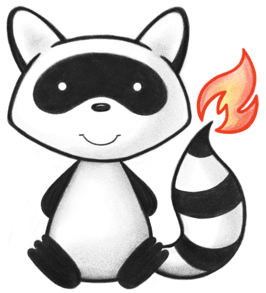
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes the intention of how one or more practitioners intend to deliver 049 * care for a particular patient, group or community for a period of time, 050 * possibly limited to care for a specific condition or set of conditions. 051 */ 052@ResourceDef(name = "CarePlan", profile = "http://hl7.org/fhir/StructureDefinition/CarePlan") 053public class CarePlan extends DomainResource { 054 055 public enum CarePlanStatus { 056 /** 057 * The request has been created but is not yet complete or ready for action. 058 */ 059 DRAFT, 060 /** 061 * The request is in force and ready to be acted upon. 062 */ 063 ACTIVE, 064 /** 065 * The request (and any implicit authorization to act) has been temporarily 066 * withdrawn but is expected to resume in the future. 067 */ 068 ONHOLD, 069 /** 070 * The request (and any implicit authorization to act) has been terminated prior 071 * to the known full completion of the intended actions. No further activity 072 * should occur. 073 */ 074 REVOKED, 075 /** 076 * The activity described by the request has been fully performed. No further 077 * activity will occur. 078 */ 079 COMPLETED, 080 /** 081 * This request should never have existed and should be considered 'void'. (It 082 * is possible that real-world decisions were based on it. If real-world 083 * activity has occurred, the status should be "revoked" rather than 084 * "entered-in-error".). 085 */ 086 ENTEREDINERROR, 087 /** 088 * The authoring/source system does not know which of the status values 089 * currently applies for this request. Note: This concept is not to be used for 090 * "other" - one of the listed statuses is presumed to apply, but the 091 * authoring/source system does not know which. 092 */ 093 UNKNOWN, 094 /** 095 * added to help the parsers with the generic types 096 */ 097 NULL; 098 099 public static CarePlanStatus fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("draft".equals(codeString)) 103 return DRAFT; 104 if ("active".equals(codeString)) 105 return ACTIVE; 106 if ("on-hold".equals(codeString)) 107 return ONHOLD; 108 if ("revoked".equals(codeString)) 109 return REVOKED; 110 if ("completed".equals(codeString)) 111 return COMPLETED; 112 if ("entered-in-error".equals(codeString)) 113 return ENTEREDINERROR; 114 if ("unknown".equals(codeString)) 115 return UNKNOWN; 116 if (Configuration.isAcceptInvalidEnums()) 117 return null; 118 else 119 throw new FHIRException("Unknown CarePlanStatus code '" + codeString + "'"); 120 } 121 122 public String toCode() { 123 switch (this) { 124 case DRAFT: 125 return "draft"; 126 case ACTIVE: 127 return "active"; 128 case ONHOLD: 129 return "on-hold"; 130 case REVOKED: 131 return "revoked"; 132 case COMPLETED: 133 return "completed"; 134 case ENTEREDINERROR: 135 return "entered-in-error"; 136 case UNKNOWN: 137 return "unknown"; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getSystem() { 146 switch (this) { 147 case DRAFT: 148 return "http://hl7.org/fhir/request-status"; 149 case ACTIVE: 150 return "http://hl7.org/fhir/request-status"; 151 case ONHOLD: 152 return "http://hl7.org/fhir/request-status"; 153 case REVOKED: 154 return "http://hl7.org/fhir/request-status"; 155 case COMPLETED: 156 return "http://hl7.org/fhir/request-status"; 157 case ENTEREDINERROR: 158 return "http://hl7.org/fhir/request-status"; 159 case UNKNOWN: 160 return "http://hl7.org/fhir/request-status"; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDefinition() { 169 switch (this) { 170 case DRAFT: 171 return "The request has been created but is not yet complete or ready for action."; 172 case ACTIVE: 173 return "The request is in force and ready to be acted upon."; 174 case ONHOLD: 175 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 176 case REVOKED: 177 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 178 case COMPLETED: 179 return "The activity described by the request has been fully performed. No further activity will occur."; 180 case ENTEREDINERROR: 181 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 182 case UNKNOWN: 183 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case DRAFT: 194 return "Draft"; 195 case ACTIVE: 196 return "Active"; 197 case ONHOLD: 198 return "On Hold"; 199 case REVOKED: 200 return "Revoked"; 201 case COMPLETED: 202 return "Completed"; 203 case ENTEREDINERROR: 204 return "Entered in Error"; 205 case UNKNOWN: 206 return "Unknown"; 207 case NULL: 208 return null; 209 default: 210 return "?"; 211 } 212 } 213 } 214 215 public static class CarePlanStatusEnumFactory implements EnumFactory<CarePlanStatus> { 216 public CarePlanStatus fromCode(String codeString) throws IllegalArgumentException { 217 if (codeString == null || "".equals(codeString)) 218 if (codeString == null || "".equals(codeString)) 219 return null; 220 if ("draft".equals(codeString)) 221 return CarePlanStatus.DRAFT; 222 if ("active".equals(codeString)) 223 return CarePlanStatus.ACTIVE; 224 if ("on-hold".equals(codeString)) 225 return CarePlanStatus.ONHOLD; 226 if ("revoked".equals(codeString)) 227 return CarePlanStatus.REVOKED; 228 if ("completed".equals(codeString)) 229 return CarePlanStatus.COMPLETED; 230 if ("entered-in-error".equals(codeString)) 231 return CarePlanStatus.ENTEREDINERROR; 232 if ("unknown".equals(codeString)) 233 return CarePlanStatus.UNKNOWN; 234 throw new IllegalArgumentException("Unknown CarePlanStatus code '" + codeString + "'"); 235 } 236 237 public Enumeration<CarePlanStatus> fromType(PrimitiveType<?> code) throws FHIRException { 238 if (code == null) 239 return null; 240 if (code.isEmpty()) 241 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.NULL, code); 242 String codeString = code.asStringValue(); 243 if (codeString == null || "".equals(codeString)) 244 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.NULL, code); 245 if ("draft".equals(codeString)) 246 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.DRAFT, code); 247 if ("active".equals(codeString)) 248 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ACTIVE, code); 249 if ("on-hold".equals(codeString)) 250 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ONHOLD, code); 251 if ("revoked".equals(codeString)) 252 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.REVOKED, code); 253 if ("completed".equals(codeString)) 254 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.COMPLETED, code); 255 if ("entered-in-error".equals(codeString)) 256 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ENTEREDINERROR, code); 257 if ("unknown".equals(codeString)) 258 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.UNKNOWN, code); 259 throw new FHIRException("Unknown CarePlanStatus code '" + codeString + "'"); 260 } 261 262 public String toCode(CarePlanStatus code) { 263 if (code == CarePlanStatus.NULL) 264 return null; 265 if (code == CarePlanStatus.DRAFT) 266 return "draft"; 267 if (code == CarePlanStatus.ACTIVE) 268 return "active"; 269 if (code == CarePlanStatus.ONHOLD) 270 return "on-hold"; 271 if (code == CarePlanStatus.REVOKED) 272 return "revoked"; 273 if (code == CarePlanStatus.COMPLETED) 274 return "completed"; 275 if (code == CarePlanStatus.ENTEREDINERROR) 276 return "entered-in-error"; 277 if (code == CarePlanStatus.UNKNOWN) 278 return "unknown"; 279 return "?"; 280 } 281 282 public String toSystem(CarePlanStatus code) { 283 return code.getSystem(); 284 } 285 } 286 287 public enum CarePlanIntent { 288 /** 289 * null 290 */ 291 PROPOSAL, 292 /** 293 * null 294 */ 295 PLAN, 296 /** 297 * null 298 */ 299 ORDER, 300 /** 301 * null 302 */ 303 OPTION, 304 /** 305 * added to help the parsers with the generic types 306 */ 307 NULL; 308 309 public static CarePlanIntent fromCode(String codeString) throws FHIRException { 310 if (codeString == null || "".equals(codeString)) 311 return null; 312 if ("proposal".equals(codeString)) 313 return PROPOSAL; 314 if ("plan".equals(codeString)) 315 return PLAN; 316 if ("order".equals(codeString)) 317 return ORDER; 318 if ("option".equals(codeString)) 319 return OPTION; 320 if (Configuration.isAcceptInvalidEnums()) 321 return null; 322 else 323 throw new FHIRException("Unknown CarePlanIntent code '" + codeString + "'"); 324 } 325 326 public String toCode() { 327 switch (this) { 328 case PROPOSAL: 329 return "proposal"; 330 case PLAN: 331 return "plan"; 332 case ORDER: 333 return "order"; 334 case OPTION: 335 return "option"; 336 case NULL: 337 return null; 338 default: 339 return "?"; 340 } 341 } 342 343 public String getSystem() { 344 switch (this) { 345 case PROPOSAL: 346 return "http://hl7.org/fhir/request-intent"; 347 case PLAN: 348 return "http://hl7.org/fhir/request-intent"; 349 case ORDER: 350 return "http://hl7.org/fhir/request-intent"; 351 case OPTION: 352 return "http://hl7.org/fhir/request-intent"; 353 case NULL: 354 return null; 355 default: 356 return "?"; 357 } 358 } 359 360 public String getDefinition() { 361 switch (this) { 362 case PROPOSAL: 363 return ""; 364 case PLAN: 365 return ""; 366 case ORDER: 367 return ""; 368 case OPTION: 369 return ""; 370 case NULL: 371 return null; 372 default: 373 return "?"; 374 } 375 } 376 377 public String getDisplay() { 378 switch (this) { 379 case PROPOSAL: 380 return "proposal"; 381 case PLAN: 382 return "plan"; 383 case ORDER: 384 return "order"; 385 case OPTION: 386 return "option"; 387 case NULL: 388 return null; 389 default: 390 return "?"; 391 } 392 } 393 } 394 395 public static class CarePlanIntentEnumFactory implements EnumFactory<CarePlanIntent> { 396 public CarePlanIntent fromCode(String codeString) throws IllegalArgumentException { 397 if (codeString == null || "".equals(codeString)) 398 if (codeString == null || "".equals(codeString)) 399 return null; 400 if ("proposal".equals(codeString)) 401 return CarePlanIntent.PROPOSAL; 402 if ("plan".equals(codeString)) 403 return CarePlanIntent.PLAN; 404 if ("order".equals(codeString)) 405 return CarePlanIntent.ORDER; 406 if ("option".equals(codeString)) 407 return CarePlanIntent.OPTION; 408 throw new IllegalArgumentException("Unknown CarePlanIntent code '" + codeString + "'"); 409 } 410 411 public Enumeration<CarePlanIntent> fromType(PrimitiveType<?> code) throws FHIRException { 412 if (code == null) 413 return null; 414 if (code.isEmpty()) 415 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.NULL, code); 416 String codeString = code.asStringValue(); 417 if (codeString == null || "".equals(codeString)) 418 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.NULL, code); 419 if ("proposal".equals(codeString)) 420 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PROPOSAL, code); 421 if ("plan".equals(codeString)) 422 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PLAN, code); 423 if ("order".equals(codeString)) 424 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.ORDER, code); 425 if ("option".equals(codeString)) 426 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.OPTION, code); 427 throw new FHIRException("Unknown CarePlanIntent code '" + codeString + "'"); 428 } 429 430 public String toCode(CarePlanIntent code) { 431 if (code == CarePlanIntent.NULL) 432 return null; 433 if (code == CarePlanIntent.PROPOSAL) 434 return "proposal"; 435 if (code == CarePlanIntent.PLAN) 436 return "plan"; 437 if (code == CarePlanIntent.ORDER) 438 return "order"; 439 if (code == CarePlanIntent.OPTION) 440 return "option"; 441 return "?"; 442 } 443 444 public String toSystem(CarePlanIntent code) { 445 return code.getSystem(); 446 } 447 } 448 449 public enum CarePlanActivityKind { 450 /** 451 * null 452 */ 453 APPOINTMENT, 454 /** 455 * null 456 */ 457 COMMUNICATIONREQUEST, 458 /** 459 * null 460 */ 461 DEVICEREQUEST, 462 /** 463 * null 464 */ 465 MEDICATIONREQUEST, 466 /** 467 * null 468 */ 469 NUTRITIONORDER, 470 /** 471 * null 472 */ 473 TASK, 474 /** 475 * null 476 */ 477 SERVICEREQUEST, 478 /** 479 * null 480 */ 481 VISIONPRESCRIPTION, 482 /** 483 * added to help the parsers with the generic types 484 */ 485 NULL; 486 487 public static CarePlanActivityKind fromCode(String codeString) throws FHIRException { 488 if (codeString == null || "".equals(codeString)) 489 return null; 490 if ("Appointment".equals(codeString)) 491 return APPOINTMENT; 492 if ("CommunicationRequest".equals(codeString)) 493 return COMMUNICATIONREQUEST; 494 if ("DeviceRequest".equals(codeString)) 495 return DEVICEREQUEST; 496 if ("MedicationRequest".equals(codeString)) 497 return MEDICATIONREQUEST; 498 if ("NutritionOrder".equals(codeString)) 499 return NUTRITIONORDER; 500 if ("Task".equals(codeString)) 501 return TASK; 502 if ("ServiceRequest".equals(codeString)) 503 return SERVICEREQUEST; 504 if ("VisionPrescription".equals(codeString)) 505 return VISIONPRESCRIPTION; 506 if (Configuration.isAcceptInvalidEnums()) 507 return null; 508 else 509 throw new FHIRException("Unknown CarePlanActivityKind code '" + codeString + "'"); 510 } 511 512 public String toCode() { 513 switch (this) { 514 case APPOINTMENT: 515 return "Appointment"; 516 case COMMUNICATIONREQUEST: 517 return "CommunicationRequest"; 518 case DEVICEREQUEST: 519 return "DeviceRequest"; 520 case MEDICATIONREQUEST: 521 return "MedicationRequest"; 522 case NUTRITIONORDER: 523 return "NutritionOrder"; 524 case TASK: 525 return "Task"; 526 case SERVICEREQUEST: 527 return "ServiceRequest"; 528 case VISIONPRESCRIPTION: 529 return "VisionPrescription"; 530 case NULL: 531 return null; 532 default: 533 return "?"; 534 } 535 } 536 537 public String getSystem() { 538 switch (this) { 539 case APPOINTMENT: 540 return "http://hl7.org/fhir/resource-types"; 541 case COMMUNICATIONREQUEST: 542 return "http://hl7.org/fhir/resource-types"; 543 case DEVICEREQUEST: 544 return "http://hl7.org/fhir/resource-types"; 545 case MEDICATIONREQUEST: 546 return "http://hl7.org/fhir/resource-types"; 547 case NUTRITIONORDER: 548 return "http://hl7.org/fhir/resource-types"; 549 case TASK: 550 return "http://hl7.org/fhir/resource-types"; 551 case SERVICEREQUEST: 552 return "http://hl7.org/fhir/resource-types"; 553 case VISIONPRESCRIPTION: 554 return "http://hl7.org/fhir/resource-types"; 555 case NULL: 556 return null; 557 default: 558 return "?"; 559 } 560 } 561 562 public String getDefinition() { 563 switch (this) { 564 case APPOINTMENT: 565 return ""; 566 case COMMUNICATIONREQUEST: 567 return ""; 568 case DEVICEREQUEST: 569 return ""; 570 case MEDICATIONREQUEST: 571 return ""; 572 case NUTRITIONORDER: 573 return ""; 574 case TASK: 575 return ""; 576 case SERVICEREQUEST: 577 return ""; 578 case VISIONPRESCRIPTION: 579 return ""; 580 case NULL: 581 return null; 582 default: 583 return "?"; 584 } 585 } 586 587 public String getDisplay() { 588 switch (this) { 589 case APPOINTMENT: 590 return "Appointment"; 591 case COMMUNICATIONREQUEST: 592 return "CommunicationRequest"; 593 case DEVICEREQUEST: 594 return "DeviceRequest"; 595 case MEDICATIONREQUEST: 596 return "MedicationRequest"; 597 case NUTRITIONORDER: 598 return "NutritionOrder"; 599 case TASK: 600 return "Task"; 601 case SERVICEREQUEST: 602 return "ServiceRequest"; 603 case VISIONPRESCRIPTION: 604 return "VisionPrescription"; 605 case NULL: 606 return null; 607 default: 608 return "?"; 609 } 610 } 611 } 612 613 public static class CarePlanActivityKindEnumFactory implements EnumFactory<CarePlanActivityKind> { 614 public CarePlanActivityKind fromCode(String codeString) throws IllegalArgumentException { 615 if (codeString == null || "".equals(codeString)) 616 if (codeString == null || "".equals(codeString)) 617 return null; 618 if ("Appointment".equals(codeString)) 619 return CarePlanActivityKind.APPOINTMENT; 620 if ("CommunicationRequest".equals(codeString)) 621 return CarePlanActivityKind.COMMUNICATIONREQUEST; 622 if ("DeviceRequest".equals(codeString)) 623 return CarePlanActivityKind.DEVICEREQUEST; 624 if ("MedicationRequest".equals(codeString)) 625 return CarePlanActivityKind.MEDICATIONREQUEST; 626 if ("NutritionOrder".equals(codeString)) 627 return CarePlanActivityKind.NUTRITIONORDER; 628 if ("Task".equals(codeString)) 629 return CarePlanActivityKind.TASK; 630 if ("ServiceRequest".equals(codeString)) 631 return CarePlanActivityKind.SERVICEREQUEST; 632 if ("VisionPrescription".equals(codeString)) 633 return CarePlanActivityKind.VISIONPRESCRIPTION; 634 throw new IllegalArgumentException("Unknown CarePlanActivityKind code '" + codeString + "'"); 635 } 636 637 public Enumeration<CarePlanActivityKind> fromType(PrimitiveType<?> code) throws FHIRException { 638 if (code == null) 639 return null; 640 if (code.isEmpty()) 641 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.NULL, code); 642 String codeString = code.asStringValue(); 643 if (codeString == null || "".equals(codeString)) 644 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.NULL, code); 645 if ("Appointment".equals(codeString)) 646 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.APPOINTMENT, code); 647 if ("CommunicationRequest".equals(codeString)) 648 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.COMMUNICATIONREQUEST, code); 649 if ("DeviceRequest".equals(codeString)) 650 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.DEVICEREQUEST, code); 651 if ("MedicationRequest".equals(codeString)) 652 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.MEDICATIONREQUEST, code); 653 if ("NutritionOrder".equals(codeString)) 654 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.NUTRITIONORDER, code); 655 if ("Task".equals(codeString)) 656 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.TASK, code); 657 if ("ServiceRequest".equals(codeString)) 658 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.SERVICEREQUEST, code); 659 if ("VisionPrescription".equals(codeString)) 660 return new Enumeration<CarePlanActivityKind>(this, CarePlanActivityKind.VISIONPRESCRIPTION, code); 661 throw new FHIRException("Unknown CarePlanActivityKind code '" + codeString + "'"); 662 } 663 664 public String toCode(CarePlanActivityKind code) { 665 if (code == CarePlanActivityKind.NULL) 666 return null; 667 if (code == CarePlanActivityKind.APPOINTMENT) 668 return "Appointment"; 669 if (code == CarePlanActivityKind.COMMUNICATIONREQUEST) 670 return "CommunicationRequest"; 671 if (code == CarePlanActivityKind.DEVICEREQUEST) 672 return "DeviceRequest"; 673 if (code == CarePlanActivityKind.MEDICATIONREQUEST) 674 return "MedicationRequest"; 675 if (code == CarePlanActivityKind.NUTRITIONORDER) 676 return "NutritionOrder"; 677 if (code == CarePlanActivityKind.TASK) 678 return "Task"; 679 if (code == CarePlanActivityKind.SERVICEREQUEST) 680 return "ServiceRequest"; 681 if (code == CarePlanActivityKind.VISIONPRESCRIPTION) 682 return "VisionPrescription"; 683 return "?"; 684 } 685 686 public String toSystem(CarePlanActivityKind code) { 687 return code.getSystem(); 688 } 689 } 690 691 public enum CarePlanActivityStatus { 692 /** 693 * Care plan activity is planned but no action has yet been taken. 694 */ 695 NOTSTARTED, 696 /** 697 * Appointment or other booking has occurred but activity has not yet begun. 698 */ 699 SCHEDULED, 700 /** 701 * Care plan activity has been started but is not yet complete. 702 */ 703 INPROGRESS, 704 /** 705 * Care plan activity was started but has temporarily ceased with an expectation 706 * of resumption at a future time. 707 */ 708 ONHOLD, 709 /** 710 * Care plan activity has been completed (more or less) as planned. 711 */ 712 COMPLETED, 713 /** 714 * The planned care plan activity has been withdrawn. 715 */ 716 CANCELLED, 717 /** 718 * The planned care plan activity has been ended prior to completion after the 719 * activity was started. 720 */ 721 STOPPED, 722 /** 723 * The current state of the care plan activity is not known. Note: This concept 724 * is not to be used for "other" - one of the listed statuses is presumed to 725 * apply, but the authoring/source system does not know which one. 726 */ 727 UNKNOWN, 728 /** 729 * Care plan activity was entered in error and voided. 730 */ 731 ENTEREDINERROR, 732 /** 733 * added to help the parsers with the generic types 734 */ 735 NULL; 736 737 public static CarePlanActivityStatus fromCode(String codeString) throws FHIRException { 738 if (codeString == null || "".equals(codeString)) 739 return null; 740 if ("not-started".equals(codeString)) 741 return NOTSTARTED; 742 if ("scheduled".equals(codeString)) 743 return SCHEDULED; 744 if ("in-progress".equals(codeString)) 745 return INPROGRESS; 746 if ("on-hold".equals(codeString)) 747 return ONHOLD; 748 if ("completed".equals(codeString)) 749 return COMPLETED; 750 if ("cancelled".equals(codeString)) 751 return CANCELLED; 752 if ("stopped".equals(codeString)) 753 return STOPPED; 754 if ("unknown".equals(codeString)) 755 return UNKNOWN; 756 if ("entered-in-error".equals(codeString)) 757 return ENTEREDINERROR; 758 if (Configuration.isAcceptInvalidEnums()) 759 return null; 760 else 761 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 762 } 763 764 public String toCode() { 765 switch (this) { 766 case NOTSTARTED: 767 return "not-started"; 768 case SCHEDULED: 769 return "scheduled"; 770 case INPROGRESS: 771 return "in-progress"; 772 case ONHOLD: 773 return "on-hold"; 774 case COMPLETED: 775 return "completed"; 776 case CANCELLED: 777 return "cancelled"; 778 case STOPPED: 779 return "stopped"; 780 case UNKNOWN: 781 return "unknown"; 782 case ENTEREDINERROR: 783 return "entered-in-error"; 784 case NULL: 785 return null; 786 default: 787 return "?"; 788 } 789 } 790 791 public String getSystem() { 792 switch (this) { 793 case NOTSTARTED: 794 return "http://hl7.org/fhir/care-plan-activity-status"; 795 case SCHEDULED: 796 return "http://hl7.org/fhir/care-plan-activity-status"; 797 case INPROGRESS: 798 return "http://hl7.org/fhir/care-plan-activity-status"; 799 case ONHOLD: 800 return "http://hl7.org/fhir/care-plan-activity-status"; 801 case COMPLETED: 802 return "http://hl7.org/fhir/care-plan-activity-status"; 803 case CANCELLED: 804 return "http://hl7.org/fhir/care-plan-activity-status"; 805 case STOPPED: 806 return "http://hl7.org/fhir/care-plan-activity-status"; 807 case UNKNOWN: 808 return "http://hl7.org/fhir/care-plan-activity-status"; 809 case ENTEREDINERROR: 810 return "http://hl7.org/fhir/care-plan-activity-status"; 811 case NULL: 812 return null; 813 default: 814 return "?"; 815 } 816 } 817 818 public String getDefinition() { 819 switch (this) { 820 case NOTSTARTED: 821 return "Care plan activity is planned but no action has yet been taken."; 822 case SCHEDULED: 823 return "Appointment or other booking has occurred but activity has not yet begun."; 824 case INPROGRESS: 825 return "Care plan activity has been started but is not yet complete."; 826 case ONHOLD: 827 return "Care plan activity was started but has temporarily ceased with an expectation of resumption at a future time."; 828 case COMPLETED: 829 return "Care plan activity has been completed (more or less) as planned."; 830 case CANCELLED: 831 return "The planned care plan activity has been withdrawn."; 832 case STOPPED: 833 return "The planned care plan activity has been ended prior to completion after the activity was started."; 834 case UNKNOWN: 835 return "The current state of the care plan activity is not known. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which one."; 836 case ENTEREDINERROR: 837 return "Care plan activity was entered in error and voided."; 838 case NULL: 839 return null; 840 default: 841 return "?"; 842 } 843 } 844 845 public String getDisplay() { 846 switch (this) { 847 case NOTSTARTED: 848 return "Not Started"; 849 case SCHEDULED: 850 return "Scheduled"; 851 case INPROGRESS: 852 return "In Progress"; 853 case ONHOLD: 854 return "On Hold"; 855 case COMPLETED: 856 return "Completed"; 857 case CANCELLED: 858 return "Cancelled"; 859 case STOPPED: 860 return "Stopped"; 861 case UNKNOWN: 862 return "Unknown"; 863 case ENTEREDINERROR: 864 return "Entered in Error"; 865 case NULL: 866 return null; 867 default: 868 return "?"; 869 } 870 } 871 } 872 873 public static class CarePlanActivityStatusEnumFactory implements EnumFactory<CarePlanActivityStatus> { 874 public CarePlanActivityStatus fromCode(String codeString) throws IllegalArgumentException { 875 if (codeString == null || "".equals(codeString)) 876 if (codeString == null || "".equals(codeString)) 877 return null; 878 if ("not-started".equals(codeString)) 879 return CarePlanActivityStatus.NOTSTARTED; 880 if ("scheduled".equals(codeString)) 881 return CarePlanActivityStatus.SCHEDULED; 882 if ("in-progress".equals(codeString)) 883 return CarePlanActivityStatus.INPROGRESS; 884 if ("on-hold".equals(codeString)) 885 return CarePlanActivityStatus.ONHOLD; 886 if ("completed".equals(codeString)) 887 return CarePlanActivityStatus.COMPLETED; 888 if ("cancelled".equals(codeString)) 889 return CarePlanActivityStatus.CANCELLED; 890 if ("stopped".equals(codeString)) 891 return CarePlanActivityStatus.STOPPED; 892 if ("unknown".equals(codeString)) 893 return CarePlanActivityStatus.UNKNOWN; 894 if ("entered-in-error".equals(codeString)) 895 return CarePlanActivityStatus.ENTEREDINERROR; 896 throw new IllegalArgumentException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 897 } 898 899 public Enumeration<CarePlanActivityStatus> fromType(PrimitiveType<?> code) throws FHIRException { 900 if (code == null) 901 return null; 902 if (code.isEmpty()) 903 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NULL, code); 904 String codeString = code.asStringValue(); 905 if (codeString == null || "".equals(codeString)) 906 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NULL, code); 907 if ("not-started".equals(codeString)) 908 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NOTSTARTED, code); 909 if ("scheduled".equals(codeString)) 910 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.SCHEDULED, code); 911 if ("in-progress".equals(codeString)) 912 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.INPROGRESS, code); 913 if ("on-hold".equals(codeString)) 914 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.ONHOLD, code); 915 if ("completed".equals(codeString)) 916 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.COMPLETED, code); 917 if ("cancelled".equals(codeString)) 918 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.CANCELLED, code); 919 if ("stopped".equals(codeString)) 920 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.STOPPED, code); 921 if ("unknown".equals(codeString)) 922 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.UNKNOWN, code); 923 if ("entered-in-error".equals(codeString)) 924 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.ENTEREDINERROR, code); 925 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 926 } 927 928 public String toCode(CarePlanActivityStatus code) { 929 if (code == CarePlanActivityStatus.NULL) 930 return null; 931 if (code == CarePlanActivityStatus.NOTSTARTED) 932 return "not-started"; 933 if (code == CarePlanActivityStatus.SCHEDULED) 934 return "scheduled"; 935 if (code == CarePlanActivityStatus.INPROGRESS) 936 return "in-progress"; 937 if (code == CarePlanActivityStatus.ONHOLD) 938 return "on-hold"; 939 if (code == CarePlanActivityStatus.COMPLETED) 940 return "completed"; 941 if (code == CarePlanActivityStatus.CANCELLED) 942 return "cancelled"; 943 if (code == CarePlanActivityStatus.STOPPED) 944 return "stopped"; 945 if (code == CarePlanActivityStatus.UNKNOWN) 946 return "unknown"; 947 if (code == CarePlanActivityStatus.ENTEREDINERROR) 948 return "entered-in-error"; 949 return "?"; 950 } 951 952 public String toSystem(CarePlanActivityStatus code) { 953 return code.getSystem(); 954 } 955 } 956 957 @Block() 958 public static class CarePlanActivityComponent extends BackboneElement implements IBaseBackboneElement { 959 /** 960 * Identifies the outcome at the point when the status of the activity is 961 * assessed. For example, the outcome of an education activity could be patient 962 * understands (or not). 963 */ 964 @Child(name = "outcomeCodeableConcept", type = { 965 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 966 @Description(shortDefinition = "Results of the activity", formalDefinition = "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).") 