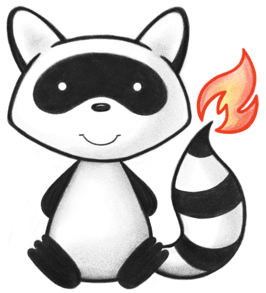
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes the intention of how one or more practitioners intend to deliver 049 * care for a particular patient, group or community for a period of time, 050 * possibly limited to care for a specific condition or set of conditions. 051 */ 052@ResourceDef(name = "CarePlan", profile = "http://hl7.org/fhir/StructureDefinition/CarePlan") 053public class CarePlan extends DomainResource { 054 055 public enum CarePlanStatus { 056 /** 057 * The request has been created but is not yet complete or ready for action. 058 */ 059 DRAFT, 060 /** 061 * The request is in force and ready to be acted upon. 062 */ 063 ACTIVE, 064 /** 065 * The request (and any implicit authorization to act) has been temporarily 066 * withdrawn but is expected to resume in the future. 067 */ 068 ONHOLD, 069 /** 070 * The request (and any implicit authorization to act) has been terminated prior 071 * to the known full completion of the intended actions. No further activity 072 * should occur. 073 */ 074 REVOKED, 075 /** 076 * The activity described by the request has been fully performed. No further 077 * activity will occur. 078 */ 079 COMPLETED, 080 /** 081 * This request should never have existed and should be considered 'void'. (It 082 * is possible that real-world decisions were based on it. If real-world 083 * activity has occurred, the status should be "revoked" rather than 084 * "entered-in-error".). 085 */ 086 ENTEREDINERROR, 087 /** 088 * The authoring/source system does not know which of the status values 089 * currently applies for this request. Note: This concept is not to be used for 090 * "other" - one of the listed statuses is presumed to apply, but the 091 * authoring/source system does not know which. 092 */ 093 UNKNOWN, 094 /** 095 * added to help the parsers with the generic types 096 */ 097 NULL; 098 099 public static CarePlanStatus fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("draft".equals(codeString)) 103 return DRAFT; 104 if ("active".equals(codeString)) 105 return ACTIVE; 106 if ("on-hold".equals(codeString)) 107 return ONHOLD; 108 if ("revoked".equals(codeString)) 109 return REVOKED; 110 if ("completed".equals(codeString)) 111 return COMPLETED; 112 if ("entered-in-error".equals(codeString)) 113 return ENTEREDINERROR; 114 if ("unknown".equals(codeString)) 115 return UNKNOWN; 116 if (Configuration.isAcceptInvalidEnums()) 117 return null; 118 else 119 throw new FHIRException("Unknown CarePlanStatus code '" + codeString + "'"); 120 } 121 122 public String toCode() { 123 switch (this) { 124 case DRAFT: 125 return "draft"; 126 case ACTIVE: 127 return "active"; 128 case ONHOLD: 129 return "on-hold"; 130 case REVOKED: 131 return "revoked"; 132 case COMPLETED: 133 return "completed"; 134 case ENTEREDINERROR: 135 return "entered-in-error"; 136 case UNKNOWN: 137 return "unknown"; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getSystem() { 146 switch (this) { 147 case DRAFT: 148 return "http://hl7.org/fhir/request-status"; 149 case ACTIVE: 150 return "http://hl7.org/fhir/request-status"; 151 case ONHOLD: 152 return "http://hl7.org/fhir/request-status"; 153 case REVOKED: 154 return "http://hl7.org/fhir/request-status"; 155 case COMPLETED: 156 return "http://hl7.org/fhir/request-status"; 157 case ENTEREDINERROR: 158 return "http://hl7.org/fhir/request-status"; 159 case UNKNOWN: 160 return "http://hl7.org/fhir/request-status"; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDefinition() { 169 switch (this) { 170 case DRAFT: 171 return "The request has been created but is not yet complete or ready for action."; 172 case ACTIVE: 173 return "The request is in force and ready to be acted upon."; 174 case ONHOLD: 175 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 176 case REVOKED: 177 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 178 case COMPLETED: 179 return "The activity described by the request has been fully performed. No further activity will occur."; 180 case ENTEREDINERROR: 181 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 182 case UNKNOWN: 183 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case DRAFT: 194 return "Draft"; 195 case ACTIVE: 196 return "Active"; 197 case ONHOLD: 198 return "On Hold"; 199 case REVOKED: 200 return "Revoked"; 201 case COMPLETED: 202 return "Completed"; 203 case ENTEREDINERROR: 204 return "Entered in Error"; 205 case UNKNOWN: 206 return "Unknown"; 207 case NULL: 208 return null; 209 default: 210 return "?"; 211 } 212 } 213 } 214 215 public static class CarePlanStatusEnumFactory implements EnumFactory<CarePlanStatus> { 216 public CarePlanStatus fromCode(String codeString) throws IllegalArgumentException { 217 if (codeString == null || "".equals(codeString)) 218 if (codeString == null || "".equals(codeString)) 219 return null; 220 if ("draft".equals(codeString)) 221 return CarePlanStatus.DRAFT; 222 if ("active".equals(codeString)) 223 return CarePlanStatus.ACTIVE; 224 if ("on-hold".equals(codeString)) 225 return CarePlanStatus.ONHOLD; 226 if ("revoked".equals(codeString)) 227 return CarePlanStatus.REVOKED; 228 if ("completed".equals(codeString)) 229 return CarePlanStatus.COMPLETED; 230 if ("entered-in-error".equals(codeString)) 231 return CarePlanStatus.ENTEREDINERROR; 232 if ("unknown".equals(codeString)) 233 return CarePlanStatus.UNKNOWN; 234 throw new IllegalArgumentException("Unknown CarePlanStatus code '" + codeString + "'"); 235 } 236 237 public Enumeration<CarePlanStatus> fromType(PrimitiveType<?> code) throws FHIRException { 238 if (code == null) 239 return null; 240 if (code.isEmpty()) 241 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.NULL, code); 242 String codeString = code.asStringValue(); 243 if (codeString == null || "".equals(codeString)) 244 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.NULL, code); 245 if ("draft".equals(codeString)) 246 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.DRAFT, code); 247 if ("active".equals(codeString)) 248 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ACTIVE, code); 249 if ("on-hold".equals(codeString)) 250 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ONHOLD, code); 251 if ("revoked".equals(codeString)) 252 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.REVOKED, code); 253 if ("completed".equals(codeString)) 254 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.COMPLETED, code); 255 if ("entered-in-error".equals(codeString)) 256 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ENTEREDINERROR, code); 257 if ("unknown".equals(codeString)) 258 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.UNKNOWN, code); 259 throw new FHIRException("Unknown CarePlanStatus code '" + codeString + "'"); 260 } 261 262 public String toCode(CarePlanStatus code) { 263 if (code == CarePlanStatus.DRAFT) 264 return "draft"; 265 if (code == CarePlanStatus.ACTIVE) 266 return "active"; 267 if (code == CarePlanStatus.ONHOLD) 268 return "on-hold"; 269 if (code == CarePlanStatus.REVOKED) 270 return "revoked"; 271 if (code == CarePlanStatus.COMPLETED) 272 return "completed"; 273 if (code == CarePlanStatus.ENTEREDINERROR) 274 return "entered-in-error"; 275 if (code == CarePlanStatus.UNKNOWN) 276 return "unknown"; 277 return "?"; 278 } 279 280 public String toSystem(CarePlanStatus code) { 281 return code.getSystem(); 282 } 283 } 284 285 public enum CarePlanIntent { 286 /** 287 * null 288 */ 289 PROPOSAL, 290 /** 291 * null 292 */ 293 PLAN, 294 /** 295 * null 296 */ 297 ORDER, 298 /** 299 * null 300 */ 301 OPTION, 302 /** 303 * added to help the parsers with the generic types 304 */ 305 NULL; 306 307 public static CarePlanIntent fromCode(String codeString) throws FHIRException { 308 if (codeString == null || "".equals(codeString)) 309 return null; 310 if ("proposal".equals(codeString)) 311 return PROPOSAL; 312 if ("plan".equals(codeString)) 313 return PLAN; 314 if ("order".equals(codeString)) 315 return ORDER; 316 if ("option".equals(codeString)) 317 return OPTION; 318 if (Configuration.isAcceptInvalidEnums()) 319 return null; 320 else 321 throw new FHIRException("Unknown CarePlanIntent code '" + codeString + "'"); 322 } 323 324 public String toCode() { 325 switch (this) { 326 case PROPOSAL: 327 return "proposal"; 328 case PLAN: 329 return "plan"; 330 case ORDER: 331 return "order"; 332 case OPTION: 333 return "option"; 334 case NULL: 335 return null; 336 default: 337 return "?"; 338 } 339 } 340 341 public String getSystem() { 342 switch (this) { 343 case PROPOSAL: 344 return "http://hl7.org/fhir/request-intent"; 345 case PLAN: 346 return "http://hl7.org/fhir/request-intent"; 347 case ORDER: 348 return "http://hl7.org/fhir/request-intent"; 349 case OPTION: 350 return "http://hl7.org/fhir/request-intent"; 351 case NULL: 352 return null; 353 default: 354 return "?"; 355 } 356 } 357 358 public String getDefinition() { 359 switch (this) { 360 case PROPOSAL: 361 return ""; 362 case PLAN: 363 return ""; 364 case ORDER: 365 return ""; 366 case OPTION: 367 return ""; 368 case NULL: 369 return null; 370 default: 371 return "?"; 372 } 373 } 374 375 public String getDisplay() { 376 switch (this) { 377 case PROPOSAL: 378 return "proposal"; 379 case PLAN: 380 return "plan"; 381 case ORDER: 382 return "order"; 383 case OPTION: 384 return "option"; 385 case NULL: 386 return null; 387 default: 388 return "?"; 389 } 390 } 391 } 392 393 public static class CarePlanIntentEnumFactory implements EnumFactory<CarePlanIntent> { 394 public CarePlanIntent fromCode(String codeString) throws IllegalArgumentException { 395 if (codeString == null || "".equals(codeString)) 396 if (codeString == null || "".equals(codeString)) 397 return null; 398 if ("proposal".equals(codeString)) 399 return CarePlanIntent.PROPOSAL; 400 if ("plan".equals(codeString)) 401 return CarePlanIntent.PLAN; 402 if ("order".equals(codeString)) 403 return CarePlanIntent.ORDER; 404 if ("option".equals(codeString)) 405 return CarePlanIntent.OPTION; 406 throw new IllegalArgumentException("Unknown CarePlanIntent code '" + codeString + "'"); 407 } 408 409 public Enumeration<CarePlanIntent> fromType(PrimitiveType<?> code) throws FHIRException { 410 if (code == null) 411 return null; 412 if (code.isEmpty()) 413 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.NULL, code); 414 String codeString = code.asStringValue(); 415 if (codeString == null || "".equals(codeString)) 416 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.NULL, code); 417 if ("proposal".equals(codeString)) 418 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PROPOSAL, code); 419 if ("plan".equals(codeString)) 420 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PLAN, code); 421 if ("order".equals(codeString)) 422 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.ORDER, code); 423 if ("option".equals(codeString)) 424 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.OPTION, code); 425 throw new FHIRException("Unknown CarePlanIntent code '" + codeString + "'"); 426 } 427 428 public String toCode(CarePlanIntent code) { 429 if (code == CarePlanIntent.PROPOSAL) 430 return "proposal"; 431 if (code == CarePlanIntent.PLAN) 432 return "plan"; 433 if (code == CarePlanIntent.ORDER) 434 return "order"; 435 if (code == CarePlanIntent.OPTION) 436 return "option"; 437 return "?"; 438 } 439 440 public String toSystem(CarePlanIntent code) { 441 return code.getSystem(); 442 } 443 } 444 445 public enum CarePlanActivityKind { 446 /** 447 * null 448 */ 449 APPOINTMENT, 450 /** 451 * null 452 */ 453 COMMUNICATIONREQUEST, 454 /** 455 * null 456 */ 457 DEVICEREQUEST, 458 /** 459 * null 460 */ 461 MEDICATIONREQUEST, 462 /** 463 * null 464 */ 465 NUTRITIONORDER, 466 /** 467 * null 468 */ 469 TASK, 470 /** 471 * null 472 */ 473 SERVICEREQUEST, 474 /** 475 * null 476 */ 477 VISIONPRESCRIPTION, 478 /** 479 * added to help the parsers with the generic types 480 */ 481 NULL; 482 483 public static CarePlanActivityKind fromCode(String codeString) throws FHIRException { 484 if (codeString == null || "".equals(codeString)) 485 return null; 486 if ("Appointment".equals(codeString)) 487 return APPOINTMENT; 488 if ("CommunicationRequest".equals(codeString)) 489 return COMMUNICATIONREQUEST; 490 if ("DeviceRequest".equals(codeString)) 491 return DEVICEREQUEST; 492 if ("MedicationRequest".equals(codeString)) 493 return MEDICATIONREQUEST; 494 if ("NutritionOrder".equals(codeString)) 495 return NUTRITIONORDER; 496 if ("Task".equals(codeString)) 497 return TASK; 498 if ("ServiceRequest".equals(codeString)) 499 return SERVICEREQUEST; 500 if ("VisionPrescription".equals(codeString)) 501 return VISIONPRESCRIPTION; 502 if (Configuration.isAcceptInvalidEnums()) 503 return null; 504 else 505 throw new FHIRException("Unknown CarePlanActivityKind code '" + codeString + "'"); 506 } 507 508 public String toCode() { 509 switch (this) { 510 case APPOINTMENT: 511 return "Appointment"; 512 case COMMUNICATIONREQUEST: 513 return "CommunicationRequest"; 514 case DEVICEREQUEST: 515 return "DeviceRequest"; 516 case MEDICATIONREQUEST: 517 return "MedicationRequest"; 518 case NUTRITIONORDER: 519 return "NutritionOrder"; 520 case TASK: 521 return "Task"; 522 case SERVICEREQUEST: 523 return "ServiceRequest"; 524 case VISIONPRESCRIPTION: 525 return "VisionPrescription"; 526 case NULL: 527 return null; 528 default: 529 return "?"; 530 } 531 } 532 533 public String getSystem() { 534 switch (this) { 535 case APPOINTMENT: 536 return "http://hl7.org/fhir/resource-types"; 537 case COMMUNICATIONREQUEST: 538 return "http://hl7.org/fhir/resource-types"; 539 case DEVICEREQUEST: 540 return "http://hl7.org/fhir/resource-types"; 541 case MEDICATIONREQUEST: 542 return "http://hl7.org/fhir/resource-types"; 543 case NUTRITIONORDER: 544 return "http://hl7.org/fhir/resource-types"; 545 case TASK: 546 return "http://hl7.org/fhir/resource-types"; 547 case SERVICEREQUEST: 548 return "http://hl7.org/fhir/resource-types"; 549 case VISIONPRESCRIPTION: 550 return "http://hl7.org/fhir/resource-types"; 551 case NULL: 552 return null; 553 default: 554 return "?"; 555 } 556 } 557 558 public String getDefinition() { 559 switch (this) { 560 case APPOINTMENT: 561 return ""; 562 case COMMUNICATIONREQUEST: 563 return ""; 564 case DEVICEREQUEST: 565 return ""; 566 case MEDICATIONREQUEST: 567 return ""; 568 case NUTRITIONORDER: 569 return ""; 570 case TASK: 571 return ""; 572 case SERVICEREQUEST: 573 return ""; 574 case VISIONPRESCRIPTION: 575 return ""; 576 case NULL: 577 return null; 578 default: 579 return "?"; 580 } 581 } 582 583 public String getDisplay() { 584 switch (this) { 585 case APPOINTMENT: 586 return "Appointment"; 587 case COMMUNICATIONREQUEST: 588 return "CommunicationRequest"; 589 case DEVICEREQUEST: 590 return "DeviceRequest"; 591 case MEDICATIONREQUEST: 592 return "MedicationRequest"; 593 case NUTRITIONORDER: 594 return "NutritionOrder"; 595 case TASK: 596 return "Task"; 597 case SERVICEREQUEST: 598 return "ServiceRequest"; 599 case VISIONPRESCRIPTION: 600 return "VisionPrescription"; 601 case NULL: 602 return null; 603 default: 604 return "?"; 605 } 606 } 607 } 608 609 public static class CarePlanActivityKindEnumFactory implements EnumFactory<CarePlanActivityKind> { 610 public CarePlanActivityKind fromCode(String codeString) throws IllegalArgumentException { 611 if (codeString == null || "".equals(codeString)) 612 if (codeString == null || "".equals(codeString)) 613 return null; 614 if ("Appointment".equals(codeString)) 615 return CarePlanActivityKind.APPOINTMENT; 616 if ("CommunicationRequest".equals(codeString)) 617 return CarePlanActivityKind.COMMUNICATIONREQUEST; 618 if ("DeviceRequest".equals(codeString)) 619 return CarePlanActivityKind.DEVICEREQUEST; 620 if ("MedicationRequest".equals(codeString)) 621 return CarePlanActivityKind.MEDICATIONREQUEST; 622 if ("NutritionOrder".equals(codeString)) 623 return CarePlanActivityKind.NUTRITIONORDER; 624 if ("Task".equals(codeString)) 625 return CarePlanActivityKind.TASK; 626 if ("ServiceRequest".equals(codeString)) 627 return CarePlanActivityKind.SERVICEREQUEST; 628 if ("VisionPrescription".equals(codeString)) 629 return CarePlanActivityKind.VISIONPRESCRIPTION; 630 throw new IllegalArgumentException("Unknown CarePlanActivityKind code '" + codeString + "'"); 631 } 632 633 public Enumeration<CarePlanActivityKind> fromType(PrimitiveType<?> code) throws FHIRException { 634 if (code == null) 635 return null; 636 if (code.isEmpty()) 637 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.NULL, code); 638 String codeString = code.asStringValue(); 639 if (codeString == null || "".equals(codeString)) 640 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.NULL, code); 641 if ("Appointment".equals(codeString)) 642 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.APPOINTMENT, code); 643 if ("CommunicationRequest".equals(codeString)) 644 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.COMMUNICATIONREQUEST, code); 645 if ("DeviceRequest".equals(codeString)) 646 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.DEVICEREQUEST, code); 647 if ("MedicationRequest".equals(codeString)) 648 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.MEDICATIONREQUEST, code); 649 if ("NutritionOrder".equals(codeString)) 650 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.NUTRITIONORDER, code); 651 if ("Task".equals(codeString)) 652 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.TASK, code); 653 if ("ServiceRequest".equals(codeString)) 654 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.SERVICEREQUEST, code); 655 if ("VisionPrescription".equals(codeString)) 656 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.VISIONPRESCRIPTION, code); 657 throw new FHIRException("Unknown CarePlanActivityKind code '" + codeString + "'"); 658 } 659 660 public String toCode(CarePlanActivityKind code) { 661 if (code == CarePlanActivityKind.APPOINTMENT) 662 return "Appointment"; 663 if (code == CarePlanActivityKind.COMMUNICATIONREQUEST) 664 return "CommunicationRequest"; 665 if (code == CarePlanActivityKind.DEVICEREQUEST) 666 return "DeviceRequest"; 667 if (code == CarePlanActivityKind.MEDICATIONREQUEST) 668 return "MedicationRequest"; 669 if (code == CarePlanActivityKind.NUTRITIONORDER) 670 return "NutritionOrder"; 671 if (code == CarePlanActivityKind.TASK) 672 return "Task"; 673 if (code == CarePlanActivityKind.SERVICEREQUEST) 674 return "ServiceRequest"; 675 if (code == CarePlanActivityKind.VISIONPRESCRIPTION) 676 return "VisionPrescription"; 677 return "?"; 678 } 679 680 public String toSystem(CarePlanActivityKind code) { 681 return code.getSystem(); 682 } 683 } 684 685 public enum CarePlanActivityStatus { 686 /** 687 * Care plan activity is planned but no action has yet been taken. 688 */ 689 NOTSTARTED, 690 /** 691 * Appointment or other booking has occurred but activity has not yet begun. 692 */ 693 SCHEDULED, 694 /** 695 * Care plan activity has been started but is not yet complete. 696 */ 697 INPROGRESS, 698 /** 699 * Care plan activity was started but has temporarily ceased with an expectation 700 * of resumption at a future time. 701 */ 702 ONHOLD, 703 /** 704 * Care plan activity has been completed (more or less) as planned. 705 */ 706 COMPLETED, 707 /** 708 * The planned care plan activity has been withdrawn. 709 */ 710 CANCELLED, 711 /** 712 * The planned care plan activity has been ended prior to completion after the 713 * activity was started. 714 */ 715 STOPPED, 716 /** 717 * The current state of the care plan activity is not known. Note: This concept 718 * is not to be used for "other" - one of the listed statuses is presumed to 719 * apply, but the authoring/source system does not know which one. 720 */ 721 UNKNOWN, 722 /** 723 * Care plan activity was entered in error and voided. 724 */ 725 ENTEREDINERROR, 726 /** 727 * added to help the parsers with the generic types 728 */ 729 NULL; 730 731 public static CarePlanActivityStatus fromCode(String codeString) throws FHIRException { 732 if (codeString == null || "".equals(codeString)) 733 return null; 734 if ("not-started".equals(codeString)) 735 return NOTSTARTED; 736 if ("scheduled".equals(codeString)) 737 return SCHEDULED; 738 if ("in-progress".equals(codeString)) 739 return INPROGRESS; 740 if ("on-hold".equals(codeString)) 741 return ONHOLD; 742 if ("completed".equals(codeString)) 743 return COMPLETED; 744 if ("cancelled".equals(codeString)) 745 return CANCELLED; 746 if ("stopped".equals(codeString)) 747 return STOPPED; 748 if ("unknown".equals(codeString)) 749 return UNKNOWN; 750 if ("entered-in-error".equals(codeString)) 751 return ENTEREDINERROR; 752 if (Configuration.isAcceptInvalidEnums()) 753 return null; 754 else 755 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 756 } 757 758 public String toCode() { 759 switch (this) { 760 case NOTSTARTED: 761 return "not-started"; 762 case SCHEDULED: 763 return "scheduled"; 764 case INPROGRESS: 765 return "in-progress"; 766 case ONHOLD: 767 return "on-hold"; 768 case COMPLETED: 769 return "completed"; 770 case CANCELLED: 771 return "cancelled"; 772 case STOPPED: 773 return "stopped"; 774 case UNKNOWN: 775 return "unknown"; 776 case ENTEREDINERROR: 777 return "entered-in-error"; 778 case NULL: 779 return null; 780 default: 781 return "?"; 782 } 783 } 784 785 public String getSystem() { 786 switch (this) { 787 case NOTSTARTED: 788 return "http://hl7.org/fhir/care-plan-activity-status"; 789 case SCHEDULED: 790 return "http://hl7.org/fhir/care-plan-activity-status"; 791 case INPROGRESS: 792 return "http://hl7.org/fhir/care-plan-activity-status"; 793 case ONHOLD: 794 return "http://hl7.org/fhir/care-plan-activity-status"; 795 case COMPLETED: 796 return "http://hl7.org/fhir/care-plan-activity-status"; 797 case CANCELLED: 798 return "http://hl7.org/fhir/care-plan-activity-status"; 799 case STOPPED: 800 return "http://hl7.org/fhir/care-plan-activity-status"; 801 case UNKNOWN: 802 return "http://hl7.org/fhir/care-plan-activity-status"; 803 case ENTEREDINERROR: 804 return "http://hl7.org/fhir/care-plan-activity-status"; 805 case NULL: 806 return null; 807 default: 808 return "?"; 809 } 810 } 811 812 public String getDefinition() { 813 switch (this) { 814 case NOTSTARTED: 815 return "Care plan activity is planned but no action has yet been taken."; 816 case SCHEDULED: 817 return "Appointment or other booking has occurred but activity has not yet begun."; 818 case INPROGRESS: 819 return "Care plan activity has been started but is not yet complete."; 820 case ONHOLD: 821 return "Care plan activity was started but has temporarily ceased with an expectation of resumption at a future time."; 822 case COMPLETED: 823 return "Care plan activity has been completed (more or less) as planned."; 824 case CANCELLED: 825 return "The planned care plan activity has been withdrawn."; 826 case STOPPED: 827 return "The planned care plan activity has been ended prior to completion after the activity was started."; 828 case UNKNOWN: 829 return "The current state of the care plan activity is not known. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which one."; 830 case ENTEREDINERROR: 831 return "Care plan activity was entered in error and voided."; 832 case NULL: 833 return null; 834 default: 835 return "?"; 836 } 837 } 838 839 public String getDisplay() { 840 switch (this) { 841 case NOTSTARTED: 842 return "Not Started"; 843 case SCHEDULED: 844 return "Scheduled"; 845 case INPROGRESS: 846 return "In Progress"; 847 case ONHOLD: 848 return "On Hold"; 849 case COMPLETED: 850 return "Completed"; 851 case CANCELLED: 852 return "Cancelled"; 853 case STOPPED: 854 return "Stopped"; 855 case UNKNOWN: 856 return "Unknown"; 857 case ENTEREDINERROR: 858 return "Entered in Error"; 859 case NULL: 860 return null; 861 default: 862 return "?"; 863 } 864 } 865 } 866 867 public static class CarePlanActivityStatusEnumFactory implements EnumFactory<CarePlanActivityStatus> { 868 public CarePlanActivityStatus fromCode(String codeString) throws IllegalArgumentException { 869 if (codeString == null || "".equals(codeString)) 870 if (codeString == null || "".equals(codeString)) 871 return null; 872 if ("not-started".equals(codeString)) 873 return CarePlanActivityStatus.NOTSTARTED; 874 if ("scheduled".equals(codeString)) 875 return CarePlanActivityStatus.SCHEDULED; 876 if ("in-progress".equals(codeString)) 877 return CarePlanActivityStatus.INPROGRESS; 878 if ("on-hold".equals(codeString)) 879 return CarePlanActivityStatus.ONHOLD; 880 if ("completed".equals(codeString)) 881 return CarePlanActivityStatus.COMPLETED; 882 if ("cancelled".equals(codeString)) 883 return CarePlanActivityStatus.CANCELLED; 884 if ("stopped".equals(codeString)) 885 return CarePlanActivityStatus.STOPPED; 886 if ("unknown".equals(codeString)) 887 return CarePlanActivityStatus.UNKNOWN; 888 if ("entered-in-error".equals(codeString)) 889 return CarePlanActivityStatus.ENTEREDINERROR; 890 throw new IllegalArgumentException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 891 } 892 893 public Enumeration<CarePlanActivityStatus> fromType(PrimitiveType<?> code) throws FHIRException { 894 if (code == null) 895 return null; 896 if (code.isEmpty()) 897 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NULL, code); 898 String codeString = code.asStringValue(); 899 if (codeString == null || "".equals(codeString)) 900 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NULL, code); 901 if ("not-started".equals(codeString)) 902 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NOTSTARTED, code); 903 if ("scheduled".equals(codeString)) 904 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.SCHEDULED, code); 905 if ("in-progress".equals(codeString)) 906 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.INPROGRESS, code); 907 if ("on-hold".equals(codeString)) 908 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.ONHOLD, code); 909 if ("completed".equals(codeString)) 910 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.COMPLETED, code); 911 if ("cancelled".equals(codeString)) 912 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.CANCELLED, code); 913 if ("stopped".equals(codeString)) 914 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.STOPPED, code); 915 if ("unknown".equals(codeString)) 916 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.UNKNOWN, code); 917 if ("entered-in-error".equals(codeString)) 918 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.ENTEREDINERROR, code); 919 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 920 } 921 922 public String toCode(CarePlanActivityStatus code) { 923 if (code == CarePlanActivityStatus.NOTSTARTED) 924 return "not-started"; 925 if (code == CarePlanActivityStatus.SCHEDULED) 926 return "scheduled"; 927 if (code == CarePlanActivityStatus.INPROGRESS) 928 return "in-progress"; 929 if (code == CarePlanActivityStatus.ONHOLD) 930 return "on-hold"; 931 if (code == CarePlanActivityStatus.COMPLETED) 932 return "completed"; 933 if (code == CarePlanActivityStatus.CANCELLED) 934 return "cancelled"; 935 if (code == CarePlanActivityStatus.STOPPED) 936 return "stopped"; 937 if (code == CarePlanActivityStatus.UNKNOWN) 938 return "unknown"; 939 if (code == CarePlanActivityStatus.ENTEREDINERROR) 940 return "entered-in-error"; 941 return "?"; 942 } 943 944 public String toSystem(CarePlanActivityStatus code) { 945 return code.getSystem(); 946 } 947 } 948 949 @Block() 950 public static class CarePlanActivityComponent extends BackboneElement implements IBaseBackboneElement { 951 /** 952 * Identifies the outcome at the point when the status of the activity is 953 * assessed. For example, the outcome of an education activity could be patient 954 * understands (or not). 955 */ 956 @Child(name = "outcomeCodeableConcept", type = { 957 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 958 @Description(shortDefinition = "Results of the activity", formalDefinition = "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).") 