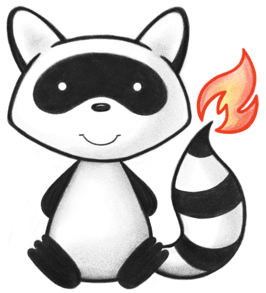
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046 047/** 048 * Catalog entries are wrappers that contextualize items included in a catalog. 049 */ 050@ResourceDef(name = "CatalogEntry", profile = "http://hl7.org/fhir/StructureDefinition/CatalogEntry") 051public class CatalogEntry extends DomainResource { 052 053 public enum CatalogEntryRelationType { 054 /** 055 * the related entry represents an activity that may be triggered by the current 056 * item. 057 */ 058 TRIGGERS, 059 /** 060 * the related entry represents an item that replaces the current retired item. 061 */ 062 ISREPLACEDBY, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 068 public static CatalogEntryRelationType fromCode(String codeString) throws FHIRException { 069 if (codeString == null || "".equals(codeString)) 070 return null; 071 if ("triggers".equals(codeString)) 072 return TRIGGERS; 073 if ("is-replaced-by".equals(codeString)) 074 return ISREPLACEDBY; 075 if (Configuration.isAcceptInvalidEnums()) 076 return null; 077 else 078 throw new FHIRException("Unknown CatalogEntryRelationType code '" + codeString + "'"); 079 } 080 081 public String toCode() { 082 switch (this) { 083 case TRIGGERS: 084 return "triggers"; 085 case ISREPLACEDBY: 086 return "is-replaced-by"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getSystem() { 095 switch (this) { 096 case TRIGGERS: 097 return "http://hl7.org/fhir/relation-type"; 098 case ISREPLACEDBY: 099 return "http://hl7.org/fhir/relation-type"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getDefinition() { 108 switch (this) { 109 case TRIGGERS: 110 return "the related entry represents an activity that may be triggered by the current item."; 111 case ISREPLACEDBY: 112 return "the related entry represents an item that replaces the current retired item."; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getDisplay() { 121 switch (this) { 122 case TRIGGERS: 123 return "Triggers"; 124 case ISREPLACEDBY: 125 return "Replaced By"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 } 133 134 public static class CatalogEntryRelationTypeEnumFactory implements EnumFactory<CatalogEntryRelationType> { 135 public CatalogEntryRelationType fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("triggers".equals(codeString)) 140 return CatalogEntryRelationType.TRIGGERS; 141 if ("is-replaced-by".equals(codeString)) 142 return CatalogEntryRelationType.ISREPLACEDBY; 143 throw new IllegalArgumentException("Unknown CatalogEntryRelationType code '" + codeString + "'"); 144 } 145 146 public Enumeration<CatalogEntryRelationType> fromType(PrimitiveType<?> code) throws FHIRException { 147 if (code == null) 148 return null; 149 if (code.isEmpty()) 150 return new Enumeration<CatalogEntryRelationType>(this, CatalogEntryRelationType.NULL, code); 151 String codeString = code.asStringValue(); 152 if (codeString == null || "".equals(codeString)) 153 return new Enumeration<CatalogEntryRelationType>(this, CatalogEntryRelationType.NULL, code); 154 if ("triggers".equals(codeString)) 155 return new Enumeration<CatalogEntryRelationType>(this, CatalogEntryRelationType.TRIGGERS, code); 156 if ("is-replaced-by".equals(codeString)) 157 return new Enumeration<CatalogEntryRelationType>(this, CatalogEntryRelationType.ISREPLACEDBY, code); 158 throw new FHIRException("Unknown CatalogEntryRelationType code '" + codeString + "'"); 159 } 160 161 public String toCode(CatalogEntryRelationType code) { 162 if (code == CatalogEntryRelationType.NULL) 163 return null; 164 if (code == CatalogEntryRelationType.TRIGGERS) 165 return "triggers"; 166 if (code == CatalogEntryRelationType.ISREPLACEDBY) 167 return "is-replaced-by"; 168 return "?"; 169 } 170 171 public String toSystem(CatalogEntryRelationType code) { 172 return code.getSystem(); 173 } 174 } 175 176 @Block() 177 public static class CatalogEntryRelatedEntryComponent extends BackboneElement implements IBaseBackboneElement { 178 /** 179 * The type of relation to the related item: child, parent, packageContent, 180 * containerPackage, usedIn, uses, requires, etc. 181 */ 182 @Child(name = "relationtype", type = { 183 CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 184 @Description(shortDefinition = "triggers | is-replaced-by", formalDefinition = "The type of relation to the related item: child, parent, packageContent, containerPackage, usedIn, uses, requires, etc.") 185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/relation-type") 186 protected Enumeration<CatalogEntryRelationType> relationtype; 187 188 /** 189 * The reference to the related item. 190 */ 191 @Child(name = "item", type = { CatalogEntry.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 192 @Description(shortDefinition = "The reference to the related item", formalDefinition = "The reference to the related item.") 193 protected Reference item; 194 195 /** 196 * The actual object that is the target of the reference (The reference to the 197 * related item.) 198 */ 199 protected CatalogEntry itemTarget; 200 201 private static final long serialVersionUID = -1367020813L; 202 203 /** 204 * Constructor 205 */ 206 public CatalogEntryRelatedEntryComponent() { 207 super(); 208 } 209 210 /** 211 * Constructor 212 */ 213 public CatalogEntryRelatedEntryComponent(Enumeration<CatalogEntryRelationType> relationtype, Reference item) { 214 super(); 215 this.relationtype = relationtype; 216 this.item = item; 217 } 218 219 /** 220 * @return {@link #relationtype} (The type of relation to the related item: 221 * child, parent, packageContent, containerPackage, usedIn, uses, 222 * requires, etc.). This is the underlying object with id, value and 223 * extensions. The accessor "getRelationtype" gives direct access to the 224 * value 225 */ 226 public Enumeration<CatalogEntryRelationType> getRelationtypeElement() { 227 if (this.relationtype == null) 228 if (Configuration.errorOnAutoCreate()) 229 throw new Error("Attempt to auto-create CatalogEntryRelatedEntryComponent.relationtype"); 230 else if (Configuration.doAutoCreate()) 231 this.relationtype = new Enumeration<CatalogEntryRelationType>(new CatalogEntryRelationTypeEnumFactory()); // bb 232 return this.relationtype; 233 } 234 235 public boolean hasRelationtypeElement() { 236 return this.relationtype != null && !this.relationtype.isEmpty(); 237 } 238 239 public boolean hasRelationtype() { 240 return this.relationtype != null && !this.relationtype.isEmpty(); 241 } 242 243 /** 244 * @param value {@link #relationtype} (The type of relation to the related item: 245 * child, parent, packageContent, containerPackage, usedIn, uses, 246 * requires, etc.). This is the underlying object with id, value 247 * and extensions. The accessor "getRelationtype" gives direct 248 * access to the value 249 */ 250 public CatalogEntryRelatedEntryComponent setRelationtypeElement(Enumeration<CatalogEntryRelationType> value) { 251 this.relationtype = value; 252 return this; 253 } 254 255 /** 256 * @return The type of relation to the related item: child, parent, 257 * packageContent, containerPackage, usedIn, uses, requires, etc. 258 */ 259 public CatalogEntryRelationType getRelationtype() { 260 return this.relationtype == null ? null : this.relationtype.getValue(); 261 } 262 263 /** 264 * @param value The type of relation to the related item: child, parent, 265 * packageContent, containerPackage, usedIn, uses, requires, etc. 266 */ 267 public CatalogEntryRelatedEntryComponent setRelationtype(CatalogEntryRelationType value) { 268 if (this.relationtype == null) 269 this.relationtype = new Enumeration<CatalogEntryRelationType>(new CatalogEntryRelationTypeEnumFactory()); 270 this.relationtype.setValue(value); 271 return this; 272 } 273 274 /** 275 * @return {@link #item} (The reference to the related item.) 276 */ 277 public Reference getItem() { 278 if (this.item == null) 279 if (Configuration.errorOnAutoCreate()) 280 throw new Error("Attempt to auto-create CatalogEntryRelatedEntryComponent.item"); 281 else if (Configuration.doAutoCreate()) 282 this.item = new Reference(); // cc 283 return this.item; 284 } 285 286 public boolean hasItem() { 287 return this.item != null && !this.item.isEmpty(); 288 } 289 290 /** 291 * @param value {@link #item} (The reference to the related item.) 292 */ 293 public CatalogEntryRelatedEntryComponent setItem(Reference value) { 294 this.item = value; 295 return this; 296 } 297 298 /** 299 * @return {@link #item} The actual object that is the target of the reference. 