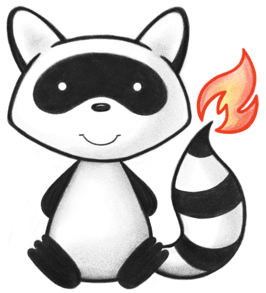
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.instance.model.api.IBaseConformance; 040import org.hl7.fhir.r4.model.Enumerations.FHIRVersion; 041import org.hl7.fhir.r4.model.Enumerations.FHIRVersionEnumFactory; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 043import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 044import org.hl7.fhir.r4.model.Enumerations.SearchParamType; 045import org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory; 046import org.hl7.fhir.utilities.Utilities; 047 048import ca.uhn.fhir.model.api.annotation.Block; 049import ca.uhn.fhir.model.api.annotation.Child; 050import ca.uhn.fhir.model.api.annotation.ChildOrder; 051import ca.uhn.fhir.model.api.annotation.Description; 052import ca.uhn.fhir.model.api.annotation.ResourceDef; 053import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 054 055/** 056 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 057 * Server for a particular version of FHIR that may be used as a statement of 058 * actual server functionality or a statement of required or desired server 059 * implementation. 060 */ 061@ResourceDef(name = "CapabilityStatement", profile = "http://hl7.org/fhir/StructureDefinition/CapabilityStatement") 062@ChildOrder(names = { "url", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", 063 "description", "useContext", "jurisdiction", "purpose", "copyright", "kind", "instantiates", "imports", "software", 064 "implementation", "fhirVersion", "format", "patchFormat", "implementationGuide", "rest", "messaging", "document" }) 065public class CapabilityStatement extends MetadataResource implements IBaseConformance { 066 067 public enum CapabilityStatementKind { 068 /** 069 * The CapabilityStatement instance represents the present capabilities of a 070 * specific system instance. This is the kind returned by /metadata for a FHIR 071 * server end-point. 072 */ 073 INSTANCE, 074 /** 075 * The CapabilityStatement instance represents the capabilities of a system or 076 * piece of software, independent of a particular installation. 077 */ 078 CAPABILITY, 079 /** 080 * The CapabilityStatement instance represents a set of requirements for other 081 * systems to meet; e.g. as part of an implementation guide or 'request for 082 * proposal'. 083 */ 084 REQUIREMENTS, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 090 public static CapabilityStatementKind fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("instance".equals(codeString)) 094 return INSTANCE; 095 if ("capability".equals(codeString)) 096 return CAPABILITY; 097 if ("requirements".equals(codeString)) 098 return REQUIREMENTS; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown CapabilityStatementKind code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case INSTANCE: 108 return "instance"; 109 case CAPABILITY: 110 return "capability"; 111 case REQUIREMENTS: 112 return "requirements"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case INSTANCE: 123 return "http://hl7.org/fhir/capability-statement-kind"; 124 case CAPABILITY: 125 return "http://hl7.org/fhir/capability-statement-kind"; 126 case REQUIREMENTS: 127 return "http://hl7.org/fhir/capability-statement-kind"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case INSTANCE: 138 return "The CapabilityStatement instance represents the present capabilities of a specific system instance. This is the kind returned by /metadata for a FHIR server end-point."; 139 case CAPABILITY: 140 return "The CapabilityStatement instance represents the capabilities of a system or piece of software, independent of a particular installation."; 141 case REQUIREMENTS: 142 return "The CapabilityStatement instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'."; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getDisplay() { 151 switch (this) { 152 case INSTANCE: 153 return "Instance"; 154 case CAPABILITY: 155 return "Capability"; 156 case REQUIREMENTS: 157 return "Requirements"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 } 165 166 public static class CapabilityStatementKindEnumFactory implements EnumFactory<CapabilityStatementKind> { 167 public CapabilityStatementKind fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("instance".equals(codeString)) 172 return CapabilityStatementKind.INSTANCE; 173 if ("capability".equals(codeString)) 174 return CapabilityStatementKind.CAPABILITY; 175 if ("requirements".equals(codeString)) 176 return CapabilityStatementKind.REQUIREMENTS; 177 throw new IllegalArgumentException("Unknown CapabilityStatementKind code '" + codeString + "'"); 178 } 179 180 public Enumeration<CapabilityStatementKind> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.NULL, code); 188 if ("instance".equals(codeString)) 189 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.INSTANCE, code); 190 if ("capability".equals(codeString)) 191 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.CAPABILITY, code); 192 if ("requirements".equals(codeString)) 193 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.REQUIREMENTS, code); 194 throw new FHIRException("Unknown CapabilityStatementKind code '" + codeString + "'"); 195 } 196 197 public String toCode(CapabilityStatementKind code) { 198 if (code == CapabilityStatementKind.INSTANCE) 199 return "instance"; 200 if (code == CapabilityStatementKind.CAPABILITY) 201 return "capability"; 202 if (code == CapabilityStatementKind.REQUIREMENTS) 203 return "requirements"; 204 return "?"; 205 } 206 207 public String toSystem(CapabilityStatementKind code) { 208 return code.getSystem(); 209 } 210 } 211 212 public enum RestfulCapabilityMode { 213 /** 214 * The application acts as a client for this resource. 215 */ 216 CLIENT, 217 /** 218 * The application acts as a server for this resource. 219 */ 220 SERVER, 221 /** 222 * added to help the parsers with the generic types 223 */ 224 NULL; 225 226 public static RestfulCapabilityMode fromCode(String codeString) throws FHIRException { 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("client".equals(codeString)) 230 return CLIENT; 231 if ("server".equals(codeString)) 232 return SERVER; 233 if (Configuration.isAcceptInvalidEnums()) 234 return null; 235 else 236 throw new FHIRException("Unknown RestfulCapabilityMode code '" + codeString + "'"); 237 } 238 239 public String toCode() { 240 switch (this) { 241 case CLIENT: 242 return "client"; 243 case SERVER: 244 return "server"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getSystem() { 253 switch (this) { 254 case CLIENT: 255 return "http://hl7.org/fhir/restful-capability-mode"; 256 case SERVER: 257 return "http://hl7.org/fhir/restful-capability-mode"; 258 case NULL: 259 return null; 260 default: 261 return "?"; 262 } 263 } 264 265 public String getDefinition() { 266 switch (this) { 267 case CLIENT: 268 return "The application acts as a client for this resource."; 269 case SERVER: 270 return "The application acts as a server for this resource."; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 278 public String getDisplay() { 279 switch (this) { 280 case CLIENT: 281 return "Client"; 282 case SERVER: 283 return "Server"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 } 291 292 public static class RestfulCapabilityModeEnumFactory implements EnumFactory<RestfulCapabilityMode> { 293 public RestfulCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 294 if (codeString == null || "".equals(codeString)) 295 if (codeString == null || "".equals(codeString)) 296 return null; 297 if ("client".equals(codeString)) 298 return RestfulCapabilityMode.CLIENT; 299 if ("server".equals(codeString)) 300 return RestfulCapabilityMode.SERVER; 301 throw new IllegalArgumentException("Unknown RestfulCapabilityMode code '" + codeString + "'"); 302 } 303 304 public Enumeration<RestfulCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 305 if (code == null) 306 return null; 307 if (code.isEmpty()) 308 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.NULL, code); 309 String codeString = code.asStringValue(); 310 if (codeString == null || "".equals(codeString)) 311 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.NULL, code); 312 if ("client".equals(codeString)) 313 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.CLIENT, code); 314 if ("server".equals(codeString)) 315 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.SERVER, code); 316 throw new FHIRException("Unknown RestfulCapabilityMode code '" + codeString + "'"); 317 } 318 319 public String toCode(RestfulCapabilityMode code) { 320 if (code == RestfulCapabilityMode.CLIENT) 321 return "client"; 322 if (code == RestfulCapabilityMode.SERVER) 323 return "server"; 324 return "?"; 325 } 326 327 public String toSystem(RestfulCapabilityMode code) { 328 return code.getSystem(); 329 } 330 } 331 332 public enum TypeRestfulInteraction { 333 /** 334 * null 335 */ 336 READ, 337 /** 338 * null 339 */ 340 VREAD, 341 /** 342 * null 343 */ 344 UPDATE, 345 /** 346 * null 347 */ 348 PATCH, 349 /** 350 * null 351 */ 352 DELETE, 353 /** 354 * null 355 */ 356 HISTORYINSTANCE, 357 /** 358 * null 359 */ 360 HISTORYTYPE, 361 /** 362 * null 363 */ 364 CREATE, 365 /** 366 * null 367 */ 368 SEARCHTYPE, 369 /** 370 * added to help the parsers with the generic types 371 */ 372 NULL; 373 374 public static TypeRestfulInteraction fromCode(String codeString) throws FHIRException { 375 if (codeString == null || "".equals(codeString)) 376 return null; 377 if ("read".equals(codeString)) 378 return READ; 379 if ("vread".equals(codeString)) 380 return VREAD; 381 if ("update".equals(codeString)) 382 return UPDATE; 383 if ("patch".equals(codeString)) 384 return PATCH; 385 if ("delete".equals(codeString)) 386 return DELETE; 387 if ("history-instance".equals(codeString)) 388 return HISTORYINSTANCE; 389 if ("history-type".equals(codeString)) 390 return HISTORYTYPE; 391 if ("create".equals(codeString)) 392 return CREATE; 393 if ("search-type".equals(codeString)) 394 return SEARCHTYPE; 395 if (Configuration.isAcceptInvalidEnums()) 396 return null; 397 else 398 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 399 } 400 401 public String toCode() { 402 switch (this) { 403 case READ: 404 return "read"; 405 case VREAD: 406 return "vread"; 407 case UPDATE: 408 return "update"; 409 case PATCH: 410 return "patch"; 411 case DELETE: 412 return "delete"; 413 case HISTORYINSTANCE: 414 return "history-instance"; 415 case HISTORYTYPE: 416 return "history-type"; 417 case CREATE: 418 return "create"; 419 case SEARCHTYPE: 420 return "search-type"; 421 case NULL: 422 return null; 423 default: 424 return "?"; 425 } 426 } 427 428 public String getSystem() { 429 switch (this) { 430 case READ: 431 return "http://hl7.org/fhir/restful-interaction"; 432 case VREAD: 433 return "http://hl7.org/fhir/restful-interaction"; 434 case UPDATE: 435 return "http://hl7.org/fhir/restful-interaction"; 436 case PATCH: 437 return "http://hl7.org/fhir/restful-interaction"; 438 case DELETE: 439 return "http://hl7.org/fhir/restful-interaction"; 440 case HISTORYINSTANCE: 441 return "http://hl7.org/fhir/restful-interaction"; 442 case HISTORYTYPE: 443 return "http://hl7.org/fhir/restful-interaction"; 444 case CREATE: 445 return "http://hl7.org/fhir/restful-interaction"; 446 case SEARCHTYPE: 447 return "http://hl7.org/fhir/restful-interaction"; 448 case NULL: 449 return null; 450 default: 451 return "?"; 452 } 453 } 454 455 public String getDefinition() { 456 switch (this) { 457 case READ: 458 return ""; 459 case VREAD: 460 return ""; 461 case UPDATE: 462 return ""; 463 case PATCH: 464 return ""; 465 case DELETE: 466 return ""; 467 case HISTORYINSTANCE: 468 return ""; 469 case HISTORYTYPE: 470 return ""; 471 case CREATE: 472 return ""; 473 case SEARCHTYPE: 474 return ""; 475 case NULL: 476 return null; 477 default: 478 return "?"; 479 } 480 } 481 482 public String getDisplay() { 483 switch (this) { 484 case READ: 485 return "read"; 486 case VREAD: 487 return "vread"; 488 case UPDATE: 489 return "update"; 490 case PATCH: 491 return "patch"; 492 case DELETE: 493 return "delete"; 494 case HISTORYINSTANCE: 495 return "history-instance"; 496 case HISTORYTYPE: 497 return "history-type"; 498 case CREATE: 499 return "create"; 500 case SEARCHTYPE: 501 return "search-type"; 502 case NULL: 503 return null; 504 default: 505 return "?"; 506 } 507 } 508 } 509 510 public static class TypeRestfulInteractionEnumFactory implements EnumFactory<TypeRestfulInteraction> { 511 public TypeRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 512 if (codeString == null || "".equals(codeString)) 513 if (codeString == null || "".equals(codeString)) 514 return null; 515 if ("read".equals(codeString)) 516 return TypeRestfulInteraction.READ; 517 if ("vread".equals(codeString)) 518 return TypeRestfulInteraction.VREAD; 519 if ("update".equals(codeString)) 520 return TypeRestfulInteraction.UPDATE; 521 if ("patch".equals(codeString)) 522 return TypeRestfulInteraction.PATCH; 523 if ("delete".equals(codeString)) 524 return TypeRestfulInteraction.DELETE; 525 if ("history-instance".equals(codeString)) 526 return TypeRestfulInteraction.HISTORYINSTANCE; 527 if ("history-type".equals(codeString)) 528 return TypeRestfulInteraction.HISTORYTYPE; 529 if ("create".equals(codeString)) 530 return TypeRestfulInteraction.CREATE; 531 if ("search-type".equals(codeString)) 532 return TypeRestfulInteraction.SEARCHTYPE; 533 throw new IllegalArgumentException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 534 } 535 536 public Enumeration<TypeRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 537 if (code == null) 538 return null; 539 if (code.isEmpty()) 540 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.NULL, code); 541 String codeString = code.asStringValue(); 542 if (codeString == null || "".equals(codeString)) 543 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.NULL, code); 544 if ("read".equals(codeString)) 545 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.READ, code); 546 if ("vread".equals(codeString)) 547 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VREAD, code); 548 if ("update".equals(codeString)) 549 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.UPDATE, code); 550 if ("patch".equals(codeString)) 551 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.PATCH, code); 552 if ("delete".equals(codeString)) 553 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.DELETE, code); 554 if ("history-instance".equals(codeString)) 555 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYINSTANCE, code); 556 if ("history-type".equals(codeString)) 557 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYTYPE, code); 558 if ("create".equals(codeString)) 559 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.CREATE, code); 560 if ("search-type".equals(codeString)) 561 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.SEARCHTYPE, code); 562 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 563 } 564 565 public String toCode(TypeRestfulInteraction code) { 566 if (code == TypeRestfulInteraction.READ) 567 return "read"; 568 if (code == TypeRestfulInteraction.VREAD) 569 return "vread"; 570 if (code == TypeRestfulInteraction.UPDATE) 571 return "update"; 572 if (code == TypeRestfulInteraction.PATCH) 573 return "patch"; 574 if (code == TypeRestfulInteraction.DELETE) 575 return "delete"; 576 if (code == TypeRestfulInteraction.HISTORYINSTANCE) 577 return "history-instance"; 578 if (code == TypeRestfulInteraction.HISTORYTYPE) 579 return "history-type"; 580 if (code == TypeRestfulInteraction.CREATE) 581 return "create"; 582 if (code == TypeRestfulInteraction.SEARCHTYPE) 583 return "search-type"; 584 return "?"; 585 } 586 587 public String toSystem(TypeRestfulInteraction code) { 588 return code.getSystem(); 589 } 590 } 591 592 public enum ResourceVersionPolicy { 593 /** 594 * VersionId meta-property is not supported (server) or used (client). 595 */ 596 NOVERSION, 597 /** 598 * VersionId meta-property is supported (server) or used (client). 599 */ 600 VERSIONED, 601 /** 602 * VersionId must be correct for updates (server) or will be specified (If-match 603 * header) for updates (client). 604 */ 605 VERSIONEDUPDATE, 606 /** 607 * added to help the parsers with the generic types 608 */ 609 NULL; 610 611 public static ResourceVersionPolicy fromCode(String codeString) throws FHIRException { 612 if (codeString == null || "".equals(codeString)) 613 return null; 614 if ("no-version".equals(codeString)) 615 return NOVERSION; 616 if ("versioned".equals(codeString)) 617 return VERSIONED; 618 if ("versioned-update".equals(codeString)) 619 return VERSIONEDUPDATE; 620 if (Configuration.isAcceptInvalidEnums()) 621 return null; 622 else 623 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 624 } 625 626 public String toCode() { 627 switch (this) { 628 case NOVERSION: 629 return "no-version"; 630 case VERSIONED: 631 return "versioned"; 632 case VERSIONEDUPDATE: 633 return "versioned-update"; 634 case NULL: 635 return null; 636 default: 637 return "?"; 638 } 639 } 640 641 public String getSystem() { 642 switch (this) { 643 case NOVERSION: 644 return "http://hl7.org/fhir/versioning-policy"; 645 case VERSIONED: 646 return "http://hl7.org/fhir/versioning-policy"; 647 case VERSIONEDUPDATE: 648 return "http://hl7.org/fhir/versioning-policy"; 649 case NULL: 650 return null; 651 default: 652 return "?"; 653 } 654 } 655 656 public String getDefinition() { 657 switch (this) { 658 case NOVERSION: 659 return "VersionId meta-property is not supported (server) or used (client)."; 660 case VERSIONED: 661 return "VersionId meta-property is supported (server) or used (client)."; 662 case VERSIONEDUPDATE: 663 return "VersionId must be correct for updates (server) or will be specified (If-match header) for updates (client)."; 664 case NULL: 665 return null; 666 default: 667 return "?"; 668 } 669 } 670 671 public String getDisplay() { 672 switch (this) { 673 case NOVERSION: 674 return "No VersionId Support"; 675 case VERSIONED: 676 return "Versioned"; 677 case VERSIONEDUPDATE: 678 return "VersionId tracked fully"; 679 case NULL: 680 return null; 681 default: 682 return "?"; 683 } 684 } 685 } 686 687 public static class ResourceVersionPolicyEnumFactory implements EnumFactory<ResourceVersionPolicy> { 688 public ResourceVersionPolicy fromCode(String codeString) throws IllegalArgumentException { 689 if (codeString == null || "".equals(codeString)) 690 if (codeString == null || "".equals(codeString)) 691 return null; 692 if ("no-version".equals(codeString)) 693 return ResourceVersionPolicy.NOVERSION; 694 if ("versioned".equals(codeString)) 695 return ResourceVersionPolicy.VERSIONED; 696 if ("versioned-update".equals(codeString)) 697 return ResourceVersionPolicy.VERSIONEDUPDATE; 698 throw new IllegalArgumentException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 699 } 700 701 public Enumeration<ResourceVersionPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 702 if (code == null) 703 return null; 704 if (code.isEmpty()) 705 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NULL, code); 706 String codeString = code.asStringValue(); 707 if (codeString == null || "".equals(codeString)) 708 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NULL, code); 709 if ("no-version".equals(codeString)) 710 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NOVERSION, code); 711 if ("versioned".equals(codeString)) 712 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONED, code); 713 if ("versioned-update".equals(codeString)) 714 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONEDUPDATE, code); 715 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 716 } 717 718 public String toCode(ResourceVersionPolicy code) { 719 if (code == ResourceVersionPolicy.NOVERSION) 720 return "no-version"; 721 if (code == ResourceVersionPolicy.VERSIONED) 722 return "versioned"; 723 if (code == ResourceVersionPolicy.VERSIONEDUPDATE) 724 return "versioned-update"; 725 return "?"; 726 } 727 728 public String toSystem(ResourceVersionPolicy code) { 729 return code.getSystem(); 730 } 731 } 732 733 public enum ConditionalReadStatus { 734 /** 735 * No support for conditional reads. 736 */ 737 NOTSUPPORTED, 738 /** 739 * Conditional reads are supported, but only with the If-Modified-Since HTTP 740 * Header. 741 */ 742 MODIFIEDSINCE, 743 /** 744 * Conditional reads are supported, but only with the If-None-Match HTTP Header. 745 */ 746 NOTMATCH, 747 /** 748 * Conditional reads are supported, with both If-Modified-Since and 749 * If-None-Match HTTP Headers. 750 */ 751 FULLSUPPORT, 752 /** 753 * added to help the parsers with the generic types 754 */ 755 NULL; 756 757 public static ConditionalReadStatus fromCode(String codeString) throws FHIRException { 758 if (codeString == null || "".equals(codeString)) 759 return null; 760 if ("not-supported".equals(codeString)) 761 return NOTSUPPORTED; 762 if ("modified-since".equals(codeString)) 763 return MODIFIEDSINCE; 764 if ("not-match".equals(codeString)) 765 return NOTMATCH; 766 if ("full-support".equals(codeString)) 767 return FULLSUPPORT; 768 if (Configuration.isAcceptInvalidEnums()) 769 return null; 770 else 771 throw new FHIRException("Unknown ConditionalReadStatus code '" + codeString + "'"); 772 } 773 774 public String toCode() { 775 switch (this) { 776 case NOTSUPPORTED: 777 return "not-supported"; 778 case MODIFIEDSINCE: 779 return "modified-since"; 780 case NOTMATCH: 781 return "not-match"; 782 case FULLSUPPORT: 783 return "full-support"; 784 case NULL: 785 return null; 786 default: 787 return "?"; 788 } 789 } 790 791 public String getSystem() { 792 switch (this) { 793 case NOTSUPPORTED: 794 return "http://hl7.org/fhir/conditional-read-status"; 795 case MODIFIEDSINCE: 796 return "http://hl7.org/fhir/conditional-read-status"; 797 case NOTMATCH: 798 return "http://hl7.org/fhir/conditional-read-status"; 799 case FULLSUPPORT: 800 return "http://hl7.org/fhir/conditional-read-status"; 801 case NULL: 802 return null; 803 default: 804 return "?"; 805 } 806 } 807 808 public String getDefinition() { 809 switch (this) { 810 case NOTSUPPORTED: 811 return "No support for conditional reads."; 812 case MODIFIEDSINCE: 813 return "Conditional reads are supported, but only with the If-Modified-Since HTTP Header."; 814 case NOTMATCH: 815 return "Conditional reads are supported, but only with the If-None-Match HTTP Header."; 816 case FULLSUPPORT: 817 return "Conditional reads are supported, with both If-Modified-Since and If-None-Match HTTP Headers."; 818 case NULL: 819 return null; 820 default: 821 return "?"; 822 } 823 } 824 825 public String getDisplay() { 826 switch (this) { 827 case NOTSUPPORTED: 828 return "Not Supported"; 829 case MODIFIEDSINCE: 830 return "If-Modified-Since"; 831 case NOTMATCH: 832 return "If-None-Match"; 833 case FULLSUPPORT: 834 return "Full Support"; 835 case NULL: 836 return null; 837 default: 838 return "?"; 839 } 840 } 841 } 842 843 public static class ConditionalReadStatusEnumFactory implements EnumFactory<ConditionalReadStatus> { 844 public ConditionalReadStatus fromCode(String codeString) throws IllegalArgumentException { 845 if (codeString == null || "".equals(codeString)) 846 if (codeString == null || "".equals(codeString)) 847 return null; 848 if ("not-supported".equals(codeString)) 849 return ConditionalReadStatus.NOTSUPPORTED; 850 if ("modified-since".equals(codeString)) 851 return ConditionalReadStatus.MODIFIEDSINCE; 852 if ("not-match".equals(codeString)) 853 return ConditionalReadStatus.NOTMATCH; 854 if ("full-support".equals(codeString)) 855 return ConditionalReadStatus.FULLSUPPORT; 856 throw new IllegalArgumentException("Unknown ConditionalReadStatus code '" + codeString + "'"); 857 } 858 859 public Enumeration<ConditionalReadStatus> fromType(PrimitiveType<?> code) throws FHIRException { 860 if (code == null) 861 return null; 862 if (code.isEmpty()) 863 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NULL, code); 864 String codeString = code.asStringValue(); 865 if (codeString == null || "".equals(codeString)) 866 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NULL, code); 867 if ("not-supported".equals(codeString)) 868 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTSUPPORTED, code); 869 if ("modified-since".equals(codeString)) 870 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.MODIFIEDSINCE, code); 871 if ("not-match".equals(codeString)) 872 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTMATCH, code); 873 if ("full-support".equals(codeString)) 874 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.FULLSUPPORT, code); 875 throw new FHIRException("Unknown ConditionalReadStatus code '" + codeString + "'"); 876 } 877 878 public String toCode(ConditionalReadStatus code) { 879 if (code == ConditionalReadStatus.NOTSUPPORTED) 880 return "not-supported"; 881 if (code == ConditionalReadStatus.MODIFIEDSINCE) 882 return "modified-since"; 883 if (code == ConditionalReadStatus.NOTMATCH) 884 return "not-match"; 885 if (code == ConditionalReadStatus.FULLSUPPORT) 886 return "full-support"; 887 return "?"; 888 } 889 890 public String toSystem(ConditionalReadStatus code) { 891 return code.getSystem(); 892 } 893 } 894 895 public enum ConditionalDeleteStatus { 896 /** 897 * No support for conditional deletes. 898 */ 899 NOTSUPPORTED, 900 /** 901 * Conditional deletes are supported, but only single resources at a time. 902 */ 903 SINGLE, 904 /** 905 * Conditional deletes are supported, and multiple resources can be deleted in a 906 * single interaction. 907 */ 908 MULTIPLE, 909 /** 910 * added to help the parsers with the generic types 911 */ 912 NULL; 913 914 public static ConditionalDeleteStatus fromCode(String codeString) throws FHIRException { 915 if (codeString == null || "".equals(codeString)) 916 return null; 917 if ("not-supported".equals(codeString)) 918 return NOTSUPPORTED; 919 if ("single".equals(codeString)) 920 return SINGLE; 921 if ("multiple".equals(codeString)) 922 return MULTIPLE; 923 if (Configuration.isAcceptInvalidEnums()) 924 return null; 925 else 926 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 927 } 928 929 public String toCode() { 930 switch (this) { 931 case NOTSUPPORTED: 932 return "not-supported"; 933 case SINGLE: 934 return "single"; 935 case MULTIPLE: 936 return "multiple"; 937 case NULL: 938 return null; 939 default: 940 return "?"; 941 } 942 } 943 944 public String getSystem() { 945 switch (this) { 946 case NOTSUPPORTED: 947 return "http://hl7.org/fhir/conditional-delete-status"; 948 case SINGLE: 949 return "http://hl7.org/fhir/conditional-delete-status"; 950 case MULTIPLE: 951 return "http://hl7.org/fhir/conditional-delete-status"; 952 case NULL: 953 return null; 954 default: 955 return "?"; 956 } 957 } 958 959 public String getDefinition() { 960 switch (this) { 961 case NOTSUPPORTED: 962 return "No support for conditional deletes."; 963 case SINGLE: 964 return "Conditional deletes are supported, but only single resources at a time."; 965 case MULTIPLE: 966 return "Conditional deletes are supported, and multiple resources can be deleted in a single interaction."; 967 case NULL: 968 return null; 969 default: 970 return "?"; 971 } 972 } 973 974 public String getDisplay() { 975 switch (this) { 976 case NOTSUPPORTED: 977 return "Not Supported"; 978 case SINGLE: 979 return "Single Deletes Supported"; 980 case MULTIPLE: 981 return "Multiple Deletes Supported"; 982 case NULL: 983 return null; 984 default: 985 return "?"; 986 } 987 } 988 } 989 990 public static class ConditionalDeleteStatusEnumFactory implements EnumFactory<ConditionalDeleteStatus> { 991 public ConditionalDeleteStatus fromCode(String codeString) throws IllegalArgumentException { 992 if (codeString == null || "".equals(codeString)) 993 if (codeString == null || "".equals(codeString)) 994 return null; 995 if ("not-supported".equals(codeString)) 996 return ConditionalDeleteStatus.NOTSUPPORTED; 997 if ("single".equals(codeString)) 998 return ConditionalDeleteStatus.SINGLE; 999 if ("multiple".equals(codeString)) 1000 return ConditionalDeleteStatus.MULTIPLE; 1001 throw new IllegalArgumentException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 1002 } 1003 1004 public Enumeration<ConditionalDeleteStatus> fromType(PrimitiveType<?> code) throws FHIRException { 1005 if (code == null) 1006 return null; 1007 if (code.isEmpty()) 1008 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NULL, code); 1009 String codeString = code.asStringValue(); 1010 if (codeString == null || "".equals(codeString)) 1011 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NULL, code); 1012 if ("not-supported".equals(codeString)) 1013 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NOTSUPPORTED, code); 1014 if ("single".equals(codeString)) 1015 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.SINGLE, code); 1016 if ("multiple".equals(codeString)) 1017 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.MULTIPLE, code); 1018 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 1019 } 1020 1021 public String toCode(ConditionalDeleteStatus code) { 1022 if (code == ConditionalDeleteStatus.NOTSUPPORTED) 1023 return "not-supported"; 1024 if (code == ConditionalDeleteStatus.SINGLE) 1025 return "single"; 1026 if (code == ConditionalDeleteStatus.MULTIPLE) 1027 return "multiple"; 1028 return "?"; 1029 } 1030 1031 public String toSystem(ConditionalDeleteStatus code) { 1032 return code.getSystem(); 1033 } 1034 } 1035 1036 public enum ReferenceHandlingPolicy { 1037 /** 1038 * The server supports and populates Literal references (i.e. using 1039 * Reference.reference) where they are known (this code does not guarantee that 1040 * all references are literal; see 'enforced'). 1041 */ 1042 LITERAL, 1043 /** 1044 * The server allows logical references (i.e. using Reference.identifier). 1045 */ 1046 LOGICAL, 1047 /** 1048 * The server will attempt to resolve logical references to literal references - 1049 * i.e. converting Reference.identifier to Reference.reference (if resolution 1050 * fails, the server may still accept resources; see logical). 1051 */ 1052 RESOLVES, 1053 /** 1054 * The server enforces that references have integrity - e.g. it ensures that 1055 * references can always be resolved. This is typically the case for clinical 1056 * record systems, but often not the case for middleware/proxy systems. 1057 */ 1058 ENFORCED, 1059 /** 1060 * The server does not support references that point to other servers. 1061 */ 1062 LOCAL, 1063 /** 1064 * added to help the parsers with the generic types 1065 */ 1066 NULL; 1067 1068 public static ReferenceHandlingPolicy fromCode(String codeString) throws FHIRException { 1069 if (codeString == null || "".equals(codeString)) 1070 return null; 1071 if ("literal".equals(codeString)) 1072 return LITERAL; 1073 if ("logical".equals(codeString)) 1074 return LOGICAL; 1075 if ("resolves".equals(codeString)) 1076 return RESOLVES; 1077 if ("enforced".equals(codeString)) 1078 return ENFORCED; 1079 if ("local".equals(codeString)) 1080 return LOCAL; 1081 if (Configuration.isAcceptInvalidEnums()) 1082 return null; 1083 else 1084 throw new FHIRException("Unknown ReferenceHandlingPolicy code '" + codeString + "'"); 1085 } 1086 1087 public String toCode() { 1088 switch (this) { 1089 case LITERAL: 1090 return "literal"; 1091 case LOGICAL: 1092 return "logical"; 1093 case RESOLVES: 1094 return "resolves"; 1095 case ENFORCED: 1096 return "enforced"; 1097 case LOCAL: 1098 return "local"; 1099 case NULL: 1100 return null; 1101 default: 1102 return "?"; 1103 } 1104 } 1105 1106 public String getSystem() { 1107 switch (this) { 1108 case LITERAL: 1109 return "http://hl7.org/fhir/reference-handling-policy"; 1110 case LOGICAL: 1111 return "http://hl7.org/fhir/reference-handling-policy"; 1112 case RESOLVES: 1113 return "http://hl7.org/fhir/reference-handling-policy"; 1114 case ENFORCED: 1115 return "http://hl7.org/fhir/reference-handling-policy"; 1116 case LOCAL: 1117 return "http://hl7.org/fhir/reference-handling-policy"; 1118 case NULL: 1119 return null; 1120 default: 1121 return "?"; 1122 } 1123 } 1124 1125 public String getDefinition() { 1126 switch (this) { 1127 case LITERAL: 1128 return "The server supports and populates Literal references (i.e. using Reference.reference) where they are known (this code does not guarantee that all references are literal; see 'enforced')."; 1129 case LOGICAL: 1130 return "The server allows logical references (i.e. using Reference.identifier)."; 1131 case RESOLVES: 1132 return "The server will attempt to resolve logical references to literal references - i.e. converting Reference.identifier to Reference.reference (if resolution fails, the server may still accept resources; see logical)."; 1133 case ENFORCED: 1134 return "The server enforces that references have integrity - e.g. it ensures that references can always be resolved. This is typically the case for clinical record systems, but often not the case for middleware/proxy systems."; 1135 case LOCAL: 1136 return "The server does not support references that point to other servers."; 1137 case NULL: 1138 return null; 1139 default: 1140 return "?"; 1141 } 1142 } 1143 1144 public String getDisplay() { 1145 switch (this) { 1146 case LITERAL: 1147 return "Literal References"; 1148 case LOGICAL: 1149 return "Logical References"; 1150 case RESOLVES: 1151 return "Resolves References"; 1152 case ENFORCED: 1153 return "Reference Integrity Enforced"; 1154 case LOCAL: 1155 return "Local References Only"; 1156 case NULL: 1157 return null; 1158 default: 1159 return "?"; 1160 } 1161 } 1162 } 1163 1164 public static class ReferenceHandlingPolicyEnumFactory implements EnumFactory<ReferenceHandlingPolicy> { 1165 public ReferenceHandlingPolicy fromCode(String codeString) throws IllegalArgumentException { 1166 if (codeString == null || "".equals(codeString)) 1167 if (codeString == null || "".equals(codeString)) 1168 return null; 1169 if ("literal".equals(codeString)) 1170 return ReferenceHandlingPolicy.LITERAL; 1171 if ("logical".equals(codeString)) 1172 return ReferenceHandlingPolicy.LOGICAL; 1173 if ("resolves".equals(codeString)) 1174 return ReferenceHandlingPolicy.RESOLVES; 1175 if ("enforced".equals(codeString)) 1176 return ReferenceHandlingPolicy.ENFORCED; 1177 if ("local".equals(codeString)) 1178 return ReferenceHandlingPolicy.LOCAL; 1179 throw new IllegalArgumentException("Unknown ReferenceHandlingPolicy code '" + codeString + "'"); 1180 } 1181 1182 public Enumeration<ReferenceHandlingPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 1183 if (code == null) 1184 return null; 1185 if (code.isEmpty()) 1186 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.NULL, code); 1187 String codeString = code.asStringValue(); 1188 if (codeString == null || "".equals(codeString)) 1189 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.NULL, code); 1190 if ("literal".equals(codeString)) 1191 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LITERAL, code); 1192 if ("logical".equals(codeString)) 1193 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOGICAL, code); 1194 if ("resolves".equals(codeString)) 1195 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.RESOLVES, code); 1196 if ("enforced".equals(codeString)) 1197 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.ENFORCED, code); 1198 if ("local".equals(codeString)) 1199 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOCAL, code); 1200 throw new FHIRException("Unknown ReferenceHandlingPolicy code '" + codeString + "'"); 1201 } 1202 1203 public String toCode(ReferenceHandlingPolicy code) { 1204 if (code == ReferenceHandlingPolicy.LITERAL) 1205 return "literal"; 1206 if (code == ReferenceHandlingPolicy.LOGICAL) 1207 return "logical"; 1208 if (code == ReferenceHandlingPolicy.RESOLVES) 1209 return "resolves"; 1210 if (code == ReferenceHandlingPolicy.ENFORCED) 1211 return "enforced"; 1212 if (code == ReferenceHandlingPolicy.LOCAL) 1213 return "local"; 1214 return "?"; 1215 } 1216 1217 public String toSystem(ReferenceHandlingPolicy code) { 1218 return code.getSystem(); 1219 } 1220 } 1221 1222 public enum SystemRestfulInteraction { 1223 /** 1224 * null 1225 */ 1226 TRANSACTION, 1227 /** 1228 * null 1229 */ 1230 BATCH, 1231 /** 1232 * null 1233 */ 1234 SEARCHSYSTEM, 1235 /** 1236 * null 1237 */ 1238 HISTORYSYSTEM, 1239 /** 1240 * added to help the parsers with the generic types 1241 */ 1242 NULL; 1243 1244 public static SystemRestfulInteraction fromCode(String codeString) throws FHIRException { 1245 if (codeString == null || "".equals(codeString)) 1246 return null; 1247 if ("transaction".equals(codeString)) 1248 return TRANSACTION; 1249 if ("batch".equals(codeString)) 1250 return BATCH; 1251 if ("search-system".equals(codeString)) 1252 return SEARCHSYSTEM; 1253 if ("history-system".equals(codeString)) 1254 return HISTORYSYSTEM; 1255 if (Configuration.isAcceptInvalidEnums()) 1256 return null; 1257 else 1258 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1259 } 1260 1261 public String toCode() { 1262 switch (this) { 1263 case TRANSACTION: 1264 return "transaction"; 1265 case BATCH: 1266 return "batch"; 1267 case SEARCHSYSTEM: 1268 return "search-system"; 1269 case HISTORYSYSTEM: 1270 return "history-system"; 1271 case NULL: 1272 return null; 1273 default: 1274 return "?"; 1275 } 1276 } 1277 1278 public String getSystem() { 1279 switch (this) { 1280 case TRANSACTION: 1281 return "http://hl7.org/fhir/restful-interaction"; 1282 case BATCH: 1283 return "http://hl7.org/fhir/restful-interaction"; 1284 case SEARCHSYSTEM: 1285 return "http://hl7.org/fhir/restful-interaction"; 1286 case HISTORYSYSTEM: 1287 return "http://hl7.org/fhir/restful-interaction"; 1288 case NULL: 1289 return null; 1290 default: 1291 return "?"; 1292 } 1293 } 1294 1295 public String getDefinition() { 1296 switch (this) { 1297 case TRANSACTION: 1298 return ""; 1299 case BATCH: 1300 return ""; 1301 case SEARCHSYSTEM: 1302 return ""; 1303 case HISTORYSYSTEM: 1304 return ""; 1305 case NULL: 1306 return null; 1307 default: 1308 return "?"; 1309 } 1310 } 1311 1312 public String getDisplay() { 1313 switch (this) { 1314 case TRANSACTION: 1315 return "transaction"; 1316 case BATCH: 1317 return "batch"; 1318 case SEARCHSYSTEM: 1319 return "search-system"; 1320 case HISTORYSYSTEM: 1321 return "history-system"; 1322 case NULL: 1323 return null; 1324 default: 1325 return "?"; 1326 } 1327 } 1328 } 1329 1330 public static class SystemRestfulInteractionEnumFactory implements EnumFactory<SystemRestfulInteraction> { 1331 public SystemRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 1332 if (codeString == null || "".equals(codeString)) 1333 if (codeString == null || "".equals(codeString)) 1334 return null; 1335 if ("transaction".equals(codeString)) 1336 return SystemRestfulInteraction.TRANSACTION; 1337 if ("batch".equals(codeString)) 1338 return SystemRestfulInteraction.BATCH; 1339 if ("search-system".equals(codeString)) 1340 return SystemRestfulInteraction.SEARCHSYSTEM; 1341 if ("history-system".equals(codeString)) 1342 return SystemRestfulInteraction.HISTORYSYSTEM; 1343 throw new IllegalArgumentException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1344 } 1345 1346 public Enumeration<SystemRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 1347 if (code == null) 1348 return null; 1349 if (code.isEmpty()) 1350 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.NULL, code); 1351 String codeString = code.asStringValue(); 1352 if (codeString == null || "".equals(codeString)) 1353 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.NULL, code); 1354 if ("transaction".equals(codeString)) 1355 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.TRANSACTION, code); 1356 if ("batch".equals(codeString)) 1357 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.BATCH, code); 1358 if ("search-system".equals(codeString)) 1359 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.SEARCHSYSTEM, code); 1360 if ("history-system".equals(codeString)) 1361 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.HISTORYSYSTEM, code); 1362 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1363 } 1364 1365 public String toCode(SystemRestfulInteraction code) { 1366 if (code == SystemRestfulInteraction.TRANSACTION) 1367 return "transaction"; 1368 if (code == SystemRestfulInteraction.BATCH) 1369 return "batch"; 1370 if (code == SystemRestfulInteraction.SEARCHSYSTEM) 1371 return "search-system"; 1372 if (code == SystemRestfulInteraction.HISTORYSYSTEM) 1373 return "history-system"; 1374 return "?"; 1375 } 1376 1377 public String toSystem(SystemRestfulInteraction code) { 1378 return code.getSystem(); 1379 } 1380 } 1381 1382 public enum EventCapabilityMode { 1383 /** 1384 * The application sends requests and receives responses. 1385 */ 1386 SENDER, 1387 /** 1388 * The application receives requests and sends responses. 1389 */ 1390 RECEIVER, 1391 /** 1392 * added to help the parsers with the generic types 1393 */ 1394 NULL; 1395 1396 public static EventCapabilityMode fromCode(String codeString) throws FHIRException { 1397 if (codeString == null || "".equals(codeString)) 1398 return null; 1399 if ("sender".equals(codeString)) 1400 return SENDER; 1401 if ("receiver".equals(codeString)) 1402 return RECEIVER; 1403 if (Configuration.isAcceptInvalidEnums()) 1404 return null; 1405 else 1406 throw new FHIRException("Unknown EventCapabilityMode code '" + codeString + "'"); 1407 } 1408 1409 public String toCode() { 1410 switch (this) { 1411 case SENDER: 1412 return "sender"; 1413 case RECEIVER: 1414 return "receiver"; 1415 case NULL: 1416 return null; 1417 default: 1418 return "?"; 1419 } 1420 } 1421 1422 public String getSystem() { 1423 switch (this) { 1424 case SENDER: 1425 return "http://hl7.org/fhir/event-capability-mode"; 1426 case RECEIVER: 1427 return "http://hl7.org/fhir/event-capability-mode"; 1428 case NULL: 1429 return null; 1430 default: 1431 return "?"; 1432 } 1433 } 1434 1435 public String getDefinition() { 1436 switch (this) { 1437 case SENDER: 1438 return "The application sends requests and receives responses."; 1439 case RECEIVER: 1440 return "The application receives requests and sends responses."; 1441 case NULL: 1442 return null; 1443 default: 1444 return "?"; 1445 } 1446 } 1447 1448 public String getDisplay() { 1449 switch (this) { 1450 case SENDER: 1451 return "Sender"; 1452 case RECEIVER: 1453 return "Receiver"; 1454 case NULL: 1455 return null; 1456 default: 1457 return "?"; 1458 } 1459 } 1460 } 1461 1462 public static class EventCapabilityModeEnumFactory implements EnumFactory<EventCapabilityMode> { 1463 public EventCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 1464 if (codeString == null || "".equals(codeString)) 1465 if (codeString == null || "".equals(codeString)) 1466 return null; 1467 if ("sender".equals(codeString)) 1468 return EventCapabilityMode.SENDER; 1469 if ("receiver".equals(codeString)) 1470 return EventCapabilityMode.RECEIVER; 1471 throw new IllegalArgumentException("Unknown EventCapabilityMode code '" + codeString + "'"); 1472 } 1473 1474 public Enumeration<EventCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 1475 if (code == null) 1476 return null; 1477 if (code.isEmpty()) 1478 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.NULL, code); 1479 String codeString = code.asStringValue(); 1480 if (codeString == null || "".equals(codeString)) 1481 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.NULL, code); 1482 if ("sender".equals(codeString)) 1483 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.SENDER, code); 1484 if ("receiver".equals(codeString)) 1485 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.RECEIVER, code); 1486 throw new FHIRException("Unknown EventCapabilityMode code '" + codeString + "'"); 1487 } 1488 1489 public String toCode(EventCapabilityMode code) { 1490 if (code == EventCapabilityMode.SENDER) 1491 return "sender"; 1492 if (code == EventCapabilityMode.RECEIVER) 1493 return "receiver"; 1494 return "?"; 1495 } 1496 1497 public String toSystem(EventCapabilityMode code) { 1498 return code.getSystem(); 1499 } 1500 } 1501 1502 public enum DocumentMode { 1503 /** 1504 * The application produces documents of the specified type. 1505 */ 1506 PRODUCER, 1507 /** 1508 * The application consumes documents of the specified type. 1509 */ 1510 CONSUMER, 1511 /** 1512 * added to help the parsers with the generic types 1513 */ 1514 NULL; 1515 1516 public static DocumentMode fromCode(String codeString) throws FHIRException { 1517 if (codeString == null || "".equals(codeString)) 1518 return null; 1519 if ("producer".equals(codeString)) 1520 return PRODUCER; 1521 if ("consumer".equals(codeString)) 1522 return CONSUMER; 1523 if (Configuration.isAcceptInvalidEnums()) 1524 return null; 1525 else 1526 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1527 } 1528 1529 public String toCode() { 1530 switch (this) { 1531 case PRODUCER: 1532 return "producer"; 1533 case CONSUMER: 1534 return "consumer"; 1535 case NULL: 1536 return null; 1537 default: 1538 return "?"; 1539 } 1540 } 1541 1542 public String getSystem() { 1543 switch (this) { 1544 case PRODUCER: 1545 return "http://hl7.org/fhir/document-mode"; 1546 case CONSUMER: 1547 return "http://hl7.org/fhir/document-mode"; 1548 case NULL: 1549 return null; 1550 default: 1551 return "?"; 1552 } 1553 } 1554 1555 public String getDefinition() { 1556 switch (this) { 1557 case PRODUCER: 1558 return "The application produces documents of the specified type."; 1559 case CONSUMER: 1560 return "The application consumes documents of the specified type."; 1561 case NULL: 1562 return null; 1563 default: 1564 return "?"; 1565 } 1566 } 1567 1568 public String getDisplay() { 1569 switch (this) { 1570 case PRODUCER: 1571 return "Producer"; 1572 case CONSUMER: 1573 return "Consumer"; 1574 case NULL: 1575 return null; 1576 default: 1577 return "?"; 1578 } 1579 } 1580 } 1581 1582 public static class DocumentModeEnumFactory implements EnumFactory<DocumentMode> { 1583 public DocumentMode fromCode(String codeString) throws IllegalArgumentException { 1584 if (codeString == null || "".equals(codeString)) 1585 if (codeString == null || "".equals(codeString)) 1586 return null; 1587 if ("producer".equals(codeString)) 1588 return DocumentMode.PRODUCER; 1589 if ("consumer".equals(codeString)) 1590 return DocumentMode.CONSUMER; 1591 throw new IllegalArgumentException("Unknown DocumentMode code '" + codeString + "'"); 1592 } 1593 1594 public Enumeration<DocumentMode> fromType(PrimitiveType<?> code) throws FHIRException { 1595 if (code == null) 1596 return null; 1597 if (code.isEmpty()) 1598 return new Enumeration<DocumentMode>(this, DocumentMode.NULL, code); 1599 String codeString = code.asStringValue(); 1600 if (codeString == null || "".equals(codeString)) 1601 return new Enumeration<DocumentMode>(this, DocumentMode.NULL, code); 1602 if ("producer".equals(codeString)) 1603 return new Enumeration<DocumentMode>(this, DocumentMode.PRODUCER, code); 1604 if ("consumer".equals(codeString)) 1605 return new Enumeration<DocumentMode>(this, DocumentMode.CONSUMER, code); 1606 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1607 } 1608 1609 public String toCode(DocumentMode code) { 1610 if (code == DocumentMode.PRODUCER) 1611 return "producer"; 1612 if (code == DocumentMode.CONSUMER) 1613 return "consumer"; 1614 return "?"; 1615 } 1616 1617 public String toSystem(DocumentMode code) { 1618 return code.getSystem(); 1619 } 1620 } 1621 1622 @Block() 1623 public static class CapabilityStatementSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 1624 /** 1625 * Name the software is known by. 1626 */ 1627 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1628 @Description(shortDefinition = "A name the software is known by", formalDefinition = "Name the software is known by.") 1629 protected StringType name; 1630 1631 /** 1632 * The version identifier for the software covered by this statement. 1633 */ 1634 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1635 @Description(shortDefinition = "Version covered by this statement", formalDefinition = "The version identifier for the software covered by this statement.") 1636 protected StringType version; 1637 1638 /** 1639 * Date this version of the software was released. 1640 */ 1641 @Child(name = "releaseDate", type = { 1642 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1643 @Description(shortDefinition = "Date this version was released", formalDefinition = "Date this version of the software was released.") 1644 protected DateTimeType releaseDate; 1645 1646 private static final long serialVersionUID = 1819769027L; 1647 1648 /** 1649 * Constructor 1650 */ 1651 public CapabilityStatementSoftwareComponent() { 1652 super(); 1653 } 1654 1655 /** 1656 * Constructor 1657 */ 1658 public CapabilityStatementSoftwareComponent(StringType name) { 1659 super(); 1660 this.name = name; 1661 } 1662 1663 /** 1664 * @return {@link #name} (Name the software is known by.). This is the 1665 * underlying object with id, value and extensions. The accessor 1666 * "getName" gives direct access to the value 1667 */ 1668 public StringType getNameElement() { 1669 if (this.name == null) 1670 if (Configuration.errorOnAutoCreate()) 1671 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.name"); 1672 else if (Configuration.doAutoCreate()) 1673 this.name = new StringType(); // bb 1674 return this.name; 1675 } 1676 1677 public boolean hasNameElement() { 1678 return this.name != null && !this.name.isEmpty(); 1679 } 1680 1681 public boolean hasName() { 1682 return this.name != null && !this.name.isEmpty(); 1683 } 1684 1685 /** 1686 * @param value {@link #name} (Name the software is known by.). This is the 1687 * underlying object with id, value and extensions. The accessor 1688 * "getName" gives direct access to the value 1689 */ 1690 public CapabilityStatementSoftwareComponent setNameElement(StringType value) { 1691 this.name = value; 1692 return this; 1693 } 1694 1695 /** 1696 * @return Name the software is known by. 1697 */ 1698 public String getName() { 1699 return this.name == null ? null : this.name.getValue(); 1700 } 1701 1702 /** 1703 * @param value Name the software is known by. 1704 */ 1705 public CapabilityStatementSoftwareComponent setName(String value) { 1706 if (this.name == null) 1707 this.name = new StringType(); 1708 this.name.setValue(value); 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #version} (The version identifier for the software covered by 1714 * this statement.). This is the underlying object with id, value and 1715 * extensions. The accessor "getVersion" gives direct access to the 1716 * value 1717 */ 1718 public StringType getVersionElement() { 1719 if (this.version == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.version"); 1722 else if (Configuration.doAutoCreate()) 1723 this.version = new StringType(); // bb 1724 return this.version; 1725 } 1726 1727 public boolean hasVersionElement() { 1728 return this.version != null && !this.version.isEmpty(); 1729 } 1730 1731 public boolean hasVersion() { 1732 return this.version != null && !this.version.isEmpty(); 1733 } 1734 1735 /** 1736 * @param value {@link #version} (The version identifier for the software 1737 * covered by this statement.). This is the underlying object with 1738 * id, value and extensions. The accessor "getVersion" gives direct 1739 * access to the value 1740 */ 1741 public CapabilityStatementSoftwareComponent setVersionElement(StringType value) { 1742 this.version = value; 1743 return this; 1744 } 1745 1746 /** 1747 * @return The version identifier for the software covered by this statement. 1748 */ 1749 public String getVersion() { 1750 return this.version == null ? null : this.version.getValue(); 1751 } 1752 1753 /** 1754 * @param value The version identifier for the software covered by this 1755 * statement. 1756 */ 1757 public CapabilityStatementSoftwareComponent setVersion(String value) { 1758 if (Utilities.noString(value)) 1759 this.version = null; 1760 else { 1761 if (this.version == null) 1762 this.version = new StringType(); 1763 this.version.setValue(value); 1764 } 1765 return this; 1766 } 1767 1768 /** 1769 * @return {@link #releaseDate} (Date this version of the software was 1770 * released.). This is the underlying object with id, value and 1771 * extensions. The accessor "getReleaseDate" gives direct access to the 1772 * value 1773 */ 1774 public DateTimeType getReleaseDateElement() { 1775 if (this.releaseDate == null) 1776 if (Configuration.errorOnAutoCreate()) 1777 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.releaseDate"); 1778 else if (Configuration.doAutoCreate()) 1779 this.releaseDate = new DateTimeType(); // bb 1780 return this.releaseDate; 1781 } 1782 1783 public boolean hasReleaseDateElement() { 1784 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1785 } 1786 1787 public boolean hasReleaseDate() { 1788 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1789 } 1790 1791 /** 1792 * @param value {@link #releaseDate} (Date this version of the software was 1793 * released.). This is the underlying object with id, value and 1794 * extensions. The accessor "getReleaseDate" gives direct access to 1795 * the value 1796 */ 1797 public CapabilityStatementSoftwareComponent setReleaseDateElement(DateTimeType value) { 1798 this.releaseDate = value; 1799 return this; 1800 } 1801 1802 /** 1803 * @return Date this version of the software was released. 