959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-activity-outcome") 960 protected List<CodeableConcept> outcomeCodeableConcept; 961 962 /** 963 * Details of the outcome or action resulting from the activity. The reference 964 * to an "event" resource, such as Procedure or Encounter or Observation, is the 965 * result/outcome of the activity itself. The activity can be conveyed using 966 * CarePlan.activity.detail OR using the CarePlan.activity.reference (a 967 * reference to a ?request? resource). 968 */ 969 @Child(name = "outcomeReference", type = { 970 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 971 @Description(shortDefinition = "Appointment, Encounter, Procedure, etc.", formalDefinition = "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).") 972 protected List<Reference> outcomeReference; 973 /** 974 * The actual objects that are the target of the reference (Details of the 975 * outcome or action resulting from the activity. The reference to an "event" 976 * resource, such as Procedure or Encounter or Observation, is the 977 * result/outcome of the activity itself. The activity can be conveyed using 978 * CarePlan.activity.detail OR using the CarePlan.activity.reference (a 979 * reference to a ?request? resource).) 980 */ 981 protected List<Resource> outcomeReferenceTarget; 982 983 /** 984 * Notes about the adherence/status/progress of the activity. 985 */ 986 @Child(name = "progress", type = { 987 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 988 @Description(shortDefinition = "Comments about the activity status/progress", formalDefinition = "Notes about the adherence/status/progress of the activity.") 989 protected List<Annotation> progress; 990 991 /** 992 * The details of the proposed activity represented in a specific resource. 993 */ 994 @Child(name = "reference", type = { Appointment.class, CommunicationRequest.class, DeviceRequest.class, 995 MedicationRequest.class, NutritionOrder.class, Task.class, ServiceRequest.class, VisionPrescription.class, 996 RequestGroup.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 997 @Description(shortDefinition = "Activity details defined in specific resource", formalDefinition = "The details of the proposed activity represented in a specific resource.") 998 protected Reference reference; 999 1000 /** 1001 * The actual object that is the target of the reference (The details of the 1002 * proposed activity represented in a specific resource.) 1003 */ 1004 protected Resource referenceTarget; 1005 1006 /** 1007 * A simple summary of a planned activity suitable for a general care plan 1008 * system (e.g. form driven) that doesn't know about specific resources such as 1009 * procedure etc. 1010 */ 1011 @Child(name = "detail", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = false) 1012 @Description(shortDefinition = "In-line definition of activity", formalDefinition = "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.") 1013 protected CarePlanActivityDetailComponent detail; 1014 1015 private static final long serialVersionUID = -609287300L; 1016 1017 /** 1018 * Constructor 1019 */ 1020 public CarePlanActivityComponent() { 1021 super(); 1022 } 1023 1024 /** 1025 * @return {@link #outcomeCodeableConcept} (Identifies the outcome at the point 1026 * when the status of the activity is assessed. For example, the outcome 1027 * of an education activity could be patient understands (or not).) 1028 */ 1029 public List<CodeableConcept> getOutcomeCodeableConcept() { 1030 if (this.outcomeCodeableConcept == null) 1031 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1032 return this.outcomeCodeableConcept; 1033 } 1034 1035 /** 1036 * @return Returns a reference to <code>this</code> for easy method chaining 1037 */ 1038 public CarePlanActivityComponent setOutcomeCodeableConcept(List<CodeableConcept> theOutcomeCodeableConcept) { 1039 this.outcomeCodeableConcept = theOutcomeCodeableConcept; 1040 return this; 1041 } 1042 1043 public boolean hasOutcomeCodeableConcept() { 1044 if (this.outcomeCodeableConcept == null) 1045 return false; 1046 for (CodeableConcept item : this.outcomeCodeableConcept) 1047 if (!item.isEmpty()) 1048 return true; 1049 return false; 1050 } 1051 1052 public CodeableConcept addOutcomeCodeableConcept() { // 3 1053 CodeableConcept t = new CodeableConcept(); 1054 if (this.outcomeCodeableConcept == null) 1055 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1056 this.outcomeCodeableConcept.add(t); 1057 return t; 1058 } 1059 1060 public CarePlanActivityComponent addOutcomeCodeableConcept(CodeableConcept t) { // 3 1061 if (t == null) 1062 return this; 1063 if (this.outcomeCodeableConcept == null) 1064 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1065 this.outcomeCodeableConcept.add(t); 1066 return this; 1067 } 1068 1069 /** 1070 * @return The first repetition of repeating field 1071 * {@link #outcomeCodeableConcept}, creating it if it does not already 1072 * exist 1073 */ 1074 public CodeableConcept getOutcomeCodeableConceptFirstRep() { 1075 if (getOutcomeCodeableConcept().isEmpty()) { 1076 addOutcomeCodeableConcept(); 1077 } 1078 return getOutcomeCodeableConcept().get(0); 1079 } 1080 1081 /** 1082 * @return {@link #outcomeReference} (Details of the outcome or action resulting 1083 * from the activity. The reference to an "event" resource, such as 1084 * Procedure or Encounter or Observation, is the result/outcome of the 1085 * activity itself. The activity can be conveyed using 1086 * CarePlan.activity.detail OR using the CarePlan.activity.reference (a 1087 * reference to a ?request? resource).) 1088 */ 1089 public List<Reference> getOutcomeReference() { 1090 if (this.outcomeReference == null) 1091 this.outcomeReference = new ArrayList<Reference>(); 1092 return this.outcomeReference; 1093 } 1094 1095 /** 1096 * @return Returns a reference to <code>this</code> for easy method chaining 1097 */ 1098 public CarePlanActivityComponent setOutcomeReference(List<Reference> theOutcomeReference) { 1099 this.outcomeReference = theOutcomeReference; 1100 return this; 1101 } 1102 1103 public boolean hasOutcomeReference() { 1104 if (this.outcomeReference == null) 1105 return false; 1106 for (Reference item : this.outcomeReference) 1107 if (!item.isEmpty()) 1108 return true; 1109 return false; 1110 } 1111 1112 public Reference addOutcomeReference() { // 3 1113 Reference t = new Reference(); 1114 if (this.outcomeReference == null) 1115 this.outcomeReference = new ArrayList<Reference>(); 1116 this.outcomeReference.add(t); 1117 return t; 1118 } 1119 1120 public CarePlanActivityComponent addOutcomeReference(Reference t) { // 3 1121 if (t == null) 1122 return this; 1123 if (this.outcomeReference == null) 1124 this.outcomeReference = new ArrayList<Reference>(); 1125 this.outcomeReference.add(t); 1126 return this; 1127 } 1128 1129 /** 1130 * @return The first repetition of repeating field {@link #outcomeReference}, 1131 * creating it if it does not already exist 1132 */ 1133 public Reference getOutcomeReferenceFirstRep() { 1134 if (getOutcomeReference().isEmpty()) { 1135 addOutcomeReference(); 1136 } 1137 return getOutcomeReference().get(0); 1138 } 1139 1140 /** 1141 * @deprecated Use Reference#setResource(IBaseResource) instead 1142 */ 1143 @Deprecated 1144 public List<Resource> getOutcomeReferenceTarget() { 1145 if (this.outcomeReferenceTarget == null) 1146 this.outcomeReferenceTarget = new ArrayList<Resource>(); 1147 return this.outcomeReferenceTarget; 1148 } 1149 1150 /** 1151 * @return {@link #progress} (Notes about the adherence/status/progress of the 1152 * activity.) 1153 */ 1154 public List<Annotation> getProgress() { 1155 if (this.progress == null) 1156 this.progress = new ArrayList<Annotation>(); 1157 return this.progress; 1158 } 1159 1160 /** 1161 * @return Returns a reference to <code>this</code> for easy method chaining 1162 */ 1163 public CarePlanActivityComponent setProgress(List<Annotation> theProgress) { 1164 this.progress = theProgress; 1165 return this; 1166 } 1167 1168 public boolean hasProgress() { 1169 if (this.progress == null) 1170 return false; 1171 for (Annotation item : this.progress) 1172 if (!item.isEmpty()) 1173 return true; 1174 return false; 1175 } 1176 1177 public Annotation addProgress() { // 3 1178 Annotation t = new Annotation(); 1179 if (this.progress == null) 1180 this.progress = new ArrayList<Annotation>(); 1181 this.progress.add(t); 1182 return t; 1183 } 1184 1185 public CarePlanActivityComponent addProgress(Annotation t) { // 3 1186 if (t == null) 1187 return this; 1188 if (this.progress == null) 1189 this.progress = new ArrayList<Annotation>(); 1190 this.progress.add(t); 1191 return this; 1192 } 1193 1194 /** 1195 * @return The first repetition of repeating field {@link #progress}, creating 1196 * it if it does not already exist 1197 */ 1198 public Annotation getProgressFirstRep() { 1199 if (getProgress().isEmpty()) { 1200 addProgress(); 1201 } 1202 return getProgress().get(0); 1203 } 1204 1205 /** 1206 * @return {@link #reference} (The details of the proposed activity represented 1207 * in a specific resource.) 1208 */ 1209 public Reference getReference() { 1210 if (this.reference == null) 1211 if (Configuration.errorOnAutoCreate()) 1212 throw new Error("Attempt to auto-create CarePlanActivityComponent.reference"); 1213 else if (Configuration.doAutoCreate()) 1214 this.reference = new Reference(); // cc 1215 return this.reference; 1216 } 1217 1218 public boolean hasReference() { 1219 return this.reference != null && !this.reference.isEmpty(); 1220 } 1221 1222 /** 1223 * @param value {@link #reference} (The details of the proposed activity 1224 * represented in a specific resource.) 1225 */ 1226 public CarePlanActivityComponent setReference(Reference value) { 1227 this.reference = value; 1228 return this; 1229 } 1230 1231 /** 1232 * @return {@link #reference} The actual object that is the target of the 1233 * reference. The reference library doesn't populate this, but you can 1234 * use it to hold the resource if you resolve it. (The details of the 1235 * proposed activity represented in a specific resource.) 1236 */ 1237 public Resource getReferenceTarget() { 1238 return this.referenceTarget; 1239 } 1240 1241 /** 1242 * @param value {@link #reference} The actual object that is the target of the 1243 * reference. The reference library doesn't use these, but you can 1244 * use it to hold the resource if you resolve it. (The details of 1245 * the proposed activity represented in a specific resource.) 1246 */ 1247 public CarePlanActivityComponent setReferenceTarget(Resource value) { 1248 this.referenceTarget = value; 1249 return this; 1250 } 1251 1252 /** 1253 * @return {@link #detail} (A simple summary of a planned activity suitable for 1254 * a general care plan system (e.g. form driven) that doesn't know about 1255 * specific resources such as procedure etc.) 1256 */ 1257 public CarePlanActivityDetailComponent getDetail() { 1258 if (this.detail == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create CarePlanActivityComponent.detail"); 1261 else if (Configuration.doAutoCreate()) 1262 this.detail = new CarePlanActivityDetailComponent(); // cc 1263 return this.detail; 1264 } 1265 1266 public boolean hasDetail() { 1267 return this.detail != null && !this.detail.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #detail} (A simple summary of a planned activity suitable 1272 * for a general care plan system (e.g. form driven) that doesn't 1273 * know about specific resources such as procedure etc.) 1274 */ 1275 public CarePlanActivityComponent setDetail(CarePlanActivityDetailComponent value) { 1276 this.detail = value; 1277 return this; 1278 } 1279 1280 protected void listChildren(List<Property> children) { 1281 super.listChildren(children); 1282 children.add(new Property("outcomeCodeableConcept", "CodeableConcept", 1283 "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).", 1284 0, java.lang.Integer.MAX_VALUE, outcomeCodeableConcept)); 1285 children.add(new Property("outcomeReference", "Reference(Any)", 1286 "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).", 1287 0, java.lang.Integer.MAX_VALUE, outcomeReference)); 1288 children.add(new Property("progress", "Annotation", "Notes about the adherence/status/progress of the activity.", 1289 0, java.lang.Integer.MAX_VALUE, progress)); 1290 children.add(new Property("reference", 1291 "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ServiceRequest|VisionPrescription|RequestGroup)", 1292 "The details of the proposed activity represented in a specific resource.", 0, 1, reference)); 1293 children.add(new Property("detail", "", 1294 "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 1295 0, 1, detail)); 1296 } 1297 1298 @Override 1299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1300 switch (_hash) { 1301 case -511913489: 1302 /* outcomeCodeableConcept */ return new Property("outcomeCodeableConcept", "CodeableConcept", 1303 "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).", 1304 0, java.lang.Integer.MAX_VALUE, outcomeCodeableConcept); 1305 case -782273511: 1306 /* outcomeReference */ return new Property("outcomeReference", "Reference(Any)", 1307 "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).", 1308 0, java.lang.Integer.MAX_VALUE, outcomeReference); 1309 case -1001078227: 1310 /* progress */ return new Property("progress", "Annotation", 1311 "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress); 1312 case -925155509: 1313 /* reference */ return new Property("reference", 1314 "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ServiceRequest|VisionPrescription|RequestGroup)", 1315 "The details of the proposed activity represented in a specific resource.", 0, 1, reference); 1316 case -1335224239: 1317 /* detail */ return new Property("detail", "", 1318 "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 1319 0, 1, detail); 1320 default: 1321 return super.getNamedProperty(_hash, _name, _checkValid); 1322 } 1323 1324 } 1325 1326 @Override 1327 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1328 switch (hash) { 1329 case -511913489: 1330 /* outcomeCodeableConcept */ return this.outcomeCodeableConcept == null ? new Base[0] 1331 : this.outcomeCodeableConcept.toArray(new Base[this.outcomeCodeableConcept.size()]); // CodeableConcept 1332 case -782273511: 1333 /* outcomeReference */ return this.outcomeReference == null ? new Base[0] 1334 : this.outcomeReference.toArray(new Base[this.outcomeReference.size()]); // Reference 1335 case -1001078227: 1336 /* progress */ return this.progress == null ? new Base[0] 1337 : this.progress.toArray(new Base[this.progress.size()]); // Annotation 1338 case -925155509: 1339 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 1340 case -1335224239: 1341 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // CarePlanActivityDetailComponent 1342 default: 1343 return super.getProperty(hash, name, checkValid); 1344 } 1345 1346 } 1347 1348 @Override 1349 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1350 switch (hash) { 1351 case -511913489: // outcomeCodeableConcept 1352 this.getOutcomeCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 1353 return value; 1354 case -782273511: // outcomeReference 1355 this.getOutcomeReference().add(castToReference(value)); // Reference 1356 return value; 1357 case -1001078227: // progress 1358 this.getProgress().add(castToAnnotation(value)); // Annotation 1359 return value; 1360 case -925155509: // reference 1361 this.reference = castToReference(value); // Reference 1362 return value; 1363 case -1335224239: // detail 1364 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1365 return value; 1366 default: 1367 return super.setProperty(hash, name, value); 1368 } 1369 1370 } 1371 1372 @Override 1373 public Base setProperty(String name, Base value) throws FHIRException { 1374 if (name.equals("outcomeCodeableConcept")) { 1375 this.getOutcomeCodeableConcept().add(castToCodeableConcept(value)); 1376 } else if (name.equals("outcomeReference")) { 1377 this.getOutcomeReference().add(castToReference(value)); 1378 } else if (name.equals("progress")) { 1379 this.getProgress().add(castToAnnotation(value)); 1380 } else if (name.equals("reference")) { 1381 this.reference = castToReference(value); // Reference 1382 } else if (name.equals("detail")) { 1383 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1384 } else 1385 return super.setProperty(name, value); 1386 return value; 1387 } 1388 1389 @Override 1390 public void removeChild(String name, Base value) throws FHIRException { 1391 if (name.equals("outcomeCodeableConcept")) { 1392 this.getOutcomeCodeableConcept().remove(castToCodeableConcept(value)); 1393 } else if (name.equals("outcomeReference")) { 1394 this.getOutcomeReference().remove(castToReference(value)); 1395 } else if (name.equals("progress")) { 1396 this.getProgress().remove(castToAnnotation(value)); 1397 } else if (name.equals("reference")) { 1398 this.reference = null; 1399 } else if (name.equals("detail")) { 1400 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1401 } else 1402 super.removeChild(name, value); 1403 1404 } 1405 1406 @Override 1407 public Base makeProperty(int hash, String name) throws FHIRException { 1408 switch (hash) { 1409 case -511913489: 1410 return addOutcomeCodeableConcept(); 1411 case -782273511: 1412 return addOutcomeReference(); 1413 case -1001078227: 1414 return addProgress(); 1415 case -925155509: 1416 return getReference(); 1417 case -1335224239: 1418 return getDetail(); 1419 default: 1420 return super.makeProperty(hash, name); 1421 } 1422 1423 } 1424 1425 @Override 1426 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1427 switch (hash) { 1428 case -511913489: 1429 /* outcomeCodeableConcept */ return new String[] { "CodeableConcept" }; 1430 case -782273511: 1431 /* outcomeReference */ return new String[] { "Reference" }; 1432 case -1001078227: 1433 /* progress */ return new String[] { "Annotation" }; 1434 case -925155509: 1435 /* reference */ return new String[] { "Reference" }; 1436 case -1335224239: 1437 /* detail */ return new String[] {}; 1438 default: 1439 return super.getTypesForProperty(hash, name); 1440 } 1441 1442 } 1443 1444 @Override 1445 public Base addChild(String name) throws FHIRException { 1446 if (name.equals("outcomeCodeableConcept")) { 1447 return addOutcomeCodeableConcept(); 1448 } else if (name.equals("outcomeReference")) { 1449 return addOutcomeReference(); 1450 } else if (name.equals("progress")) { 1451 return addProgress(); 1452 } else if (name.equals("reference")) { 1453 this.reference = new Reference(); 1454 return this.reference; 1455 } else if (name.equals("detail")) { 1456 this.detail = new CarePlanActivityDetailComponent(); 1457 return this.detail; 1458 } else 1459 return super.addChild(name); 1460 } 1461 1462 public CarePlanActivityComponent copy() { 1463 CarePlanActivityComponent dst = new CarePlanActivityComponent(); 1464 copyValues(dst); 1465 return dst; 1466 } 1467 1468 public void copyValues(CarePlanActivityComponent dst) { 1469 super.copyValues(dst); 1470 if (outcomeCodeableConcept != null) { 1471 dst.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1472 for (CodeableConcept i : outcomeCodeableConcept) 1473 dst.outcomeCodeableConcept.add(i.copy()); 1474 } 1475 ; 1476 if (outcomeReference != null) { 1477 dst.outcomeReference = new ArrayList<Reference>(); 1478 for (Reference i : outcomeReference) 1479 dst.outcomeReference.add(i.copy()); 1480 } 1481 ; 1482 if (progress != null) { 1483 dst.progress = new ArrayList<Annotation>(); 1484 for (Annotation i : progress) 1485 dst.progress.add(i.copy()); 1486 } 1487 ; 1488 dst.reference = reference == null ? null : reference.copy(); 1489 dst.detail = detail == null ? null : detail.copy(); 1490 } 1491 1492 @Override 1493 public boolean equalsDeep(Base other_) { 1494 if (!super.equalsDeep(other_)) 1495 return false; 1496 if (!(other_ instanceof CarePlanActivityComponent)) 1497 return false; 1498 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 1499 return compareDeep(outcomeCodeableConcept, o.outcomeCodeableConcept, true) 1500 && compareDeep(outcomeReference, o.outcomeReference, true) && compareDeep(progress, o.progress, true) 1501 && compareDeep(reference, o.reference, true) && compareDeep(detail, o.detail, true); 1502 } 1503 1504 @Override 1505 public boolean equalsShallow(Base other_) { 1506 if (!super.equalsShallow(other_)) 1507 return false; 1508 if (!(other_ instanceof CarePlanActivityComponent)) 1509 return false; 1510 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 1511 return true; 1512 } 1513 1514 public boolean isEmpty() { 1515 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(outcomeCodeableConcept, outcomeReference, progress, 1516 reference, detail); 1517 } 1518 1519 public String fhirType() { 1520 return "CarePlan.activity"; 1521 1522 } 1523 1524 } 1525 1526 @Block() 1527 public static class CarePlanActivityDetailComponent extends BackboneElement implements IBaseBackboneElement { 1528 /** 1529 * A description of the kind of resource the in-line definition of a care plan 1530 * activity is representing. The CarePlan.activity.detail is an in-line 1531 * definition when a resource is not referenced using 1532 * CarePlan.activity.reference. For example, a MedicationRequest, a 1533 * ServiceRequest, or a CommunicationRequest. 1534 */ 1535 @Child(name = "kind", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1536 @Description(shortDefinition = "Appointment | CommunicationRequest | DeviceRequest | MedicationRequest | NutritionOrder | Task | ServiceRequest | VisionPrescription", formalDefinition = "A description of the kind of resource the in-line definition of a care plan activity is representing. The CarePlan.activity.detail is an in-line definition when a resource is not referenced using CarePlan.activity.reference. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.") 1537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-activity-kind") 1538 protected Enumeration<CarePlanActivityKind> kind; 1539 1540 /** 1541 * The URL pointing to a FHIR-defined protocol, guideline, questionnaire or 1542 * other definition that is adhered to in whole or in part by this CarePlan 1543 * activity. 1544 */ 1545 @Child(name = "instantiatesCanonical", type = { 1546 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1547 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.") 1548 protected List<CanonicalType> instantiatesCanonical; 1549 1550 /** 1551 * The URL pointing to an externally maintained protocol, guideline, 1552 * questionnaire or other definition that is adhered to in whole or in part by 1553 * this CarePlan activity. 1554 */ 1555 @Child(name = "instantiatesUri", type = { 1556 UriType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1557 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.") 1558 protected List<UriType> instantiatesUri; 1559 1560 /** 1561 * Detailed description of the type of planned activity; e.g. what lab test, 1562 * what procedure, what kind of encounter. 1563 */ 1564 @Child(name = "code", type = { 1565 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1566 @Description(shortDefinition = "Detail type of activity", formalDefinition = "Detailed description of the type of planned activity; e.g. what lab test, what procedure, what kind of encounter.") 1567 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 1568 protected CodeableConcept code; 1569 1570 /** 1571 * Provides the rationale that drove the inclusion of this particular activity 1572 * as part of the plan or the reason why the activity was prohibited. 1573 */ 1574 @Child(name = "reasonCode", type = { 1575 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1576 @Description(shortDefinition = "Why activity should be done or why activity was prohibited", formalDefinition = "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.") 1577 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 1578 protected List<CodeableConcept> reasonCode; 1579 1580 /** 1581 * Indicates another resource, such as the health condition(s), whose existence 1582 * justifies this request and drove the inclusion of this particular activity as 1583 * part of the plan. 1584 */ 1585 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 1586 DocumentReference.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1587 @Description(shortDefinition = "Why activity is needed", formalDefinition = "Indicates another resource, such as the health condition(s), whose existence justifies this request and drove the inclusion of this particular activity as part of the plan.") 1588 protected List<Reference> reasonReference; 1589 /** 1590 * The actual objects that are the target of the reference (Indicates another 1591 * resource, such as the health condition(s), whose existence justifies this 1592 * request and drove the inclusion of this particular activity as part of the 1593 * plan.) 1594 */ 1595 protected List<Resource> reasonReferenceTarget; 1596 1597 /** 1598 * Internal reference that identifies the goals that this activity is intended 1599 * to contribute towards meeting. 1600 */ 1601 @Child(name = "goal", type = { 1602 Goal.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1603 @Description(shortDefinition = "Goals this activity relates to", formalDefinition = "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.") 1604 protected List<Reference> goal; 1605 /** 1606 * The actual objects that are the target of the reference (Internal reference 1607 * that identifies the goals that this activity is intended to contribute 1608 * towards meeting.) 1609 */ 1610 protected List<Goal> goalTarget; 1611 1612 /** 1613 * Identifies what progress is being made for the specific activity. 1614 */ 1615 @Child(name = "status", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = true, summary = false) 1616 @Description(shortDefinition = "not-started | scheduled | in-progress | on-hold | completed | cancelled | stopped | unknown | entered-in-error", formalDefinition = "Identifies what progress is being made for the specific activity.") 1617 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-activity-status") 1618 protected Enumeration<CarePlanActivityStatus> status; 1619 1620 /** 1621 * Provides reason why the activity isn't yet started, is on hold, was 1622 * cancelled, etc. 1623 */ 1624 @Child(name = "statusReason", type = { 1625 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1626 @Description(shortDefinition = "Reason for current status", formalDefinition = "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.") 1627 protected CodeableConcept statusReason; 1628 1629 /** 1630 * If true, indicates that the described activity is one that must NOT be 1631 * engaged in when following the plan. If false, or missing, indicates that the 1632 * described activity is one that should be engaged in when following the plan. 1633 */ 1634 @Child(name = "doNotPerform", type = { 1635 BooleanType.class }, order = 10, min = 0, max = 1, modifier = true, summary = false) 1636 @Description(shortDefinition = "If true, activity is prohibiting action", formalDefinition = "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, or missing, indicates that the described activity is one that should be engaged in when following the plan.") 1637 protected BooleanType doNotPerform; 1638 1639 /** 1640 * The period, timing or frequency upon which the described activity is to 1641 * occur. 1642 */ 1643 @Child(name = "scheduled", type = { Timing.class, Period.class, 1644 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1645 @Description(shortDefinition = "When activity is to occur", formalDefinition = "The period, timing or frequency upon which the described activity is to occur.") 1646 protected Type scheduled; 1647 1648 /** 1649 * Identifies the facility where the activity will occur; e.g. home, hospital, 1650 * specific clinic, etc. 1651 */ 1652 @Child(name = "location", type = { 1653 Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1654 @Description(shortDefinition = "Where it should happen", formalDefinition = "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.") 