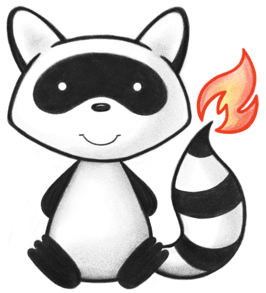
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The Care Team includes all the people and organizations who plan to 048 * participate in the coordination and delivery of care for a patient. 049 */ 050@ResourceDef(name = "CareTeam", profile = "http://hl7.org/fhir/StructureDefinition/CareTeam") 051public class CareTeam extends DomainResource { 052 053 public enum CareTeamStatus { 054 /** 055 * The care team has been drafted and proposed, but not yet participating in the 056 * coordination and delivery of patient care. 057 */ 058 PROPOSED, 059 /** 060 * The care team is currently participating in the coordination and delivery of 061 * care. 062 */ 063 ACTIVE, 064 /** 065 * The care team is temporarily on hold or suspended and not participating in 066 * the coordination and delivery of care. 067 */ 068 SUSPENDED, 069 /** 070 * The care team was, but is no longer, participating in the coordination and 071 * delivery of care. 072 */ 073 INACTIVE, 074 /** 075 * The care team should have never existed. 076 */ 077 ENTEREDINERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static CareTeamStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("proposed".equals(codeString)) 087 return PROPOSED; 088 if ("active".equals(codeString)) 089 return ACTIVE; 090 if ("suspended".equals(codeString)) 091 return SUSPENDED; 092 if ("inactive".equals(codeString)) 093 return INACTIVE; 094 if ("entered-in-error".equals(codeString)) 095 return ENTEREDINERROR; 096 if (Configuration.isAcceptInvalidEnums()) 097 return null; 098 else 099 throw new FHIRException("Unknown CareTeamStatus code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PROPOSED: 105 return "proposed"; 106 case ACTIVE: 107 return "active"; 108 case SUSPENDED: 109 return "suspended"; 110 case INACTIVE: 111 return "inactive"; 112 case ENTEREDINERROR: 113 return "entered-in-error"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 switch (this) { 123 case PROPOSED: 124 return "http://hl7.org/fhir/care-team-status"; 125 case ACTIVE: 126 return "http://hl7.org/fhir/care-team-status"; 127 case SUSPENDED: 128 return "http://hl7.org/fhir/care-team-status"; 129 case INACTIVE: 130 return "http://hl7.org/fhir/care-team-status"; 131 case ENTEREDINERROR: 132 return "http://hl7.org/fhir/care-team-status"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case PROPOSED: 143 return "The care team has been drafted and proposed, but not yet participating in the coordination and delivery of patient care."; 144 case ACTIVE: 145 return "The care team is currently participating in the coordination and delivery of care."; 146 case SUSPENDED: 147 return "The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care."; 148 case INACTIVE: 149 return "The care team was, but is no longer, participating in the coordination and delivery of care."; 150 case ENTEREDINERROR: 151 return "The care team should have never existed."; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDisplay() { 160 switch (this) { 161 case PROPOSED: 162 return "Proposed"; 163 case ACTIVE: 164 return "Active"; 165 case SUSPENDED: 166 return "Suspended"; 167 case INACTIVE: 168 return "Inactive"; 169 case ENTEREDINERROR: 170 return "Entered in Error"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 } 178 179 public static class CareTeamStatusEnumFactory implements EnumFactory<CareTeamStatus> { 180 public CareTeamStatus fromCode(String codeString) throws IllegalArgumentException { 181 if (codeString == null || "".equals(codeString)) 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("proposed".equals(codeString)) 185 return CareTeamStatus.PROPOSED; 186 if ("active".equals(codeString)) 187 return CareTeamStatus.ACTIVE; 188 if ("suspended".equals(codeString)) 189 return CareTeamStatus.SUSPENDED; 190 if ("inactive".equals(codeString)) 191 return CareTeamStatus.INACTIVE; 192 if ("entered-in-error".equals(codeString)) 193 return CareTeamStatus.ENTEREDINERROR; 194 throw new IllegalArgumentException("Unknown CareTeamStatus code '" + codeString + "'"); 195 } 196 197 public Enumeration<CareTeamStatus> fromType(PrimitiveType<?> code) throws FHIRException { 198 if (code == null) 199 return null; 200 if (code.isEmpty()) 201 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 202 String codeString = code.asStringValue(); 203 if (codeString == null || "".equals(codeString)) 204 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 205 if ("proposed".equals(codeString)) 206 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.PROPOSED, code); 207 if ("active".equals(codeString)) 208 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ACTIVE, code); 209 if ("suspended".equals(codeString)) 210 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.SUSPENDED, code); 211 if ("inactive".equals(codeString)) 212 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.INACTIVE, code); 213 if ("entered-in-error".equals(codeString)) 214 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ENTEREDINERROR, code); 215 throw new FHIRException("Unknown CareTeamStatus code '" + codeString + "'"); 216 } 217 218 public String toCode(CareTeamStatus code) { 219 if (code == CareTeamStatus.PROPOSED) 220 return "proposed"; 221 if (code == CareTeamStatus.ACTIVE) 222 return "active"; 223 if (code == CareTeamStatus.SUSPENDED) 224 return "suspended"; 225 if (code == CareTeamStatus.INACTIVE) 226 return "inactive"; 227 if (code == CareTeamStatus.ENTEREDINERROR) 228 return "entered-in-error"; 229 return "?"; 230 } 231 232 public String toSystem(CareTeamStatus code) { 233 return code.getSystem(); 234 } 235 } 236 237 @Block() 238 public static class CareTeamParticipantComponent extends BackboneElement implements IBaseBackboneElement { 239 /** 240 * Indicates specific responsibility of an individual within the care team, such 241 * as "Primary care physician", "Trained social worker counselor", "Caregiver", 242 * etc. 243 */ 244 @Child(name = "role", type = { 245 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 246 @Description(shortDefinition = "Type of involvement", formalDefinition = "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.") 247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participant-role") 248 protected List<CodeableConcept> role; 249 250 /** 251 * The specific person or organization who is participating/expected to 252 * participate in the care team. 253 */ 254 @Child(name = "member", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 255 Organization.class, CareTeam.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 256 @Description(shortDefinition = "Who is involved", formalDefinition = "The specific person or organization who is participating/expected to participate in the care team.") 257 protected Reference member; 258 259 /** 260 * The actual object that is the target of the reference (The specific person or 261 * organization who is participating/expected to participate in the care team.) 262 */ 263 protected Resource memberTarget; 264 265 /** 266 * The organization of the practitioner. 267 */ 268 @Child(name = "onBehalfOf", type = { 269 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 270 @Description(shortDefinition = "Organization of the practitioner", formalDefinition = "The organization of the practitioner.") 271 protected Reference onBehalfOf; 272 273 /** 274 * The actual object that is the target of the reference (The organization of 275 * the practitioner.) 276 */ 277 protected Organization onBehalfOfTarget; 278 279 /** 280 * Indicates when the specific member or organization did (or is intended to) 281 * come into effect and end. 282 */ 283 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 284 @Description(shortDefinition = "Time period of participant", formalDefinition = "Indicates when the specific member or organization did (or is intended to) come into effect and end.") 285 protected Period period; 286 287 private static final long serialVersionUID = -575634410L; 288 289 /** 290 * Constructor 291 */ 292 public CareTeamParticipantComponent() { 293 super(); 294 } 295 296 /** 297 * @return {@link #role} (Indicates specific responsibility of an individual 298 * within the care team, such as "Primary care physician", "Trained 299 * social worker counselor", "Caregiver", etc.) 300 */ 301 public List<CodeableConcept> getRole() { 302 if (this.role == null) 303 this.role = new ArrayList<CodeableConcept>(); 304 return this.role; 305 } 306 307 /** 308 * @return Returns a reference to <code>this</code> for easy method chaining 309 */ 310 public CareTeamParticipantComponent setRole(List<CodeableConcept> theRole) { 311 this.role = theRole; 312 return this; 313 } 314 315 public boolean hasRole() { 316 if (this.role == null) 317 return false; 318 for (CodeableConcept item : this.role) 319 if (!item.isEmpty()) 320 return true; 321 return false; 322 } 323 324 public CodeableConcept addRole() { // 3 325 CodeableConcept t = new CodeableConcept(); 326 if (this.role == null) 327 this.role = new ArrayList<CodeableConcept>(); 328 this.role.add(t); 329 return t; 330 } 331 332 public CareTeamParticipantComponent addRole(CodeableConcept t) { // 3 333 if (t == null) 334 return this; 335 if (this.role == null) 336 this.role = new ArrayList<CodeableConcept>(); 337 this.role.add(t); 338 return this; 339 } 340 341 /** 342 * @return The first repetition of repeating field {@link #role}, creating it if 343 * it does not already exist 344 */ 345 public CodeableConcept getRoleFirstRep() { 346 if (getRole().isEmpty()) { 347 addRole(); 348 } 349 return getRole().get(0); 350 } 351 352 /** 353 * @return {@link #member} (The specific person or organization who is 354 * participating/expected to participate in the care team.) 355 */ 356 public Reference getMember() { 357 if (this.member == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create CareTeamParticipantComponent.member"); 360 else if (Configuration.doAutoCreate()) 361 this.member = new Reference(); // cc 362 return this.member; 363 } 364 365 public boolean hasMember() { 366 return this.member != null && !this.member.