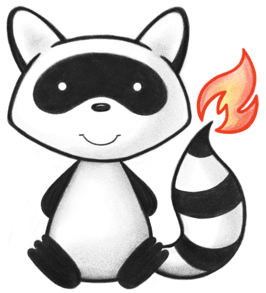
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * The resource ChargeItem describes the provision of healthcare provider 051 * products for a certain patient, therefore referring not only to the product, 052 * but containing in addition details of the provision, like date, time, amounts 053 * and participating organizations and persons. Main Usage of the ChargeItem is 054 * to enable the billing process and internal cost allocation. 055 */ 056@ResourceDef(name = "ChargeItem", profile = "http://hl7.org/fhir/StructureDefinition/ChargeItem") 057public class ChargeItem extends DomainResource { 058 059 public enum ChargeItemStatus { 060 /** 061 * The charge item has been entered, but the charged service is not yet 062 * complete, so it shall not be billed yet but might be used in the context of 063 * pre-authorization. 064 */ 065 PLANNED, 066 /** 067 * The charge item is ready for billing. 068 */ 069 BILLABLE, 070 /** 071 * The charge item has been determined to be not billable (e.g. due to rules 072 * associated with the billing code). 073 */ 074 NOTBILLABLE, 075 /** 076 * The processing of the charge was aborted. 077 */ 078 ABORTED, 079 /** 080 * The charge item has been billed (e.g. a billing engine has generated 081 * financial transactions by applying the associated ruled for the charge item 082 * to the context of the Encounter, and placed them into Claims/Invoices. 083 */ 084 BILLED, 085 /** 086 * The charge item has been entered in error and should not be processed for 087 * billing. 088 */ 089 ENTEREDINERROR, 090 /** 091 * The authoring system does not know which of the status values currently 092 * applies for this charge item Note: This concept is not to be used for "other" 093 * - one of the listed statuses is presumed to apply, it's just not known which 094 * one. 095 */ 096 UNKNOWN, 097 /** 098 * added to help the parsers with the generic types 099 */ 100 NULL; 101 102 public static ChargeItemStatus fromCode(String codeString) throws FHIRException { 103 if (codeString == null || "".equals(codeString)) 104 return null; 105 if ("planned".equals(codeString)) 106 return PLANNED; 107 if ("billable".equals(codeString)) 108 return BILLABLE; 109 if ("not-billable".equals(codeString)) 110 return NOTBILLABLE; 111 if ("aborted".equals(codeString)) 112 return ABORTED; 113 if ("billed".equals(codeString)) 114 return BILLED; 115 if ("entered-in-error".equals(codeString)) 116 return ENTEREDINERROR; 117 if ("unknown".equals(codeString)) 118 return UNKNOWN; 119 if (Configuration.isAcceptInvalidEnums()) 120 return null; 121 else 122 throw new FHIRException("Unknown ChargeItemStatus code '" + codeString + "'"); 123 } 124 125 public String toCode() { 126 switch (this) { 127 case PLANNED: 128 return "planned"; 129 case BILLABLE: 130 return "billable"; 131 case NOTBILLABLE: 132 return "not-billable"; 133 case ABORTED: 134 return "aborted"; 135 case BILLED: 136 return "billed"; 137 case ENTEREDINERROR: 138 return "entered-in-error"; 139 case UNKNOWN: 140 return "unknown"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getSystem() { 149 switch (this) { 150 case PLANNED: 151 return "http://hl7.org/fhir/chargeitem-status"; 152 case BILLABLE: 153 return "http://hl7.org/fhir/chargeitem-status"; 154 case NOTBILLABLE: 155 return "http://hl7.org/fhir/chargeitem-status"; 156 case ABORTED: 157 return "http://hl7.org/fhir/chargeitem-status"; 158 case BILLED: 159 return "http://hl7.org/fhir/chargeitem-status"; 160 case ENTEREDINERROR: 161 return "http://hl7.org/fhir/chargeitem-status"; 162 case UNKNOWN: 163 return "http://hl7.org/fhir/chargeitem-status"; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getDefinition() { 172 switch (this) { 173 case PLANNED: 174 return "The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization."; 175 case BILLABLE: 176 return "The charge item is ready for billing."; 177 case NOTBILLABLE: 178 return "The charge item has been determined to be not billable (e.g. due to rules associated with the billing code)."; 179 case ABORTED: 180 return "The processing of the charge was aborted."; 181 case BILLED: 182 return "The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices."; 183 case ENTEREDINERROR: 184 return "The charge item has been entered in error and should not be processed for billing."; 185 case UNKNOWN: 186 return "The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 187 case NULL: 188 return null; 189 default: 190 return "?"; 191 } 192 } 193 194 public String getDisplay() { 195 switch (this) { 196 case PLANNED: 197 return "Planned"; 198 case BILLABLE: 199 return "Billable"; 200 case NOTBILLABLE: 201 return "Not billable"; 202 case ABORTED: 203 return "Aborted"; 204 case BILLED: 205 return "Billed"; 206 case ENTEREDINERROR: 207 return "Entered in Error"; 208 case UNKNOWN: 209 return "Unknown"; 210 case NULL: 211 return null; 212 default: 213 return "?"; 214 } 215 } 216 } 217 218 public static class ChargeItemStatusEnumFactory implements EnumFactory<ChargeItemStatus> { 219 public ChargeItemStatus fromCode(String codeString) throws IllegalArgumentException { 220 if (codeString == null || "".equals(codeString)) 221 if (codeString == null || "".equals(codeString)) 222 return null; 223 if ("planned".equals(codeString)) 224 return ChargeItemStatus.PLANNED; 225 if ("billable".equals(codeString)) 226 return ChargeItemStatus.BILLABLE; 227 if ("not-billable".equals(codeString)) 228 return ChargeItemStatus.NOTBILLABLE; 229 if ("aborted".equals(codeString)) 230 return ChargeItemStatus.ABORTED; 231 if ("billed".equals(codeString)) 232 return ChargeItemStatus.BILLED; 233 if ("entered-in-error".equals(codeString)) 234 return ChargeItemStatus.ENTEREDINERROR; 235 if ("unknown".equals(codeString)) 236 return ChargeItemStatus.UNKNOWN; 237 throw new IllegalArgumentException("Unknown ChargeItemStatus code '" + codeString + "'"); 238 } 239 240 public Enumeration<ChargeItemStatus> fromType(PrimitiveType<?> code) throws FHIRException { 241 if (code == null) 242 return null; 243 if (code.isEmpty()) 244 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NULL, code); 245 String codeString = code.asStringValue(); 246 if (codeString == null || "".equals(codeString)) 247 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NULL, code); 248 if ("planned".equals(codeString)) 249 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.PLANNED, code); 250 if ("billable".equals(codeString)) 251 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLABLE, code); 252 if ("not-billable".equals(codeString)) 253 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NOTBILLABLE, code); 254 if ("aborted".equals(codeString)) 255 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ABORTED, code); 256 if ("billed".equals(codeString)) 257 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLED, code); 258 if ("entered-in-error".equals(codeString)) 259 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ENTEREDINERROR, code); 260 if ("unknown".equals(codeString)) 261 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.UNKNOWN, code); 262 throw new FHIRException("Unknown ChargeItemStatus code '" + codeString + "'"); 263 } 264 265 public String toCode(ChargeItemStatus code) { 266 if (code == ChargeItemStatus.PLANNED) 267 return "planned"; 268 if (code == ChargeItemStatus.BILLABLE) 269 return "billable"; 270 if (code == ChargeItemStatus.NOTBILLABLE) 271 return "not-billable"; 272 if (code == ChargeItemStatus.ABORTED) 273 return "aborted"; 274 if (code == ChargeItemStatus.BILLED) 275 return "billed"; 276 if (code == ChargeItemStatus.ENTEREDINERROR) 277 return "entered-in-error"; 278 if (code == ChargeItemStatus.UNKNOWN) 279 return "unknown"; 280 return "?"; 281 } 282 283 public String toSystem(ChargeItemStatus code) { 284 return code.getSystem(); 285 } 286 } 287 288 @Block() 289 public static class ChargeItemPerformerComponent extends BackboneElement implements IBaseBackboneElement { 290 /** 291 * Describes the type of performance or participation(e.g. primary surgeon, 292 * anesthesiologiest, etc.). 293 */ 294 @Child(name = "function", type = { 295 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 296 @Description(shortDefinition = "What type of performance was done", formalDefinition = "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).") 297 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/performer-role") 298 protected CodeableConcept function; 299 300 /** 301 * The device, practitioner, etc. who performed or participated in the service. 302 */ 303 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 304 Patient.class, Device.class, 305 RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 306 @Description(shortDefinition = "Individual who was performing", formalDefinition = "The device, practitioner, etc. who performed or participated in the service.") 307 protected Reference actor; 308 309 /** 310 * The actual object that is the target of the reference (The device, 311 * practitioner, etc. who performed or participated in the service.) 312 */ 313 protected Resource actorTarget; 314 315 private static final long serialVersionUID = 1424001049L; 316 317 /** 318 * Constructor 319 */ 320 public ChargeItemPerformerComponent() { 321 super(); 322 } 323 324 /** 325 * Constructor 326 */ 327 public ChargeItemPerformerComponent(Reference actor) { 328 super(); 329 this.actor = actor; 330 } 331 332 /** 333 * @return {@link #function} (Describes the type of performance or 334 * participation(e.g. primary surgeon, anesthesiologiest, etc.).) 335 */ 336 public CodeableConcept getFunction() { 337 if (this.function == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create ChargeItemPerformerComponent.function"); 340 else if (Configuration.doAutoCreate()) 341 this.function = new CodeableConcept(); // cc 342 return this.function; 343 } 344 345 public boolean hasFunction() { 346 return this.function != null && !this.function.isEmpty(); 347 } 348 349 /** 350 * @param value {@link #function} (Describes the type of performance or 351 * participation(e.g. primary surgeon, anesthesiologiest, etc.).) 352 */ 353 public ChargeItemPerformerComponent setFunction(CodeableConcept value) { 354 this.function = value; 355 return this; 356 } 357 358 /** 359 * @return {@link #actor} (The device, practitioner, etc. who performed or 360 * participated in the service.) 361 */ 362 public Reference getActor() { 363 if (this.actor == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create ChargeItemPerformerComponent.actor"); 366 else if (Configuration.doAutoCreate()) 367 this.actor = new Reference(); // cc 368 return this.actor; 369 } 370 371 public boolean hasActor() { 372 return this.actor != null && !this.actor.isEmpty(); 373 } 374 375 /** 376 * @param value {@link #actor} (The device, practitioner, etc. who performed or 377 * participated in the service.) 378 */ 379 public ChargeItemPerformerComponent setActor(Reference value) { 380 this.actor = value; 381 return this; 382 } 383 384 /** 385 * @return {@link #actor} The actual object that is the target of the reference. 386 * The reference library doesn't populate this, but you can use it to 387 * hold the resource if you resolve it. (The device, practitioner, etc. 388 * who performed or participated in the service.) 389 */ 390 public Resource getActorTarget() { 391 return this.actorTarget; 392 } 393 394 /** 395 * @param value {@link #actor} The actual object that is the target of the 396 * reference. The reference library doesn't use these, but you can 397 * use it to hold the resource if you resolve it. (The device, 398 * practitioner, etc. who performed or participated in the 399 * service.) 400 */ 401 public ChargeItemPerformerComponent setActorTarget(Resource value) { 402 this.actorTarget = value; 403 return this; 404 } 405 406 protected void listChildren(List<Property> children) { 407 super.listChildren(children); 408 children.add(new Property("function", "CodeableConcept", 409 "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).", 0, 1, 410 function)); 411 children.add(new Property("actor", 412 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 413 "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 414 } 415 416 @Override 417 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 418 switch (_hash) { 419 case 1380938712: 420 /* function */ return new Property("function", "CodeableConcept", 421 "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).", 0, 1, 422 function); 423 case 92645877: 424 /* actor */ return new Property("actor", 425 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 426 "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 427 default: 428 return super.getNamedProperty(_hash, _name, _checkValid); 429 } 430 431 } 432 433 @Override 434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 435 switch (hash) { 436 case 1380938712: 437 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 438 case 92645877: 439 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 440 default: 441 return super.getProperty(hash, name, checkValid); 442 } 443 444 } 445 446 @Override 447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 448 switch (hash) { 449 case 1380938712: // function 450 this.function = castToCodeableConcept(value); // CodeableConcept 451 return value; 452 case 92645877: // actor 453 this.actor = castToReference(value); // Reference 454 return value; 455 default: 456 return super.setProperty(hash, name, value); 457 } 458 459 } 460 461 @Override 462 public Base setProperty(String name, Base value) throws FHIRException { 463 if (name.equals("function")) { 464 this.function = castToCodeableConcept(value); // CodeableConcept 465 } else if (name.equals("actor")) { 466 this.actor = castToReference(value); // Reference 467 } else 468 return super.setProperty(name, value); 469 return value; 470 } 471 472 @Override 473 public void removeChild(String name, Base value) throws FHIRException { 474 if (name.equals("function")) { 475 this.function = null; 476 } else if (name.equals("actor")) { 477 this.actor = null; 478 } else 479 super.removeChild(name, value); 480 481 } 482 483 @Override 484 public Base makeProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case 1380938712: 487 return getFunction(); 488 case 92645877: 489 return getActor(); 490 default: 491 return super.makeProperty(hash, name); 492 } 493 494 } 495 496 @Override 497 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 498 switch (hash) { 499 case 1380938712: 500 /* function */ return new String[] { "CodeableConcept" }; 501 case 92645877: 502 /* actor */ return new String[] { "Reference" }; 503 default: 504 return super.getTypesForProperty(hash, name); 505 } 506 507 } 508 509 @Override 510 public Base addChild(String name) throws FHIRException { 511 if (name.equals("function")) { 512 this.function = new CodeableConcept(); 513 return this.function; 514 } else if (name.equals("actor")) { 515 this.actor = new Reference(); 516 return this.actor; 517 } else 518 return super.addChild(name); 519 } 520 521 public ChargeItemPerformerComponent copy() { 522 ChargeItemPerformerComponent dst = new ChargeItemPerformerComponent(); 523 copyValues(dst); 524 return dst; 525 } 526 527 public void copyValues(ChargeItemPerformerComponent dst) { 528 super.copyValues(dst); 529 dst.function = function == null ? null : function.copy(); 530 dst.actor = actor == null ? null : actor.copy(); 531 } 532 533 @Override 534 public boolean equalsDeep(Base other_) { 535 if (!super.equalsDeep(other_)) 536 return false; 537 if (!