1655 protected Reference location; 1656 1657 /** 1658 * The actual object that is the target of the reference (Identifies the 1659 * facility where the activity will occur; e.g. home, hospital, specific clinic, 1660 * etc.) 1661 */ 1662 protected Location locationTarget; 1663 1664 /** 1665 * Identifies who's expected to be involved in the activity. 1666 */ 1667 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, 1668 RelatedPerson.class, Patient.class, CareTeam.class, HealthcareService.class, 1669 Device.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1670 @Description(shortDefinition = "Who will be responsible?", formalDefinition = "Identifies who's expected to be involved in the activity.") 1671 protected List<Reference> performer; 1672 /** 1673 * The actual objects that are the target of the reference (Identifies who's 1674 * expected to be involved in the activity.) 1675 */ 1676 protected List<Resource> performerTarget; 1677 1678 /** 1679 * Identifies the food, drug or other product to be consumed or supplied in the 1680 * activity. 1681 */ 1682 @Child(name = "product", type = { CodeableConcept.class, Medication.class, 1683 Substance.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1684 @Description(shortDefinition = "What is to be administered/supplied", formalDefinition = "Identifies the food, drug or other product to be consumed or supplied in the activity.") 1685 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1686 protected Type product; 1687 1688 /** 1689 * Identifies the quantity expected to be consumed in a given day. 1690 */ 1691 @Child(name = "dailyAmount", type = { 1692 Quantity.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1693 @Description(shortDefinition = "How to consume/day?", formalDefinition = "Identifies the quantity expected to be consumed in a given day.") 1694 protected Quantity dailyAmount; 1695 1696 /** 1697 * Identifies the quantity expected to be supplied, administered or consumed by 1698 * the subject. 1699 */ 1700 @Child(name = "quantity", type = { 1701 Quantity.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1702 @Description(shortDefinition = "How much to administer/supply/consume", formalDefinition = "Identifies the quantity expected to be supplied, administered or consumed by the subject.") 1703 protected Quantity quantity; 1704 1705 /** 1706 * This provides a textual description of constraints on the intended activity 1707 * occurrence, including relation to other activities. It may also include 1708 * objectives, pre-conditions and end-conditions. Finally, it may convey 1709 * specifics about the activity such as body site, method, route, etc. 1710 */ 1711 @Child(name = "description", type = { 1712 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1713 @Description(shortDefinition = "Extra info describing activity to perform", formalDefinition = "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.") 1714 protected StringType description; 1715 1716 private static final long serialVersionUID = 1355568081L; 1717 1718 /** 1719 * Constructor 1720 */ 1721 public CarePlanActivityDetailComponent() { 1722 super(); 1723 } 1724 1725 /** 1726 * Constructor 1727 */ 1728 public CarePlanActivityDetailComponent(Enumeration<CarePlanActivityStatus> status) { 1729 super(); 1730 this.status = status; 1731 } 1732 1733 /** 1734 * @return {@link #kind} (A description of the kind of resource the in-line 1735 * definition of a care plan activity is representing. The 1736 * CarePlan.activity.detail is an in-line definition when a resource is 1737 * not referenced using CarePlan.activity.reference. For example, a 1738 * MedicationRequest, a ServiceRequest, or a CommunicationRequest.). 1739 * This is the underlying object with id, value and extensions. The 1740 * accessor "getKind" gives direct access to the value 1741 */ 1742 public Enumeration<CarePlanActivityKind> getKindElement() { 1743 if (this.kind == null) 1744 if (Configuration.errorOnAutoCreate()) 1745 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.kind"); 1746 else if (Configuration.doAutoCreate()) 1747 this.kind = new Enumeration<CarePlanActivityKind>(new CarePlanActivityKindEnumFactory()); // bb 1748 return this.kind; 1749 } 1750 1751 public boolean hasKindElement() { 1752 return this.kind != null && !this.kind.isEmpty(); 1753 } 1754 1755 public boolean hasKind() { 1756 return this.kind != null && !this.kind.isEmpty(); 1757 } 1758 1759 /** 1760 * @param value {@link #kind} (A description of the kind of resource the in-line 1761 * definition of a care plan activity is representing. The 1762 * CarePlan.activity.detail is an in-line definition when a 1763 * resource is not referenced using CarePlan.activity.reference. 1764 * For example, a MedicationRequest, a ServiceRequest, or a 1765 * CommunicationRequest.). This is the underlying object with id, 1766 * value and extensions. The accessor "getKind" gives direct access 1767 * to the value 1768 */ 1769 public CarePlanActivityDetailComponent setKindElement(Enumeration<CarePlanActivityKind> value) { 1770 this.kind = value; 1771 return this; 1772 } 1773 1774 /** 1775 * @return A description of the kind of resource the in-line definition of a 1776 * care plan activity is representing. The CarePlan.activity.detail is 1777 * an in-line definition when a resource is not referenced using 1778 * CarePlan.activity.reference. For example, a MedicationRequest, a 1779 * ServiceRequest, or a CommunicationRequest. 1780 */ 1781 public CarePlanActivityKind getKind() { 1782 return this.kind == null ? null : this.kind.getValue(); 1783 } 1784 1785 /** 1786 * @param value A description of the kind of resource the in-line definition of 1787 * a care plan activity is representing. The 1788 * CarePlan.activity.detail is an in-line definition when a 1789 * resource is not referenced using CarePlan.activity.reference. 1790 * For example, a MedicationRequest, a ServiceRequest, or a 1791 * CommunicationRequest. 1792 */ 1793 public CarePlanActivityDetailComponent setKind(CarePlanActivityKind value) { 1794 if (value == null) 1795 this.kind = null; 1796 else { 1797 if (this.kind == null) 1798 this.kind = new Enumeration<CarePlanActivityKind>(new CarePlanActivityKindEnumFactory()); 1799 this.kind.setValue(value); 1800 } 1801 return this; 1802 } 1803 1804 /** 1805 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1806 * protocol, guideline, questionnaire or other definition that is 1807 * adhered to in whole or in part by this CarePlan activity.) 1808 */ 1809 public List<CanonicalType> getInstantiatesCanonical() { 1810 if (this.instantiatesCanonical == null) 1811 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1812 return this.instantiatesCanonical; 1813 } 1814 1815 /** 1816 * @return Returns a reference to <code>this</code> for easy method chaining 1817 */ 1818 public CarePlanActivityDetailComponent setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1819 this.instantiatesCanonical = theInstantiatesCanonical; 1820 return this; 1821 } 1822 1823 public boolean hasInstantiatesCanonical() { 1824 if (this.instantiatesCanonical == null) 1825 return false; 1826 for (CanonicalType item : this.instantiatesCanonical) 1827 if (!item.isEmpty()) 1828 return true; 1829 return false; 1830 } 1831 1832 /** 1833 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1834 * protocol, guideline, questionnaire or other definition that is 1835 * adhered to in whole or in part by this CarePlan activity.) 1836 */ 1837 public CanonicalType addInstantiatesCanonicalElement() {// 2 1838 CanonicalType t = new CanonicalType(); 1839 if (this.instantiatesCanonical == null) 1840 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1841 this.instantiatesCanonical.add(t); 1842 return t; 1843 } 1844 1845 /** 1846 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1847 * FHIR-defined protocol, guideline, questionnaire or other 1848 * definition that is adhered to in whole or in part by this 1849 * CarePlan activity.) 1850 */ 1851 public CarePlanActivityDetailComponent addInstantiatesCanonical(String value) { // 1 1852 CanonicalType t = new CanonicalType(); 1853 t.setValue(value); 1854 if (this.instantiatesCanonical == null) 1855 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1856 this.instantiatesCanonical.add(t); 1857 return this; 1858 } 1859 1860 /** 1861 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1862 * FHIR-defined protocol, guideline, questionnaire or other 1863 * definition that is adhered to in whole or in part by this 1864 * CarePlan activity.) 1865 */ 1866 public boolean hasInstantiatesCanonical(String value) { 1867 if (this.instantiatesCanonical == null) 1868 return false; 1869 for (CanonicalType v : this.instantiatesCanonical) 1870 if (v.getValue().equals(value)) // canonical(PlanDefinition|ActivityDefinition|Questionnaire|Measure|OperationDefinition) 1871 return true; 1872 return false; 1873 } 1874 1875 /** 1876 * @return {@link #instantiatesUri} (The URL pointing to an externally 1877 * maintained protocol, guideline, questionnaire or other definition 1878 * that is adhered to in whole or in part by this CarePlan activity.) 1879 */ 1880 public List<UriType> getInstantiatesUri() { 1881 if (this.instantiatesUri == null) 1882 this.instantiatesUri = new ArrayList<UriType>(); 1883 return this.instantiatesUri; 1884 } 1885 1886 /** 1887 * @return Returns a reference to <code>this</code> for easy method chaining 1888 */ 1889 public CarePlanActivityDetailComponent setInstantiatesUri(List<UriType> theInstantiatesUri) { 1890 this.instantiatesUri = theInstantiatesUri; 1891 return this; 1892 } 1893 1894 public boolean hasInstantiatesUri() { 1895 if (this.instantiatesUri == null) 1896 return false; 1897 for (UriType item : this.instantiatesUri) 1898 if (!item.isEmpty()) 1899 return true; 1900 return false; 1901 } 1902 1903 /** 1904 * @return {@link #instantiatesUri} (The URL pointing to an externally 1905 * maintained protocol, guideline, questionnaire or other definition 1906 * that is adhered to in whole or in part by this CarePlan activity.) 1907 */ 1908 public UriType addInstantiatesUriElement() {// 2 1909 UriType t = new UriType(); 1910 if (this.instantiatesUri == null) 1911 this.instantiatesUri = new ArrayList<UriType>(); 1912 this.instantiatesUri.add(t); 1913 return t; 1914 } 1915 1916 /** 1917 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1918 * maintained protocol, guideline, questionnaire or other 1919 * definition that is adhered to in whole or in part by this 1920 * CarePlan activity.) 1921 */ 1922 public CarePlanActivityDetailComponent addInstantiatesUri(String value) { // 1 1923 UriType t = new UriType(); 1924 t.setValue(value); 1925 if (this.instantiatesUri == null) 1926 this.instantiatesUri = new ArrayList<UriType>(); 1927 this.instantiatesUri.add(t); 1928 return this; 1929 } 1930 1931 /** 1932 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1933 * maintained protocol, guideline, questionnaire or other 1934 * definition that is adhered to in whole or in part by this 1935 * CarePlan activity.) 1936 */ 1937 public boolean hasInstantiatesUri(String value) { 1938 if (this.instantiatesUri == null) 1939 return false; 1940 for (UriType v : this.instantiatesUri) 1941 if (v.getValue().equals(value)) // uri 1942 return true; 1943 return false; 1944 } 1945 1946 /** 1947 * @return {@link #code} (Detailed description of the type of planned activity; 1948 * e.g. what lab test, what procedure, what kind of encounter.) 1949 */ 1950 public CodeableConcept getCode() { 1951 if (this.code == null) 1952 if (Configuration.errorOnAutoCreate()) 1953 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.code"); 1954 else if (Configuration.doAutoCreate()) 1955 this.code = new CodeableConcept(); // cc 1956 return this.code; 1957 } 1958 1959 public boolean hasCode() { 1960 return this.code != null && !this.code.isEmpty(); 1961 } 1962 1963 /** 1964 * @param value {@link #code} (Detailed description of the type of planned 1965 * activity; e.g. what lab test, what procedure, what kind of 1966 * encounter.) 1967 */ 1968 public CarePlanActivityDetailComponent setCode(CodeableConcept value) { 1969 this.code = value; 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #reasonCode} (Provides the rationale that drove the inclusion 1975 * of this particular activity as part of the plan or the reason why the 1976 * activity was prohibited.) 1977 */ 1978 public List<CodeableConcept> getReasonCode() { 1979 if (this.reasonCode == null) 1980 this.reasonCode = new ArrayList<CodeableConcept>(); 1981 return this.reasonCode; 1982 } 1983 1984 /** 1985 * @return Returns a reference to <code>this</code> for easy method chaining 1986 */ 1987 public CarePlanActivityDetailComponent setReasonCode(List<CodeableConcept> theReasonCode) { 1988 this.reasonCode = theReasonCode; 1989 return this; 1990 } 1991 1992 public boolean hasReasonCode() { 1993 if (this.reasonCode == null) 1994 return false; 1995 for (CodeableConcept item : this.reasonCode) 1996 if (!item.isEmpty()) 1997 return true; 1998 return false; 1999 } 2000 2001 public CodeableConcept addReasonCode() { // 3 2002 CodeableConcept t = new CodeableConcept(); 2003 if (this.reasonCode == null) 2004 this.reasonCode = new ArrayList<CodeableConcept>(); 2005 this.reasonCode.add(t); 2006 return t; 2007 } 2008 2009 public CarePlanActivityDetailComponent addReasonCode(CodeableConcept t) { // 3 2010 if (t == null) 2011 return this; 2012 if (this.reasonCode == null) 2013 this.reasonCode = new ArrayList<CodeableConcept>(); 2014 this.reasonCode.add(t); 2015 return this; 2016 } 2017 2018 /** 2019 * @return The first repetition of repeating field {@link #reasonCode}, creating 2020 * it if it does not already exist 2021 */ 2022 public CodeableConcept getReasonCodeFirstRep() { 2023 if (getReasonCode().isEmpty()) { 2024 addReasonCode(); 2025 } 2026 return getReasonCode().get(0); 2027 } 2028 2029 /** 2030 * @return {@link #reasonReference} (Indicates another resource, such as the 2031 * health condition(s), whose existence justifies this request and drove 2032 * the inclusion of this particular activity as part of the plan.) 2033 */ 2034 public List<Reference> getReasonReference() { 2035 if (this.reasonReference == null) 2036 this.reasonReference = new ArrayList<Reference>(); 2037 return this.reasonReference; 2038 } 2039 2040 /** 2041 * @return Returns a reference to <code>this</code> for easy method chaining 2042 */ 2043 public CarePlanActivityDetailComponent setReasonReference(List<Reference> theReasonReference) { 2044 this.reasonReference = theReasonReference; 2045 return this; 2046 } 2047 2048 public boolean hasReasonReference() { 2049 if (this.reasonReference == null) 2050 return false; 2051 for (Reference item : this.reasonReference) 2052 if (!item.isEmpty()) 2053 return true; 2054 return false; 2055 } 2056 2057 public Reference addReasonReference() { // 3 2058 Reference t = new Reference(); 2059 if (this.reasonReference == null) 2060 this.reasonReference = new ArrayList<Reference>(); 2061 this.reasonReference.add(t); 2062 return t; 2063 } 2064 2065 public CarePlanActivityDetailComponent addReasonReference(Reference t) { // 3 2066 if (t == null) 2067 return this; 2068 if (this.reasonReference == null) 2069 this.reasonReference = new ArrayList<Reference>(); 2070 this.reasonReference.add(t); 2071 return this; 2072 } 2073 2074 /** 2075 * @return The first repetition of repeating field {@link #reasonReference}, 2076 * creating it if it does not already exist 2077 */ 2078 public Reference getReasonReferenceFirstRep() { 2079 if (getReasonReference().isEmpty()) { 2080 addReasonReference(); 2081 } 2082 return getReasonReference().get(0); 2083 } 2084 2085 /** 2086 * @deprecated Use Reference#setResource(IBaseResource) instead 2087 */ 2088 @Deprecated 2089 public List<Resource> getReasonReferenceTarget() { 2090 if (this.reasonReferenceTarget == null) 2091 this.reasonReferenceTarget = new ArrayList<Resource>(); 2092 return this.reasonReferenceTarget; 2093 } 2094 2095 /** 2096 * @return {@link #goal} (Internal reference that identifies the goals that this 2097 * activity is intended to contribute towards meeting.) 2098 */ 2099 public List<Reference> getGoal() { 2100 if (this.goal == null) 2101 this.goal = new ArrayList<Reference>(); 2102 return this.goal; 2103 } 2104 2105 /** 2106 * @return Returns a reference to <code>this</code> for easy method chaining 2107 */ 2108 public CarePlanActivityDetailComponent setGoal(List<Reference> theGoal) { 2109 this.goal = theGoal; 2110 return this; 2111 } 2112 2113 public boolean hasGoal() { 2114 if (this.goal == null) 2115 return false; 2116 for (Reference item : this.goal) 2117 if (!item.isEmpty()) 2118 return true; 2119 return false; 2120 } 2121 2122 public Reference addGoal() { // 3 2123 Reference t = new Reference(); 2124 if (this.goal == null) 2125 this.goal = new ArrayList<Reference>(); 2126 this.goal.add(t); 2127 return t; 2128 } 2129 2130 public CarePlanActivityDetailComponent addGoal(Reference t) { // 3 2131 if (t == null) 2132 return this; 2133 if (this.goal == null) 2134 this.goal = new ArrayList<Reference>(); 2135 this.goal.add(t); 2136 return this; 2137 } 2138 2139 /** 2140 * @return The first repetition of repeating field {@link #goal}, creating it if 2141 * it does not already exist 2142 */ 2143 public Reference getGoalFirstRep() { 2144 if (getGoal().isEmpty()) { 2145 addGoal(); 2146 } 2147 return getGoal().get(0); 2148 } 2149 2150 /** 2151 * @deprecated Use Reference#setResource(IBaseResource) instead 2152 */ 2153 @Deprecated 2154 public List<Goal> getGoalTarget() { 2155 if (this.goalTarget == null) 2156 this.goalTarget = new ArrayList<Goal>(); 2157 return this.goalTarget; 2158 } 2159 2160 /** 2161 * @deprecated Use Reference#setResource(IBaseResource) instead 2162 */ 2163 @Deprecated 2164 public Goal addGoalTarget() { 2165 Goal r = new Goal(); 2166 if (this.goalTarget == null) 2167 this.goalTarget = new ArrayList<Goal>(); 2168 this.goalTarget.add(r); 2169 return r; 2170 } 2171 2172 /** 2173 * @return {@link #status} (Identifies what progress is being made for the 2174 * specific activity.). This is the underlying object with id, value and 2175 * extensions. The accessor "getStatus" gives direct access to the value 2176 */ 2177 public Enumeration<CarePlanActivityStatus> getStatusElement() { 2178 if (this.status == null) 2179 if (Configuration.errorOnAutoCreate()) 2180 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.status"); 2181 else if (Configuration.doAutoCreate()) 2182 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); // bb 2183 return this.status; 2184 } 2185 2186 public boolean hasStatusElement() { 2187 return this.status != null && !this.status.isEmpty(); 2188 } 2189 2190 public boolean hasStatus() { 2191 return this.status != null && !this.status.isEmpty(); 2192 } 2193 2194 /** 2195 * @param value {@link #status} (Identifies what progress is being made for the 2196 * specific activity.). This is the underlying object with id, 2197 * value and extensions. The accessor "getStatus" gives direct 2198 * access to the value 2199 */ 2200 public CarePlanActivityDetailComponent setStatusElement(Enumeration<CarePlanActivityStatus> value) { 2201 this.status = value; 2202 return this; 2203 } 2204 2205 /** 2206 * @return Identifies what progress is being made for the specific activity. 2207 */ 2208 public CarePlanActivityStatus getStatus() { 2209 return this.status == null ? null : this.status.getValue(); 2210 } 2211 2212 /** 2213 * @param value Identifies what progress is being made for the specific 2214 * activity. 2215 */ 2216 public CarePlanActivityDetailComponent setStatus(CarePlanActivityStatus value) { 2217 if (this.status == null) 2218 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); 2219 this.status.setValue(value); 2220 return this; 2221 } 2222 2223 /** 2224 * @return {@link #statusReason} (Provides reason why the activity isn't yet 2225 * started, is on hold, was cancelled, etc.) 2226 */ 2227 public CodeableConcept getStatusReason() { 2228 if (this.statusReason == null) 2229 if (Configuration.errorOnAutoCreate()) 2230 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.statusReason"); 2231 else if (Configuration.doAutoCreate()) 2232 this.statusReason = new CodeableConcept(); // cc 2233 return this.statusReason; 2234 } 2235 2236 public boolean hasStatusReason() { 2237 return this.statusReason != null && !this.statusReason.isEmpty(); 2238 } 2239 2240 /** 2241 * @param value {@link #statusReason} (Provides reason why the activity isn't 2242 * yet started, is on hold, was cancelled, etc.) 2243 */ 2244 public CarePlanActivityDetailComponent setStatusReason(CodeableConcept value) { 2245 this.statusReason = value; 2246 return this; 2247 } 2248 2249 /** 2250 * @return {@link #doNotPerform} (If true, indicates that the described activity 2251 * is one that must NOT be engaged in when following the plan. If false, 2252 * or missing, indicates that the described activity is one that should 2253 * be engaged in when following the plan.). This is the underlying 2254 * object with id, value and extensions. The accessor "getDoNotPerform" 2255 * gives direct access to the value 2256 */ 2257 public BooleanType getDoNotPerformElement() { 2258 if (this.doNotPerform == null) 2259 if (Configuration.errorOnAutoCreate()) 2260 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.doNotPerform"); 2261 else if (Configuration.doAutoCreate()) 2262 this.doNotPerform = new BooleanType(); // bb 2263 return this.doNotPerform; 2264 } 2265 2266 public boolean hasDoNotPerformElement() { 2267 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2268 } 2269 2270 public boolean hasDoNotPerform() { 2271 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2272 } 2273 2274 /** 2275 * @param value {@link #doNotPerform} (If true, indicates that the described 2276 * activity is one that must NOT be engaged in when following the 2277 * plan. If false, or missing, indicates that the described 2278 * activity is one that should be engaged in when following the 2279 * plan.). This is the underlying object with id, value and 2280 * extensions. The accessor "getDoNotPerform" gives direct access 2281 * to the value 2282 */ 2283 public CarePlanActivityDetailComponent setDoNotPerformElement(BooleanType value) { 2284 this.doNotPerform = value; 2285 return this; 2286 } 2287 2288 /** 2289 * @return If true, indicates that the described activity is one that must NOT 2290 * be engaged in when following the plan. If false, or missing, 2291 * indicates that the described activity is one that should be engaged 2292 * in when following the plan. 2293 */ 2294 public boolean getDoNotPerform() { 2295 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 2296 } 2297 2298 /** 2299 * @param value If true, indicates that the described activity is one that must 2300 * NOT be engaged in when following the plan. If false, or missing, 2301 * indicates that the described activity is one that should be 2302 * engaged in when following the plan. 2303 */ 2304 public CarePlanActivityDetailComponent setDoNotPerform(boolean value) { 2305 if (this.doNotPerform == null) 2306 this.doNotPerform = new BooleanType(); 2307 this.doNotPerform.setValue(value); 2308 return this; 2309 } 2310 2311 /** 2312 * @return {@link #scheduled} (The period, timing or frequency upon which the 2313 * described activity is to occur.) 2314 */ 2315 public Type getScheduled() { 2316 return this.scheduled; 2317 } 2318 2319 /** 2320 * @return {@link #scheduled} (The period, timing or frequency upon which the 2321 * described activity is to occur.) 2322 */ 2323 public Timing getScheduledTiming() throws FHIRException { 2324 if (this.scheduled == null) 2325 this.scheduled = new Timing(); 2326 if (!(this.scheduled instanceof Timing)) 2327 throw new FHIRException("Type mismatch: the type Timing was expected, but " 2328 + this.scheduled.getClass().getName() + " was encountered"); 2329 return (Timing) this.scheduled; 2330 } 2331 2332 public boolean hasScheduledTiming() { 2333 return this != null && this.scheduled instanceof Timing; 2334 } 2335 2336 /** 2337 * @return {@link #scheduled} (The period, timing or frequency upon which the 2338 * described activity is to occur.) 2339 */ 2340 public Period getScheduledPeriod() throws FHIRException { 2341 if (this.scheduled == null) 2342 this.scheduled = new Period(); 2343 if (!(this.scheduled instanceof Period)) 2344 throw new FHIRException("Type mismatch: the type Period was expected, but " 2345 + this.scheduled.getClass().getName() + " was encountered"); 2346 return (Period) this.scheduled; 2347 } 2348 2349 public boolean hasScheduledPeriod() { 2350 return this != null && this.scheduled instanceof Period; 2351 } 2352 2353 /** 2354 * @return {@link #scheduled} (The period, timing or frequency upon which the 2355 * described activity is to occur.) 2356 */ 2357 public StringType getScheduledStringType() throws FHIRException { 2358 if (this.scheduled == null) 2359 this.scheduled = new StringType(); 2360 if (!(this.scheduled instanceof StringType)) 2361 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2362 + this.scheduled.getClass().getName() + " was encountered"); 2363 return (StringType) this.scheduled; 2364 } 2365 2366 public boolean hasScheduledStringType() { 2367 return this != null && this.scheduled instanceof StringType; 2368 } 2369 2370 public boolean hasScheduled() { 2371 return this.scheduled != null && !this.scheduled.isEmpty(); 2372 } 2373 2374 /** 2375 * @param value {@link #scheduled} (The period, timing or frequency upon which 2376 * the described activity is to occur.) 2377 */ 2378 public CarePlanActivityDetailComponent setScheduled(Type value) { 2379 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof StringType)) 2380 throw new Error("Not the right type for CarePlan.activity.detail.scheduled[x]: " + value.fhirType()); 2381 this.scheduled = value; 2382 return this; 2383 } 2384 2385 /** 2386 * @return {@link #location} (Identifies the facility where the activity will 2387 * occur; e.g. home, hospital, specific clinic, etc.) 2388 */ 2389 public Reference getLocation() { 2390 if (this.location == null) 2391 if (Configuration.errorOnAutoCreate()) 2392 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 2393 else if (Configuration.doAutoCreate()) 2394 this.location = new Reference(); // cc 2395 return this.location; 2396 } 2397 2398 public boolean hasLocation() { 2399 return this.location != null && !this.location.isEmpty(); 2400 } 2401 2402 /** 2403 * @param value {@link #location} (Identifies the facility where the activity 2404 * will occur; e.g. home, hospital, specific clinic, etc.) 2405 */ 2406 public CarePlanActivityDetailComponent setLocation(Reference value) { 2407 this.location = value; 2408 return this; 2409 } 2410 2411 /** 2412 * @return {@link #location} The actual object that is the target of the 2413 * reference. The reference library doesn't populate this, but you can 2414 * use it to hold the resource if you resolve it. (Identifies the 2415 * facility where the activity will occur; e.g. home, hospital, specific 2416 * clinic, etc.) 2417 */ 2418 public Location getLocationTarget() { 2419 if (this.locationTarget == null) 2420 if (Configuration.errorOnAutoCreate()) 2421 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 2422 else if (Configuration.doAutoCreate()) 2423 this.locationTarget = new Location(); // aa 2424 return this.locationTarget; 2425 } 2426 2427 /** 2428 * @param value {@link #location} The actual object that is the target of the 2429 * reference. The reference library doesn't use these, but you can 2430 * use it to hold the resource if you resolve it. (Identifies the 2431 * facility where the activity will occur; e.g. home, hospital, 2432 * specific clinic, etc.) 2433 */ 2434 public CarePlanActivityDetailComponent setLocationTarget(Location value) { 2435 this.locationTarget = value; 2436 return this; 2437 } 2438 2439 /** 2440 * @return {@link #performer} (Identifies who's expected to be involved in the 2441 * activity.) 