300 * The reference library doesn't populate this, but you can use it to 301 * hold the resource if you resolve it. (The reference to the related 302 * item.) 303 */ 304 public CatalogEntry getItemTarget() { 305 if (this.itemTarget == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create CatalogEntryRelatedEntryComponent.item"); 308 else if (Configuration.doAutoCreate()) 309 this.itemTarget = new CatalogEntry(); // aa 310 return this.itemTarget; 311 } 312 313 /** 314 * @param value {@link #item} The actual object that is the target of the 315 * reference. The reference library doesn't use these, but you can 316 * use it to hold the resource if you resolve it. (The reference to 317 * the related item.) 318 */ 319 public CatalogEntryRelatedEntryComponent setItemTarget(CatalogEntry value) { 320 this.itemTarget = value; 321 return this; 322 } 323 324 protected void listChildren(List<Property> children) { 325 super.listChildren(children); 326 children.add(new Property("relationtype", "code", 327 "The type of relation to the related item: child, parent, packageContent, containerPackage, usedIn, uses, requires, etc.", 328 0, 1, relationtype)); 329 children.add(new Property("item", "Reference(CatalogEntry)", "The reference to the related item.", 0, 1, item)); 330 } 331 332 @Override 333 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 334 switch (_hash) { 335 case -261805258: 336 /* relationtype */ return new Property("relationtype", "code", 337 "The type of relation to the related item: child, parent, packageContent, containerPackage, usedIn, uses, requires, etc.", 338 0, 1, relationtype); 339 case 3242771: 340 /* item */ return new Property("item", "Reference(CatalogEntry)", "The reference to the related item.", 0, 1, 341 item); 342 default: 343 return super.getNamedProperty(_hash, _name, _checkValid); 344 } 345 346 } 347 348 @Override 349 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 350 switch (hash) { 351 case -261805258: 352 /* relationtype */ return this.relationtype == null ? new Base[0] : new Base[] { this.relationtype }; // Enumeration<CatalogEntryRelationType> 353 case 3242771: 354 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Reference 355 default: 356 return super.getProperty(hash, name, checkValid); 357 } 358 359 } 360 361 @Override 362 public Base setProperty(int hash, String name, Base value) throws FHIRException { 363 switch (hash) { 364 case -261805258: // relationtype 365 value = new CatalogEntryRelationTypeEnumFactory().fromType(castToCode(value)); 366 this.relationtype = (Enumeration) value; // Enumeration<CatalogEntryRelationType> 367 return value; 368 case 3242771: // item 369 this.item = castToReference(value); // Reference 370 return value; 371 default: 372 return super.setProperty(hash, name, value); 373 } 374 375 } 376 377 @Override 378 public Base setProperty(String name, Base value) throws FHIRException { 379 if (name.equals("relationtype")) { 380 value = new CatalogEntryRelationTypeEnumFactory().fromType(castToCode(value)); 381 this.relationtype = (Enumeration) value; // Enumeration<CatalogEntryRelationType> 382 } else if (name.equals("item")) { 383 this.item = castToReference(value); // Reference 384 } else 385 return super.setProperty(name, value); 386 return value; 387 } 388 389 @Override 390 public void removeChild(String name, Base value) throws FHIRException { 391 if (name.equals("relationtype")) { 392 this.relationtype = null; 393 } else if (name.equals("item")) { 394 this.item = null; 395 } else 396 super.removeChild(name, value); 397 398 } 399 400 @Override 401 public Base makeProperty(int hash, String name) throws FHIRException { 402 switch (hash) { 403 case -261805258: 404 return getRelationtypeElement(); 405 case 3242771: 406 return getItem(); 407 default: 408 return super.makeProperty(hash, name); 409 } 410 411 } 412 413 @Override 414 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 415 switch (hash) { 416 case -261805258: 417 /* relationtype */ return new String[] { "code" }; 418 case 3242771: 419 /* item */ return new String[] { "Reference" }; 420 default: 421 return super.getTypesForProperty(hash, name); 422 } 423 424 } 425 426 @Override 427 public Base addChild(String name) throws FHIRException { 428 if (name.equals("relationtype")) { 429 throw new FHIRException("Cannot call addChild on a singleton property CatalogEntry.relationtype"); 430 } else if (name.equals("item")) { 431 this.item = new Reference(); 432 return this.item; 433 } else 434 return super.addChild(name); 435 } 436 437 public CatalogEntryRelatedEntryComponent copy() { 438 CatalogEntryRelatedEntryComponent dst = new CatalogEntryRelatedEntryComponent(); 439 copyValues(dst); 440 return dst; 441 } 442 443 public void copyValues(CatalogEntryRelatedEntryComponent dst) { 444 super.copyValues(dst); 445 dst.relationtype = relationtype == null ? null : relationtype.copy(); 446 dst.item = item == null ? null : item.copy(); 447 } 448 449 @Override 450 public boolean equalsDeep(Base other_) { 451 if (!super.equalsDeep(other_)) 452 return false; 453 if (!(other_ instanceof CatalogEntryRelatedEntryComponent)) 454 return false; 455 CatalogEntryRelatedEntryComponent o = (CatalogEntryRelatedEntryComponent) other_; 456 return compareDeep(relationtype, o.relationtype, true) && compareDeep(item, o.item, true); 457 } 458 459 @Override 460 public boolean equalsShallow(Base other_) { 461 if (!super.equalsShallow(other_)) 462 return false; 463 if (!(other_ instanceof CatalogEntryRelatedEntryComponent)) 464 return false; 465 CatalogEntryRelatedEntryComponent o = (CatalogEntryRelatedEntryComponent) other_; 466 return compareValues(relationtype, o.relationtype, true); 467 } 468 469 public boolean isEmpty() { 470 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relationtype, item); 471 } 472 473 public String fhirType() { 474 return "CatalogEntry.relatedEntry"; 475 476 } 477 478 } 479 480 /** 481 * Used in supporting different identifiers for the same product, e.g. 482 * manufacturer code and retailer code. 483 */ 484 @Child(name = "identifier", type = { 485 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 486 @Description(shortDefinition = "Unique identifier of the catalog item", formalDefinition = "Used in supporting different identifiers for the same product, e.g. manufacturer code and retailer code.") 487 protected List<Identifier> identifier; 488 489 /** 490 * The type of item - medication, device, service, protocol or other. 491 */ 492 @Child(name = "type", type = { 493 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 494 @Description(shortDefinition = "The type of item - medication, device, service, protocol or other", formalDefinition = "The type of item - medication, device, service, protocol or other.") 495 protected CodeableConcept type; 496 497 /** 498 * Whether the entry represents an orderable item. 499 */ 500 @Child(name = "orderable", type = { 501 BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 502 @Description(shortDefinition = "Whether the entry represents an orderable item", formalDefinition = "Whether the entry represents an orderable item.") 503 protected BooleanType orderable; 504 505 /** 506 * The item in a catalog or definition. 