967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-activity-outcome") 968 protected List<CodeableConcept> outcomeCodeableConcept; 969 970 /** 971 * Details of the outcome or action resulting from the activity. The reference 972 * to an "event" resource, such as Procedure or Encounter or Observation, is the 973 * result/outcome of the activity itself. The activity can be conveyed using 974 * CarePlan.activity.detail OR using the CarePlan.activity.reference (a 975 * reference to a ?request? resource). 976 */ 977 @Child(name = "outcomeReference", type = { 978 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 979 @Description(shortDefinition = "Appointment, Encounter, Procedure, etc.", formalDefinition = "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).") 980 protected List<Reference> outcomeReference; 981 /** 982 * The actual objects that are the target of the reference (Details of the 983 * outcome or action resulting from the activity. The reference to an "event" 984 * resource, such as Procedure or Encounter or Observation, is the 985 * result/outcome of the activity itself. The activity can be conveyed using 986 * CarePlan.activity.detail OR using the CarePlan.activity.reference (a 987 * reference to a ?request? resource).) 988 */ 989 protected List<Resource> outcomeReferenceTarget; 990 991 /** 992 * Notes about the adherence/status/progress of the activity. 993 */ 994 @Child(name = "progress", type = { 995 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 996 @Description(shortDefinition = "Comments about the activity status/progress", formalDefinition = "Notes about the adherence/status/progress of the activity.") 997 protected List<Annotation> progress; 998 999 /** 1000 * The details of the proposed activity represented in a specific resource. 1001 */ 1002 @Child(name = "reference", type = { Appointment.class, CommunicationRequest.class, DeviceRequest.class, 1003 MedicationRequest.class, NutritionOrder.class, Task.class, ServiceRequest.class, VisionPrescription.class, 1004 RequestGroup.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1005 @Description(shortDefinition = "Activity details defined in specific resource", formalDefinition = "The details of the proposed activity represented in a specific resource.") 1006 protected Reference reference; 1007 1008 /** 1009 * The actual object that is the target of the reference (The details of the 1010 * proposed activity represented in a specific resource.) 1011 */ 1012 protected Resource referenceTarget; 1013 1014 /** 1015 * A simple summary of a planned activity suitable for a general care plan 1016 * system (e.g. form driven) that doesn't know about specific resources such as 1017 * procedure etc. 1018 */ 1019 @Child(name = "detail", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = false) 1020 @Description(shortDefinition = "In-line definition of activity", formalDefinition = "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.") 1021 protected CarePlanActivityDetailComponent detail; 1022 1023 private static final long serialVersionUID = -609287300L; 1024 1025 /** 1026 * Constructor 1027 */ 1028 public CarePlanActivityComponent() { 1029 super(); 1030 } 1031 1032 /** 1033 * @return {@link #outcomeCodeableConcept} (Identifies the outcome at the point 1034 * when the status of the activity is assessed. For example, the outcome 1035 * of an education activity could be patient understands (or not).) 1036 */ 1037 public List<CodeableConcept> getOutcomeCodeableConcept() { 1038 if (this.outcomeCodeableConcept == null) 1039 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1040 return this.outcomeCodeableConcept; 1041 } 1042 1043 /** 1044 * @return Returns a reference to <code>this</code> for easy method chaining 1045 */ 1046 public CarePlanActivityComponent setOutcomeCodeableConcept(List<CodeableConcept> theOutcomeCodeableConcept) { 1047 this.outcomeCodeableConcept = theOutcomeCodeableConcept; 1048 return this; 1049 } 1050 1051 public boolean hasOutcomeCodeableConcept() { 1052 if (this.outcomeCodeableConcept == null) 1053 return false; 1054 for (CodeableConcept item : this.outcomeCodeableConcept) 1055 if (!item.isEmpty()) 1056 return true; 1057 return false; 1058 } 1059 1060 public CodeableConcept addOutcomeCodeableConcept() { // 3 1061 CodeableConcept t = new CodeableConcept(); 1062 if (this.outcomeCodeableConcept == null) 1063 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1064 this.outcomeCodeableConcept.add(t); 1065 return t; 1066 } 1067 1068 public CarePlanActivityComponent addOutcomeCodeableConcept(CodeableConcept t) { // 3 1069 if (t == null) 1070 return this; 1071 if (this.outcomeCodeableConcept == null) 1072 this.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1073 this.outcomeCodeableConcept.add(t); 1074 return this; 1075 } 1076 1077 /** 1078 * @return The first repetition of repeating field 1079 * {@link #outcomeCodeableConcept}, creating it if it does not already 1080 * exist 1081 */ 1082 public CodeableConcept getOutcomeCodeableConceptFirstRep() { 1083 if (getOutcomeCodeableConcept().isEmpty()) { 1084 addOutcomeCodeableConcept(); 1085 } 1086 return getOutcomeCodeableConcept().get(0); 1087 } 1088 1089 /** 1090 * @return {@link #outcomeReference} (Details of the outcome or action resulting 1091 * from the activity. The reference to an "event" resource, such as 1092 * Procedure or Encounter or Observation, is the result/outcome of the 1093 * activity itself. The activity can be conveyed using 1094 * CarePlan.activity.detail OR using the CarePlan.activity.reference (a 1095 * reference to a ?request? resource).) 1096 */ 1097 public List<Reference> getOutcomeReference() { 1098 if (this.outcomeReference == null) 1099 this.outcomeReference = new ArrayList<Reference>(); 1100 return this.outcomeReference; 1101 } 1102 1103 /** 1104 * @return Returns a reference to <code>this</code> for easy method chaining 1105 */ 1106 public CarePlanActivityComponent setOutcomeReference(List<Reference> theOutcomeReference) { 1107 this.outcomeReference = theOutcomeReference; 1108 return this; 1109 } 1110 1111 public boolean hasOutcomeReference() { 1112 if (this.outcomeReference == null) 1113 return false; 1114 for (Reference item : this.outcomeReference) 1115 if (!item.isEmpty()) 1116 return true; 1117 return false; 1118 } 1119 1120 public Reference addOutcomeReference() { // 3 1121 Reference t = new Reference(); 1122 if (this.outcomeReference == null) 1123 this.outcomeReference = new ArrayList<Reference>(); 1124 this.outcomeReference.add(t); 1125 return t; 1126 } 1127 1128 public CarePlanActivityComponent addOutcomeReference(Reference t) { // 3 1129 if (t == null) 1130 return this; 1131 if (this.outcomeReference == null) 1132 this.outcomeReference = new ArrayList<Reference>(); 1133 this.outcomeReference.add(t); 1134 return this; 1135 } 1136 1137 /** 1138 * @return The first repetition of repeating field {@link #outcomeReference}, 1139 * creating it if it does not already exist 1140 */ 1141 public Reference getOutcomeReferenceFirstRep() { 1142 if (getOutcomeReference().isEmpty()) { 1143 addOutcomeReference(); 1144 } 1145 return getOutcomeReference().get(0); 1146 } 1147 1148 /** 1149 * @deprecated Use Reference#setResource(IBaseResource) instead 1150 */ 1151 @Deprecated 1152 public List<Resource> getOutcomeReferenceTarget() { 1153 if (this.outcomeReferenceTarget == null) 1154 this.outcomeReferenceTarget = new ArrayList<Resource>(); 1155 return this.outcomeReferenceTarget; 1156 } 1157 1158 /** 1159 * @return {@link #progress} (Notes about the adherence/status/progress of the 1160 * activity.) 1161 */ 1162 public List<Annotation> getProgress() { 1163 if (this.progress == null) 1164 this.progress = new ArrayList<Annotation>(); 1165 return this.progress; 1166 } 1167 1168 /** 1169 * @return Returns a reference to <code>this</code> for easy method chaining 1170 */ 1171 public CarePlanActivityComponent setProgress(List<Annotation> theProgress) { 1172 this.progress = theProgress; 1173 return this; 1174 } 1175 1176 public boolean hasProgress() { 1177 if (this.progress == null) 1178 return false; 1179 for (Annotation item : this.progress) 1180 if (!item.isEmpty()) 1181 return true; 1182 return false; 1183 } 1184 1185 public Annotation addProgress() { // 3 1186 Annotation t = new Annotation(); 1187 if (this.progress == null) 1188 this.progress = new ArrayList<Annotation>(); 1189 this.progress.add(t); 1190 return t; 1191 } 1192 1193 public CarePlanActivityComponent addProgress(Annotation t) { // 3 1194 if (t == null) 1195 return this; 1196 if (this.progress == null) 1197 this.progress = new ArrayList<Annotation>(); 1198 this.progress.add(t); 1199 return this; 1200 } 1201 1202 /** 1203 * @return The first repetition of repeating field {@link #progress}, creating 1204 * it if it does not already exist 1205 */ 1206 public Annotation getProgressFirstRep() { 1207 if (getProgress().isEmpty()) { 1208 addProgress(); 1209 } 1210 return getProgress().get(0); 1211 } 1212 1213 /** 1214 * @return {@link #reference} (The details of the proposed activity represented 1215 * in a specific resource.) 1216 */ 1217 public Reference getReference() { 1218 if (this.reference == null) 1219 if (Configuration.errorOnAutoCreate()) 1220 throw new Error("Attempt to auto-create CarePlanActivityComponent.reference"); 1221 else if (Configuration.doAutoCreate()) 1222 this.reference = new Reference(); // cc 1223 return this.reference; 1224 } 1225 1226 public boolean hasReference() { 1227 return this.reference != null && !this.reference.isEmpty(); 1228 } 1229 1230 /** 1231 * @param value {@link #reference} (The details of the proposed activity 1232 * represented in a specific resource.) 1233 */ 1234 public CarePlanActivityComponent setReference(Reference value) { 1235 this.reference = value; 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #reference} The actual object that is the target of the 1241 * reference. The reference library doesn't populate this, but you can 1242 * use it to hold the resource if you resolve it. (The details of the 1243 * proposed activity represented in a specific resource.) 1244 */ 1245 public Resource getReferenceTarget() { 1246 return this.referenceTarget; 1247 } 1248 1249 /** 1250 * @param value {@link #reference} The actual object that is the target of the 1251 * reference. The reference library doesn't use these, but you can 1252 * use it to hold the resource if you resolve it. (The details of 1253 * the proposed activity represented in a specific resource.) 1254 */ 1255 public CarePlanActivityComponent setReferenceTarget(Resource value) { 1256 this.referenceTarget = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #detail} (A simple summary of a planned activity suitable for 1262 * a general care plan system (e.g. form driven) that doesn't know about 1263 * specific resources such as procedure etc.) 1264 */ 1265 public CarePlanActivityDetailComponent getDetail() { 1266 if (this.detail == null) 1267 if (Configuration.errorOnAutoCreate()) 1268 throw new Error("Attempt to auto-create CarePlanActivityComponent.detail"); 1269 else if (Configuration.doAutoCreate()) 1270 this.detail = new CarePlanActivityDetailComponent(); // cc 1271 return this.detail; 1272 } 1273 1274 public boolean hasDetail() { 1275 return this.detail != null && !this.detail.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #detail} (A simple summary of a planned activity suitable 1280 * for a general care plan system (e.g. form driven) that doesn't 1281 * know about specific resources such as procedure etc.) 1282 */ 1283 public CarePlanActivityComponent setDetail(CarePlanActivityDetailComponent value) { 1284 this.detail = value; 1285 return this; 1286 } 1287 1288 protected void listChildren(List<Property> children) { 1289 super.listChildren(children); 1290 children.add(new Property("outcomeCodeableConcept", "CodeableConcept", 1291 "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).", 1292 0, java.lang.Integer.MAX_VALUE, outcomeCodeableConcept)); 1293 children.add(new Property("outcomeReference", "Reference(Any)", 1294 "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).", 1295 0, java.lang.Integer.MAX_VALUE, outcomeReference)); 1296 children.add(new Property("progress", "Annotation", "Notes about the adherence/status/progress of the activity.", 1297 0, java.lang.Integer.MAX_VALUE, progress)); 1298 children.add(new Property("reference", 1299 "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ServiceRequest|VisionPrescription|RequestGroup)", 1300 "The details of the proposed activity represented in a specific resource.", 0, 1, reference)); 1301 children.add(new Property("detail", "", 1302 "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 1303 0, 1, detail)); 1304 } 1305 1306 @Override 1307 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1308 switch (_hash) { 1309 case -511913489: 1310 /* outcomeCodeableConcept */ return new Property("outcomeCodeableConcept", "CodeableConcept", 1311 "Identifies the outcome at the point when the status of the activity is assessed. For example, the outcome of an education activity could be patient understands (or not).", 1312 0, java.lang.Integer.MAX_VALUE, outcomeCodeableConcept); 1313 case -782273511: 1314 /* outcomeReference */ return new Property("outcomeReference", "Reference(Any)", 1315 "Details of the outcome or action resulting from the activity. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, is the result/outcome of the activity itself. The activity can be conveyed using CarePlan.activity.detail OR using the CarePlan.activity.reference (a reference to a ?request? resource).", 1316 0, java.lang.Integer.MAX_VALUE, outcomeReference); 1317 case -1001078227: 1318 /* progress */ return new Property("progress", "Annotation", 1319 "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress); 1320 case -925155509: 1321 /* reference */ return new Property("reference", 1322 "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ServiceRequest|VisionPrescription|RequestGroup)", 1323 "The details of the proposed activity represented in a specific resource.", 0, 1, reference); 1324 case -1335224239: 1325 /* detail */ return new Property("detail", "", 1326 "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 1327 0, 1, detail); 1328 default: 1329 return super.getNamedProperty(_hash, _name, _checkValid); 1330 } 1331 1332 } 1333 1334 @Override 1335 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1336 switch (hash) { 1337 case -511913489: 1338 /* outcomeCodeableConcept */ return this.outcomeCodeableConcept == null ? new Base[0] 1339 : this.outcomeCodeableConcept.toArray(new Base[this.outcomeCodeableConcept.size()]); // CodeableConcept 1340 case -782273511: 1341 /* outcomeReference */ return this.outcomeReference == null ? new Base[0] 1342 : this.outcomeReference.toArray(new Base[this.outcomeReference.size()]); // Reference 1343 case -1001078227: 1344 /* progress */ return this.progress == null ? new Base[0] 1345 : this.progress.toArray(new Base[this.progress.size()]); // Annotation 1346 case -925155509: 1347 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 1348 case -1335224239: 1349 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // CarePlanActivityDetailComponent 1350 default: 1351 return super.getProperty(hash, name, checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1358 switch (hash) { 1359 case -511913489: // outcomeCodeableConcept 1360 this.getOutcomeCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 1361 return value; 1362 case -782273511: // outcomeReference 1363 this.getOutcomeReference().add(castToReference(value)); // Reference 1364 return value; 1365 case -1001078227: // progress 1366 this.getProgress().add(castToAnnotation(value)); // Annotation 1367 return value; 1368 case -925155509: // reference 1369 this.reference = castToReference(value); // Reference 1370 return value; 1371 case -1335224239: // detail 1372 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1373 return value; 1374 default: 1375 return super.setProperty(hash, name, value); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base setProperty(String name, Base value) throws FHIRException { 1382 if (name.equals("outcomeCodeableConcept")) { 1383 this.getOutcomeCodeableConcept().add(castToCodeableConcept(value)); 1384 } else if (name.equals("outcomeReference")) { 1385 this.getOutcomeReference().add(castToReference(value)); 1386 } else if (name.equals("progress")) { 1387 this.getProgress().add(castToAnnotation(value)); 1388 } else if (name.equals("reference")) { 1389 this.reference = castToReference(value); // Reference 1390 } else if (name.equals("detail")) { 1391 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1392 } else 1393 return super.setProperty(name, value); 1394 return value; 1395 } 1396 1397 @Override 1398 public void removeChild(String name, Base value) throws FHIRException { 1399 if (name.equals("outcomeCodeableConcept")) { 1400 this.getOutcomeCodeableConcept().remove(castToCodeableConcept(value)); 1401 } else if (name.equals("outcomeReference")) { 1402 this.getOutcomeReference().remove(castToReference(value)); 1403 } else if (name.equals("progress")) { 1404 this.getProgress().remove(castToAnnotation(value)); 1405 } else if (name.equals("reference")) { 1406 this.reference = null; 1407 } else if (name.equals("detail")) { 1408 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1409 } else 1410 super.removeChild(name, value); 1411 1412 } 1413 1414 @Override 1415 public Base makeProperty(int hash, String name) throws FHIRException { 1416 switch (hash) { 1417 case -511913489: 1418 return addOutcomeCodeableConcept(); 1419 case -782273511: 1420 return addOutcomeReference(); 1421 case -1001078227: 1422 return addProgress(); 1423 case -925155509: 1424 return getReference(); 1425 case -1335224239: 1426 return getDetail(); 1427 default: 1428 return super.makeProperty(hash, name); 1429 } 1430 1431 } 1432 1433 @Override 1434 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1435 switch (hash) { 1436 case -511913489: 1437 /* outcomeCodeableConcept */ return new String[] { "CodeableConcept" }; 1438 case -782273511: 1439 /* outcomeReference */ return new String[] { "Reference" }; 1440 case -1001078227: 1441 /* progress */ return new String[] { "Annotation" }; 1442 case -925155509: 1443 /* reference */ return new String[] { "Reference" }; 1444 case -1335224239: 1445 /* detail */ return new String[] {}; 1446 default: 1447 return super.getTypesForProperty(hash, name); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base addChild(String name) throws FHIRException { 1454 if (name.equals("outcomeCodeableConcept")) { 1455 return addOutcomeCodeableConcept(); 1456 } else if (name.equals("outcomeReference")) { 1457 return addOutcomeReference(); 1458 } else if (name.equals("progress")) { 1459 return addProgress(); 1460 } else if (name.equals("reference")) { 1461 this.reference = new Reference(); 1462 return this.reference; 1463 } else if (name.equals("detail")) { 1464 this.detail = new CarePlanActivityDetailComponent(); 1465 return this.detail; 1466 } else 1467 return super.addChild(name); 1468 } 1469 1470 public CarePlanActivityComponent copy() { 1471 CarePlanActivityComponent dst = new CarePlanActivityComponent(); 1472 copyValues(dst); 1473 return dst; 1474 } 1475 1476 public void copyValues(CarePlanActivityComponent dst) { 1477 super.copyValues(dst); 1478 if (outcomeCodeableConcept != null) { 1479 dst.outcomeCodeableConcept = new ArrayList<CodeableConcept>(); 1480 for (CodeableConcept i : outcomeCodeableConcept) 1481 dst.outcomeCodeableConcept.add(i.copy()); 1482 } 1483 ; 1484 if (outcomeReference != null) { 1485 dst.outcomeReference = new ArrayList<Reference>(); 1486 for (Reference i : outcomeReference) 1487 dst.outcomeReference.add(i.copy()); 1488 } 1489 ; 1490 if (progress != null) { 1491 dst.progress = new ArrayList<Annotation>(); 1492 for (Annotation i : progress) 1493 dst.progress.add(i.copy()); 1494 } 1495 ; 1496 dst.reference = reference == null ? null : reference.copy(); 1497 dst.detail = detail == null ? null : detail.copy(); 1498 } 1499 1500 @Override 1501 public boolean equalsDeep(Base other_) { 1502 if (!super.equalsDeep(other_)) 1503 return false; 1504 if (!(other_ instanceof CarePlanActivityComponent)) 1505 return false; 1506 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 1507 return compareDeep(outcomeCodeableConcept, o.outcomeCodeableConcept, true) 1508 && compareDeep(outcomeReference, o.outcomeReference, true) && compareDeep(progress, o.progress, true) 1509 && compareDeep(reference, o.reference, true) && compareDeep(detail, o.detail, true); 1510 } 1511 1512 @Override 1513 public boolean equalsShallow(Base other_) { 1514 if (!super.equalsShallow(other_)) 1515 return false; 1516 if (!(other_ instanceof CarePlanActivityComponent)) 1517 return false; 1518 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 1519 return true; 1520 } 1521 1522 public boolean isEmpty() { 1523 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(outcomeCodeableConcept, outcomeReference, progress, 1524 reference, detail); 1525 } 1526 1527 public String fhirType() { 1528 return "CarePlan.activity"; 1529 1530 } 1531 1532 } 1533 1534 @Block() 1535 public static class CarePlanActivityDetailComponent extends BackboneElement implements IBaseBackboneElement { 1536 /** 1537 * A description of the kind of resource the in-line definition of a care plan 1538 * activity is representing. The CarePlan.activity.detail is an in-line 1539 * definition when a resource is not referenced using 1540 * CarePlan.activity.reference. For example, a MedicationRequest, a 1541 * ServiceRequest, or a CommunicationRequest. 1542 */ 1543 @Child(name = "kind", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1544 @Description(shortDefinition = "Appointment | CommunicationRequest | DeviceRequest | MedicationRequest | NutritionOrder | Task | ServiceRequest | VisionPrescription", formalDefinition = "A description of the kind of resource the in-line definition of a care plan activity is representing. The CarePlan.activity.detail is an in-line definition when a resource is not referenced using CarePlan.activity.reference. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.") 1545 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-activity-kind") 1546 protected Enumeration<CarePlanActivityKind> kind; 1547 1548 /** 1549 * The URL pointing to a FHIR-defined protocol, guideline, questionnaire or 1550 * other definition that is adhered to in whole or in part by this CarePlan 1551 * activity. 1552 */ 1553 @Child(name = "instantiatesCanonical", type = { 1554 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1555 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.") 1556 protected List<CanonicalType> instantiatesCanonical; 1557 1558 /** 1559 * The URL pointing to an externally maintained protocol, guideline, 1560 * questionnaire or other definition that is adhered to in whole or in part by 1561 * this CarePlan activity. 1562 */ 1563 @Child(name = "instantiatesUri", type = { 1564 UriType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1565 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.") 1566 protected List<UriType> instantiatesUri; 1567 1568 /** 1569 * Detailed description of the type of planned activity; e.g. what lab test, 1570 * what procedure, what kind of encounter. 1571 */ 1572 @Child(name = "code", type = { 1573 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1574 @Description(shortDefinition = "Detail type of activity", formalDefinition = "Detailed description of the type of planned activity; e.g. what lab test, what procedure, what kind of encounter.") 1575 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 1576 protected CodeableConcept code; 1577 1578 /** 1579 * Provides the rationale that drove the inclusion of this particular activity 1580 * as part of the plan or the reason why the activity was prohibited. 1581 */ 1582 @Child(name = "reasonCode", type = { 1583 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1584 @Description(shortDefinition = "Why activity should be done or why activity was prohibited", formalDefinition = "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.") 1585 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 1586 protected List<CodeableConcept> reasonCode; 1587 1588 /** 1589 * Indicates another resource, such as the health condition(s), whose existence 1590 * justifies this request and drove the inclusion of this particular activity as 1591 * part of the plan. 1592 */ 1593 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 1594 DocumentReference.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1595 @Description(shortDefinition = "Why activity is needed", formalDefinition = "Indicates another resource, such as the health condition(s), whose existence justifies this request and drove the inclusion of this particular activity as part of the plan.") 1596 protected List<Reference> reasonReference; 1597 /** 1598 * The actual objects that are the target of the reference (Indicates another 1599 * resource, such as the health condition(s), whose existence justifies this 1600 * request and drove the inclusion of this particular activity as part of the 1601 * plan.) 1602 */ 1603 protected List<Resource> reasonReferenceTarget; 1604 1605 /** 1606 * Internal reference that identifies the goals that this activity is intended 1607 * to contribute towards meeting. 1608 */ 1609 @Child(name = "goal", type = { 1610 Goal.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1611 @Description(shortDefinition = "Goals this activity relates to", formalDefinition = "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.") 1612 protected List<Reference> goal; 1613 /** 1614 * The actual objects that are the target of the reference (Internal reference 1615 * that identifies the goals that this activity is intended to contribute 1616 * towards meeting.) 1617 */ 1618 protected List<Goal> goalTarget; 1619 1620 /** 1621 * Identifies what progress is being made for the specific activity. 1622 */ 1623 @Child(name = "status", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = true, summary = false) 1624 @Description(shortDefinition = "not-started | scheduled | in-progress | on-hold | completed | cancelled | stopped | unknown | entered-in-error", formalDefinition = "Identifies what progress is being made for the specific activity.") 1625 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-activity-status") 1626 protected Enumeration<CarePlanActivityStatus> status; 1627 1628 /** 1629 * Provides reason why the activity isn't yet started, is on hold, was 1630 * cancelled, etc. 1631 */ 1632 @Child(name = "statusReason", type = { 1633 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1634 @Description(shortDefinition = "Reason for current status", formalDefinition = "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.") 1635 protected CodeableConcept statusReason; 1636 1637 /** 1638 * If true, indicates that the described activity is one that must NOT be 1639 * engaged in when following the plan. If false, or missing, indicates that the 1640 * described activity is one that should be engaged in when following the plan. 1641 */ 1642 @Child(name = "doNotPerform", type = { 1643 BooleanType.class }, order = 10, min = 0, max = 1, modifier = true, summary = false) 1644 @Description(shortDefinition = "If true, activity is prohibiting action", formalDefinition = "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, or missing, indicates that the described activity is one that should be engaged in when following the plan.") 1645 protected BooleanType doNotPerform; 1646 1647 /** 1648 * The period, timing or frequency upon which the described activity is to 1649 * occur. 1650 */ 1651 @Child(name = "scheduled", type = { Timing.class, Period.class, 1652 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1653 @Description(shortDefinition = "When activity is to occur", formalDefinition = "The period, timing or frequency upon which the described activity is to occur.") 