2442 */ 2443 public List<Reference> getPerformer() { 2444 if (this.performer == null) 2445 this.performer = new ArrayList<Reference>(); 2446 return this.performer; 2447 } 2448 2449 /** 2450 * @return Returns a reference to <code>this</code> for easy method chaining 2451 */ 2452 public CarePlanActivityDetailComponent setPerformer(List<Reference> thePerformer) { 2453 this.performer = thePerformer; 2454 return this; 2455 } 2456 2457 public boolean hasPerformer() { 2458 if (this.performer == null) 2459 return false; 2460 for (Reference item : this.performer) 2461 if (!item.isEmpty()) 2462 return true; 2463 return false; 2464 } 2465 2466 public Reference addPerformer() { // 3 2467 Reference t = new Reference(); 2468 if (this.performer == null) 2469 this.performer = new ArrayList<Reference>(); 2470 this.performer.add(t); 2471 return t; 2472 } 2473 2474 public CarePlanActivityDetailComponent addPerformer(Reference t) { // 3 2475 if (t == null) 2476 return this; 2477 if (this.performer == null) 2478 this.performer = new ArrayList<Reference>(); 2479 this.performer.add(t); 2480 return this; 2481 } 2482 2483 /** 2484 * @return The first repetition of repeating field {@link #performer}, creating 2485 * it if it does not already exist 2486 */ 2487 public Reference getPerformerFirstRep() { 2488 if (getPerformer().isEmpty()) { 2489 addPerformer(); 2490 } 2491 return getPerformer().get(0); 2492 } 2493 2494 /** 2495 * @deprecated Use Reference#setResource(IBaseResource) instead 2496 */ 2497 @Deprecated 2498 public List<Resource> getPerformerTarget() { 2499 if (this.performerTarget == null) 2500 this.performerTarget = new ArrayList<Resource>(); 2501 return this.performerTarget; 2502 } 2503 2504 /** 2505 * @return {@link #product} (Identifies the food, drug or other product to be 2506 * consumed or supplied in the activity.) 2507 */ 2508 public Type getProduct() { 2509 return this.product; 2510 } 2511 2512 /** 2513 * @return {@link #product} (Identifies the food, drug or other product to be 2514 * consumed or supplied in the activity.) 2515 */ 2516 public CodeableConcept getProductCodeableConcept() throws FHIRException { 2517 if (this.product == null) 2518 this.product = new CodeableConcept(); 2519 if (!(this.product instanceof CodeableConcept)) 2520 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2521 + this.product.getClass().getName() + " was encountered"); 2522 return (CodeableConcept) this.product; 2523 } 2524 2525 public boolean hasProductCodeableConcept() { 2526 return this != null && this.product instanceof CodeableConcept; 2527 } 2528 2529 /** 2530 * @return {@link #product} (Identifies the food, drug or other product to be 2531 * consumed or supplied in the activity.) 2532 */ 2533 public Reference getProductReference() throws FHIRException { 2534 if (this.product == null) 2535 this.product = new Reference(); 2536 if (!(this.product instanceof Reference)) 2537 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2538 + this.product.getClass().getName() + " was encountered"); 2539 return (Reference) this.product; 2540 } 2541 2542 public boolean hasProductReference() { 2543 return this != null && this.product instanceof Reference; 2544 } 2545 2546 public boolean hasProduct() { 2547 return this.product != null && !this.product.isEmpty(); 2548 } 2549 2550 /** 2551 * @param value {@link #product} (Identifies the food, drug or other product to 2552 * be consumed or supplied in the activity.) 2553 */ 2554 public CarePlanActivityDetailComponent setProduct(Type value) { 2555 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2556 throw new Error("Not the right type for CarePlan.activity.detail.product[x]: " + value.fhirType()); 2557 this.product = value; 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #dailyAmount} (Identifies the quantity expected to be consumed 2563 * in a given day.) 2564 */ 2565 public Quantity getDailyAmount() { 2566 if (this.dailyAmount == null) 2567 if (Configuration.errorOnAutoCreate()) 2568 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.dailyAmount"); 2569 else if (Configuration.doAutoCreate()) 2570 this.dailyAmount = new Quantity(); // cc 2571 return this.dailyAmount; 2572 } 2573 2574 public boolean hasDailyAmount() { 2575 return this.dailyAmount != null && !this.dailyAmount.isEmpty(); 2576 } 2577 2578 /** 2579 * @param value {@link #dailyAmount} (Identifies the quantity expected to be 2580 * consumed in a given day.) 2581 */ 2582 public CarePlanActivityDetailComponent setDailyAmount(Quantity value) { 2583 this.dailyAmount = value; 2584 return this; 2585 } 2586 2587 /** 2588 * @return {@link #quantity} (Identifies the quantity expected to be supplied, 2589 * administered or consumed by the subject.) 2590 */ 2591 public Quantity getQuantity() { 2592 if (this.quantity == null) 2593 if (Configuration.errorOnAutoCreate()) 2594 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.quantity"); 2595 else if (Configuration.doAutoCreate()) 2596 this.quantity = new Quantity(); // cc 2597 return this.quantity; 2598 } 2599 2600 public boolean hasQuantity() { 2601 return this.quantity != null && !this.quantity.isEmpty(); 2602 } 2603 2604 /** 2605 * @param value {@link #quantity} (Identifies the quantity expected to be 2606 * supplied, administered or consumed by the subject.) 2607 */ 2608 public CarePlanActivityDetailComponent setQuantity(Quantity value) { 2609 this.quantity = value; 2610 return this; 2611 } 2612 2613 /** 2614 * @return {@link #description} (This provides a textual description of 2615 * constraints on the intended activity occurrence, including relation 2616 * to other activities. It may also include objectives, pre-conditions 2617 * and end-conditions. Finally, it may convey specifics about the 2618 * activity such as body site, method, route, etc.). This is the 2619 * underlying object with id, value and extensions. The accessor 2620 * "getDescription" gives direct access to the value 2621 */ 2622 public StringType getDescriptionElement() { 2623 if (this.description == null) 2624 if (Configuration.errorOnAutoCreate()) 2625 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.description"); 2626 else if (Configuration.doAutoCreate()) 2627 this.description = new StringType(); // bb 2628 return this.description; 2629 } 2630 2631 public boolean hasDescriptionElement() { 2632 return this.description != null && !this.description.isEmpty(); 2633 } 2634 2635 public boolean hasDescription() { 2636 return this.description != null && !this.description.isEmpty(); 2637 } 2638 2639 /** 2640 * @param value {@link #description} (This provides a textual description of 2641 * constraints on the intended activity occurrence, including 2642 * relation to other activities. It may also include objectives, 2643 * pre-conditions and end-conditions. Finally, it may convey 2644 * specifics about the activity such as body site, method, route, 2645 * etc.). This is the underlying object with id, value and 2646 * extensions. The accessor "getDescription" gives direct access to 2647 * the value 2648 */ 2649 public CarePlanActivityDetailComponent setDescriptionElement(StringType value) { 2650 this.description = value; 2651 return this; 2652 } 2653 2654 /** 2655 * @return This provides a textual description of constraints on the intended 2656 * activity occurrence, including relation to other activities. It may 2657 * also include objectives, pre-conditions and end-conditions. Finally, 2658 * it may convey specifics about the activity such as body site, method, 2659 * route, etc. 2660 */ 2661 public String getDescription() { 2662 return this.description == null ? null : this.description.getValue(); 2663 } 2664 2665 /** 2666 * @param value This provides a textual description of constraints on the 2667 * intended activity occurrence, including relation to other 2668 * activities. It may also include objectives, pre-conditions and 2669 * end-conditions. Finally, it may convey specifics about the 2670 * activity such as body site, method, route, etc. 2671 */ 2672 public CarePlanActivityDetailComponent setDescription(String value) { 2673 if (Utilities.noString(value)) 2674 this.description = null; 2675 else { 2676 if (this.description == null) 2677 this.description = new StringType(); 2678 this.description.setValue(value); 2679 } 2680 return this; 2681 } 2682 2683 protected void listChildren(List<Property> children) { 2684 super.listChildren(children); 2685 children.add(new Property("kind", "code", 2686 "A description of the kind of resource the in-line definition of a care plan activity is representing. The CarePlan.activity.detail is an in-line definition when a resource is not referenced using CarePlan.activity.reference. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.", 2687 0, 1, kind)); 2688 children.add(new Property("instantiatesCanonical", 2689 "canonical(PlanDefinition|ActivityDefinition|Questionnaire|Measure|OperationDefinition)", 2690 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2691 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2692 children.add(new Property("instantiatesUri", "uri", 2693 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2694 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2695 children.add(new Property("code", "CodeableConcept", 2696 "Detailed description of the type of planned activity; e.g. what lab test, what procedure, what kind of encounter.", 2697 0, 1, code)); 2698 children.add(new Property("reasonCode", "CodeableConcept", 2699 "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.", 2700 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2701 children.add(new Property("reasonReference", 2702 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2703 "Indicates another resource, such as the health condition(s), whose existence justifies this request and drove the inclusion of this particular activity as part of the plan.", 2704 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2705 children.add(new Property("goal", "Reference(Goal)", 2706 "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 2707 0, java.lang.Integer.MAX_VALUE, goal)); 2708 children.add(new Property("status", "code", "Identifies what progress is being made for the specific activity.", 2709 0, 1, status)); 2710 children.add(new Property("statusReason", "CodeableConcept", 2711 "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 1, statusReason)); 2712 children.add(new Property("doNotPerform", "boolean", 2713 "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, or missing, indicates that the described activity is one that should be engaged in when following the plan.", 2714 0, 1, doNotPerform)); 2715 children.add(new Property("scheduled[x]", "Timing|Period|string", 2716 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled)); 2717 children.add(new Property("location", "Reference(Location)", 2718 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 2719 location)); 2720 children.add(new Property("performer", 2721 "Reference(Practitioner|PractitionerRole|Organization|RelatedPerson|Patient|CareTeam|HealthcareService|Device)", 2722 "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer)); 2723 children.add(new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2724 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product)); 2725 children.add(new Property("dailyAmount", "SimpleQuantity", 2726 "Identifies the quantity expected to be consumed in a given day.", 0, 1, dailyAmount)); 2727 children.add(new Property("quantity", "SimpleQuantity", 2728 "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 1, quantity)); 2729 children.add(new Property("description", "string", 2730 "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 2731 0, 1, description)); 2732 } 2733 2734 @Override 2735 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2736 switch (_hash) { 2737 case 3292052: 2738 /* kind */ return new Property("kind", "code", 2739 "A description of the kind of resource the in-line definition of a care plan activity is representing. The CarePlan.activity.detail is an in-line definition when a resource is not referenced using CarePlan.activity.reference. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.", 2740 0, 1, kind); 2741 case 8911915: 2742 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 2743 "canonical(PlanDefinition|ActivityDefinition|Questionnaire|Measure|OperationDefinition)", 2744 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2745 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2746 case -1926393373: 2747 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 2748 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2749 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2750 case 3059181: 2751 /* code */ return new Property("code", "CodeableConcept", 2752 "Detailed description of the type of planned activity; e.g. what lab test, what procedure, what kind of encounter.", 2753 0, 1, code); 2754 case 722137681: 2755 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2756 "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.", 2757 0, java.lang.Integer.MAX_VALUE, reasonCode); 2758 case -1146218137: 2759 /* reasonReference */ return new Property("reasonReference", 2760 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2761 "Indicates another resource, such as the health condition(s), whose existence justifies this request and drove the inclusion of this particular activity as part of the plan.", 2762 0, java.lang.Integer.MAX_VALUE, reasonReference); 2763 case 3178259: 2764 /* goal */ return new Property("goal", "Reference(Goal)", 2765 "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 2766 0, java.lang.Integer.MAX_VALUE, goal); 2767 case -892481550: 2768 /* status */ return new Property("status", "code", 2769 "Identifies what progress is being made for the specific activity.", 0, 1, status); 2770 case 2051346646: 2771 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2772 "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 1, statusReason); 2773 case -1788508167: 2774 /* doNotPerform */ return new Property("doNotPerform", "boolean", 2775 "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, or missing, indicates that the described activity is one that should be engaged in when following the plan.", 2776 0, 1, doNotPerform); 2777 case 1162627251: 2778 /* scheduled[x] */ return new Property("scheduled[x]", "Timing|Period|string", 2779 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2780 case -160710483: 2781 /* scheduled */ return new Property("scheduled[x]", "Timing|Period|string", 2782 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2783 case 998483799: 2784 /* scheduledTiming */ return new Property("scheduled[x]", "Timing|Period|string", 2785 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2786 case 880422094: 2787 /* scheduledPeriod */ return new Property("scheduled[x]", "Timing|Period|string", 2788 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2789 case 980162334: 2790 /* scheduledString */ return new Property("scheduled[x]", "Timing|Period|string", 2791 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2792 case 1901043637: 2793 /* location */ return new Property("location", "Reference(Location)", 2794 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 2795 location); 2796 case 481140686: 2797 /* performer */ return new Property("performer", 2798 "Reference(Practitioner|PractitionerRole|Organization|RelatedPerson|Patient|CareTeam|HealthcareService|Device)", 2799 "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer); 2800 case 1753005361: 2801 /* product[x] */ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2802 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2803 case -309474065: 2804 /* product */ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2805 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2806 case 906854066: 2807 /* productCodeableConcept */ return new Property("product[x]", 2808 "CodeableConcept|Reference(Medication|Substance)", 2809 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2810 case -669667556: 2811 /* productReference */ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2812 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2813 case -768908335: 2814 /* dailyAmount */ return new Property("dailyAmount", "SimpleQuantity", 2815 "Identifies the quantity expected to be consumed in a given day.", 0, 1, dailyAmount); 2816 case -1285004149: 2817 /* quantity */ return new Property("quantity", "SimpleQuantity", 2818 "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 1, 2819 quantity); 2820 case -1724546052: 2821 /* description */ return new Property("description", "string", 2822 "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 2823 0, 1, description); 2824 default: 2825 return super.getNamedProperty(_hash, _name, _checkValid); 2826 } 2827 2828 } 2829 2830 @Override 2831 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2832 switch (hash) { 2833 case 3292052: 2834 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<CarePlanActivityKind> 2835 case 8911915: 2836 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 2837 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2838 case -1926393373: 2839 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 2840 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2841 case 3059181: 2842 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2843 case 722137681: 2844 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2845 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2846 case -1146218137: 2847 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2848 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2849 case 3178259: 2850 /* goal */ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 2851 case -892481550: 2852 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CarePlanActivityStatus> 2853 case 2051346646: 2854 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2855 case -1788508167: 2856 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 2857 case -160710483: 2858 /* scheduled */ return this.scheduled == null ? new Base[0] : new Base[] { this.scheduled }; // Type 2859 case 1901043637: 2860 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 2861 case 481140686: 2862 /* performer */ return this.performer == null ? new Base[0] 2863 : this.performer.toArray(new Base[this.performer.size()]); // Reference 2864 case -309474065: 2865 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // Type 2866 case -768908335: 2867 /* dailyAmount */ return this.dailyAmount == null ? new Base[0] : new Base[] { this.dailyAmount }; // Quantity 2868 case -1285004149: 2869 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 2870 case -1724546052: 2871 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2872 default: 2873 return super.getProperty(hash, name, checkValid); 2874 } 2875 2876 } 2877 2878 @Override 2879 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2880 switch (hash) { 2881 case 3292052: // kind 2882 value = new CarePlanActivityKindEnumFactory().fromType(castToCode(value)); 2883 this.kind = (Enumeration) value; // Enumeration<CarePlanActivityKind> 2884 return value; 2885 case 8911915: // instantiatesCanonical 2886 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 2887 return value; 2888 case -1926393373: // instantiatesUri 2889 this.getInstantiatesUri().add(castToUri(value)); // UriType 2890 return value; 2891 case 3059181: // code 2892 this.code = castToCodeableConcept(value); // CodeableConcept 2893 return value; 2894 case 722137681: // reasonCode 2895 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2896 return value; 2897 case -1146218137: // reasonReference 2898 this.getReasonReference().add(castToReference(value)); // Reference 2899 return value; 2900 case 3178259: // goal 2901 this.getGoal().add(castToReference(value)); // Reference 2902 return value; 2903 case -892481550: // status 2904 value = new CarePlanActivityStatusEnumFactory().fromType(castToCode(value)); 2905 this.status = (Enumeration) value; // Enumeration<CarePlanActivityStatus> 2906 return value; 2907 case 2051346646: // statusReason 2908 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2909 return value; 2910 case -1788508167: // doNotPerform 2911 this.doNotPerform = castToBoolean(value); // BooleanType 2912 return value; 2913 case -160710483: // scheduled 2914 this.scheduled = castToType(value); // Type 2915 return value; 2916 case 1901043637: // location 2917 this.location = castToReference(value); // Reference 2918 return value; 2919 case 481140686: // performer 2920 this.getPerformer().add(castToReference(value)); // Reference 2921 return value; 2922 case -309474065: // product 2923 this.product = castToType(value); // Type 2924 return value; 2925 case -768908335: // dailyAmount 2926 this.dailyAmount = castToQuantity(value); // Quantity 2927 return value; 2928 case -1285004149: // quantity 2929 this.quantity = castToQuantity(value); // Quantity 2930 return value; 2931 case -1724546052: // description 2932 this.description = castToString(value); // StringType 2933 return value; 2934 default: 2935 return super.setProperty(hash, name, value); 2936 } 2937 2938 } 2939 2940 @Override 2941 public Base setProperty(String name, Base value) throws FHIRException { 2942 if (name.equals("kind")) { 2943 value = new CarePlanActivityKindEnumFactory().fromType(castToCode(value)); 2944 this.kind = (Enumeration) value; // Enumeration<CarePlanActivityKind> 2945 } else if (name.equals("instantiatesCanonical")) { 2946 this.getInstantiatesCanonical().add(castToCanonical(value)); 2947 } else if (name.equals("instantiatesUri")) { 2948 this.getInstantiatesUri().add(castToUri(value)); 2949 } else if (name.equals("code")) { 2950 this.code = castToCodeableConcept(value); // CodeableConcept 2951 } else if (name.equals("reasonCode")) { 2952 this.getReasonCode().add(castToCodeableConcept(value)); 2953 } else if (name.equals("reasonReference")) { 2954 this.getReasonReference().add(castToReference(value)); 2955 } else if (name.equals("goal")) { 2956 this.getGoal().add(castToReference(value)); 2957 } else if (name.equals("status")) { 2958 value = new CarePlanActivityStatusEnumFactory().fromType(castToCode(value)); 2959 this.status = (Enumeration) value; // Enumeration<CarePlanActivityStatus> 2960 } else if (name.equals("statusReason")) { 2961 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2962 } else if (name.equals("doNotPerform")) { 2963 this.doNotPerform = castToBoolean(value); // BooleanType 2964 } else if (name.equals("scheduled[x]")) { 2965 this.scheduled = castToType(value); // Type 2966 } else if (name.equals("location")) { 2967 this.location = castToReference(value); // Reference 2968 } else if (name.equals("performer")) { 2969 this.getPerformer().add(castToReference(value)); 2970 } else if (name.equals("product[x]")) { 2971 this.product = castToType(value); // Type 2972 } else if (name.equals("dailyAmount")) { 2973 this.dailyAmount = castToQuantity(value); // Quantity 2974 } else if (name.equals("quantity")) { 2975 this.quantity = castToQuantity(value); // Quantity 2976 } else if (name.equals("description")) { 2977 this.description = castToString(value); // StringType 2978 } else 2979 return super.setProperty(name, value); 2980 return value; 2981 } 2982 2983 @Override 2984 public void removeChild(String name, Base value) throws FHIRException { 2985 if (name.equals("kind")) { 2986 this.kind = null; 2987 } else if (name.equals("instantiatesCanonical")) { 2988 this.getInstantiatesCanonical().remove(castToCanonical(value)); 2989 } else if (name.equals("instantiatesUri")) { 2990 this.getInstantiatesUri().remove(castToUri(value)); 2991 } else if (name.equals("code")) { 2992 this.code = null; 2993 } else if (name.equals("reasonCode")) { 2994 this.getReasonCode().remove(castToCodeableConcept(value)); 2995 } else if (name.equals("reasonReference")) { 2996 this.getReasonReference().remove(castToReference(value)); 2997 } else if (name.equals("goal")) { 2998 this.getGoal().remove(castToReference(value)); 2999 } else if (name.equals("status")) { 3000 this.status = null; 3001 } else if (name.equals("statusReason")) { 3002 this.statusReason = null; 3003 } else if (name.equals("doNotPerform")) { 3004 this.doNotPerform = null; 3005 } else if (name.equals("scheduled[x]")) { 3006 this.scheduled = null; 3007 } else if (name.equals("location")) { 3008 this.location = null; 3009 } else if (name.equals("performer")) { 3010 this.getPerformer().remove(castToReference(value)); 3011 } else if (name.equals("product[x]")) { 3012 this.product = null; 3013 } else if (name.equals("dailyAmount")) { 3014 this.dailyAmount = null; 3015 } else if (name.equals("quantity")) { 3016 this.quantity = null; 3017 } else if (name.equals("description")) { 3018 this.description = null; 3019 } else 3020 super.removeChild(name, value); 3021 3022 } 3023 3024 @Override 3025 public Base makeProperty(int hash, String name) throws FHIRException { 3026 switch (hash) { 3027 case 3292052: 3028 return getKindElement(); 3029 case 8911915: 3030 return addInstantiatesCanonicalElement(); 3031 case -1926393373: 3032 return addInstantiatesUriElement(); 3033 case 3059181: 3034 return getCode(); 3035 case 722137681: 3036 return addReasonCode(); 3037 case -1146218137: 3038 return addReasonReference(); 3039 case 3178259: 3040 return addGoal(); 3041 case -892481550: 3042 return getStatusElement(); 3043 case 2051346646: 3044 return getStatusReason(); 3045 case -1788508167: 3046 return getDoNotPerformElement(); 3047 case 1162627251: 3048 return getScheduled(); 3049 case -160710483: 3050 return getScheduled(); 3051 case 1901043637: 3052 return getLocation(); 3053 case 481140686: 3054 return addPerformer(); 3055 case 1753005361: 3056 return getProduct(); 3057 case -309474065: 3058 return getProduct(); 3059 case -768908335: 3060 return getDailyAmount(); 3061 case -1285004149: 3062 return getQuantity(); 3063 case -1724546052: 3064 return getDescriptionElement(); 3065 default: 3066 return super.makeProperty(hash, name); 3067 } 3068 3069 } 3070 3071 @Override 3072 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3073 switch (hash) { 3074 case 3292052: 3075 /* kind */ return new String[] { "code" }; 3076 case 8911915: 3077 /* instantiatesCanonical */ return new String[] { "canonical" }; 3078 case -1926393373: 3079 /* instantiatesUri */ return new String[] { "uri" }; 3080 case 3059181: 3081 /* code */ return new String[] { "CodeableConcept" }; 3082 case 722137681: 3083 /* reasonCode */ return new String[] { "CodeableConcept" }; 3084 case -1146218137: 3085 /* reasonReference */ return new String[] { "Reference" }; 3086 case 3178259: 3087 /* goal */ return new String[] { "Reference" }; 3088 case -892481550: 3089 /* status */ return new String[] { "code" }; 3090 case 2051346646: 3091 /* statusReason */ return new String[] { "CodeableConcept" }; 3092 case -1788508167: 3093 /* doNotPerform */ return new String[] { "boolean" }; 3094 case -160710483: 3095 /* scheduled */ return new String[] { "Timing", "Period", "string" }; 3096 case 1901043637: 3097 /* location */ return new String[] { "Reference" }; 3098 case 481140686: 3099 /* performer */ return new String[] { "Reference" }; 3100 case -309474065: 3101 /* product */ return new String[] { "CodeableConcept", "Reference" }; 3102 case -768908335: 3103 /* dailyAmount */ return new String[] { "SimpleQuantity" }; 3104 case -1285004149: 3105 /* quantity */ return new String[] { "SimpleQuantity" }; 3106 case -1724546052: 3107 /* description */ return new String[] { "string" }; 3108 default: 3109 return super.getTypesForProperty(hash, name); 3110 } 3111 3112 } 3113 3114 @Override 3115 public Base addChild(String name) throws FHIRException { 3116 if (name.equals("kind")) { 3117 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.kind"); 3118 } else if (name.equals("instantiatesCanonical")) { 3119 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesCanonical"); 3120 } else if (name.equals("instantiatesUri")) { 3121 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesUri"); 3122 } else if (name.equals("code")) { 3123 this.code = new CodeableConcept(); 3124 return this.code; 3125 } else if (name.equals("reasonCode")) { 3126 return addReasonCode(); 3127 } else if (name.equals("reasonReference")) { 3128 return addReasonReference(); 3129 } else if (name.equals("goal")) { 3130 return addGoal(); 3131 } else if (name.equals("status")) { 3132 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 3133 } else if (name.equals("statusReason")) { 3134 this.statusReason = new CodeableConcept(); 3135 return this.statusReason; 3136 } else if (name.equals("doNotPerform")) { 3137 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.doNotPerform"); 3138 } else if (name.equals("scheduledTiming")) { 3139 this.scheduled = new Timing(); 3140 return this.scheduled; 3141 } else if (name.equals("scheduledPeriod")) { 3142 this.scheduled = new Period(); 3143 return this.scheduled; 3144 } else if (name.equals("scheduledString")) { 3145 this.scheduled = new StringType(); 3146 return this.scheduled; 3147 } else if (name.equals("location")) { 3148 this.location = new Reference(); 3149 return this.location; 3150 } else if (name.equals("performer")) { 3151 return addPerformer(); 3152 } else if (name.equals("productCodeableConcept")) { 3153 this.product = new CodeableConcept(); 3154 return this.product; 3155 } else if (name.equals("productReference")) { 3156 this.product = new Reference(); 3157 return this.product; 3158 } else if (name.equals("dailyAmount")) { 3159 this.dailyAmount = new Quantity(); 3160 return this.dailyAmount; 3161 } else if (name.equals("quantity")) { 3162 this.quantity = new Quantity(); 3163 return this.quantity; 3164 } else if (name.equals("description")) { 3165 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 3166 } else 3167 return super.addChild(name); 3168 } 3169 3170 public CarePlanActivityDetailComponent copy() { 3171 CarePlanActivityDetailComponent dst = new CarePlanActivityDetailComponent(); 3172 copyValues(dst); 3173 return dst; 3174 } 3175 3176 public void copyValues(CarePlanActivityDetailComponent dst) { 3177 super.copyValues(dst); 3178 dst.kind = kind == null ? null : kind.copy(); 3179 if (instantiatesCanonical != null) { 3180 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 3181 for (CanonicalType i : instantiatesCanonical) 3182 dst.instantiatesCanonical.add(i.copy()); 3183 } 3184 ; 3185 if (instantiatesUri != null) { 3186 dst.instantiatesUri = new ArrayList<UriType>(); 3187 for (UriType i : instantiatesUri) 3188 dst.instantiatesUri.add(i.copy()); 3189 } 3190 ; 3191 dst.code = code == null ? null : code.copy(); 3192 if (reasonCode != null) { 3193 dst.reasonCode = new ArrayList<CodeableConcept>(); 3194 for (CodeableConcept i : reasonCode) 3195 dst.reasonCode.add(i.copy()); 3196 } 3197 ; 3198 if (reasonReference != null) { 3199 dst.reasonReference = new ArrayList<Reference>(); 3200 for (Reference i : reasonReference) 3201 dst.reasonReference.add(i.copy()); 3202 } 3203 ; 3204 if (goal != null) { 3205 dst.goal = new ArrayList<Reference>(); 3206 for (Reference i : goal) 3207 dst.goal.add(i.copy()); 3208 } 3209 ; 3210 dst.status = status == null ? null : status.copy(); 3211 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3212 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 3213 dst.scheduled = scheduled == null ? null : scheduled.copy(); 3214 dst.location = location == null ? null : location.copy(); 3215 if (performer != null) { 3216 dst.performer = new ArrayList<Reference>(); 3217 for (Reference i : performer) 3218 dst.performer.add(i.copy()); 3219 } 3220 ; 3221 dst.product = product == null ? null : product.copy(); 3222 dst.dailyAmount = dailyAmount == null ? null : dailyAmount.copy(); 3223 dst.quantity = quantity == null ? null : quantity.copy(); 3224 dst.description = description == null ? null : description.copy(); 3225 } 3226 3227 @Override 3228 public boolean equalsDeep(Base other_) { 3229 if (!super.equalsDeep(other_)) 3230 return false; 3231 if (!