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #member} (The specific person or organization who is 371 * participating/expected to participate in the care team.) 372 */ 373 public CareTeamParticipantComponent setMember(Reference value) { 374 this.member = value; 375 return this; 376 } 377 378 /** 379 * @return {@link #member} The actual object that is the target of the 380 * reference. The reference library doesn't populate this, but you can 381 * use it to hold the resource if you resolve it. (The specific person 382 * or organization who is participating/expected to participate in the 383 * care team.) 384 */ 385 public Resource getMemberTarget() { 386 return this.memberTarget; 387 } 388 389 /** 390 * @param value {@link #member} The actual object that is the target of the 391 * reference. The reference library doesn't use these, but you can 392 * use it to hold the resource if you resolve it. (The specific 393 * person or organization who is participating/expected to 394 * participate in the care team.) 395 */ 396 public CareTeamParticipantComponent setMemberTarget(Resource value) { 397 this.memberTarget = value; 398 return this; 399 } 400 401 /** 402 * @return {@link #onBehalfOf} (The organization of the practitioner.) 403 */ 404 public Reference getOnBehalfOf() { 405 if (this.onBehalfOf == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 408 else if (Configuration.doAutoCreate()) 409 this.onBehalfOf = new Reference(); // cc 410 return this.onBehalfOf; 411 } 412 413 public boolean hasOnBehalfOf() { 414 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #onBehalfOf} (The organization of the practitioner.) 419 */ 420 public CareTeamParticipantComponent setOnBehalfOf(Reference value) { 421 this.onBehalfOf = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #onBehalfOf} The actual object that is the target of the 427 * reference. The reference library doesn't populate this, but you can 428 * use it to hold the resource if you resolve it. (The organization of 429 * the practitioner.) 430 */ 431 public Organization getOnBehalfOfTarget() { 432 if (this.onBehalfOfTarget == null) 433 if (Configuration.errorOnAutoCreate()) 434 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 435 else if (Configuration.doAutoCreate()) 436 this.onBehalfOfTarget = new Organization(); // aa 437 return this.onBehalfOfTarget; 438 } 439 440 /** 441 * @param value {@link #onBehalfOf} The actual object that is the target of the 442 * reference. The reference library doesn't use these, but you can 443 * use it to hold the resource if you resolve it. (The organization 444 * of the practitioner.) 445 */ 446 public CareTeamParticipantComponent setOnBehalfOfTarget(Organization value) { 447 this.onBehalfOfTarget = value; 448 return this; 449 } 450 451 /** 452 * @return {@link #period} (Indicates when the specific member or organization 453 * did (or is intended to) come into effect and end.) 454 */ 455 public Period getPeriod() { 456 if (this.period == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create CareTeamParticipantComponent.period"); 459 else if (Configuration.doAutoCreate()) 460 this.period = new Period(); // cc 461 return this.period; 462 } 463 464 public boolean hasPeriod() { 465 return this.period != null && !this.period.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #period} (Indicates when the specific member or 470 * organization did (or is intended to) come into effect and end.) 471 */ 472 public CareTeamParticipantComponent setPeriod(Period value) { 473 this.period = value; 474 return this; 475 } 476 477 protected void listChildren(List<Property> children) { 478 super.listChildren(children); 479 children.add(new Property("role", "CodeableConcept", 480 "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 481 0, java.lang.Integer.MAX_VALUE, role)); 482 children.add( 483 new Property("member", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", 484 "The specific person or organization who is participating/expected to participate in the care team.", 0, 485 1, member)); 486 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, 487 onBehalfOf)); 488 children.add(new Property("period", "Period", 489 "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, 490 period)); 491 } 492 493 @Override 494 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 495 switch (_hash) { 496 case 3506294: 497 /* role */ return new Property("role", "CodeableConcept", 498 "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 499 0, java.lang.Integer.MAX_VALUE, role); 500 case -1077769574: 501 /* member */ return new Property("member", 502 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", 503 "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, 504 member); 505 case -14402964: 506 /* onBehalfOf */ return new Property("onBehalfOf", "Reference(Organization)", 507 "The organization of the practitioner.", 0, 1, onBehalfOf); 508 case -991726143: 509 /* period */ return new Property("period", "Period", 510 "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 511 1, period); 512 default: 513 return super.getNamedProperty(_hash, _name, _checkValid); 514 } 515 516 } 517 518 @Override 519 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 520 switch (hash) { 521 case 3506294: 522 /* role */ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 523 case -1077769574: 524 /* member */ return this.member == null ? new Base[0] : new Base[] { this.member }; // Reference 525 case -14402964: 526 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 527 case -991726143: 528 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 529 default: 530 return super.getProperty(hash, name, checkValid); 531 } 532 533 } 534 535 @Override 536 public Base setProperty(int hash, String name, Base value) throws FHIRException { 537 switch (hash) { 538 case 3506294: // role 539 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 540 return value; 541 case -1077769574: // member 542 this.member = castToReference(value); // Reference 543 return value; 544 case -14402964: // onBehalfOf 545 this.onBehalfOf = castToReference(value); // Reference 546 return value; 547 case -991726143: // period 548 this.period = castToPeriod(value); // Period 549 return value; 550 default: 551 return super.setProperty(hash, name, value); 552 } 553 554 } 555 556 @Override 557 public Base setProperty(String name, Base value) throws FHIRException { 558 if (name.equals("role")) { 559 this.getRole().add(castToCodeableConcept(value)); 560 } else if (name.equals("member")) { 561 this.member = castToReference(value); // Reference 562 } else if (name.equals("onBehalfOf")) { 563 this.onBehalfOf = castToReference(value); // Reference 564 } else if (name.equals("period")) { 565 this.period = castToPeriod(value); // Period 566 } else 567 return super.setProperty(name, value); 568 return value; 569 } 570 571 @Override 572 public Base makeProperty(int hash, String name) throws FHIRException { 573 switch (hash) { 574 case 3506294: 575 return addRole(); 576 case -1077769574: 577 return getMember(); 578 case -14402964: 579 return getOnBehalfOf(); 580 case -991726143: 581 return getPeriod(); 582 default: 583 return super.makeProperty(hash, name); 584 } 585 586 } 587 588 @Override 589 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 590 switch (hash) { 591 case 3506294: 592 /* role */ return new String[] { "CodeableConcept" }; 593 case -1077769574: 594 /* member */ return new String[] { "Reference" }; 595 case -14402964: 596 /* onBehalfOf */ return new String[] { "Reference" }; 597 case -991726143: 598 /* period */ return new String[] { "Period" }; 599 default: 600 return super.getTypesForProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public Base addChild(String name) throws FHIRException { 607 if (name.equals("role")) { 608 return addRole(); 609 } else if (name.equals("member")) { 610 this.member = new Reference(); 611 return this.member; 612 } else if (name.equals("onBehalfOf")) { 613 this.onBehalfOf = new Reference(); 614 return this.onBehalfOf; 615 } else if (name.equals("period")) { 616 this.period = new Period(); 617 return this.period; 618 } else 619 return super.addChild(name); 620 } 621 622 public CareTeamParticipantComponent copy() { 623 CareTeamParticipantComponent dst = new CareTeamParticipantComponent(); 624 copyValues(dst); 625 return dst; 626 } 627 628 public void copyValues(CareTeamParticipantComponent dst) { 629 super.copyValues(dst); 630 if (role != null) { 631 dst.role = new ArrayList<CodeableConcept>(); 632 for (CodeableConcept i : role) 633 dst.role.add(i.copy()); 634 } 635 ; 636 dst.member = member == null ? null : member.copy(); 637 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 638 dst.period = period == null ? null : period.copy(); 639 } 640 641 @Override 642 public boolean equalsDeep(Base other_) { 643 if (!super.equalsDeep(other_)) 644 return false; 645 if (!