1804 */ 1805 public Date getReleaseDate() { 1806 return this.releaseDate == null ? null : this.releaseDate.getValue(); 1807 } 1808 1809 /** 1810 * @param value Date this version of the software was released. 1811 */ 1812 public CapabilityStatementSoftwareComponent setReleaseDate(Date value) { 1813 if (value == null) 1814 this.releaseDate = null; 1815 else { 1816 if (this.releaseDate == null) 1817 this.releaseDate = new DateTimeType(); 1818 this.releaseDate.setValue(value); 1819 } 1820 return this; 1821 } 1822 1823 protected void listChildren(List<Property> children) { 1824 super.listChildren(children); 1825 children.add(new Property("name", "string", "Name the software is known by.", 0, 1, name)); 1826 children.add(new Property("version", "string", 1827 "The version identifier for the software covered by this statement.", 0, 1, version)); 1828 children.add(new Property("releaseDate", "dateTime", "Date this version of the software was released.", 0, 1, 1829 releaseDate)); 1830 } 1831 1832 @Override 1833 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1834 switch (_hash) { 1835 case 3373707: 1836 /* name */ return new Property("name", "string", "Name the software is known by.", 0, 1, name); 1837 case 351608024: 1838 /* version */ return new Property("version", "string", 1839 "The version identifier for the software covered by this statement.", 0, 1, version); 1840 case 212873301: 1841 /* releaseDate */ return new Property("releaseDate", "dateTime", 1842 "Date this version of the software was released.", 0, 1, releaseDate); 1843 default: 1844 return super.getNamedProperty(_hash, _name, _checkValid); 1845 } 1846 1847 } 1848 1849 @Override 1850 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1851 switch (hash) { 1852 case 3373707: 1853 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1854 case 351608024: 1855 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1856 case 212873301: 1857 /* releaseDate */ return this.releaseDate == null ? new Base[0] : new Base[] { this.releaseDate }; // DateTimeType 1858 default: 1859 return super.getProperty(hash, name, checkValid); 1860 } 1861 1862 } 1863 1864 @Override 1865 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1866 switch (hash) { 1867 case 3373707: // name 1868 this.name = castToString(value); // StringType 1869 return value; 1870 case 351608024: // version 1871 this.version = castToString(value); // StringType 1872 return value; 1873 case 212873301: // releaseDate 1874 this.releaseDate = castToDateTime(value); // DateTimeType 1875 return value; 1876 default: 1877 return super.setProperty(hash, name, value); 1878 } 1879 1880 } 1881 1882 @Override 1883 public Base setProperty(String name, Base value) throws FHIRException { 1884 if (name.equals("name")) { 1885 this.name = castToString(value); // StringType 1886 } else if (name.equals("version")) { 1887 this.version = castToString(value); // StringType 1888 } else if (name.equals("releaseDate")) { 1889 this.releaseDate = castToDateTime(value); // DateTimeType 1890 } else 1891 return super.setProperty(name, value); 1892 return value; 1893 } 1894 1895 @Override 1896 public void removeChild(String name, Base value) throws FHIRException { 1897 if (name.equals("name")) { 1898 this.name = null; 1899 } else if (name.equals("version")) { 1900 this.version = null; 1901 } else if (name.equals("releaseDate")) { 1902 this.releaseDate = null; 1903 } else 1904 super.removeChild(name, value); 1905 1906 } 1907 1908 @Override 1909 public Base makeProperty(int hash, String name) throws FHIRException { 1910 switch (hash) { 1911 case 3373707: 1912 return getNameElement(); 1913 case 351608024: 1914 return getVersionElement(); 1915 case 212873301: 1916 return getReleaseDateElement(); 1917 default: 1918 return super.makeProperty(hash, name); 1919 } 1920 1921 } 1922 1923 @Override 1924 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1925 switch (hash) { 1926 case 3373707: 1927 /* name */ return new String[] { "string" }; 1928 case 351608024: 1929 /* version */ return new String[] { "string" }; 1930 case 212873301: 1931 /* releaseDate */ return new String[] { "dateTime" }; 1932 default: 1933 return super.getTypesForProperty(hash, name); 1934 } 1935 1936 } 1937 1938 @Override 1939 public Base addChild(String name) throws FHIRException { 1940 if (name.equals("name")) { 1941 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 1942 } else if (name.equals("version")) { 1943 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 1944 } else if (name.equals("releaseDate")) { 1945 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.releaseDate"); 1946 } else 1947 return super.addChild(name); 1948 } 1949 1950 public CapabilityStatementSoftwareComponent copy() { 1951 CapabilityStatementSoftwareComponent dst = new CapabilityStatementSoftwareComponent(); 1952 copyValues(dst); 1953 return dst; 1954 } 1955 1956 public void copyValues(CapabilityStatementSoftwareComponent dst) { 1957 super.copyValues(dst); 1958 dst.name = name == null ? null : name.copy(); 1959 dst.version = version == null ? null : version.copy(); 1960 dst.releaseDate = releaseDate == null ? null : releaseDate.copy(); 1961 } 1962 1963 @Override 1964 public boolean equalsDeep(Base other_) { 1965 if (!super.equalsDeep(other_)) 1966 return false; 1967 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1968 return false; 1969 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1970 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true) 1971 && compareDeep(releaseDate, o.releaseDate, true); 1972 } 1973 1974 @Override 1975 public boolean equalsShallow(Base other_) { 1976 if (!super.equalsShallow(other_)) 1977 return false; 1978 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1979 return false; 1980 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1981 return compareValues(name, o.name, true) && compareValues(version, o.version, true) 1982 && compareValues(releaseDate, o.releaseDate, true); 1983 } 1984 1985 public boolean isEmpty() { 1986 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, version, releaseDate); 1987 } 1988 1989 public String fhirType() { 1990 return "CapabilityStatement.software"; 1991 1992 } 1993 1994 } 1995 1996 @Block() 1997 public static class CapabilityStatementImplementationComponent extends BackboneElement 1998 implements IBaseBackboneElement { 1999 /** 2000 * Information about the specific installation that this capability statement 2001 * relates to. 2002 */ 2003 @Child(name = "description", type = { 2004 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2005 @Description(shortDefinition = "Describes this specific instance", formalDefinition = "Information about the specific installation that this capability statement relates to.") 2006 protected StringType description; 2007 2008 /** 2009 * An absolute base URL for the implementation. This forms the base for REST 2010 * interfaces as well as the mailbox and document interfaces. 2011 */ 2012 @Child(name = "url", type = { UrlType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2013 @Description(shortDefinition = "Base URL for the installation", formalDefinition = "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.") 2014 protected UrlType url; 2015 2016 /** 2017 * The organization responsible for the management of the instance and oversight 2018 * of the data on the server at the specified URL. 2019 */ 2020 @Child(name = "custodian", type = { 2021 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2022 @Description(shortDefinition = "Organization that manages the data", formalDefinition = "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.") 2023 protected Reference custodian; 2024 2025 /** 2026 * The actual object that is the target of the reference (The organization 2027 * responsible for the management of the instance and oversight of the data on 2028 * the server at the specified URL.) 2029 */ 2030 protected Organization custodianTarget; 2031 2032 private static final long serialVersionUID = -1705695694L; 2033 2034 /** 2035 * Constructor 2036 */ 2037 public CapabilityStatementImplementationComponent() { 2038 super(); 2039 } 2040 2041 /** 2042 * Constructor 2043 */ 2044 public CapabilityStatementImplementationComponent(StringType description) { 2045 super(); 2046 this.description = description; 2047 } 2048 2049 /** 2050 * @return {@link #description} (Information about the specific installation 2051 * that this capability statement relates to.). This is the underlying 2052 * object with id, value and extensions. The accessor "getDescription" 2053 * gives direct access to the value 2054 */ 2055 public StringType getDescriptionElement() { 2056 if (this.description == null) 2057 if (Configuration.errorOnAutoCreate()) 2058 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.description"); 2059 else if (Configuration.doAutoCreate()) 2060 this.description = new StringType(); // bb 2061 return this.description; 2062 } 2063 2064 public boolean hasDescriptionElement() { 2065 return this.description != null && !this.description.isEmpty(); 2066 } 2067 2068 public boolean hasDescription() { 2069 return this.description != null && !this.description.isEmpty(); 2070 } 2071 2072 /** 2073 * @param value {@link #description} (Information about the specific 2074 * installation that this capability statement relates to.). This 2075 * is the underlying object with id, value and extensions. The 2076 * accessor "getDescription" gives direct access to the value 2077 */ 2078 public CapabilityStatementImplementationComponent setDescriptionElement(StringType value) { 2079 this.description = value; 2080 return this; 2081 } 2082 2083 /** 2084 * @return Information about the specific installation that this capability 2085 * statement relates to. 2086 */ 2087 public String getDescription() { 2088 return this.description == null ? null : this.description.getValue(); 2089 } 2090 2091 /** 2092 * @param value Information about the specific installation that this capability 2093 * statement relates to. 2094 */ 2095 public CapabilityStatementImplementationComponent setDescription(String value) { 2096 if (this.description == null) 2097 this.description = new StringType(); 2098 this.description.setValue(value); 2099 return this; 2100 } 2101 2102 /** 2103 * @return {@link #url} (An absolute base URL for the implementation. This forms 2104 * the base for REST interfaces as well as the mailbox and document 2105 * interfaces.). This is the underlying object with id, value and 2106 * extensions. The accessor "getUrl" gives direct access to the value 2107 */ 2108 public UrlType getUrlElement() { 2109 if (this.url == null) 2110 if (Configuration.errorOnAutoCreate()) 2111 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.url"); 2112 else if (Configuration.doAutoCreate()) 2113 this.url = new UrlType(); // bb 2114 return this.url; 2115 } 2116 2117 public boolean hasUrlElement() { 2118 return this.url != null && !this.url.isEmpty(); 2119 } 2120 2121 public boolean hasUrl() { 2122 return this.url != null && !this.url.isEmpty(); 2123 } 2124 2125 /** 2126 * @param value {@link #url} (An absolute base URL for the implementation. This 2127 * forms the base for REST interfaces as well as the mailbox and 2128 * document interfaces.). This is the underlying object with id, 2129 * value and extensions. The accessor "getUrl" gives direct access 2130 * to the value 2131 */ 2132 public CapabilityStatementImplementationComponent setUrlElement(UrlType value) { 2133 this.url = value; 2134 return this; 2135 } 2136 2137 /** 2138 * @return An absolute base URL for the implementation. This forms the base for 2139 * REST interfaces as well as the mailbox and document interfaces. 2140 */ 2141 public String getUrl() { 2142 return this.url == null ? null : this.url.getValue(); 2143 } 2144 2145 /** 2146 * @param value An absolute base URL for the implementation. This forms the base 2147 * for REST interfaces as well as the mailbox and document 2148 * interfaces. 2149 */ 2150 public CapabilityStatementImplementationComponent setUrl(String value) { 2151 if (Utilities.noString(value)) 2152 this.url = null; 2153 else { 2154 if (this.url == null) 2155 this.url = new UrlType(); 2156 this.url.setValue(value); 2157 } 2158 return this; 2159 } 2160 2161 /** 2162 * @return {@link #custodian} (The organization responsible for the management 2163 * of the instance and oversight of the data on the server at the 2164 * specified URL.) 2165 */ 2166 public Reference getCustodian() { 2167 if (this.custodian == null) 2168 if (Configuration.errorOnAutoCreate()) 2169 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.custodian"); 2170 else if (Configuration.doAutoCreate()) 2171 this.custodian = new Reference(); // cc 2172 return this.custodian; 2173 } 2174 2175 public boolean hasCustodian() { 2176 return this.custodian != null && !this.custodian.isEmpty(); 2177 } 2178 2179 /** 2180 * @param value {@link #custodian} (The organization responsible for the 2181 * management of the instance and oversight of the data on the 2182 * server at the specified URL.) 2183 */ 2184 public CapabilityStatementImplementationComponent setCustodian(Reference value) { 2185 this.custodian = value; 2186 return this; 2187 } 2188 2189 /** 2190 * @return {@link #custodian} The actual object that is the target of the 2191 * reference. The reference library doesn't populate this, but you can 2192 * use it to hold the resource if you resolve it. (The organization 2193 * responsible for the management of the instance and oversight of the 2194 * data on the server at the specified URL.) 2195 */ 2196 public Organization getCustodianTarget() { 2197 if (this.custodianTarget == null) 2198 if (Configuration.errorOnAutoCreate()) 2199 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.custodian"); 2200 else if (Configuration.doAutoCreate()) 2201 this.custodianTarget = new Organization(); // aa 2202 return this.custodianTarget; 2203 } 2204 2205 /** 2206 * @param value {@link #custodian} The actual object that is the target of the 2207 * reference. The reference library doesn't use these, but you can 2208 * use it to hold the resource if you resolve it. (The organization 2209 * responsible for the management of the instance and oversight of 2210 * the data on the server at the specified URL.) 2211 */ 2212 public CapabilityStatementImplementationComponent setCustodianTarget(Organization value) { 2213 this.custodianTarget = value; 2214 return this; 2215 } 2216 2217 protected void listChildren(List<Property> children) { 2218 super.listChildren(children); 2219 children.add(new Property("description", "string", 2220 "Information about the specific installation that this capability statement relates to.", 0, 1, description)); 2221 children.add(new Property("url", "url", 2222 "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 2223 0, 1, url)); 2224 children.add(new Property("custodian", "Reference(Organization)", 2225 "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.", 2226 0, 1, custodian)); 2227 } 2228 2229 @Override 2230 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2231 switch (_hash) { 2232 case -1724546052: 2233 /* description */ return new Property("description", "string", 2234 "Information about the specific installation that this capability statement relates to.", 0, 1, 2235 description); 2236 case 116079: 2237 /* url */ return new Property("url", "url", 2238 "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 2239 0, 1, url); 2240 case 1611297262: 2241 /* custodian */ return new Property("custodian", "Reference(Organization)", 2242 "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.", 2243 0, 1, custodian); 2244 default: 2245 return super.getNamedProperty(_hash, _name, _checkValid); 2246 } 2247 2248 } 2249 2250 @Override 2251 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2252 switch (hash) { 2253 case -1724546052: 2254 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2255 case 116079: 2256 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UrlType 2257 case 1611297262: 2258 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 2259 default: 2260 return super.getProperty(hash, name, checkValid); 2261 } 2262 2263 } 2264 2265 @Override 2266 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2267 switch (hash) { 2268 case -1724546052: // description 2269 this.description = castToString(value); // StringType 2270 return value; 2271 case 116079: // url 2272 this.url = castToUrl(value); // UrlType 2273 return value; 2274 case 1611297262: // custodian 2275 this.custodian = castToReference(value); // Reference 2276 return value; 2277 default: 2278 return super.setProperty(hash, name, value); 2279 } 2280 2281 } 2282 2283 @Override 2284 public Base setProperty(String name, Base value) throws FHIRException { 2285 if (name.equals("description")) { 2286 this.description = castToString(value); // StringType 2287 } else if (name.equals("url")) { 2288 this.url = castToUrl(value); // UrlType 2289 } else if (name.equals("custodian")) { 2290 this.custodian = castToReference(value); // Reference 2291 } else 2292 return super.setProperty(name, value); 2293 return value; 2294 } 2295 2296 @Override 2297 public void removeChild(String name, Base value) throws FHIRException { 2298 if (name.equals("description")) { 2299 this.description = null; 2300 } else if (name.equals("url")) { 2301 this.url = null; 2302 } else if (name.equals("custodian")) { 2303 this.custodian = null; 2304 } else 2305 super.removeChild(name, value); 2306 2307 } 2308 2309 @Override 2310 public Base makeProperty(int hash, String name) throws FHIRException { 2311 switch (hash) { 2312 case -1724546052: 2313 return getDescriptionElement(); 2314 case 116079: 2315 return getUrlElement(); 2316 case 1611297262: 2317 return getCustodian(); 2318 default: 2319 return super.makeProperty(hash, name); 2320 } 2321 2322 } 2323 2324 @Override 2325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2326 switch (hash) { 2327 case -1724546052: 2328 /* description */ return new String[] { "string" }; 2329 case 116079: 2330 /* url */ return new String[] { "url" }; 2331 case 1611297262: 2332 /* custodian */ return new String[] { "Reference" }; 2333 default: 2334 return super.getTypesForProperty(hash, name); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base addChild(String name) throws FHIRException { 2341 if (name.equals("description")) { 2342 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 2343 } else if (name.equals("url")) { 2344 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 2345 } else if (name.equals("custodian")) { 2346 this.custodian = new Reference(); 2347 return this.custodian; 2348 } else 2349 return super.addChild(name); 2350 } 2351 2352 public CapabilityStatementImplementationComponent copy() { 2353 CapabilityStatementImplementationComponent dst = new CapabilityStatementImplementationComponent(); 2354 copyValues(dst); 2355 return dst; 2356 } 2357 2358 public void copyValues(CapabilityStatementImplementationComponent dst) { 2359 super.copyValues(dst); 2360 dst.description = description == null ? null : description.copy(); 2361 dst.url = url == null ? null : url.copy(); 2362 dst.custodian = custodian == null ? null : custodian.copy(); 2363 } 2364 2365 @Override 2366 public boolean equalsDeep(Base other_) { 2367 if (!super.equalsDeep(other_)) 2368 return false; 2369 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 2370 return false; 2371 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 2372 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true) 2373 && compareDeep(custodian, o.custodian, true); 2374 } 2375 2376 @Override 2377 public boolean equalsShallow(Base other_) { 2378 if (!super.equalsShallow(other_)) 2379 return false; 2380 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 2381 return false; 2382 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 2383 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 2384 } 2385 2386 public boolean isEmpty() { 2387 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, url, custodian); 2388 } 2389 2390 public String fhirType() { 2391 return "CapabilityStatement.implementation"; 2392 2393 } 2394 2395 } 2396 2397 @Block() 2398 public static class CapabilityStatementRestComponent extends BackboneElement implements IBaseBackboneElement { 2399 /** 2400 * Identifies whether this portion of the statement is describing the ability to 2401 * initiate or receive restful operations. 2402 */ 2403 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2404 @Description(shortDefinition = "client | server", formalDefinition = "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.") 2405 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/restful-capability-mode") 2406 protected Enumeration<RestfulCapabilityMode> mode; 2407 2408 /** 2409 * Information about the system's restful capabilities that apply across all 2410 * applications, such as security. 2411 */ 2412 @Child(name = "documentation", type = { 2413 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2414 @Description(shortDefinition = "General description of implementation", formalDefinition = "Information about the system's restful capabilities that apply across all applications, such as security.") 2415 protected MarkdownType documentation; 2416 2417 /** 2418 * Information about security implementation from an interface perspective - 2419 * what a client needs to know. 2420 */ 2421 @Child(name = "security", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = true) 2422 @Description(shortDefinition = "Information about security of implementation", formalDefinition = "Information about security implementation from an interface perspective - what a client needs to know.") 2423 protected CapabilityStatementRestSecurityComponent security; 2424 2425 /** 2426 * A specification of the restful capabilities of the solution for a specific 2427 * resource type. 2428 */ 2429 @Child(name = "resource", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2430 @Description(shortDefinition = "Resource served on the REST interface", formalDefinition = "A specification of the restful capabilities of the solution for a specific resource type.") 2431 protected List<CapabilityStatementRestResourceComponent> resource; 2432 2433 /** 2434 * A specification of restful operations supported by the system. 2435 */ 2436 @Child(name = "interaction", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2437 @Description(shortDefinition = "What operations are supported?", formalDefinition = "A specification of restful operations supported by the system.") 2438 protected List<SystemInteractionComponent> interaction; 2439 2440 /** 2441 * Search parameters that are supported for searching all resources for 2442 * implementations to support and/or make use of - either references to ones 2443 * defined in the specification, or additional ones defined for/by the 2444 * implementation. 2445 */ 2446 @Child(name = "searchParam", type = { 2447 CapabilityStatementRestResourceSearchParamComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2448 @Description(shortDefinition = "Search parameters for searching all resources", formalDefinition = "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 2449 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 2450 2451 /** 2452 * Definition of an operation or a named query together with its parameters and 2453 * their meaning and type. 2454 */ 2455 @Child(name = "operation", type = { 2456 CapabilityStatementRestResourceOperationComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2457 @Description(shortDefinition = "Definition of a system level operation", formalDefinition = "Definition of an operation or a named query together with its parameters and their meaning and type.") 2458 protected List<CapabilityStatementRestResourceOperationComponent> operation; 2459 2460 /** 2461 * An absolute URI which is a reference to the definition of a compartment that 2462 * the system supports. The reference is to a CompartmentDefinition resource by 2463 * its canonical URL . 2464 */ 2465 @Child(name = "compartment", type = { 2466 CanonicalType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2467 @Description(shortDefinition = "Compartments served/used by system", formalDefinition = "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .") 2468 protected List<CanonicalType> compartment; 2469 2470 private static final long serialVersionUID = -1442029817L; 2471 2472 /** 2473 * Constructor 2474 */ 2475 public CapabilityStatementRestComponent() { 2476 super(); 2477 } 2478 2479 /** 2480 * Constructor 2481 */ 2482 public CapabilityStatementRestComponent(Enumeration<RestfulCapabilityMode> mode) { 2483 super(); 2484 this.mode = mode; 2485 } 2486 2487 /** 2488 * @return {@link #mode} (Identifies whether this portion of the statement is 2489 * describing the ability to initiate or receive restful operations.). 2490 * This is the underlying object with id, value and extensions. The 2491 * accessor "getMode" gives direct access to the value 2492 */ 2493 public Enumeration<RestfulCapabilityMode> getModeElement() { 2494 if (this.mode == null) 2495 if (Configuration.errorOnAutoCreate()) 2496 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.mode"); 2497 else if (Configuration.doAutoCreate()) 2498 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); // bb 2499 return this.mode; 2500 } 2501 2502 public boolean hasModeElement() { 2503 return this.mode != null && !this.mode.isEmpty(); 2504 } 2505 2506 public boolean hasMode() { 2507 return this.mode != null && !this.mode.isEmpty(); 2508 } 2509 2510 /** 2511 * @param value {@link #mode} (Identifies whether this portion of the statement 2512 * is describing the ability to initiate or receive restful 2513 * operations.). This is the underlying object with id, value and 2514 * extensions. The accessor "getMode" gives direct access to the 2515 * value 2516 */ 2517 public CapabilityStatementRestComponent setModeElement(Enumeration<RestfulCapabilityMode> value) { 2518 this.mode = value; 2519 return this; 2520 } 2521 2522 /** 2523 * @return Identifies whether this portion of the statement is describing the 2524 * ability to initiate or receive restful operations. 2525 */ 2526 public RestfulCapabilityMode getMode() { 2527 return this.mode == null ? null : this.mode.getValue(); 2528 } 2529 2530 /** 2531 * @param value Identifies whether this portion of the statement is describing 2532 * the ability to initiate or receive restful operations. 2533 */ 2534 public CapabilityStatementRestComponent setMode(RestfulCapabilityMode value) { 2535 if (this.mode == null) 2536 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); 2537 this.mode.setValue(value); 2538 return this; 2539 } 2540 2541 /** 2542 * @return {@link #documentation} (Information about the system's restful 2543 * capabilities that apply across all applications, such as security.). 2544 * This is the underlying object with id, value and extensions. The 2545 * accessor "getDocumentation" gives direct access to the value 2546 */ 2547 public MarkdownType getDocumentationElement() { 2548 if (this.documentation == null) 2549 if (Configuration.errorOnAutoCreate()) 2550 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.documentation"); 2551 else if (Configuration.doAutoCreate()) 2552 this.documentation = new MarkdownType(); // bb 2553 return this.documentation; 2554 } 2555 2556 public boolean hasDocumentationElement() { 2557 return this.documentation != null && !this.documentation.isEmpty(); 2558 } 2559 2560 public boolean hasDocumentation() { 2561 return this.documentation != null && !this.documentation.isEmpty(); 2562 } 2563 2564 /** 2565 * @param value {@link #documentation} (Information about the system's restful 2566 * capabilities that apply across all applications, such as 2567 * security.). This is the underlying object with id, value and 2568 * extensions. The accessor "getDocumentation" gives direct access 2569 * to the value 2570 */ 2571 public CapabilityStatementRestComponent setDocumentationElement(MarkdownType value) { 2572 this.documentation = value; 2573 return this; 2574 } 2575 2576 /** 2577 * @return Information about the system's restful capabilities that apply across 2578 * all applications, such as security. 2579 */ 2580 public String getDocumentation() { 2581 return this.documentation == null ? null : this.documentation.getValue(); 2582 } 2583 2584 /** 2585 * @param value Information about the system's restful capabilities that apply 2586 * across all applications, such as security. 2587 */ 2588 public CapabilityStatementRestComponent setDocumentation(String value) { 2589 if (value == null) 2590 this.documentation = null; 2591 else { 2592 if (this.documentation == null) 2593 this.documentation = new MarkdownType(); 2594 this.documentation.setValue(value); 2595 } 2596 return this; 2597 } 2598 2599 /** 2600 * @return {@link #security} (Information about security implementation from an 2601 * interface perspective - what a client needs to know.) 2602 */ 2603 public CapabilityStatementRestSecurityComponent getSecurity() { 2604 if (this.security == null) 2605 if (Configuration.errorOnAutoCreate()) 2606 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.security"); 2607 else if (Configuration.doAutoCreate()) 2608 this.security = new CapabilityStatementRestSecurityComponent(); // cc 2609 return this.security; 2610 } 2611 2612 public boolean hasSecurity() { 2613 return this.security != null && !this.security.isEmpty(); 2614 } 2615 2616 /** 2617 * @param value {@link #security} (Information about security implementation 2618 * from an interface perspective - what a client needs to know.) 2619 */ 2620 public CapabilityStatementRestComponent setSecurity(CapabilityStatementRestSecurityComponent value) { 2621 this.security = value; 2622 return this; 2623 } 2624 2625 /** 2626 * @return {@link #resource} (A specification of the restful capabilities of the 2627 * solution for a specific resource type.) 2628 */ 2629 public List<CapabilityStatementRestResourceComponent> getResource() { 2630 if (this.resource == null) 2631 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2632 return this.resource; 2633 } 2634 2635 /** 2636 * @return Returns a reference to <code>this</code> for easy method chaining 2637 */ 2638 public CapabilityStatementRestComponent setResource(List<CapabilityStatementRestResourceComponent> theResource) { 2639 this.resource = theResource; 2640 return this; 2641 } 2642 2643 public boolean hasResource() { 2644 if (this.resource == null) 2645 return false; 2646 for (CapabilityStatementRestResourceComponent item : this.resource) 2647 if (!item.isEmpty()) 2648 return true; 2649 return false; 2650 } 2651 2652 public CapabilityStatementRestResourceComponent addResource() { // 3 2653 CapabilityStatementRestResourceComponent t = new CapabilityStatementRestResourceComponent(); 2654 if (this.resource == null) 2655 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2656 this.resource.add(t); 2657 return t; 2658 } 2659 2660 public CapabilityStatementRestComponent addResource(CapabilityStatementRestResourceComponent t) { // 3 2661 if (t == null) 2662 return this; 2663 if (this.resource == null) 2664 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2665 this.resource.add(t); 2666 return this; 2667 } 2668 2669 /** 2670 * @return The first repetition of repeating field {@link #resource}, creating 2671 * it if it does not already exist 2672 */ 2673 public CapabilityStatementRestResourceComponent getResourceFirstRep() { 2674 if (getResource().isEmpty()) { 2675 addResource(); 2676 } 2677 return getResource().get(0); 2678 } 2679 2680 /** 2681 * @return {@link #interaction} (A specification of restful operations supported 2682 * by the system.) 2683 */ 2684 public List<SystemInteractionComponent> getInteraction() { 2685 if (this.interaction == null) 2686 this.interaction = new ArrayList<SystemInteractionComponent>(); 2687 return this.interaction; 2688 } 2689 2690 /** 2691 * @return Returns a reference to <code>this</code> for easy method chaining 2692 */ 2693 public CapabilityStatementRestComponent setInteraction(List<SystemInteractionComponent> theInteraction) { 2694 this.interaction = theInteraction; 2695 return this; 2696 } 2697 2698 public boolean hasInteraction() { 2699 if (this.interaction == null) 2700 return false; 2701 for (SystemInteractionComponent item : this.interaction) 2702 if (!item.isEmpty()) 2703 return true; 2704 return false; 2705 } 2706 2707 public SystemInteractionComponent addInteraction() { // 3 2708 SystemInteractionComponent t = new SystemInteractionComponent(); 2709 if (this.interaction == null) 2710 this.interaction = new ArrayList<SystemInteractionComponent>(); 2711 this.interaction.add(t); 2712 return t; 2713 } 2714 2715 public CapabilityStatementRestComponent addInteraction(SystemInteractionComponent t) { // 3 2716 if (t == null) 2717 return this; 2718 if (this.interaction == null) 2719 this.interaction = new ArrayList<SystemInteractionComponent>(); 2720 this.interaction.add(t); 2721 return this; 2722 } 2723 2724 /** 2725 * @return The first repetition of repeating field {@link #interaction}, 2726 * creating it if it does not already exist 2727 */ 2728 public SystemInteractionComponent getInteractionFirstRep() { 2729 if (getInteraction().isEmpty()) { 2730 addInteraction(); 2731 } 2732 return getInteraction().get(0); 2733 } 2734 2735 /** 2736 * @return {@link #searchParam} (Search parameters that are supported for 2737 * searching all resources for implementations to support and/or make 2738 * use of - either references to ones defined in the specification, or 2739 * additional ones defined for/by the implementation.) 2740 */ 2741 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 2742 if (this.searchParam == null) 2743 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2744 return this.searchParam; 2745 } 2746 2747 /** 2748 * @return Returns a reference to <code>this</code> for easy method chaining 2749 */ 2750 public CapabilityStatementRestComponent setSearchParam( 2751 List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 2752 this.searchParam = theSearchParam; 2753 return this; 2754 } 2755 2756 public boolean hasSearchParam() { 2757 if (this.searchParam == null) 2758 return false; 2759 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 2760 if (!item.isEmpty()) 2761 return true; 2762 return false; 2763 } 2764 2765 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { // 3 2766 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 2767 if (this.searchParam == null) 2768 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2769 this.searchParam.add(t); 2770 return t; 2771 } 2772 2773 public CapabilityStatementRestComponent addSearchParam(CapabilityStatementRestResourceSearchParamComponent t) { // 3 2774 if (t == null) 2775 return this; 2776 if (this.searchParam == null) 2777 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2778 this.searchParam.add(t); 2779 return this; 2780 } 2781 2782 /** 2783 * @return The first repetition of repeating field {@link #searchParam}, 2784 * creating it if it does not already exist 2785 */ 2786 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 2787 if (getSearchParam().isEmpty()) { 2788 addSearchParam(); 2789 } 2790 return getSearchParam().get(0); 2791 } 2792 2793 /** 2794 * @return {@link #operation} (Definition of an operation or a named query 2795 * together with its parameters and their meaning and type.) 2796 */ 2797 public List<CapabilityStatementRestResourceOperationComponent> getOperation() { 2798 if (this.operation == null) 2799 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2800 return this.operation; 2801 } 2802 2803 /** 2804 * @return Returns a reference to <code>this</code> for easy method chaining 2805 */ 2806 public CapabilityStatementRestComponent setOperation( 2807 List<CapabilityStatementRestResourceOperationComponent> theOperation) { 2808 this.operation = theOperation; 2809 return this; 2810 } 2811 2812 public boolean hasOperation() { 2813 if (this.operation == null) 2814 return false; 2815 for (CapabilityStatementRestResourceOperationComponent item : this.operation) 2816 if (!item.isEmpty()) 2817 return true; 2818 return false; 2819 } 2820 2821 public CapabilityStatementRestResourceOperationComponent addOperation() { // 3 2822 CapabilityStatementRestResourceOperationComponent t = new CapabilityStatementRestResourceOperationComponent(); 2823 if (this.operation == null) 2824 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2825 this.operation.add(t); 2826 return t; 2827 } 2828 2829 public CapabilityStatementRestComponent addOperation(CapabilityStatementRestResourceOperationComponent t) { // 3 2830 if (t == null) 2831 return this; 2832 if (this.operation == null) 2833 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2834 this.operation.add(t); 2835 return this; 2836 } 2837 2838 /** 2839 * @return The first repetition of repeating field {@link #operation}, creating 2840 * it if it does not already exist 2841 */ 2842 public CapabilityStatementRestResourceOperationComponent getOperationFirstRep() { 2843 if (getOperation().isEmpty()) { 2844 addOperation(); 2845 } 2846 return getOperation().get(0); 2847 } 2848 2849 /** 2850 * @return {@link #compartment} (An absolute URI which is a reference to the 2851 * definition of a compartment that the system supports. The reference 2852 * is to a CompartmentDefinition resource by its canonical URL .) 2853 */ 2854 public List<CanonicalType> getCompartment() { 2855 if (this.compartment == null) 2856 this.compartment = new ArrayList<CanonicalType>(); 2857 return this.compartment; 2858 } 2859 2860 /** 2861 * @return Returns a reference to <code>this</code> for easy method chaining 2862 */ 2863 public CapabilityStatementRestComponent setCompartment(List<CanonicalType> theCompartment) { 2864 this.compartment = theCompartment; 2865 return this; 2866 } 2867 2868 public boolean hasCompartment() { 2869 if (this.compartment == null) 2870 return false; 2871 for (CanonicalType item : this.compartment) 2872 if (!item.isEmpty()) 2873 return true; 2874 return false; 2875 } 2876 2877 /** 2878 * @return {@link #compartment} (An absolute URI which is a reference to the 2879 * definition of a compartment that the system supports. The reference 2880 * is to a CompartmentDefinition resource by its canonical URL .) 2881 */ 2882 public CanonicalType addCompartmentElement() {// 2 2883 CanonicalType t = new CanonicalType(); 2884 if (this.compartment == null) 2885 this.compartment = new ArrayList<CanonicalType>(); 2886 this.compartment.add(t); 2887 return t; 2888 } 2889 2890 /** 2891 * @param value {@link #compartment} (An absolute URI which is a reference to 2892 * the definition of a compartment that the system supports. The 2893 * reference is to a CompartmentDefinition resource by its 2894 * canonical URL .) 2895 */ 2896 public CapabilityStatementRestComponent addCompartment(String value) { // 1 2897 CanonicalType t = new CanonicalType(); 2898 t.setValue(value); 2899 if (this.compartment == null) 2900 this.compartment = new ArrayList<CanonicalType>(); 2901 this.compartment.add(t); 2902 return this; 2903 } 2904 2905 /** 2906 * @param value {@link #compartment} (An absolute URI which is a reference to 2907 * the definition of a compartment that the system supports. The 2908 * reference is to a CompartmentDefinition resource by its 2909 * canonical URL .) 2910 */ 2911 public boolean hasCompartment(String value) { 2912 if (this.compartment == null) 2913 return false; 2914 for (CanonicalType v : this.compartment) 2915 if (v.getValue().equals(value)) // canonical(CompartmentDefinition) 2916 return true; 2917 return false; 2918 } 2919 2920 protected void listChildren(List<Property> children) { 2921 super.listChildren(children); 2922 children.add(new Property("mode", "code", 2923 "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 2924 0, 1, mode)); 2925 children.add(new Property("documentation", "markdown", 2926 "Information about the system's restful capabilities that apply across all applications, such as security.", 2927 0, 1, documentation)); 2928 children.add(new Property("security", "", 2929 "Information about security implementation from an interface perspective - what a client needs to know.", 0, 2930 1, security)); 2931 children.add(new Property("resource", "", 2932 "A specification of the restful capabilities of the solution for a specific resource type.", 0, 2933 java.lang.Integer.MAX_VALUE, resource)); 2934 children.add(new Property("interaction", "", "A specification of restful operations supported by the system.", 0, 2935 java.lang.Integer.MAX_VALUE, interaction)); 2936 children.add(new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", 2937 "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 2938 0, java.lang.Integer.MAX_VALUE, searchParam)); 2939 children.add(new Property("operation", "@CapabilityStatement.rest.resource.operation", 2940 "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, 2941 java.lang.Integer.MAX_VALUE, operation)); 2942 children.add(new Property("compartment", "canonical(CompartmentDefinition)", 2943 "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 2944 0, java.lang.Integer.MAX_VALUE, compartment)); 2945 } 2946 2947 @Override 2948 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2949 switch (_hash) { 2950 case 3357091: 2951 /* mode */ return new Property("mode", "code", 2952 "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 2953 0, 1, mode); 2954 case 1587405498: 2955 /* documentation */ return new Property("documentation", "markdown", 2956 "Information about the system's restful capabilities that apply across all applications, such as security.", 2957 0, 1, documentation); 2958 case 949122880: 2959 /* security */ return new Property("security", "", 2960 "Information about security implementation from an interface perspective - what a client needs to know.", 0, 2961 1, security); 2962 case -341064690: 2963 /* resource */ return new Property("resource", "", 2964 "A specification of the restful capabilities of the solution for a specific resource type.", 0, 2965 java.lang.Integer.MAX_VALUE, resource); 2966 case 1844104722: 2967 /* interaction */ return new Property("interaction", "", 2968 "A specification of restful operations supported by the system.", 0, java.lang.Integer.MAX_VALUE, 2969 interaction); 2970 case -553645115: 2971 /* searchParam */ return new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", 2972 "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 2973 0, java.lang.Integer.MAX_VALUE, searchParam); 2974 case 1662702951: 2975 /* operation */ return new Property("operation", "@CapabilityStatement.rest.resource.operation", 2976 "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, 2977 java.lang.Integer.MAX_VALUE, operation); 2978 case -397756334: 2979 /* compartment */ return new Property("compartment", "canonical(CompartmentDefinition)", 2980 "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 2981 0, java.lang.Integer.MAX_VALUE, compartment); 2982 default: 2983 return super.getNamedProperty(_hash, _name, _checkValid); 2984 } 2985 2986 } 2987 2988 @Override 2989 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2990 switch (hash) { 2991 case 3357091: 2992 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<RestfulCapabilityMode> 2993 case 1587405498: 2994 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 2995 case 949122880: 2996 /* security */ return this.security == null ? new Base[0] : new Base[] { this.security }; // CapabilityStatementRestSecurityComponent 2997 case -341064690: 2998 /* resource */ return this.resource == null ? new Base[0] 2999 : this.resource.toArray(new Base[this.resource.size()]); // CapabilityStatementRestResourceComponent 3000 case 1844104722: 3001 /* interaction */ return this.interaction == null ? new Base[0] 3002 : this.interaction.toArray(new Base[this.interaction.size()]); // SystemInteractionComponent 3003 case -553645115: 3004 /* searchParam */ return this.searchParam == null ? new Base[0] 3005 : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 3006 case 1662702951: 3007 /* operation */ return this.operation == null ? new Base[0] 3008 : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestResourceOperationComponent 3009 case -397756334: 3010 /* compartment */ return this.compartment == null ? new Base[0] 3011 : this.compartment.toArray(new Base[this.compartment.size()]); // CanonicalType 3012 default: 3013 return super.getProperty(hash, name, checkValid); 3014 } 3015 3016 } 3017 3018 @Override 3019 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3020 switch (hash) { 3021 case 3357091: // mode 3022 value = new RestfulCapabilityModeEnumFactory().fromType(castToCode(value)); 3023 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 3024 return value; 3025 case 1587405498: // documentation 3026 this.documentation = castToMarkdown(value); // MarkdownType 3027 return value; 3028 case 949122880: // security 3029 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 3030 return value; 3031 case -341064690: // resource 3032 this.getResource().add((CapabilityStatementRestResourceComponent) value); // CapabilityStatementRestResourceComponent 3033 return value; 3034 case 1844104722: // interaction 3035 this.getInteraction().add((SystemInteractionComponent) value); // SystemInteractionComponent 3036 return value; 3037 case -553645115: // searchParam 3038 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 3039 return value; 3040 case 1662702951: // operation 3041 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); // CapabilityStatementRestResourceOperationComponent 3042 return value; 3043 case -397756334: // compartment 3044 this.getCompartment().add(castToCanonical(value)); // CanonicalType 3045 return value; 3046 default: 3047 return super.setProperty(hash, name, value); 3048 } 3049 3050 } 3051 3052 @Override 3053 public Base setProperty(String name, Base value) throws FHIRException { 3054 if (name.equals("mode")) { 3055 value = new RestfulCapabilityModeEnumFactory().fromType(castToCode(value)); 3056 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 3057 } else if (name.equals("documentation")) { 3058 this.documentation = castToMarkdown(value); // MarkdownType 3059 } else if (name.equals("security")) { 3060 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 3061 } else if (name.equals("resource")) { 3062 this.getResource().add((CapabilityStatementRestResourceComponent) value); 3063 } else if (name.equals("interaction")) { 3064 this.getInteraction().add((SystemInteractionComponent) value); 3065 } else if (name.equals("searchParam")) { 3066 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 3067 } else if (name.equals("operation")) { 3068 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); 3069 } else if (name.equals("compartment")) { 3070 this.getCompartment().add(castToCanonical(value)); 3071 } else 3072 return super.setProperty(name, value); 3073 return value; 3074 } 3075 3076 @Override 3077 public void removeChild(String name, Base value) throws FHIRException { 3078 if (name.equals("mode")) { 3079 this.mode = null; 3080 } else if (name.equals("documentation")) { 3081 this.documentation = null; 3082 } else if (name.equals("security")) { 3083 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 3084 } else if (name.equals("resource")) { 3085 this.getResource().remove((CapabilityStatementRestResourceComponent) value); 3086 } else if (name.equals("interaction")) { 3087 this.getInteraction().remove((SystemInteractionComponent) value); 3088 } else if (name.equals("searchParam")) { 3089 this.getSearchParam().remove((CapabilityStatementRestResourceSearchParamComponent) value); 3090 } else if (name.equals("operation")) { 3091 this.getOperation().remove((CapabilityStatementRestResourceOperationComponent) value); 3092 } else if (name.equals("compartment")) { 3093 this.getCompartment().remove(castToCanonical(value)); 3094 } else 3095 super.removeChild(name, value); 3096 3097 } 3098 3099 @Override 3100 public Base makeProperty(int hash, String name) throws FHIRException { 3101 switch (hash) { 3102 case 3357091: 3103 return getModeElement(); 3104 case 1587405498: 3105 return getDocumentationElement(); 3106 case 949122880: 3107 return getSecurity(); 3108 case -341064690: 3109 return addResource(); 3110 case 1844104722: 3111 return addInteraction(); 3112 case -553645115: 3113 return addSearchParam(); 3114 case 1662702951: 3115 return addOperation(); 3116 case -397756334: 3117 return addCompartmentElement(); 3118 default: 3119 return super.makeProperty(hash, name); 3120 } 3121 3122 } 3123 3124 @Override 3125 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3126 switch (hash) { 3127 case 3357091: 3128 /* mode */ return new String[] { "code" }; 3129 case 1587405498: 3130 /* documentation */ return new String[] { "markdown" }; 3131 case 949122880: 3132 /* security */ return new String[] {}; 3133 case -341064690: 3134 /* resource */ return new String[] {}; 3135 case 1844104722: 3136 /* interaction */ return new String[] {}; 3137 case -553645115: 3138 /* searchParam */ return new String[] { "@CapabilityStatement.rest.resource.searchParam" }; 3139 case 1662702951: 3140 /* operation */ return new String[] { "@CapabilityStatement.rest.resource.operation" }; 3141 case -397756334: 3142 /* compartment */ return new String[] { "canonical" }; 3143 default: 3144 return super.getTypesForProperty(hash, name); 3145 } 3146 3147 } 3148 3149 @Override 3150 public Base addChild(String name) throws FHIRException { 3151 if (name.equals("mode")) { 3152 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 3153 } else if (name.equals("documentation")) { 3154 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 3155 } else if (name.equals("security")) { 3156 this.security = new CapabilityStatementRestSecurityComponent(); 3157 return this.security; 3158 } else if (name.equals("resource")) { 3159 return addResource(); 3160 } else if (name.equals("interaction")) { 3161 return addInteraction(); 3162 } else if (name.equals("searchParam")) { 3163 return addSearchParam(); 3164 } else if (name.equals("operation")) { 3165 return addOperation(); 3166 } else if (name.equals("compartment")) { 3167 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.compartment"); 3168 } else 3169 return super.addChild(name); 3170 } 3171 3172 public CapabilityStatementRestComponent copy() { 3173 CapabilityStatementRestComponent dst = new CapabilityStatementRestComponent(); 3174 copyValues(dst); 3175 return dst; 3176 } 3177 3178 public void copyValues(CapabilityStatementRestComponent dst) { 3179 super.copyValues(dst); 3180 dst.mode = mode == null ? null : mode.copy(); 3181 dst.documentation = documentation == null ? null : documentation.copy(); 3182 dst.security = security == null ? null : security.copy(); 3183 if (resource != null) { 3184 dst.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 3185 for (CapabilityStatementRestResourceComponent i : resource) 3186 dst.resource.add(i.copy()); 3187 } 3188 ; 3189 if (interaction != null) { 3190 dst.interaction = new ArrayList<SystemInteractionComponent>(); 3191 for (SystemInteractionComponent i : interaction) 3192 dst.interaction.add(i.copy()); 3193 } 3194 ; 3195 if (searchParam != null) { 3196 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 3197 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 3198 dst.searchParam.add(i.copy()); 3199 } 3200 ; 3201 if (operation != null) { 3202 dst.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 3203 for (CapabilityStatementRestResourceOperationComponent i : operation) 3204 dst.operation.add(i.copy()); 3205 } 3206 ; 3207 if (compartment != null) { 3208 dst.compartment = new ArrayList<CanonicalType>(); 3209 for (CanonicalType i : compartment) 3210 dst.compartment.add(i.copy()); 3211 } 3212 ; 3213 } 3214 3215 @Override 3216 public boolean equalsDeep(Base other_) { 3217 if (!super.equalsDeep(other_)) 3218 return false; 3219 if (!