507 */ 508 @Child(name = "referencedItem", type = { Medication.class, Device.class, Organization.class, Practitioner.class, 509 PractitionerRole.class, HealthcareService.class, ActivityDefinition.class, PlanDefinition.class, 510 SpecimenDefinition.class, ObservationDefinition.class, 511 Binary.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 512 @Description(shortDefinition = "The item that is being defined", formalDefinition = "The item in a catalog or definition.") 513 protected Reference referencedItem; 514 515 /** 516 * The actual object that is the target of the reference (The item in a catalog 517 * or definition.) 518 */ 519 protected Resource referencedItemTarget; 520 521 /** 522 * Used in supporting related concepts, e.g. NDC to RxNorm. 523 */ 524 @Child(name = "additionalIdentifier", type = { 525 Identifier.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 526 @Description(shortDefinition = "Any additional identifier(s) for the catalog item, in the same granularity or concept", formalDefinition = "Used in supporting related concepts, e.g. NDC to RxNorm.") 527 protected List<Identifier> additionalIdentifier; 528 529 /** 530 * Classes of devices, or ATC for medication. 531 */ 532 @Child(name = "classification", type = { 533 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 534 @Description(shortDefinition = "Classification (category or class) of the item entry", formalDefinition = "Classes of devices, or ATC for medication.") 535 protected List<CodeableConcept> classification; 536 537 /** 538 * Used to support catalog exchange even for unsupported products, e.g. getting 539 * list of medications even if not prescribable. 540 */ 541 @Child(name = "status", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 542 @Description(shortDefinition = "draft | active | retired | unknown", formalDefinition = "Used to support catalog exchange even for unsupported products, e.g. getting list of medications even if not prescribable.") 543 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/publication-status") 544 protected Enumeration<PublicationStatus> status; 545 546 /** 547 * The time period in which this catalog entry is expected to be active. 548 */ 549 @Child(name = "validityPeriod", type = { 550 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 551 @Description(shortDefinition = "The time period in which this catalog entry is expected to be active", formalDefinition = "The time period in which this catalog entry is expected to be active.") 552 protected Period validityPeriod; 553 554 /** 555 * The date until which this catalog entry is expected to be active. 556 */ 557 @Child(name = "validTo", type = { 558 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 559 @Description(shortDefinition = "The date until which this catalog entry is expected to be active", formalDefinition = "The date until which this catalog entry is expected to be active.") 560 protected DateTimeType validTo; 561 562 /** 563 * Typically date of issue is different from the beginning of the validity. This 564 * can be used to see when an item was last updated. 565 */ 566 @Child(name = "lastUpdated", type = { 567 DateTimeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 568 @Description(shortDefinition = "When was this catalog last updated", formalDefinition = "Typically date of issue is different from the beginning of the validity. This can be used to see when an item was last updated.") 569 protected DateTimeType lastUpdated; 570 571 /** 572 * Used for examplefor Out of Formulary, or any specifics. 573 */ 574 @Child(name = "additionalCharacteristic", type = { 575 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 576 @Description(shortDefinition = "Additional characteristics of the catalog entry", formalDefinition = "Used for examplefor Out of Formulary, or any specifics.") 577 protected List<CodeableConcept> additionalCharacteristic; 578 579 /** 580 * User for example for ATC classification, or. 581 */ 582 @Child(name = "additionalClassification", type = { 583 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 584 @Description(shortDefinition = "Additional classification of the catalog entry", formalDefinition = "User for example for ATC classification, or.") 585 protected List<CodeableConcept> additionalClassification; 586 587 /** 588 * Used for example, to point to a substance, or to a device used to administer 589 * a medication. 590 */ 591 @Child(name = "relatedEntry", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 592 @Description(shortDefinition = "An item that this catalog entry is related to", formalDefinition = "Used for example, to point to a substance, or to a device used to administer a medication.") 593 protected List<CatalogEntryRelatedEntryComponent> relatedEntry; 594 595 private static final long serialVersionUID = 57448275L; 596 597 /** 598 * Constructor 599 */ 600 public CatalogEntry() { 601 super(); 602 } 603 604 /** 605 * Constructor 606 */ 607 public CatalogEntry(BooleanType orderable, Reference referencedItem) { 608 super(); 609 this.orderable = orderable; 610 this.referencedItem = referencedItem; 611 } 612 613 /** 614 * @return {@link #identifier} (Used in supporting different identifiers for the 615 * same product, e.g. manufacturer code and retailer code.) 616 */ 617 public List<Identifier> getIdentifier() { 618 if (this.identifier == null) 619 this.identifier = new ArrayList<Identifier>(); 620 return this.identifier; 621 } 622 623 /** 624 * @return Returns a reference to <code>this</code> for easy method chaining 625 */ 626 public CatalogEntry setIdentifier(List<Identifier> theIdentifier) { 627 this.identifier = theIdentifier; 628 return this; 629 } 630 631 public boolean hasIdentifier() { 632 if (this.identifier == null) 633 return false; 634 for (Identifier item : this.identifier) 635 if (!item.isEmpty()) 636 return true; 637 return false; 638 } 639 640 public Identifier addIdentifier() { // 3 641 Identifier t = new Identifier(); 642 if (this.identifier == null) 643 this.identifier = new ArrayList<Identifier>(); 644 this.identifier.add(t); 645 return t; 646 } 647 648 public CatalogEntry addIdentifier(Identifier t) { // 3 649 if (t == null) 650 return this; 651 if (this.identifier == null) 652 this.identifier = new ArrayList<Identifier>(); 653 this.identifier.add(t); 654 return this; 655 } 656 657 /** 658 * @return The first repetition of repeating field {@link #identifier}, creating 659 * it if it does not already exist 660 */ 661 public Identifier getIdentifierFirstRep() { 662 if (getIdentifier().isEmpty()) { 663 addIdentifier(); 664 } 665 return getIdentifier().get(0); 666 } 667 668 /** 669 * @return {@link #type} (The type of item - medication, device, service, 670 * protocol or other.) 671 */ 672 public CodeableConcept getType() { 673 if (this.type == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create CatalogEntry.type"); 676 else if (Configuration.doAutoCreate()) 677 this.type = new CodeableConcept(); // cc 678 return this.type; 679 } 680 681 public boolean hasType() { 682 return this.type != null && !this.type.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #type} (The type of item - medication, device, service, 687 * protocol or other.) 688 */ 689 public CatalogEntry setType(CodeableConcept value) { 690 this.type = value; 691 return this; 692 } 693 694 /** 695 * @return {@link #orderable} (Whether the entry represents an orderable item.). 