(other_ instanceof ChargeItemPerformerComponent)) 538 return false; 539 ChargeItemPerformerComponent o = (ChargeItemPerformerComponent) other_; 540 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 541 } 542 543 @Override 544 public boolean equalsShallow(Base other_) { 545 if (!super.equalsShallow(other_)) 546 return false; 547 if (!(other_ instanceof ChargeItemPerformerComponent)) 548 return false; 549 ChargeItemPerformerComponent o = (ChargeItemPerformerComponent) other_; 550 return true; 551 } 552 553 public boolean isEmpty() { 554 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 555 } 556 557 public String fhirType() { 558 return "ChargeItem.performer"; 559 560 } 561 562 } 563 564 /** 565 * Identifiers assigned to this event performer or other systems. 566 */ 567 @Child(name = "identifier", type = { 568 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 569 @Description(shortDefinition = "Business Identifier for item", formalDefinition = "Identifiers assigned to this event performer or other systems.") 570 protected List<Identifier> identifier; 571 572 /** 573 * References the (external) source of pricing information, rules of application 574 * for the code this ChargeItem uses. 575 */ 576 @Child(name = "definitionUri", type = { 577 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 578 @Description(shortDefinition = "Defining information about the code of this charge item", formalDefinition = "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.") 579 protected List<UriType> definitionUri; 580 581 /** 582 * References the source of pricing information, rules of application for the 583 * code this ChargeItem uses. 584 */ 585 @Child(name = "definitionCanonical", type = { 586 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 587 @Description(shortDefinition = "Resource defining the code of this ChargeItem", formalDefinition = "References the source of pricing information, rules of application for the code this ChargeItem uses.") 588 protected List<CanonicalType> definitionCanonical; 589 590 /** 591 * The current state of the ChargeItem. 592 */ 593 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 594 @Description(shortDefinition = "planned | billable | not-billable | aborted | billed | entered-in-error | unknown", formalDefinition = "The current state of the ChargeItem.") 595 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chargeitem-status") 596 protected Enumeration<ChargeItemStatus> status; 597 598 /** 599 * ChargeItems can be grouped to larger ChargeItems covering the whole set. 600 */ 601 @Child(name = "partOf", type = { 602 ChargeItem.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 603 @Description(shortDefinition = "Part of referenced ChargeItem", formalDefinition = "ChargeItems can be grouped to larger ChargeItems covering the whole set.") 604 protected List<Reference> partOf; 605 /** 606 * The actual objects that are the target of the reference (ChargeItems can be 607 * grouped to larger ChargeItems covering the whole set.) 608 */ 609 protected List<ChargeItem> partOfTarget; 610 611 /** 612 * A code that identifies the charge, like a billing code. 613 */ 614 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 615 @Description(shortDefinition = "A code that identifies the charge, like a billing code", formalDefinition = "A code that identifies the charge, like a billing code.") 616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 617 protected CodeableConcept code; 618 619 /** 620 * The individual or set of individuals the action is being or was performed on. 621 */ 622 @Child(name = "subject", type = { Patient.class, 623 Group.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 624 @Description(shortDefinition = "Individual service was done for/to", formalDefinition = "The individual or set of individuals the action is being or was performed on.") 625 protected Reference subject; 626 627 /** 628 * The actual object that is the target of the reference (The individual or set 629 * of individuals the action is being or was performed on.) 630 */ 631 protected Resource subjectTarget; 632 633 /** 634 * The encounter or episode of care that establishes the context for this event. 635 */ 636 @Child(name = "context", type = { Encounter.class, 637 EpisodeOfCare.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 638 @Description(shortDefinition = "Encounter / Episode associated with event", formalDefinition = "The encounter or episode of care that establishes the context for this event.") 639 protected Reference context; 640 641 /** 642 * The actual object that is the target of the reference (The encounter or 643 * episode of care that establishes the context for this event.) 644 */ 645 protected Resource contextTarget; 646 647 /** 648 * Date/time(s) or duration when the charged service was applied. 649 */ 650 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 651 Timing.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 652 @Description(shortDefinition = "When the charged service was applied", formalDefinition = "Date/time(s) or duration when the charged service was applied.") 653 protected Type occurrence; 654 655 /** 656 * Indicates who or what performed or participated in the charged service. 657 */ 658 @Child(name = "performer", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 659 @Description(shortDefinition = "Who performed charged service", formalDefinition = "Indicates who or what performed or participated in the charged service.") 660 protected List<ChargeItemPerformerComponent> performer; 661 662 /** 663 * The organization requesting the service. 664 */ 665 @Child(name = "performingOrganization", type = { 666 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 667 @Description(shortDefinition = "Organization providing the charged service", formalDefinition = "The organization requesting the service.") 668 protected Reference performingOrganization; 669 670 /** 671 * The actual object that is the target of the reference (The organization 672 * requesting the service.) 673 */ 674 protected Organization performingOrganizationTarget; 675 676 /** 677 * The organization performing the service. 678 */ 679 @Child(name = "requestingOrganization", type = { 680 Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 681 @Description(shortDefinition = "Organization requesting the charged service", formalDefinition = "The organization performing the service.") 682 protected Reference requestingOrganization; 683 684 /** 685 * The actual object that is the target of the reference (The organization 686 * performing the service.) 687 */ 688 protected Organization requestingOrganizationTarget; 689 690 /** 691 * The financial cost center permits the tracking of charge attribution. 692 */ 693 @Child(name = "costCenter", type = { 694 Organization.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 695 @Description(shortDefinition = "Organization that has ownership of the (potential, future) revenue", formalDefinition = "The financial cost center permits the tracking of charge attribution.") 696 protected Reference costCenter; 697 698 /** 699 * The actual object that is the target of the reference (The financial cost 700 * center permits the tracking of charge attribution.) 701 */ 702 protected Organization costCenterTarget; 703 704 /** 705 * Quantity of which the charge item has been serviced. 706 */ 707 @Child(name = "quantity", type = { Quantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 708 @Description(shortDefinition = "Quantity of which the charge item has been serviced", formalDefinition = "Quantity of which the charge item has been serviced.") 709 protected Quantity quantity; 710 711 /** 712 * The anatomical location where the related service has been applied. 713 */ 714 @Child(name = "bodysite", type = { 715 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 716 @Description(shortDefinition = "Anatomical location, if relevant", formalDefinition = "The anatomical location where the related service has been applied.") 717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 718 protected List<CodeableConcept> bodysite; 719 720 /** 721 * Factor overriding the factor determined by the rules associated with the 722 * code. 723 */ 724 @Child(name = "factorOverride", type = { 725 DecimalType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 726 @Description(shortDefinition = "Factor overriding the associated rules", formalDefinition = "Factor overriding the factor determined by the rules associated with the code.") 727 protected DecimalType factorOverride; 728 729 /** 730 * Total price of the charge overriding the list price associated with the code. 731 */ 732 @Child(name = "priceOverride", type = { 733 Money.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 734 @Description(shortDefinition = "Price overriding the associated rules", formalDefinition = "Total price of the charge overriding the list price associated with the code.") 735 protected Money priceOverride; 736 737 /** 738 * If the list price or the rule-based factor associated with the code is 739 * overridden, this attribute can capture a text to indicate the reason for this 740 * action. 741 */ 742 @Child(name = "overrideReason", type = { 743 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 744 @Description(shortDefinition = "Reason for overriding the list price/factor", formalDefinition = "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.") 745 protected StringType overrideReason; 746 747 /** 748 * The device, practitioner, etc. who entered the charge item. 749 */ 750 @Child(name = "enterer", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 751 Device.class, RelatedPerson.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 752 @Description(shortDefinition = "Individual who was entering", formalDefinition = "The device, practitioner, etc. who entered the charge item.") 753 protected Reference enterer; 754 755 /** 756 * The actual object that is the target of the reference (The device, 757 * practitioner, etc. who entered the charge item.) 758 */ 759 protected Resource entererTarget; 760 761 /** 762 * Date the charge item was entered. 763 */ 764 @Child(name = "enteredDate", type = { 765 DateTimeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 766 @Description(shortDefinition = "Date the charge item was entered", formalDefinition = "Date the charge item was entered.") 767 protected DateTimeType enteredDate; 768 769 /** 770 * Describes why the event occurred in coded or textual form. 771 */ 772 @Child(name = "reason", type = { 773 CodeableConcept.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 774 @Description(shortDefinition = "Why was the charged service rendered?", formalDefinition = "Describes why the event occurred in coded or textual form.") 775 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10") 776 protected List<CodeableConcept> reason; 777 778 /** 779 * Indicated the rendered service that caused this charge. 780 */ 781 @Child(name = "service", type = { DiagnosticReport.class, ImagingStudy.class, Immunization.class, 782 MedicationAdministration.class, MedicationDispense.class, Observation.class, Procedure.class, 783 SupplyDelivery.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 784 @Description(shortDefinition = "Which rendered service is being charged?", formalDefinition = "Indicated the rendered service that caused this charge.") 785 protected List<Reference> service; 786 /** 787 * The actual objects that are the target of the reference (Indicated the 788 * rendered service that caused this charge.) 789 */ 790 protected List<Resource> serviceTarget; 791 792 /** 793 * Identifies the device, food, drug or other product being charged either by 794 * type code or reference to an instance. 795 */ 796 @Child(name = "product", type = { Device.class, Medication.class, Substance.class, 797 CodeableConcept.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 798 @Description(shortDefinition = "Product charged", formalDefinition = "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.") 799 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-kind") 800 protected Type product; 801 802 /** 803 * Account into which this ChargeItems belongs. 804 */ 805 @Child(name = "account", type = { 806 Account.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 807 @Description(shortDefinition = "Account to place this charge", formalDefinition = "Account into which this ChargeItems belongs.") 808 protected List<Reference> account; 809 /** 810 * The actual objects that are the target of the reference (Account into which 811 * this ChargeItems belongs.) 812 */ 813 protected List<Account> accountTarget; 814 815 /** 816 * Comments made about the event by the performer, subject or other 817 * participants. 818 */ 819 @Child(name = "note", type = { 820 Annotation.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 821 @Description(shortDefinition = "Comments made about the ChargeItem", formalDefinition = "Comments made about the event by the performer, subject or other participants.") 822 protected List<Annotation> note; 823 824 /** 825 * Further information supporting this charge. 826 */ 827 @Child(name = "supportingInformation", type = { 828 Reference.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 829 @Description(shortDefinition = "Further information supporting this charge", formalDefinition = "Further information supporting this charge.") 830 protected List<Reference> supportingInformation; 831 /** 832 * The actual objects that are the target of the reference (Further information 833 * supporting this charge.) 834 */ 835 protected List<Resource> supportingInformationTarget; 836 837 private static final long serialVersionUID = 1748644267L; 838 839 /** 840 * Constructor 841 */ 842 public ChargeItem() { 843 super(); 844 } 845 846 /** 847 * Constructor 848 */ 849 public ChargeItem(Enumeration<ChargeItemStatus> status, CodeableConcept code, Reference subject) { 850 super(); 851 this.status = status; 852 this.code = code; 853 this.subject = subject; 854 } 855 856 /** 857 * @return {@link #identifier} (Identifiers assigned to this event performer or 858 * other systems.) 