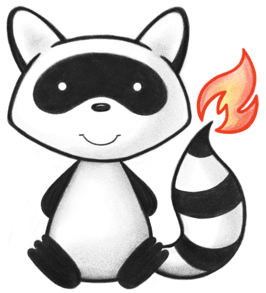
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The Care Team includes all the people and organizations who plan to 048 * participate in the coordination and delivery of care for a patient. 049 */ 050@ResourceDef(name = "CareTeam", profile = "http://hl7.org/fhir/StructureDefinition/CareTeam") 051public class CareTeam extends DomainResource { 052 053 public enum CareTeamStatus { 054 /** 055 * The care team has been drafted and proposed, but not yet participating in the 056 * coordination and delivery of patient care. 057 */ 058 PROPOSED, 059 /** 060 * The care team is currently participating in the coordination and delivery of 061 * care. 062 */ 063 ACTIVE, 064 /** 065 * The care team is temporarily on hold or suspended and not participating in 066 * the coordination and delivery of care. 067 */ 068 SUSPENDED, 069 /** 070 * The care team was, but is no longer, participating in the coordination and 071 * delivery of care. 072 */ 073 INACTIVE, 074 /** 075 * The care team should have never existed. 076 */ 077 ENTEREDINERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static CareTeamStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("proposed".equals(codeString)) 087 return PROPOSED; 088 if ("active".equals(codeString)) 089 return ACTIVE; 090 if ("suspended".equals(codeString)) 091 return SUSPENDED; 092 if ("inactive".equals(codeString)) 093 return INACTIVE; 094 if ("entered-in-error".equals(codeString)) 095 return ENTEREDINERROR; 096 if (Configuration.isAcceptInvalidEnums()) 097 return null; 098 else 099 throw new FHIRException("Unknown CareTeamStatus code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PROPOSED: 105 return "proposed"; 106 case ACTIVE: 107 return "active"; 108 case SUSPENDED: 109 return "suspended"; 110 case INACTIVE: 111 return "inactive"; 112 case ENTEREDINERROR: 113 return "entered-in-error"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 switch (this) { 123 case PROPOSED: 124 return "http://hl7.org/fhir/care-team-status"; 125 case ACTIVE: 126 return "http://hl7.org/fhir/care-team-status"; 127 case SUSPENDED: 128 return "http://hl7.org/fhir/care-team-status"; 129 case INACTIVE: 130 return "http://hl7.org/fhir/care-team-status"; 131 case ENTEREDINERROR: 132 return "http://hl7.org/fhir/care-team-status"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case PROPOSED: 143 return "The care team has been drafted and proposed, but not yet participating in the coordination and delivery of patient care."; 144 case ACTIVE: 145 return "The care team is currently participating in the coordination and delivery of care."; 146 case SUSPENDED: 147 return "The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care."; 148 case INACTIVE: 149 return "The care team was, but is no longer, participating in the coordination and delivery of care."; 150 case ENTEREDINERROR: 151 return "The care team should have never existed."; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDisplay() { 160 switch (this) { 161 case PROPOSED: 162 return "Proposed"; 163 case ACTIVE: 164 return "Active"; 165 case SUSPENDED: 166 return "Suspended"; 167 case INACTIVE: 168 return "Inactive"; 169 case ENTEREDINERROR: 170 return "Entered in Error"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 } 178 179 public static class CareTeamStatusEnumFactory implements EnumFactory<CareTeamStatus> { 180 public CareTeamStatus fromCode(String codeString) throws IllegalArgumentException { 181 if (codeString == null || "".equals(codeString)) 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("proposed".equals(codeString)) 185 return CareTeamStatus.PROPOSED; 186 if ("active".equals(codeString)) 187 return CareTeamStatus.ACTIVE; 188 if ("suspended".equals(codeString)) 189 return CareTeamStatus.SUSPENDED; 190 if ("inactive".equals(codeString)) 191 return CareTeamStatus.INACTIVE; 192 if ("entered-in-error".equals(codeString)) 193 return CareTeamStatus.ENTEREDINERROR; 194 throw new IllegalArgumentException("Unknown CareTeamStatus code '" + codeString + "'"); 195 } 196 197 public Enumeration<CareTeamStatus> fromType(PrimitiveType<?> code) throws FHIRException { 198 if (code == null) 199 return null; 200 if (code.isEmpty()) 201 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 202 String codeString = code.asStringValue(); 203 if (codeString == null || "".equals(codeString)) 204 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 205 if ("proposed".equals(codeString)) 206 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.PROPOSED, code); 207 if ("active".equals(codeString)) 208 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ACTIVE, code); 209 if ("suspended".equals(codeString)) 210 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.SUSPENDED, code); 211 if ("inactive".equals(codeString)) 212 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.INACTIVE, code); 213 if ("entered-in-error".equals(codeString)) 214 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ENTEREDINERROR, code); 215 throw new FHIRException("Unknown CareTeamStatus code '" + codeString + "'"); 216 } 217 218 public String toCode(CareTeamStatus code) { 219 if (code == CareTeamStatus.NULL) 220 return null; 221 if (code == CareTeamStatus.PROPOSED) 222 return "proposed"; 223 if (code == CareTeamStatus.ACTIVE) 224 return "active"; 225 if (code == CareTeamStatus.SUSPENDED) 226 return "suspended"; 227 if (code == CareTeamStatus.INACTIVE) 228 return "inactive"; 229 if (code == CareTeamStatus.ENTEREDINERROR) 230 return "entered-in-error"; 231 return "?"; 232 } 233 234 public String toSystem(CareTeamStatus code) { 235 return code.getSystem(); 236 } 237 } 238 239 @Block() 240 public static class CareTeamParticipantComponent extends BackboneElement implements IBaseBackboneElement { 241 /** 242 * Indicates specific responsibility of an individual within the care team, such 243 * as "Primary care physician", "Trained social worker counselor", "Caregiver", 244 * etc. 245 */ 246 @Child(name = "role", type = { 247 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 248 @Description(shortDefinition = "Type of involvement", formalDefinition = "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.") 249 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participant-role") 250 protected List<CodeableConcept> role; 251 252 /** 253 * The specific person or organization who is participating/expected to 254 * participate in the care team. 255 */ 256 @Child(name = "member", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 257 Organization.class, CareTeam.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 258 @Description(shortDefinition = "Who is involved", formalDefinition = "The specific person or organization who is participating/expected to participate in the care team.") 259 protected Reference member; 260 261 /** 262 * The actual object that is the target of the reference (The specific person or 263 * organization who is participating/expected to participate in the care team.) 264 */ 265 protected Resource memberTarget; 266 267 /** 268 * The organization of the practitioner. 269 */ 270 @Child(name = "onBehalfOf", type = { 271 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 272 @Description(shortDefinition = "Organization of the practitioner", formalDefinition = "The organization of the practitioner.") 273 protected Reference onBehalfOf; 274 275 /** 276 * The actual object that is the target of the reference (The organization of 277 * the practitioner.) 278 */ 279 protected Organization onBehalfOfTarget; 280 281 /** 282 * Indicates when the specific member or organization did (or is intended to) 283 * come into effect and end. 284 */ 285 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 286 @Description(shortDefinition = "Time period of participant", formalDefinition = "Indicates when the specific member or organization did (or is intended to) come into effect and end.") 287 protected Period period; 288 289 private static final long serialVersionUID = -575634410L; 290 291 /** 292 * Constructor 293 */ 294 public CareTeamParticipantComponent() { 295 super(); 296 } 297 298 /** 299 * @return {@link #role} (Indicates specific responsibility of an individual 300 * within the care team, such as "Primary care physician", "Trained 301 * social worker counselor", "Caregiver", etc.) 302 */ 303 public List<CodeableConcept> getRole() { 304 if (this.role == null) 305 this.role = new ArrayList<CodeableConcept>(); 306 return this.role; 307 } 308 309 /** 310 * @return Returns a reference to <code>this</code> for easy method chaining 311 */ 312 public CareTeamParticipantComponent setRole(List<CodeableConcept> theRole) { 313 this.role = theRole; 314 return this; 315 } 316 317 public boolean hasRole() { 318 if (this.role == null) 319 return false; 320 for (CodeableConcept item : this.role) 321 if (!item.isEmpty()) 322 return true; 323 return false; 324 } 325 326 public CodeableConcept addRole() { // 3 327 CodeableConcept t = new CodeableConcept(); 328 if (this.role == null) 329 this.role = new