 939 public String toCode() { 940 switch (this) { 941 case NOTSUPPORTED: 942 return "not-supported"; 943 case SINGLE: 944 return "single"; 945 case MULTIPLE: 946 return "multiple"; 947 case NULL: 948 return null; 949 default: 950 return "?"; 951 } 952 } 953 954 public String getSystem() { 955 switch (this) { 956 case NOTSUPPORTED: 957 return "http://hl7.org/fhir/conditional-delete-status"; 958 case SINGLE: 959 return "http://hl7.org/fhir/conditional-delete-status"; 960 case MULTIPLE: 961 return "http://hl7.org/fhir/conditional-delete-status"; 962 case NULL: 963 return null; 964 default: 965 return "?"; 966 } 967 } 968 969 public String getDefinition() { 970 switch (this) { 971 case NOTSUPPORTED: 972 return "No support for conditional deletes."; 973 case SINGLE: 974 return "Conditional deletes are supported, but only single resources at a time."; 975 case MULTIPLE: 976 return "Conditional deletes are supported, and multiple resources can be deleted in a single interaction."; 977 case NULL: 978 return null; 979 default: 980 return "?"; 981 } 982 } 983 984 public String getDisplay() { 985 switch (this) { 986 case NOTSUPPORTED: 987 return "Not Supported"; 988 case SINGLE: 989 return "Single Deletes Supported"; 990 case MULTIPLE: 991 return "Multiple Deletes Supported"; 992 case NULL: 993 return null; 994 default: 995 return "?"; 996 } 997 } 998 } 999 1000 public static class ConditionalDeleteStatusEnumFactory implements EnumFactory<ConditionalDeleteStatus> { 1001 public ConditionalDeleteStatus fromCode(String codeString) throws IllegalArgumentException { 1002 if (codeString == null || "".equals(codeString)) 1003 if (codeString == null || "".equals(codeString)) 1004 return null; 1005 if ("not-supported".equals(codeString)) 1006 return ConditionalDeleteStatus.NOTSUPPORTED; 1007 if ("single".equals(codeString)) 1008 return ConditionalDeleteStatus.SINGLE; 1009 if ("multiple".equals(codeString)) 1010 return ConditionalDeleteStatus.MULTIPLE; 1011 throw new IllegalArgumentException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 1012 } 1013 1014 public Enumeration<ConditionalDeleteStatus> fromType(PrimitiveType<?> code) throws FHIRException { 1015 if (code == null) 1016 return null; 1017 if (code.isEmpty()) 1018 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NULL, code); 1019 String codeString = code.asStringValue(); 1020 if (codeString == null || "".equals(codeString)) 1021 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NULL, code); 1022 if ("not-supported".equals(codeString)) 1023 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NOTSUPPORTED, code); 1024 if ("single".equals(codeString)) 1025 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.SINGLE, code); 1026 if ("multiple".equals(codeString)) 1027 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.MULTIPLE, code); 1028 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 1029 } 1030 1031 public String toCode(ConditionalDeleteStatus code) { 1032 if (code == ConditionalDeleteStatus.NULL) 1033 return null; 1034 if (code == ConditionalDeleteStatus.NOTSUPPORTED) 1035 return "not-supported"; 1036 if (code == ConditionalDeleteStatus.SINGLE) 1037 return "single"; 1038 if (code == ConditionalDeleteStatus.MULTIPLE) 1039 return "multiple"; 1040 return "?"; 1041 } 1042 1043 public String toSystem(ConditionalDeleteStatus code) { 1044 return code.getSystem(); 1045 } 1046 } 1047 1048 public enum ReferenceHandlingPolicy { 1049 /** 1050 * The server supports and populates Literal references (i.e. using 1051 * Reference.reference) where they are known (this code does not guarantee that 1052 * all references are literal; see 'enforced'). 1053 */ 1054 LITERAL, 1055 /** 1056 * The server allows logical references (i.e. using Reference.identifier). 1057 */ 1058 LOGICAL, 1059 /** 1060 * The server will attempt to resolve logical references to literal references - 1061 * i.e. converting Reference.identifier to Reference.reference (if resolution 1062 * fails, the server may still accept resources; see logical). 1063 */ 1064 RESOLVES, 1065 /** 1066 * The server enforces that references have integrity - e.g. it ensures that 1067 * references can always be resolved. This is typically the case for clinical 1068 * record systems, but often not the case for middleware/proxy systems. 1069 */ 1070 ENFORCED, 1071 /** 1072 * The server does not support references that point to other servers. 1073 */ 1074 LOCAL, 1075 /** 1076 * added to help the parsers with the generic types 1077 */ 1078 NULL; 1079 1080 public static ReferenceHandlingPolicy fromCode(String codeString) throws FHIRException { 1081 if (codeString == null || "".equals(codeString)) 1082 return null; 1083 if ("literal".equals(codeString)) 1084 return LITERAL; 1085 if ("logical".equals(codeString)) 1086 return LOGICAL; 1087 if ("resolves".equals(codeString)) 1088 return RESOLVES; 1089 if ("enforced".equals(codeString)) 1090 return ENFORCED; 1091 if ("local".equals(codeString)) 1092 return LOCAL; 1093 if (Configuration.isAcceptInvalidEnums()) 1094 return null; 1095 else 1096 throw new FHIRException("Unknown ReferenceHandlingPolicy code '" + codeString + "'"); 1097 } 1098 1099 public String toCode() { 1100 switch (this) { 1101 case LITERAL: 1102 return "literal"; 1103 case LOGICAL: 1104 return "logical"; 1105 case RESOLVES: 1106 return "resolves"; 1107 case ENFORCED: 1108 return "enforced"; 1109 case LOCAL: 1110 return "local"; 1111 case NULL: 1112 return null; 1113 default: 1114 return "?"; 1115 } 1116 } 1117 1118 public String getSystem() { 1119 switch (this) { 1120 case LITERAL: 1121 return "http://hl7.org/fhir/reference-handling-policy"; 1122 case LOGICAL: 1123 return "http://hl7.org/fhir/reference-handling-policy"; 1124 case RESOLVES: 1125 return "http://hl7.org/fhir/reference-handling-policy"; 1126 case ENFORCED: 1127 return "http://hl7.org/fhir/reference-handling-policy"; 1128 case LOCAL: 1129 return "http://hl7.org/fhir/reference-handling-policy"; 1130 case NULL: 1131 return null; 1132 default: 1133 return "?"; 1134 } 1135 } 1136 1137 public String getDefinition() { 1138 switch (this) { 1139 case LITERAL: 1140 return "The server supports and populates Literal references (i.e. using Reference.reference) where they are known (this code does not guarantee that all references are literal; see 'enforced')."; 1141 case LOGICAL: 1142 return "The server allows logical references (i.e. using Reference.identifier)."; 1143 case RESOLVES: 1144 return "The server will attempt to resolve logical references to literal references - i.e. converting Reference.identifier to Reference.reference (if resolution fails, the server may still accept resources; see logical)."; 1145 case ENFORCED: 1146 return "The server enforces that references have integrity - e.g. it ensures that references can always be resolved. This is typically the case for clinical record systems, but often not the case for middleware/proxy systems."; 1147 case LOCAL: 1148 return "The server does not support references that point to other servers."; 1149 case NULL: 1150 return null; 1151 default: 1152 return "?"; 1153 } 1154 } 1155 1156 public String getDisplay() { 1157 switch (this) { 1158 case LITERAL: 1159 return "Literal References"; 1160 case LOGICAL: 1161 return "Logical References"; 1162 case RESOLVES: 1163 return "Resolves References"; 1164 case ENFORCED: 1165 return "Reference Integrity Enforced"; 1166 case LOCAL: 1167 return "Local References Only"; 1168 case NULL: 1169 return null; 1170 default: 1171 return "?"; 1172 } 1173 } 1174 } 1175 1176 public static class ReferenceHandlingPolicyEnumFactory implements EnumFactory<ReferenceHandlingPolicy> { 1177 public ReferenceHandlingPolicy fromCode(String codeString) throws IllegalArgumentException { 1178 if (codeString == null || "".equals(codeString)) 1179 if (codeString == null || "".equals(codeString)) 1180 return null; 1181 if ("literal".equals(codeString)) 1182 return ReferenceHandlingPolicy.LITERAL; 1183 if ("logical".equals(codeString)) 1184 return ReferenceHandlingPolicy.LOGICAL; 1185 if ("resolves".equals(codeString)) 1186 return ReferenceHandlingPolicy.RESOLVES; 1187 if ("enforced".equals(codeString)) 1188 return ReferenceHandlingPolicy.ENFORCED; 1189 if ("local".equals(codeString)) 1190 return ReferenceHandlingPolicy.LOCAL; 1191 throw new IllegalArgumentException("Unknown ReferenceHandlingPolicy code '" + codeString + "'"); 1192 } 1193 1194 public Enumeration<ReferenceHandlingPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 1195 if (code == null) 1196 return null; 1197 if (code.isEmpty()) 1198 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.NULL, code); 1199 String codeString = code.asStringValue(); 1200 if (codeString == null || "".equals(codeString)) 1201 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.NULL, code); 1202 if ("literal".equals(codeString)) 1203 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LITERAL, code); 1204 if ("logical".equals(codeString)) 1205 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOGICAL, code); 1206 if ("resolves".equals(codeString)) 1207 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.RESOLVES, code); 1208 if ("enforced".equals(codeString)) 1209 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.ENFORCED, code); 1210 if ("local".equals(codeString)) 1211 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOCAL, code); 1212 throw new FHIRException("Unknown ReferenceHandlingPolicy code '" + codeString + "'"); 1213 } 1214 1215 public String toCode(ReferenceHandlingPolicy code) { 1216 if (code == ReferenceHandlingPolicy.NULL) 1217 return null; 1218 if (code == ReferenceHandlingPolicy.LITERAL) 1219 return "literal"; 1220 if (code == ReferenceHandlingPolicy.LOGICAL) 1221 return "logical"; 1222 if (code == ReferenceHandlingPolicy.RESOLVES) 1223 return "resolves"; 1224 if (code == ReferenceHandlingPolicy.ENFORCED) 1225 return "enforced"; 1226 if (code == ReferenceHandlingPolicy.LOCAL) 1227 return "local"; 1228 return "?"; 1229 } 1230 1231 public String toSystem(ReferenceHandlingPolicy code) { 1232 return code.getSystem(); 1233 } 1234 } 1235 1236 public enum SystemRestfulInteraction { 1237 /** 1238 * null 1239 */ 1240 TRANSACTION, 1241 /** 1242 * null 1243 */ 1244 BATCH, 1245 /** 1246 * null 1247 */ 1248 SEARCHSYSTEM, 1249 /** 1250 * null 1251 */ 1252 HISTORYSYSTEM, 1253 /** 1254 * added to help the parsers with the generic types 1255 */ 1256 NULL; 1257 1258 public static SystemRestfulInteraction fromCode(String codeString) throws FHIRException { 1259 if (codeString == null || "".equals(codeString)) 1260 return null; 1261 if ("transaction".equals(codeString)) 1262 return TRANSACTION; 1263 if ("batch".equals(codeString)) 1264 return BATCH; 1265 if ("search-system".equals(codeString)) 1266 return SEARCHSYSTEM; 1267 if ("history-system".equals(codeString)) 1268 return HISTORYSYSTEM; 1269 if (Configuration.isAcceptInvalidEnums()) 1270 return null; 1271 else 1272 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1273 } 1274 1275 public String toCode() { 1276 switch (this) { 1277 case TRANSACTION: 1278 return "transaction"; 1279 case BATCH: 1280 return "batch"; 1281 case SEARCHSYSTEM: 1282 return "search-system"; 1283 case HISTORYSYSTEM: 1284 return "history-system"; 1285 case NULL: 1286 return null; 1287 default: 1288 return "?"; 1289 } 1290 } 1291 1292 public String getSystem() { 1293 switch (this) { 1294 case TRANSACTION: 1295 return "http://hl7.org/fhir/restful-interaction"; 1296 case BATCH: 1297 return "http://hl7.org/fhir/restful-interaction"; 1298 case SEARCHSYSTEM: 1299 return "http://hl7.org/fhir/restful-interaction"; 1300 case HISTORYSYSTEM: 1301 return "http://hl7.org/fhir/restful-interaction"; 1302 case NULL: 1303 return null; 1304 default: 1305 return "?"; 1306 } 1307 } 1308 1309 public String getDefinition() { 1310 switch (this) { 1311 case TRANSACTION: 1312 return ""; 1313 case BATCH: 1314 return ""; 1315 case SEARCHSYSTEM: 1316 return ""; 1317 case HISTORYSYSTEM: 1318 return ""; 1319 case NULL: 1320 return null; 1321 default: 1322 return "?"; 1323 } 1324 } 1325 1326 public String getDisplay() { 1327 switch (this) { 1328 case TRANSACTION: 1329 return "transaction"; 1330 case BATCH: 1331 return "batch"; 1332 case SEARCHSYSTEM: 1333 return "search-system"; 1334 case HISTORYSYSTEM: 1335 return "history-system"; 1336 case NULL: 1337 return null; 1338 default: 1339 return "?"; 1340 } 1341 } 1342 } 1343 1344 public static class SystemRestfulInteractionEnumFactory implements EnumFactory<SystemRestfulInteraction> { 1345 public SystemRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 1346 if (codeString == null || "".equals(codeString)) 1347 if (codeString == null || "".equals(codeString)) 1348 return null; 1349 if ("transaction".equals(codeString)) 1350 return SystemRestfulInteraction.TRANSACTION; 1351 if ("batch".equals(codeString)) 1352 return SystemRestfulInteraction.BATCH; 1353 if ("search-system".equals(codeString)) 1354 return SystemRestfulInteraction.SEARCHSYSTEM; 1355 if ("history-system".equals(codeString)) 1356 return SystemRestfulInteraction.HISTORYSYSTEM; 1357 throw new IllegalArgumentException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1358 } 1359 1360 public Enumeration<SystemRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 1361 if (code == null) 1362 return null; 1363 if (code.isEmpty()) 1364 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.NULL, code); 1365 String codeString = code.asStringValue(); 1366 if (codeString == null || "".equals(codeString)) 1367 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.NULL, code); 1368 if ("transaction".equals(codeString)) 1369 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.TRANSACTION, code); 1370 if ("batch".equals(codeString)) 1371 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.BATCH, code); 1372 if ("search-system".equals(codeString)) 1373 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.SEARCHSYSTEM, code); 1374 if ("history-system".equals(codeString)) 1375 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.HISTORYSYSTEM, code); 1376 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1377 } 1378 1379 public String toCode(SystemRestfulInteraction code) { 1380 if (code == SystemRestfulInteraction.NULL) 1381 return null; 1382 if (code == SystemRestfulInteraction.TRANSACTION) 1383 return "transaction"; 1384 if (code == SystemRestfulInteraction.BATCH) 1385 return "batch"; 1386 if (code == SystemRestfulInteraction.SEARCHSYSTEM) 1387 return "search-system"; 1388 if (code == SystemRestfulInteraction.HISTORYSYSTEM) 1389 return "history-system"; 1390 return "?"; 1391 } 1392 1393 public String toSystem(SystemRestfulInteraction code) { 1394 return code.getSystem(); 1395 } 1396 } 1397 1398 public enum EventCapabilityMode { 1399 /** 1400 * The application sends requests and receives responses. 1401 */ 1402 SENDER, 1403 /** 1404 * The application receives requests and sends responses. 1405 */ 1406 RECEIVER, 1407 /** 1408 * added to help the parsers with the generic types 1409 */ 1410 NULL; 1411 1412 public static EventCapabilityMode fromCode(String codeString) throws FHIRException { 1413 if (codeString == null || "".equals(codeString)) 1414 return null; 1415 if ("sender".equals(codeString)) 1416 return SENDER; 1417 if ("receiver".equals(codeString)) 1418 return RECEIVER; 1419 if (Configuration.isAcceptInvalidEnums()) 1420 return null; 1421 else 1422 throw new FHIRException("Unknown EventCapabilityMode code '" + codeString + "'"); 1423 } 1424 1425 public String toCode() { 1426 switch (this) { 1427 case SENDER: 1428 return "sender"; 1429 case RECEIVER: 1430 return "receiver"; 1431 case NULL: 1432 return null; 1433 default: 1434 return "?"; 1435 } 1436 } 1437 1438 public String getSystem() { 1439 switch (this) { 1440 case SENDER: 1441 return "http://hl7.org/fhir/event-capability-mode"; 1442 case RECEIVER: 1443 return "http://hl7.org/fhir/event-capability-mode"; 1444 case NULL: 1445 return null; 1446 default: 1447 return "?"; 1448 } 1449 } 1450 1451 public String getDefinition() { 1452 switch (this) { 1453 case SENDER: 1454 return "The application sends requests and receives responses."; 1455 case RECEIVER: 1456 return "The application receives requests and sends responses."; 1457 case NULL: 1458 return null; 1459 default: 1460 return "?"; 1461 } 1462 } 1463 1464 public String getDisplay() { 1465 switch (this) { 1466 case SENDER: 1467 return "Sender"; 1468 case RECEIVER: 1469 return "Receiver"; 1470 case NULL: 1471 return null; 1472 default: 1473 return "?"; 1474 } 1475 } 1476 } 1477 1478 public static class EventCapabilityModeEnumFactory implements EnumFactory<EventCapabilityMode> { 1479 public EventCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 1480 if (codeString == null || "".equals(codeString)) 1481 if (codeString == null || "".equals(codeString)) 1482 return null; 1483 if ("sender".equals(codeString)) 1484 return EventCapabilityMode.SENDER; 1485 if ("receiver".equals(codeString)) 1486 return EventCapabilityMode.RECEIVER; 1487 throw new IllegalArgumentException("Unknown EventCapabilityMode code '" + codeString + "'"); 1488 } 1489 1490 public Enumeration<EventCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 1491 if (code == null) 1492 return null; 1493 if (code.isEmpty()) 1494 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.NULL, code); 1495 String codeString = code.asStringValue(); 1496 if (codeString == null || "".equals(codeString)) 1497 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.NULL, code); 1498 if ("sender".equals(codeString)) 1499 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.SENDER, code); 1500 if ("receiver".equals(codeString)) 1501 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.RECEIVER, code); 1502 throw new FHIRException("Unknown EventCapabilityMode code '" + codeString + "'"); 1503 } 1504 1505 public String toCode(EventCapabilityMode code) { 1506 if (code == EventCapabilityMode.NULL) 1507 return null; 1508 if (code == EventCapabilityMode.SENDER) 1509 return "sender"; 1510 if (code == EventCapabilityMode.RECEIVER) 1511 return "receiver"; 1512 return "?"; 1513 } 1514 1515 public String toSystem(EventCapabilityMode code) { 1516 return code.getSystem(); 1517 } 1518 } 1519 1520 public enum DocumentMode { 1521 /** 1522 * The application produces documents of the specified type. 1523 */ 1524 PRODUCER, 1525 /** 1526 * The application consumes documents of the specified type. 1527 */ 1528 CONSUMER, 1529 /** 1530 * added to help the parsers with the generic types 1531 */ 1532 NULL; 1533 1534 public static DocumentMode fromCode(String codeString) throws FHIRException { 1535 if (codeString == null || "".equals(codeString)) 1536 return null; 1537 if ("producer".equals(codeString)) 1538 return PRODUCER; 1539 if ("consumer".equals(codeString)) 1540 return CONSUMER; 1541 if (Configuration.isAcceptInvalidEnums()) 1542 return null; 1543 else 1544 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1545 } 1546 1547 public String toCode() { 1548 switch (this) { 1549 case PRODUCER: 1550 return "producer"; 1551 case CONSUMER: 1552 return "consumer"; 1553 case NULL: 1554 return null; 1555 default: 1556 return "?"; 1557 } 1558 } 1559 1560 public String getSystem() { 1561 switch (this) { 1562 case PRODUCER: 1563 return "http://hl7.org/fhir/document-mode"; 1564 case CONSUMER: 1565 return "http://hl7.org/fhir/document-mode"; 1566 case NULL: 1567 return null; 1568 default: 1569 return "?"; 1570 } 1571 } 1572 1573 public String getDefinition() { 1574 switch (this) { 1575 case PRODUCER: 1576 return "The application produces documents of the specified type."; 1577 case CONSUMER: 1578 return "The application consumes documents of the specified type."; 1579 case NULL: 1580 return null; 1581 default: 1582 return "?"; 1583 } 1584 } 1585 1586 public String getDisplay() { 1587 switch (this) { 1588 case PRODUCER: 1589 return "Producer"; 1590 case CONSUMER: 1591 return "Consumer"; 1592 case NULL: 1593 return null; 1594 default: 1595 return "?"; 1596 } 1597 } 1598 } 1599 1600 public static class DocumentModeEnumFactory implements EnumFactory<DocumentMode> { 1601 public DocumentMode fromCode(String codeString) throws IllegalArgumentException { 1602 if (codeString == null || "".equals(codeString)) 1603 if (codeString == null || "".equals(codeString)) 1604 return null; 1605 if ("producer".equals(codeString)) 1606 return DocumentMode.PRODUCER; 1607 if ("consumer".equals(codeString)) 1608 return DocumentMode.CONSUMER; 1609 throw new IllegalArgumentException("Unknown DocumentMode code '" + codeString + "'"); 1610 } 1611 1612 public Enumeration<DocumentMode> fromType(PrimitiveType<?> code) throws FHIRException { 1613 if (code == null) 1614 return null; 1615 if (code.isEmpty()) 1616 return new Enumeration<DocumentMode>(this, DocumentMode.NULL, code); 1617 String codeString = code.asStringValue(); 1618 if (codeString == null || "".equals(codeString)) 1619 return new Enumeration<DocumentMode>(this, DocumentMode.NULL, code); 1620 if ("producer".equals(codeString)) 1621 return new Enumeration<DocumentMode>(this, DocumentMode.PRODUCER, code); 1622 if ("consumer".equals(codeString)) 1623 return new Enumeration<DocumentMode>(this, DocumentMode.CONSUMER, code); 1624 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1625 } 1626 1627 public String toCode(DocumentMode code) { 1628 if (code == DocumentMode.NULL) 1629 return null; 1630 if (code == DocumentMode.PRODUCER) 1631 return "producer"; 1632 if (code == DocumentMode.CONSUMER) 1633 return "consumer"; 1634 return "?"; 1635 } 1636 1637 public String toSystem(DocumentMode code) { 1638 return code.getSystem(); 1639 } 1640 } 1641 1642 @Block() 1643 public static class CapabilityStatementSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 1644 /** 1645 * Name the software is known by. 1646 */ 1647 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1648 @Description(shortDefinition = "A name the software is known by", formalDefinition = "Name the software is known by.") 1649 protected StringType name; 1650 1651 /** 1652 * The version identifier for the software covered by this statement. 1653 */ 1654 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1655 @Description(shortDefinition = "Version covered by this statement", formalDefinition = "The version identifier for the software covered by this statement.") 1656 protected StringType version; 1657 1658 /** 1659 * Date this version of the software was released. 1660 */ 1661 @Child(name = "releaseDate", type = { 1662 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1663 @Description(shortDefinition = "Date this version was released", formalDefinition = "Date this version of the software was released.") 1664 protected DateTimeType releaseDate; 1665 1666 private static final long serialVersionUID = 1819769027L; 1667 1668 /** 1669 * Constructor 1670 */ 1671 public CapabilityStatementSoftwareComponent() { 1672 super(); 1673 } 1674 1675 /** 1676 * Constructor 1677 */ 1678 public CapabilityStatementSoftwareComponent(StringType name) { 1679 super(); 1680 this.name = name; 1681 } 1682 1683 /** 1684 * @return {@link #name} (Name the software is known by.). This is the 1685 * underlying object with id, value and extensions. The accessor 1686 * "getName" gives direct access to the value 1687 */ 1688 public StringType getNameElement() { 1689 if (this.name == null) 1690 if (Configuration.errorOnAutoCreate()) 1691 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.name"); 1692 else if (Configuration.doAutoCreate()) 1693 this.name = new StringType(); // bb 1694 return this.name; 1695 } 1696 1697 public boolean hasNameElement() { 1698 return this.name != null && !this.name.isEmpty(); 1699 } 1700 1701 public boolean hasName() { 1702 return this.name != null && !this.name.isEmpty(); 1703 } 1704 1705 /** 1706 * @param value {@link #name} (Name the software is known by.). This is the 1707 * underlying object with id, value and extensions. The accessor 1708 * "getName" gives direct access to the value 1709 */ 1710 public CapabilityStatementSoftwareComponent setNameElement(StringType value) { 1711 this.name = value; 1712 return this; 1713 } 1714 1715 /** 1716 * @return Name the software is known by. 1717 */ 1718 public String getName() { 1719 return this.name == null ? null : this.name.getValue(); 1720 } 1721 1722 /** 1723 * @param value Name the software is known by. 1724 */ 1725 public CapabilityStatementSoftwareComponent setName(String value) { 1726 if (this.name == null) 1727 this.name = new StringType(); 1728 this.name.setValue(value); 1729 return this; 1730 } 1731 1732 /** 1733 * @return {@link #version} (The version identifier for the software covered by 1734 * this statement.). This is the underlying object with id, value and 1735 * extensions. The accessor "getVersion" gives direct access to the 1736 * value 1737 */ 1738 public StringType getVersionElement() { 1739 if (this.version == null) 1740 if (Configuration.errorOnAutoCreate()) 1741 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.version"); 1742 else if (Configuration.doAutoCreate()) 1743 this.version = new StringType(); // bb 1744 return this.version; 1745 } 1746 1747 public boolean hasVersionElement() { 1748 return this.version != null && !this.version.isEmpty(); 1749 } 1750 1751 public boolean hasVersion() { 1752 return this.version != null && !this.version.isEmpty(); 1753 } 1754 1755 /** 1756 * @param value {@link #version} (The version identifier for the software 1757 * covered by this statement.). This is the underlying object with 1758 * id, value and extensions. The accessor "getVersion" gives direct 1759 * access to the value 1760 */ 1761 public CapabilityStatementSoftwareComponent setVersionElement(StringType value) { 1762 this.version = value; 1763 return this; 1764 } 1765 1766 /** 1767 * @return The version identifier for the software covered by this statement. 1768 */ 1769 public String getVersion() { 1770 return this.version == null ? null : this.version.getValue(); 1771 } 1772 1773 /** 1774 * @param value The version identifier for the software covered by this 1775 * statement. 1776 */ 1777 public CapabilityStatementSoftwareComponent setVersion(String value) { 1778 if (Utilities.noString(value)) 1779 this.version = null; 1780 else { 1781 if (this.version == null) 1782 this.version = new StringType(); 1783 this.version.setValue(value); 1784 } 1785 return this; 1786 } 1787 1788 /** 1789 * @return {@link #releaseDate} (Date this version of the software was 1790 * released.). This is the underlying object with id, value and 1791 * extensions. The accessor "getReleaseDate" gives direct access to the 1792 * value 1793 */ 1794 public DateTimeType getReleaseDateElement() { 1795 if (this.releaseDate == null) 1796 if (Configuration.errorOnAutoCreate()) 1797 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.releaseDate"); 1798 else if (Configuration.doAutoCreate()) 1799 this.releaseDate = new DateTimeType(); // bb 1800 return this.releaseDate; 1801 } 1802 1803 public boolean hasReleaseDateElement() { 1804 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1805 } 1806 1807 public boolean hasReleaseDate() { 1808 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1809 } 1810 1811 /** 1812 * @param value {@link #releaseDate} (Date this version of the software was 1813 * released.). This is the underlying object with id, value and 1814 * extensions. The accessor "getReleaseDate" gives direct access to 1815 * the value 1816 */ 1817 public CapabilityStatementSoftwareComponent setReleaseDateElement(DateTimeType value) { 1818 this.releaseDate = value; 1819 return this; 1820 } 1821 1822 /** 1823 * @return Date this version of the software was released. 1824 */ 1825 public Date getReleaseDate() { 1826 return this.releaseDate == null ? null : this.releaseDate.getValue(); 1827 } 1828 1829 /** 1830 * @param value Date this version of the software was released. 1831 */ 1832 public CapabilityStatementSoftwareComponent setReleaseDate(Date value) { 1833 if (value == null) 1834 this.releaseDate = null; 1835 else { 1836 if (this.releaseDate == null) 1837 this.releaseDate = new DateTimeType(); 1838 this.releaseDate.setValue(value); 1839 } 1840 return this; 1841 } 1842 1843 protected void listChildren(List<Property> children) { 1844 super.listChildren(children); 1845 children.add(new Property("name", "string", "Name the software is known by.", 0, 1, name)); 1846 children.add(new Property("version", "string", 1847 "The version identifier for the software covered by this statement.", 0, 1, version)); 1848 children.add(new Property("releaseDate", "dateTime", "Date this version of the software was released.", 0, 1, 1849 releaseDate)); 1850 } 1851 1852 @Override 1853 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1854 switch (_hash) { 1855 case 3373707: 1856 /* name */ return new Property("name", "string", "Name the software is known by.", 0, 1, name); 1857 case 351608024: 1858 /* version */ return new Property("version", "string", 1859 "The version identifier for the software covered by this statement.", 0, 1, version); 1860 case 212873301: 1861 /* releaseDate */ return new Property("releaseDate", "dateTime", 1862 "Date this version of the software was released.", 0, 1, releaseDate); 1863 default: 1864 return super.getNamedProperty(_hash, _name, _checkValid); 1865 } 1866 1867 } 1868 1869 @Override 1870 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1871 switch (hash) { 1872 case 3373707: 1873 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1874 case 351608024: 1875 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1876 case 212873301: 1877 /* releaseDate */ return this.releaseDate == null ? new Base[0] : new Base[] { this.releaseDate }; // DateTimeType 1878 default: 1879 return super.getProperty(hash, name, checkValid); 1880 } 1881 1882 } 1883 1884 @Override 1885 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1886 switch (hash) { 1887 case 3373707: // name 1888 this.name = castToString(value); // StringType 1889 return value; 1890 case 351608024: // version 1891 this.version = castToString(value); // StringType 1892 return value; 1893 case 212873301: // releaseDate 1894 this.releaseDate = castToDateTime(value); // DateTimeType 1895 return value; 1896 default: 1897 return super.setProperty(hash, name, value); 1898 } 1899 1900 } 1901 1902 @Override 1903 public Base setProperty(String name, Base value) throws FHIRException { 1904 if (name.equals("name")) { 1905 this.name = castToString(value); // StringType 1906 } else if (name.equals("version")) { 1907 this.version = castToString(value); // StringType 1908 } else if (name.equals("releaseDate")) { 1909 this.releaseDate = castToDateTime(value); // DateTimeType 1910 } else 1911 return super.setProperty(name, value); 1912 return value; 1913 } 1914 1915 @Override 1916 public void removeChild(String name, Base value) throws FHIRException { 1917 if (name.equals("name")) { 1918 this.name = null; 1919 } else if (name.equals("version")) { 1920 this.version = null; 1921 } else if (name.equals("releaseDate")) { 1922 this.releaseDate = null; 1923 } else 1924 super.removeChild(name, value); 1925 1926 } 1927 1928 @Override 1929 public Base makeProperty(int hash, String name) throws FHIRException { 1930 switch (hash) { 1931 case 3373707: 1932 return getNameElement(); 1933 case 351608024: 1934 return getVersionElement(); 1935 case 212873301: 1936 return getReleaseDateElement(); 1937 default: 1938 return super.makeProperty(hash, name); 1939 } 1940 1941 } 1942 1943 @Override 1944 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1945 switch (hash) { 1946 case 3373707: 1947 /* name */ return new String[] { "string" }; 1948 case 351608024: 1949 /* version */ return new String[] { "string" }; 1950 case 212873301: 1951 /* releaseDate */ return new String[] { "dateTime" }; 1952 default: 1953 return super.getTypesForProperty(hash, name); 1954 } 1955 1956 } 1957 1958 @Override 1959 public Base addChild(String name) throws FHIRException { 1960 if (name.equals("name")) { 1961 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 1962 } else if (name.equals("version")) { 1963 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 1964 } else if (name.equals("releaseDate")) { 1965 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.releaseDate"); 1966 } else 1967 return super.addChild(name); 1968 } 1969 1970 public CapabilityStatementSoftwareComponent copy() { 1971 CapabilityStatementSoftwareComponent dst = new CapabilityStatementSoftwareComponent(); 1972 copyValues(dst); 1973 return dst; 1974 } 1975 1976 public void copyValues(CapabilityStatementSoftwareComponent dst) { 1977 super.copyValues(dst); 1978 dst.name = name == null ? null : name.copy(); 1979 dst.version = version == null ? null : version.copy(); 1980 dst.releaseDate = releaseDate == null ? null : releaseDate.copy(); 1981 } 1982 1983 @Override 1984 public boolean equalsDeep(Base other_) { 1985 if (!super.equalsDeep(other_)) 1986 return false; 1987 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1988 return false; 1989 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1990 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true) 1991 && compareDeep(releaseDate, o.releaseDate, true); 1992 } 1993 1994 @Override 1995 public boolean equalsShallow(Base other_) { 1996 if (!super.equalsShallow(other_)) 1997 return false; 1998 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1999 return false; 2000 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 2001 return compareValues(name, o.name, true) && compareValues(version, o.version, true) 2002 && compareValues(releaseDate, o.releaseDate, true); 2003 } 2004 2005 public boolean isEmpty() { 2006 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, version, releaseDate); 2007 } 2008 2009 public String fhirType() { 2010 return "CapabilityStatement.software"; 2011 2012 } 2013 2014 } 2015 2016 @Block() 2017 public static class CapabilityStatementImplementationComponent extends BackboneElement 2018 implements IBaseBackboneElement { 2019 /** 2020 * Information about the specific installation that this capability statement 2021 * relates to. 2022 */ 2023 @Child(name = "description", type = { 2024 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2025 @Description(shortDefinition = "Describes this specific instance", formalDefinition = "Information about the specific installation that this capability statement relates to.") 2026 protected StringType description; 2027 2028 /** 2029 * An absolute base URL for the implementation. This forms the base for REST 2030 * interfaces as well as the mailbox and document interfaces. 2031 */ 2032 @Child(name = "url", type = { UrlType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2033 @Description(shortDefinition = "Base URL for the installation", formalDefinition = "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.") 2034 protected UrlType url; 2035 2036 /** 2037 * The organization responsible for the management of the instance and oversight 2038 * of the data on the server at the specified URL. 2039 */ 2040 @Child(name = "custodian", type = { 2041 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2042 @Description(shortDefinition = "Organization that manages the data", formalDefinition = "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.") 2043 protected Reference custodian; 2044 2045 /** 2046 * The actual object that is the target of the reference (The organization 2047 * responsible for the management of the instance and oversight of the data on 2048 * the server at the specified URL.) 2049 */ 2050 protected Organization custodianTarget; 2051 2052 private static final long serialVersionUID = -1705695694L; 2053 2054 /** 2055 * Constructor 2056 */ 2057 public CapabilityStatementImplementationComponent() { 2058 super(); 2059 } 2060 2061 /** 2062 * Constructor 2063 */ 2064 public CapabilityStatementImplementationComponent(StringType description) { 2065 super(); 2066 this.description = description; 2067 } 2068 2069 /** 2070 * @return {@link #description} (Information about the specific installation 2071 * that this capability statement relates to.). This is the underlying 2072 * object with id, value and extensions. The accessor "getDescription" 2073 * gives direct access to the value 2074 */ 2075 public StringType getDescriptionElement() { 2076 if (this.description == null) 2077 if (Configuration.errorOnAutoCreate()) 2078 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.description"); 2079 else if (Configuration.doAutoCreate()) 2080 this.description = new StringType(); // bb 2081 return this.description; 2082 } 2083 2084 public boolean hasDescriptionElement() { 2085 return this.description != null && !this.description.isEmpty(); 2086 } 2087 2088 public boolean hasDescription() { 2089 return this.description != null && !this.description.isEmpty(); 2090 } 2091 2092 /** 2093 * @param value {@link #description} (Information about the specific 2094 * installation that this capability statement relates to.). This 2095 * is the underlying object with id, value and extensions. The 2096 * accessor "getDescription" gives direct access to the value 2097 */ 2098 public CapabilityStatementImplementationComponent setDescriptionElement(StringType value) { 2099 this.description = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return Information about the specific installation that this capability 2105 * statement relates to. 2106 */ 2107 public String getDescription() { 2108 return this.description == null ? null : this.description.getValue(); 2109 } 2110 2111 /** 2112 * @param value Information about the specific installation that this capability 2113 * statement relates to. 2114 */ 2115 public CapabilityStatementImplementationComponent setDescription(String value) { 2116 if (this.description == null) 2117 this.description = new StringType(); 2118 this.description.setValue(value); 2119 return this; 2120 } 2121 2122 /** 2123 * @return {@link #url} (An absolute base URL for the implementation. This forms 2124 * the base for REST interfaces as well as the mailbox and document 2125 * interfaces.). This is the underlying object with id, value and 2126 * extensions. The accessor "getUrl" gives direct access to the value 2127 */ 2128 public UrlType getUrlElement() { 2129 if (this.url == null) 2130 if (Configuration.errorOnAutoCreate()) 2131 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.url"); 2132 else if (Configuration.doAutoCreate()) 2133 this.url = new UrlType(); // bb 2134 return this.url; 2135 } 2136 2137 public boolean hasUrlElement() { 2138 return this.url != null && !this.url.isEmpty(); 2139 } 2140 2141 public boolean hasUrl() { 2142 return this.url != null && !this.url.isEmpty(); 2143 } 2144 2145 /** 2146 * @param value {@link #url} (An absolute base URL for the implementation. This 2147 * forms the base for REST interfaces as well as the mailbox and 2148 * document interfaces.). This is the underlying object with id, 2149 * value and extensions. The accessor "getUrl" gives direct access 2150 * to the value 2151 */ 2152 public CapabilityStatementImplementationComponent setUrlElement(UrlType value) { 2153 this.url = value; 2154 return this; 2155 } 2156 2157 /** 2158 * @return An absolute base URL for the implementation. This forms the base for 2159 * REST interfaces as well as the mailbox and document interfaces. 2160 */ 2161 public String getUrl() { 2162 return this.url == null ? null : this.url.getValue(); 2163 } 2164 2165 /** 2166 * @param value An absolute base URL for the implementation. This forms the base 2167 * for REST interfaces as well as the mailbox and document 2168 * interfaces. 2169 */ 2170 public CapabilityStatementImplementationComponent setUrl(String value) { 2171 if (Utilities.noString(value)) 2172 this.url = null; 2173 else { 2174 if (this.url == null) 2175 this.url = new UrlType(); 2176 this.url.setValue(value); 2177 } 2178 return this; 2179 } 2180 2181 /** 2182 * @return {@link #custodian} (The organization responsible for the management 2183 * of the instance and oversight of the data on the server at the 2184 * specified URL.) 2185 */ 2186 public Reference getCustodian() { 2187 if (this.custodian == null) 2188 if (Configuration.errorOnAutoCreate()) 2189 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.custodian"); 2190 else if (Configuration.doAutoCreate()) 2191 this.custodian = new Reference(); // cc 2192 return this.custodian; 2193 } 2194 2195 public boolean hasCustodian() { 2196 return this.custodian != null && !this.custodian.isEmpty(); 2197 } 2198 2199 /** 2200 * @param value {@link #custodian} (The organization responsible for the 2201 * management of the instance and oversight of the data on the 2202 * server at the specified URL.) 2203 */ 2204 public CapabilityStatementImplementationComponent setCustodian(Reference value) { 2205 this.custodian = value; 2206 return this; 2207 } 2208 2209 /** 2210 * @return {@link #custodian} The actual object that is the target of the 2211 * reference. The reference library doesn't populate this, but you can 2212 * use it to hold the resource if you resolve it. (The organization 2213 * responsible for the management of the instance and oversight of the 2214 * data on the server at the specified URL.) 2215 */ 2216 public Organization getCustodianTarget() { 2217 if (this.custodianTarget == null) 2218 if (Configuration.errorOnAutoCreate()) 2219 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.custodian"); 2220 else if (Configuration.doAutoCreate()) 2221 this.custodianTarget = new Organization(); // aa 2222 return this.custodianTarget; 2223 } 2224 2225 /** 2226 * @param value {@link #custodian} The actual object that is the target of the 2227 * reference. The reference library doesn't use these, but you can 2228 * use it to hold the resource if you resolve it. (The organization 2229 * responsible for the management of the instance and oversight of 2230 * the data on the server at the specified URL.) 2231 */ 2232 public CapabilityStatementImplementationComponent setCustodianTarget(Organization value) { 2233 this.custodianTarget = value; 2234 return this; 2235 } 2236 2237 protected void listChildren(List<Property> children) { 2238 super.listChildren(children); 2239 children.add(new Property("description", "string", 2240 "Information about the specific installation that this capability statement relates to.", 0, 1, description)); 2241 children.add(new Property("url", "url", 2242 "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 2243 0, 1, url)); 2244 children.add(new Property("custodian", "Reference(Organization)", 2245 "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.", 2246 0, 1, custodian)); 2247 } 2248 2249 @Override 2250 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2251 switch (_hash) { 2252 case -1724546052: 2253 /* description */ return new Property("description", "string", 2254 "Information about the specific installation that this capability statement relates to.", 0, 1, 2255 description); 2256 case 116079: 2257 /* url */ return new Property("url", "url", 2258 "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 2259 0, 1, url); 2260 case 1611297262: 2261 /* custodian */ return new Property("custodian", "Reference(Organization)", 2262 "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.", 2263 0, 1, custodian); 2264 default: 2265 return super.getNamedProperty(_hash, _name, _checkValid); 2266 } 2267 2268 } 2269 2270 @Override 2271 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2272 switch (hash) { 2273 case -1724546052: 2274 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2275 case 116079: 2276 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UrlType 2277 case 1611297262: 2278 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 2279 default: 2280 return super.getProperty(hash, name, checkValid); 2281 } 2282 2283 } 2284 2285 @Override 2286 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2287 switch (hash) { 2288 case -1724546052: // description 2289 this.description = castToString(value); // StringType 2290 return value; 2291 case 116079: // url 2292 this.url = castToUrl(value); // UrlType 2293 return value; 2294 case 1611297262: // custodian 2295 this.custodian = castToReference(value); // Reference 2296 return value; 2297 default: 2298 return super.setProperty(hash, name, value); 2299 } 2300 2301 } 2302 2303 @Override 2304 public Base setProperty(String name, Base value) throws FHIRException { 2305 if (name.equals("description")) { 2306 this.description = castToString(value); // StringType 2307 } else if (name.equals("url")) { 2308 this.url = castToUrl(value); // UrlType 2309 } else if (name.equals("custodian")) { 2310 this.custodian = castToReference(value); // Reference 2311 } else 2312 return super.setProperty(name, value); 2313 return value; 2314 } 2315 2316 @Override 2317 public void removeChild(String name, Base value) throws FHIRException { 2318 if (name.equals("description")) { 2319 this.description = null; 2320 } else if (name.equals("url")) { 2321 this.url = null; 2322 } else if (name.equals("custodian")) { 2323 this.custodian = null; 2324 } else 2325 super.removeChild(name, value); 2326 2327 } 2328 2329 @Override 2330 public Base makeProperty(int hash, String name) throws FHIRException { 2331 switch (hash) { 2332 case -1724546052: 2333 return getDescriptionElement(); 2334 case 116079: 2335 return getUrlElement(); 2336 case 1611297262: 2337 return getCustodian(); 2338 default: 2339 return super.makeProperty(hash, name); 2340 } 2341 2342 } 2343 2344 @Override 2345 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2346 switch (hash) { 2347 case -1724546052: 2348 /* description */ return new String[] { "string" }; 2349 case 116079: 2350 /* url */ return new String[] { "url" }; 2351 case 1611297262: 2352 /* custodian */ return new String[] { "Reference" }; 2353 default: 2354 return super.getTypesForProperty(hash, name); 2355 } 2356 2357 } 2358 2359 @Override 2360 public Base addChild(String name) throws FHIRException { 2361 if (name.equals("description")) { 2362 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 2363 } else if (name.equals("url")) { 2364 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 2365 } else if (name.equals("custodian")) { 2366 this.custodian = new Reference(); 2367 return this.custodian; 2368 } else 2369 return super.addChild(name); 2370 } 2371 2372 public CapabilityStatementImplementationComponent copy() { 2373 CapabilityStatementImplementationComponent dst = new CapabilityStatementImplementationComponent(); 2374 copyValues(dst); 2375 return dst; 2376 } 2377 2378 public void copyValues(CapabilityStatementImplementationComponent dst) { 2379 super.copyValues(dst); 2380 dst.description = description == null ? null : description.copy(); 2381 dst.url = url == null ? null : url.copy(); 2382 dst.custodian = custodian == null ? null : custodian.copy(); 2383 } 2384 2385 @Override 2386 public boolean equalsDeep(Base other_) { 2387 if (!super.equalsDeep(other_)) 2388 return false; 2389 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 2390 return false; 2391 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 2392 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true) 2393 && compareDeep(custodian, o.custodian, true); 2394 } 2395 2396 @Override 2397 public boolean equalsShallow(Base other_) { 2398 if (!super.equalsShallow(other_)) 2399 return false; 2400 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 2401 return false; 2402 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 2403 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 2404 } 2405 2406 public boolean isEmpty() { 2407 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, url, custodian); 2408 } 2409 2410 public String fhirType() { 2411 return "CapabilityStatement.implementation"; 2412 2413 } 2414 2415 } 2416 2417 @Block() 2418 public static class CapabilityStatementRestComponent extends BackboneElement implements IBaseBackboneElement { 2419 /** 2420 * Identifies whether this portion of the statement is describing the ability to 2421 * initiate or receive restful operations. 2422 */ 2423 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2424 @Description(shortDefinition = "client | server", formalDefinition = "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.") 2425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/restful-capability-mode") 2426 protected Enumeration<RestfulCapabilityMode> mode; 2427 2428 /** 2429 * Information about the system's restful capabilities that apply across all 2430 * applications, such as security. 2431 */ 2432 @Child(name = "documentation", type = { 2433 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2434 @Description(shortDefinition = "General description of implementation", formalDefinition = "Information about the system's restful capabilities that apply across all applications, such as security.") 2435 protected MarkdownType documentation; 2436 2437 /** 2438 * Information about security implementation from an interface perspective - 2439 * what a client needs to know. 2440 */ 2441 @Child(name = "security", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = true) 2442 @Description(shortDefinition = "Information about security of implementation", formalDefinition = "Information about security implementation from an interface perspective - what a client needs to know.") 2443 protected CapabilityStatementRestSecurityComponent security; 2444 2445 /** 2446 * A specification of the restful capabilities of the solution for a specific 2447 * resource type. 2448 */ 2449 @Child(name = "resource", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2450 @Description(shortDefinition = "Resource served on the REST interface", formalDefinition = "A specification of the restful capabilities of the solution for a specific resource type.") 2451 protected List<CapabilityStatementRestResourceComponent> resource; 2452 2453 /** 2454 * A specification of restful operations supported by the system. 