(other_ instanceof CareTeamParticipantComponent)) 646 return false; 647 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 648 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true) 649 && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(period, o.period, true); 650 } 651 652 @Override 653 public boolean equalsShallow(Base other_) { 654 if (!super.equalsShallow(other_)) 655 return false; 656 if (!(other_ instanceof CareTeamParticipantComponent)) 657 return false; 658 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 659 return true; 660 } 661 662 public boolean isEmpty() { 663 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, member, onBehalfOf, period); 664 } 665 666 public String fhirType() { 667 return "CareTeam.participant"; 668 669 } 670 671 } 672 673 /** 674 * Business identifiers assigned to this care team by the performer or other 675 * systems which remain constant as the resource is updated and propagates from 676 * server to server. 677 */ 678 @Child(name = "identifier", type = { 679 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 680 @Description(shortDefinition = "External Ids for this team", formalDefinition = "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 681 protected List<Identifier> identifier; 682 683 /** 684 * Indicates the current state of the care team. 685 */ 686 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 687 @Description(shortDefinition = "proposed | active | suspended | inactive | entered-in-error", formalDefinition = "Indicates the current state of the care team.") 688 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-team-status") 689 protected Enumeration<CareTeamStatus> status; 690 691 /** 692 * Identifies what kind of team. This is to support differentiation between 693 * multiple co-existing teams, such as care plan team, episode of care team, 694 * longitudinal care team. 695 */ 696 @Child(name = "category", type = { 697 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 698 @Description(shortDefinition = "Type of team", formalDefinition = "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.") 699 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-team-category") 700 protected List<CodeableConcept> category; 701 702 /** 703 * A label for human use intended to distinguish like teams. E.g. the "red" vs. 704 * "green" trauma teams. 705 */ 706 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 707 @Description(shortDefinition = "Name of the team, such as crisis assessment team", formalDefinition = "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.") 708 protected StringType name; 709 710 /** 711 * Identifies the patient or group whose intended care is handled by the team. 712 */ 713 @Child(name = "subject", type = { Patient.class, 714 Group.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 715 @Description(shortDefinition = "Who care team is for", formalDefinition = "Identifies the patient or group whose intended care is handled by the team.") 716 protected Reference subject; 717 718 /** 719 * The actual object that is the target of the reference (Identifies the patient 720 * or group whose intended care is handled by the team.) 721 */ 722 protected Resource subjectTarget; 723 724 /** 725 * The Encounter during which this CareTeam was created or to which the creation 726 * of this record is tightly associated. 727 */ 728 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 729 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.") 730 protected Reference encounter; 731 732 /** 733 * The actual object that is the target of the reference (The Encounter during 734 * which this CareTeam was created or to which the creation of this record is 735 * tightly associated.) 736 */ 737 protected Encounter encounterTarget; 738 739 /** 740 * Indicates when the team did (or is intended to) come into effect and end. 741 */ 742 @Child(name = "period", type = { Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 743 @Description(shortDefinition = "Time period team covers", formalDefinition = "Indicates when the team did (or is intended to) come into effect and end.") 744 protected Period period; 745 746 /** 747 * Identifies all people and organizations who are expected to be involved in 748 * the care team. 749 */ 750 @Child(name = "participant", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 751 @Description(shortDefinition = "Members of the team", formalDefinition = "Identifies all people and organizations who are expected to be involved in the care team.") 752 protected List<CareTeamParticipantComponent> participant; 753 754 /** 755 * Describes why the care team exists. 756 */ 757 @Child(name = "reasonCode", type = { 758 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 759 @Description(shortDefinition = "Why the care team exists", formalDefinition = "Describes why the care team exists.") 760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 761 protected List<CodeableConcept> reasonCode; 762 763 /** 764 * Condition(s) that this care team addresses. 765 */ 766 @Child(name = "reasonReference", type = { 767 Condition.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 768 @Description(shortDefinition = "Why the care team exists", formalDefinition = "Condition(s) that this care team addresses.") 769 protected List<Reference> reasonReference; 770 /** 771 * The actual objects that are the target of the reference (Condition(s) that 772 * this care team addresses.) 773 */ 774 protected List<Condition> reasonReferenceTarget; 775 776 /** 777 * The organization responsible for the care team. 778 */ 779 @Child(name = "managingOrganization", type = { 780 Organization.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 781 @Description(shortDefinition = "Organization responsible for the care team", formalDefinition = "The organization responsible for the care team.") 782 protected List<Reference> managingOrganization; 783 /** 784 * The actual objects that are the target of the reference (The organization 785 * responsible for the care team.) 786 */ 787 protected List<Organization> managingOrganizationTarget; 788 789 /** 790 * A central contact detail for the care team (that applies to all members). 791 */ 792 @Child(name = "telecom", type = { 793 ContactPoint.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 794 @Description(shortDefinition = "A contact detail for the care team (that applies to all members)", formalDefinition = "A central contact detail for the care team (that applies to all members).") 795 protected List<ContactPoint> telecom; 796 797 /** 798 * Comments made about the CareTeam. 799 */ 800 @Child(name = "note", type = { 801 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 802 @Description(shortDefinition = "Comments made about the CareTeam", formalDefinition = "Comments made about the CareTeam.") 803 protected List<Annotation> note; 804 805 private static final long serialVersionUID = 1793069286L; 806 807 /** 808 * Constructor 809 */ 810 public CareTeam() { 811 super(); 812 } 813 814 /** 815 * @return {@link #identifier} (Business identifiers assigned to this care team 816 * by the performer or other systems which remain constant as the 817 * resource is updated and propagates from server to server.) 