ArrayList<CodeableConcept>(); 330 this.role.add(t); 331 return t; 332 } 333 334 public CareTeamParticipantComponent addRole(CodeableConcept t) { // 3 335 if (t == null) 336 return this; 337 if (this.role == null) 338 this.role = new ArrayList<CodeableConcept>(); 339 this.role.add(t); 340 return this; 341 } 342 343 /** 344 * @return The first repetition of repeating field {@link #role}, creating it if 345 * it does not already exist 346 */ 347 public CodeableConcept getRoleFirstRep() { 348 if (getRole().isEmpty()) { 349 addRole(); 350 } 351 return getRole().get(0); 352 } 353 354 /** 355 * @return {@link #member} (The specific person or organization who is 356 * participating/expected to participate in the care team.) 357 */ 358 public Reference getMember() { 359 if (this.member == null) 360 if (Configuration.errorOnAutoCreate()) 361 throw new Error("Attempt to auto-create CareTeamParticipantComponent.member"); 362 else if (Configuration.doAutoCreate()) 363 this.member = new Reference(); // cc 364 return this.member; 365 } 366 367 public boolean hasMember() { 368 return this.member != null && !this.member.isEmpty(); 369 } 370 371 /** 372 * @param value {@link #member} (The specific person or organization who is 373 * participating/expected to participate in the care team.) 374 */ 375 public CareTeamParticipantComponent setMember(Reference value) { 376 this.member = value; 377 return this; 378 } 379 380 /** 381 * @return {@link #member} The actual object that is the target of the 382 * reference. The reference library doesn't populate this, but you can 383 * use it to hold the resource if you resolve it. (The specific person 384 * or organization who is participating/expected to participate in the 385 * care team.) 386 */ 387 public Resource getMemberTarget() { 388 return this.memberTarget; 389 } 390 391 /** 392 * @param value {@link #member} The actual object that is the target of the 393 * reference. The reference library doesn't use these, but you can 394 * use it to hold the resource if you resolve it. (The specific 395 * person or organization who is participating/expected to 396 * participate in the care team.) 397 */ 398 public CareTeamParticipantComponent setMemberTarget(Resource value) { 399 this.memberTarget = value; 400 return this; 401 } 402 403 /** 404 * @return {@link #onBehalfOf} (The organization of the practitioner.) 405 */ 406 public Reference getOnBehalfOf() { 407 if (this.onBehalfOf == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 410 else if (Configuration.doAutoCreate()) 411 this.onBehalfOf = new Reference(); // cc 412 return this.onBehalfOf; 413 } 414 415 public boolean hasOnBehalfOf() { 416 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #onBehalfOf} (The organization of the practitioner.) 421 */ 422 public CareTeamParticipantComponent setOnBehalfOf(Reference value) { 423 this.onBehalfOf = value; 424 return this; 425 } 426 427 /** 428 * @return {@link #onBehalfOf} The actual object that is the target of the 429 * reference. The reference library doesn't populate this, but you can 430 * use it to hold the resource if you resolve it. (The organization of 431 * the practitioner.) 432 */ 433 public Organization getOnBehalfOfTarget() { 434 if (this.onBehalfOfTarget == null) 435 if (Configuration.errorOnAutoCreate()) 436 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 437 else if (Configuration.doAutoCreate()) 438 this.onBehalfOfTarget = new Organization(); // aa 439 return this.onBehalfOfTarget; 440 } 441 442 /** 443 * @param value {@link #onBehalfOf} The actual object that is the target of the 444 * reference. The reference library doesn't use these, but you can 445 * use it to hold the resource if you resolve it. (The organization 446 * of the practitioner.) 447 */ 448 public CareTeamParticipantComponent setOnBehalfOfTarget(Organization value) { 449 this.onBehalfOfTarget = value; 450 return this; 451 } 452 453 /** 454 * @return {@link #period} (Indicates when the specific member or organization 455 * did (or is intended to) come into effect and end.) 456 */ 457 public Period getPeriod() { 458 if (this.period == null) 459 if (Configuration.errorOnAutoCreate()) 460 throw new Error("Attempt to auto-create CareTeamParticipantComponent.period"); 461 else if (Configuration.doAutoCreate()) 462 this.period = new Period(); // cc 463 return this.period; 464 } 465 466 public boolean hasPeriod() { 467 return this.period != null && !this.period.isEmpty(); 468 } 469 470 /** 471 * @param value {@link #period} (Indicates when the specific member or 472 * organization did (or is intended to) come into effect and end.) 473 */ 474 public CareTeamParticipantComponent setPeriod(Period value) { 475 this.period = value; 476 return this; 477 } 478 479 protected void listChildren(List<Property> children) { 480 super.listChildren(children); 481 children.add(new Property("role", "CodeableConcept", 482 "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 483 0, java.lang.Integer.MAX_VALUE, role)); 484 children.add( 485 new Property("member", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", 486 "The specific person or organization who is participating/expected to participate in the care team.", 0, 487 1, member)); 488 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, 489 onBehalfOf)); 490 children.add(new Property("period", "Period", 491 "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, 492 period)); 493 } 494 495 @Override 496 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 497 switch (_hash) { 498 case 3506294: 499 /* role */ return new Property("role", "CodeableConcept", 500 "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 501 0, java.lang.Integer.MAX_VALUE, role); 502 case -1077769574: 503 /* member */ return new Property("member", 504 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", 505 "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, 506 member); 507 case -14402964: 508 /* onBehalfOf */ return new Property("onBehalfOf", "Reference(Organization)", 509 "The organization of the practitioner.", 0, 1, onBehalfOf); 510 case -991726143: 511 /* period */ return new Property("period", "Period", 512 "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 513 1, period); 514 default: 515 return super.getNamedProperty(_hash, _name, _checkValid); 516 } 517 518 } 519 520 @Override 521 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 522 switch (hash) { 523 case 3506294: 524 /* role */ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 525 case -1077769574: 526 /* member */ return this.member == null ? new Base[0] : new Base[] { this.member }; // Reference 527 case -14402964: 528 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 529 case -991726143: 530 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 531 default: 532 return super.getProperty(hash, name, checkValid); 533 } 534 535 } 536 537 @Override 538 public Base setProperty(int hash, String name, Base value) throws FHIRException { 539 switch (hash) { 540 case 3506294: // role 541 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 542 return value; 543 case -1077769574: // member 544 this.member = castToReference(value); // Reference 545 return value; 546 case -14402964: // onBehalfOf 547 this.onBehalfOf = castToReference(value); // Reference 548 return value; 549 case -991726143: // period 550 this.period = castToPeriod(value); // Period 551 return value; 552 default: 553 return super.setProperty(hash, name, value); 554 } 555 556 } 557 558 @Override 559 public Base setProperty(String name, Base value) throws FHIRException { 560 if (name.equals("role")) { 561 this.getRole().add(castToCodeableConcept(value)); 562 } else if (name.equals("member")) { 563 this.member = castToReference(value); // Reference 564 } else if (name.equals("onBehalfOf")) { 565 this.onBehalfOf = castToReference(value); // Reference 566 } else if (name.equals("period")) { 567 this.period = castToPeriod(value); // Period 568 } else 569 return super.setProperty(name, value); 570 return value; 571 } 572 573 @Override 574 public void removeChild(String name, Base value) throws FHIRException { 575 if (name.equals("role")) { 576 this.getRole().remove(castToCodeableConcept(value)); 577 } else if (name.equals("member")) { 578 this.member = null; 579 } else if (name.equals("onBehalfOf")) { 580 this.onBehalfOf = null; 581 } else if (name.equals("period")) { 582 this.period = null; 583 } else 584 super.removeChild(name, value); 585 586 } 587 588 @Override 589 public Base makeProperty(int hash, String name) throws FHIRException { 590 switch (hash) { 591 case 3506294: 592 return addRole(); 593 case -1077769574: 594 return getMember(); 595 case -14402964: 596 return getOnBehalfOf(); 597 case -991726143: 598 return getPeriod(); 599 default: 600 return super.makeProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 607 switch (hash) { 608 case 3506294: 609 /* role */ return new String[] { "CodeableConcept" }; 610 case -1077769574: 611 /* member */ return new String[] { "Reference" }; 612 case -14402964: 613 /* onBehalfOf */ return new