2455 */ 2456 @Child(name = "interaction", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2457 @Description(shortDefinition = "What operations are supported?", formalDefinition = "A specification of restful operations supported by the system.") 2458 protected List<SystemInteractionComponent> interaction; 2459 2460 /** 2461 * Search parameters that are supported for searching all resources for 2462 * implementations to support and/or make use of - either references to ones 2463 * defined in the specification, or additional ones defined for/by the 2464 * implementation. 2465 */ 2466 @Child(name = "searchParam", type = { 2467 CapabilityStatementRestResourceSearchParamComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2468 @Description(shortDefinition = "Search parameters for searching all resources", formalDefinition = "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 2469 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 2470 2471 /** 2472 * Definition of an operation or a named query together with its parameters and 2473 * their meaning and type. 2474 */ 2475 @Child(name = "operation", type = { 2476 CapabilityStatementRestResourceOperationComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2477 @Description(shortDefinition = "Definition of a system level operation", formalDefinition = "Definition of an operation or a named query together with its parameters and their meaning and type.") 2478 protected List<CapabilityStatementRestResourceOperationComponent> operation; 2479 2480 /** 2481 * An absolute URI which is a reference to the definition of a compartment that 2482 * the system supports. The reference is to a CompartmentDefinition resource by 2483 * its canonical URL . 2484 */ 2485 @Child(name = "compartment", type = { 2486 CanonicalType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2487 @Description(shortDefinition = "Compartments served/used by system", formalDefinition = "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .") 2488 protected List<CanonicalType> compartment; 2489 2490 private static final long serialVersionUID = -1442029817L; 2491 2492 /** 2493 * Constructor 2494 */ 2495 public CapabilityStatementRestComponent() { 2496 super(); 2497 } 2498 2499 /** 2500 * Constructor 2501 */ 2502 public CapabilityStatementRestComponent(Enumeration<RestfulCapabilityMode> mode) { 2503 super(); 2504 this.mode = mode; 2505 } 2506 2507 /** 2508 * @return {@link #mode} (Identifies whether this portion of the statement is 2509 * describing the ability to initiate or receive restful operations.). 2510 * This is the underlying object with id, value and extensions. The 2511 * accessor "getMode" gives direct access to the value 2512 */ 2513 public Enumeration<RestfulCapabilityMode> getModeElement() { 2514 if (this.mode == null) 2515 if (Configuration.errorOnAutoCreate()) 2516 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.mode"); 2517 else if (Configuration.doAutoCreate()) 2518 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); // bb 2519 return this.mode; 2520 } 2521 2522 public boolean hasModeElement() { 2523 return this.mode != null && !this.mode.isEmpty(); 2524 } 2525 2526 public boolean hasMode() { 2527 return this.mode != null && !this.mode.isEmpty(); 2528 } 2529 2530 /** 2531 * @param value {@link #mode} (Identifies whether this portion of the statement 2532 * is describing the ability to initiate or receive restful 2533 * operations.). This is the underlying object with id, value and 2534 * extensions. The accessor "getMode" gives direct access to the 2535 * value 2536 */ 2537 public CapabilityStatementRestComponent setModeElement(Enumeration<RestfulCapabilityMode> value) { 2538 this.mode = value; 2539 return this; 2540 } 2541 2542 /** 2543 * @return Identifies whether this portion of the statement is describing the 2544 * ability to initiate or receive restful operations. 2545 */ 2546 public RestfulCapabilityMode getMode() { 2547 return this.mode == null ? null : this.mode.getValue(); 2548 } 2549 2550 /** 2551 * @param value Identifies whether this portion of the statement is describing 2552 * the ability to initiate or receive restful operations. 2553 */ 2554 public CapabilityStatementRestComponent setMode(RestfulCapabilityMode value) { 2555 if (this.mode == null) 2556 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); 2557 this.mode.setValue(value); 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #documentation} (Information about the system's restful 2563 * capabilities that apply across all applications, such as security.). 2564 * This is the underlying object with id, value and extensions. The 2565 * accessor "getDocumentation" gives direct access to the value 2566 */ 2567 public MarkdownType getDocumentationElement() { 2568 if (this.documentation == null) 2569 if (Configuration.errorOnAutoCreate()) 2570 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.documentation"); 2571 else if (Configuration.doAutoCreate()) 2572 this.documentation = new MarkdownType(); // bb 2573 return this.documentation; 2574 } 2575 2576 public boolean hasDocumentationElement() { 2577 return this.documentation != null && !this.documentation.isEmpty(); 2578 } 2579 2580 public boolean hasDocumentation() { 2581 return this.documentation != null && !this.documentation.isEmpty(); 2582 } 2583 2584 /** 2585 * @param value {@link #documentation} (Information about the system's restful 2586 * capabilities that apply across all applications, such as 2587 * security.). This is the underlying object with id, value and 2588 * extensions. The accessor "getDocumentation" gives direct access 2589 * to the value 2590 */ 2591 public CapabilityStatementRestComponent setDocumentationElement(MarkdownType value) { 2592 this.documentation = value; 2593 return this; 2594 } 2595 2596 /** 2597 * @return Information about the system's restful capabilities that apply across 2598 * all applications, such as security. 2599 */ 2600 public String getDocumentation() { 2601 return this.documentation == null ? null : this.documentation.getValue(); 2602 } 2603 2604 /** 2605 * @param value Information about the system's restful capabilities that apply 2606 * across all applications, such as security. 2607 */ 2608 public CapabilityStatementRestComponent setDocumentation(String value) { 2609 if (value == null) 2610 this.documentation = null; 2611 else { 2612 if (this.documentation == null) 2613 this.documentation = new MarkdownType(); 2614 this.documentation.setValue(value); 2615 } 2616 return this; 2617 } 2618 2619 /** 2620 * @return {@link #security} (Information about security implementation from an 2621 * interface perspective - what a client needs to know.) 2622 */ 2623 public CapabilityStatementRestSecurityComponent getSecurity() { 2624 if (this.security == null) 2625 if (Configuration.errorOnAutoCreate()) 2626 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.security"); 2627 else if (Configuration.doAutoCreate()) 2628 this.security = new CapabilityStatementRestSecurityComponent(); // cc 2629 return this.security; 2630 } 2631 2632 public boolean hasSecurity() { 2633 return this.security != null && !this.security.isEmpty(); 2634 } 2635 2636 /** 2637 * @param value {@link #security} (Information about security implementation 2638 * from an interface perspective - what a client needs to know.) 2639 */ 2640 public CapabilityStatementRestComponent setSecurity(CapabilityStatementRestSecurityComponent value) { 2641 this.security = value; 2642 return this; 2643 } 2644 2645 /** 2646 * @return {@link #resource} (A specification of the restful capabilities of the 2647 * solution for a specific resource type.) 2648 */ 2649 public List<CapabilityStatementRestResourceComponent> getResource() { 2650 if (this.resource == null) 2651 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2652 return this.resource; 2653 } 2654 2655 /** 2656 * @return Returns a reference to <code>this</code> for easy method chaining 2657 */ 2658 public CapabilityStatementRestComponent setResource(List<CapabilityStatementRestResourceComponent> theResource) { 2659 this.resource = theResource; 2660 return this; 2661 } 2662 2663 public boolean hasResource() { 2664 if (this.resource == null) 2665 return false; 2666 for (CapabilityStatementRestResourceComponent item : this.resource) 2667 if (!item.isEmpty()) 2668 return true; 2669 return false; 2670 } 2671 2672 public CapabilityStatementRestResourceComponent addResource() { // 3 2673 CapabilityStatementRestResourceComponent t = new CapabilityStatementRestResourceComponent(); 2674 if (this.resource == null) 2675 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2676 this.resource.add(t); 2677 return t; 2678 } 2679 2680 public CapabilityStatementRestComponent addResource(CapabilityStatementRestResourceComponent t) { // 3 2681 if (t == null) 2682 return this; 2683 if (this.resource == null) 2684 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2685 this.resource.add(t); 2686 return this; 2687 } 2688 2689 /** 2690 * @return The first repetition of repeating field {@link #resource}, creating 2691 * it if it does not already exist 2692 */ 2693 public CapabilityStatementRestResourceComponent getResourceFirstRep() { 2694 if (getResource().isEmpty()) { 2695 addResource(); 2696 } 2697 return getResource().get(0); 2698 } 2699 2700 /** 2701 * @return {@link #interaction} (A specification of restful operations supported 2702 * by the system.) 2703 */ 2704 public List<SystemInteractionComponent> getInteraction() { 2705 if (this.interaction == null) 2706 this.interaction = new ArrayList<SystemInteractionComponent>(); 2707 return this.interaction; 2708 } 2709 2710 /** 2711 * @return Returns a reference to <code>this</code> for easy method chaining 2712 */ 2713 public CapabilityStatementRestComponent setInteraction(List<SystemInteractionComponent> theInteraction) { 2714 this.interaction = theInteraction; 2715 return this; 2716 } 2717 2718 public boolean hasInteraction() { 2719 if (this.interaction == null) 2720 return false; 2721 for (SystemInteractionComponent item : this.interaction) 2722 if (!item.isEmpty()) 2723 return true; 2724 return false; 2725 } 2726 2727 public SystemInteractionComponent addInteraction() { // 3 2728 SystemInteractionComponent t = new SystemInteractionComponent(); 2729 if (this.interaction == null) 2730 this.interaction = new ArrayList<SystemInteractionComponent>(); 2731 this.interaction.add(t); 2732 return t; 2733 } 2734 2735 public CapabilityStatementRestComponent addInteraction(SystemInteractionComponent t) { // 3 2736 if (t == null) 2737 return this; 2738 if (this.interaction == null) 2739 this.interaction = new ArrayList<SystemInteractionComponent>(); 2740 this.interaction.add(t); 2741 return this; 2742 } 2743 2744 /** 2745 * @return The first repetition of repeating field {@link #interaction}, 2746 * creating it if it does not already exist 2747 */ 2748 public SystemInteractionComponent getInteractionFirstRep() { 2749 if (getInteraction().isEmpty()) { 2750 addInteraction(); 2751 } 2752 return getInteraction().get(0); 2753 } 2754 2755 /** 2756 * @return {@link #searchParam} (Search parameters that are supported for 2757 * searching all resources for implementations to support and/or make 2758 * use of - either references to ones defined in the specification, or 2759 * additional ones defined for/by the implementation.) 2760 */ 2761 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 2762 if (this.searchParam == null) 2763 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2764 return this.searchParam; 2765 } 2766 2767 /** 2768 * @return Returns a reference to <code>this</code> for easy method chaining 2769 */ 2770 public CapabilityStatementRestComponent setSearchParam( 2771 List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 2772 this.searchParam = theSearchParam; 2773 return this; 2774 } 2775 2776 public boolean hasSearchParam() { 2777 if (this.searchParam == null) 2778 return false; 2779 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 2780 if (!item.isEmpty()) 2781 return true; 2782 return false; 2783 } 2784 2785 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { // 3 2786 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 2787 if (this.searchParam == null) 2788 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2789 this.searchParam.add(t); 2790 return t; 2791 } 2792 2793 public CapabilityStatementRestComponent addSearchParam(CapabilityStatementRestResourceSearchParamComponent t) { // 3 2794 if (t == null) 2795 return this; 2796 if (this.searchParam == null) 2797 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2798 this.searchParam.add(t); 2799 return this; 2800 } 2801 2802 /** 2803 * @return The first repetition of repeating field {@link #searchParam}, 2804 * creating it if it does not already exist 2805 */ 2806 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 2807 if (getSearchParam().isEmpty()) { 2808 addSearchParam(); 2809 } 2810 return getSearchParam().get(0); 2811 } 2812 2813 /** 2814 * @return {@link #operation} (Definition of an operation or a named query 2815 * together with its parameters and their meaning and type.) 2816 */ 2817 public List<CapabilityStatementRestResourceOperationComponent> getOperation() { 2818 if (this.operation == null) 2819 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2820 return this.operation; 2821 } 2822 2823 /** 2824 * @return Returns a reference to <code>this</code> for easy method chaining 2825 */ 2826 public CapabilityStatementRestComponent setOperation( 2827 List<CapabilityStatementRestResourceOperationComponent> theOperation) { 2828 this.operation = theOperation; 2829 return this; 2830 } 2831 2832 public boolean hasOperation() { 2833 if (this.operation == null) 2834 return false; 2835 for (CapabilityStatementRestResourceOperationComponent item : this.operation) 2836 if (!item.isEmpty()) 2837 return true; 2838 return false; 2839 } 2840 2841 public CapabilityStatementRestResourceOperationComponent addOperation() { // 3 2842 CapabilityStatementRestResourceOperationComponent t = new CapabilityStatementRestResourceOperationComponent(); 2843 if (this.operation == null) 2844 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2845 this.operation.add(t); 2846 return t; 2847 } 2848 2849 public CapabilityStatementRestComponent addOperation(CapabilityStatementRestResourceOperationComponent t) { // 3 2850 if (t == null) 2851 return this; 2852 if (this.operation == null) 2853 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2854 this.operation.add(t); 2855 return this; 2856 } 2857 2858 /** 2859 * @return The first repetition of repeating field {@link #operation}, creating 2860 * it if it does not already exist 2861 */ 2862 public CapabilityStatementRestResourceOperationComponent getOperationFirstRep() { 2863 if (getOperation().isEmpty()) { 2864 addOperation(); 2865 } 2866 return getOperation().get(0); 2867 } 2868 2869 /** 2870 * @return {@link #compartment} (An absolute URI which is a reference to the 2871 * definition of a compartment that the system supports. The reference 2872 * is to a CompartmentDefinition resource by its canonical URL .) 2873 */ 2874 public List<CanonicalType> getCompartment() { 2875 if (this.compartment == null) 2876 this.compartment = new ArrayList<CanonicalType>(); 2877 return this.compartment; 2878 } 2879 2880 /** 2881 * @return Returns a reference to <code>this</code> for easy method chaining 2882 */ 2883 public CapabilityStatementRestComponent setCompartment(List<CanonicalType> theCompartment) { 2884 this.compartment = theCompartment; 2885 return this; 2886 } 2887 2888 public boolean hasCompartment() { 2889 if (this.compartment == null) 2890 return false; 2891 for (CanonicalType item : this.compartment) 2892 if (!item.isEmpty()) 2893 return true; 2894 return false; 2895 } 2896 2897 /** 2898 * @return {@link #compartment} (An absolute URI which is a reference to the 2899 * definition of a compartment that the system supports. The reference 2900 * is to a CompartmentDefinition resource by its canonical URL .) 2901 */ 2902 public CanonicalType addCompartmentElement() {// 2 2903 CanonicalType t = new CanonicalType(); 2904 if (this.compartment == null) 2905 this.compartment = new ArrayList<CanonicalType>(); 2906 this.compartment.add(t); 2907 return t; 2908 } 2909 2910 /** 2911 * @param value {@link #compartment} (An absolute URI which is a reference to 2912 * the definition of a compartment that the system supports. The 2913 * reference is to a CompartmentDefinition resource by its 2914 * canonical URL .) 2915 */ 2916 public CapabilityStatementRestComponent addCompartment(String value) { // 1 2917 CanonicalType t = new CanonicalType(); 2918 t.setValue(value); 2919 if (this.compartment == null) 2920 this.compartment = new ArrayList<CanonicalType>(); 2921 this.compartment.add(t); 2922 return this; 2923 } 2924 2925 /** 2926 * @param value {@link #compartment} (An absolute URI which is a reference to 2927 * the definition of a compartment that the system supports. The 2928 * reference is to a CompartmentDefinition resource by its 2929 * canonical URL .) 2930 */ 2931 public boolean hasCompartment(String value) { 2932 if (this.compartment == null) 2933 return false; 2934 for (CanonicalType v : this.compartment) 2935 if (v.getValue().equals(value)) // canonical(CompartmentDefinition) 2936 return true; 2937 return false; 2938 } 2939 2940 protected void listChildren(List<Property> children) { 2941 super.listChildren(children); 2942 children.add(new Property("mode", "code", 2943 "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 2944 0, 1, mode)); 2945 children.add(new Property("documentation", "markdown", 2946 "Information about the system's restful capabilities that apply across all applications, such as security.", 2947 0, 1, documentation)); 2948 children.add(new Property("security", "", 2949 "Information about security implementation from an interface perspective - what a client needs to know.", 0, 2950 1, security)); 2951 children.add(new Property("resource", "", 2952 "A specification of the restful capabilities of the solution for a specific resource type.", 0, 2953 java.lang.Integer.MAX_VALUE, resource)); 2954 children.add(new Property("interaction", "", "A specification of restful operations supported by the system.", 0, 2955 java.lang.Integer.MAX_VALUE, interaction)); 2956 children.add(new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", 2957 "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 2958 0, java.lang.Integer.MAX_VALUE, searchParam)); 2959 children.add(new Property("operation", "@CapabilityStatement.rest.resource.operation", 2960 "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, 2961 java.lang.Integer.MAX_VALUE, operation)); 2962 children.add(new Property("compartment", "canonical(CompartmentDefinition)", 2963 "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 2964 0, java.lang.Integer.MAX_VALUE, compartment)); 2965 } 2966 2967 @Override 2968 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2969 switch (_hash) { 2970 case 3357091: 2971 /* mode */ return new Property("mode", "code", 2972 "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 2973 0, 1, mode); 2974 case 1587405498: 2975 /* documentation */ return new Property("documentation", "markdown", 2976 "Information about the system's restful capabilities that apply across all applications, such as security.", 2977 0, 1, documentation); 2978 case 949122880: 2979 /* security */ return new Property("security", "", 2980 "Information about security implementation from an interface perspective - what a client needs to know.", 0, 2981 1, security); 2982 case -341064690: 2983 /* resource */ return new Property("resource", "", 2984 "A specification of the restful capabilities of the solution for a specific resource type.", 0, 2985 java.lang.Integer.MAX_VALUE, resource); 2986 case 1844104722: 2987 /* interaction */ return new Property("interaction", "", 2988 "A specification of restful operations supported by the system.", 0, java.lang.Integer.MAX_VALUE, 2989 interaction); 2990 case -553645115: 2991 /* searchParam */ return new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", 2992 "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 2993 0, java.lang.Integer.MAX_VALUE, searchParam); 2994 case 1662702951: 2995 /* operation */ return new Property("operation", "@CapabilityStatement.rest.resource.operation", 2996 "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, 2997 java.lang.Integer.MAX_VALUE, operation); 2998 case -397756334: 2999 /* compartment */ return new Property("compartment", "canonical(CompartmentDefinition)", 3000 "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 3001 0, java.lang.Integer.MAX_VALUE, compartment); 3002 default: 3003 return super.getNamedProperty(_hash, _name, _checkValid); 3004 } 3005 3006 } 3007 3008 @Override 3009 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3010 switch (hash) { 3011 case 3357091: 3012 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<RestfulCapabilityMode> 3013 case 1587405498: 3014 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 3015 case 949122880: 3016 /* security */ return this.security == null ? new Base[0] : new Base[] { this.security }; // CapabilityStatementRestSecurityComponent 3017 case -341064690: 3018 /* resource */ return this.resource == null ? new Base[0] 3019 : this.resource.toArray(new Base[this.resource.size()]); // CapabilityStatementRestResourceComponent 3020 case 1844104722: 3021 /* interaction */ return this.interaction == null ? new Base[0] 3022 : this.interaction.toArray(new Base[this.interaction.size()]); // SystemInteractionComponent 3023 case -553645115: 3024 /* searchParam */ return this.searchParam == null ? new Base[0] 3025 : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 3026 case 1662702951: 3027 /* operation */ return this.operation == null ? new Base[0] 3028 : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestResourceOperationComponent 3029 case -397756334: 3030 /* compartment */ return this.compartment == null ? new Base[0] 3031 : this.compartment.toArray(new Base[this.compartment.size()]); // CanonicalType 3032 default: 3033 return super.getProperty(hash, name, checkValid); 3034 } 3035 3036 } 3037 3038 @Override 3039 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3040 switch (hash) { 3041 case 3357091: // mode 3042 value = new RestfulCapabilityModeEnumFactory().fromType(castToCode(value)); 3043 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 3044 return value; 3045 case 1587405498: // documentation 3046 this.documentation = castToMarkdown(value); // MarkdownType 3047 return value; 3048 case 949122880: // security 3049 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 3050 return value; 3051 case -341064690: // resource 3052 this.getResource().add((CapabilityStatementRestResourceComponent) value); // CapabilityStatementRestResourceComponent 3053 return value; 3054 case 1844104722: // interaction 3055 this.getInteraction().add((SystemInteractionComponent) value); // SystemInteractionComponent 3056 return value; 3057 case -553645115: // searchParam 3058 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 3059 return value; 3060 case 1662702951: // operation 3061 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); // CapabilityStatementRestResourceOperationComponent 3062 return value; 3063 case -397756334: // compartment 3064 this.getCompartment().add(castToCanonical(value)); // CanonicalType 3065 return value; 3066 default: 3067 return super.setProperty(hash, name, value); 3068 } 3069 3070 } 3071 3072 @Override 3073 public Base setProperty(String name, Base value) throws FHIRException { 3074 if (name.equals("mode")) { 3075 value = new RestfulCapabilityModeEnumFactory().fromType(castToCode(value)); 3076 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 3077 } else if (name.equals("documentation")) { 3078 this.documentation = castToMarkdown(value); // MarkdownType 3079 } else if (name.equals("security")) { 3080 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 3081 } else if (name.equals("resource")) { 3082 this.getResource().add((CapabilityStatementRestResourceComponent) value); 3083 } else if (name.equals("interaction")) { 3084 this.getInteraction().add((SystemInteractionComponent) value); 3085 } else if (name.equals("searchParam")) { 3086 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 3087 } else if (name.equals("operation")) { 3088 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); 3089 } else if (name.equals("compartment")) { 3090 this.getCompartment().add(castToCanonical(value)); 3091 } else 3092 return super.setProperty(name, value); 3093 return value; 3094 } 3095 3096 @Override 3097 public void removeChild(String name, Base value) throws FHIRException { 3098 if (name.equals("mode")) { 3099 this.mode = null; 3100 } else if (name.equals("documentation")) { 3101 this.documentation = null; 3102 } else if (name.equals("security")) { 3103 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 3104 } else if (name.equals("resource")) { 3105 this.getResource().remove((CapabilityStatementRestResourceComponent) value); 3106 } else if (name.equals("interaction")) { 3107 this.getInteraction().remove((SystemInteractionComponent) value); 3108 } else if (name.equals("searchParam")) { 3109 this.getSearchParam().remove((CapabilityStatementRestResourceSearchParamComponent) value); 3110 } else if (name.equals("operation")) { 3111 this.getOperation().remove((CapabilityStatementRestResourceOperationComponent) value); 3112 } else if (name.equals("compartment")) { 3113 this.getCompartment().remove(castToCanonical(value)); 3114 } else 3115 super.removeChild(name, value); 3116 3117 } 3118 3119 @Override 3120 public Base makeProperty(int hash, String name) throws FHIRException { 3121 switch (hash) { 3122 case 3357091: 3123 return getModeElement(); 3124 case 1587405498: 3125 return getDocumentationElement(); 3126 case 949122880: 3127 return getSecurity(); 3128 case -341064690: 3129 return addResource(); 3130 case 1844104722: 3131 return addInteraction(); 3132 case -553645115: 3133 return addSearchParam(); 3134 case 1662702951: 3135 return addOperation(); 3136 case -397756334: 3137 return addCompartmentElement(); 3138 default: 3139 return super.makeProperty(hash, name); 3140 } 3141 3142 } 3143 3144 @Override 3145 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3146 switch (hash) { 3147 case 3357091: 3148 /* mode */ return new String[] { "code" }; 3149 case 1587405498: 3150 /* documentation */ return new String[] { "markdown" }; 3151 case 949122880: 3152 /* security */ return new String[] {}; 3153 case -341064690: 3154 /* resource */ return new String[] {}; 3155 case 1844104722: 3156 /* interaction */ return new String[] {}; 3157 case -553645115: 3158 /* searchParam */ return new String[] { "@CapabilityStatement.rest.resource.searchParam" }; 3159 case 1662702951: 3160 /* operation */ return new String[] { "@CapabilityStatement.rest.resource.operation" }; 3161 case -397756334: 3162 /* compartment */ return new String[] { "canonical" }; 3163 default: 3164 return super.getTypesForProperty(hash, name); 3165 } 3166 3167 } 3168 3169 @Override 3170 public Base addChild(String name) throws FHIRException { 3171 if (name.equals("mode")) { 3172 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 3173 } else if (name.equals("documentation")) { 3174 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 3175 } else if (name.equals("security")) { 3176 this.security = new CapabilityStatementRestSecurityComponent(); 3177 return this.security; 3178 } else if (name.equals("resource")) { 3179 return addResource(); 3180 } else if (name.equals("interaction")) { 3181 return addInteraction(); 3182 } else if (name.equals("searchParam")) { 3183 return addSearchParam(); 3184 } else if (name.equals("operation")) { 3185 return addOperation(); 3186 } else if (name.equals("compartment")) { 3187 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.compartment"); 3188 } else 3189 return super.addChild(name); 3190 } 3191 3192 public CapabilityStatementRestComponent copy() { 3193 CapabilityStatementRestComponent dst = new CapabilityStatementRestComponent(); 3194 copyValues(dst); 3195 return dst; 3196 } 3197 3198 public void copyValues(CapabilityStatementRestComponent dst) { 3199 super.copyValues(dst); 3200 dst.mode = mode == null ? null : mode.copy(); 3201 dst.documentation = documentation == null ? null : documentation.copy(); 3202 dst.security = security == null ? null : security.copy(); 3203 if (resource != null) { 3204 dst.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 3205 for (CapabilityStatementRestResourceComponent i : resource) 3206 dst.resource.add(i.copy()); 3207 } 3208 ; 3209 if (interaction != null) { 3210 dst.interaction = new ArrayList<SystemInteractionComponent>(); 3211 for (SystemInteractionComponent i : interaction) 3212 dst.interaction.add(i.copy()); 3213 } 3214 ; 3215 if (searchParam != null) { 3216 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 3217 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 3218 dst.searchParam.add(i.copy()); 3219 } 3220 ; 3221 if (operation != null) { 3222 dst.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 3223 for (CapabilityStatementRestResourceOperationComponent i : operation) 3224 dst.operation.add(i.copy()); 3225 } 3226 ; 3227 if (compartment != null) { 3228 dst.compartment = new ArrayList<CanonicalType>(); 3229 for (CanonicalType i : compartment) 3230 dst.compartment.add(i.copy()); 3231 } 3232 ; 3233 } 3234 3235 @Override 3236 public boolean equalsDeep(Base other_) { 3237 if (!super.equalsDeep(other_)) 3238 return false; 3239 if (!(other_ instanceof CapabilityStatementRestComponent)) 3240 return false; 3241 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 3242 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 3243 && compareDeep(security, o.security, true) && compareDeep(resource, o.resource, true) 3244 && compareDeep(interaction, o.interaction, true) && compareDeep(searchParam, o.searchParam, true) 3245 && compareDeep(operation, o.operation, true) && compareDeep(compartment, o.compartment, true); 3246 } 3247 3248 @Override 3249 public boolean equalsShallow(Base other_) { 3250 if (!super.equalsShallow(other_)) 3251 return false; 3252 if (!(other_ instanceof CapabilityStatementRestComponent)) 3253 return false; 3254 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 3255 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 3256 } 3257 3258 public boolean isEmpty() { 3259 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, security, resource, 3260 interaction, searchParam, operation, compartment); 3261 } 3262 3263 public String fhirType() { 3264 return "CapabilityStatement.rest"; 3265 3266 } 3267 3268 } 3269 3270 @Block() 3271 public static class CapabilityStatementRestSecurityComponent extends BackboneElement implements IBaseBackboneElement { 3272 /** 3273 * Server adds CORS headers when responding to requests - this enables 3274 * Javascript applications to use the server. 3275 */ 3276 @Child(name = "cors", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3277 @Description(shortDefinition = "Adds CORS Headers (http://enable-cors.org/)", formalDefinition = "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.") 3278 protected BooleanType cors; 3279 3280 /** 3281 * Types of security services that are supported/required by the system. 3282 */ 3283 @Child(name = "service", type = { 3284 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3285 @Description(shortDefinition = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", formalDefinition = "Types of security services that are supported/required by the system.") 3286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/restful-security-service") 3287 protected List<CodeableConcept> service; 3288 3289 /** 3290 * General description of how security works. 3291 */ 3292 @Child(name = "description", type = { 3293 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3294 @Description(shortDefinition = "General description of how security works", formalDefinition = "General description of how security works.") 3295 protected MarkdownType description; 3296 3297 private static final long serialVersionUID = -1348900500L; 3298 3299 /** 3300 * Constructor 3301 */ 3302 public CapabilityStatementRestSecurityComponent() { 3303 super(); 3304 } 3305 3306 /** 3307 * @return {@link #cors} (Server adds CORS headers when responding to requests - 3308 * this enables Javascript applications to use the server.). This is the 3309 * underlying object with id, value and extensions. The accessor 3310 * "getCors" gives direct access to the value 3311 */ 3312 public BooleanType getCorsElement() { 3313 if (this.cors == null) 3314 if (Configuration.errorOnAutoCreate()) 3315 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.cors"); 3316 else if (Configuration.doAutoCreate()) 3317 this.cors = new BooleanType(); // bb 3318 return this.cors; 3319 } 3320 3321 public boolean hasCorsElement() { 3322 return this.cors != null && !this.cors.isEmpty(); 3323 } 3324 3325 public boolean hasCors() { 3326 return this.cors != null && !this.cors.isEmpty(); 3327 } 3328 3329 /** 3330 * @param value {@link #cors} (Server adds CORS headers when responding to 3331 * requests - this enables Javascript applications to use the 3332 * server.). This is the underlying object with id, value and 3333 * extensions. The accessor "getCors" gives direct access to the 3334 * value 3335 */ 3336 public CapabilityStatementRestSecurityComponent setCorsElement(BooleanType value) { 3337 this.cors = value; 3338 return this; 3339 } 3340 3341 /** 3342 * @return Server adds CORS headers when responding to requests - this enables 3343 * Javascript applications to use the server. 3344 */ 3345 public boolean getCors() { 3346 return this.cors == null || this.cors.isEmpty() ? false : this.cors.getValue(); 3347 } 3348 3349 /** 3350 * @param value Server adds CORS headers when responding to requests - this 3351 * enables Javascript applications to use the server. 3352 */ 3353 public CapabilityStatementRestSecurityComponent setCors(boolean value) { 3354 if (this.cors == null) 3355 this.cors = new BooleanType(); 3356 this.cors.setValue(value); 3357 return this; 3358 } 3359 3360 /** 3361 * @return {@link #service} (Types of security services that are 3362 * supported/required by the system.) 3363 */ 3364 public List<CodeableConcept> getService() { 3365 if (this.service == null) 3366 this.service = new ArrayList<CodeableConcept>(); 3367 return this.service; 3368 } 3369 3370 /** 3371 * @return Returns a reference to <code>this</code> for easy method chaining 3372 */ 3373 public CapabilityStatementRestSecurityComponent setService(List<CodeableConcept> theService) { 3374 this.service = theService; 3375 return this; 3376 } 3377 3378 public boolean hasService() { 3379 if (this.service == null) 3380 return false; 3381 for (CodeableConcept item : this.service) 3382 if (!item.isEmpty()) 3383 return true; 3384 return false; 3385 } 3386 3387 public CodeableConcept addService() { // 3 3388 CodeableConcept t = new CodeableConcept(); 3389 if (this.service == null) 3390 this.service = new ArrayList<CodeableConcept>(); 3391 this.service.add(t); 3392 return t; 3393 } 3394 3395 public CapabilityStatementRestSecurityComponent addService(CodeableConcept t) { // 3 3396 if (t == null) 3397 return this; 3398 if (this.service == null) 3399 this.service = new ArrayList<CodeableConcept>(); 3400 this.service.add(t); 3401 return this; 3402 } 3403 3404 /** 3405 * @return The first repetition of repeating field {@link #service}, creating it 3406 * if it does not already exist 3407 */ 3408 public CodeableConcept getServiceFirstRep() { 3409 if (getService().isEmpty()) { 3410 addService(); 3411 } 3412 return getService().get(0); 3413 } 3414 3415 /** 3416 * @return {@link #description} (General description of how security works.). 3417 * This is the underlying object with id, value and extensions. The 3418 * accessor "getDescription" gives direct access to the value 3419 */ 3420 public MarkdownType getDescriptionElement() { 3421 if (this.description == null) 3422 if (Configuration.errorOnAutoCreate()) 3423 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.description"); 3424 else if (Configuration.doAutoCreate()) 3425 this.description = new MarkdownType(); // bb 3426 return this.description; 3427 } 3428 3429 public boolean hasDescriptionElement() { 3430 return this.description != null && !this.description.isEmpty(); 3431 } 3432 3433 public boolean hasDescription() { 3434 return this.description != null && !this.description.isEmpty(); 3435 } 3436 3437 /** 3438 * @param value {@link #description} (General description of how security 3439 * works.). This is the underlying object with id, value and 3440 * extensions. The accessor "getDescription" gives direct access to 3441 * the value 3442 */ 3443 public CapabilityStatementRestSecurityComponent setDescriptionElement(MarkdownType value) { 3444 this.description = value; 3445 return this; 3446 } 3447 3448 /** 3449 * @return General description of how security works. 3450 */ 3451 public String getDescription() { 3452 return this.description == null ? null : this.description.getValue(); 3453 } 3454 3455 /** 3456 * @param value General description of how security works. 3457 */ 3458 public CapabilityStatementRestSecurityComponent setDescription(String value) { 3459 if (value == null) 3460 this.description = null; 3461 else { 3462 if (this.description == null) 3463 this.description = new MarkdownType(); 3464 this.description.setValue(value); 3465 } 3466 return this; 3467 } 3468 3469 protected void listChildren(List<Property> children) { 3470 super.listChildren(children); 3471 children.add(new Property("cors", "boolean", 3472 "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.", 3473 0, 1, cors)); 3474 children.add(new Property("service", "CodeableConcept", 3475 "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, 3476 service)); 3477 children.add( 3478 new Property("description", "markdown", "General description of how security works.", 0, 1, description)); 3479 } 3480 3481 @Override 3482 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3483 switch (_hash) { 3484 case 3059629: 3485 /* cors */ return new Property("cors", "boolean", 3486 "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.", 3487 0, 1, cors); 3488 case 1984153269: 3489 /* service */ return new Property("service", "CodeableConcept", 3490 "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, 3491 service); 3492 case -1724546052: 3493 /* description */ return new Property("description", "markdown", "General description of how security works.", 3494 0, 1, description); 3495 default: 3496 return super.getNamedProperty(_hash, _name, _checkValid); 3497 } 3498 3499 } 3500 3501 @Override 3502 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3503 switch (hash) { 3504 case 3059629: 3505 /* cors */ return this.cors == null ? new Base[0] : new Base[] { this.cors }; // BooleanType 3506 case 1984153269: 3507 /* service */ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // CodeableConcept 3508 case -1724546052: 3509 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3510 default: 3511 return super.getProperty(hash, name, checkValid); 3512 } 3513 3514 } 3515 3516 @Override 3517 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3518 switch (hash) { 3519 case 3059629: // cors 3520 this.cors = castToBoolean(value); // BooleanType 3521 return value; 3522 case 1984153269: // service 3523 this.getService().add(castToCodeableConcept(value)); // CodeableConcept 3524 return value; 3525 case -1724546052: // description 3526 this.description = castToMarkdown(value); // MarkdownType 3527 return value; 3528 default: 3529 return super.setProperty(hash, name, value); 3530 } 3531 3532 } 3533 3534 @Override 3535 public Base setProperty(String name, Base value) throws FHIRException { 3536 if (name.equals("cors")) { 3537 this.cors = castToBoolean(value); // BooleanType 3538 } else if (name.equals("service")) { 3539 this.getService().add(castToCodeableConcept(value)); 3540 } else if (name.equals("description")) { 3541 this.description = castToMarkdown(value); // MarkdownType 3542 } else 3543 return super.setProperty(name, value); 3544 return value; 3545 } 3546 3547 @Override 3548 public void removeChild(String name, Base value) throws FHIRException { 3549 if (name.equals("cors")) { 3550 this.cors = null; 3551 } else if (name.equals("service")) { 3552 this.getService().remove(castToCodeableConcept(value)); 3553 } else if (name.equals("description")) { 3554 this.description = null; 3555 } else 3556 super.removeChild(name, value); 3557 3558 } 3559 3560 @Override 3561 public Base makeProperty(int hash, String name) throws FHIRException { 3562 switch (hash) { 3563 case 3059629: 3564 return getCorsElement(); 3565 case 1984153269: 3566 return addService(); 3567 case -1724546052: 3568 return getDescriptionElement(); 3569 default: 3570 return super.makeProperty(hash, name); 3571 } 3572 3573 } 3574 3575 @Override 3576 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3577 switch (hash) { 3578 case 3059629: 3579 /* cors */ return new String[] { "boolean" }; 3580 case 1984153269: 3581 /* service */ return new String[] { "CodeableConcept" }; 3582 case -1724546052: 3583 /* description */ return new String[] { "markdown" }; 3584 default: 3585 return super.getTypesForProperty(hash, name); 3586 } 3587 3588 } 3589 3590 @Override 3591 public Base addChild(String name) throws FHIRException { 3592 if (name.equals("cors")) { 3593 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.cors"); 3594 } else if (name.equals("service")) { 3595 return addService(); 3596 } else if (name.equals("description")) { 3597 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 3598 } else 3599 return super.addChild(name); 3600 } 3601 3602 public CapabilityStatementRestSecurityComponent copy() { 3603 CapabilityStatementRestSecurityComponent dst = new CapabilityStatementRestSecurityComponent(); 3604 copyValues(dst); 3605 return dst; 3606 } 3607 3608 public void copyValues(CapabilityStatementRestSecurityComponent dst) { 3609 super.copyValues(dst); 3610 dst.cors = cors == null ? null : cors.copy(); 3611 if (service != null) { 3612 dst.service = new ArrayList<CodeableConcept>(); 3613 for (CodeableConcept i : service) 3614 dst.service.add(i.copy()); 3615 } 3616 ; 3617 dst.description = description == null ? null : description.copy(); 3618 } 3619 3620 @Override 3621 public boolean equalsDeep(Base other_) { 3622 if (!super.equalsDeep(other_)) 3623 return false; 3624 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 3625 return false; 3626 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 3627 return compareDeep(cors, o.cors, true) && compareDeep(service, o.service, true) 3628 && compareDeep(description, o.description, true); 3629 } 3630 3631 @Override 3632 public boolean equalsShallow(Base other_) { 3633 if (!super.equalsShallow(other_)) 3634 return false; 3635 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 3636 return false; 3637 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 3638 return compareValues(cors, o.cors, true) && compareValues(description, o.description, true); 3639 } 3640 3641 public boolean isEmpty() { 3642 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cors, service, description); 3643 } 3644 3645 public String fhirType() { 3646 return "CapabilityStatement.rest.security"; 3647 3648 } 3649 3650 } 3651 3652 @Block() 3653 public static class CapabilityStatementRestResourceComponent extends BackboneElement implements IBaseBackboneElement { 3654 /** 3655 * A type of resource exposed via the restful interface. 3656 */ 3657 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3658 @Description(shortDefinition = "A resource type that is supported", formalDefinition = "A type of resource exposed via the restful interface.") 3659 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 3660 protected CodeType type; 3661 3662 /** 3663 * A specification of the profile that describes the solution's overall support 3664 * for the resource, including any constraints on cardinality, bindings, lengths 3665 * or other limitations. See further discussion in [Using 3666 * Profiles](profiling.html#profile-uses). 3667 */ 3668 @Child(name = "profile", type = { 3669 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3670 @Description(shortDefinition = "Base System profile for all uses of resource", formalDefinition = "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).") 3671 protected CanonicalType profile; 3672 3673 /** 3674 * A list of profiles that represent different use cases supported by the 3675 * system. For a server, "supported by the system" means the system 3676 * hosts/produces a set of resources that are conformant to a particular 3677 * profile, and allows clients that use its services to search using this 3678 * profile and to find appropriate data. For a client, it means the system will 3679 * search by this profile and process data according to the guidance implicit in 3680 * the profile. See further discussion in [Using 3681 * Profiles](profiling.html#profile-uses). 3682 */ 3683 @Child(name = "supportedProfile", type = { 3684 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3685 @Description(shortDefinition = "Profiles for use cases supported", formalDefinition = "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).") 3686 protected List<CanonicalType> supportedProfile; 3687 3688 /** 3689 * Additional information about the resource type used by the system. 3690 */ 3691 @Child(name = "documentation", type = { 3692 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3693 @Description(shortDefinition = "Additional information about the use of the resource type", formalDefinition = "Additional information about the resource type used by the system.") 3694 protected MarkdownType documentation; 3695 3696 /** 3697 * Identifies a restful operation supported by the solution. 3698 */ 3699 @Child(name = "interaction", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3700 @Description(shortDefinition = "What operations are supported?", formalDefinition = "Identifies a restful operation supported by the solution.") 3701 protected List<ResourceInteractionComponent> interaction; 3702 3703 /** 3704 * This field is set to no-version to specify that the system does not support 3705 * (server) or use (client) versioning for this resource type. If this has some 3706 * other value, the server must at least correctly track and populate the 3707 * versionId meta-property on resources. If the value is 'versioned-update', 3708 * then the server supports all the versioning features, including using e-tags 3709 * for version integrity in the API. 3710 */ 3711 @Child(name = "versioning", type = { 3712 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3713 @Description(shortDefinition = "no-version | versioned | versioned-update", formalDefinition = "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.") 3714 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/versioning-policy") 3715 protected Enumeration<ResourceVersionPolicy> versioning; 3716 3717 /** 3718 * A flag for whether the server is able to return past versions as part of the 3719 * vRead operation. 3720 */ 3721 @Child(name = "readHistory", type = { 3722 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3723 @Description(shortDefinition = "Whether vRead can return past versions", formalDefinition = "A flag for whether the server is able to return past versions as part of the vRead operation.") 3724 protected BooleanType readHistory; 3725 3726 /** 3727 * A flag to indicate that the server allows or needs to allow the client to 3728 * create new identities on the server (that is, the client PUTs to a location 3729 * where there is no existing resource). Allowing this operation means that the 3730 * server allows the client to create new identities on the server. 3731 */ 3732 @Child(name = "updateCreate", type = { 3733 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3734 @Description(shortDefinition = "If update can commit to a new identity", formalDefinition = "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.") 3735 protected BooleanType updateCreate; 3736 3737 /** 3738 * A flag that indicates that the server supports conditional create. 3739 */ 3740 @Child(name = "conditionalCreate", type = { 3741 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 3742 @Description(shortDefinition = "If allows/uses conditional create", formalDefinition = "A flag that indicates that the server supports conditional create.") 