(other_ instanceof CapabilityStatementRestComponent)) 3220 return false; 3221 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 3222 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 3223 && compareDeep(security, o.security, true) && compareDeep(resource, o.resource, true) 3224 && compareDeep(interaction, o.interaction, true) && compareDeep(searchParam, o.searchParam, true) 3225 && compareDeep(operation, o.operation, true) && compareDeep(compartment, o.compartment, true); 3226 } 3227 3228 @Override 3229 public boolean equalsShallow(Base other_) { 3230 if (!super.equalsShallow(other_)) 3231 return false; 3232 if (!(other_ instanceof CapabilityStatementRestComponent)) 3233 return false; 3234 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 3235 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 3236 } 3237 3238 public boolean isEmpty() { 3239 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, security, resource, 3240 interaction, searchParam, operation, compartment); 3241 } 3242 3243 public String fhirType() { 3244 return "CapabilityStatement.rest"; 3245 3246 } 3247 3248 } 3249 3250 @Block() 3251 public static class CapabilityStatementRestSecurityComponent extends BackboneElement implements IBaseBackboneElement { 3252 /** 3253 * Server adds CORS headers when responding to requests - this enables 3254 * Javascript applications to use the server. 3255 */ 3256 @Child(name = "cors", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3257 @Description(shortDefinition = "Adds CORS Headers (http://enable-cors.org/)", formalDefinition = "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.") 3258 protected BooleanType cors; 3259 3260 /** 3261 * Types of security services that are supported/required by the system. 3262 */ 3263 @Child(name = "service", type = { 3264 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3265 @Description(shortDefinition = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", formalDefinition = "Types of security services that are supported/required by the system.") 3266 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/restful-security-service") 3267 protected List<CodeableConcept> service; 3268 3269 /** 3270 * General description of how security works. 3271 */ 3272 @Child(name = "description", type = { 3273 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3274 @Description(shortDefinition = "General description of how security works", formalDefinition = "General description of how security works.") 3275 protected MarkdownType description; 3276 3277 private static final long serialVersionUID = -1348900500L; 3278 3279 /** 3280 * Constructor 3281 */ 3282 public CapabilityStatementRestSecurityComponent() { 3283 super(); 3284 } 3285 3286 /** 3287 * @return {@link #cors} (Server adds CORS headers when responding to requests - 3288 * this enables Javascript applications to use the server.). This is the 3289 * underlying object with id, value and extensions. The accessor 3290 * "getCors" gives direct access to the value 3291 */ 3292 public BooleanType getCorsElement() { 3293 if (this.cors == null) 3294 if (Configuration.errorOnAutoCreate()) 3295 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.cors"); 3296 else if (Configuration.doAutoCreate()) 3297 this.cors = new BooleanType(); // bb 3298 return this.cors; 3299 } 3300 3301 public boolean hasCorsElement() { 3302 return this.cors != null && !this.cors.isEmpty(); 3303 } 3304 3305 public boolean hasCors() { 3306 return this.cors != null && !this.cors.isEmpty(); 3307 } 3308 3309 /** 3310 * @param value {@link #cors} (Server adds CORS headers when responding to 3311 * requests - this enables Javascript applications to use the 3312 * server.). This is the underlying object with id, value and 3313 * extensions. The accessor "getCors" gives direct access to the 3314 * value 3315 */ 3316 public CapabilityStatementRestSecurityComponent setCorsElement(BooleanType value) { 3317 this.cors = value; 3318 return this; 3319 } 3320 3321 /** 3322 * @return Server adds CORS headers when responding to requests - this enables 3323 * Javascript applications to use the server. 3324 */ 3325 public boolean getCors() { 3326 return this.cors == null || this.cors.isEmpty() ? false : this.cors.getValue(); 3327 } 3328 3329 /** 3330 * @param value Server adds CORS headers when responding to requests - this 3331 * enables Javascript applications to use the server. 3332 */ 3333 public CapabilityStatementRestSecurityComponent setCors(boolean value) { 3334 if (this.cors == null) 3335 this.cors = new BooleanType(); 3336 this.cors.setValue(value); 3337 return this; 3338 } 3339 3340 /** 3341 * @return {@link #service} (Types of security services that are 3342 * supported/required by the system.) 3343 */ 3344 public List<CodeableConcept> getService() { 3345 if (this.service == null) 3346 this.service = new ArrayList<CodeableConcept>(); 3347 return this.service; 3348 } 3349 3350 /** 3351 * @return Returns a reference to <code>this</code> for easy method chaining 3352 */ 3353 public CapabilityStatementRestSecurityComponent setService(List<CodeableConcept> theService) { 3354 this.service = theService; 3355 return this; 3356 } 3357 3358 public boolean hasService() { 3359 if (this.service == null) 3360 return false; 3361 for (CodeableConcept item : this.service) 3362 if (!item.isEmpty()) 3363 return true; 3364 return false; 3365 } 3366 3367 public CodeableConcept addService() { // 3 3368 CodeableConcept t = new CodeableConcept(); 3369 if (this.service == null) 3370 this.service = new ArrayList<CodeableConcept>(); 3371 this.service.add(t); 3372 return t; 3373 } 3374 3375 public CapabilityStatementRestSecurityComponent addService(CodeableConcept t) { // 3 3376 if (t == null) 3377 return this; 3378 if (this.service == null) 3379 this.service = new ArrayList<CodeableConcept>(); 3380 this.service.add(t); 3381 return this; 3382 } 3383 3384 /** 3385 * @return The first repetition of repeating field {@link #service}, creating it 3386 * if it does not already exist 3387 */ 3388 public CodeableConcept getServiceFirstRep() { 3389 if (getService().isEmpty()) { 3390 addService(); 3391 } 3392 return getService().get(0); 3393 } 3394 3395 /** 3396 * @return {@link #description} (General description of how security works.). 3397 * This is the underlying object with id, value and extensions. The 3398 * accessor "getDescription" gives direct access to the value 3399 */ 3400 public MarkdownType getDescriptionElement() { 3401 if (this.description == null) 3402 if (Configuration.errorOnAutoCreate()) 3403 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.description"); 3404 else if (Configuration.doAutoCreate()) 3405 this.description = new MarkdownType(); // bb 3406 return this.description; 3407 } 3408 3409 public boolean hasDescriptionElement() { 3410 return this.description != null && !this.description.isEmpty(); 3411 } 3412 3413 public boolean hasDescription() { 3414 return this.description != null && !this.description.isEmpty(); 3415 } 3416 3417 /** 3418 * @param value {@link #description} (General description of how security 3419 * works.). This is the underlying object with id, value and 3420 * extensions. The accessor "getDescription" gives direct access to 3421 * the value 3422 */ 3423 public CapabilityStatementRestSecurityComponent setDescriptionElement(MarkdownType value) { 3424 this.description = value; 3425 return this; 3426 } 3427 3428 /** 3429 * @return General description of how security works. 3430 */ 3431 public String getDescription() { 3432 return this.description == null ? null : this.description.getValue(); 3433 } 3434 3435 /** 3436 * @param value General description of how security works. 3437 */ 3438 public CapabilityStatementRestSecurityComponent setDescription(String value) { 3439 if (value == null) 3440 this.description = null; 3441 else { 3442 if (this.description == null) 3443 this.description = new MarkdownType(); 3444 this.description.setValue(value); 3445 } 3446 return this; 3447 } 3448 3449 protected void listChildren(List<Property> children) { 3450 super.listChildren(children); 3451 children.add(new Property("cors", "boolean", 3452 "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.", 3453 0, 1, cors)); 3454 children.add(new Property("service", "CodeableConcept", 3455 "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, 3456 service)); 3457 children.add( 3458 new Property("description", "markdown", "General description of how security works.", 0, 1, description)); 3459 } 3460 3461 @Override 3462 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3463 switch (_hash) { 3464 case 3059629: 3465 /* cors */ return new Property("cors", "boolean", 3466 "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.", 3467 0, 1, cors); 3468 case 1984153269: 3469 /* service */ return new Property("service", "CodeableConcept", 3470 "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, 3471 service); 3472 case -1724546052: 3473 /* description */ return new Property("description", "markdown", "General description of how security works.", 3474 0, 1, description); 3475 default: 3476 return super.getNamedProperty(_hash, _name, _checkValid); 3477 } 3478 3479 } 3480 3481 @Override 3482 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3483 switch (hash) { 3484 case 3059629: 3485 /* cors */ return this.cors == null ? new Base[0] : new Base[] { this.cors }; // BooleanType 3486 case 1984153269: 3487 /* service */ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // CodeableConcept 3488 case -1724546052: 3489 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3490 default: 3491 return super.getProperty(hash, name, checkValid); 3492 } 3493 3494 } 3495 3496 @Override 3497 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3498 switch (hash) { 3499 case 3059629: // cors 3500 this.cors = castToBoolean(value); // BooleanType 3501 return value; 3502 case 1984153269: // service 3503 this.getService().add(castToCodeableConcept(value)); // CodeableConcept 3504 return value; 3505 case -1724546052: // description 3506 this.description = castToMarkdown(value); // MarkdownType 3507 return value; 3508 default: 3509 return super.setProperty(hash, name, value); 3510 } 3511 3512 } 3513 3514 @Override 3515 public Base setProperty(String name, Base value) throws FHIRException { 3516 if (name.equals("cors")) { 3517 this.cors = castToBoolean(value); // BooleanType 3518 } else if (name.equals("service")) { 3519 this.getService().add(castToCodeableConcept(value)); 3520 } else if (name.equals("description")) { 3521 this.description = castToMarkdown(value); // MarkdownType 3522 } else 3523 return super.setProperty(name, value); 3524 return value; 3525 } 3526 3527 @Override 3528 public void removeChild(String name, Base value) throws FHIRException { 3529 if (name.equals("cors")) { 3530 this.cors = null; 3531 } else if (name.equals("service")) { 3532 this.getService().remove(castToCodeableConcept(value)); 3533 } else if (name.equals("description")) { 3534 this.description = null; 3535 } else 3536 super.removeChild(name, value); 3537 3538 } 3539 3540 @Override 3541 public Base makeProperty(int hash, String name) throws FHIRException { 3542 switch (hash) { 3543 case 3059629: 3544 return getCorsElement(); 3545 case 1984153269: 3546 return addService(); 3547 case -1724546052: 3548 return getDescriptionElement(); 3549 default: 3550 return super.makeProperty(hash, name); 3551 } 3552 3553 } 3554 3555 @Override 3556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3557 switch (hash) { 3558 case 3059629: 3559 /* cors */ return new String[] { "boolean" }; 3560 case 1984153269: 3561 /* service */ return new String[] { "CodeableConcept" }; 3562 case -1724546052: 3563 /* description */ return new String[] { "markdown" }; 3564 default: 3565 return super.getTypesForProperty(hash, name); 3566 } 3567 3568 } 3569 3570 @Override 3571 public Base addChild(String name) throws FHIRException { 3572 if (name.equals("cors")) { 3573 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.cors"); 3574 } else if (name.equals("service")) { 3575 return addService(); 3576 } else if (name.equals("description")) { 3577 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 3578 } else 3579 return super.addChild(name); 3580 } 3581 3582 public CapabilityStatementRestSecurityComponent copy() { 3583 CapabilityStatementRestSecurityComponent dst = new CapabilityStatementRestSecurityComponent(); 3584 copyValues(dst); 3585 return dst; 3586 } 3587 3588 public void copyValues(CapabilityStatementRestSecurityComponent dst) { 3589 super.copyValues(dst); 3590 dst.cors = cors == null ? null : cors.copy(); 3591 if (service != null) { 3592 dst.service = new ArrayList<CodeableConcept>(); 3593 for (CodeableConcept i : service) 3594 dst.service.add(i.copy()); 3595 } 3596 ; 3597 dst.description = description == null ? null : description.copy(); 3598 } 3599 3600 @Override 3601 public boolean equalsDeep(Base other_) { 3602 if (!super.equalsDeep(other_)) 3603 return false; 3604 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 3605 return false; 3606 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 3607 return compareDeep(cors, o.cors, true) && compareDeep(service, o.service, true) 3608 && compareDeep(description, o.description, true); 3609 } 3610 3611 @Override 3612 public boolean equalsShallow(Base other_) { 3613 if (!super.equalsShallow(other_)) 3614 return false; 3615 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 3616 return false; 3617 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 3618 return compareValues(cors, o.cors, true) && compareValues(description, o.description, true); 3619 } 3620 3621 public boolean isEmpty() { 3622 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cors, service, description); 3623 } 3624 3625 public String fhirType() { 3626 return "CapabilityStatement.rest.security"; 3627 3628 } 3629 3630 } 3631 3632 @Block() 3633 public static class CapabilityStatementRestResourceComponent extends BackboneElement implements IBaseBackboneElement { 3634 /** 3635 * A type of resource exposed via the restful interface. 3636 */ 3637 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3638 @Description(shortDefinition = "A resource type that is supported", formalDefinition = "A type of resource exposed via the restful interface.") 3639 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 3640 protected CodeType type; 3641 3642 /** 3643 * A specification of the profile that describes the solution's overall support 3644 * for the resource, including any constraints on cardinality, bindings, lengths 3645 * or other limitations. See further discussion in [Using 3646 * Profiles](profiling.html#profile-uses). 3647 */ 3648 @Child(name = "profile", type = { 3649 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3650 @Description(shortDefinition = "Base System profile for all uses of resource", formalDefinition = "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).") 3651 protected CanonicalType profile; 3652 3653 /** 3654 * A list of profiles that represent different use cases supported by the 3655 * system. For a server, "supported by the system" means the system 3656 * hosts/produces a set of resources that are conformant to a particular 3657 * profile, and allows clients that use its services to search using this 3658 * profile and to find appropriate data. For a client, it means the system will 3659 * search by this profile and process data according to the guidance implicit in 3660 * the profile. See further discussion in [Using 3661 * Profiles](profiling.html#profile-uses). 3662 */ 3663 @Child(name = "supportedProfile", type = { 3664 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3665 @Description(shortDefinition = "Profiles for use cases supported", formalDefinition = "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).") 3666 protected List<CanonicalType> supportedProfile; 3667 3668 /** 3669 * Additional information about the resource type used by the system. 3670 */ 3671 @Child(name = "documentation", type = { 3672 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3673 @Description(shortDefinition = "Additional information about the use of the resource type", formalDefinition = "Additional information about the resource type used by the system.") 3674 protected MarkdownType documentation; 3675 3676 /** 3677 * Identifies a restful operation supported by the solution. 3678 */ 3679 @Child(name = "interaction", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3680 @Description(shortDefinition = "What operations are supported?", formalDefinition = "Identifies a restful operation supported by the solution.") 3681 protected List<ResourceInteractionComponent> interaction; 3682 3683 /** 3684 * This field is set to no-version to specify that the system does not support 3685 * (server) or use (client) versioning for this resource type. If this has some 3686 * other value, the server must at least correctly track and populate the 3687 * versionId meta-property on resources. If the value is 'versioned-update', 3688 * then the server supports all the versioning features, including using e-tags 3689 * for version integrity in the API. 3690 */ 3691 @Child(name = "versioning", type = { 3692 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3693 @Description(shortDefinition = "no-version | versioned | versioned-update", formalDefinition = "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.") 3694 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/versioning-policy") 3695 protected Enumeration<ResourceVersionPolicy> versioning; 3696 3697 /** 3698 * A flag for whether the server is able to return past versions as part of the 3699 * vRead operation. 3700 */ 3701 @Child(name = "readHistory", type = { 3702 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3703 @Description(shortDefinition = "Whether vRead can return past versions", formalDefinition = "A flag for whether the server is able to return past versions as part of the vRead operation.") 3704 protected BooleanType readHistory; 3705 3706 /** 3707 * A flag to indicate that the server allows or needs to allow the client to 3708 * create new identities on the server (that is, the client PUTs to a location 3709 * where there is no existing resource). Allowing this operation means that the 3710 * server allows the client to create new identities on the server. 3711 */ 3712 @Child(name = "updateCreate", type = { 3713 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3714 @Description(shortDefinition = "If update can commit to a new identity", formalDefinition = "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.") 3715 protected BooleanType updateCreate; 3716 3717 /** 3718 * A flag that indicates that the server supports conditional create. 3719 */ 3720 @Child(name = "conditionalCreate", type = { 3721 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 3722 @Description(shortDefinition = "If allows/uses conditional create", formalDefinition = "A flag that indicates that the server supports conditional create.") 3723 protected BooleanType conditionalCreate; 3724 3725 /** 3726 * A code that indicates how the server supports conditional read. 3727 */ 3728 @Child(name = "conditionalRead", type = { 3729 CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 3730 @Description(shortDefinition = "not-supported | modified-since | not-match | full-support", formalDefinition = "A code that indicates how the server supports conditional read.") 3731 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/conditional-read-status") 3732 protected Enumeration<ConditionalReadStatus> conditionalRead; 3733 3734 /** 3735 * A flag that indicates that the server supports conditional update. 3736 */ 3737 @Child(name = "conditionalUpdate", type = { 3738 BooleanType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 3739 @Description(shortDefinition = "If allows/uses conditional update", formalDefinition = "A flag that indicates that the server supports conditional update.") 3740 protected BooleanType conditionalUpdate; 3741 3742 /** 3743 * A code that indicates how the server supports conditional delete. 3744 */ 3745 @Child(name = "conditionalDelete", type = { 3746 CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 3747 @Description(shortDefinition = "not-supported | single | multiple - how conditional delete is supported", formalDefinition = "A code that indicates how the server supports conditional delete.") 3748 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/conditional-delete-status") 3749 protected Enumeration<ConditionalDeleteStatus> conditionalDelete; 3750 3751 /** 3752 * A set of flags that defines how references are supported. 3753 */ 3754 @Child(name = "referencePolicy", type = { 3755 CodeType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3756 @Description(shortDefinition = "literal | logical | resolves | enforced | local", formalDefinition = "A set of flags that defines how references are supported.") 3757 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reference-handling-policy") 3758 protected List<Enumeration<ReferenceHandlingPolicy>> referencePolicy; 3759 3760 /** 3761 * A list of _include values supported by the server. 3762 */ 3763 @Child(name = "searchInclude", type = { 3764 StringType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3765 @Description(shortDefinition = "_include values supported by the server", formalDefinition = "A list of _include values supported by the server.") 3766 protected List<StringType> searchInclude; 3767 3768 /** 3769 * A list of _revinclude (reverse include) values supported by the server. 3770 */ 3771 @Child(name = "searchRevInclude", type = { 3772 StringType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3773 @Description(shortDefinition = "_revinclude values supported by the server", formalDefinition = "A list of _revinclude (reverse include) values supported by the server.") 3774 protected List<StringType> searchRevInclude; 3775 3776 /** 3777 * Search parameters for implementations to support and/or make use of - either 3778 * references to ones defined in the specification, or additional ones defined 3779 * for/by the implementation. 3780 */ 3781 @Child(name = "searchParam", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3782 @Description(shortDefinition = "Search parameters supported by implementation", formalDefinition = "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 3783 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 3784 3785 /** 3786 * Definition of an operation or a named query together with its parameters and 3787 * their meaning and type. Consult the definition of the operation for details 3788 * about how to invoke the operation, and the parameters. 3789 */ 3790 @Child(name = "operation", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3791 @Description(shortDefinition = "Definition of a resource operation", formalDefinition = "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.") 3792 protected List<CapabilityStatementRestResourceOperationComponent> operation; 3793 3794 private static final long serialVersionUID = -1843372337L; 3795 3796 /** 3797 * Constructor 3798 */ 3799 public CapabilityStatementRestResourceComponent() { 3800 super(); 3801 } 3802 3803 /** 3804 * Constructor 3805 */ 3806 public CapabilityStatementRestResourceComponent(CodeType type) { 3807 super(); 3808 this.type = type; 3809 } 3810 3811 /** 3812 * @return {@link #type} (A type of resource exposed via the restful 3813 * interface.). This is the underlying object with id, value and 3814 * extensions. The accessor "getType" gives direct access to the value 3815 */ 3816 public CodeType getTypeElement() { 3817 if (this.type == null) 3818 if (Configuration.errorOnAutoCreate()) 3819 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.type"); 3820 else if (Configuration.doAutoCreate()) 3821 this.type = new CodeType(); // bb 3822 return this.type; 3823 } 3824 3825 public boolean hasTypeElement() { 3826 return this.type != null && !this.type.isEmpty(); 3827 } 3828 3829 public boolean hasType() { 3830 return this.type != null && !this.type.isEmpty(); 3831 } 3832 3833 /** 3834 * @param value {@link #type} (A type of resource exposed via the restful 3835 * interface.). This is the underlying object with id, value and 3836 * extensions. The accessor "getType" gives direct access to the 3837 * value 3838 */ 3839 public CapabilityStatementRestResourceComponent setTypeElement(CodeType value) { 3840 this.type = value; 3841 return this; 3842 } 3843 3844 /** 3845 * @return A type of resource exposed via the restful interface. 3846 */ 3847 public String getType() { 3848 return this.type == null ? null : this.type.getValue(); 3849 } 3850 3851 /** 3852 * @param value A type of resource exposed via the restful interface. 3853 */ 3854 public CapabilityStatementRestResourceComponent setType(String value) { 3855 if (this.type == null) 3856 this.type = new CodeType(); 3857 this.type.setValue(value); 3858 return this; 3859 } 3860 3861 /** 3862 * @return {@link #profile} (A specification of the profile that describes the 3863 * solution's overall support for the resource, including any 3864 * constraints on cardinality, bindings, lengths or other limitations. 3865 * See further discussion in [Using 3866 * Profiles](profiling.html#profile-uses).). This is the underlying 3867 * object with id, value and extensions. The accessor "getProfile" gives 3868 * direct access to the value 3869 */ 3870 public CanonicalType getProfileElement() { 3871 if (this.profile == null) 3872 if (Configuration.errorOnAutoCreate()) 3873 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.profile"); 3874 else if (Configuration.doAutoCreate()) 3875 this.profile = new CanonicalType(); // bb 3876 return this.profile; 3877 } 3878 3879 public boolean hasProfileElement() { 3880 return this.profile != null && !this.profile.isEmpty(); 3881 } 3882 3883 public boolean hasProfile() { 3884 return this.profile != null && !this.profile.isEmpty(); 3885 } 3886 3887 /** 3888 * @param value {@link #profile} (A specification of the profile that describes 3889 * the solution's overall support for the resource, including any 3890 * constraints on cardinality, bindings, lengths or other 3891 * limitations. See further discussion in [Using 3892 * Profiles](profiling.html#profile-uses).). This is the underlying 3893 * object with id, value and extensions. The accessor "getProfile" 3894 * gives direct access to the value 3895 */ 3896 public CapabilityStatementRestResourceComponent setProfileElement(CanonicalType value) { 3897 this.profile = value; 3898 return this; 3899 } 3900 3901 /** 3902 * @return A specification of the profile that describes the solution's overall 3903 * support for the resource, including any constraints on cardinality, 3904 * bindings, lengths or other limitations. See further discussion in 3905 * [Using Profiles](profiling.html#profile-uses). 3906 */ 3907 public String getProfile() { 3908 return this.profile == null ? null : this.profile.getValue(); 3909 } 3910 3911 /** 3912 * @param value A specification of the profile that describes the solution's 3913 * overall support for the resource, including any constraints on 3914 * cardinality, bindings, lengths or other limitations. See further 3915 * discussion in [Using Profiles](profiling.html#profile-uses). 3916 */ 3917 public CapabilityStatementRestResourceComponent setProfile(String value) { 3918 if (Utilities.noString(value)) 3919 this.profile = null; 3920 else { 3921 if (this.profile == null) 3922 this.profile = new CanonicalType(); 3923 this.profile.setValue(value); 3924 } 3925 return this; 3926 } 3927 3928 /** 3929 * @return {@link #supportedProfile} (A list of profiles that represent 3930 * different use cases supported by the system. For a server, "supported 3931 * by the system" means the system hosts/produces a set of resources 3932 * that are conformant to a particular profile, and allows clients that 3933 * use its services to search using this profile and to find appropriate 3934 * data. For a client, it means the system will search by this profile 3935 * and process data according to the guidance implicit in the profile. 3936 * See further discussion in [Using 3937 * Profiles](profiling.html#profile-uses).) 3938 */ 3939 public List<CanonicalType> getSupportedProfile() { 3940 if (this.supportedProfile == null) 3941 this.supportedProfile = new ArrayList<CanonicalType>(); 3942 return this.supportedProfile; 3943 } 3944 3945 /** 3946 * @return Returns a reference to <code>this</code> for easy method chaining 3947 */ 3948 public CapabilityStatementRestResourceComponent setSupportedProfile(List<CanonicalType> theSupportedProfile) { 3949 this.supportedProfile = theSupportedProfile; 3950 return this; 3951 } 3952 3953 public boolean hasSupportedProfile() { 3954 if (this.supportedProfile == null) 3955 return false; 3956 for (CanonicalType item : this.supportedProfile) 3957 if (!item.isEmpty()) 3958 return true; 3959 return false; 3960 } 3961 3962 /** 3963 * @return {@link #supportedProfile} (A list of profiles that represent 3964 * different use cases supported by the system. For a server, "supported 3965 * by the system" means the system hosts/produces a set of resources 3966 * that are conformant to a particular profile, and allows clients that 3967 * use its services to search using this profile and to find appropriate 3968 * data. For a client, it means the system will search by this profile 3969 * and process data according to the guidance implicit in the profile. 3970 * See further discussion in [Using 3971 * Profiles](profiling.html#profile-uses).) 3972 */ 3973 public CanonicalType addSupportedProfileElement() {// 2 3974 CanonicalType t = new CanonicalType(); 3975 if (this.supportedProfile == null) 3976 this.supportedProfile = new ArrayList<CanonicalType>(); 3977 this.supportedProfile.add(t); 3978 return t; 3979 } 3980 3981 /** 3982 * @param value {@link #supportedProfile} (A list of profiles that represent 3983 * different use cases supported by the system. For a server, 3984 * "supported by the system" means the system hosts/produces a set 3985 * of resources that are conformant to a particular profile, and 3986 * allows clients that use its services to search using this 3987 * profile and to find appropriate data. For a client, it means the 3988 * system will search by this profile and process data according to 3989 * the guidance implicit in the profile. See further discussion in 3990 * [Using Profiles](profiling.html#profile-uses).) 3991 */ 3992 public CapabilityStatementRestResourceComponent addSupportedProfile(String value) { // 1 3993 CanonicalType t = new CanonicalType(); 3994 t.setValue(value); 3995 if (this.supportedProfile == null) 3996 this.supportedProfile = new ArrayList<CanonicalType>(); 3997 this.supportedProfile.add(t); 3998 return this; 3999 } 4000 4001 /** 4002 * @param value {@link #supportedProfile} (A list of profiles that represent 4003 * different use cases supported by the system. For a server, 4004 * "supported by the system" means the system hosts/produces a set 4005 * of resources that are conformant to a particular profile, and 4006 * allows clients that use its services to search using this 4007 * profile and to find appropriate data. For a client, it means the 4008 * system will search by this profile and process data according to 4009 * the guidance implicit in the profile. See further discussion in 4010 * [Using Profiles](profiling.html#profile-uses).) 4011 */ 4012 public boolean hasSupportedProfile(String value) { 4013 if (this.supportedProfile == null) 4014 return false; 4015 for (CanonicalType v : this.supportedProfile) 4016 if (v.getValue().equals(value)) // canonical(StructureDefinition) 4017 return true; 4018 return false; 4019 } 4020 4021 /** 4022 * @return {@link #documentation} (Additional information about the resource 4023 * type used by the system.). This is the underlying object with id, 4024 * value and extensions. The accessor "getDocumentation" gives direct 4025 * access to the value 4026 */ 4027 public MarkdownType getDocumentationElement() { 4028 if (this.documentation == null) 4029 if (Configuration.errorOnAutoCreate()) 4030 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.documentation"); 4031 else if (Configuration.doAutoCreate()) 4032 this.documentation = new MarkdownType(); // bb 4033 return this.documentation; 4034 } 4035 4036 public boolean hasDocumentationElement() { 4037 return this.documentation != null && !this.documentation.isEmpty(); 4038 } 4039 4040 public boolean hasDocumentation() { 4041 return this.documentation != null && !this.documentation.isEmpty(); 4042 } 4043 4044 /** 4045 * @param value {@link #documentation} (Additional information about the 4046 * resource type used by the system.). This is the underlying 4047 * object with id, value and extensions. The accessor 4048 * "getDocumentation" gives direct access to the value 4049 */ 4050 public CapabilityStatementRestResourceComponent setDocumentationElement(MarkdownType value) { 4051 this.documentation = value; 4052 return this; 4053 } 4054 4055 /** 4056 * @return Additional information about the resource type used by the system. 4057 */ 4058 public String getDocumentation() { 4059 return this.documentation == null ? null : this.documentation.getValue(); 4060 } 4061 4062 /** 4063 * @param value Additional information about the resource type used by the 4064 * system. 4065 */ 4066 public CapabilityStatementRestResourceComponent setDocumentation(String value) { 4067 if (value == null) 4068 this.documentation = null; 4069 else { 4070 if (this.documentation == null) 4071 this.documentation = new MarkdownType(); 4072 this.documentation.setValue(value); 4073 } 4074 return this; 4075 } 4076 4077 /** 4078 * @return {@link #interaction} (Identifies a restful operation supported by the 4079 * solution.) 4080 */ 4081 public List<ResourceInteractionComponent> getInteraction() { 4082 if (this.interaction == null) 4083 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4084 return this.interaction; 4085 } 4086 4087 /** 4088 * @return Returns a reference to <code>this</code> for easy method chaining 4089 */ 4090 public CapabilityStatementRestResourceComponent setInteraction(List<ResourceInteractionComponent> theInteraction) { 4091 this.interaction = theInteraction; 4092 return this; 4093 } 4094 4095 public boolean hasInteraction() { 4096 if (this.interaction == null) 4097 return false; 4098 for (ResourceInteractionComponent item : this.interaction) 4099 if (!item.isEmpty()) 4100 return true; 4101 return false; 4102 } 4103 4104 public ResourceInteractionComponent addInteraction() { // 3 4105 ResourceInteractionComponent t = new ResourceInteractionComponent(); 4106 if (this.interaction == null) 4107 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4108 this.interaction.add(t); 4109 return t; 4110 } 4111 4112 public CapabilityStatementRestResourceComponent addInteraction(ResourceInteractionComponent t) { // 3 4113 if (t == null) 4114 return this; 4115 if (this.interaction == null) 4116 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4117 this.interaction.add(t); 4118 return this; 4119 } 4120 4121 /** 4122 * @return The first repetition of repeating field {@link #interaction}, 4123 * creating it if it does not already exist 4124 */ 4125 public ResourceInteractionComponent getInteractionFirstRep() { 4126 if (getInteraction().isEmpty()) { 4127 addInteraction(); 4128 } 4129 return getInteraction().get(0); 4130 } 4131 4132 /** 4133 * @return {@link #versioning} (This field is set to no-version to specify that 4134 * the system does not support (server) or use (client) versioning for 4135 * this resource type. If this has some other value, the server must at 4136 * least correctly track and populate the versionId meta-property on 4137 * resources. If the value is 'versioned-update', then the server 4138 * supports all the versioning features, including using e-tags for 4139 * version integrity in the API.). This is the underlying object with 4140 * id, value and extensions. The accessor "getVersioning" gives direct 4141 * access to the value 4142 */ 4143 public Enumeration<ResourceVersionPolicy> getVersioningElement() { 4144 if (this.versioning == null) 4145 if (Configuration.errorOnAutoCreate()) 4146 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.versioning"); 4147 else if (Configuration.doAutoCreate()) 4148 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); // bb 4149 return this.versioning; 4150 } 4151 4152 public boolean hasVersioningElement() { 4153 return this.versioning != null && !this.versioning.isEmpty(); 4154 } 4155 4156 public boolean hasVersioning() { 4157 return this.versioning != null && !this.versioning.isEmpty(); 4158 } 4159 4160 /** 4161 * @param value {@link #versioning} (This field is set to no-version to specify 4162 * that the system does not support (server) or use (client) 4163 * versioning for this resource type. If this has some other value, 4164 * the server must at least correctly track and populate the 4165 * versionId meta-property on resources. If the value is 4166 * 'versioned-update', then the server supports all the versioning 4167 * features, including using e-tags for version integrity in the 4168 * API.). This is the underlying object with id, value and 4169 * extensions. The accessor "getVersioning" gives direct access to 4170 * the value 4171 */ 4172 public CapabilityStatementRestResourceComponent setVersioningElement(Enumeration<ResourceVersionPolicy> value) { 4173 this.versioning = value; 4174 return this; 4175 } 4176 4177 /** 4178 * @return This field is set to no-version to specify that the system does not 4179 * support (server) or use (client) versioning for this resource type. 4180 * If this has some other value, the server must at least correctly 4181 * track and populate the versionId meta-property on resources. If the 4182 * value is 'versioned-update', then the server supports all the 4183 * versioning features, including using e-tags for version integrity in 4184 * the API. 4185 */ 4186 public ResourceVersionPolicy getVersioning() { 4187 return this.versioning == null ? null : this.versioning.getValue(); 4188 } 4189 4190 /** 4191 * @param value This field is set to no-version to specify that the system does 4192 * not support (server) or use (client) versioning for this 4193 * resource type. If this has some other value, the server must at 4194 * least correctly track and populate the versionId meta-property 4195 * on resources. If the value is 'versioned-update', then the 4196 * server supports all the versioning features, including using 4197 * e-tags for version integrity in the API. 4198 */ 4199 public CapabilityStatementRestResourceComponent setVersioning(ResourceVersionPolicy value) { 4200 if (value == null) 4201 this.versioning = null; 4202 else { 4203 if (this.versioning == null) 4204 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); 4205 this.versioning.setValue(value); 4206 } 4207 return this; 4208 } 4209 4210 /** 4211 * @return {@link #readHistory} (A flag for whether the server is able to return 4212 * past versions as part of the vRead operation.). This is the 4213 * underlying object with id, value and extensions. The accessor 4214 * "getReadHistory" gives direct access to the value 4215 */ 4216 public BooleanType getReadHistoryElement() { 4217 if (this.readHistory == null) 4218 if (Configuration.errorOnAutoCreate()) 4219 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.readHistory"); 4220 else if (Configuration.doAutoCreate()) 4221 this.readHistory = new BooleanType(); // bb 4222 return this.readHistory; 4223 } 4224 4225 public boolean hasReadHistoryElement() { 4226 return this.readHistory != null && !this.readHistory.isEmpty(); 4227 } 4228 4229 public boolean hasReadHistory() { 4230 return this.readHistory != null && !this.readHistory.isEmpty(); 4231 } 4232 4233 /** 4234 * @param value {@link #readHistory} (A flag for whether the server is able to 4235 * return past versions as part of the vRead operation.). This is 4236 * the underlying object with id, value and extensions. The 4237 * accessor "getReadHistory" gives direct access to the value 4238 */ 4239 public CapabilityStatementRestResourceComponent setReadHistoryElement(BooleanType value) { 4240 this.readHistory = value; 4241 return this; 4242 } 4243 4244 /** 4245 * @return A flag for whether the server is able to return past versions as part 4246 * of the vRead operation. 4247 */ 4248 public boolean getReadHistory() { 4249 return this.readHistory == null || this.readHistory.isEmpty() ? false : this.readHistory.getValue(); 4250 } 4251 4252 /** 4253 * @param value A flag for whether the server is able to return past versions as 4254 * part of the vRead operation. 4255 */ 4256 public CapabilityStatementRestResourceComponent setReadHistory(boolean value) { 4257 if (this.readHistory == null) 4258 this.readHistory = new BooleanType(); 4259 this.readHistory.setValue(value); 4260 return this; 4261 } 4262 4263 /** 4264 * @return {@link #updateCreate} (A flag to indicate that the server allows or 4265 * needs to allow the client to create new identities on the server 4266 * (that is, the client PUTs to a location where there is no existing 4267 * resource). Allowing this operation means that the server allows the 4268 * client to create new identities on the server.). This is the 4269 * underlying object with id, value and extensions. The accessor 4270 * "getUpdateCreate" gives direct access to the value 4271 */ 4272 public BooleanType getUpdateCreateElement() { 4273 if (this.updateCreate == null) 4274 if (Configuration.errorOnAutoCreate()) 4275 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.updateCreate"); 4276 else if (Configuration.doAutoCreate()) 4277 this.updateCreate = new BooleanType(); // bb 4278 return this.updateCreate; 4279 } 4280 4281 public boolean hasUpdateCreateElement() { 4282 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4283 } 4284 4285 public boolean hasUpdateCreate() { 4286 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4287 } 4288 4289 /** 4290 * @param value {@link #updateCreate} (A flag to indicate that the server allows 4291 * or needs to allow the client to create new identities on the 4292 * server (that is, the client PUTs to a location where there is no 4293 * existing resource). Allowing this operation means that the 4294 * server allows the client to create new identities on the 4295 * server.). This is the underlying object with id, value and 4296 * extensions. The accessor "getUpdateCreate" gives direct access 4297 * to the value 4298 */ 4299 public CapabilityStatementRestResourceComponent setUpdateCreateElement(BooleanType value) { 4300 this.updateCreate = value; 4301 return this; 4302 } 4303 4304 /** 4305 * @return A flag to indicate that the server allows or needs to allow the 4306 * client to create new identities on the server (that is, the client 4307 * PUTs to a location where there is no existing resource). Allowing 4308 * this operation means that the server allows the client to create new 4309 * identities on the server. 4310 */ 4311 public boolean getUpdateCreate() { 4312 return this.updateCreate == null || this.updateCreate.isEmpty() ? false : this.updateCreate.getValue(); 4313 } 4314 4315 /** 4316 * @param value A flag to indicate that the server allows or needs to allow the 4317 * client to create new identities on the server (that is, the 4318 * client PUTs to a location where there is no existing resource). 4319 * Allowing this operation means that the server allows the client 4320 * to create new identities on the server. 4321 */ 4322 public CapabilityStatementRestResourceComponent setUpdateCreate(boolean value) { 4323 if (this.updateCreate == null) 4324 this.updateCreate = new BooleanType(); 4325 this.updateCreate.setValue(value); 4326 return this; 4327 } 4328 4329 /** 4330 * @return {@link #conditionalCreate} (A flag that indicates that the server 4331 * supports conditional create.). This is the underlying object with id, 4332 * value and extensions. The accessor "getConditionalCreate" gives 4333 * direct access to the value 4334 */ 4335 public BooleanType getConditionalCreateElement() { 4336 if (this.conditionalCreate == null) 4337 if (Configuration.errorOnAutoCreate()) 4338 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalCreate"); 4339 else if (Configuration.doAutoCreate()) 4340 this.conditionalCreate = new BooleanType(); // bb 4341 return this.conditionalCreate; 4342 } 4343 4344 public boolean hasConditionalCreateElement() { 4345 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4346 } 4347 4348 public boolean hasConditionalCreate() { 4349 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4350 } 4351 4352 /** 4353 * @param value {@link #conditionalCreate} (A flag that indicates that the 4354 * server supports conditional create.). This is the underlying 4355 * object with id, value and extensions. The accessor 4356 * "getConditionalCreate" gives direct access to the value 4357 */ 4358 public CapabilityStatementRestResourceComponent setConditionalCreateElement(BooleanType value) { 4359 this.conditionalCreate = value; 4360 return this; 4361 } 4362 4363 /** 4364 * @return A flag that indicates that the server supports conditional create. 4365 */ 4366 public boolean getConditionalCreate() { 4367 return this.conditionalCreate == null || this.conditionalCreate.isEmpty() ? false 4368 : this.conditionalCreate.getValue(); 4369 } 4370 4371 /** 4372 * @param value A flag that indicates that the server supports conditional 4373 * create. 4374 */ 4375 public CapabilityStatementRestResourceComponent setConditionalCreate(boolean value) { 4376 if (this.conditionalCreate == null) 4377 this.conditionalCreate = new BooleanType(); 4378 this.conditionalCreate.setValue(value); 4379 return this; 4380 } 4381 4382 /** 4383 * @return {@link #conditionalRead} (A code that indicates how the server 4384 * supports conditional read.). This is the underlying object with id, 4385 * value and extensions. The accessor "getConditionalRead" gives direct 4386 * access to the value 4387 */ 4388 public Enumeration<ConditionalReadStatus> getConditionalReadElement() { 4389 if (this.conditionalRead == null) 4390 if (Configuration.errorOnAutoCreate()) 4391 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalRead"); 4392 else if (Configuration.doAutoCreate()) 4393 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); // bb 4394 return this.conditionalRead; 4395 } 4396 4397 public boolean hasConditionalReadElement() { 4398 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 4399 } 4400 4401 public boolean hasConditionalRead() { 4402 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 4403 } 4404 4405 /** 4406 * @param value {@link #conditionalRead} (A code that indicates how the server 4407 * supports conditional read.). This is the underlying object with 4408 * id, value and extensions. The accessor "getConditionalRead" 4409 * gives direct access to the value 4410 */ 4411 public CapabilityStatementRestResourceComponent setConditionalReadElement( 4412 Enumeration<ConditionalReadStatus> value) { 4413 this.conditionalRead = value; 4414 return this; 4415 } 4416 4417 /** 4418 * @return A code that indicates how the server supports conditional read. 4419 */ 4420 public ConditionalReadStatus getConditionalRead() { 4421 return this.conditionalRead == null ? null : this.conditionalRead.getValue(); 4422 } 4423 4424 /** 4425 * @param value A code that indicates how the server supports conditional read. 4426 */ 4427 public CapabilityStatementRestResourceComponent setConditionalRead(ConditionalReadStatus value) { 4428 if (value == null) 4429 this.conditionalRead = null; 4430 else { 4431 if (this.conditionalRead == null) 4432 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); 4433 this.conditionalRead.setValue(value); 4434 } 4435 return this; 4436 } 4437 4438 /** 4439 * @return {@link #conditionalUpdate} (A flag that indicates that the server 4440 * supports conditional update.). This is the underlying object with id, 4441 * value and extensions. The accessor "getConditionalUpdate" gives 4442 * direct access to the value 4443 */ 4444 public BooleanType getConditionalUpdateElement() { 4445 if (this.conditionalUpdate == null) 4446 if (Configuration.errorOnAutoCreate()) 4447 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalUpdate"); 4448 else if (Configuration.doAutoCreate()) 4449 this.conditionalUpdate = new BooleanType(); // bb 4450 return this.conditionalUpdate; 4451 } 4452 4453 public boolean hasConditionalUpdateElement() { 4454 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4455 } 4456 4457 public boolean hasConditionalUpdate() { 4458 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4459 } 4460 4461 /** 4462 * @param value {@link #conditionalUpdate} (A flag that indicates that the 4463 * server supports conditional update.). This is the underlying 4464 * object with id, value and extensions. The accessor 4465 * "getConditionalUpdate" gives direct access to the value 4466 */ 4467 public CapabilityStatementRestResourceComponent setConditionalUpdateElement(BooleanType value) { 4468 this.conditionalUpdate = value; 4469 return this; 4470 } 4471 4472 /** 4473 * @return A flag that indicates that the server supports conditional update. 4474 */ 4475 public boolean getConditionalUpdate() { 4476 return this.conditionalUpdate == null || this.conditionalUpdate.isEmpty() ? false 4477 : this.conditionalUpdate.getValue(); 4478 } 4479 4480 /** 4481 * @param value A flag that indicates that the server supports conditional 4482 * update. 4483 */ 4484 public CapabilityStatementRestResourceComponent setConditionalUpdate(boolean value) { 4485 if (this.conditionalUpdate == null) 4486 this.conditionalUpdate = new BooleanType(); 4487 this.conditionalUpdate.setValue(value); 4488 return this; 4489 } 4490 4491 /** 4492 * @return {@link #conditionalDelete} (A code that indicates how the server 4493 * supports conditional delete.). This is the underlying object with id, 4494 * value and extensions. The accessor "getConditionalDelete" gives 4495 * direct access to the value 4496 */ 4497 public Enumeration<ConditionalDeleteStatus> getConditionalDeleteElement() { 4498 if (this.conditionalDelete == null) 4499 if (Configuration.errorOnAutoCreate()) 4500 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalDelete"); 4501 else if (Configuration.doAutoCreate()) 4502 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); // bb 4503 return this.conditionalDelete; 4504 } 4505 4506 public boolean hasConditionalDeleteElement() { 4507 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4508 } 4509 4510 public boolean hasConditionalDelete() { 4511 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4512 } 4513 4514 /** 4515 * @param value {@link #conditionalDelete} (A code that indicates how the server 4516 * supports conditional delete.). This is the underlying object 4517 * with id, value and extensions. The accessor 4518 * "getConditionalDelete" gives direct access to the value 4519 */ 4520 public CapabilityStatementRestResourceComponent setConditionalDeleteElement( 4521 Enumeration<ConditionalDeleteStatus> value) { 4522 this.conditionalDelete = value; 4523 return this; 4524 } 4525 4526 /** 4527 * @return A code that indicates how the server supports conditional delete. 4528 */ 4529 public ConditionalDeleteStatus getConditionalDelete() { 4530 return this.conditionalDelete == null ? null : this.conditionalDelete.getValue(); 4531 } 4532 4533 /** 4534 * @param value A code that indicates how the server supports conditional 4535 * delete. 4536 */ 4537 public CapabilityStatementRestResourceComponent setConditionalDelete(ConditionalDeleteStatus value) { 4538 if (value == null) 4539 this.conditionalDelete = null; 4540 else { 4541 if (this.conditionalDelete == null) 4542 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); 4543 this.conditionalDelete.setValue(value); 4544 } 4545 return this; 4546 } 4547 4548 /** 4549 * @return {@link #referencePolicy} (A set of flags that defines how references 4550 * are supported.) 4551 */ 4552 public List<Enumeration<ReferenceHandlingPolicy>> getReferencePolicy() { 4553 if (this.referencePolicy == null) 4554 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4555 return this.referencePolicy; 4556 } 4557 4558 /** 4559 * @return Returns a reference to <code>this</code> for easy method chaining 4560 */ 4561 public CapabilityStatementRestResourceComponent setReferencePolicy( 4562 List<Enumeration<ReferenceHandlingPolicy>> theReferencePolicy) { 4563 this.referencePolicy = theReferencePolicy; 4564 return this; 4565 } 4566 4567 public boolean hasReferencePolicy() { 4568 if (this.referencePolicy == null) 4569 return false; 4570 for (Enumeration<ReferenceHandlingPolicy> item : this.referencePolicy) 4571 if (!item.isEmpty()) 4572 return true; 4573 return false; 4574 } 4575 4576 /** 4577 * @return {@link #referencePolicy} (A set of flags that defines how references 4578 * are supported.) 