696 * This is the underlying object with id, value and extensions. The 697 * accessor "getOrderable" gives direct access to the value 698 */ 699 public BooleanType getOrderableElement() { 700 if (this.orderable == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create CatalogEntry.orderable"); 703 else if (Configuration.doAutoCreate()) 704 this.orderable = new BooleanType(); // bb 705 return this.orderable; 706 } 707 708 public boolean hasOrderableElement() { 709 return this.orderable != null && !this.orderable.isEmpty(); 710 } 711 712 public boolean hasOrderable() { 713 return this.orderable != null && !this.orderable.isEmpty(); 714 } 715 716 /** 717 * @param value {@link #orderable} (Whether the entry represents an orderable 718 * item.). This is the underlying object with id, value and 719 * extensions. The accessor "getOrderable" gives direct access to 720 * the value 721 */ 722 public CatalogEntry setOrderableElement(BooleanType value) { 723 this.orderable = value; 724 return this; 725 } 726 727 /** 728 * @return Whether the entry represents an orderable item. 729 */ 730 public boolean getOrderable() { 731 return this.orderable == null || this.orderable.isEmpty() ? false : this.orderable.getValue(); 732 } 733 734 /** 735 * @param value Whether the entry represents an orderable item. 736 */ 737 public CatalogEntry setOrderable(boolean value) { 738 if (this.orderable == null) 739 this.orderable = new BooleanType(); 740 this.orderable.setValue(value); 741 return this; 742 } 743 744 /** 745 * @return {@link #referencedItem} (The item in a catalog or definition.) 746 */ 747 public Reference getReferencedItem() { 748 if (this.referencedItem == null) 749 if (Configuration.errorOnAutoCreate()) 750 throw new Error("Attempt to auto-create CatalogEntry.referencedItem"); 751 else if (Configuration.doAutoCreate()) 752 this.referencedItem = new Reference(); // cc 753 return this.referencedItem; 754 } 755 756 public boolean hasReferencedItem() { 757 return this.referencedItem != null && !this.referencedItem.isEmpty(); 758 } 759 760 /** 761 * @param value {@link #referencedItem} (The item in a catalog or definition.) 762 */ 763 public CatalogEntry setReferencedItem(Reference value) { 764 this.referencedItem = value; 765 return this; 766 } 767 768 /** 769 * @return {@link #referencedItem} The actual object that is the target of the 770 * reference. The reference library doesn't populate this, but you can 771 * use it to hold the resource if you resolve it. (The item in a catalog 772 * or definition.) 773 */ 774 public Resource getReferencedItemTarget() { 775 return this.referencedItemTarget; 776 } 777 778 /** 779 * @param value {@link #referencedItem} The actual object that is the target of 780 * the reference. The reference library doesn't use these, but you 781 * can use it to hold the resource if you resolve it. (The item in 782 * a catalog or definition.) 783 */ 784 public CatalogEntry setReferencedItemTarget(Resource value) { 785 this.referencedItemTarget = value; 786 return this; 787 } 788 789 /** 790 * @return {@link #additionalIdentifier} (Used in supporting related concepts, 791 * e.g. NDC to RxNorm.) 792 */ 793 public List<Identifier> getAdditionalIdentifier() { 794 if (this.additionalIdentifier == null) 795 this.additionalIdentifier = new ArrayList<Identifier>(); 796 return this.additionalIdentifier; 797 } 798 799 /** 800 * @return Returns a reference to <code>this</code> for easy method chaining 801 */ 802 public CatalogEntry setAdditionalIdentifier(List<Identifier> theAdditionalIdentifier) { 803 this.additionalIdentifier = theAdditionalIdentifier; 804 return this; 805 } 806 807 public boolean hasAdditionalIdentifier() { 808 if (this.additionalIdentifier == null) 809 return false; 810 for (Identifier item : this.additionalIdentifier) 811 if (!item.isEmpty()) 812 return true; 813 return false; 814 } 815 816 public Identifier addAdditionalIdentifier() { // 3 817 Identifier t = new Identifier(); 818 if (this.additionalIdentifier == null) 819 this.additionalIdentifier = new ArrayList<Identifier>(); 820 this.additionalIdentifier.add(t); 821 return t; 822 } 823 824 public CatalogEntry addAdditionalIdentifier(Identifier t) { // 3 825 if (t == null) 826 return this; 827 if (this.additionalIdentifier == null) 828 this.additionalIdentifier = new ArrayList<Identifier>(); 829 this.additionalIdentifier.add(t); 830 return this; 831 } 832 833 /** 834 * @return The first repetition of repeating field 835 * {@link #additionalIdentifier}, creating it if it does not already 836 * exist 837 */ 838 public Identifier getAdditionalIdentifierFirstRep() { 839 if (getAdditionalIdentifier().isEmpty()) { 840 addAdditionalIdentifier(); 841 } 842 return getAdditionalIdentifier().get(0); 843 } 844 845 /** 846 * @return {@link #classification} (Classes of devices, or ATC for medication.) 847 */ 848 public List<CodeableConcept> getClassification() { 849 if (this.classification == null) 850 this.classification = new ArrayList<CodeableConcept>(); 851 return this.classification; 852 } 853 854 /** 855 * @return Returns a reference to <code>this</code> for easy method chaining 856 */ 857 public CatalogEntry setClassification(List<CodeableConcept> theClassification) { 858 this.classification = theClassification; 859 return this; 860 } 861 862 public boolean hasClassification() { 863 if (this.classification == null) 864 return false; 865 for (CodeableConcept item : this.classification) 866 if (!item.isEmpty()) 867 return true; 868 return false; 869 } 870 871 public CodeableConcept addClassification() { // 3 872 CodeableConcept t = new CodeableConcept(); 873 if (this.classification == null) 874 this.classification = new ArrayList<CodeableConcept>(); 875 this.classification.add(t); 876 return t; 877 } 878 879 public CatalogEntry addClassification(CodeableConcept t) { // 3 880 if (t == null) 881 return this; 882 if (this.classification == null) 883 this.classification = new ArrayList<CodeableConcept>(); 884 this.classification.add(t); 885 return this; 886 } 887 888 /** 889 * @return The first repetition of repeating field {@link #classification}, 890 * creating it if it does not already exist 891 */ 892 public CodeableConcept getClassificationFirstRep() { 893 if (getClassification().isEmpty()) { 894 addClassification(); 895 } 896 return getClassification().get(0); 897 } 898 899 /** 900 * @return {@link #status} (Used to support catalog exchange even for 901 * unsupported products, e.g. getting list of medications even if not 902 * prescribable.). This is the underlying object with id, value and 903 * extensions. The accessor "getStatus" gives direct access to the value 904 */ 905 public Enumeration<PublicationStatus> getStatusElement() { 906 if (this.status == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create CatalogEntry.status"); 909 else if (Configuration.doAutoCreate()) 910 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 911 return this.status; 912 } 913 914 public boolean hasStatusElement() { 915 return this.status != null && !this.status.isEmpty(); 916 } 917 918 public boolean hasStatus() { 919 return this.status != null && !this.status.isEmpty(); 920 } 921 922 /** 923 * @param value {@link #status} (Used to support catalog exchange even for 924 * unsupported products, e.g. getting list of medications even if 925 * not prescribable.). This is the underlying object with id, value 926 * and extensions. The accessor "getStatus" gives direct access to 927 * the value 928 */ 929 public CatalogEntry setStatusElement(Enumeration<PublicationStatus> value) { 930 this.status = value; 931 return this; 932 } 933 934 /** 935 * @return Used to support catalog exchange even for unsupported products, e.g. 936 * getting list of medications even if not prescribable. 937 */ 938 public PublicationStatus getStatus() { 939 return this.status == null ? null : this.status.getValue(); 940 } 941 942 /** 943 * @param value Used to support catalog exchange even for unsupported products, 944 * e.g. getting list of medications even if not prescribable. 