3743 protected BooleanType conditionalCreate; 3744 3745 /** 3746 * A code that indicates how the server supports conditional read. 3747 */ 3748 @Child(name = "conditionalRead", type = { 3749 CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 3750 @Description(shortDefinition = "not-supported | modified-since | not-match | full-support", formalDefinition = "A code that indicates how the server supports conditional read.") 3751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/conditional-read-status") 3752 protected Enumeration<ConditionalReadStatus> conditionalRead; 3753 3754 /** 3755 * A flag that indicates that the server supports conditional update. 3756 */ 3757 @Child(name = "conditionalUpdate", type = { 3758 BooleanType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 3759 @Description(shortDefinition = "If allows/uses conditional update", formalDefinition = "A flag that indicates that the server supports conditional update.") 3760 protected BooleanType conditionalUpdate; 3761 3762 /** 3763 * A code that indicates how the server supports conditional delete. 3764 */ 3765 @Child(name = "conditionalDelete", type = { 3766 CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 3767 @Description(shortDefinition = "not-supported | single | multiple - how conditional delete is supported", formalDefinition = "A code that indicates how the server supports conditional delete.") 3768 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/conditional-delete-status") 3769 protected Enumeration<ConditionalDeleteStatus> conditionalDelete; 3770 3771 /** 3772 * A set of flags that defines how references are supported. 3773 */ 3774 @Child(name = "referencePolicy", type = { 3775 CodeType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3776 @Description(shortDefinition = "literal | logical | resolves | enforced | local", formalDefinition = "A set of flags that defines how references are supported.") 3777 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reference-handling-policy") 3778 protected List<Enumeration<ReferenceHandlingPolicy>> referencePolicy; 3779 3780 /** 3781 * A list of _include values supported by the server. 3782 */ 3783 @Child(name = "searchInclude", type = { 3784 StringType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3785 @Description(shortDefinition = "_include values supported by the server", formalDefinition = "A list of _include values supported by the server.") 3786 protected List<StringType> searchInclude; 3787 3788 /** 3789 * A list of _revinclude (reverse include) values supported by the server. 3790 */ 3791 @Child(name = "searchRevInclude", type = { 3792 StringType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3793 @Description(shortDefinition = "_revinclude values supported by the server", formalDefinition = "A list of _revinclude (reverse include) values supported by the server.") 3794 protected List<StringType> searchRevInclude; 3795 3796 /** 3797 * Search parameters for implementations to support and/or make use of - either 3798 * references to ones defined in the specification, or additional ones defined 3799 * for/by the implementation. 3800 */ 3801 @Child(name = "searchParam", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3802 @Description(shortDefinition = "Search parameters supported by implementation", formalDefinition = "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 3803 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 3804 3805 /** 3806 * Definition of an operation or a named query together with its parameters and 3807 * their meaning and type. Consult the definition of the operation for details 3808 * about how to invoke the operation, and the parameters. 3809 */ 3810 @Child(name = "operation", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3811 @Description(shortDefinition = "Definition of a resource operation", formalDefinition = "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.") 3812 protected List<CapabilityStatementRestResourceOperationComponent> operation; 3813 3814 private static final long serialVersionUID = -1843372337L; 3815 3816 /** 3817 * Constructor 3818 */ 3819 public CapabilityStatementRestResourceComponent() { 3820 super(); 3821 } 3822 3823 /** 3824 * Constructor 3825 */ 3826 public CapabilityStatementRestResourceComponent(CodeType type) { 3827 super(); 3828 this.type = type; 3829 } 3830 3831 /** 3832 * @return {@link #type} (A type of resource exposed via the restful 3833 * interface.). This is the underlying object with id, value and 3834 * extensions. The accessor "getType" gives direct access to the value 3835 */ 3836 public CodeType getTypeElement() { 3837 if (this.type == null) 3838 if (Configuration.errorOnAutoCreate()) 3839 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.type"); 3840 else if (Configuration.doAutoCreate()) 3841 this.type = new CodeType(); // bb 3842 return this.type; 3843 } 3844 3845 public boolean hasTypeElement() { 3846 return this.type != null && !this.type.isEmpty(); 3847 } 3848 3849 public boolean hasType() { 3850 return this.type != null && !this.type.isEmpty(); 3851 } 3852 3853 /** 3854 * @param value {@link #type} (A type of resource exposed via the restful 3855 * interface.). This is the underlying object with id, value and 3856 * extensions. The accessor "getType" gives direct access to the 3857 * value 3858 */ 3859 public CapabilityStatementRestResourceComponent setTypeElement(CodeType value) { 3860 this.type = value; 3861 return this; 3862 } 3863 3864 /** 3865 * @return A type of resource exposed via the restful interface. 3866 */ 3867 public String getType() { 3868 return this.type == null ? null : this.type.getValue(); 3869 } 3870 3871 /** 3872 * @param value A type of resource exposed via the restful interface. 3873 */ 3874 public CapabilityStatementRestResourceComponent setType(String value) { 3875 if (this.type == null) 3876 this.type = new CodeType(); 3877 this.type.setValue(value); 3878 return this; 3879 } 3880 3881 /** 3882 * @return {@link #profile} (A specification of the profile that describes the 3883 * solution's overall support for the resource, including any 3884 * constraints on cardinality, bindings, lengths or other limitations. 3885 * See further discussion in [Using 3886 * Profiles](profiling.html#profile-uses).). This is the underlying 3887 * object with id, value and extensions. The accessor "getProfile" gives 3888 * direct access to the value 3889 */ 3890 public CanonicalType getProfileElement() { 3891 if (this.profile == null) 3892 if (Configuration.errorOnAutoCreate()) 3893 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.profile"); 3894 else if (Configuration.doAutoCreate()) 3895 this.profile = new CanonicalType(); // bb 3896 return this.profile; 3897 } 3898 3899 public boolean hasProfileElement() { 3900 return this.profile != null && !this.profile.isEmpty(); 3901 } 3902 3903 public boolean hasProfile() { 3904 return this.profile != null && !this.profile.isEmpty(); 3905 } 3906 3907 /** 3908 * @param value {@link #profile} (A specification of the profile that describes 3909 * the solution's overall support for the resource, including any 3910 * constraints on cardinality, bindings, lengths or other 3911 * limitations. See further discussion in [Using 3912 * Profiles](profiling.html#profile-uses).). This is the underlying 3913 * object with id, value and extensions. The accessor "getProfile" 3914 * gives direct access to the value 3915 */ 3916 public CapabilityStatementRestResourceComponent setProfileElement(CanonicalType value) { 3917 this.profile = value; 3918 return this; 3919 } 3920 3921 /** 3922 * @return A specification of the profile that describes the solution's overall 3923 * support for the resource, including any constraints on cardinality, 3924 * bindings, lengths or other limitations. See further discussion in 3925 * [Using Profiles](profiling.html#profile-uses). 3926 */ 3927 public String getProfile() { 3928 return this.profile == null ? null : this.profile.getValue(); 3929 } 3930 3931 /** 3932 * @param value A specification of the profile that describes the solution's 3933 * overall support for the resource, including any constraints on 3934 * cardinality, bindings, lengths or other limitations. See further 3935 * discussion in [Using Profiles](profiling.html#profile-uses). 3936 */ 3937 public CapabilityStatementRestResourceComponent setProfile(String value) { 3938 if (Utilities.noString(value)) 3939 this.profile = null; 3940 else { 3941 if (this.profile == null) 3942 this.profile = new CanonicalType(); 3943 this.profile.setValue(value); 3944 } 3945 return this; 3946 } 3947 3948 /** 3949 * @return {@link #supportedProfile} (A list of profiles that represent 3950 * different use cases supported by the system. For a server, "supported 3951 * by the system" means the system hosts/produces a set of resources 3952 * that are conformant to a particular profile, and allows clients that 3953 * use its services to search using this profile and to find appropriate 3954 * data. For a client, it means the system will search by this profile 3955 * and process data according to the guidance implicit in the profile. 3956 * See further discussion in [Using 3957 * Profiles](profiling.html#profile-uses).) 3958 */ 3959 public List<CanonicalType> getSupportedProfile() { 3960 if (this.supportedProfile == null) 3961 this.supportedProfile = new ArrayList<CanonicalType>(); 3962 return this.supportedProfile; 3963 } 3964 3965 /** 3966 * @return Returns a reference to <code>this</code> for easy method chaining 3967 */ 3968 public CapabilityStatementRestResourceComponent setSupportedProfile(List<CanonicalType> theSupportedProfile) { 3969 this.supportedProfile = theSupportedProfile; 3970 return this; 3971 } 3972 3973 public boolean hasSupportedProfile() { 3974 if (this.supportedProfile == null) 3975 return false; 3976 for (CanonicalType item : this.supportedProfile) 3977 if (!item.isEmpty()) 3978 return true; 3979 return false; 3980 } 3981 3982 /** 3983 * @return {@link #supportedProfile} (A list of profiles that represent 3984 * different use cases supported by the system. For a server, "supported 3985 * by the system" means the system hosts/produces a set of resources 3986 * that are conformant to a particular profile, and allows clients that 3987 * use its services to search using this profile and to find appropriate 3988 * data. For a client, it means the system will search by this profile 3989 * and process data according to the guidance implicit in the profile. 3990 * See further discussion in [Using 3991 * Profiles](profiling.html#profile-uses).) 3992 */ 3993 public CanonicalType addSupportedProfileElement() {// 2 3994 CanonicalType t = new CanonicalType(); 3995 if (this.supportedProfile == null) 3996 this.supportedProfile = new ArrayList<CanonicalType>(); 3997 this.supportedProfile.add(t); 3998 return t; 3999 } 4000 4001 /** 4002 * @param value {@link #supportedProfile} (A list of profiles that represent 4003 * different use cases supported by the system. For a server, 4004 * "supported by the system" means the system hosts/produces a set 4005 * of resources that are conformant to a particular profile, and 4006 * allows clients that use its services to search using this 4007 * profile and to find appropriate data. For a client, it means the 4008 * system will search by this profile and process data according to 4009 * the guidance implicit in the profile. See further discussion in 4010 * [Using Profiles](profiling.html#profile-uses).) 4011 */ 4012 public CapabilityStatementRestResourceComponent addSupportedProfile(String value) { // 1 4013 CanonicalType t = new CanonicalType(); 4014 t.setValue(value); 4015 if (this.supportedProfile == null) 4016 this.supportedProfile = new ArrayList<CanonicalType>(); 4017 this.supportedProfile.add(t); 4018 return this; 4019 } 4020 4021 /** 4022 * @param value {@link #supportedProfile} (A list of profiles that represent 4023 * different use cases supported by the system. For a server, 4024 * "supported by the system" means the system hosts/produces a set 4025 * of resources that are conformant to a particular profile, and 4026 * allows clients that use its services to search using this 4027 * profile and to find appropriate data. For a client, it means the 4028 * system will search by this profile and process data according to 4029 * the guidance implicit in the profile. See further discussion in 4030 * [Using Profiles](profiling.html#profile-uses).) 4031 */ 4032 public boolean hasSupportedProfile(String value) { 4033 if (this.supportedProfile == null) 4034 return false; 4035 for (CanonicalType v : this.supportedProfile) 4036 if (v.getValue().equals(value)) // canonical(StructureDefinition) 4037 return true; 4038 return false; 4039 } 4040 4041 /** 4042 * @return {@link #documentation} (Additional information about the resource 4043 * type used by the system.). This is the underlying object with id, 4044 * value and extensions. The accessor "getDocumentation" gives direct 4045 * access to the value 4046 */ 4047 public MarkdownType getDocumentationElement() { 4048 if (this.documentation == null) 4049 if (Configuration.errorOnAutoCreate()) 4050 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.documentation"); 4051 else if (Configuration.doAutoCreate()) 4052 this.documentation = new MarkdownType(); // bb 4053 return this.documentation; 4054 } 4055 4056 public boolean hasDocumentationElement() { 4057 return this.documentation != null && !this.documentation.isEmpty(); 4058 } 4059 4060 public boolean hasDocumentation() { 4061 return this.documentation != null && !this.documentation.isEmpty(); 4062 } 4063 4064 /** 4065 * @param value {@link #documentation} (Additional information about the 4066 * resource type used by the system.). This is the underlying 4067 * object with id, value and extensions. The accessor 4068 * "getDocumentation" gives direct access to the value 4069 */ 4070 public CapabilityStatementRestResourceComponent setDocumentationElement(MarkdownType value) { 4071 this.documentation = value; 4072 return this; 4073 } 4074 4075 /** 4076 * @return Additional information about the resource type used by the system. 4077 */ 4078 public String getDocumentation() { 4079 return this.documentation == null ? null : this.documentation.getValue(); 4080 } 4081 4082 /** 4083 * @param value Additional information about the resource type used by the 4084 * system. 4085 */ 4086 public CapabilityStatementRestResourceComponent setDocumentation(String value) { 4087 if (value == null) 4088 this.documentation = null; 4089 else { 4090 if (this.documentation == null) 4091 this.documentation = new MarkdownType(); 4092 this.documentation.setValue(value); 4093 } 4094 return this; 4095 } 4096 4097 /** 4098 * @return {@link #interaction} (Identifies a restful operation supported by the 4099 * solution.) 4100 */ 4101 public List<ResourceInteractionComponent> getInteraction() { 4102 if (this.interaction == null) 4103 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4104 return this.interaction; 4105 } 4106 4107 /** 4108 * @return Returns a reference to <code>this</code> for easy method chaining 4109 */ 4110 public CapabilityStatementRestResourceComponent setInteraction(List<ResourceInteractionComponent> theInteraction) { 4111 this.interaction = theInteraction; 4112 return this; 4113 } 4114 4115 public boolean hasInteraction() { 4116 if (this.interaction == null) 4117 return false; 4118 for (ResourceInteractionComponent item : this.interaction) 4119 if (!item.isEmpty()) 4120 return true; 4121 return false; 4122 } 4123 4124 public ResourceInteractionComponent addInteraction() { // 3 4125 ResourceInteractionComponent t = new ResourceInteractionComponent(); 4126 if (this.interaction == null) 4127 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4128 this.interaction.add(t); 4129 return t; 4130 } 4131 4132 public CapabilityStatementRestResourceComponent addInteraction(ResourceInteractionComponent t) { // 3 4133 if (t == null) 4134 return this; 4135 if (this.interaction == null) 4136 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4137 this.interaction.add(t); 4138 return this; 4139 } 4140 4141 /** 4142 * @return The first repetition of repeating field {@link #interaction}, 4143 * creating it if it does not already exist 4144 */ 4145 public ResourceInteractionComponent getInteractionFirstRep() { 4146 if (getInteraction().isEmpty()) { 4147 addInteraction(); 4148 } 4149 return getInteraction().get(0); 4150 } 4151 4152 /** 4153 * @return {@link #versioning} (This field is set to no-version to specify that 4154 * the system does not support (server) or use (client) versioning for 4155 * this resource type. If this has some other value, the server must at 4156 * least correctly track and populate the versionId meta-property on 4157 * resources. If the value is 'versioned-update', then the server 4158 * supports all the versioning features, including using e-tags for 4159 * version integrity in the API.). This is the underlying object with 4160 * id, value and extensions. The accessor "getVersioning" gives direct 4161 * access to the value 4162 */ 4163 public Enumeration<ResourceVersionPolicy> getVersioningElement() { 4164 if (this.versioning == null) 4165 if (Configuration.errorOnAutoCreate()) 4166 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.versioning"); 4167 else if (Configuration.doAutoCreate()) 4168 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); // bb 4169 return this.versioning; 4170 } 4171 4172 public boolean hasVersioningElement() { 4173 return this.versioning != null && !this.versioning.isEmpty(); 4174 } 4175 4176 public boolean hasVersioning() { 4177 return this.versioning != null && !this.versioning.isEmpty(); 4178 } 4179 4180 /** 4181 * @param value {@link #versioning} (This field is set to no-version to specify 4182 * that the system does not support (server) or use (client) 4183 * versioning for this resource type. If this has some other value, 4184 * the server must at least correctly track and populate the 4185 * versionId meta-property on resources. If the value is 4186 * 'versioned-update', then the server supports all the versioning 4187 * features, including using e-tags for version integrity in the 4188 * API.). This is the underlying object with id, value and 4189 * extensions. The accessor "getVersioning" gives direct access to 4190 * the value 4191 */ 4192 public CapabilityStatementRestResourceComponent setVersioningElement(Enumeration<ResourceVersionPolicy> value) { 4193 this.versioning = value; 4194 return this; 4195 } 4196 4197 /** 4198 * @return This field is set to no-version to specify that the system does not 4199 * support (server) or use (client) versioning for this resource type. 4200 * If this has some other value, the server must at least correctly 4201 * track and populate the versionId meta-property on resources. If the 4202 * value is 'versioned-update', then the server supports all the 4203 * versioning features, including using e-tags for version integrity in 4204 * the API. 4205 */ 4206 public ResourceVersionPolicy getVersioning() { 4207 return this.versioning == null ? null : this.versioning.getValue(); 4208 } 4209 4210 /** 4211 * @param value This field is set to no-version to specify that the system does 4212 * not support (server) or use (client) versioning for this 4213 * resource type. If this has some other value, the server must at 4214 * least correctly track and populate the versionId meta-property 4215 * on resources. If the value is 'versioned-update', then the 4216 * server supports all the versioning features, including using 4217 * e-tags for version integrity in the API. 4218 */ 4219 public CapabilityStatementRestResourceComponent setVersioning(ResourceVersionPolicy value) { 4220 if (value == null) 4221 this.versioning = null; 4222 else { 4223 if (this.versioning == null) 4224 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); 4225 this.versioning.setValue(value); 4226 } 4227 return this; 4228 } 4229 4230 /** 4231 * @return {@link #readHistory} (A flag for whether the server is able to return 4232 * past versions as part of the vRead operation.). This is the 4233 * underlying object with id, value and extensions. The accessor 4234 * "getReadHistory" gives direct access to the value 4235 */ 4236 public BooleanType getReadHistoryElement() { 4237 if (this.readHistory == null) 4238 if (Configuration.errorOnAutoCreate()) 4239 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.readHistory"); 4240 else if (Configuration.doAutoCreate()) 4241 this.readHistory = new BooleanType(); // bb 4242 return this.readHistory; 4243 } 4244 4245 public boolean hasReadHistoryElement() { 4246 return this.readHistory != null && !this.readHistory.isEmpty(); 4247 } 4248 4249 public boolean hasReadHistory() { 4250 return this.readHistory != null && !this.readHistory.isEmpty(); 4251 } 4252 4253 /** 4254 * @param value {@link #readHistory} (A flag for whether the server is able to 4255 * return past versions as part of the vRead operation.). This is 4256 * the underlying object with id, value and extensions. The 4257 * accessor "getReadHistory" gives direct access to the value 4258 */ 4259 public CapabilityStatementRestResourceComponent setReadHistoryElement(BooleanType value) { 4260 this.readHistory = value; 4261 return this; 4262 } 4263 4264 /** 4265 * @return A flag for whether the server is able to return past versions as part 4266 * of the vRead operation. 4267 */ 4268 public boolean getReadHistory() { 4269 return this.readHistory == null || this.readHistory.isEmpty() ? false : this.readHistory.getValue(); 4270 } 4271 4272 /** 4273 * @param value A flag for whether the server is able to return past versions as 4274 * part of the vRead operation. 4275 */ 4276 public CapabilityStatementRestResourceComponent setReadHistory(boolean value) { 4277 if (this.readHistory == null) 4278 this.readHistory = new BooleanType(); 4279 this.readHistory.setValue(value); 4280 return this; 4281 } 4282 4283 /** 4284 * @return {@link #updateCreate} (A flag to indicate that the server allows or 4285 * needs to allow the client to create new identities on the server 4286 * (that is, the client PUTs to a location where there is no existing 4287 * resource). Allowing this operation means that the server allows the 4288 * client to create new identities on the server.). This is the 4289 * underlying object with id, value and extensions. The accessor 4290 * "getUpdateCreate" gives direct access to the value 4291 */ 4292 public BooleanType getUpdateCreateElement() { 4293 if (this.updateCreate == null) 4294 if (Configuration.errorOnAutoCreate()) 4295 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.updateCreate"); 4296 else if (Configuration.doAutoCreate()) 4297 this.updateCreate = new BooleanType(); // bb 4298 return this.updateCreate; 4299 } 4300 4301 public boolean hasUpdateCreateElement() { 4302 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4303 } 4304 4305 public boolean hasUpdateCreate() { 4306 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4307 } 4308 4309 /** 4310 * @param value {@link #updateCreate} (A flag to indicate that the server allows 4311 * or needs to allow the client to create new identities on the 4312 * server (that is, the client PUTs to a location where there is no 4313 * existing resource). Allowing this operation means that the 4314 * server allows the client to create new identities on the 4315 * server.). This is the underlying object with id, value and 4316 * extensions. The accessor "getUpdateCreate" gives direct access 4317 * to the value 4318 */ 4319 public CapabilityStatementRestResourceComponent setUpdateCreateElement(BooleanType value) { 4320 this.updateCreate = value; 4321 return this; 4322 } 4323 4324 /** 4325 * @return A flag to indicate that the server allows or needs to allow the 4326 * client to create new identities on the server (that is, the client 4327 * PUTs to a location where there is no existing resource). Allowing 4328 * this operation means that the server allows the client to create new 4329 * identities on the server. 4330 */ 4331 public boolean getUpdateCreate() { 4332 return this.updateCreate == null || this.updateCreate.isEmpty() ? false : this.updateCreate.getValue(); 4333 } 4334 4335 /** 4336 * @param value A flag to indicate that the server allows or needs to allow the 4337 * client to create new identities on the server (that is, the 4338 * client PUTs to a location where there is no existing resource). 4339 * Allowing this operation means that the server allows the client 4340 * to create new identities on the server. 4341 */ 4342 public CapabilityStatementRestResourceComponent setUpdateCreate(boolean value) { 4343 if (this.updateCreate == null) 4344 this.updateCreate = new BooleanType(); 4345 this.updateCreate.setValue(value); 4346 return this; 4347 } 4348 4349 /** 4350 * @return {@link #conditionalCreate} (A flag that indicates that the server 4351 * supports conditional create.). This is the underlying object with id, 4352 * value and extensions. The accessor "getConditionalCreate" gives 4353 * direct access to the value 4354 */ 4355 public BooleanType getConditionalCreateElement() { 4356 if (this.conditionalCreate == null) 4357 if (Configuration.errorOnAutoCreate()) 4358 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalCreate"); 4359 else if (Configuration.doAutoCreate()) 4360 this.conditionalCreate = new BooleanType(); // bb 4361 return this.conditionalCreate; 4362 } 4363 4364 public boolean hasConditionalCreateElement() { 4365 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4366 } 4367 4368 public boolean hasConditionalCreate() { 4369 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4370 } 4371 4372 /** 4373 * @param value {@link #conditionalCreate} (A flag that indicates that the 4374 * server supports conditional create.). This is the underlying 4375 * object with id, value and extensions. The accessor 4376 * "getConditionalCreate" gives direct access to the value 4377 */ 4378 public CapabilityStatementRestResourceComponent setConditionalCreateElement(BooleanType value) { 4379 this.conditionalCreate = value; 4380 return this; 4381 } 4382 4383 /** 4384 * @return A flag that indicates that the server supports conditional create. 4385 */ 4386 public boolean getConditionalCreate() { 4387 return this.conditionalCreate == null || this.conditionalCreate.isEmpty() ? false 4388 : this.conditionalCreate.getValue(); 4389 } 4390 4391 /** 4392 * @param value A flag that indicates that the server supports conditional 4393 * create. 4394 */ 4395 public CapabilityStatementRestResourceComponent setConditionalCreate(boolean value) { 4396 if (this.conditionalCreate == null) 4397 this.conditionalCreate = new BooleanType(); 4398 this.conditionalCreate.setValue(value); 4399 return this; 4400 } 4401 4402 /** 4403 * @return {@link #conditionalRead} (A code that indicates how the server 4404 * supports conditional read.). This is the underlying object with id, 4405 * value and extensions. The accessor "getConditionalRead" gives direct 4406 * access to the value 4407 */ 4408 public Enumeration<ConditionalReadStatus> getConditionalReadElement() { 4409 if (this.conditionalRead == null) 4410 if (Configuration.errorOnAutoCreate()) 4411 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalRead"); 4412 else if (Configuration.doAutoCreate()) 4413 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); // bb 4414 return this.conditionalRead; 4415 } 4416 4417 public boolean hasConditionalReadElement() { 4418 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 4419 } 4420 4421 public boolean hasConditionalRead() { 4422 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 4423 } 4424 4425 /** 4426 * @param value {@link #conditionalRead} (A code that indicates how the server 4427 * supports conditional read.). This is the underlying object with 4428 * id, value and extensions. The accessor "getConditionalRead" 4429 * gives direct access to the value 4430 */ 4431 public CapabilityStatementRestResourceComponent setConditionalReadElement( 4432 Enumeration<ConditionalReadStatus> value) { 4433 this.conditionalRead = value; 4434 return this; 4435 } 4436 4437 /** 4438 * @return A code that indicates how the server supports conditional read. 4439 */ 4440 public ConditionalReadStatus getConditionalRead() { 4441 return this.conditionalRead == null ? null : this.conditionalRead.getValue(); 4442 } 4443 4444 /** 4445 * @param value A code that indicates how the server supports conditional read. 4446 */ 4447 public CapabilityStatementRestResourceComponent setConditionalRead(ConditionalReadStatus value) { 4448 if (value == null) 4449 this.conditionalRead = null; 4450 else { 4451 if (this.conditionalRead == null) 4452 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); 4453 this.conditionalRead.setValue(value); 4454 } 4455 return this; 4456 } 4457 4458 /** 4459 * @return {@link #conditionalUpdate} (A flag that indicates that the server 4460 * supports conditional update.). This is the underlying object with id, 4461 * value and extensions. The accessor "getConditionalUpdate" gives 4462 * direct access to the value 4463 */ 4464 public BooleanType getConditionalUpdateElement() { 4465 if (this.conditionalUpdate == null) 4466 if (Configuration.errorOnAutoCreate()) 4467 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalUpdate"); 4468 else if (Configuration.doAutoCreate()) 4469 this.conditionalUpdate = new BooleanType(); // bb 4470 return this.conditionalUpdate; 4471 } 4472 4473 public boolean hasConditionalUpdateElement() { 4474 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4475 } 4476 4477 public boolean hasConditionalUpdate() { 4478 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4479 } 4480 4481 /** 4482 * @param value {@link #conditionalUpdate} (A flag that indicates that the 4483 * server supports conditional update.). This is the underlying 4484 * object with id, value and extensions. The accessor 4485 * "getConditionalUpdate" gives direct access to the value 4486 */ 4487 public CapabilityStatementRestResourceComponent setConditionalUpdateElement(BooleanType value) { 4488 this.conditionalUpdate = value; 4489 return this; 4490 } 4491 4492 /** 4493 * @return A flag that indicates that the server supports conditional update. 4494 */ 4495 public boolean getConditionalUpdate() { 4496 return this.conditionalUpdate == null || this.conditionalUpdate.isEmpty() ? false 4497 : this.conditionalUpdate.getValue(); 4498 } 4499 4500 /** 4501 * @param value A flag that indicates that the server supports conditional 4502 * update. 4503 */ 4504 public CapabilityStatementRestResourceComponent setConditionalUpdate(boolean value) { 4505 if (this.conditionalUpdate == null) 4506 this.conditionalUpdate = new BooleanType(); 4507 this.conditionalUpdate.setValue(value); 4508 return this; 4509 } 4510 4511 /** 4512 * @return {@link #conditionalDelete} (A code that indicates how the server 4513 * supports conditional delete.). This is the underlying object with id, 4514 * value and extensions. The accessor "getConditionalDelete" gives 4515 * direct access to the value 4516 */ 4517 public Enumeration<ConditionalDeleteStatus> getConditionalDeleteElement() { 4518 if (this.conditionalDelete == null) 4519 if (Configuration.errorOnAutoCreate()) 4520 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalDelete"); 4521 else if (Configuration.doAutoCreate()) 4522 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); // bb 4523 return this.conditionalDelete; 4524 } 4525 4526 public boolean hasConditionalDeleteElement() { 4527 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4528 } 4529 4530 public boolean hasConditionalDelete() { 4531 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4532 } 4533 4534 /** 4535 * @param value {@link #conditionalDelete} (A code that indicates how the server 4536 * supports conditional delete.). This is the underlying object 4537 * with id, value and extensions. The accessor 4538 * "getConditionalDelete" gives direct access to the value 4539 */ 4540 public CapabilityStatementRestResourceComponent setConditionalDeleteElement( 4541 Enumeration<ConditionalDeleteStatus> value) { 4542 this.conditionalDelete = value; 4543 return this; 4544 } 4545 4546 /** 4547 * @return A code that indicates how the server supports conditional delete. 4548 */ 4549 public ConditionalDeleteStatus getConditionalDelete() { 4550 return this.conditionalDelete == null ? null : this.conditionalDelete.getValue(); 4551 } 4552 4553 /** 4554 * @param value A code that indicates how the server supports conditional 4555 * delete. 4556 */ 4557 public CapabilityStatementRestResourceComponent setConditionalDelete(ConditionalDeleteStatus value) { 4558 if (value == null) 4559 this.conditionalDelete = null; 4560 else { 4561 if (this.conditionalDelete == null) 4562 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); 4563 this.conditionalDelete.setValue(value); 4564 } 4565 return this; 4566 } 4567 4568 /** 4569 * @return {@link #referencePolicy} (A set of flags that defines how references 4570 * are supported.) 4571 */ 4572 public List<Enumeration<ReferenceHandlingPolicy>> getReferencePolicy() { 4573 if (this.referencePolicy == null) 4574 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4575 return this.referencePolicy; 4576 } 4577 4578 /** 4579 * @return Returns a reference to <code>this</code> for easy method chaining 4580 */ 4581 public CapabilityStatementRestResourceComponent setReferencePolicy( 4582 List<Enumeration<ReferenceHandlingPolicy>> theReferencePolicy) { 4583 this.referencePolicy = theReferencePolicy; 4584 return this; 4585 } 4586 4587 public boolean hasReferencePolicy() { 4588 if (this.referencePolicy == null) 4589 return false; 4590 for (Enumeration<ReferenceHandlingPolicy> item : this.referencePolicy) 4591 if (!item.isEmpty()) 4592 return true; 4593 return false; 4594 } 4595 4596 /** 4597 * @return {@link #referencePolicy} (A set of flags that defines how references 4598 * are supported.) 4599 */ 4600 public Enumeration<ReferenceHandlingPolicy> addReferencePolicyElement() {// 2 4601 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>( 4602 new ReferenceHandlingPolicyEnumFactory()); 4603 if (this.referencePolicy == null) 4604 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4605 this.referencePolicy.add(t); 4606 return t; 4607 } 4608 4609 /** 4610 * @param value {@link #referencePolicy} (A set of flags that defines how 4611 * references are supported.) 4612 */ 4613 public CapabilityStatementRestResourceComponent addReferencePolicy(ReferenceHandlingPolicy value) { // 1 4614 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>( 4615 new ReferenceHandlingPolicyEnumFactory()); 4616 t.setValue(value); 4617 if (this.referencePolicy == null) 4618 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4619 this.referencePolicy.add(t); 4620 return this; 4621 } 4622 4623 /** 4624 * @param value {@link #referencePolicy} (A set of flags that defines how 4625 * references are supported.) 4626 */ 4627 public boolean hasReferencePolicy(ReferenceHandlingPolicy value) { 4628 if (this.referencePolicy == null) 4629 return false; 4630 for (Enumeration<ReferenceHandlingPolicy> v : this.referencePolicy) 4631 if (v.getValue().equals(value)) // code 4632 return true; 4633 return false; 4634 } 4635 4636 /** 4637 * @return {@link #searchInclude} (A list of _include values supported by the 4638 * server.) 4639 */ 4640 public List<StringType> getSearchInclude() { 4641 if (this.searchInclude == null) 4642 this.searchInclude = new ArrayList<StringType>(); 4643 return this.searchInclude; 4644 } 4645 4646 /** 4647 * @return Returns a reference to <code>this</code> for easy method chaining 4648 */ 4649 public CapabilityStatementRestResourceComponent setSearchInclude(List<StringType> theSearchInclude) { 4650 this.searchInclude = theSearchInclude; 4651 return this; 4652 } 4653 4654 public boolean hasSearchInclude() { 4655 if (this.searchInclude == null) 4656 return false; 4657 for (StringType item : this.searchInclude) 4658 if (!item.isEmpty()) 4659 return true; 4660 return false; 4661 } 4662 4663 /** 4664 * @return {@link #searchInclude} (A list of _include values supported by the 4665 * server.) 4666 */ 4667 public StringType addSearchIncludeElement() {// 2 4668 StringType t = new StringType(); 4669 if (this.searchInclude == null) 4670 this.searchInclude = new ArrayList<StringType>(); 4671 this.searchInclude.add(t); 4672 return t; 4673 } 4674 4675 /** 4676 * @param value {@link #searchInclude} (A list of _include values supported by 4677 * the server.) 4678 */ 4679 public CapabilityStatementRestResourceComponent addSearchInclude(String value) { // 1 4680 StringType t = new StringType(); 4681 t.setValue(value); 4682 if (this.searchInclude == null) 4683 this.searchInclude = new ArrayList<StringType>(); 4684 this.searchInclude.add(t); 4685 return this; 4686 } 4687 4688 /** 4689 * @param value {@link #searchInclude} (A list of _include values supported by 4690 * the server.) 4691 */ 4692 public boolean hasSearchInclude(String value) { 4693 if (this.searchInclude == null) 4694 return false; 4695 for (StringType v : this.searchInclude) 4696 if (v.getValue().equals(value)) // string 4697 return true; 4698 return false; 4699 } 4700 4701 /** 4702 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4703 * values supported by the server.) 4704 */ 4705 public List<StringType> getSearchRevInclude() { 4706 if (this.searchRevInclude == null) 4707 this.searchRevInclude = new ArrayList<StringType>(); 4708 return this.searchRevInclude; 4709 } 4710 4711 /** 4712 * @return Returns a reference to <code>this</code> for easy method chaining 4713 */ 4714 public CapabilityStatementRestResourceComponent setSearchRevInclude(List<StringType> theSearchRevInclude) { 4715 this.searchRevInclude = theSearchRevInclude; 4716 return this; 4717 } 4718 4719 public boolean hasSearchRevInclude() { 4720 if (this.searchRevInclude == null) 4721 return false; 4722 for (StringType item : this.searchRevInclude) 4723 if (!item.isEmpty()) 4724 return true; 4725 return false; 4726 } 4727 4728 /** 4729 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4730 * values supported by the server.) 4731 */ 4732 public StringType addSearchRevIncludeElement() {// 2 4733 StringType t = new StringType(); 4734 if (this.searchRevInclude == null) 4735 this.searchRevInclude = new ArrayList<StringType>(); 4736 this.searchRevInclude.add(t); 4737 return t; 4738 } 4739 4740 /** 4741 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4742 * include) values supported by the server.) 4743 */ 4744 public CapabilityStatementRestResourceComponent addSearchRevInclude(String value) { // 1 4745 StringType t = new StringType(); 4746 t.setValue(value); 4747 if (this.searchRevInclude == null) 4748 this.searchRevInclude = new ArrayList<StringType>(); 4749 this.searchRevInclude.add(t); 4750 return this; 4751 } 4752 4753 /** 4754 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4755 * include) values supported by the server.) 4756 */ 4757 public boolean hasSearchRevInclude(String value) { 4758 if (this.searchRevInclude == null) 4759 return false; 4760 for (StringType v : this.searchRevInclude) 4761 if (v.getValue().equals(value)) // string 4762 return true; 4763 return false; 4764 } 4765 4766 /** 4767 * @return {@link #searchParam} (Search parameters for implementations to 4768 * support and/or make use of - either references to ones defined in the 4769 * specification, or additional ones defined for/by the implementation.) 4770 */ 4771 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 4772 if (this.searchParam == null) 4773 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4774 return this.searchParam; 4775 } 4776 4777 /** 4778 * @return Returns a reference to <code>this</code> for easy method chaining 4779 */ 4780 public CapabilityStatementRestResourceComponent setSearchParam( 4781 List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 4782 this.searchParam = theSearchParam; 4783 return this; 4784 } 4785 4786 public boolean hasSearchParam() { 4787 if (this.searchParam == null) 4788 return false; 4789 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 4790 if (!item.isEmpty()) 4791 return true; 4792 return false; 4793 } 4794 4795 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { // 3 4796 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 4797 if (this.searchParam == null) 4798 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4799 this.searchParam.add(t); 4800 return t; 4801 } 4802 4803 public CapabilityStatementRestResourceComponent addSearchParam( 4804 CapabilityStatementRestResourceSearchParamComponent t) { // 3 4805 if (t == null) 4806 return this; 4807 if (this.searchParam == null) 4808 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4809 this.searchParam.add(t); 4810 return this; 4811 } 4812 4813 /** 4814 * @return The first repetition of repeating field {@link #searchParam}, 4815 * creating it if it does not already exist 4816 */ 4817 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 4818 if (getSearchParam().isEmpty()) { 4819 addSearchParam(); 4820 } 4821 return getSearchParam().get(0); 4822 } 4823 4824 /** 4825 * @return {@link #operation} (Definition of an operation or a named query 4826 * together with its parameters and their meaning and type. Consult the 4827 * definition of the operation for details about how to invoke the 4828 * operation, and the parameters.) 4829 */ 4830 public List<CapabilityStatementRestResourceOperationComponent> getOperation() { 4831 if (this.operation == null) 4832 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4833 return this.operation; 4834 } 4835 4836 /** 4837 * @return Returns a reference to <code>this</code> for easy method chaining 4838 */ 4839 public CapabilityStatementRestResourceComponent setOperation( 4840 List<CapabilityStatementRestResourceOperationComponent> theOperation) { 4841 this.operation = theOperation; 4842 return this; 4843 } 4844 4845 public boolean hasOperation() { 4846 if (this.operation == null) 4847 return false; 4848 for (CapabilityStatementRestResourceOperationComponent item : this.operation) 4849 if (!item.isEmpty()) 4850 return true; 4851 return false; 4852 } 4853 4854 public CapabilityStatementRestResourceOperationComponent addOperation() { // 3 4855 CapabilityStatementRestResourceOperationComponent t = new CapabilityStatementRestResourceOperationComponent(); 4856 if (this.operation == null) 4857 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4858 this.operation.add(t); 4859 return t; 4860 } 4861 4862 public CapabilityStatementRestResourceComponent addOperation(CapabilityStatementRestResourceOperationComponent t) { // 3 4863 if (t == null) 4864 return this; 4865 if (this.operation == null) 4866 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4867 this.operation.add(t); 4868 return this; 4869 } 4870 4871 /** 4872 * @return The first repetition of repeating field {@link #operation}, creating 4873 * it if it does not already exist 4874 */ 4875 public CapabilityStatementRestResourceOperationComponent getOperationFirstRep() { 4876 if (getOperation().isEmpty()) { 4877 addOperation(); 4878 } 4879 return getOperation().get(0); 4880 } 4881 4882 protected void listChildren(List<Property> children) { 4883 super.listChildren(children); 4884 children.add(new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, type)); 4885 children.add(new Property("profile", "canonical(StructureDefinition)", 4886 "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4887 0, 1, profile)); 4888 children.add(new Property("supportedProfile", "canonical(StructureDefinition)", 4889 "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4890 0, java.lang.Integer.MAX_VALUE, supportedProfile)); 4891 children.add(new Property("documentation", "markdown", 4892 "Additional information about the resource type used by the system.", 0, 1, documentation)); 4893 children.add(new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, 4894 java.lang.Integer.MAX_VALUE, interaction)); 4895 children.add(new Property("versioning", "code", 4896 "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 4897 0, 1, versioning)); 4898 children.add(new Property("readHistory", "boolean", 4899 "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, 4900 readHistory)); 4901 children.add(new Property("updateCreate", "boolean", 4902 "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 4903 0, 1, updateCreate)); 4904 children.add(new Property("conditionalCreate", "boolean", 4905 "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate)); 4906 children.add(new Property("conditionalRead", "code", 4907 "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead)); 4908 children.add(new Property("conditionalUpdate", "boolean", 4909 "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate)); 4910 children.add(new Property("conditionalDelete", "code", 4911 "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete)); 4912 children.add(new Property("referencePolicy", "code", "A set of flags that defines how references are supported.", 4913 0, java.lang.Integer.MAX_VALUE, referencePolicy)); 4914 children.add(new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, 4915 java.lang.Integer.MAX_VALUE, searchInclude)); 4916 children.add(new Property("searchRevInclude", "string", 4917 "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, 4918 searchRevInclude)); 4919 children.add(new Property("searchParam", "", 4920 "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 4921 0, java.lang.Integer.MAX_VALUE, searchParam)); 4922 children.add(new Property("operation", "", 4923 "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.", 4924 0, java.lang.Integer.MAX_VALUE, operation)); 4925 } 4926 4927 @Override 4928 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4929 switch (_hash) { 4930 case 3575610: 4931 /* type */ return new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, 4932 type); 4933 case -309425751: 4934 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 4935 "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4936 0, 1, profile); 4937 case 1225477403: 4938 /* supportedProfile */ return new Property("supportedProfile", "canonical(StructureDefinition)", 4939 "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4940 0, java.lang.Integer.MAX_VALUE, supportedProfile); 4941 case 1587405498: 4942 /* documentation */ return new Property("documentation", "markdown", 4943 "Additional information about the resource type used by the system.", 0, 1, documentation); 4944 case 1844104722: 4945 /* interaction */ return new Property("interaction", "", 4946 "Identifies a restful operation supported by the solution.", 0, java.lang.Integer.MAX_VALUE, interaction); 4947 case -670487542: 4948 /* versioning */ return new Property("versioning", "code", 4949 "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 4950 0, 1, versioning); 4951 case 187518494: 4952 /* readHistory */ return new Property("readHistory", "boolean", 4953 "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, 4954 readHistory); 4955 case -1400550619: 4956 /* updateCreate */ return new Property("updateCreate", "boolean", 4957 "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 4958 0, 1, updateCreate); 4959 case 6401826: 4960 /* conditionalCreate */ return new Property("conditionalCreate", "boolean", 4961 "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate); 4962 case 822786364: 4963 /* conditionalRead */ return new Property("conditionalRead", "code", 4964 "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead); 4965 case 519849711: 4966 /* conditionalUpdate */ return new Property("conditionalUpdate", "boolean", 4967 "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate); 4968 case 23237585: 4969 /* conditionalDelete */ return new Property("conditionalDelete", "code", 4970 "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete); 4971 case 796257373: 4972 /* referencePolicy */ return new Property("referencePolicy", "code", 4973 "A set of flags that defines how references are supported.", 0, java.lang.Integer.MAX_VALUE, 4974 referencePolicy); 4975 case -1035904544: 4976 /* searchInclude */ return new Property("searchInclude", "string", 4977 "A list of _include values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchInclude); 4978 case -2123884979: 4979 /* searchRevInclude */ return new Property("searchRevInclude", "string", 4980 "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, 4981 searchRevInclude); 4982 case -553645115: 4983 /* searchParam */ return new Property("searchParam", "", 4984 "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 4985 0, java.lang.Integer.MAX_VALUE, searchParam); 4986 case 1662702951: 4987 /* operation */ return new Property("operation", "", 4988 "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.", 4989 0, java.lang.Integer.MAX_VALUE, operation); 4990 default: 4991 return super.getNamedProperty(_hash, _name, _checkValid); 4992 } 4993 4994 } 4995 4996 @Override 4997 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4998 switch (hash) { 4999 case 3575610: 5000 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 5001 case -309425751: 5002 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 5003 case 1225477403: 5004 /* supportedProfile */ return this.supportedProfile == null ? new Base[0] 5005 : this.supportedProfile.toArray(new Base[this.supportedProfile.size()]); // CanonicalType 5006 case 1587405498: 5007 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 5008 case 1844104722: 5009 /* interaction */ return this.interaction == null ? new Base[0] 5010 : this.interaction.toArray(new Base[this.interaction.size()]); // ResourceInteractionComponent 5011 case -670487542: 5012 /* versioning */ return this.versioning == null ? new Base[0] : new Base[] { this.versioning }; // Enumeration<ResourceVersionPolicy> 5013 case 187518494: 5014 /* readHistory */ return this.readHistory == null ? new Base[0] : new Base[] { this.readHistory }; // BooleanType 5015 case -1400550619: 5016 /* updateCreate */ return this.updateCreate == null ? new Base[0] : new Base[] { this.updateCreate }; // BooleanType 5017 case 6401826: 5018 /* conditionalCreate */ return this.conditionalCreate == null ? new Base[0] 5019 : new Base[] { this.conditionalCreate }; // BooleanType 5020 case 822786364: 5021 /* conditionalRead */ return this.conditionalRead == null ? new Base[0] : new Base[] { this.conditionalRead }; // Enumeration<ConditionalReadStatus> 5022 case 519849711: 5023 /* conditionalUpdate */ return this.conditionalUpdate == null ? new Base[0] 5024 : new Base[] { this.conditionalUpdate }; // BooleanType 5025 case 23237585: 5026 /* conditionalDelete */ return this.conditionalDelete == null ? new Base[0] 5027 : new Base[] { this.conditionalDelete }; // Enumeration<ConditionalDeleteStatus> 5028 case 796257373: 5029 /* referencePolicy */ return this.referencePolicy == null ? new Base[0] 5030 : this.referencePolicy.toArray(new Base[this.referencePolicy.size()]); // Enumeration<ReferenceHandlingPolicy> 5031 case -1035904544: 5032 /* searchInclude */ return this.searchInclude == null ? new Base[0] 5033 : this.searchInclude.toArray(new Base[this.searchInclude.size()]); // StringType 5034 case -2123884979: 5035 /* searchRevInclude */ return this.searchRevInclude == null ? new Base[0] 5036 : this.searchRevInclude.toArray(new Base[this.searchRevInclude.size()]); // StringType 5037 case -553645115: 5038 /* searchParam */ return this.searchParam == null ? new Base[0] 5039 : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 5040 case 1662702951: 5041 /* operation */ return this.operation == null ? new Base[0] 5042 : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestResourceOperationComponent 5043 default: 5044 return super.getProperty(hash, name, checkValid); 5045 } 5046 5047 } 5048 5049 @Override 5050 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5051 switch (hash) { 5052 case 3575610: // type 5053 this.type = castToCode(value); // CodeType 5054 return value; 5055 case -309425751: // profile 5056 this.profile = castToCanonical(value); // CanonicalType 5057 return value; 5058 case 1225477403: // supportedProfile 5059 this.getSupportedProfile().add(castToCanonical(value)); // CanonicalType 5060 return value; 5061 case 1587405498: // documentation 5062 this.documentation = castToMarkdown(value); // MarkdownType 5063 return value; 5064 case 1844104722: // interaction 5065 this.getInteraction().add((ResourceInteractionComponent) value); // ResourceInteractionComponent 5066 return value; 5067 case -670487542: // versioning 5068 value = new ResourceVersionPolicyEnumFactory().fromType(castToCode(value)); 5069 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 5070 return value; 5071 case 187518494: // readHistory 5072 this.readHistory = castToBoolean(value); // BooleanType 5073 return value; 5074 case -1400550619: // updateCreate 5075 this.updateCreate = castToBoolean(value); // BooleanType 5076 return value; 5077 case 6401826: // conditionalCreate 5078 this.conditionalCreate = castToBoolean(value); // BooleanType 5079 return value; 5080 case 822786364: // conditionalRead 5081 value = new ConditionalReadStatusEnumFactory().fromType(castToCode(value)); 5082 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 5083 return value; 5084 case 519849711: // conditionalUpdate 5085 this.conditionalUpdate = castToBoolean(value); // BooleanType 5086 return value; 5087 case 23237585: // conditionalDelete 5088 value = new ConditionalDeleteStatusEnumFactory().fromType(castToCode(value)); 5089 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 5090 return value; 5091 case 796257373: // referencePolicy 5092 value = new ReferenceHandlingPolicyEnumFactory().fromType(castToCode(value)); 5093 this.getReferencePolicy().add((Enumeration) value); // Enumeration<ReferenceHandlingPolicy> 5094 return value; 5095 case -1035904544: // searchInclude 5096 this.getSearchInclude().add(castToString(value)); // StringType 5097 return value; 5098 case -2123884979: // searchRevInclude 5099 this.getSearchRevInclude().add(castToString(value)); // StringType 5100 return value; 5101 case -553645115: // searchParam 5102 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 5103 return value; 5104 case 1662702951: // operation 5105 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); // CapabilityStatementRestResourceOperationComponent 5106 return value; 5107 default: 5108 return super.setProperty(hash, name, value); 5109 } 5110 5111 } 5112 5113 @Override 5114 public Base setProperty(String name, Base value) throws FHIRException { 5115 if (name.equals("type")) { 5116 this.type = castToCode(value); // CodeType 5117 } else if (name.equals("profile")) { 5118 this.profile = castToCanonical(value); // CanonicalType 5119 } else if (name.equals("supportedProfile")) { 5120 this.getSupportedProfile().add(castToCanonical(value)); 5121 } else if (name.equals("documentation")) { 5122 this.documentation = castToMarkdown(value); // MarkdownType 5123 } else if (name.equals("interaction")) { 5124 this.getInteraction().add((ResourceInteractionComponent) value); 5125 } else if (name.equals("versioning")) { 5126 value = new ResourceVersionPolicyEnumFactory().fromType(castToCode(value)); 5127 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 5128 } else if (name.equals("readHistory")) { 5129 this.readHistory = castToBoolean(value); // BooleanType 5130 } else if (name.equals("updateCreate")) { 5131 this.updateCreate = castToBoolean(value); // BooleanType 5132 } else if (name.equals("conditionalCreate")) { 5133 this.conditionalCreate = castToBoolean(value); // BooleanType 5134 } else if (name.equals("conditionalRead")) { 5135 value = new ConditionalReadStatusEnumFactory().fromType(castToCode(value)); 5136 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 5137 } else if (name.equals("conditionalUpdate")) { 5138 this.conditionalUpdate = castToBoolean(value); // BooleanType 5139 } else if (name.equals("conditionalDelete")) { 5140 value = new ConditionalDeleteStatusEnumFactory().fromType(castToCode(value)); 5141 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 5142 } else if (name.equals("referencePolicy")) { 5143 value = new ReferenceHandlingPolicyEnumFactory().fromType(castToCode(value)); 5144 this.getReferencePolicy().add((Enumeration) value); 5145 } else if (name.equals("searchInclude")) { 5146 this.getSearchInclude().add(castToString(value)); 5147 } else if (name.equals("searchRevInclude")) { 5148 this.getSearchRevInclude().add(castToString(value)); 5149 } else if (name.equals("searchParam")) { 5150 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 5151 } else if (name.equals("operation")) { 5152 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); 5153 } else 5154 return super.setProperty(name, value); 5155 return value; 5156 } 5157 5158 @Override 5159 public void removeChild(String name, Base value) throws FHIRException { 5160 if (name.equals("type")) { 5161 this.type = null; 5162 } else if (name.equals("profile")) { 5163 this.profile = null; 5164 } else if (name.equals("supportedProfile")) { 5165 this.getSupportedProfile().remove(castToCanonical(value)); 5166 } else if (name.equals("documentation")) { 5167 this.documentation = null; 5168 } else if (name.equals("interaction")) { 5169 this.getInteraction().remove((ResourceInteractionComponent) value); 5170 } else if (name.equals("versioning")) { 5171 this.versioning = null; 5172 } else if (name.equals("readHistory")) { 5173 this.readHistory = null; 5174 } else if (name.equals("updateCreate")) { 5175 this.updateCreate = null; 5176 } else if (name.equals("conditionalCreate")) { 5177 this.conditionalCreate = null; 5178 } else if (name.equals("conditionalRead")) { 5179 this.conditionalRead = null; 5180 } else if (name.equals("conditionalUpdate")) { 5181 this.conditionalUpdate = null; 5182 } else if (name.equals("conditionalDelete")) { 5183 this.conditionalDelete = null; 5184 } else if (name.equals("referencePolicy")) { 5185 this.getReferencePolicy().remove((Enumeration) value); 5186 } else if (name.equals("searchInclude")) { 5187 this.getSearchInclude().remove(castToString(value)); 5188 } else if (name.equals("searchRevInclude")) { 5189 this.getSearchRevInclude().remove(castToString(value)); 5190 } else if (name.equals("searchParam")) { 5191 this.getSearchParam().remove((CapabilityStatementRestResourceSearchParamComponent) value); 5192 } else if (name.equals("operation")) { 5193 this.getOperation().remove((CapabilityStatementRestResourceOperationComponent) value); 5194 } else 5195 super.removeChild(name, value); 5196 5197 } 5198 5199 @Override 5200 public Base makeProperty(int hash, String name) throws FHIRException { 5201 switch (hash) { 5202 case 3575610: 5203 return getTypeElement(); 5204 case -309425751: 5205 return getProfileElement(); 5206 case 1225477403: 5207 return addSupportedProfileElement(); 5208 case 1587405498: 5209 return getDocumentationElement(); 5210 case 1844104722: 5211 return addInteraction(); 5212 case -670487542: 5213 return getVersioningElement(); 5214 case 187518494: 5215 return getReadHistoryElement(); 5216 case -1400550619: 5217 return getUpdateCreateElement(); 5218 case 6401826: 5219 return getConditionalCreateElement(); 5220 case 822786364: 5221 return getConditionalReadElement(); 5222 case 519849711: 5223 return getConditionalUpdateElement(); 5224 case 23237585: 5225 return getConditionalDeleteElement(); 5226 case 796257373: 5227 return addReferencePolicyElement(); 5228 case -1035904544: 5229 return addSearchIncludeElement(); 5230 case -2123884979: 5231 return addSearchRevIncludeElement(); 5232 case -553645115: 5233 return addSearchParam(); 5234 case 1662702951: 5235 return addOperation(); 5236 default: 5237 return super.makeProperty(hash, name); 5238 } 5239 5240 } 5241 5242 @Override 5243 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5244 switch (hash) { 5245 case 3575610: 5246 /* type */ return new String[] { "code" }; 5247 case -309425751: 5248 /* profile */ return new String[] { "canonical" }; 5249 case 1225477403: 5250 /* supportedProfile */ return new String[] { "canonical" }; 5251 case 1587405498: 5252 /* documentation */ return new String[] { "markdown" }; 5253 case 1844104722: 5254 /* interaction */ return new String[] {}; 5255 case -670487542: 5256 /* versioning */ return new String[] { "code" }; 5257 case 187518494: 5258 /* readHistory */ return new String[] { "boolean" }; 5259 case -1400550619: 5260 /* updateCreate */ return new String[] { "boolean" }; 5261 case 6401826: 5262 /* conditionalCreate */ return new String[] { "boolean" }; 5263 case 822786364: 5264 /* conditionalRead */ return new String[] { "code" }; 5265 case 519849711: 5266 /* conditionalUpdate */ return new String[] { "boolean" }; 5267 case 23237585: 5268 /* conditionalDelete */ return new String[] { "code" }; 5269 case 796257373: 5270 /* referencePolicy */ return new String[] { "code" }; 5271 case -1035904544: 5272 /* searchInclude */ return new String[] { "string" }; 5273 case -2123884979: 5274 /* searchRevInclude */ return new String[] { "string" }; 5275 case -553645115: 5276 /* searchParam */ return new String[] {}; 5277 case 1662702951: 5278 /* operation */ return new String[] {}; 5279 default: 5280 return super.getTypesForProperty(hash, name); 5281 } 5282 5283 } 5284 5285 @Override 5286 public Base addChild(String name) throws FHIRException { 5287 if (name.equals("type")) { 5288 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 5289 } else if (name.equals("profile")) { 5290 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.profile"); 5291 } else if (name.equals("supportedProfile")) { 5292 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.supportedProfile"); 5293 } else if (name.equals("documentation")) { 5294 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 5295 } else if (name.equals("interaction")) { 5296 return addInteraction(); 5297 } else if (name.equals("versioning")) { 5298 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.versioning"); 5299 } else if (name.equals("readHistory")) { 5300 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.readHistory"); 5301 } else if (name.equals("updateCreate")) { 5302 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.updateCreate"); 5303 } else if (name.equals("conditionalCreate")) { 5304 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalCreate"); 5305 } else if (name.equals("conditionalRead")) { 5306 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalRead"); 5307 } else if (name.equals("conditionalUpdate")) { 5308 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalUpdate"); 5309 } else if (name.equals("conditionalDelete")) { 5310 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalDelete"); 5311 } else if (name.equals("referencePolicy")) { 5312 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.referencePolicy"); 5313 } else if (name.equals("searchInclude")) { 5314 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.searchInclude"); 5315 } else if (name.equals("searchRevInclude")) { 5316 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.searchRevInclude"); 5317 } else if (name.equals("searchParam")) { 5318 return addSearchParam(); 5319 } else if (name.equals("operation")) { 5320 return addOperation(); 5321 } else 5322 return super.addChild(name); 5323 } 5324 5325 public CapabilityStatementRestResourceComponent copy() { 5326 CapabilityStatementRestResourceComponent dst = new CapabilityStatementRestResourceComponent(); 5327 copyValues(dst); 5328 return dst; 5329 } 5330 5331 public void copyValues(CapabilityStatementRestResourceComponent dst) { 5332 super.copyValues(dst); 5333 dst.type = type == null ? null : type.copy(); 5334 dst.profile = profile == null ? null : profile.copy(); 5335 if (supportedProfile != null) { 5336 dst.supportedProfile = new ArrayList<CanonicalType>(); 5337 for (CanonicalType i : supportedProfile) 5338 dst.supportedProfile.add(i.copy()); 5339 } 5340 ; 5341 dst.documentation = documentation == null ? null : documentation.copy(); 5342 if (interaction != null) { 5343 dst.interaction = new ArrayList<ResourceInteractionComponent>(); 5344 for (ResourceInteractionComponent i : interaction) 5345 dst.interaction.add(i.copy()); 5346 } 5347 ; 5348 dst.versioning = versioning == null ? null : versioning.copy(); 5349 dst.readHistory = readHistory == null ? null : readHistory.copy(); 5350 dst.updateCreate = updateCreate == null ? null : updateCreate.copy(); 5351 dst.conditionalCreate = conditionalCreate == null ? null : conditionalCreate.copy(); 5352 dst.conditionalRead = conditionalRead == null ? null : conditionalRead.copy(); 5353 dst.conditionalUpdate = conditionalUpdate == null ? null : conditionalUpdate.copy(); 5354 dst.conditionalDelete = conditionalDelete == null ? null : conditionalDelete.copy(); 5355 if (referencePolicy != null) { 5356 dst.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 5357 for (Enumeration<ReferenceHandlingPolicy> i : referencePolicy) 5358 dst.referencePolicy.add(i.copy()); 5359 } 5360 ; 5361 if (searchInclude != null) { 5362 dst.searchInclude = new ArrayList<StringType>(); 5363 for (StringType i : searchInclude) 5364 dst.searchInclude.add(i.copy()); 5365 } 5366 ; 5367 if (searchRevInclude != null) { 5368 dst.searchRevInclude = new ArrayList<StringType>(); 5369 for (StringType i : searchRevInclude) 5370 dst.searchRevInclude.add(i.copy()); 5371 } 5372 ; 5373 if (searchParam != null) { 5374 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 5375 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 5376 dst.searchParam.add(i.copy()); 5377 } 5378 ; 5379 if (operation != null) { 5380 dst.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 5381 for (CapabilityStatementRestResourceOperationComponent i : operation) 5382 dst.operation.add(i.copy()); 5383 } 5384 ; 5385 } 5386 5387 @Override 5388 public boolean equalsDeep(Base other_) { 5389 if (!super.equalsDeep(other_)) 5390 return false; 5391 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 5392 return false; 5393 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 5394 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) 5395 && compareDeep(supportedProfile, o.supportedProfile, true) 5396 && compareDeep(documentation, o.documentation, true) && compareDeep(interaction, o.interaction, true) 5397 && compareDeep(versioning, o.versioning, true) && compareDeep(readHistory, o.readHistory, true) 5398 && compareDeep(updateCreate, o.updateCreate, true) 5399 && compareDeep(conditionalCreate, o.conditionalCreate, true) 5400 && compareDeep(conditionalRead, o.conditionalRead, true) 5401 && compareDeep(conditionalUpdate, o.conditionalUpdate, true) 5402 && compareDeep(conditionalDelete, o.conditionalDelete, true) 5403 && compareDeep(referencePolicy, o.referencePolicy, true) && compareDeep(searchInclude, o.searchInclude, true) 5404 && compareDeep(searchRevInclude, o.searchRevInclude, true) && compareDeep(searchParam, o.searchParam, true) 5405 && compareDeep(operation, o.operation, true); 5406 } 5407 5408 @Override 5409 public boolean equalsShallow(Base other_) { 5410 if (!super.equalsShallow(other_)) 5411 return false; 5412 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 5413 return false; 5414 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 5415 return compareValues(type, o.type, true) && compareValues(documentation, o.documentation, true) 5416 && compareValues(versioning, o.versioning, true) && compareValues(readHistory, o.readHistory, true) 5417 && compareValues(updateCreate, o.updateCreate, true) 5418 && compareValues(conditionalCreate, o.conditionalCreate, true) 5419 && compareValues(conditionalRead, o.conditionalRead, true) 5420 && compareValues(conditionalUpdate, o.conditionalUpdate, true) 5421 && compareValues(conditionalDelete, o.conditionalDelete, true) 5422 && compareValues(referencePolicy, o.referencePolicy, true) 5423 && compareValues(searchInclude, o.searchInclude, true) 5424 && compareValues(searchRevInclude, o.searchRevInclude, true); 5425 } 5426 5427 public boolean isEmpty() { 5428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, supportedProfile, documentation, 5429 interaction, versioning, readHistory, updateCreate, conditionalCreate, conditionalRead, conditionalUpdate, 5430 conditionalDelete, referencePolicy, searchInclude, searchRevInclude, searchParam, operation); 5431 } 5432 5433 public String fhirType() { 5434 return "CapabilityStatement.rest.resource"; 5435 5436 } 5437 5438 } 5439 5440 @Block() 5441 public static class ResourceInteractionComponent extends BackboneElement implements IBaseBackboneElement { 5442 /** 5443 * Coded identifier of the operation, supported by the system resource. 5444 */ 5445 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5446 @Description(shortDefinition = "read | vread | update | patch | delete | history-instance | history-type | create | search-type", formalDefinition = "Coded identifier of the operation, supported by the system resource.") 5447 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/type-restful-interaction") 5448 protected Enumeration<TypeRestfulInteraction> code; 5449 5450 /** 5451 * Guidance specific to the implementation of this operation, such as 'delete is 5452 * a logical delete' or 'updates are only allowed with version id' or 'creates 5453 * permitted from pre-authorized certificates only'. 5454 */ 5455 @Child(name = "documentation", type = { 5456 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5457 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.") 5458 protected MarkdownType documentation; 5459 5460 private static final long serialVersionUID = 2128937796L; 5461 5462 /** 5463 * Constructor 5464 */ 5465 public ResourceInteractionComponent() { 5466 super(); 5467 } 5468 5469 /** 5470 * Constructor 5471 */ 5472 public ResourceInteractionComponent(Enumeration<TypeRestfulInteraction> code) { 5473 super(); 5474 this.code = code; 5475 } 5476 5477 /** 5478 * @return {@link #code} (Coded identifier of the operation, supported by the 5479 * system resource.). This is the underlying object with id, value and 5480 * extensions. The accessor "getCode" gives direct access to the value 5481 */ 5482 public Enumeration<TypeRestfulInteraction> getCodeElement() { 5483 if (this.code == null) 5484 if (Configuration.errorOnAutoCreate()) 5485 throw new Error("Attempt to auto-create ResourceInteractionComponent.code"); 5486 else if (Configuration.doAutoCreate()) 5487 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); // bb 5488 return this.code; 5489 } 5490 5491 public boolean hasCodeElement() { 5492 return this.code != null && !this.code.isEmpty(); 5493 } 5494 5495 public boolean hasCode() { 5496 return this.code != null && !this.code.isEmpty(); 5497 } 5498 5499 /** 5500 * @param value {@link #code} (Coded identifier of the operation, supported by 5501 * the system resource.). This is the underlying object with id, 5502 * value and extensions. The accessor "getCode" gives direct access 5503 * to the value 5504 */ 5505 public ResourceInteractionComponent setCodeElement(Enumeration<TypeRestfulInteraction> value) { 5506 this.code = value; 5507 return this; 5508 } 5509 5510 /** 5511 * @return Coded identifier of the operation, supported by the system resource. 5512 */ 5513 public TypeRestfulInteraction getCode() { 5514 return this.code == null ? null : this.code.getValue(); 5515 } 5516 5517 /** 5518 * @param value Coded identifier of the operation, supported by the system 5519 * resource. 5520 */ 5521 public ResourceInteractionComponent setCode(TypeRestfulInteraction value) { 5522 if (this.code == null) 5523 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); 5524 this.code.setValue(value); 5525 return this; 5526 } 5527 5528 /** 5529 * @return {@link #documentation} (Guidance specific to the implementation of 5530 * this operation, such as 'delete is a logical delete' or 'updates are 5531 * only allowed with version id' or 'creates permitted from 5532 * pre-authorized certificates only'.). This is the underlying object 5533 * with id, value and extensions. The accessor "getDocumentation" gives 5534 * direct access to the value 5535 */ 5536 public MarkdownType getDocumentationElement() { 5537 if (this.documentation == null) 5538 if (Configuration.errorOnAutoCreate()) 5539 throw new Error("Attempt to auto-create ResourceInteractionComponent.documentation"); 5540 else if (Configuration.doAutoCreate()) 5541 this.documentation = new MarkdownType(); // bb 5542 return this.documentation; 5543 } 5544 5545 public boolean hasDocumentationElement() { 5546 return this.documentation != null && !this.documentation.isEmpty(); 5547 } 5548 5549 public boolean hasDocumentation() { 5550 return this.documentation != null && !this.documentation.isEmpty(); 5551 } 5552 5553 /** 5554 * @param value {@link #documentation} (Guidance specific to the implementation 5555 * of this operation, such as 'delete is a logical delete' or 5556 * 'updates are only allowed with version id' or 'creates permitted 5557 * from pre-authorized certificates only'.). This is the underlying 5558 * object with id, value and extensions. The accessor 5559 * "getDocumentation" gives direct access to the value 5560 */ 5561 public ResourceInteractionComponent setDocumentationElement(MarkdownType value) { 5562 this.documentation = value; 5563 return this; 5564 } 5565 5566 /** 5567 * @return Guidance specific to the implementation of this operation, such as 5568 * 'delete is a logical delete' or 'updates are only allowed with 5569 * version id' or 'creates permitted from pre-authorized certificates 5570 * only'. 5571 */ 5572 public String getDocumentation() { 5573 return this.documentation == null ? null : this.documentation.getValue(); 5574 } 5575 5576 /** 5577 * @param value Guidance specific to the implementation of this operation, such 5578 * as 'delete is a logical delete' or 'updates are only allowed 5579 * with version id' or 'creates permitted from pre-authorized 5580 * certificates only'. 5581 */ 5582 public ResourceInteractionComponent setDocumentation(String value) { 5583 if (value == null) 5584 this.documentation = null; 5585 else { 5586 if (this.documentation == null) 5587 this.documentation = new MarkdownType(); 5588 this.documentation.setValue(value); 5589 } 5590 return this; 5591 } 5592 5593 protected void listChildren(List<Property> children) { 5594 super.listChildren(children); 5595 children.add(new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 5596 0, 1, code)); 5597 children.add(new Property("documentation", "markdown", 5598 "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 5599 0, 1, documentation)); 5600 } 5601 5602 @Override 5603 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5604 switch (_hash) { 5605 case 3059181: 5606 /* code */ return new Property("code", "code", 5607 "Coded identifier of the operation, supported by the system resource.", 0, 1, code); 5608 case 1587405498: 5609 /* documentation */ return new Property("documentation", "markdown", 5610 "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 5611 0, 1, documentation); 5612 default: 5613 return super.getNamedProperty(_hash, _name, _checkValid); 5614 } 5615 5616 } 5617 5618 @Override 5619 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5620 switch (hash) { 5621 case 3059181: 5622 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<TypeRestfulInteraction> 5623 case 1587405498: 5624 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 5625 default: 5626 return super.getProperty(hash, name, checkValid); 5627 } 5628 5629 } 5630 5631 @Override 5632 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5633 switch (hash) { 5634 case 3059181: // code 5635 value = new TypeRestfulInteractionEnumFactory().fromType(castToCode(value)); 5636 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 5637 return value; 5638 case 1587405498: // documentation 5639 this.documentation = castToMarkdown(value); // MarkdownType 5640 return value; 5641 default: 5642 return super.setProperty(hash, name, value); 5643 } 5644 5645 } 5646 5647 @Override 5648 public Base setProperty(String name, Base value) throws FHIRException { 5649 if (name.equals("code")) { 5650 value = new TypeRestfulInteractionEnumFactory().fromType(castToCode(value)); 5651 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 5652 } else if (name.equals("documentation")) { 5653 this.documentation = castToMarkdown(value); // MarkdownType 5654 } else 5655 return super.setProperty(name, value); 5656 return value; 5657 } 5658 5659 @Override 5660 public void removeChild(String name, Base value) throws FHIRException { 5661 if (name.equals("code")) { 5662 this.code = null; 5663 } else if (name.equals("documentation")) { 5664 this.documentation = null; 5665 } else 5666 super.removeChild(name, value); 5667 5668 } 5669 5670 @Override 5671 public Base makeProperty(int hash, String name) throws FHIRException { 5672 switch (hash) { 5673 case 3059181: 5674 return getCodeElement(); 5675 case 1587405498: 5676 return getDocumentationElement(); 5677 default: 5678 return super.makeProperty(hash, name); 5679 } 5680 5681 } 5682 5683 @Override 5684 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5685 switch (hash) { 5686 case 3059181: 5687 /* code */ return new String[] { "code" }; 5688 case 1587405498: 5689 /* documentation */ return new String[] { "markdown" }; 5690 default: 5691 return super.getTypesForProperty(hash, name); 5692 } 5693 5694 } 5695 5696 @Override 5697 public Base addChild(String name) throws FHIRException { 5698 if (name.equals("code")) { 5699 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.code"); 5700 } else if (name.equals("documentation")) { 5701 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 5702 } else 5703 return super.addChild(name); 5704 } 5705 5706 public ResourceInteractionComponent copy() { 5707 ResourceInteractionComponent dst = new ResourceInteractionComponent(); 5708 copyValues(dst); 5709 return dst; 5710 } 5711 5712 public void copyValues(ResourceInteractionComponent dst) { 5713 super.copyValues(dst); 5714 dst.code = code == null ? null : code.copy(); 5715 dst.documentation = documentation == null ? null : documentation.copy(); 5716 } 5717 5718 @Override 5719 public boolean equalsDeep(Base other_) { 5720 if (!super.equalsDeep(other_)) 5721 return false; 5722 if (!(other_ instanceof ResourceInteractionComponent)) 5723 return false; 5724 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 5725 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5726 } 5727 5728 @Override 5729 public boolean equalsShallow(Base other_) { 5730 if (!super.equalsShallow(other_)) 5731 return false; 5732 if (!(other_ instanceof ResourceInteractionComponent)) 5733 return false; 5734 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 5735 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5736 } 5737 5738 public boolean isEmpty() { 5739 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 5740 } 5741 5742 public String fhirType() { 5743 return "CapabilityStatement.rest.resource.interaction"; 5744 5745 } 5746 5747 } 5748 5749 @Block() 5750 public static class CapabilityStatementRestResourceSearchParamComponent extends BackboneElement 5751 implements IBaseBackboneElement { 5752 /** 5753 * The name of the search parameter used in the interface. 5754 */ 5755 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5756 @Description(shortDefinition = "Name of search parameter", formalDefinition = "The name of the search parameter used in the interface.") 5757 protected StringType name; 5758 5759 /** 5760 * An absolute URI that is a formal reference to where this parameter was first 5761 * defined, so that a client can be confident of the meaning of the search 5762 * parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be 5763 * populated if the search parameter refers to a SearchParameter defined by the 5764 * FHIR core specification or externally defined IGs. 5765 */ 5766 @Child(name = "definition", type = { 5767 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5768 @Description(shortDefinition = "Source of definition for parameter", formalDefinition = "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.") 5769 protected CanonicalType definition; 5770 5771 /** 5772 * The type of value a search parameter refers to, and how the content is 5773 * interpreted. 5774 */ 5775 @Child(name = "type", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 5776 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri | special", formalDefinition = "The type of value a search parameter refers to, and how the content is interpreted.") 5777 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-param-type") 5778 protected Enumeration<SearchParamType> type; 5779 5780 /** 5781 * This allows documentation of any distinct behaviors about how the search 5782 * parameter is used. For example, text matching algorithms. 5783 */ 5784 @Child(name = "documentation", type = { 5785 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5786 @Description(shortDefinition = "Server-specific usage", formalDefinition = "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.") 5787 protected MarkdownType documentation; 5788 5789 private static final long serialVersionUID = -171123928L; 5790 5791 /** 5792 * Constructor 5793 */ 5794 public CapabilityStatementRestResourceSearchParamComponent() { 5795 super(); 5796 } 5797 5798 /** 5799 * Constructor 5800 */ 5801 public CapabilityStatementRestResourceSearchParamComponent(StringType name, Enumeration<SearchParamType> type) { 5802 super(); 5803 this.name = name; 5804 this.type = type; 5805 } 5806 5807 /** 5808 * @return {@link #name} (The name of the search parameter used in the 5809 * interface.). This is the underlying object with id, value and 5810 * extensions. The accessor "getName" gives direct access to the value 5811 */ 5812 public StringType getNameElement() { 5813 if (this.name == null) 5814 if (Configuration.errorOnAutoCreate()) 5815 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.name"); 5816 else if (Configuration.doAutoCreate()) 5817 this.name = new StringType(); // bb 5818 return this.name; 5819 } 5820 5821 public boolean hasNameElement() { 5822 return this.name != null && !this.name.isEmpty(); 5823 } 5824 5825 public boolean hasName() { 5826 return this.name != null && !this.name.isEmpty(); 5827 } 5828 5829 /** 5830 * @param value {@link #name} (The name of the search parameter used in the 5831 * interface.). This is the underlying object with id, value and 5832 * extensions. The accessor "getName" gives direct access to the 5833 * value 5834 */ 5835 public CapabilityStatementRestResourceSearchParamComponent setNameElement(StringType value) { 5836 this.name = value; 5837 return this; 5838 } 5839 5840 /** 5841 * @return The name of the search parameter used in the interface. 5842 */ 5843 public String getName() { 5844 return this.name == null ? null : this.name.getValue(); 5845 } 5846 5847 /** 5848 * @param value The name of the search parameter used in the interface. 5849 */ 5850 public CapabilityStatementRestResourceSearchParamComponent setName(String value) { 5851 if (this.name == null) 5852 this.name = new StringType(); 5853 this.name.setValue(value); 5854 return this; 5855 } 5856 5857 /** 5858 * @return {@link #definition} (An absolute URI that is a formal reference to 5859 * where this parameter was first defined, so that a client can be 5860 * confident of the meaning of the search parameter (a reference to 5861 * [[[SearchParameter.url]]]). This element SHALL be populated if the 5862 * search parameter refers to a SearchParameter defined by the FHIR core 5863 * specification or externally defined IGs.). This is the underlying 5864 * object with id, value and extensions. The accessor "getDefinition" 5865 * gives direct access to the value 5866 */ 5867 public CanonicalType getDefinitionElement() { 5868 if (this.definition == null) 5869 if (Configuration.errorOnAutoCreate()) 5870 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.definition"); 5871 else if (Configuration.doAutoCreate()) 5872 this.definition = new CanonicalType(); // bb 5873 return this.definition; 5874 } 5875 5876 public boolean hasDefinitionElement() { 5877 return this.definition != null && !this.definition.isEmpty(); 5878 } 5879 5880 public boolean hasDefinition() { 5881 return this.definition != null && !this.definition.isEmpty(); 5882 } 5883 5884 /** 5885 * @param value {@link #definition} (An absolute URI that is a formal reference 5886 * to where this parameter was first defined, so that a client can 5887 * be confident of the meaning of the search parameter (a reference 5888 * to [[[SearchParameter.url]]]). This element SHALL be populated 5889 * if the search parameter refers to a SearchParameter defined by 5890 * the FHIR core specification or externally defined IGs.). This is 5891 * the underlying object with id, value and extensions. The 5892 * accessor "getDefinition" gives direct access to the value 5893 */ 5894 public CapabilityStatementRestResourceSearchParamComponent setDefinitionElement(CanonicalType value) { 5895 this.definition = value; 5896 return this; 5897 } 5898 5899 /** 5900 * @return An absolute URI that is a formal reference to where this parameter 5901 * was first defined, so that a client can be confident of the meaning 5902 * of the search parameter (a reference to [[[SearchParameter.url]]]). 5903 * This element SHALL be populated if the search parameter refers to a 5904 * SearchParameter defined by the FHIR core specification or externally 5905 * defined IGs. 5906 */ 5907 public String getDefinition() { 5908 return this.definition == null ? null : this.definition.getValue(); 5909 } 5910 5911 /** 5912 * @param value An absolute URI that is a formal reference to where this 5913 * parameter was first defined, so that a client can be confident 5914 * of the meaning of the search parameter (a reference to 5915 * [[[SearchParameter.url]]]). This element SHALL be populated if 5916 * the search parameter refers to a SearchParameter defined by the 5917 * FHIR core specification or externally defined IGs. 5918 */ 5919 public CapabilityStatementRestResourceSearchParamComponent setDefinition(String value) { 5920 if (Utilities.noString(value)) 5921 this.definition = null; 5922 else { 5923 if (this.definition == null) 5924 this.definition = new CanonicalType(); 5925 this.definition.setValue(value); 5926 } 5927 return this; 5928 } 5929 5930 /** 5931 * @return {@link #type} (The type of value a search parameter refers to, and 5932 * how the content is interpreted.). This is the underlying object with 5933 * id, value and extensions. The accessor "getType" gives direct access 5934 * to the value 5935 */ 5936 public Enumeration<SearchParamType> getTypeElement() { 5937 if (this.type == null) 5938 if (Configuration.errorOnAutoCreate()) 5939 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.type"); 5940 else if (Configuration.doAutoCreate()) 5941 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 5942 return this.type; 5943 } 5944 5945 public boolean hasTypeElement() { 5946 return this.type != null && !this.type.isEmpty(); 5947 } 5948 5949 public boolean hasType() { 5950 return this.type != null && !this.type.isEmpty(); 5951 } 5952 5953 /** 5954 * @param value {@link #type} (The type of value a search parameter refers to, 5955 * and how the content is interpreted.). This is the underlying 5956 * object with id, value and extensions. The accessor "getType" 5957 * gives direct access to the value 5958 */ 5959 public CapabilityStatementRestResourceSearchParamComponent setTypeElement(Enumeration<SearchParamType> value) { 5960 this.type = value; 5961 return this; 5962 } 5963 5964 /** 5965 * @return The type of value a search parameter refers to, and how the content 5966 * is interpreted. 5967 */ 5968 public SearchParamType getType() { 5969 return this.type == null ? null : this.type.getValue(); 5970 } 5971 5972 /** 5973 * @param value The type of value a search parameter refers to, and how the 5974 * content is interpreted. 5975 */ 5976 public CapabilityStatementRestResourceSearchParamComponent setType(SearchParamType value) { 5977 if (this.type == null) 5978 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 5979 this.type.setValue(value); 5980 return this; 5981 } 5982 5983 /** 5984 * @return {@link #documentation} (This allows documentation of any distinct 5985 * behaviors about how the search parameter is used. For example, text 5986 * matching algorithms.). This is the underlying object with id, value 5987 * and extensions. The accessor "getDocumentation" gives direct access 5988 * to the value 5989 */ 5990 public MarkdownType getDocumentationElement() { 5991 if (this.documentation == null) 5992 if (Configuration.errorOnAutoCreate()) 5993 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.documentation"); 5994 else if (Configuration.doAutoCreate()) 5995 this.documentation = new MarkdownType(); // bb 5996 return this.documentation; 5997 } 5998 5999 public boolean hasDocumentationElement() { 6000 return this.documentation != null && !this.documentation.isEmpty(); 6001 } 6002 6003 public boolean hasDocumentation() { 6004 return this.documentation != null && !this.documentation.isEmpty(); 6005 } 6006 6007 /** 6008 * @param value {@link #documentation} (This allows documentation of any 6009 * distinct behaviors about how the search parameter is used. For 6010 * example, text matching algorithms.). This is the underlying 6011 * object with id, value and extensions. The accessor 6012 * "getDocumentation" gives direct access to the value 6013 */ 6014 public CapabilityStatementRestResourceSearchParamComponent setDocumentationElement(MarkdownType value) { 6015 this.documentation = value; 6016 return this; 6017 } 6018 6019 /** 6020 * @return This allows documentation of any distinct behaviors about how the 6021 * search parameter is used. For example, text matching algorithms. 6022 */ 6023 public String getDocumentation() { 6024 return this.documentation == null ? null : this.documentation.getValue(); 6025 } 6026 6027 /** 6028 * @param value This allows documentation of any distinct behaviors about how 6029 * the search parameter is used. For example, text matching 6030 * algorithms. 6031 */ 6032 public CapabilityStatementRestResourceSearchParamComponent setDocumentation(String value) { 6033 if (value == null) 6034 this.documentation = null; 6035 else { 6036 if (this.documentation == null) 6037 this.documentation = new MarkdownType(); 6038 this.documentation.setValue(value); 6039 } 6040 return this; 6041 } 6042 6043 protected void listChildren(List<Property> children) { 6044 super.listChildren(children); 6045 children 6046 .add(new Property("name", "string", "The name of the search parameter used in the interface.", 0, 1, name)); 6047 children.add(new Property("definition", "canonical(SearchParameter)", 6048 "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.", 6049 0, 1, definition)); 6050 children.add(new Property("type", "code", 6051 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type)); 6052 children.add(new Property("documentation", "markdown", 6053 "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 6054 0, 1, documentation)); 6055 } 6056 6057 @Override 6058 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6059 switch (_hash) { 6060 case 3373707: 6061 /* name */ return new Property("name", "string", "The name of the search parameter used in the interface.", 0, 6062 1, name); 6063 case -1014418093: 6064 /* definition */ return new Property("definition", "canonical(SearchParameter)", 6065 "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.", 6066 0, 1, definition); 6067 case 3575610: 6068 /* type */ return new Property("type", "code", 6069 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type); 6070 case 1587405498: 6071 /* documentation */ return new Property("documentation", "markdown", 6072 "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 6073 0, 1, documentation); 6074 default: 6075 return super.getNamedProperty(_hash, _name, _checkValid); 6076 } 6077 6078 } 6079 6080 @Override 6081 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6082 switch (hash) { 6083 case 3373707: 6084 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 6085 case -1014418093: 6086 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 6087 case 3575610: 6088 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SearchParamType> 6089 case 1587405498: 6090 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 6091 default: 6092 return super.getProperty(hash, name, checkValid); 6093 } 6094 6095 } 6096 6097 @Override 6098 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6099 switch (hash) { 6100 case 3373707: // name 6101 this.name = castToString(value); // StringType 6102 return value; 6103 case -1014418093: // definition 6104 this.definition = castToCanonical(value); // CanonicalType 6105 return value; 6106 case 3575610: // type 6107 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 6108 this.type = (Enumeration) value; // Enumeration<SearchParamType> 6109 return value; 6110 case 1587405498: // documentation 6111 this.documentation = castToMarkdown(value); // MarkdownType 6112 return value; 6113 default: 6114 return super.setProperty(hash, name, value); 6115 } 6116 6117 } 6118 6119 @Override 6120 public Base setProperty(String name, Base value) throws FHIRException { 6121 if (name.equals("name")) { 6122 this.name = castToString(value); // StringType 6123 } else if (name.equals("definition")) { 6124 this.definition = castToCanonical(value); // CanonicalType 6125 } else if (name.equals("type")) { 6126 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 6127 this.type = (Enumeration) value; // Enumeration<SearchParamType> 6128 } else if (name.equals("documentation")) { 6129 this.documentation = castToMarkdown(value); // MarkdownType 6130 } else 6131 return super.setProperty(name, value); 6132 return value; 6133 } 6134 6135 @Override 6136 public void removeChild(String name, Base value) throws FHIRException { 6137 if (name.equals("name")) { 6138 this.name = null; 6139 } else if (name.equals("definition")) { 6140 this.definition = null; 6141 } else if (name.equals("type")) { 6142 this.type = null; 6143 } else if (name.equals("documentation")) { 6144 this.documentation = null; 6145 } else 6146 super.removeChild(name, value); 6147 6148 } 6149 6150 @Override 6151 public Base makeProperty(int hash, String name) throws FHIRException { 6152 switch (hash) { 6153 case 3373707: 6154 return getNameElement(); 6155 case -1014418093: 6156 return getDefinitionElement(); 6157 case 3575610: 6158 return getTypeElement(); 6159 case 1587405498: 6160 return getDocumentationElement(); 6161 default: 6162 return super.makeProperty(hash, name); 6163 } 6164 6165 } 6166 6167 @Override 6168 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6169 switch (hash) { 6170 case 3373707: 6171 /* name */ return new String[] { "string" }; 6172 case -1014418093: 6173 /* definition */ return new String[] { "canonical" }; 6174 case 3575610: 6175 /* type */ return new String[] { "code" }; 6176 case 1587405498: 6177 /* documentation */ return new String[] { "markdown" }; 6178 default: 6179 return super.getTypesForProperty(hash, name); 6180 } 6181 6182 } 6183 6184 @Override 6185 public Base addChild(String name) throws FHIRException { 6186 if (name.equals("name")) { 6187 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 6188 } else if (name.equals("definition")) { 6189 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 6190 } else if (name.equals("type")) { 6191 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 6192 } else if (name.equals("documentation")) { 6193 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6194 } else 6195 return super.addChild(name); 6196 } 6197 6198 public CapabilityStatementRestResourceSearchParamComponent copy() { 6199 CapabilityStatementRestResourceSearchParamComponent dst = new CapabilityStatementRestResourceSearchParamComponent(); 6200 copyValues(dst); 6201 return dst; 6202 } 6203 6204 public void copyValues(CapabilityStatementRestResourceSearchParamComponent dst) { 6205 super.copyValues(dst); 6206 dst.name = name == null ? null : name.copy(); 6207 dst.definition = definition == null ? null : definition.copy(); 6208 dst.type = type == null ? null : type.copy(); 6209 dst.documentation = documentation == null ? null : documentation.copy(); 6210 } 6211 6212 @Override 6213 public boolean equalsDeep(Base other_) { 6214 if (!super.equalsDeep(other_)) 6215 return false; 6216 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 6217 return false; 6218 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 6219 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) 6220 && compareDeep(type, o.type, true) && compareDeep(documentation, o.documentation, true); 6221 } 6222 6223 @Override 6224 public boolean equalsShallow(Base other_) { 6225 if (!super.equalsShallow(other_)) 6226 return false; 6227 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 6228 return false; 6229 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 6230 return compareValues(name, o.name, true) && compareValues(type, o.type, true) 6231 && compareValues(documentation, o.documentation, true); 6232 } 6233 6234 public boolean isEmpty() { 6235 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, type, documentation); 6236 } 6237 6238 public String fhirType() { 6239 return "CapabilityStatement.rest.resource.searchParam"; 6240 6241 } 6242 6243 } 6244 6245 @Block() 6246 public static class CapabilityStatementRestResourceOperationComponent extends BackboneElement 6247 implements IBaseBackboneElement { 6248 /** 6249 * The name of the operation or query. For an operation, this is the name 6250 * prefixed with $ and used in the URL. For a query, this is the name used in 6251 * the _query parameter when the query is called. 6252 */ 6253 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 6254 @Description(shortDefinition = "Name by which the operation/query is invoked", formalDefinition = "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.") 6255 protected StringType name; 6256 6257 /** 6258 * Where the formal definition can be found. If a server references the base 6259 * definition of an Operation (i.e. from the specification itself such as 6260 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it 6261 * supports the full capabilities of the operation - e.g. both GET and POST 6262 * invocation. If it only supports a subset, it must define its own custom 6263 * [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. 6264 * The custom definition would describe the specific subset of functionality 6265 * supported. 6266 */ 6267 @Child(name = "definition", type = { 6268 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 6269 @Description(shortDefinition = "The defined operation/query", formalDefinition = "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.") 6270 protected CanonicalType definition; 6271 6272 /** 6273 * Documentation that describes anything special about the operation behavior, 6274 * possibly detailing different behavior for system, type and instance-level 6275 * invocation of the operation. 