4579 */ 4580 public Enumeration<ReferenceHandlingPolicy> addReferencePolicyElement() {// 2 4581 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>( 4582 new ReferenceHandlingPolicyEnumFactory()); 4583 if (this.referencePolicy == null) 4584 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4585 this.referencePolicy.add(t); 4586 return t; 4587 } 4588 4589 /** 4590 * @param value {@link #referencePolicy} (A set of flags that defines how 4591 * references are supported.) 4592 */ 4593 public CapabilityStatementRestResourceComponent addReferencePolicy(ReferenceHandlingPolicy value) { // 1 4594 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>( 4595 new ReferenceHandlingPolicyEnumFactory()); 4596 t.setValue(value); 4597 if (this.referencePolicy == null) 4598 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4599 this.referencePolicy.add(t); 4600 return this; 4601 } 4602 4603 /** 4604 * @param value {@link #referencePolicy} (A set of flags that defines how 4605 * references are supported.) 4606 */ 4607 public boolean hasReferencePolicy(ReferenceHandlingPolicy value) { 4608 if (this.referencePolicy == null) 4609 return false; 4610 for (Enumeration<ReferenceHandlingPolicy> v : this.referencePolicy) 4611 if (v.getValue().equals(value)) // code 4612 return true; 4613 return false; 4614 } 4615 4616 /** 4617 * @return {@link #searchInclude} (A list of _include values supported by the 4618 * server.) 4619 */ 4620 public List<StringType> getSearchInclude() { 4621 if (this.searchInclude == null) 4622 this.searchInclude = new ArrayList<StringType>(); 4623 return this.searchInclude; 4624 } 4625 4626 /** 4627 * @return Returns a reference to <code>this</code> for easy method chaining 4628 */ 4629 public CapabilityStatementRestResourceComponent setSearchInclude(List<StringType> theSearchInclude) { 4630 this.searchInclude = theSearchInclude; 4631 return this; 4632 } 4633 4634 public boolean hasSearchInclude() { 4635 if (this.searchInclude == null) 4636 return false; 4637 for (StringType item : this.searchInclude) 4638 if (!item.isEmpty()) 4639 return true; 4640 return false; 4641 } 4642 4643 /** 4644 * @return {@link #searchInclude} (A list of _include values supported by the 4645 * server.) 4646 */ 4647 public StringType addSearchIncludeElement() {// 2 4648 StringType t = new StringType(); 4649 if (this.searchInclude == null) 4650 this.searchInclude = new ArrayList<StringType>(); 4651 this.searchInclude.add(t); 4652 return t; 4653 } 4654 4655 /** 4656 * @param value {@link #searchInclude} (A list of _include values supported by 4657 * the server.) 4658 */ 4659 public CapabilityStatementRestResourceComponent addSearchInclude(String value) { // 1 4660 StringType t = new StringType(); 4661 t.setValue(value); 4662 if (this.searchInclude == null) 4663 this.searchInclude = new ArrayList<StringType>(); 4664 this.searchInclude.add(t); 4665 return this; 4666 } 4667 4668 /** 4669 * @param value {@link #searchInclude} (A list of _include values supported by 4670 * the server.) 4671 */ 4672 public boolean hasSearchInclude(String value) { 4673 if (this.searchInclude == null) 4674 return false; 4675 for (StringType v : this.searchInclude) 4676 if (v.getValue().equals(value)) // string 4677 return true; 4678 return false; 4679 } 4680 4681 /** 4682 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4683 * values supported by the server.) 4684 */ 4685 public List<StringType> getSearchRevInclude() { 4686 if (this.searchRevInclude == null) 4687 this.searchRevInclude = new ArrayList<StringType>(); 4688 return this.searchRevInclude; 4689 } 4690 4691 /** 4692 * @return Returns a reference to <code>this</code> for easy method chaining 4693 */ 4694 public CapabilityStatementRestResourceComponent setSearchRevInclude(List<StringType> theSearchRevInclude) { 4695 this.searchRevInclude = theSearchRevInclude; 4696 return this; 4697 } 4698 4699 public boolean hasSearchRevInclude() { 4700 if (this.searchRevInclude == null) 4701 return false; 4702 for (StringType item : this.searchRevInclude) 4703 if (!item.isEmpty()) 4704 return true; 4705 return false; 4706 } 4707 4708 /** 4709 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4710 * values supported by the server.) 4711 */ 4712 public StringType addSearchRevIncludeElement() {// 2 4713 StringType t = new StringType(); 4714 if (this.searchRevInclude == null) 4715 this.searchRevInclude = new ArrayList<StringType>(); 4716 this.searchRevInclude.add(t); 4717 return t; 4718 } 4719 4720 /** 4721 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4722 * include) values supported by the server.) 4723 */ 4724 public CapabilityStatementRestResourceComponent addSearchRevInclude(String value) { // 1 4725 StringType t = new StringType(); 4726 t.setValue(value); 4727 if (this.searchRevInclude == null) 4728 this.searchRevInclude = new ArrayList<StringType>(); 4729 this.searchRevInclude.add(t); 4730 return this; 4731 } 4732 4733 /** 4734 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4735 * include) values supported by the server.) 4736 */ 4737 public boolean hasSearchRevInclude(String value) { 4738 if (this.searchRevInclude == null) 4739 return false; 4740 for (StringType v : this.searchRevInclude) 4741 if (v.getValue().equals(value)) // string 4742 return true; 4743 return false; 4744 } 4745 4746 /** 4747 * @return {@link #searchParam} (Search parameters for implementations to 4748 * support and/or make use of - either references to ones defined in the 4749 * specification, or additional ones defined for/by the implementation.) 4750 */ 4751 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 4752 if (this.searchParam == null) 4753 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4754 return this.searchParam; 4755 } 4756 4757 /** 4758 * @return Returns a reference to <code>this</code> for easy method chaining 4759 */ 4760 public CapabilityStatementRestResourceComponent setSearchParam( 4761 List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 4762 this.searchParam = theSearchParam; 4763 return this; 4764 } 4765 4766 public boolean hasSearchParam() { 4767 if (this.searchParam == null) 4768 return false; 4769 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 4770 if (!item.isEmpty()) 4771 return true; 4772 return false; 4773 } 4774 4775 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { // 3 4776 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 4777 if (this.searchParam == null) 4778 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4779 this.searchParam.add(t); 4780 return t; 4781 } 4782 4783 public CapabilityStatementRestResourceComponent addSearchParam( 4784 CapabilityStatementRestResourceSearchParamComponent t) { // 3 4785 if (t == null) 4786 return this; 4787 if (this.searchParam == null) 4788 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4789 this.searchParam.add(t); 4790 return this; 4791 } 4792 4793 /** 4794 * @return The first repetition of repeating field {@link #searchParam}, 4795 * creating it if it does not already exist 4796 */ 4797 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 4798 if (getSearchParam().isEmpty()) { 4799 addSearchParam(); 4800 } 4801 return getSearchParam().get(0); 4802 } 4803 4804 /** 4805 * @return {@link #operation} (Definition of an operation or a named query 4806 * together with its parameters and their meaning and type. Consult the 4807 * definition of the operation for details about how to invoke the 4808 * operation, and the parameters.) 4809 */ 4810 public List<CapabilityStatementRestResourceOperationComponent> getOperation() { 4811 if (this.operation == null) 4812 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4813 return this.operation; 4814 } 4815 4816 /** 4817 * @return Returns a reference to <code>this</code> for easy method chaining 4818 */ 4819 public CapabilityStatementRestResourceComponent setOperation( 4820 List<CapabilityStatementRestResourceOperationComponent> theOperation) { 4821 this.operation = theOperation; 4822 return this; 4823 } 4824 4825 public boolean hasOperation() { 4826 if (this.operation == null) 4827 return false; 4828 for (CapabilityStatementRestResourceOperationComponent item : this.operation) 4829 if (!item.isEmpty()) 4830 return true; 4831 return false; 4832 } 4833 4834 public CapabilityStatementRestResourceOperationComponent addOperation() { // 3 4835 CapabilityStatementRestResourceOperationComponent t = new CapabilityStatementRestResourceOperationComponent(); 4836 if (this.operation == null) 4837 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4838 this.operation.add(t); 4839 return t; 4840 } 4841 4842 public CapabilityStatementRestResourceComponent addOperation(CapabilityStatementRestResourceOperationComponent t) { // 3 4843 if (t == null) 4844 return this; 4845 if (this.operation == null) 4846 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4847 this.operation.add(t); 4848 return this; 4849 } 4850 4851 /** 4852 * @return The first repetition of repeating field {@link #operation}, creating 4853 * it if it does not already exist 4854 */ 4855 public CapabilityStatementRestResourceOperationComponent getOperationFirstRep() { 4856 if (getOperation().isEmpty()) { 4857 addOperation(); 4858 } 4859 return getOperation().get(0); 4860 } 4861 4862 protected void listChildren(List<Property> children) { 4863 super.listChildren(children); 4864 children.add(new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, type)); 4865 children.add(new Property("profile", "canonical(StructureDefinition)", 4866 "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4867 0, 1, profile)); 4868 children.add(new Property("supportedProfile", "canonical(StructureDefinition)", 4869 "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4870 0, java.lang.Integer.MAX_VALUE, supportedProfile)); 4871 children.add(new Property("documentation", "markdown", 4872 "Additional information about the resource type used by the system.", 0, 1, documentation)); 4873 children.add(new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, 4874 java.lang.Integer.MAX_VALUE, interaction)); 4875 children.add(new Property("versioning", "code", 4876 "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 4877 0, 1, versioning)); 4878 children.add(new Property("readHistory", "boolean", 4879 "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, 4880 readHistory)); 4881 children.add(new Property("updateCreate", "boolean", 4882 "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 4883 0, 1, updateCreate)); 4884 children.add(new Property("conditionalCreate", "boolean", 4885 "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate)); 4886 children.add(new Property("conditionalRead", "code", 4887 "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead)); 4888 children.add(new Property("conditionalUpdate", "boolean", 4889 "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate)); 4890 children.add(new Property("conditionalDelete", "code", 4891 "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete)); 4892 children.add(new Property("referencePolicy", "code", "A set of flags that defines how references are supported.", 4893 0, java.lang.Integer.MAX_VALUE, referencePolicy)); 4894 children.add(new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, 4895 java.lang.Integer.MAX_VALUE, searchInclude)); 4896 children.add(new Property("searchRevInclude", "string", 4897 "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, 4898 searchRevInclude)); 4899 children.add(new Property("searchParam", "", 4900 "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 4901 0, java.lang.Integer.MAX_VALUE, searchParam)); 4902 children.add(new Property("operation", "", 4903 "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.", 4904 0, java.lang.Integer.MAX_VALUE, operation)); 4905 } 4906 4907 @Override 4908 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4909 switch (_hash) { 4910 case 3575610: 4911 /* type */ return new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, 4912 type); 4913 case -309425751: 4914 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 4915 "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4916 0, 1, profile); 4917 case 1225477403: 4918 /* supportedProfile */ return new Property("supportedProfile", "canonical(StructureDefinition)", 4919 "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles](profiling.html#profile-uses).", 4920 0, java.lang.Integer.MAX_VALUE, supportedProfile); 4921 case 1587405498: 4922 /* documentation */ return new Property("documentation", "markdown", 4923 "Additional information about the resource type used by the system.", 0, 1, documentation); 4924 case 1844104722: 4925 /* interaction */ return new Property("interaction", "", 4926 "Identifies a restful operation supported by the solution.", 0, java.lang.Integer.MAX_VALUE, interaction); 4927 case -670487542: 4928 /* versioning */ return new Property("versioning", "code", 4929 "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 4930 0, 1, versioning); 4931 case 187518494: 4932 /* readHistory */ return new Property("readHistory", "boolean", 4933 "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, 4934 readHistory); 4935 case -1400550619: 4936 /* updateCreate */ return new Property("updateCreate", "boolean", 4937 "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 4938 0, 1, updateCreate); 4939 case 6401826: 4940 /* conditionalCreate */ return new Property("conditionalCreate", "boolean", 4941 "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate); 4942 case 822786364: 4943 /* conditionalRead */ return new Property("conditionalRead", "code", 4944 "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead); 4945 case 519849711: 4946 /* conditionalUpdate */ return new Property("conditionalUpdate", "boolean", 4947 "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate); 4948 case 23237585: 4949 /* conditionalDelete */ return new Property("conditionalDelete", "code", 4950 "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete); 4951 case 796257373: 4952 /* referencePolicy */ return new Property("referencePolicy", "code", 4953 "A set of flags that defines how references are supported.", 0, java.lang.Integer.MAX_VALUE, 4954 referencePolicy); 4955 case -1035904544: 4956 /* searchInclude */ return new Property("searchInclude", "string", 4957 "A list of _include values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchInclude); 4958 case -2123884979: 4959 /* searchRevInclude */ return new Property("searchRevInclude", "string", 4960 "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, 4961 searchRevInclude); 4962 case -553645115: 4963 /* searchParam */ return new Property("searchParam", "", 4964 "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 4965 0, java.lang.Integer.MAX_VALUE, searchParam); 4966 case 1662702951: 4967 /* operation */ return new Property("operation", "", 4968 "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.", 4969 0, java.lang.Integer.MAX_VALUE, operation); 4970 default: 4971 return super.getNamedProperty(_hash, _name, _checkValid); 4972 } 4973 4974 } 4975 4976 @Override 4977 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4978 switch (hash) { 4979 case 3575610: 4980 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 4981 case -309425751: 4982 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 4983 case 1225477403: 4984 /* supportedProfile */ return this.supportedProfile == null ? new Base[0] 4985 : this.supportedProfile.toArray(new Base[this.supportedProfile.size()]); // CanonicalType 4986 case 1587405498: 4987 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 4988 case 1844104722: 4989 /* interaction */ return this.interaction == null ? new Base[0] 4990 : this.interaction.toArray(new Base[this.interaction.size()]); // ResourceInteractionComponent 4991 case -670487542: 4992 /* versioning */ return this.versioning == null ? new Base[0] : new Base[] { this.versioning }; // Enumeration<ResourceVersionPolicy> 4993 case 187518494: 4994 /* readHistory */ return this.readHistory == null ? new Base[0] : new Base[] { this.readHistory }; // BooleanType 4995 case -1400550619: 4996 /* updateCreate */ return this.updateCreate == null ? new Base[0] : new Base[] { this.updateCreate }; // BooleanType 4997 case 6401826: 4998 /* conditionalCreate */ return this.conditionalCreate == null ? new Base[0] 4999 : new Base[] { this.conditionalCreate }; // BooleanType 5000 case 822786364: 5001 /* conditionalRead */ return this.conditionalRead == null ? new Base[0] : new Base[] { this.conditionalRead }; // Enumeration<ConditionalReadStatus> 5002 case 519849711: 5003 /* conditionalUpdate */ return this.conditionalUpdate == null ? new Base[0] 5004 : new Base[] { this.conditionalUpdate }; // BooleanType 5005 case 23237585: 5006 /* conditionalDelete */ return this.conditionalDelete == null ? new Base[0] 5007 : new Base[] { this.conditionalDelete }; // Enumeration<ConditionalDeleteStatus> 5008 case 796257373: 5009 /* referencePolicy */ return this.referencePolicy == null ? new Base[0] 5010 : this.referencePolicy.toArray(new Base[this.referencePolicy.size()]); // Enumeration<ReferenceHandlingPolicy> 5011 case -1035904544: 5012 /* searchInclude */ return this.searchInclude == null ? new Base[0] 5013 : this.searchInclude.toArray(new Base[this.searchInclude.size()]); // StringType 5014 case -2123884979: 5015 /* searchRevInclude */ return this.searchRevInclude == null ? new Base[0] 5016 : this.searchRevInclude.toArray(new Base[this.searchRevInclude.size()]); // StringType 5017 case -553645115: 5018 /* searchParam */ return this.searchParam == null ? new Base[0] 5019 : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 5020 case 1662702951: 5021 /* operation */ return this.operation == null ? new Base[0] 5022 : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestResourceOperationComponent 5023 default: 5024 return super.getProperty(hash, name, checkValid); 5025 } 5026 5027 } 5028 5029 @Override 5030 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5031 switch (hash) { 5032 case 3575610: // type 5033 this.type = castToCode(value); // CodeType 5034 return value; 5035 case -309425751: // profile 5036 this.profile = castToCanonical(value); // CanonicalType 5037 return value; 5038 case 1225477403: // supportedProfile 5039 this.getSupportedProfile().add(castToCanonical(value)); // CanonicalType 5040 return value; 5041 case 1587405498: // documentation 5042 this.documentation = castToMarkdown(value); // MarkdownType 5043 return value; 5044 case 1844104722: // interaction 5045 this.getInteraction().add((ResourceInteractionComponent) value); // ResourceInteractionComponent 5046 return value; 5047 case -670487542: // versioning 5048 value = new ResourceVersionPolicyEnumFactory().fromType(castToCode(value)); 5049 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 5050 return value; 5051 case 187518494: // readHistory 5052 this.readHistory = castToBoolean(value); // BooleanType 5053 return value; 5054 case -1400550619: // updateCreate 5055 this.updateCreate = castToBoolean(value); // BooleanType 5056 return value; 5057 case 6401826: // conditionalCreate 5058 this.conditionalCreate = castToBoolean(value); // BooleanType 5059 return value; 5060 case 822786364: // conditionalRead 5061 value = new ConditionalReadStatusEnumFactory().fromType(castToCode(value)); 5062 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 5063 return value; 5064 case 519849711: // conditionalUpdate 5065 this.conditionalUpdate = castToBoolean(value); // BooleanType 5066 return value; 5067 case 23237585: // conditionalDelete 5068 value = new ConditionalDeleteStatusEnumFactory().fromType(castToCode(value)); 5069 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 5070 return value; 5071 case 796257373: // referencePolicy 5072 value = new ReferenceHandlingPolicyEnumFactory().fromType(castToCode(value)); 5073 this.getReferencePolicy().add((Enumeration) value); // Enumeration<ReferenceHandlingPolicy> 5074 return value; 5075 case -1035904544: // searchInclude 5076 this.getSearchInclude().add(castToString(value)); // StringType 5077 return value; 5078 case -2123884979: // searchRevInclude 5079 this.getSearchRevInclude().add(castToString(value)); // StringType 5080 return value; 5081 case -553645115: // searchParam 5082 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 5083 return value; 5084 case 1662702951: // operation 5085 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); // CapabilityStatementRestResourceOperationComponent 5086 return value; 5087 default: 5088 return super.setProperty(hash, name, value); 5089 } 5090 5091 } 5092 5093 @Override 5094 public Base setProperty(String name, Base value) throws FHIRException { 5095 if (name.equals("type")) { 5096 this.type = castToCode(value); // CodeType 5097 } else if (name.equals("profile")) { 5098 this.profile = castToCanonical(value); // CanonicalType 5099 } else if (name.equals("supportedProfile")) { 5100 this.getSupportedProfile().add(castToCanonical(value)); 5101 } else if (name.equals("documentation")) { 5102 this.documentation = castToMarkdown(value); // MarkdownType 5103 } else if (name.equals("interaction")) { 5104 this.getInteraction().add((ResourceInteractionComponent) value); 5105 } else if (name.equals("versioning")) { 5106 value = new ResourceVersionPolicyEnumFactory().fromType(castToCode(value)); 5107 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 5108 } else if (name.equals("readHistory")) { 5109 this.readHistory = castToBoolean(value); // BooleanType 5110 } else if (name.equals("updateCreate")) { 5111 this.updateCreate = castToBoolean(value); // BooleanType 5112 } else if (name.equals("conditionalCreate")) { 5113 this.conditionalCreate = castToBoolean(value); // BooleanType 5114 } else if (name.equals("conditionalRead")) { 5115 value = new ConditionalReadStatusEnumFactory().fromType(castToCode(value)); 5116 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 5117 } else if (name.equals("conditionalUpdate")) { 5118 this.conditionalUpdate = castToBoolean(value); // BooleanType 5119 } else if (name.equals("conditionalDelete")) { 5120 value = new ConditionalDeleteStatusEnumFactory().fromType(castToCode(value)); 5121 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 5122 } else if (name.equals("referencePolicy")) { 5123 value = new ReferenceHandlingPolicyEnumFactory().fromType(castToCode(value)); 5124 this.getReferencePolicy().add((Enumeration) value); 5125 } else if (name.equals("searchInclude")) { 5126 this.getSearchInclude().add(castToString(value)); 5127 } else if (name.equals("searchRevInclude")) { 5128 this.getSearchRevInclude().add(castToString(value)); 5129 } else if (name.equals("searchParam")) { 5130 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 5131 } else if (name.equals("operation")) { 5132 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); 5133 } else 5134 return super.setProperty(name, value); 5135 return value; 5136 } 5137 5138 @Override 5139 public void removeChild(String name, Base value) throws FHIRException { 5140 if (name.equals("type")) { 5141 this.type = null; 5142 } else if (name.equals("profile")) { 5143 this.profile = null; 5144 } else if (name.equals("supportedProfile")) { 5145 this.getSupportedProfile().remove(castToCanonical(value)); 5146 } else if (name.equals("documentation")) { 5147 this.documentation = null; 5148 } else if (name.equals("interaction")) { 5149 this.getInteraction().remove((ResourceInteractionComponent) value); 5150 } else if (name.equals("versioning")) { 5151 this.versioning = null; 5152 } else if (name.equals("readHistory")) { 5153 this.readHistory = null; 5154 } else if (name.equals("updateCreate")) { 5155 this.updateCreate = null; 5156 } else if (name.equals("conditionalCreate")) { 5157 this.conditionalCreate = null; 5158 } else if (name.equals("conditionalRead")) { 5159 this.conditionalRead = null; 5160 } else if (name.equals("conditionalUpdate")) { 5161 this.conditionalUpdate = null; 5162 } else if (name.equals("conditionalDelete")) { 5163 this.conditionalDelete = null; 5164 } else if (name.equals("referencePolicy")) { 5165 this.getReferencePolicy().remove((Enumeration) value); 5166 } else if (name.equals("searchInclude")) { 5167 this.getSearchInclude().remove(castToString(value)); 5168 } else if (name.equals("searchRevInclude")) { 5169 this.getSearchRevInclude().remove(castToString(value)); 5170 } else if (name.equals("searchParam")) { 5171 this.getSearchParam().remove((CapabilityStatementRestResourceSearchParamComponent) value); 5172 } else if (name.equals("operation")) { 5173 this.getOperation().remove((CapabilityStatementRestResourceOperationComponent) value); 5174 } else 5175 super.removeChild(name, value); 5176 5177 } 5178 5179 @Override 5180 public Base makeProperty(int hash, String name) throws FHIRException { 5181 switch (hash) { 5182 case 3575610: 5183 return getTypeElement(); 5184 case -309425751: 5185 return getProfileElement(); 5186 case 1225477403: 5187 return addSupportedProfileElement(); 5188 case 1587405498: 5189 return getDocumentationElement(); 5190 case 1844104722: 5191 return addInteraction(); 5192 case -670487542: 5193 return getVersioningElement(); 5194 case 187518494: 5195 return getReadHistoryElement(); 5196 case -1400550619: 5197 return getUpdateCreateElement(); 5198 case 6401826: 5199 return getConditionalCreateElement(); 5200 case 822786364: 5201 return getConditionalReadElement(); 5202 case 519849711: 5203 return getConditionalUpdateElement(); 5204 case 23237585: 5205 return getConditionalDeleteElement(); 5206 case 796257373: 5207 return addReferencePolicyElement(); 5208 case -1035904544: 5209 return addSearchIncludeElement(); 5210 case -2123884979: 5211 return addSearchRevIncludeElement(); 5212 case -553645115: 5213 return addSearchParam(); 5214 case 1662702951: 5215 return addOperation(); 5216 default: 5217 return super.makeProperty(hash, name); 5218 } 5219 5220 } 5221 5222 @Override 5223 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5224 switch (hash) { 5225 case 3575610: 5226 /* type */ return new String[] { "code" }; 5227 case -309425751: 5228 /* profile */ return new String[] { "canonical" }; 5229 case 1225477403: 5230 /* supportedProfile */ return new String[] { "canonical" }; 5231 case 1587405498: 5232 /* documentation */ return new String[] { "markdown" }; 5233 case 1844104722: 5234 /* interaction */ return new String[] {}; 5235 case -670487542: 5236 /* versioning */ return new String[] { "code" }; 5237 case 187518494: 5238 /* readHistory */ return new String[] { "boolean" }; 5239 case -1400550619: 5240 /* updateCreate */ return new String[] { "boolean" }; 5241 case 6401826: 5242 /* conditionalCreate */ return new String[] { "boolean" }; 5243 case 822786364: 5244 /* conditionalRead */ return new String[] { "code" }; 5245 case 519849711: 5246 /* conditionalUpdate */ return new String[] { "boolean" }; 5247 case 23237585: 5248 /* conditionalDelete */ return new String[] { "code" }; 5249 case 796257373: 5250 /* referencePolicy */ return new String[] { "code" }; 5251 case -1035904544: 5252 /* searchInclude */ return new String[] { "string" }; 5253 case -2123884979: 5254 /* searchRevInclude */ return new String[] { "string" }; 5255 case -553645115: 5256 /* searchParam */ return new String[] {}; 5257 case 1662702951: 5258 /* operation */ return new String[] {}; 5259 default: 5260 return super.getTypesForProperty(hash, name); 5261 } 5262 5263 } 5264 5265 @Override 5266 public Base addChild(String name) throws FHIRException { 5267 if (name.equals("type")) { 5268 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 5269 } else if (name.equals("profile")) { 5270 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.profile"); 5271 } else if (name.equals("supportedProfile")) { 5272 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.supportedProfile"); 5273 } else if (name.equals("documentation")) { 5274 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 5275 } else if (name.equals("interaction")) { 5276 return addInteraction(); 5277 } else if (name.equals("versioning")) { 5278 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.versioning"); 5279 } else if (name.equals("readHistory")) { 5280 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.readHistory"); 5281 } else if (name.equals("updateCreate")) { 5282 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.updateCreate"); 5283 } else if (name.equals("conditionalCreate")) { 5284 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalCreate"); 5285 } else if (name.equals("conditionalRead")) { 5286 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalRead"); 5287 } else if (name.equals("conditionalUpdate")) { 5288 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalUpdate"); 5289 } else if (name.equals("conditionalDelete")) { 5290 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.conditionalDelete"); 5291 } else if (name.equals("referencePolicy")) { 5292 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.referencePolicy"); 5293 } else if (name.equals("searchInclude")) { 5294 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.searchInclude"); 5295 } else if (name.equals("searchRevInclude")) { 5296 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.searchRevInclude"); 5297 } else if (name.equals("searchParam")) { 5298 return addSearchParam(); 5299 } else if (name.equals("operation")) { 5300 return addOperation(); 5301 } else 5302 return super.addChild(name); 5303 } 5304 5305 public CapabilityStatementRestResourceComponent copy() { 5306 CapabilityStatementRestResourceComponent dst = new CapabilityStatementRestResourceComponent(); 5307 copyValues(dst); 5308 return dst; 5309 } 5310 5311 public void copyValues(CapabilityStatementRestResourceComponent dst) { 5312 super.copyValues(dst); 5313 dst.type = type == null ? null : type.copy(); 5314 dst.profile = profile == null ? null : profile.copy(); 5315 if (supportedProfile != null) { 5316 dst.supportedProfile = new ArrayList<CanonicalType>(); 5317 for (CanonicalType i : supportedProfile) 5318 dst.supportedProfile.add(i.copy()); 5319 } 5320 ; 5321 dst.documentation = documentation == null ? null : documentation.copy(); 5322 if (interaction != null) { 5323 dst.interaction = new ArrayList<ResourceInteractionComponent>(); 5324 for (ResourceInteractionComponent i : interaction) 5325 dst.interaction.add(i.copy()); 5326 } 5327 ; 5328 dst.versioning = versioning == null ? null : versioning.copy(); 5329 dst.readHistory = readHistory == null ? null : readHistory.copy(); 5330 dst.updateCreate = updateCreate == null ? null : updateCreate.copy(); 5331 dst.conditionalCreate = conditionalCreate == null ? null : conditionalCreate.copy(); 5332 dst.conditionalRead = conditionalRead == null ? null : conditionalRead.copy(); 5333 dst.conditionalUpdate = conditionalUpdate == null ? null : conditionalUpdate.copy(); 5334 dst.conditionalDelete = conditionalDelete == null ? null : conditionalDelete.copy(); 5335 if (referencePolicy != null) { 5336 dst.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 5337 for (Enumeration<ReferenceHandlingPolicy> i : referencePolicy) 5338 dst.referencePolicy.add(i.copy()); 5339 } 5340 ; 5341 if (searchInclude != null) { 5342 dst.searchInclude = new ArrayList<StringType>(); 5343 for (StringType i : searchInclude) 5344 dst.searchInclude.add(i.copy()); 5345 } 5346 ; 5347 if (searchRevInclude != null) { 5348 dst.searchRevInclude = new ArrayList<StringType>(); 5349 for (StringType i : searchRevInclude) 5350 dst.searchRevInclude.add(i.copy()); 5351 } 5352 ; 5353 if (searchParam != null) { 5354 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 5355 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 5356 dst.searchParam.add(i.copy()); 5357 } 5358 ; 5359 if (operation != null) { 5360 dst.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 5361 for (CapabilityStatementRestResourceOperationComponent i : operation) 5362 dst.operation.add(i.copy()); 5363 } 5364 ; 5365 } 5366 5367 @Override 5368 public boolean equalsDeep(Base other_) { 5369 if (!super.equalsDeep(other_)) 5370 return false; 5371 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 5372 return false; 5373 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 5374 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) 5375 && compareDeep(supportedProfile, o.supportedProfile, true) 5376 && compareDeep(documentation, o.documentation, true) && compareDeep(interaction, o.interaction, true) 5377 && compareDeep(versioning, o.versioning, true) && compareDeep(readHistory, o.readHistory, true) 5378 && compareDeep(updateCreate, o.updateCreate, true) 5379 && compareDeep(conditionalCreate, o.conditionalCreate, true) 5380 && compareDeep(conditionalRead, o.conditionalRead, true) 5381 && compareDeep(conditionalUpdate, o.conditionalUpdate, true) 5382 && compareDeep(conditionalDelete, o.conditionalDelete, true) 5383 && compareDeep(referencePolicy, o.referencePolicy, true) && compareDeep(searchInclude, o.searchInclude, true) 5384 && compareDeep(searchRevInclude, o.searchRevInclude, true) && compareDeep(searchParam, o.searchParam, true) 5385 && compareDeep(operation, o.operation, true); 5386 } 5387 5388 @Override 5389 public boolean equalsShallow(Base other_) { 5390 if (!super.equalsShallow(other_)) 5391 return false; 5392 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 5393 return false; 5394 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 5395 return compareValues(type, o.type, true) && compareValues(documentation, o.documentation, true) 5396 && compareValues(versioning, o.versioning, true) && compareValues(readHistory, o.readHistory, true) 5397 && compareValues(updateCreate, o.updateCreate, true) 5398 && compareValues(conditionalCreate, o.conditionalCreate, true) 5399 && compareValues(conditionalRead, o.conditionalRead, true) 5400 && compareValues(conditionalUpdate, o.conditionalUpdate, true) 5401 && compareValues(conditionalDelete, o.conditionalDelete, true) 5402 && compareValues(referencePolicy, o.referencePolicy, true) 5403 && compareValues(searchInclude, o.searchInclude, true) 5404 && compareValues(searchRevInclude, o.searchRevInclude, true); 5405 } 5406 5407 public boolean isEmpty() { 5408 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, supportedProfile, documentation, 5409 interaction, versioning, readHistory, updateCreate, conditionalCreate, conditionalRead, conditionalUpdate, 5410 conditionalDelete, referencePolicy, searchInclude, searchRevInclude, searchParam, operation); 5411 } 5412 5413 public String fhirType() { 5414 return "CapabilityStatement.rest.resource"; 5415 5416 } 5417 5418 } 5419 5420 @Block() 5421 public static class ResourceInteractionComponent extends BackboneElement implements IBaseBackboneElement { 5422 /** 5423 * Coded identifier of the operation, supported by the system resource. 5424 */ 5425 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5426 @Description(shortDefinition = "read | vread | update | patch | delete | history-instance | history-type | create | search-type", formalDefinition = "Coded identifier of the operation, supported by the system resource.") 5427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/type-restful-interaction") 5428 protected Enumeration<TypeRestfulInteraction> code; 5429 5430 /** 5431 * Guidance specific to the implementation of this operation, such as 'delete is 5432 * a logical delete' or 'updates are only allowed with version id' or 'creates 5433 * permitted from pre-authorized certificates only'. 5434 */ 5435 @Child(name = "documentation", type = { 5436 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5437 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.") 5438 protected MarkdownType documentation; 5439 5440 private static final long serialVersionUID = 2128937796L; 5441 5442 /** 5443 * Constructor 5444 */ 5445 public ResourceInteractionComponent() { 5446 super(); 5447 } 5448 5449 /** 5450 * Constructor 5451 */ 5452 public ResourceInteractionComponent(Enumeration<TypeRestfulInteraction> code) { 5453 super(); 5454 this.code = code; 5455 } 5456 5457 /** 5458 * @return {@link #code} (Coded identifier of the operation, supported by the 5459 * system resource.). This is the underlying object with id, value and 5460 * extensions. The accessor "getCode" gives direct access to the value 5461 */ 5462 public Enumeration<TypeRestfulInteraction> getCodeElement() { 5463 if (this.code == null) 5464 if (Configuration.errorOnAutoCreate()) 5465 throw new Error("Attempt to auto-create ResourceInteractionComponent.code"); 5466 else if (Configuration.doAutoCreate()) 5467 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); // bb 5468 return this.code; 5469 } 5470 5471 public boolean hasCodeElement() { 5472 return this.code != null && !this.code.isEmpty(); 5473 } 5474 5475 public boolean hasCode() { 5476 return this.code != null && !this.code.isEmpty(); 5477 } 5478 5479 /** 5480 * @param value {@link #code} (Coded identifier of the operation, supported by 5481 * the system resource.). This is the underlying object with id, 5482 * value and extensions. The accessor "getCode" gives direct access 5483 * to the value 5484 */ 5485 public ResourceInteractionComponent setCodeElement(Enumeration<TypeRestfulInteraction> value) { 5486 this.code = value; 5487 return this; 5488 } 5489 5490 /** 5491 * @return Coded identifier of the operation, supported by the system resource. 5492 */ 5493 public TypeRestfulInteraction getCode() { 5494 return this.code == null ? null : this.code.getValue(); 5495 } 5496 5497 /** 5498 * @param value Coded identifier of the operation, supported by the system 5499 * resource. 5500 */ 5501 public ResourceInteractionComponent setCode(TypeRestfulInteraction value) { 5502 if (this.code == null) 5503 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); 5504 this.code.setValue(value); 5505 return this; 5506 } 5507 5508 /** 5509 * @return {@link #documentation} (Guidance specific to the implementation of 5510 * this operation, such as 'delete is a logical delete' or 'updates are 5511 * only allowed with version id' or 'creates permitted from 5512 * pre-authorized certificates only'.). This is the underlying object 5513 * with id, value and extensions. The accessor "getDocumentation" gives 5514 * direct access to the value 5515 */ 5516 public MarkdownType getDocumentationElement() { 5517 if (this.documentation == null) 5518 if (Configuration.errorOnAutoCreate()) 5519 throw new Error("Attempt to auto-create ResourceInteractionComponent.documentation"); 5520 else if (Configuration.doAutoCreate()) 5521 this.documentation = new MarkdownType(); // bb 5522 return this.documentation; 5523 } 5524 5525 public boolean hasDocumentationElement() { 5526 return this.documentation != null && !this.documentation.isEmpty(); 5527 } 5528 5529 public boolean hasDocumentation() { 5530 return this.documentation != null && !this.documentation.isEmpty(); 5531 } 5532 5533 /** 5534 * @param value {@link #documentation} (Guidance specific to the implementation 5535 * of this operation, such as 'delete is a logical delete' or 5536 * 'updates are only allowed with version id' or 'creates permitted 5537 * from pre-authorized certificates only'.). This is the underlying 5538 * object with id, value and extensions. The accessor 5539 * "getDocumentation" gives direct access to the value 5540 */ 5541 public ResourceInteractionComponent setDocumentationElement(MarkdownType value) { 5542 this.documentation = value; 5543 return this; 5544 } 5545 5546 /** 5547 * @return Guidance specific to the implementation of this operation, such as 5548 * 'delete is a logical delete' or 'updates are only allowed with 5549 * version id' or 'creates permitted from pre-authorized certificates 5550 * only'. 5551 */ 5552 public String getDocumentation() { 5553 return this.documentation == null ? null : this.documentation.getValue(); 5554 } 5555 5556 /** 5557 * @param value Guidance specific to the implementation of this operation, such 5558 * as 'delete is a logical delete' or 'updates are only allowed 5559 * with version id' or 'creates permitted from pre-authorized 5560 * certificates only'. 5561 */ 5562 public ResourceInteractionComponent setDocumentation(String value) { 5563 if (value == null) 5564 this.documentation = null; 5565 else { 5566 if (this.documentation == null) 5567 this.documentation = new MarkdownType(); 5568 this.documentation.setValue(value); 5569 } 5570 return this; 5571 } 5572 5573 protected void listChildren(List<Property> children) { 5574 super.listChildren(children); 5575 children.add(new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 5576 0, 1, code)); 5577 children.add(new Property("documentation", "markdown", 5578 "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 5579 0, 1, documentation)); 5580 } 5581 5582 @Override 5583 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5584 switch (_hash) { 5585 case 3059181: 5586 /* code */ return new Property("code", "code", 5587 "Coded identifier of the operation, supported by the system resource.", 0, 1, code); 5588 case 1587405498: 5589 /* documentation */ return new Property("documentation", "markdown", 5590 "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 5591 0, 1, documentation); 5592 default: 5593 return super.getNamedProperty(_hash, _name, _checkValid); 5594 } 5595 5596 } 5597 5598 @Override 5599 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5600 switch (hash) { 5601 case 3059181: 5602 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<TypeRestfulInteraction> 5603 case 1587405498: 5604 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 5605 default: 5606 return super.getProperty(hash, name, checkValid); 5607 } 5608 5609 } 5610 5611 @Override 5612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5613 switch (hash) { 5614 case 3059181: // code 5615 value = new TypeRestfulInteractionEnumFactory().fromType(castToCode(value)); 5616 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 5617 return value; 5618 case 1587405498: // documentation 5619 this.documentation = castToMarkdown(value); // MarkdownType 5620 return value; 5621 default: 5622 return super.setProperty(hash, name, value); 5623 } 5624 5625 } 5626 5627 @Override 5628 public Base setProperty(String name, Base value) throws FHIRException { 5629 if (name.equals("code")) { 5630 value = new TypeRestfulInteractionEnumFactory().fromType(castToCode(value)); 5631 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 5632 } else if (name.equals("documentation")) { 5633 this.documentation = castToMarkdown(value); // MarkdownType 5634 } else 5635 return super.setProperty(name, value); 5636 return value; 5637 } 5638 5639 @Override 5640 public void removeChild(String name, Base value) throws FHIRException { 5641 if (name.equals("code")) { 5642 this.code = null; 5643 } else if (name.equals("documentation")) { 5644 this.documentation = null; 5645 } else 5646 super.removeChild(name, value); 5647 5648 } 5649 5650 @Override 5651 public Base makeProperty(int hash, String name) throws FHIRException { 5652 switch (hash) { 5653 case 3059181: 5654 return getCodeElement(); 5655 case 1587405498: 5656 return getDocumentationElement(); 5657 default: 5658 return super.makeProperty(hash, name); 5659 } 5660 5661 } 5662 5663 @Override 5664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5665 switch (hash) { 5666 case 3059181: 5667 /* code */ return new String[] { "code" }; 5668 case 1587405498: 5669 /* documentation */ return new String[] { "markdown" }; 5670 default: 5671 return super.getTypesForProperty(hash, name); 5672 } 5673 5674 } 5675 5676 @Override 5677 public Base addChild(String name) throws FHIRException { 5678 if (name.equals("code")) { 5679 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.code"); 5680 } else if (name.equals("documentation")) { 5681 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 5682 } else 5683 return super.addChild(name); 5684 } 5685 5686 public ResourceInteractionComponent copy() { 5687 ResourceInteractionComponent dst = new ResourceInteractionComponent(); 5688 copyValues(dst); 5689 return dst; 5690 } 5691 5692 public void copyValues(ResourceInteractionComponent dst) { 5693 super.copyValues(dst); 5694 dst.code = code == null ? null : code.copy(); 5695 dst.documentation = documentation == null ? null : documentation.copy(); 5696 } 5697 5698 @Override 5699 public boolean equalsDeep(Base other_) { 5700 if (!super.equalsDeep(other_)) 5701 return false; 5702 if (!(other_ instanceof ResourceInteractionComponent)) 5703 return false; 5704 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 5705 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5706 } 5707 5708 @Override 5709 public boolean equalsShallow(Base other_) { 5710 if (!super.equalsShallow(other_)) 5711 return false; 5712 if (!(other_ instanceof ResourceInteractionComponent)) 5713 return false; 5714 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 5715 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5716 } 5717 5718 public boolean isEmpty() { 5719 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 5720 } 5721 5722 public String fhirType() { 5723 return "CapabilityStatement.rest.resource.interaction"; 5724 5725 } 5726 5727 } 5728 5729 @Block() 5730 public static class CapabilityStatementRestResourceSearchParamComponent extends BackboneElement 5731 implements IBaseBackboneElement { 5732 /** 5733 * The name of the search parameter used in the interface. 5734 */ 5735 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5736 @Description(shortDefinition = "Name of search parameter", formalDefinition = "The name of the search parameter used in the interface.") 5737 protected StringType name; 5738 5739 /** 5740 * An absolute URI that is a formal reference to where this parameter was first 5741 * defined, so that a client can be confident of the meaning of the search 5742 * parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be 5743 * populated if the search parameter refers to a SearchParameter defined by the 5744 * FHIR core specification or externally defined IGs. 5745 */ 5746 @Child(name = "definition", type = { 5747 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5748 @Description(shortDefinition = "Source of definition for parameter", formalDefinition = "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.") 5749 protected CanonicalType definition; 5750 5751 /** 5752 * The type of value a search parameter refers to, and how the content is 5753 * interpreted. 5754 */ 5755 @Child(name = "type", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 5756 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri | special", formalDefinition = "The type of value a search parameter refers to, and how the content is interpreted.") 5757 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-param-type") 5758 protected Enumeration<SearchParamType> type; 5759 5760 /** 5761 * This allows documentation of any distinct behaviors about how the search 5762 * parameter is used. For example, text matching algorithms. 5763 */ 5764 @Child(name = "documentation", type = { 5765 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5766 @Description(shortDefinition = "Server-specific usage", formalDefinition = "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.") 5767 protected MarkdownType documentation; 5768 5769 private static final long serialVersionUID = -171123928L; 5770 5771 /** 5772 * Constructor 5773 */ 5774 public CapabilityStatementRestResourceSearchParamComponent() { 5775 super(); 5776 } 5777 5778 /** 5779 * Constructor 5780 */ 5781 public CapabilityStatementRestResourceSearchParamComponent(StringType name, Enumeration<SearchParamType> type) { 5782 super(); 5783 this.name = name; 5784 this.type = type; 5785 } 5786 5787 /** 5788 * @return {@link #name} (The name of the search parameter used in the 5789 * interface.). This is the underlying object with id, value and 5790 * extensions. The accessor "getName" gives direct access to the value 5791 */ 5792 public StringType getNameElement() { 5793 if (this.name == null) 5794 if (Configuration.errorOnAutoCreate()) 5795 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.name"); 5796 else if (Configuration.doAutoCreate()) 5797 this.name = new StringType(); // bb 5798 return this.name; 5799 } 5800 5801 public boolean hasNameElement() { 5802 return this.name != null && !this.name.isEmpty(); 5803 } 5804 5805 public boolean hasName() { 5806 return this.name != null && !this.name.isEmpty(); 5807 } 5808 5809 /** 5810 * @param value {@link #name} (The name of the search parameter used in the 5811 * interface.). This is the underlying object with id, value and 5812 * extensions. The accessor "getName" gives direct access to the 5813 * value 5814 */ 5815 public CapabilityStatementRestResourceSearchParamComponent setNameElement(StringType value) { 5816 this.name = value; 5817 return this; 5818 } 5819 5820 /** 5821 * @return The name of the search parameter used in the interface. 5822 */ 5823 public String getName() { 5824 return this.name == null ? null : this.name.getValue(); 5825 } 5826 5827 /** 5828 * @param value The name of the search parameter used in the interface. 5829 */ 5830 public CapabilityStatementRestResourceSearchParamComponent setName(String value) { 5831 if (this.name == null) 5832 this.name = new StringType(); 5833 this.name.setValue(value); 5834 return this; 5835 } 5836 5837 /** 5838 * @return {@link #definition} (An absolute URI that is a formal reference to 5839 * where this parameter was first defined, so that a client can be 5840 * confident of the meaning of the search parameter (a reference to 5841 * [[[SearchParameter.url]]]). This element SHALL be populated if the 5842 * search parameter refers to a SearchParameter defined by the FHIR core 5843 * specification or externally defined IGs.). This is the underlying 5844 * object with id, value and extensions. The accessor "getDefinition" 5845 * gives direct access to the value 5846 */ 5847 public CanonicalType getDefinitionElement() { 5848 if (this.definition == null) 5849 if (Configuration.errorOnAutoCreate()) 5850 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.definition"); 5851 else if (Configuration.doAutoCreate()) 5852 this.definition = new CanonicalType(); // bb 5853 return this.definition; 5854 } 5855 5856 public boolean hasDefinitionElement() { 5857 return this.definition != null && !this.definition.isEmpty(); 5858 } 5859 5860 public boolean hasDefinition() { 5861 return this.definition != null && !this.definition.isEmpty(); 5862 } 5863 5864 /** 5865 * @param value {@link #definition} (An absolute URI that is a formal reference 5866 * to where this parameter was first defined, so that a client can 5867 * be confident of the meaning of the search parameter (a reference 5868 * to [[[SearchParameter.url]]]). This element SHALL be populated 5869 * if the search parameter refers to a SearchParameter defined by 5870 * the FHIR core specification or externally defined IGs.). This is 5871 * the underlying object with id, value and extensions. The 5872 * accessor "getDefinition" gives direct access to the value 5873 */ 5874 public CapabilityStatementRestResourceSearchParamComponent setDefinitionElement(CanonicalType value) { 5875 this.definition = value; 5876 return this; 5877 } 5878 5879 /** 5880 * @return An absolute URI that is a formal reference to where this parameter 5881 * was first defined, so that a client can be confident of the meaning 5882 * of the search parameter (a reference to [[[SearchParameter.url]]]). 