945 */ 946 public CatalogEntry setStatus(PublicationStatus value) { 947 if (value == null) 948 this.status = null; 949 else { 950 if (this.status == null) 951 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 952 this.status.setValue(value); 953 } 954 return this; 955 } 956 957 /** 958 * @return {@link #validityPeriod} (The time period in which this catalog entry 959 * is expected to be active.) 960 */ 961 public Period getValidityPeriod() { 962 if (this.validityPeriod == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create CatalogEntry.validityPeriod"); 965 else if (Configuration.doAutoCreate()) 966 this.validityPeriod = new Period(); // cc 967 return this.validityPeriod; 968 } 969 970 public boolean hasValidityPeriod() { 971 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #validityPeriod} (The time period in which this catalog 976 * entry is expected to be active.) 977 */ 978 public CatalogEntry setValidityPeriod(Period value) { 979 this.validityPeriod = value; 980 return this; 981 } 982 983 /** 984 * @return {@link #validTo} (The date until which this catalog entry is expected 985 * to be active.). This is the underlying object with id, value and 986 * extensions. The accessor "getValidTo" gives direct access to the 987 * value 988 */ 989 public DateTimeType getValidToElement() { 990 if (this.validTo == null) 991 if (Configuration.errorOnAutoCreate()) 992 throw new Error("Attempt to auto-create CatalogEntry.validTo"); 993 else if (Configuration.doAutoCreate()) 994 this.validTo = new DateTimeType(); // bb 995 return this.validTo; 996 } 997 998 public boolean hasValidToElement() { 999 return this.validTo != null && !this.validTo.isEmpty(); 1000 } 1001 1002 public boolean hasValidTo() { 1003 return this.validTo != null && !this.validTo.isEmpty(); 1004 } 1005 1006 /** 1007 * @param value {@link #validTo} (The date until which this catalog entry is 1008 * expected to be active.). This is the underlying object with id, 1009 * value and extensions. The accessor "getValidTo" gives direct 1010 * access to the value 1011 */ 1012 public CatalogEntry setValidToElement(DateTimeType value) { 1013 this.validTo = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return The date until which this catalog entry is expected to be active. 1019 */ 1020 public Date getValidTo() { 1021 return this.validTo == null ? null : this.validTo.getValue(); 1022 } 1023 1024 /** 1025 * @param value The date until which this catalog entry is expected to be 1026 * active. 1027 */ 1028 public CatalogEntry setValidTo(Date value) { 1029 if (value == null) 1030 this.validTo = null; 1031 else { 1032 if (this.validTo == null) 1033 this.validTo = new DateTimeType(); 1034 this.validTo.setValue(value); 1035 } 1036 return this; 1037 } 1038 1039 /** 1040 * @return {@link #lastUpdated} (Typically date of issue is different from the 1041 * beginning of the validity. This can be used to see when an item was 1042 * last updated.). This is the underlying object with id, value and 1043 * extensions. The accessor "getLastUpdated" gives direct access to the 1044 * value 1045 */ 1046 public DateTimeType getLastUpdatedElement() { 1047 if (this.lastUpdated == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create CatalogEntry.lastUpdated"); 1050 else if (Configuration.doAutoCreate()) 1051 this.lastUpdated = new DateTimeType(); // bb 1052 return this.lastUpdated; 1053 } 1054 1055 public boolean hasLastUpdatedElement() { 1056 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 1057 } 1058 1059 public boolean hasLastUpdated() { 1060 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #lastUpdated} (Typically date of issue is different from 1065 * the beginning of the validity. This can be used to see when an 1066 * item was last updated.). This is the underlying object with id, 1067 * value and extensions. The accessor "getLastUpdated" gives direct 1068 * access to the value 1069 */ 1070 public CatalogEntry setLastUpdatedElement(DateTimeType value) { 1071 this.lastUpdated = value; 1072 return this; 1073 } 1074 1075 /** 1076 * @return Typically date of issue is different from the beginning of the 1077 * validity. This can be used to see when an item was last updated. 1078 */ 1079 public Date getLastUpdated() { 1080 return this.lastUpdated == null ? null : this.lastUpdated.getValue(); 1081 } 1082 1083 /** 1084 * @param value Typically date of issue is different from the beginning of the 1085 * validity. This can be used to see when an item was last updated. 1086 */ 1087 public CatalogEntry setLastUpdated(Date value) { 1088 if (value == null) 1089 this.lastUpdated = null; 1090 else { 1091 if (this.lastUpdated == null) 1092 this.lastUpdated = new DateTimeType(); 1093 this.lastUpdated.setValue(value); 1094 } 1095 return this; 1096 } 1097 1098 /** 1099 * @return {@link #additionalCharacteristic} (Used for examplefor Out of 1100 * Formulary, or any specifics.) 1101 */ 1102 public List<CodeableConcept> getAdditionalCharacteristic() { 1103 if (this.additionalCharacteristic == null) 1104 this.additionalCharacteristic = new ArrayList<CodeableConcept>(); 1105 return this.additionalCharacteristic; 1106 } 1107 1108 /** 1109 * @return Returns a reference to <code>this</code> for easy method chaining 1110 */ 1111 public CatalogEntry setAdditionalCharacteristic(List<CodeableConcept> theAdditionalCharacteristic) { 1112 this.additionalCharacteristic = theAdditionalCharacteristic; 1113 return this; 1114 } 1115 1116 public boolean hasAdditionalCharacteristic() { 1117 if (this.additionalCharacteristic == null) 1118 return false; 1119 for (CodeableConcept item : this.additionalCharacteristic) 1120 if (!item.isEmpty()) 1121 return true; 1122 return false; 1123 } 1124 1125 public CodeableConcept addAdditionalCharacteristic() { // 3 1126 CodeableConcept t = new CodeableConcept(); 1127 if (this.additionalCharacteristic == null) 1128 this.additionalCharacteristic = new ArrayList<CodeableConcept>(); 1129 this.additionalCharacteristic.add(t); 1130 return t; 1131 } 1132 1133 public CatalogEntry addAdditionalCharacteristic(CodeableConcept t) { // 3 1134 if (t == null) 1135 return this; 1136 if (this.additionalCharacteristic == null) 1137 this.additionalCharacteristic = new ArrayList<CodeableConcept>(); 1138 this.additionalCharacteristic.add(t); 1139 return this; 1140 } 1141 1142 /** 1143 * @return The first repetition of repeating field 1144 * {@link #additionalCharacteristic}, creating it if it does not already 1145 * exist 1146 */ 1147 public CodeableConcept getAdditionalCharacteristicFirstRep() { 1148 if (getAdditionalCharacteristic().isEmpty()) { 1149 addAdditionalCharacteristic(); 1150 } 1151 return getAdditionalCharacteristic().get(0); 1152 } 1153 1154 /** 1155 * @return {@link #additionalClassification} (User for example for ATC 1156 * classification, or.) 1157 */ 1158 public List<CodeableConcept> getAdditionalClassification() { 1159 if (this.additionalClassification == null) 1160 this.additionalClassification = new ArrayList<CodeableConcept>(); 1161 return this.additionalClassification; 1162 } 1163 1164 /** 1165 * @return Returns a reference to <code>this</code> for easy method chaining 1166 */ 1167 public CatalogEntry setAdditionalClassification(List<CodeableConcept> theAdditionalClassification) { 1168 this.additionalClassification = theAdditionalClassification; 1169 return this; 1170 } 1171 1172 public boolean hasAdditionalClassification() { 1173 if (this.additionalClassification == null) 1174 return false; 1175 for (CodeableConcept item : this.additionalClassification) 1176 if (!item.isEmpty()) 1177 return true; 1178 return false; 1179 } 1180 1181 public CodeableConcept addAdditionalClassification() { // 3 1182 CodeableConcept t = new CodeableConcept(); 1183 if (this.additionalClassification == null) 1184 this.additionalClassification = new ArrayList<CodeableConcept>(); 1185 this.additionalClassification.add(t); 1186 return t; 1187 } 1188 1189 public CatalogEntry addAdditionalClassification(CodeableConcept t) { // 3 1190 if (t == null) 1191 return this; 1192 if (this.additionalClassification == null) 1193 this.additionalClassification = new ArrayList<CodeableConcept>(); 1194 this.additionalClassification.add(t); 1195 return this; 1196 } 1197 1198 /** 1199 * @return The first repetition of repeating field 1200 * {@link #additionalClassification}, creating it if it does not already 1201 * exist 1202 */ 1203 public CodeableConcept getAdditionalClassificationFirstRep() { 1204 if (getAdditionalClassification().isEmpty()) { 1205 addAdditionalClassification(); 1206 } 1207 return getAdditionalClassification().get(0); 1208 } 1209 1210 /** 1211 * @return {@link #relatedEntry} (Used for example, to point to a substance, or 1212 * to a device used to administer a medication.) 