859 */ 860 public List<Identifier> getIdentifier() { 861 if (this.identifier == null) 862 this.identifier = new ArrayList<Identifier>(); 863 return this.identifier; 864 } 865 866 /** 867 * @return Returns a reference to <code>this</code> for easy method chaining 868 */ 869 public ChargeItem setIdentifier(List<Identifier> theIdentifier) { 870 this.identifier = theIdentifier; 871 return this; 872 } 873 874 public boolean hasIdentifier() { 875 if (this.identifier == null) 876 return false; 877 for (Identifier item : this.identifier) 878 if (!item.isEmpty()) 879 return true; 880 return false; 881 } 882 883 public Identifier addIdentifier() { // 3 884 Identifier t = new Identifier(); 885 if (this.identifier == null) 886 this.identifier = new ArrayList<Identifier>(); 887 this.identifier.add(t); 888 return t; 889 } 890 891 public ChargeItem addIdentifier(Identifier t) { // 3 892 if (t == null) 893 return this; 894 if (this.identifier == null) 895 this.identifier = new ArrayList<Identifier>(); 896 this.identifier.add(t); 897 return this; 898 } 899 900 /** 901 * @return The first repetition of repeating field {@link #identifier}, creating 902 * it if it does not already exist 903 */ 904 public Identifier getIdentifierFirstRep() { 905 if (getIdentifier().isEmpty()) { 906 addIdentifier(); 907 } 908 return getIdentifier().get(0); 909 } 910 911 /** 912 * @return {@link #definitionUri} (References the (external) source of pricing 913 * information, rules of application for the code this ChargeItem uses.) 914 */ 915 public List<UriType> getDefinitionUri() { 916 if (this.definitionUri == null) 917 this.definitionUri = new ArrayList<UriType>(); 918 return this.definitionUri; 919 } 920 921 /** 922 * @return Returns a reference to <code>this</code> for easy method chaining 923 */ 924 public ChargeItem setDefinitionUri(List<UriType> theDefinitionUri) { 925 this.definitionUri = theDefinitionUri; 926 return this; 927 } 928 929 public boolean hasDefinitionUri() { 930 if (this.definitionUri == null) 931 return false; 932 for (UriType item : this.definitionUri) 933 if (!item.isEmpty()) 934 return true; 935 return false; 936 } 937 938 /** 939 * @return {@link #definitionUri} (References the (external) source of pricing 940 * information, rules of application for the code this ChargeItem uses.) 941 */ 942 public UriType addDefinitionUriElement() {// 2 943 UriType t = new UriType(); 944 if (this.definitionUri == null) 945 this.definitionUri = new ArrayList<UriType>(); 946 this.definitionUri.add(t); 947 return t; 948 } 949 950 /** 951 * @param value {@link #definitionUri} (References the (external) source of 952 * pricing information, rules of application for the code this 953 * ChargeItem uses.) 954 */ 955 public ChargeItem addDefinitionUri(String value) { // 1 956 UriType t = new UriType(); 957 t.setValue(value); 958 if (this.definitionUri == null) 959 this.definitionUri = new ArrayList<UriType>(); 960 this.definitionUri.add(t); 961 return this; 962 } 963 964 /** 965 * @param value {@link #definitionUri} (References the (external) source of 966 * pricing information, rules of application for the code this 967 * ChargeItem uses.) 968 */ 969 public boolean hasDefinitionUri(String value) { 970 if (this.definitionUri == null) 971 return false; 972 for (UriType v : this.definitionUri) 973 if (v.getValue().equals(value)) // uri 974 return true; 975 return false; 976 } 977 978 /** 979 * @return {@link #definitionCanonical} (References the source of pricing 980 * information, rules of application for the code this ChargeItem uses.) 981 */ 982 public List<CanonicalType> getDefinitionCanonical() { 983 if (this.definitionCanonical == null) 984 this.definitionCanonical = new ArrayList<CanonicalType>(); 985 return this.definitionCanonical; 986 } 987 988 /** 989 * @return Returns a reference to <code>this</code> for easy method chaining 990 */ 991 public ChargeItem setDefinitionCanonical(List<CanonicalType> theDefinitionCanonical) { 992 this.definitionCanonical = theDefinitionCanonical; 993 return this; 994 } 995 996 public boolean hasDefinitionCanonical() { 997 if (this.definitionCanonical == null) 998 return false; 999 for (CanonicalType item : this.definitionCanonical) 1000 if (!item.isEmpty()) 1001 return true; 1002 return false; 1003 } 1004 1005 /** 1006 * @return {@link #definitionCanonical} (References the source of pricing 1007 * information, rules of application for the code this ChargeItem uses.) 1008 */ 1009 public CanonicalType addDefinitionCanonicalElement() {// 2 1010 CanonicalType t = new CanonicalType(); 1011 if (this.definitionCanonical == null) 1012 this.definitionCanonical = new ArrayList<CanonicalType>(); 1013 this.definitionCanonical.add(t); 1014 return t; 1015 } 1016 1017 /** 1018 * @param value {@link #definitionCanonical} (References the source of pricing 1019 * information, rules of application for the code this ChargeItem 1020 * uses.) 1021 */ 1022 public ChargeItem addDefinitionCanonical(String value) { // 1 1023 CanonicalType t = new CanonicalType(); 1024 t.setValue(value); 1025 if (this.definitionCanonical == null) 1026 this.definitionCanonical = new ArrayList<CanonicalType>(); 1027 this.definitionCanonical.add(t); 1028 return this; 1029 } 1030 1031 /** 1032 * @param value {@link #definitionCanonical} (References the source of pricing 1033 * information, rules of application for the code this ChargeItem 1034 * uses.) 1035 */ 1036 public boolean hasDefinitionCanonical(String value) { 1037 if (this.definitionCanonical == null) 1038 return false; 1039 for (CanonicalType v : this.definitionCanonical) 1040 if (v.getValue().equals(value)) // canonical(ChargeItemDefinition) 1041 return true; 1042 return false; 1043 } 1044 1045 /** 1046 * @return {@link #status} (The current state of the ChargeItem.). This is the 1047 * underlying object with id, value and extensions. The accessor 1048 * "getStatus" gives direct access to the value 1049 */ 1050 public Enumeration<ChargeItemStatus> getStatusElement() { 1051 if (this.status == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create ChargeItem.status"); 1054 else if (Configuration.doAutoCreate()) 1055 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); // bb 1056 return this.status; 1057 } 1058 1059 public boolean hasStatusElement() { 1060 return this.status != null && !this.status.isEmpty(); 1061 } 1062 1063 public boolean hasStatus() { 1064 return this.status != null && !this.status.isEmpty(); 1065 } 1066 1067 /** 1068 * @param value {@link #status} (The current state of the ChargeItem.). This is 1069 * the underlying object with id, value and extensions. The 1070 * accessor "getStatus" gives direct access to the value 1071 */ 1072 public ChargeItem setStatusElement(Enumeration<ChargeItemStatus> value) { 1073 this.status = value; 1074 return this; 1075 } 1076 1077 /** 1078 * @return The current state of the ChargeItem. 1079 */ 1080 public ChargeItemStatus getStatus() { 1081 return this.status == null ? null : this.status.getValue(); 1082 } 1083 1084 /** 1085 * @param value The current state of the ChargeItem. 1086 */ 1087 public ChargeItem setStatus(ChargeItemStatus value) { 1088 if (this.status == null) 1089 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); 1090 this.status.setValue(value); 1091 return this; 1092 } 1093 1094 /** 1095 * @return {@link #partOf} (ChargeItems can be grouped to larger ChargeItems 1096 * covering the whole set.) 1097 */ 1098 public List<Reference> getPartOf() { 1099 if (this.partOf == null) 1100 this.partOf = new ArrayList<Reference>(); 1101 return this.partOf; 1102 } 1103 1104 /** 1105 * @return Returns a reference to <code>this</code> for easy method chaining 1106 */ 1107 public ChargeItem setPartOf(List<Reference> thePartOf) { 1108 this.partOf = thePartOf; 1109 return this; 1110 } 1111 1112 public boolean hasPartOf() { 1113 if (this.partOf == null) 1114 return false; 1115 for (Reference item : this.partOf) 1116 if (!item.isEmpty()) 1117 return true; 1118 return false; 1119 } 1120 1121 public Reference addPartOf() { // 3 1122 Reference t = new Reference(); 1123 if (this.partOf == null) 1124 this.partOf = new ArrayList<Reference>(); 1125 this.partOf.add(t); 1126 return t; 1127 } 1128 1129 public ChargeItem addPartOf(Reference t) { // 3 1130 if (t == null) 1131 return this; 1132 if (this.partOf == null) 1133 this.partOf = new ArrayList<Reference>(); 1134 this.partOf.add(t); 1135 return this; 1136 } 1137 1138 /** 1139 * @return The first repetition of repeating field {@link #partOf}, creating it 1140 * if it does not already exist 1141 */ 1142 public Reference getPartOfFirstRep() { 1143 if (getPartOf().isEmpty()) { 1144 addPartOf(); 1145 } 1146 return getPartOf().get(0); 1147 } 1148 1149 /** 1150 * @deprecated Use Reference#setResource(IBaseResource) instead 1151 */ 1152 @Deprecated 1153 public List<ChargeItem> getPartOfTarget() { 1154 if (this.partOfTarget == null) 1155 this.partOfTarget = new ArrayList<ChargeItem>(); 1156 return this.partOfTarget; 1157 } 1158 1159 /** 1160 * @deprecated Use Reference#setResource(IBaseResource) instead 1161 */ 1162 @Deprecated 1163 public ChargeItem addPartOfTarget() { 1164 ChargeItem r = new ChargeItem(); 1165 if (this.partOfTarget == null) 1166 this.partOfTarget = new ArrayList<ChargeItem>(); 1167 this.partOfTarget.add(r); 1168 return r; 1169 } 1170 1171 /** 1172 * @return {@link #code} (A code that identifies the charge, like a billing 1173 * code.) 1174 */ 1175 public CodeableConcept getCode() { 1176 if (this.code == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create ChargeItem.code"); 1179 else if (Configuration.doAutoCreate()) 1180 this.code = new CodeableConcept(); // cc 1181 return this.code; 1182 } 1183 1184 public boolean hasCode() { 1185 return this.code != null && !this.code.isEmpty(); 1186 } 1187 1188 /** 1189 * @param value {@link #code} (A code that identifies the charge, like a billing 1190 * code.) 1191 */ 1192 public ChargeItem setCode(CodeableConcept value) { 1193 this.code = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return {@link #subject} (The individual or set of individuals the action is 1199 * being or was performed on.) 1200 */ 1201 public Reference getSubject() { 1202 if (this.subject == null) 1203 if (Configuration.errorOnAutoCreate()) 1204 throw new Error("Attempt to auto-create ChargeItem.subject"); 1205 else if (Configuration.doAutoCreate()) 1206 this.subject = new Reference(); // cc 1207 return this.subject; 1208 } 1209 1210 public boolean hasSubject() { 1211 return this.subject != null && !this.subject.isEmpty(); 1212 } 1213 1214 /** 1215 * @param value {@link #subject} (The individual or set of individuals the 1216 * action is being or was performed on.) 1217 */ 1218 public ChargeItem setSubject(Reference value) { 1219 this.subject = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return {@link #subject} The actual object that is the target of the 1225 * reference. The reference library doesn't populate this, but you can 1226 * use it to hold the resource if you resolve it. (The individual or set 1227 * of individuals the action is being or was performed on.) 1228 */ 1229 public Resource getSubjectTarget() { 1230 return this.subjectTarget; 1231 } 1232 1233 /** 1234 * @param value {@link #subject} The actual object that is the target of the 1235 * reference. The reference library doesn't use these, but you can 1236 * use it to hold the resource if you resolve it. (The individual 1237 * or set of individuals the action is being or was performed on.) 1238 */ 1239 public ChargeItem setSubjectTarget(Resource value) { 1240 this.subjectTarget = value; 1241 return this; 1242 } 1243 1244 /** 1245 * @return {@link #context} (The encounter or episode of care that establishes 1246 * the context for this event.) 1247 */ 1248 public Reference getContext() { 1249 if (this.context == null) 1250 if (Configuration.errorOnAutoCreate()) 1251 throw new Error("Attempt to auto-create ChargeItem.context"); 1252 else if (Configuration.doAutoCreate()) 1253 this.context = new Reference(); // cc 1254 return this.context; 1255 } 1256 1257 public boolean hasContext() { 1258 return this.context != null && !this.context.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #context} (The encounter or episode of care that 1263 * establishes the context for this event.) 1264 */ 1265 public ChargeItem setContext(Reference value) { 1266 this.context = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return {@link #context} The actual object that is the target of the 1272 * reference. The reference library doesn't populate this, but you can 1273 * use it to hold the resource if you resolve it. (The encounter or 1274 * episode of care that establishes the context for this event.) 1275 */ 1276 public Resource getContextTarget() { 1277 return this.contextTarget; 1278 } 1279 1280 /** 1281 * @param value {@link #context} The actual object that is the target of the 1282 * reference. The reference library doesn't use these, but you can 1283 * use it to hold the resource if you resolve it. (The encounter or 1284 * episode of care that establishes the context for this event.) 1285 */ 1286 public ChargeItem setContextTarget(Resource value) { 1287 this.contextTarget = value; 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1293 * service was applied.) 1294 */ 1295 public Type getOccurrence() { 1296 return this.occurrence; 1297 } 1298 1299 /** 1300 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1301 * service was applied.) 1302 */ 1303 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1304 if (this.occurrence == null) 1305 this.occurrence = new DateTimeType(); 1306 if (!(this.occurrence instanceof DateTimeType)) 1307 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1308 + this.occurrence.getClass().getName() + " was encountered"); 1309 return (DateTimeType) this.occurrence; 1310 } 1311 1312 public boolean hasOccurrenceDateTimeType() { 1313 return this != null && this.occurrence instanceof DateTimeType; 1314 } 1315 1316 /** 1317 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1318 * service was applied.) 1319 */ 1320 public Period getOccurrencePeriod() throws FHIRException { 1321 if (this.occurrence == null) 1322 this.occurrence = new Period(); 1323 if (!(this.occurrence instanceof Period)) 1324 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1325 + " was encountered"); 1326 return (Period) this.occurrence; 1327 } 1328 1329 public boolean hasOccurrencePeriod() { 1330 return this != null && this.occurrence instanceof Period; 1331 } 1332 1333 /** 1334 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1335 * service was applied.) 1336 */ 1337 public Timing getOccurrenceTiming() throws FHIRException { 1338 if (this.occurrence == null) 1339 this.occurrence = new Timing(); 1340 if (!(this.occurrence instanceof Timing)) 1341 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 1342 + " was encountered"); 1343 return (Timing) this.occurrence; 1344 } 1345 1346 public boolean hasOccurrenceTiming() { 1347 return this != null && this.occurrence instanceof Timing; 1348 } 1349 1350 public boolean hasOccurrence() { 1351 return this.occurrence != null && !this.occurrence.isEmpty(); 1352 } 1353 1354 /** 1355 * @param value {@link #occurrence} (Date/time(s) or duration when the charged 1356 * service was applied.) 1357 */ 1358 public ChargeItem setOccurrence(Type value) { 1359 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1360 throw new Error("Not the right type for ChargeItem.occurrence[x]: " + value.fhirType()); 1361 this.occurrence = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #performer} (Indicates who or what performed or participated 1367 * in the charged service.) 1368 */ 1369 public List<ChargeItemPerformerComponent> getPerformer() { 1370 if (this.performer == null) 1371 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1372 return this.performer; 1373 } 1374 1375 /** 1376 * @return Returns a reference to <code>this</code> for easy method chaining 1377 */ 1378 public ChargeItem setPerformer(List<ChargeItemPerformerComponent> thePerformer) { 1379 this.performer = thePerformer; 1380 return this; 1381 } 1382 1383 public boolean hasPerformer() { 1384 if (this.performer == null) 1385 return false; 1386 for (ChargeItemPerformerComponent item : this.performer) 1387 if (!item.isEmpty()) 1388 return true; 1389 return false; 1390 } 1391 1392 public ChargeItemPerformerComponent addPerformer() { // 3 1393 ChargeItemPerformerComponent t = new ChargeItemPerformerComponent(); 1394 if (this.performer == null) 1395 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1396 this.performer.add(t); 1397 return t; 1398 } 1399 1400 public ChargeItem addPerformer(ChargeItemPerformerComponent t) { // 3 1401 if (t == null) 1402 return this; 1403 if (this.performer == null) 1404 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1405 this.performer.add(t); 1406 return this; 1407 } 1408 1409 /** 1410 * @return The first repetition of repeating field {@link #performer}, creating 1411 * it if it does not already exist 1412 */ 1413 public ChargeItemPerformerComponent getPerformerFirstRep() { 1414 if (getPerformer().isEmpty()) { 1415 addPerformer(); 1416 } 1417 return getPerformer().get(0); 1418 } 1419 1420 /** 1421 * @return {@link #performingOrganization} (The organization requesting the 1422 * service.) 