1654 protected Type scheduled; 1655 1656 /** 1657 * Identifies the facility where the activity will occur; e.g. home, hospital, 1658 * specific clinic, etc. 1659 */ 1660 @Child(name = "location", type = { 1661 Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1662 @Description(shortDefinition = "Where it should happen", formalDefinition = "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.") 1663 protected Reference location; 1664 1665 /** 1666 * The actual object that is the target of the reference (Identifies the 1667 * facility where the activity will occur; e.g. home, hospital, specific clinic, 1668 * etc.) 1669 */ 1670 protected Location locationTarget; 1671 1672 /** 1673 * Identifies who's expected to be involved in the activity. 1674 */ 1675 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, 1676 RelatedPerson.class, Patient.class, CareTeam.class, HealthcareService.class, 1677 Device.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1678 @Description(shortDefinition = "Who will be responsible?", formalDefinition = "Identifies who's expected to be involved in the activity.") 1679 protected List<Reference> performer; 1680 /** 1681 * The actual objects that are the target of the reference (Identifies who's 1682 * expected to be involved in the activity.) 1683 */ 1684 protected List<Resource> performerTarget; 1685 1686 /** 1687 * Identifies the food, drug or other product to be consumed or supplied in the 1688 * activity. 1689 */ 1690 @Child(name = "product", type = { CodeableConcept.class, Medication.class, 1691 Substance.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1692 @Description(shortDefinition = "What is to be administered/supplied", formalDefinition = "Identifies the food, drug or other product to be consumed or supplied in the activity.") 1693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1694 protected Type product; 1695 1696 /** 1697 * Identifies the quantity expected to be consumed in a given day. 1698 */ 1699 @Child(name = "dailyAmount", type = { 1700 Quantity.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1701 @Description(shortDefinition = "How to consume/day?", formalDefinition = "Identifies the quantity expected to be consumed in a given day.") 1702 protected Quantity dailyAmount; 1703 1704 /** 1705 * Identifies the quantity expected to be supplied, administered or consumed by 1706 * the subject. 1707 */ 1708 @Child(name = "quantity", type = { 1709 Quantity.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1710 @Description(shortDefinition = "How much to administer/supply/consume", formalDefinition = "Identifies the quantity expected to be supplied, administered or consumed by the subject.") 1711 protected Quantity quantity; 1712 1713 /** 1714 * This provides a textual description of constraints on the intended activity 1715 * occurrence, including relation to other activities. It may also include 1716 * objectives, pre-conditions and end-conditions. Finally, it may convey 1717 * specifics about the activity such as body site, method, route, etc. 1718 */ 1719 @Child(name = "description", type = { 1720 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1721 @Description(shortDefinition = "Extra info describing activity to perform", formalDefinition = "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.") 1722 protected StringType description; 1723 1724 private static final long serialVersionUID = 1355568081L; 1725 1726 /** 1727 * Constructor 1728 */ 1729 public CarePlanActivityDetailComponent() { 1730 super(); 1731 } 1732 1733 /** 1734 * Constructor 1735 */ 1736 public CarePlanActivityDetailComponent(Enumeration<CarePlanActivityStatus> status) { 1737 super(); 1738 this.status = status; 1739 } 1740 1741 /** 1742 * @return {@link #kind} (A description of the kind of resource the in-line 1743 * definition of a care plan activity is representing. The 1744 * CarePlan.activity.detail is an in-line definition when a resource is 1745 * not referenced using CarePlan.activity.reference. For example, a 1746 * MedicationRequest, a ServiceRequest, or a CommunicationRequest.). 1747 * This is the underlying object with id, value and extensions. The 1748 * accessor "getKind" gives direct access to the value 1749 */ 1750 public Enumeration<CarePlanActivityKind> getKindElement() { 1751 if (this.kind == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.kind"); 1754 else if (Configuration.doAutoCreate()) 1755 this.kind = new Enumeration<CarePlanActivityKind>(new CarePlanActivityKindEnumFactory()); // bb 1756 return this.kind; 1757 } 1758 1759 public boolean hasKindElement() { 1760 return this.kind != null && !this.kind.isEmpty(); 1761 } 1762 1763 public boolean hasKind() { 1764 return this.kind != null && !this.kind.isEmpty(); 1765 } 1766 1767 /** 1768 * @param value {@link #kind} (A description of the kind of resource the in-line 1769 * definition of a care plan activity is representing. The 1770 * CarePlan.activity.detail is an in-line definition when a 1771 * resource is not referenced using CarePlan.activity.reference. 1772 * For example, a MedicationRequest, a ServiceRequest, or a 1773 * CommunicationRequest.). This is the underlying object with id, 1774 * value and extensions. The accessor "getKind" gives direct access 1775 * to the value 1776 */ 1777 public CarePlanActivityDetailComponent setKindElement(Enumeration<CarePlanActivityKind> value) { 1778 this.kind = value; 1779 return this; 1780 } 1781 1782 /** 1783 * @return A description of the kind of resource the in-line definition of a 1784 * care plan activity is representing. The CarePlan.activity.detail is 1785 * an in-line definition when a resource is not referenced using 1786 * CarePlan.activity.reference. For example, a MedicationRequest, a 1787 * ServiceRequest, or a CommunicationRequest. 1788 */ 1789 public CarePlanActivityKind getKind() { 1790 return this.kind == null ? null : this.kind.getValue(); 1791 } 1792 1793 /** 1794 * @param value A description of the kind of resource the in-line definition of 1795 * a care plan activity is representing. The 1796 * CarePlan.activity.detail is an in-line definition when a 1797 * resource is not referenced using CarePlan.activity.reference. 1798 * For example, a MedicationRequest, a ServiceRequest, or a 1799 * CommunicationRequest. 1800 */ 1801 public CarePlanActivityDetailComponent setKind(CarePlanActivityKind value) { 1802 if (value == null) 1803 this.kind = null; 1804 else { 1805 if (this.kind == null) 1806 this.kind = new Enumeration<CarePlanActivityKind>(new CarePlanActivityKindEnumFactory()); 1807 this.kind.setValue(value); 1808 } 1809 return this; 1810 } 1811 1812 /** 1813 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1814 * protocol, guideline, questionnaire or other definition that is 1815 * adhered to in whole or in part by this CarePlan activity.) 1816 */ 1817 public List<CanonicalType> getInstantiatesCanonical() { 1818 if (this.instantiatesCanonical == null) 1819 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1820 return this.instantiatesCanonical; 1821 } 1822 1823 /** 1824 * @return Returns a reference to <code>this</code> for easy method chaining 1825 */ 1826 public CarePlanActivityDetailComponent setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1827 this.instantiatesCanonical = theInstantiatesCanonical; 1828 return this; 1829 } 1830 1831 public boolean hasInstantiatesCanonical() { 1832 if (this.instantiatesCanonical == null) 1833 return false; 1834 for (CanonicalType item : this.instantiatesCanonical) 1835 if (!item.isEmpty()) 1836 return true; 1837 return false; 1838 } 1839 1840 /** 1841 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1842 * protocol, guideline, questionnaire or other definition that is 1843 * adhered to in whole or in part by this CarePlan activity.) 1844 */ 1845 public CanonicalType addInstantiatesCanonicalElement() {// 2 1846 CanonicalType t = new CanonicalType(); 1847 if (this.instantiatesCanonical == null) 1848 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1849 this.instantiatesCanonical.add(t); 1850 return t; 1851 } 1852 1853 /** 1854 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1855 * FHIR-defined protocol, guideline, questionnaire or other 1856 * definition that is adhered to in whole or in part by this 1857 * CarePlan activity.) 1858 */ 1859 public CarePlanActivityDetailComponent addInstantiatesCanonical(String value) { // 1 1860 CanonicalType t = new CanonicalType(); 1861 t.setValue(value); 1862 if (this.instantiatesCanonical == null) 1863 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1864 this.instantiatesCanonical.add(t); 1865 return this; 1866 } 1867 1868 /** 1869 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1870 * FHIR-defined protocol, guideline, questionnaire or other 1871 * definition that is adhered to in whole or in part by this 1872 * CarePlan activity.) 1873 */ 1874 public boolean hasInstantiatesCanonical(String value) { 1875 if (this.instantiatesCanonical == null) 1876 return false; 1877 for (CanonicalType v : this.instantiatesCanonical) 1878 if (v.getValue().equals(value)) // canonical(PlanDefinition|ActivityDefinition|Questionnaire|Measure|OperationDefinition) 1879 return true; 1880 return false; 1881 } 1882 1883 /** 1884 * @return {@link #instantiatesUri} (The URL pointing to an externally 1885 * maintained protocol, guideline, questionnaire or other definition 1886 * that is adhered to in whole or in part by this CarePlan activity.) 1887 */ 1888 public List<UriType> getInstantiatesUri() { 1889 if (this.instantiatesUri == null) 1890 this.instantiatesUri = new ArrayList<UriType>(); 1891 return this.instantiatesUri; 1892 } 1893 1894 /** 1895 * @return Returns a reference to <code>this</code> for easy method chaining 1896 */ 1897 public CarePlanActivityDetailComponent setInstantiatesUri(List<UriType> theInstantiatesUri) { 1898 this.instantiatesUri = theInstantiatesUri; 1899 return this; 1900 } 1901 1902 public boolean hasInstantiatesUri() { 1903 if (this.instantiatesUri == null) 1904 return false; 1905 for (UriType item : this.instantiatesUri) 1906 if (!item.isEmpty()) 1907 return true; 1908 return false; 1909 } 1910 1911 /** 1912 * @return {@link #instantiatesUri} (The URL pointing to an externally 1913 * maintained protocol, guideline, questionnaire or other definition 1914 * that is adhered to in whole or in part by this CarePlan activity.) 1915 */ 1916 public UriType addInstantiatesUriElement() {// 2 1917 UriType t = new UriType(); 1918 if (this.instantiatesUri == null) 1919 this.instantiatesUri = new ArrayList<UriType>(); 1920 this.instantiatesUri.add(t); 1921 return t; 1922 } 1923 1924 /** 1925 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1926 * maintained protocol, guideline, questionnaire or other 1927 * definition that is adhered to in whole or in part by this 1928 * CarePlan activity.) 1929 */ 1930 public CarePlanActivityDetailComponent addInstantiatesUri(String value) { // 1 1931 UriType t = new UriType(); 1932 t.setValue(value); 1933 if (this.instantiatesUri == null) 1934 this.instantiatesUri = new ArrayList<UriType>(); 1935 this.instantiatesUri.add(t); 1936 return this; 1937 } 1938 1939 /** 1940 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1941 * maintained protocol, guideline, questionnaire or other 1942 * definition that is adhered to in whole or in part by this 1943 * CarePlan activity.) 1944 */ 1945 public boolean hasInstantiatesUri(String value) { 1946 if (this.instantiatesUri == null) 1947 return false; 1948 for (UriType v : this.instantiatesUri) 1949 if (v.getValue().equals(value)) // uri 1950 return true; 1951 return false; 1952 } 1953 1954 /** 1955 * @return {@link #code} (Detailed description of the type of planned activity; 1956 * e.g. what lab test, what procedure, what kind of encounter.) 1957 */ 1958 public CodeableConcept getCode() { 1959 if (this.code == null) 1960 if (Configuration.errorOnAutoCreate()) 1961 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.code"); 1962 else if (Configuration.doAutoCreate()) 1963 this.code = new CodeableConcept(); // cc 1964 return this.code; 1965 } 1966 1967 public boolean hasCode() { 1968 return this.code != null && !this.code.isEmpty(); 1969 } 1970 1971 /** 1972 * @param value {@link #code} (Detailed description of the type of planned 1973 * activity; e.g. what lab test, what procedure, what kind of 1974 * encounter.) 1975 */ 1976 public CarePlanActivityDetailComponent setCode(CodeableConcept value) { 1977 this.code = value; 1978 return this; 1979 } 1980 1981 /** 1982 * @return {@link #reasonCode} (Provides the rationale that drove the inclusion 1983 * of this particular activity as part of the plan or the reason why the 1984 * activity was prohibited.) 1985 */ 1986 public List<CodeableConcept> getReasonCode() { 1987 if (this.reasonCode == null) 1988 this.reasonCode = new ArrayList<CodeableConcept>(); 1989 return this.reasonCode; 1990 } 1991 1992 /** 1993 * @return Returns a reference to <code>this</code> for easy method chaining 1994 */ 1995 public CarePlanActivityDetailComponent setReasonCode(List<CodeableConcept> theReasonCode) { 1996 this.reasonCode = theReasonCode; 1997 return this; 1998 } 1999 2000 public boolean hasReasonCode() { 2001 if (this.reasonCode == null) 2002 return false; 2003 for (CodeableConcept item : this.reasonCode) 2004 if (!item.isEmpty()) 2005 return true; 2006 return false; 2007 } 2008 2009 public CodeableConcept addReasonCode() { // 3 2010 CodeableConcept t = new CodeableConcept(); 2011 if (this.reasonCode == null) 2012 this.reasonCode = new ArrayList<CodeableConcept>(); 2013 this.reasonCode.add(t); 2014 return t; 2015 } 2016 2017 public CarePlanActivityDetailComponent addReasonCode(CodeableConcept t) { // 3 2018 if (t == null) 2019 return this; 2020 if (this.reasonCode == null) 2021 this.reasonCode = new ArrayList<CodeableConcept>(); 2022 this.reasonCode.add(t); 2023 return this; 2024 } 2025 2026 /** 2027 * @return The first repetition of repeating field {@link #reasonCode}, creating 2028 * it if it does not already exist 2029 */ 2030 public CodeableConcept getReasonCodeFirstRep() { 2031 if (getReasonCode().isEmpty()) { 2032 addReasonCode(); 2033 } 2034 return getReasonCode().get(0); 2035 } 2036 2037 /** 2038 * @return {@link #reasonReference} (Indicates another resource, such as the 2039 * health condition(s), whose existence justifies this request and drove 2040 * the inclusion of this particular activity as part of the plan.) 2041 */ 2042 public List<Reference> getReasonReference() { 2043 if (this.reasonReference == null) 2044 this.reasonReference = new ArrayList<Reference>(); 2045 return this.reasonReference; 2046 } 2047 2048 /** 2049 * @return Returns a reference to <code>this</code> for easy method chaining 2050 */ 2051 public CarePlanActivityDetailComponent setReasonReference(List<Reference> theReasonReference) { 2052 this.reasonReference = theReasonReference; 2053 return this; 2054 } 2055 2056 public boolean hasReasonReference() { 2057 if (this.reasonReference == null) 2058 return false; 2059 for (Reference item : this.reasonReference) 2060 if (!item.isEmpty()) 2061 return true; 2062 return false; 2063 } 2064 2065 public Reference addReasonReference() { // 3 2066 Reference t = new Reference(); 2067 if (this.reasonReference == null) 2068 this.reasonReference = new ArrayList<Reference>(); 2069 this.reasonReference.add(t); 2070 return t; 2071 } 2072 2073 public CarePlanActivityDetailComponent addReasonReference(Reference t) { // 3 2074 if (t == null) 2075 return this; 2076 if (this.reasonReference == null) 2077 this.reasonReference = new ArrayList<Reference>(); 2078 this.reasonReference.add(t); 2079 return this; 2080 } 2081 2082 /** 2083 * @return The first repetition of repeating field {@link #reasonReference}, 2084 * creating it if it does not already exist 2085 */ 2086 public Reference getReasonReferenceFirstRep() { 2087 if (getReasonReference().isEmpty()) { 2088 addReasonReference(); 2089 } 2090 return getReasonReference().get(0); 2091 } 2092 2093 /** 2094 * @deprecated Use Reference#setResource(IBaseResource) instead 2095 */ 2096 @Deprecated 2097 public List<Resource> getReasonReferenceTarget() { 2098 if (this.reasonReferenceTarget == null) 2099 this.reasonReferenceTarget = new ArrayList<Resource>(); 2100 return this.reasonReferenceTarget; 2101 } 2102 2103 /** 2104 * @return {@link #goal} (Internal reference that identifies the goals that this 2105 * activity is intended to contribute towards meeting.) 2106 */ 2107 public List<Reference> getGoal() { 2108 if (this.goal == null) 2109 this.goal = new ArrayList<Reference>(); 2110 return this.goal; 2111 } 2112 2113 /** 2114 * @return Returns a reference to <code>this</code> for easy method chaining 2115 */ 2116 public CarePlanActivityDetailComponent setGoal(List<Reference> theGoal) { 2117 this.goal = theGoal; 2118 return this; 2119 } 2120 2121 public boolean hasGoal() { 2122 if (this.goal == null) 2123 return false; 2124 for (Reference item : this.goal) 2125 if (!item.isEmpty()) 2126 return true; 2127 return false; 2128 } 2129 2130 public Reference addGoal() { // 3 2131 Reference t = new Reference(); 2132 if (this.goal == null) 2133 this.goal = new ArrayList<Reference>(); 2134 this.goal.add(t); 2135 return t; 2136 } 2137 2138 public CarePlanActivityDetailComponent addGoal(Reference t) { // 3 2139 if (t == null) 2140 return this; 2141 if (this.goal == null) 2142 this.goal = new ArrayList<Reference>(); 2143 this.goal.add(t); 2144 return this; 2145 } 2146 2147 /** 2148 * @return The first repetition of repeating field {@link #goal}, creating it if 2149 * it does not already exist 2150 */ 2151 public Reference getGoalFirstRep() { 2152 if (getGoal().isEmpty()) { 2153 addGoal(); 2154 } 2155 return getGoal().get(0); 2156 } 2157 2158 /** 2159 * @deprecated Use Reference#setResource(IBaseResource) instead 2160 */ 2161 @Deprecated 2162 public List<Goal> getGoalTarget() { 2163 if (this.goalTarget == null) 2164 this.goalTarget = new ArrayList<Goal>(); 2165 return this.goalTarget; 2166 } 2167 2168 /** 2169 * @deprecated Use Reference#setResource(IBaseResource) instead 2170 */ 2171 @Deprecated 2172 public Goal addGoalTarget() { 2173 Goal r = new Goal(); 2174 if (this.goalTarget == null) 2175 this.goalTarget = new ArrayList<Goal>(); 2176 this.goalTarget.add(r); 2177 return r; 2178 } 2179 2180 /** 2181 * @return {@link #status} (Identifies what progress is being made for the 2182 * specific activity.). This is the underlying object with id, value and 2183 * extensions. The accessor "getStatus" gives direct access to the value 2184 */ 2185 public Enumeration<CarePlanActivityStatus> getStatusElement() { 2186 if (this.status == null) 2187 if (Configuration.errorOnAutoCreate()) 2188 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.status"); 2189 else if (Configuration.doAutoCreate()) 2190 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); // bb 2191 return this.status; 2192 } 2193 2194 public boolean hasStatusElement() { 2195 return this.status != null && !this.status.isEmpty(); 2196 } 2197 2198 public boolean hasStatus() { 2199 return this.status != null && !this.status.isEmpty(); 2200 } 2201 2202 /** 2203 * @param value {@link #status} (Identifies what progress is being made for the 2204 * specific activity.). This is the underlying object with id, 2205 * value and extensions. The accessor "getStatus" gives direct 2206 * access to the value 2207 */ 2208 public CarePlanActivityDetailComponent setStatusElement(Enumeration<CarePlanActivityStatus> value) { 2209 this.status = value; 2210 return this; 2211 } 2212 2213 /** 2214 * @return Identifies what progress is being made for the specific activity. 2215 */ 2216 public CarePlanActivityStatus getStatus() { 2217 return this.status == null ? null : this.status.getValue(); 2218 } 2219 2220 /** 2221 * @param value Identifies what progress is being made for the specific 2222 * activity. 2223 */ 2224 public CarePlanActivityDetailComponent setStatus(CarePlanActivityStatus value) { 2225 if (this.status == null) 2226 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); 2227 this.status.setValue(value); 2228 return this; 2229 } 2230 2231 /** 2232 * @return {@link #statusReason} (Provides reason why the activity isn't yet 2233 * started, is on hold, was cancelled, etc.) 2234 */ 2235 public CodeableConcept getStatusReason() { 2236 if (this.statusReason == null) 2237 if (Configuration.errorOnAutoCreate()) 2238 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.statusReason"); 2239 else if (Configuration.doAutoCreate()) 2240 this.statusReason = new CodeableConcept(); // cc 2241 return this.statusReason; 2242 } 2243 2244 public boolean hasStatusReason() { 2245 return this.statusReason != null && !this.statusReason.isEmpty(); 2246 } 2247 2248 /** 2249 * @param value {@link #statusReason} (Provides reason why the activity isn't 2250 * yet started, is on hold, was cancelled, etc.) 2251 */ 2252 public CarePlanActivityDetailComponent setStatusReason(CodeableConcept value) { 2253 this.statusReason = value; 2254 return this; 2255 } 2256 2257 /** 2258 * @return {@link #doNotPerform} (If true, indicates that the described activity 2259 * is one that must NOT be engaged in when following the plan. If false, 2260 * or missing, indicates that the described activity is one that should 2261 * be engaged in when following the plan.). This is the underlying 2262 * object with id, value and extensions. The accessor "getDoNotPerform" 2263 * gives direct access to the value 2264 */ 2265 public BooleanType getDoNotPerformElement() { 2266 if (this.doNotPerform == null) 2267 if (Configuration.errorOnAutoCreate()) 2268 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.doNotPerform"); 2269 else if (Configuration.doAutoCreate()) 2270 this.doNotPerform = new BooleanType(); // bb 2271 return this.doNotPerform; 2272 } 2273 2274 public boolean hasDoNotPerformElement() { 2275 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2276 } 2277 2278 public boolean hasDoNotPerform() { 2279 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2280 } 2281 2282 /** 2283 * @param value {@link #doNotPerform} (If true, indicates that the described 2284 * activity is one that must NOT be engaged in when following the 2285 * plan. If false, or missing, indicates that the described 2286 * activity is one that should be engaged in when following the 2287 * plan.). This is the underlying object with id, value and 2288 * extensions. The accessor "getDoNotPerform" gives direct access 2289 * to the value 2290 */ 2291 public CarePlanActivityDetailComponent setDoNotPerformElement(BooleanType value) { 2292 this.doNotPerform = value; 2293 return this; 2294 } 2295 2296 /** 2297 * @return If true, indicates that the described activity is one that must NOT 2298 * be engaged in when following the plan. If false, or missing, 2299 * indicates that the described activity is one that should be engaged 2300 * in when following the plan. 2301 */ 2302 public boolean getDoNotPerform() { 2303 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 2304 } 2305 2306 /** 2307 * @param value If true, indicates that the described activity is one that must 2308 * NOT be engaged in when following the plan. If false, or missing, 2309 * indicates that the described activity is one that should be 2310 * engaged in when following the plan. 2311 */ 2312 public CarePlanActivityDetailComponent setDoNotPerform(boolean value) { 2313 if (this.doNotPerform == null) 2314 this.doNotPerform = new BooleanType(); 2315 this.doNotPerform.setValue(value); 2316 return this; 2317 } 2318 2319 /** 2320 * @return {@link #scheduled} (The period, timing or frequency upon which the 2321 * described activity is to occur.) 2322 */ 2323 public Type getScheduled() { 2324 return this.scheduled; 2325 } 2326 2327 /** 2328 * @return {@link #scheduled} (The period, timing or frequency upon which the 2329 * described activity is to occur.) 2330 */ 2331 public Timing getScheduledTiming() throws FHIRException { 2332 if (this.scheduled == null) 2333 this.scheduled = new Timing(); 2334 if (!(this.scheduled instanceof Timing)) 2335 throw new FHIRException("Type mismatch: the type Timing was expected, but " 2336 + this.scheduled.getClass().getName() + " was encountered"); 2337 return (Timing) this.scheduled; 2338 } 2339 2340 public boolean hasScheduledTiming() { 2341 return this != null && this.scheduled instanceof Timing; 2342 } 2343 2344 /** 2345 * @return {@link #scheduled} (The period, timing or frequency upon which the 2346 * described activity is to occur.) 2347 */ 2348 public Period getScheduledPeriod() throws FHIRException { 2349 if (this.scheduled == null) 2350 this.scheduled = new Period(); 2351 if (!(this.scheduled instanceof Period)) 2352 throw new FHIRException("Type mismatch: the type Period was expected, but " 2353 + this.scheduled.getClass().getName() + " was encountered"); 2354 return (Period) this.scheduled; 2355 } 2356 2357 public boolean hasScheduledPeriod() { 2358 return this != null && this.scheduled instanceof Period; 2359 } 2360 2361 /** 2362 * @return {@link #scheduled} (The period, timing or frequency upon which the 2363 * described activity is to occur.) 2364 */ 2365 public StringType getScheduledStringType() throws FHIRException { 2366 if (this.scheduled == null) 2367 this.scheduled = new StringType(); 2368 if (!(this.scheduled instanceof StringType)) 2369 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2370 + this.scheduled.getClass().getName() + " was encountered"); 2371 return (StringType) this.scheduled; 2372 } 2373 2374 public boolean hasScheduledStringType() { 2375 return this != null && this.scheduled instanceof StringType; 2376 } 2377 2378 public boolean hasScheduled() { 2379 return this.scheduled != null && !this.scheduled.isEmpty(); 2380 } 2381 2382 /** 2383 * @param value {@link #scheduled} (The period, timing or frequency upon which 2384 * the described activity is to occur.) 2385 */ 2386 public CarePlanActivityDetailComponent setScheduled(Type value) { 2387 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof StringType)) 2388 throw new Error("Not the right type for CarePlan.activity.detail.scheduled[x]: " + value.fhirType()); 2389 this.scheduled = value; 2390 return this; 2391 } 2392 2393 /** 2394 * @return {@link #location} (Identifies the facility where the activity will 2395 * occur; e.g. home, hospital, specific clinic, etc.) 2396 */ 2397 public Reference getLocation() { 2398 if (this.location == null) 2399 if (Configuration.errorOnAutoCreate()) 2400 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 2401 else if (Configuration.doAutoCreate()) 2402 this.location = new Reference(); // cc 2403 return this.location; 2404 } 2405 2406 public boolean hasLocation() { 2407 return this.location != null && !this.location.isEmpty(); 2408 } 2409 2410 /** 2411 * @param value {@link #location} (Identifies the facility where the activity 2412 * will occur; e.g. home, hospital, specific clinic, etc.) 2413 */ 2414 public CarePlanActivityDetailComponent setLocation(Reference value) { 2415 this.location = value; 2416 return this; 2417 } 2418 2419 /** 2420 * @return {@link #location} The actual object that is the target of the 2421 * reference. The reference library doesn't populate this, but you can 2422 * use it to hold the resource if you resolve it. (Identifies the 2423 * facility where the activity will occur; e.g. home, hospital, specific 2424 * clinic, etc.) 2425 */ 2426 public Location getLocationTarget() { 2427 if (this.locationTarget == null) 2428 if (Configuration.errorOnAutoCreate()) 2429 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 2430 else if (Configuration.doAutoCreate()) 2431 this.locationTarget = new Location(); // aa 2432 return this.locationTarget; 2433 } 2434 2435 /** 2436 * @param value {@link #location} The actual object that is the target of the 2437 * reference. The reference library doesn't use these, but you can 2438 * use it to hold the resource if you resolve it. (Identifies the 2439 * facility where the activity will occur; e.g. home, hospital, 2440 * specific clinic, etc.) 2441 */ 2442 public CarePlanActivityDetailComponent setLocationTarget(Location value) { 2443 this.locationTarget = value; 2444 return this; 2445 } 2446 2447 /** 2448 * @return {@link #performer} (Identifies who's expected to be involved in the 2449 * activity.) 