(other_ instanceof CarePlanActivityDetailComponent)) 3232 return false; 3233 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other_; 3234 return compareDeep(kind, o.kind, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3235 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(code, o.code, true) 3236 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3237 && compareDeep(goal, o.goal, true) && compareDeep(status, o.status, true) 3238 && compareDeep(statusReason, o.statusReason, true) && compareDeep(doNotPerform, o.doNotPerform, true) 3239 && compareDeep(scheduled, o.scheduled, true) && compareDeep(location, o.location, true) 3240 && compareDeep(performer, o.performer, true) && compareDeep(product, o.product, true) 3241 && compareDeep(dailyAmount, o.dailyAmount, true) && compareDeep(quantity, o.quantity, true) 3242 && compareDeep(description, o.description, true); 3243 } 3244 3245 @Override 3246 public boolean equalsShallow(Base other_) { 3247 if (!super.equalsShallow(other_)) 3248 return false; 3249 if (!(other_ instanceof CarePlanActivityDetailComponent)) 3250 return false; 3251 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other_; 3252 return compareValues(kind, o.kind, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 3253 && compareValues(status, o.status, true) && compareValues(doNotPerform, o.doNotPerform, true) 3254 && compareValues(description, o.description, true); 3255 } 3256 3257 public boolean isEmpty() { 3258 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, instantiatesCanonical, instantiatesUri, code, 3259 reasonCode, reasonReference, goal, status, statusReason, doNotPerform, scheduled, location, performer, 3260 product, dailyAmount, quantity, description); 3261 } 3262 3263 public String fhirType() { 3264 return "CarePlan.activity.detail"; 3265 3266 } 3267 3268 } 3269 3270 /** 3271 * Business identifiers assigned to this care plan by the performer or other 3272 * systems which remain constant as the resource is updated and propagates from 3273 * server to server. 3274 */ 3275 @Child(name = "identifier", type = { 3276 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3277 @Description(shortDefinition = "External Ids for this plan", formalDefinition = "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 3278 protected List<Identifier> identifier; 3279 3280 /** 3281 * The URL pointing to a FHIR-defined protocol, guideline, questionnaire or 3282 * other definition that is adhered to in whole or in part by this CarePlan. 3283 */ 3284 @Child(name = "instantiatesCanonical", type = { 3285 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3286 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.") 3287 protected List<CanonicalType> instantiatesCanonical; 3288 3289 /** 3290 * The URL pointing to an externally maintained protocol, guideline, 3291 * questionnaire or other definition that is adhered to in whole or in part by 3292 * this CarePlan. 3293 */ 3294 @Child(name = "instantiatesUri", type = { 3295 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3296 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.") 3297 protected List<UriType> instantiatesUri; 3298 3299 /** 3300 * A care plan that is fulfilled in whole or in part by this care plan. 3301 */ 3302 @Child(name = "basedOn", type = { 3303 CarePlan.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3304 @Description(shortDefinition = "Fulfills CarePlan", formalDefinition = "A care plan that is fulfilled in whole or in part by this care plan.") 3305 protected List<Reference> basedOn; 3306 /** 3307 * The actual objects that are the target of the reference (A care plan that is 3308 * fulfilled in whole or in part by this care plan.) 3309 */ 3310 protected List<CarePlan> basedOnTarget; 3311 3312 /** 3313 * Completed or terminated care plan whose function is taken by this new care 3314 * plan. 3315 */ 3316 @Child(name = "replaces", type = { 3317 CarePlan.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3318 @Description(shortDefinition = "CarePlan replaced by this CarePlan", formalDefinition = "Completed or terminated care plan whose function is taken by this new care plan.") 3319 protected List<Reference> replaces; 3320 /** 3321 * The actual objects that are the target of the reference (Completed or 3322 * terminated care plan whose function is taken by this new care plan.) 3323 */ 3324 protected List<CarePlan> replacesTarget; 3325 3326 /** 3327 * A larger care plan of which this particular care plan is a component or step. 3328 */ 3329 @Child(name = "partOf", type = { 3330 CarePlan.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3331 @Description(shortDefinition = "Part of referenced CarePlan", formalDefinition = "A larger care plan of which this particular care plan is a component or step.") 3332 protected List<Reference> partOf; 3333 /** 3334 * The actual objects that are the target of the reference (A larger care plan 3335 * of which this particular care plan is a component or step.) 3336 */ 3337 protected List<CarePlan> partOfTarget; 3338 3339 /** 3340 * Indicates whether the plan is currently being acted upon, represents future 3341 * intentions or is now a historical record. 3342 */ 3343 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 3344 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.") 3345 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 3346 protected Enumeration<CarePlanStatus> status; 3347 3348 /** 3349 * Indicates the level of authority/intentionality associated with the care plan 3350 * and where the care plan fits into the workflow chain. 3351 */ 3352 @Child(name = "intent", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = true, summary = true) 3353 @Description(shortDefinition = "proposal | plan | order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.") 3354 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-intent") 3355 protected Enumeration<CarePlanIntent> intent; 3356 3357 /** 3358 * Identifies what "kind" of plan this is to support differentiation between 3359 * multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", 3360 * "disease management", "wellness plan", etc. 3361 */ 3362 @Child(name = "category", type = { 3363 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3364 @Description(shortDefinition = "Type of plan", formalDefinition = "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.") 3365 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-category") 3366 protected List<CodeableConcept> category; 3367 3368 /** 3369 * Human-friendly name for the care plan. 3370 */ 3371 @Child(name = "title", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3372 @Description(shortDefinition = "Human-friendly name for the care plan", formalDefinition = "Human-friendly name for the care plan.") 3373 protected StringType title; 3374 3375 /** 3376 * A description of the scope and nature of the plan. 3377 */ 3378 @Child(name = "description", type = { 3379 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 3380 @Description(shortDefinition = "Summary of nature of plan", formalDefinition = "A description of the scope and nature of the plan.") 3381 protected StringType description; 3382 3383 /** 3384 * Identifies the patient or group whose intended care is described by the plan. 3385 */ 3386 @Child(name = "subject", type = { Patient.class, 3387 Group.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 3388 @Description(shortDefinition = "Who the care plan is for", formalDefinition = "Identifies the patient or group whose intended care is described by the plan.") 3389 protected Reference subject; 3390 3391 /** 3392 * The actual object that is the target of the reference (Identifies the patient 3393 * or group whose intended care is described by the plan.) 3394 */ 3395 protected Resource subjectTarget; 3396 3397 /** 3398 * The Encounter during which this CarePlan was created or to which the creation 3399 * of this record is tightly associated. 3400 */ 3401 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 3402 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.") 3403 protected Reference encounter; 3404 3405 /** 3406 * The actual object that is the target of the reference (The Encounter during 3407 * which this CarePlan was created or to which the creation of this record is 3408 * tightly associated.) 3409 */ 3410 protected Encounter encounterTarget; 3411 3412 /** 3413 * Indicates when the plan did (or is intended to) come into effect and end. 3414 */ 3415 @Child(name = "period", type = { Period.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 3416 @Description(shortDefinition = "Time period plan covers", formalDefinition = "Indicates when the plan did (or is intended to) come into effect and end.") 3417 protected Period period; 3418 3419 /** 3420 * Represents when this particular CarePlan record was created in the system, 3421 * which is often a system-generated date. 3422 */ 3423 @Child(name = "created", type = { 3424 DateTimeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 3425 @Description(shortDefinition = "Date record was first recorded", formalDefinition = "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.") 3426 protected DateTimeType created; 3427 3428 /** 3429 * When populated, the author is responsible for the care plan. The care plan is 3430 * attributed to the author. 3431 */ 3432 @Child(name = "author", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 3433 RelatedPerson.class, Organization.class, 3434 CareTeam.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 3435 @Description(shortDefinition = "Who is the designated responsible party", formalDefinition = "When populated, the author is responsible for the care plan. The care plan is attributed to the author.") 3436 protected Reference author; 3437 3438 /** 3439 * The actual object that is the target of the reference (When populated, the 3440 * author is responsible for the care plan. The care plan is attributed to the 3441 * author.) 3442 */ 3443 protected Resource authorTarget; 3444 3445 /** 3446 * Identifies the individual(s) or organization who provided the contents of the 3447 * care plan. 3448 */ 3449 @Child(name = "contributor", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 3450 RelatedPerson.class, Organization.class, 3451 CareTeam.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3452 @Description(shortDefinition = "Who provided the content of the care plan", formalDefinition = "Identifies the individual(s) or organization who provided the contents of the care plan.") 3453 protected List<Reference> contributor; 3454 /** 3455 * The actual objects that are the target of the reference (Identifies the 3456 * individual(s) or organization who provided the contents of the care plan.) 3457 */ 3458 protected List<Resource> contributorTarget; 3459 3460 /** 3461 * Identifies all people and organizations who are expected to be involved in 3462 * the care envisioned by this plan. 3463 */ 3464 @Child(name = "careTeam", type = { 3465 CareTeam.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3466 @Description(shortDefinition = "Who's involved in plan?", formalDefinition = "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.") 3467 protected List<Reference> careTeam; 3468 /** 3469 * The actual objects that are the target of the reference (Identifies all 3470 * people and organizations who are expected to be involved in the care 3471 * envisioned by this plan.) 3472 */ 3473 protected List<CareTeam> careTeamTarget; 3474 3475 /** 3476 * Identifies the conditions/problems/concerns/diagnoses/etc. whose management 3477 * and/or mitigation are handled by this plan. 3478 */ 3479 @Child(name = "addresses", type = { 3480 Condition.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3481 @Description(shortDefinition = "Health issues this plan addresses", formalDefinition = "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.") 3482 protected List<Reference> addresses; 3483 /** 3484 * The actual objects that are the target of the reference (Identifies the 3485 * conditions/problems/concerns/diagnoses/etc. whose management and/or 3486 * mitigation are handled by this plan.) 3487 */ 3488 protected List<Condition> addressesTarget; 3489 3490 /** 3491 * Identifies portions of the patient's record that specifically influenced the 3492 * formation of the plan. These might include comorbidities, recent procedures, 3493 * limitations, recent assessments, etc. 3494 */ 3495 @Child(name = "supportingInfo", type = { 3496 Reference.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3497 @Description(shortDefinition = "Information considered as part of plan", formalDefinition = "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.") 3498 protected List<Reference> supportingInfo; 3499 /** 3500 * The actual objects that are the target of the reference (Identifies portions 3501 * of the patient's record that specifically influenced the formation of the 3502 * plan. These might include comorbidities, recent procedures, limitations, 3503 * recent assessments, etc.) 3504 */ 3505 protected List<Resource> supportingInfoTarget; 3506 3507 /** 3508 * Describes the intended objective(s) of carrying out the care plan. 3509 */ 3510 @Child(name = "goal", type = { 3511 Goal.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3512 @Description(shortDefinition = "Desired outcome of plan", formalDefinition = "Describes the intended objective(s) of carrying out the care plan.") 3513 protected List<Reference> goal; 3514 /** 3515 * The actual objects that are the target of the reference (Describes the 3516 * intended objective(s) of carrying out the care plan.) 3517 */ 3518 protected List<Goal> goalTarget; 3519 3520 /** 3521 * Identifies a planned action to occur as part of the plan. For example, a 3522 * medication to be used, lab tests to perform, self-monitoring, education, etc. 3523 */ 3524 @Child(name = "activity", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3525 @Description(shortDefinition = "Action to occur as part of plan", formalDefinition = "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.") 3526 protected List<CarePlanActivityComponent> activity; 3527 3528 /** 3529 * General notes about the care plan not covered elsewhere. 3530 */ 3531 @Child(name = "note", type = { 3532 Annotation.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3533 @Description(shortDefinition = "Comments about the plan", formalDefinition = "General notes about the care plan not covered elsewhere.") 3534 protected List<Annotation> note; 3535 3536 private static final long serialVersionUID = -584930613L; 3537 3538 /** 3539 * Constructor 3540 */ 3541 public CarePlan() { 3542 super(); 3543 } 3544 3545 /** 3546 * Constructor 3547 */ 3548 public CarePlan(Enumeration<CarePlanStatus> status, Enumeration<CarePlanIntent> intent, Reference subject) { 3549 super(); 3550 this.status = status; 3551 this.intent = intent; 3552 this.subject = subject; 3553 } 3554 3555 /** 3556 * @return {@link #identifier} (Business identifiers assigned to this care plan 3557 * by the performer or other systems which remain constant as the 3558 * resource is updated and propagates from server to server.) 3559 */ 3560 public List<Identifier> getIdentifier() { 3561 if (this.identifier == null) 3562 this.identifier = new ArrayList<Identifier>(); 3563 return this.identifier; 3564 } 3565 3566 /** 3567 * @return Returns a reference to <code>this</code> for easy method chaining 3568 */ 3569 public CarePlan setIdentifier(List<Identifier> theIdentifier) { 3570 this.identifier = theIdentifier; 3571 return this; 3572 } 3573 3574 public boolean hasIdentifier() { 3575 if (this.identifier == null) 3576 return false; 3577 for (Identifier item : this.identifier) 3578 if (!item.isEmpty()) 3579 return true; 3580 return false; 3581 } 3582 3583 public Identifier addIdentifier() { // 3 3584 Identifier t = new Identifier(); 3585 if (this.identifier == null) 3586 this.identifier = new ArrayList<Identifier>(); 3587 this.identifier.add(t); 3588 return t; 3589 } 3590 3591 public CarePlan addIdentifier(Identifier t) { // 3 3592 if (t == null) 3593 return this; 3594 if (this.identifier == null) 3595 this.identifier = new ArrayList<Identifier>(); 3596 this.identifier.add(t); 3597 return this; 3598 } 3599 3600 /** 3601 * @return The first repetition of repeating field {@link #identifier}, creating 3602 * it if it does not already exist 3603 */ 3604 public Identifier getIdentifierFirstRep() { 3605 if (getIdentifier().isEmpty()) { 3606 addIdentifier(); 3607 } 3608 return getIdentifier().get(0); 3609 } 3610 3611 /** 3612 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 3613 * protocol, guideline, questionnaire or other definition that is 3614 * adhered to in whole or in part by this CarePlan.) 3615 */ 3616 public List<CanonicalType> getInstantiatesCanonical() { 3617 if (this.instantiatesCanonical == null) 3618 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3619 return this.instantiatesCanonical; 3620 } 3621 3622 /** 3623 * @return Returns a reference to <code>this</code> for easy method chaining 3624 */ 3625 public CarePlan setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 3626 this.instantiatesCanonical = theInstantiatesCanonical; 3627 return this; 3628 } 3629 3630 public boolean hasInstantiatesCanonical() { 3631 if (this.instantiatesCanonical == null) 3632 return false; 3633 for (CanonicalType item : this.instantiatesCanonical) 3634 if (!item.isEmpty()) 3635 return true; 3636 return false; 3637 } 3638 3639 /** 3640 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 3641 * protocol, guideline, questionnaire or other definition that is 3642 * adhered to in whole or in part by this CarePlan.) 3643 */ 3644 public CanonicalType addInstantiatesCanonicalElement() {// 2 3645 CanonicalType t = new CanonicalType(); 3646 if (this.instantiatesCanonical == null) 3647 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3648 this.instantiatesCanonical.add(t); 3649 return t; 3650 } 3651 3652 /** 3653 * @param value {@link #instantiatesCanonical} (The URL pointing to a 3654 * FHIR-defined protocol, guideline, questionnaire or other 3655 * definition that is adhered to in whole or in part by this 3656 * CarePlan.) 3657 */ 3658 public CarePlan addInstantiatesCanonical(String value) { // 1 3659 CanonicalType t = new CanonicalType(); 3660 t.setValue(value); 3661 if (this.instantiatesCanonical == null) 3662 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3663 this.instantiatesCanonical.add(t); 3664 return this; 3665 } 3666 3667 /** 3668 * @param value {@link #instantiatesCanonical} (The URL pointing to a 3669 * FHIR-defined protocol, guideline, questionnaire or other 3670 * definition that is adhered to in whole or in part by this 3671 * CarePlan.) 3672 */ 3673 public boolean hasInstantiatesCanonical(String value) { 3674 if (this.instantiatesCanonical == null) 3675 return false; 3676 for (CanonicalType v : this.instantiatesCanonical) 3677 if (v.getValue().equals(value)) // canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition) 3678 return true; 3679 return false; 3680 } 3681 3682 /** 3683 * @return {@link #instantiatesUri} (The URL pointing to an externally 3684 * maintained protocol, guideline, questionnaire or other definition 3685 * that is adhered to in whole or in part by this CarePlan.) 3686 */ 3687 public List<UriType> getInstantiatesUri() { 3688 if (this.instantiatesUri == null) 3689 this.instantiatesUri = new ArrayList<UriType>(); 3690 return this.instantiatesUri; 3691 } 3692 3693 /** 3694 * @return Returns a reference to <code>this</code> for easy method chaining 3695 */ 3696 public CarePlan setInstantiatesUri(List<UriType> theInstantiatesUri) { 3697 this.instantiatesUri = theInstantiatesUri; 3698 return this; 3699 } 3700 3701 public boolean hasInstantiatesUri() { 3702 if (this.instantiatesUri == null) 3703 return false; 3704 for (UriType item : this.instantiatesUri) 3705 if (!item.isEmpty()) 3706 return true; 3707 return false; 3708 } 3709 3710 /** 3711 * @return {@link #instantiatesUri} (The URL pointing to an externally 3712 * maintained protocol, guideline, questionnaire or other definition 3713 * that is adhered to in whole or in part by this CarePlan.) 3714 */ 3715 public UriType addInstantiatesUriElement() {// 2 3716 UriType t = new UriType(); 3717 if (this.instantiatesUri == null) 3718 this.instantiatesUri = new ArrayList<UriType>(); 3719 this.instantiatesUri.add(t); 3720 return t; 3721 } 3722 3723 /** 3724 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3725 * maintained protocol, guideline, questionnaire or other 3726 * definition that is adhered to in whole or in part by this 3727 * CarePlan.) 3728 */ 3729 public CarePlan addInstantiatesUri(String value) { // 1 3730 UriType t = new UriType(); 3731 t.setValue(value); 3732 if (this.instantiatesUri == null) 3733 this.instantiatesUri = new ArrayList<UriType>(); 3734 this.instantiatesUri.add(t); 3735 return this; 3736 } 3737 3738 /** 3739 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3740 * maintained protocol, guideline, questionnaire or other 3741 * definition that is adhered to in whole or in part by this 3742 * CarePlan.) 