5883 * This element SHALL be populated if the search parameter refers to a 5884 * SearchParameter defined by the FHIR core specification or externally 5885 * defined IGs. 5886 */ 5887 public String getDefinition() { 5888 return this.definition == null ? null : this.definition.getValue(); 5889 } 5890 5891 /** 5892 * @param value An absolute URI that is a formal reference to where this 5893 * parameter was first defined, so that a client can be confident 5894 * of the meaning of the search parameter (a reference to 5895 * [[[SearchParameter.url]]]). This element SHALL be populated if 5896 * the search parameter refers to a SearchParameter defined by the 5897 * FHIR core specification or externally defined IGs. 5898 */ 5899 public CapabilityStatementRestResourceSearchParamComponent setDefinition(String value) { 5900 if (Utilities.noString(value)) 5901 this.definition = null; 5902 else { 5903 if (this.definition == null) 5904 this.definition = new CanonicalType(); 5905 this.definition.setValue(value); 5906 } 5907 return this; 5908 } 5909 5910 /** 5911 * @return {@link #type} (The type of value a search parameter refers to, and 5912 * how the content is interpreted.). This is the underlying object with 5913 * id, value and extensions. The accessor "getType" gives direct access 5914 * to the value 5915 */ 5916 public Enumeration<SearchParamType> getTypeElement() { 5917 if (this.type == null) 5918 if (Configuration.errorOnAutoCreate()) 5919 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.type"); 5920 else if (Configuration.doAutoCreate()) 5921 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 5922 return this.type; 5923 } 5924 5925 public boolean hasTypeElement() { 5926 return this.type != null && !this.type.isEmpty(); 5927 } 5928 5929 public boolean hasType() { 5930 return this.type != null && !this.type.isEmpty(); 5931 } 5932 5933 /** 5934 * @param value {@link #type} (The type of value a search parameter refers to, 5935 * and how the content is interpreted.). This is the underlying 5936 * object with id, value and extensions. The accessor "getType" 5937 * gives direct access to the value 5938 */ 5939 public CapabilityStatementRestResourceSearchParamComponent setTypeElement(Enumeration<SearchParamType> value) { 5940 this.type = value; 5941 return this; 5942 } 5943 5944 /** 5945 * @return The type of value a search parameter refers to, and how the content 5946 * is interpreted. 5947 */ 5948 public SearchParamType getType() { 5949 return this.type == null ? null : this.type.getValue(); 5950 } 5951 5952 /** 5953 * @param value The type of value a search parameter refers to, and how the 5954 * content is interpreted. 5955 */ 5956 public CapabilityStatementRestResourceSearchParamComponent setType(SearchParamType value) { 5957 if (this.type == null) 5958 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 5959 this.type.setValue(value); 5960 return this; 5961 } 5962 5963 /** 5964 * @return {@link #documentation} (This allows documentation of any distinct 5965 * behaviors about how the search parameter is used. For example, text 5966 * matching algorithms.). This is the underlying object with id, value 5967 * and extensions. The accessor "getDocumentation" gives direct access 5968 * to the value 5969 */ 5970 public MarkdownType getDocumentationElement() { 5971 if (this.documentation == null) 5972 if (Configuration.errorOnAutoCreate()) 5973 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.documentation"); 5974 else if (Configuration.doAutoCreate()) 5975 this.documentation = new MarkdownType(); // bb 5976 return this.documentation; 5977 } 5978 5979 public boolean hasDocumentationElement() { 5980 return this.documentation != null && !this.documentation.isEmpty(); 5981 } 5982 5983 public boolean hasDocumentation() { 5984 return this.documentation != null && !this.documentation.isEmpty(); 5985 } 5986 5987 /** 5988 * @param value {@link #documentation} (This allows documentation of any 5989 * distinct behaviors about how the search parameter is used. For 5990 * example, text matching algorithms.). This is the underlying 5991 * object with id, value and extensions. The accessor 5992 * "getDocumentation" gives direct access to the value 5993 */ 5994 public CapabilityStatementRestResourceSearchParamComponent setDocumentationElement(MarkdownType value) { 5995 this.documentation = value; 5996 return this; 5997 } 5998 5999 /** 6000 * @return This allows documentation of any distinct behaviors about how the 6001 * search parameter is used. For example, text matching algorithms. 6002 */ 6003 public String getDocumentation() { 6004 return this.documentation == null ? null : this.documentation.getValue(); 6005 } 6006 6007 /** 6008 * @param value This allows documentation of any distinct behaviors about how 6009 * the search parameter is used. For example, text matching 6010 * algorithms. 6011 */ 6012 public CapabilityStatementRestResourceSearchParamComponent setDocumentation(String value) { 6013 if (value == null) 6014 this.documentation = null; 6015 else { 6016 if (this.documentation == null) 6017 this.documentation = new MarkdownType(); 6018 this.documentation.setValue(value); 6019 } 6020 return this; 6021 } 6022 6023 protected void listChildren(List<Property> children) { 6024 super.listChildren(children); 6025 children 6026 .add(new Property("name", "string", "The name of the search parameter used in the interface.", 0, 1, name)); 6027 children.add(new Property("definition", "canonical(SearchParameter)", 6028 "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.", 6029 0, 1, definition)); 6030 children.add(new Property("type", "code", 6031 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type)); 6032 children.add(new Property("documentation", "markdown", 6033 "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 6034 0, 1, documentation)); 6035 } 6036 6037 @Override 6038 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6039 switch (_hash) { 6040 case 3373707: 6041 /* name */ return new Property("name", "string", "The name of the search parameter used in the interface.", 0, 6042 1, name); 6043 case -1014418093: 6044 /* definition */ return new Property("definition", "canonical(SearchParameter)", 6045 "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.", 6046 0, 1, definition); 6047 case 3575610: 6048 /* type */ return new Property("type", "code", 6049 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type); 6050 case 1587405498: 6051 /* documentation */ return new Property("documentation", "markdown", 6052 "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 6053 0, 1, documentation); 6054 default: 6055 return super.getNamedProperty(_hash, _name, _checkValid); 6056 } 6057 6058 } 6059 6060 @Override 6061 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6062 switch (hash) { 6063 case 3373707: 6064 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 6065 case -1014418093: 6066 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 6067 case 3575610: 6068 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SearchParamType> 6069 case 1587405498: 6070 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 6071 default: 6072 return super.getProperty(hash, name, checkValid); 6073 } 6074 6075 } 6076 6077 @Override 6078 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6079 switch (hash) { 6080 case 3373707: // name 6081 this.name = castToString(value); // StringType 6082 return value; 6083 case -1014418093: // definition 6084 this.definition = castToCanonical(value); // CanonicalType 6085 return value; 6086 case 3575610: // type 6087 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 6088 this.type = (Enumeration) value; // Enumeration<SearchParamType> 6089 return value; 6090 case 1587405498: // documentation 6091 this.documentation = castToMarkdown(value); // MarkdownType 6092 return value; 6093 default: 6094 return super.setProperty(hash, name, value); 6095 } 6096 6097 } 6098 6099 @Override 6100 public Base setProperty(String name, Base value) throws FHIRException { 6101 if (name.equals("name")) { 6102 this.name = castToString(value); // StringType 6103 } else if (name.equals("definition")) { 6104 this.definition = castToCanonical(value); // CanonicalType 6105 } else if (name.equals("type")) { 6106 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 6107 this.type = (Enumeration) value; // Enumeration<SearchParamType> 6108 } else if (name.equals("documentation")) { 6109 this.documentation = castToMarkdown(value); // MarkdownType 6110 } else 6111 return super.setProperty(name, value); 6112 return value; 6113 } 6114 6115 @Override 6116 public void removeChild(String name, Base value) throws FHIRException { 6117 if (name.equals("name")) { 6118 this.name = null; 6119 } else if (name.equals("definition")) { 6120 this.definition = null; 6121 } else if (name.equals("type")) { 6122 this.type = null; 6123 } else if (name.equals("documentation")) { 6124 this.documentation = null; 6125 } else 6126 super.removeChild(name, value); 6127 6128 } 6129 6130 @Override 6131 public Base makeProperty(int hash, String name) throws FHIRException { 6132 switch (hash) { 6133 case 3373707: 6134 return getNameElement(); 6135 case -1014418093: 6136 return getDefinitionElement(); 6137 case 3575610: 6138 return getTypeElement(); 6139 case 1587405498: 6140 return getDocumentationElement(); 6141 default: 6142 return super.makeProperty(hash, name); 6143 } 6144 6145 } 6146 6147 @Override 6148 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6149 switch (hash) { 6150 case 3373707: 6151 /* name */ return new String[] { "string" }; 6152 case -1014418093: 6153 /* definition */ return new String[] { "canonical" }; 6154 case 3575610: 6155 /* type */ return new String[] { "code" }; 6156 case 1587405498: 6157 /* documentation */ return new String[] { "markdown" }; 6158 default: 6159 return super.getTypesForProperty(hash, name); 6160 } 6161 6162 } 6163 6164 @Override 6165 public Base addChild(String name) throws FHIRException { 6166 if (name.equals("name")) { 6167 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 6168 } else if (name.equals("definition")) { 6169 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 6170 } else if (name.equals("type")) { 6171 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.type"); 6172 } else if (name.equals("documentation")) { 6173 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6174 } else 6175 return super.addChild(name); 6176 } 6177 6178 public CapabilityStatementRestResourceSearchParamComponent copy() { 6179 CapabilityStatementRestResourceSearchParamComponent dst = new CapabilityStatementRestResourceSearchParamComponent(); 6180 copyValues(dst); 6181 return dst; 6182 } 6183 6184 public void copyValues(CapabilityStatementRestResourceSearchParamComponent dst) { 6185 super.copyValues(dst); 6186 dst.name = name == null ? null : name.copy(); 6187 dst.definition = definition == null ? null : definition.copy(); 6188 dst.type = type == null ? null : type.copy(); 6189 dst.documentation = documentation == null ? null : documentation.copy(); 6190 } 6191 6192 @Override 6193 public boolean equalsDeep(Base other_) { 6194 if (!super.equalsDeep(other_)) 6195 return false; 6196 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 6197 return false; 6198 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 6199 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) 6200 && compareDeep(type, o.type, true) && compareDeep(documentation, o.documentation, true); 6201 } 6202 6203 @Override 6204 public boolean equalsShallow(Base other_) { 6205 if (!super.equalsShallow(other_)) 6206 return false; 6207 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 6208 return false; 6209 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 6210 return compareValues(name, o.name, true) && compareValues(type, o.type, true) 6211 && compareValues(documentation, o.documentation, true); 6212 } 6213 6214 public boolean isEmpty() { 6215 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, type, documentation); 6216 } 6217 6218 public String fhirType() { 6219 return "CapabilityStatement.rest.resource.searchParam"; 6220 6221 } 6222 6223 } 6224 6225 @Block() 6226 public static class CapabilityStatementRestResourceOperationComponent extends BackboneElement 6227 implements IBaseBackboneElement { 6228 /** 6229 * The name of the operation or query. For an operation, this is the name 6230 * prefixed with $ and used in the URL. For a query, this is the name used in 6231 * the _query parameter when the query is called. 6232 */ 6233 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 6234 @Description(shortDefinition = "Name by which the operation/query is invoked", formalDefinition = "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.") 6235 protected StringType name; 6236 6237 /** 6238 * Where the formal definition can be found. If a server references the base 6239 * definition of an Operation (i.e. from the specification itself such as 6240 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it 6241 * supports the full capabilities of the operation - e.g. both GET and POST 6242 * invocation. If it only supports a subset, it must define its own custom 6243 * [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. 6244 * The custom definition would describe the specific subset of functionality 6245 * supported. 6246 */ 6247 @Child(name = "definition", type = { 6248 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 6249 @Description(shortDefinition = "The defined operation/query", formalDefinition = "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.") 6250 protected CanonicalType definition; 6251 6252 /** 6253 * Documentation that describes anything special about the operation behavior, 6254 * possibly detailing different behavior for system, type and instance-level 6255 * invocation of the operation. 6256 */ 6257 @Child(name = "documentation", type = { 6258 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6259 @Description(shortDefinition = "Specific details about operation behavior", formalDefinition = "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.") 6260 protected MarkdownType documentation; 6261 6262 private static final long serialVersionUID = -388608084L; 6263 6264 /** 6265 * Constructor 6266 */ 6267 public CapabilityStatementRestResourceOperationComponent() { 6268 super(); 6269 } 6270 6271 /** 6272 * Constructor 6273 */ 6274 public CapabilityStatementRestResourceOperationComponent(StringType name, CanonicalType definition) { 6275 super(); 6276 this.name = name; 6277 this.definition = definition; 6278 } 6279 6280 /** 6281 * @return {@link #name} (The name of the operation or query. For an operation, 6282 * this is the name prefixed with $ and used in the URL. For a query, 6283 * this is the name used in the _query parameter when the query is 6284 * called.). This is the underlying object with id, value and 6285 * extensions. The accessor "getName" gives direct access to the value 6286 */ 6287 public StringType getNameElement() { 6288 if (this.name == null) 6289 if (Configuration.errorOnAutoCreate()) 6290 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.name"); 6291 else if (Configuration.doAutoCreate()) 6292 this.name = new StringType(); // bb 6293 return this.name; 6294 } 6295 6296 public boolean hasNameElement() { 6297 return this.name != null && !this.name.isEmpty(); 6298 } 6299 6300 public boolean hasName() { 6301 return this.name != null && !this.name.isEmpty(); 6302 } 6303 6304 /** 6305 * @param value {@link #name} (The name of the operation or query. For an 6306 * operation, this is the name prefixed with $ and used in the URL. 6307 * For a query, this is the name used in the _query parameter when 6308 * the query is called.). This is the underlying object with id, 6309 * value and extensions. The accessor "getName" gives direct access 6310 * to the value 6311 */ 6312 public CapabilityStatementRestResourceOperationComponent setNameElement(StringType value) { 6313 this.name = value; 6314 return this; 6315 } 6316 6317 /** 6318 * @return The name of the operation or query. For an operation, this is the 6319 * name prefixed with $ and used in the URL. For a query, this is the 6320 * name used in the _query parameter when the query is called. 6321 */ 6322 public String getName() { 6323 return this.name == null ? null : this.name.getValue(); 6324 } 6325 6326 /** 6327 * @param value The name of the operation or query. For an operation, this is 6328 * the name prefixed with $ and used in the URL. For a query, this 6329 * is the name used in the _query parameter when the query is 6330 * called. 6331 */ 6332 public CapabilityStatementRestResourceOperationComponent setName(String value) { 6333 if (this.name == null) 6334 this.name = new StringType(); 6335 this.name.setValue(value); 6336 return this; 6337 } 6338 6339 /** 6340 * @return {@link #definition} (Where the formal definition can be found. If a 6341 * server references the base definition of an Operation (i.e. from the 6342 * specification itself such as 6343 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that 6344 * means it supports the full capabilities of the operation - e.g. both 6345 * GET and POST invocation. If it only supports a subset, it must define 6346 * its own custom [[[OperationDefinition]]] with a 'base' of the 6347 * original OperationDefinition. The custom definition would describe 6348 * the specific subset of functionality supported.). This is the 6349 * underlying object with id, value and extensions. The accessor 6350 * "getDefinition" gives direct access to the value 6351 */ 6352 public CanonicalType getDefinitionElement() { 6353 if (this.definition == null) 6354 if (Configuration.errorOnAutoCreate()) 6355 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.definition"); 6356 else if (Configuration.doAutoCreate()) 6357 this.definition = new CanonicalType(); // bb 6358 return this.definition; 6359 } 6360 6361 public boolean hasDefinitionElement() { 6362 return this.definition != null && !this.definition.isEmpty(); 6363 } 6364 6365 public boolean hasDefinition() { 6366 return this.definition != null && !this.definition.isEmpty(); 6367 } 6368 6369 /** 6370 * @param value {@link #definition} (Where the formal definition can be found. 6371 * If a server references the base definition of an Operation (i.e. 6372 * from the specification itself such as 6373 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), 6374 * that means it supports the full capabilities of the operation - 6375 * e.g. both GET and POST invocation. If it only supports a subset, 6376 * it must define its own custom [[[OperationDefinition]]] with a 6377 * 'base' of the original OperationDefinition. The custom 6378 * definition would describe the specific subset of functionality 6379 * supported.). This is the underlying object with id, value and 6380 * extensions. The accessor "getDefinition" gives direct access to 6381 * the value 6382 */ 6383 public CapabilityStatementRestResourceOperationComponent setDefinitionElement(CanonicalType value) { 6384 this.definition = value; 6385 return this; 6386 } 6387 6388 /** 6389 * @return Where the formal definition can be found. If a server references the 6390 * base definition of an Operation (i.e. from the specification itself 6391 * such as 6392 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that 6393 * means it supports the full capabilities of the operation - e.g. both 6394 * GET and POST invocation. If it only supports a subset, it must define 6395 * its own custom [[[OperationDefinition]]] with a 'base' of the 6396 * original OperationDefinition. The custom definition would describe 6397 * the specific subset of functionality supported. 6398 */ 6399 public String getDefinition() { 6400 return this.definition == null ? null : this.definition.getValue(); 6401 } 6402 6403 /** 6404 * @param value Where the formal definition can be found. If a server references 6405 * the base definition of an Operation (i.e. from the specification 6406 * itself such as 6407 * ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), 6408 * that means it supports the full capabilities of the operation - 6409 * e.g. both GET and POST invocation. If it only supports a subset, 6410 * it must define its own custom [[[OperationDefinition]]] with a 6411 * 'base' of the original OperationDefinition. The custom 6412 * definition would describe the specific subset of functionality 6413 * supported. 6414 */ 6415 public CapabilityStatementRestResourceOperationComponent setDefinition(String value) { 6416 if (this.definition == null) 6417 this.definition = new CanonicalType(); 6418 this.definition.setValue(value); 6419 return this; 6420 } 6421 6422 /** 6423 * @return {@link #documentation} (Documentation that describes anything special 6424 * about the operation behavior, possibly detailing different behavior 6425 * for system, type and instance-level invocation of the operation.). 6426 * This is the underlying object with id, value and extensions. The 6427 * accessor "getDocumentation" gives direct access to the value 6428 */ 6429 public MarkdownType getDocumentationElement() { 6430 if (this.documentation == null) 6431 if (Configuration.errorOnAutoCreate()) 6432 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.documentation"); 6433 else if (Configuration.doAutoCreate()) 6434 this.documentation = new MarkdownType(); // bb 6435 return this.documentation; 6436 } 6437 6438 public boolean hasDocumentationElement() { 6439 return this.documentation != null && !this.documentation.isEmpty(); 6440 } 6441 6442 public boolean hasDocumentation() { 6443 return this.documentation != null && !this.documentation.isEmpty(); 6444 } 6445 6446 /** 6447 * @param value {@link #documentation} (Documentation that describes anything 6448 * special about the operation behavior, possibly detailing 6449 * different behavior for system, type and instance-level 6450 * invocation of the operation.). This is the underlying object 6451 * with id, value and extensions. The accessor "getDocumentation" 6452 * gives direct access to the value 6453 */ 6454 public CapabilityStatementRestResourceOperationComponent setDocumentationElement(MarkdownType value) { 6455 this.documentation = value; 6456 return this; 6457 } 6458 6459 /** 6460 * @return Documentation that describes anything special about the operation 6461 * behavior, possibly detailing different behavior for system, type and 6462 * instance-level invocation of the operation. 6463 */ 6464 public String getDocumentation() { 6465 return this.documentation == null ? null : this.documentation.getValue(); 6466 } 6467 6468 /** 6469 * @param value Documentation that describes anything special about the 6470 * operation behavior, possibly detailing different behavior for 6471 * system, type and instance-level invocation of the operation. 6472 */ 6473 public CapabilityStatementRestResourceOperationComponent setDocumentation(String value) { 6474 if (value == null) 6475 this.documentation = null; 6476 else { 6477 if (this.documentation == null) 6478 this.documentation = new MarkdownType(); 6479 this.documentation.setValue(value); 6480 } 6481 return this; 6482 } 6483 6484 protected void listChildren(List<Property> children) { 6485 super.listChildren(children); 6486 children.add(new Property("name", "string", 6487 "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.", 6488 0, 1, name)); 6489 children.add(new Property("definition", "canonical(OperationDefinition)", 6490 "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.", 6491 0, 1, definition)); 6492 children.add(new Property("documentation", "markdown", 6493 "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.", 6494 0, 1, documentation)); 6495 } 6496 6497 @Override 6498 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6499 switch (_hash) { 6500 case 3373707: 6501 /* name */ return new Property("name", "string", 6502 "The name of the operation or query. For an operation, this is the name prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called.", 6503 0, 1, name); 6504 case -1014418093: 6505 /* definition */ return new Property("definition", "canonical(OperationDefinition)", 6506 "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [[[OperationDefinition]]] with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.", 6507 0, 1, definition); 6508 case 1587405498: 6509 /* documentation */ return new Property("documentation", "markdown", 6510 "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.", 6511 0, 1, documentation); 6512 default: 6513 return super.getNamedProperty(_hash, _name, _checkValid); 6514 } 6515 6516 } 6517 6518 @Override 6519 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6520 switch (hash) { 6521 case 3373707: 6522 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 6523 case -1014418093: 6524 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 6525 case 1587405498: 6526 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 6527 default: 6528 return super.getProperty(hash, name, checkValid); 6529 } 6530 6531 } 6532 6533 @Override 6534 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6535 switch (hash) { 6536 case 3373707: // name 6537 this.name = castToString(value); // StringType 6538 return value; 6539 case -1014418093: // definition 6540 this.definition = castToCanonical(value); // CanonicalType 6541 return value; 6542 case 1587405498: // documentation 6543 this.documentation = castToMarkdown(value); // MarkdownType 6544 return value; 6545 default: 6546 return super.setProperty(hash, name, value); 6547 } 6548 6549 } 6550 6551 @Override 6552 public Base setProperty(String name, Base value) throws FHIRException { 6553 if (name.equals("name")) { 6554 this.name = castToString(value); // StringType 6555 } else if (name.equals("definition")) { 6556 this.definition = castToCanonical(value); // CanonicalType 6557 } else if (name.equals("documentation")) { 6558 this.documentation = castToMarkdown(value); // MarkdownType 6559 } else 6560 return super.setProperty(name, value); 6561 return value; 6562 } 6563 6564 @Override 6565 public void removeChild(String name, Base value) throws FHIRException { 6566 if (name.equals("name")) { 6567 this.name = null; 6568 } else if (name.equals("definition")) { 6569 this.definition = null; 6570 } else if (name.equals("documentation")) { 6571 this.documentation = null; 6572 } else 6573 super.removeChild(name, value); 6574 6575 } 6576 6577 @Override 6578 public Base makeProperty(int hash, String name) throws FHIRException { 6579 switch (hash) { 6580 case 3373707: 6581 return getNameElement(); 6582 case -1014418093: 6583 return getDefinitionElement(); 6584 case 1587405498: 6585 return getDocumentationElement(); 6586 default: 6587 return super.makeProperty(hash, name); 6588 } 6589 6590 } 6591 6592 @Override 6593 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6594 switch (hash) { 6595 case 3373707: 6596 /* name */ return new String[] { "string" }; 6597 case -1014418093: 6598 /* definition */ return new String[] { "canonical" }; 6599 case 1587405498: 6600 /* documentation */ return new String[] { "markdown" }; 6601 default: 6602 return super.getTypesForProperty(hash, name); 6603 } 6604 6605 } 6606 6607 @Override 6608 public Base addChild(String name) throws FHIRException { 6609 if (name.equals("name")) { 6610 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 6611 } else if (name.equals("definition")) { 6612 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 6613 } else if (name.equals("documentation")) { 6614 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6615 } else 6616 return super.addChild(name); 6617 } 6618 6619 public CapabilityStatementRestResourceOperationComponent copy() { 6620 CapabilityStatementRestResourceOperationComponent dst = new CapabilityStatementRestResourceOperationComponent(); 6621 copyValues(dst); 6622 return dst; 6623 } 6624 6625 public void copyValues(CapabilityStatementRestResourceOperationComponent dst) { 6626 super.copyValues(dst); 6627 dst.name = name == null ? null : name.copy(); 6628 dst.definition = definition == null ? null : definition.copy(); 6629 dst.documentation = documentation == null ? null : documentation.copy(); 6630 } 6631 6632 @Override 6633 public boolean equalsDeep(Base other_) { 6634 if (!super.equalsDeep(other_)) 6635 return false; 6636 if (!(other_ instanceof CapabilityStatementRestResourceOperationComponent)) 6637 return false; 6638 CapabilityStatementRestResourceOperationComponent o = (CapabilityStatementRestResourceOperationComponent) other_; 6639 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) 6640 && compareDeep(documentation, o.documentation, true); 6641 } 6642 6643 @Override 6644 public boolean equalsShallow(Base other_) { 6645 if (!super.equalsShallow(other_)) 6646 return false; 6647 if (!(other_ instanceof CapabilityStatementRestResourceOperationComponent)) 6648 return false; 6649 CapabilityStatementRestResourceOperationComponent o = (CapabilityStatementRestResourceOperationComponent) other_; 6650 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 6651 } 6652 6653 public boolean isEmpty() { 6654 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, documentation); 6655 } 6656 6657 public String fhirType() { 6658 return "CapabilityStatement.rest.resource.operation"; 6659 6660 } 6661 6662 } 6663 6664 @Block() 6665 public static class SystemInteractionComponent extends BackboneElement implements IBaseBackboneElement { 6666 /** 6667 * A coded identifier of the operation, supported by the system. 6668 */ 6669 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6670 @Description(shortDefinition = "transaction | batch | search-system | history-system", formalDefinition = "A coded identifier of the operation, supported by the system.") 6671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/system-restful-interaction") 6672 protected Enumeration<SystemRestfulInteraction> code; 6673 6674 /** 6675 * Guidance specific to the implementation of this operation, such as 6676 * limitations on the kind of transactions allowed, or information about system 6677 * wide search is implemented. 6678 */ 6679 @Child(name = "documentation", type = { 6680 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6681 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.") 6682 protected MarkdownType documentation; 6683 6684 private static final long serialVersionUID = -1495143879L; 6685 6686 /** 6687 * Constructor 6688 */ 6689 public SystemInteractionComponent() { 6690 super(); 6691 } 6692 6693 /** 6694 * Constructor 6695 */ 6696 public SystemInteractionComponent(Enumeration<SystemRestfulInteraction> code) { 6697 super(); 6698 this.code = code; 6699 } 6700 6701 /** 6702 * @return {@link #code} (A coded identifier of the operation, supported by the 6703 * system.). This is the underlying object with id, value and 6704 * extensions. The accessor "getCode" gives direct access to the value 6705 */ 6706 public Enumeration<SystemRestfulInteraction> getCodeElement() { 6707 if (this.code == null) 6708 if (Configuration.errorOnAutoCreate()) 6709 throw new Error("Attempt to auto-create SystemInteractionComponent.code"); 6710 else if (Configuration.doAutoCreate()) 6711 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); // bb 6712 return this.code; 6713 } 6714 6715 public boolean hasCodeElement() { 6716 return this.code != null && !this.code.isEmpty(); 6717 } 6718 6719 public boolean hasCode() { 6720 return this.code != null && !this.code.isEmpty(); 6721 } 6722 6723 /** 6724 * @param value {@link #code} (A coded identifier of the operation, supported by 6725 * the system.). This is the underlying object with id, value and 6726 * extensions. The accessor "getCode" gives direct access to the 6727 * value 6728 */ 6729 public SystemInteractionComponent setCodeElement(Enumeration<SystemRestfulInteraction> value) { 6730 this.code = value; 6731 return this; 6732 } 6733 6734 /** 6735 * @return A coded identifier of the operation, supported by the system. 6736 */ 6737 public SystemRestfulInteraction getCode() { 6738 return this.code == null ? null : this.code.getValue(); 6739 } 6740 6741 /** 6742 * @param value A coded identifier of the operation, supported by the system. 6743 */ 6744 public SystemInteractionComponent setCode(SystemRestfulInteraction value) { 6745 if (this.code == null) 6746 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); 6747 this.code.setValue(value); 6748 return this; 6749 } 6750 6751 /** 6752 * @return {@link #documentation} (Guidance specific to the implementation of 6753 * this operation, such as limitations on the kind of transactions 6754 * allowed, or information about system wide search is implemented.). 6755 * This is the underlying object with id, value and extensions. The 6756 * accessor "getDocumentation" gives direct access to the value 6757 */ 6758 public MarkdownType getDocumentationElement() { 6759 if (this.documentation == null) 6760 if (Configuration.errorOnAutoCreate()) 6761 throw new Error("Attempt to auto-create SystemInteractionComponent.documentation"); 6762 else if (Configuration.doAutoCreate()) 6763 this.documentation = new MarkdownType(); // bb 6764 return this.documentation; 6765 } 6766 6767 public boolean hasDocumentationElement() { 6768 return this.documentation != null && !this.documentation.isEmpty(); 6769 } 6770 6771 public boolean hasDocumentation() { 6772 return this.documentation != null && !this.documentation.isEmpty(); 6773 } 6774 6775 /** 6776 * @param value {@link #documentation} (Guidance specific to the implementation 6777 * of this operation, such as limitations on the kind of 6778 * transactions allowed, or information about system wide search is 6779 * implemented.). This is the underlying object with id, value and 6780 * extensions. The accessor "getDocumentation" gives direct access 6781 * to the value 6782 */ 6783 public SystemInteractionComponent setDocumentationElement(MarkdownType value) { 6784 this.documentation = value; 6785 return this; 6786 } 6787 6788 /** 6789 * @return Guidance specific to the implementation of this operation, such as 6790 * limitations on the kind of transactions allowed, or information about 6791 * system wide search is implemented. 6792 */ 6793 public String getDocumentation() { 6794 return this.documentation == null ? null : this.documentation.getValue(); 6795 } 6796 6797 /** 6798 * @param value Guidance specific to the implementation of this operation, such 6799 * as limitations on the kind of transactions allowed, or 6800 * information about system wide search is implemented. 6801 */ 6802 public SystemInteractionComponent setDocumentation(String value) { 6803 if (value == null) 6804 this.documentation = null; 6805 else { 6806 if (this.documentation == null) 6807 this.documentation = new MarkdownType(); 6808 this.documentation.setValue(value); 6809 } 6810 return this; 6811 } 6812 6813 protected void listChildren(List<Property> children) { 6814 super.listChildren(children); 6815 children.add( 6816 new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 1, code)); 6817 children.add(new Property("documentation", "markdown", 6818 "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 6819 0, 1, documentation)); 6820 } 6821 6822 @Override 6823 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6824 switch (_hash) { 6825 case 3059181: 6826 /* code */ return new Property("code", "code", "A coded identifier of the operation, supported by the system.", 6827 0, 1, code); 6828 case 1587405498: 6829 /* documentation */ return new Property("documentation", "markdown", 6830 "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 6831 0, 1, documentation); 6832 default: 6833 return super.getNamedProperty(_hash, _name, _checkValid); 6834 } 6835 6836 } 6837 6838 @Override 6839 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6840 switch (hash) { 6841 case 3059181: 6842 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<SystemRestfulInteraction> 6843 case 1587405498: 6844 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 6845 default: 6846 return super.getProperty(hash, name, checkValid); 6847 } 6848 6849 } 6850 6851 @Override 6852 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6853 switch (hash) { 6854 case 3059181: // code 6855 value = new SystemRestfulInteractionEnumFactory().fromType(castToCode(value)); 6856 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 6857 return value; 6858 case 1587405498: // documentation 6859 this.documentation = castToMarkdown(value); // MarkdownType 6860 return value; 6861 default: 6862 return super.setProperty(hash, name, value); 6863 } 6864 6865 } 6866 6867 @Override 6868 public Base setProperty(String name, Base value) throws FHIRException { 6869 if (name.equals("code")) { 6870 value = new SystemRestfulInteractionEnumFactory().fromType(castToCode(value)); 6871 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 6872 } else if (name.equals("documentation")) { 6873 this.documentation = castToMarkdown(value); // MarkdownType 6874 } else 6875 return super.setProperty(name, value); 6876 return value; 6877 } 6878 6879 @Override 6880 public void removeChild(String name, Base value) throws FHIRException { 6881 if (name.equals("code")) { 6882 this.code = null; 6883 } else if (name.equals("documentation")) { 6884 this.documentation = null; 6885 } else 6886 super.removeChild(name, value); 6887 6888 } 6889 6890 @Override 6891 public Base makeProperty(int hash, String name) throws FHIRException { 6892 switch (hash) { 6893 case 3059181: 6894 return getCodeElement(); 6895 case 1587405498: 6896 return getDocumentationElement(); 6897 default: 6898 return super.makeProperty(hash, name); 6899 } 6900 6901 } 6902 6903 @Override 6904 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6905 switch (hash) { 6906 case 3059181: 6907 /* code */ return new String[] { "code" }; 6908 case 1587405498: 6909 /* documentation */ return new String[] { "markdown" }; 6910 default: 6911 return super.getTypesForProperty(hash, name); 6912 } 6913 6914 } 6915 6916 @Override 6917 public Base addChild(String name) throws FHIRException { 6918 if (name.equals("code")) { 6919 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.code"); 6920 } else if (name.equals("documentation")) { 6921 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 6922 } else 6923 return super.addChild(name); 6924 } 6925 6926 public SystemInteractionComponent copy() { 6927 SystemInteractionComponent dst = new SystemInteractionComponent(); 6928 copyValues(dst); 6929 return dst; 6930 } 6931 6932 public void copyValues(SystemInteractionComponent dst) { 6933 super.copyValues(dst); 6934 dst.code = code == null ? null : code.copy(); 6935 dst.documentation = documentation == null ? null : documentation.copy(); 6936 } 6937 6938 @Override 6939 public boolean equalsDeep(Base other_) { 6940 if (!super.equalsDeep(other_)) 6941 return false; 6942 if (!(other_ instanceof SystemInteractionComponent)) 6943 return false; 6944 SystemInteractionComponent o = (SystemInteractionComponent) other_; 6945 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 6946 } 6947 6948 @Override 6949 public boolean equalsShallow(Base other_) { 6950 if (!super.equalsShallow(other_)) 6951 return false; 6952 if (!(other_ instanceof SystemInteractionComponent)) 6953 return false; 6954 SystemInteractionComponent o = (SystemInteractionComponent) other_; 6955 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 6956 } 6957 6958 public boolean isEmpty() { 6959 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 6960 } 6961 6962 public String fhirType() { 6963 return "CapabilityStatement.rest.interaction"; 6964 6965 } 6966 6967 } 6968 6969 @Block() 6970 public static class CapabilityStatementMessagingComponent extends BackboneElement implements IBaseBackboneElement { 6971 /** 6972 * An endpoint (network accessible address) to which messages and/or replies are 6973 * to be sent. 6974 */ 6975 @Child(name = "endpoint", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6976 @Description(shortDefinition = "Where messages should be sent", formalDefinition = "An endpoint (network accessible address) to which messages and/or replies are to be sent.") 6977 protected List<CapabilityStatementMessagingEndpointComponent> endpoint; 6978 6979 /** 6980 * Length if the receiver's reliable messaging cache in minutes (if a receiver) 6981 * or how long the cache length on the receiver should be (if a sender). 6982 */ 6983 @Child(name = "reliableCache", type = { 6984 UnsignedIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6985 @Description(shortDefinition = "Reliable Message Cache Length (min)", formalDefinition = "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).") 6986 protected UnsignedIntType reliableCache; 6987 6988 /** 6989 * Documentation about the system's messaging capabilities for this endpoint not 6990 * otherwise documented by the capability statement. For example, the process 6991 * for becoming an authorized messaging exchange partner. 6992 */ 6993 @Child(name = "documentation", type = { 6994 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6995 @Description(shortDefinition = "Messaging interface behavior details", formalDefinition = "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.") 6996 protected MarkdownType documentation; 6997 6998 /** 6999 * References to message definitions for messages this system can send or 7000 * receive. 7001 */ 7002 @Child(name = "supportedMessage", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7003 @Description(shortDefinition = "Messages supported by this system", formalDefinition = "References to message definitions for messages this system can send or receive.") 7004 protected List<CapabilityStatementMessagingSupportedMessageComponent> supportedMessage; 7005 7006 private static final long serialVersionUID = 300411231L; 7007 7008 /** 7009 * Constructor 7010 */ 7011 public CapabilityStatementMessagingComponent() { 7012 super(); 7013 } 7014 7015 /** 7016 * @return {@link #endpoint} (An endpoint (network accessible address) to which 7017 * messages and/or replies are to be sent.) 7018 */ 7019 public List<CapabilityStatementMessagingEndpointComponent> getEndpoint() { 7020 if (this.endpoint == null) 7021 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7022 return this.endpoint; 7023 } 7024 7025 /** 7026 * @return Returns a reference to <code>this</code> for easy method chaining 7027 */ 7028 public CapabilityStatementMessagingComponent setEndpoint( 7029 List<CapabilityStatementMessagingEndpointComponent> theEndpoint) { 7030 this.endpoint = theEndpoint; 7031 return this; 7032 } 7033 7034 public boolean hasEndpoint() { 7035 if (this.endpoint == null) 7036 return false; 7037 for (CapabilityStatementMessagingEndpointComponent item : this.endpoint) 7038 if (!item.isEmpty()) 7039 return true; 7040 return false; 7041 } 7042 7043 public CapabilityStatementMessagingEndpointComponent addEndpoint() { // 3 7044 CapabilityStatementMessagingEndpointComponent t = new CapabilityStatementMessagingEndpointComponent(); 7045 if (this.endpoint == null) 7046 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7047 this.endpoint.add(t); 7048 return t; 7049 } 7050 7051 public CapabilityStatementMessagingComponent addEndpoint(CapabilityStatementMessagingEndpointComponent t) { // 3 7052 if (t == null) 7053 return this; 7054 if (this.endpoint == null) 7055 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7056 this.endpoint.add(t); 7057 return this; 7058 } 7059 7060 /** 7061 * @return The first repetition of repeating field {@link #endpoint}, creating 7062 * it if it does not already exist 7063 */ 7064 public CapabilityStatementMessagingEndpointComponent getEndpointFirstRep() { 7065 if (getEndpoint().isEmpty()) { 7066 addEndpoint(); 7067 } 7068 return getEndpoint().get(0); 7069 } 7070 7071 /** 7072 * @return {@link #reliableCache} (Length if the receiver's reliable messaging 7073 * cache in minutes (if a receiver) or how long the cache length on the 7074 * receiver should be (if a sender).). This is the underlying object 7075 * with id, value and extensions. The accessor "getReliableCache" gives 7076 * direct access to the value 7077 */ 7078 public UnsignedIntType getReliableCacheElement() { 7079 if (this.reliableCache == null) 7080 if (Configuration.errorOnAutoCreate()) 7081 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.reliableCache"); 7082 else if (Configuration.doAutoCreate()) 7083 this.reliableCache = new UnsignedIntType(); // bb 7084 return this.reliableCache; 7085 } 7086 7087 public boolean hasReliableCacheElement() { 7088 return this.reliableCache != null && !this.reliableCache.isEmpty(); 7089 } 7090 7091 public boolean hasReliableCache() { 7092 return this.reliableCache != null && !this.reliableCache.isEmpty(); 7093 } 7094 7095 /** 7096 * @param value {@link #reliableCache} (Length if the receiver's reliable 7097 * messaging cache in minutes (if a receiver) or how long the cache 7098 * length on the receiver should be (if a sender).). This is the 7099 * underlying object with id, value and extensions. The accessor 7100 * "getReliableCache" gives direct access to the value 7101 */ 7102 public CapabilityStatementMessagingComponent setReliableCacheElement(UnsignedIntType value) { 7103 this.reliableCache = value; 7104 return this; 7105 } 7106 7107 /** 7108 * @return Length if the receiver's reliable messaging cache in minutes (if a 7109 * receiver) or how long the cache length on the receiver should be (if 7110 * a sender). 7111 */ 7112 public int getReliableCache() { 7113 return this.reliableCache == null || this.reliableCache.isEmpty() ? 0 : this.reliableCache.getValue(); 7114 } 7115 7116 /** 7117 * @param value Length if the receiver's reliable messaging cache in minutes (if 7118 * a receiver) or how long the cache length on the receiver should 7119 * be (if a sender). 7120 */ 7121 public CapabilityStatementMessagingComponent setReliableCache(int value) { 7122 if (this.reliableCache == null) 7123 this.reliableCache = new UnsignedIntType(); 7124 this.reliableCache.setValue(value); 7125 return this; 7126 } 7127 7128 /** 7129 * @return {@link #documentation} (Documentation about the system's messaging 7130 * capabilities for this endpoint not otherwise documented by the 7131 * capability statement. For example, the process for becoming an 7132 * authorized messaging exchange partner.). This is the underlying 7133 * object with id, value and extensions. The accessor "getDocumentation" 7134 * gives direct access to the value 7135 */ 7136 public MarkdownType getDocumentationElement() { 7137 if (this.documentation == null) 7138 if (Configuration.errorOnAutoCreate()) 7139 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.documentation"); 7140 else if (Configuration.doAutoCreate()) 7141 this.documentation = new MarkdownType(); // bb 7142 return this.documentation; 7143 } 7144 7145 public boolean hasDocumentationElement() { 7146 return this.documentation != null && !this.documentation.isEmpty(); 7147 } 7148 7149 public boolean hasDocumentation() { 7150 return this.documentation != null && !this.documentation.isEmpty(); 7151 } 7152 7153 /** 7154 * @param value {@link #documentation} (Documentation about the system's 7155 * messaging capabilities for this endpoint not otherwise 7156 * documented by the capability statement. For example, the process 7157 * for becoming an authorized messaging exchange partner.). This is 7158 * the underlying object with id, value and extensions. The 7159 * accessor "getDocumentation" gives direct access to the value 7160 */ 7161 public CapabilityStatementMessagingComponent setDocumentationElement(MarkdownType value) { 7162 this.documentation = value; 7163 return this; 7164 } 7165 7166 /** 7167 * @return Documentation about the system's messaging capabilities for this 7168 * endpoint not otherwise documented by the capability statement. For 7169 * example, the process for becoming an authorized messaging exchange 7170 * partner. 7171 */ 7172 public String getDocumentation() { 7173 return this.documentation == null ? null : this.documentation.getValue(); 7174 } 7175 7176 /** 7177 * @param value Documentation about the system's messaging capabilities for this 7178 * endpoint not otherwise documented by the capability statement. 7179 * For example, the process for becoming an authorized messaging 7180 * exchange partner. 7181 */ 7182 public CapabilityStatementMessagingComponent setDocumentation(String value) { 7183 if (value == null) 7184 this.documentation = null; 7185 else { 7186 if (this.documentation == null) 7187 this.documentation = new MarkdownType(); 7188 this.documentation.setValue(value); 7189 } 7190 return this; 7191 } 7192 7193 /** 7194 * @return {@link #supportedMessage} (References to message definitions for 7195 * messages this system can send or receive.) 7196 */ 7197 public List<CapabilityStatementMessagingSupportedMessageComponent> getSupportedMessage() { 7198 if (this.supportedMessage == null) 7199 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7200 return this.supportedMessage; 7201 } 7202 7203 /** 7204 * @return Returns a reference to <code>this</code> for easy method chaining 7205 */ 7206 public CapabilityStatementMessagingComponent setSupportedMessage( 7207 List<CapabilityStatementMessagingSupportedMessageComponent> theSupportedMessage) { 7208 this.supportedMessage = theSupportedMessage; 7209 return this; 7210 } 7211 7212 public boolean hasSupportedMessage() { 7213 if (this.supportedMessage == null) 7214 return false; 7215 for (CapabilityStatementMessagingSupportedMessageComponent item : this.supportedMessage) 7216 if (!item.isEmpty()) 7217 return true; 7218 return false; 7219 } 7220 7221 public CapabilityStatementMessagingSupportedMessageComponent addSupportedMessage() { // 3 7222 CapabilityStatementMessagingSupportedMessageComponent t = new CapabilityStatementMessagingSupportedMessageComponent(); 7223 if (this.supportedMessage == null) 7224 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7225 this.supportedMessage.add(t); 7226 return t; 7227 } 7228 7229 public CapabilityStatementMessagingComponent addSupportedMessage( 7230 CapabilityStatementMessagingSupportedMessageComponent t) { // 3 7231 if (t == null) 7232 return this; 7233 if (this.supportedMessage == null) 7234 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7235 this.supportedMessage.add(t); 7236 return this; 7237 } 7238 7239 /** 7240 * @return The first repetition of repeating field {@link #supportedMessage}, 7241 * creating it if it does not already exist 7242 */ 7243 public CapabilityStatementMessagingSupportedMessageComponent getSupportedMessageFirstRep() { 7244 if (getSupportedMessage().isEmpty()) { 7245 addSupportedMessage(); 7246 } 7247 return getSupportedMessage().get(0); 7248 } 7249 7250 protected void listChildren(List<Property> children) { 7251 super.listChildren(children); 7252 children.add(new Property("endpoint", "", 7253 "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, 7254 java.lang.Integer.MAX_VALUE, endpoint)); 7255 children.add(new Property("reliableCache", "unsignedInt", 7256 "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 7257 0, 1, reliableCache)); 7258 children.add(new Property("documentation", "markdown", 7259 "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 7260 0, 1, documentation)); 7261 children.add(new Property("supportedMessage", "", 7262 "References to message definitions for messages this system can send or receive.", 0, 7263 java.lang.Integer.MAX_VALUE, supportedMessage)); 7264 } 7265 7266 @Override 7267 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7268 switch (_hash) { 7269 case 1741102485: 7270 /* endpoint */ return new Property("endpoint", "", 7271 "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, 7272 java.lang.Integer.MAX_VALUE, endpoint); 7273 case 897803608: 7274 /* reliableCache */ return new Property("reliableCache", "unsignedInt", 7275 "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 7276 0, 1, reliableCache); 7277 case 1587405498: 7278 /* documentation */ return new Property("documentation", "markdown", 7279 "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 7280 0, 1, documentation); 7281 case -1805139079: 7282 /* supportedMessage */ return new Property("supportedMessage", "", 7283 "References to message definitions for messages this system can send or receive.", 0, 7284 java.lang.Integer.MAX_VALUE, supportedMessage); 7285 default: 7286 return super.getNamedProperty(_hash, _name, _checkValid); 7287 } 7288 7289 } 7290 7291 @Override 7292 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7293 switch (hash) { 7294 case 1741102485: 7295 /* endpoint */ return this.endpoint == null ? new Base[0] 7296 : this.endpoint.toArray(new Base[this.endpoint.size()]); // CapabilityStatementMessagingEndpointComponent 7297 case 897803608: 7298 /* reliableCache */ return this.reliableCache == null ? new Base[0] : new Base[] { this.reliableCache }; // UnsignedIntType 7299 case 1587405498: 7300 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 7301 case -1805139079: 7302 /* supportedMessage */ return this.supportedMessage == null ? new Base[0] 7303 : this.supportedMessage.toArray(new Base[this.supportedMessage.size()]); // CapabilityStatementMessagingSupportedMessageComponent 7304 default: 7305 return super.getProperty(hash, name, checkValid); 7306 } 7307 7308 } 7309 7310 @Override 7311 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7312 switch (hash) { 7313 case 1741102485: // endpoint 7314 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); // CapabilityStatementMessagingEndpointComponent 7315 return value; 7316 case 897803608: // reliableCache 7317 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 7318 return value; 7319 case 1587405498: // documentation 7320 this.documentation = castToMarkdown(value); // MarkdownType 7321 return value; 7322 case -1805139079: // supportedMessage 7323 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); // CapabilityStatementMessagingSupportedMessageComponent 7324 return value; 7325 default: 7326 return super.setProperty(hash, name, value); 7327 } 7328 7329 } 7330 7331 @Override 7332 public Base setProperty(String name, Base value) throws FHIRException { 7333 if (name.equals("endpoint")) { 7334 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); 7335 } else if (name.equals("reliableCache")) { 7336 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 7337 } else if (name.equals("documentation")) { 7338 this.documentation = castToMarkdown(value); // MarkdownType 7339 } else if (name.equals("supportedMessage")) { 7340 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); 7341 } else 7342 return super.setProperty(name, value); 7343 return value; 7344 } 7345 7346 @Override 7347 public void removeChild(String name, Base value) throws FHIRException { 7348 if (name.equals("endpoint")) { 7349 this.getEndpoint().remove((CapabilityStatementMessagingEndpointComponent) value); 7350 } else if (name.equals("reliableCache")) { 7351 this.reliableCache = null; 7352 } else if (name.equals("documentation")) { 7353 this.documentation = null; 7354 } else if (name.equals("supportedMessage")) { 7355 this.getSupportedMessage().remove((CapabilityStatementMessagingSupportedMessageComponent) value); 7356 } else 7357 super.removeChild(name, value); 7358 7359 } 7360 7361 @Override 7362 public Base makeProperty(int hash, String name) throws FHIRException { 7363 switch (hash) { 7364 case 1741102485: 7365 return addEndpoint(); 7366 case 897803608: 7367 return getReliableCacheElement(); 7368 case 1587405498: 7369 return getDocumentationElement(); 7370 case -1805139079: 7371 return addSupportedMessage(); 7372 default: 7373 return super.makeProperty(hash, name); 7374 } 7375 7376 } 7377 7378 @Override 7379 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7380 switch (hash) { 7381 case 1741102485: 7382 /* endpoint */ return new String[] {}; 7383 case 897803608: 7384 /* reliableCache */ return new String[] { "unsignedInt" }; 7385 case 1587405498: 7386 /* documentation */ return new String[] { "markdown" }; 7387 case -1805139079: 7388 /* supportedMessage */ return new String[] {}; 7389 default: 7390 return super.getTypesForProperty(hash, name); 7391 } 7392 7393 } 7394 7395 @Override 7396 public Base addChild(String name) throws FHIRException { 7397 if (name.equals("endpoint")) { 7398 return addEndpoint(); 7399 } else if (name.equals("reliableCache")) { 7400 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.reliableCache"); 7401 } else if (name.equals("documentation")) { 7402 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 7403 } else if (name.equals("supportedMessage")) { 7404 return addSupportedMessage(); 7405 } else 7406 return super.addChild(name); 7407 } 7408 7409 public CapabilityStatementMessagingComponent copy() { 7410 CapabilityStatementMessagingComponent dst = new CapabilityStatementMessagingComponent(); 7411 copyValues(dst); 7412 return dst; 7413 } 7414 7415 public void copyValues(CapabilityStatementMessagingComponent dst) { 7416 super.copyValues(dst); 7417 if (endpoint != null) { 7418 dst.