1423 */ 1424 public Reference getPerformingOrganization() { 1425 if (this.performingOrganization == null) 1426 if (Configuration.errorOnAutoCreate()) 1427 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1428 else if (Configuration.doAutoCreate()) 1429 this.performingOrganization = new Reference(); // cc 1430 return this.performingOrganization; 1431 } 1432 1433 public boolean hasPerformingOrganization() { 1434 return this.performingOrganization != null && !this.performingOrganization.isEmpty(); 1435 } 1436 1437 /** 1438 * @param value {@link #performingOrganization} (The organization requesting the 1439 * service.) 1440 */ 1441 public ChargeItem setPerformingOrganization(Reference value) { 1442 this.performingOrganization = value; 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #performingOrganization} The actual object that is the target 1448 * of the reference. The reference library doesn't populate this, but 1449 * you can use it to hold the resource if you resolve it. (The 1450 * organization requesting the service.) 1451 */ 1452 public Organization getPerformingOrganizationTarget() { 1453 if (this.performingOrganizationTarget == null) 1454 if (Configuration.errorOnAutoCreate()) 1455 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1456 else if (Configuration.doAutoCreate()) 1457 this.performingOrganizationTarget = new Organization(); // aa 1458 return this.performingOrganizationTarget; 1459 } 1460 1461 /** 1462 * @param value {@link #performingOrganization} The actual object that is the 1463 * target of the reference. The reference library doesn't use 1464 * these, but you can use it to hold the resource if you resolve 1465 * it. (The organization requesting the service.) 1466 */ 1467 public ChargeItem setPerformingOrganizationTarget(Organization value) { 1468 this.performingOrganizationTarget = value; 1469 return this; 1470 } 1471 1472 /** 1473 * @return {@link #requestingOrganization} (The organization performing the 1474 * service.) 1475 */ 1476 public Reference getRequestingOrganization() { 1477 if (this.requestingOrganization == null) 1478 if (Configuration.errorOnAutoCreate()) 1479 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1480 else if (Configuration.doAutoCreate()) 1481 this.requestingOrganization = new Reference(); // cc 1482 return this.requestingOrganization; 1483 } 1484 1485 public boolean hasRequestingOrganization() { 1486 return this.requestingOrganization != null && !this.requestingOrganization.isEmpty(); 1487 } 1488 1489 /** 1490 * @param value {@link #requestingOrganization} (The organization performing the 1491 * service.) 1492 */ 1493 public ChargeItem setRequestingOrganization(Reference value) { 1494 this.requestingOrganization = value; 1495 return this; 1496 } 1497 1498 /** 1499 * @return {@link #requestingOrganization} The actual object that is the target 1500 * of the reference. The reference library doesn't populate this, but 1501 * you can use it to hold the resource if you resolve it. (The 1502 * organization performing the service.) 1503 */ 1504 public Organization getRequestingOrganizationTarget() { 1505 if (this.requestingOrganizationTarget == null) 1506 if (Configuration.errorOnAutoCreate()) 1507 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1508 else if (Configuration.doAutoCreate()) 1509 this.requestingOrganizationTarget = new Organization(); // aa 1510 return this.requestingOrganizationTarget; 1511 } 1512 1513 /** 1514 * @param value {@link #requestingOrganization} The actual object that is the 1515 * target of the reference. The reference library doesn't use 1516 * these, but you can use it to hold the resource if you resolve 1517 * it. (The organization performing the service.) 1518 */ 1519 public ChargeItem setRequestingOrganizationTarget(Organization value) { 1520 this.requestingOrganizationTarget = value; 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #costCenter} (The financial cost center permits the tracking 1526 * of charge attribution.) 1527 */ 1528 public Reference getCostCenter() { 1529 if (this.costCenter == null) 1530 if (Configuration.errorOnAutoCreate()) 1531 throw new Error("Attempt to auto-create ChargeItem.costCenter"); 1532 else if (Configuration.doAutoCreate()) 1533 this.costCenter = new Reference(); // cc 1534 return this.costCenter; 1535 } 1536 1537 public boolean hasCostCenter() { 1538 return this.costCenter != null && !this.costCenter.isEmpty(); 1539 } 1540 1541 /** 1542 * @param value {@link #costCenter} (The financial cost center permits the 1543 * tracking of charge attribution.) 1544 */ 1545 public ChargeItem setCostCenter(Reference value) { 1546 this.costCenter = value; 1547 return this; 1548 } 1549 1550 /** 1551 * @return {@link #costCenter} The actual object that is the target of the 1552 * reference. The reference library doesn't populate this, but you can 1553 * use it to hold the resource if you resolve it. (The financial cost 1554 * center permits the tracking of charge attribution.) 1555 */ 1556 public Organization getCostCenterTarget() { 1557 if (this.costCenterTarget == null) 1558 if (Configuration.errorOnAutoCreate()) 1559 throw new Error("Attempt to auto-create ChargeItem.costCenter"); 1560 else if (Configuration.doAutoCreate()) 1561 this.costCenterTarget = new Organization(); // aa 1562 return this.costCenterTarget; 1563 } 1564 1565 /** 1566 * @param value {@link #costCenter} The actual object that is the target of the 1567 * reference. The reference library doesn't use these, but you can 1568 * use it to hold the resource if you resolve it. (The financial 1569 * cost center permits the tracking of charge attribution.) 1570 */ 1571 public ChargeItem setCostCenterTarget(Organization value) { 1572 this.costCenterTarget = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return {@link #quantity} (Quantity of which the charge item has been 1578 * serviced.) 1579 */ 1580 public Quantity getQuantity() { 1581 if (this.quantity == null) 1582 if (Configuration.errorOnAutoCreate()) 1583 throw new Error("Attempt to auto-create ChargeItem.quantity"); 1584 else if (Configuration.doAutoCreate()) 1585 this.quantity = new Quantity(); // cc 1586 return this.quantity; 1587 } 1588 1589 public boolean hasQuantity() { 1590 return this.quantity != null && !this.quantity.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #quantity} (Quantity of which the charge item has been 1595 * serviced.) 1596 */ 1597 public ChargeItem setQuantity(Quantity value) { 1598 this.quantity = value; 1599 return this; 1600 } 1601 1602 /** 1603 * @return {@link #bodysite} (The anatomical location where the related service 1604 * has been applied.) 1605 */ 1606 public List<CodeableConcept> getBodysite() { 1607 if (this.bodysite == null) 1608 this.bodysite = new ArrayList<CodeableConcept>(); 1609 return this.bodysite; 1610 } 1611 1612 /** 1613 * @return Returns a reference to <code>this</code> for easy method chaining 1614 */ 1615 public ChargeItem setBodysite(List<CodeableConcept> theBodysite) { 1616 this.bodysite = theBodysite; 1617 return this; 1618 } 1619 1620 public boolean hasBodysite() { 1621 if (this.bodysite == null) 1622 return false; 1623 for (CodeableConcept item : this.bodysite) 1624 if (!item.isEmpty()) 1625 return true; 1626 return false; 1627 } 1628 1629 public CodeableConcept addBodysite() { // 3 1630 CodeableConcept t = new CodeableConcept(); 1631 if (this.bodysite == null) 1632 this.bodysite = new ArrayList<CodeableConcept>(); 1633 this.bodysite.add(t); 1634 return t; 1635 } 1636 1637 public ChargeItem addBodysite(CodeableConcept t) { // 3 1638 if (t == null) 1639 return this; 1640 if (this.bodysite == null) 1641 this.bodysite = new ArrayList<CodeableConcept>(); 1642 this.bodysite.add(t); 1643 return this; 1644 } 1645 1646 /** 1647 * @return The first repetition of repeating field {@link #bodysite}, creating 1648 * it if it does not already exist 1649 */ 1650 public CodeableConcept getBodysiteFirstRep() { 1651 if (getBodysite().isEmpty()) { 1652 addBodysite(); 1653 } 1654 return getBodysite().get(0); 1655 } 1656 1657 /** 1658 * @return {@link #factorOverride} (Factor overriding the factor determined by 1659 * the rules associated with the code.). This is the underlying object 1660 * with id, value and extensions. The accessor "getFactorOverride" gives 1661 * direct access to the value 1662 */ 1663 public DecimalType getFactorOverrideElement() { 1664 if (this.factorOverride == null) 1665 if (Configuration.errorOnAutoCreate()) 1666 throw new Error("Attempt to auto-create ChargeItem.factorOverride"); 1667 else if (Configuration.doAutoCreate()) 1668 this.factorOverride = new DecimalType(); // bb 1669 return this.factorOverride; 1670 } 1671 1672 public boolean hasFactorOverrideElement() { 1673 return this.factorOverride != null && !this.factorOverride.isEmpty(); 1674 } 1675 1676 public boolean hasFactorOverride() { 1677 return this.factorOverride != null && !this.factorOverride.isEmpty(); 1678 } 1679 1680 /** 1681 * @param value {@link #factorOverride} (Factor overriding the factor determined 1682 * by the rules associated with the code.). This is the underlying 1683 * object with id, value and extensions. The accessor 1684 * "getFactorOverride" gives direct access to the value 1685 */ 1686 public ChargeItem setFactorOverrideElement(DecimalType value) { 1687 this.factorOverride = value; 1688 return this; 1689 } 1690 1691 /** 1692 * @return Factor overriding the factor determined by the rules associated with 1693 * the code. 1694 */ 1695 public BigDecimal getFactorOverride() { 1696 return this.factorOverride == null ? null : this.factorOverride.getValue(); 1697 } 1698 1699 /** 1700 * @param value Factor overriding the factor determined by the rules associated 1701 * with the code. 1702 */ 1703 public ChargeItem setFactorOverride(BigDecimal value) { 1704 if (value == null) 1705 this.factorOverride = null; 1706 else { 1707 if (this.factorOverride == null) 1708 this.factorOverride = new DecimalType(); 1709 this.factorOverride.setValue(value); 1710 } 1711 return this; 1712 } 1713 1714 /** 1715 * @param value Factor overriding the factor determined by the rules associated 1716 * with the code. 1717 */ 1718 public ChargeItem setFactorOverride(long value) { 1719 this.factorOverride = new DecimalType(); 1720 this.factorOverride.setValue(value); 1721 return this; 1722 } 1723 1724 /** 1725 * @param value Factor overriding the factor determined by the rules associated 1726 * with the code. 1727 */ 1728 public ChargeItem setFactorOverride(double value) { 1729 this.factorOverride = new DecimalType(); 1730 this.factorOverride.setValue(value); 1731 return this; 1732 } 1733 1734 /** 1735 * @return {@link #priceOverride} (Total price of the charge overriding the list 1736 * price associated with the code.) 1737 */ 1738 public Money getPriceOverride() { 1739 if (this.priceOverride == null) 1740 if (Configuration.errorOnAutoCreate()) 1741 throw new Error("Attempt to auto-create ChargeItem.priceOverride"); 1742 else if (Configuration.doAutoCreate()) 1743 this.priceOverride = new Money(); // cc 1744 return this.priceOverride; 1745 } 1746 1747 public boolean hasPriceOverride() { 1748 return this.priceOverride != null && !this.priceOverride.isEmpty(); 1749 } 1750 1751 /** 1752 * @param value {@link #priceOverride} (Total price of the charge overriding the 1753 * list price associated with the code.) 1754 */ 1755 public ChargeItem setPriceOverride(Money value) { 1756 this.priceOverride = value; 1757 return this; 1758 } 1759 1760 /** 1761 * @return {@link #overrideReason} (If the list price or the rule-based factor 1762 * associated with the code is overridden, this attribute can capture a 1763 * text to indicate the reason for this action.). This is the underlying 1764 * object with id, value and extensions. The accessor 1765 * "getOverrideReason" gives direct access to the value 1766 */ 1767 public StringType getOverrideReasonElement() { 1768 if (this.overrideReason == null) 1769 if (Configuration.errorOnAutoCreate()) 1770 throw new Error("Attempt to auto-create ChargeItem.overrideReason"); 1771 else if (Configuration.doAutoCreate()) 1772 this.overrideReason = new StringType(); // bb 1773 return this.overrideReason; 1774 } 1775 1776 public boolean hasOverrideReasonElement() { 1777 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1778 } 1779 1780 public boolean hasOverrideReason() { 1781 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1782 } 1783 1784 /** 1785 * @param value {@link #overrideReason} (If the list price or the rule-based 1786 * factor associated with the code is overridden, this attribute 1787 * can capture a text to indicate the reason for this action.). 1788 * This is the underlying object with id, value and extensions. The 1789 * accessor "getOverrideReason" gives direct access to the value 1790 */ 1791 public ChargeItem setOverrideReasonElement(StringType value) { 1792 this.overrideReason = value; 1793 return this; 1794 } 1795 1796 /** 1797 * @return If the list price or the rule-based factor associated with the code 1798 * is overridden, this attribute can capture a text to indicate the 1799 * reason for this action. 1800 */ 1801 public String getOverrideReason() { 1802 return this.overrideReason == null ? null : this.overrideReason.getValue(); 1803 } 1804 1805 /** 1806 * @param value If the list price or the rule-based factor associated with the 1807 * code is overridden, this attribute can capture a text to 1808 * indicate the reason for this action. 1809 */ 1810 public ChargeItem setOverrideReason(String value) { 1811 if (Utilities.noString(value)) 1812 this.overrideReason = null; 1813 else { 1814 if (this.overrideReason == null) 1815 this.overrideReason = new StringType(); 1816 this.overrideReason.setValue(value); 1817 } 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #enterer} (The device, practitioner, etc. who entered the 1823 * charge item.) 1824 */ 1825 public Reference getEnterer() { 1826 if (this.enterer == null) 1827 if (Configuration.errorOnAutoCreate()) 1828 throw new Error("Attempt to auto-create ChargeItem.enterer"); 1829 else if (Configuration.doAutoCreate()) 1830 this.enterer = new Reference(); // cc 1831 return this.enterer; 1832 } 1833 1834 public boolean hasEnterer() { 1835 return this.enterer != null && !this.enterer.isEmpty(); 1836 } 1837 1838 /** 1839 * @param value {@link #enterer} (The device, practitioner, etc. who entered the 1840 * charge item.) 1841 */ 1842 public ChargeItem setEnterer(Reference value) { 1843 this.enterer = value; 1844 return this; 1845 } 1846 1847 /** 1848 * @return {@link #enterer} The actual object that is the target of the 1849 * reference. The reference library doesn't populate this, but you can 1850 * use it to hold the resource if you resolve it. (The device, 1851 * practitioner, etc. who entered the charge item.) 1852 */ 1853 public Resource getEntererTarget() { 1854 return this.entererTarget; 1855 } 1856 1857 /** 1858 * @param value {@link #enterer} The actual object that is the target of the 1859 * reference. The reference library doesn't use these, but you can 1860 * use it to hold the resource if you resolve it. (The device, 1861 * practitioner, etc. who entered the charge item.) 1862 */ 1863 public ChargeItem setEntererTarget(Resource value) { 1864 this.entererTarget = value; 1865 return this; 1866 } 1867 1868 /** 1869 * @return {@link #enteredDate} (Date the charge item was entered.). This is the 1870 * underlying object with id, value and extensions. The accessor 1871 * "getEnteredDate" gives direct access to the value 1872 */ 1873 public DateTimeType getEnteredDateElement() { 1874 if (this.enteredDate == null) 1875 if (Configuration.errorOnAutoCreate()) 1876 throw new Error("Attempt to auto-create ChargeItem.enteredDate"); 1877 else if (Configuration.doAutoCreate()) 1878 this.enteredDate = new DateTimeType(); // bb 1879 return this.enteredDate; 1880 } 1881 1882 public boolean hasEnteredDateElement() { 1883 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1884 } 1885 1886 public boolean hasEnteredDate() { 1887 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1888 } 1889 1890 /** 1891 * @param value {@link #enteredDate} (Date the charge item was entered.). This 1892 * is the underlying object with id, value and extensions. The 1893 * accessor "getEnteredDate" gives direct access to the value 1894 */ 1895 public ChargeItem setEnteredDateElement(DateTimeType value) { 1896 this.enteredDate = value; 1897 return this; 1898 } 1899 1900 /** 1901 * @return Date the charge item was entered. 