String[] { "Reference" }; 614 case -991726143: 615 /* period */ return new String[] { "Period" }; 616 default: 617 return super.getTypesForProperty(hash, name); 618 } 619 620 } 621 622 @Override 623 public Base addChild(String name) throws FHIRException { 624 if (name.equals("role")) { 625 return addRole(); 626 } else if (name.equals("member")) { 627 this.member = new Reference(); 628 return this.member; 629 } else if (name.equals("onBehalfOf")) { 630 this.onBehalfOf = new Reference(); 631 return this.onBehalfOf; 632 } else if (name.equals("period")) { 633 this.period = new Period(); 634 return this.period; 635 } else 636 return super.addChild(name); 637 } 638 639 public CareTeamParticipantComponent copy() { 640 CareTeamParticipantComponent dst = new CareTeamParticipantComponent(); 641 copyValues(dst); 642 return dst; 643 } 644 645 public void copyValues(CareTeamParticipantComponent dst) { 646 super.copyValues(dst); 647 if (role != null) { 648 dst.role = new ArrayList<CodeableConcept>(); 649 for (CodeableConcept i : role) 650 dst.role.add(i.copy()); 651 } 652 ; 653 dst.member = member == null ? null : member.copy(); 654 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 655 dst.period = period == null ? null : period.copy(); 656 } 657 658 @Override 659 public boolean equalsDeep(Base other_) { 660 if (!super.equalsDeep(other_)) 661 return false; 662 if (!(other_ instanceof CareTeamParticipantComponent)) 663 return false; 664 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 665 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true) 666 && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(period, o.period, true); 667 } 668 669 @Override 670 public boolean equalsShallow(Base other_) { 671 if (!super.equalsShallow(other_)) 672 return false; 673 if (!(other_ instanceof CareTeamParticipantComponent)) 674 return false; 675 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 676 return true; 677 } 678 679 public boolean isEmpty() { 680 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, member, onBehalfOf, period); 681 } 682 683 public String fhirType() { 684 return "CareTeam.participant"; 685 686 } 687 688 } 689 690 /** 691 * Business identifiers assigned to this care team by the performer or other 692 * systems which remain constant as the resource is updated and propagates from 693 * server to server. 694 */ 695 @Child(name = "identifier", type = { 696 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 697 @Description(shortDefinition = "External Ids for this team", formalDefinition = "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 698 protected List<Identifier> identifier; 699 700 /** 701 * Indicates the current state of the care team. 702 */ 703 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 704 @Description(shortDefinition = "proposed | active | suspended | inactive | entered-in-error", formalDefinition = "Indicates the current state of the care team.") 705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-team-status") 706 protected Enumeration<CareTeamStatus> status; 707 708 /** 709 * Identifies what kind of team. This is to support differentiation between 710 * multiple co-existing teams, such as care plan team, episode of care team, 711 * longitudinal care team. 712 */ 713 @Child(name = "category", type = { 714 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 715 @Description(shortDefinition = "Type of team", formalDefinition = "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.") 716 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-team-category") 717 protected List<CodeableConcept> category; 718 719 /** 720 * A label for human use intended to distinguish like teams. E.g. the "red" vs. 721 * "green" trauma teams. 722 */ 723 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 724 @Description(shortDefinition = "Name of the team, such as crisis assessment team", formalDefinition = "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.") 725 protected StringType name; 726 727 /** 728 * Identifies the patient or group whose intended care is handled by the team. 729 */ 730 @Child(name = "subject", type = { Patient.class, 731 Group.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 732 @Description(shortDefinition = "Who care team is for", formalDefinition = "Identifies the patient or group whose intended care is handled by the team.") 733 protected Reference subject; 734 735 /** 736 * The actual object that is the target of the reference (Identifies the patient 737 * or group whose intended care is handled by the team.) 738 */ 739 protected Resource subjectTarget; 740 741 /** 742 * The Encounter during which this CareTeam was created or to which the creation 743 * of this record is tightly associated. 744 */ 745 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 746 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.") 747 protected Reference encounter; 748 749 /** 750 * The actual object that is the target of the reference (The Encounter during 751 * which this CareTeam was created or to which the creation of this record is 752 * tightly associated.) 753 */ 754 protected Encounter encounterTarget; 755 756 /** 757 * Indicates when the team did (or is intended to) come into effect and end. 758 */ 759 @Child(name = "period", type = { Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 760 @Description(shortDefinition = "Time period team covers", formalDefinition = "Indicates when the team did (or is intended to) come into effect and end.") 761 protected Period period; 762 763 /** 764 * Identifies all people and organizations who are expected to be involved in 765 * the care team. 766 */ 767 @Child(name = "participant", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 768 @Description(shortDefinition = "Members of the team", formalDefinition = "Identifies all people and organizations who are expected to be involved in the care team.") 769 protected List<CareTeamParticipantComponent> participant; 770 771 /** 772 * Describes why the care team exists. 773 */ 774 @Child(name = "reasonCode", type = { 775 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 776 @Description(shortDefinition = "Why the care team exists", formalDefinition = "Describes why the care team exists.") 777 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 778 protected List<CodeableConcept> reasonCode; 779 780 /** 781 * Condition(s) that this care team addresses. 782 */ 783 @Child(name = "reasonReference", type = { 784 Condition.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 785 @Description(shortDefinition = "Why the care team exists", formalDefinition = "Condition(s) that this care team addresses.") 786 protected List<Reference> reasonReference; 787 /** 788 * The actual objects that are the target of the reference (Condition(s) that 789 * this care team addresses.) 790 */ 791 protected List<Condition> reasonReferenceTarget; 792 793 /** 794 * The organization responsible for the care team. 795 */ 796 @Child(name = "managingOrganization", type = { 797 Organization.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 798 @Description(shortDefinition = "Organization responsible for the care team", formalDefinition = "The organization responsible for the care team.") 799 protected List<Reference> managingOrganization; 800 /** 801 * The actual objects that are the target of the reference (The organization 802 * responsible for the care team.) 803 */ 804 protected List<Organization> managingOrganizationTarget; 805 806 /** 807 * A central contact detail for the care team (that applies to all members). 808 */ 809 @Child(name = "telecom", type = { 810 ContactPoint.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 811 @Description(shortDefinition = "A contact detail for the care team (that applies to all members)", formalDefinition = "A central contact detail for the care team (that applies to all members).") 812 protected List<ContactPoint> telecom; 813 814 /** 815 * Comments made about the CareTeam. 816 */ 817 @Child(name = "note", type = { 818 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 819 @Description(shortDefinition = "Comments made about the CareTeam", formalDefinition = "Comments made about the CareTeam.") 820 protected List<Annotation> note; 821 822 private static final long serialVersionUID = 1793069286L; 823 824 /** 825 * Constructor 826 */ 827 public CareTeam() { 828 super(); 829 } 830 831 /** 832 * @return {@link #identifier} (Business identifiers assigned to this care team 833 * by the performer or other systems which remain constant as the 834 * resource is updated and propagates from server to server.) 