3743 */ 3744 public boolean hasInstantiatesUri(String value) { 3745 if (this.instantiatesUri == null) 3746 return false; 3747 for (UriType v : this.instantiatesUri) 3748 if (v.getValue().equals(value)) // uri 3749 return true; 3750 return false; 3751 } 3752 3753 /** 3754 * @return {@link #basedOn} (A care plan that is fulfilled in whole or in part 3755 * by this care plan.) 3756 */ 3757 public List<Reference> getBasedOn() { 3758 if (this.basedOn == null) 3759 this.basedOn = new ArrayList<Reference>(); 3760 return this.basedOn; 3761 } 3762 3763 /** 3764 * @return Returns a reference to <code>this</code> for easy method chaining 3765 */ 3766 public CarePlan setBasedOn(List<Reference> theBasedOn) { 3767 this.basedOn = theBasedOn; 3768 return this; 3769 } 3770 3771 public boolean hasBasedOn() { 3772 if (this.basedOn == null) 3773 return false; 3774 for (Reference item : this.basedOn) 3775 if (!item.isEmpty()) 3776 return true; 3777 return false; 3778 } 3779 3780 public Reference addBasedOn() { // 3 3781 Reference t = new Reference(); 3782 if (this.basedOn == null) 3783 this.basedOn = new ArrayList<Reference>(); 3784 this.basedOn.add(t); 3785 return t; 3786 } 3787 3788 public CarePlan addBasedOn(Reference t) { // 3 3789 if (t == null) 3790 return this; 3791 if (this.basedOn == null) 3792 this.basedOn = new ArrayList<Reference>(); 3793 this.basedOn.add(t); 3794 return this; 3795 } 3796 3797 /** 3798 * @return The first repetition of repeating field {@link #basedOn}, creating it 3799 * if it does not already exist 3800 */ 3801 public Reference getBasedOnFirstRep() { 3802 if (getBasedOn().isEmpty()) { 3803 addBasedOn(); 3804 } 3805 return getBasedOn().get(0); 3806 } 3807 3808 /** 3809 * @deprecated Use Reference#setResource(IBaseResource) instead 3810 */ 3811 @Deprecated 3812 public List<CarePlan> getBasedOnTarget() { 3813 if (this.basedOnTarget == null) 3814 this.basedOnTarget = new ArrayList<CarePlan>(); 3815 return this.basedOnTarget; 3816 } 3817 3818 /** 3819 * @deprecated Use Reference#setResource(IBaseResource) instead 3820 */ 3821 @Deprecated 3822 public CarePlan addBasedOnTarget() { 3823 CarePlan r = new CarePlan(); 3824 if (this.basedOnTarget == null) 3825 this.basedOnTarget = new ArrayList<CarePlan>(); 3826 this.basedOnTarget.add(r); 3827 return r; 3828 } 3829 3830 /** 3831 * @return {@link #replaces} (Completed or terminated care plan whose function 3832 * is taken by this new care plan.) 3833 */ 3834 public List<Reference> getReplaces() { 3835 if (this.replaces == null) 3836 this.replaces = new ArrayList<Reference>(); 3837 return this.replaces; 3838 } 3839 3840 /** 3841 * @return Returns a reference to <code>this</code> for easy method chaining 3842 */ 3843 public CarePlan setReplaces(List<Reference> theReplaces) { 3844 this.replaces = theReplaces; 3845 return this; 3846 } 3847 3848 public boolean hasReplaces() { 3849 if (this.replaces == null) 3850 return false; 3851 for (Reference item : this.replaces) 3852 if (!item.isEmpty()) 3853 return true; 3854 return false; 3855 } 3856 3857 public Reference addReplaces() { // 3 3858 Reference t = new Reference(); 3859 if (this.replaces == null) 3860 this.replaces = new ArrayList<Reference>(); 3861 this.replaces.add(t); 3862 return t; 3863 } 3864 3865 public CarePlan addReplaces(Reference t) { // 3 3866 if (t == null) 3867 return this; 3868 if (this.replaces == null) 3869 this.replaces = new ArrayList<Reference>(); 3870 this.replaces.add(t); 3871 return this; 3872 } 3873 3874 /** 3875 * @return The first repetition of repeating field {@link #replaces}, creating 3876 * it if it does not already exist 3877 */ 3878 public Reference getReplacesFirstRep() { 3879 if (getReplaces().isEmpty()) { 3880 addReplaces(); 3881 } 3882 return getReplaces().get(0); 3883 } 3884 3885 /** 3886 * @deprecated Use Reference#setResource(IBaseResource) instead 3887 */ 3888 @Deprecated 3889 public List<CarePlan> getReplacesTarget() { 3890 if (this.replacesTarget == null) 3891 this.replacesTarget = new ArrayList<CarePlan>(); 3892 return this.replacesTarget; 3893 } 3894 3895 /** 3896 * @deprecated Use Reference#setResource(IBaseResource) instead 3897 */ 3898 @Deprecated 3899 public CarePlan addReplacesTarget() { 3900 CarePlan r = new CarePlan(); 3901 if (this.replacesTarget == null) 3902 this.replacesTarget = new ArrayList<CarePlan>(); 3903 this.replacesTarget.add(r); 3904 return r; 3905 } 3906 3907 /** 3908 * @return {@link #partOf} (A larger care plan of which this particular care 3909 * plan is a component or step.) 3910 */ 3911 public List<Reference> getPartOf() { 3912 if (this.partOf == null) 3913 this.partOf = new ArrayList<Reference>(); 3914 return this.partOf; 3915 } 3916 3917 /** 3918 * @return Returns a reference to <code>this</code> for easy method chaining 3919 */ 3920 public CarePlan setPartOf(List<Reference> thePartOf) { 3921 this.partOf = thePartOf; 3922 return this; 3923 } 3924 3925 public boolean hasPartOf() { 3926 if (this.partOf == null) 3927 return false; 3928 for (Reference item : this.partOf) 3929 if (!item.isEmpty()) 3930 return true; 3931 return false; 3932 } 3933 3934 public Reference addPartOf() { // 3 3935 Reference t = new Reference(); 3936 if (this.partOf == null) 3937 this.partOf = new ArrayList<Reference>(); 3938 this.partOf.add(t); 3939 return t; 3940 } 3941 3942 public CarePlan addPartOf(Reference t) { // 3 3943 if (t == null) 3944 return this; 3945 if (this.partOf == null) 3946 this.partOf = new ArrayList<Reference>(); 3947 this.partOf.add(t); 3948 return this; 3949 } 3950 3951 /** 3952 * @return The first repetition of repeating field {@link #partOf}, creating it 3953 * if it does not already exist 3954 */ 3955 public Reference getPartOfFirstRep() { 3956 if (getPartOf().isEmpty()) { 3957 addPartOf(); 3958 } 3959 return getPartOf().get(0); 3960 } 3961 3962 /** 3963 * @deprecated Use Reference#setResource(IBaseResource) instead 3964 */ 3965 @Deprecated 3966 public List<CarePlan> getPartOfTarget() { 3967 if (this.partOfTarget == null) 3968 this.partOfTarget = new ArrayList<CarePlan>(); 3969 return this.partOfTarget; 3970 } 3971 3972 /** 3973 * @deprecated Use Reference#setResource(IBaseResource) instead 3974 */ 3975 @Deprecated 3976 public CarePlan addPartOfTarget() { 3977 CarePlan r = new CarePlan(); 3978 if (this.partOfTarget == null) 3979 this.partOfTarget = new ArrayList<CarePlan>(); 3980 this.partOfTarget.add(r); 3981 return r; 3982 } 3983 3984 /** 3985 * @return {@link #status} (Indicates whether the plan is currently being acted 3986 * upon, represents future intentions or is now a historical record.). 3987 * This is the underlying object with id, value and extensions. The 3988 * accessor "getStatus" gives direct access to the value 3989 */ 3990 public Enumeration<CarePlanStatus> getStatusElement() { 3991 if (this.status == null) 3992 if (Configuration.errorOnAutoCreate()) 3993 throw new Error("Attempt to auto-create CarePlan.status"); 3994 else if (Configuration.doAutoCreate()) 3995 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); // bb 3996 return this.status; 3997 } 3998 3999 public boolean hasStatusElement() { 4000 return this.status != null && !this.status.isEmpty(); 4001 } 4002 4003 public boolean hasStatus() { 4004 return this.status != null && !this.status.isEmpty(); 4005 } 4006 4007 /** 4008 * @param value {@link #status} (Indicates whether the plan is currently being 4009 * acted upon, represents future intentions or is now a historical 4010 * record.). This is the underlying object with id, value and 4011 * extensions. The accessor "getStatus" gives direct access to the 4012 * value 4013 */ 4014 public CarePlan setStatusElement(Enumeration<CarePlanStatus> value) { 4015 this.status = value; 4016 return this; 4017 } 4018 4019 /** 4020 * @return Indicates whether the plan is currently being acted upon, represents 4021 * future intentions or is now a historical record. 4022 */ 4023 public CarePlanStatus getStatus() { 4024 return this.status == null ? null : this.status.getValue(); 4025 } 4026 4027 /** 4028 * @param value Indicates whether the plan is currently being acted upon, 4029 * represents future intentions or is now a historical record. 4030 */ 4031 public CarePlan setStatus(CarePlanStatus value) { 4032 if (this.status == null) 4033 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); 4034 this.status.setValue(value); 4035 return this; 4036 } 4037 4038 /** 4039 * @return {@link #intent} (Indicates the level of authority/intentionality 4040 * associated with the care plan and where the care plan fits into the 4041 * workflow chain.). This is the underlying object with id, value and 4042 * extensions. The accessor "getIntent" gives direct access to the value 4043 */ 4044 public Enumeration<CarePlanIntent> getIntentElement() { 4045 if (this.intent == null) 4046 if (Configuration.errorOnAutoCreate()) 4047 throw new Error("Attempt to auto-create CarePlan.intent"); 4048 else if (Configuration.doAutoCreate()) 4049 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); // bb 4050 return this.intent; 4051 } 4052 4053 public boolean hasIntentElement() { 4054 return this.intent != null && !this.intent.isEmpty(); 4055 } 4056 4057 public boolean hasIntent() { 4058 return this.intent != null && !this.intent.isEmpty(); 4059 } 4060 4061 /** 4062 * @param value {@link #intent} (Indicates the level of authority/intentionality 4063 * associated with the care plan and where the care plan fits into 4064 * the workflow chain.). This is the underlying object with id, 4065 * value and extensions. The accessor "getIntent" gives direct 4066 * access to the value 4067 */ 4068 public CarePlan setIntentElement(Enumeration<CarePlanIntent> value) { 4069 this.intent = value; 4070 return this; 4071 } 4072 4073 /** 4074 * @return Indicates the level of authority/intentionality associated with the 4075 * care plan and where the care plan fits into the workflow chain. 4076 */ 4077 public CarePlanIntent getIntent() { 4078 return this.intent == null ? null : this.intent.getValue(); 4079 } 4080 4081 /** 4082 * @param value Indicates the level of authority/intentionality associated with 4083 * the care plan and where the care plan fits into the workflow 4084 * chain. 4085 */ 4086 public CarePlan setIntent(CarePlanIntent value) { 4087 if (this.intent == null) 4088 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); 4089 this.intent.setValue(value); 4090 return this; 4091 } 4092 4093 /** 4094 * @return {@link #category} (Identifies what "kind" of plan this is to support 4095 * differentiation between multiple co-existing plans; e.g. "Home 4096 * health", "psychiatric", "asthma", "disease management", "wellness 4097 * plan", etc.) 4098 */ 4099 public List<CodeableConcept> getCategory() { 4100 if (this.category == null) 4101 this.category = new ArrayList<CodeableConcept>(); 4102 return this.category; 4103 } 4104 4105 /** 4106 * @return Returns a reference to <code>this</code> for easy method chaining 4107 */ 4108 public CarePlan setCategory(List<CodeableConcept> theCategory) { 4109 this.category = theCategory; 4110 return this; 4111 } 4112 4113 public boolean hasCategory() { 4114 if (this.category == null) 4115 return false; 4116 for (CodeableConcept item : this.category) 4117 if (!item.isEmpty()) 4118 return true; 4119 return false; 4120 } 4121 4122 public CodeableConcept addCategory() { // 3 4123 CodeableConcept t = new CodeableConcept(); 4124 if (this.category == null) 4125 this.category = new ArrayList<CodeableConcept>(); 4126 this.category.add(t); 4127 return t; 4128 } 4129 4130 public CarePlan addCategory(CodeableConcept t) { // 3 4131 if (t == null) 4132 return this; 4133 if (this.category == null) 4134 this.category = new ArrayList<CodeableConcept>(); 4135 this.category.add(t); 4136 return this; 4137 } 4138 4139 /** 4140 * @return The first repetition of repeating field {@link #category}, creating 4141 * it if it does not already exist 4142 */ 4143 public CodeableConcept getCategoryFirstRep() { 4144 if (getCategory().isEmpty()) { 4145 addCategory(); 4146 } 4147 return getCategory().get(0); 4148 } 4149 4150 /** 4151 * @return {@link #title} (Human-friendly name for the care plan.). This is the 4152 * underlying object with id, value and extensions. The accessor 4153 * "getTitle" gives direct access to the value 4154 */ 4155 public StringType getTitleElement() { 4156 if (this.title == null) 4157 if (Configuration.errorOnAutoCreate()) 4158 throw new Error("Attempt to auto-create CarePlan.title"); 4159 else if (Configuration.doAutoCreate()) 4160 this.title = new StringType(); // bb 4161 return this.title; 4162 } 4163 4164 public boolean hasTitleElement() { 4165 return this.title != null && !this.title.isEmpty(); 4166 } 4167 4168 public boolean hasTitle() { 4169 return this.title != null && !this.title.isEmpty(); 4170 } 4171 4172 /** 4173 * @param value {@link #title} (Human-friendly name for the care plan.). This is 4174 * the underlying object with id, value and extensions. The 4175 * accessor "getTitle" gives direct access to the value 4176 */ 4177 public CarePlan setTitleElement(StringType value) { 4178 this.title = value; 4179 return this; 4180 } 4181 4182 /** 4183 * @return Human-friendly name for the care plan. 4184 */ 4185 public String getTitle() { 4186 return this.title == null ? null : this.title.getValue(); 4187 } 4188 4189 /** 4190 * @param value Human-friendly name for the care plan. 4191 */ 4192 public CarePlan setTitle(String value) { 4193 if (Utilities.noString(value)) 4194 this.title = null; 4195 else { 4196 if (this.title == null) 4197 this.title = new StringType(); 4198 this.title.setValue(value); 4199 } 4200 return this; 4201 } 4202 4203 /** 4204 * @return {@link #description} (A description of the scope and nature of the 4205 * plan.). This is the underlying object with id, value and extensions. 4206 * The accessor "getDescription" gives direct access to the value 4207 */ 4208 public StringType getDescriptionElement() { 4209 if (this.description == null) 4210 if (Configuration.errorOnAutoCreate()) 4211 throw new Error("Attempt to auto-create CarePlan.description"); 4212 else if (Configuration.doAutoCreate()) 4213 this.description = new StringType(); // bb 4214 return this.description; 4215 } 4216 4217 public boolean hasDescriptionElement() { 4218 return this.description != null && !this.description.isEmpty(); 4219 } 4220 4221 public boolean hasDescription() { 4222 return this.description != null && !this.description.isEmpty(); 4223 } 4224 4225 /** 4226 * @param value {@link #description} (A description of the scope and nature of 4227 * the plan.). This is the underlying object with id, value and 4228 * extensions. The accessor "getDescription" gives direct access to 4229 * the value 4230 */ 4231 public CarePlan setDescriptionElement(StringType value) { 4232 this.description = value; 4233 return this; 4234 } 4235 4236 /** 4237 * @return A description of the scope and nature of the plan. 4238 */ 4239 public String getDescription() { 4240 return this.description == null ? null : this.description.getValue(); 4241 } 4242 4243 /** 4244 * @param value A description of the scope and nature of the plan. 4245 */ 4246 public CarePlan setDescription(String value) { 4247 if (Utilities.noString(value)) 4248 this.description = null; 4249 else { 4250 if (this.description == null) 4251 this.description = new StringType(); 4252 this.description.setValue(value); 4253 } 4254 return this; 4255 } 4256 4257 /** 4258 * @return {@link #subject} (Identifies the patient or group whose intended care 4259 * is described by the plan.) 4260 */ 4261 public Reference getSubject() { 4262 if (this.subject == null) 4263 if (Configuration.errorOnAutoCreate()) 4264 throw new Error("Attempt to auto-create CarePlan.subject"); 4265 else if (Configuration.doAutoCreate()) 4266 this.subject = new Reference(); // cc 4267 return this.subject; 4268 } 4269 4270 public boolean hasSubject() { 4271 return this.subject != null && !this.subject.isEmpty(); 4272 } 4273 4274 /** 4275 * @param value {@link #subject} (Identifies the patient or group whose intended 4276 * care is described by the plan.) 4277 */ 4278 public CarePlan setSubject(Reference value) { 4279 this.subject = value; 4280 return this; 4281 } 4282 4283 /** 4284 * @return {@link #subject} The actual object that is the target of the 4285 * reference. The reference library doesn't populate this, but you can 4286 * use it to hold the resource if you resolve it. (Identifies the 4287 * patient or group whose intended care is described by the plan.) 4288 */ 4289 public Resource getSubjectTarget() { 4290 return this.subjectTarget; 4291 } 4292 4293 /** 4294 * @param value {@link #subject} The actual object that is the target of the 4295 * reference. The reference library doesn't use these, but you can 4296 * use it to hold the resource if you resolve it. (Identifies the 4297 * patient or group whose intended care is described by the plan.) 4298 */ 4299 public CarePlan setSubjectTarget(Resource value) { 4300 this.subjectTarget = value; 4301 return this; 4302 } 4303 4304 /** 4305 * @return {@link #encounter} (The Encounter during which this CarePlan was 4306 * created or to which the creation of this record is tightly 4307 * associated.) 4308 */ 4309 public Reference getEncounter() { 4310 if (this.encounter == null) 4311 if (Configuration.errorOnAutoCreate()) 4312 throw new Error("Attempt to auto-create CarePlan.encounter"); 4313 else if (Configuration.doAutoCreate()) 4314 this.encounter = new Reference(); // cc 4315 return this.encounter; 4316 } 4317 4318 public boolean hasEncounter() { 4319 return this.encounter != null && !this.encounter.isEmpty(); 4320 } 4321 4322 /** 4323 * @param value {@link #encounter} (The Encounter during which this CarePlan was 4324 * created or to which the creation of this record is tightly 4325 * associated.) 4326 */ 4327 public CarePlan setEncounter(Reference value) { 4328 this.encounter = value; 4329 return this; 4330 } 4331 4332 /** 4333 * @return {@link #encounter} The actual object that is the target of the 4334 * reference. The reference library doesn't populate this, but you can 4335 * use it to hold the resource if you resolve it. (The Encounter during 4336 * which this CarePlan was created or to which the creation of this 4337 * record is tightly associated.) 4338 */ 4339 public Encounter getEncounterTarget() { 4340 if (this.encounterTarget == null) 4341 if (Configuration.errorOnAutoCreate()) 4342 throw new Error("Attempt to auto-create CarePlan.encounter"); 4343 else if (Configuration.doAutoCreate()) 4344 this.encounterTarget = new Encounter(); // aa 4345 return this.encounterTarget; 4346 } 4347 4348 /** 4349 * @param value {@link #encounter} The actual object that is the target of the 4350 * reference. The reference library doesn't use these, but you can 4351 * use it to hold the resource if you resolve it. (The Encounter 4352 * during which this CarePlan was created or to which the creation 4353 * of this record is tightly associated.) 4354 */ 4355 public CarePlan setEncounterTarget(Encounter value) { 4356 this.encounterTarget = value; 4357 return this; 4358 } 4359 4360 /** 4361 * @return {@link #period} (Indicates when the plan did (or is intended to) come 4362 * into effect and end.) 4363 */ 4364 public Period getPeriod() { 4365 if (this.period == null) 4366 if (Configuration.errorOnAutoCreate()) 4367 throw new Error("Attempt to auto-create CarePlan.period"); 4368 else if (Configuration.doAutoCreate()) 4369 this.period = new Period(); // cc 4370 return this.period; 4371 } 4372 4373 public boolean hasPeriod() { 4374 return this.period != null && !this.period.isEmpty(); 4375 } 4376 4377 /** 4378 * @param value {@link #period} (Indicates when the plan did (or is intended to) 4379 * come into effect and end.) 4380 */ 4381 public CarePlan setPeriod(Period value) { 4382 this.period = value; 4383 return this; 4384 } 4385 4386 /** 4387 * @return {@link #created} (Represents when this particular CarePlan record was 4388 * created in the system, which is often a system-generated date.). This 4389 * is the underlying object with id, value and extensions. The accessor 4390 * "getCreated" gives direct access to the value 4391 */ 4392 public DateTimeType getCreatedElement() { 4393 if (this.created == null) 4394 if (Configuration.errorOnAutoCreate()) 4395 throw new Error("Attempt to auto-create CarePlan.created"); 4396 else if (Configuration.doAutoCreate()) 4397 this.created = new DateTimeType(); // bb 4398 return this.created; 4399 } 4400 4401 public boolean hasCreatedElement() { 4402 return this.created != null && !this.created.isEmpty(); 4403 } 4404 4405 public boolean hasCreated() { 4406 return this.created != null && !this.created.isEmpty(); 4407 } 4408 4409 /** 4410 * @param value {@link #created} (Represents when this particular CarePlan 4411 * record was created in the system, which is often a 4412 * system-generated date.). This is the underlying object with id, 4413 * value and extensions. The accessor "getCreated" gives direct 4414 * access to the value 4415 */ 4416 public CarePlan setCreatedElement(DateTimeType value) { 4417 this.created = value; 4418 return this; 4419 } 4420 4421 /** 4422 * @return Represents when this particular CarePlan record was created in the 4423 * system, which is often a system-generated date. 4424 */ 4425 public Date getCreated() { 4426 return this.created == null ? null : this.created.getValue(); 4427 } 4428 4429 /** 4430 * @param value Represents when this particular CarePlan record was created in 4431 * the system, which is often a system-generated date. 4432 */ 4433 public CarePlan setCreated(Date value) { 4434 if (value == null) 4435 this.created = null; 4436 else { 4437 if (this.created == null) 4438 this.created = new DateTimeType(); 4439 this.created.setValue(value); 4440 } 4441 return this; 4442 } 4443 4444 /** 4445 * @return {@link #author} (When populated, the author is responsible for the 4446 * care plan. The care plan is attributed to the author.) 4447 */ 4448 public Reference getAuthor() { 4449 if (this.author == null) 4450 if (Configuration.errorOnAutoCreate()) 4451 throw new Error("Attempt to auto-create CarePlan.author"); 4452 else if (Configuration.doAutoCreate()) 4453 this.author = new Reference(); // cc 4454 return this.author; 4455 } 4456 4457 public boolean hasAuthor() { 4458 return this.author != null && !this.author.isEmpty(); 4459 } 4460 4461 /** 4462 * @param value {@link #author} (When populated, the author is responsible for 4463 * the care plan. The care plan is attributed to the author.) 4464 */ 4465 public CarePlan setAuthor(Reference value) { 4466 this.author = value; 4467 return this; 4468 } 4469 4470 /** 4471 * @return {@link #author} The actual object that is the target of the 4472 * reference. The reference library doesn't populate this, but you can 4473 * use it to hold the resource if you resolve it. (When populated, the 4474 * author is responsible for the care plan. The care plan is attributed 4475 * to the author.) 4476 */ 4477 public Resource getAuthorTarget() { 4478 return this.authorTarget; 4479 } 4480 4481 /** 4482 * @param value {@link #author} The actual object that is the target of the 4483 * reference. The reference library doesn't use these, but you can 4484 * use it to hold the resource if you resolve it. (When populated, 4485 * the author is responsible for the care plan. The care plan is 4486 * attributed to the author.) 4487 */ 4488 public CarePlan setAuthorTarget(Resource value) { 4489 this.authorTarget = value; 4490 return this; 4491 } 4492 4493 /** 4494 * @return {@link #contributor} (Identifies the individual(s) or organization 4495 * who provided the contents of the care plan.) 4496 */ 4497 public List<Reference> getContributor() { 4498 if (this.contributor == null) 4499 this.contributor = new ArrayList<Reference>(); 4500 return this.contributor; 4501 } 4502 4503 /** 4504 * @return Returns a reference to <code>this</code> for easy method chaining 4505 */ 4506 public CarePlan setContributor(List<Reference> theContributor) { 4507 this.contributor = theContributor; 4508 return this; 4509 } 4510 4511 public boolean hasContributor() { 4512 if (this.contributor == null) 4513 return false; 4514 for (Reference item : this.contributor) 4515 if (!item.isEmpty()) 4516 return true; 4517 return false; 4518 } 4519 4520 public Reference addContributor() { // 3 4521 Reference t = new Reference(); 4522 if (this.contributor == null) 4523 this.contributor = new ArrayList<Reference>(); 4524 this.contributor.add(t); 4525 return t; 4526 } 4527 4528 public CarePlan addContributor(Reference t) { // 3 4529 if (t == null) 4530 return this; 4531 if (this.contributor == null) 4532 this.contributor = new ArrayList<Reference>(); 4533 this.contributor.add(t); 4534 return this; 4535 } 4536 4537 /** 4538 * @return The first repetition of repeating field {@link #contributor}, 4539 * creating it if it does not already exist 4540 */ 4541 public Reference getContributorFirstRep() { 4542 if (getContributor().isEmpty()) { 4543 addContributor(); 4544 } 4545 return getContributor().get(0); 4546 } 4547 4548 /** 4549 * @deprecated Use Reference#setResource(IBaseResource) instead 4550 */ 4551 @Deprecated 4552 public List<Resource> getContributorTarget() { 4553 if (this.contributorTarget == null) 4554 this.contributorTarget = new ArrayList<Resource>(); 4555 return this.contributorTarget; 4556 } 4557 4558 /** 4559 * @return {@link #careTeam} (Identifies all people and organizations who are 4560 * expected to be involved in the care envisioned by this plan.) 4561 */ 4562 public List<Reference> getCareTeam() { 4563 if (this.careTeam == null) 4564 this.careTeam = new ArrayList<Reference>(); 4565 return this.careTeam; 4566 } 4567 4568 /** 4569 * @return Returns a reference to <code>this</code> for easy method chaining 4570 */ 4571 public CarePlan setCareTeam(List<Reference> theCareTeam) { 4572 this.careTeam = theCareTeam; 4573 return this; 4574 } 4575 4576 public boolean hasCareTeam() { 4577 if (this.careTeam == null) 4578 return false; 4579 for (Reference item : this.careTeam) 4580 if (!item.isEmpty()) 4581 return true; 4582 return false; 4583 } 4584 4585 public Reference addCareTeam() { // 3 4586 Reference t = new Reference(); 4587 if (this.careTeam == null) 4588 this.careTeam = new ArrayList<Reference>(); 4589 this.careTeam.add(t); 4590 return t; 4591 } 4592 4593 public CarePlan addCareTeam(Reference t) { // 3 4594 if (t == null) 4595 return this; 4596 if (this.careTeam == null) 4597 this.careTeam = new ArrayList<Reference>(); 4598 this.careTeam.add(t); 4599 return this; 4600 } 4601 4602 /** 4603 * @return The first repetition of repeating field {@link #careTeam}, creating 4604 * it if it does not already exist 4605 */ 4606 public Reference getCareTeamFirstRep() { 4607 if (getCareTeam().isEmpty()) { 4608 addCareTeam(); 4609 } 4610 return getCareTeam().get(0); 4611 } 4612 4613 /** 4614 * @deprecated Use Reference#setResource(IBaseResource) instead 4615 */ 4616 @Deprecated 4617 public List<CareTeam> getCareTeamTarget() { 4618 if (this.careTeamTarget == null) 4619 this.careTeamTarget = new ArrayList<CareTeam>(); 4620 return this.careTeamTarget; 4621 } 4622 4623 /** 4624 * @deprecated Use Reference#setResource(IBaseResource) instead 4625 */ 4626 @Deprecated 4627 public CareTeam addCareTeamTarget() { 4628 CareTeam r = new CareTeam(); 4629 if (this.careTeamTarget == null) 4630 this.careTeamTarget = new ArrayList<CareTeam>(); 4631 this.careTeamTarget.add(r); 4632 return r; 4633 } 4634 4635 /** 4636 * @return {@link #addresses} (Identifies the 4637 * conditions/problems/concerns/diagnoses/etc. whose management and/or 4638 * mitigation are handled by this plan.) 4639 */ 4640 public List<Reference> getAddresses() { 4641 if (this.addresses == null) 4642 this.addresses = new ArrayList<Reference>(); 4643 return this.addresses; 4644 } 4645 4646 /** 4647 * @return Returns a reference to <code>this</code> for easy method chaining 4648 */ 4649 public CarePlan setAddresses(List<Reference> theAddresses) { 4650 this.addresses = theAddresses; 4651 return this; 4652 } 4653 4654 public boolean hasAddresses() { 4655 if (this.addresses == null) 4656 return false; 4657 for (Reference item : this.addresses) 4658 if (!item.isEmpty()) 4659 return true; 4660 return false; 4661 } 4662 4663 public Reference addAddresses() { // 3 4664 Reference t = new Reference(); 4665 if (this.addresses == null) 4666 this.addresses = new ArrayList<Reference>(); 4667 this.addresses.add(t); 4668 return t; 4669 } 4670 4671 public CarePlan addAddresses(Reference t) { // 3 4672 if (t == null) 4673 return this; 4674 if (this.addresses == null) 4675 this.addresses = new ArrayList<Reference>(); 4676 this.addresses.add(t); 4677 return this; 4678 } 4679 4680 /** 4681 * @return The first repetition of repeating field {@link #addresses}, creating 4682 * it if it does not already exist 4683 */ 4684 public Reference getAddressesFirstRep() { 4685 if (getAddresses().isEmpty()) { 4686 addAddresses(); 4687 } 4688 return getAddresses().get(0); 4689 } 4690 4691 /** 4692 * @deprecated Use Reference#setResource(IBaseResource) instead 4693 */ 4694 @Deprecated 4695 public List<Condition> getAddressesTarget() { 4696 if (this.addressesTarget == null) 4697 this.addressesTarget = new ArrayList<Condition>(); 4698 return this.addressesTarget; 4699 } 4700 4701 /** 4702 * @deprecated Use Reference#setResource(IBaseResource) instead 4703 */ 4704 @Deprecated 4705 public Condition addAddressesTarget() { 4706 Condition r = new Condition(); 4707 if (this.addressesTarget == null) 4708 this.addressesTarget = new ArrayList<Condition>(); 4709 this.addressesTarget.add(r); 4710 return r; 4711 } 4712 4713 /** 4714 * @return {@link #supportingInfo} (Identifies portions of the patient's record 4715 * that specifically influenced the formation of the plan. These might 4716 * include comorbidities, recent procedures, limitations, recent 4717 * assessments, etc.) 4718 */ 4719 public List<Reference> getSupportingInfo() { 4720 if (this.supportingInfo == null) 4721 this.supportingInfo = new ArrayList<Reference>(); 4722 return this.supportingInfo; 4723 } 4724 4725 /** 4726 * @return Returns a reference to <code>this</code> for easy method chaining 4727 */ 4728 public CarePlan setSupportingInfo(List<Reference> theSupportingInfo) { 4729 this.supportingInfo = theSupportingInfo; 4730 return this; 4731 } 4732 4733 public boolean hasSupportingInfo() { 4734 if (this.supportingInfo == null) 4735 return false; 4736 for (Reference item : this.supportingInfo) 4737 if (!item.isEmpty()) 4738 return true; 4739 return false; 4740 } 4741 4742 public Reference