1902 */ 1903 public Date getEnteredDate() { 1904 return this.enteredDate == null ? null : this.enteredDate.getValue(); 1905 } 1906 1907 /** 1908 * @param value Date the charge item was entered. 1909 */ 1910 public ChargeItem setEnteredDate(Date value) { 1911 if (value == null) 1912 this.enteredDate = null; 1913 else { 1914 if (this.enteredDate == null) 1915 this.enteredDate = new DateTimeType(); 1916 this.enteredDate.setValue(value); 1917 } 1918 return this; 1919 } 1920 1921 /** 1922 * @return {@link #reason} (Describes why the event occurred in coded or textual 1923 * form.) 1924 */ 1925 public List<CodeableConcept> getReason() { 1926 if (this.reason == null) 1927 this.reason = new ArrayList<CodeableConcept>(); 1928 return this.reason; 1929 } 1930 1931 /** 1932 * @return Returns a reference to <code>this</code> for easy method chaining 1933 */ 1934 public ChargeItem setReason(List<CodeableConcept> theReason) { 1935 this.reason = theReason; 1936 return this; 1937 } 1938 1939 public boolean hasReason() { 1940 if (this.reason == null) 1941 return false; 1942 for (CodeableConcept item : this.reason) 1943 if (!item.isEmpty()) 1944 return true; 1945 return false; 1946 } 1947 1948 public CodeableConcept addReason() { // 3 1949 CodeableConcept t = new CodeableConcept(); 1950 if (this.reason == null) 1951 this.reason = new ArrayList<CodeableConcept>(); 1952 this.reason.add(t); 1953 return t; 1954 } 1955 1956 public ChargeItem addReason(CodeableConcept t) { // 3 1957 if (t == null) 1958 return this; 1959 if (this.reason == null) 1960 this.reason = new ArrayList<CodeableConcept>(); 1961 this.reason.add(t); 1962 return this; 1963 } 1964 1965 /** 1966 * @return The first repetition of repeating field {@link #reason}, creating it 1967 * if it does not already exist 1968 */ 1969 public CodeableConcept getReasonFirstRep() { 1970 if (getReason().isEmpty()) { 1971 addReason(); 1972 } 1973 return getReason().get(0); 1974 } 1975 1976 /** 1977 * @return {@link #service} (Indicated the rendered service that caused this 1978 * charge.) 1979 */ 1980 public List<Reference> getService() { 1981 if (this.service == null) 1982 this.service = new ArrayList<Reference>(); 1983 return this.service; 1984 } 1985 1986 /** 1987 * @return Returns a reference to <code>this</code> for easy method chaining 1988 */ 1989 public ChargeItem setService(List<Reference> theService) { 1990 this.service = theService; 1991 return this; 1992 } 1993 1994 public boolean hasService() { 1995 if (this.service == null) 1996 return false; 1997 for (Reference item : this.service) 1998 if (!item.isEmpty()) 1999 return true; 2000 return false; 2001 } 2002 2003 public Reference addService() { // 3 2004 Reference t = new Reference(); 2005 if (this.service == null) 2006 this.service = new ArrayList<Reference>(); 2007 this.service.add(t); 2008 return t; 2009 } 2010 2011 public ChargeItem addService(Reference t) { // 3 2012 if (t == null) 2013 return this; 2014 if (this.service == null) 2015 this.service = new ArrayList<Reference>(); 2016 this.service.add(t); 2017 return this; 2018 } 2019 2020 /** 2021 * @return The first repetition of repeating field {@link #service}, creating it 2022 * if it does not already exist 2023 */ 2024 public Reference getServiceFirstRep() { 2025 if (getService().isEmpty()) { 2026 addService(); 2027 } 2028 return getService().get(0); 2029 } 2030 2031 /** 2032 * @deprecated Use Reference#setResource(IBaseResource) instead 2033 */ 2034 @Deprecated 2035 public List<Resource> getServiceTarget() { 2036 if (this.serviceTarget == null) 2037 this.serviceTarget = new ArrayList<Resource>(); 2038 return this.serviceTarget; 2039 } 2040 2041 /** 2042 * @return {@link #product} (Identifies the device, food, drug or other product 2043 * being charged either by type code or reference to an instance.) 2044 */ 2045 public Type getProduct() { 2046 return this.product; 2047 } 2048 2049 /** 2050 * @return {@link #product} (Identifies the device, food, drug or other product 2051 * being charged either by type code or reference to an instance.) 2052 */ 2053 public Reference getProductReference() throws FHIRException { 2054 if (this.product == null) 2055 this.product = new Reference(); 2056 if (!(this.product instanceof Reference)) 2057 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.product.getClass().getName() 2058 + " was encountered"); 2059 return (Reference) this.product; 2060 } 2061 2062 public boolean hasProductReference() { 2063 return this != null && this.product instanceof Reference; 2064 } 2065 2066 /** 2067 * @return {@link #product} (Identifies the device, food, drug or other product 2068 * being charged either by type code or reference to an instance.) 2069 */ 2070 public CodeableConcept getProductCodeableConcept() throws FHIRException { 2071 if (this.product == null) 2072 this.product = new CodeableConcept(); 2073 if (!(this.product instanceof CodeableConcept)) 2074 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2075 + this.product.getClass().getName() + " was encountered"); 2076 return (CodeableConcept) this.product; 2077 } 2078 2079 public boolean hasProductCodeableConcept() { 2080 return this != null && this.product instanceof CodeableConcept; 2081 } 2082 2083 public boolean hasProduct() { 2084 return this.product != null && !this.product.isEmpty(); 2085 } 2086 2087 /** 2088 * @param value {@link #product} (Identifies the device, food, drug or other 2089 * product being charged either by type code or reference to an 2090 * instance.) 2091 */ 2092 public ChargeItem setProduct(Type value) { 2093 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 2094 throw new Error("Not the right type for ChargeItem.product[x]: " + value.fhirType()); 2095 this.product = value; 2096 return this; 2097 } 2098 2099 /** 2100 * @return {@link #account} (Account into which this ChargeItems belongs.) 2101 */ 2102 public List<Reference> getAccount() { 2103 if (this.account == null) 2104 this.account = new ArrayList<Reference>(); 2105 return this.account; 2106 } 2107 2108 /** 2109 * @return Returns a reference to <code>this</code> for easy method chaining 2110 */ 2111 public ChargeItem setAccount(List<Reference> theAccount) { 2112 this.account = theAccount; 2113 return this; 2114 } 2115 2116 public boolean hasAccount() { 2117 if (this.account == null) 2118 return false; 2119 for (Reference item : this.account) 2120 if (!item.isEmpty()) 2121 return true; 2122 return false; 2123 } 2124 2125 public Reference addAccount() { // 3 2126 Reference t = new Reference(); 2127 if (this.account == null) 2128 this.account = new ArrayList<Reference>(); 2129 this.account.add(t); 2130 return t; 2131 } 2132 2133 public ChargeItem addAccount(Reference t) { // 3 2134 if (t == null) 2135 return this; 2136 if (this.account == null) 2137 this.account = new ArrayList<Reference>(); 2138 this.account.add(t); 2139 return this; 2140 } 2141 2142 /** 2143 * @return The first repetition of repeating field {@link #account}, creating it 2144 * if it does not already exist 2145 */ 2146 public Reference getAccountFirstRep() { 2147 if (getAccount().isEmpty()) { 2148 addAccount(); 2149 } 2150 return getAccount().get(0); 2151 } 2152 2153 /** 2154 * @deprecated Use Reference#setResource(IBaseResource) instead 2155 */ 2156 @Deprecated 2157 public List<Account> getAccountTarget() { 2158 if (this.accountTarget == null) 2159 this.accountTarget = new ArrayList<Account>(); 2160 return this.accountTarget; 2161 } 2162 2163 /** 2164 * @deprecated Use Reference#setResource(IBaseResource) instead 2165 */ 2166 @Deprecated 2167 public Account addAccountTarget() { 2168 Account r = new Account(); 2169 if (this.accountTarget == null) 2170 this.accountTarget = new ArrayList<Account>(); 2171 this.accountTarget.add(r); 2172 return r; 2173 } 2174 2175 /** 2176 * @return {@link #note} (Comments made about the event by the performer, 2177 * subject or other participants.) 2178 */ 2179 public List<Annotation> getNote() { 2180 if (this.note == null) 2181 this.note = new ArrayList<Annotation>(); 2182 return this.note; 2183 } 2184 2185 /** 2186 * @return Returns a reference to <code>this</code> for easy method chaining 2187 */ 2188 public ChargeItem setNote(List<Annotation> theNote) { 2189 this.note = theNote; 2190 return this; 2191 } 2192 2193 public boolean hasNote() { 2194 if (this.note == null) 2195 return false; 2196 for (Annotation item : this.note) 2197 if (!item.isEmpty()) 2198 return true; 2199 return false; 2200 } 2201 2202 public Annotation addNote() { // 3 2203 Annotation t = new Annotation(); 2204 if (this.note == null) 2205 this.note = new ArrayList<Annotation>(); 2206 this.note.add(t); 2207 return t; 2208 } 2209 2210 public ChargeItem addNote(Annotation t) { // 3 2211 if (t == null) 2212 return this; 2213 if (this.note == null) 2214 this.note = new ArrayList<Annotation>(); 2215 this.note.add(t); 2216 return this; 2217 } 2218 2219 /** 2220 * @return The first repetition of repeating field {@link #note}, creating it if 2221 * it does not already exist 2222 */ 2223 public Annotation getNoteFirstRep() { 2224 if (getNote().isEmpty()) { 2225 addNote(); 2226 } 2227 return getNote().get(0); 2228 } 2229 2230 /** 2231 * @return {@link #supportingInformation} (Further information supporting this 2232 * charge.) 2233 */ 2234 public List<Reference> getSupportingInformation() { 2235 if (this.supportingInformation == null) 2236 this.supportingInformation = new ArrayList<Reference>(); 2237 return this.supportingInformation; 2238 } 2239 2240 /** 2241 * @return Returns a reference to <code>this</code> for easy method chaining 2242 */ 2243 public ChargeItem setSupportingInformation(List<Reference> theSupportingInformation) { 2244 this.supportingInformation = theSupportingInformation; 2245 return this; 2246 } 2247 2248 public boolean hasSupportingInformation() { 2249 if (this.supportingInformation == null) 2250 return false; 2251 for (Reference item : this.supportingInformation) 2252 if (!item.isEmpty()) 2253 return true; 2254 return false; 2255 } 2256 2257 public Reference addSupportingInformation() { // 3 2258 Reference t = new Reference(); 2259 if (this.supportingInformation == null) 2260 this.supportingInformation = new ArrayList<Reference>(); 2261 this.supportingInformation.add(t); 2262 return t; 2263 } 2264 2265 public ChargeItem addSupportingInformation(Reference t) { // 3 2266 if (t == null) 2267 return this; 2268 if (this.supportingInformation == null) 2269 this.supportingInformation = new ArrayList<Reference>(); 2270 this.supportingInformation.add(t); 2271 return this; 2272 } 2273 2274 /** 2275 * @return The first repetition of repeating field 2276 * {@link #supportingInformation}, creating it if it does not already 2277 * exist 2278 */ 2279 public Reference getSupportingInformationFirstRep() { 2280 if (getSupportingInformation().isEmpty()) { 2281 addSupportingInformation(); 2282 } 2283 return getSupportingInformation().get(0); 2284 } 2285 2286 /** 2287 * @deprecated Use Reference#setResource(IBaseResource) instead 2288 */ 2289 @Deprecated 2290 public List<Resource> getSupportingInformationTarget() { 2291 if (this.supportingInformationTarget == null) 2292 this.supportingInformationTarget = new ArrayList<Resource>(); 2293 return this.supportingInformationTarget; 2294 } 2295 2296 protected void listChildren(List<Property> children) { 2297 super.listChildren(children); 2298 children.add(new Property("identifier", "Identifier", 2299 "Identifiers assigned to this event performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2300 children.add(new Property("definitionUri", "uri", 2301 "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.", 2302 0, java.lang.Integer.MAX_VALUE, definitionUri)); 2303 children.add(new Property("definitionCanonical", "canonical(ChargeItemDefinition)", 2304 "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, 2305 java.lang.Integer.MAX_VALUE, definitionCanonical)); 2306 children.add(new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status)); 2307 children.add(new Property("partOf", "Reference(ChargeItem)", 2308 "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, 2309 partOf)); 2310 children.add( 2311 new Property("code", "CodeableConcept", "A code that identifies the charge, like a billing code.", 0, 1, code)); 2312 children.add(new Property("subject", "Reference(Patient|Group)", 2313 "The individual or set of individuals the action is being or was performed on.", 0, 1, subject)); 2314 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 2315 "The encounter or episode of care that establishes the context for this event.", 0, 1, context)); 2316 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", 2317 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence)); 2318 children 2319 .add(new Property("performer", "", "Indicates who or what performed or participated in the charged service.", 0, 2320 java.lang.Integer.MAX_VALUE, performer)); 2321 children.add(new Property("performingOrganization", "Reference(Organization)", 2322 "The organization requesting the service.", 0, 1, performingOrganization)); 2323 children.add(new Property("requestingOrganization", "Reference(Organization)", 2324 "The organization performing the service.", 0, 1, requestingOrganization)); 2325 children.add(new Property("costCenter", "Reference(Organization)", 2326 "The financial cost center permits the tracking of charge attribution.", 0, 1, costCenter)); 2327 children.add( 2328 new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 0, 1, quantity)); 2329 children.add(new Property("bodysite", "CodeableConcept", 2330 "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, 2331 bodysite)); 2332 children.add(new Property("factorOverride", "decimal", 2333 "Factor overriding the factor determined by the rules associated with the code.", 0, 1, factorOverride)); 2334 children.add(new Property("priceOverride", "Money", 2335 "Total price of the charge overriding the list price associated with the code.", 0, 1, priceOverride)); 2336 children.add(new Property("overrideReason", "string", 