818 */ 819 public List<Identifier> getIdentifier() { 820 if (this.identifier == null) 821 this.identifier = new ArrayList<Identifier>(); 822 return this.identifier; 823 } 824 825 /** 826 * @return Returns a reference to <code>this</code> for easy method chaining 827 */ 828 public CareTeam setIdentifier(List<Identifier> theIdentifier) { 829 this.identifier = theIdentifier; 830 return this; 831 } 832 833 public boolean hasIdentifier() { 834 if (this.identifier == null) 835 return false; 836 for (Identifier item : this.identifier) 837 if (!item.isEmpty()) 838 return true; 839 return false; 840 } 841 842 public Identifier addIdentifier() { // 3 843 Identifier t = new Identifier(); 844 if (this.identifier == null) 845 this.identifier = new ArrayList<Identifier>(); 846 this.identifier.add(t); 847 return t; 848 } 849 850 public CareTeam addIdentifier(Identifier t) { // 3 851 if (t == null) 852 return this; 853 if (this.identifier == null) 854 this.identifier = new ArrayList<Identifier>(); 855 this.identifier.add(t); 856 return this; 857 } 858 859 /** 860 * @return The first repetition of repeating field {@link #identifier}, creating 861 * it if it does not already exist 862 */ 863 public Identifier getIdentifierFirstRep() { 864 if (getIdentifier().isEmpty()) { 865 addIdentifier(); 866 } 867 return getIdentifier().get(0); 868 } 869 870 /** 871 * @return {@link #status} (Indicates the current state of the care team.). This 872 * is the underlying object with id, value and extensions. The accessor 873 * "getStatus" gives direct access to the value 874 */ 875 public Enumeration<CareTeamStatus> getStatusElement() { 876 if (this.status == null) 877 if (Configuration.errorOnAutoCreate()) 878 throw new Error("Attempt to auto-create CareTeam.status"); 879 else if (Configuration.doAutoCreate()) 880 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); // bb 881 return this.status; 882 } 883 884 public boolean hasStatusElement() { 885 return this.status != null && !this.status.isEmpty(); 886 } 887 888 public boolean hasStatus() { 889 return this.status != null && !this.status.isEmpty(); 890 } 891 892 /** 893 * @param value {@link #status} (Indicates the current state of the care team.). 894 * This is the underlying object with id, value and extensions. The 895 * accessor "getStatus" gives direct access to the value 896 */ 897 public CareTeam setStatusElement(Enumeration<CareTeamStatus> value) { 898 this.status = value; 899 return this; 900 } 901 902 /** 903 * @return Indicates the current state of the care team. 904 */ 905 public CareTeamStatus getStatus() { 906 return this.status == null ? null : this.status.getValue(); 907 } 908 909 /** 910 * @param value Indicates the current state of the care team. 911 */ 912 public CareTeam setStatus(CareTeamStatus value) { 913 if (value == null) 914 this.status = null; 915 else { 916 if (this.status == null) 917 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); 918 this.status.setValue(value); 919 } 920 return this; 921 } 922 923 /** 924 * @return {@link #category} (Identifies what kind of team. This is to support 925 * differentiation between multiple co-existing teams, such as care plan 926 * team, episode of care team, longitudinal care team.) 927 */ 928 public List<CodeableConcept> getCategory() { 929 if (this.category == null) 930 this.category = new ArrayList<CodeableConcept>(); 931 return this.category; 932 } 933 934 /** 935 * @return Returns a reference to <code>this</code> for easy method chaining 936 */ 937 public CareTeam setCategory(List<CodeableConcept> theCategory) { 938 this.category = theCategory; 939 return this; 940 } 941 942 public boolean hasCategory() { 943 if (this.category == null) 944 return false; 945 for (CodeableConcept item : this.category) 946 if (!item.isEmpty()) 947 return true; 948 return false; 949 } 950 951 public CodeableConcept addCategory() { // 3 952 CodeableConcept t = new CodeableConcept(); 953 if (this.category == null) 954 this.category = new ArrayList<CodeableConcept>(); 955 this.category.add(t); 956 return t; 957 } 958 959 public CareTeam addCategory(CodeableConcept t) { // 3 960 if (t == null) 961 return this; 962 if (this.category == null) 963 this.category = new ArrayList<CodeableConcept>(); 964 this.category.add(t); 965 return this; 966 } 967 968 /** 969 * @return The first repetition of repeating field {@link #category}, creating 970 * it if it does not already exist 971 */ 972 public CodeableConcept getCategoryFirstRep() { 973 if (getCategory().isEmpty()) { 974 addCategory(); 975 } 976 return getCategory().get(0); 977 } 978 979 /** 980 * @return {@link #name} (A label for human use intended to distinguish like 981 * teams. E.g. the "red" vs. "green" trauma teams.). This is the 982 * underlying object with id, value and extensions. The accessor 983 * "getName" gives direct access to the value 984 */ 985 public StringType getNameElement() { 986 if (this.name == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create CareTeam.name"); 989 else if (Configuration.doAutoCreate()) 990 this.name = new StringType(); // bb 991 return this.name; 992 } 993 994 public boolean hasNameElement() { 995 return this.name != null && !this.name.isEmpty(); 996 } 997 998 public boolean hasName() { 999 return this.name != null && !this.name.isEmpty(); 1000 } 1001 1002 /** 1003 * @param value {@link #name} (A label for human use intended to distinguish 1004 * like teams. E.g. the "red" vs. "green" trauma teams.). This is 1005 * the underlying object with id, value and extensions. The 1006 * accessor "getName" gives direct access to the value 1007 */ 1008 public CareTeam setNameElement(StringType value) { 1009 this.name = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return A label for human use intended to distinguish like teams. E.g. the 1015 * "red" vs. "green" trauma teams. 1016 */ 1017 public String getName() { 1018 return this.name == null ? null : this.name.getValue(); 1019 } 1020 1021 /** 1022 * @param value A label for human use intended to distinguish like teams. E.g. 1023 * the "red" vs. "green" trauma teams. 1024 */ 1025 public CareTeam setName(String value) { 1026 if (Utilities.noString(value)) 1027 this.name = null; 1028 else { 1029 if (this.name == null) 1030 this.name = new StringType(); 1031 this.name.setValue(value); 1032 } 1033 return this; 1034 } 1035 1036 /** 1037 * @return {@link #subject} (Identifies the patient or group whose intended care 1038 * is handled by the team.) 1039 */ 1040 public Reference getSubject() { 1041 if (this.subject == null) 1042 if (Configuration.errorOnAutoCreate()) 1043 throw new Error("Attempt to auto-create CareTeam.subject"); 1044 else if (Configuration.doAutoCreate()) 1045 this.subject = new Reference(); // cc 1046 return this.subject; 1047 } 1048 1049 public boolean hasSubject() { 1050 return this.subject != null && !this.subject.isEmpty(); 1051 } 1052 1053 /** 1054 * @param value {@link #subject} (Identifies the patient or group whose intended 1055 * care is handled by the team.) 1056 */ 1057 public CareTeam setSubject(Reference value) { 1058 this.subject = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return {@link #subject} The actual object that is the target of the 1064 * reference. The reference library doesn't populate this, but you can 1065 * use it to hold the resource if you resolve it. (Identifies the 1066 * patient or group whose intended care is handled by the team.) 1067 */ 1068 public Resource getSubjectTarget() { 1069 return this.subjectTarget; 1070 } 1071 1072 /** 1073 * @param value {@link #subject} The actual object that is the target of the 1074 * reference. The reference library doesn't use these, but you can 1075 * use it to hold the resource if you resolve it. (Identifies the 1076 * patient or group whose intended care is handled by the team.) 1077 */ 1078 public CareTeam setSubjectTarget(Resource value) { 1079 this.subjectTarget = value; 1080 return this; 1081 } 1082 1083 /** 1084 * @return {@link #encounter} (The Encounter during which this CareTeam was 1085 * created or to which the creation of this record is tightly 1086 * associated.) 1087 */ 1088 public Reference getEncounter() { 1089 if (this.encounter == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create CareTeam.encounter"); 1092 else if (Configuration.doAutoCreate()) 1093 this.encounter = new Reference(); // cc 1094 return this.encounter; 1095 } 1096 1097 public boolean hasEncounter() { 1098 return this.encounter != null && !this.encounter.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #encounter} (The Encounter during which this CareTeam was 1103 * created or to which the creation of this record is tightly 1104 * associated.) 1105 */ 1106 public CareTeam setEncounter(Reference value) { 1107 this.encounter = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return {@link #encounter} The actual object that is the target of the 1113 * reference. The reference library doesn't populate this, but you can 1114 * use it to hold the resource if you resolve it. (The Encounter during 1115 * which this CareTeam was created or to which the creation of this 1116 * record is tightly associated.) 