6276 */ 6277 @Child(name = "documentation", type = { 6278 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6279 @Description(shortDefinition = "Specific details about operation behavior", formalDefinition = "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.") 6280 protected MarkdownType documentation; 6281 6282 private static final long serialVersionUID = -388608084L; 6283 6284 /** 6285 * Constructor 6286 */ 6287 public CapabilityStatementRestResourceOperationComponent() { 6288 super(); 6289 } 6290 6291 /** 6292 * Constructor 6293 */ 6294 public CapabilityStatementRestResourceOperationComponent(StringType name, CanonicalType definition) { 6295 super(); 6296 this.name = name; 6297 this.definition = definition; 6298 } 6299 6300 /** 6301 * @return {@link #name} (The name of the operation or query. For an operation, 6302 * this is the name prefixed with $ and used in the URL. For a query, 6303 * this is the name used in the _query parameter when the query is 6304 * called.). This is the underlying object with id, value and 6305 * extensions. The accessor "getName" gives direct access to the value 6306 */ 6307 public StringType getNameElement() { 6308 if (this.name == null) 6309 if (Configuration.errorOnAutoCreate()) 6310 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.name"); 6311 else if (Configuration.doAutoCreate()) 6312 this.name = new StringType(); // bb 6313 return this.name; 6314 } 6315 6316 public boolean hasNameElement() { 6317 return this.name != null && !this.name.isEmpty(); 6318 } 6319 6320 public boolean hasName() { 6321 return this.name != null && !this.name.isEmpty(); 6322 } 6323 6324 /** 6325 * @param value {@link #name} (The name of the operation or query. For an 6326 * operation, this is the name prefixed with $ and used in the URL. 6327 * For a query, this is the name used in the _query parameter when 6328 * the query is called.). This is the underlying object with id, 6329 * value and extensions. The accessor "getName" gives direct access 6330 * to the value 6331 */ 6332 public CapabilityStatementRestResourceOperationComponent setNameElement(StringType value) { 6333 this.name = value; 6334 return this; 6335 } 6336 6337 /** 6338 * @return The name of the operation or query. For an operation, this is the 6339 * name prefixed with $ and used in the URL. For a query, this is the 6340 * name used in the _query parameter when the query is called. 6341 */ 6342 public String getName() { 6343 return this.name == null ? null : this.name.getValue(); 6344 } 6345 6346 /** 6347 * @param value The name of the operation or query. For an operation, this is 6348 * the name prefixed with $ and used in the URL. For a query, this 6349 * is the name used in the _query parameter when the query is 6350 * called. 6351 */ 6352 public CapabilityStatementRestResourceOperationComponent setName(String value) { 6353 if (this.name == null) 6354 this.name = new StringType(); 6355 this.name.setValue(value); 6356 return this; 6357 } 6358 6359 /** 6360 * @return {@link #definition} (Where the formal definition can be found. If a 6361 * server references the base definition of an Operation (i.e. from the 6362 * specification itself such as 6363 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that 6364 * means it supports the full capabilities of the operation - e.g. both 6365 * GET and POST invocation. If it only supports a subset, it must define 6366 * its own custom [[[OperationDefinition]]] with a 'base' of the 6367 * original OperationDefinition. The custom definition would describe 6368 * the specific subset of functionality supported.). This is the 6369 * underlying object with id, value and extensions. The accessor 6370 * "getDefinition" gives direct access to the value 6371 */ 6372 public CanonicalType getDefinitionElement() { 6373 if (this.definition == null) 6374 if (Configuration.errorOnAutoCreate()) 6375 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.definition"); 6376 else if (Configuration.doAutoCreate()) 6377 this.definition = new CanonicalType(); // bb 6378 return this.definition; 6379 } 6380 6381 public boolean hasDefinitionElement() { 6382 return this.definition != null && !this.definition.isEmpty(); 6383 } 6384 6385 public boolean hasDefinition() { 6386 return this.definition != null && !this.definition.isEmpty(); 6387 } 6388 6389 /** 6390 * @param value {@link #definition} (Where the formal definition can be found. 6391 * If a server references the base definition of an Operation (i.e. 6392 * from the specification itself such as 6393 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), 6394 * that means it supports the full capabilities of the operation - 6395 * e.g. both GET and POST invocation. If it only supports a subset, 6396 * it must define its own custom [[[OperationDefinition]]] with a 6397 * 'base' of the original OperationDefinition. The custom 6398 * definition would describe the specific subset of functionality 6399 * supported.). This is the underlying object with id, value and 6400 * extensions. The accessor "getDefinition" gives direct access to 6401 * the value 6402 */ 6403 public CapabilityStatementRestResourceOperationComponent setDefinitionElement(CanonicalType value) { 6404 this.definition = value; 6405 return this; 6406 } 6407 6408 /** 6409 * @return Where the formal definition can be found. If a server references the 6410 * base definition of an Operation (i.e. from the specification itself 6411 * such as 6412 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that 6413 * means it supports the full capabilities of the operation - e.g. both 6414 * GET and POST invocation. If it only supports a subset, it must define 6415 * its own custom [[[OperationDefinition]]] with a 'base' of the 6416 * original OperationDefinition. The custom definition would describe 6417 * the specific subset of functionality supported. 6418 */ 6419 public String getDefinition() { 6420 return this.definition == null ? null : this.definition.getValue(); 6421 } 6422 6423 /** 6424 * @param value Where the formal definition can be found. If a server references 6425 * the base definition of an Operation (i.e. from the specification 6426 * itself such as 6427 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), 6428 * that means it supports the full capabilities of the operation - 6429 * e.g. both GET and POST invocation. If it only supports a subset, 6430 * it must define its own custom [[[OperationDefinition]]] with a 6431 * 'base' of the original OperationDefinition. The custom 6432 * definition would describe the specific subset of functionality 6433 * supported. 6434 */ 6435 public CapabilityStatementRestResourceOperationComponent setDefinition(String value) { 6436 if (this.definition == null) 6437 this.definition = new CanonicalType(); 6438 this.definition.setValue(value); 6439 return this; 6440 } 6441 6442 /** 6443 * @return {@link #documentation} (Documentation that describes anything special 6444 * about the operation behavior, possibly detailing different behavior 6445 * for system, type and instance-level invocation of the operation.). 6446 * This is the underlying object with id, value and extensions. The 6447 * accessor "getDocumentation" gives direct access to the value 6448 */ 6449 public MarkdownType getDocumentationElement() { 6450 if (this.documentation == null) 6451 if (Configuration.errorOnAutoCreate()) 6452 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.documentation"); 6453 else if (Configuration.doAutoCreate()) 6454 this.documentation = new MarkdownType(); // bb 6455 return this.documentation; 6456 } 6457 6458 public boolean hasDocumentationElement() { 6459 return this.documentation != null && !this.documentation.isEmpty(); 6460 } 6461 6462 public boolean hasDocumentation() { 6463 return this.documentation != null && !this.documentation.isEmpty(); 6464 } 6465 6466 /** 6467 * @param value {@link #documentation} (Documentation that describes anything 6468 * special about the operation behavior, possibly detailing 6469 * different behavior for system, type and instance-level 6470 * invocation of the operation.). This is the underlying object 6471 * with id, value and extensions. The accessor "getDocumentation" 6472 * gives direct access to the value 6473 */ 6474 public CapabilityStatementRestResourceOperationComponent setDocumentationElement(MarkdownType value) { 6475 this.documentation = value; 6476 return this; 6477 } 6478 6479 /** 6480 * @return Documentation that describes anything special about the operation 6481 * behavior, possibly detailing different behavior for system, type and 6482 * instance-level invocation of the operation. 6483 */ 6484 public String getDocumentation() { 6485 return this.documentation == null ? null : this.documentation.getValue(); 6486 } 6487 6488 /** 6489 * @param value Documentation that describes anything special about the 6490 * operation behavior, possibly detailing different behavior for 6491 * system, type and instance-level invocation of the operation. 6492 */ 6493 public CapabilityStatementRestResourceOperationComponent setDocumentation(String value) { 6494 if (value == null) 6495 this.documentation = null; 6496 else { 6497 if (this.documentation == null) 6498 this.documentation = new MarkdownType(); 6499 this.documentation.setValue(value); 6500 } 6501 return this; 6502 } 6503 6504 protected void listChildren(List<Property> children) { 6505 super.listChildren(children); 6506 children.add(new Property("name", "string", 6507 "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.", 6508 0, 1, name)); 6509 children.add(new Property("definition", "canonical(OperationDefinition)", 6510 "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.", 6511 0, 1, definition)); 6512 children.add(new Property("documentation", "markdown", 6513 "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.", 6514 0, 1, documentation)); 6515 } 6516 6517 @Override 6518 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6519 switch (_hash) { 6520 case 3373707: 6521 /* name */ return new Property("name", "string", 6522 "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.", 6523 0, 1, name); 6524 case -1014418093: 6525 /* definition */ return new Property("definition", "canonical(OperationDefinition)", 6526 "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.", 6527 0, 1, definition); 6528 case 1587405498: 6529 /* documentation */ return new Property("documentation", "markdown", 6530 "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.", 6531 0, 1, documentation); 6532 default: 6533 return super.getNamedProperty(_hash, _name, _checkValid); 6534 } 6535 6536 } 6537 6538 @Override 6539 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6540 switch (hash) { 6541 case 3373707: 6542 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 6543 case -1014418093: 6544 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 6545 case 1587405498: 6546 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 6547 default: 6548 return super.getProperty(hash, name, checkValid); 6549 } 6550 6551 } 6552 6553 @Override 6554 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6555 switch (hash) { 6556 case 3373707: // name 6557 this.name = castToString(value); // StringType 6558 return value; 6559 case -1014418093: // definition 6560 this.definition = castToCanonical(value); // CanonicalType 6561 return value; 6562 case 1587405498: // documentation 6563 this.documentation = castToMarkdown(value); // MarkdownType 6564 return value; 6565 default: 6566 return super.setProperty(hash, name, value); 6567 } 6568 6569 } 6570 6571 @Override 6572 public Base setProperty(String name, Base value) throws FHIRException { 6573 if (name.equals("name")) { 6574 this.name = castToString(value); // StringType 6575 } else if (name.equals("definition")) { 6576 this.definition = castToCanonical(value); // CanonicalType 6577 } else if (name.equals("documentation")) { 6578 this.documentation = castToMarkdown(value); // MarkdownType 6579 } else 6580 return super.setProperty(name, value); 6581 return value; 6582 } 6583 6584 @Override 6585 public void removeChild(String name, Base value) throws FHIRException { 6586 if (name.equals("name")) { 6587 this.name = null; 6588 } else if (name.equals("definition")) { 6589 this.definition = null; 6590 } else if (name.equals("documentation")) { 6591 this.documentation = null; 6592 } else 6593 super.removeChild(name, value); 6594 6595 } 6596 6597 @Override 6598 public Base makeProperty(int hash, String name) throws FHIRException { 6599 switch (hash) { 6600 case 3373707: 6601 return getNameElement(); 6602 case -1014418093: 6603 return getDefinitionElement(); 6604 case 1587405498: 6605 return getDocumentationElement(); 6606 default: 6607 return super.makeProperty(hash, name); 6608 } 6609 6610 } 6611 6612 @Override 6613 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6614 switch (hash) { 6615 case 3373707: 6616 /* name */ return new String[] { "string" }; 6617 case -1014418093: 6618 /* definition */ return new String[] { "canonical" }; 6619 case 1587405498: 6620 /* documentation */ return new String[] { "markdown" }; 6621 default: 6622 return super.getTypesForProperty(hash, name); 6623 } 6624 6625 } 6626 6627 @Override 6628 public Base addChild(String name) throws FHIRException { 6629 if (name.equals("name")) { 6630 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 6631 } else if (name.equals("definition")) { 6632 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 6633 } else if (name.equals("documentation")) { 6634 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6635 } else 6636 return super.addChild(name); 6637 } 6638 6639 public CapabilityStatementRestResourceOperationComponent copy() { 6640 CapabilityStatementRestResourceOperationComponent dst = new CapabilityStatementRestResourceOperationComponent(); 6641 copyValues(dst); 6642 return dst; 6643 } 6644 6645 public void copyValues(CapabilityStatementRestResourceOperationComponent dst) { 6646 super.copyValues(dst); 6647 dst.name = name == null ? null : name.copy(); 6648 dst.definition = definition == null ? null : definition.copy(); 6649 dst.documentation = documentation == null ? null : documentation.copy(); 6650 } 6651 6652 @Override 6653 public boolean equalsDeep(Base other_) { 6654 if (!super.equalsDeep(other_)) 6655 return false; 6656 if (!(other_ instanceof CapabilityStatementRestResourceOperationComponent)) 6657 return false; 6658 CapabilityStatementRestResourceOperationComponent o = (CapabilityStatementRestResourceOperationComponent) other_; 6659 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) 6660 && compareDeep(documentation, o.documentation, true); 6661 } 6662 6663 @Override 6664 public boolean equalsShallow(Base other_) { 6665 if (!super.equalsShallow(other_)) 6666 return false; 6667 if (!(other_ instanceof CapabilityStatementRestResourceOperationComponent)) 6668 return false; 6669 CapabilityStatementRestResourceOperationComponent o = (CapabilityStatementRestResourceOperationComponent) other_; 6670 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 6671 } 6672 6673 public boolean isEmpty() { 6674 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, documentation); 6675 } 6676 6677 public String fhirType() { 6678 return "CapabilityStatement.rest.resource.operation"; 6679 6680 } 6681 6682 } 6683 6684 @Block() 6685 public static class SystemInteractionComponent extends BackboneElement implements IBaseBackboneElement { 6686 /** 6687 * A coded identifier of the operation, supported by the system. 6688 */ 6689 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6690 @Description(shortDefinition = "transaction | batch | search-system | history-system", formalDefinition = "A coded identifier of the operation, supported by the system.") 6691 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/system-restful-interaction") 6692 protected Enumeration<SystemRestfulInteraction> code; 6693 6694 /** 6695 * Guidance specific to the implementation of this operation, such as 6696 * limitations on the kind of transactions allowed, or information about system 6697 * wide search is implemented. 6698 */ 6699 @Child(name = "documentation", type = { 6700 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6701 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.") 6702 protected MarkdownType documentation; 6703 6704 private static final long serialVersionUID = -1495143879L; 6705 6706 /** 6707 * Constructor 6708 */ 6709 public SystemInteractionComponent() { 6710 super(); 6711 } 6712 6713 /** 6714 * Constructor 6715 */ 6716 public SystemInteractionComponent(Enumeration<SystemRestfulInteraction> code) { 6717 super(); 6718 this.code = code; 6719 } 6720 6721 /** 6722 * @return {@link #code} (A coded identifier of the operation, supported by the 6723 * system.). This is the underlying object with id, value and 6724 * extensions. The accessor "getCode" gives direct access to the value 6725 */ 6726 public Enumeration<SystemRestfulInteraction> getCodeElement() { 6727 if (this.code == null) 6728 if (Configuration.errorOnAutoCreate()) 6729 throw new Error("Attempt to auto-create SystemInteractionComponent.code"); 6730 else if (Configuration.doAutoCreate()) 6731 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); // bb 6732 return this.code; 6733 } 6734 6735 public boolean hasCodeElement() { 6736 return this.code != null && !this.code.isEmpty(); 6737 } 6738 6739 public boolean hasCode() { 6740 return this.code != null && !this.code.isEmpty(); 6741 } 6742 6743 /** 6744 * @param value {@link #code} (A coded identifier of the operation, supported by 6745 * the system.). This is the underlying object with id, value and 6746 * extensions. The accessor "getCode" gives direct access to the 6747 * value 6748 */ 6749 public SystemInteractionComponent setCodeElement(Enumeration<SystemRestfulInteraction> value) { 6750 this.code = value; 6751 return this; 6752 } 6753 6754 /** 6755 * @return A coded identifier of the operation, supported by the system. 6756 */ 6757 public SystemRestfulInteraction getCode() { 6758 return this.code == null ? null : this.code.getValue(); 6759 } 6760 6761 /** 6762 * @param value A coded identifier of the operation, supported by the system. 6763 */ 6764 public SystemInteractionComponent setCode(SystemRestfulInteraction value) { 6765 if (this.code == null) 6766 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); 6767 this.code.setValue(value); 6768 return this; 6769 } 6770 6771 /** 6772 * @return {@link #documentation} (Guidance specific to the implementation of 6773 * this operation, such as limitations on the kind of transactions 6774 * allowed, or information about system wide search is implemented.). 6775 * This is the underlying object with id, value and extensions. The 6776 * accessor "getDocumentation" gives direct access to the value 6777 */ 6778 public MarkdownType getDocumentationElement() { 6779 if (this.documentation == null) 6780 if (Configuration.errorOnAutoCreate()) 6781 throw new Error("Attempt to auto-create SystemInteractionComponent.documentation"); 6782 else if (Configuration.doAutoCreate()) 6783 this.documentation = new MarkdownType(); // bb 6784 return this.documentation; 6785 } 6786 6787 public boolean hasDocumentationElement() { 6788 return this.documentation != null && !this.documentation.isEmpty(); 6789 } 6790 6791 public boolean hasDocumentation() { 6792 return this.documentation != null && !this.documentation.isEmpty(); 6793 } 6794 6795 /** 6796 * @param value {@link #documentation} (Guidance specific to the implementation 6797 * of this operation, such as limitations on the kind of 6798 * transactions allowed, or information about system wide search is 6799 * implemented.). This is the underlying object with id, value and 6800 * extensions. The accessor "getDocumentation" gives direct access 6801 * to the value 6802 */ 6803 public SystemInteractionComponent setDocumentationElement(MarkdownType value) { 6804 this.documentation = value; 6805 return this; 6806 } 6807 6808 /** 6809 * @return Guidance specific to the implementation of this operation, such as 6810 * limitations on the kind of transactions allowed, or information about 6811 * system wide search is implemented. 6812 */ 6813 public String getDocumentation() { 6814 return this.documentation == null ? null : this.documentation.getValue(); 6815 } 6816 6817 /** 6818 * @param value Guidance specific to the implementation of this operation, such 6819 * as limitations on the kind of transactions allowed, or 6820 * information about system wide search is implemented. 6821 */ 6822 public SystemInteractionComponent setDocumentation(String value) { 6823 if (value == null) 6824 this.documentation = null; 6825 else { 6826 if (this.documentation == null) 6827 this.documentation = new MarkdownType(); 6828 this.documentation.setValue(value); 6829 } 6830 return this; 6831 } 6832 6833 protected void listChildren(List<Property> children) { 6834 super.listChildren(children); 6835 children.add( 6836 new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 1, code)); 6837 children.add(new Property("documentation", "markdown", 6838 "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 6839 0, 1, documentation)); 6840 } 6841 6842 @Override 6843 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6844 switch (_hash) { 6845 case 3059181: 6846 /* code */ return new Property("code", "code", "A coded identifier of the operation, supported by the system.", 6847 0, 1, code); 6848 case 1587405498: 6849 /* documentation */ return new Property("documentation", "markdown", 6850 "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 6851 0, 1, documentation); 6852 default: 6853 return super.getNamedProperty(_hash, _name, _checkValid); 6854 } 6855 6856 } 6857 6858 @Override 6859 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6860 switch (hash) { 6861 case 3059181: 6862 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<SystemRestfulInteraction> 6863 case 1587405498: 6864 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 6865 default: 6866 return super.getProperty(hash, name, checkValid); 6867 } 6868 6869 } 6870 6871 @Override 6872 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6873 switch (hash) { 6874 case 3059181: // code 6875 value = new SystemRestfulInteractionEnumFactory().fromType(castToCode(value)); 6876 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 6877 return value; 6878 case 1587405498: // documentation 6879 this.documentation = castToMarkdown(value); // MarkdownType 6880 return value; 6881 default: 6882 return super.setProperty(hash, name, value); 6883 } 6884 6885 } 6886 6887 @Override 6888 public Base setProperty(String name, Base value) throws FHIRException { 6889 if (name.equals("code")) { 6890 value = new SystemRestfulInteractionEnumFactory().fromType(castToCode(value)); 6891 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 6892 } else if (name.equals("documentation")) { 6893 this.documentation = castToMarkdown(value); // MarkdownType 6894 } else 6895 return super.setProperty(name, value); 6896 return value; 6897 } 6898 6899 @Override 6900 public void removeChild(String name, Base value) throws FHIRException { 6901 if (name.equals("code")) { 6902 this.code = null; 6903 } else if (name.equals("documentation")) { 6904 this.documentation = null; 6905 } else 6906 super.removeChild(name, value); 6907 6908 } 6909 6910 @Override 6911 public Base makeProperty(int hash, String name) throws FHIRException { 6912 switch (hash) { 6913 case 3059181: 6914 return getCodeElement(); 6915 case 1587405498: 6916 return getDocumentationElement(); 6917 default: 6918 return super.makeProperty(hash, name); 6919 } 6920 6921 } 6922 6923 @Override 6924 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6925 switch (hash) { 6926 case 3059181: 6927 /* code */ return new String[] { "code" }; 6928 case 1587405498: 6929 /* documentation */ return new String[] { "markdown" }; 6930 default: 6931 return super.getTypesForProperty(hash, name); 6932 } 6933 6934 } 6935 6936 @Override 6937 public Base addChild(String name) throws FHIRException { 6938 if (name.equals("code")) { 6939 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.code"); 6940 } else if (name.equals("documentation")) { 6941 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6942 } else 6943 return super.addChild(name); 6944 } 6945 6946 public SystemInteractionComponent copy() { 6947 SystemInteractionComponent dst = new SystemInteractionComponent(); 6948 copyValues(dst); 6949 return dst; 6950 } 6951 6952 public void copyValues(SystemInteractionComponent dst) { 6953 super.copyValues(dst); 6954 dst.code = code == null ? null : code.copy(); 6955 dst.documentation = documentation == null ? null : documentation.copy(); 6956 } 6957 6958 @Override 6959 public boolean equalsDeep(Base other_) { 6960 if (!super.equalsDeep(other_)) 6961 return false; 6962 if (!(other_ instanceof SystemInteractionComponent)) 6963 return false; 6964 SystemInteractionComponent o = (SystemInteractionComponent) other_; 6965 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 6966 } 6967 6968 @Override 6969 public boolean equalsShallow(Base other_) { 6970 if (!super.equalsShallow(other_)) 6971 return false; 6972 if (!(other_ instanceof SystemInteractionComponent)) 6973 return false; 6974 SystemInteractionComponent o = (SystemInteractionComponent) other_; 6975 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 6976 } 6977 6978 public boolean isEmpty() { 6979 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 6980 } 6981 6982 public String fhirType() { 6983 return "CapabilityStatement.rest.interaction"; 6984 6985 } 6986 6987 } 6988 6989 @Block() 6990 public static class CapabilityStatementMessagingComponent extends BackboneElement implements IBaseBackboneElement { 6991 /** 6992 * An endpoint (network accessible address) to which messages and/or replies are 6993 * to be sent. 6994 */ 6995 @Child(name = "endpoint", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6996 @Description(shortDefinition = "Where messages should be sent", formalDefinition = "An endpoint (network accessible address) to which messages and/or replies are to be sent.") 6997 protected List<CapabilityStatementMessagingEndpointComponent> endpoint; 6998 6999 /** 7000 * Length if the receiver's reliable messaging cache in minutes (if a receiver) 7001 * or how long the cache length on the receiver should be (if a sender). 7002 */ 7003 @Child(name = "reliableCache", type = { 7004 UnsignedIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7005 @Description(shortDefinition = "Reliable Message Cache Length (min)", formalDefinition = "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).") 7006 protected UnsignedIntType reliableCache; 7007 7008 /** 7009 * Documentation about the system's messaging capabilities for this endpoint not 7010 * otherwise documented by the capability statement. For example, the process 7011 * for becoming an authorized messaging exchange partner. 7012 */ 7013 @Child(name = "documentation", type = { 7014 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 7015 @Description(shortDefinition = "Messaging interface behavior details", formalDefinition = "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.") 7016 protected MarkdownType documentation; 7017 7018 /** 7019 * References to message definitions for messages this system can send or 7020 * receive. 7021 */ 7022 @Child(name = "supportedMessage", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7023 @Description(shortDefinition = "Messages supported by this system", formalDefinition = "References to message definitions for messages this system can send or receive.") 7024 protected List<CapabilityStatementMessagingSupportedMessageComponent> supportedMessage; 7025 7026 private static final long serialVersionUID = 300411231L; 7027 7028 /** 7029 * Constructor 7030 */ 7031 public CapabilityStatementMessagingComponent() { 7032 super(); 7033 } 7034 7035 /** 7036 * @return {@link #endpoint} (An endpoint (network accessible address) to which 7037 * messages and/or replies are to be sent.) 7038 */ 7039 public List<CapabilityStatementMessagingEndpointComponent> getEndpoint() { 7040 if (this.endpoint == null) 7041 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7042 return this.endpoint; 7043 } 7044 7045 /** 7046 * @return Returns a reference to <code>this</code> for easy method chaining 7047 */ 7048 public CapabilityStatementMessagingComponent setEndpoint( 7049 List<CapabilityStatementMessagingEndpointComponent> theEndpoint) { 7050 this.endpoint = theEndpoint; 7051 return this; 7052 } 7053 7054 public boolean hasEndpoint() { 7055 if (this.endpoint == null) 7056 return false; 7057 for (CapabilityStatementMessagingEndpointComponent item : this.endpoint) 7058 if (!item.isEmpty()) 7059 return true; 7060 return false; 7061 } 7062 7063 public CapabilityStatementMessagingEndpointComponent addEndpoint() { // 3 7064 CapabilityStatementMessagingEndpointComponent t = new CapabilityStatementMessagingEndpointComponent(); 7065 if (this.endpoint == null) 7066 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7067 this.endpoint.add(t); 7068 return t; 7069 } 7070 7071 public CapabilityStatementMessagingComponent addEndpoint(CapabilityStatementMessagingEndpointComponent t) { // 3 7072 if (t == null) 7073 return this; 7074 if (this.endpoint == null) 7075 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7076 this.endpoint.add(t); 7077 return this; 7078 } 7079 7080 /** 7081 * @return The first repetition of repeating field {@link #endpoint}, creating 7082 * it if it does not already exist 7083 */ 7084 public CapabilityStatementMessagingEndpointComponent getEndpointFirstRep() { 7085 if (getEndpoint().isEmpty()) { 7086 addEndpoint(); 7087 } 7088 return getEndpoint().get(0); 7089 } 7090 7091 /** 7092 * @return {@link #reliableCache} (Length if the receiver's reliable messaging 7093 * cache in minutes (if a receiver) or how long the cache length on the 7094 * receiver should be (if a sender).). This is the underlying object 7095 * with id, value and extensions. The accessor "getReliableCache" gives 7096 * direct access to the value 7097 */ 7098 public UnsignedIntType getReliableCacheElement() { 7099 if (this.reliableCache == null) 7100 if (Configuration.errorOnAutoCreate()) 7101 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.reliableCache"); 7102 else if (Configuration.doAutoCreate()) 7103 this.reliableCache = new UnsignedIntType(); // bb 7104 return this.reliableCache; 7105 } 7106 7107 public boolean hasReliableCacheElement() { 7108 return this.reliableCache != null && !this.reliableCache.isEmpty(); 7109 } 7110 7111 public boolean hasReliableCache() { 7112 return this.reliableCache != null && !this.reliableCache.isEmpty(); 7113 } 7114 7115 /** 7116 * @param value {@link #reliableCache} (Length if the receiver's reliable 7117 * messaging cache in minutes (if a receiver) or how long the cache 7118 * length on the receiver should be (if a sender).). This is the 7119 * underlying object with id, value and extensions. The accessor 7120 * "getReliableCache" gives direct access to the value 7121 */ 7122 public CapabilityStatementMessagingComponent setReliableCacheElement(UnsignedIntType value) { 7123 this.reliableCache = value; 7124 return this; 7125 } 7126 7127 /** 7128 * @return Length if the receiver's reliable messaging cache in minutes (if a 7129 * receiver) or how long the cache length on the receiver should be (if 7130 * a sender). 7131 */ 7132 public int getReliableCache() { 7133 return this.reliableCache == null || this.reliableCache.isEmpty() ? 0 : this.reliableCache.getValue(); 7134 } 7135 7136 /** 7137 * @param value Length if the receiver's reliable messaging cache in minutes (if 7138 * a receiver) or how long the cache length on the receiver should 7139 * be (if a sender). 7140 */ 7141 public CapabilityStatementMessagingComponent setReliableCache(int value) { 7142 if (this.reliableCache == null) 7143 this.reliableCache = new UnsignedIntType(); 7144 this.reliableCache.setValue(value); 7145 return this; 7146 } 7147 7148 /** 7149 * @return {@link #documentation} (Documentation about the system's messaging 7150 * capabilities for this endpoint not otherwise documented by the 7151 * capability statement. For example, the process for becoming an 7152 * authorized messaging exchange partner.). This is the underlying 7153 * object with id, value and extensions. The accessor "getDocumentation" 7154 * gives direct access to the value 7155 */ 7156 public MarkdownType getDocumentationElement() { 7157 if (this.documentation == null) 7158 if (Configuration.errorOnAutoCreate()) 7159 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.documentation"); 7160 else if (Configuration.doAutoCreate()) 7161 this.documentation = new MarkdownType(); // bb 7162 return this.documentation; 7163 } 7164 7165 public boolean hasDocumentationElement() { 7166 return this.documentation != null && !this.documentation.isEmpty(); 7167 } 7168 7169 public boolean hasDocumentation() { 7170 return this.documentation != null && !this.documentation.isEmpty(); 7171 } 7172 7173 /** 7174 * @param value {@link #documentation} (Documentation about the system's 7175 * messaging capabilities for this endpoint not otherwise 7176 * documented by the capability statement. For example, the process 7177 * for becoming an authorized messaging exchange partner.). This is 7178 * the underlying object with id, value and extensions. The 7179 * accessor "getDocumentation" gives direct access to the value 7180 */ 7181 public CapabilityStatementMessagingComponent setDocumentationElement(MarkdownType value) { 7182 this.documentation = value; 7183 return this; 7184 } 7185 7186 /** 7187 * @return Documentation about the system's messaging capabilities for this 7188 * endpoint not otherwise documented by the capability statement. For 7189 * example, the process for becoming an authorized messaging exchange 7190 * partner. 7191 */ 7192 public String getDocumentation() { 7193 return this.documentation == null ? null : this.documentation.getValue(); 7194 } 7195 7196 /** 7197 * @param value Documentation about the system's messaging capabilities for this 7198 * endpoint not otherwise documented by the capability statement. 7199 * For example, the process for becoming an authorized messaging 7200 * exchange partner. 7201 */ 7202 public CapabilityStatementMessagingComponent setDocumentation(String value) { 7203 if (value == null) 7204 this.documentation = null; 7205 else { 7206 if (this.documentation == null) 7207 this.documentation = new MarkdownType(); 7208 this.documentation.setValue(value); 7209 } 7210 return this; 7211 } 7212 7213 /** 7214 * @return {@link #supportedMessage} (References to message definitions for 7215 * messages this system can send or receive.) 7216 */ 7217 public List<CapabilityStatementMessagingSupportedMessageComponent> getSupportedMessage() { 7218 if (this.supportedMessage == null) 7219 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7220 return this.supportedMessage; 7221 } 7222 7223 /** 7224 * @return Returns a reference to <code>this</code> for easy method chaining 7225 */ 7226 public CapabilityStatementMessagingComponent setSupportedMessage( 7227 List<CapabilityStatementMessagingSupportedMessageComponent> theSupportedMessage) { 7228 this.supportedMessage = theSupportedMessage; 7229 return this; 7230 } 7231 7232 public boolean hasSupportedMessage() { 7233 if (this.supportedMessage == null) 7234 return false; 7235 for (CapabilityStatementMessagingSupportedMessageComponent item : this.supportedMessage) 7236 if (!item.isEmpty()) 7237 return true; 7238 return false; 7239 } 7240 7241 public CapabilityStatementMessagingSupportedMessageComponent addSupportedMessage() { // 3 7242 CapabilityStatementMessagingSupportedMessageComponent t = new CapabilityStatementMessagingSupportedMessageComponent(); 7243 if (this.supportedMessage == null) 7244 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7245 this.supportedMessage.add(t); 7246 return t; 7247 } 7248 7249 public CapabilityStatementMessagingComponent addSupportedMessage( 7250 CapabilityStatementMessagingSupportedMessageComponent t) { // 3 7251 if (t == null) 7252 return this; 7253 if (this.supportedMessage == null) 7254 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7255 this.supportedMessage.add(t); 7256 return this; 7257 } 7258 7259 /** 7260 * @return The first repetition of repeating field {@link #supportedMessage}, 7261 * creating it if it does not already exist 7262 */ 7263 public CapabilityStatementMessagingSupportedMessageComponent getSupportedMessageFirstRep() { 7264 if (getSupportedMessage().isEmpty()) { 7265 addSupportedMessage(); 7266 } 7267 return getSupportedMessage().get(0); 7268 } 7269 7270 protected void listChildren(List<Property> children) { 7271 super.listChildren(children); 7272 children.add(new Property("endpoint", "", 7273 "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, 7274 java.lang.Integer.MAX_VALUE, endpoint)); 7275 children.add(new Property("reliableCache", "unsignedInt", 7276 "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 7277 0, 1, reliableCache)); 7278 children.add(new Property("documentation", "markdown", 7279 "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 7280 0, 1, documentation)); 7281 children.add(new Property("supportedMessage", "", 7282 "References to message definitions for messages this system can send or receive.", 0, 7283 java.lang.Integer.MAX_VALUE, supportedMessage)); 7284 } 7285 7286 @Override 7287 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7288 switch (_hash) { 7289 case 1741102485: 7290 /* endpoint */ return new Property("endpoint", "", 7291 "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, 7292 java.lang.Integer.MAX_VALUE, endpoint); 7293 case 897803608: 7294 /* reliableCache */ return new Property("reliableCache", "unsignedInt", 7295 "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 7296 0, 1, reliableCache); 7297 case 1587405498: 7298 /* documentation */ return new Property("documentation", "markdown", 7299 "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 7300 0, 1, documentation); 7301 case -1805139079: 7302 /* supportedMessage */ return new Property("supportedMessage", "", 7303 "References to message definitions for messages this system can send or receive.", 0, 7304 java.lang.Integer.MAX_VALUE, supportedMessage); 7305 default: 7306 return super.getNamedProperty(_hash, _name, _checkValid); 7307 } 7308 7309 } 7310 7311 @Override 7312 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7313 switch (hash) { 7314 case 1741102485: 7315 /* endpoint */ return this.endpoint == null ? new Base[0] 7316 : this.endpoint.toArray(new Base[this.endpoint.size()]); // CapabilityStatementMessagingEndpointComponent 7317 case 897803608: 7318 /* reliableCache */ return this.reliableCache == null ? new Base[0] : new Base[] { this.reliableCache }; // UnsignedIntType 7319 case 1587405498: 7320 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 7321 case -1805139079: 7322 /* supportedMessage */ return this.supportedMessage == null ? new Base[0] 7323 : this.supportedMessage.toArray(new Base[this.supportedMessage.size()]); // CapabilityStatementMessagingSupportedMessageComponent 7324 default: 7325 return super.getProperty(hash, name, checkValid); 7326 } 7327 7328 } 7329 7330 @Override 7331 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7332 switch (hash) { 7333 case 1741102485: // endpoint 7334 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); // CapabilityStatementMessagingEndpointComponent 7335 return value; 7336 case 897803608: // reliableCache 7337 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 7338 return value; 7339 case 1587405498: // documentation 7340 this.documentation = castToMarkdown(value); // MarkdownType 7341 return value; 7342 case -1805139079: // supportedMessage 7343 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); // CapabilityStatementMessagingSupportedMessageComponent 7344 return value; 7345 default: 7346 return super.setProperty(hash, name, value); 7347 } 7348 7349 } 7350 7351 @Override 7352 public Base setProperty(String name, Base value) throws FHIRException { 7353 if (name.equals("endpoint")) { 7354 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); 7355 } else if (name.equals("reliableCache")) { 7356 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 7357 } else if (name.equals("documentation")) { 7358 this.documentation = castToMarkdown(value); // MarkdownType 7359 } else if (name.equals("supportedMessage")) { 7360 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); 7361 } else 7362 return super.setProperty(name, value); 7363 return value; 7364 } 7365 7366 @Override 7367 public void removeChild(String name, Base value) throws FHIRException { 7368 if (name.equals("endpoint")) { 7369 this.getEndpoint().remove((CapabilityStatementMessagingEndpointComponent) value); 7370 } else if (name.equals("reliableCache")) { 7371 this.reliableCache = null; 7372 } else if (name.equals("documentation")) { 7373 this.documentation = null; 7374 } else if (name.equals("supportedMessage")) { 7375 this.getSupportedMessage().remove((CapabilityStatementMessagingSupportedMessageComponent) value); 7376 } else 7377 super.removeChild(name, value); 7378 7379 } 7380 7381 @Override 7382 public Base makeProperty(int hash, String name) throws FHIRException { 7383 switch (hash) { 7384 case 1741102485: 7385 return addEndpoint(); 7386 case 897803608: 7387 return getReliableCacheElement(); 7388 case 1587405498: 7389 return getDocumentationElement(); 7390 case -1805139079: 7391 return addSupportedMessage(); 7392 default: 7393 return super.makeProperty(hash, name); 7394 } 7395 7396 } 7397 7398 @Override 7399 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7400 switch (hash) { 7401 case 1741102485: 7402 /* endpoint */ return new String[] {}; 7403 case 897803608: 7404 /* reliableCache */ return new String[] { "unsignedInt" }; 7405 case 1587405498: 7406 /* documentation */ return new String[] { "markdown" }; 7407 case -1805139079: 7408 /* supportedMessage */ return new String[] {}; 7409 default: 7410 return super.getTypesForProperty(hash, name); 7411 } 7412 7413 } 7414 7415 @Override 7416 public Base addChild(String name) throws FHIRException { 7417 if (name.equals("endpoint")) { 7418 return addEndpoint(); 7419 } else if (name.equals("reliableCache")) { 7420 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.reliableCache"); 7421 } else if (name.equals("documentation")) { 7422 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 7423 } else if (name.equals("supportedMessage")) { 7424 return addSupportedMessage(); 7425 } else 7426 return super.addChild(name); 7427 } 7428 7429 public CapabilityStatementMessagingComponent copy() { 7430 CapabilityStatementMessagingComponent dst = new CapabilityStatementMessagingComponent(); 7431 copyValues(dst); 7432 return dst; 7433 } 7434 7435 public void copyValues(CapabilityStatementMessagingComponent dst) { 7436 super.copyValues(dst); 7437 if (endpoint != null) { 7438 dst.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7439 for (CapabilityStatementMessagingEndpointComponent i : endpoint) 7440 dst.endpoint.add(i.copy()); 7441 } 7442 ; 7443 dst.reliableCache = reliableCache == null ? null : reliableCache.copy(); 7444 dst.documentation = documentation == null ? null : documentation.copy(); 7445 if (supportedMessage != null) { 7446 dst.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7447 for (CapabilityStatementMessagingSupportedMessageComponent i : supportedMessage) 7448 dst.supportedMessage.add(i.copy()); 7449 } 7450 ; 7451 } 7452 7453 @Override 7454 public boolean equalsDeep(Base other_) { 7455 if (!super.equalsDeep(other_)) 7456 return false; 7457 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 7458 return false; 7459 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 7460 return compareDeep(endpoint, o.endpoint, true) && compareDeep(reliableCache, o.reliableCache, true) 7461 && compareDeep(documentation, o.documentation, true) 7462 && compareDeep(supportedMessage, o.supportedMessage, true); 7463 } 7464 7465 @Override 7466 public boolean equalsShallow(Base other_) { 7467 if (!super.equalsShallow(other_)) 7468 return false; 7469 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 7470 return false; 7471 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 7472 return compareValues(reliableCache, o.reliableCache, true) && compareValues(documentation, o.documentation, true); 7473 } 7474 7475 public boolean isEmpty() { 7476 return super.isEmpty() 7477 && ca.uhn.fhir.util.ElementUtil.isEmpty(endpoint, reliableCache, documentation, supportedMessage); 7478 } 7479 7480 public String fhirType() { 7481 return "CapabilityStatement.messaging"; 7482 7483 } 7484 7485 } 7486 7487 @Block() 7488 public static class CapabilityStatementMessagingEndpointComponent extends BackboneElement 7489 implements IBaseBackboneElement { 7490 /** 7491 * A list of the messaging transport protocol(s) identifiers, supported by this 7492 * endpoint. 7493 */ 7494 @Child(name = "protocol", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7495 @Description(shortDefinition = "http | ftp | mllp +", formalDefinition = "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.") 7496 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-transport") 7497 protected Coding protocol; 7498 7499 /** 7500 * The network address of the endpoint. For solutions that do not use network 7501 * addresses for routing, it can be just an identifier. 7502 */ 7503 @Child(name = "address", type = { UrlType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 7504 @Description(shortDefinition = "Network address or identifier of the end-point", formalDefinition = "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.") 7505 protected UrlType address; 7506 7507 private static final long serialVersionUID = -236946103L; 7508 7509 /** 7510 * Constructor 7511 */ 7512 public CapabilityStatementMessagingEndpointComponent() { 7513 super(); 7514 } 7515 7516 /** 7517 * Constructor 7518 */ 7519 public CapabilityStatementMessagingEndpointComponent(Coding protocol, UrlType address) { 7520 super(); 7521 this.protocol = protocol; 7522 this.address = address; 7523 } 7524 7525 /** 7526 * @return {@link #protocol} (A list of the messaging transport protocol(s) 7527 * identifiers, supported by this endpoint.) 7528 */ 7529 public Coding getProtocol() { 7530 if (this.protocol == null) 7531 if (Configuration.errorOnAutoCreate()) 7532 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.protocol"); 7533 else if (Configuration.doAutoCreate()) 7534 this.protocol = new Coding(); // cc 7535 return this.protocol; 7536 } 7537 7538 public boolean hasProtocol() { 7539 return this.protocol != null && !this.protocol.isEmpty(); 7540 } 7541 7542 /** 7543 * @param value {@link #protocol} (A list of the messaging transport protocol(s) 7544 * identifiers, supported by this endpoint.) 7545 */ 7546 public CapabilityStatementMessagingEndpointComponent setProtocol(Coding value) { 7547 this.protocol = value; 7548 return this; 7549 } 7550 7551 /** 7552 * @return {@link #address} (The network address of the endpoint. For solutions 7553 * that do not use network addresses for routing, it can be just an 7554 * identifier.). This is the underlying object with id, value and 7555 * extensions. The accessor "getAddress" gives direct access to the 7556 * value 7557 */ 7558 public UrlType getAddressElement() { 7559 if (this.address == null) 7560 if (Configuration.errorOnAutoCreate()) 7561 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.address"); 7562 else if (Configuration.doAutoCreate()) 7563 this.address = new UrlType(); // bb 7564 return this.address; 7565 } 7566 7567 public boolean hasAddressElement() { 7568 return this.address != null && !this.address.isEmpty(); 7569 } 7570 7571 public boolean hasAddress() { 7572 return this.address != null && !this.address.isEmpty(); 7573 } 7574 7575 /** 7576 * @param value {@link #address} (The network address of the endpoint. For 7577 * solutions that do not use network addresses for routing, it can 7578 * be just an identifier.). This is the underlying object with id, 7579 * value and extensions. The accessor "getAddress" gives direct 7580 * access to the value 7581 */ 7582 public CapabilityStatementMessagingEndpointComponent setAddressElement(UrlType value) { 7583 this.address = value; 7584 return this; 7585 } 7586 7587 /** 7588 * @return The network address of the endpoint. For solutions that do not use 7589 * network addresses for routing, it can be just an identifier. 7590 */ 7591 public String getAddress() { 7592 return this.address == null ? null : this.address.getValue(); 7593 } 7594 7595 /** 7596 * @param value The network address of the endpoint. For solutions that do not 7597 * use network addresses for routing, it can be just an identifier. 