835 */ 836 public List<Identifier> getIdentifier() { 837 if (this.identifier == null) 838 this.identifier = new ArrayList<Identifier>(); 839 return this.identifier; 840 } 841 842 /** 843 * @return Returns a reference to <code>this</code> for easy method chaining 844 */ 845 public CareTeam setIdentifier(List<Identifier> theIdentifier) { 846 this.identifier = theIdentifier; 847 return this; 848 } 849 850 public boolean hasIdentifier() { 851 if (this.identifier == null) 852 return false; 853 for (Identifier item : this.identifier) 854 if (!item.isEmpty()) 855 return true; 856 return false; 857 } 858 859 public Identifier addIdentifier() { // 3 860 Identifier t = new Identifier(); 861 if (this.identifier == null) 862 this.identifier = new ArrayList<Identifier>(); 863 this.identifier.add(t); 864 return t; 865 } 866 867 public CareTeam addIdentifier(Identifier t) { // 3 868 if (t == null) 869 return this; 870 if (this.identifier == null) 871 this.identifier = new ArrayList<Identifier>(); 872 this.identifier.add(t); 873 return this; 874 } 875 876 /** 877 * @return The first repetition of repeating field {@link #identifier}, creating 878 * it if it does not already exist 879 */ 880 public Identifier getIdentifierFirstRep() { 881 if (getIdentifier().isEmpty()) { 882 addIdentifier(); 883 } 884 return getIdentifier().get(0); 885 } 886 887 /** 888 * @return {@link #status} (Indicates the current state of the care team.). This 889 * is the underlying object with id, value and extensions. The accessor 890 * "getStatus" gives direct access to the value 891 */ 892 public Enumeration<CareTeamStatus> getStatusElement() { 893 if (this.status == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create CareTeam.status"); 896 else if (Configuration.doAutoCreate()) 897 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); // bb 898 return this.status; 899 } 900 901 public boolean hasStatusElement() { 902 return this.status != null && !this.status.isEmpty(); 903 } 904 905 public boolean hasStatus() { 906 return this.status != null && !this.status.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #status} (Indicates the current state of the care team.). 911 * This is the underlying object with id, value and extensions. The 912 * accessor "getStatus" gives direct access to the value 913 */ 914 public CareTeam setStatusElement(Enumeration<CareTeamStatus> value) { 915 this.status = value; 916 return this; 917 } 918 919 /** 920 * @return Indicates the current state of the care team. 921 */ 922 public CareTeamStatus getStatus() { 923 return this.status == null ? null : this.status.getValue(); 924 } 925 926 /** 927 * @param value Indicates the current state of the care team. 928 */ 929 public CareTeam setStatus(CareTeamStatus value) { 930 if (value == null) 931 this.status = null; 932 else { 933 if (this.status == null) 934 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); 935 this.status.setValue(value); 936 } 937 return this; 938 } 939 940 /** 941 * @return {@link #category} (Identifies what kind of team. This is to support 942 * differentiation between multiple co-existing teams, such as care plan 943 * team, episode of care team, longitudinal care team.) 944 */ 945 public List<CodeableConcept> getCategory() { 946 if (this.category == null) 947 this.category = new ArrayList<CodeableConcept>(); 948 return this.category; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public CareTeam setCategory(List<CodeableConcept> theCategory) { 955 this.category = theCategory; 956 return this; 957 } 958 959 public boolean hasCategory() { 960 if (this.category == null) 961 return false; 962 for (CodeableConcept item : this.category) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 public CodeableConcept addCategory() { // 3 969 CodeableConcept t = new CodeableConcept(); 970 if (this.category == null) 971 this.category = new ArrayList<CodeableConcept>(); 972 this.category.add(t); 973 return t; 974 } 975 976 public CareTeam addCategory(CodeableConcept t) { // 3 977 if (t == null) 978 return this; 979 if (this.category == null) 980 this.category = new ArrayList<CodeableConcept>(); 981 this.category.add(t); 982 return this; 983 } 984 985 /** 986 * @return The first repetition of repeating field {@link #category}, creating 987 * it if it does not already exist 988 */ 989 public CodeableConcept getCategoryFirstRep() { 990 if (getCategory().isEmpty()) { 991 addCategory(); 992 } 993 return getCategory().get(0); 994 } 995 996 /** 997 * @return {@link #name} (A label for human use intended to distinguish like 998 * teams. E.g. the "red" vs. "green" trauma teams.). This is the 999 * underlying object with id, value and extensions. The accessor 1000 * "getName" gives direct access to the value 1001 */ 1002 public StringType getNameElement() { 1003 if (this.name == null) 1004 if (Configuration.errorOnAutoCreate()) 1005 throw new Error("Attempt to auto-create CareTeam.name"); 1006 else if (Configuration.doAutoCreate()) 1007 this.name = new StringType(); // bb 1008 return this.name; 1009 } 1010 1011 public boolean hasNameElement() { 1012 return this.name != null && !this.name.isEmpty(); 1013 } 1014 1015 public boolean hasName() { 1016 return this.name != null && !this.name.isEmpty(); 1017 } 1018 1019 /** 1020 * @param value {@link #name} (A label for human use intended to distinguish 1021 * like teams. E.g. the "red" vs. "green" trauma teams.). This is 1022 * the underlying object with id, value and extensions. The 1023 * accessor "getName" gives direct access to the value 1024 */ 1025 public CareTeam setNameElement(StringType value) { 1026 this.name = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return A label for human use intended to distinguish like teams. E.g. the 1032 * "red" vs. "green" trauma teams. 1033 */ 1034 public String getName() { 1035 return this.name == null ? null : this.name.getValue(); 1036 } 1037 1038 /** 1039 * @param value A label for human use intended to distinguish like teams. E.g. 1040 * the "red" vs. "green" trauma teams. 1041 */ 1042 public CareTeam setName(String value) { 1043 if (Utilities.noString(value)) 1044 this.name = null; 1045 else { 1046 if (this.name == null) 1047 this.name = new StringType(); 1048 this.name.setValue(value); 1049 } 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #subject} (Identifies the patient or group whose intended care 1055 * is handled by the team.) 1056 */ 1057 public Reference getSubject() { 1058 if (this.subject == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create CareTeam.subject"); 1061 else if (Configuration.doAutoCreate()) 1062 this.subject = new Reference(); // cc 1063 return this.subject; 1064 } 1065 1066 public boolean hasSubject() { 1067 return this.subject != null && !this.subject.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #subject} (Identifies the patient or group whose intended 1072 * care is handled by the team.) 1073 */ 1074 public CareTeam setSubject(Reference value) { 1075 this.subject = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #subject} The actual object that is the target of the 1081 * reference. The reference library doesn't populate this, but you can 1082 * use it to hold the resource if you resolve it. (Identifies the 1083 * patient or group whose intended care is handled by the team.) 1084 */ 1085 public Resource getSubjectTarget() { 1086 return this.subjectTarget; 1087 } 1088 1089 /** 1090 * @param value {@link #subject} The actual object that is the target of the 1091 * reference. The reference library doesn't use these, but you can 1092 * use it to hold the resource if you resolve it. (Identifies the 1093 * patient or group whose intended care is handled by the team.) 1094 */ 1095 public CareTeam setSubjectTarget(Resource value) { 1096 this.subjectTarget = value; 1097 return this; 1098 } 1099 1100 /** 1101 * @return {@link #encounter} (The Encounter during which this CareTeam was 1102 * created or to which the creation of this record is tightly 1103 * associated.) 1104 */ 1105 public Reference getEncounter() { 1106 if (this.encounter == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create CareTeam.encounter"); 1109 else if (Configuration.doAutoCreate()) 1110 this.encounter = new Reference(); // cc 1111 return this.encounter; 1112 } 1113 1114 public boolean hasEncounter() { 1115 return this.encounter != null && !this.encounter.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #encounter} (The Encounter during which this CareTeam was 1120 * created or to which the creation of this record is tightly 1121 * associated.) 1122 */ 1123 public CareTeam setEncounter(Reference value) { 1124 this.encounter = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #encounter} The actual object that is the target of the 1130 * reference. The reference library doesn't populate this, but you can 1131 * use it to hold the resource if you resolve it. (The Encounter during 1132 * which this CareTeam was created or to which the creation of this 1133 * record is tightly associated.) 1134 */ 1135 public Encounter getEncounterTarget() { 1136 if (this.encounterTarget == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create CareTeam.encounter"); 1139 else if (Configuration.doAutoCreate()) 1140 this.encounterTarget = new Encounter(); // aa 1141 return this.encounterTarget; 1142 } 1143 1144 /** 1145 * @param value {@link #encounter} The actual object that is the target of the 1146 * reference. The reference library doesn't use these, but you can 1147 * use it to hold the resource if you resolve it. (The Encounter 1148 * during which this CareTeam was created or to which the creation 1149 * of this record is tightly associated.) 