2450 */ 2451 public List<Reference> getPerformer() { 2452 if (this.performer == null) 2453 this.performer = new ArrayList<Reference>(); 2454 return this.performer; 2455 } 2456 2457 /** 2458 * @return Returns a reference to <code>this</code> for easy method chaining 2459 */ 2460 public CarePlanActivityDetailComponent setPerformer(List<Reference> thePerformer) { 2461 this.performer = thePerformer; 2462 return this; 2463 } 2464 2465 public boolean hasPerformer() { 2466 if (this.performer == null) 2467 return false; 2468 for (Reference item : this.performer) 2469 if (!item.isEmpty()) 2470 return true; 2471 return false; 2472 } 2473 2474 public Reference addPerformer() { // 3 2475 Reference t = new Reference(); 2476 if (this.performer == null) 2477 this.performer = new ArrayList<Reference>(); 2478 this.performer.add(t); 2479 return t; 2480 } 2481 2482 public CarePlanActivityDetailComponent addPerformer(Reference t) { // 3 2483 if (t == null) 2484 return this; 2485 if (this.performer == null) 2486 this.performer = new ArrayList<Reference>(); 2487 this.performer.add(t); 2488 return this; 2489 } 2490 2491 /** 2492 * @return The first repetition of repeating field {@link #performer}, creating 2493 * it if it does not already exist 2494 */ 2495 public Reference getPerformerFirstRep() { 2496 if (getPerformer().isEmpty()) { 2497 addPerformer(); 2498 } 2499 return getPerformer().get(0); 2500 } 2501 2502 /** 2503 * @deprecated Use Reference#setResource(IBaseResource) instead 2504 */ 2505 @Deprecated 2506 public List<Resource> getPerformerTarget() { 2507 if (this.performerTarget == null) 2508 this.performerTarget = new ArrayList<Resource>(); 2509 return this.performerTarget; 2510 } 2511 2512 /** 2513 * @return {@link #product} (Identifies the food, drug or other product to be 2514 * consumed or supplied in the activity.) 2515 */ 2516 public Type getProduct() { 2517 return this.product; 2518 } 2519 2520 /** 2521 * @return {@link #product} (Identifies the food, drug or other product to be 2522 * consumed or supplied in the activity.) 2523 */ 2524 public CodeableConcept getProductCodeableConcept() throws FHIRException { 2525 if (this.product == null) 2526 this.product = new CodeableConcept(); 2527 if (!(this.product instanceof CodeableConcept)) 2528 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2529 + this.product.getClass().getName() + " was encountered"); 2530 return (CodeableConcept) this.product; 2531 } 2532 2533 public boolean hasProductCodeableConcept() { 2534 return this != null && this.product instanceof CodeableConcept; 2535 } 2536 2537 /** 2538 * @return {@link #product} (Identifies the food, drug or other product to be 2539 * consumed or supplied in the activity.) 2540 */ 2541 public Reference getProductReference() throws FHIRException { 2542 if (this.product == null) 2543 this.product = new Reference(); 2544 if (!(this.product instanceof Reference)) 2545 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2546 + this.product.getClass().getName() + " was encountered"); 2547 return (Reference) this.product; 2548 } 2549 2550 public boolean hasProductReference() { 2551 return this != null && this.product instanceof Reference; 2552 } 2553 2554 public boolean hasProduct() { 2555 return this.product != null && !this.product.isEmpty(); 2556 } 2557 2558 /** 2559 * @param value {@link #product} (Identifies the food, drug or other product to 2560 * be consumed or supplied in the activity.) 2561 */ 2562 public CarePlanActivityDetailComponent setProduct(Type value) { 2563 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2564 throw new Error("Not the right type for CarePlan.activity.detail.product[x]: " + value.fhirType()); 2565 this.product = value; 2566 return this; 2567 } 2568 2569 /** 2570 * @return {@link #dailyAmount} (Identifies the quantity expected to be consumed 2571 * in a given day.) 2572 */ 2573 public Quantity getDailyAmount() { 2574 if (this.dailyAmount == null) 2575 if (Configuration.errorOnAutoCreate()) 2576 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.dailyAmount"); 2577 else if (Configuration.doAutoCreate()) 2578 this.dailyAmount = new Quantity(); // cc 2579 return this.dailyAmount; 2580 } 2581 2582 public boolean hasDailyAmount() { 2583 return this.dailyAmount != null && !this.dailyAmount.isEmpty(); 2584 } 2585 2586 /** 2587 * @param value {@link #dailyAmount} (Identifies the quantity expected to be 2588 * consumed in a given day.) 2589 */ 2590 public CarePlanActivityDetailComponent setDailyAmount(Quantity value) { 2591 this.dailyAmount = value; 2592 return this; 2593 } 2594 2595 /** 2596 * @return {@link #quantity} (Identifies the quantity expected to be supplied, 2597 * administered or consumed by the subject.) 2598 */ 2599 public Quantity getQuantity() { 2600 if (this.quantity == null) 2601 if (Configuration.errorOnAutoCreate()) 2602 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.quantity"); 2603 else if (Configuration.doAutoCreate()) 2604 this.quantity = new Quantity(); // cc 2605 return this.quantity; 2606 } 2607 2608 public boolean hasQuantity() { 2609 return this.quantity != null && !this.quantity.isEmpty(); 2610 } 2611 2612 /** 2613 * @param value {@link #quantity} (Identifies the quantity expected to be 2614 * supplied, administered or consumed by the subject.) 2615 */ 2616 public CarePlanActivityDetailComponent setQuantity(Quantity value) { 2617 this.quantity = value; 2618 return this; 2619 } 2620 2621 /** 2622 * @return {@link #description} (This provides a textual description of 2623 * constraints on the intended activity occurrence, including relation 2624 * to other activities. It may also include objectives, pre-conditions 2625 * and end-conditions. Finally, it may convey specifics about the 2626 * activity such as body site, method, route, etc.). This is the 2627 * underlying object with id, value and extensions. The accessor 2628 * "getDescription" gives direct access to the value 2629 */ 2630 public StringType getDescriptionElement() { 2631 if (this.description == null) 2632 if (Configuration.errorOnAutoCreate()) 2633 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.description"); 2634 else if (Configuration.doAutoCreate()) 2635 this.description = new StringType(); // bb 2636 return this.description; 2637 } 2638 2639 public boolean hasDescriptionElement() { 2640 return this.description != null && !this.description.isEmpty(); 2641 } 2642 2643 public boolean hasDescription() { 2644 return this.description != null && !this.description.isEmpty(); 2645 } 2646 2647 /** 2648 * @param value {@link #description} (This provides a textual description of 2649 * constraints on the intended activity occurrence, including 2650 * relation to other activities. It may also include objectives, 2651 * pre-conditions and end-conditions. Finally, it may convey 2652 * specifics about the activity such as body site, method, route, 2653 * etc.). This is the underlying object with id, value and 2654 * extensions. The accessor "getDescription" gives direct access to 2655 * the value 2656 */ 2657 public CarePlanActivityDetailComponent setDescriptionElement(StringType value) { 2658 this.description = value; 2659 return this; 2660 } 2661 2662 /** 2663 * @return This provides a textual description of constraints on the intended 2664 * activity occurrence, including relation to other activities. It may 2665 * also include objectives, pre-conditions and end-conditions. Finally, 2666 * it may convey specifics about the activity such as body site, method, 2667 * route, etc. 2668 */ 2669 public String getDescription() { 2670 return this.description == null ? null : this.description.getValue(); 2671 } 2672 2673 /** 2674 * @param value This provides a textual description of constraints on the 2675 * intended activity occurrence, including relation to other 2676 * activities. It may also include objectives, pre-conditions and 2677 * end-conditions. Finally, it may convey specifics about the 2678 * activity such as body site, method, route, etc. 2679 */ 2680 public CarePlanActivityDetailComponent setDescription(String value) { 2681 if (Utilities.noString(value)) 2682 this.description = null; 2683 else { 2684 if (this.description == null) 2685 this.description = new StringType(); 2686 this.description.setValue(value); 2687 } 2688 return this; 2689 } 2690 2691 protected void listChildren(List<Property> children) { 2692 super.listChildren(children); 2693 children.add(new Property("kind", "code", 2694 "A description of the kind of resource the in-line definition of a care plan activity is representing. The CarePlan.activity.detail is an in-line definition when a resource is not referenced using CarePlan.activity.reference. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.", 2695 0, 1, kind)); 2696 children.add(new Property("instantiatesCanonical", 2697 "canonical(PlanDefinition|ActivityDefinition|Questionnaire|Measure|OperationDefinition)", 2698 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2699 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2700 children.add(new Property("instantiatesUri", "uri", 2701 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2702 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2703 children.add(new Property("code", "CodeableConcept", 2704 "Detailed description of the type of planned activity; e.g. what lab test, what procedure, what kind of encounter.", 2705 0, 1, code)); 2706 children.add(new Property("reasonCode", "CodeableConcept", 2707 "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.", 2708 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2709 children.add(new Property("reasonReference", 2710 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2711 "Indicates another resource, such as the health condition(s), whose existence justifies this request and drove the inclusion of this particular activity as part of the plan.", 2712 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2713 children.add(new Property("goal", "Reference(Goal)", 2714 "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 2715 0, java.lang.Integer.MAX_VALUE, goal)); 2716 children.add(new Property("status", "code", "Identifies what progress is being made for the specific activity.", 2717 0, 1, status)); 2718 children.add(new Property("statusReason", "CodeableConcept", 2719 "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 1, statusReason)); 2720 children.add(new Property("doNotPerform", "boolean", 2721 "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, or missing, indicates that the described activity is one that should be engaged in when following the plan.", 2722 0, 1, doNotPerform)); 2723 children.add(new Property("scheduled[x]", "Timing|Period|string", 2724 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled)); 2725 children.add(new Property("location", "Reference(Location)", 2726 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 2727 location)); 2728 children.add(new Property("performer", 2729 "Reference(Practitioner|PractitionerRole|Organization|RelatedPerson|Patient|CareTeam|HealthcareService|Device)", 2730 "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer)); 2731 children.add(new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2732 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product)); 2733 children.add(new Property("dailyAmount", "SimpleQuantity", 2734 "Identifies the quantity expected to be consumed in a given day.", 0, 1, dailyAmount)); 2735 children.add(new Property("quantity", "SimpleQuantity", 2736 "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 1, quantity)); 2737 children.add(new Property("description", "string", 2738 "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 2739 0, 1, description)); 2740 } 2741 2742 @Override 2743 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2744 switch (_hash) { 2745 case 3292052: 2746 /* kind */ return new Property("kind", "code", 2747 "A description of the kind of resource the in-line definition of a care plan activity is representing. The CarePlan.activity.detail is an in-line definition when a resource is not referenced using CarePlan.activity.reference. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.", 2748 0, 1, kind); 2749 case 8911915: 2750 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 2751 "canonical(PlanDefinition|ActivityDefinition|Questionnaire|Measure|OperationDefinition)", 2752 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2753 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2754 case -1926393373: 2755 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 2756 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan activity.", 2757 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2758 case 3059181: 2759 /* code */ return new Property("code", "CodeableConcept", 2760 "Detailed description of the type of planned activity; e.g. what lab test, what procedure, what kind of encounter.", 2761 0, 1, code); 2762 case 722137681: 2763 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2764 "Provides the rationale that drove the inclusion of this particular activity as part of the plan or the reason why the activity was prohibited.", 2765 0, java.lang.Integer.MAX_VALUE, reasonCode); 2766 case -1146218137: 2767 /* reasonReference */ return new Property("reasonReference", 2768 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2769 "Indicates another resource, such as the health condition(s), whose existence justifies this request and drove the inclusion of this particular activity as part of the plan.", 2770 0, java.lang.Integer.MAX_VALUE, reasonReference); 2771 case 3178259: 2772 /* goal */ return new Property("goal", "Reference(Goal)", 2773 "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 2774 0, java.lang.Integer.MAX_VALUE, goal); 2775 case -892481550: 2776 /* status */ return new Property("status", "code", 2777 "Identifies what progress is being made for the specific activity.", 0, 1, status); 2778 case 2051346646: 2779 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2780 "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 1, statusReason); 2781 case -1788508167: 2782 /* doNotPerform */ return new Property("doNotPerform", "boolean", 2783 "If true, indicates that the described activity is one that must NOT be engaged in when following the plan. If false, or missing, indicates that the described activity is one that should be engaged in when following the plan.", 2784 0, 1, doNotPerform); 2785 case 1162627251: 2786 /* scheduled[x] */ return new Property("scheduled[x]", "Timing|Period|string", 2787 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2788 case -160710483: 2789 /* scheduled */ return new Property("scheduled[x]", "Timing|Period|string", 2790 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2791 case 998483799: 2792 /* scheduledTiming */ return new Property("scheduled[x]", "Timing|Period|string", 2793 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2794 case 880422094: 2795 /* scheduledPeriod */ return new Property("scheduled[x]", "Timing|Period|string", 2796 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2797 case 980162334: 2798 /* scheduledString */ return new Property("scheduled[x]", "Timing|Period|string", 2799 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, scheduled); 2800 case 1901043637: 2801 /* location */ return new Property("location", "Reference(Location)", 2802 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 2803 location); 2804 case 481140686: 2805 /* performer */ return new Property("performer", 2806 "Reference(Practitioner|PractitionerRole|Organization|RelatedPerson|Patient|CareTeam|HealthcareService|Device)", 2807 "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer); 2808 case 1753005361: 2809 /* product[x] */ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2810 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2811 case -309474065: 2812 /* product */ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2813 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2814 case 906854066: 2815 /* productCodeableConcept */ return new Property("product[x]", 2816 "CodeableConcept|Reference(Medication|Substance)", 2817 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2818 case -669667556: 2819 /* productReference */ return new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2820 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 1, product); 2821 case -768908335: 2822 /* dailyAmount */ return new Property("dailyAmount", "SimpleQuantity", 2823 "Identifies the quantity expected to be consumed in a given day.", 0, 1, dailyAmount); 2824 case -1285004149: 2825 /* quantity */ return new Property("quantity", "SimpleQuantity", 2826 "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 1, 2827 quantity); 2828 case -1724546052: 2829 /* description */ return new Property("description", "string", 2830 "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 2831 0, 1, description); 2832 default: 2833 return super.getNamedProperty(_hash, _name, _checkValid); 2834 } 2835 2836 } 2837 2838 @Override 2839 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2840 switch (hash) { 2841 case 3292052: 2842 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<CarePlanActivityKind> 2843 case 8911915: 2844 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 2845 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2846 case -1926393373: 2847 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 2848 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2849 case 3059181: 2850 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2851 case 722137681: 2852 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2853 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2854 case -1146218137: 2855 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2856 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2857 case 3178259: 2858 /* goal */ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 2859 case -892481550: 2860 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CarePlanActivityStatus> 2861 case 2051346646: 2862 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2863 case -1788508167: 2864 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 2865 case -160710483: 2866 /* scheduled */ return this.scheduled == null ? new Base[0] : new Base[] { this.scheduled }; // Type 2867 case 1901043637: 2868 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 2869 case 481140686: 2870 /* performer */ return this.performer == null ? new Base[0] 2871 : this.performer.toArray(new Base[this.performer.size()]); // Reference 2872 case -309474065: 2873 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // Type 2874 case -768908335: 2875 /* dailyAmount */ return this.dailyAmount == null ? new Base[0] : new Base[] { this.dailyAmount }; // Quantity 2876 case -1285004149: 2877 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 2878 case -1724546052: 2879 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2880 default: 2881 return super.getProperty(hash, name, checkValid); 2882 } 2883 2884 } 2885 2886 @Override 2887 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2888 switch (hash) { 2889 case 3292052: // kind 2890 value = new CarePlanActivityKindEnumFactory().fromType(castToCode(value)); 2891 this.kind = (Enumeration) value; // Enumeration<CarePlanActivityKind> 2892 return value; 2893 case 8911915: // instantiatesCanonical 2894 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 2895 return value; 2896 case -1926393373: // instantiatesUri 2897 this.getInstantiatesUri().add(castToUri(value)); // UriType 2898 return value; 2899 case 3059181: // code 2900 this.code = castToCodeableConcept(value); // CodeableConcept 2901 return value; 2902 case 722137681: // reasonCode 2903 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2904 return value; 2905 case -1146218137: // reasonReference 2906 this.getReasonReference().add(castToReference(value)); // Reference 2907 return value; 2908 case 3178259: // goal 2909 this.getGoal().add(castToReference(value)); // Reference 2910 return value; 2911 case -892481550: // status 2912 value = new CarePlanActivityStatusEnumFactory().fromType(castToCode(value)); 2913 this.status = (Enumeration) value; // Enumeration<CarePlanActivityStatus> 2914 return value; 2915 case 2051346646: // statusReason 2916 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2917 return value; 2918 case -1788508167: // doNotPerform 2919 this.doNotPerform = castToBoolean(value); // BooleanType 2920 return value; 2921 case -160710483: // scheduled 2922 this.scheduled = castToType(value); // Type 2923 return value; 2924 case 1901043637: // location 2925 this.location = castToReference(value); // Reference 2926 return value; 2927 case 481140686: // performer 2928 this.getPerformer().add(castToReference(value)); // Reference 2929 return value; 2930 case -309474065: // product 2931 this.product = castToType(value); // Type 2932 return value; 2933 case -768908335: // dailyAmount 2934 this.dailyAmount = castToQuantity(value); // Quantity 2935 return value; 2936 case -1285004149: // quantity 2937 this.quantity = castToQuantity(value); // Quantity 2938 return value; 2939 case -1724546052: // description 2940 this.description = castToString(value); // StringType 2941 return value; 2942 default: 2943 return super.setProperty(hash, name, value); 2944 } 2945 2946 } 2947 2948 @Override 2949 public Base setProperty(String name, Base value) throws FHIRException { 2950 if (name.equals("kind")) { 2951 value = new CarePlanActivityKindEnumFactory().fromType(castToCode(value)); 2952 this.kind = (Enumeration) value; // Enumeration<CarePlanActivityKind> 2953 } else if (name.equals("instantiatesCanonical")) { 2954 this.getInstantiatesCanonical().add(castToCanonical(value)); 2955 } else if (name.equals("instantiatesUri")) { 2956 this.getInstantiatesUri().add(castToUri(value)); 2957 } else if (name.equals("code")) { 2958 this.code = castToCodeableConcept(value); // CodeableConcept 2959 } else if (name.equals("reasonCode")) { 2960 this.getReasonCode().add(castToCodeableConcept(value)); 2961 } else if (name.equals("reasonReference")) { 2962 this.getReasonReference().add(castToReference(value)); 2963 } else if (name.equals("goal")) { 2964 this.getGoal().add(castToReference(value)); 2965 } else if (name.equals("status")) { 2966 value = new CarePlanActivityStatusEnumFactory().fromType(castToCode(value)); 2967 this.status = (Enumeration) value; // Enumeration<CarePlanActivityStatus> 2968 } else if (name.equals("statusReason")) { 2969 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2970 } else if (name.equals("doNotPerform")) { 2971 this.doNotPerform = castToBoolean(value); // BooleanType 2972 } else if (name.equals("scheduled[x]")) { 2973 this.scheduled = castToType(value); // Type 2974 } else if (name.equals("location")) { 2975 this.location = castToReference(value); // Reference 2976 } else if (name.equals("performer")) { 2977 this.getPerformer().add(castToReference(value)); 2978 } else if (name.equals("product[x]")) { 2979 this.product = castToType(value); // Type 2980 } else if (name.equals("dailyAmount")) { 2981 this.dailyAmount = castToQuantity(value); // Quantity 2982 } else if (name.equals("quantity")) { 2983 this.quantity = castToQuantity(value); // Quantity 2984 } else if (name.equals("description")) { 2985 this.description = castToString(value); // StringType 2986 } else 2987 return super.setProperty(name, value); 2988 return value; 2989 } 2990 2991 @Override 2992 public void removeChild(String name, Base value) throws FHIRException { 2993 if (name.equals("kind")) { 2994 this.kind = null; 2995 } else if (name.equals("instantiatesCanonical")) { 2996 this.getInstantiatesCanonical().remove(castToCanonical(value)); 2997 } else if (name.equals("instantiatesUri")) { 2998 this.getInstantiatesUri().remove(castToUri(value)); 2999 } else if (name.equals("code")) { 3000 this.code = null; 3001 } else if (name.equals("reasonCode")) { 3002 this.getReasonCode().remove(castToCodeableConcept(value)); 3003 } else if (name.equals("reasonReference")) { 3004 this.getReasonReference().remove(castToReference(value)); 3005 } else if (name.equals("goal")) { 3006 this.getGoal().remove(castToReference(value)); 3007 } else if (name.equals("status")) { 3008 this.status = null; 3009 } else if (name.equals("statusReason")) { 3010 this.statusReason = null; 3011 } else if (name.equals("doNotPerform")) { 3012 this.doNotPerform = null; 3013 } else if (name.equals("scheduled[x]")) { 3014 this.scheduled = null; 3015 } else if (name.equals("location")) { 3016 this.location = null; 3017 } else if (name.equals("performer")) { 3018 this.getPerformer().remove(castToReference(value)); 3019 } else if (name.equals("product[x]")) { 3020 this.product = null; 3021 } else if (name.equals("dailyAmount")) { 3022 this.dailyAmount = null; 3023 } else if (name.equals("quantity")) { 3024 this.quantity = null; 3025 } else if (name.equals("description")) { 3026 this.description = null; 3027 } else 3028 super.removeChild(name, value); 3029 3030 } 3031 3032 @Override 3033 public Base makeProperty(int hash, String name) throws FHIRException { 3034 switch (hash) { 3035 case 3292052: 3036 return getKindElement(); 3037 case 8911915: 3038 return addInstantiatesCanonicalElement(); 3039 case -1926393373: 3040 return addInstantiatesUriElement(); 3041 case 3059181: 3042 return getCode(); 3043 case 722137681: 3044 return addReasonCode(); 3045 case -1146218137: 3046 return addReasonReference(); 3047 case 3178259: 3048 return addGoal(); 3049 case -892481550: 3050 return getStatusElement(); 3051 case 2051346646: 3052 return getStatusReason(); 3053 case -1788508167: 3054 return getDoNotPerformElement(); 3055 case 1162627251: 3056 return getScheduled(); 3057 case -160710483: 3058 return getScheduled(); 3059 case 1901043637: 3060 return getLocation(); 3061 case 481140686: 3062 return addPerformer(); 3063 case 1753005361: 3064 return getProduct(); 3065 case -309474065: 3066 return getProduct(); 3067 case -768908335: 3068 return getDailyAmount(); 3069 case -1285004149: 3070 return getQuantity(); 3071 case -1724546052: 3072 return getDescriptionElement(); 3073 default: 3074 return super.makeProperty(hash, name); 3075 } 3076 3077 } 3078 3079 @Override 3080 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3081 switch (hash) { 3082 case 3292052: 3083 /* kind */ return new String[] { "code" }; 3084 case 8911915: 3085 /* instantiatesCanonical */ return new String[] { "canonical" }; 3086 case -1926393373: 3087 /* instantiatesUri */ return new String[] { "uri" }; 3088 case 3059181: 3089 /* code */ return new String[] { "CodeableConcept" }; 3090 case 722137681: 3091 /* reasonCode */ return new String[] { "CodeableConcept" }; 3092 case -1146218137: 3093 /* reasonReference */ return new String[] { "Reference" }; 3094 case 3178259: 3095 /* goal */ return new String[] { "Reference" }; 3096 case -892481550: 3097 /* status */ return new String[] { "code" }; 3098 case 2051346646: 3099 /* statusReason */ return new String[] { "CodeableConcept" }; 3100 case -1788508167: 3101 /* doNotPerform */ return new String[] { "boolean" }; 3102 case -160710483: 3103 /* scheduled */ return new String[] { "Timing", "Period", "string" }; 3104 case 1901043637: 3105 /* location */ return new String[] { "Reference" }; 3106 case 481140686: 3107 /* performer */ return new String[] { "Reference" }; 3108 case -309474065: 3109 /* product */ return new String[] { "CodeableConcept", "Reference" }; 3110 case -768908335: 3111 /* dailyAmount */ return new String[] { "SimpleQuantity" }; 3112 case -1285004149: 3113 /* quantity */ return new String[] { "SimpleQuantity" }; 3114 case -1724546052: 3115 /* description */ return new String[] { "string" }; 3116 default: 3117 return super.getTypesForProperty(hash, name); 3118 } 3119 3120 } 3121 3122 @Override 3123 public Base addChild(String name) throws FHIRException { 3124 if (name.equals("kind")) { 3125 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.kind"); 3126 } else if (name.equals("instantiatesCanonical")) { 3127 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesCanonical"); 3128 } else if (name.equals("instantiatesUri")) { 3129 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesUri"); 3130 } else if (name.equals("code")) { 3131 this.code = new CodeableConcept(); 3132 return this.code; 3133 } else if (name.equals("reasonCode")) { 3134 return addReasonCode(); 3135 } else if (name.equals("reasonReference")) { 3136 return addReasonReference(); 3137 } else if (name.equals("goal")) { 3138 return addGoal(); 3139 } else if (name.equals("status")) { 3140 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 3141 } else if (name.equals("statusReason")) { 3142 this.statusReason = new CodeableConcept(); 3143 return this.statusReason; 3144 } else if (name.equals("doNotPerform")) { 3145 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.doNotPerform"); 3146 } else if (name.equals("scheduledTiming")) { 3147 this.scheduled = new Timing(); 3148 return this.scheduled; 3149 } else if (name.equals("scheduledPeriod")) { 3150 this.scheduled = new Period(); 3151 return this.scheduled; 3152 } else if (name.equals("scheduledString")) { 3153 this.scheduled = new StringType(); 3154 return this.scheduled; 3155 } else if (name.equals("location")) { 3156 this.location = new Reference(); 3157 return this.location; 3158 } else if (name.equals("performer")) { 3159 return addPerformer(); 3160 } else if (name.equals("productCodeableConcept")) { 3161 this.product = new CodeableConcept(); 3162 return this.product; 3163 } else if (name.equals("productReference")) { 3164 this.product = new Reference(); 3165 return this.product; 3166 } else if (name.equals("dailyAmount")) { 3167 this.dailyAmount = new Quantity(); 3168 return this.dailyAmount; 3169 } else if (name.equals("quantity")) { 3170 this.quantity = new Quantity(); 3171 return this.quantity; 3172 } else if (name.equals("description")) { 3173 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 3174 } else 3175 return super.addChild(name); 3176 } 3177 3178 public CarePlanActivityDetailComponent copy() { 3179 CarePlanActivityDetailComponent dst = new CarePlanActivityDetailComponent(); 3180 copyValues(dst); 3181 return dst; 3182 } 3183 3184 public void copyValues(CarePlanActivityDetailComponent dst) { 3185 super.copyValues(dst); 3186 dst.kind = kind == null ? null : kind.copy(); 3187 if (instantiatesCanonical != null) { 3188 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 3189 for (CanonicalType i : instantiatesCanonical) 3190 dst.instantiatesCanonical.add(i.copy()); 3191 } 3192 ; 3193 if (instantiatesUri != null) { 3194 dst.instantiatesUri = new ArrayList<UriType>(); 3195 for (UriType i : instantiatesUri) 3196 dst.instantiatesUri.add(i.copy()); 3197 } 3198 ; 3199 dst.code = code == null ? null : code.copy(); 3200 if (reasonCode != null) { 3201 dst.reasonCode = new ArrayList<CodeableConcept>(); 3202 for (CodeableConcept i : reasonCode) 3203 dst.reasonCode.add(i.copy()); 3204 } 3205 ; 3206 if (reasonReference != null) { 3207 dst.reasonReference = new ArrayList<Reference>(); 3208 for (Reference i : reasonReference) 3209 dst.reasonReference.add(i.copy()); 3210 } 3211 ; 3212 if (goal != null) { 3213 dst.goal = new ArrayList<Reference>(); 3214 for (Reference i : goal) 3215 dst.goal.add(i.copy()); 3216 } 3217 ; 3218 dst.status = status == null ? null : status.copy(); 3219 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3220 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 3221 dst.scheduled = scheduled == null ? null : scheduled.copy(); 3222 dst.location = location == null ? null : location.copy(); 3223 if (performer != null) { 3224 dst.performer = new ArrayList<Reference>(); 3225 for (Reference i : performer) 3226 dst.performer.add(i.copy()); 3227 } 3228 ; 3229 dst.product = product == null ? null : product.copy(); 3230 dst.dailyAmount = dailyAmount == null ? null : dailyAmount.copy(); 3231 dst.quantity = quantity == null ? null : quantity.copy(); 3232 dst.description = description == null ? null : description.copy(); 3233 } 3234 3235 @Override 3236 public boolean equalsDeep(Base other_) { 3237 if (!super.equalsDeep(other_)) 3238 return false; 3239 if (!(other_ instanceof CarePlanActivityDetailComponent)) 3240 return false; 3241 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other_; 3242 return compareDeep(kind, o.kind, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3243 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(code, o.code, true) 3244 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3245 && compareDeep(goal, o.goal, true) && compareDeep(status, o.status, true) 3246 && compareDeep(statusReason, o.statusReason, true) && compareDeep(doNotPerform, o.doNotPerform, true) 3247 && compareDeep(scheduled, o.scheduled, true) && compareDeep(location, o.location, true) 3248 && compareDeep(performer, o.performer, true) && compareDeep(product, o.product, true) 3249 && compareDeep(dailyAmount, o.dailyAmount, true) && compareDeep(quantity, o.quantity, true) 3250 && compareDeep(description, o.description, true); 3251 } 3252 3253 @Override 3254 public boolean equalsShallow(Base other_) { 3255 if (!super.equalsShallow(other_)) 3256 return false; 3257 if (!(other_ instanceof CarePlanActivityDetailComponent)) 3258 return false; 3259 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other_; 3260 return compareValues(kind, o.kind, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 3261 && compareValues(status, o.status, true) && compareValues(doNotPerform, o.doNotPerform, true) 3262 && compareValues(description, o.description, true); 3263 } 3264 3265 public boolean isEmpty() { 3266 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, instantiatesCanonical, instantiatesUri, code, 3267 reasonCode, reasonReference, goal, status, statusReason, doNotPerform, scheduled, location, performer, 3268 product, dailyAmount, quantity, description); 3269 } 3270 3271 public String fhirType() { 3272 return "CarePlan.activity.detail"; 3273 3274 } 3275 3276 } 3277 3278 /** 3279 * Business identifiers assigned to this care plan by the performer or other 3280 * systems which remain constant as the resource is updated and propagates from 3281 * server to server. 3282 */ 3283 @Child(name = "identifier", type = { 3284 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3285 @Description(shortDefinition = "External Ids for this plan", formalDefinition = "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 3286 protected List<Identifier> identifier; 3287 3288 /** 3289 * The URL pointing to a FHIR-defined protocol, guideline, questionnaire or 3290 * other definition that is adhered to in whole or in part by this CarePlan. 3291 */ 3292 @Child(name = "instantiatesCanonical", type = { 3293 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3294 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.") 3295 protected List<CanonicalType> instantiatesCanonical; 3296 3297 /** 3298 * The URL pointing to an externally maintained protocol, guideline, 3299 * questionnaire or other definition that is adhered to in whole or in part by 3300 * this CarePlan. 3301 */ 3302 @Child(name = "instantiatesUri", type = { 3303 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3304 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.") 3305 protected List<UriType> instantiatesUri; 3306 3307 /** 3308 * A care plan that is fulfilled in whole or in part by this care plan. 3309 */ 3310 @Child(name = "basedOn", type = { 3311 CarePlan.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3312 @Description(shortDefinition = "Fulfills CarePlan", formalDefinition = "A care plan that is fulfilled in whole or in part by this care plan.") 3313 protected List<Reference> basedOn; 3314 /** 3315 * The actual objects that are the target of the reference (A care plan that is 3316 * fulfilled in whole or in part by this care plan.) 3317 */ 3318 protected List<CarePlan> basedOnTarget; 3319 3320 /** 3321 * Completed or terminated care plan whose function is taken by this new care 3322 * plan. 3323 */ 3324 @Child(name = "replaces", type = { 3325 CarePlan.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3326 @Description(shortDefinition = "CarePlan replaced by this CarePlan", formalDefinition = "Completed or terminated care plan whose function is taken by this new care plan.") 3327 protected List<Reference> replaces; 3328 /** 3329 * The actual objects that are the target of the reference (Completed or 3330 * terminated care plan whose function is taken by this new care plan.) 3331 */ 3332 protected List<CarePlan> replacesTarget; 3333 3334 /** 3335 * A larger care plan of which this particular care plan is a component or step. 3336 */ 3337 @Child(name = "partOf", type = { 3338 CarePlan.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3339 @Description(shortDefinition = "Part of referenced CarePlan", formalDefinition = "A larger care plan of which this particular care plan is a component or step.") 3340 protected List<Reference> partOf; 3341 /** 3342 * The actual objects that are the target of the reference (A larger care plan 3343 * of which this particular care plan is a component or step.) 3344 */ 3345 protected List<CarePlan> partOfTarget; 3346 3347 /** 3348 * Indicates whether the plan is currently being acted upon, represents future 3349 * intentions or is now a historical record. 3350 */ 3351 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 3352 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.") 3353 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 3354 protected Enumeration<CarePlanStatus> status; 3355 3356 /** 3357 * Indicates the level of authority/intentionality associated with the care plan 3358 * and where the care plan fits into the workflow chain. 3359 */ 3360 @Child(name = "intent", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = true, summary = true) 3361 @Description(shortDefinition = "proposal | plan | order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.") 3362 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-intent") 3363 protected Enumeration<CarePlanIntent> intent; 3364 3365 /** 3366 * Identifies what "kind" of plan this is to support differentiation between 3367 * multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", 3368 * "disease management", "wellness plan", etc. 3369 */ 3370 @Child(name = "category", type = { 3371 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3372 @Description(shortDefinition = "Type of plan", formalDefinition = "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.") 3373 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-plan-category") 3374 protected List<CodeableConcept> category; 3375 3376 /** 3377 * Human-friendly name for the care plan. 3378 */ 3379 @Child(name = "title", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3380 @Description(shortDefinition = "Human-friendly name for the care plan", formalDefinition = "Human-friendly name for the care plan.") 3381 protected StringType title; 3382 3383 /** 3384 * A description of the scope and nature of the plan. 3385 */ 3386 @Child(name = "description", type = { 3387 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 3388 @Description(shortDefinition = "Summary of nature of plan", formalDefinition = "A description of the scope and nature of the plan.") 3389 protected StringType description; 3390 3391 /** 3392 * Identifies the patient or group whose intended care is described by the plan. 3393 */ 3394 @Child(name = "subject", type = { Patient.class, 3395 Group.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 3396 @Description(shortDefinition = "Who the care plan is for", formalDefinition = "Identifies the patient or group whose intended care is described by the plan.") 3397 protected Reference subject; 3398 3399 /** 3400 * The actual object that is the target of the reference (Identifies the patient 3401 * or group whose intended care is described by the plan.) 3402 */ 3403 protected Resource subjectTarget; 3404 3405 /** 3406 * The Encounter during which this CarePlan was created or to which the creation 3407 * of this record is tightly associated. 3408 */ 3409 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 3410 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.") 3411 protected Reference encounter; 3412 3413 /** 3414 * The actual object that is the target of the reference (The Encounter during 3415 * which this CarePlan was created or to which the creation of this record is 3416 * tightly associated.) 3417 */ 3418 protected Encounter encounterTarget; 3419 3420 /** 3421 * Indicates when the plan did (or is intended to) come into effect and end. 3422 */ 3423 @Child(name = "period", type = { Period.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 3424 @Description(shortDefinition = "Time period plan covers", formalDefinition = "Indicates when the plan did (or is intended to) come into effect and end.") 3425 protected Period period; 3426 3427 /** 3428 * Represents when this particular CarePlan record was created in the system, 3429 * which is often a system-generated date. 3430 */ 3431 @Child(name = "created", type = { 3432 DateTimeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 3433 @Description(shortDefinition = "Date record was first recorded", formalDefinition = "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.") 3434 protected DateTimeType created; 3435 3436 /** 3437 * When populated, the author is responsible for the care plan. The care plan is 3438 * attributed to the author. 3439 */ 3440 @Child(name = "author", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 3441 RelatedPerson.class, Organization.class, 3442 CareTeam.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 3443 @Description(shortDefinition = "Who is the designated responsible party", formalDefinition = "When populated, the author is responsible for the care plan. The care plan is attributed to the author.") 3444 protected Reference author; 3445 3446 /** 3447 * The actual object that is the target of the reference (When populated, the 3448 * author is responsible for the care plan. The care plan is attributed to the 3449 * author.) 3450 */ 3451 protected Resource authorTarget; 3452 3453 /** 3454 * Identifies the individual(s) or organization who provided the contents of the 3455 * care plan. 3456 */ 3457 @Child(name = "contributor", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 3458 RelatedPerson.class, Organization.class, 3459 CareTeam.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3460 @Description(shortDefinition = "Who provided the content of the care plan", formalDefinition = "Identifies the individual(s) or organization who provided the contents of the care plan.") 3461 protected List<Reference> contributor; 3462 /** 3463 * The actual objects that are the target of the reference (Identifies the 3464 * individual(s) or organization who provided the contents of the care plan.) 3465 */ 3466 protected List<Resource> contributorTarget; 3467 3468 /** 3469 * Identifies all people and organizations who are expected to be involved in 3470 * the care envisioned by this plan. 3471 */ 3472 @Child(name = "careTeam", type = { 3473 CareTeam.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3474 @Description(shortDefinition = "Who's involved in plan?", formalDefinition = "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.") 3475 protected List<Reference> careTeam; 3476 /** 3477 * The actual objects that are the target of the reference (Identifies all 3478 * people and organizations who are expected to be involved in the care 3479 * envisioned by this plan.) 3480 */ 3481 protected List<CareTeam> careTeamTarget; 3482 3483 /** 3484 * Identifies the conditions/problems/concerns/diagnoses/etc. whose management 3485 * and/or mitigation are handled by this plan. 3486 */ 3487 @Child(name = "addresses", type = { 3488 Condition.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3489 @Description(shortDefinition = "Health issues this plan addresses", formalDefinition = "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.") 3490 protected List<Reference> addresses; 3491 /** 3492 * The actual objects that are the target of the reference (Identifies the 3493 * conditions/problems/concerns/diagnoses/etc. whose management and/or 3494 * mitigation are handled by this plan.) 3495 */ 3496 protected List<Condition> addressesTarget; 3497 3498 /** 3499 * Identifies portions of the patient's record that specifically influenced the 3500 * formation of the plan. These might include comorbidities, recent procedures, 3501 * limitations, recent assessments, etc. 3502 */ 3503 @Child(name = "supportingInfo", type = { 3504 Reference.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3505 @Description(shortDefinition = "Information considered as part of plan", formalDefinition = "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.") 3506 protected List<Reference> supportingInfo; 3507 /** 3508 * The actual objects that are the target of the reference (Identifies portions 3509 * of the patient's record that specifically influenced the formation of the 3510 * plan. These might include comorbidities, recent procedures, limitations, 3511 * recent assessments, etc.) 3512 */ 3513 protected List<Resource> supportingInfoTarget; 3514 3515 /** 3516 * Describes the intended objective(s) of carrying out the care plan. 3517 */ 3518 @Child(name = "goal", type = { 3519 Goal.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3520 @Description(shortDefinition = "Desired outcome of plan", formalDefinition = "Describes the intended objective(s) of carrying out the care plan.") 3521 protected List<Reference> goal; 3522 /** 3523 * The actual objects that are the target of the reference (Describes the 3524 * intended objective(s) of carrying out the care plan.) 3525 */ 3526 protected List<Goal> goalTarget; 3527 3528 /** 3529 * Identifies a planned action to occur as part of the plan. For example, a 3530 * medication to be used, lab tests to perform, self-monitoring, education, etc. 3531 */ 3532 @Child(name = "activity", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3533 @Description(shortDefinition = "Action to occur as part of plan", formalDefinition = "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.") 3534 protected List<CarePlanActivityComponent> activity; 3535 3536 /** 3537 * General notes about the care plan not covered elsewhere. 3538 */ 3539 @Child(name = "note", type = { 3540 Annotation.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3541 @Description(shortDefinition = "Comments about the plan", formalDefinition = "General notes about the care plan not covered elsewhere.") 3542 protected List<Annotation> note; 3543 3544 private static final long serialVersionUID = -584930613L; 3545 3546 /** 3547 * Constructor 3548 */ 3549 public CarePlan() { 3550 super(); 3551 } 3552 3553 /** 3554 * Constructor 3555 */ 3556 public CarePlan(Enumeration<CarePlanStatus> status, Enumeration<CarePlanIntent> intent, Reference subject) { 3557 super(); 3558 this.status = status; 3559 this.intent = intent; 3560 this.subject = subject; 3561 } 3562 3563 /** 3564 * @return {@link #identifier} (Business identifiers assigned to this care plan 3565 * by the performer or other systems which remain constant as the 3566 * resource is updated and propagates from server to server.) 3567 */ 3568 public List<Identifier> getIdentifier() { 3569 if (this.identifier == null) 3570 this.identifier = new ArrayList<Identifier>(); 3571 return this.identifier; 3572 } 3573 3574 /** 3575 * @return Returns a reference to <code>this</code> for easy method chaining 3576 */ 3577 public CarePlan setIdentifier(List<Identifier> theIdentifier) { 3578 this.identifier = theIdentifier; 3579 return this; 3580 } 3581 3582 public boolean hasIdentifier() { 3583 if (this.identifier == null) 3584 return false; 3585 for (Identifier item : this.identifier) 3586 if (!item.isEmpty()) 3587 return true; 3588 return false; 3589 } 3590 3591 public Identifier addIdentifier() { // 3 3592 Identifier t = new Identifier(); 3593 if (this.identifier == null) 3594 this.identifier = new ArrayList<Identifier>(); 3595 this.identifier.add(t); 3596 return t; 3597 } 3598 3599 public CarePlan addIdentifier(Identifier t) { // 3 3600 if (t == null) 3601 return this; 3602 if (this.identifier == null) 3603 this.identifier = new ArrayList<Identifier>(); 3604 this.identifier.add(t); 3605 return this; 3606 } 3607 3608 /** 3609 * @return The first repetition of repeating field {@link #identifier}, creating 3610 * it if it does not already exist 3611 */ 3612 public Identifier getIdentifierFirstRep() { 3613 if (getIdentifier().isEmpty()) { 3614 addIdentifier(); 3615 } 3616 return getIdentifier().get(0); 3617 } 3618 3619 /** 3620 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 3621 * protocol, guideline, questionnaire or other definition that is 3622 * adhered to in whole or in part by this CarePlan.) 3623 */ 3624 public List<CanonicalType> getInstantiatesCanonical() { 3625 if (this.instantiatesCanonical == null) 3626 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3627 return this.instantiatesCanonical; 3628 } 3629 3630 /** 3631 * @return Returns a reference to <code>this</code> for easy method chaining 3632 */ 3633 public CarePlan setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 3634 this.instantiatesCanonical = theInstantiatesCanonical; 3635 return this; 3636 } 3637 3638 public boolean hasInstantiatesCanonical() { 3639 if (this.instantiatesCanonical == null) 3640 return false; 3641 for (CanonicalType item : this.instantiatesCanonical) 3642 if (!item.isEmpty()) 3643 return true; 3644 return false; 3645 } 3646 3647 /** 3648 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 3649 * protocol, guideline, questionnaire or other definition that is 3650 * adhered to in whole or in part by this CarePlan.) 3651 */ 3652 public CanonicalType addInstantiatesCanonicalElement() {// 2 3653 CanonicalType t = new CanonicalType(); 3654 if (this.instantiatesCanonical == null) 3655 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3656 this.instantiatesCanonical.add(t); 3657 return t; 3658 } 3659 3660 /** 3661 * @param value {@link #instantiatesCanonical} (The URL pointing to a 3662 * FHIR-defined protocol, guideline, questionnaire or other 3663 * definition that is adhered to in whole or in part by this 3664 * CarePlan.) 3665 */ 3666 public CarePlan addInstantiatesCanonical(String value) { // 1 3667 CanonicalType t = new CanonicalType(); 3668 t.setValue(value); 3669 if (this.instantiatesCanonical == null) 3670 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3671 this.instantiatesCanonical.add(t); 3672 return this; 3673 } 3674 3675 /** 3676 * @param value {@link #instantiatesCanonical} (The URL pointing to a 3677 * FHIR-defined protocol, guideline, questionnaire or other 3678 * definition that is adhered to in whole or in part by this 3679 * CarePlan.) 3680 */ 3681 public boolean hasInstantiatesCanonical(String value) { 3682 if (this.instantiatesCanonical == null) 3683 return false; 3684 for (CanonicalType v : this.instantiatesCanonical) 3685 if (v.getValue().equals(value)) // canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition) 3686 return true; 3687 return false; 3688 } 3689 3690 /** 3691 * @return {@link #instantiatesUri} (The URL pointing to an externally 3692 * maintained protocol, guideline, questionnaire or other definition 3693 * that is adhered to in whole or in part by this CarePlan.) 3694 */ 3695 public List<UriType> getInstantiatesUri() { 3696 if (this.instantiatesUri == null) 3697 this.instantiatesUri = new ArrayList<UriType>(); 3698 return this.instantiatesUri; 3699 } 3700 3701 /** 3702 * @return Returns a reference to <code>this</code> for easy method chaining 3703 */ 3704 public CarePlan setInstantiatesUri(List<UriType> theInstantiatesUri) { 3705 this.instantiatesUri = theInstantiatesUri; 3706 return this; 3707 } 3708 3709 public boolean hasInstantiatesUri() { 3710 if (this.instantiatesUri == null) 3711 return false; 3712 for (UriType item : this.instantiatesUri) 3713 if (!item.isEmpty()) 3714 return true; 3715 return false; 3716 } 3717 3718 /** 3719 * @return {@link #instantiatesUri} (The URL pointing to an externally 3720 * maintained protocol, guideline, questionnaire or other definition 3721 * that is adhered to in whole or in part by this CarePlan.) 3722 */ 3723 public UriType addInstantiatesUriElement() {// 2 3724 UriType t = new UriType(); 3725 if (this.instantiatesUri == null) 3726 this.instantiatesUri = new ArrayList<UriType>(); 3727 this.instantiatesUri.add(t); 3728 return t; 3729 } 3730 3731 /** 3732 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3733 * maintained protocol, guideline, questionnaire or other 3734 * definition that is adhered to in whole or in part by this 3735 * CarePlan.) 3736 */ 3737 public CarePlan addInstantiatesUri(String value) { // 1 3738 UriType t = new UriType(); 3739 t.setValue(value); 3740 if (this.instantiatesUri == null) 3741 this.instantiatesUri = new ArrayList<UriType>(); 3742 this.instantiatesUri.add(t); 3743 return this; 3744 } 3745 3746 /** 3747 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3748 * maintained protocol, guideline, questionnaire or other 3749 * definition that is adhered to in whole or in part by this 3750 * CarePlan.) 