addSupportingInfo() { // 3 4743 Reference t = new Reference(); 4744 if (this.supportingInfo == null) 4745 this.supportingInfo = new ArrayList<Reference>(); 4746 this.supportingInfo.add(t); 4747 return t; 4748 } 4749 4750 public CarePlan addSupportingInfo(Reference t) { // 3 4751 if (t == null) 4752 return this; 4753 if (this.supportingInfo == null) 4754 this.supportingInfo = new ArrayList<Reference>(); 4755 this.supportingInfo.add(t); 4756 return this; 4757 } 4758 4759 /** 4760 * @return The first repetition of repeating field {@link #supportingInfo}, 4761 * creating it if it does not already exist 4762 */ 4763 public Reference getSupportingInfoFirstRep() { 4764 if (getSupportingInfo().isEmpty()) { 4765 addSupportingInfo(); 4766 } 4767 return getSupportingInfo().get(0); 4768 } 4769 4770 /** 4771 * @deprecated Use Reference#setResource(IBaseResource) instead 4772 */ 4773 @Deprecated 4774 public List<Resource> getSupportingInfoTarget() { 4775 if (this.supportingInfoTarget == null) 4776 this.supportingInfoTarget = new ArrayList<Resource>(); 4777 return this.supportingInfoTarget; 4778 } 4779 4780 /** 4781 * @return {@link #goal} (Describes the intended objective(s) of carrying out 4782 * the care plan.) 4783 */ 4784 public List<Reference> getGoal() { 4785 if (this.goal == null) 4786 this.goal = new ArrayList<Reference>(); 4787 return this.goal; 4788 } 4789 4790 /** 4791 * @return Returns a reference to <code>this</code> for easy method chaining 4792 */ 4793 public CarePlan setGoal(List<Reference> theGoal) { 4794 this.goal = theGoal; 4795 return this; 4796 } 4797 4798 public boolean hasGoal() { 4799 if (this.goal == null) 4800 return false; 4801 for (Reference item : this.goal) 4802 if (!item.isEmpty()) 4803 return true; 4804 return false; 4805 } 4806 4807 public Reference addGoal() { // 3 4808 Reference t = new Reference(); 4809 if (this.goal == null) 4810 this.goal = new ArrayList<Reference>(); 4811 this.goal.add(t); 4812 return t; 4813 } 4814 4815 public CarePlan addGoal(Reference t) { // 3 4816 if (t == null) 4817 return this; 4818 if (this.goal == null) 4819 this.goal = new ArrayList<Reference>(); 4820 this.goal.add(t); 4821 return this; 4822 } 4823 4824 /** 4825 * @return The first repetition of repeating field {@link #goal}, creating it if 4826 * it does not already exist 4827 */ 4828 public Reference getGoalFirstRep() { 4829 if (getGoal().isEmpty()) { 4830 addGoal(); 4831 } 4832 return getGoal().get(0); 4833 } 4834 4835 /** 4836 * @deprecated Use Reference#setResource(IBaseResource) instead 4837 */ 4838 @Deprecated 4839 public List<Goal> getGoalTarget() { 4840 if (this.goalTarget == null) 4841 this.goalTarget = new ArrayList<Goal>(); 4842 return this.goalTarget; 4843 } 4844 4845 /** 4846 * @deprecated Use Reference#setResource(IBaseResource) instead 4847 */ 4848 @Deprecated 4849 public Goal addGoalTarget() { 4850 Goal r = new Goal(); 4851 if (this.goalTarget == null) 4852 this.goalTarget = new ArrayList<Goal>(); 4853 this.goalTarget.add(r); 4854 return r; 4855 } 4856 4857 /** 4858 * @return {@link #activity} (Identifies a planned action to occur as part of 4859 * the plan. For example, a medication to be used, lab tests to perform, 4860 * self-monitoring, education, etc.) 4861 */ 4862 public List<CarePlanActivityComponent> getActivity() { 4863 if (this.activity == null) 4864 this.activity = new ArrayList<CarePlanActivityComponent>(); 4865 return this.activity; 4866 } 4867 4868 /** 4869 * @return Returns a reference to <code>this</code> for easy method chaining 4870 */ 4871 public CarePlan setActivity(List<CarePlanActivityComponent> theActivity) { 4872 this.activity = theActivity; 4873 return this; 4874 } 4875 4876 public boolean hasActivity() { 4877 if (this.activity == null) 4878 return false; 4879 for (CarePlanActivityComponent item : this.activity) 4880 if (!item.isEmpty()) 4881 return true; 4882 return false; 4883 } 4884 4885 public CarePlanActivityComponent addActivity() { // 3 4886 CarePlanActivityComponent t = new CarePlanActivityComponent(); 4887 if (this.activity == null) 4888 this.activity = new ArrayList<CarePlanActivityComponent>(); 4889 this.activity.add(t); 4890 return t; 4891 } 4892 4893 public CarePlan addActivity(CarePlanActivityComponent t) { // 3 4894 if (t == null) 4895 return this; 4896 if (this.activity == null) 4897 this.activity = new ArrayList<CarePlanActivityComponent>(); 4898 this.activity.add(t); 4899 return this; 4900 } 4901 4902 /** 4903 * @return The first repetition of repeating field {@link #activity}, creating 4904 * it if it does not already exist 4905 */ 4906 public CarePlanActivityComponent getActivityFirstRep() { 4907 if (getActivity().isEmpty()) { 4908 addActivity(); 4909 } 4910 return getActivity().get(0); 4911 } 4912 4913 /** 4914 * @return {@link #note} (General notes about the care plan not covered 4915 * elsewhere.) 4916 */ 4917 public List<Annotation> getNote() { 4918 if (this.note == null) 4919 this.note = new ArrayList<Annotation>(); 4920 return this.note; 4921 } 4922 4923 /** 4924 * @return Returns a reference to <code>this</code> for easy method chaining 4925 */ 4926 public CarePlan setNote(List<Annotation> theNote) { 4927 this.note = theNote; 4928 return this; 4929 } 4930 4931 public boolean hasNote() { 4932 if (this.note == null) 4933 return false; 4934 for (Annotation item : this.note) 4935 if (!item.isEmpty()) 4936 return true; 4937 return false; 4938 } 4939 4940 public Annotation addNote() { // 3 4941 Annotation t = new Annotation(); 4942 if (this.note == null) 4943 this.note = new ArrayList<Annotation>(); 4944 this.note.add(t); 4945 return t; 4946 } 4947 4948 public CarePlan addNote(Annotation t) { // 3 4949 if (t == null) 4950 return this; 4951 if (this.note == null) 4952 this.note = new ArrayList<Annotation>(); 4953 this.note.add(t); 4954 return this; 4955 } 4956 4957 /** 4958 * @return The first repetition of repeating field {@link #note}, creating it if 4959 * it does not already exist 4960 */ 4961 public Annotation getNoteFirstRep() { 4962 if (getNote().isEmpty()) { 4963 addNote(); 4964 } 4965 return getNote().get(0); 4966 } 4967 4968 protected void listChildren(List<Property> children) { 4969 super.listChildren(children); 4970 children.add(new Property("identifier", "Identifier", 4971 "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 4972 0, java.lang.Integer.MAX_VALUE, identifier)); 4973 children.add(new Property("instantiatesCanonical", 4974 "canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition)", 4975 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 4976 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 4977 children.add(new Property("instantiatesUri", "uri", 4978 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 4979 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 4980 children.add(new Property("basedOn", "Reference(CarePlan)", 4981 "A care plan that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, 4982 basedOn)); 4983 children.add(new Property("replaces", "Reference(CarePlan)", 4984 "Completed or terminated care plan whose function is taken by this new care plan.", 0, 4985 java.lang.Integer.MAX_VALUE, replaces)); 4986 children.add(new Property("partOf", "Reference(CarePlan)", 4987 "A larger care plan of which this particular care plan is a component or step.", 0, java.lang.Integer.MAX_VALUE, 4988 partOf)); 4989 children.add(new Property("status", "code", 4990 "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 4991 0, 1, status)); 4992 children.add(new Property("intent", "code", 4993 "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 4994 0, 1, intent)); 4995 children.add(new Property("category", "CodeableConcept", 4996 "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 4997 0, java.lang.Integer.MAX_VALUE, category)); 4998 children.add(new Property("title", "string", "Human-friendly name for the care plan.", 0, 1, title)); 4999 children.add( 5000 new Property("description", "string", "A description of the scope and nature of the plan.", 0, 1, description)); 5001 children.add(new Property("subject", "Reference(Patient|Group)", 5002 "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject)); 5003 children.add(new Property("encounter", "Reference(Encounter)", 5004 "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.", 5005 0, 1, encounter)); 5006 children.add(new Property("period", "Period", 5007 "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period)); 5008 children.add(new Property("created", "dateTime", 5009 "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.", 5010 0, 1, created)); 5011 children.add(new Property("author", 5012 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5013 "When populated, the author is responsible for the care plan. The care plan is attributed to the author.", 0, 5014 1, author)); 5015 children.add(new Property("contributor", 5016 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5017 "Identifies the individual(s) or organization who provided the contents of the care plan.", 0, 5018 java.lang.Integer.MAX_VALUE, contributor)); 5019 children.add(new Property("careTeam", "Reference(CareTeam)", 5020 "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 5021 0, java.lang.Integer.MAX_VALUE, careTeam)); 5022 children.add(new Property("addresses", "Reference(Condition)", 5023 "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 5024 0, java.lang.Integer.MAX_VALUE, addresses)); 5025 children.add(new Property("supportingInfo", "Reference(Any)", 5026 "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.", 5027 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 5028 children.add(new Property("goal", "Reference(Goal)", 5029 "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal)); 5030 children.add(new Property("activity", "", 5031 "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 5032 0, java.lang.Integer.MAX_VALUE, activity)); 5033 children.add(new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, 5034 java.lang.Integer.MAX_VALUE, note)); 5035 } 5036 5037 @Override 5038 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5039 switch (_hash) { 5040 case -1618432855: 5041 /* identifier */ return new Property("identifier", "Identifier", 5042 "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 5043 0, java.lang.Integer.MAX_VALUE, identifier); 5044 case 8911915: 5045 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 5046 "canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition)", 5047 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 5048 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 5049 case -1926393373: 5050 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 5051 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 5052 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 5053 case -332612366: 5054 /* basedOn */ return new Property("basedOn", "Reference(CarePlan)", 5055 "A care plan that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, 5056 basedOn); 5057 case -430332865: 5058 /* replaces */ return new Property("replaces", "Reference(CarePlan)", 5059 "Completed or terminated care plan whose function is taken by this new care plan.", 0, 5060 java.lang.Integer.MAX_VALUE, replaces); 5061 case -995410646: 5062 /* partOf */ return new Property("partOf", "Reference(CarePlan)", 5063 "A larger care plan of which this particular care plan is a component or step.", 0, 5064 java.lang.Integer.MAX_VALUE, partOf); 5065 case -892481550: 5066 /* status */ return new Property("status", "code", 5067 "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 5068 0, 1, status); 5069 case -1183762788: 5070 /* intent */ return new Property("intent", "code", 5071 "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 5072 0, 1, intent); 5073 case 50511102: 5074 /* category */ return new Property("category", "CodeableConcept", 5075 "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 5076 0, java.lang.Integer.MAX_VALUE, category); 5077 case 110371416: 5078 /* title */ return new Property("title", "string", "Human-friendly name for the care plan.", 0, 1, title); 5079 case -1724546052: 5080 /* description */ return new Property("description", "string", 5081 "A description of the scope and nature of the plan.", 0, 1, description); 5082 case -1867885268: 5083 /* subject */ return new Property("subject", "Reference(Patient|Group)", 5084 "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject); 5085 case 1524132147: 5086 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5087 "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.", 5088 0, 1, encounter); 5089 case -991726143: 5090 /* period */ return new Property("period", "Period", 5091 "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period); 5092 case 1028554472: 5093 /* created */ return new Property("created", "dateTime", 5094 "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.", 5095 0, 1, created); 5096 case -1406328437: 5097 /* author */ return new Property("author", 5098 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5099 "When populated, the author is responsible for the care plan. The care plan is attributed to the author.", 0, 5100 1, author); 5101 case -1895276325: 5102 /* contributor */ return new Property("contributor", 5103 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5104 "Identifies the individual(s) or organization who provided the contents of the care plan.", 0, 5105 java.lang.Integer.MAX_VALUE, contributor); 5106 case -7323378: 5107 /* careTeam */ return new Property("careTeam", "Reference(CareTeam)", 5108 "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 5109 0, java.lang.Integer.MAX_VALUE, careTeam); 5110 case 874544034: 5111 /* addresses */ return new Property("addresses", "Reference(Condition)", 5112 "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 5113 0, java.lang.Integer.MAX_VALUE, addresses); 5114 case 1922406657: 5115 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 5116 "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.", 5117 0, java.lang.Integer.MAX_VALUE, supportingInfo); 5118 case 3178259: 5119 /* goal */ return new Property("goal", "Reference(Goal)", 5120 "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal); 5121 case -1655966961: 5122 /* activity */ return new Property("activity", "", 5123 "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 5124 0, java.lang.Integer.MAX_VALUE, activity); 5125 case 3387378: 5126 /* note */ return new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 5127 0, java.lang.Integer.MAX_VALUE, note); 5128 default: 5129 return super.getNamedProperty(_hash, _name, _checkValid); 5130 } 5131 5132 } 5133 5134 @Override 5135 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5136 switch (hash) { 5137 case -1618432855: 5138 /* identifier */ return this.identifier == null ? new Base[0] 5139 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5140 case 8911915: 5141 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 5142 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 5143 case -1926393373: 5144 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 5145 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 5146 case -332612366: 5147 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 5148 case -430332865: 5149 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 5150 case -995410646: 5151 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 5152 case -892481550: 5153 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CarePlanStatus> 5154 case -1183762788: 5155 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<CarePlanIntent> 5156 case 50511102: 5157 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 5158 case 110371416: 5159 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5160 case -1724546052: 5161 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 5162 case -1867885268: 5163 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 5164 case 1524132147: 5165 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 5166 case -991726143: 5167 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 5168 case 1028554472: 5169 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 5170 case -1406328437: 5171 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 5172 case -1895276325: 5173 /* contributor */ return this.contributor == null ? new Base[0] 5174 : this.contributor.toArray(new Base[this.contributor.size()]); // Reference 5175 case -7323378: 5176 /* careTeam */ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // Reference 5177 case 874544034: 5178 /* addresses */ return this.addresses == null ? new Base[0] 5179 : this.addresses.toArray(new Base[this.addresses.size()]); // Reference 5180 case 1922406657: 5181 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 5182 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 5183 case 3178259: 5184 /* goal */ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 5185 case -1655966961: 5186 /* activity */ return this.activity == null ? new Base[0] : this.activity.toArray(new Base[this.activity.size()]); // CarePlanActivityComponent 5187 case 3387378: 5188 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5189 default: 5190 return super.getProperty(hash, name, checkValid); 5191 } 5192 5193 } 5194 5195 @Override 5196 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5197 switch (hash) { 5198 case -1618432855: // identifier 5199 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5200 return value; 5201 case 8911915: // instantiatesCanonical 5202 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 5203 return value; 5204 case -1926393373: // instantiatesUri 5205 this.getInstantiatesUri().add(castToUri(value)); // UriType 5206 return value; 5207 case -332612366: // basedOn 5208 this.getBasedOn().add(castToReference(value)); // Reference 5209 return value; 5210 case -430332865: // replaces 5211 this.getReplaces().add(castToReference(value)); // Reference 5212 return value; 5213 case -995410646: // partOf 5214 this.getPartOf().add(castToReference(value)); // Reference 5215 return value; 5216 case -892481550: // status 5217 value = new CarePlanStatusEnumFactory().fromType(castToCode(value)); 5218 this.status = (Enumeration) value; // Enumeration<CarePlanStatus> 5219 return value; 5220 case -1183762788: // intent 5221 value = new CarePlanIntentEnumFactory().fromType(castToCode(value)); 5222 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 5223 return value; 5224 case 50511102: // category 5225 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 5226 return value; 5227 case 110371416: // title 5228 this.title = castToString(value); // StringType 5229 return value; 5230 case -1724546052: // description 5231 this.description = castToString(value); // StringType 5232 return value; 5233 case -1867885268: // subject 5234 this.subject = castToReference(value); // Reference 5235 return value; 5236 case 1524132147: // encounter 5237 this.encounter = castToReference(value); // Reference 5238 return value; 5239 case -991726143: // period 5240 this.period = castToPeriod(value); // Period 5241 return value; 5242 case 1028554472: // created 5243 this.created = castToDateTime(value); // DateTimeType 5244 return value; 5245 case -1406328437: // author 5246 this.author = castToReference(value); // Reference 5247 return value; 5248 case -1895276325: // contributor 5249 this.getContributor().add(castToReference(value)); // Reference 5250 return value; 5251 case -7323378: // careTeam 5252 this.getCareTeam().add(castToReference(value)); // Reference 5253 return value; 5254 case 874544034: // addresses 5255 this.getAddresses().add(castToReference(value)); // Reference 5256 return value; 5257 case 1922406657: // supportingInfo 5258 this.getSupportingInfo().add(castToReference(value)); // Reference 5259 return value; 5260 case 3178259: // goal 5261 this.getGoal().add(castToReference(value)); // Reference 5262 return value; 5263 case -1655966961: // activity 5264 this.getActivity().add((CarePlanActivityComponent) value); // CarePlanActivityComponent 5265 return value; 5266 case 3387378: // note 5267 this.getNote().add(castToAnnotation(value)); // Annotation 5268 return value; 5269 default: 5270 return super.setProperty(hash, name, value); 5271 } 5272 5273 } 5274 5275 @Override 5276 public Base setProperty(String name, Base value) throws FHIRException { 5277 if (name.equals("identifier")) { 5278 this.getIdentifier().add(castToIdentifier(value)); 5279 } else if (name.equals("instantiatesCanonical")) { 5280 this.getInstantiatesCanonical().add(castToCanonical(value)); 5281 } else if (name.equals("instantiatesUri")) { 5282 this.getInstantiatesUri().add(castToUri(value)); 5283 } else if (name.equals("basedOn")) { 5284 this.getBasedOn().add(castToReference(value)); 5285 } else if (name.equals("replaces")) { 5286 this.getReplaces().add(castToReference(value)); 5287 } else if (name.equals("partOf")) { 5288 this.getPartOf().add(castToReference(value)); 5289 } else if (name.equals("status")) { 5290 value = new CarePlanStatusEnumFactory().fromType(castToCode(value)); 5291 this.status = (Enumeration) value; // Enumeration<CarePlanStatus> 5292 } else if (name.equals("intent")) { 5293 value = new CarePlanIntentEnumFactory().fromType(castToCode(value)); 5294 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 5295 } else if (name.equals("category")) { 5296 this.getCategory().add(castToCodeableConcept(value)); 5297 } else if (name.equals("title")) { 5298 this.title = castToString(value); // StringType 5299 } else if (name.equals("description")) { 5300 this.description = castToString(value); // StringType 5301 } else if (name.equals("subject")) { 5302 this.subject = castToReference(value); // Reference 5303 } else if (name.equals("encounter")) { 5304 this.encounter = castToReference(value); // Reference 5305 } else if (name.equals("period")) { 5306 this.period = castToPeriod(value); // Period 5307 } else if (name.equals("created")) { 5308 this.created = castToDateTime(value); // DateTimeType 5309 } else if (name.equals("author")) { 5310 this.author = castToReference(value); // Reference 5311 } else if (name.equals("contributor")) { 5312 this.getContributor().add(castToReference(value)); 5313 } else if (name.equals("careTeam")) { 5314 this.getCareTeam().add(castToReference(value)); 5315 } else if (name.equals("addresses")) { 5316 this.getAddresses().add(castToReference(value)); 5317 } else if (name.equals("supportingInfo")) { 5318 this.getSupportingInfo().add(castToReference(value)); 5319 } else if (name.equals("goal")) { 5320 this.getGoal().add(castToReference(value)); 5321 } else if (name.equals("activity")) { 5322 this.getActivity().add((CarePlanActivityComponent) value); 5323 } else if (name.equals("note")) { 5324 this.getNote().add(castToAnnotation(value)); 5325 } else 5326 return super.setProperty(name, value); 5327 return value; 5328 } 5329 5330 @Override 5331 public void removeChild(String name, Base value) throws FHIRException { 5332 if (name.equals("identifier")) { 5333 this.getIdentifier().remove(castToIdentifier(value)); 5334 } else if (name.equals("instantiatesCanonical")) { 5335 this.getInstantiatesCanonical().remove(castToCanonical(value)); 5336 } else if (name.equals("instantiatesUri")) { 5337 this.getInstantiatesUri().remove(castToUri(value)); 5338 } else if (name.equals("basedOn")) { 5339 this.getBasedOn().remove(castToReference(value)); 5340 } else if (name.equals("replaces")) { 5341 this.getReplaces().remove(castToReference(value)); 5342 } else if (name.equals("partOf")) { 5343 this.getPartOf().remove(castToReference(value)); 5344 } else if (name.equals("status")) { 5345 this.status = null; 5346 } else if (name.equals("intent")) { 5347 this.intent = null; 5348 } else if (name.equals("category")) { 5349 this.getCategory().remove(castToCodeableConcept(value)); 5350 } else if (name.equals("title")) { 5351 this.title = null; 5352 } else if (name.equals("description")) { 5353 this.description = null; 5354 } else if (name.equals("subject")) { 5355 this.subject = null; 5356 } else if (name.equals("encounter")) { 5357 this.encounter = null; 5358 } else if (name.equals("period")) { 5359 this.period = null; 5360 } else if (name.equals("created")) { 5361 this.created = null; 5362 } else if (name.equals("author")) { 5363 this.author = null; 5364 } else if (name.equals("contributor")) { 5365 this.getContributor().remove(castToReference(value)); 5366 } else if (name.equals("careTeam")) { 5367 this.getCareTeam().remove(castToReference(value)); 5368 } else if (name.equals("addresses")) { 5369 this.getAddresses().remove(castToReference(value)); 5370 } else if (name.equals("supportingInfo")) { 5371 this.getSupportingInfo().remove(castToReference(value)); 5372 } else if (name.equals("goal")) { 5373 this.getGoal().remove(castToReference(value)); 5374 } else if (name.equals("activity")) { 5375 this.getActivity().remove((CarePlanActivityComponent) value); 5376 } else if (name.equals("note")) { 5377 this.getNote().remove(castToAnnotation(value)); 5378 } else 5379 super.removeChild(name, value); 5380 5381 } 5382 5383 @Override 5384 public Base makeProperty(int hash, String name) throws FHIRException { 5385 switch (hash) { 5386 case -1618432855: 5387 return addIdentifier(); 5388 case 8911915: 5389 return addInstantiatesCanonicalElement(); 5390 case -1926393373: 5391 return addInstantiatesUriElement(); 5392 case -332612366: 5393 return addBasedOn(); 5394 case -430332865: 5395 return addReplaces(); 5396 case -995410646: 5397 return addPartOf(); 5398 case -892481550: 5399 return getStatusElement(); 5400 case -1183762788: 5401 return getIntentElement(); 5402 case 50511102: 5403 return addCategory(); 5404 case 110371416: 5405 return getTitleElement(); 5406 case -1724546052: 5407 return getDescriptionElement(); 5408 case -1867885268: 5409 return getSubject(); 5410 case 1524132147: 5411 return getEncounter(); 5412 case -991726143: 5413 return getPeriod(); 5414 case 1028554472: 5415 return getCreatedElement(); 5416 case -1406328437: 5417 return getAuthor(); 5418 case -1895276325: 5419 return addContributor(); 5420 case -7323378: 5421 return addCareTeam(); 5422 case 874544034: 5423 return addAddresses(); 5424 case 1922406657: 5425 return addSupportingInfo(); 5426 case 3178259: 5427 return addGoal(); 5428 case -1655966961: 5429 return addActivity(); 5430 case 3387378: 5431 return addNote(); 5432 default: 5433 return super.makeProperty(hash, name); 5434 } 5435 5436 } 5437 5438 @Override 5439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5440 switch (hash) { 5441 case -1618432855: 5442 /* identifier */ return new String[] { "Identifier" }; 5443 case 8911915: 5444 /* instantiatesCanonical */ return new String[] { "canonical" }; 5445 case -1926393373: 5446 /* instantiatesUri */ return new String[] { "uri" }; 5447 case -332612366: 5448 /* basedOn */ return new String[] { "Reference" }; 5449 case -430332865: 5450 /* replaces */ return new String[] { "Reference" }; 5451 case -995410646: 5452 /* partOf */ return new String[] { "Reference" }; 5453 case -892481550: 5454 /* status */ return new String[] { "code" }; 5455 case -1183762788: 5456 /* intent */ return new String[] { "code" }; 5457 case 50511102: 5458 /* category */ return new String[] { "CodeableConcept" }; 5459 case 110371416: 5460 /* title */ return new String[] { "string" }; 5461 case -1724546052: 5462 /* description */ return new String[] { "string" }; 5463 case -1867885268: 5464 /* subject */ return new String[] { "Reference" }; 5465 case 1524132147: 5466 /* encounter */ return new String[] { "Reference" }; 5467 case -991726143: 5468 /* period */ return new String[] { "Period" }; 5469 case 1028554472: 5470 /* created */ return new String[] { "dateTime" }; 5471 case -1406328437: 5472 /* author */ return new String[] { "Reference" }; 5473 case -1895276325: 5474 /* contributor */ return new String[] { "Reference" }; 5475 case -7323378: 5476 /* careTeam */ return new String[] { "Reference" }; 5477 case 874544034: 5478 /* addresses */ return new String[] { "Reference" }; 