7598 */ 7599 public CapabilityStatementMessagingEndpointComponent setAddress(String value) { 7600 if (this.address == null) 7601 this.address = new UrlType(); 7602 this.address.setValue(value); 7603 return this; 7604 } 7605 7606 protected void listChildren(List<Property> children) { 7607 super.listChildren(children); 7608 children.add(new Property("protocol", "Coding", 7609 "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol)); 7610 children.add(new Property("address", "url", 7611 "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.", 7612 0, 1, address)); 7613 } 7614 7615 @Override 7616 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7617 switch (_hash) { 7618 case -989163880: 7619 /* protocol */ return new Property("protocol", "Coding", 7620 "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol); 7621 case -1147692044: 7622 /* address */ return new Property("address", "url", 7623 "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.", 7624 0, 1, address); 7625 default: 7626 return super.getNamedProperty(_hash, _name, _checkValid); 7627 } 7628 7629 } 7630 7631 @Override 7632 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7633 switch (hash) { 7634 case -989163880: 7635 /* protocol */ return this.protocol == null ? new Base[0] : new Base[] { this.protocol }; // Coding 7636 case -1147692044: 7637 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // UrlType 7638 default: 7639 return super.getProperty(hash, name, checkValid); 7640 } 7641 7642 } 7643 7644 @Override 7645 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7646 switch (hash) { 7647 case -989163880: // protocol 7648 this.protocol = castToCoding(value); // Coding 7649 return value; 7650 case -1147692044: // address 7651 this.address = castToUrl(value); // UrlType 7652 return value; 7653 default: 7654 return super.setProperty(hash, name, value); 7655 } 7656 7657 } 7658 7659 @Override 7660 public Base setProperty(String name, Base value) throws FHIRException { 7661 if (name.equals("protocol")) { 7662 this.protocol = castToCoding(value); // Coding 7663 } else if (name.equals("address")) { 7664 this.address = castToUrl(value); // UrlType 7665 } else 7666 return super.setProperty(name, value); 7667 return value; 7668 } 7669 7670 @Override 7671 public void removeChild(String name, Base value) throws FHIRException { 7672 if (name.equals("protocol")) { 7673 this.protocol = null; 7674 } else if (name.equals("address")) { 7675 this.address = null; 7676 } else 7677 super.removeChild(name, value); 7678 7679 } 7680 7681 @Override 7682 public Base makeProperty(int hash, String name) throws FHIRException { 7683 switch (hash) { 7684 case -989163880: 7685 return getProtocol(); 7686 case -1147692044: 7687 return getAddressElement(); 7688 default: 7689 return super.makeProperty(hash, name); 7690 } 7691 7692 } 7693 7694 @Override 7695 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7696 switch (hash) { 7697 case -989163880: 7698 /* protocol */ return new String[] { "Coding" }; 7699 case -1147692044: 7700 /* address */ return new String[] { "url" }; 7701 default: 7702 return super.getTypesForProperty(hash, name); 7703 } 7704 7705 } 7706 7707 @Override 7708 public Base addChild(String name) throws FHIRException { 7709 if (name.equals("protocol")) { 7710 this.protocol = new Coding(); 7711 return this.protocol; 7712 } else if (name.equals("address")) { 7713 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.address"); 7714 } else 7715 return super.addChild(name); 7716 } 7717 7718 public CapabilityStatementMessagingEndpointComponent copy() { 7719 CapabilityStatementMessagingEndpointComponent dst = new CapabilityStatementMessagingEndpointComponent(); 7720 copyValues(dst); 7721 return dst; 7722 } 7723 7724 public void copyValues(CapabilityStatementMessagingEndpointComponent dst) { 7725 super.copyValues(dst); 7726 dst.protocol = protocol == null ? null : protocol.copy(); 7727 dst.address = address == null ? null : address.copy(); 7728 } 7729 7730 @Override 7731 public boolean equalsDeep(Base other_) { 7732 if (!super.equalsDeep(other_)) 7733 return false; 7734 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 7735 return false; 7736 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 7737 return compareDeep(protocol, o.protocol, true) && compareDeep(address, o.address, true); 7738 } 7739 7740 @Override 7741 public boolean equalsShallow(Base other_) { 7742 if (!super.equalsShallow(other_)) 7743 return false; 7744 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 7745 return false; 7746 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 7747 return compareValues(address, o.address, true); 7748 } 7749 7750 public boolean isEmpty() { 7751 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(protocol, address); 7752 } 7753 7754 public String fhirType() { 7755 return "CapabilityStatement.messaging.endpoint"; 7756 7757 } 7758 7759 } 7760 7761 @Block() 7762 public static class CapabilityStatementMessagingSupportedMessageComponent extends BackboneElement 7763 implements IBaseBackboneElement { 7764 /** 7765 * The mode of this event declaration - whether application is sender or 7766 * receiver. 7767 */ 7768 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 7769 @Description(shortDefinition = "sender | receiver", formalDefinition = "The mode of this event declaration - whether application is sender or receiver.") 7770 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-capability-mode") 7771 protected Enumeration<EventCapabilityMode> mode; 7772 7773 /** 7774 * Points to a message definition that identifies the messaging event, message 7775 * structure, allowed responses, etc. 7776 */ 7777 @Child(name = "definition", type = { 7778 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 7779 @Description(shortDefinition = "Message supported by this system", formalDefinition = "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.") 7780 protected CanonicalType definition; 7781 7782 private static final long serialVersionUID = -1172840676L; 7783 7784 /** 7785 * Constructor 7786 */ 7787 public CapabilityStatementMessagingSupportedMessageComponent() { 7788 super(); 7789 } 7790 7791 /** 7792 * Constructor 7793 */ 7794 public CapabilityStatementMessagingSupportedMessageComponent(Enumeration<EventCapabilityMode> mode, 7795 CanonicalType definition) { 7796 super(); 7797 this.mode = mode; 7798 this.definition = definition; 7799 } 7800 7801 /** 7802 * @return {@link #mode} (The mode of this event declaration - whether 7803 * application is sender or receiver.). This is the underlying object 7804 * with id, value and extensions. The accessor "getMode" gives direct 7805 * access to the value 7806 */ 7807 public Enumeration<EventCapabilityMode> getModeElement() { 7808 if (this.mode == null) 7809 if (Configuration.errorOnAutoCreate()) 7810 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.mode"); 7811 else if (Configuration.doAutoCreate()) 7812 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); // bb 7813 return this.mode; 7814 } 7815 7816 public boolean hasModeElement() { 7817 return this.mode != null && !this.mode.isEmpty(); 7818 } 7819 7820 public boolean hasMode() { 7821 return this.mode != null && !this.mode.isEmpty(); 7822 } 7823 7824 /** 7825 * @param value {@link #mode} (The mode of this event declaration - whether 7826 * application is sender or receiver.). This is the underlying 7827 * object with id, value and extensions. The accessor "getMode" 7828 * gives direct access to the value 7829 */ 7830 public CapabilityStatementMessagingSupportedMessageComponent setModeElement( 7831 Enumeration<EventCapabilityMode> value) { 7832 this.mode = value; 7833 return this; 7834 } 7835 7836 /** 7837 * @return The mode of this event declaration - whether application is sender or 7838 * receiver. 7839 */ 7840 public EventCapabilityMode getMode() { 7841 return this.mode == null ? null : this.mode.getValue(); 7842 } 7843 7844 /** 7845 * @param value The mode of this event declaration - whether application is 7846 * sender or receiver. 7847 */ 7848 public CapabilityStatementMessagingSupportedMessageComponent setMode(EventCapabilityMode value) { 7849 if (this.mode == null) 7850 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); 7851 this.mode.setValue(value); 7852 return this; 7853 } 7854 7855 /** 7856 * @return {@link #definition} (Points to a message definition that identifies 7857 * the messaging event, message structure, allowed responses, etc.). 7858 * This is the underlying object with id, value and extensions. The 7859 * accessor "getDefinition" gives direct access to the value 7860 */ 7861 public CanonicalType getDefinitionElement() { 7862 if (this.definition == null) 7863 if (Configuration.errorOnAutoCreate()) 7864 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.definition"); 7865 else if (Configuration.doAutoCreate()) 7866 this.definition = new CanonicalType(); // bb 7867 return this.definition; 7868 } 7869 7870 public boolean hasDefinitionElement() { 7871 return this.definition != null && !this.definition.isEmpty(); 7872 } 7873 7874 public boolean hasDefinition() { 7875 return this.definition != null && !this.definition.isEmpty(); 7876 } 7877 7878 /** 7879 * @param value {@link #definition} (Points to a message definition that 7880 * identifies the messaging event, message structure, allowed 7881 * responses, etc.). This is the underlying object with id, value 7882 * and extensions. The accessor "getDefinition" gives direct access 7883 * to the value 7884 */ 7885 public CapabilityStatementMessagingSupportedMessageComponent setDefinitionElement(CanonicalType value) { 7886 this.definition = value; 7887 return this; 7888 } 7889 7890 /** 7891 * @return Points to a message definition that identifies the messaging event, 7892 * message structure, allowed responses, etc. 7893 */ 7894 public String getDefinition() { 7895 return this.definition == null ? null : this.definition.getValue(); 7896 } 7897 7898 /** 7899 * @param value Points to a message definition that identifies the messaging 7900 * event, message structure, allowed responses, etc. 7901 */ 7902 public CapabilityStatementMessagingSupportedMessageComponent setDefinition(String value) { 7903 if (this.definition == null) 7904 this.definition = new CanonicalType(); 7905 this.definition.setValue(value); 7906 return this; 7907 } 7908 7909 protected void listChildren(List<Property> children) { 7910 super.listChildren(children); 7911 children.add(new Property("mode", "code", 7912 "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode)); 7913 children.add(new Property("definition", "canonical(MessageDefinition)", 7914 "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 7915 0, 1, definition)); 7916 } 7917 7918 @Override 7919 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7920 switch (_hash) { 7921 case 3357091: 7922 /* mode */ return new Property("mode", "code", 7923 "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode); 7924 case -1014418093: 7925 /* definition */ return new Property("definition", "canonical(MessageDefinition)", 7926 "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 7927 0, 1, definition); 7928 default: 7929 return super.getNamedProperty(_hash, _name, _checkValid); 7930 } 7931 7932 } 7933 7934 @Override 7935 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7936 switch (hash) { 7937 case 3357091: 7938 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<EventCapabilityMode> 7939 case -1014418093: 7940 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 7941 default: 7942 return super.getProperty(hash, name, checkValid); 7943 } 7944 7945 } 7946 7947 @Override 7948 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7949 switch (hash) { 7950 case 3357091: // mode 7951 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 7952 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 7953 return value; 7954 case -1014418093: // definition 7955 this.definition = castToCanonical(value); // CanonicalType 7956 return value; 7957 default: 7958 return super.setProperty(hash, name, value); 7959 } 7960 7961 } 7962 7963 @Override 7964 public Base setProperty(String name, Base value) throws FHIRException { 7965 if (name.equals("mode")) { 7966 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 7967 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 7968 } else if (name.equals("definition")) { 7969 this.definition = castToCanonical(value); // CanonicalType 7970 } else 7971 return super.setProperty(name, value); 7972 return value; 7973 } 7974 7975 @Override 7976 public void removeChild(String name, Base value) throws FHIRException { 7977 if (name.equals("mode")) { 7978 this.mode = null; 7979 } else if (name.equals("definition")) { 7980 this.definition = null; 7981 } else 7982 super.removeChild(name, value); 7983 7984 } 7985 7986 @Override 7987 public Base makeProperty(int hash, String name) throws FHIRException { 7988 switch (hash) { 7989 case 3357091: 7990 return getModeElement(); 7991 case -1014418093: 7992 return getDefinitionElement(); 7993 default: 7994 return super.makeProperty(hash, name); 7995 } 7996 7997 } 7998 7999 @Override 8000 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8001 switch (hash) { 8002 case 3357091: 8003 /* mode */ return new String[] { "code" }; 8004 case -1014418093: 8005 /* definition */ return new String[] { "canonical" }; 8006 default: 8007 return super.getTypesForProperty(hash, name); 8008 } 8009 8010 } 8011 8012 @Override 8013 public Base addChild(String name) throws FHIRException { 8014 if (name.equals("mode")) { 8015 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 8016 } else if (name.equals("definition")) { 8017 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 8018 } else 8019 return super.addChild(name); 8020 } 8021 8022 public CapabilityStatementMessagingSupportedMessageComponent copy() { 8023 CapabilityStatementMessagingSupportedMessageComponent dst = new CapabilityStatementMessagingSupportedMessageComponent(); 8024 copyValues(dst); 8025 return dst; 8026 } 8027 8028 public void copyValues(CapabilityStatementMessagingSupportedMessageComponent dst) { 8029 super.copyValues(dst); 8030 dst.mode = mode == null ? null : mode.copy(); 8031 dst.definition = definition == null ? null : definition.copy(); 8032 } 8033 8034 @Override 8035 public boolean equalsDeep(Base other_) { 8036 if (!super.equalsDeep(other_)) 8037 return false; 8038 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 8039 return false; 8040 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 8041 return compareDeep(mode, o.mode, true) && compareDeep(definition, o.definition, true); 8042 } 8043 8044 @Override 8045 public boolean equalsShallow(Base other_) { 8046 if (!super.equalsShallow(other_)) 8047 return false; 8048 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 8049 return false; 8050 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 8051 return compareValues(mode, o.mode, true); 8052 } 8053 8054 public boolean isEmpty() { 8055 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, definition); 8056 } 8057 8058 public String fhirType() { 8059 return "CapabilityStatement.messaging.supportedMessage"; 8060 8061 } 8062 8063 } 8064 8065 @Block() 8066 public static class CapabilityStatementDocumentComponent extends BackboneElement implements IBaseBackboneElement { 8067 /** 8068 * Mode of this document declaration - whether an application is a producer or 8069 * consumer. 8070 */ 8071 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 8072 @Description(shortDefinition = "producer | consumer", formalDefinition = "Mode of this document declaration - whether an application is a producer or consumer.") 8073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-mode") 8074 protected Enumeration<DocumentMode> mode; 8075 8076 /** 8077 * A description of how the application supports or uses the specified document 8078 * profile. For example, when documents are created, what action is taken with 8079 * consumed documents, etc. 8080 */ 8081 @Child(name = "documentation", type = { 8082 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 8083 @Description(shortDefinition = "Description of document support", formalDefinition = "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.") 8084 protected MarkdownType documentation; 8085 8086 /** 8087 * A profile on the document Bundle that constrains which resources are present, 8088 * and their contents. 8089 */ 8090 @Child(name = "profile", type = { 8091 CanonicalType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 8092 @Description(shortDefinition = "Constraint on the resources used in the document", formalDefinition = "A profile on the document Bundle that constrains which resources are present, and their contents.") 8093 protected CanonicalType profile; 8094 8095 private static final long serialVersionUID = 18026632L; 8096 8097 /** 8098 * Constructor 8099 */ 8100 public CapabilityStatementDocumentComponent() { 8101 super(); 8102 } 8103 8104 /** 8105 * Constructor 8106 */ 8107 public CapabilityStatementDocumentComponent(Enumeration<DocumentMode> mode, CanonicalType profile) { 8108 super(); 8109 this.mode = mode; 8110 this.profile = profile; 8111 } 8112 8113 /** 8114 * @return {@link #mode} (Mode of this document declaration - whether an 8115 * application is a producer or consumer.). This is the underlying 8116 * object with id, value and extensions. The accessor "getMode" gives 8117 * direct access to the value 8118 */ 8119 public Enumeration<DocumentMode> getModeElement() { 8120 if (this.mode == null) 8121 if (Configuration.errorOnAutoCreate()) 8122 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.mode"); 8123 else if (Configuration.doAutoCreate()) 8124 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); // bb 8125 return this.mode; 8126 } 8127 8128 public boolean hasModeElement() { 8129 return this.mode != null && !this.mode.isEmpty(); 8130 } 8131 8132 public boolean hasMode() { 8133 return this.mode != null && !this.mode.isEmpty(); 8134 } 8135 8136 /** 8137 * @param value {@link #mode} (Mode of this document declaration - whether an 8138 * application is a producer or consumer.). This is the underlying 8139 * object with id, value and extensions. The accessor "getMode" 8140 * gives direct access to the value 8141 */ 8142 public CapabilityStatementDocumentComponent setModeElement(Enumeration<DocumentMode> value) { 8143 this.mode = value; 8144 return this; 8145 } 8146 8147 /** 8148 * @return Mode of this document declaration - whether an application is a 8149 * producer or consumer. 8150 */ 8151 public DocumentMode getMode() { 8152 return this.mode == null ? null : this.mode.getValue(); 8153 } 8154 8155 /** 8156 * @param value Mode of this document declaration - whether an application is a 8157 * producer or consumer. 8158 */ 8159 public CapabilityStatementDocumentComponent setMode(DocumentMode value) { 8160 if (this.mode == null) 8161 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); 8162 this.mode.setValue(value); 8163 return this; 8164 } 8165 8166 /** 8167 * @return {@link #documentation} (A description of how the application supports 8168 * or uses the specified document profile. For example, when documents 8169 * are created, what action is taken with consumed documents, etc.). 8170 * This is the underlying object with id, value and extensions. The 8171 * accessor "getDocumentation" gives direct access to the value 8172 */ 8173 public MarkdownType getDocumentationElement() { 8174 if (this.documentation == null) 8175 if (Configuration.errorOnAutoCreate()) 8176 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.documentation"); 8177 else if (Configuration.doAutoCreate()) 8178 this.documentation = new MarkdownType(); // bb 8179 return this.documentation; 8180 } 8181 8182 public boolean hasDocumentationElement() { 8183 return this.documentation != null && !this.documentation.isEmpty(); 8184 } 8185 8186 public boolean hasDocumentation() { 8187 return this.documentation != null && !this.documentation.isEmpty(); 8188 } 8189 8190 /** 8191 * @param value {@link #documentation} (A description of how the application 8192 * supports or uses the specified document profile. For example, 8193 * when documents are created, what action is taken with consumed 8194 * documents, etc.). This is the underlying object with id, value 8195 * and extensions. The accessor "getDocumentation" gives direct 8196 * access to the value 8197 */ 8198 public CapabilityStatementDocumentComponent setDocumentationElement(MarkdownType value) { 8199 this.documentation = value; 8200 return this; 8201 } 8202 8203 /** 8204 * @return A description of how the application supports or uses the specified 8205 * document profile. For example, when documents are created, what 8206 * action is taken with consumed documents, etc. 8207 */ 8208 public String getDocumentation() { 8209 return this.documentation == null ? null : this.documentation.getValue(); 8210 } 8211 8212 /** 8213 * @param value A description of how the application supports or uses the 8214 * specified document profile. For example, when documents are 8215 * created, what action is taken with consumed documents, etc. 8216 */ 8217 public CapabilityStatementDocumentComponent setDocumentation(String value) { 8218 if (value == null) 8219 this.documentation = null; 8220 else { 8221 if (this.documentation == null) 8222 this.documentation = new MarkdownType(); 8223 this.documentation.setValue(value); 8224 } 8225 return this; 8226 } 8227 8228 /** 8229 * @return {@link #profile} (A profile on the document Bundle that constrains 8230 * which resources are present, and their contents.). This is the 8231 * underlying object with id, value and extensions. The accessor 8232 * "getProfile" gives direct access to the value 8233 */ 8234 public CanonicalType getProfileElement() { 8235 if (this.profile == null) 8236 if (Configuration.errorOnAutoCreate()) 8237 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.profile"); 8238 else if (Configuration.doAutoCreate()) 8239 this.profile = new CanonicalType(); // bb 8240 return this.profile; 8241 } 8242 8243 public boolean hasProfileElement() { 8244 return this.profile != null && !this.profile.isEmpty(); 8245 } 8246 8247 public boolean hasProfile() { 8248 return this.profile != null && !this.profile.isEmpty(); 8249 } 8250 8251 /** 8252 * @param value {@link #profile} (A profile on the document Bundle that 8253 * constrains which resources are present, and their contents.). 8254 * This is the underlying object with id, value and extensions. The 8255 * accessor "getProfile" gives direct access to the value 8256 */ 8257 public CapabilityStatementDocumentComponent setProfileElement(CanonicalType value) { 8258 this.profile = value; 8259 return this; 8260 } 8261 8262 /** 8263 * @return A profile on the document Bundle that constrains which resources are 8264 * present, and their contents. 8265 */ 8266 public String getProfile() { 8267 return this.profile == null ? null : this.profile.getValue(); 8268 } 8269 8270 /** 8271 * @param value A profile on the document Bundle that constrains which resources 8272 * are present, and their contents. 8273 */ 8274 public CapabilityStatementDocumentComponent setProfile(String value) { 8275 if (this.profile == null) 8276 this.profile = new CanonicalType(); 8277 this.profile.setValue(value); 8278 return this; 8279 } 8280 8281 protected void listChildren(List<Property> children) { 8282 super.listChildren(children); 8283 children.add(new Property("mode", "code", 8284 "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode)); 8285 children.add(new Property("documentation", "markdown", 8286 "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 8287 0, 1, documentation)); 8288 children.add(new Property("profile", "canonical(StructureDefinition)", 8289 "A profile on the document Bundle that constrains which resources are present, and their contents.", 0, 1, 8290 profile)); 8291 } 8292 8293 @Override 8294 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8295 switch (_hash) { 8296 case 3357091: 8297 /* mode */ return new Property("mode", "code", 8298 "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode); 8299 case 1587405498: 8300 /* documentation */ return new Property("documentation", "markdown", 8301 "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 8302 0, 1, documentation); 8303 case -309425751: 8304 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 8305 "A profile on the document Bundle that constrains which resources are present, and their contents.", 0, 1, 8306 profile); 8307 default: 8308 return super.getNamedProperty(_hash, _name, _checkValid); 8309 } 8310 8311 } 8312 8313 @Override 8314 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8315 switch (hash) { 8316 case 3357091: 8317 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<DocumentMode> 8318 case 1587405498: 8319 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 8320 case -309425751: 8321 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 8322 default: 8323 return super.getProperty(hash, name, checkValid); 8324 } 8325 8326 } 8327 8328 @Override 8329 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8330 switch (hash) { 8331 case 3357091: // mode 8332 value = new DocumentModeEnumFactory().fromType(castToCode(value)); 8333 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 8334 return value; 8335 case 1587405498: // documentation 8336 this.documentation = castToMarkdown(value); // MarkdownType 8337 return value; 8338 case -309425751: // profile 8339 this.profile = castToCanonical(value); // CanonicalType 8340 return value; 8341 default: 8342 return super.setProperty(hash, name, value); 8343 } 8344 8345 } 8346 8347 @Override 8348 public Base setProperty(String name, Base value) throws FHIRException { 8349 if (name.equals("mode")) { 8350 value = new DocumentModeEnumFactory().fromType(castToCode(value)); 8351 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 8352 } else if (name.equals("documentation")) { 8353 this.documentation = castToMarkdown(value); // MarkdownType 8354 } else if (name.equals("profile")) { 8355 this.profile = castToCanonical(value); // CanonicalType 8356 } else 8357 return super.setProperty(name, value); 8358 return value; 8359 } 8360 8361 @Override 8362 public void removeChild(String name, Base value) throws FHIRException { 8363 if (name.equals("mode")) { 8364 this.mode = null; 8365 } else if (name.equals("documentation")) { 8366 this.documentation = null; 8367 } else if (name.equals("profile")) { 8368 this.profile = null; 8369 } else 8370 super.removeChild(name, value); 8371 8372 } 8373 8374 @Override 8375 public Base makeProperty(int hash, String name) throws FHIRException { 8376 switch (hash) { 8377 case 3357091: 8378 return getModeElement(); 8379 case 1587405498: 8380 return getDocumentationElement(); 8381 case -309425751: 8382 return getProfileElement(); 8383 default: 8384 return super.makeProperty(hash, name); 8385 } 8386 8387 } 8388 8389 @Override 8390 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8391 switch (hash) { 8392 case 3357091: 8393 /* mode */ return new String[] { "code" }; 8394 case 1587405498: 8395 /* documentation */ return new String[] { "markdown" }; 8396 case -309425751: 8397 /* profile */ return new String[] { "canonical" }; 8398 default: 8399 return super.getTypesForProperty(hash, name); 8400 } 8401 8402 } 8403 8404 @Override 8405 public Base addChild(String name) throws FHIRException { 8406 if (name.equals("mode")) { 8407 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 8408 } else if (name.equals("documentation")) { 8409 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 8410 } else if (name.equals("profile")) { 8411 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.profile"); 8412 } else 8413 return super.addChild(name); 8414 } 8415 8416 public CapabilityStatementDocumentComponent copy() { 8417 CapabilityStatementDocumentComponent dst = new CapabilityStatementDocumentComponent(); 8418 copyValues(dst); 8419 return dst; 8420 } 8421 8422 public void copyValues(CapabilityStatementDocumentComponent dst) { 8423 super.copyValues(dst); 8424 dst.mode = mode == null ? null : mode.copy(); 8425 dst.documentation = documentation == null ? null : documentation.copy(); 8426 dst.profile = profile == null ? null : profile.copy(); 8427 } 8428 8429 @Override 8430 public boolean equalsDeep(Base other_) { 8431 if (!super.equalsDeep(other_)) 8432 return false; 8433 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 8434 return false; 8435 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 8436 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 8437 && compareDeep(profile, o.profile, true); 8438 } 8439 8440 @Override 8441 public boolean equalsShallow(Base other_) { 8442 if (!super.equalsShallow(other_)) 8443 return false; 8444 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 8445 return false; 8446 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 8447 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 8448 } 8449 8450 public boolean isEmpty() { 8451 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, profile); 8452 } 8453 8454 public String fhirType() { 8455 return "CapabilityStatement.document"; 8456 8457 } 8458 8459 } 8460 8461 /** 8462 * Explanation of why this capability statement is needed and why it has been 8463 * designed as it has. 8464 */ 8465 @Child(name = "purpose", type = { 8466 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 8467 @Description(shortDefinition = "Why this capability statement is defined", formalDefinition = "Explanation of why this capability statement is needed and why it has been designed as it has.") 8468 protected MarkdownType purpose; 8469 8470 /** 8471 * A copyright statement relating to the capability statement and/or its 8472 * contents. Copyright statements are generally legal restrictions on the use 8473 * and publishing of the capability statement. 8474 */ 8475 @Child(name = "copyright", type = { 8476 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 8477 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.") 8478 protected MarkdownType copyright; 8479 8480 /** 8481 * The way that this statement is intended to be used, to describe an actual 8482 * running instance of software, a particular product (kind, not instance of 8483 * software) or a class of implementation (e.g. a desired purchase). 8484 */ 8485 @Child(name = "kind", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 8486 @Description(shortDefinition = "instance | capability | requirements", formalDefinition = "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).") 8487 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/capability-statement-kind") 8488 protected Enumeration<CapabilityStatementKind> kind; 8489 8490 /** 8491 * Reference to a canonical URL of another CapabilityStatement that this 8492 * software implements. This capability statement is a published API description 8493 * that corresponds to a business service. The server may actually implement a 8494 * subset of the capability statement it claims to implement, so the capability 8495 * statement must specify the full capability details. 8496 */ 8497 @Child(name = "instantiates", type = { 8498 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8499 @Description(shortDefinition = "Canonical URL of another capability statement this implements", formalDefinition = "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.") 8500 protected List<CanonicalType> instantiates; 8501 8502 /** 8503 * Reference to a canonical URL of another CapabilityStatement that this 8504 * software adds to. The capability statement automatically includes everything 8505 * in the other statement, and it is not duplicated, though the server may 8506 * repeat the same resources, interactions and operations to add additional 8507 * details to them. 8508 */ 8509 @Child(name = "imports", type = { 8510 CanonicalType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8511 @Description(shortDefinition = "Canonical URL of another capability statement this adds to", formalDefinition = "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.") 8512 protected List<CanonicalType> imports; 8513 8514 /** 8515 * Software that is covered by this capability statement. It is used when the 8516 * capability statement describes the capabilities of a particular software 8517 * version, independent of an installation. 8518 */ 8519 @Child(name = "software", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 8520 @Description(shortDefinition = "Software that is covered by this capability statement", formalDefinition = "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.") 8521 protected CapabilityStatementSoftwareComponent software; 8522 8523 /** 8524 * Identifies a specific implementation instance that is described by the 8525 * capability statement - i.e. a particular installation, rather than the 8526 * capabilities of a software program. 8527 */ 8528 @Child(name = "implementation", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 8529 @Description(shortDefinition = "If this describes a specific instance", formalDefinition = "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.") 8530 protected CapabilityStatementImplementationComponent implementation; 8531 8532 /** 8533 * The version of the FHIR specification that this CapabilityStatement describes 8534 * (which SHALL be the same as the FHIR version of the CapabilityStatement 8535 * itself). There is no default value. 8536 */ 8537 @Child(name = "fhirVersion", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 8538 @Description(shortDefinition = "FHIR Version the system supports", formalDefinition = "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.") 8539 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/FHIR-version") 8540 protected Enumeration<FHIRVersion> fhirVersion; 8541 8542 /** 8543 * A list of the formats supported by this implementation using their content 8544 * types. 8545 */ 8546 @Child(name = "format", type = { 8547 CodeType.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8548 @Description(shortDefinition = "formats supported (xml | json | ttl | mime type)", formalDefinition = "A list of the formats supported by this implementation using their content types.") 8549 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 8550 protected List<CodeType> format; 8551 8552 /** 8553 * A list of the patch formats supported by this implementation using their 8554 * content types. 8555 */ 8556 @Child(name = "patchFormat", type = { 8557 CodeType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8558 @Description(shortDefinition = "Patch formats supported", formalDefinition = "A list of the patch formats supported by this implementation using their content types.") 8559 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 8560 protected List<CodeType> patchFormat; 8561 8562 /** 8563 * A list of implementation guides that the server does (or should) support in 8564 * their entirety. 8565 */ 8566 @Child(name = "implementationGuide", type = { 8567 CanonicalType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8568 @Description(shortDefinition = "Implementation guides supported", formalDefinition = "A list of implementation guides that the server does (or should) support in their entirety.") 8569 protected List<CanonicalType> implementationGuide; 8570 8571 /** 8572 * A definition of the restful capabilities of the solution, if any. 8573 */ 8574 @Child(name = "rest", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8575 @Description(shortDefinition = "If the endpoint is a RESTful one", formalDefinition = "A definition of the restful capabilities of the solution, if any.") 8576 protected List<CapabilityStatementRestComponent> rest; 8577 8578 /** 8579 * A description of the messaging capabilities of the solution. 8580 */ 8581 @Child(name = "messaging", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8582 @Description(shortDefinition = "If messaging is supported", formalDefinition = "A description of the messaging capabilities of the solution.") 8583 protected List<CapabilityStatementMessagingComponent> messaging; 8584 8585 /** 8586 * A document definition. 8587 */ 8588 @Child(name = "document", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8589 @Description(shortDefinition = "Document definition", formalDefinition = "A document definition.") 8590 protected List<CapabilityStatementDocumentComponent> document; 8591 8592 private static final long serialVersionUID = -1050288843L; 8593 8594 /** 8595 * Constructor 8596 */ 8597 public CapabilityStatement() { 8598 super(); 8599 } 8600 8601 /** 8602 * Constructor 8603 */ 8604 public CapabilityStatement(Enumeration<PublicationStatus> status, DateTimeType date, 8605 Enumeration<CapabilityStatementKind> kind, Enumeration<FHIRVersion> fhirVersion) { 8606 super(); 8607 this.status = status; 8608 this.date = date; 8609 this.kind = kind; 8610 this.fhirVersion = fhirVersion; 8611 } 8612 8613 /** 8614 * @return {@link #url} (An absolute URI that is used to identify this 8615 * capability statement when it is referenced in a specification, model, 8616 * design or an instance; also called its canonical identifier. This 8617 * SHOULD be globally unique and SHOULD be a literal address at which at 8618 * which an authoritative instance of this capability statement is (or 8619 * will be) published. This URL can be the target of a canonical 8620 * reference. It SHALL remain the same when the capability statement is 8621 * stored on different servers.). This is the underlying object with id, 8622 * value and extensions. The accessor "getUrl" gives direct access to 8623 * the value 8624 */ 8625 public UriType getUrlElement() { 8626 if (this.url == null) 8627 if (Configuration.errorOnAutoCreate()) 8628 throw new Error("Attempt to auto-create CapabilityStatement.url"); 8629 else if (Configuration.doAutoCreate()) 8630 this.url = new UriType(); // bb 8631 return this.url; 8632 } 8633 8634 public boolean hasUrlElement() { 8635 return this.url != null && !this.url.isEmpty(); 8636 } 8637 8638 public boolean hasUrl() { 8639 return this.url != null && !this.url.isEmpty(); 8640 } 8641 8642 /** 8643 * @param value {@link #url} (An absolute URI that is used to identify this 8644 * capability statement when it is referenced in a specification, 8645 * model, design or an instance; also called its canonical 8646 * identifier. This SHOULD be globally unique and SHOULD be a 8647 * literal address at which at which an authoritative instance of 8648 * this capability statement is (or will be) published. This URL 8649 * can be the target of a canonical reference. It SHALL remain the 8650 * same when the capability statement is stored on different 8651 * servers.). This is the underlying object with id, value and 8652 * extensions. The accessor "getUrl" gives direct access to the 8653 * value 8654 */ 8655 public CapabilityStatement setUrlElement(UriType value) { 8656 this.url = value; 8657 return this; 8658 } 8659 8660 /** 8661 * @return An absolute URI that is used to identify this capability statement 8662 * when it is referenced in a specification, model, design or an 8663 * instance; also called its canonical identifier. This SHOULD be 8664 * globally unique and SHOULD be a literal address at which at which an 8665 * authoritative instance of this capability statement is (or will be) 8666 * published. This URL can be the target of a canonical reference. It 8667 * SHALL remain the same when the capability statement is stored on 8668 * different servers. 8669 */ 8670 public String getUrl() { 8671 return this.url == null ? null : this.url.getValue(); 8672 } 8673 8674 /** 8675 * @param value An absolute URI that is used to identify this capability 8676 * statement when it is referenced in a specification, model, 8677 * design or an instance; also called its canonical identifier. 8678 * This SHOULD be globally unique and SHOULD be a literal address 8679 * at which at which an authoritative instance of this capability 8680 * statement is (or will be) published. This URL can be the target 8681 * of a canonical reference. It SHALL remain the same when the 8682 * capability statement is stored on different servers. 8683 */ 8684 public CapabilityStatement setUrl(String value) { 8685 if (Utilities.noString(value)) 8686 this.url = null; 8687 else { 8688 if (this.url == null) 8689 this.url = new UriType(); 8690 this.url.setValue(value); 8691 } 8692 return this; 8693 } 8694 8695 /** 8696 * @return {@link #version} (The identifier that is used to identify this 8697 * version of the capability statement when it is referenced in a 8698 * specification, model, design or instance. This is an arbitrary value 8699 * managed by the capability statement author and is not expected to be 8700 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 8701 * if a managed version is not available. There is also no expectation 8702 * that versions can be placed in a lexicographical sequence.). This is 8703 * the underlying object with id, value and extensions. The accessor 8704 * "getVersion" gives direct access to the value 8705 */ 8706 public StringType getVersionElement() { 8707 if (this.version == null) 8708 if (Configuration.errorOnAutoCreate()) 8709 throw new Error("Attempt to auto-create CapabilityStatement.version"); 8710 else if (Configuration.doAutoCreate()) 8711 this.version = new StringType(); // bb 8712 return this.version; 8713 } 8714 8715 public boolean hasVersionElement() { 8716 return this.version != null && !this.version.isEmpty(); 8717 } 8718 8719 public boolean hasVersion() { 8720 return this.version != null && !this.version.isEmpty(); 8721 } 8722 8723 /** 8724 * @param value {@link #version} (The identifier that is used to identify this 8725 * version of the capability statement when it is referenced in a 8726 * specification, model, design or instance. This is an arbitrary 8727 * value managed by the capability statement author and is not 8728 * expected to be globally unique. For example, it might be a 8729 * timestamp (e.g. yyyymmdd) if a managed version is not available. 8730 * There is also no expectation that versions can be placed in a 8731 * lexicographical sequence.). This is the underlying object with 8732 * id, value and extensions. The accessor "getVersion" gives direct 8733 * access to the value 8734 */ 8735 public CapabilityStatement setVersionElement(StringType value) { 8736 this.version = value; 8737 return this; 8738 } 8739 8740 /** 8741 * @return The identifier that is used to identify this version of the 8742 * capability statement when it is referenced in a specification, model, 8743 * design or instance. This is an arbitrary value managed by the 8744 * capability statement author and is not expected to be globally 8745 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 8746 * managed version is not available. There is also no expectation that 8747 * versions can be placed in a lexicographical sequence. 8748 */ 8749 public String getVersion() { 8750 return this.version == null ? null : this.version.getValue(); 8751 } 8752 8753 /** 8754 * @param value The identifier that is used to identify this version of the 8755 * capability statement when it is referenced in a specification, 8756 * model, design or instance. This is an arbitrary value managed by 8757 * the capability statement author and is not expected to be 8758 * globally unique. For example, it might be a timestamp (e.g. 8759 * yyyymmdd) if a managed version is not available. There is also 8760 * no expectation that versions can be placed in a lexicographical 8761 * sequence. 8762 */ 8763 public CapabilityStatement setVersion(String value) { 8764 if (Utilities.noString(value)) 8765 this.version = null; 8766 else { 8767 if (this.version == null) 8768 this.version = new StringType(); 8769 this.version.setValue(value); 8770 } 8771 return this; 8772 } 8773 8774 /** 8775 * @return {@link #name} (A natural language name identifying the capability 8776 * statement. This name should be usable as an identifier for the module 8777 * by machine processing applications such as code generation.). This is 8778 * the underlying object with id, value and extensions. The accessor 8779 * "getName" gives direct access to the value 8780 */ 8781 public StringType getNameElement() { 8782 if (this.name == null) 8783 if (Configuration.errorOnAutoCreate()) 8784 throw new Error("Attempt to auto-create CapabilityStatement.name"); 8785 else if (Configuration.doAutoCreate()) 8786 this.name = new StringType(); // bb 8787 return this.name; 8788 } 8789 8790 public boolean hasNameElement() { 8791 return this.name != null && !this.name.isEmpty(); 8792 } 8793 8794 public boolean hasName() { 8795 return this.name != null && !this.name.isEmpty(); 8796 } 8797 8798 /** 8799 * @param value {@link #name} (A natural language name identifying the 8800 * capability statement. This name should be usable as an 8801 * identifier for the module by machine processing applications 8802 * such as code generation.). This is the underlying object with 8803 * id, value and extensions. The accessor "getName" gives direct 8804 * access to the value 8805 */ 8806 public CapabilityStatement setNameElement(StringType value) { 8807 this.name = value; 8808 return this; 8809 } 8810 8811 /** 8812 * @return A natural language name identifying the capability statement. This 8813 * name should be usable as an identifier for the module by machine 8814 * processing applications such as code generation. 8815 */ 8816 public String getName() { 8817 return this.name == null ? null : this.name.getValue(); 8818 } 8819 8820 /** 8821 * @param value A natural language name identifying the capability statement. 8822 * This name should be usable as an identifier for the module by 8823 * machine processing applications such as code generation. 8824 */ 8825 public CapabilityStatement setName(String value) { 8826 if (Utilities.noString(value)) 8827 this.name = null; 8828 else { 8829 if (this.name == null) 8830 this.name = new StringType(); 8831 this.name.setValue(value); 8832 } 8833 return this; 8834 } 8835 8836 /** 8837 * @return {@link #title} (A short, descriptive, user-friendly title for the 8838 * capability statement.). This is the underlying object with id, value 8839 * and extensions. The accessor "getTitle" gives direct access to the 8840 * value 8841 */ 8842 public StringType getTitleElement() { 8843 if (this.title == null) 8844 if (Configuration.errorOnAutoCreate()) 8845 throw new Error("Attempt to auto-create CapabilityStatement.title"); 8846 else if (Configuration.doAutoCreate()) 8847 this.title = new StringType(); // bb 8848 return this.title; 8849 } 8850 8851 public boolean hasTitleElement() { 8852 return this.title != null && !this.title.isEmpty(); 8853 } 8854 8855 public boolean hasTitle() { 8856 return this.title != null && !this.title.isEmpty(); 8857 } 8858 8859 /** 8860 * @param value {@link #title} (A short, descriptive, user-friendly title for 8861 * the capability statement.). This is the underlying object with 8862 * id, value and extensions. The accessor "getTitle" gives direct 8863 * access to the value 8864 */ 8865 public CapabilityStatement setTitleElement(StringType value) { 8866 this.title = value; 8867 return this; 8868 } 8869 8870 /** 8871 * @return A short, descriptive, user-friendly title for the capability 8872 * statement. 8873 */ 8874 public String getTitle() { 8875 return this.title == null ? null : this.title.getValue(); 8876 } 8877 8878 /** 8879 * @param value A short, descriptive, user-friendly title for the capability 8880 * statement. 8881 */ 8882 public CapabilityStatement setTitle(String value) { 8883 if (Utilities.noString(value)) 8884 this.title = null; 8885 else { 8886 if (this.title == null) 8887 this.title = new StringType(); 8888 this.title.setValue(value); 8889 } 8890 return this; 8891 } 8892 8893 /** 8894 * @return {@link #status} (The status of this capability statement. Enables 8895 * tracking the life-cycle of the content.). This is the underlying 8896 * object with id, value and extensions. The accessor "getStatus" gives 8897 * direct access to the value 8898 */ 8899 public Enumeration<PublicationStatus> getStatusElement() { 8900 if (this.status == null) 8901 if (Configuration.errorOnAutoCreate()) 8902 throw new Error("Attempt to auto-create CapabilityStatement.status"); 8903 else if (Configuration.doAutoCreate()) 8904 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 8905 return this.status; 8906 } 8907 8908 public boolean hasStatusElement() { 8909 return this.status != null && !this.status.isEmpty(); 8910 } 8911 8912 public boolean hasStatus() { 8913 return this.status != null && !this.status.isEmpty(); 8914 } 8915 8916 /** 8917 * @param value {@link #status} (The status of this capability statement. 8918 * Enables tracking the life-cycle of the content.). This is the 8919 * underlying object with id, value and extensions. The accessor 8920 * "getStatus" gives direct access to the value 8921 */ 8922 public CapabilityStatement setStatusElement(Enumeration<PublicationStatus> value) { 8923 this.status = value; 8924 return this; 8925 } 8926 8927 /** 8928 * @return The status of this capability statement. Enables tracking the 8929 * life-cycle of the content. 8930 */ 8931 public PublicationStatus getStatus() { 8932 return this.status == null ? null : this.status.getValue(); 8933 } 8934 8935 /** 8936 * @param value The status of this capability statement. Enables tracking the 8937 * life-cycle of the content. 