1117 */ 1118 public Encounter getEncounterTarget() { 1119 if (this.encounterTarget == null) 1120 if (Configuration.errorOnAutoCreate()) 1121 throw new Error("Attempt to auto-create CareTeam.encounter"); 1122 else if (Configuration.doAutoCreate()) 1123 this.encounterTarget = new Encounter(); // aa 1124 return this.encounterTarget; 1125 } 1126 1127 /** 1128 * @param value {@link #encounter} The actual object that is the target of the 1129 * reference. The reference library doesn't use these, but you can 1130 * use it to hold the resource if you resolve it. (The Encounter 1131 * during which this CareTeam was created or to which the creation 1132 * of this record is tightly associated.) 1133 */ 1134 public CareTeam setEncounterTarget(Encounter value) { 1135 this.encounterTarget = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #period} (Indicates when the team did (or is intended to) come 1141 * into effect and end.) 1142 */ 1143 public Period getPeriod() { 1144 if (this.period == null) 1145 if (Configuration.errorOnAutoCreate()) 1146 throw new Error("Attempt to auto-create CareTeam.period"); 1147 else if (Configuration.doAutoCreate()) 1148 this.period = new Period(); // cc 1149 return this.period; 1150 } 1151 1152 public boolean hasPeriod() { 1153 return this.period != null && !this.period.isEmpty(); 1154 } 1155 1156 /** 1157 * @param value {@link #period} (Indicates when the team did (or is intended to) 1158 * come into effect and end.) 1159 */ 1160 public CareTeam setPeriod(Period value) { 1161 this.period = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return {@link #participant} (Identifies all people and organizations who are 1167 * expected to be involved in the care team.) 1168 */ 1169 public List<CareTeamParticipantComponent> getParticipant() { 1170 if (this.participant == null) 1171 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1172 return this.participant; 1173 } 1174 1175 /** 1176 * @return Returns a reference to <code>this</code> for easy method chaining 1177 */ 1178 public CareTeam setParticipant(List<CareTeamParticipantComponent> theParticipant) { 1179 this.participant = theParticipant; 1180 return this; 1181 } 1182 1183 public boolean hasParticipant() { 1184 if (this.participant == null) 1185 return false; 1186 for (CareTeamParticipantComponent item : this.participant) 1187 if (!item.isEmpty()) 1188 return true; 1189 return false; 1190 } 1191 1192 public CareTeamParticipantComponent addParticipant() { // 3 1193 CareTeamParticipantComponent t = new CareTeamParticipantComponent(); 1194 if (this.participant == null) 1195 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1196 this.participant.add(t); 1197 return t; 1198 } 1199 1200 public CareTeam addParticipant(CareTeamParticipantComponent t) { // 3 1201 if (t == null) 1202 return this; 1203 if (this.participant == null) 1204 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1205 this.participant.add(t); 1206 return this; 1207 } 1208 1209 /** 1210 * @return The first repetition of repeating field {@link #participant}, 1211 * creating it if it does not already exist 1212 */ 1213 public CareTeamParticipantComponent getParticipantFirstRep() { 1214 if (getParticipant().isEmpty()) { 1215 addParticipant(); 1216 } 1217 return getParticipant().get(0); 1218 } 1219 1220 /** 1221 * @return {@link #reasonCode} (Describes why the care team exists.) 1222 */ 1223 public List<CodeableConcept> getReasonCode() { 1224 if (this.reasonCode == null) 1225 this.reasonCode = new ArrayList<CodeableConcept>(); 1226 return this.reasonCode; 1227 } 1228 1229 /** 1230 * @return Returns a reference to <code>this</code> for easy method chaining 1231 */ 1232 public CareTeam setReasonCode(List<CodeableConcept> theReasonCode) { 1233 this.reasonCode = theReasonCode; 1234 return this; 1235 } 1236 1237 public boolean hasReasonCode() { 1238 if (this.reasonCode == null) 1239 return false; 1240 for (CodeableConcept item : this.reasonCode) 1241 if (!item.isEmpty()) 1242 return true; 1243 return false; 1244 } 1245 1246 public CodeableConcept addReasonCode() { // 3 1247 CodeableConcept t = new CodeableConcept(); 1248 if (this.reasonCode == null) 1249 this.reasonCode = new ArrayList<CodeableConcept>(); 1250 this.reasonCode.add(t); 1251 return t; 1252 } 1253 1254 public CareTeam addReasonCode(CodeableConcept t) { // 3 1255 if (t == null) 1256 return this; 1257 if (this.reasonCode == null) 1258 this.reasonCode = new ArrayList<CodeableConcept>(); 1259 this.reasonCode.add(t); 1260 return this; 1261 } 1262 1263 /** 1264 * @return The first repetition of repeating field {@link #reasonCode}, creating 1265 * it if it does not already exist 1266 */ 1267 public CodeableConcept getReasonCodeFirstRep() { 1268 if (getReasonCode().isEmpty()) { 1269 addReasonCode(); 1270 } 1271 return getReasonCode().get(0); 1272 } 1273 1274 /** 1275 * @return {@link #reasonReference} (Condition(s) that this care team 1276 * addresses.) 1277 */ 1278 public List<Reference> getReasonReference() { 1279 if (this.reasonReference == null) 1280 this.reasonReference = new ArrayList<Reference>(); 1281 return this.reasonReference; 1282 } 1283 1284 /** 1285 * @return Returns a reference to <code>this</code> for easy method chaining 1286 */ 1287 public CareTeam setReasonReference(List<Reference> theReasonReference) { 1288 this.reasonReference = theReasonReference; 1289 return this; 1290 } 1291 1292 public boolean hasReasonReference() { 1293 if (this.reasonReference == null) 1294 return false; 1295 for (Reference item : this.reasonReference) 1296 if (!item.isEmpty()) 1297 return true; 1298 return false; 1299 } 1300 1301 public Reference addReasonReference() { // 3 1302 Reference t = new Reference(); 1303 if (this.reasonReference == null) 1304 this.reasonReference = new ArrayList<Reference>(); 1305 this.reasonReference.add(t); 1306 return t; 1307 } 1308 1309 public CareTeam addReasonReference(Reference t) { // 3 1310 if (t == null) 1311 return this; 1312 if (this.reasonReference == null) 1313 this.reasonReference = new ArrayList<Reference>(); 1314 this.reasonReference.add(t); 1315 return this; 1316 } 1317 1318 /** 1319 * @return The first repetition of repeating field {@link #reasonReference}, 1320 * creating it if it does not already exist 1321 */ 1322 public Reference getReasonReferenceFirstRep() { 1323 if (getReasonReference().isEmpty()) { 1324 addReasonReference(); 1325 } 1326 return getReasonReference().get(0); 1327 } 1328 1329 /** 1330 * @deprecated Use Reference#setResource(IBaseResource) instead 1331 */ 1332 @Deprecated 1333 public List<Condition> getReasonReferenceTarget() { 1334 if (this.reasonReferenceTarget == null) 1335 this.reasonReferenceTarget = new ArrayList<Condition>(); 1336 return this.reasonReferenceTarget; 1337 } 1338 1339 /** 1340 * @deprecated Use Reference#setResource(IBaseResource) instead 1341 */ 1342 @Deprecated 1343 public Condition addReasonReferenceTarget() { 1344 Condition r = new Condition(); 1345 if (this.reasonReferenceTarget == null) 1346 this.reasonReferenceTarget = new ArrayList<Condition>(); 1347 this.reasonReferenceTarget.add(r); 1348 return r; 1349 } 1350 1351 /** 1352 * @return {@link #managingOrganization} (The organization responsible for the 1353 * care team.) 1354 */ 1355 public List<Reference> getManagingOrganization() { 1356 if (this.managingOrganization == null) 1357 this.managingOrganization = new ArrayList<Reference>(); 1358 return this.managingOrganization; 1359 } 1360 1361 /** 1362 * @return Returns a reference to <code>this</code> for easy method chaining 1363 */ 1364 public CareTeam setManagingOrganization(List<Reference> theManagingOrganization) { 1365 this.managingOrganization = theManagingOrganization; 1366 return this; 1367 } 1368 1369 public boolean hasManagingOrganization() { 1370 if (this.managingOrganization == null) 1371 return false; 1372 for (Reference item : this.managingOrganization) 1373 if (!item.isEmpty()) 1374 return true; 1375 return false; 1376 } 1377 1378 public Reference addManagingOrganization() { // 3 1379 Reference t = new Reference(); 1380 if (this.managingOrganization == null) 1381 this.managingOrganization = new ArrayList<Reference>(); 1382 this.managingOrganization.add(t); 1383 return t; 1384 } 1385 1386 public CareTeam addManagingOrganization(Reference t) { // 3 1387 if (t == null) 1388 return this; 1389 if (this.managingOrganization == null) 1390 this.managingOrganization = new ArrayList<Reference>(); 1391 this.managingOrganization.add(t); 1392 return this; 1393 } 1394 1395 /** 1396 * @return The first repetition of repeating field 1397 * {@link #managingOrganization}, creating it if it does not already 1398 * exist 1399 */ 1400 public Reference getManagingOrganizationFirstRep() { 1401 if (getManagingOrganization().isEmpty()) { 1402 addManagingOrganization(); 1403 } 1404 return getManagingOrganization().get(0); 1405 } 1406 1407 /** 1408 * @deprecated Use Reference#setResource(IBaseResource) instead 1409 */ 1410 @Deprecated 1411 public List<Organization> getManagingOrganizationTarget() { 1412 if (this.managingOrganizationTarget == null) 1413 this.managingOrganizationTarget = new ArrayList<Organization>(); 1414 return this.managingOrganizationTarget; 1415 } 1416 1417 /** 1418 * @deprecated Use Reference#setResource(IBaseResource) instead 1419 */ 1420 @Deprecated 1421 public Organization addManagingOrganizationTarget() { 1422 Organization r = new Organization(); 1423 if (this.managingOrganizationTarget == null) 1424 this.managingOrganizationTarget = new ArrayList<Organization>(); 1425 this.managingOrganizationTarget.add(r); 1426 return r; 1427 } 1428 1429 /** 1430 * @return {@link #telecom} (A central contact detail for the care team (that 1431 * applies to all members).) 