1150 */ 1151 public CareTeam setEncounterTarget(Encounter value) { 1152 this.encounterTarget = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #period} (Indicates when the team did (or is intended to) come 1158 * into effect and end.) 1159 */ 1160 public Period getPeriod() { 1161 if (this.period == null) 1162 if (Configuration.errorOnAutoCreate()) 1163 throw new Error("Attempt to auto-create CareTeam.period"); 1164 else if (Configuration.doAutoCreate()) 1165 this.period = new Period(); // cc 1166 return this.period; 1167 } 1168 1169 public boolean hasPeriod() { 1170 return this.period != null && !this.period.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #period} (Indicates when the team did (or is intended to) 1175 * come into effect and end.) 1176 */ 1177 public CareTeam setPeriod(Period value) { 1178 this.period = value; 1179 return this; 1180 } 1181 1182 /** 1183 * @return {@link #participant} (Identifies all people and organizations who are 1184 * expected to be involved in the care team.) 1185 */ 1186 public List<CareTeamParticipantComponent> getParticipant() { 1187 if (this.participant == null) 1188 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1189 return this.participant; 1190 } 1191 1192 /** 1193 * @return Returns a reference to <code>this</code> for easy method chaining 1194 */ 1195 public CareTeam setParticipant(List<CareTeamParticipantComponent> theParticipant) { 1196 this.participant = theParticipant; 1197 return this; 1198 } 1199 1200 public boolean hasParticipant() { 1201 if (this.participant == null) 1202 return false; 1203 for (CareTeamParticipantComponent item : this.participant) 1204 if (!item.isEmpty()) 1205 return true; 1206 return false; 1207 } 1208 1209 public CareTeamParticipantComponent addParticipant() { // 3 1210 CareTeamParticipantComponent t = new CareTeamParticipantComponent(); 1211 if (this.participant == null) 1212 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1213 this.participant.add(t); 1214 return t; 1215 } 1216 1217 public CareTeam addParticipant(CareTeamParticipantComponent t) { // 3 1218 if (t == null) 1219 return this; 1220 if (this.participant == null) 1221 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1222 this.participant.add(t); 1223 return this; 1224 } 1225 1226 /** 1227 * @return The first repetition of repeating field {@link #participant}, 1228 * creating it if it does not already exist 1229 */ 1230 public CareTeamParticipantComponent getParticipantFirstRep() { 1231 if (getParticipant().isEmpty()) { 1232 addParticipant(); 1233 } 1234 return getParticipant().get(0); 1235 } 1236 1237 /** 1238 * @return {@link #reasonCode} (Describes why the care team exists.) 1239 */ 1240 public List<CodeableConcept> getReasonCode() { 1241 if (this.reasonCode == null) 1242 this.reasonCode = new ArrayList<CodeableConcept>(); 1243 return this.reasonCode; 1244 } 1245 1246 /** 1247 * @return Returns a reference to <code>this</code> for easy method chaining 1248 */ 1249 public CareTeam setReasonCode(List<CodeableConcept> theReasonCode) { 1250 this.reasonCode = theReasonCode; 1251 return this; 1252 } 1253 1254 public boolean hasReasonCode() { 1255 if (this.reasonCode == null) 1256 return false; 1257 for (CodeableConcept item : this.reasonCode) 1258 if (!item.isEmpty()) 1259 return true; 1260 return false; 1261 } 1262 1263 public CodeableConcept addReasonCode() { // 3 1264 CodeableConcept t = new CodeableConcept(); 1265 if (this.reasonCode == null) 1266 this.reasonCode = new ArrayList<CodeableConcept>(); 1267 this.reasonCode.add(t); 1268 return t; 1269 } 1270 1271 public CareTeam addReasonCode(CodeableConcept t) { // 3 1272 if (t == null) 1273 return this; 1274 if (this.reasonCode == null) 1275 this.reasonCode = new ArrayList<CodeableConcept>(); 1276 this.reasonCode.add(t); 1277 return this; 1278 } 1279 1280 /** 1281 * @return The first repetition of repeating field {@link #reasonCode}, creating 1282 * it if it does not already exist 1283 */ 1284 public CodeableConcept getReasonCodeFirstRep() { 1285 if (getReasonCode().isEmpty()) { 1286 addReasonCode(); 1287 } 1288 return getReasonCode().get(0); 1289 } 1290 1291 /** 1292 * @return {@link #reasonReference} (Condition(s) that this care team 1293 * addresses.) 1294 */ 1295 public List<Reference> getReasonReference() { 1296 if (this.reasonReference == null) 1297 this.reasonReference = new ArrayList<Reference>(); 1298 return this.reasonReference; 1299 } 1300 1301 /** 1302 * @return Returns a reference to <code>this</code> for easy method chaining 1303 */ 1304 public CareTeam setReasonReference(List<Reference> theReasonReference) { 1305 this.reasonReference = theReasonReference; 1306 return this; 1307 } 1308 1309 public boolean hasReasonReference() { 1310 if (this.reasonReference == null) 1311 return false; 1312 for (Reference item : this.reasonReference) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 public Reference addReasonReference() { // 3 1319 Reference t = new Reference(); 1320 if (this.reasonReference == null) 1321 this.reasonReference = new ArrayList<Reference>(); 1322 this.reasonReference.add(t); 1323 return t; 1324 } 1325 1326 public CareTeam addReasonReference(Reference t) { // 3 1327 if (t == null) 1328 return this; 1329 if (this.reasonReference == null) 1330 this.reasonReference = new ArrayList<Reference>(); 1331 this.reasonReference.add(t); 1332 return this; 1333 } 1334 1335 /** 1336 * @return The first repetition of repeating field {@link #reasonReference}, 1337 * creating it if it does not already exist 1338 */ 1339 public Reference getReasonReferenceFirstRep() { 1340 if (getReasonReference().isEmpty()) { 1341 addReasonReference(); 1342 } 1343 return getReasonReference().get(0); 1344 } 1345 1346 /** 1347 * @deprecated Use Reference#setResource(IBaseResource) instead 1348 */ 1349 @Deprecated 1350 public List<Condition> getReasonReferenceTarget() { 1351 if (this.reasonReferenceTarget == null) 1352 this.reasonReferenceTarget = new ArrayList<Condition>(); 1353 return this.reasonReferenceTarget; 1354 } 1355 1356 /** 1357 * @deprecated Use Reference#setResource(IBaseResource) instead 1358 */ 1359 @Deprecated 1360 public Condition addReasonReferenceTarget() { 1361 Condition r = new Condition(); 1362 if (this.reasonReferenceTarget == null) 1363 this.reasonReferenceTarget = new ArrayList<Condition>(); 1364 this.reasonReferenceTarget.add(r); 1365 return r; 1366 } 1367 1368 /** 1369 * @return {@link #managingOrganization} (The organization responsible for the 1370 * care team.) 1371 */ 1372 public List<Reference> getManagingOrganization() { 1373 if (this.managingOrganization == null) 1374 this.managingOrganization = new ArrayList<Reference>(); 1375 return this.managingOrganization; 1376 } 1377 1378 /** 1379 * @return Returns a reference to <code>this</code> for easy method chaining 1380 */ 1381 public CareTeam setManagingOrganization(List<Reference> theManagingOrganization) { 1382 this.managingOrganization = theManagingOrganization; 1383 return this; 1384 } 1385 1386 public boolean hasManagingOrganization() { 1387 if (this.managingOrganization == null) 1388 return false; 1389 for (Reference item : this.managingOrganization) 1390 if (!item.isEmpty()) 1391 return true; 1392 return false; 1393 } 1394 1395 public Reference addManagingOrganization() { // 3 1396 Reference t = new Reference(); 1397 if (this.managingOrganization == null) 1398 this.managingOrganization = new ArrayList<Reference>(); 1399 this.managingOrganization.add(t); 1400 return t; 1401 } 1402 1403 public CareTeam addManagingOrganization(Reference t) { // 3 1404 if (t == null) 1405 return this; 1406 if (this.managingOrganization == null) 1407 this.managingOrganization = new ArrayList<Reference>(); 1408 this.managingOrganization.add(t); 1409 return this; 1410 } 1411 1412 /** 1413 * @return The first repetition of repeating field 1414 * {@link #managingOrganization}, creating it if it does not already 1415 * exist 1416 */ 1417 public Reference getManagingOrganizationFirstRep() { 1418 if (getManagingOrganization().isEmpty()) { 1419 addManagingOrganization(); 1420 } 1421 return getManagingOrganization().get(0); 1422 } 1423 1424 /** 1425 * @deprecated Use Reference#setResource(IBaseResource) instead 1426 */ 1427 @Deprecated 1428 public List<Organization> getManagingOrganizationTarget() { 1429 if (this.managingOrganizationTarget == null) 1430 this.managingOrganizationTarget = new ArrayList<Organization>(); 1431 return this.managingOrganizationTarget; 1432 } 1433 1434 /** 1435 * @deprecated Use Reference#setResource(IBaseResource) instead 1436 */ 1437 @Deprecated 1438 public Organization addManagingOrganizationTarget() { 1439 Organization r = new Organization(); 1440 if (this.managingOrganizationTarget == null) 1441 this.managingOrganizationTarget = new ArrayList<Organization>(); 1442 this.managingOrganizationTarget.add(r); 1443 return r; 1444 } 1445 1446 /** 1447 * @return {@link #telecom} (A central contact detail for the care team (that 1448 * applies to all members).) 