8938 */ 8939 public CapabilityStatement setStatus(PublicationStatus value) { 8940 if (this.status == null) 8941 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 8942 this.status.setValue(value); 8943 return this; 8944 } 8945 8946 /** 8947 * @return {@link #experimental} (A Boolean value to indicate that this 8948 * capability statement is authored for testing purposes (or 8949 * education/evaluation/marketing) and is not intended to be used for 8950 * genuine usage.). This is the underlying object with id, value and 8951 * extensions. The accessor "getExperimental" gives direct access to the 8952 * value 8953 */ 8954 public BooleanType getExperimentalElement() { 8955 if (this.experimental == null) 8956 if (Configuration.errorOnAutoCreate()) 8957 throw new Error("Attempt to auto-create CapabilityStatement.experimental"); 8958 else if (Configuration.doAutoCreate()) 8959 this.experimental = new BooleanType(); // bb 8960 return this.experimental; 8961 } 8962 8963 public boolean hasExperimentalElement() { 8964 return this.experimental != null && !this.experimental.isEmpty(); 8965 } 8966 8967 public boolean hasExperimental() { 8968 return this.experimental != null && !this.experimental.isEmpty(); 8969 } 8970 8971 /** 8972 * @param value {@link #experimental} (A Boolean value to indicate that this 8973 * capability statement is authored for testing purposes (or 8974 * education/evaluation/marketing) and is not intended to be used 8975 * for genuine usage.). This is the underlying object with id, 8976 * value and extensions. The accessor "getExperimental" gives 8977 * direct access to the value 8978 */ 8979 public CapabilityStatement setExperimentalElement(BooleanType value) { 8980 this.experimental = value; 8981 return this; 8982 } 8983 8984 /** 8985 * @return A Boolean value to indicate that this capability statement is 8986 * authored for testing purposes (or education/evaluation/marketing) and 8987 * is not intended to be used for genuine usage. 8988 */ 8989 public boolean getExperimental() { 8990 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 8991 } 8992 8993 /** 8994 * @param value A Boolean value to indicate that this capability statement is 8995 * authored for testing purposes (or 8996 * education/evaluation/marketing) and is not intended to be used 8997 * for genuine usage. 8998 */ 8999 public CapabilityStatement setExperimental(boolean value) { 9000 if (this.experimental == null) 9001 this.experimental = new BooleanType(); 9002 this.experimental.setValue(value); 9003 return this; 9004 } 9005 9006 /** 9007 * @return {@link #date} (The date (and optionally time) when the capability 9008 * statement was published. The date must change when the business 9009 * version changes and it must change if the status code changes. In 9010 * addition, it should change when the substantive content of the 9011 * capability statement changes.). This is the underlying object with 9012 * id, value and extensions. The accessor "getDate" gives direct access 9013 * to the value 9014 */ 9015 public DateTimeType getDateElement() { 9016 if (this.date == null) 9017 if (Configuration.errorOnAutoCreate()) 9018 throw new Error("Attempt to auto-create CapabilityStatement.date"); 9019 else if (Configuration.doAutoCreate()) 9020 this.date = new DateTimeType(); // bb 9021 return this.date; 9022 } 9023 9024 public boolean hasDateElement() { 9025 return this.date != null && !this.date.isEmpty(); 9026 } 9027 9028 public boolean hasDate() { 9029 return this.date != null && !this.date.isEmpty(); 9030 } 9031 9032 /** 9033 * @param value {@link #date} (The date (and optionally time) when the 9034 * capability statement was published. The date must change when 9035 * the business version changes and it must change if the status 9036 * code changes. In addition, it should change when the substantive 9037 * content of the capability statement changes.). This is the 9038 * underlying object with id, value and extensions. The accessor 9039 * "getDate" gives direct access to the value 9040 */ 9041 public CapabilityStatement setDateElement(DateTimeType value) { 9042 this.date = value; 9043 return this; 9044 } 9045 9046 /** 9047 * @return The date (and optionally time) when the capability statement was 9048 * published. The date must change when the business version changes and 9049 * it must change if the status code changes. In addition, it should 9050 * change when the substantive content of the capability statement 9051 * changes. 9052 */ 9053 public Date getDate() { 9054 return this.date == null ? null : this.date.getValue(); 9055 } 9056 9057 /** 9058 * @param value The date (and optionally time) when the capability statement was 9059 * published. The date must change when the business version 9060 * changes and it must change if the status code changes. In 9061 * addition, it should change when the substantive content of the 9062 * capability statement changes. 9063 */ 9064 public CapabilityStatement setDate(Date value) { 9065 if (this.date == null) 9066 this.date = new DateTimeType(); 9067 this.date.setValue(value); 9068 return this; 9069 } 9070 9071 /** 9072 * @return {@link #publisher} (The name of the organization or individual that 9073 * published the capability statement.). This is the underlying object 9074 * with id, value and extensions. The accessor "getPublisher" gives 9075 * direct access to the value 9076 */ 9077 public StringType getPublisherElement() { 9078 if (this.publisher == null) 9079 if (Configuration.errorOnAutoCreate()) 9080 throw new Error("Attempt to auto-create CapabilityStatement.publisher"); 9081 else if (Configuration.doAutoCreate()) 9082 this.publisher = new StringType(); // bb 9083 return this.publisher; 9084 } 9085 9086 public boolean hasPublisherElement() { 9087 return this.publisher != null && !this.publisher.isEmpty(); 9088 } 9089 9090 public boolean hasPublisher() { 9091 return this.publisher != null && !this.publisher.isEmpty(); 9092 } 9093 9094 /** 9095 * @param value {@link #publisher} (The name of the organization or individual 9096 * that published the capability statement.). This is the 9097 * underlying object with id, value and extensions. The accessor 9098 * "getPublisher" gives direct access to the value 9099 */ 9100 public CapabilityStatement setPublisherElement(StringType value) { 9101 this.publisher = value; 9102 return this; 9103 } 9104 9105 /** 9106 * @return The name of the organization or individual that published the 9107 * capability statement. 9108 */ 9109 public String getPublisher() { 9110 return this.publisher == null ? null : this.publisher.getValue(); 9111 } 9112 9113 /** 9114 * @param value The name of the organization or individual that published the 9115 * capability statement. 9116 */ 9117 public CapabilityStatement setPublisher(String value) { 9118 if (Utilities.noString(value)) 9119 this.publisher = null; 9120 else { 9121 if (this.publisher == null) 9122 this.publisher = new StringType(); 9123 this.publisher.setValue(value); 9124 } 9125 return this; 9126 } 9127 9128 /** 9129 * @return {@link #contact} (Contact details to assist a user in finding and 9130 * communicating with the publisher.) 9131 */ 9132 public List<ContactDetail> getContact() { 9133 if (this.contact == null) 9134 this.contact = new ArrayList<ContactDetail>(); 9135 return this.contact; 9136 } 9137 9138 /** 9139 * @return Returns a reference to <code>this</code> for easy method chaining 9140 */ 9141 public CapabilityStatement setContact(List<ContactDetail> theContact) { 9142 this.contact = theContact; 9143 return this; 9144 } 9145 9146 public boolean hasContact() { 9147 if (this.contact == null) 9148 return false; 9149 for (ContactDetail item : this.contact) 9150 if (!item.isEmpty()) 9151 return true; 9152 return false; 9153 } 9154 9155 public ContactDetail addContact() { // 3 9156 ContactDetail t = new ContactDetail(); 9157 if (this.contact == null) 9158 this.contact = new ArrayList<ContactDetail>(); 9159 this.contact.add(t); 9160 return t; 9161 } 9162 9163 public CapabilityStatement addContact(ContactDetail t) { // 3 9164 if (t == null) 9165 return this; 9166 if (this.contact == null) 9167 this.contact = new ArrayList<ContactDetail>(); 9168 this.contact.add(t); 9169 return this; 9170 } 9171 9172 /** 9173 * @return The first repetition of repeating field {@link #contact}, creating it 9174 * if it does not already exist 9175 */ 9176 public ContactDetail getContactFirstRep() { 9177 if (getContact().isEmpty()) { 9178 addContact(); 9179 } 9180 return getContact().get(0); 9181 } 9182 9183 /** 9184 * @return {@link #description} (A free text natural language description of the 9185 * capability statement from a consumer's perspective. Typically, this 9186 * is used when the capability statement describes a desired rather than 9187 * an actual solution, for example as a formal expression of 9188 * requirements as part of an RFP.). This is the underlying object with 9189 * id, value and extensions. The accessor "getDescription" gives direct 9190 * access to the value 9191 */ 9192 public MarkdownType getDescriptionElement() { 9193 if (this.description == null) 9194 if (Configuration.errorOnAutoCreate()) 9195 throw new Error("Attempt to auto-create CapabilityStatement.description"); 9196 else if (Configuration.doAutoCreate()) 9197 this.description = new MarkdownType(); // bb 9198 return this.description; 9199 } 9200 9201 public boolean hasDescriptionElement() { 9202 return this.description != null && !this.description.isEmpty(); 9203 } 9204 9205 public boolean hasDescription() { 9206 return this.description != null && !this.description.isEmpty(); 9207 } 9208 9209 /** 9210 * @param value {@link #description} (A free text natural language description 9211 * of the capability statement from a consumer's perspective. 9212 * Typically, this is used when the capability statement describes 9213 * a desired rather than an actual solution, for example as a 9214 * formal expression of requirements as part of an RFP.). This is 9215 * the underlying object with id, value and extensions. The 9216 * accessor "getDescription" gives direct access to the value 9217 */ 9218 public CapabilityStatement setDescriptionElement(MarkdownType value) { 9219 this.description = value; 9220 return this; 9221 } 9222 9223 /** 9224 * @return A free text natural language description of the capability statement 9225 * from a consumer's perspective. Typically, this is used when the 9226 * capability statement describes a desired rather than an actual 9227 * solution, for example as a formal expression of requirements as part 9228 * of an RFP. 9229 */ 9230 public String getDescription() { 9231 return this.description == null ? null : this.description.getValue(); 9232 } 9233 9234 /** 9235 * @param value A free text natural language description of the capability 9236 * statement from a consumer's perspective. Typically, this is used 9237 * when the capability statement describes a desired rather than an 9238 * actual solution, for example as a formal expression of 9239 * requirements as part of an RFP. 9240 */ 9241 public CapabilityStatement setDescription(String value) { 9242 if (value == null) 9243 this.description = null; 9244 else { 9245 if (this.description == null) 9246 this.description = new MarkdownType(); 9247 this.description.setValue(value); 9248 } 9249 return this; 9250 } 9251 9252 /** 9253 * @return {@link #useContext} (The content was developed with a focus and 9254 * intent of supporting the contexts that are listed. These contexts may 9255 * be general categories (gender, age, ...) or may be references to 9256 * specific programs (insurance plans, studies, ...) and may be used to 9257 * assist with indexing and searching for appropriate capability 9258 * statement instances.) 9259 */ 9260 public List<UsageContext> getUseContext() { 9261 if (this.useContext == null) 9262 this.useContext = new ArrayList<UsageContext>(); 9263 return this.useContext; 9264 } 9265 9266 /** 9267 * @return Returns a reference to <code>this</code> for easy method chaining 9268 */ 9269 public CapabilityStatement setUseContext(List<UsageContext> theUseContext) { 9270 this.useContext = theUseContext; 9271 return this; 9272 } 9273 9274 public boolean hasUseContext() { 9275 if (this.useContext == null) 9276 return false; 9277 for (UsageContext item : this.useContext) 9278 if (!item.isEmpty()) 9279 return true; 9280 return false; 9281 } 9282 9283 public UsageContext addUseContext() { // 3 9284 UsageContext t = new UsageContext(); 9285 if (this.useContext == null) 9286 this.useContext = new ArrayList<UsageContext>(); 9287 this.useContext.add(t); 9288 return t; 9289 } 9290 9291 public CapabilityStatement addUseContext(UsageContext t) { // 3 9292 if (t == null) 9293 return this; 9294 if (this.useContext == null) 9295 this.useContext = new ArrayList<UsageContext>(); 9296 this.useContext.add(t); 9297 return this; 9298 } 9299 9300 /** 9301 * @return The first repetition of repeating field {@link #useContext}, creating 9302 * it if it does not already exist 9303 */ 9304 public UsageContext getUseContextFirstRep() { 9305 if (getUseContext().isEmpty()) { 9306 addUseContext(); 9307 } 9308 return getUseContext().get(0); 9309 } 9310 9311 /** 9312 * @return {@link #jurisdiction} (A legal or geographic region in which the 9313 * capability statement is intended to be used.) 9314 */ 9315 public List<CodeableConcept> getJurisdiction() { 9316 if (this.jurisdiction == null) 9317 this.jurisdiction = new ArrayList<CodeableConcept>(); 9318 return this.jurisdiction; 9319 } 9320 9321 /** 9322 * @return Returns a reference to <code>this</code> for easy method chaining 9323 */ 9324 public CapabilityStatement setJurisdiction(List<CodeableConcept> theJurisdiction) { 9325 this.jurisdiction = theJurisdiction; 9326 return this; 9327 } 9328 9329 public boolean hasJurisdiction() { 9330 if (this.jurisdiction == null) 9331 return false; 9332 for (CodeableConcept item : this.jurisdiction) 9333 if (!item.isEmpty()) 9334 return true; 9335 return false; 9336 } 9337 9338 public CodeableConcept addJurisdiction() { // 3 9339 CodeableConcept t = new CodeableConcept(); 9340 if (this.jurisdiction == null) 9341 this.jurisdiction = new ArrayList<CodeableConcept>(); 9342 this.jurisdiction.add(t); 9343 return t; 9344 } 9345 9346 public CapabilityStatement addJurisdiction(CodeableConcept t) { // 3 9347 if (t == null) 9348 return this; 9349 if (this.jurisdiction == null) 9350 this.jurisdiction = new ArrayList<CodeableConcept>(); 9351 this.jurisdiction.add(t); 9352 return this; 9353 } 9354 9355 /** 9356 * @return The first repetition of repeating field {@link #jurisdiction}, 9357 * creating it if it does not already exist 9358 */ 9359 public CodeableConcept getJurisdictionFirstRep() { 9360 if (getJurisdiction().isEmpty()) { 9361 addJurisdiction(); 9362 } 9363 return getJurisdiction().get(0); 9364 } 9365 9366 /** 9367 * @return {@link #purpose} (Explanation of why this capability statement is 9368 * needed and why it has been designed as it has.). This is the 9369 * underlying object with id, value and extensions. The accessor 9370 * "getPurpose" gives direct access to the value 9371 */ 9372 public MarkdownType getPurposeElement() { 9373 if (this.purpose == null) 9374 if (Configuration.errorOnAutoCreate()) 9375 throw new Error("Attempt to auto-create CapabilityStatement.purpose"); 9376 else if (Configuration.doAutoCreate()) 9377 this.purpose = new MarkdownType(); // bb 9378 return this.purpose; 9379 } 9380 9381 public boolean hasPurposeElement() { 9382 return this.purpose != null && !this.purpose.isEmpty(); 9383 } 9384 9385 public boolean hasPurpose() { 9386 return this.purpose != null && !this.purpose.isEmpty(); 9387 } 9388 9389 /** 9390 * @param value {@link #purpose} (Explanation of why this capability statement 9391 * is needed and why it has been designed as it has.). This is the 9392 * underlying object with id, value and extensions. The accessor 9393 * "getPurpose" gives direct access to the value 9394 */ 9395 public CapabilityStatement setPurposeElement(MarkdownType value) { 9396 this.purpose = value; 9397 return this; 9398 } 9399 9400 /** 9401 * @return Explanation of why this capability statement is needed and why it has 9402 * been designed as it has. 9403 */ 9404 public String getPurpose() { 9405 return this.purpose == null ? null : this.purpose.getValue(); 9406 } 9407 9408 /** 9409 * @param value Explanation of why this capability statement is needed and why 9410 * it has been designed as it has. 9411 */ 9412 public CapabilityStatement setPurpose(String value) { 9413 if (value == null) 9414 this.purpose = null; 9415 else { 9416 if (this.purpose == null) 9417 this.purpose = new MarkdownType(); 9418 this.purpose.setValue(value); 9419 } 9420 return this; 9421 } 9422 9423 /** 9424 * @return {@link #copyright} (A copyright statement relating to the capability 9425 * statement and/or its contents. Copyright statements are generally 9426 * legal restrictions on the use and publishing of the capability 9427 * statement.). This is the underlying object with id, value and 9428 * extensions. The accessor "getCopyright" gives direct access to the 9429 * value 9430 */ 9431 public MarkdownType getCopyrightElement() { 9432 if (this.copyright == null) 9433 if (Configuration.errorOnAutoCreate()) 9434 throw new Error("Attempt to auto-create CapabilityStatement.copyright"); 9435 else if (Configuration.doAutoCreate()) 9436 this.copyright = new MarkdownType(); // bb 9437 return this.copyright; 9438 } 9439 9440 public boolean hasCopyrightElement() { 9441 return this.copyright != null && !this.copyright.isEmpty(); 9442 } 9443 9444 public boolean hasCopyright() { 9445 return this.copyright != null && !this.copyright.isEmpty(); 9446 } 9447 9448 /** 9449 * @param value {@link #copyright} (A copyright statement relating to the 9450 * capability statement and/or its contents. Copyright statements 9451 * are generally legal restrictions on the use and publishing of 9452 * the capability statement.). This is the underlying object with 9453 * id, value and extensions. The accessor "getCopyright" gives 9454 * direct access to the value 9455 */ 9456 public CapabilityStatement setCopyrightElement(MarkdownType value) { 9457 this.copyright = value; 9458 return this; 9459 } 9460 9461 /** 9462 * @return A copyright statement relating to the capability statement and/or its 9463 * contents. Copyright statements are generally legal restrictions on 9464 * the use and publishing of the capability statement. 9465 */ 9466 public String getCopyright() { 9467 return this.copyright == null ? null : this.copyright.getValue(); 9468 } 9469 9470 /** 9471 * @param value A copyright statement relating to the capability statement 9472 * and/or its contents. Copyright statements are generally legal 9473 * restrictions on the use and publishing of the capability 9474 * statement. 9475 */ 9476 public CapabilityStatement setCopyright(String value) { 9477 if (value == null) 9478 this.copyright = null; 9479 else { 9480 if (this.copyright == null) 9481 this.copyright = new MarkdownType(); 9482 this.copyright.setValue(value); 9483 } 9484 return this; 9485 } 9486 9487 /** 9488 * @return {@link #kind} (The way that this statement is intended to be used, to 9489 * describe an actual running instance of software, a particular product 9490 * (kind, not instance of software) or a class of implementation (e.g. a 9491 * desired purchase).). This is the underlying object with id, value and 9492 * extensions. The accessor "getKind" gives direct access to the value 9493 */ 9494 public Enumeration<CapabilityStatementKind> getKindElement() { 9495 if (this.kind == null) 9496 if (Configuration.errorOnAutoCreate()) 9497 throw new Error("Attempt to auto-create CapabilityStatement.kind"); 9498 else if (Configuration.doAutoCreate()) 9499 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); // bb 9500 return this.kind; 9501 } 9502 9503 public boolean hasKindElement() { 9504 return this.kind != null && !this.kind.isEmpty(); 9505 } 9506 9507 public boolean hasKind() { 9508 return this.kind != null && !this.kind.isEmpty(); 9509 } 9510 9511 /** 9512 * @param value {@link #kind} (The way that this statement is intended to be 9513 * used, to describe an actual running instance of software, a 9514 * particular product (kind, not instance of software) or a class 9515 * of implementation (e.g. a desired purchase).). This is the 9516 * underlying object with id, value and extensions. The accessor 9517 * "getKind" gives direct access to the value 9518 */ 9519 public CapabilityStatement setKindElement(Enumeration<CapabilityStatementKind> value) { 9520 this.kind = value; 9521 return this; 9522 } 9523 9524 /** 9525 * @return The way that this statement is intended to be used, to describe an 9526 * actual running instance of software, a particular product (kind, not 9527 * instance of software) or a class of implementation (e.g. a desired 9528 * purchase). 9529 */ 9530 public CapabilityStatementKind getKind() { 9531 return this.kind == null ? null : this.kind.getValue(); 9532 } 9533 9534 /** 9535 * @param value The way that this statement is intended to be used, to describe 9536 * an actual running instance of software, a particular product 9537 * (kind, not instance of software) or a class of implementation 9538 * (e.g. a desired purchase). 9539 */ 9540 public CapabilityStatement setKind(CapabilityStatementKind value) { 9541 if (this.kind == null) 9542 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); 9543 this.kind.setValue(value); 9544 return this; 9545 } 9546 9547 /** 9548 * @return {@link #instantiates} (Reference to a canonical URL of another 9549 * CapabilityStatement that this software implements. This capability 9550 * statement is a published API description that corresponds to a 9551 * business service. The server may actually implement a subset of the 9552 * capability statement it claims to implement, so the capability 9553 * statement must specify the full capability details.) 9554 */ 9555 public List<CanonicalType> getInstantiates() { 9556 if (this.instantiates == null) 9557 this.instantiates = new ArrayList<CanonicalType>(); 9558 return this.instantiates; 9559 } 9560 9561 /** 9562 * @return Returns a reference to <code>this</code> for easy method chaining 9563 */ 9564 public CapabilityStatement setInstantiates(List<CanonicalType> theInstantiates) { 9565 this.instantiates = theInstantiates; 9566 return this; 9567 } 9568 9569 public boolean hasInstantiates() { 9570 if (this.instantiates == null) 9571 return false; 9572 for (CanonicalType item : this.instantiates) 9573 if (!item.isEmpty()) 9574 return true; 9575 return false; 9576 } 9577 9578 /** 9579 * @return {@link #instantiates} (Reference to a canonical URL of another 9580 * CapabilityStatement that this software implements. This capability 9581 * statement is a published API description that corresponds to a 9582 * business service. The server may actually implement a subset of the 9583 * capability statement it claims to implement, so the capability 9584 * statement must specify the full capability details.) 9585 */ 9586 public CanonicalType addInstantiatesElement() {// 2 9587 CanonicalType t = new CanonicalType(); 9588 if (this.instantiates == null) 9589 this.instantiates = new ArrayList<CanonicalType>(); 9590 this.instantiates.add(t); 9591 return t; 9592 } 9593 9594 /** 9595 * @param value {@link #instantiates} (Reference to a canonical URL of another 9596 * CapabilityStatement that this software implements. This 9597 * capability statement is a published API description that 9598 * corresponds to a business service. The server may actually 9599 * implement a subset of the capability statement it claims to 9600 * implement, so the capability statement must specify the full 9601 * capability details.) 9602 */ 9603 public CapabilityStatement addInstantiates(String value) { // 1 9604 CanonicalType t = new CanonicalType(); 9605 t.setValue(value); 9606 if (this.instantiates == null) 9607 this.instantiates = new ArrayList<CanonicalType>(); 9608 this.instantiates.add(t); 9609 return this; 9610 } 9611 9612 /** 9613 * @param value {@link #instantiates} (Reference to a canonical URL of another 9614 * CapabilityStatement that this software implements. This 9615 * capability statement is a published API description that 9616 * corresponds to a business service. The server may actually 9617 * implement a subset of the capability statement it claims to 9618 * implement, so the capability statement must specify the full 9619 * capability details.) 9620 */ 9621 public boolean hasInstantiates(String value) { 9622 if (this.instantiates == null) 9623 return false; 9624 for (CanonicalType v : this.instantiates) 9625 if (v.getValue().equals(value)) // canonical(CapabilityStatement) 9626 return true; 9627 return false; 9628 } 9629 9630 /** 9631 * @return {@link #imports} (Reference to a canonical URL of another 9632 * CapabilityStatement that this software adds to. The capability 9633 * statement automatically includes everything in the other statement, 9634 * and it is not duplicated, though the server may repeat the same 9635 * resources, interactions and operations to add additional details to 9636 * them.) 9637 */ 9638 public List<CanonicalType> getImports() { 9639 if (this.imports == null) 9640 this.imports = new ArrayList<CanonicalType>(); 9641 return this.imports; 9642 } 9643 9644 /** 9645 * @return Returns a reference to <code>this</code> for easy method chaining 9646 */ 9647 public CapabilityStatement setImports(List<CanonicalType> theImports) { 9648 this.imports = theImports; 9649 return this; 9650 } 9651 9652 public boolean hasImports() { 9653 if (this.imports == null) 9654 return false; 9655 for (CanonicalType item : this.imports) 9656 if (!item.isEmpty()) 9657 return true; 9658 return false; 9659 } 9660 9661 /** 9662 * @return {@link #imports} (Reference to a canonical URL of another 9663 * CapabilityStatement that this software adds to. The capability 9664 * statement automatically includes everything in the other statement, 9665 * and it is not duplicated, though the server may repeat the same 9666 * resources, interactions and operations to add additional details to 9667 * them.) 9668 */ 9669 public CanonicalType addImportsElement() {// 2 9670 CanonicalType t = new CanonicalType(); 9671 if (this.imports == null) 9672 this.imports = new ArrayList<CanonicalType>(); 9673 this.imports.add(t); 9674 return t; 9675 } 9676 9677 /** 9678 * @param value {@link #imports} (Reference to a canonical URL of another 9679 * CapabilityStatement that this software adds to. The capability 9680 * statement automatically includes everything in the other 9681 * statement, and it is not duplicated, though the server may 9682 * repeat the same resources, interactions and operations to add 9683 * additional details to them.) 9684 */ 9685 public CapabilityStatement addImports(String value) { // 1 9686 CanonicalType t = new CanonicalType(); 9687 t.setValue(value); 9688 if (this.imports == null) 9689 this.imports = new ArrayList<CanonicalType>(); 9690 this.imports.add(t); 9691 return this; 9692 } 9693 9694 /** 9695 * @param value {@link #imports} (Reference to a canonical URL of another 9696 * CapabilityStatement that this software adds to. The capability 9697 * statement automatically includes everything in the other 9698 * statement, and it is not duplicated, though the server may 9699 * repeat the same resources, interactions and operations to add 9700 * additional details to them.) 9701 */ 9702 public boolean hasImports(String value) { 9703 if (this.imports == null) 9704 return false; 9705 for (CanonicalType v : this.imports) 9706 if (v.getValue().equals(value)) // canonical(CapabilityStatement) 9707 return true; 9708 return false; 9709 } 9710 9711 /** 9712 * @return {@link #software} (Software that is covered by this capability 9713 * statement. It is used when the capability statement describes the 9714 * capabilities of a particular software version, independent of an 9715 * installation.) 9716 */ 9717 public CapabilityStatementSoftwareComponent getSoftware() { 9718 if (this.software == null) 9719 if (Configuration.errorOnAutoCreate()) 9720 throw new Error("Attempt to auto-create CapabilityStatement.software"); 9721 else if (Configuration.doAutoCreate()) 9722 this.software = new CapabilityStatementSoftwareComponent(); // cc 9723 return this.software; 9724 } 9725 9726 public boolean hasSoftware() { 9727 return this.software != null && !this.software.isEmpty(); 9728 } 9729 9730 /** 9731 * @param value {@link #software} (Software that is covered by this capability 9732 * statement. It is used when the capability statement describes 9733 * the capabilities of a particular software version, independent 9734 * of an installation.) 9735 */ 9736 public CapabilityStatement setSoftware(CapabilityStatementSoftwareComponent value) { 9737 this.software = value; 9738 return this; 9739 } 9740 9741 /** 9742 * @return {@link #implementation} (Identifies a specific implementation 9743 * instance that is described by the capability statement - i.e. a 9744 * particular installation, rather than the capabilities of a software 9745 * program.) 9746 */ 9747 public CapabilityStatementImplementationComponent getImplementation() { 9748 if (this.implementation == null) 9749 if (Configuration.errorOnAutoCreate()) 9750 throw new Error("Attempt to auto-create CapabilityStatement.implementation"); 9751 else if (Configuration.doAutoCreate()) 9752 this.implementation = new CapabilityStatementImplementationComponent(); // cc 9753 return this.implementation; 9754 } 9755 9756 public boolean hasImplementation() { 9757 return this.implementation != null && !this.implementation.isEmpty(); 9758 } 9759 9760 /** 9761 * @param value {@link #implementation} (Identifies a specific implementation 9762 * instance that is described by the capability statement - i.e. a 9763 * particular installation, rather than the capabilities of a 9764 * software program.) 9765 */ 9766 public CapabilityStatement setImplementation(CapabilityStatementImplementationComponent value) { 9767 this.implementation = value; 9768 return this; 9769 } 9770 9771 /** 9772 * @return {@link #fhirVersion} (The version of the FHIR specification that this 9773 * CapabilityStatement describes (which SHALL be the same as the FHIR 9774 * version of the CapabilityStatement itself). There is no default 9775 * value.). This is the underlying object with id, value and extensions. 9776 * The accessor "getFhirVersion" gives direct access to the value 9777 */ 9778 public Enumeration<FHIRVersion> getFhirVersionElement() { 9779 if (this.fhirVersion == null) 9780 if (Configuration.errorOnAutoCreate()) 9781 throw new Error("Attempt to auto-create CapabilityStatement.fhirVersion"); 9782 else if (Configuration.doAutoCreate()) 9783 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); // bb 9784 return this.fhirVersion; 9785 } 9786 9787 public boolean hasFhirVersionElement() { 9788 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 9789 } 9790 9791 public boolean hasFhirVersion() { 9792 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 9793 } 9794 9795 /** 9796 * @param value {@link #fhirVersion} (The version of the FHIR specification that 9797 * this CapabilityStatement describes (which SHALL be the same as 9798 * the FHIR version of the CapabilityStatement itself). There is no 9799 * default value.). This is the underlying object with id, value 9800 * and extensions. The accessor "getFhirVersion" gives direct 9801 * access to the value 9802 */ 9803 public CapabilityStatement setFhirVersionElement(Enumeration<FHIRVersion> value) { 9804 this.fhirVersion = value; 9805 return this; 9806 } 9807 9808 /** 9809 * @return The version of the FHIR specification that this CapabilityStatement 9810 * describes (which SHALL be the same as the FHIR version of the 9811 * CapabilityStatement itself). There is no default value. 9812 */ 9813 public FHIRVersion getFhirVersion() { 9814 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 9815 } 9816 9817 /** 9818 * @param value The version of the FHIR specification that this 9819 * CapabilityStatement describes (which SHALL be the same as the 9820 * FHIR version of the CapabilityStatement itself). There is no 9821 * default value. 9822 */ 9823 public CapabilityStatement setFhirVersion(FHIRVersion value) { 9824 if (this.fhirVersion == null) 9825 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 9826 this.fhirVersion.setValue(value); 9827 return this; 9828 } 9829 9830 /** 9831 * @return {@link #format} (A list of the formats supported by this 9832 * implementation using their content types.) 9833 */ 9834 public List<CodeType> getFormat() { 9835 if (this.format == null) 9836 this.format = new ArrayList<CodeType>(); 9837 return this.format; 9838 } 9839 9840 /** 9841 * @return Returns a reference to <code>this</code> for easy method chaining 9842 */ 9843 public CapabilityStatement setFormat(List<CodeType> theFormat) { 9844 this.format = theFormat; 9845 return this; 9846 } 9847 9848 public boolean hasFormat() { 9849 if (this.format == null) 9850 return false; 9851 for (CodeType item : this.format) 9852 if (!item.isEmpty()) 9853 return true; 9854 return false; 9855 } 9856 9857 /** 9858 * @return {@link #format} (A list of the formats supported by this 9859 * implementation using their content types.) 9860 */ 9861 public CodeType addFormatElement() {// 2 9862 CodeType t = new CodeType(); 9863 if (this.format == null) 9864 this.format = new ArrayList<CodeType>(); 9865 this.format.add(t); 9866 return t; 9867 } 9868 9869 /** 9870 * @param value {@link #format} (A list of the formats supported by this 9871 * implementation using their content types.) 9872 */ 9873 public CapabilityStatement addFormat(String value) { // 1 9874 CodeType t = new CodeType(); 9875 t.setValue(value); 9876 if (this.format == null) 9877 this.format = new ArrayList<CodeType>(); 9878 this.format.add(t); 9879 return this; 9880 } 9881 9882 /** 9883 * @param value {@link #format} (A list of the formats supported by this 9884 * implementation using their content types.) 9885 */ 9886 public boolean hasFormat(String value) { 9887 if (this.format == null) 9888 return false; 9889 for (CodeType v : this.format) 9890 if (v.getValue().equals(value)) // code 9891 return true; 9892 return false; 9893 } 9894 9895 /** 9896 * @return {@link #patchFormat} (A list of the patch formats supported by this 9897 * implementation using their content types.) 9898 */ 9899 public List<CodeType> getPatchFormat() { 9900 if (this.patchFormat == null) 9901 this.patchFormat = new ArrayList<CodeType>(); 9902 return this.patchFormat; 9903 } 9904 9905 /** 9906 * @return Returns a reference to <code>this</code> for easy method chaining 9907 */ 9908 public CapabilityStatement setPatchFormat(List<CodeType> thePatchFormat) { 9909 this.patchFormat = thePatchFormat; 9910 return this; 9911 } 9912 9913 public boolean hasPatchFormat() { 9914 if (this.patchFormat == null) 9915 return false; 9916 for (CodeType item : this.patchFormat) 9917 if (!item.isEmpty()) 9918 return true; 9919 return false; 9920 } 9921 9922 /** 9923 * @return {@link #patchFormat} (A list of the patch formats supported by this 9924 * implementation using their content types.) 9925 */ 9926 public CodeType addPatchFormatElement() {// 2 9927 CodeType t = new CodeType(); 9928 if (this.patchFormat == null) 9929 this.patchFormat = new ArrayList<CodeType>(); 9930 this.patchFormat.add(t); 9931 return t; 9932 } 9933 9934 /** 9935 * @param value {@link #patchFormat} (A list of the patch formats supported by 9936 * this implementation using their content types.) 9937 */ 9938 public CapabilityStatement addPatchFormat(String value) { // 1 9939 CodeType t = new CodeType(); 9940 t.setValue(value); 9941 if (this.patchFormat == null) 9942 this.patchFormat = new ArrayList<CodeType>(); 9943 this.patchFormat.add(t); 9944 return this; 9945 } 9946 9947 /** 9948 * @param value {@link #patchFormat} (A list of the patch formats supported by 9949 * this implementation using their content types.) 9950 */ 9951 public boolean hasPatchFormat(String value) { 9952 if (this.patchFormat == null) 9953 return false; 9954 for (CodeType v : this.patchFormat) 9955 if (v.getValue().equals(value)) // code 9956 return true; 9957 return false; 9958 } 9959 9960 /** 9961 * @return {@link #implementationGuide} (A list of implementation guides that 9962 * the server does (or should) support in their entirety.) 9963 */ 9964 public List<CanonicalType> getImplementationGuide() { 9965 if (this.implementationGuide == null) 9966 this.implementationGuide = new ArrayList<CanonicalType>(); 9967 return this.implementationGuide; 9968 } 9969 9970 /** 9971 * @return Returns a reference to <code>this</code> for easy method chaining 9972 */ 9973 public CapabilityStatement setImplementationGuide(List<CanonicalType> theImplementationGuide) { 9974 this.implementationGuide = theImplementationGuide; 9975 return this; 9976 } 9977 9978 public boolean hasImplementationGuide() { 9979 if (this.implementationGuide == null) 9980 return false; 9981 for (CanonicalType item : this.implementationGuide) 9982 if (!item.isEmpty()) 9983 return true; 9984 return false; 9985 } 9986 9987 /** 9988 * @return {@link #implementationGuide} (A list of implementation guides that 9989 * the server does (or should) support in their entirety.) 9990 */ 9991 public CanonicalType addImplementationGuideElement() {// 2 9992 CanonicalType t = new CanonicalType(); 9993 if (this.implementationGuide == null) 9994 this.implementationGuide = new ArrayList<CanonicalType>(); 9995 this.implementationGuide.add(t); 9996 return t; 9997 } 9998 9999 /** 10000 * @param value {@link #implementationGuide} (A list of implementation guides 10001 * that the server does (or should) support in their entirety.) 10002 */ 10003 public CapabilityStatement addImplementationGuide(String value) { // 1 10004 CanonicalType t = new CanonicalType(); 10005 t.setValue(value); 10006 if (this.implementationGuide == null) 10007 this.implementationGuide = new ArrayList<CanonicalType>(); 10008 this.implementationGuide.add(t); 10009 return this; 10010 } 10011 10012 /** 10013 * @param value {@link #implementationGuide} (A list of implementation guides 10014 * that the server does (or should) support in their entirety.) 10015 */ 10016 public boolean hasImplementationGuide(String value) { 10017 if (this.implementationGuide == null) 10018 return false; 10019 for (CanonicalType v : this.implementationGuide) 10020 if (v.getValue().equals(value)) // canonical(ImplementationGuide) 10021 return true; 10022 return false; 10023 } 10024 10025 /** 10026 * @return {@link #rest} (A definition of the restful capabilities of the 10027 * solution, if any.) 10028 */ 10029 public List<CapabilityStatementRestComponent> getRest() { 10030 if (this.rest == null) 10031 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 10032 return this.rest; 10033 } 10034 10035 /** 10036 * @return Returns a reference to <code>this</code> for easy method chaining 10037 */ 10038 public CapabilityStatement setRest(List<CapabilityStatementRestComponent> theRest) { 10039 this.rest = theRest; 10040 return this; 10041 } 10042 10043 public boolean hasRest() { 10044 if (this.rest == null) 10045 return false; 10046 for (CapabilityStatementRestComponent item : this.rest) 10047 if (!item.isEmpty()) 10048 return true; 10049 return false; 10050 } 10051 10052 public CapabilityStatementRestComponent addRest() { // 3 10053 CapabilityStatementRestComponent t = new CapabilityStatementRestComponent(); 10054 if (this.rest == null) 10055 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 10056 this.rest.add(t); 10057 return t; 10058 } 10059 10060 public CapabilityStatement addRest(CapabilityStatementRestComponent t) { // 3 10061 if (t == null) 10062 return this; 10063 if (this.rest == null) 10064 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 10065 this.rest.add(t); 10066 return this; 10067 } 10068 10069 /** 10070 * @return The first repetition of repeating field {@link #rest}, creating it if 10071 * it does not already exist 10072 */ 10073 public CapabilityStatementRestComponent getRestFirstRep() { 10074 if (getRest().isEmpty()) { 10075 addRest(); 10076 } 10077 return getRest().get(0); 10078 } 10079 10080 /** 10081 * @return {@link #messaging} (A description of the messaging capabilities of 10082 * the solution.) 10083 */ 10084 public List<CapabilityStatementMessagingComponent> getMessaging() { 10085 if (this.messaging == null) 10086 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10087 return this.messaging; 10088 } 10089 10090 /** 10091 * @return Returns a reference to <code>this</code> for easy method chaining 10092 */ 10093 public CapabilityStatement setMessaging(List<CapabilityStatementMessagingComponent> theMessaging) { 10094 this.messaging = theMessaging; 10095 return this; 10096 } 10097 10098 public boolean hasMessaging() { 10099 if (this.messaging == null) 10100 return false; 10101 for (CapabilityStatementMessagingComponent item : this.messaging) 10102 if (!item.isEmpty()) 10103 return true; 10104 return false; 10105 } 10106 10107 public CapabilityStatementMessagingComponent addMessaging() { // 3 10108 CapabilityStatementMessagingComponent t = new CapabilityStatementMessagingComponent(); 10109 if (this.messaging == null) 10110 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10111 this.messaging.add(t); 10112 return t; 10113 } 10114 10115 public CapabilityStatement addMessaging(CapabilityStatementMessagingComponent t) { // 3 10116 if (t == null) 10117 return this; 10118 if (this.messaging == null) 10119 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10120 this.messaging.add(t); 10121 return this; 10122 } 10123 10124 /** 10125 * @return The first repetition of repeating field {@link #messaging}, creating 10126 * it if it does not already exist 10127 */ 10128 public CapabilityStatementMessagingComponent getMessagingFirstRep() { 10129 if (getMessaging().isEmpty()) { 10130 addMessaging(); 10131 } 10132 return getMessaging().get(0); 10133 } 10134 10135 /** 10136 * @return {@link #document} (A document definition.) 10137 */ 10138 public List<CapabilityStatementDocumentComponent> getDocument() { 10139 if (this.document == null) 10140 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10141 return this.document; 10142 } 10143 10144 /** 10145 * @return Returns a reference to <code>this</code> for easy method chaining 10146 */ 10147 public CapabilityStatement setDocument(List<CapabilityStatementDocumentComponent> theDocument) { 10148 this.document = theDocument; 10149 return this; 10150 } 10151 10152 public boolean hasDocument() { 10153 if (this.document == null) 10154 return false; 10155 for (CapabilityStatementDocumentComponent item : this.document) 10156 if (!item.isEmpty()) 10157 return true; 10158 return false; 10159 } 10160 10161 public CapabilityStatementDocumentComponent addDocument() { // 3 10162 CapabilityStatementDocumentComponent t = new CapabilityStatementDocumentComponent(); 10163 if (this.document == null) 10164 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10165 this.document.add(t); 10166 return t; 10167 } 10168 10169 public CapabilityStatement addDocument(CapabilityStatementDocumentComponent t) { // 3 10170 if (t == null) 10171 return this; 10172 if (this.document == null) 10173 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10174 this.document.add(t); 10175 return this; 10176 } 10177 10178 /** 10179 * @return The first repetition of repeating field {@link #document}, creating 10180 * it if it does not already exist 10181 */ 10182 public CapabilityStatementDocumentComponent getDocumentFirstRep() { 10183 if (getDocument().isEmpty()) { 10184 addDocument(); 10185 } 10186 return getDocument().get(0); 10187 } 10188 10189 protected void listChildren(List<Property> children) { 10190 super.listChildren(children); 10191 children.add(new Property("url", "uri", 10192 "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.", 10193 0, 1, url)); 10194 children.add(new Property("version", "string", 10195 "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 10196 0, 1, version)); 10197 children.add(new Property("name", "string", 10198 "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 10199 0, 1, name)); 10200 children.add(new Property("title", "string", 10201 "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title)); 10202 children.add(new Property("status", "code", 10203 "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status)); 10204 children.add(new Property("experimental", "boolean", 10205 "A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 10206 0, 1, experimental)); 10207 children.add(new Property("date", "dateTime", 10208 "The date (and optionally time) when the capability statement was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 10209 0, 1, date)); 10210 children.add(new Property("publisher", "string", 10211 "The name of the organization or individual that published the capability statement.", 0, 1, publisher)); 10212 children.add(new Property("contact", "ContactDetail", 10213 "Contact details to assist a user in finding and communicating with the publisher.", 0, 10214 java.lang.Integer.MAX_VALUE, contact)); 10215 children.add(new Property("description", "markdown", 10216 "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 10217 0, 1, description)); 10218 children.add(new Property("useContext", "UsageContext", 10219 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.", 10220 0, java.lang.Integer.MAX_VALUE, useContext)); 10221 children.add(new Property("jurisdiction", "CodeableConcept", 10222 "A legal or geographic region in which the capability statement is intended to be used.", 0, 10223 java.lang.Integer.MAX_VALUE, jurisdiction)); 10224 children.add(new Property("purpose", "markdown", 10225 "Explanation of why this capability statement is needed and why it has been designed as it has.", 0, 1, 10226 purpose)); 10227 children.add(new Property("copyright", "markdown", 10228 "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 10229 0, 1, copyright)); 10230 children.add(new Property("kind", "code", 10231 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 10232 0, 1, kind)); 10233 children.add(new Property("instantiates", "canonical(CapabilityStatement)", 10234 "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.", 10235 0, java.lang.Integer.MAX_VALUE, instantiates)); 10236 children.add(new Property("imports", "canonical(CapabilityStatement)", 10237 "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.", 10238 0, java.lang.Integer.MAX_VALUE, imports)); 10239 children.add(new Property("software", "", 10240 "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 10241 0, 1, software)); 10242 children.add(new Property("implementation", "", 10243 "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 10244 0, 1, implementation)); 10245 children.add(new Property("fhirVersion", "code", 10246 "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.", 10247 0, 1, fhirVersion)); 10248 children.add(new Property("format", "code", 10249 "A list of the formats supported by this implementation using their content types.", 0, 10250 java.lang.Integer.MAX_VALUE, format)); 10251 children.add(new Property("patchFormat", "code", 10252 "A list of the patch formats supported by this implementation using their content types.", 0, 10253 java.lang.Integer.MAX_VALUE, patchFormat)); 10254 children.add(new Property("implementationGuide", "canonical(ImplementationGuide)", 10255 "A list of implementation guides that the server does (or should) support in their entirety.", 0, 10256 java.lang.Integer.MAX_VALUE, implementationGuide)); 10257 children.add(new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, 10258 java.lang.Integer.MAX_VALUE, rest)); 10259 children.add(new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, 10260 java.lang.Integer.MAX_VALUE, messaging)); 10261 children.add(new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document)); 10262 } 10263 10264 @Override 10265 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10266 switch (_hash) { 10267 case 116079: 10268 /* url */ return new Property("url", "uri", 10269 "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.", 10270 0, 1, url); 10271 case 351608024: 10272 /* version */ return new Property("version", "string", 10273 "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 10274 0, 1, version); 10275 case 3373707: 10276 /* name */ return new Property("name", "string", 10277 "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 10278 0, 1, name); 10279 case 110371416: 10280 /* title */ return new Property("title", "string", 10281 "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title); 10282 case -892481550: 10283 /* status */ return new Property("status", "code", 10284 "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status); 10285 case -404562712: 10286 /* experimental */ return new Property("experimental", "boolean", 10287 "A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 10288 0, 1, experimental); 10289 case 3076014: 10290 /* date */ return new Property("date", "dateTime", 10291 "The date (and optionally time) when the capability statement was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 10292 0, 1, date); 10293 case 1447404028: 10294 /* publisher */ return new Property("publisher", "string", 10295 "The name of the organization or individual that published the capability statement.", 0, 1, publisher); 10296 case 951526432: 10297 /* contact */ return new Property("contact", "ContactDetail", 10298 "Contact details to assist a user in finding and communicating with the publisher.", 0, 10299 java.lang.Integer.MAX_VALUE, contact); 10300 case -1724546052: 10301 /* description */ return new Property("description", "markdown", 10302 "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 10303 0, 1, description); 10304 case -669707736: 10305 /* useContext */ return new Property("useContext", "UsageContext", 10306 