3751 */ 3752 public boolean hasInstantiatesUri(String value) { 3753 if (this.instantiatesUri == null) 3754 return false; 3755 for (UriType v : this.instantiatesUri) 3756 if (v.getValue().equals(value)) // uri 3757 return true; 3758 return false; 3759 } 3760 3761 /** 3762 * @return {@link #basedOn} (A care plan that is fulfilled in whole or in part 3763 * by this care plan.) 3764 */ 3765 public List<Reference> getBasedOn() { 3766 if (this.basedOn == null) 3767 this.basedOn = new ArrayList<Reference>(); 3768 return this.basedOn; 3769 } 3770 3771 /** 3772 * @return Returns a reference to <code>this</code> for easy method chaining 3773 */ 3774 public CarePlan setBasedOn(List<Reference> theBasedOn) { 3775 this.basedOn = theBasedOn; 3776 return this; 3777 } 3778 3779 public boolean hasBasedOn() { 3780 if (this.basedOn == null) 3781 return false; 3782 for (Reference item : this.basedOn) 3783 if (!item.isEmpty()) 3784 return true; 3785 return false; 3786 } 3787 3788 public Reference addBasedOn() { // 3 3789 Reference t = new Reference(); 3790 if (this.basedOn == null) 3791 this.basedOn = new ArrayList<Reference>(); 3792 this.basedOn.add(t); 3793 return t; 3794 } 3795 3796 public CarePlan addBasedOn(Reference t) { // 3 3797 if (t == null) 3798 return this; 3799 if (this.basedOn == null) 3800 this.basedOn = new ArrayList<Reference>(); 3801 this.basedOn.add(t); 3802 return this; 3803 } 3804 3805 /** 3806 * @return The first repetition of repeating field {@link #basedOn}, creating it 3807 * if it does not already exist 3808 */ 3809 public Reference getBasedOnFirstRep() { 3810 if (getBasedOn().isEmpty()) { 3811 addBasedOn(); 3812 } 3813 return getBasedOn().get(0); 3814 } 3815 3816 /** 3817 * @deprecated Use Reference#setResource(IBaseResource) instead 3818 */ 3819 @Deprecated 3820 public List<CarePlan> getBasedOnTarget() { 3821 if (this.basedOnTarget == null) 3822 this.basedOnTarget = new ArrayList<CarePlan>(); 3823 return this.basedOnTarget; 3824 } 3825 3826 /** 3827 * @deprecated Use Reference#setResource(IBaseResource) instead 3828 */ 3829 @Deprecated 3830 public CarePlan addBasedOnTarget() { 3831 CarePlan r = new CarePlan(); 3832 if (this.basedOnTarget == null) 3833 this.basedOnTarget = new ArrayList<CarePlan>(); 3834 this.basedOnTarget.add(r); 3835 return r; 3836 } 3837 3838 /** 3839 * @return {@link #replaces} (Completed or terminated care plan whose function 3840 * is taken by this new care plan.) 3841 */ 3842 public List<Reference> getReplaces() { 3843 if (this.replaces == null) 3844 this.replaces = new ArrayList<Reference>(); 3845 return this.replaces; 3846 } 3847 3848 /** 3849 * @return Returns a reference to <code>this</code> for easy method chaining 3850 */ 3851 public CarePlan setReplaces(List<Reference> theReplaces) { 3852 this.replaces = theReplaces; 3853 return this; 3854 } 3855 3856 public boolean hasReplaces() { 3857 if (this.replaces == null) 3858 return false; 3859 for (Reference item : this.replaces) 3860 if (!item.isEmpty()) 3861 return true; 3862 return false; 3863 } 3864 3865 public Reference addReplaces() { // 3 3866 Reference t = new Reference(); 3867 if (this.replaces == null) 3868 this.replaces = new ArrayList<Reference>(); 3869 this.replaces.add(t); 3870 return t; 3871 } 3872 3873 public CarePlan addReplaces(Reference t) { // 3 3874 if (t == null) 3875 return this; 3876 if (this.replaces == null) 3877 this.replaces = new ArrayList<Reference>(); 3878 this.replaces.add(t); 3879 return this; 3880 } 3881 3882 /** 3883 * @return The first repetition of repeating field {@link #replaces}, creating 3884 * it if it does not already exist 3885 */ 3886 public Reference getReplacesFirstRep() { 3887 if (getReplaces().isEmpty()) { 3888 addReplaces(); 3889 } 3890 return getReplaces().get(0); 3891 } 3892 3893 /** 3894 * @deprecated Use Reference#setResource(IBaseResource) instead 3895 */ 3896 @Deprecated 3897 public List<CarePlan> getReplacesTarget() { 3898 if (this.replacesTarget == null) 3899 this.replacesTarget = new ArrayList<CarePlan>(); 3900 return this.replacesTarget; 3901 } 3902 3903 /** 3904 * @deprecated Use Reference#setResource(IBaseResource) instead 3905 */ 3906 @Deprecated 3907 public CarePlan addReplacesTarget() { 3908 CarePlan r = new CarePlan(); 3909 if (this.replacesTarget == null) 3910 this.replacesTarget = new ArrayList<CarePlan>(); 3911 this.replacesTarget.add(r); 3912 return r; 3913 } 3914 3915 /** 3916 * @return {@link #partOf} (A larger care plan of which this particular care 3917 * plan is a component or step.) 3918 */ 3919 public List<Reference> getPartOf() { 3920 if (this.partOf == null) 3921 this.partOf = new ArrayList<Reference>(); 3922 return this.partOf; 3923 } 3924 3925 /** 3926 * @return Returns a reference to <code>this</code> for easy method chaining 3927 */ 3928 public CarePlan setPartOf(List<Reference> thePartOf) { 3929 this.partOf = thePartOf; 3930 return this; 3931 } 3932 3933 public boolean hasPartOf() { 3934 if (this.partOf == null) 3935 return false; 3936 for (Reference item : this.partOf) 3937 if (!item.isEmpty()) 3938 return true; 3939 return false; 3940 } 3941 3942 public Reference addPartOf() { // 3 3943 Reference t = new Reference(); 3944 if (this.partOf == null) 3945 this.partOf = new ArrayList<Reference>(); 3946 this.partOf.add(t); 3947 return t; 3948 } 3949 3950 public CarePlan addPartOf(Reference t) { // 3 3951 if (t == null) 3952 return this; 3953 if (this.partOf == null) 3954 this.partOf = new ArrayList<Reference>(); 3955 this.partOf.add(t); 3956 return this; 3957 } 3958 3959 /** 3960 * @return The first repetition of repeating field {@link #partOf}, creating it 3961 * if it does not already exist 3962 */ 3963 public Reference getPartOfFirstRep() { 3964 if (getPartOf().isEmpty()) { 3965 addPartOf(); 3966 } 3967 return getPartOf().get(0); 3968 } 3969 3970 /** 3971 * @deprecated Use Reference#setResource(IBaseResource) instead 3972 */ 3973 @Deprecated 3974 public List<CarePlan> getPartOfTarget() { 3975 if (this.partOfTarget == null) 3976 this.partOfTarget = new ArrayList<CarePlan>(); 3977 return this.partOfTarget; 3978 } 3979 3980 /** 3981 * @deprecated Use Reference#setResource(IBaseResource) instead 3982 */ 3983 @Deprecated 3984 public CarePlan addPartOfTarget() { 3985 CarePlan r = new CarePlan(); 3986 if (this.partOfTarget == null) 3987 this.partOfTarget = new ArrayList<CarePlan>(); 3988 this.partOfTarget.add(r); 3989 return r; 3990 } 3991 3992 /** 3993 * @return {@link #status} (Indicates whether the plan is currently being acted 3994 * upon, represents future intentions or is now a historical record.). 3995 * This is the underlying object with id, value and extensions. The 3996 * accessor "getStatus" gives direct access to the value 3997 */ 3998 public Enumeration<CarePlanStatus> getStatusElement() { 3999 if (this.status == null) 4000 if (Configuration.errorOnAutoCreate()) 4001 throw new Error("Attempt to auto-create CarePlan.status"); 4002 else if (Configuration.doAutoCreate()) 4003 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); // bb 4004 return this.status; 4005 } 4006 4007 public boolean hasStatusElement() { 4008 return this.status != null && !this.status.isEmpty(); 4009 } 4010 4011 public boolean hasStatus() { 4012 return this.status != null && !this.status.isEmpty(); 4013 } 4014 4015 /** 4016 * @param value {@link #status} (Indicates whether the plan is currently being 4017 * acted upon, represents future intentions or is now a historical 4018 * record.). This is the underlying object with id, value and 4019 * extensions. The accessor "getStatus" gives direct access to the 4020 * value 4021 */ 4022 public CarePlan setStatusElement(Enumeration<CarePlanStatus> value) { 4023 this.status = value; 4024 return this; 4025 } 4026 4027 /** 4028 * @return Indicates whether the plan is currently being acted upon, represents 4029 * future intentions or is now a historical record. 4030 */ 4031 public CarePlanStatus getStatus() { 4032 return this.status == null ? null : this.status.getValue(); 4033 } 4034 4035 /** 4036 * @param value Indicates whether the plan is currently being acted upon, 4037 * represents future intentions or is now a historical record. 4038 */ 4039 public CarePlan setStatus(CarePlanStatus value) { 4040 if (this.status == null) 4041 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); 4042 this.status.setValue(value); 4043 return this; 4044 } 4045 4046 /** 4047 * @return {@link #intent} (Indicates the level of authority/intentionality 4048 * associated with the care plan and where the care plan fits into the 4049 * workflow chain.). This is the underlying object with id, value and 4050 * extensions. The accessor "getIntent" gives direct access to the value 4051 */ 4052 public Enumeration<CarePlanIntent> getIntentElement() { 4053 if (this.intent == null) 4054 if (Configuration.errorOnAutoCreate()) 4055 throw new Error("Attempt to auto-create CarePlan.intent"); 4056 else if (Configuration.doAutoCreate()) 4057 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); // bb 4058 return this.intent; 4059 } 4060 4061 public boolean hasIntentElement() { 4062 return this.intent != null && !this.intent.isEmpty(); 4063 } 4064 4065 public boolean hasIntent() { 4066 return this.intent != null && !this.intent.isEmpty(); 4067 } 4068 4069 /** 4070 * @param value {@link #intent} (Indicates the level of authority/intentionality 4071 * associated with the care plan and where the care plan fits into 4072 * the workflow chain.). This is the underlying object with id, 4073 * value and extensions. The accessor "getIntent" gives direct 4074 * access to the value 4075 */ 4076 public CarePlan setIntentElement(Enumeration<CarePlanIntent> value) { 4077 this.intent = value; 4078 return this; 4079 } 4080 4081 /** 4082 * @return Indicates the level of authority/intentionality associated with the 4083 * care plan and where the care plan fits into the workflow chain. 4084 */ 4085 public CarePlanIntent getIntent() { 4086 return this.intent == null ? null : this.intent.getValue(); 4087 } 4088 4089 /** 4090 * @param value Indicates the level of authority/intentionality associated with 4091 * the care plan and where the care plan fits into the workflow 4092 * chain. 4093 */ 4094 public CarePlan setIntent(CarePlanIntent value) { 4095 if (this.intent == null) 4096 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); 4097 this.intent.setValue(value); 4098 return this; 4099 } 4100 4101 /** 4102 * @return {@link #category} (Identifies what "kind" of plan this is to support 4103 * differentiation between multiple co-existing plans; e.g. "Home 4104 * health", "psychiatric", "asthma", "disease management", "wellness 4105 * plan", etc.) 4106 */ 4107 public List<CodeableConcept> getCategory() { 4108 if (this.category == null) 4109 this.category = new ArrayList<CodeableConcept>(); 4110 return this.category; 4111 } 4112 4113 /** 4114 * @return Returns a reference to <code>this</code> for easy method chaining 4115 */ 4116 public CarePlan setCategory(List<CodeableConcept> theCategory) { 4117 this.category = theCategory; 4118 return this; 4119 } 4120 4121 public boolean hasCategory() { 4122 if (this.category == null) 4123 return false; 4124 for (CodeableConcept item : this.category) 4125 if (!item.isEmpty()) 4126 return true; 4127 return false; 4128 } 4129 4130 public CodeableConcept addCategory() { // 3 4131 CodeableConcept t = new CodeableConcept(); 4132 if (this.category == null) 4133 this.category = new ArrayList<CodeableConcept>(); 4134 this.category.add(t); 4135 return t; 4136 } 4137 4138 public CarePlan addCategory(CodeableConcept t) { // 3 4139 if (t == null) 4140 return this; 4141 if (this.category == null) 4142 this.category = new ArrayList<CodeableConcept>(); 4143 this.category.add(t); 4144 return this; 4145 } 4146 4147 /** 4148 * @return The first repetition of repeating field {@link #category}, creating 4149 * it if it does not already exist 4150 */ 4151 public CodeableConcept getCategoryFirstRep() { 4152 if (getCategory().isEmpty()) { 4153 addCategory(); 4154 } 4155 return getCategory().get(0); 4156 } 4157 4158 /** 4159 * @return {@link #title} (Human-friendly name for the care plan.). This is the 4160 * underlying object with id, value and extensions. The accessor 4161 * "getTitle" gives direct access to the value 4162 */ 4163 public StringType getTitleElement() { 4164 if (this.title == null) 4165 if (Configuration.errorOnAutoCreate()) 4166 throw new Error("Attempt to auto-create CarePlan.title"); 4167 else if (Configuration.doAutoCreate()) 4168 this.title = new StringType(); // bb 4169 return this.title; 4170 } 4171 4172 public boolean hasTitleElement() { 4173 return this.title != null && !this.title.isEmpty(); 4174 } 4175 4176 public boolean hasTitle() { 4177 return this.title != null && !this.title.isEmpty(); 4178 } 4179 4180 /** 4181 * @param value {@link #title} (Human-friendly name for the care plan.). This is 4182 * the underlying object with id, value and extensions. The 4183 * accessor "getTitle" gives direct access to the value 4184 */ 4185 public CarePlan setTitleElement(StringType value) { 4186 this.title = value; 4187 return this; 4188 } 4189 4190 /** 4191 * @return Human-friendly name for the care plan. 4192 */ 4193 public String getTitle() { 4194 return this.title == null ? null : this.title.getValue(); 4195 } 4196 4197 /** 4198 * @param value Human-friendly name for the care plan. 4199 */ 4200 public CarePlan setTitle(String value) { 4201 if (Utilities.noString(value)) 4202 this.title = null; 4203 else { 4204 if (this.title == null) 4205 this.title = new StringType(); 4206 this.title.setValue(value); 4207 } 4208 return this; 4209 } 4210 4211 /** 4212 * @return {@link #description} (A description of the scope and nature of the 4213 * plan.). This is the underlying object with id, value and extensions. 4214 * The accessor "getDescription" gives direct access to the value 4215 */ 4216 public StringType getDescriptionElement() { 4217 if (this.description == null) 4218 if (Configuration.errorOnAutoCreate()) 4219 throw new Error("Attempt to auto-create CarePlan.description"); 4220 else if (Configuration.doAutoCreate()) 4221 this.description = new StringType(); // bb 4222 return this.description; 4223 } 4224 4225 public boolean hasDescriptionElement() { 4226 return this.description != null && !this.description.isEmpty(); 4227 } 4228 4229 public boolean hasDescription() { 4230 return this.description != null && !this.description.isEmpty(); 4231 } 4232 4233 /** 4234 * @param value {@link #description} (A description of the scope and nature of 4235 * the plan.). This is the underlying object with id, value and 4236 * extensions. The accessor "getDescription" gives direct access to 4237 * the value 4238 */ 4239 public CarePlan setDescriptionElement(StringType value) { 4240 this.description = value; 4241 return this; 4242 } 4243 4244 /** 4245 * @return A description of the scope and nature of the plan. 4246 */ 4247 public String getDescription() { 4248 return this.description == null ? null : this.description.getValue(); 4249 } 4250 4251 /** 4252 * @param value A description of the scope and nature of the plan. 4253 */ 4254 public CarePlan setDescription(String value) { 4255 if (Utilities.noString(value)) 4256 this.description = null; 4257 else { 4258 if (this.description == null) 4259 this.description = new StringType(); 4260 this.description.setValue(value); 4261 } 4262 return this; 4263 } 4264 4265 /** 4266 * @return {@link #subject} (Identifies the patient or group whose intended care 4267 * is described by the plan.) 4268 */ 4269 public Reference getSubject() { 4270 if (this.subject == null) 4271 if (Configuration.errorOnAutoCreate()) 4272 throw new Error("Attempt to auto-create CarePlan.subject"); 4273 else if (Configuration.doAutoCreate()) 4274 this.subject = new Reference(); // cc 4275 return this.subject; 4276 } 4277 4278 public boolean hasSubject() { 4279 return this.subject != null && !this.subject.isEmpty(); 4280 } 4281 4282 /** 4283 * @param value {@link #subject} (Identifies the patient or group whose intended 4284 * care is described by the plan.) 4285 */ 4286 public CarePlan setSubject(Reference value) { 4287 this.subject = value; 4288 return this; 4289 } 4290 4291 /** 4292 * @return {@link #subject} The actual object that is the target of the 4293 * reference. The reference library doesn't populate this, but you can 4294 * use it to hold the resource if you resolve it. (Identifies the 4295 * patient or group whose intended care is described by the plan.) 4296 */ 4297 public Resource getSubjectTarget() { 4298 return this.subjectTarget; 4299 } 4300 4301 /** 4302 * @param value {@link #subject} The actual object that is the target of the 4303 * reference. The reference library doesn't use these, but you can 4304 * use it to hold the resource if you resolve it. (Identifies the 4305 * patient or group whose intended care is described by the plan.) 4306 */ 4307 public CarePlan setSubjectTarget(Resource value) { 4308 this.subjectTarget = value; 4309 return this; 4310 } 4311 4312 /** 4313 * @return {@link #encounter} (The Encounter during which this CarePlan was 4314 * created or to which the creation of this record is tightly 4315 * associated.) 4316 */ 4317 public Reference getEncounter() { 4318 if (this.encounter == null) 4319 if (Configuration.errorOnAutoCreate()) 4320 throw new Error("Attempt to auto-create CarePlan.encounter"); 4321 else if (Configuration.doAutoCreate()) 4322 this.encounter = new Reference(); // cc 4323 return this.encounter; 4324 } 4325 4326 public boolean hasEncounter() { 4327 return this.encounter != null && !this.encounter.isEmpty(); 4328 } 4329 4330 /** 4331 * @param value {@link #encounter} (The Encounter during which this CarePlan was 4332 * created or to which the creation of this record is tightly 4333 * associated.) 4334 */ 4335 public CarePlan setEncounter(Reference value) { 4336 this.encounter = value; 4337 return this; 4338 } 4339 4340 /** 4341 * @return {@link #encounter} The actual object that is the target of the 4342 * reference. The reference library doesn't populate this, but you can 4343 * use it to hold the resource if you resolve it. (The Encounter during 4344 * which this CarePlan was created or to which the creation of this 4345 * record is tightly associated.) 4346 */ 4347 public Encounter getEncounterTarget() { 4348 if (this.encounterTarget == null) 4349 if (Configuration.errorOnAutoCreate()) 4350 throw new Error("Attempt to auto-create CarePlan.encounter"); 4351 else if (Configuration.doAutoCreate()) 4352 this.encounterTarget = new Encounter(); // aa 4353 return this.encounterTarget; 4354 } 4355 4356 /** 4357 * @param value {@link #encounter} The actual object that is the target of the 4358 * reference. The reference library doesn't use these, but you can 4359 * use it to hold the resource if you resolve it. (The Encounter 4360 * during which this CarePlan was created or to which the creation 4361 * of this record is tightly associated.) 4362 */ 4363 public CarePlan setEncounterTarget(Encounter value) { 4364 this.encounterTarget = value; 4365 return this; 4366 } 4367 4368 /** 4369 * @return {@link #period} (Indicates when the plan did (or is intended to) come 4370 * into effect and end.) 4371 */ 4372 public Period getPeriod() { 4373 if (this.period == null) 4374 if (Configuration.errorOnAutoCreate()) 4375 throw new Error("Attempt to auto-create CarePlan.period"); 4376 else if (Configuration.doAutoCreate()) 4377 this.period = new Period(); // cc 4378 return this.period; 4379 } 4380 4381 public boolean hasPeriod() { 4382 return this.period != null && !this.period.isEmpty(); 4383 } 4384 4385 /** 4386 * @param value {@link #period} (Indicates when the plan did (or is intended to) 4387 * come into effect and end.) 4388 */ 4389 public CarePlan setPeriod(Period value) { 4390 this.period = value; 4391 return this; 4392 } 4393 4394 /** 4395 * @return {@link #created} (Represents when this particular CarePlan record was 4396 * created in the system, which is often a system-generated date.). This 4397 * is the underlying object with id, value and extensions. The accessor 4398 * "getCreated" gives direct access to the value 4399 */ 4400 public DateTimeType getCreatedElement() { 4401 if (this.created == null) 4402 if (Configuration.errorOnAutoCreate()) 4403 throw new Error("Attempt to auto-create CarePlan.created"); 4404 else if (Configuration.doAutoCreate()) 4405 this.created = new DateTimeType(); // bb 4406 return this.created; 4407 } 4408 4409 public boolean hasCreatedElement() { 4410 return this.created != null && !this.created.isEmpty(); 4411 } 4412 4413 public boolean hasCreated() { 4414 return this.created != null && !this.created.isEmpty(); 4415 } 4416 4417 /** 4418 * @param value {@link #created} (Represents when this particular CarePlan 4419 * record was created in the system, which is often a 4420 * system-generated date.). This is the underlying object with id, 4421 * value and extensions. The accessor "getCreated" gives direct 4422 * access to the value 4423 */ 4424 public CarePlan setCreatedElement(DateTimeType value) { 4425 this.created = value; 4426 return this; 4427 } 4428 4429 /** 4430 * @return Represents when this particular CarePlan record was created in the 4431 * system, which is often a system-generated date. 4432 */ 4433 public Date getCreated() { 4434 return this.created == null ? null : this.created.getValue(); 4435 } 4436 4437 /** 4438 * @param value Represents when this particular CarePlan record was created in 4439 * the system, which is often a system-generated date. 4440 */ 4441 public CarePlan setCreated(Date value) { 4442 if (value == null) 4443 this.created = null; 4444 else { 4445 if (this.created == null) 4446 this.created = new DateTimeType(); 4447 this.created.setValue(value); 4448 } 4449 return this; 4450 } 4451 4452 /** 4453 * @return {@link #author} (When populated, the author is responsible for the 4454 * care plan. The care plan is attributed to the author.) 4455 */ 4456 public Reference getAuthor() { 4457 if (this.author == null) 4458 if (Configuration.errorOnAutoCreate()) 4459 throw new Error("Attempt to auto-create CarePlan.author"); 4460 else if (Configuration.doAutoCreate()) 4461 this.author = new Reference(); // cc 4462 return this.author; 4463 } 4464 4465 public boolean hasAuthor() { 4466 return this.author != null && !this.author.isEmpty(); 4467 } 4468 4469 /** 4470 * @param value {@link #author} (When populated, the author is responsible for 4471 * the care plan. The care plan is attributed to the author.) 4472 */ 4473 public CarePlan setAuthor(Reference value) { 4474 this.author = value; 4475 return this; 4476 } 4477 4478 /** 4479 * @return {@link #author} The actual object that is the target of the 4480 * reference. The reference library doesn't populate this, but you can 4481 * use it to hold the resource if you resolve it. (When populated, the 4482 * author is responsible for the care plan. The care plan is attributed 4483 * to the author.) 4484 */ 4485 public Resource getAuthorTarget() { 4486 return this.authorTarget; 4487 } 4488 4489 /** 4490 * @param value {@link #author} The actual object that is the target of the 4491 * reference. The reference library doesn't use these, but you can 4492 * use it to hold the resource if you resolve it. (When populated, 4493 * the author is responsible for the care plan. The care plan is 4494 * attributed to the author.) 4495 */ 4496 public CarePlan setAuthorTarget(Resource value) { 4497 this.authorTarget = value; 4498 return this; 4499 } 4500 4501 /** 4502 * @return {@link #contributor} (Identifies the individual(s) or organization 4503 * who provided the contents of the care plan.) 4504 */ 4505 public List<Reference> getContributor() { 4506 if (this.contributor == null) 4507 this.contributor = new ArrayList<Reference>(); 4508 return this.contributor; 4509 } 4510 4511 /** 4512 * @return Returns a reference to <code>this</code> for easy method chaining 4513 */ 4514 public CarePlan setContributor(List<Reference> theContributor) { 4515 this.contributor = theContributor; 4516 return this; 4517 } 4518 4519 public boolean hasContributor() { 4520 if (this.contributor == null) 4521 return false; 4522 for (Reference item : this.contributor) 4523 if (!item.isEmpty()) 4524 return true; 4525 return false; 4526 } 4527 4528 public Reference addContributor() { // 3 4529 Reference t = new Reference(); 4530 if (this.contributor == null) 4531 this.contributor = new ArrayList<Reference>(); 4532 this.contributor.add(t); 4533 return t; 4534 } 4535 4536 public CarePlan addContributor(Reference t) { // 3 4537 if (t == null) 4538 return this; 4539 if (this.contributor == null) 4540 this.contributor = new ArrayList<Reference>(); 4541 this.contributor.add(t); 4542 return this; 4543 } 4544 4545 /** 4546 * @return The first repetition of repeating field {@link #contributor}, 4547 * creating it if it does not already exist 4548 */ 4549 public Reference getContributorFirstRep() { 4550 if (getContributor().isEmpty()) { 4551 addContributor(); 4552 } 4553 return getContributor().get(0); 4554 } 4555 4556 /** 4557 * @deprecated Use Reference#setResource(IBaseResource) instead 4558 */ 4559 @Deprecated 4560 public List<Resource> getContributorTarget() { 4561 if (this.contributorTarget == null) 4562 this.contributorTarget = new ArrayList<Resource>(); 4563 return this.contributorTarget; 4564 } 4565 4566 /** 4567 * @return {@link #careTeam} (Identifies all people and organizations who are 4568 * expected to be involved in the care envisioned by this plan.) 4569 */ 4570 public List<Reference> getCareTeam() { 4571 if (this.careTeam == null) 4572 this.careTeam = new ArrayList<Reference>(); 4573 return this.careTeam; 4574 } 4575 4576 /** 4577 * @return Returns a reference to <code>this</code> for easy method chaining 4578 */ 4579 public CarePlan setCareTeam(List<Reference> theCareTeam) { 4580 this.careTeam = theCareTeam; 4581 return this; 4582 } 4583 4584 public boolean hasCareTeam() { 4585 if (this.careTeam == null) 4586 return false; 4587 for (Reference item : this.careTeam) 4588 if (!item.isEmpty()) 4589 return true; 4590 return false; 4591 } 4592 4593 public Reference addCareTeam() { // 3 4594 Reference t = new Reference(); 4595 if (this.careTeam == null) 4596 this.careTeam = new ArrayList<Reference>(); 4597 this.careTeam.add(t); 4598 return t; 4599 } 4600 4601 public CarePlan addCareTeam(Reference t) { // 3 4602 if (t == null) 4603 return this; 4604 if (this.careTeam == null) 4605 this.careTeam = new ArrayList<Reference>(); 4606 this.careTeam.add(t); 4607 return this; 4608 } 4609 4610 /** 4611 * @return The first repetition of repeating field {@link #careTeam}, creating 4612 * it if it does not already exist 4613 */ 4614 public Reference getCareTeamFirstRep() { 4615 if (getCareTeam().isEmpty()) { 4616 addCareTeam(); 4617 } 4618 return getCareTeam().get(0); 4619 } 4620 4621 /** 4622 * @deprecated Use Reference#setResource(IBaseResource) instead 4623 */ 4624 @Deprecated 4625 public List<CareTeam> getCareTeamTarget() { 4626 if (this.careTeamTarget == null) 4627 this.careTeamTarget = new ArrayList<CareTeam>(); 4628 return this.careTeamTarget; 4629 } 4630 4631 /** 4632 * @deprecated Use Reference#setResource(IBaseResource) instead 4633 */ 4634 @Deprecated 4635 public CareTeam addCareTeamTarget() { 4636 CareTeam r = new CareTeam(); 4637 if (this.careTeamTarget == null) 4638 this.careTeamTarget = new ArrayList<CareTeam>(); 4639 this.careTeamTarget.add(r); 4640 return r; 4641 } 4642 4643 /** 4644 * @return {@link #addresses} (Identifies the 4645 * conditions/problems/concerns/diagnoses/etc. whose management and/or 4646 * mitigation are handled by this plan.) 4647 */ 4648 public List<Reference> getAddresses() { 4649 if (this.addresses == null) 4650 this.addresses = new ArrayList<Reference>(); 4651 return this.addresses; 4652 } 4653 4654 /** 4655 * @return Returns a reference to <code>this</code> for easy method chaining 4656 */ 4657 public CarePlan setAddresses(List<Reference> theAddresses) { 4658 this.addresses = theAddresses; 4659 return this; 4660 } 4661 4662 public boolean hasAddresses() { 4663 if (this.addresses == null) 4664 return false; 4665 for (Reference item : this.addresses) 4666 if (!item.isEmpty()) 4667 return true; 4668 return false; 4669 } 4670 4671 public Reference addAddresses() { // 3 4672 Reference t = new Reference(); 4673 if (this.addresses == null) 4674 this.addresses = new ArrayList<Reference>(); 4675 this.addresses.add(t); 4676 return t; 4677 } 4678 4679 public CarePlan addAddresses(Reference t) { // 3 4680 if (t == null) 4681 return this; 4682 if (this.addresses == null) 4683 this.addresses = new ArrayList<Reference>(); 4684 this.addresses.add(t); 4685 return this; 4686 } 4687 4688 /** 4689 * @return The first repetition of repeating field {@link #addresses}, creating 4690 * it if it does not already exist 4691 */ 4692 public Reference getAddressesFirstRep() { 4693 if (getAddresses().isEmpty()) { 4694 addAddresses(); 4695 } 4696 return getAddresses().get(0); 4697 } 4698 4699 /** 4700 * @deprecated Use Reference#setResource(IBaseResource) instead 4701 */ 4702 @Deprecated 4703 public List<Condition> getAddressesTarget() { 4704 if (this.addressesTarget == null) 4705 this.addressesTarget = new ArrayList<Condition>(); 4706 return this.addressesTarget; 4707 } 4708 4709 /** 4710 * @deprecated Use Reference#setResource(IBaseResource) instead 4711 */ 4712 @Deprecated 4713 public Condition addAddressesTarget() { 4714 Condition r = new Condition(); 4715 if (this.addressesTarget == null) 4716 this.addressesTarget = new ArrayList<Condition>(); 4717 this.addressesTarget.add(r); 4718 return r; 4719 } 4720 4721 /** 4722 * @return {@link #supportingInfo} (Identifies portions of the patient's record 4723 * that specifically influenced the formation of the plan. These might 4724 * include comorbidities, recent procedures, limitations, recent 4725 * assessments, etc.) 4726 */ 4727 public List<Reference> getSupportingInfo() { 4728 if (this.supportingInfo == null) 4729 this.supportingInfo = new ArrayList<Reference>(); 4730 return this.supportingInfo; 4731 } 4732 4733 /** 4734 * @return Returns a reference to <code>this</code> for easy method chaining 4735 */ 4736 public CarePlan setSupportingInfo(List<Reference> theSupportingInfo) { 4737 this.supportingInfo = theSupportingInfo; 4738 return this; 4739 } 4740 4741 public boolean hasSupportingInfo() { 4742 if (this.supportingInfo == null) 4743 return false; 4744 for (Reference item : this.supportingInfo) 4745 if (!item.isEmpty()) 4746 return true; 4747 return false; 4748 } 4749 4750 public Reference addSupportingInfo() { // 3 4751 Reference t = new Reference(); 4752 if (this.supportingInfo == null) 4753 this.supportingInfo = new ArrayList<Reference>(); 4754 this.supportingInfo.add(t); 4755 return t; 4756 } 4757 4758 public CarePlan addSupportingInfo(Reference t) { // 3 4759 if (t == null) 4760 return this; 4761 if (this.supportingInfo == null) 4762 this.supportingInfo = new ArrayList<Reference>(); 4763 this.supportingInfo.add(t); 4764 return this; 4765 } 4766 4767 /** 4768 * @return The first repetition of repeating field {@link #supportingInfo}, 4769 * creating it if it does not already exist 4770 */ 4771 public Reference getSupportingInfoFirstRep() { 4772 if (getSupportingInfo().isEmpty()) { 4773 addSupportingInfo(); 4774 } 4775 return getSupportingInfo().get(0); 4776 } 4777 4778 /** 4779 * @deprecated Use Reference#setResource(IBaseResource) instead 4780 */ 4781 @Deprecated 4782 public List<Resource> getSupportingInfoTarget() { 4783 if (this.supportingInfoTarget == null) 4784 this.supportingInfoTarget = new ArrayList<Resource>(); 4785 return this.supportingInfoTarget; 4786 } 4787 4788 /** 4789 * @return {@link #goal} (Describes the intended objective(s) of carrying out 4790 * the care plan.) 4791 */ 4792 public List<Reference> getGoal() { 4793 if (this.goal == null) 4794 this.goal = new ArrayList<Reference>(); 4795 return this.goal; 4796 } 4797 4798 /** 4799 * @return Returns a reference to <code>this</code> for easy method chaining 4800 */ 4801 public CarePlan setGoal(List<Reference> theGoal) { 4802 this.goal = theGoal; 4803 return this; 4804 } 4805 4806 public boolean hasGoal() { 4807 if (this.goal == null) 4808 return false; 4809 for (Reference item : this.goal) 4810 if (!item.isEmpty()) 4811 return true; 4812 return false; 4813 } 4814 4815 public Reference addGoal() { // 3 4816 Reference t = new Reference(); 4817 if (this.goal == null) 4818 this.goal = new ArrayList<Reference>(); 4819 this.goal.add(t); 4820 return t; 4821 } 4822 4823 public CarePlan addGoal(Reference t) { // 3 4824 if (t == null) 4825 return this; 4826 if (this.goal == null) 4827 this.goal = new ArrayList<Reference>(); 4828 this.goal.add(t); 4829 return this; 4830 } 4831 4832 /** 4833 * @return The first repetition of repeating field {@link #goal}, creating it if 4834 * it does not already exist 4835 */ 4836 public Reference getGoalFirstRep() { 4837 if (getGoal().isEmpty()) { 4838 addGoal(); 4839 } 4840 return getGoal().get(0); 4841 } 4842 4843 /** 4844 * @deprecated Use Reference#setResource(IBaseResource) instead 4845 */ 4846 @Deprecated 4847 public List<Goal> getGoalTarget() { 4848 if (this.goalTarget == null) 4849 this.goalTarget = new ArrayList<Goal>(); 4850 return this.goalTarget; 4851 } 4852 4853 /** 4854 * @deprecated Use Reference#setResource(IBaseResource) instead 4855 */ 4856 @Deprecated 4857 public Goal addGoalTarget() { 4858 Goal r = new Goal(); 4859 if (this.goalTarget == null) 4860 this.goalTarget = new ArrayList<Goal>(); 4861 this.goalTarget.add(r); 4862 return r; 4863 } 4864 4865 /** 4866 * @return {@link #activity} (Identifies a planned action to occur as part of 4867 * the plan. For example, a medication to be used, lab tests to perform, 4868 * self-monitoring, education, etc.) 4869 */ 4870 public List<CarePlanActivityComponent> getActivity() { 4871 if (this.activity == null) 4872 this.activity = new ArrayList<CarePlanActivityComponent>(); 4873 return this.activity; 4874 } 4875 4876 /** 4877 * @return Returns a reference to <code>this</code> for easy method chaining 4878 */ 4879 public CarePlan setActivity(List<CarePlanActivityComponent> theActivity) { 4880 this.activity = theActivity; 4881 return this; 4882 } 4883 4884 public boolean hasActivity() { 4885 if (this.activity == null) 4886 return false; 4887 for (CarePlanActivityComponent item : this.activity) 4888 if (!item.isEmpty()) 4889 return true; 4890 return false; 4891 } 4892 4893 public CarePlanActivityComponent addActivity() { // 3 4894 CarePlanActivityComponent t = new CarePlanActivityComponent(); 4895 if (this.activity == null) 4896 this.activity = new ArrayList<CarePlanActivityComponent>(); 4897 this.activity.add(t); 4898 return t; 4899 } 4900 4901 public CarePlan addActivity(CarePlanActivityComponent t) { // 3 4902 if (t == null) 4903 return this; 4904 if (this.activity == null) 4905 this.activity = new ArrayList<CarePlanActivityComponent>(); 4906 this.activity.add(t); 4907 return this; 4908 } 4909 4910 /** 4911 * @return The first repetition of repeating field {@link #activity}, creating 4912 * it if it does not already exist 4913 */ 4914 public CarePlanActivityComponent getActivityFirstRep() { 4915 if (getActivity().isEmpty()) { 4916 addActivity(); 4917 } 4918 return getActivity().get(0); 4919 } 4920 4921 /** 4922 * @return {@link #note} (General notes about the care plan not covered 4923 * elsewhere.) 4924 */ 4925 public List<Annotation> getNote() { 4926 if (this.note == null) 4927 this.note = new ArrayList<Annotation>(); 4928 return this.note; 4929 } 4930 4931 /** 4932 * @return Returns a reference to <code>this</code> for easy method chaining 4933 */ 4934 public CarePlan setNote(List<Annotation> theNote) { 4935 this.note = theNote; 4936 return this; 4937 } 4938 4939 public boolean hasNote() { 4940 if (this.note == null) 4941 return false; 4942 for (Annotation item : this.note) 4943 if (!item.isEmpty()) 4944 return true; 4945 return false; 4946 } 4947 4948 public Annotation addNote() { // 3 4949 Annotation t = new Annotation(); 4950 if (this.note == null) 4951 this.note = new ArrayList<Annotation>(); 4952 this.note.add(t); 4953 return t; 4954 } 4955 4956 public CarePlan addNote(Annotation t) { // 3 4957 if (t == null) 4958 return this; 4959 if (this.note == null) 4960 this.note = new ArrayList<Annotation>(); 4961 this.note.add(t); 4962 return this; 4963 } 4964 4965 /** 4966 * @return The first repetition of repeating field {@link #note}, creating it if 4967 * it does not already exist 4968 */ 4969 public Annotation getNoteFirstRep() { 4970 if (getNote().isEmpty()) { 4971 addNote(); 4972 } 4973 return getNote().get(0); 4974 } 4975 4976 protected void listChildren(List<Property> children) { 4977 super.listChildren(children); 4978 children.add(new Property("identifier", "Identifier", 4979 "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 4980 0, java.lang.Integer.MAX_VALUE, identifier)); 4981 children.add(new Property("instantiatesCanonical", 4982 "canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition)", 4983 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 4984 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 4985 children.add(new Property("instantiatesUri", "uri", 4986 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 4987 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 4988 children.add(new Property("basedOn", "Reference(CarePlan)", 4989 "A care plan that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, 4990 basedOn)); 4991 children.add(new Property("replaces", "Reference(CarePlan)", 4992 "Completed or terminated care plan whose function is taken by this new care plan.", 0, 4993 java.lang.Integer.MAX_VALUE, replaces)); 4994 children.add(new Property("partOf", "Reference(CarePlan)", 4995 "A larger care plan of which this particular care plan is a component or step.", 0, java.lang.Integer.MAX_VALUE, 4996 partOf)); 4997 children.add(new Property("status", "code", 4998 "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 4999 0, 1, status)); 5000 children.add(new Property("intent", "code", 5001 "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 5002 0, 1, intent)); 5003 children.add(new Property("category", "CodeableConcept", 5004 "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 5005 0, java.lang.Integer.MAX_VALUE, category)); 5006 children.add(new Property("title", "string", "Human-friendly name for the care plan.", 0, 1, title)); 5007 children.add( 5008 new Property("description", "string", "A description of the scope and nature of the plan.", 0, 1, description)); 5009 children.add(new Property("subject", "Reference(Patient|Group)", 5010 "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject)); 5011 children.add(new Property("encounter", "Reference(Encounter)", 5012 "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.", 5013 0, 1, encounter)); 5014 children.add(new Property("period", "Period", 5015 "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period)); 5016 children.add(new Property("created", "dateTime", 5017 "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.", 5018 0, 1, created)); 5019 children.add(new Property("author", 5020 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5021 "When populated, the author is responsible for the care plan. The care plan is attributed to the author.", 0, 5022 1, author)); 5023 children.add(new Property("contributor", 5024 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5025 "Identifies the individual(s) or organization who provided the contents of the care plan.", 0, 5026 java.lang.Integer.MAX_VALUE, contributor)); 5027 children.add(new Property("careTeam", "Reference(CareTeam)", 5028 "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 5029 0, java.lang.Integer.MAX_VALUE, careTeam)); 5030 children.add(new Property("addresses", "Reference(Condition)", 5031 "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 5032 0, java.lang.Integer.MAX_VALUE, addresses)); 5033 children.add(new Property("supportingInfo", "Reference(Any)", 5034 "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.", 5035 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 5036 children.add(new Property("goal", "Reference(Goal)", 5037 "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal)); 5038 children.add(new Property("activity", "", 5039 "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 5040 0, java.lang.Integer.MAX_VALUE, activity)); 5041 children.add(new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, 5042 java.lang.Integer.MAX_VALUE, note)); 5043 } 5044 5045 @Override 5046 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5047 switch (_hash) { 5048 case -1618432855: 5049 /* identifier */ return new Property("identifier", "Identifier", 5050 "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 5051 0, java.lang.Integer.MAX_VALUE, identifier); 5052 case 8911915: 5053 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 5054 "canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition)", 5055 "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 5056 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 5057 case -1926393373: 5058 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 5059 "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 5060 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 5061 case -332612366: 5062 /* basedOn */ return new Property("basedOn", "Reference(CarePlan)", 5063 "A care plan that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, 5064 basedOn); 5065 case -430332865: 5066 /* replaces */ return new Property("replaces", "Reference(CarePlan)", 5067 "Completed or terminated care plan whose function is taken by this new care plan.", 0, 5068 java.lang.Integer.MAX_VALUE, replaces); 5069 case -995410646: 5070 /* partOf */ return new Property("partOf", "Reference(CarePlan)", 5071 "A larger care plan of which this particular care plan is a component or step.", 0, 5072 java.lang.Integer.MAX_VALUE, partOf); 5073 case -892481550: 5074 /* status */ return new Property("status", "code", 5075 "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 5076 0, 1, status); 5077 case -1183762788: 5078 /* intent */ return new Property("intent", "code", 5079 "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 5080 0, 1, intent); 5081 case 50511102: 5082 /* category */ return new Property("category", "CodeableConcept", 5083 "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 5084 0, java.lang.Integer.MAX_VALUE, category); 5085 case 110371416: 5086 /* title */ return new Property("title", "string", "Human-friendly name for the care plan.", 0, 1, title); 5087 case -1724546052: 5088 /* description */ return new Property("description", "string", 5089 "A description of the scope and nature of the plan.", 0, 1, description); 5090 case -1867885268: 5091 /* subject */ return new Property("subject", "Reference(Patient|Group)", 5092 "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject); 5093 case 1524132147: 5094 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5095 "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.", 5096 0, 1, encounter); 5097 case -991726143: 5098 /* period */ return new Property("period", "Period", 5099 "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period); 5100 case 1028554472: 5101 /* created */ return new Property("created", "dateTime", 5102 "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.", 5103 0, 1, created); 5104 case -1406328437: 5105 /* author */ return new Property("author", 5106 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5107 "When populated, the author is responsible for the care plan. The care plan is attributed to the author.", 0, 5108 1, author); 5109 case -1895276325: 5110 /* contributor */ return new Property("contributor", 5111 "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", 5112 "Identifies the individual(s) or organization who provided the contents of the care plan.", 0, 5113 java.lang.Integer.MAX_VALUE, contributor); 5114 case -7323378: 5115 /* careTeam */ return new Property("careTeam", "Reference(CareTeam)", 5116 "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 5117 0, java.lang.Integer.MAX_VALUE, careTeam); 5118 case 874544034: 5119 /* addresses */ return new Property("addresses", "Reference(Condition)", 5120 "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 5121 0, java.lang.Integer.MAX_VALUE, addresses); 5122 case 1922406657: 5123 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 5124 "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.", 5125 0, java.lang.Integer.MAX_VALUE, supportingInfo); 5126 case 3178259: 5127 /* goal */ return new Property("goal", "Reference(Goal)", 5128 "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal); 5129 case -1655966961: 5130 /* activity */ return new Property("activity", "", 5131 "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 5132 0, java.lang.Integer.MAX_VALUE, activity); 5133 case 3387378: 5134 /* note */ return new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 5135 0, java.lang.Integer.MAX_VALUE, note); 5136 default: 5137 return super.getNamedProperty(_hash, _name, _checkValid); 5138 } 5139 5140 } 5141 5142 @Override 5143 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5144 switch (hash) { 5145 case -1618432855: 5146 /* identifier */ return this.identifier == null ? new Base[0] 5147 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5148 case 8911915: 5149 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 5150 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 5151 case -1926393373: 5152 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 5153 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 5154 case -332612366: 5155 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 5156 case -430332865: 5157 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 5158 case -995410646: 5159 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 5160 case -892481550: 5161 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CarePlanStatus> 5162 case -1183762788: 5163 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<CarePlanIntent> 5164 case 50511102: 5165 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 5166 case 110371416: 5167 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5168 case -1724546052: 5169 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 5170 case -1867885268: 5171 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 5172 case 1524132147: 5173 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 5174 case -991726143: 5175 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 5176 case 1028554472: 5177 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 5178 case -1406328437: 5179 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 5180 case -1895276325: 5181 /* contributor */ return this.contributor == null ? new Base[0] 5182 : this.contributor.toArray(new Base[this.contributor.size()]); // Reference 5183 case -7323378: 5184 /* careTeam */ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // Reference 5185 case 874544034: 5186 /* addresses */ return this.addresses == null ? new Base[0] 5187 : this.addresses.toArray(new Base[this.addresses.size()]); // Reference 5188 case 1922406657: 5189 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 5190 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 5191 case 3178259: 5192 /* goal */ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 5193 case -1655966961: 5194 /* activity */ return this.activity == null ? new Base[0] : this.activity.toArray(new Base[this.activity.size()]); // CarePlanActivityComponent 5195 case 3387378: 5196 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5197 default: 5198 return super.getProperty(hash, name, checkValid); 5199 } 5200 5201 } 5202 5203 @Override 5204 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5205 switch (hash) { 5206 case -1618432855: // identifier 5207 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5208 return value; 5209 case 8911915: // instantiatesCanonical 5210 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 5211 return value; 5212 case -1926393373: // instantiatesUri 5213 this.getInstantiatesUri().add(castToUri(value)); // UriType 5214 return value; 5215 case -332612366: // basedOn 5216 this.getBasedOn().add(castToReference(value)); // Reference 5217 return value; 5218 case -430332865: // replaces 5219 this.getReplaces().add(castToReference(value)); // Reference 5220 return value; 5221 case -995410646: // partOf 5222 this.getPartOf().add(castToReference(value)); // Reference 5223 return value; 5224 case -892481550: // status 5225 value = new CarePlanStatusEnumFactory().fromType(castToCode(value)); 5226 this.status = (Enumeration) value; // Enumeration<CarePlanStatus> 5227 return value; 5228 case -1183762788: // intent 5229 value = new CarePlanIntentEnumFactory().fromType(castToCode(value)); 5230 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 5231 return value; 5232 case 50511102: // category 5233 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 5234 return value; 5235 case 110371416: // title 5236 this.title = castToString(value); // StringType 5237 return value; 5238 case -1724546052: // description 5239 this.description = castToString(value); // StringType 5240 return value; 5241 case -1867885268: // subject 5242 this.subject = castToReference(value); // Reference 5243 return value; 5244 case 1524132147: // encounter 5245 this.encounter = castToReference(value); // Reference 5246 return value; 5247 case -991726143: // period 5248 this.period = castToPeriod(value); // Period 5249 return value; 5250 case 1028554472: // created 5251 this.created = castToDateTime(value); // DateTimeType 5252 return value; 5253 case -1406328437: // author 5254 this.author = castToReference(value); // Reference 5255 return value; 5256 case -1895276325: // contributor 5257 this.getContributor().add(castToReference(value)); // Reference 5258 return value; 5259 case -7323378: // careTeam 5260 this.getCareTeam().add(castToReference(value)); // Reference 5261 return value; 5262 case 874544034: // addresses 5263 this.getAddresses().add(castToReference(value)); // Reference 5264 return value; 5265 case 1922406657: // supportingInfo 5266 this.getSupportingInfo().add(castToReference(value)); // Reference 5267 return value; 5268 case 3178259: // goal 5269 this.getGoal().add(castToReference(value)); // Reference 5270 return value; 5271 case -1655966961: // activity 5272 this.getActivity().add((CarePlanActivityComponent) value); // CarePlanActivityComponent 5273 return value; 5274 case 3387378: // note 5275 this.getNote().add(castToAnnotation(value)); // Annotation 5276 return value; 5277 default: 5278 return super.setProperty(hash, name, value); 5279 } 5280 5281 } 5282 5283 @Override 5284 public Base setProperty(String name, Base value) throws FHIRException { 5285 if (name.equals("identifier")) { 5286 this.getIdentifier().add(castToIdentifier(value)); 5287 } else if (name.equals("instantiatesCanonical")) { 5288 this.getInstantiatesCanonical().add(castToCanonical(value)); 5289 } else if (name.equals("instantiatesUri")) { 5290 this.getInstantiatesUri().add(castToUri(value)); 5291 } else if (name.equals("basedOn")) { 5292 this.getBasedOn().add(castToReference(value)); 5293 } else if (name.equals("replaces")) { 5294 this.getReplaces().add(castToReference(value)); 5295 } else if (name.equals("partOf")) { 5296 this.getPartOf().add(castToReference(value)); 5297 } else if (name.equals("status")) { 5298 value = new CarePlanStatusEnumFactory().fromType(castToCode(value)); 5299 this.status = (Enumeration) value; // Enumeration<CarePlanStatus> 5300 } else if (name.equals("intent")) { 5301 value = new CarePlanIntentEnumFactory().fromType(castToCode(value)); 5302 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 5303 } else if (name.equals("category")) { 5304 this.getCategory().add(castToCodeableConcept(value)); 5305 } else if (name.equals("title")) { 5306 this.title = castToString(value); // StringType 5307 } else if (name.equals("description")) { 5308 this.description = castToString(value); // StringType 5309 } else if (name.equals("subject")) { 5310 this.subject = castToReference(value); // Reference 5311 } else if (name.equals("encounter")) { 5312 this.encounter = castToReference(value); // Reference 5313 } else if (name.equals("period")) { 5314 this.period = castToPeriod(value); // Period 5315 } else if (name.equals("created")) { 5316 this.created = castToDateTime(value); // DateTimeType 5317 } else if (name.equals("author")) { 5318 this.author = castToReference(value); // Reference 5319 } else if (name.equals("contributor")) { 5320 this.getContributor().add(castToReference(value)); 5321 } else if (name.equals("careTeam")) { 5322 this.getCareTeam().add(castToReference(value)); 5323 } else if (name.equals("addresses")) { 5324 this.getAddresses().add(castToReference(value)); 5325 } else if (name.equals("supportingInfo")) { 5326 this.getSupportingInfo().add(castToReference(value)); 5327 } else if (name.equals("goal")) { 5328 this.getGoal().add(castToReference(value)); 5329 } else if (name.equals("activity")) { 5330 this.getActivity().add((CarePlanActivityComponent) value); 5331 } else if (name.equals("note")) { 5332 this.getNote().add(castToAnnotation(value)); 5333 } else 5334 return super.setProperty(name, value); 5335 return value; 5336 } 5337 5338 @Override 5339 public void removeChild(String name, Base value) throws FHIRException { 5340 if (name.equals("identifier")) { 5341 this.getIdentifier().remove(castToIdentifier(value)); 5342 } else if (name.equals("instantiatesCanonical")) { 5343 this.getInstantiatesCanonical().remove(castToCanonical(value)); 5344 } else if (name.equals("instantiatesUri")) { 5345 this.getInstantiatesUri().remove(castToUri(value)); 5346 } else if (name.equals("basedOn")) { 5347 this.getBasedOn().remove(castToReference(value)); 5348 } else if (name.equals("replaces")) { 5349 this.getReplaces().remove(castToReference(value)); 5350 } else if (name.equals("partOf")) { 5351 this.getPartOf().remove(castToReference(value)); 5352 } else if (name.equals("status")) { 5353 this.status = null; 5354 } else if (name.equals("intent")) { 5355 this.intent = null; 5356 } else if (name.equals("category")) { 5357 this.getCategory().remove(castToCodeableConcept(value)); 5358 } else if (name.equals("title")) { 5359 this.title = null; 5360 } else if (name.equals("description")) { 5361 this.description = null; 5362 } else if (name.equals("subject")) { 5363 this.subject = null; 5364 } else if (name.equals("encounter")) { 5365 this.encounter = null; 5366 } else if (name.equals("period")) { 5367 this.period = null; 5368 } else if (name.equals("created")) { 5369 this.created = null; 5370 } else if (name.equals("author")) { 5371 this.author = null; 5372 } else if (name.equals("contributor")) { 5373 this.getContributor().remove(castToReference(value)); 5374 } else if (name.equals("careTeam")) { 5375 this.getCareTeam().remove(castToReference(value)); 5376 } else if (name.equals("addresses")) { 5377 this.getAddresses().remove(castToReference(value)); 5378 } else if (name.equals("supportingInfo")) { 5379 this.getSupportingInfo().remove(castToReference(value)); 5380 } else if (name.equals("goal")) { 5381 this.getGoal().remove(castToReference(value)); 5382 } else if (name.equals("activity")) { 5383 this.getActivity().remove((CarePlanActivityComponent) value); 5384 } else if (name.equals("note")) { 5385 this.getNote().remove(castToAnnotation(value)); 5386 } else 5387 super.removeChild(name, value); 5388 5389 } 5390 5391 @Override 5392 public Base makeProperty(int hash, String name) throws FHIRException { 5393 switch (hash) { 5394 case -1618432855: 5395 return addIdentifier(); 5396 case 8911915: 5397 return addInstantiatesCanonicalElement(); 5398 case -1926393373: 5399 return addInstantiatesUriElement(); 5400 case -332612366: 5401 return addBasedOn(); 5402 case -430332865: 5403 return addReplaces(); 5404 case -995410646: 5405 return addPartOf(); 5406 case -892481550: 5407 return getStatusElement(); 5408 case -1183762788: 5409 return getIntentElement(); 5410 case 50511102: 5411 return addCategory(); 5412 case 110371416: 5413 return getTitleElement(); 5414 case -1724546052: 5415 return getDescriptionElement(); 5416 case -1867885268: 5417 return getSubject(); 5418 case 1524132147: 5419 return getEncounter(); 5420 case -991726143: 5421 return getPeriod(); 5422 case 1028554472: 5423 return getCreatedElement(); 5424 case -1406328437: 5425 return getAuthor(); 5426 case -1895276325: 5427 return addContributor(); 5428 case -7323378: 5429 return addCareTeam(); 5430 case 874544034: 5431 return addAddresses(); 5432 case 1922406657: 5433 return addSupportingInfo(); 5434 case 3178259: 5435 return addGoal(); 5436 case -1655966961: 5437 return addActivity(); 5438 case 3387378: 5439 return addNote(); 5440 default: 5441 return super.makeProperty(hash, name); 5442 } 5443 5444 } 5445 5446 @Override 5447 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5448 switch (hash) { 5449 case -1618432855: 5450 /* identifier */ return new String[] { "Identifier" }; 5451 case 8911915: 5452 /* instantiatesCanonical */ return new String[] { "canonical" }; 5453 case -1926393373: 5454 /* instantiatesUri */ return new String[] { "uri" }; 5455 case -332612366: 5456 /* basedOn */ return new String[] { "Reference" }; 5457 case -430332865: 5458 /* replaces */ return new String[] { "Reference" }; 5459 case -995410646: 5460 /* partOf */ return new String[] { "Reference" }; 5461 case -892481550: 5462 /* status */ return new String[] { "code" }; 5463 case -1183762788: 5464 /* intent */ return new String[] { "code" }; 5465 case 50511102: 5466 /* category */ return new String[] { "CodeableConcept" }; 5467 case 110371416: 5468 /* title */ return new String[] { "string" }; 5469 case -1724546052: 5470 /* description */ return new String[] { "string" }; 5471 case -1867885268: 5472 /* subject */ return new String[] { "Reference" }; 5473 case 1524132147: 5474 /* encounter */ return new String[] { "Reference" }; 5475 case -991726143: 5476 /* period */ return new String[] { "Period" }; 5477 case 1028554472: 5478 /* created */ return new String[] { "dateTime" }; 5479 case -1406328437: 5480 /* author */ return new String[] { "Reference" }; 5481 case -1895276325: 5482 /* contributor */ return new String[] { "Reference" }; 5483 case -7323378: 5484 /* careTeam */ return new