1432 */ 1433 public List<ContactPoint> getTelecom() { 1434 if (this.telecom == null) 1435 this.telecom = new ArrayList<ContactPoint>(); 1436 return this.telecom; 1437 } 1438 1439 /** 1440 * @return Returns a reference to <code>this</code> for easy method chaining 1441 */ 1442 public CareTeam setTelecom(List<ContactPoint> theTelecom) { 1443 this.telecom = theTelecom; 1444 return this; 1445 } 1446 1447 public boolean hasTelecom() { 1448 if (this.telecom == null) 1449 return false; 1450 for (ContactPoint item : this.telecom) 1451 if (!item.isEmpty()) 1452 return true; 1453 return false; 1454 } 1455 1456 public ContactPoint addTelecom() { // 3 1457 ContactPoint t = new ContactPoint(); 1458 if (this.telecom == null) 1459 this.telecom = new ArrayList<ContactPoint>(); 1460 this.telecom.add(t); 1461 return t; 1462 } 1463 1464 public CareTeam addTelecom(ContactPoint t) { // 3 1465 if (t == null) 1466 return this; 1467 if (this.telecom == null) 1468 this.telecom = new ArrayList<ContactPoint>(); 1469 this.telecom.add(t); 1470 return this; 1471 } 1472 1473 /** 1474 * @return The first repetition of repeating field {@link #telecom}, creating it 1475 * if it does not already exist 1476 */ 1477 public ContactPoint getTelecomFirstRep() { 1478 if (getTelecom().isEmpty()) { 1479 addTelecom(); 1480 } 1481 return getTelecom().get(0); 1482 } 1483 1484 /** 1485 * @return {@link #note} (Comments made about the CareTeam.) 1486 */ 1487 public List<Annotation> getNote() { 1488 if (this.note == null) 1489 this.note = new ArrayList<Annotation>(); 1490 return this.note; 1491 } 1492 1493 /** 1494 * @return Returns a reference to <code>this</code> for easy method chaining 1495 */ 1496 public CareTeam setNote(List<Annotation> theNote) { 1497 this.note = theNote; 1498 return this; 1499 } 1500 1501 public boolean hasNote() { 1502 if (this.note == null) 1503 return false; 1504 for (Annotation item : this.note) 1505 if (!item.isEmpty()) 1506 return true; 1507 return false; 1508 } 1509 1510 public Annotation addNote() { // 3 1511 Annotation t = new Annotation(); 1512 if (this.note == null) 1513 this.note = new ArrayList<Annotation>(); 1514 this.note.add(t); 1515 return t; 1516 } 1517 1518 public CareTeam addNote(Annotation t) { // 3 1519 if (t == null) 1520 return this; 1521 if (this.note == null) 1522 this.note = new ArrayList<Annotation>(); 1523 this.note.add(t); 1524 return this; 1525 } 1526 1527 /** 1528 * @return The first repetition of repeating field {@link #note}, creating it if 1529 * it does not already exist 1530 */ 1531 public Annotation getNoteFirstRep() { 1532 if (getNote().isEmpty()) { 1533 addNote(); 1534 } 1535 return getNote().get(0); 1536 } 1537 1538 protected void listChildren(List<Property> children) { 1539 super.listChildren(children); 1540 children.add(new Property("identifier", "Identifier", 1541 "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1542 0, java.lang.Integer.MAX_VALUE, identifier)); 1543 children.add(new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status)); 1544 children.add(new Property("category", "CodeableConcept", 1545 "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 1546 0, java.lang.Integer.MAX_VALUE, category)); 1547 children.add(new Property("name", "string", 1548 "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, 1549 name)); 1550 children.add(new Property("subject", "Reference(Patient|Group)", 1551 "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject)); 1552 children.add(new Property("encounter", "Reference(Encounter)", 1553 "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.", 1554 0, 1, encounter)); 1555 children.add(new Property("period", "Period", 1556 "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period)); 1557 children.add(new Property("participant", "", 1558 "Identifies all people and organizations who are expected to be involved in the care team.", 0, 1559 java.lang.Integer.MAX_VALUE, participant)); 1560 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, 1561 java.lang.Integer.MAX_VALUE, reasonCode)); 1562 children.add(new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 1563 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1564 children.add(new Property("managingOrganization", "Reference(Organization)", 1565 "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization)); 1566 children.add(new Property("telecom", "ContactPoint", 1567 "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, 1568 telecom)); 1569 children.add( 1570 new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note)); 1571 } 1572 1573 @Override 1574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1575 switch (_hash) { 1576 case -1618432855: 1577 /* identifier */ return new Property("identifier", "Identifier", 1578 "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1579 0, java.lang.Integer.MAX_VALUE, identifier); 1580 case -892481550: 1581 /* status */ return new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status); 1582 case 50511102: 1583 /* category */ return new Property("category", "CodeableConcept", 1584 "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 1585 0, java.lang.Integer.MAX_VALUE, category); 1586 case 3373707: 1587 /* name */ return new Property("name", "string", 1588 "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1589 1, name); 1590 case -1867885268: 1591 /* subject */ return new Property("subject", "Reference(Patient|Group)", 1592 "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject); 1593 case 1524132147: 1594 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1595 "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.", 1596 0, 1, encounter); 1597 case -991726143: 1598 /* period */ return new Property("period", "Period", 1599 "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period); 1600 case 767422259: 1601 /* participant */ return new Property("participant", "", 1602 "Identifies all people and organizations who are expected to be involved in the care team.", 0, 1603 java.lang.Integer.MAX_VALUE, participant); 1604 case 722137681: 1605 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, 1606 java.lang.Integer.MAX_VALUE, reasonCode); 1607 case -1146218137: 1608 /* reasonReference */ return new Property("reasonReference", "Reference(Condition)", 1609 "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1610 case -2058947787: 1611 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1612 "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization); 1613 case -1429363305: 1614 /* telecom */ return new Property("telecom", "ContactPoint", 1615 "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, 1616 telecom); 1617 case 3387378: 1618 /* note */ return new Property("note", "Annotation", "Comments made about the CareTeam.", 0, 1619 java.lang.Integer.MAX_VALUE, note); 1620 default: 1621 return super.getNamedProperty(_hash, _name, _checkValid); 1622 } 1623 1624 } 1625 1626 @Override 1627 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1628 switch (hash) { 1629 case -1618432855: 1630 /* identifier */ return this.identifier == null ? new Base[0] 1631 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1632 case -892481550: 1633 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CareTeamStatus> 1634 case 50511102: 1635 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1636 case 3373707: 1637 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1638 case -1867885268: 1639 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1640 case 1524132147: 1641 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1642 case -991726143: 1643 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1644 case 767422259: 1645 /* participant */ return this.participant == null ? new Base[0] 1646 : this.participant.toArray(new Base[this.participant.size()]); // CareTeamParticipantComponent 1647 case 722137681: 1648 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1649 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1650 case -1146218137: 1651 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1652 