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 7419 for (CapabilityStatementMessagingEndpointComponent i : endpoint) 7420 dst.endpoint.add(i.copy()); 7421 } 7422 ; 7423 dst.reliableCache = reliableCache == null ? null : reliableCache.copy(); 7424 dst.documentation = documentation == null ? null : documentation.copy(); 7425 if (supportedMessage != null) { 7426 dst.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 7427 for (CapabilityStatementMessagingSupportedMessageComponent i : supportedMessage) 7428 dst.supportedMessage.add(i.copy()); 7429 } 7430 ; 7431 } 7432 7433 @Override 7434 public boolean equalsDeep(Base other_) { 7435 if (!super.equalsDeep(other_)) 7436 return false; 7437 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 7438 return false; 7439 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 7440 return compareDeep(endpoint, o.endpoint, true) && compareDeep(reliableCache, o.reliableCache, true) 7441 && compareDeep(documentation, o.documentation, true) 7442 && compareDeep(supportedMessage, o.supportedMessage, true); 7443 } 7444 7445 @Override 7446 public boolean equalsShallow(Base other_) { 7447 if (!super.equalsShallow(other_)) 7448 return false; 7449 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 7450 return false; 7451 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 7452 return compareValues(reliableCache, o.reliableCache, true) && compareValues(documentation, o.documentation, true); 7453 } 7454 7455 public boolean isEmpty() { 7456 return super.isEmpty() 7457 && ca.uhn.fhir.util.ElementUtil.isEmpty(endpoint, reliableCache, documentation, supportedMessage); 7458 } 7459 7460 public String fhirType() { 7461 return "CapabilityStatement.messaging"; 7462 7463 } 7464 7465 } 7466 7467 @Block() 7468 public static class CapabilityStatementMessagingEndpointComponent extends BackboneElement 7469 implements IBaseBackboneElement { 7470 /** 7471 * A list of the messaging transport protocol(s) identifiers, supported by this 7472 * endpoint. 7473 */ 7474 @Child(name = "protocol", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7475 @Description(shortDefinition = "http | ftp | mllp +", formalDefinition = "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.") 7476 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-transport") 7477 protected Coding protocol; 7478 7479 /** 7480 * The network address of the endpoint. For solutions that do not use network 7481 * addresses for routing, it can be just an identifier. 7482 */ 7483 @Child(name = "address", type = { UrlType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 7484 @Description(shortDefinition = "Network address or identifier of the end-point", formalDefinition = "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.") 7485 protected UrlType address; 7486 7487 private static final long serialVersionUID = -236946103L; 7488 7489 /** 7490 * Constructor 7491 */ 7492 public CapabilityStatementMessagingEndpointComponent() { 7493 super(); 7494 } 7495 7496 /** 7497 * Constructor 7498 */ 7499 public CapabilityStatementMessagingEndpointComponent(Coding protocol, UrlType address) { 7500 super(); 7501 this.protocol = protocol; 7502 this.address = address; 7503 } 7504 7505 /** 7506 * @return {@link #protocol} (A list of the messaging transport protocol(s) 7507 * identifiers, supported by this endpoint.) 7508 */ 7509 public Coding getProtocol() { 7510 if (this.protocol == null) 7511 if (Configuration.errorOnAutoCreate()) 7512 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.protocol"); 7513 else if (Configuration.doAutoCreate()) 7514 this.protocol = new Coding(); // cc 7515 return this.protocol; 7516 } 7517 7518 public boolean hasProtocol() { 7519 return this.protocol != null && !this.protocol.isEmpty(); 7520 } 7521 7522 /** 7523 * @param value {@link #protocol} (A list of the messaging transport protocol(s) 7524 * identifiers, supported by this endpoint.) 7525 */ 7526 public CapabilityStatementMessagingEndpointComponent setProtocol(Coding value) { 7527 this.protocol = value; 7528 return this; 7529 } 7530 7531 /** 7532 * @return {@link #address} (The network address of the endpoint. For solutions 7533 * that do not use network addresses for routing, it can be just an 7534 * identifier.). This is the underlying object with id, value and 7535 * extensions. The accessor "getAddress" gives direct access to the 7536 * value 7537 */ 7538 public UrlType getAddressElement() { 7539 if (this.address == null) 7540 if (Configuration.errorOnAutoCreate()) 7541 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.address"); 7542 else if (Configuration.doAutoCreate()) 7543 this.address = new UrlType(); // bb 7544 return this.address; 7545 } 7546 7547 public boolean hasAddressElement() { 7548 return this.address != null && !this.address.isEmpty(); 7549 } 7550 7551 public boolean hasAddress() { 7552 return this.address != null && !this.address.isEmpty(); 7553 } 7554 7555 /** 7556 * @param value {@link #address} (The network address of the endpoint. For 7557 * solutions that do not use network addresses for routing, it can 7558 * be just an identifier.). This is the underlying object with id, 7559 * value and extensions. The accessor "getAddress" gives direct 7560 * access to the value 7561 */ 7562 public CapabilityStatementMessagingEndpointComponent setAddressElement(UrlType value) { 7563 this.address = value; 7564 return this; 7565 } 7566 7567 /** 7568 * @return The network address of the endpoint. For solutions that do not use 7569 * network addresses for routing, it can be just an identifier. 7570 */ 7571 public String getAddress() { 7572 return this.address == null ? null : this.address.getValue(); 7573 } 7574 7575 /** 7576 * @param value The network address of the endpoint. For solutions that do not 7577 * use network addresses for routing, it can be just an identifier. 7578 */ 7579 public CapabilityStatementMessagingEndpointComponent setAddress(String value) { 7580 if (this.address == null) 7581 this.address = new UrlType(); 7582 this.address.setValue(value); 7583 return this; 7584 } 7585 7586 protected void listChildren(List<Property> children) { 7587 super.listChildren(children); 7588 children.add(new Property("protocol", "Coding", 7589 "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol)); 7590 children.add(new Property("address", "url", 7591 "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.", 7592 0, 1, address)); 7593 } 7594 7595 @Override 7596 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7597 switch (_hash) { 7598 case -989163880: 7599 /* protocol */ return new Property("protocol", "Coding", 7600 "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol); 7601 case -1147692044: 7602 /* address */ return new Property("address", "url", 7603 "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.", 7604 0, 1, address); 7605 default: 7606 return super.getNamedProperty(_hash, _name, _checkValid); 7607 } 7608 7609 } 7610 7611 @Override 7612 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7613 switch (hash) { 7614 case -989163880: 7615 /* protocol */ return this.protocol == null ? new Base[0] : new Base[] { this.protocol }; // Coding 7616 case -1147692044: 7617 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // UrlType 7618 default: 7619 return super.getProperty(hash, name, checkValid); 7620 } 7621 7622 } 7623 7624 @Override 7625 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7626 switch (hash) { 7627 case -989163880: // protocol 7628 this.protocol = castToCoding(value); // Coding 7629 return value; 7630 case -1147692044: // address 7631 this.address = castToUrl(value); // UrlType 7632 return value; 7633 default: 7634 return super.setProperty(hash, name, value); 7635 } 7636 7637 } 7638 7639 @Override 7640 public Base setProperty(String name, Base value) throws FHIRException { 7641 if (name.equals("protocol")) { 7642 this.protocol = castToCoding(value); // Coding 7643 } else if (name.equals("address")) { 7644 this.address = castToUrl(value); // UrlType 7645 } else 7646 return super.setProperty(name, value); 7647 return value; 7648 } 7649 7650 @Override 7651 public void removeChild(String name, Base value) throws FHIRException { 7652 if (name.equals("protocol")) { 7653 this.protocol = null; 7654 } else if (name.equals("address")) { 7655 this.address = null; 7656 } else 7657 super.removeChild(name, value); 7658 7659 } 7660 7661 @Override 7662 public Base makeProperty(int hash, String name) throws FHIRException { 7663 switch (hash) { 7664 case -989163880: 7665 return getProtocol(); 7666 case -1147692044: 7667 return getAddressElement(); 7668 default: 7669 return super.makeProperty(hash, name); 7670 } 7671 7672 } 7673 7674 @Override 7675 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7676 switch (hash) { 7677 case -989163880: 7678 /* protocol */ return new String[] { "Coding" }; 7679 case -1147692044: 7680 /* address */ return new String[] { "url" }; 7681 default: 7682 return super.getTypesForProperty(hash, name); 7683 } 7684 7685 } 7686 7687 @Override 7688 public Base addChild(String name) throws FHIRException { 7689 if (name.equals("protocol")) { 7690 this.protocol = new Coding(); 7691 return this.protocol; 7692 } else if (name.equals("address")) { 7693 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.address"); 7694 } else 7695 return super.addChild(name); 7696 } 7697 7698 public CapabilityStatementMessagingEndpointComponent copy() { 7699 CapabilityStatementMessagingEndpointComponent dst = new CapabilityStatementMessagingEndpointComponent(); 7700 copyValues(dst); 7701 return dst; 7702 } 7703 7704 public void copyValues(CapabilityStatementMessagingEndpointComponent dst) { 7705 super.copyValues(dst); 7706 dst.protocol = protocol == null ? null : protocol.copy(); 7707 dst.address = address == null ? null : address.copy(); 7708 } 7709 7710 @Override 7711 public boolean equalsDeep(Base other_) { 7712 if (!super.equalsDeep(other_)) 7713 return false; 7714 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 7715 return false; 7716 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 7717 return compareDeep(protocol, o.protocol, true) && compareDeep(address, o.address, true); 7718 } 7719 7720 @Override 7721 public boolean equalsShallow(Base other_) { 7722 if (!super.equalsShallow(other_)) 7723 return false; 7724 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 7725 return false; 7726 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 7727 return compareValues(address, o.address, true); 7728 } 7729 7730 public boolean isEmpty() { 7731 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(protocol, address); 7732 } 7733 7734 public String fhirType() { 7735 return "CapabilityStatement.messaging.endpoint"; 7736 7737 } 7738 7739 } 7740 7741 @Block() 7742 public static class CapabilityStatementMessagingSupportedMessageComponent extends BackboneElement 7743 implements IBaseBackboneElement { 7744 /** 7745 * The mode of this event declaration - whether application is sender or 7746 * receiver. 7747 */ 7748 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 7749 @Description(shortDefinition = "sender | receiver", formalDefinition = "The mode of this event declaration - whether application is sender or receiver.") 7750 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-capability-mode") 7751 protected Enumeration<EventCapabilityMode> mode; 7752 7753 /** 7754 * Points to a message definition that identifies the messaging event, message 7755 * structure, allowed responses, etc. 7756 */ 7757 @Child(name = "definition", type = { 7758 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 7759 @Description(shortDefinition = "Message supported by this system", formalDefinition = "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.") 7760 protected CanonicalType definition; 7761 7762 private static final long serialVersionUID = -1172840676L; 7763 7764 /** 7765 * Constructor 7766 */ 7767 public CapabilityStatementMessagingSupportedMessageComponent() { 7768 super(); 7769 } 7770 7771 /** 7772 * Constructor 7773 */ 7774 public CapabilityStatementMessagingSupportedMessageComponent(Enumeration<EventCapabilityMode> mode, 7775 CanonicalType definition) { 7776 super(); 7777 this.mode = mode; 7778 this.definition = definition; 7779 } 7780 7781 /** 7782 * @return {@link #mode} (The mode of this event declaration - whether 7783 * application is sender or receiver.). This is the underlying object 7784 * with id, value and extensions. The accessor "getMode" gives direct 7785 * access to the value 7786 */ 7787 public Enumeration<EventCapabilityMode> getModeElement() { 7788 if (this.mode == null) 7789 if (Configuration.errorOnAutoCreate()) 7790 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.mode"); 7791 else if (Configuration.doAutoCreate()) 7792 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); // bb 7793 return this.mode; 7794 } 7795 7796 public boolean hasModeElement() { 7797 return this.mode != null && !this.mode.isEmpty(); 7798 } 7799 7800 public boolean hasMode() { 7801 return this.mode != null && !this.mode.isEmpty(); 7802 } 7803 7804 /** 7805 * @param value {@link #mode} (The mode of this event declaration - whether 7806 * application is sender or receiver.). This is the underlying 7807 * object with id, value and extensions. The accessor "getMode" 7808 * gives direct access to the value 7809 */ 7810 public CapabilityStatementMessagingSupportedMessageComponent setModeElement( 7811 Enumeration<EventCapabilityMode> value) { 7812 this.mode = value; 7813 return this; 7814 } 7815 7816 /** 7817 * @return The mode of this event declaration - whether application is sender or 7818 * receiver. 7819 */ 7820 public EventCapabilityMode getMode() { 7821 return this.mode == null ? null : this.mode.getValue(); 7822 } 7823 7824 /** 7825 * @param value The mode of this event declaration - whether application is 7826 * sender or receiver. 7827 */ 7828 public CapabilityStatementMessagingSupportedMessageComponent setMode(EventCapabilityMode value) { 7829 if (this.mode == null) 7830 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); 7831 this.mode.setValue(value); 7832 return this; 7833 } 7834 7835 /** 7836 * @return {@link #definition} (Points to a message definition that identifies 7837 * the messaging event, message structure, allowed responses, etc.). 7838 * This is the underlying object with id, value and extensions. The 7839 * accessor "getDefinition" gives direct access to the value 7840 */ 7841 public CanonicalType getDefinitionElement() { 7842 if (this.definition == null) 7843 if (Configuration.errorOnAutoCreate()) 7844 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.definition"); 7845 else if (Configuration.doAutoCreate()) 7846 this.definition = new CanonicalType(); // bb 7847 return this.definition; 7848 } 7849 7850 public boolean hasDefinitionElement() { 7851 return this.definition != null && !this.definition.isEmpty(); 7852 } 7853 7854 public boolean hasDefinition() { 7855 return this.definition != null && !this.definition.isEmpty(); 7856 } 7857 7858 /** 7859 * @param value {@link #definition} (Points to a message definition that 7860 * identifies the messaging event, message structure, allowed 7861 * responses, etc.). This is the underlying object with id, value 7862 * and extensions. The accessor "getDefinition" gives direct access 7863 * to the value 7864 */ 7865 public CapabilityStatementMessagingSupportedMessageComponent setDefinitionElement(CanonicalType value) { 7866 this.definition = value; 7867 return this; 7868 } 7869 7870 /** 7871 * @return Points to a message definition that identifies the messaging event, 7872 * message structure, allowed responses, etc. 7873 */ 7874 public String getDefinition() { 7875 return this.definition == null ? null : this.definition.getValue(); 7876 } 7877 7878 /** 7879 * @param value Points to a message definition that identifies the messaging 7880 * event, message structure, allowed responses, etc. 7881 */ 7882 public CapabilityStatementMessagingSupportedMessageComponent setDefinition(String value) { 7883 if (this.definition == null) 7884 this.definition = new CanonicalType(); 7885 this.definition.setValue(value); 7886 return this; 7887 } 7888 7889 protected void listChildren(List<Property> children) { 7890 super.listChildren(children); 7891 children.add(new Property("mode", "code", 7892 "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode)); 7893 children.add(new Property("definition", "canonical(MessageDefinition)", 7894 "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 7895 0, 1, definition)); 7896 } 7897 7898 @Override 7899 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7900 switch (_hash) { 7901 case 3357091: 7902 /* mode */ return new Property("mode", "code", 7903 "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode); 7904 case -1014418093: 7905 /* definition */ return new Property("definition", "canonical(MessageDefinition)", 7906 "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 7907 0, 1, definition); 7908 default: 7909 return super.getNamedProperty(_hash, _name, _checkValid); 7910 } 7911 7912 } 7913 7914 @Override 7915 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7916 switch (hash) { 7917 case 3357091: 7918 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<EventCapabilityMode> 7919 case -1014418093: 7920 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 7921 default: 7922 return super.getProperty(hash, name, checkValid); 7923 } 7924 7925 } 7926 7927 @Override 7928 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7929 switch (hash) { 7930 case 3357091: // mode 7931 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 7932 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 7933 return value; 7934 case -1014418093: // definition 7935 this.definition = castToCanonical(value); // CanonicalType 7936 return value; 7937 default: 7938 return super.setProperty(hash, name, value); 7939 } 7940 7941 } 7942 7943 @Override 7944 public Base setProperty(String name, Base value) throws FHIRException { 7945 if (name.equals("mode")) { 7946 value = new EventCapabilityModeEnumFactory().fromType(castToCode(value)); 7947 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 7948 } else if (name.equals("definition")) { 7949 this.definition = castToCanonical(value); // CanonicalType 7950 } else 7951 return super.setProperty(name, value); 7952 return value; 7953 } 7954 7955 @Override 7956 public void removeChild(String name, Base value) throws FHIRException { 7957 if (name.equals("mode")) { 7958 this.mode = null; 7959 } else if (name.equals("definition")) { 7960 this.definition = null; 7961 } else 7962 super.removeChild(name, value); 7963 7964 } 7965 7966 @Override 7967 public Base makeProperty(int hash, String name) throws FHIRException { 7968 switch (hash) { 7969 case 3357091: 7970 return getModeElement(); 7971 case -1014418093: 7972 return getDefinitionElement(); 7973 default: 7974 return super.makeProperty(hash, name); 7975 } 7976 7977 } 7978 7979 @Override 7980 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7981 switch (hash) { 7982 case 3357091: 7983 /* mode */ return new String[] { "code" }; 7984 case -1014418093: 7985 /* definition */ return new String[] { "canonical" }; 7986 default: 7987 return super.getTypesForProperty(hash, name); 7988 } 7989 7990 } 7991 7992 @Override 7993 public Base addChild(String name) throws FHIRException { 7994 if (name.equals("mode")) { 7995 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 7996 } else if (name.equals("definition")) { 7997 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.definition"); 7998 } else 7999 return super.addChild(name); 8000 } 8001 8002 public CapabilityStatementMessagingSupportedMessageComponent copy() { 8003 CapabilityStatementMessagingSupportedMessageComponent dst = new CapabilityStatementMessagingSupportedMessageComponent(); 8004 copyValues(dst); 8005 return dst; 8006 } 8007 8008 public void copyValues(CapabilityStatementMessagingSupportedMessageComponent dst) { 8009 super.copyValues(dst); 8010 dst.mode = mode == null ? null : mode.copy(); 8011 dst.definition = definition == null ? null : definition.copy(); 8012 } 8013 8014 @Override 8015 public boolean equalsDeep(Base other_) { 8016 if (!super.equalsDeep(other_)) 8017 return false; 8018 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 8019 return false; 8020 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 8021 return compareDeep(mode, o.mode, true) && compareDeep(definition, o.definition, true); 8022 } 8023 8024 @Override 8025 public boolean equalsShallow(Base other_) { 8026 if (!super.equalsShallow(other_)) 8027 return false; 8028 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 8029 return false; 8030 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 8031 return compareValues(mode, o.mode, true); 8032 } 8033 8034 public boolean isEmpty() { 8035 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, definition); 8036 } 8037 8038 public String fhirType() { 8039 return "CapabilityStatement.messaging.supportedMessage"; 8040 8041 } 8042 8043 } 8044 8045 @Block() 8046 public static class CapabilityStatementDocumentComponent extends BackboneElement implements IBaseBackboneElement { 8047 /** 8048 * Mode of this document declaration - whether an application is a producer or 8049 * consumer. 8050 */ 8051 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 8052 @Description(shortDefinition = "producer | consumer", formalDefinition = "Mode of this document declaration - whether an application is a producer or consumer.") 8053 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-mode") 8054 protected Enumeration<DocumentMode> mode; 8055 8056 /** 8057 * A description of how the application supports or uses the specified document 8058 * profile. For example, when documents are created, what action is taken with 8059 * consumed documents, etc. 8060 */ 8061 @Child(name = "documentation", type = { 8062 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 8063 @Description(shortDefinition = "Description of document support", formalDefinition = "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.") 8064 protected MarkdownType documentation; 8065 8066 /** 8067 * A profile on the document Bundle that constrains which resources are present, 8068 * and their contents. 8069 */ 8070 @Child(name = "profile", type = { 8071 CanonicalType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 8072 @Description(shortDefinition = "Constraint on the resources used in the document", formalDefinition = "A profile on the document Bundle that constrains which resources are present, and their contents.") 8073 protected CanonicalType profile; 8074 8075 private static final long serialVersionUID = 18026632L; 8076 8077 /** 8078 * Constructor 8079 */ 8080 public CapabilityStatementDocumentComponent() { 8081 super(); 8082 } 8083 8084 /** 8085 * Constructor 8086 */ 8087 public CapabilityStatementDocumentComponent(Enumeration<DocumentMode> mode, CanonicalType profile) { 8088 super(); 8089 this.mode = mode; 8090 this.profile = profile; 8091 } 8092 8093 /** 8094 * @return {@link #mode} (Mode of this document declaration - whether an 8095 * application is a producer or consumer.). This is the underlying 8096 * object with id, value and extensions. The accessor "getMode" gives 8097 * direct access to the value 8098 */ 8099 public Enumeration<DocumentMode> getModeElement() { 8100 if (this.mode == null) 8101 if (Configuration.errorOnAutoCreate()) 8102 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.mode"); 8103 else if (Configuration.doAutoCreate()) 8104 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); // bb 8105 return this.mode; 8106 } 8107 8108 public boolean hasModeElement() { 8109 return this.mode != null && !this.mode.isEmpty(); 8110 } 8111 8112 public boolean hasMode() { 8113 return this.mode != null && !this.mode.isEmpty(); 8114 } 8115 8116 /** 8117 * @param value {@link #mode} (Mode of this document declaration - whether an 8118 * application is a producer or consumer.). This is the underlying 8119 * object with id, value and extensions. The accessor "getMode" 8120 * gives direct access to the value 8121 */ 8122 public CapabilityStatementDocumentComponent setModeElement(Enumeration<DocumentMode> value) { 8123 this.mode = value; 8124 return this; 8125 } 8126 8127 /** 8128 * @return Mode of this document declaration - whether an application is a 8129 * producer or consumer. 8130 */ 8131 public DocumentMode getMode() { 8132 return this.mode == null ? null : this.mode.getValue(); 8133 } 8134 8135 /** 8136 * @param value Mode of this document declaration - whether an application is a 8137 * producer or consumer. 8138 */ 8139 public CapabilityStatementDocumentComponent setMode(DocumentMode value) { 8140 if (this.mode == null) 8141 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); 8142 this.mode.setValue(value); 8143 return this; 8144 } 8145 8146 /** 8147 * @return {@link #documentation} (A description of how the application supports 8148 * or uses the specified document profile. For example, when documents 8149 * are created, what action is taken with consumed documents, etc.). 8150 * This is the underlying object with id, value and extensions. The 8151 * accessor "getDocumentation" gives direct access to the value 8152 */ 8153 public MarkdownType getDocumentationElement() { 8154 if (this.documentation == null) 8155 if (Configuration.errorOnAutoCreate()) 8156 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.documentation"); 8157 else if (Configuration.doAutoCreate()) 8158 this.documentation = new MarkdownType(); // bb 8159 return this.documentation; 8160 } 8161 8162 public boolean hasDocumentationElement() { 8163 return this.documentation != null && !this.documentation.isEmpty(); 8164 } 8165 8166 public boolean hasDocumentation() { 8167 return this.documentation != null && !this.documentation.isEmpty(); 8168 } 8169 8170 /** 8171 * @param value {@link #documentation} (A description of how the application 8172 * supports or uses the specified document profile. For example, 8173 * when documents are created, what action is taken with consumed 8174 * documents, etc.). This is the underlying object with id, value 8175 * and extensions. The accessor "getDocumentation" gives direct 8176 * access to the value 8177 */ 8178 public CapabilityStatementDocumentComponent setDocumentationElement(MarkdownType value) { 8179 this.documentation = value; 8180 return this; 8181 } 8182 8183 /** 8184 * @return A description of how the application supports or uses the specified 8185 * document profile. For example, when documents are created, what 8186 * action is taken with consumed documents, etc. 8187 */ 8188 public String getDocumentation() { 8189 return this.documentation == null ? null : this.documentation.getValue(); 8190 } 8191 8192 /** 8193 * @param value A description of how the application supports or uses the 8194 * specified document profile. For example, when documents are 8195 * created, what action is taken with consumed documents, etc. 8196 */ 8197 public CapabilityStatementDocumentComponent setDocumentation(String value) { 8198 if (value == null) 8199 this.documentation = null; 8200 else { 8201 if (this.documentation == null) 8202 this.documentation = new MarkdownType(); 8203 this.documentation.setValue(value); 8204 } 8205 return this; 8206 } 8207 8208 /** 8209 * @return {@link #profile} (A profile on the document Bundle that constrains 8210 * which resources are present, and their contents.). This is the 8211 * underlying object with id, value and extensions. The accessor 8212 * "getProfile" gives direct access to the value 8213 */ 8214 public CanonicalType getProfileElement() { 8215 if (this.profile == null) 8216 if (Configuration.errorOnAutoCreate()) 8217 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.profile"); 8218 else if (Configuration.doAutoCreate()) 8219 this.profile = new CanonicalType(); // bb 8220 return this.profile; 8221 } 8222 8223 public boolean hasProfileElement() { 8224 return this.profile != null && !this.profile.isEmpty(); 8225 } 8226 8227 public boolean hasProfile() { 8228 return this.profile != null && !this.profile.isEmpty(); 8229 } 8230 8231 /** 8232 * @param value {@link #profile} (A profile on the document Bundle that 8233 * constrains which resources are present, and their contents.). 8234 * This is the underlying object with id, value and extensions. The 8235 * accessor "getProfile" gives direct access to the value 8236 */ 8237 public CapabilityStatementDocumentComponent setProfileElement(CanonicalType value) { 8238 this.profile = value; 8239 return this; 8240 } 8241 8242 /** 8243 * @return A profile on the document Bundle that constrains which resources are 8244 * present, and their contents. 8245 */ 8246 public String getProfile() { 8247 return this.profile == null ? null : this.profile.getValue(); 8248 } 8249 8250 /** 8251 * @param value A profile on the document Bundle that constrains which resources 8252 * are present, and their contents. 8253 */ 8254 public CapabilityStatementDocumentComponent setProfile(String value) { 8255 if (this.profile == null) 8256 this.profile = new CanonicalType(); 8257 this.profile.setValue(value); 8258 return this; 8259 } 8260 8261 protected void listChildren(List<Property> children) { 8262 super.listChildren(children); 8263 children.add(new Property("mode", "code", 8264 "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode)); 8265 children.add(new Property("documentation", "markdown", 8266 "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 8267 0, 1, documentation)); 8268 children.add(new Property("profile", "canonical(StructureDefinition)", 8269 "A profile on the document Bundle that constrains which resources are present, and their contents.", 0, 1, 8270 profile)); 8271 } 8272 8273 @Override 8274 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8275 switch (_hash) { 8276 case 3357091: 8277 /* mode */ return new Property("mode", "code", 8278 "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode); 8279 case 1587405498: 8280 /* documentation */ return new Property("documentation", "markdown", 8281 "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 8282 0, 1, documentation); 8283 case -309425751: 8284 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 8285 "A profile on the document Bundle that constrains which resources are present, and their contents.", 0, 1, 8286 profile); 8287 default: 8288 return super.getNamedProperty(_hash, _name, _checkValid); 8289 } 8290 8291 } 8292 8293 @Override 8294 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8295 switch (hash) { 8296 case 3357091: 8297 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<DocumentMode> 8298 case 1587405498: 8299 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // MarkdownType 8300 case -309425751: 8301 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 8302 default: 8303 return super.getProperty(hash, name, checkValid); 8304 } 8305 8306 } 8307 8308 @Override 8309 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8310 switch (hash) { 8311 case 3357091: // mode 8312 value = new DocumentModeEnumFactory().fromType(castToCode(value)); 8313 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 8314 return value; 8315 case 1587405498: // documentation 8316 this.documentation = castToMarkdown(value); // MarkdownType 8317 return value; 8318 case -309425751: // profile 8319 this.profile = castToCanonical(value); // CanonicalType 8320 return value; 8321 default: 8322 return super.setProperty(hash, name, value); 8323 } 8324 8325 } 8326 8327 @Override 8328 public Base setProperty(String name, Base value) throws FHIRException { 8329 if (name.equals("mode")) { 8330 value = new DocumentModeEnumFactory().fromType(castToCode(value)); 8331 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 8332 } else if (name.equals("documentation")) { 8333 this.documentation = castToMarkdown(value); // MarkdownType 8334 } else if (name.equals("profile")) { 8335 this.profile = castToCanonical(value); // CanonicalType 8336 } else 8337 return super.setProperty(name, value); 8338 return value; 8339 } 8340 8341 @Override 8342 public void removeChild(String name, Base value) throws FHIRException { 8343 if (name.equals("mode")) { 8344 this.mode = null; 8345 } else if (name.equals("documentation")) { 8346 this.documentation = null; 8347 } else if (name.equals("profile")) { 8348 this.profile = null; 8349 } else 8350 super.removeChild(name, value); 8351 8352 } 8353 8354 @Override 8355 public Base makeProperty(int hash, String name) throws FHIRException { 8356 switch (hash) { 8357 case 3357091: 8358 return getModeElement(); 8359 case 1587405498: 8360 return getDocumentationElement(); 8361 case -309425751: 8362 return getProfileElement(); 8363 default: 8364 return super.makeProperty(hash, name); 8365 } 8366 8367 } 8368 8369 @Override 8370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8371 switch (hash) { 8372 case 3357091: 8373 /* mode */ return new String[] { "code" }; 8374 case 1587405498: 8375 /* documentation */ return new String[] { "markdown" }; 8376 case -309425751: 8377 /* profile */ return new String[] { "canonical" }; 8378 default: 8379 return super.getTypesForProperty(hash, name); 8380 } 8381 8382 } 8383 8384 @Override 8385 public Base addChild(String name) throws FHIRException { 8386 if (name.equals("mode")) { 8387 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.mode"); 8388 } else if (name.equals("documentation")) { 8389 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.documentation"); 8390 } else if (name.equals("profile")) { 8391 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.profile"); 8392 } else 8393 return super.addChild(name); 8394 } 8395 8396 public CapabilityStatementDocumentComponent copy() { 8397 CapabilityStatementDocumentComponent dst = new CapabilityStatementDocumentComponent(); 8398 copyValues(dst); 8399 return dst; 8400 } 8401 8402 public void copyValues(CapabilityStatementDocumentComponent dst) { 8403 super.copyValues(dst); 8404 dst.mode = mode == null ? null : mode.copy(); 8405 dst.documentation = documentation == null ? null : documentation.copy(); 8406 dst.profile = profile == null ? null : profile.copy(); 8407 } 8408 8409 @Override 8410 public boolean equalsDeep(Base other_) { 8411 if (!super.equalsDeep(other_)) 8412 return false; 8413 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 8414 return false; 8415 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 8416 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 8417 && compareDeep(profile, o.profile, true); 8418 } 8419 8420 @Override 8421 public boolean equalsShallow(Base other_) { 8422 if (!super.equalsShallow(other_)) 8423 return false; 8424 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 8425 return false; 8426 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 8427 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 8428 } 8429 8430 public boolean isEmpty() { 8431 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, profile); 8432 } 8433 8434 public String fhirType() { 8435 return "CapabilityStatement.document"; 8436 8437 } 8438 8439 } 8440 8441 /** 8442 * Explanation of why this capability statement is needed and why it has been 8443 * designed as it has. 8444 */ 8445 @Child(name = "purpose", type = { 8446 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 8447 @Description(shortDefinition = "Why this capability statement is defined", formalDefinition = "Explanation of why this capability statement is needed and why it has been designed as it has.") 8448 protected MarkdownType purpose; 8449 8450 /** 8451 * A copyright statement relating to the capability statement and/or its 8452 * contents. Copyright statements are generally legal restrictions on the use 8453 * and publishing of the capability statement. 8454 */ 8455 @Child(name = "copyright", type = { 8456 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 8457 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.") 8458 protected MarkdownType copyright; 8459 8460 /** 8461 * The way that this statement is intended to be used, to describe an actual 8462 * running instance of software, a particular product (kind, not instance of 8463 * software) or a class of implementation (e.g. a desired purchase). 8464 */ 8465 @Child(name = "kind", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 8466 @Description(shortDefinition = "instance | capability | requirements", formalDefinition = "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).") 8467 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/capability-statement-kind") 8468 protected Enumeration<CapabilityStatementKind> kind; 8469 8470 /** 8471 * Reference to a canonical URL of another CapabilityStatement that this 8472 * software implements. This capability statement is a published API description 8473 * that corresponds to a business service. The server may actually implement a 8474 * subset of the capability statement it claims to implement, so the capability 8475 * statement must specify the full capability details. 8476 */ 8477 @Child(name = "instantiates", type = { 8478 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8479 @Description(shortDefinition = "Canonical URL of another capability statement this implements", formalDefinition = "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.") 8480 protected List<CanonicalType> instantiates; 8481 8482 /** 8483 * Reference to a canonical URL of another CapabilityStatement that this 8484 * software adds to. The capability statement automatically includes everything 8485 * in the other statement, and it is not duplicated, though the server may 8486 * repeat the same resources, interactions and operations to add additional 8487 * details to them. 8488 */ 8489 @Child(name = "imports", type = { 8490 CanonicalType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8491 @Description(shortDefinition = "Canonical URL of another capability statement this adds to", formalDefinition = "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.") 8492 protected List<CanonicalType> imports; 8493 8494 /** 8495 * Software that is covered by this capability statement. It is used when the 8496 * capability statement describes the capabilities of a particular software 8497 * version, independent of an installation. 8498 */ 8499 @Child(name = "software", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 8500 @Description(shortDefinition = "Software that is covered by this capability statement", formalDefinition = "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.") 8501 protected CapabilityStatementSoftwareComponent software; 8502 8503 /** 8504 * Identifies a specific implementation instance that is described by the 8505 * capability statement - i.e. a particular installation, rather than the 8506 * capabilities of a software program. 8507 */ 8508 @Child(name = "implementation", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 8509 @Description(shortDefinition = "If this describes a specific instance", formalDefinition = "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.") 8510 protected CapabilityStatementImplementationComponent implementation; 8511 8512 /** 8513 * The version of the FHIR specification that this CapabilityStatement describes 8514 * (which SHALL be the same as the FHIR version of the CapabilityStatement 8515 * itself). There is no default value. 8516 */ 8517 @Child(name = "fhirVersion", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 8518 @Description(shortDefinition = "FHIR Version the system supports", formalDefinition = "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.") 8519 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/FHIR-version") 8520 protected Enumeration<FHIRVersion> fhirVersion; 8521 8522 /** 8523 * A list of the formats supported by this implementation using their content 8524 * types. 8525 */ 8526 @Child(name = "format", type = { 8527 CodeType.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8528 @Description(shortDefinition = "formats supported (xml | json | ttl | mime type)", formalDefinition = "A list of the formats supported by this implementation using their content types.") 8529 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 8530 protected List<CodeType> format; 8531 8532 /** 8533 * A list of the patch formats supported by this implementation using their 8534 * content types. 8535 */ 8536 @Child(name = "patchFormat", type = { 8537 CodeType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8538 @Description(shortDefinition = "Patch formats supported", formalDefinition = "A list of the patch formats supported by this implementation using their content types.") 8539 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 8540 protected List<CodeType> patchFormat; 8541 8542 /** 8543 * A list of implementation guides that the server does (or should) support in 8544 * their entirety. 8545 */ 8546 @Child(name = "implementationGuide", type = { 8547 CanonicalType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8548 @Description(shortDefinition = "Implementation guides supported", formalDefinition = "A list of implementation guides that the server does (or should) support in their entirety.") 8549 protected List<CanonicalType> implementationGuide; 8550 8551 /** 8552 * A definition of the restful capabilities of the solution, if any. 8553 */ 8554 @Child(name = "rest", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8555 @Description(shortDefinition = "If the endpoint is a RESTful one", formalDefinition = "A definition of the restful capabilities of the solution, if any.") 8556 protected List<CapabilityStatementRestComponent> rest; 8557 8558 /** 8559 * A description of the messaging capabilities of the solution. 8560 */ 8561 @Child(name = "messaging", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8562 @Description(shortDefinition = "If messaging is supported", formalDefinition = "A description of the messaging capabilities of the solution.") 8563 protected List<CapabilityStatementMessagingComponent> messaging; 8564 8565 /** 8566 * A document definition. 8567 */ 8568 @Child(name = "document", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8569 @Description(shortDefinition = "Document definition", formalDefinition = "A document definition.") 8570 protected List<CapabilityStatementDocumentComponent> document; 8571 8572 private static final long serialVersionUID = -1050288843L; 8573 8574 /** 8575 * Constructor 8576 */ 8577 public CapabilityStatement() { 8578 super(); 8579 } 8580 8581 /** 8582 * Constructor 8583 */ 8584 public CapabilityStatement(Enumeration<PublicationStatus> status, DateTimeType date, 8585 Enumeration<CapabilityStatementKind> kind, Enumeration<FHIRVersion> fhirVersion) { 8586 super(); 8587 this.status = status; 8588 this.date = date; 8589 this.kind = kind; 8590 this.fhirVersion = fhirVersion; 8591 } 8592 8593 /** 8594 * @return {@link #url} (An absolute URI that is used to identify this 8595 * capability statement when it is referenced in a specification, model, 8596 * design or an instance; also called its canonical identifier. This 8597 * SHOULD be globally unique and SHOULD be a literal address at which at 8598 * which an authoritative instance of this capability statement is (or 8599 * will be) published. This URL can be the target of a canonical 8600 * reference. It SHALL remain the same when the capability statement is 8601 * stored on different servers.). This is the underlying object with id, 8602 * value and extensions. The accessor "getUrl" gives direct access to 8603 * the value 8604 */ 8605 public UriType getUrlElement() { 8606 if (this.url == null) 8607 if (Configuration.errorOnAutoCreate()) 8608 throw new Error("Attempt to auto-create CapabilityStatement.url"); 8609 else if (Configuration.doAutoCreate()) 8610 this.url = new UriType(); // bb 8611 return this.url; 8612 } 8613 8614 public boolean hasUrlElement() { 8615 return this.url != null && !this.url.isEmpty(); 8616 } 8617 8618 public boolean hasUrl() { 8619 return this.url != null && !this.url.isEmpty(); 8620 } 8621 8622 /** 8623 * @param value {@link #url} (An absolute URI that is used to identify this 8624 * capability statement when it is referenced in a specification, 8625 * model, design or an instance; also called its canonical 8626 * identifier. This SHOULD be globally unique and SHOULD be a 8627 * literal address at which at which an authoritative instance of 8628 * this capability statement is (or will be) published. This URL 8629 * can be the target of a canonical reference. It SHALL remain the 8630 * same when the capability statement is stored on different 8631 * servers.). This is the underlying object with id, value and 8632 * extensions. The accessor "getUrl" gives direct access to the 8633 * value 8634 */ 8635 public CapabilityStatement setUrlElement(UriType value) { 8636 this.url = value; 8637 return this; 8638 } 8639 8640 /** 8641 * @return An absolute URI that is used to identify this capability statement 8642 * when it is referenced in a specification, model, design or an 8643 * instance; also called its canonical identifier. This SHOULD be 8644 * globally unique and SHOULD be a literal address at which at which an 8645 * authoritative instance of this capability statement is (or will be) 8646 * published. This URL can be the target of a canonical reference. It 8647 * SHALL remain the same when the capability statement is stored on 8648 * different servers. 8649 */ 8650 public String getUrl() { 8651 return this.url == null ? null : this.url.getValue(); 8652 } 8653 8654 /** 8655 * @param value An absolute URI that is used to identify this capability 8656 * statement when it is referenced in a specification, model, 8657 * design or an instance; also called its canonical identifier. 8658 * This SHOULD be globally unique and SHOULD be a literal address 8659 * at which at which an authoritative instance of this capability 8660 * statement is (or will be) published. This URL can be the target 8661 * of a canonical reference. It SHALL remain the same when the 8662 * capability statement is stored on different servers. 8663 */ 8664 public CapabilityStatement setUrl(String value) { 8665 if (Utilities.noString(value)) 8666 this.url = null; 8667 else { 8668 if (this.url == null) 8669 this.url = new UriType(); 8670 this.url.setValue(value); 8671 } 8672 return this; 8673 } 8674 8675 /** 8676 * @return {@link #version} (The identifier that is used to identify this 8677 * version of the capability statement when it is referenced in a 8678 * specification, model, design or instance. This is an arbitrary value 8679 * managed by the capability statement author and is not expected to be 8680 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 8681 * if a managed version is not available. There is also no expectation 8682 * that versions can be placed in a lexicographical sequence.). This is 8683 * the underlying object with id, value and extensions. The accessor 8684 * "getVersion" gives direct access to the value 8685 */ 8686 public StringType getVersionElement() { 8687 if (this.version == null) 8688 if (Configuration.errorOnAutoCreate()) 8689 throw new Error("Attempt to auto-create CapabilityStatement.version"); 8690 else if (Configuration.doAutoCreate()) 8691 this.version = new StringType(); // bb 8692 return this.version; 8693 } 8694 8695 public boolean hasVersionElement() { 8696 return this.version != null && !this.version.isEmpty(); 8697 } 8698 8699 public boolean hasVersion() { 8700 return this.version != null && !this.version.isEmpty(); 8701 } 8702 8703 /** 8704 * @param value {@link #version} (The identifier that is used to identify this 8705 * version of the capability statement when it is referenced in a 8706 * specification, model, design or instance. This is an arbitrary 8707 * value managed by the capability statement author and is not 8708 * expected to be globally unique. For example, it might be a 8709 * timestamp (e.g. yyyymmdd) if a managed version is not available. 8710 * There is also no expectation that versions can be placed in a 8711 * lexicographical sequence.). This is the underlying object with 8712 * id, value and extensions. The accessor "getVersion" gives direct 8713 * access to the value 8714 */ 8715 public CapabilityStatement setVersionElement(StringType value) { 8716 this.version = value; 8717 return this; 8718 } 8719 8720 /** 8721 * @return The identifier that is used to identify this version of the 8722 * capability statement when it is referenced in a specification, model, 8723 * design or instance. This is an arbitrary value managed by the 8724 * capability statement author and is not expected to be globally 8725 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 8726 * managed version is not available. There is also no expectation that 8727 * versions can be placed in a lexicographical sequence. 8728 */ 8729 public String getVersion() { 8730 return this.version == null ? null : this.version.getValue(); 8731 } 8732 8733 /** 8734 * @param value The identifier that is used to identify this version of the 8735 * capability statement when it is referenced in a specification, 8736 * model, design or instance. This is an arbitrary value managed by 8737 * the capability statement author and is not expected to be 8738 * globally unique. For example, it might be a timestamp (e.g. 8739 * yyyymmdd) if a managed version is not available. There is also 8740 * no expectation that versions can be placed in a lexicographical 8741 * sequence. 