2337 "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 2338 0, 1, overrideReason)); 2339 children.add( 2340 new Property("enterer", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", 2341 "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer)); 2342 children.add(new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, enteredDate)); 2343 children.add(new Property("reason", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 2344 0, java.lang.Integer.MAX_VALUE, reason)); 2345 children.add(new Property("service", 2346 "Reference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|Observation|Procedure|SupplyDelivery)", 2347 "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service)); 2348 children.add(new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2349 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2350 0, 1, product)); 2351 children.add(new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 0, 2352 java.lang.Integer.MAX_VALUE, account)); 2353 children.add(new Property("note", "Annotation", 2354 "Comments made about the event by the performer, subject or other participants.", 0, 2355 java.lang.Integer.MAX_VALUE, note)); 2356 children.add(new Property("supportingInformation", "Reference(Any)", "Further information supporting this charge.", 2357 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2358 } 2359 2360 @Override 2361 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2362 switch (_hash) { 2363 case -1618432855: 2364 /* identifier */ return new Property("identifier", "Identifier", 2365 "Identifiers assigned to this event performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 2366 case -1139428583: 2367 /* definitionUri */ return new Property("definitionUri", "uri", 2368 "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.", 2369 0, java.lang.Integer.MAX_VALUE, definitionUri); 2370 case 933485793: 2371 /* definitionCanonical */ return new Property("definitionCanonical", "canonical(ChargeItemDefinition)", 2372 "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, 2373 java.lang.Integer.MAX_VALUE, definitionCanonical); 2374 case -892481550: 2375 /* status */ return new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status); 2376 case -995410646: 2377 /* partOf */ return new Property("partOf", "Reference(ChargeItem)", 2378 "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, 2379 partOf); 2380 case 3059181: 2381 /* code */ return new Property("code", "CodeableConcept", 2382 "A code that identifies the charge, like a billing code.", 0, 1, code); 2383 case -1867885268: 2384 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2385 "The individual or set of individuals the action is being or was performed on.", 0, 1, subject); 2386 case 951530927: 2387 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 2388 "The encounter or episode of care that establishes the context for this event.", 0, 1, context); 2389 case -2022646513: 2390 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2391 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2392 case 1687874001: 2393 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2394 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2395 case -298443636: 2396 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2397 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2398 case 1397156594: 2399 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2400 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2401 case 1515218299: 2402 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2403 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2404 case 481140686: 2405 /* performer */ return new Property("performer", "", 2406 "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, 2407 performer); 2408 case 1273192628: 2409 /* performingOrganization */ return new Property("performingOrganization", "Reference(Organization)", 2410 "The organization requesting the service.", 0, 1, performingOrganization); 2411 case 1279054790: 2412 /* requestingOrganization */ return new Property("requestingOrganization", "Reference(Organization)", 2413 "The organization performing the service.", 0, 1, requestingOrganization); 2414 case -593192318: 2415 /* costCenter */ return new Property("costCenter", "Reference(Organization)", 2416 "The financial cost center permits the tracking of charge attribution.", 0, 1, costCenter); 2417 case -1285004149: 2418 /* quantity */ return new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 2419 0, 1, quantity); 2420 case 1703573481: 2421 /* bodysite */ return new Property("bodysite", "CodeableConcept", 2422 "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, 2423 bodysite); 2424 case -451233221: 2425 /* factorOverride */ return new Property("factorOverride", "decimal", 2426 "Factor overriding the factor determined by the rules associated with the code.", 0, 1, factorOverride); 2427 case -216803275: 2428 /* priceOverride */ return new Property("priceOverride", "Money", 2429 "Total price of the charge overriding the list price associated with the code.", 0, 1, priceOverride); 2430 case -742878928: 2431 /* overrideReason */ return new Property("overrideReason", "string", 2432 "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 2433 0, 1, overrideReason); 2434 case -1591951995: 2435 /* enterer */ return new Property("enterer", 2436 "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", 2437 "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer); 2438 case 555978181: 2439 /* enteredDate */ return new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, 2440 enteredDate); 2441 case -934964668: 2442 /* reason */ return new Property("reason", "CodeableConcept", 2443 "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason); 2444 case 1984153269: 2445 /* service */ return new Property("service", 2446 "Reference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|Observation|Procedure|SupplyDelivery)", 2447 "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service); 2448 case 1753005361: 2449 /* product[x] */ return new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2450 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2451 0, 1, product); 2452 case -309474065: 2453 /* product */ return new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2454 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2455 0, 1, product); 2456 case -669667556: 2457 /* productReference */ return new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2458 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2459 0, 1, product); 2460 case 906854066: 2461 /* productCodeableConcept */ return new Property("product[x]", 2462 "Reference(Device|Medication|Substance)|CodeableConcept", 2463 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2464 0, 1, product); 2465 case -1177318867: 2466 /* account */ return new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 2467 0, java.lang.Integer.MAX_VALUE, account); 2468 case 3387378: 2469 /* note */ return new Property("note", "Annotation", 2470 "Comments made about the event by the performer, subject or other participants.", 0, 2471 java.lang.Integer.MAX_VALUE, note); 2472 case -1248768647: 2473 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2474 "Further information supporting this charge.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2475 default: 2476 return super.getNamedProperty(_hash, _name, _checkValid); 2477 } 2478 2479 } 2480 2481 @Override 2482 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2483 switch (hash) { 2484 case -1618432855: 2485 /* identifier */ return this.identifier == null ? new Base[0] 2486 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2487 case -1139428583: 2488 /* definitionUri */ return this.definitionUri == null ? new Base[0] 2489 : this.definitionUri.toArray(new Base[this.definitionUri.size()]); // UriType 2490 case 933485793: 2491 /* definitionCanonical */ return this.definitionCanonical == null ? new Base[0] 2492 : this.definitionCanonical.toArray(new Base[this.definitionCanonical.size()]); // CanonicalType 2493 case -892481550: 2494 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ChargeItemStatus> 2495 case -995410646: 2496 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2497 case 3059181: 2498 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2499 case -1867885268: 2500 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2501 case 951530927: 2502 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 2503 case 1687874001: 2504 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2505 case 481140686: 2506 /* performer */ return this.performer == null ? new Base[0] 2507 : this.performer.toArray(new Base[this.performer.size()]); // ChargeItemPerformerComponent 2508 case 1273192628: 2509 /* performingOrganization */ return this.performingOrganization == null ? new Base[0] 2510 : new Base[] { this.performingOrganization }; // Reference 2511 case 1279054790: 2512 /* requestingOrganization */ return this.requestingOrganization == null ? new Base[0] 2513 : new Base[] { this.requestingOrganization }; // Reference 2514 case -593192318: 2515 /* costCenter */ return this.costCenter == null ? new Base[0] : new Base[] { this.costCenter }; // Reference 2516 case -1285004149: 2517 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 2518 case 1703573481: 2519 /* bodysite */ return this.bodysite == null ? new Base[0] : this.bodysite.toArray(new Base[this.bodysite.size()]); // CodeableConcept 2520 case -451233221: 2521 /* factorOverride */ return this.factorOverride == null ? new Base[0] : new Base[] { this.factorOverride }; // DecimalType 2522 case -216803275: 2523 /* priceOverride */ return this.priceOverride == null ? new Base[0] : new Base[] { this.priceOverride }; // Money 2524 case -742878928: 2525 /* overrideReason */ return this.overrideReason == null ? new Base[0] : new Base[] { this.overrideReason }; // StringType 2526 case -1591951995: 2527 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 2528 case 555978181: 2529 /* enteredDate */ return this.enteredDate == null ? new Base[0] : new Base[] { this.enteredDate }; // DateTimeType 2530 case -934964668: 2531 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 2532 case 1984153269: 2533 /* service */ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // Reference 2534 case -309474065: 2535 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // Type 2536 case -1177318867: 2537 /* account */ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 2538 case 3387378: 2539 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2540 case -1248768647: 2541 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2542 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2543 default: 2544 return super.getProperty(hash, name, checkValid); 2545 } 2546 2547 } 2548 2549 @Override 2550 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2551 switch (hash) { 2552 case -1618432855: // identifier 2553 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2554 return value; 2555 case -1139428583: // definitionUri 2556 this.getDefinitionUri().add(castToUri(value)); // UriType 2557 return value; 2558 case 933485793: // definitionCanonical 2559 this.getDefinitionCanonical().add(castToCanonical(value)); // CanonicalType 2560 return value; 2561 case -892481550: // status 2562 value = new ChargeItemStatusEnumFactory().fromType(castToCode(value)); 2563 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 2564 return value; 2565 case -995410646: // partOf 2566 this.getPartOf().add(castToReference(value)); // Reference 2567 return value; 2568 case 3059181: // code 2569 this.code = castToCodeableConcept(value); // CodeableConcept 2570 return value; 2571 case -1867885268: // subject 2572 this.subject = castToReference(value); // Reference 2573 return value; 2574 case 951530927: // context 2575 this.context = castToReference(value); // Reference 2576 return value; 2577 case 1687874001: // occurrence 2578 this.occurrence = castToType(value); // Type 2579 return value; 2580 case 481140686: // performer 2581 this.getPerformer().add((ChargeItemPerformerComponent) value); // ChargeItemPerformerComponent 2582 return value; 2583 case 1273192628: // performingOrganization 2584 this.performingOrganization = castToReference(value); // Reference 2585 return value; 2586 case 1279054790: // requestingOrganization 2587 this.requestingOrganization = castToReference(value); // Reference 2588 return value; 2589 case -593192318: // costCenter 2590 this.costCenter = castToReference(value); // Reference 2591 return value; 2592 case -1285004149: // quantity 2593 this.quantity = castToQuantity(value); // Quantity 2594 return value; 2595 case 1703573481: // bodysite 2596 this.getBodysite().add(castToCodeableConcept(value)); // CodeableConcept 2597 return value; 2598 case -451233221: // factorOverride 2599 this.factorOverride = castToDecimal(value); // DecimalType 2600 return value; 2601 case -216803275: // priceOverride 2602 this.priceOverride = castToMoney(value); // Money 2603 return value; 2604 case -742878928: // overrideReason 2605 this.overrideReason = castToString(value); // StringType 2606 return value; 2607 case -1591951995: // enterer 2608 this.enterer = castToReference(value); // Reference 2609 return value; 2610 case 555978181: // enteredDate 2611 this.enteredDate = castToDateTime(value); // DateTimeType 2612 return value; 2613 case -934964668: // reason 2614 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 2615 return value; 2616 case 1984153269: // service 2617 this.getService().add(castToReference(value)); // Reference 2618 return value; 2619 case -309474065: // product 2620 this.product = castToType(value); // Type 2621 return value; 2622 case -1177318867: // account 2623 this.getAccount().add(castToReference(value)); // Reference 2624 return value; 2625 case 3387378: // note 2626 this.getNote().add(castToAnnotation(value)); // Annotation 2627 return value; 2628 case -1248768647: // supportingInformation 2629 this.getSupportingInformation().add(castToReference(value)); // Reference 2630 return value; 2631 default: 2632 return super.setProperty(hash, name, value); 2633 } 2634 2635 } 2636 2637 @Override 2638 public Base setProperty(String name, Base value) throws FHIRException { 2639 if (name.equals("identifier")) { 2640 this.getIdentifier().add(castToIdentifier(value)); 2641 } else if (name.equals("definitionUri")) { 2642 this.getDefinitionUri().add(castToUri(value)); 2643 } else if (name.equals("definitionCanonical")) { 2644 this.getDefinitionCanonical().add(castToCanonical(value)); 2645 } else if (name.equals("status")) { 2646 value = new ChargeItemStatusEnumFactory().fromType(castToCode(value)); 2647 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 2648 } else if (name.equals("partOf")) { 2649 this.getPartOf().add(castToReference(value)); 2650 } else if (name.equals("code")) { 2651 this.code = castToCodeableConcept(value); // CodeableConcept 2652 } else if (name.equals("subject")) { 2653 this.subject = castToReference(value); // Reference 2654 } else if (name.equals("context")) { 2655 this.context = castToReference(value); // Reference 2656 } else if (name.equals("occurrence[x]")) { 2657 this.occurrence = castToType(value); // Type 2658 } else if (name.equals("performer")) { 2659 this.getPerformer().add((ChargeItemPerformerComponent) value); 2660 } else if (name.equals("performingOrganization")) { 2661 this.performingOrganization = castToReference(value); // Reference 2662 } else if (name.equals("requestingOrganization")) { 2663 this.requestingOrganization = castToReference(value); // Reference 2664 } else if (name.equals("costCenter")) { 2665 this.costCenter = castToReference(value); // Reference 2666 } else if (name.equals("quantity")) { 2667 this.quantity = castToQuantity(value); // Quantity 2668 } else if (name.equals("bodysite")) { 2669 this.getBodysite().add(castToCodeableConcept(value)); 2670 } else if (name.equals("factorOverride")) { 2671 this.factorOverride = castToDecimal(value); // DecimalType 2672 } else if (name.equals("priceOverride")) { 2673 this.priceOverride = castToMoney(value); // Money 2674 } else if (name.equals("overrideReason")) { 2675 this.overrideReason = castToString(value); // StringType 2676 } else if (name.equals("enterer")) { 2677 this.enterer = castToReference(value); // Reference 2678 } else if (name.equals("enteredDate")) { 2679 this.enteredDate = castToDateTime(value); // DateTimeType 2680 } else if (name.equals("reason")) { 2681 this.getReason().add(castToCodeableConcept(value)); 2682 } else if (name.equals("service")) { 2683 this.getService().add(castToReference(value)); 2684 } else if (name.equals("product[x]")) { 2685 this.product = castToType(value); // Type 2686 } else if (name.equals("account")) { 2687 this.getAccount().add(castToReference(value)); 2688 } else if (name.equals("note")) { 2689 this.getNote().add(castToAnnotation(value)); 2690 } else if (name.equals("supportingInformation")) { 2691 this.getSupportingInformation().add(castToReference(value)); 2692 } else 2693 return super.setProperty(name, value); 2694 return value; 2695 } 2696 2697 @Override 2698 public void removeChild(String name, Base value) throws FHIRException { 2699 if (name.equals("identifier")) { 2700 this.getIdentifier().remove(castToIdentifier(value)); 2701 } else if (name.equals("definitionUri")) { 2702 this.getDefinitionUri().remove(castToUri(value)); 2703 } else if (name.equals("definitionCanonical")) { 2704 this.getDefinitionCanonical().remove(castToCanonical(value)); 2705 } else if (name.equals("status")) { 2706 this.status = null; 2707 } else if (name.equals("partOf")) { 2708 this.getPartOf().remove(castToReference(value)); 2709 } else if (name.equals("code")) { 2710 this.code = null; 2711 } else if (name.equals("subject")) { 2712 this.subject = null; 2713 } else if (name.equals("context")) { 2714 this.context = null; 2715 } else if (name.equals("occurrence[x]")) { 2716 this.occurrence = null; 2717 } else if (name.equals("performer")) { 2718 this.getPerformer().remove((ChargeItemPerformerComponent) value); 2719 } else if (name.equals("performingOrganization")) { 2720 this.performingOrganization = null; 2721 } else if (name.equals("requestingOrganization")) { 2722 this.requestingOrganization = null; 2723 } else if (name.equals("costCenter")) { 2724 this.costCenter = null; 2725 } else if (name.equals("quantity")) { 2726 this.quantity = null; 2727 } else if (name.equals("bodysite")) { 2728 this.getBodysite().remove(castToCodeableConcept(value)); 2729 } else if (name.equals("factorOverride")) { 2730 this.factorOverride = null; 2731 } else if (name.equals("priceOverride")) { 2732 this.priceOverride = null; 2733 } else if (name.equals("overrideReason")) { 2734 this.overrideReason = null; 2735 } else if (name.equals("enterer")) { 2736 this.enterer = null; 2737 } else if (name.equals("enteredDate")) { 2738 this.enteredDate = null; 2739 } else if (name.equals("reason")) { 2740 this.getReason().remove(castToCodeableConcept(value)); 2741 } else if (name.equals("service")) { 2742 this.getService().remove(castToReference(value)); 2743 } else if (name.equals("product[x]")) { 2744 this.product = null; 2745 } else if (name.equals("account")) { 2746 this.getAccount().remove(castToReference(value)); 2747 } else if (name.equals("note")) { 2748 this.getNote().remove(castToAnnotation(value)); 2749 } else if (name.equals("supportingInformation")) { 2750 this.getSupportingInformation().remove(castToReference(value)); 2751 } else 2752 super.removeChild(name, value); 2753 2754 } 2755 2756 @Override 2757 public Base makeProperty(int hash, String name) throws FHIRException { 2758 switch (hash) { 2759 case -1618432855: 2760 return addIdentifier(); 2761 case -1139428583: 2762 return addDefinitionUriElement(); 2763 case 933485793: 2764 return addDefinitionCanonicalElement(); 2765 case -892481550: 2766 return getStatusElement(); 2767 case -995410646: 2768 return addPartOf(); 2769 case 3059181: 2770 return getCode(); 2771 case -1867885268: 2772 return getSubject(); 2773 case 951530927: 2774 return getContext(); 2775 case -2022646513: 2776 return getOccurrence(); 2777 case 1687874001: 2778 return getOccurrence(); 2779 case 481140686: 2780 return addPerformer(); 2781 case 1273192628: 2782 return getPerformingOrganization(); 2783 case 1279054790: 2784 return getRequestingOrganization(); 2785 case -593192318: 2786 return getCostCenter(); 2787 case -1285004149: 2788 return getQuantity(); 2789 case 1703573481: 2790 return addBodysite(); 2791 case -451233221: 2792 return getFactorOverrideElement(); 2793 case -216803275: 2794 return getPriceOverride(); 2795 case -742878928: 2796 return getOverrideReasonElement(); 2797 case -1591951995: 2798 return getEnterer(); 2799 case 555978181: 2800 return getEnteredDateElement(); 2801 case -934964668: 2802 return addReason(); 2803 case 1984153269: 2804 return addService(); 2805 case 1753005361: 2806 return getProduct(); 2807 case -309474065: 2808 return getProduct(); 2809 case -1177318867: 2810 return addAccount(); 2811 case 3387378: 2812 return addNote(); 2813 case -1248768647: 2814 return addSupportingInformation(); 2815 default: 2816 return super.makeProperty(hash, name); 2817 } 2818 2819 } 2820 2821 @Override 2822 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2823 switch (hash) { 2824 case -1618432855: 2825 /* identifier */ return new String[] { "Identifier" }; 2826 case -1139428583: 2827 /* definitionUri */ return new String[] { "uri" }; 2828 case 933485793: 2829 /* definitionCanonical */ return new String[] { "canonical" }; 2830 case -892481550: 2831 /* status */ return new String[] { "code" }; 2832 case -995410646: 2833 /* partOf */ return new String[] { "Reference" }; 2834 case 3059181: 2835 /* code */ return new String[] { "CodeableConcept" }; 2836 case -1867885268: 2837 /* subject */ return new String[] { "Reference" }; 2838 case 951530927: 2839 /* context */ return new String[] { "Reference" }; 2840 case 1687874001: 2841 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 2842 case 481140686: 2843 /* performer */ return new String[] {}; 2844 case 1273192628: 2845 /* performingOrganization */ return new String[] { "Reference" }; 2846 case 1279054790: 2847 /* requestingOrganization */ return new String[] { "Reference" }; 2848 case -593192318: 2849 /* costCenter */ return new String[] { "Reference" }; 2850 case -1285004149: 2851 /* quantity */ return new String[] { "Quantity" }; 2852 case 1703573481: 2853 /* bodysite */ return new String[] { "CodeableConcept" }; 2854 case -451233221: 2855 /* factorOverride */ return new String[] { "decimal" }; 2856 case -216803275: 2857 /* priceOverride */ return new String[] { "Money" }; 2858 case -742878928: 2859 /* overrideReason */ return new String[] { "string" }; 2860 case -1591951995: 2861 /* enterer */ return new String[] { "Reference" }; 2862 case 555978181: 2863 /* enteredDate */ return new String[] { "dateTime" }; 2864 case -934964668: 2865 /* reason */ return new String[] { "CodeableConcept" }; 2866 case 1984153269: 2867 /* service */ return new String[] { "Reference" }; 2868 case -309474065: 2869 /* product */ return new String[] { "Reference", "CodeableConcept" }; 2870 case -1177318867: 2871 /* account */ return new String[] { "Reference" }; 2872 case 3387378: 2873 /* note */ return new String[] { "Annotation" }; 2874 case -1248768647: 2875 /* supportingInformation */ return new String[] { "Reference" }; 2876 default: 2877 return super.getTypesForProperty(hash, name); 2878 } 2879 2880 } 2881 2882 @Override 2883 public Base addChild(String name) throws FHIRException { 2884 if (name.equals("identifier")) { 2885 return addIdentifier(); 2886 } else if (name.equals("definitionUri")) { 2887 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definitionUri"); 2888 } else if (name.equals("definitionCanonical")) { 2889 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definitionCanonical"); 2890 } else if (name.equals("status")) { 2891 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.status"); 2892 } else if (name.equals("partOf")) { 2893 return addPartOf(); 2894 } else if (name.equals("code")) { 2895 this.code = new CodeableConcept(); 2896 return this.code; 2897 } else if (name.equals("subject")) { 2898 this.subject = new Reference(); 2899 return this.subject; 2900 } else if (name.equals("context")) { 2901 this.context = new Reference(); 2902 return this.context; 2903 } else if (name.equals("occurrenceDateTime")) { 2904 this.occurrence = new DateTimeType(); 2905 return this.occurrence; 2906 } else if (name.equals("occurrencePeriod")) { 2907 this.occurrence = new Period(); 2908 return this.occurrence; 2909 } else if (name.equals("occurrenceTiming")) { 2910 this.occurrence = new Timing(); 2911 return this.occurrence; 2912 } else if (name.equals("performer")) { 2913 return addPerformer(); 2914 } else if (name.equals("performingOrganization")) { 2915 this.performingOrganization = new Reference(); 2916 return this.performingOrganization; 2917 } else if (name.equals("requestingOrganization")) { 2918 this.requestingOrganization = new Reference(); 2919 return this.requestingOrganization; 2920 } else if (name.equals("costCenter")) { 2921 this.costCenter = new Reference(); 2922 return this.costCenter; 2923 } else if (name.equals("quantity")) { 2924 this.quantity = new Quantity(); 2925 return this.quantity; 2926 } else if (name.equals("bodysite")) { 2927 return addBodysite(); 2928 } else if (name.equals("factorOverride")) { 2929 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.factorOverride"); 2930 } else if (name.equals("priceOverride")) { 2931 this.priceOverride = new Money(); 2932 return this.priceOverride; 2933 } else if (name.equals("overrideReason")) { 2934 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.overrideReason"); 2935 } else if (name.equals("enterer")) { 2936 this.enterer = new Reference(); 2937 return this.enterer; 2938 } else if (name.equals("enteredDate")) { 2939 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.enteredDate"); 2940 } else if (name.equals("reason")) { 2941 return addReason(); 2942 } else if (name.equals("service")) { 2943 return addService(); 2944 } else if (name.equals("productReference")) { 2945 this.product = new Reference(); 2946 return this.product; 2947 } else if (name.equals("productCodeableConcept")) { 2948 this.product = new CodeableConcept(); 2949 return this.product; 2950 } else if (name.equals("account")) { 2951 return addAccount(); 2952 } else if (name.equals("note")) { 2953 return addNote(); 2954 } else if (name.equals("supportingInformation")) { 2955 return addSupportingInformation(); 2956 } else 2957 return super.addChild(name); 2958 } 2959 2960 public String fhirType() { 2961 return "ChargeItem"; 2962 2963 } 2964 2965 public ChargeItem copy() { 2966 ChargeItem dst = new ChargeItem(); 2967 copyValues(dst); 2968 return dst; 2969 } 2970 2971 public void copyValues(ChargeItem dst) { 2972 super.copyValues(dst); 2973 if (identifier != null) { 2974 dst.identifier = new ArrayList<Identifier>(); 2975 for (Identifier i : identifier) 2976 dst.identifier.add(i.copy()); 2977 } 2978 ; 2979 if (definitionUri != null) { 2980 dst.definitionUri = new ArrayList<UriType>(); 2981 for (UriType i : definitionUri) 2982 dst.definitionUri.add(i.copy()); 2983 } 2984 ; 2985 if (definitionCanonical != null) { 2986 dst.definitionCanonical = new ArrayList<CanonicalType>(); 2987 for (CanonicalType i : definitionCanonical) 2988 dst.definitionCanonical.add(i.copy()); 2989 } 2990 ; 2991 dst.status = status == null ? null : status.copy(); 2992 if (partOf != null) { 2993 dst.partOf = new ArrayList<Reference>(); 2994 for (Reference i : partOf) 2995 dst.partOf.add(i.copy()); 2996 } 2997 ; 2998 dst.code = code == null ? null : code.copy(); 2999 dst.subject = subject == null ? null : subject.copy(); 3000 dst.context = context == null ? null : context.copy(); 3001 dst.occurrence = occurrence == null ? null : occurrence.copy(); 3002 if (performer != null) { 3003 dst.performer = new ArrayList<ChargeItemPerformerComponent>(); 3004 for (ChargeItemPerformerComponent i : performer) 3005 dst.performer.add(i.copy()); 3006 } 3007 ; 3008 dst.performingOrganization = performingOrganization == null ? null : performingOrganization.copy(); 3009 dst.requestingOrganization = requestingOrganization == null ? null : requestingOrganization.copy(); 3010 dst.costCenter = costCenter == null ? null : costCenter.copy(); 3011 dst.quantity = quantity == null ? null : quantity.copy(); 3012 if (bodysite != null) { 3013 dst.bodysite = new ArrayList<CodeableConcept>(); 3014 for (CodeableConcept i : bodysite) 3015 dst.bodysite.add(i.copy()); 3016 } 3017 ; 3018 dst.factorOverride = factorOverride == null ? null : factorOverride.copy(); 3019 dst.priceOverride = priceOverride == null ? null : priceOverride.copy(); 3020 dst.overrideReason = overrideReason == null ? null : overrideReason.copy(); 3021 dst.enterer = enterer == null ? null : enterer.copy(); 3022 dst.enteredDate = enteredDate == null ? null : enteredDate.copy(); 3023 if (reason != null) { 3024 dst.reason = new ArrayList<CodeableConcept>(); 3025 for (CodeableConcept i : reason) 3026 dst.reason.add(i.copy()); 3027 } 3028 ; 3029 if (service != null) { 3030 dst.service = new ArrayList<Reference>(); 3031 for (Reference i : service) 3032 dst.service.add(i.copy()); 3033 } 3034 ; 3035 dst.product = product == null ? null : product.copy(); 3036 if (account != null) { 3037 dst.account = new ArrayList<Reference>(); 3038 for (Reference i : account) 3039 dst.account.add(i.copy()); 3040 } 3041 ; 3042 if (note != null) { 3043 dst.note = new ArrayList<Annotation>(); 3044 for (Annotation i : note) 3045 dst.note.add(i.copy()); 3046 } 3047 ; 3048 if (supportingInformation != null) { 3049 dst.supportingInformation = new ArrayList<Reference>(); 3050 for (Reference i : supportingInformation) 3051 dst.supportingInformation.add(i.copy()); 3052 } 3053 ; 3054 } 3055 3056 protected ChargeItem typedCopy() { 3057 return copy(); 3058 } 3059 3060 @Override 3061 public boolean equalsDeep(Base other_) { 3062 if (!super.equalsDeep(other_)) 3063 return false; 3064 if (!(other_ instanceof ChargeItem)) 3065 return false; 3066 ChargeItem o = (ChargeItem) other_; 3067 return compareDeep(identifier, o.identifier, true) && compareDeep(definitionUri, o.definitionUri, true) 3068 && compareDeep(definitionCanonical, o.definitionCanonical, true) && compareDeep(status, o.status, true) 3069 && compareDeep(partOf, o.partOf, true) && compareDeep(code, o.code, true) 3070 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 3071 && compareDeep(occurrence, o.occurrence, true) && compareDeep(performer, o.performer, true) 3072 && compareDeep(performingOrganization, o.performingOrganization, true) 3073 && compareDeep(requestingOrganization, o.requestingOrganization, true) 3074 && compareDeep(costCenter, o.costCenter, true) && compareDeep(quantity, o.quantity, true) 3075 && compareDeep(bodysite, o.bodysite, true) && compareDeep(factorOverride, o.factorOverride, true) 3076 && compareDeep(priceOverride, o.priceOverride, true) && compareDeep(overrideReason, o.overrideReason, true) 3077 && compareDeep(enterer, o.enterer, true) && compareDeep(enteredDate, o.enteredDate, true) 3078 && compareDeep(reason, o.reason, true) && compareDeep(service, o.service, true) 3079 && compareDeep(product, o.product, true) && compareDeep(account, o.account, true) 3080 && compareDeep(note, o.note, true) && compareDeep(supportingInformation, o.supportingInformation, true); 3081 } 3082 3083 @Override 3084 public boolean equalsShallow(Base other_) { 3085 if (!super.equalsShallow(other_)) 3086 return false; 3087 if (!