1213 */ 1214 public List<CatalogEntryRelatedEntryComponent> getRelatedEntry() { 1215 if (this.relatedEntry == null) 1216 this.relatedEntry = new ArrayList<CatalogEntryRelatedEntryComponent>(); 1217 return this.relatedEntry; 1218 } 1219 1220 /** 1221 * @return Returns a reference to <code>this</code> for easy method chaining 1222 */ 1223 public CatalogEntry setRelatedEntry(List<CatalogEntryRelatedEntryComponent> theRelatedEntry) { 1224 this.relatedEntry = theRelatedEntry; 1225 return this; 1226 } 1227 1228 public boolean hasRelatedEntry() { 1229 if (this.relatedEntry == null) 1230 return false; 1231 for (CatalogEntryRelatedEntryComponent item : this.relatedEntry) 1232 if (!item.isEmpty()) 1233 return true; 1234 return false; 1235 } 1236 1237 public CatalogEntryRelatedEntryComponent addRelatedEntry() { // 3 1238 CatalogEntryRelatedEntryComponent t = new CatalogEntryRelatedEntryComponent(); 1239 if (this.relatedEntry == null) 1240 this.relatedEntry = new ArrayList<CatalogEntryRelatedEntryComponent>(); 1241 this.relatedEntry.add(t); 1242 return t; 1243 } 1244 1245 public CatalogEntry addRelatedEntry(CatalogEntryRelatedEntryComponent t) { // 3 1246 if (t == null) 1247 return this; 1248 if (this.relatedEntry == null) 1249 this.relatedEntry = new ArrayList<CatalogEntryRelatedEntryComponent>(); 1250 this.relatedEntry.add(t); 1251 return this; 1252 } 1253 1254 /** 1255 * @return The first repetition of repeating field {@link #relatedEntry}, 1256 * creating it if it does not already exist 1257 */ 1258 public CatalogEntryRelatedEntryComponent getRelatedEntryFirstRep() { 1259 if (getRelatedEntry().isEmpty()) { 1260 addRelatedEntry(); 1261 } 1262 return getRelatedEntry().get(0); 1263 } 1264 1265 protected void listChildren(List<Property> children) { 1266 super.listChildren(children); 1267 children.add(new Property("identifier", "Identifier", 1268 "Used in supporting different identifiers for the same product, e.g. manufacturer code and retailer code.", 0, 1269 java.lang.Integer.MAX_VALUE, identifier)); 1270 children.add(new Property("type", "CodeableConcept", 1271 "The type of item - medication, device, service, protocol or other.", 0, 1, type)); 1272 children 1273 .add(new Property("orderable", "boolean", "Whether the entry represents an orderable item.", 0, 1, orderable)); 1274 children.add(new Property("referencedItem", 1275 "Reference(Medication|Device|Organization|Practitioner|PractitionerRole|HealthcareService|ActivityDefinition|PlanDefinition|SpecimenDefinition|ObservationDefinition|Binary)", 1276 "The item in a catalog or definition.", 0, 1, referencedItem)); 1277 children.add( 1278 new Property("additionalIdentifier", "Identifier", "Used in supporting related concepts, e.g. NDC to RxNorm.", 1279 0, java.lang.Integer.MAX_VALUE, additionalIdentifier)); 1280 children.add(new Property("classification", "CodeableConcept", "Classes of devices, or ATC for medication.", 0, 1281 java.lang.Integer.MAX_VALUE, classification)); 1282 children.add(new Property("status", "code", 1283 "Used to support catalog exchange even for unsupported products, e.g. getting list of medications even if not prescribable.", 1284 0, 1, status)); 1285 children.add(new Property("validityPeriod", "Period", 1286 "The time period in which this catalog entry is expected to be active.", 0, 1, validityPeriod)); 1287 children.add(new Property("validTo", "dateTime", 1288 "The date until which this catalog entry is expected to be active.", 0, 1, validTo)); 1289 children.add(new Property("lastUpdated", "dateTime", 1290 "Typically date of issue is different from the beginning of the validity. This can be used to see when an item was last updated.", 1291 0, 1, lastUpdated)); 1292 children.add(new Property("additionalCharacteristic", "CodeableConcept", 1293 "Used for examplefor Out of Formulary, or any specifics.", 0, java.lang.Integer.MAX_VALUE, 1294 additionalCharacteristic)); 1295 children.add(new Property("additionalClassification", "CodeableConcept", 1296 "User for example for ATC classification, or.", 0, java.lang.Integer.MAX_VALUE, additionalClassification)); 1297 children.add(new Property("relatedEntry", "", 1298 "Used for example, to point to a substance, or to a device used to administer a medication.", 0, 1299 java.lang.Integer.MAX_VALUE, relatedEntry)); 1300 } 1301 1302 @Override 1303 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1304 switch (_hash) { 1305 case -1618432855: 1306 /* identifier */ return new Property("identifier", "Identifier", 1307 "Used in supporting different identifiers for the same product, e.g. manufacturer code and retailer code.", 0, 1308 java.lang.Integer.MAX_VALUE, identifier); 1309 case 3575610: 1310 /* type */ return new Property("type", "CodeableConcept", 1311 "The type of item - medication, device, service, protocol or other.", 0, 1, type); 1312 case -391199320: 1313 /* orderable */ return new Property("orderable", "boolean", "Whether the entry represents an orderable item.", 0, 1314 1, orderable); 1315 case -1896630996: 1316 /* referencedItem */ return new Property("referencedItem", 1317 "Reference(Medication|Device|Organization|Practitioner|PractitionerRole|HealthcareService|ActivityDefinition|PlanDefinition|SpecimenDefinition|ObservationDefinition|Binary)", 1318 "The item in a catalog or definition.", 0, 1, referencedItem); 1319 case 1195162672: 1320 /* additionalIdentifier */ return new Property("additionalIdentifier", "Identifier", 1321 "Used in supporting related concepts, e.g. NDC to RxNorm.", 0, java.lang.Integer.MAX_VALUE, 1322 additionalIdentifier); 1323 case 382350310: 1324 /* classification */ return new Property("classification", "CodeableConcept", 1325 "Classes of devices, or ATC for medication.", 0, java.lang.Integer.MAX_VALUE, classification); 1326 case -892481550: 1327 /* status */ return new Property("status", "code", 1328 "Used to support catalog exchange even for unsupported products, e.g. getting list of medications even if not prescribable.", 1329 0, 1, status); 1330 case -1434195053: 1331 /* validityPeriod */ return new Property("validityPeriod", "Period", 1332 "The time period in which this catalog entry is expected to be active.", 0, 1, validityPeriod); 1333 case 231246743: 1334 /* validTo */ return new Property("validTo", "dateTime", 1335 "The date until which this catalog entry is expected to be active.", 0, 1, validTo); 1336 case 1649733957: 1337 /* lastUpdated */ return new Property("lastUpdated", "dateTime", 1338 "Typically date of issue is different from the beginning of the validity. This can be used to see when an item was last updated.", 1339 0, 1, lastUpdated); 1340 case -1638369886: 1341 /* additionalCharacteristic */ return new Property("additionalCharacteristic", "CodeableConcept", 1342 "Used for examplefor Out of