8742 */ 8743 public CapabilityStatement setVersion(String value) { 8744 if (Utilities.noString(value)) 8745 this.version = null; 8746 else { 8747 if (this.version == null) 8748 this.version = new StringType(); 8749 this.version.setValue(value); 8750 } 8751 return this; 8752 } 8753 8754 /** 8755 * @return {@link #name} (A natural language name identifying the capability 8756 * statement. This name should be usable as an identifier for the module 8757 * by machine processing applications such as code generation.). This is 8758 * the underlying object with id, value and extensions. The accessor 8759 * "getName" gives direct access to the value 8760 */ 8761 public StringType getNameElement() { 8762 if (this.name == null) 8763 if (Configuration.errorOnAutoCreate()) 8764 throw new Error("Attempt to auto-create CapabilityStatement.name"); 8765 else if (Configuration.doAutoCreate()) 8766 this.name = new StringType(); // bb 8767 return this.name; 8768 } 8769 8770 public boolean hasNameElement() { 8771 return this.name != null && !this.name.isEmpty(); 8772 } 8773 8774 public boolean hasName() { 8775 return this.name != null && !this.name.isEmpty(); 8776 } 8777 8778 /** 8779 * @param value {@link #name} (A natural language name identifying the 8780 * capability statement. This name should be usable as an 8781 * identifier for the module by machine processing applications 8782 * such as code generation.). This is the underlying object with 8783 * id, value and extensions. The accessor "getName" gives direct 8784 * access to the value 8785 */ 8786 public CapabilityStatement setNameElement(StringType value) { 8787 this.name = value; 8788 return this; 8789 } 8790 8791 /** 8792 * @return A natural language name identifying the capability statement. This 8793 * name should be usable as an identifier for the module by machine 8794 * processing applications such as code generation. 8795 */ 8796 public String getName() { 8797 return this.name == null ? null : this.name.getValue(); 8798 } 8799 8800 /** 8801 * @param value A natural language name identifying the capability statement. 8802 * This name should be usable as an identifier for the module by 8803 * machine processing applications such as code generation. 8804 */ 8805 public CapabilityStatement setName(String value) { 8806 if (Utilities.noString(value)) 8807 this.name = null; 8808 else { 8809 if (this.name == null) 8810 this.name = new StringType(); 8811 this.name.setValue(value); 8812 } 8813 return this; 8814 } 8815 8816 /** 8817 * @return {@link #title} (A short, descriptive, user-friendly title for the 8818 * capability statement.). This is the underlying object with id, value 8819 * and extensions. The accessor "getTitle" gives direct access to the 8820 * value 8821 */ 8822 public StringType getTitleElement() { 8823 if (this.title == null) 8824 if (Configuration.errorOnAutoCreate()) 8825 throw new Error("Attempt to auto-create CapabilityStatement.title"); 8826 else if (Configuration.doAutoCreate()) 8827 this.title = new StringType(); // bb 8828 return this.title; 8829 } 8830 8831 public boolean hasTitleElement() { 8832 return this.title != null && !this.title.isEmpty(); 8833 } 8834 8835 public boolean hasTitle() { 8836 return this.title != null && !this.title.isEmpty(); 8837 } 8838 8839 /** 8840 * @param value {@link #title} (A short, descriptive, user-friendly title for 8841 * the capability statement.). This is the underlying object with 8842 * id, value and extensions. The accessor "getTitle" gives direct 8843 * access to the value 8844 */ 8845 public CapabilityStatement setTitleElement(StringType value) { 8846 this.title = value; 8847 return this; 8848 } 8849 8850 /** 8851 * @return A short, descriptive, user-friendly title for the capability 8852 * statement. 8853 */ 8854 public String getTitle() { 8855 return this.title == null ? null : this.title.getValue(); 8856 } 8857 8858 /** 8859 * @param value A short, descriptive, user-friendly title for the capability 8860 * statement. 8861 */ 8862 public CapabilityStatement setTitle(String value) { 8863 if (Utilities.noString(value)) 8864 this.title = null; 8865 else { 8866 if (this.title == null) 8867 this.title = new StringType(); 8868 this.title.setValue(value); 8869 } 8870 return this; 8871 } 8872 8873 /** 8874 * @return {@link #status} (The status of this capability statement. Enables 8875 * tracking the life-cycle of the content.). This is the underlying 8876 * object with id, value and extensions. The accessor "getStatus" gives 8877 * direct access to the value 8878 */ 8879 public Enumeration<PublicationStatus> getStatusElement() { 8880 if (this.status == null) 8881 if (Configuration.errorOnAutoCreate()) 8882 throw new Error("Attempt to auto-create CapabilityStatement.status"); 8883 else if (Configuration.doAutoCreate()) 8884 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 8885 return this.status; 8886 } 8887 8888 public boolean hasStatusElement() { 8889 return this.status != null && !this.status.isEmpty(); 8890 } 8891 8892 public boolean hasStatus() { 8893 return this.status != null && !this.status.isEmpty(); 8894 } 8895 8896 /** 8897 * @param value {@link #status} (The status of this capability statement. 8898 * Enables tracking the life-cycle of the content.). This is the 8899 * underlying object with id, value and extensions. The accessor 8900 * "getStatus" gives direct access to the value 8901 */ 8902 public CapabilityStatement setStatusElement(Enumeration<PublicationStatus> value) { 8903 this.status = value; 8904 return this; 8905 } 8906 8907 /** 8908 * @return The status of this capability statement. Enables tracking the 8909 * life-cycle of the content. 8910 */ 8911 public PublicationStatus getStatus() { 8912 return this.status == null ? null : this.status.getValue(); 8913 } 8914 8915 /** 8916 * @param value The status of this capability statement. Enables tracking the 8917 * life-cycle of the content. 8918 */ 8919 public CapabilityStatement setStatus(PublicationStatus value) { 8920 if (this.status == null) 8921 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 8922 this.status.setValue(value); 8923 return this; 8924 } 8925 8926 /** 8927 * @return {@link #experimental} (A Boolean value to indicate that this 8928 * capability statement is authored for testing purposes (or 8929 * education/evaluation/marketing) and is not intended to be used for 8930 * genuine usage.). This is the underlying object with id, value and 8931 * extensions. The accessor "getExperimental" gives direct access to the 8932 * value 8933 */ 8934 public BooleanType getExperimentalElement() { 8935 if (this.experimental == null) 8936 if (Configuration.errorOnAutoCreate()) 8937 throw new Error("Attempt to auto-create CapabilityStatement.experimental"); 8938 else if (Configuration.doAutoCreate()) 8939 this.experimental = new BooleanType(); // bb 8940 return this.experimental; 8941 } 8942 8943 public boolean hasExperimentalElement() { 8944 return this.experimental != null && !this.experimental.isEmpty(); 8945 } 8946 8947 public boolean hasExperimental() { 8948 return this.experimental != null && !this.experimental.isEmpty(); 8949 } 8950 8951 /** 8952 * @param value {@link #experimental} (A Boolean value to indicate that this 8953 * capability statement is authored for testing purposes (or 8954 * education/evaluation/marketing) and is not intended to be used 8955 * for genuine usage.). This is the underlying object with id, 8956 * value and extensions. The accessor "getExperimental" gives 8957 * direct access to the value 8958 */ 8959 public CapabilityStatement setExperimentalElement(BooleanType value) { 8960 this.experimental = value; 8961 return this; 8962 } 8963 8964 /** 8965 * @return A Boolean value to indicate that this capability statement is 8966 * authored for testing purposes (or education/evaluation/marketing) and 8967 * is not intended to be used for genuine usage. 8968 */ 8969 public boolean getExperimental() { 8970 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 8971 } 8972 8973 /** 8974 * @param value A Boolean value to indicate that this capability statement is 8975 * authored for testing purposes (or 8976 * education/evaluation/marketing) and is not intended to be used 8977 * for genuine usage. 8978 */ 8979 public CapabilityStatement setExperimental(boolean value) { 8980 if (this.experimental == null) 8981 this.experimental = new BooleanType(); 8982 this.experimental.setValue(value); 8983 return this; 8984 } 8985 8986 /** 8987 * @return {@link #date} (The date (and optionally time) when the capability 8988 * statement was published. The date must change when the business 8989 * version changes and it must change if the status code changes. In 8990 * addition, it should change when the substantive content of the 8991 * capability statement changes.). This is the underlying object with 8992 * id, value and extensions. The accessor "getDate" gives direct access 8993 * to the value 8994 */ 8995 public DateTimeType getDateElement() { 8996 if (this.date == null) 8997 if (Configuration.errorOnAutoCreate()) 8998 throw new Error("Attempt to auto-create CapabilityStatement.date"); 8999 else if (Configuration.doAutoCreate()) 9000 this.date = new DateTimeType(); // bb 9001 return this.date; 9002 } 9003 9004 public boolean hasDateElement() { 9005 return this.date != null && !this.date.isEmpty(); 9006 } 9007 9008 public boolean hasDate() { 9009 return this.date != null && !this.date.isEmpty(); 9010 } 9011 9012 /** 9013 * @param value {@link #date} (The date (and optionally time) when the 9014 * capability statement was published. The date must change when 9015 * the business version changes and it must change if the status 9016 * code changes. In addition, it should change when the substantive 9017 * content of the capability statement changes.). This is the 9018 * underlying object with id, value and extensions. The accessor 9019 * "getDate" gives direct access to the value 9020 */ 9021 public CapabilityStatement setDateElement(DateTimeType value) { 9022 this.date = value; 9023 return this; 9024 } 9025 9026 /** 9027 * @return The date (and optionally time) when the capability statement was 9028 * published. The date must change when the business version changes and 9029 * it must change if the status code changes. In addition, it should 9030 * change when the substantive content of the capability statement 9031 * changes. 9032 */ 9033 public Date getDate() { 9034 return this.date == null ? null : this.date.getValue(); 9035 } 9036 9037 /** 9038 * @param value The date (and optionally time) when the capability statement was 9039 * published. The date must change when the business version 9040 * changes and it must change if the status code changes. In 9041 * addition, it should change when the substantive content of the 9042 * capability statement changes. 9043 */ 9044 public CapabilityStatement setDate(Date value) { 9045 if (this.date == null) 9046 this.date = new DateTimeType(); 9047 this.date.setValue(value); 9048 return this; 9049 } 9050 9051 /** 9052 * @return {@link #publisher} (The name of the organization or individual that 9053 * published the capability statement.). This is the underlying object 9054 * with id, value and extensions. The accessor "getPublisher" gives 9055 * direct access to the value 9056 */ 9057 public StringType getPublisherElement() { 9058 if (this.publisher == null) 9059 if (Configuration.errorOnAutoCreate()) 9060 throw new Error("Attempt to auto-create CapabilityStatement.publisher"); 9061 else if (Configuration.doAutoCreate()) 9062 this.publisher = new StringType(); // bb 9063 return this.publisher; 9064 } 9065 9066 public boolean hasPublisherElement() { 9067 return this.publisher != null && !this.publisher.isEmpty(); 9068 } 9069 9070 public boolean hasPublisher() { 9071 return this.publisher != null && !this.publisher.isEmpty(); 9072 } 9073 9074 /** 9075 * @param value {@link #publisher} (The name of the organization or individual 9076 * that published the capability statement.). This is the 9077 * underlying object with id, value and extensions. The accessor 9078 * "getPublisher" gives direct access to the value 9079 */ 9080 public CapabilityStatement setPublisherElement(StringType value) { 9081 this.publisher = value; 9082 return this; 9083 } 9084 9085 /** 9086 * @return The name of the organization or individual that published the 9087 * capability statement. 9088 */ 9089 public String getPublisher() { 9090 return this.publisher == null ? null : this.publisher.getValue(); 9091 } 9092 9093 /** 9094 * @param value The name of the organization or individual that published the 9095 * capability statement. 9096 */ 9097 public CapabilityStatement setPublisher(String value) { 9098 if (Utilities.noString(value)) 9099 this.publisher = null; 9100 else { 9101 if (this.publisher == null) 9102 this.publisher = new StringType(); 9103 this.publisher.setValue(value); 9104 } 9105 return this; 9106 } 9107 9108 /** 9109 * @return {@link #contact} (Contact details to assist a user in finding and 9110 * communicating with the publisher.) 9111 */ 9112 public List<ContactDetail> getContact() { 9113 if (this.contact == null) 9114 this.contact = new ArrayList<ContactDetail>(); 9115 return this.contact; 9116 } 9117 9118 /** 9119 * @return Returns a reference to <code>this</code> for easy method chaining 9120 */ 9121 public CapabilityStatement setContact(List<ContactDetail> theContact) { 9122 this.contact = theContact; 9123 return this; 9124 } 9125 9126 public boolean hasContact() { 9127 if (this.contact == null) 9128 return false; 9129 for (ContactDetail item : this.contact) 9130 if (!item.isEmpty()) 9131 return true; 9132 return false; 9133 } 9134 9135 public ContactDetail addContact() { // 3 9136 ContactDetail t = new ContactDetail(); 9137 if (this.contact == null) 9138 this.contact = new ArrayList<ContactDetail>(); 9139 this.contact.add(t); 9140 return t; 9141 } 9142 9143 public CapabilityStatement addContact(ContactDetail t) { // 3 9144 if (t == null) 9145 return this; 9146 if (this.contact == null) 9147 this.contact = new ArrayList<ContactDetail>(); 9148 this.contact.add(t); 9149 return this; 9150 } 9151 9152 /** 9153 * @return The first repetition of repeating field {@link #contact}, creating it 9154 * if it does not already exist 9155 */ 9156 public ContactDetail getContactFirstRep() { 9157 if (getContact().isEmpty()) { 9158 addContact(); 9159 } 9160 return getContact().get(0); 9161 } 9162 9163 /** 9164 * @return {@link #description} (A free text natural language description of the 9165 * capability statement from a consumer's perspective. Typically, this 9166 * is used when the capability statement describes a desired rather than 9167 * an actual solution, for example as a formal expression of 9168 * requirements as part of an RFP.). This is the underlying object with 9169 * id, value and extensions. The accessor "getDescription" gives direct 9170 * access to the value 9171 */ 9172 public MarkdownType getDescriptionElement() { 9173 if (this.description == null) 9174 if (Configuration.errorOnAutoCreate()) 9175 throw new Error("Attempt to auto-create CapabilityStatement.description"); 9176 else if (Configuration.doAutoCreate()) 9177 this.description = new MarkdownType(); // bb 9178 return this.description; 9179 } 9180 9181 public boolean hasDescriptionElement() { 9182 return this.description != null && !this.description.isEmpty(); 9183 } 9184 9185 public boolean hasDescription() { 9186 return this.description != null && !this.description.isEmpty(); 9187 } 9188 9189 /** 9190 * @param value {@link #description} (A free text natural language description 9191 * of the capability statement from a consumer's perspective. 9192 * Typically, this is used when the capability statement describes 9193 * a desired rather than an actual solution, for example as a 9194 * formal expression of requirements as part of an RFP.). This is 9195 * the underlying object with id, value and extensions. The 9196 * accessor "getDescription" gives direct access to the value 9197 */ 9198 public CapabilityStatement setDescriptionElement(MarkdownType value) { 9199 this.description = value; 9200 return this; 9201 } 9202 9203 /** 9204 * @return A free text natural language description of the capability statement 9205 * from a consumer's perspective. Typically, this is used when the 9206 * capability statement describes a desired rather than an actual 9207 * solution, for example as a formal expression of requirements as part 9208 * of an RFP. 9209 */ 9210 public String getDescription() { 9211 return this.description == null ? null : this.description.getValue(); 9212 } 9213 9214 /** 9215 * @param value A free text natural language description of the capability 9216 * statement from a consumer's perspective. Typically, this is used 9217 * when the capability statement describes a desired rather than an 9218 * actual solution, for example as a formal expression of 9219 * requirements as part of an RFP. 9220 */ 9221 public CapabilityStatement setDescription(String value) { 9222 if (value == null) 9223 this.description = null; 9224 else { 9225 if (this.description == null) 9226 this.description = new MarkdownType(); 9227 this.description.setValue(value); 9228 } 9229 return this; 9230 } 9231 9232 /** 9233 * @return {@link #useContext} (The content was developed with a focus and 9234 * intent of supporting the contexts that are listed. These contexts may 9235 * be general categories (gender, age, ...) or may be references to 9236 * specific programs (insurance plans, studies, ...) and may be used to 9237 * assist with indexing and searching for appropriate capability 9238 * statement instances.) 9239 */ 9240 public List<UsageContext> getUseContext() { 9241 if (this.useContext == null) 9242 this.useContext = new ArrayList<UsageContext>(); 9243 return this.useContext; 9244 } 9245 9246 /** 9247 * @return Returns a reference to <code>this</code> for easy method chaining 9248 */ 9249 public CapabilityStatement setUseContext(List<UsageContext> theUseContext) { 9250 this.useContext = theUseContext; 9251 return this; 9252 } 9253 9254 public boolean hasUseContext() { 9255 if (this.useContext == null) 9256 return false; 9257 for (UsageContext item : this.useContext) 9258 if (!item.isEmpty()) 9259 return true; 9260 return false; 9261 } 9262 9263 public UsageContext addUseContext() { // 3 9264 UsageContext t = new UsageContext(); 9265 if (this.useContext == null) 9266 this.useContext = new ArrayList<UsageContext>(); 9267 this.useContext.add(t); 9268 return t; 9269 } 9270 9271 public CapabilityStatement addUseContext(UsageContext t) { // 3 9272 if (t == null) 9273 return this; 9274 if (this.useContext == null) 9275 this.useContext = new ArrayList<UsageContext>(); 9276 this.useContext.add(t); 9277 return this; 9278 } 9279 9280 /** 9281 * @return The first repetition of repeating field {@link #useContext}, creating 9282 * it if it does not already exist 9283 */ 9284 public UsageContext getUseContextFirstRep() { 9285 if (getUseContext().isEmpty()) { 9286 addUseContext(); 9287 } 9288 return getUseContext().get(0); 9289 } 9290 9291 /** 9292 * @return {@link #jurisdiction} (A legal or geographic region in which the 9293 * capability statement is intended to be used.) 9294 */ 9295 public List<CodeableConcept> getJurisdiction() { 9296 if (this.jurisdiction == null) 9297 this.jurisdiction = new ArrayList<CodeableConcept>(); 9298 return this.jurisdiction; 9299 } 9300 9301 /** 9302 * @return Returns a reference to <code>this</code> for easy method chaining 9303 */ 9304 public CapabilityStatement setJurisdiction(List<CodeableConcept> theJurisdiction) { 9305 this.jurisdiction = theJurisdiction; 9306 return this; 9307 } 9308 9309 public boolean hasJurisdiction() { 9310 if (this.jurisdiction == null) 9311 return false; 9312 for (CodeableConcept item : this.jurisdiction) 9313 if (!item.isEmpty()) 9314 return true; 9315 return false; 9316 } 9317 9318 public CodeableConcept addJurisdiction() { // 3 9319 CodeableConcept t = new CodeableConcept(); 9320 if (this.jurisdiction == null) 9321 this.jurisdiction = new ArrayList<CodeableConcept>(); 9322 this.jurisdiction.add(t); 9323 return t; 9324 } 9325 9326 public CapabilityStatement addJurisdiction(CodeableConcept t) { // 3 9327 if (t == null) 9328 return this; 9329 if (this.jurisdiction == null) 9330 this.jurisdiction = new ArrayList<CodeableConcept>(); 9331 this.jurisdiction.add(t); 9332 return this; 9333 } 9334 9335 /** 9336 * @return The first repetition of repeating field {@link #jurisdiction}, 9337 * creating it if it does not already exist 9338 */ 9339 public CodeableConcept getJurisdictionFirstRep() { 9340 if (getJurisdiction().isEmpty()) { 9341 addJurisdiction(); 9342 } 9343 return getJurisdiction().get(0); 9344 } 9345 9346 /** 9347 * @return {@link #purpose} (Explanation of why this capability statement is 9348 * needed and why it has been designed as it has.). This is the 9349 * underlying object with id, value and extensions. The accessor 9350 * "getPurpose" gives direct access to the value 9351 */ 9352 public MarkdownType getPurposeElement() { 9353 if (this.purpose == null) 9354 if (Configuration.errorOnAutoCreate()) 9355 throw new Error("Attempt to auto-create CapabilityStatement.purpose"); 9356 else if (Configuration.doAutoCreate()) 9357 this.purpose = new MarkdownType(); // bb 9358 return this.purpose; 9359 } 9360 9361 public boolean hasPurposeElement() { 9362 return this.purpose != null && !this.purpose.isEmpty(); 9363 } 9364 9365 public boolean hasPurpose() { 9366 return this.purpose != null && !this.purpose.isEmpty(); 9367 } 9368 9369 /** 9370 * @param value {@link #purpose} (Explanation of why this capability statement 9371 * is needed and why it has been designed as it has.). This is the 9372 * underlying object with id, value and extensions. The accessor 9373 * "getPurpose" gives direct access to the value 9374 */ 9375 public CapabilityStatement setPurposeElement(MarkdownType value) { 9376 this.purpose = value; 9377 return this; 9378 } 9379 9380 /** 9381 * @return Explanation of why this capability statement is needed and why it has 9382 * been designed as it has. 9383 */ 9384 public String getPurpose() { 9385 return this.purpose == null ? null : this.purpose.getValue(); 9386 } 9387 9388 /** 9389 * @param value Explanation of why this capability statement is needed and why 9390 * it has been designed as it has. 9391 */ 9392 public CapabilityStatement setPurpose(String value) { 9393 if (value == null) 9394 this.purpose = null; 9395 else { 9396 if (this.purpose == null) 9397 this.purpose = new MarkdownType(); 9398 this.purpose.setValue(value); 9399 } 9400 return this; 9401 } 9402 9403 /** 9404 * @return {@link #copyright} (A copyright statement relating to the capability 9405 * statement and/or its contents. Copyright statements are generally 9406 * legal restrictions on the use and publishing of the capability 9407 * statement.). This is the underlying object with id, value and 9408 * extensions. The accessor "getCopyright" gives direct access to the 9409 * value 9410 */ 9411 public MarkdownType getCopyrightElement() { 9412 if (this.copyright == null) 9413 if (Configuration.errorOnAutoCreate()) 9414 throw new Error("Attempt to auto-create CapabilityStatement.copyright"); 9415 else if (Configuration.doAutoCreate()) 9416 this.copyright = new MarkdownType(); // bb 9417 return this.copyright; 9418 } 9419 9420 public boolean hasCopyrightElement() { 9421 return this.copyright != null && !this.copyright.isEmpty(); 9422 } 9423 9424 public boolean hasCopyright() { 9425 return this.copyright != null && !this.copyright.isEmpty(); 9426 } 9427 9428 /** 9429 * @param value {@link #copyright} (A copyright statement relating to the 9430 * capability statement and/or its contents. Copyright statements 9431 * are generally legal restrictions on the use and publishing of 9432 * the capability statement.). This is the underlying object with 9433 * id, value and extensions. The accessor "getCopyright" gives 9434 * direct access to the value 9435 */ 9436 public CapabilityStatement setCopyrightElement(MarkdownType value) { 9437 this.copyright = value; 9438 return this; 9439 } 9440 9441 /** 9442 * @return A copyright statement relating to the capability statement and/or its 9443 * contents. Copyright statements are generally legal restrictions on 9444 * the use and publishing of the capability statement. 9445 */ 9446 public String getCopyright() { 9447 return this.copyright == null ? null : this.copyright.getValue(); 9448 } 9449 9450 /** 9451 * @param value A copyright statement relating to the capability statement 9452 * and/or its contents. Copyright statements are generally legal 9453 * restrictions on the use and publishing of the capability 9454 * statement. 9455 */ 9456 public CapabilityStatement setCopyright(String value) { 9457 if (value == null) 9458 this.copyright = null; 9459 else { 9460 if (this.copyright == null) 9461 this.copyright = new MarkdownType(); 9462 this.copyright.setValue(value); 9463 } 9464 return this; 9465 } 9466 9467 /** 9468 * @return {@link #kind} (The way that this statement is intended to be used, to 9469 * describe an actual running instance of software, a particular product 9470 * (kind, not instance of software) or a class of implementation (e.g. a 9471 * desired purchase).). This is the underlying object with id, value and 9472 * extensions. The accessor "getKind" gives direct access to the value 9473 */ 9474 public Enumeration<CapabilityStatementKind> getKindElement() { 9475 if (this.kind == null) 9476 if (Configuration.errorOnAutoCreate()) 9477 throw new Error("Attempt to auto-create CapabilityStatement.kind"); 9478 else if (Configuration.doAutoCreate()) 9479 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); // bb 9480 return this.kind; 9481 } 9482 9483 public boolean hasKindElement() { 9484 return this.kind != null && !this.kind.isEmpty(); 9485 } 9486 9487 public boolean hasKind() { 9488 return this.kind != null && !this.kind.isEmpty(); 9489 } 9490 9491 /** 9492 * @param value {@link #kind} (The way that this statement is intended to be 9493 * used, to describe an actual running instance of software, a 9494 * particular product (kind, not instance of software) or a class 9495 * of implementation (e.g. a desired purchase).). This is the 9496 * underlying object with id, value and extensions. The accessor 9497 * "getKind" gives direct access to the value 9498 */ 9499 public CapabilityStatement setKindElement(Enumeration<CapabilityStatementKind> value) { 9500 this.kind = value; 9501 return this; 9502 } 9503 9504 /** 9505 * @return The way that this statement is intended to be used, to describe an 9506 * actual running instance of software, a particular product (kind, not 9507 * instance of software) or a class of implementation (e.g. a desired 9508 * purchase). 9509 */ 9510 public CapabilityStatementKind getKind() { 9511 return this.kind == null ? null : this.kind.getValue(); 9512 } 9513 9514 /** 9515 * @param value The way that this statement is intended to be used, to describe 9516 * an actual running instance of software, a particular product 9517 * (kind, not instance of software) or a class of implementation 9518 * (e.g. a desired purchase). 9519 */ 9520 public CapabilityStatement setKind(CapabilityStatementKind value) { 9521 if (this.kind == null) 9522 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); 9523 this.kind.setValue(value); 9524 return this; 9525 } 9526 9527 /** 9528 * @return {@link #instantiates} (Reference to a canonical URL of another 9529 * CapabilityStatement that this software implements. This capability 9530 * statement is a published API description that corresponds to a 9531 * business service. The server may actually implement a subset of the 9532 * capability statement it claims to implement, so the capability 9533 * statement must specify the full capability details.) 9534 */ 9535 public List<CanonicalType> getInstantiates() { 9536 if (this.instantiates == null) 9537 this.instantiates = new ArrayList<CanonicalType>(); 9538 return this.instantiates; 9539 } 9540 9541 /** 9542 * @return Returns a reference to <code>this</code> for easy method chaining 9543 */ 9544 public CapabilityStatement setInstantiates(List<CanonicalType> theInstantiates) { 9545 this.instantiates = theInstantiates; 9546 return this; 9547 } 9548 9549 public boolean hasInstantiates() { 9550 if (this.instantiates == null) 9551 return false; 9552 for (CanonicalType item : this.instantiates) 9553 if (!item.isEmpty()) 9554 return true; 9555 return false; 9556 } 9557 9558 /** 9559 * @return {@link #instantiates} (Reference to a canonical URL of another 9560 * CapabilityStatement that this software implements. This capability 9561 * statement is a published API description that corresponds to a 9562 * business service. The server may actually implement a subset of the 9563 * capability statement it claims to implement, so the capability 9564 * statement must specify the full capability details.) 9565 */ 9566 public CanonicalType addInstantiatesElement() {// 2 9567 CanonicalType t = new CanonicalType(); 9568 if (this.instantiates == null) 9569 this.instantiates = new ArrayList<CanonicalType>(); 9570 this.instantiates.add(t); 9571 return t; 9572 } 9573 9574 /** 9575 * @param value {@link #instantiates} (Reference to a canonical URL of another 9576 * CapabilityStatement that this software implements. This 9577 * capability statement is a published API description that 9578 * corresponds to a business service. The server may actually 9579 * implement a subset of the capability statement it claims to 9580 * implement, so the capability statement must specify the full 9581 * capability details.) 9582 */ 9583 public CapabilityStatement addInstantiates(String value) { // 1 9584 CanonicalType t = new CanonicalType(); 9585 t.setValue(value); 9586 if (this.instantiates == null) 9587 this.instantiates = new ArrayList<CanonicalType>(); 9588 this.instantiates.add(t); 9589 return this; 9590 } 9591 9592 /** 9593 * @param value {@link #instantiates} (Reference to a canonical URL of another 9594 * CapabilityStatement that this software implements. This 9595 * capability statement is a published API description that 9596 * corresponds to a business service. The server may actually 9597 * implement a subset of the capability statement it claims to 9598 * implement, so the capability statement must specify the full 9599 * capability details.) 9600 */ 9601 public boolean hasInstantiates(String value) { 9602 if (this.instantiates == null) 9603 return false; 9604 for (CanonicalType v : this.instantiates) 9605 if (v.getValue().equals(value)) // canonical(CapabilityStatement) 9606 return true; 9607 return false; 9608 } 9609 9610 /** 9611 * @return {@link #imports} (Reference to a canonical URL of another 9612 * CapabilityStatement that this software adds to. The capability 9613 * statement automatically includes everything in the other statement, 9614 * and it is not duplicated, though the server may repeat the same 9615 * resources, interactions and operations to add additional details to 9616 * them.) 9617 */ 9618 public List<CanonicalType> getImports() { 9619 if (this.imports == null) 9620 this.imports = new ArrayList<CanonicalType>(); 9621 return this.imports; 9622 } 9623 9624 /** 9625 * @return Returns a reference to <code>this</code> for easy method chaining 9626 */ 9627 public CapabilityStatement setImports(List<CanonicalType> theImports) { 9628 this.imports = theImports; 9629 return this; 9630 } 9631 9632 public boolean hasImports() { 9633 if (this.imports == null) 9634 return false; 9635 for (CanonicalType item : this.imports) 9636 if (!item.isEmpty()) 9637 return true; 9638 return false; 9639 } 9640 9641 /** 9642 * @return {@link #imports} (Reference to a canonical URL of another 9643 * CapabilityStatement that this software adds to. The capability 9644 * statement automatically includes everything in the other statement, 9645 * and it is not duplicated, though the server may repeat the same 9646 * resources, interactions and operations to add additional details to 9647 * them.) 9648 */ 9649 public CanonicalType addImportsElement() {// 2 9650 CanonicalType t = new CanonicalType(); 9651 if (this.imports == null) 9652 this.imports = new ArrayList<CanonicalType>(); 9653 this.imports.add(t); 9654 return t; 9655 } 9656 9657 /** 9658 * @param value {@link #imports} (Reference to a canonical URL of another 9659 * CapabilityStatement that this software adds to. The capability 9660 * statement automatically includes everything in the other 9661 * statement, and it is not duplicated, though the server may 9662 * repeat the same resources, interactions and operations to add 9663 * additional details to them.) 9664 */ 9665 public CapabilityStatement addImports(String value) { // 1 9666 CanonicalType t = new CanonicalType(); 9667 t.setValue(value); 9668 if (this.imports == null) 9669 this.imports = new ArrayList<CanonicalType>(); 9670 this.imports.add(t); 9671 return this; 9672 } 9673 9674 /** 9675 * @param value {@link #imports} (Reference to a canonical URL of another 9676 * CapabilityStatement that this software adds to. The capability 9677 * statement automatically includes everything in the other 9678 * statement, and it is not duplicated, though the server may 9679 * repeat the same resources, interactions and operations to add 9680 * additional details to them.) 9681 */ 9682 public boolean hasImports(String value) { 9683 if (this.imports == null) 9684 return false; 9685 for (CanonicalType v : this.imports) 9686 if (v.getValue().equals(value)) // canonical(CapabilityStatement) 9687 return true; 9688 return false; 9689 } 9690 9691 /** 9692 * @return {@link #software} (Software that is covered by this capability 9693 * statement. It is used when the capability statement describes the 9694 * capabilities of a particular software version, independent of an 9695 * installation.) 9696 */ 9697 public CapabilityStatementSoftwareComponent getSoftware() { 9698 if (this.software == null) 9699 if (Configuration.errorOnAutoCreate()) 9700 throw new Error("Attempt to auto-create CapabilityStatement.software"); 9701 else if (Configuration.doAutoCreate()) 9702 this.software = new CapabilityStatementSoftwareComponent(); // cc 9703 return this.software; 9704 } 9705 9706 public boolean hasSoftware() { 9707 return this.software != null && !this.software.isEmpty(); 9708 } 9709 9710 /** 9711 * @param value {@link #software} (Software that is covered by this capability 9712 * statement. It is used when the capability statement describes 9713 * the capabilities of a particular software version, independent 9714 * of an installation.) 9715 */ 9716 public CapabilityStatement setSoftware(CapabilityStatementSoftwareComponent value) { 9717 this.software = value; 9718 return this; 9719 } 9720 9721 /** 9722 * @return {@link #implementation} (Identifies a specific implementation 9723 * instance that is described by the capability statement - i.e. a 9724 * particular installation, rather than the capabilities of a software 9725 * program.) 9726 */ 9727 public CapabilityStatementImplementationComponent getImplementation() { 9728 if (this.implementation == null) 9729 if (Configuration.errorOnAutoCreate()) 9730 throw new Error("Attempt to auto-create CapabilityStatement.implementation"); 9731 else if (Configuration.doAutoCreate()) 9732 this.implementation = new CapabilityStatementImplementationComponent(); // cc 9733 return this.implementation; 9734 } 9735 9736 public boolean hasImplementation() { 9737 return this.implementation != null && !this.implementation.isEmpty(); 9738 } 9739 9740 /** 9741 * @param value {@link #implementation} (Identifies a specific implementation 9742 * instance that is described by the capability statement - i.e. a 9743 * particular installation, rather than the capabilities of a 9744 * software program.) 9745 */ 9746 public CapabilityStatement setImplementation(CapabilityStatementImplementationComponent value) { 9747 this.implementation = value; 9748 return this; 9749 } 9750 9751 /** 9752 * @return {@link #fhirVersion} (The version of the FHIR specification that this 9753 * CapabilityStatement describes (which SHALL be the same as the FHIR 9754 * version of the CapabilityStatement itself). There is no default 9755 * value.). This is the underlying object with id, value and extensions. 9756 * The accessor "getFhirVersion" gives direct access to the value 9757 */ 9758 public Enumeration<FHIRVersion> getFhirVersionElement() { 9759 if (this.fhirVersion == null) 9760 if (Configuration.errorOnAutoCreate()) 9761 throw new Error("Attempt to auto-create CapabilityStatement.fhirVersion"); 9762 else if (Configuration.doAutoCreate()) 9763 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); // bb 9764 return this.fhirVersion; 9765 } 9766 9767 public boolean hasFhirVersionElement() { 9768 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 9769 } 9770 9771 public boolean hasFhirVersion() { 9772 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 9773 } 9774 9775 /** 9776 * @param value {@link #fhirVersion} (The version of the FHIR specification that 9777 * this CapabilityStatement describes (which SHALL be the same as 9778 * the FHIR version of the CapabilityStatement itself). There is no 9779 * default value.). This is the underlying object with id, value 9780 * and extensions. The accessor "getFhirVersion" gives direct 9781 * access to the value 9782 */ 9783 public CapabilityStatement setFhirVersionElement(Enumeration<FHIRVersion> value) { 9784 this.fhirVersion = value; 9785 return this; 9786 } 9787 9788 /** 9789 * @return The version of the FHIR specification that this CapabilityStatement 9790 * describes (which SHALL be the same as the FHIR version of the 9791 * CapabilityStatement itself). There is no default value. 9792 */ 9793 public FHIRVersion getFhirVersion() { 9794 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 9795 } 9796 9797 /** 9798 * @param value The version of the FHIR specification that this 9799 * CapabilityStatement describes (which SHALL be the same as the 9800 * FHIR version of the CapabilityStatement itself). There is no 9801 * default value. 9802 */ 9803 public CapabilityStatement setFhirVersion(FHIRVersion value) { 9804 if (this.fhirVersion == null) 9805 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 9806 this.fhirVersion.setValue(value); 9807 return this; 9808 } 9809 9810 /** 9811 * @return {@link #format} (A list of the formats supported by this 9812 * implementation using their content types.) 9813 */ 9814 public List<CodeType> getFormat() { 9815 if (this.format == null) 9816 this.format = new ArrayList<CodeType>(); 9817 return this.format; 9818 } 9819 9820 /** 9821 * @return Returns a reference to <code>this</code> for easy method chaining 9822 */ 9823 public CapabilityStatement setFormat(List<CodeType> theFormat) { 9824 this.format = theFormat; 9825 return this; 9826 } 9827 9828 public boolean hasFormat() { 9829 if (this.format == null) 9830 return false; 9831 for (CodeType item : this.format) 9832 if (!item.isEmpty()) 9833 return true; 9834 return false; 9835 } 9836 9837 /** 9838 * @return {@link #format} (A list of the formats supported by this 9839 * implementation using their content types.) 9840 */ 9841 public CodeType addFormatElement() {// 2 9842 CodeType t = new CodeType(); 9843 if (this.format == null) 9844 this.format = new ArrayList<CodeType>(); 9845 this.format.add(t); 9846 return t; 9847 } 9848 9849 /** 9850 * @param value {@link #format} (A list of the formats supported by this 9851 * implementation using their content types.) 9852 */ 9853 public CapabilityStatement addFormat(String value) { // 1 9854 CodeType t = new CodeType(); 9855 t.setValue(value); 9856 if (this.format == null) 9857 this.format = new ArrayList<CodeType>(); 9858 this.format.add(t); 9859 return this; 9860 } 9861 9862 /** 9863 * @param value {@link #format} (A list of the formats supported by this 9864 * implementation using their content types.) 9865 */ 9866 public boolean hasFormat(String value) { 9867 if (this.format == null) 9868 return false; 9869 for (CodeType v : this.format) 9870 if (v.getValue().equals(value)) // code 9871 return true; 9872 return false; 9873 } 9874 9875 /** 9876 * @return {@link #patchFormat} (A list of the patch formats supported by this 9877 * implementation using their content types.) 9878 */ 9879 public List<CodeType> getPatchFormat() { 9880 if (this.patchFormat == null) 9881 this.patchFormat = new ArrayList<CodeType>(); 9882 return this.patchFormat; 9883 } 9884 9885 /** 9886 * @return Returns a reference to <code>this</code> for easy method chaining 9887 */ 9888 public CapabilityStatement setPatchFormat(List<CodeType> thePatchFormat) { 9889 this.patchFormat = thePatchFormat; 9890 return this; 9891 } 9892 9893 public boolean hasPatchFormat() { 9894 if (this.patchFormat == null) 9895 return false; 9896 for (CodeType item : this.patchFormat) 9897 if (!item.isEmpty()) 9898 return true; 9899 return false; 9900 } 9901 9902 /** 9903 * @return {@link #patchFormat} (A list of the patch formats supported by this 9904 * implementation using their content types.) 9905 */ 9906 public CodeType addPatchFormatElement() {// 2 9907 CodeType t = new CodeType(); 9908 if (this.patchFormat == null) 9909 this.patchFormat = new ArrayList<CodeType>(); 9910 this.patchFormat.add(t); 9911 return t; 9912 } 9913 9914 /** 9915 * @param value {@link #patchFormat} (A list of the patch formats supported by 9916 * this implementation using their content types.) 9917 */ 9918 public CapabilityStatement addPatchFormat(String value) { // 1 9919 CodeType t = new CodeType(); 9920 t.setValue(value); 9921 if (this.patchFormat == null) 9922 this.patchFormat = new ArrayList<CodeType>(); 9923 this.patchFormat.add(t); 9924 return this; 9925 } 9926 9927 /** 9928 * @param value {@link #patchFormat} (A list of the patch formats supported by 9929 * this implementation using their content types.) 9930 */ 9931 public boolean hasPatchFormat(String value) { 9932 if (this.patchFormat == null) 9933 return false; 9934 for (CodeType v : this.patchFormat) 9935 if (v.getValue().equals(value)) // code 9936 return true; 9937 return false; 9938 } 9939 9940 /** 9941 * @return {@link #implementationGuide} (A list of implementation guides that 9942 * the server does (or should) support in their entirety.) 9943 */ 9944 public List<CanonicalType> getImplementationGuide() { 9945 if (this.implementationGuide == null) 9946 this.implementationGuide = new ArrayList<CanonicalType>(); 9947 return this.implementationGuide; 9948 } 9949 9950 /** 9951 * @return Returns a reference to <code>this</code> for easy method chaining 9952 */ 9953 public CapabilityStatement setImplementationGuide(List<CanonicalType> theImplementationGuide) { 9954 this.implementationGuide = theImplementationGuide; 9955 return this; 9956 } 9957 9958 public boolean hasImplementationGuide() { 9959 if (this.implementationGuide == null) 9960 return false; 9961 for (CanonicalType item : this.implementationGuide) 9962 if (!item.isEmpty()) 9963 return true; 9964 return false; 9965 } 9966 9967 /** 9968 * @return {@link #implementationGuide} (A list of implementation guides that 9969 * the server does (or should) support in their entirety.) 9970 */ 9971 public CanonicalType addImplementationGuideElement() {// 2 9972 CanonicalType t = new CanonicalType(); 9973 if (this.implementationGuide == null) 9974 this.implementationGuide = new ArrayList<CanonicalType>(); 9975 this.implementationGuide.add(t); 9976 return t; 9977 } 9978 9979 /** 9980 * @param value {@link #implementationGuide} (A list of implementation guides 9981 * that the server does (or should) support in their entirety.) 9982 */ 9983 public CapabilityStatement addImplementationGuide(String value) { // 1 9984 CanonicalType t = new CanonicalType(); 9985 t.setValue(value); 9986 if (this.implementationGuide == null) 9987 this.implementationGuide = new ArrayList<CanonicalType>(); 9988 this.implementationGuide.add(t); 9989 return this; 9990 } 9991 9992 /** 9993 * @param value {@link #implementationGuide} (A list of implementation guides 9994 * that the server does (or should) support in their entirety.) 9995 */ 9996 public boolean hasImplementationGuide(String value) { 9997 if (this.implementationGuide == null) 9998 return false; 9999 for (CanonicalType v : this.implementationGuide) 10000 if (v.getValue().equals(value)) // canonical(ImplementationGuide) 10001 return true; 10002 return false; 10003 } 10004 10005 /** 10006 * @return {@link #rest} (A definition of the restful capabilities of the 10007 * solution, if any.) 10008 */ 10009 public List<CapabilityStatementRestComponent> getRest() { 10010 if (this.rest == null) 10011 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 10012 return this.rest; 10013 } 10014 10015 /** 10016 * @return Returns a reference to <code>this</code> for easy method chaining 10017 */ 10018 public CapabilityStatement setRest(List<CapabilityStatementRestComponent> theRest) { 10019 this.rest = theRest; 10020 return this; 10021 } 10022 10023 public boolean hasRest() { 10024 if (this.rest == null) 10025 return false; 10026 for (CapabilityStatementRestComponent item : this.rest) 10027 if (!item.isEmpty()) 10028 return true; 10029 return false; 10030 } 10031 10032 public CapabilityStatementRestComponent addRest() { // 3 10033 CapabilityStatementRestComponent t = new CapabilityStatementRestComponent(); 10034 if (this.rest == null) 10035 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 10036 this.rest.add(t); 10037 return t; 10038 } 10039 10040 public CapabilityStatement addRest(CapabilityStatementRestComponent t) { // 3 10041 if (t == null) 10042 return this; 10043 if (this.rest == null) 10044 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 10045 this.rest.add(t); 10046 return this; 10047 } 10048 10049 /** 10050 * @return The first repetition of repeating field {@link #rest}, creating it if 10051 * it does not already exist 10052 */ 10053 public CapabilityStatementRestComponent getRestFirstRep() { 10054 if (getRest().isEmpty()) { 10055 addRest(); 10056 } 10057 return getRest().get(0); 10058 } 10059 10060 /** 10061 * @return {@link #messaging} (A description of the messaging capabilities of 10062 * the solution.) 10063 */ 10064 public List<CapabilityStatementMessagingComponent> getMessaging() { 10065 if (this.messaging == null) 10066 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10067 return this.messaging; 10068 } 10069 10070 /** 10071 * @return Returns a reference to <code>this</code> for easy method chaining 10072 */ 10073 public CapabilityStatement setMessaging(List<CapabilityStatementMessagingComponent> theMessaging) { 10074 this.messaging = theMessaging; 10075 return this; 10076 } 10077 10078 public boolean hasMessaging() { 10079 if (this.messaging == null) 10080 return false; 10081 for (CapabilityStatementMessagingComponent item : this.messaging) 10082 if (!item.isEmpty()) 10083 return true; 10084 return false; 10085 } 10086 10087 public CapabilityStatementMessagingComponent addMessaging() { // 3 10088 CapabilityStatementMessagingComponent t = new CapabilityStatementMessagingComponent(); 10089 if (this.messaging == null) 10090 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10091 this.messaging.add(t); 10092 return t; 10093 } 10094 10095 public CapabilityStatement addMessaging(CapabilityStatementMessagingComponent t) { // 3 10096 if (t == null) 10097 return this; 10098 if (this.messaging == null) 10099 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10100 this.messaging.add(t); 10101 return this; 10102 } 10103 10104 /** 10105 * @return The first repetition of repeating field {@link #messaging}, creating 10106 * it if it does not already exist 10107 */ 10108 public CapabilityStatementMessagingComponent getMessagingFirstRep() { 10109 if (getMessaging().isEmpty()) { 10110 addMessaging(); 10111 } 10112 return getMessaging().get(0); 10113 } 10114 10115 /** 10116 * @return {@link #document} (A document definition.) 