1449 */ 1450 public List<ContactPoint> getTelecom() { 1451 if (this.telecom == null) 1452 this.telecom = new ArrayList<ContactPoint>(); 1453 return this.telecom; 1454 } 1455 1456 /** 1457 * @return Returns a reference to <code>this</code> for easy method chaining 1458 */ 1459 public CareTeam setTelecom(List<ContactPoint> theTelecom) { 1460 this.telecom = theTelecom; 1461 return this; 1462 } 1463 1464 public boolean hasTelecom() { 1465 if (this.telecom == null) 1466 return false; 1467 for (ContactPoint item : this.telecom) 1468 if (!item.isEmpty()) 1469 return true; 1470 return false; 1471 } 1472 1473 public ContactPoint addTelecom() { // 3 1474 ContactPoint t = new ContactPoint(); 1475 if (this.telecom == null) 1476 this.telecom = new ArrayList<ContactPoint>(); 1477 this.telecom.add(t); 1478 return t; 1479 } 1480 1481 public CareTeam addTelecom(ContactPoint t) { // 3 1482 if (t == null) 1483 return this; 1484 if (this.telecom == null) 1485 this.telecom = new ArrayList<ContactPoint>(); 1486 this.telecom.add(t); 1487 return this; 1488 } 1489 1490 /** 1491 * @return The first repetition of repeating field {@link #telecom}, creating it 1492 * if it does not already exist 1493 */ 1494 public ContactPoint getTelecomFirstRep() { 1495 if (getTelecom().isEmpty()) { 1496 addTelecom(); 1497 } 1498 return getTelecom().get(0); 1499 } 1500 1501 /** 1502 * @return {@link #note} (Comments made about the CareTeam.) 1503 */ 1504 public List<Annotation> getNote() { 1505 if (this.note == null) 1506 this.note = new ArrayList<Annotation>(); 1507 return this.note; 1508 } 1509 1510 /** 1511 * @return Returns a reference to <code>this</code> for easy method chaining 1512 */ 1513 public CareTeam setNote(List<Annotation> theNote) { 1514 this.note = theNote; 1515 return this; 1516 } 1517 1518 public boolean hasNote() { 1519 if (this.note == null) 1520 return false; 1521 for (Annotation item : this.note) 1522 if (!item.isEmpty()) 1523 return true; 1524 return false; 1525 } 1526 1527 public Annotation addNote() { // 3 1528 Annotation t = new Annotation(); 1529 if (this.note == null) 1530 this.note = new ArrayList<Annotation>(); 1531 this.note.add(t); 1532 return t; 1533 } 1534 1535 public CareTeam addNote(Annotation t) { // 3 1536 if (t == null) 1537 return this; 1538 if (this.note == null) 1539 this.note = new ArrayList<Annotation>(); 1540 this.note.add(t); 1541 return this; 1542 } 1543 1544 /** 1545 * @return The first repetition of repeating field {@link #note}, creating it if 1546 * it does not already exist 1547 */ 1548 public Annotation getNoteFirstRep() { 1549 if (getNote().isEmpty()) { 1550 addNote(); 1551 } 1552 return getNote().get(0); 1553 } 1554 1555 protected void listChildren(List<Property> children) { 1556 super.listChildren(children); 1557 children.add(new Property("identifier", "Identifier", 1558 "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1559 0, java.lang.Integer.MAX_VALUE, identifier)); 1560 children.add(new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status)); 1561 children.add(new Property("category", "CodeableConcept", 1562 "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 1563 0, java.lang.Integer.MAX_VALUE, category)); 1564 children.add(new Property("name", "string", 1565 "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, 1566 name)); 1567 children.add(new Property("subject", "Reference(Patient|Group)", 1568 "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject)); 1569 children.add(new Property("encounter", "Reference(Encounter)", 1570 "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.", 1571 0, 1, encounter)); 1572 children.add(new Property("period", "Period", 1573 "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period)); 1574 children.add(new Property("participant", "", 1575 "Identifies all people and organizations who are expected to be involved in the care team.", 0, 1576 java.lang.Integer.MAX_VALUE, participant)); 1577 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, 1578 java.lang.Integer.MAX_VALUE, reasonCode)); 1579 children.add(new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 1580 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1581 children.add(new Property("managingOrganization", "Reference(Organization)", 1582 "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization)); 1583 children.add(new Property("telecom", "ContactPoint", 1584 "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, 1585 telecom)); 1586 children.add( 1587 new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note)); 1588 } 1589 1590 @Override 1591 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1592 switch (_hash) { 1593 case -1618432855: 1594 /* identifier */ return new Property("identifier", "Identifier", 1595 "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1596 0, java.lang.Integer.MAX_VALUE, identifier); 1597 case -892481550: 1598 /* status */ return new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status); 1599 case 50511102: 1600 /* category */ return new Property("category", "CodeableConcept", 1601 "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 1602 0, java.lang.Integer.MAX_VALUE, category); 1603 case 3373707: 1604 /* name */ return new Property("name", "string", 1605 "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1606 1, name); 1607 case -1867885268: 1608 /* subject */ return new Property("subject", "Reference(Patient|Group)", 1609 "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject); 1610 case 1524132147: 1611 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1612 "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.", 1613 0, 1, encounter); 1614 case -991726143: 1615 /* period */ return new Property("period", "Period", 1616 "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period); 1617 case 767422259: 1618 /* participant */ return new Property("participant", "", 1619 "Identifies all people and organizations who are expected to be involved in the care team.", 0, 1620 java.lang.Integer.MAX_VALUE, participant); 1621 case 722137681: 1622 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, 1623 java.lang.Integer.MAX_VALUE, reasonCode); 1624 case -1146218137: 1625 /* reasonReference */ return new Property("reasonReference", "Reference(Condition)", 1626 "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1627 case -2058947787: 1628 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1629 "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization); 1630 case -1429363305: 1631 /* telecom */ return new Property("telecom", "ContactPoint", 1632 "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, 1633 telecom); 1634 case 3387378: 1635 /* note */ return new Property("note", "Annotation", "Comments made about the CareTeam.", 0, 1636 java.lang.Integer.MAX_VALUE, note); 1637 default: 1638 return super.getNamedProperty(_hash, _name, _checkValid); 1639 } 1640 1641 } 1642 1643 @Override 1644 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1645 switch (hash) { 1646 case -1618432855: 1647 /* identifier */ return this.identifier == null ? new Base[0] 1648 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1649 case -892481550: 1650 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CareTeamStatus> 1651 case 50511102: 1652 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1653 case 3373707: 1654 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1655 case -1867885268: 1656 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1657 case 1524132147: 1658 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1659 case -991726143: 1660 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1661 case 767422259: 1662 /* participant */ return this.participant == null ? new Base[0] 1663 : this.participant.toArray(new Base[this.participant.size()]); // CareTeamParticipantComponent 1664 case 722137681: 1665 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1666 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1667 case -1146218137: 1668 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1669 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1670 case -2058947787: 1671 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1672 : this.managingOrganization.toArray(new Base[this.managingOrganization.size()]); // Reference 1673 case -1429363305: 1674 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1675 case 3387378: 1676 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1677 default: 1678 return super.getProperty(hash, name, checkValid); 1679 } 1680 1681 } 1682 1683 @Override 1684 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1685 switch (hash) { 1686 case -1618432855: // identifier 1687 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1688 return value; 1689 case -892481550: // status 1690 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1691 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1692 return value; 1693 case 50511102: // category 1694 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1695 