5479 case 1922406657: 5480 /* supportingInfo */ return new String[] { "Reference" }; 5481 case 3178259: 5482 /* goal */ return new String[] { "Reference" }; 5483 case -1655966961: 5484 /* activity */ return new String[] {}; 5485 case 3387378: 5486 /* note */ return new String[] { "Annotation" }; 5487 default: 5488 return super.getTypesForProperty(hash, name); 5489 } 5490 5491 } 5492 5493 @Override 5494 public Base addChild(String name) throws FHIRException { 5495 if (name.equals("identifier")) { 5496 return addIdentifier(); 5497 } else if (name.equals("instantiatesCanonical")) { 5498 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesCanonical"); 5499 } else if (name.equals("instantiatesUri")) { 5500 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesUri"); 5501 } else if (name.equals("basedOn")) { 5502 return addBasedOn(); 5503 } else if (name.equals("replaces")) { 5504 return addReplaces(); 5505 } else if (name.equals("partOf")) { 5506 return addPartOf(); 5507 } else if (name.equals("status")) { 5508 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 5509 } else if (name.equals("intent")) { 5510 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.intent"); 5511 } else if (name.equals("category")) { 5512 return addCategory(); 5513 } else if (name.equals("title")) { 5514 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.title"); 5515 } else if (name.equals("description")) { 5516 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 5517 } else if (name.equals("subject")) { 5518 this.subject = new Reference(); 5519 return this.subject; 5520 } else if (name.equals("encounter")) { 5521 this.encounter = new Reference(); 5522 return this.encounter; 5523 } else if (name.equals("period")) { 5524 this.period = new Period(); 5525 return this.period; 5526 } else if (name.equals("created")) { 5527 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.created"); 5528 } else if (name.equals("author")) { 5529 this.author = new Reference(); 5530 return this.author; 5531 } else if (name.equals("contributor")) { 5532 return addContributor(); 5533 } else if (name.equals("careTeam")) { 5534 return addCareTeam(); 5535 } else if (name.equals("addresses")) { 5536 return addAddresses(); 5537 } else if (name.equals("supportingInfo")) { 5538 return addSupportingInfo(); 5539 } else if (name.equals("goal")) { 5540 return addGoal(); 5541 } else if (name.equals("activity")) { 5542 return addActivity(); 5543 } else if (name.equals("note")) { 5544 return addNote(); 5545 } else 5546 return super.addChild(name); 5547 } 5548 5549 public String fhirType() { 5550 return "CarePlan"; 5551 5552 } 5553 5554 public CarePlan copy() { 5555 CarePlan dst = new CarePlan(); 5556 copyValues(dst); 5557 return dst; 5558 } 5559 5560 public void copyValues(CarePlan dst) { 5561 super.copyValues(dst); 5562 if (identifier != null) { 5563 dst.identifier = new ArrayList<Identifier>(); 5564 for (Identifier i : identifier) 5565 dst.identifier.add(i.copy()); 5566 } 5567 ; 5568 if (instantiatesCanonical != null) { 5569 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 5570 for (CanonicalType i : instantiatesCanonical) 5571 dst.instantiatesCanonical.add(i.copy()); 5572 } 5573 ; 5574 if (instantiatesUri != null) { 5575 dst.instantiatesUri = new ArrayList<UriType>(); 5576 for (UriType i : instantiatesUri) 5577 dst.instantiatesUri.add(i.copy()); 5578 } 5579 ; 5580 if (basedOn != null) { 5581 dst.basedOn = new ArrayList<Reference>(); 5582 for (Reference i : basedOn) 5583 dst.basedOn.add(i.copy()); 5584 } 5585 ; 5586 if (replaces != null) { 5587 dst.replaces = new ArrayList<Reference>(); 5588 for (Reference i : replaces) 5589 dst.replaces.add(i.copy()); 5590 } 5591 ; 5592 if (partOf != null) { 5593 dst.partOf = new ArrayList<Reference>(); 5594 for (Reference i : partOf) 5595 dst.partOf.add(i.copy()); 5596 } 5597 ; 5598 dst.status = status == null ? null : status.copy(); 5599 dst.intent = intent == null ? null : intent.copy(); 5600 if (category != null) { 5601 dst.category = new ArrayList<CodeableConcept>(); 5602 for (CodeableConcept i : category) 5603 dst.category.add(i.copy()); 5604 } 5605 ; 5606 dst.title = title == null ? null : title.copy(); 5607 dst.description = description == null ? null : description.copy(); 5608 dst.subject = subject == null ? null : subject.copy(); 5609 dst.encounter = encounter == null ? null : encounter.copy(); 5610 dst.period = period == null ? null : period.copy(); 5611 dst.created = created == null ? null : created.copy(); 5612 dst.author = author == null ? null : author.copy(); 5613 if (contributor != null) { 5614 dst.contributor = new ArrayList<Reference>(); 5615 for (Reference i : contributor) 5616 dst.contributor.add(i.copy()); 5617 } 5618 ; 5619 if (careTeam != null) { 5620 dst.careTeam = new ArrayList<Reference>(); 5621 for (Reference i : careTeam) 5622 dst.careTeam.add(i.copy()); 5623 } 5624 ; 5625 if (addresses != null) { 5626 dst.addresses = new ArrayList<Reference>(); 5627 for (Reference i : addresses) 5628 dst.addresses.add(i.copy()); 5629 } 5630 ; 5631 if (supportingInfo != null) { 5632 dst.supportingInfo = new ArrayList<Reference>(); 5633 for (Reference i : supportingInfo) 5634 dst.supportingInfo.add(i.copy()); 5635 } 5636 ; 5637 if (goal != null) { 5638 dst.goal = new ArrayList<Reference>(); 5639 for (Reference i : goal) 5640 dst.goal.add(i.copy()); 5641 } 5642 ; 5643 if (activity != null) { 5644 dst.activity = new ArrayList<CarePlanActivityComponent>(); 5645 for (CarePlanActivityComponent i : activity) 5646 dst.activity.add(i.copy()); 5647 } 5648 ; 5649 if (note != null) { 5650 dst.note = new ArrayList<Annotation>(); 5651 for (Annotation i : note) 5652 dst.note.add(i.copy()); 5653 } 5654 ; 5655 } 5656 5657 protected CarePlan typedCopy() { 5658 return copy(); 5659 } 5660 5661 @Override 5662 public boolean equalsDeep(Base other_) { 5663 if (!super.equalsDeep(other_)) 5664 return false; 5665 if (!(other_ instanceof CarePlan)) 5666 return false; 5667 CarePlan o = (CarePlan) other_; 5668 return compareDeep(identifier, o.identifier, true) 5669 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5670 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 5671 && compareDeep(replaces, o.replaces, true) && compareDeep(partOf, o.partOf, true) 5672 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 5673 && compareDeep(category, o.category, true) && compareDeep(title, o.title, true) 5674 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) 5675 && compareDeep(encounter, o.encounter, true) && compareDeep(period, o.period, true) 5676 && compareDeep(created, o.created, true) && compareDeep(author, o.author, true) 5677 && compareDeep(contributor, o.contributor, true) && compareDeep(careTeam, o.careTeam, true) 5678 && compareDeep(addresses, o.addresses, true) && compareDeep(supportingInfo, o.supportingInfo, true) 5679 && compareDeep(goal, o.goal, true) && compareDeep(activity, o.activity, true) 5680 && compareDeep(note, o.note, true); 5681 } 5682 5683 @Override 5684 public boolean equalsShallow(Base other_) { 5685 if (!super.equalsShallow(other_)) 5686 return false; 5687 if (!(other_ instanceof CarePlan)) 5688 return false; 5689 CarePlan o = (CarePlan) other_; 5690 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 5691 && compareValues(intent, o.intent, true) && compareValues(title, o.title, true) 5692 && compareValues(description, o.description, true) && compareValues(created, o.created, true); 5693 } 5694 5695 public boolean isEmpty() { 5696 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 5697 basedOn, replaces, partOf, status, intent, category, title, description, subject, encounter, period, created, 5698 author, contributor, careTeam, addresses, supportingInfo, goal, activity, note); 5699 } 5700 5701 @Override 5702 public ResourceType getResourceType() { 5703 return ResourceType.CarePlan; 5704 } 5705 5706 /** 5707 * Search parameter: <b>date</b> 5708 * <p> 5709 * Description: <b>Time period plan covers</b><br> 5710 * Type: <b>date</b><br> 5711 * Path: <b>CarePlan.period</b><br> 5712 * </p> 5713 */ 5714 @SearchParamDefinition(name = "date", path = "CarePlan.period", description = "Time period plan covers", type = "date") 5715 public static final String SP_DATE = "date"; 5716 /** 5717 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5718 * <p> 5719 * Description: <b>Time period plan covers</b><br> 5720 * Type: <b>date</b><br> 5721 * Path: <b>CarePlan.period</b><br> 5722 * </p> 5723 */ 5724 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5725 SP_DATE); 5726 5727 /** 5728 * Search parameter: <b>care-team</b> 5729 * <p> 5730 * Description: <b>Who's involved in plan?</b><br> 5731 * Type: <b>reference</b><br> 5732 * Path: <b>CarePlan.careTeam</b><br> 5733 * </p> 5734 */ 5735 @SearchParamDefinition(name = "care-team", path = "CarePlan.careTeam", description = "Who's involved in plan?", type = "reference", target = { 5736 CareTeam.class }) 5737 public static final String SP_CARE_TEAM = "care-team"; 5738 /** 5739 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 5740 * <p> 5741 * Description: <b>Who's involved in plan?</b><br> 5742 * Type: <b>reference</b><br> 5743 * Path: <b>CarePlan.careTeam</b><br> 5744 * </p> 5745 */ 5746 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5747 SP_CARE_TEAM); 5748 5749 /** 5750 * Constant for fluent queries to be used to add include statements. Specifies 5751 * the path value of "<b>CarePlan:care-team</b>". 5752 */ 5753 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include( 5754 "CarePlan:care-team").toLocked(); 5755 5756 /** 5757 * Search parameter: <b>identifier</b> 5758 * <p> 5759 * Description: <b>External Ids for this plan</b><br> 5760 * Type: <b>token</b><br> 5761 * Path: <b>CarePlan.identifier</b><br> 5762 * </p> 5763 */ 5764 @SearchParamDefinition(name = "identifier", path = "CarePlan.identifier", description = "External Ids for this plan", type = "token") 5765 public static final String SP_IDENTIFIER = "identifier"; 5766 /** 5767 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5768 * <p> 5769 * Description: <b>External Ids for this plan</b><br> 5770 * Type: <b>token</b><br> 5771 * Path: <b>CarePlan.identifier</b><br> 5772 * </p> 5773 */ 5774 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5775 SP_IDENTIFIER); 5776 5777 /** 5778 * Search parameter: <b>performer</b> 5779 * <p> 5780 * Description: <b>Matches if the practitioner is listed as a performer in any 5781 * of the "simple" activities. (For performers of the detailed activities, chain 5782 * through the activitydetail search parameter.)</b><br> 5783 * Type: <b>reference</b><br> 5784 * Path: <b>CarePlan.activity.detail.performer</b><br> 5785 * </p> 5786 */ 5787 @SearchParamDefinition(name = "performer", path = "CarePlan.activity.detail.performer", description = "Matches if the practitioner is listed as a performer in any of the \"simple\" activities. (For performers of the detailed activities, chain through the activitydetail search parameter.)", type = "reference", providesMembershipIn = { 5788 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 5789 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 5790 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 5791 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 5792 RelatedPerson.class }) 5793 public static final String SP_PERFORMER = "performer"; 5794 /** 5795 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 5796 * <p> 5797 * Description: <b>Matches if the practitioner is listed as a performer in any 5798 * of the "simple" activities. (For performers of the detailed activities, chain 5799 * through the activitydetail search parameter.)</b><br> 5800 * Type: <b>reference</b><br> 5801 * Path: <b>CarePlan.activity.detail.performer</b><br> 5802 * </p> 5803 */ 5804 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5805 SP_PERFORMER); 5806 5807 /** 5808 * Constant for fluent queries to be used to add include statements. Specifies 5809 * the path value of "<b>CarePlan:performer</b>". 5810 */ 5811 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 5812 "CarePlan:performer").toLocked(); 5813 5814 /** 5815 * Search parameter: <b>goal</b> 5816 * <p> 5817 * Description: <b>Desired outcome of plan</b><br> 5818 * Type: <b>reference</b><br> 5819 * Path: <b>CarePlan.goal</b><br> 5820 * </p> 5821 */ 5822 @SearchParamDefinition(name = "goal", path = "CarePlan.goal", description = "Desired outcome of plan", type = "reference", target = { 5823 Goal.class }) 5824 public static final String SP_GOAL = "goal"; 5825 /** 5826 * <b>Fluent Client</b> search parameter constant for <b>goal</b> 5827 * <p> 5828 * Description: <b>Desired outcome of plan</b><br> 5829 * Type: <b>reference</b><br> 5830 * Path: <b>CarePlan.goal</b><br> 5831 * </p> 5832 */ 5833 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GOAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5834 SP_GOAL); 5835 5836 /** 5837 * Constant for fluent queries to be used to add include statements. Specifies 5838 * the path value of "<b>CarePlan:goal</b>". 5839 */ 5840 public static final ca.uhn.fhir.model.api.Include INCLUDE_GOAL = new ca.uhn.fhir.model.api.Include("CarePlan:goal") 5841 .toLocked(); 5842 5843 /** 5844 * Search parameter: <b>subject</b> 5845 * <p> 5846 * Description: <b>Who the care plan is for</b><br> 5847 * Type: <b>reference</b><br> 5848 * Path: <b>CarePlan.subject</b><br> 5849 * </p> 5850 */ 5851 @SearchParamDefinition(name = "subject", path = "CarePlan.subject", description = "Who the care plan is for", type = "reference", target = { 5852 Group.class, Patient.class }) 5853 public static final String SP_SUBJECT = "subject"; 5854 /** 5855 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5856 * <p> 5857 * Description: <b>Who the care plan is for</b><br> 5858 * Type: <b>reference</b><br> 5859 * Path: <b>CarePlan.subject</b><br> 5860 * </p> 5861 */ 5862 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5863 SP_SUBJECT); 5864 5865 /** 5866 * Constant for fluent queries to be used to add include statements. Specifies 5867 * the path value of "<b>CarePlan:subject</b>". 5868 */ 5869 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 5870 "CarePlan:subject").toLocked(); 5871 5872 /** 5873 * Search parameter: <b>replaces</b> 5874 * <p> 5875 * Description: <b>CarePlan replaced by this CarePlan</b><br> 5876 * Type: <b>reference</b><br> 5877 * Path: <b>CarePlan.replaces</b><br> 5878 * </p> 5879 */ 5880 @SearchParamDefinition(name = "replaces", path = "CarePlan.replaces", description = "CarePlan replaced by this CarePlan", type = "reference", target = { 5881 CarePlan.class }) 5882 public static final String SP_REPLACES = "replaces"; 5883 /** 5884 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 5885 * <p> 5886 * Description: <b>CarePlan replaced by this CarePlan</b><br> 5887 * Type: <b>reference</b><br> 5888 * Path: <b>CarePlan.replaces</b><br> 5889 * </p> 5890 */ 5891 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5892 SP_REPLACES); 5893 5894 /** 5895 * Constant for fluent queries to be used to add include statements. Specifies 5896 * the path value of "<b>CarePlan:replaces</b>". 5897 */ 5898 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include( 5899 "CarePlan:replaces").toLocked(); 5900 5901 /** 5902 * Search parameter: <b>instantiates-canonical</b> 5903 * <p> 5904 * Description: <b>Instantiates FHIR protocol or definition</b><br> 5905 * Type: <b>reference</b><br> 5906 * Path: <b>CarePlan.instantiatesCanonical</b><br> 5907 * </p> 5908 */ 5909 @SearchParamDefinition(name = "instantiates-canonical", path = "CarePlan.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 5910 ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class }) 5911 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 5912 /** 5913 * <b>Fluent Client</b> search parameter constant for 5914 * <b>instantiates-canonical</b> 5915 * <p> 5916 * Description: <b>Instantiates FHIR protocol or definition</b><br> 5917 * Type: <b>reference</b><br> 5918 * Path: <b>CarePlan.instantiatesCanonical</b><br> 5919 * </p> 5920 */ 5921 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5922 SP_INSTANTIATES_CANONICAL); 5923 5924 /** 5925 * Constant for fluent queries to be used to add include statements. Specifies 5926 * the path value of "<b>CarePlan:instantiates-canonical</b>". 5927 */ 5928 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 5929 "CarePlan:instantiates-canonical").toLocked(); 5930 5931 /** 5932 * Search parameter: <b>part-of</b> 5933 * <p> 5934 * Description: <b>Part of referenced CarePlan</b><br> 5935 * Type: <b>reference</b><br> 5936 * Path: <b>CarePlan.partOf</b><br> 5937 * </p> 5938 */ 5939 @SearchParamDefinition(name = "part-of", path = "CarePlan.partOf", description = "Part of referenced CarePlan", type = "reference", target = { 5940 CarePlan.class }) 5941 public static final String SP_PART_OF = "part-of"; 5942 /** 5943 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5944 * <p> 5945 * Description: <b>Part of referenced CarePlan</b><br> 5946 * Type: <b>reference</b><br> 5947 * Path: <b>CarePlan.partOf</b><br> 5948 * </p> 5949 */ 5950 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5951 SP_PART_OF); 5952 5953 /** 5954 * Constant for fluent queries to be used to add include statements. Specifies 5955 * the path value of "<b>CarePlan:part-of</b>". 5956 */ 5957 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 5958 "CarePlan:part-of").toLocked(); 5959 5960 /** 5961 * Search parameter: <b>encounter</b> 5962 * <p> 5963 * Description: <b>Encounter created as part of</b><br> 5964 * Type: <b>reference</b><br> 5965 * Path: <b>CarePlan.encounter</b><br> 5966 * </p> 5967 */ 5968 @SearchParamDefinition(name = "encounter", path = "CarePlan.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 5969 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 5970 public static final String SP_ENCOUNTER = "encounter"; 5971 /** 5972 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5973 * <p> 5974 * Description: <b>Encounter created as part of</b><br> 5975 * Type: <b>reference</b><br> 5976 * Path: <b>CarePlan.encounter</b><br> 5977 * </p> 5978 */ 5979 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5980 SP_ENCOUNTER); 5981 5982 /** 5983 * Constant for fluent queries to be used to add include statements. Specifies 5984 * the path value of "<b>CarePlan:encounter</b>". 5985 */ 5986 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5987 "CarePlan:encounter").toLocked(); 5988 5989 /** 5990 * Search parameter: <b>intent</b> 5991 * <p> 5992 * Description: <b>proposal | plan | order | option</b><br> 5993 * Type: <b>token</b><br> 5994 * Path: <b>CarePlan.intent</b><br> 5995 * </p> 5996 */ 5997 @SearchParamDefinition(name = "intent", path = "CarePlan.intent", description = "proposal | plan | order | option", type = "token") 5998 public static final String SP_INTENT = "intent"; 5999 /** 6000 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 6001 * <p> 6002 * Description: <b>proposal | plan | order | option</b><br> 6003 * Type: <b>token</b><br> 6004 * Path: <b>CarePlan.intent</b><br> 6005 * </p> 6006 */ 6007 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6008 SP_INTENT); 6009 6010 /** 6011 * Search parameter: <b>activity-reference</b> 6012 * <p> 6013 * Description: <b>Activity details defined in specific resource</b><br> 6014 * Type: <b>reference</b><br> 6015 * Path: <b>CarePlan.activity.reference</b><br> 6016 * </p> 6017 */ 6018 @SearchParamDefinition(name = "activity-reference", path = "CarePlan.activity.reference", description = "Activity details defined in specific resource", type = "reference", target = { 6019 Appointment.class, CommunicationRequest.class, DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, 6020 RequestGroup.class, ServiceRequest.class, Task.class, VisionPrescription.class }) 6021 public static final String SP_ACTIVITY_REFERENCE = "activity-reference"; 6022 /** 6023 * <b>Fluent Client</b> search parameter constant for <b>activity-reference</b> 6024 * <p> 6025 * Description: <b>Activity details defined in specific resource</b><br> 6026 * Type: <b>reference</b><br> 6027 * Path: <b>CarePlan.activity.reference</b><br> 6028 * </p> 6029 */ 6030 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTIVITY_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6031 SP_ACTIVITY_REFERENCE); 6032 6033 /** 6034 * Constant for fluent queries to be used to add include statements. Specifies 6035 * the path value of "<b>CarePlan:activity-reference</b>". 6036 */ 6037 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTIVITY_REFERENCE = new ca.uhn.fhir.model.api.Include( 6038 "CarePlan:activity-reference").toLocked(); 6039 6040 /** 6041 * Search parameter: <b>condition</b> 6042 * <p> 6043 * Description: <b>Health issues this plan addresses</b><br> 6044 * Type: <b>reference</b><br> 6045 * Path: <b>CarePlan.addresses</b><br> 6046 * </p> 6047 */ 6048 @SearchParamDefinition(name = "condition", path = "CarePlan.addresses", description = "Health issues this plan addresses", type = "reference", target = { 6049 Condition.class }) 6050 public static final String SP_CONDITION = "condition"; 6051 /** 6052 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 6053 * <p> 6054 * Description: <b>Health issues this plan addresses</b><br> 6055 * Type: <b>reference</b><br> 6056 * Path: <b>CarePlan.addresses</b><br> 6057 * </p> 6058 */ 6059 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6060 SP_CONDITION); 6061 6062 /** 6063 * Constant for fluent queries to be used to add include statements. Specifies 6064 * the path value of "<b>CarePlan:condition</b>". 6065 */ 6066 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include( 6067 "CarePlan:condition").toLocked(); 6068 6069 /** 6070 * Search parameter: <b>based-on</b> 6071 * <p> 6072 * Description: <b>Fulfills CarePlan</b><br> 6073 * Type: <b>reference</b><br> 6074 * Path: <b>CarePlan.basedOn</b><br> 6075 * </p> 6076 */ 6077 @SearchParamDefinition(name = "based-on", path = "CarePlan.basedOn", description = "Fulfills CarePlan", type = "reference", target = { 6078 CarePlan.class }) 6079 public static final String SP_BASED_ON = "based-on"; 6080 /** 6081 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 6082 * <p> 6083 * Description: <b>Fulfills CarePlan</b><br> 6084 * Type: <b>reference</b><br> 6085 * Path: <b>CarePlan.basedOn</b><br> 6086 * </p> 6087 */ 6088 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6089 SP_BASED_ON); 6090 6091 /** 6092 * Constant for fluent queries to be used to add include statements. Specifies 6093 * the path value of "<b>CarePlan:based-on</b>". 6094 */ 6095 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 6096 "CarePlan:based-on").toLocked(); 6097 6098 /** 6099 * Search parameter: <b>patient</b> 6100 * <p> 6101 * Description: <b>Who the care plan is for</b><br> 6102 * Type: <b>reference</b><br> 6103 * Path: <b>CarePlan.subject</b><br> 6104 * </p> 6105 */ 6106 @SearchParamDefinition(name = "patient", path = "CarePlan.subject.where(resolve() is Patient)", description = "Who the care plan is for", type = "reference", providesMembershipIn = { 6107 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 6108 public static final String SP_PATIENT = "patient"; 6109 /** 6110 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6111 * <p> 6112 * Description: <b>Who the care plan is for</b><br> 6113 * Type: <b>reference</b><br> 6114 * Path: <b>CarePlan.subject</b><br> 6115 * </p> 6116 */ 6117 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6118 SP_PATIENT); 6119 6120 /** 6121 * Constant for fluent queries to be used to add include statements. Specifies 6122 * the path value of "<b>CarePlan:patient</b>". 6123 */ 6124 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 6125 "CarePlan:patient").toLocked(); 6126 6127 /** 6128 * Search parameter: <b>activity-date</b> 6129 * <p> 6130 * Description: <b>Specified date occurs within period specified by 6131 * CarePlan.activity.detail.scheduled[x]</b><br> 6132 * Type: <b>date</b><br> 6133 * Path: <b>CarePlan.activity.detail.scheduled[x]</b><br> 6134 * </p> 6135 */ 6136 @SearchParamDefinition(name = "activity-date", path = "CarePlan.activity.detail.scheduled", description = "Specified date occurs within period specified by CarePlan.activity.detail.scheduled[x]", type = "date") 6137 public static final String SP_ACTIVITY_DATE = "activity-date"; 6138 /** 6139 * <b>Fluent Client</b> search parameter constant for <b>activity-date</b> 6140 * <p> 6141 * Description: <b>Specified date occurs within period specified by 6142 * CarePlan.activity.detail.scheduled[x]</b><br> 6143 * Type: <b>date</b><br> 6144 * Path: <b>CarePlan.activity.detail.scheduled[x]</b><br> 6145 * </p> 6146 */ 6147 public static final ca.uhn.fhir.rest.gclient.DateClientParam ACTIVITY_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6148 SP_ACTIVITY_DATE); 6149 6150 /** 6151 * Search parameter: <b>instantiates-uri</b> 6152 * <p> 6153 * Description: <b>Instantiates external protocol or definition</b><br> 6154 * Type: <b>uri</b><br> 6155 * Path: <b>CarePlan.instantiatesUri</b><br> 6156 * </p> 6157 */ 6158 @SearchParamDefinition(name = "instantiates-uri", path = "CarePlan.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 6159 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 6160 /** 6161 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 6162 * <p> 6163 * Description: <b>Instantiates external protocol or definition</b><br> 6164 * Type: <b>uri</b><br> 6165 * Path: <b>CarePlan.instantiatesUri</b><br> 6166 * </p> 6167 */ 6168 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 6169 SP_INSTANTIATES_URI); 6170 6171 /** 6172 * Search parameter: <b>category</b> 6173 * <p> 6174 * Description: <b>Type of plan</b><br> 6175 * Type: <b>token</b><br> 6176 * Path: <b>CarePlan.category</b><br> 6177 * </p> 6178 */ 6179 @SearchParamDefinition(name = "category", path = "CarePlan.category", description = "Type of plan", type = "token") 6180 public static final String SP_CATEGORY = "category"; 6181 /** 6182 * <b>Fluent Client</b> search parameter constant for <b>category</b> 6183 * <p> 6184 * Description: <b>Type of plan</b><br> 6185 * Type: <b>token</b><br> 6186 * Path: <b>CarePlan.category</b><br> 6187 * </p> 6188 */ 6189 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6190 SP_CATEGORY); 6191 6192 /** 6193 * Search parameter: <b>activity-code</b> 6194 * <p> 6195 * Description: <b>Detail type of activity</b><br> 6196 * Type: <b>token</b><br> 6197 * Path: <b>CarePlan.activity.detail.code</b><br> 6198 * </p> 6199 */ 6200 @SearchParamDefinition(name = "activity-code", path = "CarePlan.activity.detail.code", description = "Detail type of activity", type = "token") 6201 public static final String SP_ACTIVITY_CODE = "activity-code"; 6202 /** 6203 * <b>Fluent Client</b> search parameter constant for <b>activity-code</b> 6204 * <p> 6205 * Description: <b>Detail type of activity</b><br> 6206 * Type: <b>token</b><br> 6207 * Path: <b>CarePlan.activity.detail.code</b><br> 6208 * </p> 6209 */ 6210 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVITY_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6211 SP_ACTIVITY_CODE); 6212 6213 /** 6214 * Search parameter: <b>status</b> 6215 * <p> 6216 * Description: <b>draft | active | on-hold | revoked | completed | 6217 * entered-in-error | unknown</b><br> 6218 * Type: <b>token</b><br> 6219 * Path: <b>CarePlan.status</b><br> 6220 * </p> 6221 */ 6222 @SearchParamDefinition(name = "status", path = "CarePlan.status", description = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", type = "token") 6223 public static final String SP_STATUS = "status"; 6224 /** 6225 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6226 * <p> 6227 * Description: <b>draft | active | on-hold | revoked | completed | 6228 * entered-in-error | unknown</b><br> 6229 * Type: <b>token</b><br> 6230 * Path: <b>CarePlan.status</b><br> 6231 * </p> 6232 */ 6233 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6234 SP_STATUS); 6235 6236}