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1653 case -2058947787: 1654 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1655 : this.managingOrganization.toArray(new Base[this.managingOrganization.size()]); // Reference 1656 case -1429363305: 1657 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1658 case 3387378: 1659 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1660 default: 1661 return super.getProperty(hash, name, checkValid); 1662 } 1663 1664 } 1665 1666 @Override 1667 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1668 switch (hash) { 1669 case -1618432855: // identifier 1670 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1671 return value; 1672 case -892481550: // status 1673 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1674 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1675 return value; 1676 case 50511102: // category 1677 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1678 return value; 1679 case 3373707: // name 1680 this.name = castToString(value); // StringType 1681 return value; 1682 case -1867885268: // subject 1683 this.subject = castToReference(value); // Reference 1684 return value; 1685 case 1524132147: // encounter 1686 this.encounter = castToReference(value); // Reference 1687 return value; 1688 case -991726143: // period 1689 this.period = castToPeriod(value); // Period 1690 return value; 1691 case 767422259: // participant 1692 this.getParticipant().add((CareTeamParticipantComponent) value); // CareTeamParticipantComponent 1693 return value; 1694 case 722137681: // reasonCode 1695 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1696 return value; 1697 case -1146218137: // reasonReference 1698 this.getReasonReference().add(castToReference(value)); // Reference 1699 return value; 1700 case -2058947787: // managingOrganization 1701 this.getManagingOrganization().add(castToReference(value)); // Reference 1702 return value; 1703 case -1429363305: // telecom 1704 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1705 return value; 1706 case 3387378: // note 1707 this.getNote().add(castToAnnotation(value)); // Annotation 1708 return value; 1709 default: 1710 return super.setProperty(hash, name, value); 1711 } 1712 1713 } 1714 1715 @Override 1716 public Base setProperty(String name, Base value) throws FHIRException { 1717 if (name.equals("identifier")) { 1718 this.getIdentifier().add(castToIdentifier(value)); 1719 } else if (name.equals("status")) { 1720 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1721 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1722 } else if (name.equals("category")) { 1723 this.getCategory().add(castToCodeableConcept(value)); 1724 } else if (name.equals("name")) { 1725 this.name = castToString(value); // StringType 1726 } else if (name.equals("subject")) { 1727 this.subject = castToReference(value); // Reference 1728 } else if (name.equals("encounter")) { 1729 this.encounter = castToReference(value); // Reference 1730 } else if (name.equals("period")) { 1731 this.period = castToPeriod(value); // Period 1732 } else if (name.equals("participant")) { 1733 this.getParticipant().add((CareTeamParticipantComponent) value); 1734 } else if (name.equals("reasonCode")) { 1735 this.getReasonCode().add(castToCodeableConcept(value)); 1736 } else if (name.equals("reasonReference")) { 1737 this.getReasonReference().add(castToReference(value)); 1738 } else if (name.equals("managingOrganization")) { 1739 this.getManagingOrganization().add(castToReference(value)); 1740 } else if (name.equals("telecom")) { 1741 this.getTelecom().add(castToContactPoint(value)); 1742 } else if (name.equals("note")) { 1743 this.getNote().add(castToAnnotation(value)); 1744 } else 1745 return super.setProperty(name, value); 1746 return value; 1747 } 1748 1749 @Override 1750 public Base makeProperty(int hash, String name) throws FHIRException { 1751 switch (hash) { 1752 case -1618432855: 1753 return addIdentifier(); 1754 case -892481550: 1755 return getStatusElement(); 1756 case 50511102: 1757 return addCategory(); 1758 case 3373707: 1759 return getNameElement(); 1760 case -1867885268: 1761 return getSubject(); 1762 case 1524132147: 1763 return getEncounter(); 1764 case -991726143: 1765 return getPeriod(); 1766 case 767422259: 1767 return addParticipant(); 1768 case 722137681: 1769 return addReasonCode(); 1770 case -1146218137: 1771 return addReasonReference(); 1772 case -2058947787: 1773 return addManagingOrganization(); 1774 case -1429363305: 1775 return addTelecom(); 1776 case 3387378: 1777 return addNote(); 1778 default: 1779 return super.makeProperty(hash, name); 1780 } 1781 1782 } 1783 1784 @Override 1785 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1786 switch (hash) { 1787 case -1618432855: 1788 /* identifier */ return new String[] { "Identifier" }; 1789 case -892481550: 1790 /* status */ return new String[] { "code" }; 1791 case 50511102: 1792 /* category */ return new String[] { "CodeableConcept" }; 1793 case 3373707: 1794 /* name */ return new String[] { "string" }; 1795 case -1867885268: 1796 /* subject */ return new String[] { "Reference" }; 1797 case 1524132147: 1798 /* encounter */ return new String[] { "Reference" }; 1799 case -991726143: 1800 /* period */ return new String[] { "Period" }; 1801 case 767422259: 1802 /* participant */ return new String[] {}; 1803 case 722137681: 1804 /* reasonCode */ return new String[] { "CodeableConcept" }; 1805 case -1146218137: 1806 /* reasonReference */ return new String[] { "Reference" }; 1807 case -2058947787: 1808 /* managingOrganization */ return new String[] { "Reference" }; 1809 case -1429363305: 1810 /* telecom */ return new String[] { "ContactPoint" }; 1811 case 3387378: 1812 /* note */ return new String[] { "Annotation" }; 1813 default: 1814 return super.getTypesForProperty(hash, name); 1815 } 1816 1817 } 1818 1819 @Override 1820 public Base addChild(String name) throws FHIRException { 1821 if (name.equals("identifier")) { 1822 return addIdentifier(); 1823 } else if (name.equals("status")) { 1824 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.status"); 1825 } else if (name.equals("category")) { 1826 return addCategory(); 1827 } else if (name.equals("name")) { 1828 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.name"); 1829 } else if (name.equals("subject")) { 1830 this.subject = new Reference(); 1831 return this.subject; 1832 } else if (name.equals("encounter")) { 1833 this.encounter = new Reference(); 1834 return this.encounter; 1835 } else if (name.equals("period")) { 1836 this.period = new Period(); 1837 return this.period; 1838 } else if (name.equals("participant")) { 1839 return addParticipant(); 1840 } else if (name.equals("reasonCode")) { 1841 return addReasonCode(); 1842 } else if (name.equals("reasonReference")) { 1843 return addReasonReference(); 1844 } else if (name.equals("managingOrganization")) { 1845 return addManagingOrganization(); 1846 } else if (name.equals("telecom")) { 1847 return addTelecom(); 1848 } else if (name.equals("note")) { 1849 return addNote(); 1850 } else 1851 return super.addChild(name); 1852 } 1853 1854 public String fhirType() { 1855 return "CareTeam"; 1856 1857 } 1858 1859 public CareTeam copy() { 1860 CareTeam dst = new CareTeam(); 1861 copyValues(dst); 1862 return dst; 1863 } 1864 1865 public void copyValues(CareTeam dst) { 1866 super.copyValues(dst); 1867 if (identifier != null) { 1868 dst.identifier = new ArrayList<Identifier>(); 1869 for (Identifier i : identifier) 1870 dst.identifier.add(i.copy()); 1871 } 1872 ; 1873 dst.status = status == null ? null : status.copy(); 1874 if (category != null) { 1875 dst.category = new ArrayList<CodeableConcept>(); 1876 for (CodeableConcept i : category) 1877 dst.category.add(i.copy()); 1878 } 1879 ; 1880 dst.name = name == null ? null : name.copy(); 1881 dst.subject = subject == null ? null : subject.copy(); 1882 dst.encounter = encounter == null ? null : encounter.copy(); 1883 dst.period = period == null ? null : period.copy(); 1884 if (participant != null) { 1885 dst.participant = new ArrayList<CareTeamParticipantComponent>(); 1886 for (CareTeamParticipantComponent i : participant) 1887 dst.participant.add(i.copy()); 1888 } 1889 ; 1890 if (reasonCode != null) { 1891 dst.reasonCode = new ArrayList<CodeableConcept>(); 1892 for (CodeableConcept i : reasonCode) 1893 dst.reasonCode.add(i.copy()); 1894 } 1895 ; 1896 if (reasonReference != null) { 1897 dst.reasonReference = new ArrayList<Reference>(); 1898 for (Reference i : reasonReference) 1899 dst.reasonReference.add(i.copy()); 1900 } 1901 ; 1902 if (managingOrganization != null) { 1903 dst.managingOrganization = new ArrayList<Reference>(); 1904 for (Reference i : managingOrganization) 1905 dst.managingOrganization.add(i.copy()); 1906 } 1907 ; 1908 if (telecom != null) { 1909 dst.telecom = new ArrayList<ContactPoint>(); 1910 for (ContactPoint i : telecom) 1911 dst.telecom.add(i.copy()); 1912 } 1913 ; 1914 if (note != null) { 1915 dst.note = new ArrayList<Annotation>(); 1916 for (Annotation i : note) 1917 dst.note.add(i.copy()); 1918 } 1919 ; 1920 } 1921 1922 protected CareTeam typedCopy() { 1923 return copy(); 1924 } 1925 1926 @Override 1927 public boolean equalsDeep(Base other_) { 1928 if (!super.equalsDeep(other_)) 1929 return false; 1930 if (!