String[] { "Reference" }; 5485 case 874544034: 5486 /* addresses */ return new String[] { "Reference" }; 5487 case 1922406657: 5488 /* supportingInfo */ return new String[] { "Reference" }; 5489 case 3178259: 5490 /* goal */ return new String[] { "Reference" }; 5491 case -1655966961: 5492 /* activity */ return new String[] {}; 5493 case 3387378: 5494 /* note */ return new String[] { "Annotation" }; 5495 default: 5496 return super.getTypesForProperty(hash, name); 5497 } 5498 5499 } 5500 5501 @Override 5502 public Base addChild(String name) throws FHIRException { 5503 if (name.equals("identifier")) { 5504 return addIdentifier(); 5505 } else if (name.equals("instantiatesCanonical")) { 5506 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesCanonical"); 5507 } else if (name.equals("instantiatesUri")) { 5508 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesUri"); 5509 } else if (name.equals("basedOn")) { 5510 return addBasedOn(); 5511 } else if (name.equals("replaces")) { 5512 return addReplaces(); 5513 } else if (name.equals("partOf")) { 5514 return addPartOf(); 5515 } else if (name.equals("status")) { 5516 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 5517 } else if (name.equals("intent")) { 5518 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.intent"); 5519 } else if (name.equals("category")) { 5520 return addCategory(); 5521 } else if (name.equals("title")) { 5522 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.title"); 5523 } else if (name.equals("description")) { 5524 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 5525 } else if (name.equals("subject")) { 5526 this.subject = new Reference(); 5527 return this.subject; 5528 } else if (name.equals("encounter")) { 5529 this.encounter = new Reference(); 5530 return this.encounter; 5531 } else if (name.equals("period")) { 5532 this.period = new Period(); 5533 return this.period; 5534 } else if (name.equals("created")) { 5535 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.created"); 5536 } else if (name.equals("author")) { 5537 this.author = new Reference(); 5538 return this.author; 5539 } else if (name.equals("contributor")) { 5540 return addContributor(); 5541 } else if (name.equals("careTeam")) { 5542 return addCareTeam(); 5543 } else if (name.equals("addresses")) { 5544 return addAddresses(); 5545 } else if (name.equals("supportingInfo")) { 5546 return addSupportingInfo(); 5547 } else if (name.equals("goal")) { 5548 return addGoal(); 5549 } else if (name.equals("activity")) { 5550 return addActivity(); 5551 } else if (name.equals("note")) { 5552 return addNote(); 5553 } else 5554 return super.addChild(name); 5555 } 5556 5557 public String fhirType() { 5558 return "CarePlan"; 5559 5560 } 5561 5562 public CarePlan copy() { 5563 CarePlan dst = new CarePlan(); 5564 copyValues(dst); 5565 return dst; 5566 } 5567 5568 public void copyValues(CarePlan dst) { 5569 super.copyValues(dst); 5570 if (identifier != null) { 5571 dst.identifier = new ArrayList<Identifier>(); 5572 for (Identifier i : identifier) 5573 dst.identifier.add(i.copy()); 5574 } 5575 ; 5576 if (instantiatesCanonical != null) { 5577 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 5578 for (CanonicalType i : instantiatesCanonical) 5579 dst.instantiatesCanonical.add(i.copy()); 5580 } 5581 ; 5582 if (instantiatesUri != null) { 5583 dst.instantiatesUri = new ArrayList<UriType>(); 5584 for (UriType i : instantiatesUri) 5585 dst.instantiatesUri.add(i.copy()); 5586 } 5587 ; 5588 if (basedOn != null) { 5589 dst.basedOn = new ArrayList<Reference>(); 5590 for (Reference i : basedOn) 5591 dst.basedOn.add(i.copy()); 5592 } 5593 ; 5594 if (replaces != null) { 5595 dst.replaces = new ArrayList<Reference>(); 5596 for (Reference i : replaces) 5597 dst.replaces.add(i.copy()); 5598 } 5599 ; 5600 if (partOf != null) { 5601 dst.partOf = new ArrayList<Reference>(); 5602 for (Reference i : partOf) 5603 dst.partOf.add(i.copy()); 5604 } 5605 ; 5606 dst.status = status == null ? null : status.copy(); 5607 dst.intent = intent == null ? null : intent.copy(); 5608 if (category != null) { 5609 dst.category = new ArrayList<CodeableConcept>(); 5610 for (CodeableConcept i : category) 5611 dst.category.add(i.copy()); 5612 } 5613 ; 5614 dst.title = title == null ? null : title.copy(); 5615 dst.description = description == null ? null : description.copy(); 5616 dst.subject = subject == null ? null : subject.copy(); 5617 dst.encounter = encounter == null ? null : encounter.copy(); 5618 dst.period = period == null ? null : period.copy(); 5619 dst.created = created == null ? null : created.copy(); 5620 dst.author = author == null ? null : author.copy(); 5621 if (contributor != null) { 5622 dst.contributor = new ArrayList<Reference>(); 5623 for (Reference i : contributor) 5624 dst.contributor.add(i.copy()); 5625 } 5626 ; 5627 if (careTeam != null) { 5628 dst.careTeam = new ArrayList<Reference>(); 5629 for (Reference i : careTeam) 5630 dst.careTeam.add(i.copy()); 5631 } 5632 ; 5633 if (addresses != null) { 5634 dst.addresses = new ArrayList<Reference>(); 5635 for (Reference i : addresses) 5636 dst.addresses.add(i.copy()); 5637 } 5638 ; 5639 if (supportingInfo != null) { 5640 dst.supportingInfo = new ArrayList<Reference>(); 5641 for (Reference i : supportingInfo) 5642 dst.supportingInfo.add(i.copy()); 5643 } 5644 ; 5645 if (goal != null) { 5646 dst.goal = new ArrayList<Reference>(); 5647 for (Reference i : goal) 5648 dst.goal.add(i.copy()); 5649 } 5650 ; 5651 if (activity != null) { 5652 dst.activity = new ArrayList<CarePlanActivityComponent>(); 5653 for (CarePlanActivityComponent i : activity) 5654 dst.activity.add(i.copy()); 5655 } 5656 ; 5657 if (note != null) { 5658 dst.note = new ArrayList<Annotation>(); 5659 for (Annotation i : note) 5660 dst.note.add(i.copy()); 5661 } 5662 ; 5663 } 5664 5665 protected CarePlan typedCopy() { 5666 return copy(); 5667 } 5668 5669 @Override 5670 public boolean equalsDeep(Base other_) { 5671 if (!super.equalsDeep(other_)) 5672 return false; 5673 if (!(other_ instanceof CarePlan)) 5674 return false; 5675 CarePlan o = (CarePlan) other_; 5676 return compareDeep(identifier, o.identifier, true) 5677 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5678 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 5679 && compareDeep(replaces, o.replaces, true) && compareDeep(partOf, o.partOf, true) 5680 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 5681 && compareDeep(category, o.category, true) && compareDeep(title, o.title, true) 5682 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) 5683 && compareDeep(encounter, o.encounter, true) && compareDeep(period, o.period, true) 5684 && compareDeep(created, o.created, true) && compareDeep(author, o.author, true) 5685 && compareDeep(contributor, o.contributor, true) && compareDeep(careTeam, o.careTeam, true) 5686 && compareDeep(addresses, o.addresses, true) && compareDeep(supportingInfo, o.supportingInfo, true) 5687 && compareDeep(goal, o.goal, true) && compareDeep(activity, o.activity, true) 5688 && compareDeep(note, o.note, true); 5689 } 5690 5691 @Override 5692 public boolean equalsShallow(Base other_) { 5693 if (!super.equalsShallow(other_)) 5694 return false; 5695 if (!(other_ instanceof CarePlan)) 5696 return false; 5697 CarePlan o = (CarePlan) other_; 5698 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 5699 && compareValues(intent, o.intent, true) && compareValues(title, o.title, true) 5700 && compareValues(description, o.description, true) && compareValues(created, o.created, true); 5701 } 5702 5703 public boolean isEmpty() { 5704 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 5705 basedOn, replaces, partOf, status, intent, category, title, description, subject, encounter, period, created, 5706 author, contributor, careTeam, addresses, supportingInfo, goal, activity, note); 5707 } 5708 5709 @Override 5710 public ResourceType getResourceType() { 5711 return ResourceType.CarePlan; 5712 } 5713 5714 /** 5715 * Search parameter: <b>date</b> 5716 * <p> 5717 * Description: <b>Time period plan covers</b><br> 5718 * Type: <b>date</b><br> 5719 * Path: <b>CarePlan.period</b><br> 5720 * </p> 5721 */ 5722 @SearchParamDefinition(name = "date", path = "CarePlan.period", description = "Time period plan covers", type = "date") 5723 public static final String SP_DATE = "date"; 5724 /** 5725 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5726 * <p> 5727 * Description: <b>Time period plan covers</b><br> 5728 * Type: <b>date</b><br> 5729 * Path: <b>CarePlan.period</b><br> 5730 * </p> 5731 */ 5732 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5733 SP_DATE); 5734 5735 /** 5736 * Search parameter: <b>care-team</b> 5737 * <p> 5738 * Description: <b>Who's involved in plan?</b><br> 5739 * Type: <b>reference</b><br> 5740 * Path: <b>CarePlan.careTeam</b><br> 5741 * </p> 5742 */ 5743 @SearchParamDefinition(name = "care-team", path = "CarePlan.careTeam", description = "Who's involved in plan?", type = "reference", target = { 5744 CareTeam.class }) 5745 public static final String SP_CARE_TEAM = "care-team"; 5746 /** 5747 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 5748 * <p> 5749 * Description: <b>Who's involved in plan?</b><br> 5750 * Type: <b>reference</b><br> 5751 * Path: <b>CarePlan.careTeam</b><br> 5752 * </p> 5753 */ 5754 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5755 SP_CARE_TEAM); 5756 5757 /** 5758 * Constant for fluent queries to be used to add include statements. Specifies 5759 * the path value of "<b>CarePlan:care-team</b>". 5760 */ 5761 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include( 5762 "CarePlan:care-team").toLocked(); 5763 5764 /** 5765 * Search parameter: <b>identifier</b> 5766 * <p> 5767 * Description: <b>External Ids for this plan</b><br> 5768 * Type: <b>token</b><br> 5769 * Path: <b>CarePlan.identifier</b><br> 5770 * </p> 5771 */ 5772 @SearchParamDefinition(name = "identifier", path = "CarePlan.identifier", description = "External Ids for this plan", type = "token") 5773 public static final String SP_IDENTIFIER = "identifier"; 5774 /** 5775 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5776 * <p> 5777 * Description: <b>External Ids for this plan</b><br> 5778 * Type: <b>token</b><br> 5779 * Path: <b>CarePlan.identifier</b><br> 5780 * </p> 5781 */ 5782 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5783 SP_IDENTIFIER); 5784 5785 /** 5786 * Search parameter: <b>performer</b> 5787 * <p> 5788 * Description: <b>Matches if the practitioner is listed as a performer in any 5789 * of the "simple" activities. (For performers of the detailed activities, chain 5790 * through the activitydetail search parameter.)</b><br> 5791 * Type: <b>reference</b><br> 5792 * Path: <b>CarePlan.activity.detail.performer</b><br> 5793 * </p> 5794 */ 5795 @SearchParamDefinition(name = "performer", path = "CarePlan.activity.detail.performer", description = "Matches if the practitioner is listed as a performer in any of the \"simple\" activities. (For performers of the detailed activities, chain through the activitydetail search parameter.)", type = "reference", providesMembershipIn = { 5796 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 5797 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 5798 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 5799 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 5800 RelatedPerson.class }) 5801 public static final String SP_PERFORMER = "performer"; 5802 /** 5803 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 5804 * <p> 5805 * Description: <b>Matches if the practitioner is listed as a performer in any 5806 * of the "simple" activities. (For performers of the detailed activities, chain 5807 * through the activitydetail search parameter.)</b><br> 5808 * Type: <b>reference</b><br> 5809 * Path: <b>CarePlan.activity.detail.performer</b><br> 5810 * </p> 5811 */ 5812 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5813 SP_PERFORMER); 5814 5815 /** 5816 * Constant for fluent queries to be used to add include statements. Specifies 5817 * the path value of "<b>CarePlan:performer</b>". 5818 */ 5819 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 5820 "CarePlan:performer").toLocked(); 5821 5822 /** 5823 * Search parameter: <b>goal</b> 5824 * <p> 5825 * Description: <b>Desired outcome of plan</b><br> 5826 * Type: <b>reference</b><br> 5827 * Path: <b>CarePlan.goal</b><br> 5828 * </p> 5829 */ 5830 @SearchParamDefinition(name = "goal", path = "CarePlan.goal", description = "Desired outcome of plan", type = "reference", target = { 5831 Goal.class }) 5832 public static final String SP_GOAL = "goal"; 5833 /** 5834 * <b>Fluent Client</b> search parameter constant for <b>goal</b> 5835 * <p> 5836 * Description: <b>Desired outcome of plan</b><br> 5837 * Type: <b>reference</b><br> 5838 * Path: <b>CarePlan.goal</b><br> 5839 * </p> 5840 */ 5841 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GOAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5842 SP_GOAL); 5843 5844 /** 5845 * Constant for fluent queries to be used to add include statements. Specifies 5846 * the path value of "<b>CarePlan:goal</b>". 5847 */ 5848 public static final ca.uhn.fhir.model.api.Include INCLUDE_GOAL = new ca.uhn.fhir.model.api.Include("CarePlan:goal") 5849 .toLocked(); 5850 5851 /** 5852 * Search parameter: <b>subject</b> 5853 * <p> 5854 * Description: <b>Who the care plan is for</b><br> 5855 * Type: <b>reference</b><br> 5856 * Path: <b>CarePlan.subject</b><br> 5857 * </p> 5858 */ 5859 @SearchParamDefinition(name = "subject", path = "CarePlan.subject", description = "Who the care plan is for", type = "reference", target = { 5860 Group.class, Patient.class }) 5861 public static final String SP_SUBJECT = "subject"; 5862 /** 5863 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5864 * <p> 5865 * Description: <b>Who the care plan is for</b><br> 5866 * Type: <b>reference</b><br> 5867 * Path: <b>CarePlan.subject</b><br> 5868 * </p> 5869 */ 5870 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5871 SP_SUBJECT); 5872 5873 /** 5874 * Constant for fluent queries to be used to add include statements. Specifies 5875 * the path value of "<b>CarePlan:subject</b>". 5876 */ 5877 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 5878 "CarePlan:subject").toLocked(); 5879 5880 /** 5881 * Search parameter: <b>replaces</b> 5882 * <p> 5883 * Description: <b>CarePlan replaced by this CarePlan</b><br> 5884 * Type: <b>reference</b><br> 5885 * Path: <b>CarePlan.replaces</b><br> 5886 * </p> 5887 */ 5888 @SearchParamDefinition(name = "replaces", path = "CarePlan.replaces", description = "CarePlan replaced by this CarePlan", type = "reference", target = { 5889 CarePlan.class }) 5890 public static final String SP_REPLACES = "replaces"; 5891 /** 5892 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 5893 * <p> 5894 * Description: <b>CarePlan replaced by this CarePlan</b><br> 5895 * Type: <b>reference</b><br> 5896 * Path: <b>CarePlan.replaces</b><br> 5897 * </p> 5898 */ 5899 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5900 SP_REPLACES); 5901 5902 /** 5903 * Constant for fluent queries to be used to add include statements. Specifies 5904 * the path value of "<b>CarePlan:replaces</b>". 5905 */ 5906 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include( 5907 "CarePlan:replaces").toLocked(); 5908 5909 /** 5910 * Search parameter: <b>instantiates-canonical</b> 5911 * <p> 5912 * Description: <b>Instantiates FHIR protocol or definition</b><br> 5913 * Type: <b>reference</b><br> 5914 * Path: <b>CarePlan.instantiatesCanonical</b><br> 5915 * </p> 5916 */ 5917 @SearchParamDefinition(name = "instantiates-canonical", path = "CarePlan.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 5918 ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class }) 5919 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 5920 /** 5921 * <b>Fluent Client</b> search parameter constant for 5922 * <b>instantiates-canonical</b> 5923 * <p> 5924 * Description: <b>Instantiates FHIR protocol or definition</b><br> 5925 * Type: <b>reference</b><br> 5926 * Path: <b>CarePlan.instantiatesCanonical</b><br> 5927 * </p> 5928 */ 5929 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5930 SP_INSTANTIATES_CANONICAL); 5931 5932 /** 5933 * Constant for fluent queries to be used to add include statements. Specifies 5934 * the path value of "<b>CarePlan:instantiates-canonical</b>". 5935 */ 5936 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 5937 "CarePlan:instantiates-canonical").toLocked(); 5938 5939 /** 5940 * Search parameter: <b>part-of</b> 5941 * <p> 5942 * Description: <b>Part of referenced CarePlan</b><br> 5943 * Type: <b>reference</b><br> 5944 * Path: <b>CarePlan.partOf</b><br> 5945 * </p> 5946 */ 5947 @SearchParamDefinition(name = "part-of", path = "CarePlan.partOf", description = "Part of referenced CarePlan", type = "reference", target = { 5948 CarePlan.class }) 5949 public static final String SP_PART_OF = "part-of"; 5950 /** 5951 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5952 * <p> 5953 * Description: <b>Part of referenced CarePlan</b><br> 5954 * Type: <b>reference</b><br> 5955 * Path: <b>CarePlan.partOf</b><br> 5956 * </p> 5957 */ 5958 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5959 SP_PART_OF); 5960 5961 /** 5962 * Constant for fluent queries to be used to add include statements. Specifies 5963 * the path value of "<b>CarePlan:part-of</b>". 5964 */ 5965 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 5966 "CarePlan:part-of").toLocked(); 5967 5968 /** 5969 * Search parameter: <b>encounter</b> 5970 * <p> 5971 * Description: <b>Encounter created as part of</b><br> 5972 * Type: <b>reference</b><br> 5973 * Path: <b>CarePlan.encounter</b><br> 5974 * </p> 5975 */ 5976 @SearchParamDefinition(name = "encounter", path = "CarePlan.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 5977 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 5978 public static final String SP_ENCOUNTER = "encounter"; 5979 /** 5980 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5981 * <p> 5982 * Description: <b>Encounter created as part of</b><br> 5983 * Type: <b>reference</b><br> 5984 * Path: <b>CarePlan.encounter</b><br> 5985 * </p> 5986 */ 5987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5988 SP_ENCOUNTER); 5989 5990 /** 5991 * Constant for fluent queries to be used to add include statements. Specifies 5992 * the path value of "<b>CarePlan:encounter</b>". 5993 */ 5994 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5995 "CarePlan:encounter").toLocked(); 5996 5997 /** 5998 * Search parameter: <b>intent</b> 5999 * <p> 6000 * Description: <b>proposal | plan | order | option</b><br> 6001 * Type: <b>token</b><br> 6002 * Path: <b>CarePlan.intent</b><br> 6003 * </p> 6004 */ 6005 @SearchParamDefinition(name = "intent", path = "CarePlan.intent", description = "proposal | plan | order | option", type = "token") 6006 public static final String SP_INTENT = "intent"; 6007 /** 6008 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 6009 * <p> 6010 * Description: <b>proposal | plan | order | option</b><br> 6011 * Type: <b>token</b><br> 6012 * Path: <b>CarePlan.intent</b><br> 6013 * </p> 6014 */ 6015 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6016 SP_INTENT); 6017 6018 /** 6019 * Search parameter: <b>activity-reference</b> 6020 * <p> 6021 * Description: <b>Activity details defined in specific resource</b><br> 6022 * Type: <b>reference</b><br> 6023 * Path: <b>CarePlan.activity.reference</b><br> 6024 * </p> 6025 */ 6026 @SearchParamDefinition(name = "activity-reference", path = "CarePlan.activity.reference", description = "Activity details defined in specific resource", type = "reference", target = { 6027 Appointment.class, CommunicationRequest.class, DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, 6028 RequestGroup.class, ServiceRequest.class, Task.class, VisionPrescription.class }) 6029 public static final String SP_ACTIVITY_REFERENCE = "activity-reference"; 6030 /** 6031 * <b>Fluent Client</b> search parameter constant for <b>activity-reference</b> 6032 * <p> 6033 * Description: <b>Activity details defined in specific resource</b><br> 6034 * Type: <b>reference</b><br> 6035 * Path: <b>CarePlan.activity.reference</b><br> 6036 * </p> 6037 */ 6038 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTIVITY_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6039 SP_ACTIVITY_REFERENCE); 6040 6041 /** 6042 * Constant for fluent queries to be used to add include statements. Specifies 6043 * the path value of "<b>CarePlan:activity-reference</b>". 6044 */ 6045 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTIVITY_REFERENCE = new ca.uhn.fhir.model.api.Include( 6046 "CarePlan:activity-reference").toLocked(); 6047 6048 /** 6049 * Search parameter: <b>condition</b> 6050 * <p> 6051 * Description: <b>Health issues this plan addresses</b><br> 6052 * Type: <b>reference</b><br> 6053 * Path: <b>CarePlan.addresses</b><br> 6054 * </p> 6055 */ 6056 @SearchParamDefinition(name = "condition", path = "CarePlan.addresses", description = "Health issues this plan addresses", type = "reference", target = { 6057 Condition.class }) 6058 public static final String SP_CONDITION = "condition"; 6059 /** 6060 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 6061 * <p> 6062 * Description: <b>Health issues this plan addresses</b><br> 6063 * Type: <b>reference</b><br> 6064 * Path: <b>CarePlan.addresses</b><br> 6065 * </p> 6066 */ 6067 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6068 SP_CONDITION); 6069 6070 /** 6071 * Constant for fluent queries to be used to add include statements. Specifies 6072 * the path value of "<b>CarePlan:condition</b>". 6073 */ 6074 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include( 6075 "CarePlan:condition").toLocked(); 6076 6077 /** 6078 * Search parameter: <b>based-on</b> 6079 * <p> 6080 * Description: <b>Fulfills CarePlan</b><br> 6081 * Type: <b>reference</b><br> 6082 * Path: <b>CarePlan.basedOn</b><br> 6083 * </p> 6084 */ 6085 @SearchParamDefinition(name = "based-on", path = "CarePlan.basedOn", description = "Fulfills CarePlan", type = "reference", target = { 6086 CarePlan.class }) 6087 public static final String SP_BASED_ON = "based-on"; 6088 /** 6089 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 6090 * <p> 6091 * Description: <b>Fulfills CarePlan</b><br> 6092 * Type: <b>reference</b><br> 6093 * Path: <b>CarePlan.basedOn</b><br> 6094 * </p> 6095 */ 6096 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6097 SP_BASED_ON); 6098 6099 /** 6100 * Constant for fluent queries to be used to add include statements. Specifies 6101 * the path value of "<b>CarePlan:based-on</b>". 6102 */ 6103 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 6104 "CarePlan:based-on").toLocked(); 6105 6106 /** 6107 * Search parameter: <b>patient</b> 6108 * <p> 6109 * Description: <b>Who the care plan is for</b><br> 6110 * Type: <b>reference</b><br> 6111 * Path: <b>CarePlan.subject</b><br> 6112 * </p> 6113 */ 6114 @SearchParamDefinition(name = "patient", path = "CarePlan.subject.where(resolve() is Patient)", description = "Who the care plan is for", type = "reference", providesMembershipIn = { 6115 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 6116 public static final String SP_PATIENT = "patient"; 6117 /** 6118 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6119 * <p> 6120 * Description: <b>Who the care plan is for</b><br> 6121 * Type: <b>reference</b><br> 6122 * Path: <b>CarePlan.subject</b><br> 6123 * </p> 6124 */ 6125 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6126 SP_PATIENT); 6127 6128 /** 6129 * Constant for fluent queries to be used to add include statements. Specifies 6130 * the path value of "<b>CarePlan:patient</b>". 6131 */ 6132 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 6133 "CarePlan:patient").toLocked(); 6134 6135 /** 6136 * Search parameter: <b>activity-date</b> 6137 * <p> 6138 * Description: <b>Specified date occurs within period specified by 6139 * CarePlan.activity.detail.scheduled[x]</b><br> 6140 * Type: <b>date</b><br> 6141 * Path: <b>CarePlan.activity.detail.scheduled[x]</b><br> 6142 * </p> 6143 */ 6144 @SearchParamDefinition(name = "activity-date", path = "CarePlan.activity.detail.scheduled", description = "Specified date occurs within period specified by CarePlan.activity.detail.scheduled[x]", type = "date") 6145 public static final String SP_ACTIVITY_DATE = "activity-date"; 6146 /** 6147 * <b>Fluent Client</b> search parameter constant for <b>activity-date</b> 6148 * <p> 6149 * Description: <b>Specified date occurs within period specified by 6150 * CarePlan.activity.detail.scheduled[x]</b><br> 6151 * Type: <b>date</b><br> 6152 * Path: <b>CarePlan.activity.detail.scheduled[x]</b><br> 6153 * </p> 6154 */ 6155 public static final ca.uhn.fhir.rest.gclient.DateClientParam ACTIVITY_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6156 SP_ACTIVITY_DATE); 6157 6158 /** 6159 * Search parameter: <b>instantiates-uri</b> 6160 * <p> 6161 * Description: <b>Instantiates external protocol or definition</b><br> 6162 * Type: <b>uri</b><br> 6163 * Path: <b>CarePlan.instantiatesUri</b><br> 6164 * </p> 6165 */ 6166 @SearchParamDefinition(name = "instantiates-uri", path = "CarePlan.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 6167 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 6168 /** 6169 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 6170 * <p> 6171 * Description: <b>Instantiates external protocol or definition</b><br> 6172 * Type: <b>uri</b><br> 6173 * Path: <b>CarePlan.instantiatesUri</b><br> 6174 * </p> 6175 */ 6176 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 6177 SP_INSTANTIATES_URI); 6178 6179 /** 6180 * Search parameter: <b>category</b> 6181 * <p> 6182 * Description: <b>Type of plan</b><br> 6183 * Type: <b>token</b><br> 6184 * Path: <b>CarePlan.category</b><br> 6185 * </p> 6186 */ 6187 @SearchParamDefinition(name = "category", path = "CarePlan.category", description = "Type of plan", type = "token") 6188 public static final String SP_CATEGORY = "category"; 6189 /** 6190 * <b>Fluent Client</b> search parameter constant for <b>category</b> 6191 * <p> 6192 * Description: <b>Type of plan</b><br> 6193 * Type: <b>token</b><br> 6194 * Path: <b>CarePlan.category</b><br> 6195 * </p> 6196 */ 6197 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6198 SP_CATEGORY); 6199 6200 /** 6201 * Search parameter: <b>activity-code</b> 6202 * <p> 6203 * Description: <b>Detail type of activity</b><br> 6204 * Type: <b>token</b><br> 6205 * Path: <b>CarePlan.activity.detail.code</b><br> 6206 * </p> 6207 */ 6208 @SearchParamDefinition(name = "activity-code", path = "CarePlan.activity.detail.code", description = "Detail type of activity", type = "token") 6209 public static final String SP_ACTIVITY_CODE = "activity-code"; 6210 /** 6211 * <b>Fluent Client</b> search parameter constant for <b>activity-code</b> 6212 * <p> 6213 * Description: <b>Detail type of activity</b><br> 6214 * Type: <b>token</b><br> 6215 * Path: <b>CarePlan.activity.detail.code</b><br> 6216 * </p> 6217 */ 6218 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVITY_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6219 SP_ACTIVITY_CODE); 6220 6221 /** 6222 * Search parameter: <b>status</b> 6223 * <p> 6224 * Description: <b>draft | active | on-hold | revoked | completed | 6225 * entered-in-error | unknown</b><br> 6226 * Type: <b>token</b><br> 6227 * Path: <b>CarePlan.status</b><br> 6228 * </p> 6229 */ 6230 @SearchParamDefinition(name = "status", path = "CarePlan.status", description = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", type = "token") 6231 public static final String SP_STATUS = "status"; 6232 /** 6233 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6234 * <p> 6235 * Description: <b>draft | active | on-hold | revoked | completed | 6236 * entered-in-error | unknown</b><br> 6237 * Type: <b>token</b><br> 6238 * Path: <b>CarePlan.status</b><br> 6239 * </p> 6240 */ 6241 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6242 SP_STATUS); 6243 6244}