10117 */ 10118 public List<CapabilityStatementDocumentComponent> getDocument() { 10119 if (this.document == null) 10120 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10121 return this.document; 10122 } 10123 10124 /** 10125 * @return Returns a reference to <code>this</code> for easy method chaining 10126 */ 10127 public CapabilityStatement setDocument(List<CapabilityStatementDocumentComponent> theDocument) { 10128 this.document = theDocument; 10129 return this; 10130 } 10131 10132 public boolean hasDocument() { 10133 if (this.document == null) 10134 return false; 10135 for (CapabilityStatementDocumentComponent item : this.document) 10136 if (!item.isEmpty()) 10137 return true; 10138 return false; 10139 } 10140 10141 public CapabilityStatementDocumentComponent addDocument() { // 3 10142 CapabilityStatementDocumentComponent t = new CapabilityStatementDocumentComponent(); 10143 if (this.document == null) 10144 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10145 this.document.add(t); 10146 return t; 10147 } 10148 10149 public CapabilityStatement addDocument(CapabilityStatementDocumentComponent t) { // 3 10150 if (t == null) 10151 return this; 10152 if (this.document == null) 10153 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10154 this.document.add(t); 10155 return this; 10156 } 10157 10158 /** 10159 * @return The first repetition of repeating field {@link #document}, creating 10160 * it if it does not already exist 10161 */ 10162 public CapabilityStatementDocumentComponent getDocumentFirstRep() { 10163 if (getDocument().isEmpty()) { 10164 addDocument(); 10165 } 10166 return getDocument().get(0); 10167 } 10168 10169 protected void listChildren(List<Property> children) { 10170 super.listChildren(children); 10171 children.add(new Property("url", "uri", 10172 "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.", 10173 0, 1, url)); 10174 children.add(new Property("version", "string", 10175 "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 10176 0, 1, version)); 10177 children.add(new Property("name", "string", 10178 "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 10179 0, 1, name)); 10180 children.add(new Property("title", "string", 10181 "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title)); 10182 children.add(new Property("status", "code", 10183 "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status)); 10184 children.add(new Property("experimental", "boolean", 10185 "A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 10186 0, 1, experimental)); 10187 children.add(new Property("date", "dateTime", 10188 "The date (and optionally time) when the capability statement was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 10189 0, 1, date)); 10190 children.add(new Property("publisher", "string", 10191 "The name of the organization or individual that published the capability statement.", 0, 1, publisher)); 10192 children.add(new Property("contact", "ContactDetail", 10193 "Contact details to assist a user in finding and communicating with the publisher.", 0, 10194 java.lang.Integer.MAX_VALUE, contact)); 10195 children.add(new Property("description", "markdown", 10196 "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 10197 0, 1, description)); 10198 children.add(new Property("useContext", "UsageContext", 10199 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.", 10200 0, java.lang.Integer.MAX_VALUE, useContext)); 10201 children.add(new Property("jurisdiction", "CodeableConcept", 10202 "A legal or geographic region in which the capability statement is intended to be used.", 0, 10203 java.lang.Integer.MAX_VALUE, jurisdiction)); 10204 children.add(new Property("purpose", "markdown", 10205 "Explanation of why this capability statement is needed and why it has been designed as it has.", 0, 1, 10206 purpose)); 10207 children.add(new Property("copyright", "markdown", 10208 "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 10209 0, 1, copyright)); 10210 children.add(new Property("kind", "code", 10211 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 10212 0, 1, kind)); 10213 children.add(new Property("instantiates", "canonical(CapabilityStatement)", 10214 "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.", 10215 0, java.lang.Integer.MAX_VALUE, instantiates)); 10216 children.add(new Property("imports", "canonical(CapabilityStatement)", 10217 "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.", 10218 0, java.lang.Integer.MAX_VALUE, imports)); 10219 children.add(new Property("software", "", 10220 "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 10221 0, 1, software)); 10222 children.add(new Property("implementation", "", 10223 "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 10224 0, 1, implementation)); 10225 children.add(new Property("fhirVersion", "code", 10226 "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.", 10227 0, 1, fhirVersion)); 10228 children.add(new Property("format", "code", 10229 "A list of the formats supported by this implementation using their content types.", 0, 10230 java.lang.Integer.MAX_VALUE, format)); 10231 children.add(new Property("patchFormat", "code", 10232 "A list of the patch formats supported by this implementation using their content types.", 0, 10233 java.lang.Integer.MAX_VALUE, patchFormat)); 10234 children.add(new Property("implementationGuide", "canonical(ImplementationGuide)", 10235 "A list of implementation guides that the server does (or should) support in their entirety.", 0, 10236 java.lang.Integer.MAX_VALUE, implementationGuide)); 10237 children.add(new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, 10238 java.lang.Integer.MAX_VALUE, rest)); 10239 children.add(new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, 10240 java.lang.Integer.MAX_VALUE, messaging)); 10241 children.add(new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document)); 10242 } 10243 10244 @Override 10245 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10246 switch (_hash) { 10247 case 116079: 10248 /* url */ return new Property("url", "uri", 10249 "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.", 10250 0, 1, url); 10251 case 351608024: 10252 /* version */ return new Property("version", "string", 10253 "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 10254 0, 1, version); 10255 case 3373707: 10256 /* name */ return new Property("name", "string", 10257 "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 10258 0, 1, name); 10259 case 110371416: 10260 /* title */ return new Property("title", "string", 10261 "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title); 10262 case -892481550: 10263 /* status */ return new Property("status", "code", 10264 "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status); 10265 case -404562712: 10266 /* experimental */ return new Property("experimental", "boolean", 10267 "A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 10268 0, 1, experimental); 10269 case 3076014: 10270 /* date */ return new Property("date", "dateTime", 10271 "The date (and optionally time) when the capability statement was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 10272 0, 1, date); 10273 case 1447404028: 10274 /* publisher */ return new Property("publisher", "string", 10275 "The name of the organization or individual that published the capability statement.", 0, 1, publisher); 10276 case 951526432: 10277 /* contact */ return new Property("contact", "ContactDetail", 10278 "Contact details to assist a user in finding and communicating with the publisher.", 0, 10279 java.lang.Integer.MAX_VALUE, contact); 10280 case -1724546052: 10281 /* description */ return new Property("description", "markdown", 10282 "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 10283 0, 1, description); 10284 case -669707736: 10285 /* useContext */ return new Property("useContext", "UsageContext", 10286 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.", 10287 0, java.lang.Integer.MAX_VALUE, useContext); 10288 case -507075711: 10289 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 10290 "A legal or geographic region in which the capability statement is intended to be used.", 0, 10291 java.lang.Integer.MAX_VALUE, jurisdiction); 10292 case -220463842: 10293 /* purpose */ return new Property("purpose", "markdown", 10294 "Explanation of why this capability statement is needed and why it has been designed as it has.", 0, 1, 10295 purpose); 10296 case 1522889671: 10297 /* copyright */ return new Property("copyright", "markdown", 10298 "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 10299 0, 1, copyright); 10300 case 3292052: 10301 /* kind */ return new Property("kind", "code", 10302 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 10303 0, 1, kind); 10304 case -246883639: 10305 /* instantiates */ return new Property("instantiates", "canonical(CapabilityStatement)", 10306 "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.", 10307 0, java.lang.Integer.MAX_VALUE, instantiates); 10308 case 1926037870: 10309 /* imports */ return new Property("imports", "canonical(CapabilityStatement)", 10310 "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.", 10311 0, java.lang.Integer.MAX_VALUE, imports); 10312 case 1319330215: 10313 /* software */ return new Property("software", "", 10314 "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 10315 0, 1, software); 10316 case 1683336114: 10317 /* implementation */ return new Property("implementation", "", 10318 "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 10319 0, 1, implementation); 10320 case 461006061: 10321 /* fhirVersion */ return new Property("fhirVersion", "code", 10322 "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.", 10323 0, 1, fhirVersion); 10324 case -1268779017: 10325 /* format */ return new Property("format", "code", 10326 "A list of the formats supported by this implementation using their content types.", 0, 10327 java.lang.Integer.MAX_VALUE, format); 10328 case 172338783: 10329 /* patchFormat */ return new Property("patchFormat", "code", 10330 "A list of the patch formats supported by this implementation using their content types.", 0, 10331 java.lang.Integer.MAX_VALUE, patchFormat); 10332 case 156966506: 10333 /* implementationGuide */ return new Property("implementationGuide", "canonical(ImplementationGuide)", 10334 "A list of implementation guides that the server does (or should) support in their entirety.", 0, 10335 java.lang.Integer.MAX_VALUE, implementationGuide); 10336 case 3496916: 10337 /* rest */ return new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, 10338 java.lang.Integer.MAX_VALUE, rest); 10339 case -1440008444: 10340 /* messaging */ return new Property("messaging", "", 10341 "A description of the messaging capabilities of the solution.", 0, java.lang.Integer.MAX_VALUE, messaging); 10342 case 861720859: 10343 /* document */ return new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, 10344 document); 10345 default: 10346 return super.getNamedProperty(_hash, _name, _checkValid); 10347 } 10348 10349 } 10350 10351 @Override 10352 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10353 switch (hash) { 10354 case 116079: 10355 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 10356 case 351608024: 10357 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 10358 case 3373707: 10359 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 10360 case 110371416: 10361 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 10362 case -892481550: 10363 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 10364 case -404562712: 10365 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 10366 case 3076014: 10367 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 10368 case 1447404028: 10369 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 10370 case 951526432: 10371 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 10372 case -1724546052: 10373 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 10374 case -669707736: 10375 /* useContext */ return this.useContext == null ? new Base[0] 10376 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 10377 case -507075711: 10378 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 10379 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 10380 case -220463842: 10381 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 10382 case 1522889671: 10383 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 10384 case 3292052: 10385 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<CapabilityStatementKind> 10386 case -246883639: 10387 /* instantiates */ return this.instantiates == null ? new Base[0] 10388 : this.instantiates.toArray(new Base[this.instantiates.size()]); // CanonicalType 10389 case 1926037870: 10390 /* imports */ return this.imports == null ? new Base[0] : this.imports.toArray(new Base[this.imports.size()]); // CanonicalType 10391 case 1319330215: 10392 /* software */ return this.software == null ? new Base[0] : new Base[] { this.software }; // CapabilityStatementSoftwareComponent 10393 case 1683336114: 10394 /* implementation */ return this.implementation == null ? new Base[0] : new Base[] { this.implementation }; // CapabilityStatementImplementationComponent 10395 case 461006061: 10396 /* fhirVersion */ return this.fhirVersion == null ? new Base[0] : new Base[] { this.fhirVersion }; // Enumeration<FHIRVersion> 10397 case -1268779017: 10398 /* format */ return this.format == null ? new Base[0] : this.format.toArray(new Base[this.format.size()]); // CodeType 10399 case 172338783: 10400 /* patchFormat */ return this.patchFormat == null ? new Base[0] 10401 : this.patchFormat.toArray(new Base[this.patchFormat.size()]); // CodeType 10402 case 156966506: 10403 /* implementationGuide */ return this.implementationGuide == null ? new Base[0] 10404 : this.implementationGuide.toArray(new Base[this.implementationGuide.size()]); // CanonicalType 10405 case 3496916: 10406 /* rest */ return this.rest == null ? new Base[0] : this.rest.toArray(new Base[this.rest.size()]); // CapabilityStatementRestComponent 10407 case -1440008444: 10408 /* messaging */ return this.messaging == null ? new Base[0] 10409 : this.messaging.toArray(new Base[this.messaging.size()]); // CapabilityStatementMessagingComponent 10410 case 861720859: 10411 /* document */ return this.document == null ? new Base[0] : this.document.toArray(new Base[this.document.size()]); // CapabilityStatementDocumentComponent 10412 default: 10413 return super.getProperty(hash, name, checkValid); 10414 } 10415 10416 } 10417 10418 @Override 10419 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10420 switch (hash) { 10421 case 116079: // url 10422 this.url = castToUri(value); // UriType 10423 return value; 10424 case 351608024: // version 10425 this.version = castToString(value); // StringType 10426 return value; 10427 case 3373707: // name 10428 this.name = castToString(value); // StringType 10429 return value; 10430 case 110371416: // title 10431 this.title = castToString(value); // StringType 10432 return value; 10433 case -892481550: // status 10434 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 10435 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10436 return value; 10437 case -404562712: // experimental 10438 this.experimental = castToBoolean(value); // BooleanType 10439 return value; 10440 case 3076014: // date 10441 this.date = castToDateTime(value); // DateTimeType 10442 return value; 10443 case 1447404028: // publisher 10444 this.publisher = castToString(value); // StringType 10445 return value; 10446 case 951526432: // contact 10447 this.getContact().add(castToContactDetail(value)); // ContactDetail 10448 return value; 10449 case -1724546052: // description 10450 this.description = castToMarkdown(value); // MarkdownType 10451 return value; 10452 case -669707736: // useContext 10453 this.getUseContext().add(castToUsageContext(value)); // UsageContext 10454 return value; 10455 case -507075711: // jurisdiction 10456 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 10457 return value; 10458 case -220463842: // purpose 10459 this.purpose = castToMarkdown(value); // MarkdownType 10460 return value; 10461 case 1522889671: // copyright 10462 this.copyright = castToMarkdown(value); // MarkdownType 10463 return value; 10464 case 3292052: // kind 10465 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 10466 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 10467 return value; 10468 case -246883639: // instantiates 10469 this.getInstantiates().add(castToCanonical(value)); // CanonicalType 10470 return value; 10471 case 1926037870: // imports 10472 this.getImports().add(castToCanonical(value)); // CanonicalType 10473 return value; 10474 case 1319330215: // software 10475 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 10476 return value; 10477 case 1683336114: // implementation 10478 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 10479 return value; 10480 case 461006061: // fhirVersion 10481 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 10482 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 10483 return value; 10484 case -1268779017: // format 10485 this.getFormat().add(castToCode(value)); // CodeType 10486 return value; 10487 case 172338783: // patchFormat 10488 this.getPatchFormat().add(castToCode(value)); // CodeType 10489 return value; 10490 case 156966506: // implementationGuide 10491 this.getImplementationGuide().add(castToCanonical(value)); // CanonicalType 10492 return value; 10493 case 3496916: // rest 10494 this.getRest().add((CapabilityStatementRestComponent) value); // CapabilityStatementRestComponent 10495 return value; 10496 case -1440008444: // messaging 10497 this.getMessaging().add((CapabilityStatementMessagingComponent) value); // CapabilityStatementMessagingComponent 10498 return value; 10499 case 861720859: // document 10500 this.getDocument().add((CapabilityStatementDocumentComponent) value); // CapabilityStatementDocumentComponent 10501 return value; 10502 default: 10503 return super.setProperty(hash, name, value); 10504 } 10505 10506 } 10507 10508 @Override 10509 public Base setProperty(String name, Base value) throws FHIRException { 10510 if (name.equals("url")) { 10511 this.url = castToUri(value); // UriType 10512 } else if (name.equals("version")) { 10513 this.version = castToString(value); // StringType 10514 } else if (name.equals("name")) { 10515 this.name = castToString(value); // StringType 10516 } else if (name.equals("title")) { 10517 this.title = castToString(value); // StringType 10518 } else if (name.equals("status")) { 10519 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 10520 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10521 } else if (name.equals("experimental")) { 10522 this.experimental = castToBoolean(value); // BooleanType 10523 } else if (name.equals("date")) { 10524 this.date = castToDateTime(value); // DateTimeType 10525 } else if (name.equals("publisher")) { 10526 this.publisher = castToString(value); // StringType 10527 } else if (name.equals("contact")) { 10528 this.getContact().add(castToContactDetail(value)); 10529 } else if (name.equals("description")) { 10530 this.description = castToMarkdown(value); // MarkdownType 10531 } else if (name.equals("useContext")) { 10532 this.getUseContext().add(castToUsageContext(value)); 10533 } else if (name.equals("jurisdiction")) { 10534 this.getJurisdiction().add(castToCodeableConcept(value)); 10535 } else if (name.equals("purpose")) { 10536 this.purpose = castToMarkdown(value); // MarkdownType 10537 } else if (name.equals("copyright")) { 10538 this.copyright = castToMarkdown(value); // MarkdownType 10539 } else if (name.equals("kind")) { 10540 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 10541 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 10542 } else if (name.equals("instantiates")) { 10543 this.getInstantiates().add(castToCanonical(value)); 10544 } else if (name.equals("imports")) { 10545 this.getImports().add(castToCanonical(value)); 10546 } else if (name.equals("software")) { 10547 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 10548 } else if (name.equals("implementation")) { 10549 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 10550 } else if (name.equals("fhirVersion")) { 10551 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 10552 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 10553 } else if (name.equals("format")) { 10554 this.getFormat().add(castToCode(value)); 10555 } else if (name.equals("patchFormat")) { 10556 this.getPatchFormat().add(castToCode(value)); 10557 } else if (name.equals("implementationGuide")) { 10558 this.getImplementationGuide().add(castToCanonical(value)); 10559 } else if (name.equals("rest")) { 10560 this.getRest().add((CapabilityStatementRestComponent) value); 10561 } else if (name.equals("messaging")) { 10562 this.getMessaging().add((CapabilityStatementMessagingComponent) value); 10563 } else if (name.equals("document")) { 10564 this.getDocument().add((CapabilityStatementDocumentComponent) value); 10565 } else 10566 return super.setProperty(name, value); 10567 return value; 10568 } 10569 10570 @Override 10571 public void removeChild(String name, Base value) throws FHIRException { 10572 if (name.equals("url")) { 10573 this.url = null; 10574 } else if (name.equals("version")) { 10575 this.version = null; 10576 } else if (name.equals("name")) { 10577 this.name = null; 10578 } else if (name.equals("title")) { 10579 this.title = null; 10580 } else if (name.equals("status")) { 10581 this.status = null; 10582 } else if (name.equals("experimental")) { 10583 this.experimental = null; 10584 } else if (name.equals("date")) { 10585 this.date = null; 10586 } else if (name.equals("publisher")) { 10587 this.publisher = null; 10588 } else if (name.equals("contact")) { 10589 this.getContact().remove(castToContactDetail(value)); 10590 } else if (name.equals("description")) { 10591 this.description = null; 10592 } else if (name.equals("useContext")) { 10593 this.getUseContext().remove(castToUsageContext(value)); 10594 } else if (name.equals("jurisdiction")) { 10595 this.getJurisdiction().remove(castToCodeableConcept(value)); 10596 } else if (name.equals("purpose")) { 10597 this.purpose = null; 10598 } else if (name.equals("copyright")) { 10599 this.copyright = null; 10600 } else if (name.equals("kind")) { 10601 this.kind = null; 10602 } else if (name.equals("instantiates")) { 10603 this.getInstantiates().remove(castToCanonical(value)); 10604 } else if (name.equals("imports")) { 10605 this.getImports().remove(castToCanonical(value)); 10606 } else if (name.equals("software")) { 10607 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 10608 } else if (name.equals("implementation")) { 10609 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 10610 } else if (name.equals("fhirVersion")) { 10611 this.fhirVersion = null; 10612 } else if (name.equals("format")) { 10613 this.getFormat().remove(castToCode(value)); 10614 } else if (name.equals("patchFormat")) { 10615 this.getPatchFormat().remove(castToCode(value)); 10616 } else if (name.equals("implementationGuide")) { 10617 this.getImplementationGuide().remove(castToCanonical(value)); 10618 } else if (name.equals("rest")) { 10619 this.getRest().remove((CapabilityStatementRestComponent) value); 10620 } else if (name.equals("messaging")) { 10621 this.getMessaging().remove((CapabilityStatementMessagingComponent) value); 10622 } else if (name.equals("document")) { 10623 this.getDocument().remove((CapabilityStatementDocumentComponent) value); 10624 } else 10625 super.removeChild(name, value); 10626 10627 } 10628 10629 @Override 10630 public Base makeProperty(int hash, String name) throws FHIRException { 10631 switch (hash) { 10632 case 116079: 10633 return getUrlElement(); 10634 case 351608024: 10635 return getVersionElement(); 10636 case 3373707: 10637 return getNameElement(); 10638 case 110371416: 10639 return getTitleElement(); 10640 case -892481550: 10641 return getStatusElement(); 10642 case -404562712: 10643 return getExperimentalElement(); 10644 case 3076014: 10645 return getDateElement(); 10646 case 1447404028: 10647 return getPublisherElement(); 10648 case 951526432: 10649 return addContact(); 10650 case -1724546052: 10651 return getDescriptionElement(); 10652 case -669707736: 10653 return addUseContext(); 10654 case -507075711: 10655 return addJurisdiction(); 10656 case -220463842: 10657 return getPurposeElement(); 10658 case 1522889671: 10659 return getCopyrightElement(); 10660 case 3292052: 10661 return getKindElement(); 10662 case -246883639: 10663 return addInstantiatesElement(); 10664 case 1926037870: 10665 return addImportsElement(); 10666 case 1319330215: 10667 return getSoftware(); 10668 case 1683336114: 10669 return getImplementation(); 10670 case 461006061: 10671 return getFhirVersionElement(); 10672 case -1268779017: 10673 return addFormatElement(); 10674 case 172338783: 10675 return addPatchFormatElement(); 10676 case 156966506: 10677 return addImplementationGuideElement(); 10678 case 3496916: 10679 return addRest(); 10680 case -1440008444: 10681 return addMessaging(); 10682 case 861720859: 10683 return addDocument(); 10684 default: 10685 return super.makeProperty(hash, name); 10686 } 10687 10688 } 10689 10690 @Override 10691 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10692 switch (hash) { 10693 case 116079: 10694 /* url */ return new String[] { "uri" }; 10695 case 351608024: 10696 /* version */ return new String[] { "string" }; 10697 case 3373707: 10698 /* name */ return new String[] { "string" }; 10699 case 110371416: 10700 /* title */ return new String[] { "string" }; 10701 case -892481550: 10702 /* status */ return new String[] { "code" }; 10703 case -404562712: 10704 /* experimental */ return new String[] { "boolean" }; 10705 case 3076014: 10706 /* date */ return new String[] { "dateTime" }; 10707 case 1447404028: 10708 /* publisher */ return new String[] { "string" }; 10709 case 951526432: 10710 /* contact */ return new String[] { "ContactDetail" }; 10711 case -1724546052: 10712 /* description */ return new String[] { "markdown" }; 10713 case -669707736: 10714 /* useContext */ return new String[] { "UsageContext" }; 10715 case -507075711: 10716 /* jurisdiction */ return new String[] { "CodeableConcept" }; 10717 case -220463842: 10718 /* purpose */ return new String[] { "markdown" }; 10719 case 1522889671: 10720 /* copyright */ return new String[] { "markdown" }; 10721 case 3292052: 10722 /* kind */ return new String[] { "code" }; 10723 case -246883639: 10724 /* instantiates */ return new String[] { "canonical" }; 10725 case 1926037870: 10726 /* imports */ return new String[] { "canonical" }; 10727 case 1319330215: 10728 /* software */ return new String[] {}; 10729 case 1683336114: 10730 /* implementation */ return new String[] {}; 10731 case 461006061: 10732 /* fhirVersion */ return new String[] { "code" }; 10733 case -1268779017: 10734 /* format */ return new String[] { "code" }; 10735 case 172338783: 10736 /* patchFormat */ return new String[] { "code" }; 10737 case 156966506: 10738 /* implementationGuide */ return new String[] { "canonical" }; 10739 case 3496916: 10740 /* rest */ return new String[] {}; 10741 case -1440008444: 10742 /* messaging */ return new String[] {}; 10743 case 861720859: 10744 /* document */ return new String[] {}; 10745 default: 10746 return super.getTypesForProperty(hash, name); 10747 } 10748 10749 } 10750 10751 @Override 10752 public Base addChild(String name) throws FHIRException { 10753 if (name.equals("url")) { 10754 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 10755 } else if (name.equals("version")) { 10756 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 10757 } else if (name.equals("name")) { 10758 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 10759 } else if (name.equals("title")) { 10760 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.title"); 10761 } else if (name.equals("status")) { 10762 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.status"); 10763 } else if (name.equals("experimental")) { 10764 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.experimental"); 10765 } else if (name.equals("date")) { 10766 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.date"); 10767 } else if (name.equals("publisher")) { 10768 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.publisher"); 10769 } else if (name.equals("contact")) { 10770 return addContact(); 10771 } else if (name.equals("description")) { 10772 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 10773 } else if (name.equals("useContext")) { 10774 return addUseContext(); 10775 } else if (name.equals("jurisdiction")) { 10776 return addJurisdiction(); 10777 } else if (name.equals("purpose")) { 10778 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.purpose"); 10779 } else if (name.equals("copyright")) { 10780 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.copyright"); 10781 } else if (name.equals("kind")) { 10782 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.kind"); 10783 } else if (name.equals("instantiates")) { 10784 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.instantiates"); 10785 } else if (name.equals("imports")) { 10786 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.imports"); 10787 } else if (name.equals("software")) { 10788 this.software = new CapabilityStatementSoftwareComponent(); 10789 return this.software; 10790 } else if (name.equals("implementation")) { 10791 this.implementation = new CapabilityStatementImplementationComponent(); 10792 return this.implementation; 10793 } else if (name.equals("fhirVersion")) { 10794 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.fhirVersion"); 10795 } else if (name.equals("format")) { 10796 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.format"); 10797 } else if (name.equals("patchFormat")) { 10798 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.patchFormat"); 10799 } else if (name.equals("implementationGuide")) { 10800 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.implementationGuide"); 10801 } else if (name.equals("rest")) { 10802 return addRest(); 10803 } else if (name.equals("messaging")) { 10804 return addMessaging(); 10805 } else if (name.equals("document")) { 10806 return addDocument(); 10807 } else 10808 return super.addChild(name); 10809 } 10810 10811 public String fhirType() { 10812 return "CapabilityStatement"; 10813 10814 } 10815 10816 public CapabilityStatement copy() { 10817 CapabilityStatement dst = new CapabilityStatement(); 10818 copyValues(dst); 10819 return dst; 10820 } 10821 10822 public void copyValues(CapabilityStatement dst) { 10823 super.copyValues(dst); 10824 dst.url = url == null ? null : url.copy(); 10825 dst.version = version == null ? null : version.copy(); 10826 dst.name = name == null ? null : name.copy(); 10827 dst.title = title == null ? null : title.copy(); 10828 dst.status = status == null ? null : status.copy(); 10829 dst.experimental = experimental == null ? null : experimental.copy(); 10830 dst.date = date == null ? null : date.copy(); 10831 dst.publisher = publisher == null ? null : publisher.copy(); 10832 if (contact != null) { 10833 dst.contact = new ArrayList<ContactDetail>(); 10834 for (ContactDetail i : contact) 10835 dst.contact.add(i.copy()); 10836 } 10837 ; 10838 dst.description = description == null ? null : description.copy(); 10839 if (useContext != null) { 10840 dst.useContext = new ArrayList<UsageContext>(); 10841 for (UsageContext i : useContext) 10842 dst.useContext.add(i.copy()); 10843 } 10844 ; 10845 if (jurisdiction != null) { 10846 dst.jurisdiction = new ArrayList<CodeableConcept>(); 10847 for (CodeableConcept i : jurisdiction) 10848 dst.jurisdiction.add(i.copy()); 10849 } 10850 ; 10851 dst.purpose = purpose == null ? null : purpose.copy(); 10852 dst.copyright = copyright == null ? null : copyright.copy(); 10853 dst.kind = kind == null ? null : kind.copy(); 10854 if (instantiates != null) { 10855 dst.instantiates = new ArrayList<CanonicalType>(); 10856 for (CanonicalType i : instantiates) 10857 dst.instantiates.add(i.copy()); 10858 } 10859 ; 10860 if (imports != null) { 10861 dst.imports = new ArrayList<CanonicalType>(); 10862 for (CanonicalType i : imports) 10863 dst.imports.add(i.copy()); 10864 } 10865 ; 10866 dst.software = software == null ? null : software.copy(); 10867 dst.implementation = implementation == null ? null : implementation.copy(); 10868 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 10869 if (format != null) { 10870 dst.format = new ArrayList<CodeType>(); 10871 for (CodeType i : format) 10872 dst.format.add(i.copy()); 10873 } 10874 ; 10875 if (patchFormat != null) { 10876 dst.patchFormat = new ArrayList<CodeType>(); 10877 for (CodeType i : patchFormat) 10878 dst.patchFormat.add(i.copy()); 10879 } 10880 ; 10881 if (implementationGuide != null) { 10882 dst.implementationGuide = new ArrayList<CanonicalType>(); 10883 for (CanonicalType i : implementationGuide) 10884 dst.implementationGuide.add(i.copy()); 10885 } 10886 ; 10887 if (rest != null) { 10888 dst.rest = new ArrayList<CapabilityStatementRestComponent>(); 10889 for (CapabilityStatementRestComponent i : rest) 10890 dst.rest.add(i.copy()); 10891 } 10892 ; 10893 if (messaging != null) { 10894 dst.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 10895 for (CapabilityStatementMessagingComponent i : messaging) 10896 dst.messaging.add(i.copy()); 10897 } 10898 ; 10899 if (document != null) { 10900 dst.document = new ArrayList<CapabilityStatementDocumentComponent>(); 10901 for (CapabilityStatementDocumentComponent i : document) 10902 dst.document.add(i.copy()); 10903 } 10904 ; 10905 } 10906 10907 protected CapabilityStatement typedCopy() { 10908 return copy(); 10909 } 10910 10911 @Override 10912 public boolean equalsDeep(Base other_) { 10913 if (!super.equalsDeep(other_)) 10914 return false; 10915 if (!(other_ instanceof CapabilityStatement)) 10916 return false; 10917 CapabilityStatement o = (CapabilityStatement) other_; 10918 return compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 10919 && compareDeep(kind, o.kind, true) && compareDeep(instantiates, o.instantiates, true) 10920 && compareDeep(imports, o.imports, true) && compareDeep(software, o.software, true) 10921 && compareDeep(implementation, o.implementation, true) && compareDeep(fhirVersion, o.fhirVersion, true) 10922 && compareDeep(format, o.format, true) && compareDeep(patchFormat, o.patchFormat, true) 10923 && compareDeep(implementationGuide, o.implementationGuide, true) && compareDeep(rest, o.rest, true) 10924 && compareDeep(messaging, o.messaging, true) && compareDeep(document, o.document, true); 10925 } 10926 10927 @Override 10928 public boolean equalsShallow(Base other_) { 10929 if (!super.equalsShallow(other_)) 10930 return false; 10931 if (!(other_ instanceof CapabilityStatement)) 10932 return false; 10933 CapabilityStatement o = (CapabilityStatement) other_; 10934 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 10935 && compareValues(kind, o.kind, true) && compareValues(fhirVersion, o.fhirVersion, true) 10936 && compareValues(format, o.format, true) && compareValues(patchFormat, o.patchFormat, true); 10937 } 10938 10939 public boolean isEmpty() { 10940 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, copyright, kind, instantiates, imports, 10941 software, implementation, fhirVersion, format, patchFormat, implementationGuide, rest, messaging, document); 10942 } 10943 10944 @Override 10945 public ResourceType getResourceType() { 10946 return ResourceType.CapabilityStatement; 10947 } 10948 10949 /** 10950 * Search parameter: <b>date</b> 10951 * <p> 10952 * Description: <b>The capability statement publication date</b><br> 10953 * Type: <b>date</b><br> 10954 * Path: <b>CapabilityStatement.date</b><br> 10955 * </p> 10956 */ 10957 @SearchParamDefinition(name = "date", path = "CapabilityStatement.date", description = "The capability statement publication date", type = "date") 10958 public static final String SP_DATE = "date"; 10959 /** 10960 * <b>Fluent Client</b> search parameter constant for <b>date</b> 10961 * <p> 10962 * Description: <b>The capability statement publication date</b><br> 10963 * Type: <b>date</b><br> 10964 * Path: <b>CapabilityStatement.date</b><br> 10965 * </p> 10966 */ 10967 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 10968 SP_DATE); 10969 10970 /** 10971 * Search parameter: <b>resource-profile</b> 10972 * <p> 10973 * Description: <b>A profile id invoked in a capability statement</b><br> 10974 * Type: <b>reference</b><br> 10975 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 10976 * </p> 10977 */ 10978 @SearchParamDefinition(name = "resource-profile", path = "CapabilityStatement.rest.resource.profile", description = "A profile id invoked in a capability statement", type = "reference", target = { 10979 StructureDefinition.class }) 10980 public static final String SP_RESOURCE_PROFILE = "resource-profile"; 10981 /** 10982 * <b>Fluent Client</b> search parameter constant for <b>resource-profile</b> 10983 * <p> 10984 * Description: <b>A profile id invoked in a capability statement</b><br> 10985 * Type: <b>reference</b><br> 10986 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 10987 * </p> 10988 */ 10989 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10990 SP_RESOURCE_PROFILE); 10991 10992 /** 10993 * Constant for fluent queries to be used to add include statements. Specifies 10994 * the path value of "<b>CapabilityStatement:resource-profile</b>". 10995 */ 10996 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE_PROFILE = new ca.uhn.fhir.model.api.Include( 10997 "CapabilityStatement:resource-profile").toLocked(); 10998 10999 /** 11000 * Search parameter: <b>context-type-value</b> 11001 * <p> 11002 * Description: <b>A use context type and value assigned to the capability 11003 * statement</b><br> 11004 * Type: <b>composite</b><br> 11005 * Path: <b></b><br> 11006 * </p> 11007 */ 11008 @SearchParamDefinition(name = "context-type-value", path = "CapabilityStatement.useContext", description = "A use context type and value assigned to the capability statement", type = "composite", compositeOf = { 11009 "context-type", "context" }) 11010 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 11011 /** 11012 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 11013 * <p> 11014 * Description: <b>A use context type and value assigned to the capability 11015 * statement</b><br> 11016 * Type: <b>composite</b><br> 11017 * Path: <b></b><br> 11018 * </p> 11019 */ 11020 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 11021 SP_CONTEXT_TYPE_VALUE); 11022 11023 /** 11024 * Search parameter: <b>software</b> 11025 * <p> 11026 * Description: <b>Part of the name of a software application</b><br> 11027 * Type: <b>string</b><br> 11028 * Path: <b>CapabilityStatement.software.name</b><br> 11029 * </p> 11030 */ 11031 @SearchParamDefinition(name = "software", path = "CapabilityStatement.software.name", description = "Part of the name of a software application", type = "string") 11032 public static final String SP_SOFTWARE = "software"; 11033 /** 11034 * <b>Fluent Client</b> search parameter constant for <b>software</b> 11035 * <p> 11036 * Description: <b>Part of the name of a software application</b><br> 11037 * Type: <b>string</b><br> 11038 * Path: <b>CapabilityStatement.software.name</b><br> 11039 * </p> 11040 */ 11041 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOFTWARE = new ca.uhn.fhir.rest.gclient.StringClientParam( 11042 SP_SOFTWARE); 11043 11044 /** 11045 * Search parameter: <b>resource</b> 11046 * <p> 11047 * Description: <b>Name of a resource mentioned in a capability 11048 * statement</b><br> 11049 * Type: <b>token</b><br> 11050 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 11051 * </p> 11052 */ 11053 @SearchParamDefinition(name = "resource", path = "CapabilityStatement.rest.resource.type", description = "Name of a resource mentioned in a capability statement", type = "token") 11054 public static final String SP_RESOURCE = "resource"; 11055 /** 11056 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 11057 * <p> 11058 * Description: <b>Name of a resource mentioned in a capability 11059 * statement</b><br> 11060 * Type: <b>token</b><br> 11061 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 11062 * </p> 11063 */ 11064 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11065 SP_RESOURCE); 11066 11067 /** 11068 * Search parameter: <b>jurisdiction</b> 11069 * <p> 11070 * Description: <b>Intended jurisdiction for the capability statement</b><br> 11071 * Type: <b>token</b><br> 11072 * Path: <b>CapabilityStatement.jurisdiction</b><br> 11073 * </p> 11074 */ 11075 @SearchParamDefinition(name = "jurisdiction", path = "CapabilityStatement.jurisdiction", description = "Intended jurisdiction for the capability statement", type = "token") 11076 public static final String SP_JURISDICTION = "jurisdiction"; 11077 /** 11078 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 11079 * <p> 11080 * Description: <b>Intended jurisdiction for the capability statement</b><br> 11081 * Type: <b>token</b><br> 11082 * Path: <b>CapabilityStatement.jurisdiction</b><br> 11083 * </p> 11084 */ 11085 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11086 SP_JURISDICTION); 11087 11088 /** 11089 * Search parameter: <b>format</b> 11090 * <p> 11091 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 11092 * Type: <b>token</b><br> 11093 * Path: <b>CapabilityStatement.format</b><br> 11094 * </p> 11095 */ 11096 @SearchParamDefinition(name = "format", path = "CapabilityStatement.format", description = "formats supported (xml | json | ttl | mime type)", type = "token") 11097 public static final String SP_FORMAT = "format"; 11098 /** 11099 * <b>Fluent Client</b> search parameter constant for <b>format</b> 11100 * <p> 11101 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 11102 * Type: <b>token</b><br> 11103 * Path: <b>CapabilityStatement.format</b><br> 11104 * </p> 11105 */ 11106 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11107 SP_FORMAT); 11108 11109 /** 11110 * Search parameter: <b>description</b> 11111 * <p> 11112 * Description: <b>The description of the capability statement</b><br> 11113 * Type: <b>string</b><br> 11114 * Path: <b>CapabilityStatement.description</b><br> 11115 * </p> 11116 */ 11117 @SearchParamDefinition(name = "description", path = "CapabilityStatement.description", description = "The description of the capability statement", type = "string") 11118 public static final String SP_DESCRIPTION = "description"; 11119 /** 11120 * <b>Fluent Client</b> search parameter constant for <b>description</b> 11121 * <p> 11122 * Description: <b>The description of the capability statement</b><br> 11123 * Type: <b>string</b><br> 11124 * Path: <b>CapabilityStatement.description</b><br> 11125 * </p> 11126 */ 11127 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 11128 SP_DESCRIPTION); 11129 11130 /** 11131 * Search parameter: <b>context-type</b> 11132 * <p> 11133 * Description: <b>A type of use context assigned to the capability 11134 * statement</b><br> 11135 * Type: <b>token</b><br> 11136 * Path: <b>CapabilityStatement.useContext.code</b><br> 11137 * </p> 11138 */ 11139 @SearchParamDefinition(name = "context-type", path = "CapabilityStatement.useContext.code", description = "A type of use context assigned to the capability statement", type = "token") 11140 public static final String SP_CONTEXT_TYPE = "context-type"; 11141 /** 11142 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 11143 * <p> 11144 * Description: <b>A type of use context assigned to the capability 11145 * statement</b><br> 11146 * Type: <b>token</b><br> 11147 * Path: <b>CapabilityStatement.useContext.code</b><br> 11148 * </p> 11149 */ 11150 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11151 SP_CONTEXT_TYPE); 11152 11153 /** 11154 * Search parameter: <b>title</b> 11155 * <p> 11156 * Description: <b>The human-friendly name of the capability statement</b><br> 11157 * Type: <b>string</b><br> 11158 * Path: <b>CapabilityStatement.title</b><br> 11159 * </p> 11160 */ 11161 @SearchParamDefinition(name = "title", path = "CapabilityStatement.title", description = "The human-friendly name of the capability statement", type = "string") 11162 public static final String SP_TITLE = "title"; 11163 /** 11164 * <b>Fluent Client</b> search parameter constant for <b>title</b> 11165 * <p> 11166 * Description: <b>The human-friendly name of the capability statement</b><br> 11167 * Type: <b>string</b><br> 11168 * Path: <b>CapabilityStatement.title</b><br> 11169 * </p> 11170 */ 11171 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 11172 SP_TITLE); 11173 11174 /** 11175 * Search parameter: <b>fhirversion</b> 11176 * <p> 11177 * Description: <b>The version of FHIR</b><br> 11178 * Type: <b>token</b><br> 11179 * Path: <b>CapabilityStatement.version</b><br> 11180 * </p> 11181 */ 11182 @SearchParamDefinition(name = "fhirversion", path = "CapabilityStatement.version", description = "The version of FHIR", type = "token") 11183 public static final String SP_FHIRVERSION = "fhirversion"; 11184 /** 11185 * <b>Fluent Client</b> search parameter constant for <b>fhirversion</b> 11186 * <p> 11187 * Description: <b>The version of FHIR</b><br> 11188 * Type: <b>token</b><br> 11189 * Path: <b>CapabilityStatement.version</b><br> 11190 * </p> 11191 */ 11192 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FHIRVERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11193 SP_FHIRVERSION); 11194 11195 /** 11196 * Search parameter: <b>version</b> 11197 * <p> 11198 * Description: <b>The business version of the capability statement</b><br> 11199 * Type: <b>token</b><br> 11200 * Path: <b>CapabilityStatement.version</b><br> 11201 * </p> 11202 */ 11203 @SearchParamDefinition(name = "version", path = "CapabilityStatement.version", description = "The business version of the capability statement", type = "token") 11204 public static final String SP_VERSION = "version"; 11205 /** 11206 * <b>Fluent Client</b> search parameter constant for <b>version</b> 11207 * <p> 11208 * Description: <b>The business version of the capability statement</b><br> 11209 * Type: <b>token</b><br> 11210 * Path: <b>CapabilityStatement.version</b><br> 11211 * </p> 11212 */ 11213 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11214 SP_VERSION); 11215 11216 /** 11217 * Search parameter: <b>url</b> 11218 * <p> 11219 * Description: <b>The uri that identifies the capability statement</b><br> 11220 * Type: <b>uri</b><br> 11221 * Path: <b>CapabilityStatement.url</b><br> 11222 * </p> 11223 */ 11224 @SearchParamDefinition(name = "url", path = "CapabilityStatement.url", description = "The uri that identifies the capability statement", type = "uri") 11225 public static final String SP_URL = "url"; 11226 /** 11227 * <b>Fluent Client</b> search parameter constant for <b>url</b> 11228 * <p> 11229 * Description: <b>The uri that identifies the capability statement</b><br> 11230 * Type: <b>uri</b><br> 11231 * Path: <b>CapabilityStatement.url</b><br> 11232 * </p> 11233 */ 11234 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 11235 11236 /** 11237 * Search parameter: <b>supported-profile</b> 11238 * <p> 11239 * Description: <b>Profiles for use cases supported</b><br> 11240 * Type: <b>reference</b><br> 11241 * Path: <b>CapabilityStatement.rest.resource.supportedProfile</b><br> 11242 * </p> 11243 */ 11244 @SearchParamDefinition(name = "supported-profile", path = "CapabilityStatement.rest.resource.supportedProfile", description = "Profiles for use cases supported", type = "reference", target = { 11245 StructureDefinition.class }) 11246 public static final String SP_SUPPORTED_PROFILE = "supported-profile"; 11247 /** 11248 * <b>Fluent Client</b> search parameter constant for <b>supported-profile</b> 11249 * <p> 11250 * Description: <b>Profiles for use cases supported</b><br> 11251 * Type: <b>reference</b><br> 11252 * Path: <b>CapabilityStatement.rest.resource.supportedProfile</b><br> 11253 * </p> 11254 */ 11255 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTED_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11256 SP_SUPPORTED_PROFILE); 11257 11258 /** 11259 * Constant for fluent queries to be used to add include statements. Specifies 11260 * the path value of "<b>CapabilityStatement:supported-profile</b>". 11261 */ 11262 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTED_PROFILE = new ca.uhn.fhir.model.api.Include( 11263 "CapabilityStatement:supported-profile").toLocked(); 11264 11265 /** 11266 * Search parameter: <b>mode</b> 11267 * <p> 11268 * Description: <b>Mode - restful (server/client) or messaging 11269 * (sender/receiver)</b><br> 11270 * Type: <b>token</b><br> 11271 * Path: <b>CapabilityStatement.rest.mode</b><br> 11272 * </p> 11273 */ 11274 @SearchParamDefinition(name = "mode", path = "CapabilityStatement.rest.mode", description = "Mode - restful (server/client) or messaging (sender/receiver)", type = "token") 11275 public static final String SP_MODE = "mode"; 11276 /** 11277 * <b>Fluent Client</b> search parameter constant for <b>mode</b> 11278 * <p> 11279 * Description: <b>Mode - restful (server/client) or messaging 11280 * (sender/receiver)</b><br> 11281 * Type: <b>token</b><br> 11282 * Path: <b>CapabilityStatement.rest.mode</b><br> 11283 * </p> 11284 */ 11285 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11286 SP_MODE); 11287 11288 /** 11289 * Search parameter: <b>context-quantity</b> 11290 * <p> 11291 * Description: <b>A quantity- or range-valued use context assigned to the 11292 * capability statement</b><br> 11293 * Type: <b>quantity</b><br> 11294 * Path: <b>CapabilityStatement.useContext.valueQuantity, 11295 * CapabilityStatement.useContext.valueRange</b><br> 11296 * </p> 11297 */ 11298 @SearchParamDefinition(name = "context-quantity", path = "(CapabilityStatement.useContext.value as Quantity) | (CapabilityStatement.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the capability statement", type = "quantity") 11299 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 11300 /** 11301 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 11302 * <p> 11303 * Description: <b>A quantity- or range-valued use context assigned to the 11304 * capability statement</b><br> 11305 * Type: <b>quantity</b><br> 11306 * Path: <b>CapabilityStatement.useContext.valueQuantity, 11307 * CapabilityStatement.useContext.valueRange</b><br> 11308 * </p> 11309 */ 11310 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 11311 SP_CONTEXT_QUANTITY); 11312 11313 /** 11314 * Search parameter: <b>security-service</b> 11315 * <p> 11316 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | 11317 * Certificates</b><br> 11318 * Type: <b>token</b><br> 11319 * Path: <b>CapabilityStatement.rest.security.service</b><br> 11320 * </p> 11321 */ 11322 @SearchParamDefinition(name = "security-service", path = "CapabilityStatement.rest.security.service", description = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", type = "token") 11323 public static final String SP_SECURITY_SERVICE = "security-service"; 11324 /** 11325 * <b>Fluent Client</b> search parameter constant for <b>security-service</b> 11326 * <p> 11327 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | 11328 * Certificates</b><br> 11329 * Type: <b>token</b><br> 11330 * Path: <b>CapabilityStatement.rest.security.service</b><br> 11331 * </p> 11332 */ 11333 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_SERVICE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11334 SP_SECURITY_SERVICE); 11335 11336 /** 11337 * Search parameter: <b>name</b> 11338 * <p> 11339 * Description: <b>Computationally friendly name of the capability 11340 * statement</b><br> 11341 * Type: <b>string</b><br> 11342 * Path: <b>CapabilityStatement.name</b><br> 11343 * </p> 11344 */ 11345 @SearchParamDefinition(name = "name", path = "CapabilityStatement.name", description = "Computationally friendly name of the capability statement", type = "string") 11346 public static final String SP_NAME = "name"; 11347 /** 11348 * <b>Fluent Client</b> search parameter constant for <b>name</b> 11349 * <p> 11350 * Description: <b>Computationally friendly name of the capability 11351 * statement</b><br> 11352 * Type: <b>string</b><br> 11353 * Path: <b>CapabilityStatement.name</b><br> 11354 * </p> 11355 */ 11356 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 11357 SP_NAME); 11358 11359 /** 11360 * Search parameter: <b>context</b> 11361 * <p> 11362 * Description: <b>A use context assigned to the capability statement</b><br> 11363 * Type: <b>token</b><br> 11364 * Path: <b>CapabilityStatement.useContext.valueCodeableConcept</b><br> 11365 * </p> 11366 */ 11367 @SearchParamDefinition(name = "context", path = "(CapabilityStatement.useContext.value as CodeableConcept)", description = "A use context assigned to the capability statement", type = "token") 11368 public static final String SP_CONTEXT = "context"; 11369 /** 11370 * <b>Fluent Client</b> search parameter constant for <b>context</b> 11371 * <p> 11372 * Description: <b>A use context assigned to the capability statement</b><br> 11373 * Type: <b>token</b><br> 11374 * Path: <b>CapabilityStatement.useContext.valueCodeableConcept</b><br> 11375 * </p> 11376 */ 11377 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11378 SP_CONTEXT); 11379 11380 /** 11381 * Search parameter: <b>publisher</b> 11382 * <p> 11383 * Description: <b>Name of the publisher of the capability statement</b><br> 11384 * Type: <b>string</b><br> 11385 * Path: <b>CapabilityStatement.publisher</b><br> 11386 * </p> 11387 */ 11388 @SearchParamDefinition(name = "publisher", path = "CapabilityStatement.publisher", description = "Name of the publisher of the capability statement", type = "string") 11389 public static final String SP_PUBLISHER = "publisher"; 11390 /** 11391 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 11392 * <p> 11393 * Description: <b>Name of the publisher of the capability statement</b><br> 11394 * Type: <b>string</b><br> 11395 * Path: <b>CapabilityStatement.publisher</b><br> 11396 * </p> 11397 */ 11398 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 11399 SP_PUBLISHER); 11400 11401 /** 11402 * Search parameter: <b>context-type-quantity</b> 11403 * <p> 11404 * Description: <b>A use context type and quantity- or range-based value 11405 * assigned to the capability statement</b><br> 11406 * Type: <b>composite</b><br> 11407 * Path: <b></b><br> 11408 * </p> 11409 */ 11410 @SearchParamDefinition(name = "context-type-quantity", path = "CapabilityStatement.useContext", description = "A use context type and quantity- or range-based value assigned to the capability statement", type = "composite", compositeOf = { 11411 "context-type", "context-quantity" }) 11412 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 11413 /** 11414 * <b>Fluent Client</b> search parameter constant for 11415 * <b>context-type-quantity</b> 11416 * <p> 11417 * Description: <b>A use context type and quantity- or range-based value 11418 * assigned to the capability statement</b><br> 11419 * Type: <b>composite</b><br> 11420 * Path: <b></b><br> 11421 * </p> 11422 */ 11423 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 11424 SP_CONTEXT_TYPE_QUANTITY); 11425 11426 /** 11427 * Search parameter: <b>guide</b> 11428 * <p> 11429 * Description: <b>Implementation guides supported</b><br> 11430 * Type: <b>reference</b><br> 11431 * Path: <b>CapabilityStatement.implementationGuide</b><br> 11432 * </p> 11433 */ 11434 @SearchParamDefinition(name = "guide", path = "CapabilityStatement.implementationGuide", description = "Implementation guides supported", type = "reference", target = { 11435 ImplementationGuide.class }) 11436 public static final String SP_GUIDE = "guide"; 11437 /** 11438 * <b>Fluent Client</b> search parameter constant for <b>guide</b> 11439 * <p> 11440 * Description: <b>Implementation guides supported</b><br> 11441 * Type: <b>reference</b><br> 11442 * Path: <b>CapabilityStatement.implementationGuide</b><br> 11443 * </p> 11444 */ 11445 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GUIDE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11446 SP_GUIDE); 11447 11448 /** 11449 * Constant for fluent queries to be used to add include statements. Specifies 11450 * the path value of "<b>CapabilityStatement:guide</b>". 11451 */ 11452 public static final ca.uhn.fhir.model.api.Include INCLUDE_GUIDE = new ca.uhn.fhir.model.api.Include( 11453 "CapabilityStatement:guide").toLocked(); 11454 11455 /** 11456 * Search parameter: <b>status</b> 11457 * <p> 11458 * Description: <b>The current status of the capability statement</b><br> 11459 * Type: <b>token</b><br> 11460 * Path: <b>CapabilityStatement.status</b><br> 11461 * </p> 11462 */ 11463 @SearchParamDefinition(name = "status", path = "CapabilityStatement.status", description = "The current status of the capability statement", type = "token") 11464 public static final String SP_STATUS = "status"; 11465 /** 11466 * <b>Fluent Client</b> search parameter constant for <b>status</b> 11467 * <p> 11468 * Description: <b>The current status of the capability statement</b><br> 11469 * Type: <b>token</b><br> 11470 * Path: <b>CapabilityStatement.status</b><br> 11471 * </p> 11472 */ 11473 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11474 SP_STATUS); 11475 11476}