return value; 1696 case 3373707: // name 1697 this.name = castToString(value); // StringType 1698 return value; 1699 case -1867885268: // subject 1700 this.subject = castToReference(value); // Reference 1701 return value; 1702 case 1524132147: // encounter 1703 this.encounter = castToReference(value); // Reference 1704 return value; 1705 case -991726143: // period 1706 this.period = castToPeriod(value); // Period 1707 return value; 1708 case 767422259: // participant 1709 this.getParticipant().add((CareTeamParticipantComponent) value); // CareTeamParticipantComponent 1710 return value; 1711 case 722137681: // reasonCode 1712 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1713 return value; 1714 case -1146218137: // reasonReference 1715 this.getReasonReference().add(castToReference(value)); // Reference 1716 return value; 1717 case -2058947787: // managingOrganization 1718 this.getManagingOrganization().add(castToReference(value)); // Reference 1719 return value; 1720 case -1429363305: // telecom 1721 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1722 return value; 1723 case 3387378: // note 1724 this.getNote().add(castToAnnotation(value)); // Annotation 1725 return value; 1726 default: 1727 return super.setProperty(hash, name, value); 1728 } 1729 1730 } 1731 1732 @Override 1733 public Base setProperty(String name, Base value) throws FHIRException { 1734 if (name.equals("identifier")) { 1735 this.getIdentifier().add(castToIdentifier(value)); 1736 } else if (name.equals("status")) { 1737 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1738 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1739 } else if (name.equals("category")) { 1740 this.getCategory().add(castToCodeableConcept(value)); 1741 } else if (name.equals("name")) { 1742 this.name = castToString(value); // StringType 1743 } else if (name.equals("subject")) { 1744 this.subject = castToReference(value); // Reference 1745 } else if (name.equals("encounter")) { 1746 this.encounter = castToReference(value); // Reference 1747 } else if (name.equals("period")) { 1748 this.period = castToPeriod(value); // Period 1749 } else if (name.equals("participant")) { 1750 this.getParticipant().add((CareTeamParticipantComponent) value); 1751 } else if (name.equals("reasonCode")) { 1752 this.getReasonCode().add(castToCodeableConcept(value)); 1753 } else if (name.equals("reasonReference")) { 1754 this.getReasonReference().add(castToReference(value)); 1755 } else if (name.equals("managingOrganization")) { 1756 this.getManagingOrganization().add(castToReference(value)); 1757 } else if (name.equals("telecom")) { 1758 this.getTelecom().add(castToContactPoint(value)); 1759 } else if (name.equals("note")) { 1760 this.getNote().add(castToAnnotation(value)); 1761 } else 1762 return super.setProperty(name, value); 1763 return value; 1764 } 1765 1766 @Override 1767 public void removeChild(String name, Base value) throws FHIRException { 1768 if (name.equals("identifier")) { 1769 this.getIdentifier().remove(castToIdentifier(value)); 1770 } else if (name.equals("status")) { 1771 this.status = null; 1772 } else if (name.equals("category")) { 1773 this.getCategory().remove(castToCodeableConcept(value)); 1774 } else if (name.equals("name")) { 1775 this.name = null; 1776 } else if (name.equals("subject")) { 1777 this.subject = null; 1778 } else if (name.equals("encounter")) { 1779 this.encounter = null; 1780 } else if (name.equals("period")) { 1781 this.period = null; 1782 } else if (name.equals("participant")) { 1783 this.getParticipant().remove((CareTeamParticipantComponent) value); 1784 } else if (name.equals("reasonCode")) { 1785 this.getReasonCode().remove(castToCodeableConcept(value)); 1786 } else if (name.equals("reasonReference")) { 1787 this.getReasonReference().remove(castToReference(value)); 1788 } else if (name.equals("managingOrganization")) { 1789 this.getManagingOrganization().remove(castToReference(value)); 1790 } else if (name.equals("telecom")) { 1791 this.getTelecom().remove(castToContactPoint(value)); 1792 } else if (name.equals("note")) { 1793 this.getNote().remove(castToAnnotation(value)); 1794 } else 1795 super.removeChild(name, value); 1796 1797 } 1798 1799 @Override 1800 public Base makeProperty(int hash, String name) throws FHIRException { 1801 switch (hash) { 1802 case -1618432855: 1803 return addIdentifier(); 1804 case -892481550: 1805 return getStatusElement(); 1806 case 50511102: 1807 return addCategory(); 1808 case 3373707: 1809 return getNameElement(); 1810 case -1867885268: 1811 return getSubject(); 1812 case 1524132147: 1813 return getEncounter(); 1814 case -991726143: 1815 return getPeriod(); 1816 case 767422259: 1817 return addParticipant(); 1818 case 722137681: 1819 return addReasonCode(); 1820 case -1146218137: 1821 return addReasonReference(); 1822 case -2058947787: 1823 return addManagingOrganization(); 1824 case -1429363305: 1825 return addTelecom(); 1826 case 3387378: 1827 return addNote(); 1828 default: 1829 return super.makeProperty(hash, name); 1830 } 1831 1832 } 1833 1834 @Override 1835 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1836 switch (hash) { 1837 case -1618432855: 1838 /* identifier */ return new String[] { "Identifier" }; 1839 case -892481550: 1840 /* status */ return new String[] { "code" }; 1841 case 50511102: 1842 /* category */ return new String[] { "CodeableConcept" }; 1843 case 3373707: 1844 /* name */ return new String[] { "string" }; 1845 case -1867885268: 1846 /* subject */ return new String[] { "Reference" }; 1847 case 1524132147: 1848 /* encounter */ return new String[] { "Reference" }; 1849 case -991726143: 1850 /* period */ return new String[] { "Period" }; 1851 case 767422259: 1852 /* participant */ return new String[] {}; 1853 case 722137681: 1854 /* reasonCode */ return new String[] { "CodeableConcept" }; 1855 case -1146218137: 1856 /* reasonReference */ return new String[] { "Reference" }; 1857 case -2058947787: 1858 /* managingOrganization */ return new String[] { "Reference" }; 1859 case -1429363305: 1860 /* telecom */ return new String[] { "ContactPoint" }; 1861 case 3387378: 1862 /* note */ return new String[] { "Annotation" }; 1863 default: 1864 return super.getTypesForProperty(hash, name); 1865 } 1866 1867 } 1868 1869 @Override 1870 public Base addChild(String name) throws FHIRException { 1871 if (name.equals("identifier")) { 1872 return addIdentifier(); 1873 } else if (name.equals("status")) { 1874 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.status"); 1875 } else if (name.equals("category")) { 1876 return addCategory(); 1877 } else if (name.equals("name")) { 1878 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.name"); 1879 } else if (name.equals("subject")) { 1880 this.subject = new Reference(); 1881 return this.subject; 1882 } else if (name.equals("encounter")) { 1883 this.encounter = new Reference(); 1884 return this.encounter; 1885 } else if (name.equals("period")) { 1886 this.period = new Period(); 1887 return this.period; 1888 } else if (name.equals("participant")) { 1889 return addParticipant(); 1890 } else if (name.equals("reasonCode")) { 1891 return addReasonCode(); 1892 } else if (name.equals("reasonReference")) { 1893 return addReasonReference(); 1894 } else if (name.equals("managingOrganization")) { 1895 return addManagingOrganization(); 1896 } else if (name.equals("telecom")) { 1897 return addTelecom(); 1898 } else if (name.equals("note")) { 1899 return addNote(); 1900 } else 1901 return super.addChild(name); 1902 } 1903 1904 public String fhirType() { 1905 return "CareTeam"; 1906 1907 } 1908 1909 public CareTeam copy() { 1910 CareTeam dst = new CareTeam(); 1911 copyValues(dst); 1912 return dst; 1913 } 1914 1915 public void copyValues(CareTeam dst) { 1916 super.copyValues(dst); 1917 if (identifier != null) { 1918 dst.identifier = new ArrayList<Identifier>(); 1919 for (Identifier i : identifier) 1920 dst.identifier.add(i.copy()); 1921 } 1922 ; 1923 dst.status = status == null ? null : status.copy(); 1924 if (category != null) { 1925 dst.category = new ArrayList<CodeableConcept>(); 1926 for (CodeableConcept i : category) 1927 dst.category.add(i.copy()); 1928 } 1929 ; 1930 dst.name = name == null ? null : name.copy(); 1931 dst.subject = subject == null ? null : subject.copy(); 1932 dst.encounter = encounter == null ? null : encounter.copy(); 1933 dst.period = period == null ? null : period.copy(); 1934 if (participant != null) { 1935 dst.participant = new ArrayList<CareTeamParticipantComponent>(); 1936 for (CareTeamParticipantComponent i : participant) 1937 dst.participant.add(i.copy()); 1938 } 1939 ; 1940 if (reasonCode != null) { 1941 dst.reasonCode = new ArrayList<CodeableConcept>(); 1942 for (CodeableConcept i : reasonCode) 1943 dst.reasonCode.add(i.copy()); 1944 } 1945 ; 1946 if (reasonReference != null) { 1947 dst.reasonReference = new ArrayList<Reference>(); 1948 for (Reference i : reasonReference) 1949 dst.reasonReference.add(i.copy()); 1950 } 1951 ; 1952 if (managingOrganization != null) { 1953 dst.managingOrganization = new ArrayList<Reference>(); 1954 for (Reference i : managingOrganization) 1955 dst.managingOrganization.add(i.copy()); 1956 } 1957 ; 1958 if (telecom != null) { 1959 dst.telecom = new ArrayList<ContactPoint>(); 1960 for (ContactPoint i : telecom) 1961 dst.telecom.add(i.copy()); 1962 } 1963 ; 1964 if (note != null) { 1965 dst.note = new ArrayList<Annotation>(); 1966 for (Annotation i : note) 1967 dst.note.add(i.copy()); 1968 } 1969 ; 1970 } 1971 1972 protected CareTeam typedCopy() { 1973 return copy(); 1974 } 1975 1976 @Override 1977 public boolean equalsDeep(Base other_) { 1978 if (!super.equalsDeep(other_)) 1979 return false; 1980 if (!