Formulary, or any specifics.", 0, java.lang.Integer.MAX_VALUE, 1343 additionalCharacteristic); 1344 case -1622333459: 1345 /* additionalClassification */ return new Property("additionalClassification", "CodeableConcept", 1346 "User for example for ATC classification, or.", 0, java.lang.Integer.MAX_VALUE, additionalClassification); 1347 case 130178823: 1348 /* relatedEntry */ return new Property("relatedEntry", "", 1349 "Used for example, to point to a substance, or to a device used to administer a medication.", 0, 1350 java.lang.Integer.MAX_VALUE, relatedEntry); 1351 default: 1352 return super.getNamedProperty(_hash, _name, _checkValid); 1353 } 1354 1355 } 1356 1357 @Override 1358 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1359 switch (hash) { 1360 case -1618432855: 1361 /* identifier */ return this.identifier == null ? new Base[0] 1362 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1363 case 3575610: 1364 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1365 case -391199320: 1366 /* orderable */ return this.orderable == null ? new Base[0] : new Base[] { this.orderable }; // BooleanType 1367 case -1896630996: 1368 /* referencedItem */ return this.referencedItem == null ? new Base[0] : new Base[] { this.referencedItem }; // Reference 1369 case 1195162672: 1370 /* additionalIdentifier */ return this.additionalIdentifier == null ? new Base[0] 1371 : this.additionalIdentifier.toArray(new Base[this.additionalIdentifier.size()]); // Identifier 1372 case 382350310: 1373 /* classification */ return this.classification == null ? new Base[0] 1374 : this.classification.toArray(new Base[this.classification.size()]); // CodeableConcept 1375 case -892481550: 1376 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 1377 case -1434195053: 1378 /* validityPeriod */ return this.validityPeriod == null ? new Base[0] : new Base[] { this.validityPeriod }; // Period 1379 case 231246743: 1380 /* validTo */ return this.validTo == null ? new Base[0] : new Base[] { this.validTo }; // DateTimeType 1381 case 1649733957: 1382 /* lastUpdated */ return this.lastUpdated == null ? new Base[0] : new Base[] { this.lastUpdated }; // DateTimeType 1383 case -1638369886: 1384 /* additionalCharacteristic */ return this.additionalCharacteristic == null ? new Base[0] 1385 : this.additionalCharacteristic.toArray(new Base[this.additionalCharacteristic.size()]); // CodeableConcept 1386 case -1622333459: 1387 /* additionalClassification */ return this.additionalClassification == null ? new Base[0] 1388 : this.additionalClassification.toArray(new Base[this.additionalClassification.size()]); // CodeableConcept 1389 case 130178823: 1390 /* relatedEntry */ return this.relatedEntry == null ? new Base[0] 1391 : this.relatedEntry.toArray(new Base[this.relatedEntry.size()]); // CatalogEntryRelatedEntryComponent 1392 default: 1393 return super.getProperty(hash, name, checkValid); 1394 } 1395 1396 } 1397 1398 @Override 1399 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1400 switch (hash) { 1401 case -1618432855: // identifier 1402 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1403 return value; 1404 case 3575610: // type 1405 this.type = castToCodeableConcept(value); // CodeableConcept 1406 return value; 1407 case -391199320: // orderable 1408 this.orderable = castToBoolean(value); // BooleanType 1409 return value; 1410 case -1896630996: // referencedItem 1411 this.referencedItem = castToReference(value); // Reference 1412 return value; 1413 case 1195162672: // additionalIdentifier 1414 this.getAdditionalIdentifier().add(castToIdentifier(value)); // Identifier 1415 return value; 1416 case 382350310: // classification 1417 this.getClassification().add(castToCodeableConcept(value)); // CodeableConcept 1418 return value; 1419 case -892481550: // status 1420 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1421 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1422 return value; 1423 case -1434195053: // validityPeriod 1424 this.validityPeriod = castToPeriod(value); // Period 1425 return value; 1426 case 231246743: // validTo 1427 this.validTo = castToDateTime(value); // DateTimeType 1428 return value; 1429 case 1649733957: // lastUpdated 1430 this.lastUpdated = castToDateTime(value); // DateTimeType 1431 return value; 1432 case -1638369886: // additionalCharacteristic 1433 this.getAdditionalCharacteristic().add(castToCodeableConcept(value)); // CodeableConcept 1434 return value; 1435 case -1622333459: // additionalClassification 1436 this.getAdditionalClassification().add(castToCodeableConcept(value)); // CodeableConcept 1437 return value; 1438 case 130178823: // relatedEntry 1439 this.getRelatedEntry().add((CatalogEntryRelatedEntryComponent) value); // CatalogEntryRelatedEntryComponent 1440 return value; 1441 default: 1442 return super.setProperty(hash, name, value); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base setProperty(String name, Base value) throws FHIRException { 1449 if (name.equals("identifier")) { 1450 this.getIdentifier().add(castToIdentifier(value)); 1451 } else if (name.equals("type")) { 1452 this.type = castToCodeableConcept(value); // CodeableConcept 1453 } else if (name.equals("orderable")) { 1454 this.orderable = castToBoolean(value); // BooleanType 1455 } else if (name.equals("referencedItem")) { 1456 this.referencedItem = castToReference(value); // Reference 1457 } else if (name.equals("additionalIdentifier")) { 1458 this.getAdditionalIdentifier().add(castToIdentifier(value)); 1459 } else if (name.equals("classification")) { 1460 this.getClassification().add(castToCodeableConcept(value)); 1461 } else if (name.equals("status")) { 1462 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1463 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1464 } else if (name.equals("validityPeriod")) { 1465 this.validityPeriod = castToPeriod(value); // Period 1466 } else if (name.equals("validTo")) { 1467 this.validTo = castToDateTime(value); // DateTimeType 1468 } else if (name.equals("lastUpdated")) { 1469 this.lastUpdated = castToDateTime(value); // DateTimeType 1470 } else if (name.equals("additionalCharacteristic")) { 1471 this.getAdditionalCharacteristic().add(castToCodeableConcept(value)); 1472 } else if (name.equals("additionalClassification")) { 1473 this.getAdditionalClassification().add(castToCodeableConcept(value)); 1474 } else if (name.equals("relatedEntry")) { 1475 this.getRelatedEntry().add((CatalogEntryRelatedEntryComponent) value); 1476 } else 1477 return super.setProperty(name, value); 1478 return value; 1479 } 1480 1481 @Override 1482 public void removeChild(String name, Base value) throws FHIRException { 1483 if (name.equals("identifier")) { 1484 this.getIdentifier().remove(castToIdentifier(value)); 1485 } else if (name.equals("type")) { 1486 this.type = null; 1487 } else if (name.equals("orderable")) { 1488 this.orderable = null; 1489 } else if (name.equals("referencedItem")) { 1490 this.referencedItem = null; 1491 } else if (name.equals("additionalIdentifier")) { 1492 this.getAdditionalIdentifier().remove(castToIdentifier(value)); 1493 } else if (name.equals("classification")) { 1494 this.getClassification().remove(castToCodeableConcept(value)); 1495 } else if (name.equals("status")) { 1496 this.status = null; 1497 } else if (name.equals("validityPeriod")) { 1498 this.validityPeriod = null; 1499 } else if (name.equals("validTo")) { 1500 this.validTo = null; 1501 } else if (name.equals("lastUpdated")) { 1502 this.lastUpdated = null; 1503 } else if (name.equals("additionalCharacteristic")) { 1504 this.getAdditionalCharacteristic().remove(castToCodeableConcept(value)); 1505 } else if (name.equals("additionalClassification")) { 1506 this.getAdditionalClassification().remove(castToCodeableConcept(value)); 1507 } else if (name.equals("relatedEntry")) { 1508 this.getRelatedEntry().remove((CatalogEntryRelatedEntryComponent) value); 1509 } else 1510 super.removeChild(name, value); 1511 1512 } 1513 1514 @Override 1515 public Base makeProperty(int hash, String name) throws FHIRException { 1516 switch (hash) { 1517 case -1618432855: 1518 return addIdentifier(); 1519 case 3575610: 1520 return getType(); 1521 case -391199320: 1522 return getOrderableElement(); 1523 case -1896630996: 1524 return getReferencedItem(); 1525 case 1195162672: 1526 return addAdditionalIdentifier(); 1527 case 382350310: 1528 return addClassification(); 1529 case -892481550: 1530 return getStatusElement(); 1531 case -1434195053: 1532 return getValidityPeriod(); 1533 case 231246743: 1534 return getValidToElement(); 1535 case 1649733957: 1536 return getLastUpdatedElement(); 1537 case -1638369886: 1538 return addAdditionalCharacteristic(); 1539 case -1622333459: 1540 return addAdditionalClassification(); 1541 case 130178823: 1542 return addRelatedEntry(); 1543 default: 1544 return super.makeProperty(hash, name); 1545 } 1546 1547 } 1548 1549 @Override 1550 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1551 switch (hash) { 1552 case -1618432855: 1553 /* identifier */ return new String[] { "Identifier" }; 1554 case 3575610: 1555 /* type */ return new String[] { "CodeableConcept" }; 1556 case -391199320: 1557 /* orderable */ return new String[] { "boolean" }; 1558 case -1896630996: 1559 /* referencedItem */ return new String[] { "Reference" }; 1560 case 1195162672: 1561 /* additionalIdentifier */ return new String[] { "Identifier" }; 1562 case 382350310: 1563 /* classification */ return new String[] { "CodeableConcept" }; 1564 case -892481550: 1565 /* status */ return new String[] { "code" }; 1566 case -1434195053: 1567 /* validityPeriod */ return new String[] { "Period" }; 1568 case 231246743: 1569 /* validTo */ return new String[] { "dateTime" }; 1570 case 1649733957: 1571 /* lastUpdated */ return new String[] { "dateTime" }; 1572 case -1638369886: 1573 /* additionalCharacteristic */ return new String[] { "CodeableConcept" }; 1574 case -1622333459: 1575 /* additionalClassification */ return new String[] { "CodeableConcept" }; 1576 case 130178823: 1577 /* relatedEntry */ return new String[] {}; 1578 default: 1579 return super.getTypesForProperty(hash, name); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base addChild(String name) throws FHIRException { 1586 if (name.equals("identifier")) { 1587 return addIdentifier(); 1588 } else if (name.equals("type")) { 1589 this.type = new CodeableConcept(); 1590 return this.type; 1591 } else if (name.equals("orderable")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property CatalogEntry.orderable"); 1593 } else if (name.equals("referencedItem")) { 1594 this.referencedItem = new Reference(); 1595 return this.referencedItem; 1596 } else if (name.equals("additionalIdentifier")) { 1597 return addAdditionalIdentifier(); 1598 } else if (name.equals("classification")) { 1599 return addClassification(); 1600 } else if (name.equals("status")) { 1601 throw new FHIRException("Cannot call addChild on a singleton property CatalogEntry.status"); 1602 } else if (name.equals("validityPeriod")) { 1603 this.validityPeriod = new Period(); 1604 return this.validityPeriod; 1605 } else if (name.equals("validTo")) { 1606 throw new FHIRException("Cannot call addChild on a singleton property CatalogEntry.validTo"); 1607 } else if (name.equals("lastUpdated")) { 1608 throw new FHIRException("Cannot call addChild on a singleton property CatalogEntry.lastUpdated"); 1609 } else if (name.equals("additionalCharacteristic")) { 1610 return addAdditionalCharacteristic(); 1611 } else if (name.equals("additionalClassification")) { 1612 return addAdditionalClassification(); 1613 } else if (name.equals("relatedEntry")) { 1614 return addRelatedEntry(); 1615 } else 1616 return super.addChild(name); 1617 } 1618 1619 public String fhirType() { 1620 return "CatalogEntry"; 1621 1622 } 1623 1624 public CatalogEntry copy() { 1625 CatalogEntry dst = new CatalogEntry(); 1626 copyValues(dst); 1627 return dst; 1628 } 1629 1630 public void copyValues(CatalogEntry dst) { 1631 super.copyValues(dst); 1632 if (identifier != null) { 1633 dst.identifier = new ArrayList<Identifier>(); 1634 for (Identifier i : identifier) 1635 dst.identifier.add(i.copy()); 1636 } 1637 ; 1638 dst.type = type == null ? null : type.copy(); 1639 dst.orderable = orderable == null ? null : orderable.copy(); 1640 dst.referencedItem = referencedItem == null ? null : referencedItem.copy(); 1641 if (additionalIdentifier != null) { 1642 dst.additionalIdentifier = new ArrayList<Identifier>(); 1643 for (Identifier i : additionalIdentifier) 1644 dst.additionalIdentifier.add(i.copy()); 1645 } 1646 ; 1647 if (classification != null) { 1648 dst.classification = new ArrayList<CodeableConcept>(); 1649 for (CodeableConcept i : classification) 1650 dst.classification.add(i.copy()); 1651 } 1652 ; 1653 dst.status = status == null ? null : status.copy(); 1654 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1655 dst.validTo = validTo == null ? null : validTo.copy(); 1656 dst.lastUpdated = lastUpdated == null ? null : lastUpdated.copy(); 1657 if (additionalCharacteristic != null) { 1658 dst.additionalCharacteristic = new ArrayList<CodeableConcept>(); 1659 for (CodeableConcept i : additionalCharacteristic) 1660 dst.additionalCharacteristic.add(i.copy()); 1661 } 1662 ; 1663 if (additionalClassification != null) { 1664 dst.additionalClassification = new ArrayList<CodeableConcept>(); 1665 for (CodeableConcept i : additionalClassification) 1666 dst.additionalClassification.add(i.copy()); 1667 } 1668 ; 1669 if (relatedEntry != null) { 1670 dst.relatedEntry = new ArrayList<CatalogEntryRelatedEntryComponent>(); 1671 for (CatalogEntryRelatedEntryComponent i : relatedEntry) 1672 dst.relatedEntry.add(i.copy()); 1673 } 1674 ; 1675 } 1676 1677 protected CatalogEntry typedCopy() { 1678 return copy(); 1679 } 1680 1681 @Override 1682 public boolean equalsDeep(Base other_) { 1683 if (!super.equalsDeep(other_)) 1684 return false; 1685 if (!(other_ instanceof CatalogEntry)) 1686 return false; 1687 CatalogEntry o = (CatalogEntry) other_; 1688 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 1689 && compareDeep(orderable, o.orderable, true) && compareDeep(referencedItem, o.referencedItem, true) 1690 && compareDeep(additionalIdentifier, o.additionalIdentifier, true) 1691 && compareDeep(classification, o.classification, true) && compareDeep(status, o.status, true) 1692 && compareDeep(validityPeriod, o.validityPeriod, true) && compareDeep(validTo, o.validTo, true) 1693 && compareDeep(lastUpdated, o.lastUpdated, true) 1694 && compareDeep(additionalCharacteristic, o.additionalCharacteristic, true) 1695 && compareDeep(additionalClassification, o.additionalClassification, true) 1696 && compareDeep(relatedEntry, o.relatedEntry, true); 1697 } 1698 1699 @Override 1700 public boolean equalsShallow(Base other_) { 1701 if (!super.equalsShallow(other_)) 1702 return false; 1703 if (!(other_ instanceof CatalogEntry)) 1704 return false; 1705 CatalogEntry o = (CatalogEntry) other_; 1706 return compareValues(orderable, o.orderable, true) && compareValues(status, o.status, true) 1707 && compareValues(validTo, o.validTo, true) && compareValues(lastUpdated, o.lastUpdated, true); 1708 } 1709 1710 public boolean isEmpty() { 1711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, orderable, referencedItem, 1712 additionalIdentifier, classification, status, validityPeriod, validTo, lastUpdated, additionalCharacteristic, 1713 additionalClassification, relatedEntry); 1714 } 1715 1716 @Override 1717 public ResourceType getResourceType() { 1718 return ResourceType.CatalogEntry; 1719 } 1720 1721}