(other_ instanceof CareTeam)) 1931 return false; 1932 CareTeam o = (CareTeam) other_; 1933 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1934 && compareDeep(category, o.category, true) && compareDeep(name, o.name, true) 1935 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1936 && compareDeep(period, o.period, true) && compareDeep(participant, o.participant, true) 1937 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 1938 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(telecom, o.telecom, true) 1939 && compareDeep(note, o.note, true); 1940 } 1941 1942 @Override 1943 public boolean equalsShallow(Base other_) { 1944 if (!super.equalsShallow(other_)) 1945 return false; 1946 if (!(other_ instanceof CareTeam)) 1947 return false; 1948 CareTeam o = (CareTeam) other_; 1949 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 1950 } 1951 1952 public boolean isEmpty() { 1953 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, name, subject, 1954 encounter, period, participant, reasonCode, reasonReference, managingOrganization, telecom, note); 1955 } 1956 1957 @Override 1958 public ResourceType getResourceType() { 1959 return ResourceType.CareTeam; 1960 } 1961 1962 /** 1963 * Search parameter: <b>date</b> 1964 * <p> 1965 * Description: <b>Time period team covers</b><br> 1966 * Type: <b>date</b><br> 1967 * Path: <b>CareTeam.period</b><br> 1968 * </p> 1969 */ 1970 @SearchParamDefinition(name = "date", path = "CareTeam.period", description = "Time period team covers", type = "date") 1971 public static final String SP_DATE = "date"; 1972 /** 1973 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1974 * <p> 1975 * Description: <b>Time period team covers</b><br> 1976 * Type: <b>date</b><br> 1977 * Path: <b>CareTeam.period</b><br> 1978 * </p> 1979 */ 1980 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1981 SP_DATE); 1982 1983 /** 1984 * Search parameter: <b>identifier</b> 1985 * <p> 1986 * Description: <b>External Ids for this team</b><br> 1987 * Type: <b>token</b><br> 1988 * Path: <b>CareTeam.identifier</b><br> 1989 * </p> 1990 */ 1991 @SearchParamDefinition(name = "identifier", path = "CareTeam.identifier", description = "External Ids for this team", type = "token") 1992 public static final String SP_IDENTIFIER = "identifier"; 1993 /** 1994 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1995 * <p> 1996 * Description: <b>External Ids for this team</b><br> 1997 * Type: <b>token</b><br> 1998 * Path: <b>CareTeam.identifier</b><br> 1999 * </p> 2000 */ 2001 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2002 SP_IDENTIFIER); 2003 2004 /** 2005 * Search parameter: <b>patient</b> 2006 * <p> 2007 * Description: <b>Who care team is for</b><br> 2008 * Type: <b>reference</b><br> 2009 * Path: <b>CareTeam.subject</b><br> 2010 * </p> 2011 */ 2012 @SearchParamDefinition(name = "patient", path = "CareTeam.subject.where(resolve() is Patient)", description = "Who care team is for", type = "reference", providesMembershipIn = { 2013 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2014 public static final String SP_PATIENT = "patient"; 2015 /** 2016 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2017 * <p> 2018 * Description: <b>Who care team is for</b><br> 2019 * Type: <b>reference</b><br> 2020 * Path: <b>CareTeam.subject</b><br> 2021 * </p> 2022 */ 2023 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2024 SP_PATIENT); 2025 2026 /** 2027 * Constant for fluent queries to be used to add include statements. Specifies 2028 * the path value of "<b>CareTeam:patient</b>". 2029 */ 2030 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2031 "CareTeam:patient").toLocked(); 2032 2033 /** 2034 * Search parameter: <b>subject</b> 2035 * <p> 2036 * Description: <b>Who care team is for</b><br> 2037 * Type: <b>reference</b><br> 2038 * Path: <b>CareTeam.subject</b><br> 2039 * </p> 2040 */ 2041 @SearchParamDefinition(name = "subject", path = "CareTeam.subject", description = "Who care team is for", type = "reference", target = { 2042 Group.class, Patient.class }) 2043 public static final String SP_SUBJECT = "subject"; 2044 /** 2045 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2046 * <p> 2047 * Description: <b>Who care team is for</b><br> 2048 * Type: <b>reference</b><br> 2049 * Path: <b>CareTeam.subject</b><br> 2050 * </p> 2051 */ 2052 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2053 SP_SUBJECT); 2054 2055 /** 2056 * Constant for fluent queries to be used to add include statements. Specifies 2057 * the path value of "<b>CareTeam:subject</b>". 2058 */ 2059 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2060 "CareTeam:subject").toLocked(); 2061 2062 /** 2063 * Search parameter: <b>encounter</b> 2064 * <p> 2065 * Description: <b>Encounter created as part of</b><br> 2066 * Type: <b>reference</b><br> 2067 * Path: <b>CareTeam.encounter</b><br> 2068 * </p> 2069 */ 2070 @SearchParamDefinition(name = "encounter", path = "CareTeam.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2071 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2072 public static final String SP_ENCOUNTER = "encounter"; 2073 /** 2074 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2075 * <p> 2076 * Description: <b>Encounter created as part of</b><br> 2077 * Type: <b>reference</b><br> 2078 * Path: <b>CareTeam.encounter</b><br> 2079 * </p> 2080 */ 2081 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2082 SP_ENCOUNTER); 2083 2084 /** 2085 * Constant for fluent queries to be used to add include statements. Specifies 2086 * the path value of "<b>CareTeam:encounter</b>". 2087 */ 2088 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2089 "CareTeam:encounter").toLocked(); 2090 2091 /** 2092 * Search parameter: <b>category</b> 2093 * <p> 2094 * Description: <b>Type of team</b><br> 2095 * Type: <b>token</b><br> 2096 * Path: <b>CareTeam.category</b><br> 2097 * </p> 2098 */ 2099 @SearchParamDefinition(name = "category", path = "CareTeam.category", description = "Type of team", type = "token") 2100 public static final String SP_CATEGORY = "category"; 2101 /** 2102 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2103 * <p> 2104 * Description: <b>Type of team</b><br> 2105 * Type: <b>token</b><br> 2106 * Path: <b>CareTeam.category</b><br> 2107 * </p> 2108 */ 2109 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2110 SP_CATEGORY); 2111 2112 /** 2113 * Search parameter: <b>participant</b> 2114 * <p> 2115 * Description: <b>Who is involved</b><br> 2116 * Type: <b>reference</b><br> 2117 * Path: <b>CareTeam.participant.member</b><br> 2118 * </p> 2119 */ 2120 @SearchParamDefinition(name = "participant", path = "CareTeam.participant.member", description = "Who is involved", type = "reference", providesMembershipIn = { 2121 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2122 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2123 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, 2124 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2125 public static final String SP_PARTICIPANT = "participant"; 2126 /** 2127 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 2128 * <p> 2129 * Description: <b>Who is involved</b><br> 2130 * Type: <b>reference</b><br> 2131 * Path: <b>CareTeam.participant.member</b><br> 2132 * </p> 2133 */ 2134 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2135 SP_PARTICIPANT); 2136 2137 /** 2138 * Constant for fluent queries to be used to add include statements. Specifies 2139 * the path value of "<b>CareTeam:participant</b>". 2140 */ 2141 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 2142 "CareTeam:participant").toLocked(); 2143 2144 /** 2145 * Search parameter: <b>status</b> 2146 * <p> 2147 * Description: <b>proposed | active | suspended | inactive | 2148 * entered-in-error</b><br> 2149 * Type: <b>token</b><br> 2150 * Path: <b>CareTeam.status</b><br> 2151 * </p> 2152 */ 2153 @SearchParamDefinition(name = "status", path = "CareTeam.status", description = "proposed | active | suspended | inactive | entered-in-error", type = "token") 2154 public static final String SP_STATUS = "status"; 2155 /** 2156 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2157 * <p> 2158 * Description: <b>proposed | active | suspended | inactive | 2159 * entered-in-error</b><br> 2160 * Type: <b>token</b><br> 2161 * Path: <b>CareTeam.status</b><br> 2162 * </p> 2163 */ 2164 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2165 SP_STATUS); 2166 2167}