(other_ instanceof CareTeam)) 1981 return false; 1982 CareTeam o = (CareTeam) other_; 1983 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1984 && compareDeep(category, o.category, true) && compareDeep(name, o.name, true) 1985 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1986 && compareDeep(period, o.period, true) && compareDeep(participant, o.participant, true) 1987 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 1988 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(telecom, o.telecom, true) 1989 && compareDeep(note, o.note, true); 1990 } 1991 1992 @Override 1993 public boolean equalsShallow(Base other_) { 1994 if (!super.equalsShallow(other_)) 1995 return false; 1996 if (!(other_ instanceof CareTeam)) 1997 return false; 1998 CareTeam o = (CareTeam) other_; 1999 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 2000 } 2001 2002 public boolean isEmpty() { 2003 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, name, subject, 2004 encounter, period, participant, reasonCode, reasonReference, managingOrganization, telecom, note); 2005 } 2006 2007 @Override 2008 public ResourceType getResourceType() { 2009 return ResourceType.CareTeam; 2010 } 2011 2012 /** 2013 * Search parameter: <b>date</b> 2014 * <p> 2015 * Description: <b>Time period team covers</b><br> 2016 * Type: <b>date</b><br> 2017 * Path: <b>CareTeam.period</b><br> 2018 * </p> 2019 */ 2020 @SearchParamDefinition(name = "date", path = "CareTeam.period", description = "Time period team covers", type = "date") 2021 public static final String SP_DATE = "date"; 2022 /** 2023 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2024 * <p> 2025 * Description: <b>Time period team covers</b><br> 2026 * Type: <b>date</b><br> 2027 * Path: <b>CareTeam.period</b><br> 2028 * </p> 2029 */ 2030 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2031 SP_DATE); 2032 2033 /** 2034 * Search parameter: <b>identifier</b> 2035 * <p> 2036 * Description: <b>External Ids for this team</b><br> 2037 * Type: <b>token</b><br> 2038 * Path: <b>CareTeam.identifier</b><br> 2039 * </p> 2040 */ 2041 @SearchParamDefinition(name = "identifier", path = "CareTeam.identifier", description = "External Ids for this team", type = "token") 2042 public static final String SP_IDENTIFIER = "identifier"; 2043 /** 2044 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2045 * <p> 2046 * Description: <b>External Ids for this team</b><br> 2047 * Type: <b>token</b><br> 2048 * Path: <b>CareTeam.identifier</b><br> 2049 * </p> 2050 */ 2051 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2052 SP_IDENTIFIER); 2053 2054 /** 2055 * Search parameter: <b>patient</b> 2056 * <p> 2057 * Description: <b>Who care team is for</b><br> 2058 * Type: <b>reference</b><br> 2059 * Path: <b>CareTeam.subject</b><br> 2060 * </p> 2061 */ 2062 @SearchParamDefinition(name = "patient", path = "CareTeam.subject.where(resolve() is Patient)", description = "Who care team is for", type = "reference", providesMembershipIn = { 2063 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2064 public static final String SP_PATIENT = "patient"; 2065 /** 2066 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2067 * <p> 2068 * Description: <b>Who care team is for</b><br> 2069 * Type: <b>reference</b><br> 2070 * Path: <b>CareTeam.subject</b><br> 2071 * </p> 2072 */ 2073 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2074 SP_PATIENT); 2075 2076 /** 2077 * Constant for fluent queries to be used to add include statements. Specifies 2078 * the path value of "<b>CareTeam:patient</b>". 2079 */ 2080 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2081 "CareTeam:patient").toLocked(); 2082 2083 /** 2084 * Search parameter: <b>subject</b> 2085 * <p> 2086 * Description: <b>Who care team is for</b><br> 2087 * Type: <b>reference</b><br> 2088 * Path: <b>CareTeam.subject</b><br> 2089 * </p> 2090 */ 2091 @SearchParamDefinition(name = "subject", path = "CareTeam.subject", description = "Who care team is for", type = "reference", target = { 2092 Group.class, Patient.class }) 2093 public static final String SP_SUBJECT = "subject"; 2094 /** 2095 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2096 * <p> 2097 * Description: <b>Who care team is for</b><br> 2098 * Type: <b>reference</b><br> 2099 * Path: <b>CareTeam.subject</b><br> 2100 * </p> 2101 */ 2102 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2103 SP_SUBJECT); 2104 2105 /** 2106 * Constant for fluent queries to be used to add include statements. Specifies 2107 * the path value of "<b>CareTeam:subject</b>". 2108 */ 2109 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2110 "CareTeam:subject").toLocked(); 2111 2112 /** 2113 * Search parameter: <b>encounter</b> 2114 * <p> 2115 * Description: <b>Encounter created as part of</b><br> 2116 * Type: <b>reference</b><br> 2117 * Path: <b>CareTeam.encounter</b><br> 2118 * </p> 2119 */ 2120 @SearchParamDefinition(name = "encounter", path = "CareTeam.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2121 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2122 public static final String SP_ENCOUNTER = "encounter"; 2123 /** 2124 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2125 * <p> 2126 * Description: <b>Encounter created as part of</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>CareTeam.encounter</b><br> 2129 * </p> 2130 */ 2131 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2132 SP_ENCOUNTER); 2133 2134 /** 2135 * Constant for fluent queries to be used to add include statements. Specifies 2136 * the path value of "<b>CareTeam:encounter</b>". 2137 */ 2138 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2139 "CareTeam:encounter").toLocked(); 2140 2141 /** 2142 * Search parameter: <b>category</b> 2143 * <p> 2144 * Description: <b>Type of team</b><br> 2145 * Type: <b>token</b><br> 2146 * Path: <b>CareTeam.category</b><br> 2147 * </p> 2148 */ 2149 @SearchParamDefinition(name = "category", path = "CareTeam.category", description = "Type of team", type = "token") 2150 public static final String SP_CATEGORY = "category"; 2151 /** 2152 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2153 * <p> 2154 * Description: <b>Type of team</b><br> 2155 * Type: <b>token</b><br> 2156 * Path: <b>CareTeam.category</b><br> 2157 * </p> 2158 */ 2159 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2160 SP_CATEGORY); 2161 2162 /** 2163 * Search parameter: <b>participant</b> 2164 * <p> 2165 * Description: <b>Who is involved</b><br> 2166 * Type: <b>reference</b><br> 2167 * Path: <b>CareTeam.participant.member</b><br> 2168 * </p> 2169 */ 2170 @SearchParamDefinition(name = "participant", path = "CareTeam.participant.member", description = "Who is involved", type = "reference", providesMembershipIn = { 2171 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2172 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2173 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, 2174 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2175 public static final String SP_PARTICIPANT = "participant"; 2176 /** 2177 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 2178 * <p> 2179 * Description: <b>Who is involved</b><br> 2180 * Type: <b>reference</b><br> 2181 * Path: <b>CareTeam.participant.member</b><br> 2182 * </p> 2183 */ 2184 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2185 SP_PARTICIPANT); 2186 2187 /** 2188 * Constant for fluent queries to be used to add include statements. Specifies 2189 * the path value of "<b>CareTeam:participant</b>". 2190 */ 2191 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 2192 "CareTeam:participant").toLocked(); 2193 2194 /** 2195 * Search parameter: <b>status</b> 2196 * <p> 2197 * Description: <b>proposed | active | suspended | inactive | 2198 * entered-in-error</b><br> 2199 * Type: <b>token</b><br> 2200 * Path: <b>CareTeam.status</b><br> 2201 * </p> 2202 */ 2203 @SearchParamDefinition(name = "status", path = "CareTeam.status", description = "proposed | active | suspended | inactive | entered-in-error", type = "token") 2204 public static final String SP_STATUS = "status"; 2205 /** 2206 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2207 * <p> 2208 * Description: <b>proposed | active | suspended | inactive | 2209 * entered-in-error</b><br> 2210 * Type: <b>token</b><br> 2211 * Path: <b>CareTeam.status</b><br> 2212 * </p> 2213 */ 2214 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2215 SP_STATUS); 2216 2217}