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.", 10307 0, java.lang.Integer.MAX_VALUE, useContext); 10308 case -507075711: 10309 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 10310 "A legal or geographic region in which the capability statement is intended to be used.", 0, 10311 java.lang.Integer.MAX_VALUE, jurisdiction); 10312 case -220463842: 10313 /* purpose */ return new Property("purpose", "markdown", 10314 "Explanation of why this capability statement is needed and why it has been designed as it has.", 0, 1, 10315 purpose); 10316 case 1522889671: 10317 /* copyright */ return new Property("copyright", "markdown", 10318 "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 10319 0, 1, copyright); 10320 case 3292052: 10321 /* kind */ return new Property("kind", "code", 10322 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 10323 0, 1, kind); 10324 case -246883639: 10325 /* instantiates */ return new Property("instantiates", "canonical(CapabilityStatement)", 10326 "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.", 10327 0, java.lang.Integer.MAX_VALUE, instantiates); 10328 case 1926037870: 10329 /* imports */ return new Property("imports", "canonical(CapabilityStatement)", 10330 "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.", 10331 0, java.lang.Integer.MAX_VALUE, imports); 10332 case 1319330215: 10333 /* software */ return new Property("software", "", 10334 "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 10335 0, 1, software); 10336 case 1683336114: 10337 /* implementation */ return new Property("implementation", "", 10338 "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 10339 0, 1, implementation); 10340 case 461006061: 10341 /* fhirVersion */ return new Property("fhirVersion", "code", 10342 "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.", 10343 0, 1, fhirVersion); 10344 case -1268779017: 10345 /* format */ return new Property("format", "code", 10346 "A list of the formats supported by this implementation using their content types.", 0, 10347 java.lang.Integer.MAX_VALUE, format); 10348 case 172338783: 10349 /* patchFormat */ return new Property("patchFormat", "code", 10350 "A list of the patch formats supported by this implementation using their content types.", 0, 10351 java.lang.Integer.MAX_VALUE, patchFormat); 10352 case 156966506: 10353 /* implementationGuide */ return new Property("implementationGuide", "canonical(ImplementationGuide)", 10354 "A list of implementation guides that the server does (or should) support in their entirety.", 0, 10355 java.lang.Integer.MAX_VALUE, implementationGuide); 10356 case 3496916: 10357 /* rest */ return new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, 10358 java.lang.Integer.MAX_VALUE, rest); 10359 case -1440008444: 10360 /* messaging */ return new Property("messaging", "", 10361 "A description of the messaging capabilities of the solution.", 0, java.lang.Integer.MAX_VALUE, messaging); 10362 case 861720859: 10363 /* document */ return new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, 10364 document); 10365 default: 10366 return super.getNamedProperty(_hash, _name, _checkValid); 10367 } 10368 10369 } 10370 10371 @Override 10372 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10373 switch (hash) { 10374 case 116079: 10375 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 10376 case 351608024: 10377 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 10378 case 3373707: 10379 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 10380 case 110371416: 10381 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 10382 case -892481550: 10383 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 10384 case -404562712: 10385 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 10386 case 3076014: 10387 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 10388 case 1447404028: 10389 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 10390 case 951526432: 10391 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 10392 case -1724546052: 10393 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 10394 case -669707736: 10395 /* useContext */ return this.useContext == null ? new Base[0] 10396 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 10397 case -507075711: 10398 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 10399 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 10400 case -220463842: 10401 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 10402 case 1522889671: 10403 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 10404 case 3292052: 10405 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<CapabilityStatementKind> 10406 case -246883639: 10407 /* instantiates */ return this.instantiates == null ? new Base[0] 10408 : this.instantiates.toArray(new Base[this.instantiates.size()]); // CanonicalType 10409 case 1926037870: 10410 /* imports */ return this.imports == null ? new Base[0] : this.imports.toArray(new Base[this.imports.size()]); // CanonicalType 10411 case 1319330215: 10412 /* software */ return this.software == null ? new Base[0] : new Base[] { this.software }; // CapabilityStatementSoftwareComponent 10413 case 1683336114: 10414 /* implementation */ return this.implementation == null ? new Base[0] : new Base[] { this.implementation }; // CapabilityStatementImplementationComponent 10415 case 461006061: 10416 /* fhirVersion */ return this.fhirVersion == null ? new Base[0] : new Base[] { this.fhirVersion }; // Enumeration<FHIRVersion> 10417 case -1268779017: 10418 /* format */ return this.format == null ? new Base[0] : this.format.toArray(new Base[this.format.size()]); // CodeType 10419 case 172338783: 10420 /* patchFormat */ return this.patchFormat == null ? new Base[0] 10421 : this.patchFormat.toArray(new Base[this.patchFormat.size()]); // CodeType 10422 case 156966506: 10423 /* implementationGuide */ return this.implementationGuide == null ? new Base[0] 10424 : this.implementationGuide.toArray(new Base[this.implementationGuide.size()]); // CanonicalType 10425 case 3496916: 10426 /* rest */ return this.rest == null ? new Base[0] : this.rest.toArray(new Base[this.rest.size()]); // CapabilityStatementRestComponent 10427 case -1440008444: 10428 /* messaging */ return this.messaging == null ? new Base[0] 10429 : this.messaging.toArray(new Base[this.messaging.size()]); // CapabilityStatementMessagingComponent 10430 case 861720859: 10431 /* document */ return this.document == null ? new Base[0] : this.document.toArray(new Base[this.document.size()]); // CapabilityStatementDocumentComponent 10432 default: 10433 return super.getProperty(hash, name, checkValid); 10434 } 10435 10436 } 10437 10438 @Override 10439 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10440 switch (hash) { 10441 case 116079: // url 10442 this.url = castToUri(value); // UriType 10443 return value; 10444 case 351608024: // version 10445 this.version = castToString(value); // StringType 10446 return value; 10447 case 3373707: // name 10448 this.name = castToString(value); // StringType 10449 return value; 10450 case 110371416: // title 10451 this.title = castToString(value); // StringType 10452 return value; 10453 case -892481550: // status 10454 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 10455 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10456 return value; 10457 case -404562712: // experimental 10458 this.experimental = castToBoolean(value); // BooleanType 10459 return value; 10460 case 3076014: // date 10461 this.date = castToDateTime(value); // DateTimeType 10462 return value; 10463 case 1447404028: // publisher 10464 this.publisher = castToString(value); // StringType 10465 return value; 10466 case 951526432: // contact 10467 this.getContact().add(castToContactDetail(value)); // ContactDetail 10468 return value; 10469 case -1724546052: // description 10470 this.description = castToMarkdown(value); // MarkdownType 10471 return value; 10472 case -669707736: // useContext 10473 this.getUseContext().add(castToUsageContext(value)); // UsageContext 10474 return value; 10475 case -507075711: // jurisdiction 10476 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 10477 return value; 10478 case -220463842: // purpose 10479 this.purpose = castToMarkdown(value); // MarkdownType 10480 return value; 10481 case 1522889671: // copyright 10482 this.copyright = castToMarkdown(value); // MarkdownType 10483 return value; 10484 case 3292052: // kind 10485 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 10486 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 10487 return value; 10488 case -246883639: // instantiates 10489 this.getInstantiates().add(castToCanonical(value)); // CanonicalType 10490 return value; 10491 case 1926037870: // imports 10492 this.getImports().add(castToCanonical(value)); // CanonicalType 10493 return value; 10494 case 1319330215: // software 10495 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 10496 return value; 10497 case 1683336114: // implementation 10498 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 10499 return value; 10500 case 461006061: // fhirVersion 10501 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 10502 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 10503 return value; 10504 case -1268779017: // format 10505 this.getFormat().add(castToCode(value)); // CodeType 10506 return value; 10507 case 172338783: // patchFormat 10508 this.getPatchFormat().add(castToCode(value)); // CodeType 10509 return value; 10510 case 156966506: // implementationGuide 10511 this.getImplementationGuide().add(castToCanonical(value)); // CanonicalType 10512 return value; 10513 case 3496916: // rest 10514 this.getRest().add((CapabilityStatementRestComponent) value); // CapabilityStatementRestComponent 10515 return value; 10516 case -1440008444: // messaging 10517 this.getMessaging().add((CapabilityStatementMessagingComponent) value); // CapabilityStatementMessagingComponent 10518 return value; 10519 case 861720859: // document 10520 this.getDocument().add((CapabilityStatementDocumentComponent) value); // CapabilityStatementDocumentComponent 10521 return value; 10522 default: 10523 return super.setProperty(hash, name, value); 10524 } 10525 10526 } 10527 10528 @Override 10529 public Base setProperty(String name, Base value) throws FHIRException { 10530 if (name.equals("url")) { 10531 this.url = castToUri(value); // UriType 10532 } else if (name.equals("version")) { 10533 this.version = castToString(value); // StringType 10534 } else if (name.equals("name")) { 10535 this.name = castToString(value); // StringType 10536 } else if (name.equals("title")) { 10537 this.title = castToString(value); // StringType 10538 } else if (name.equals("status")) { 10539 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 10540 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10541 } else if (name.equals("experimental")) { 10542 this.experimental = castToBoolean(value); // BooleanType 10543 } else if (name.equals("date")) { 10544 this.date = castToDateTime(value); // DateTimeType 10545 } else if (name.equals("publisher")) { 10546 this.publisher = castToString(value); // StringType 10547 } else if (name.equals("contact")) { 10548 this.getContact().add(castToContactDetail(value)); 10549 } else if (name.equals("description")) { 10550 this.description = castToMarkdown(value); // MarkdownType 10551 } else if (name.equals("useContext")) { 10552 this.getUseContext().add(castToUsageContext(value)); 10553 } else if (name.equals("jurisdiction")) { 10554 this.getJurisdiction().add(castToCodeableConcept(value)); 10555 } else if (name.equals("purpose")) { 10556 this.purpose = castToMarkdown(value); // MarkdownType 10557 } else if (name.equals("copyright")) { 10558 this.copyright = castToMarkdown(value); // MarkdownType 10559 } else if (name.equals("kind")) { 10560 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 10561 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 10562 } else if (name.equals("instantiates")) { 10563 this.getInstantiates().add(castToCanonical(value)); 10564 } else if (name.equals("imports")) { 10565 this.getImports().add(castToCanonical(value)); 10566 } else if (name.equals("software")) { 10567 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 10568 } else if (name.equals("implementation")) { 10569 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 10570 } else if (name.equals("fhirVersion")) { 10571 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 10572 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 10573 } else if (name.equals("format")) { 10574 this.getFormat().add(castToCode(value)); 10575 } else if (name.equals("patchFormat")) { 10576 this.getPatchFormat().add(castToCode(value)); 10577 } else if (name.equals("implementationGuide")) { 10578 this.getImplementationGuide().add(castToCanonical(value)); 10579 } else if (name.equals("rest")) { 10580 this.getRest().add((CapabilityStatementRestComponent) value); 10581 } else if (name.equals("messaging")) { 10582 this.getMessaging().add((CapabilityStatementMessagingComponent) value); 10583 } else if (name.equals("document")) { 10584 this.getDocument().add((CapabilityStatementDocumentComponent) value); 10585 } else 10586 return super.setProperty(name, value); 10587 return value; 10588 } 10589 10590 @Override 10591 public void removeChild(String name, Base value) throws FHIRException { 10592 if (name.equals("url")) { 10593 this.url = null; 10594 } else if (name.equals("version")) { 10595 this.version = null; 10596 } else if (name.equals("name")) { 10597 this.name = null; 10598 } else if (name.equals("title")) { 10599 this.title = null; 10600 } else if (name.equals("status")) { 10601 this.status = null; 10602 } else if (name.equals("experimental")) { 10603 this.experimental = null; 10604 } else if (name.equals("date")) { 10605 this.date = null; 10606 } else if (name.equals("publisher")) { 10607 this.publisher = null; 10608 } else if (name.equals("contact")) { 10609 this.getContact().remove(castToContactDetail(value)); 10610 } else if (name.equals("description")) { 10611 this.description = null; 10612 } else if (name.equals("useContext")) { 10613 this.getUseContext().remove(castToUsageContext(value)); 10614 } else if (name.equals("jurisdiction")) { 10615 this.getJurisdiction().remove(castToCodeableConcept(value)); 10616 } else if (name.equals("purpose")) { 10617 this.purpose = null; 10618 } else if (name.equals("copyright")) { 10619 this.copyright = null; 10620 } else if (name.equals("kind")) { 10621 this.kind = null; 10622 } else if (name.equals("instantiates")) { 10623 this.getInstantiates().remove(castToCanonical(value)); 10624 } else if (name.equals("imports")) { 10625 this.getImports().remove(castToCanonical(value)); 10626 } else if (name.equals("software")) { 10627 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 10628 } else if (name.equals("implementation")) { 10629 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 10630 } else if (name.equals("fhirVersion")) { 10631 this.fhirVersion = null; 10632 } else if (name.equals("format")) { 10633 this.getFormat().remove(castToCode(value)); 10634 } else if (name.equals("patchFormat")) { 10635 this.getPatchFormat().remove(castToCode(value)); 10636 } else if (name.equals("implementationGuide")) { 10637 this.getImplementationGuide().remove(castToCanonical(value)); 10638 } else if (name.equals("rest")) { 10639 this.getRest().remove((CapabilityStatementRestComponent) value); 10640 } else if (name.equals("messaging")) { 10641 this.getMessaging().remove((CapabilityStatementMessagingComponent) value); 10642 } else if (name.equals("document")) { 10643 this.getDocument().remove((CapabilityStatementDocumentComponent) value); 10644 } else 10645 super.removeChild(name, value); 10646 10647 } 10648 10649 @Override 10650 public Base makeProperty(int hash, String name) throws FHIRException { 10651 switch (hash) { 10652 case 116079: 10653 return getUrlElement(); 10654 case 351608024: 10655 return getVersionElement(); 10656 case 3373707: 10657 return getNameElement(); 10658 case 110371416: 10659 return getTitleElement(); 10660 case -892481550: 10661 return getStatusElement(); 10662 case -404562712: 10663 return getExperimentalElement(); 10664 case 3076014: 10665 return getDateElement(); 10666 case 1447404028: 10667 return getPublisherElement(); 10668 case 951526432: 10669 return addContact(); 10670 case -1724546052: 10671 return getDescriptionElement(); 10672 case -669707736: 10673 return addUseContext(); 10674 case -507075711: 10675 return addJurisdiction(); 10676 case -220463842: 10677 return getPurposeElement(); 10678 case 1522889671: 10679 return getCopyrightElement(); 10680 case 3292052: 10681 return getKindElement(); 10682 case -246883639: 10683 return addInstantiatesElement(); 10684 case 1926037870: 10685 return addImportsElement(); 10686 case 1319330215: 10687 return getSoftware(); 10688 case 1683336114: 10689 return getImplementation(); 10690 case 461006061: 10691 return getFhirVersionElement(); 10692 case -1268779017: 10693 return addFormatElement(); 10694 case 172338783: 10695 return addPatchFormatElement(); 10696 case 156966506: 10697 return addImplementationGuideElement(); 10698 case 3496916: 10699 return addRest(); 10700 case -1440008444: 10701 return addMessaging(); 10702 case 861720859: 10703 return addDocument(); 10704 default: 10705 return super.makeProperty(hash, name); 10706 } 10707 10708 } 10709 10710 @Override 10711 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10712 switch (hash) { 10713 case 116079: 10714 /* url */ return new String[] { "uri" }; 10715 case 351608024: 10716 /* version */ return new String[] { "string" }; 10717 case 3373707: 10718 /* name */ return new String[] { "string" }; 10719 case 110371416: 10720 /* title */ return new String[] { "string" }; 10721 case -892481550: 10722 /* status */ return new String[] { "code" }; 10723 case -404562712: 10724 /* experimental */ return new String[] { "boolean" }; 10725 case 3076014: 10726 /* date */ return new String[] { "dateTime" }; 10727 case 1447404028: 10728 /* publisher */ return new String[] { "string" }; 10729 case 951526432: 10730 /* contact */ return new String[] { "ContactDetail" }; 10731 case -1724546052: 10732 /* description */ return new String[] { "markdown" }; 10733 case -669707736: 10734 /* useContext */ return new String[] { "UsageContext" }; 10735 case -507075711: 10736 /* jurisdiction */ return new String[] { "CodeableConcept" }; 10737 case -220463842: 10738 /* purpose */ return new String[] { "markdown" }; 10739 case 1522889671: 10740 /* copyright */ return new String[] { "markdown" }; 10741 case 3292052: 10742 /* kind */ return new String[] { "code" }; 10743 case -246883639: 10744 /* instantiates */ return new String[] { "canonical" }; 10745 case 1926037870: 10746 /* imports */ return new String[] { "canonical" }; 10747 case 1319330215: 10748 /* software */ return new String[] {}; 10749 case 1683336114: 10750 /* implementation */ return new String[] {}; 10751 case 461006061: 10752 /* fhirVersion */ return new String[] { "code" }; 10753 case -1268779017: 10754 /* format */ return new String[] { "code" }; 10755 case 172338783: 10756 /* patchFormat */ return new String[] { "code" }; 10757 case 156966506: 10758 /* implementationGuide */ return new String[] { "canonical" }; 10759 case 3496916: 10760 /* rest */ return new String[] {}; 10761 case -1440008444: 10762 /* messaging */ return new String[] {}; 10763 case 861720859: 10764 /* document */ return new String[] {}; 10765 default: 10766 return super.getTypesForProperty(hash, name); 10767 } 10768 10769 } 10770 10771 @Override 10772 public Base addChild(String name) throws FHIRException { 10773 if (name.equals("url")) { 10774 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 10775 } else if (name.equals("version")) { 10776 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 10777 } else if (name.equals("name")) { 10778 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 10779 } else if (name.equals("title")) { 10780 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.title"); 10781 } else if (name.equals("status")) { 10782 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.status"); 10783 } else if (name.equals("experimental")) { 10784 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.experimental"); 10785 } else if (name.equals("date")) { 10786 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.date"); 10787 } else if (name.equals("publisher")) { 10788 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.publisher"); 10789 } else if (name.equals("contact")) { 10790 return addContact(); 10791 } else if (name.equals("description")) { 10792 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 10793 } else if (name.equals("useContext")) { 10794 return addUseContext(); 10795 } else if (name.equals("jurisdiction")) { 10796 return addJurisdiction(); 10797 } else if (name.equals("purpose")) { 10798 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.purpose"); 10799 } else if (name.equals("copyright")) { 10800 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.copyright"); 10801 } else if (name.equals("kind")) { 10802 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.kind"); 10803 } else if (name.equals("instantiates")) { 10804 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.instantiates"); 10805 } else if (name.equals("imports")) { 10806 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.imports"); 10807 } else if (name.equals("software")) { 10808 this.software = new CapabilityStatementSoftwareComponent(); 10809 return this.software; 10810 } else if (name.equals("implementation")) { 10811 this.implementation = new CapabilityStatementImplementationComponent(); 10812 return this.implementation; 10813 } else if (name.equals("fhirVersion")) { 10814 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.fhirVersion"); 10815 } else if (name.equals("format")) { 10816 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.format"); 10817 } else if (name.equals("patchFormat")) { 10818 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.patchFormat"); 10819 } else if (name.equals("implementationGuide")) { 10820 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.implementationGuide"); 10821 } else if (name.equals("rest")) { 10822 return addRest(); 10823 } else if (name.equals("messaging")) { 10824 return addMessaging(); 10825 } else if (name.equals("document")) { 10826 return addDocument(); 10827 } else 10828 return super.addChild(name); 10829 } 10830 10831 public String fhirType() { 10832 return "CapabilityStatement"; 10833 10834 } 10835 10836 public CapabilityStatement copy() { 10837 CapabilityStatement dst = new CapabilityStatement(); 10838 copyValues(dst); 10839 return dst; 10840 } 10841 10842 public void copyValues(CapabilityStatement dst) { 10843 super.copyValues(dst); 10844 dst.url = url == null ? null : url.copy(); 10845 dst.version = version == null ? null : version.copy(); 10846 dst.name = name == null ? null : name.copy(); 10847 dst.title = title == null ? null : title.copy(); 10848 dst.status = status == null ? null : status.copy(); 10849 dst.experimental = experimental == null ? null : experimental.copy(); 10850 dst.date = date == null ? null : date.copy(); 10851 dst.publisher = publisher == null ? null : publisher.copy(); 10852 if (contact != null) { 10853 dst.contact = new ArrayList<ContactDetail>(); 10854 for (ContactDetail i : contact) 10855 dst.contact.add(i.copy()); 10856 } 10857 ; 10858 dst.description = description == null ? null : description.copy(); 10859 if (useContext != null) { 10860 dst.useContext = new ArrayList<UsageContext>(); 10861 for (UsageContext i : useContext) 10862 dst.useContext.add(i.copy()); 10863 } 10864 ; 10865 if (jurisdiction != null) { 10866 dst.jurisdiction = new ArrayList<CodeableConcept>(); 10867 for (CodeableConcept i : jurisdiction) 10868 dst.jurisdiction.add(i.copy()); 10869 } 10870 ; 10871 dst.purpose = purpose == null ? null : purpose.copy(); 10872 dst.copyright = copyright == null ? null : copyright.copy(); 10873 dst.kind = kind == null ? null : kind.copy(); 10874 if (instantiates != null) { 10875 dst.instantiates = new ArrayList<CanonicalType>(); 10876 for (CanonicalType i : instantiates) 10877 dst.instantiates.add(i.copy()); 10878 } 10879 ; 10880 if (imports != null) { 10881 dst.imports = new ArrayList<CanonicalType>(); 10882 for (CanonicalType i : imports) 10883 dst.imports.add(i.copy()); 10884 } 10885 ; 10886 dst.software = software == null ? null : software.copy(); 10887 dst.implementation = implementation == null ? null : implementation.copy(); 10888 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 10889 if (format != null) { 10890 dst.format = new ArrayList<CodeType>(); 10891 for (CodeType i : format) 10892 dst.format.add(i.copy()); 10893 } 10894 ; 10895 if (patchFormat != null) { 10896 dst.patchFormat = new ArrayList<CodeType>(); 10897 for (CodeType i : patchFormat) 10898 dst.patchFormat.add(i.copy()); 10899 } 10900 ; 10901 if (implementationGuide != null) { 10902 dst.implementationGuide = new ArrayList<CanonicalType>(); 10903 for (CanonicalType i : implementationGuide) 10904 dst.implementationGuide.add(i.copy()); 10905 } 10906 ; 10907 if (rest != null) { 10908 dst.rest = new ArrayList<CapabilityStatementRestComponent>(); 10909 for (CapabilityStatementRestComponent i : rest) 10910 dst.rest.add(i.copy()); 10911 } 10912 ; 10913 if (messaging != null) { 10914 dst.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10915 for (CapabilityStatementMessagingComponent i : messaging) 10916 dst.messaging.add(i.copy()); 10917 } 10918 ; 10919 if (document != null) { 10920 dst.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10921 for (CapabilityStatementDocumentComponent i : document) 10922 dst.document.add(i.copy()); 10923 } 10924 ; 10925 } 10926 10927 protected CapabilityStatement typedCopy() { 10928 return copy(); 10929 } 10930 10931 @Override 10932 public boolean equalsDeep(Base other_) { 10933 if (!super.equalsDeep(other_)) 10934 return false; 10935 if (!(other_ instanceof CapabilityStatement)) 10936 return false; 10937 CapabilityStatement o = (CapabilityStatement) other_; 10938 return compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 10939 && compareDeep(kind, o.kind, true) && compareDeep(instantiates, o.instantiates, true) 10940 && compareDeep(imports, o.imports, true) && compareDeep(software, o.software, true) 10941 && compareDeep(implementation, o.implementation, true) && compareDeep(fhirVersion, o.fhirVersion, true) 10942 && compareDeep(format, o.format, true) && compareDeep(patchFormat, o.patchFormat, true) 10943 && compareDeep(implementationGuide, o.implementationGuide, true) && compareDeep(rest, o.rest, true) 10944 && compareDeep(messaging, o.messaging, true) && compareDeep(document, o.document, true); 10945 } 10946 10947 @Override 10948 public boolean equalsShallow(Base other_) { 10949 if (!super.equalsShallow(other_)) 10950 return false; 10951 if (!(other_ instanceof CapabilityStatement)) 10952 return false; 10953 CapabilityStatement o = (CapabilityStatement) other_; 10954 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 10955 && compareValues(kind, o.kind, true) && compareValues(fhirVersion, o.fhirVersion, true) 10956 && compareValues(format, o.format, true) && compareValues(patchFormat, o.patchFormat, true); 10957 } 10958 10959 public boolean isEmpty() { 10960 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, copyright, kind, instantiates, imports, 10961 software, implementation, fhirVersion, format, patchFormat, implementationGuide, rest, messaging, document); 10962 } 10963 10964 @Override 10965 public ResourceType getResourceType() { 10966 return ResourceType.CapabilityStatement; 10967 } 10968 10969 /** 10970 * Search parameter: <b>date</b> 10971 * <p> 10972 * Description: <b>The capability statement publication date</b><br> 10973 * Type: <b>date</b><br> 10974 * Path: <b>CapabilityStatement.date</b><br> 10975 * </p> 10976 */ 10977 @SearchParamDefinition(name = "date", path = "CapabilityStatement.date", description = "The capability statement publication date", type = "date") 10978 public static final String SP_DATE = "date"; 10979 /** 10980 * <b>Fluent Client</b> search parameter constant for <b>date</b> 10981 * <p> 10982 * Description: <b>The capability statement publication date</b><br> 10983 * Type: <b>date</b><br> 10984 * Path: <b>CapabilityStatement.date</b><br> 10985 * </p> 10986 */ 10987 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 10988 SP_DATE); 10989 10990 /** 10991 * Search parameter: <b>resource-profile</b> 10992 * <p> 10993 * Description: <b>A profile id invoked in a capability statement</b><br> 10994 * Type: <b>reference</b><br> 10995 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 10996 * </p> 10997 */ 10998 @SearchParamDefinition(name = "resource-profile", path = "CapabilityStatement.rest.resource.profile", description = "A profile id invoked in a capability statement", type = "reference", target = { 10999 StructureDefinition.class }) 11000 public static final String SP_RESOURCE_PROFILE = "resource-profile"; 11001 /** 11002 * <b>Fluent Client</b> search parameter constant for <b>resource-profile</b> 11003 * <p> 11004 * Description: <b>A profile id invoked in a capability statement</b><br> 11005 * Type: <b>reference</b><br> 11006 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 11007 * </p> 11008 */ 11009 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11010 SP_RESOURCE_PROFILE); 11011 11012 /** 11013 * Constant for fluent queries to be used to add include statements. Specifies 11014 * the path value of "<b>CapabilityStatement:resource-profile</b>". 11015 */ 11016 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE_PROFILE = new ca.uhn.fhir.model.api.Include( 11017 "CapabilityStatement:resource-profile").toLocked(); 11018 11019 /** 11020 * Search parameter: <b>context-type-value</b> 11021 * <p> 11022 * Description: <b>A use context type and value assigned to the capability 11023 * statement</b><br> 11024 * Type: <b>composite</b><br> 11025 * Path: <b></b><br> 11026 * </p> 11027 */ 11028 @SearchParamDefinition(name = "context-type-value", path = "CapabilityStatement.useContext", description = "A use context type and value assigned to the capability statement", type = "composite", compositeOf = { 11029 "context-type", "context" }) 11030 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 11031 /** 11032 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 11033 * <p> 11034 * Description: <b>A use context type and value assigned to the capability 11035 * statement</b><br> 11036 * Type: <b>composite</b><br> 11037 * Path: <b></b><br> 11038 * </p> 11039 */ 11040 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 11041 SP_CONTEXT_TYPE_VALUE); 11042 11043 /** 11044 * Search parameter: <b>software</b> 11045 * <p> 11046 * Description: <b>Part of the name of a software application</b><br> 11047 * Type: <b>string</b><br> 11048 * Path: <b>CapabilityStatement.software.name</b><br> 11049 * </p> 11050 */ 11051 @SearchParamDefinition(name = "software", path = "CapabilityStatement.software.name", description = "Part of the name of a software application", type = "string") 11052 public static final String SP_SOFTWARE = "software"; 11053 /** 11054 * <b>Fluent Client</b> search parameter constant for <b>software</b> 11055 * <p> 11056 * Description: <b>Part of the name of a software application</b><br> 11057 * Type: <b>string</b><br> 11058 * Path: <b>CapabilityStatement.software.name</b><br> 11059 * </p> 11060 */ 11061 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOFTWARE = new ca.uhn.fhir.rest.gclient.StringClientParam( 11062 SP_SOFTWARE); 11063 11064 /** 11065 * Search parameter: <b>resource</b> 11066 * <p> 11067 * Description: <b>Name of a resource mentioned in a capability 11068 * statement</b><br> 11069 * Type: <b>token</b><br> 11070 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 11071 * </p> 11072 */ 11073 @SearchParamDefinition(name = "resource", path = "CapabilityStatement.rest.resource.type", description = "Name of a resource mentioned in a capability statement", type = "token") 11074 public static final String SP_RESOURCE = "resource"; 11075 /** 11076 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 11077 * <p> 11078 * Description: <b>Name of a resource mentioned in a capability 11079 * statement</b><br> 11080 * Type: <b>token</b><br> 11081 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 11082 * </p> 11083 */ 11084 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11085 SP_RESOURCE); 11086 11087 /** 11088 * Search parameter: <b>jurisdiction</b> 11089 * <p> 11090 * Description: <b>Intended jurisdiction for the capability statement</b><br> 11091 * Type: <b>token</b><br> 11092 * Path: <b>CapabilityStatement.jurisdiction</b><br> 11093 * </p> 11094 */ 11095 @SearchParamDefinition(name = "jurisdiction", path = "CapabilityStatement.jurisdiction", description = "Intended jurisdiction for the capability statement", type = "token") 11096 public static final String SP_JURISDICTION = "jurisdiction"; 11097 /** 11098 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 11099 * <p> 11100 * Description: <b>Intended jurisdiction for the capability statement</b><br> 11101 * Type: <b>token</b><br> 11102 * Path: <b>CapabilityStatement.jurisdiction</b><br> 11103 * </p> 11104 */ 11105 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11106 SP_JURISDICTION); 11107 11108 /** 11109 * Search parameter: <b>format</b> 11110 * <p> 11111 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 11112 * Type: <b>token</b><br> 11113 * Path: <b>CapabilityStatement.format</b><br> 11114 * </p> 11115 */ 11116 @SearchParamDefinition(name = "format", path = "CapabilityStatement.format", description = "formats supported (xml | json | ttl | mime type)", type = "token") 11117 public static final String SP_FORMAT = "format"; 11118 /** 11119 * <b>Fluent Client</b> search parameter constant for <b>format</b> 11120 * <p> 11121 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 11122 * Type: <b>token</b><br> 11123 * Path: <b>CapabilityStatement.format</b><br> 11124 * </p> 11125 */ 11126 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11127 SP_FORMAT); 11128 11129 /** 11130 * Search parameter: <b>description</b> 11131 * <p> 11132 * Description: <b>The description of the capability statement</b><br> 11133 * Type: <b>string</b><br> 11134 * Path: <b>CapabilityStatement.description</b><br> 11135 * </p> 11136 */ 11137 @SearchParamDefinition(name = "description", path = "CapabilityStatement.description", description = "The description of the capability statement", type = "string") 11138 public static final String SP_DESCRIPTION = "description"; 11139 /** 11140 * <b>Fluent Client</b> search parameter constant for <b>description</b> 11141 * <p> 11142 * Description: <b>The description of the capability statement</b><br> 11143 * Type: <b>string</b><br> 11144 * Path: <b>CapabilityStatement.description</b><br> 11145 * </p> 11146 */ 11147 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 11148 SP_DESCRIPTION); 11149 11150 /** 11151 * Search parameter: <b>context-type</b> 11152 * <p> 11153 * Description: <b>A type of use context assigned to the capability 11154 * statement</b><br> 11155 * Type: <b>token</b><br> 11156 * Path: <b>CapabilityStatement.useContext.code</b><br> 11157 * </p> 11158 */ 11159 @SearchParamDefinition(name = "context-type", path = "CapabilityStatement.useContext.code", description = "A type of use context assigned to the capability statement", type = "token") 11160 public static final String SP_CONTEXT_TYPE = "context-type"; 11161 /** 11162 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 11163 * <p> 11164 * Description: <b>A type of use context assigned to the capability 11165 * statement</b><br> 11166 * Type: <b>token</b><br> 11167 * Path: <b>CapabilityStatement.useContext.code</b><br> 11168 * </p> 11169 */ 11170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11171 SP_CONTEXT_TYPE); 11172 11173 /** 11174 * Search parameter: <b>title</b> 11175 * <p> 11176 * Description: <b>The human-friendly name of the capability statement</b><br> 11177 * Type: <b>string</b><br> 11178 * Path: <b>CapabilityStatement.title</b><br> 11179 * </p> 11180 */ 11181 @SearchParamDefinition(name = "title", path = "CapabilityStatement.title", description = "The human-friendly name of the capability statement", type = "string") 11182 public static final String SP_TITLE = "title"; 11183 /** 11184 * <b>Fluent Client</b> search parameter constant for <b>title</b> 11185 * <p> 11186 * Description: <b>The human-friendly name of the capability statement</b><br> 11187 * Type: <b>string</b><br> 11188 * Path: <b>CapabilityStatement.title</b><br> 11189 * </p> 11190 */ 11191 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 11192 SP_TITLE); 11193 11194 /** 11195 * Search parameter: <b>fhirversion</b> 11196 * <p> 11197 * Description: <b>The version of FHIR</b><br> 11198 * Type: <b>token</b><br> 11199 * Path: <b>CapabilityStatement.version</b><br> 11200 * </p> 11201 */ 11202 @SearchParamDefinition(name = "fhirversion", path = "CapabilityStatement.version", description = "The version of FHIR", type = "token") 11203 public static final String SP_FHIRVERSION = "fhirversion"; 11204 /** 11205 * <b>Fluent Client</b> search parameter constant for <b>fhirversion</b> 11206 * <p> 11207 * Description: <b>The version of FHIR</b><br> 11208 * Type: <b>token</b><br> 11209 * Path: <b>CapabilityStatement.version</b><br> 11210 * </p> 11211 */ 11212 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FHIRVERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11213 SP_FHIRVERSION); 11214 11215 /** 11216 * Search parameter: <b>version</b> 11217 * <p> 11218 * Description: <b>The business version of the capability statement</b><br> 11219 * Type: <b>token</b><br> 11220 * Path: <b>CapabilityStatement.version</b><br> 11221 * </p> 11222 */ 11223 @SearchParamDefinition(name = "version", path = "CapabilityStatement.version", description = "The business version of the capability statement", type = "token") 11224 public static final String SP_VERSION = "version"; 11225 /** 11226 * <b>Fluent Client</b> search parameter constant for <b>version</b> 11227 * <p> 11228 * Description: <b>The business version of the capability statement</b><br> 11229 * Type: <b>token</b><br> 11230 * Path: <b>CapabilityStatement.version</b><br> 11231 * </p> 11232 */ 11233 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11234 SP_VERSION); 11235 11236 /** 11237 * Search parameter: <b>url</b> 11238 * <p> 11239 * Description: <b>The uri that identifies the capability statement</b><br> 11240 * Type: <b>uri</b><br> 11241 * Path: <b>CapabilityStatement.url</b><br> 11242 * </p> 11243 */ 11244 @SearchParamDefinition(name = "url", path = "CapabilityStatement.url", description = "The uri that identifies the capability statement", type = "uri") 11245 public static final String SP_URL = "url"; 11246 /** 11247 * <b>Fluent Client</b> search parameter constant for <b>url</b> 11248 * <p> 11249 * Description: <b>The uri that identifies the capability statement</b><br> 11250 * Type: <b>uri</b><br> 11251 * Path: <b>CapabilityStatement.url</b><br> 11252 * </p> 11253 */ 11254 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 11255 11256 /** 11257 * Search parameter: <b>supported-profile</b> 11258 * <p> 11259 * Description: <b>Profiles for use cases supported</b><br> 11260 * Type: <b>reference</b><br> 11261 * Path: <b>CapabilityStatement.rest.resource.supportedProfile</b><br> 11262 * </p> 11263 */ 11264 @SearchParamDefinition(name = "supported-profile", path = "CapabilityStatement.rest.resource.supportedProfile", description = "Profiles for use cases supported", type = "reference", target = { 11265 StructureDefinition.class }) 11266 public static final String SP_SUPPORTED_PROFILE = "supported-profile"; 11267 /** 11268 * <b>Fluent Client</b> search parameter constant for <b>supported-profile</b> 11269 * <p> 11270 * Description: <b>Profiles for use cases supported</b><br> 11271 * Type: <b>reference</b><br> 11272 * Path: <b>CapabilityStatement.rest.resource.supportedProfile</b><br> 11273 * </p> 11274 */ 11275 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTED_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11276 SP_SUPPORTED_PROFILE); 11277 11278 /** 11279 * Constant for fluent queries to be used to add include statements. Specifies 11280 * the path value of "<b>CapabilityStatement:supported-profile</b>". 11281 */ 11282 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTED_PROFILE = new ca.uhn.fhir.model.api.Include( 11283 "CapabilityStatement:supported-profile").toLocked(); 11284 11285 /** 11286 * Search parameter: <b>mode</b> 11287 * <p> 11288 * Description: <b>Mode - restful (server/client) or messaging 11289 * (sender/receiver)</b><br> 11290 * Type: <b>token</b><br> 11291 * Path: <b>CapabilityStatement.rest.mode</b><br> 11292 * </p> 11293 */ 11294 @SearchParamDefinition(name = "mode", path = "CapabilityStatement.rest.mode", description = "Mode - restful (server/client) or messaging (sender/receiver)", type = "token") 11295 public static final String SP_MODE = "mode"; 11296 /** 11297 * <b>Fluent Client</b> search parameter constant for <b>mode</b> 11298 * <p> 11299 * Description: <b>Mode - restful (server/client) or messaging 11300 * (sender/receiver)</b><br> 11301 * Type: <b>token</b><br> 11302 * Path: <b>CapabilityStatement.rest.mode</b><br> 11303 * </p> 11304 */ 11305 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11306 SP_MODE); 11307 11308 /** 11309 * Search parameter: <b>context-quantity</b> 11310 * <p> 11311 * Description: <b>A quantity- or range-valued use context assigned to the 11312 * capability statement</b><br> 11313 * Type: <b>quantity</b><br> 11314 * Path: <b>CapabilityStatement.useContext.valueQuantity, 11315 * CapabilityStatement.useContext.valueRange</b><br> 11316 * </p> 11317 */ 11318 @SearchParamDefinition(name = "context-quantity", path = "(CapabilityStatement.useContext.value as Quantity) | (CapabilityStatement.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the capability statement", type = "quantity") 11319 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 11320 /** 11321 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 11322 * <p> 11323 * Description: <b>A quantity- or range-valued use context assigned to the 11324 * capability statement</b><br> 11325 * Type: <b>quantity</b><br> 11326 * Path: <b>CapabilityStatement.useContext.valueQuantity, 11327 * CapabilityStatement.useContext.valueRange</b><br> 11328 * </p> 11329 */ 11330 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 11331 SP_CONTEXT_QUANTITY); 11332 11333 /** 11334 * Search parameter: <b>security-service</b> 11335 * <p> 11336 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | 11337 * Certificates</b><br> 11338 * Type: <b>token</b><br> 11339 * Path: <b>CapabilityStatement.rest.security.service</b><br> 11340 * </p> 11341 */ 11342 @SearchParamDefinition(name = "security-service", path = "CapabilityStatement.rest.security.service", description = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", type = "token") 11343 public static final String SP_SECURITY_SERVICE = "security-service"; 11344 /** 11345 * <b>Fluent Client</b> search parameter constant for <b>security-service</b> 11346 * <p> 11347 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | 11348 * Certificates</b><br> 11349 * Type: <b>token</b><br> 11350 * Path: <b>CapabilityStatement.rest.security.service</b><br> 11351 * </p> 11352 */ 11353 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_SERVICE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11354 SP_SECURITY_SERVICE); 11355 11356 /** 11357 * Search parameter: <b>name</b> 11358 * <p> 11359 * Description: <b>Computationally friendly name of the capability 11360 * statement</b><br> 11361 * Type: <b>string</b><br> 11362 * Path: <b>CapabilityStatement.name</b><br> 11363 * </p> 11364 */ 11365 @SearchParamDefinition(name = "name", path = "CapabilityStatement.name", description = "Computationally friendly name of the capability statement", type = "string") 11366 public static final String SP_NAME = "name"; 11367 /** 11368 * <b>Fluent Client</b> search parameter constant for <b>name</b> 11369 * <p> 11370 * Description: <b>Computationally friendly name of the capability 11371 * statement</b><br> 11372 * Type: <b>string</b><br> 11373 * Path: <b>CapabilityStatement.name</b><br> 11374 * </p> 11375 */ 11376 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 11377 SP_NAME); 11378 11379 /** 11380 * Search parameter: <b>context</b> 11381 * <p> 11382 * Description: <b>A use context assigned to the capability statement</b><br> 11383 * Type: <b>token</b><br> 11384 * Path: <b>CapabilityStatement.useContext.valueCodeableConcept</b><br> 11385 * </p> 11386 */ 11387 @SearchParamDefinition(name = "context", path = "(CapabilityStatement.useContext.value as CodeableConcept)", description = "A use context assigned to the capability statement", type = "token") 11388 public static final String SP_CONTEXT = "context"; 11389 /** 11390 * <b>Fluent Client</b> search parameter constant for <b>context</b> 11391 * <p> 11392 * Description: <b>A use context assigned to the capability statement</b><br> 11393 * Type: <b>token</b><br> 11394 * Path: <b>CapabilityStatement.useContext.valueCodeableConcept</b><br> 11395 * </p> 11396 */ 11397 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11398 SP_CONTEXT); 11399 11400 /** 11401 * Search parameter: <b>publisher</b> 11402 * <p> 11403 * Description: <b>Name of the publisher of the capability statement</b><br> 11404 * Type: <b>string</b><br> 11405 * Path: <b>CapabilityStatement.publisher</b><br> 11406 * </p> 11407 */ 11408 @SearchParamDefinition(name = "publisher", path = "CapabilityStatement.publisher", description = "Name of the publisher of the capability statement", type = "string") 11409 public static final String SP_PUBLISHER = "publisher"; 11410 /** 11411 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 11412 * <p> 11413 * Description: <b>Name of the publisher of the capability statement</b><br> 11414 * Type: <b>string</b><br> 11415 * Path: <b>CapabilityStatement.publisher</b><br> 11416 * </p> 11417 */ 11418 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 11419 SP_PUBLISHER); 11420 11421 /** 11422 * Search parameter: <b>context-type-quantity</b> 11423 * <p> 11424 * Description: <b>A use context type and quantity- or range-based value 11425 * assigned to the capability statement</b><br> 11426 * Type: <b>composite</b><br> 11427 * Path: <b></b><br> 11428 * </p> 11429 */ 11430 @SearchParamDefinition(name = "context-type-quantity", path = "CapabilityStatement.useContext", description = "A use context type and quantity- or range-based value assigned to the capability statement", type = "composite", compositeOf = { 11431 "context-type", "context-quantity" }) 11432 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 11433 /** 11434 * <b>Fluent Client</b> search parameter constant for 11435 * <b>context-type-quantity</b> 11436 * <p> 11437 * Description: <b>A use context type and quantity- or range-based value 11438 * assigned to the capability statement</b><br> 11439 * Type: <b>composite</b><br> 11440 * Path: <b></b><br> 11441 * </p> 11442 */ 11443 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 11444 SP_CONTEXT_TYPE_QUANTITY); 11445 11446 /** 11447 * Search parameter: <b>guide</b> 11448 * <p> 11449 * Description: <b>Implementation guides supported</b><br> 11450 * Type: <b>reference</b><br> 11451 * Path: <b>CapabilityStatement.implementationGuide</b><br> 11452 * </p> 11453 */ 11454 @SearchParamDefinition(name = "guide", path = "CapabilityStatement.implementationGuide", description = "Implementation guides supported", type = "reference", target = { 11455 ImplementationGuide.class }) 11456 public static final String SP_GUIDE = "guide"; 11457 /** 11458 * <b>Fluent Client</b> search parameter constant for <b>guide</b> 11459 * <p> 11460 * Description: <b>Implementation guides supported</b><br> 11461 * Type: <b>reference</b><br> 11462 * Path: <b>CapabilityStatement.implementationGuide</b><br> 11463 * </p> 11464 */ 11465 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GUIDE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11466 SP_GUIDE); 11467 11468 /** 11469 * Constant for fluent queries to be used to add include statements. Specifies 11470 * the path value of "<b>CapabilityStatement:guide</b>". 11471 */ 11472 public static final ca.uhn.fhir.model.api.Include INCLUDE_GUIDE = new ca.uhn.fhir.model.api.Include( 11473 "CapabilityStatement:guide").toLocked(); 11474 11475 /** 11476 * Search parameter: <b>status</b> 11477 * <p> 11478 * Description: <b>The current status of the capability statement</b><br> 11479 * Type: <b>token</b><br> 11480 * Path: <b>CapabilityStatement.status</b><br> 11481 * </p> 11482 */ 11483 @SearchParamDefinition(name = "status", path = "CapabilityStatement.status", description = "The current status of the capability statement", type = "token") 11484 public static final String SP_STATUS = "status"; 11485 /** 11486 * <b>Fluent Client</b> search parameter constant for <b>status</b> 11487 * <p> 11488 * Description: <b>The current status of the capability statement</b><br> 11489 * Type: <b>token</b><br> 11490 * Path: <b>CapabilityStatement.status</b><br> 11491 * </p> 11492 */ 11493 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11494 SP_STATUS); 11495 11496}