(other_ instanceof ChargeItem)) 3088 return false; 3089 ChargeItem o = (ChargeItem) other_; 3090 return compareValues(definitionUri, o.definitionUri, true) && compareValues(status, o.status, true) 3091 && compareValues(factorOverride, o.factorOverride, true) 3092 && compareValues(overrideReason, o.overrideReason, true) && compareValues(enteredDate, o.enteredDate, true); 3093 } 3094 3095 public boolean isEmpty() { 3096 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definitionUri, definitionCanonical, 3097 status, partOf, code, subject, context, occurrence, performer, performingOrganization, requestingOrganization, 3098 costCenter, quantity, bodysite, factorOverride, priceOverride, overrideReason, enterer, enteredDate, reason, 3099 service, product, account, note, supportingInformation); 3100 } 3101 3102 @Override 3103 public ResourceType getResourceType() { 3104 return ResourceType.ChargeItem; 3105 } 3106 3107 /** 3108 * Search parameter: <b>identifier</b> 3109 * <p> 3110 * Description: <b>Business Identifier for item</b><br> 3111 * Type: <b>token</b><br> 3112 * Path: <b>ChargeItem.identifier</b><br> 3113 * </p> 3114 */ 3115 @SearchParamDefinition(name = "identifier", path = "ChargeItem.identifier", description = "Business Identifier for item", type = "token") 3116 public static final String SP_IDENTIFIER = "identifier"; 3117 /** 3118 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3119 * <p> 3120 * Description: <b>Business Identifier for item</b><br> 3121 * Type: <b>token</b><br> 3122 * Path: <b>ChargeItem.identifier</b><br> 3123 * </p> 3124 */ 3125 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3126 SP_IDENTIFIER); 3127 3128 /** 3129 * Search parameter: <b>performing-organization</b> 3130 * <p> 3131 * Description: <b>Organization providing the charged service</b><br> 3132 * Type: <b>reference</b><br> 3133 * Path: <b>ChargeItem.performingOrganization</b><br> 3134 * </p> 3135 */ 3136 @SearchParamDefinition(name = "performing-organization", path = "ChargeItem.performingOrganization", description = "Organization providing the charged service", type = "reference", target = { 3137 Organization.class }) 3138 public static final String SP_PERFORMING_ORGANIZATION = "performing-organization"; 3139 /** 3140 * <b>Fluent Client</b> search parameter constant for 3141 * <b>performing-organization</b> 3142 * <p> 3143 * Description: <b>Organization providing the charged service</b><br> 3144 * Type: <b>reference</b><br> 3145 * Path: <b>ChargeItem.performingOrganization</b><br> 3146 * </p> 3147 */ 3148 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3149 SP_PERFORMING_ORGANIZATION); 3150 3151 /** 3152 * Constant for fluent queries to be used to add include statements. Specifies 3153 * the path value of "<b>ChargeItem:performing-organization</b>". 3154 */ 3155 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMING_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3156 "ChargeItem:performing-organization").toLocked(); 3157 3158 /** 3159 * Search parameter: <b>code</b> 3160 * <p> 3161 * Description: <b>A code that identifies the charge, like a billing 3162 * code</b><br> 3163 * Type: <b>token</b><br> 3164 * Path: <b>ChargeItem.code</b><br> 3165 * </p> 3166 */ 3167 @SearchParamDefinition(name = "code", path = "ChargeItem.code", description = "A code that identifies the charge, like a billing code", type = "token") 3168 public static final String SP_CODE = "code"; 3169 /** 3170 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3171 * <p> 3172 * Description: <b>A code that identifies the charge, like a billing 3173 * code</b><br> 3174 * Type: <b>token</b><br> 3175 * Path: <b>ChargeItem.code</b><br> 3176 * </p> 3177 */ 3178 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3179 SP_CODE); 3180 3181 /** 3182 * Search parameter: <b>quantity</b> 3183 * <p> 3184 * Description: <b>Quantity of which the charge item has been serviced</b><br> 3185 * Type: <b>quantity</b><br> 3186 * Path: <b>ChargeItem.quantity</b><br> 3187 * </p> 3188 */ 3189 @SearchParamDefinition(name = "quantity", path = "ChargeItem.quantity", description = "Quantity of which the charge item has been serviced", type = "quantity") 3190 public static final String SP_QUANTITY = "quantity"; 3191 /** 3192 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 3193 * <p> 3194 * Description: <b>Quantity of which the charge item has been serviced</b><br> 3195 * Type: <b>quantity</b><br> 3196 * Path: <b>ChargeItem.quantity</b><br> 3197 * </p> 3198 */ 3199 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3200 SP_QUANTITY); 3201 3202 /** 3203 * Search parameter: <b>subject</b> 3204 * <p> 3205 * Description: <b>Individual service was done for/to</b><br> 3206 * Type: <b>reference</b><br> 3207 * Path: <b>ChargeItem.subject</b><br> 3208 * </p> 3209 */ 3210 @SearchParamDefinition(name = "subject", path = "ChargeItem.subject", description = "Individual service was done for/to", type = "reference", providesMembershipIn = { 3211 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3212 public static final String SP_SUBJECT = "subject"; 3213 /** 3214 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3215 * <p> 3216 * Description: <b>Individual service was done for/to</b><br> 3217 * Type: <b>reference</b><br> 3218 * Path: <b>ChargeItem.subject</b><br> 3219 * </p> 3220 */ 3221 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3222 SP_SUBJECT); 3223 3224 /** 3225 * Constant for fluent queries to be used to add include statements. Specifies 3226 * the path value of "<b>ChargeItem:subject</b>". 3227 */ 3228 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3229 "ChargeItem:subject").toLocked(); 3230 3231 /** 3232 * Search parameter: <b>occurrence</b> 3233 * <p> 3234 * Description: <b>When the charged service was applied</b><br> 3235 * Type: <b>date</b><br> 3236 * Path: <b>ChargeItem.occurrence[x]</b><br> 3237 * </p> 3238 */ 3239 @SearchParamDefinition(name = "occurrence", path = "ChargeItem.occurrence", description = "When the charged service was applied", type = "date") 3240 public static final String SP_OCCURRENCE = "occurrence"; 3241 /** 3242 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3243 * <p> 3244 * Description: <b>When the charged service was applied</b><br> 3245 * Type: <b>date</b><br> 3246 * Path: <b>ChargeItem.occurrence[x]</b><br> 3247 * </p> 3248 */ 3249 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3250 SP_OCCURRENCE); 3251 3252 /** 3253 * Search parameter: <b>entered-date</b> 3254 * <p> 3255 * Description: <b>Date the charge item was entered</b><br> 3256 * Type: <b>date</b><br> 3257 * Path: <b>ChargeItem.enteredDate</b><br> 3258 * </p> 3259 */ 3260 @SearchParamDefinition(name = "entered-date", path = "ChargeItem.enteredDate", description = "Date the charge item was entered", type = "date") 3261 public static final String SP_ENTERED_DATE = "entered-date"; 3262 /** 3263 * <b>Fluent Client</b> search parameter constant for <b>entered-date</b> 3264 * <p> 3265 * Description: <b>Date the charge item was entered</b><br> 3266 * Type: <b>date</b><br> 3267 * Path: <b>ChargeItem.enteredDate</b><br> 3268 * </p> 3269 */ 3270 public static final ca.uhn.fhir.rest.gclient.DateClientParam ENTERED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3271 SP_ENTERED_DATE); 3272 3273 /** 3274 * Search parameter: <b>performer-function</b> 3275 * <p> 3276 * Description: <b>What type of performance was done</b><br> 3277 * Type: <b>token</b><br> 3278 * Path: <b>ChargeItem.performer.function</b><br> 3279 * </p> 3280 */ 3281 @SearchParamDefinition(name = "performer-function", path = "ChargeItem.performer.function", description = "What type of performance was done", type = "token") 3282 public static final String SP_PERFORMER_FUNCTION = "performer-function"; 3283 /** 3284 * <b>Fluent Client</b> search parameter constant for <b>performer-function</b> 3285 * <p> 3286 * Description: <b>What type of performance was done</b><br> 3287 * Type: <b>token</b><br> 3288 * Path: <b>ChargeItem.performer.function</b><br> 3289 * </p> 3290 */ 3291 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_FUNCTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3292 SP_PERFORMER_FUNCTION); 3293 3294 /** 3295 * Search parameter: <b>patient</b> 3296 * <p> 3297 * Description: <b>Individual service was done for/to</b><br> 3298 * Type: <b>reference</b><br> 3299 * Path: <b>ChargeItem.subject</b><br> 3300 * </p> 3301 */ 3302 @SearchParamDefinition(name = "patient", path = "ChargeItem.subject.where(resolve() is Patient)", description = "Individual service was done for/to", type = "reference", target = { 3303 Patient.class }) 3304 public static final String SP_PATIENT = "patient"; 3305 /** 3306 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3307 * <p> 3308 * Description: <b>Individual service was done for/to</b><br> 3309 * Type: <b>reference</b><br> 3310 * Path: <b>ChargeItem.subject</b><br> 3311 * </p> 3312 */ 3313 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3314 SP_PATIENT); 3315 3316 /** 3317 * Constant for fluent queries to be used to add include statements. Specifies 3318 * the path value of "<b>ChargeItem:patient</b>". 3319 */ 3320 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3321 "ChargeItem:patient").toLocked(); 3322 3323 /** 3324 * Search parameter: <b>factor-override</b> 3325 * <p> 3326 * Description: <b>Factor overriding the associated rules</b><br> 3327 * Type: <b>number</b><br> 3328 * Path: <b>ChargeItem.factorOverride</b><br> 3329 * </p> 3330 */ 3331 @SearchParamDefinition(name = "factor-override", path = "ChargeItem.factorOverride", description = "Factor overriding the associated rules", type = "number") 3332 public static final String SP_FACTOR_OVERRIDE = "factor-override"; 3333 /** 3334 * <b>Fluent Client</b> search parameter constant for <b>factor-override</b> 3335 * <p> 3336 * Description: <b>Factor overriding the associated rules</b><br> 3337 * Type: <b>number</b><br> 3338 * Path: <b>ChargeItem.factorOverride</b><br> 3339 * </p> 3340 */ 3341 public static final ca.uhn.fhir.rest.gclient.NumberClientParam FACTOR_OVERRIDE = new ca.uhn.fhir.rest.gclient.NumberClientParam( 3342 SP_FACTOR_OVERRIDE); 3343 3344 /** 3345 * Search parameter: <b>service</b> 3346 * <p> 3347 * Description: <b>Which rendered service is being charged?</b><br> 3348 * Type: <b>reference</b><br> 3349 * Path: <b>ChargeItem.service</b><br> 3350 * </p> 3351 */ 3352 @SearchParamDefinition(name = "service", path = "ChargeItem.service", description = "Which rendered service is being charged?", type = "reference", target = { 3353 DiagnosticReport.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, 3354 MedicationDispense.class, Observation.class, Procedure.class, SupplyDelivery.class }) 3355 public static final String SP_SERVICE = "service"; 3356 /** 3357 * <b>Fluent Client</b> search parameter constant for <b>service</b> 3358 * <p> 3359 * Description: <b>Which rendered service is being charged?</b><br> 3360 * Type: <b>reference</b><br> 3361 * Path: <b>ChargeItem.service</b><br> 3362 * </p> 3363 */ 3364 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3365 SP_SERVICE); 3366 3367 /** 3368 * Constant for fluent queries to be used to add include statements. Specifies 3369 * the path value of "<b>ChargeItem:service</b>". 3370 */ 3371 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include( 3372 "ChargeItem:service").toLocked(); 3373 3374 /** 3375 * Search parameter: <b>price-override</b> 3376 * <p> 3377 * Description: <b>Price overriding the associated rules</b><br> 3378 * Type: <b>quantity</b><br> 3379 * Path: <b>ChargeItem.priceOverride</b><br> 3380 * </p> 3381 */ 3382 @SearchParamDefinition(name = "price-override", path = "ChargeItem.priceOverride", description = "Price overriding the associated rules", type = "quantity") 3383 public static final String SP_PRICE_OVERRIDE = "price-override"; 3384 /** 3385 * <b>Fluent Client</b> search parameter constant for <b>price-override</b> 3386 * <p> 3387 * Description: <b>Price overriding the associated rules</b><br> 3388 * Type: <b>quantity</b><br> 3389 * Path: <b>ChargeItem.priceOverride</b><br> 3390 * </p> 3391 */ 3392 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam PRICE_OVERRIDE = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3393 SP_PRICE_OVERRIDE); 3394 3395 /** 3396 * Search parameter: <b>context</b> 3397 * <p> 3398 * Description: <b>Encounter / Episode associated with event</b><br> 3399 * Type: <b>reference</b><br> 3400 * Path: <b>ChargeItem.context</b><br> 3401 * </p> 3402 */ 3403 @SearchParamDefinition(name = "context", path = "ChargeItem.context", description = "Encounter / Episode associated with event", type = "reference", providesMembershipIn = { 3404 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3405 EpisodeOfCare.class }) 3406 public static final String SP_CONTEXT = "context"; 3407 /** 3408 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3409 * <p> 3410 * Description: <b>Encounter / Episode associated with event</b><br> 3411 * Type: <b>reference</b><br> 3412 * Path: <b>ChargeItem.context</b><br> 3413 * </p> 3414 */ 3415 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3416 SP_CONTEXT); 3417 3418 /** 3419 * Constant for fluent queries to be used to add include statements. Specifies 3420 * the path value of "<b>ChargeItem:context</b>". 3421 */ 3422 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include( 3423 "ChargeItem:context").toLocked(); 3424 3425 /** 3426 * Search parameter: <b>enterer</b> 3427 * <p> 3428 * Description: <b>Individual who was entering</b><br> 3429 * Type: <b>reference</b><br> 3430 * Path: <b>ChargeItem.enterer</b><br> 3431 * </p> 3432 */ 3433 @SearchParamDefinition(name = "enterer", path = "ChargeItem.enterer", description = "Individual who was entering", type = "reference", providesMembershipIn = { 3434 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3435 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3436 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3437 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3438 public static final String SP_ENTERER = "enterer"; 3439 /** 3440 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 3441 * <p> 3442 * Description: <b>Individual who was entering</b><br> 3443 * Type: <b>reference</b><br> 3444 * Path: <b>ChargeItem.enterer</b><br> 3445 * </p> 3446 */ 3447 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3448 SP_ENTERER); 3449 3450 /** 3451 * Constant for fluent queries to be used to add include statements. Specifies 3452 * the path value of "<b>ChargeItem:enterer</b>". 3453 */ 3454 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include( 3455 "ChargeItem:enterer").toLocked(); 3456 3457 /** 3458 * Search parameter: <b>performer-actor</b> 3459 * <p> 3460 * Description: <b>Individual who was performing</b><br> 3461 * Type: <b>reference</b><br> 3462 * Path: <b>ChargeItem.performer.actor</b><br> 3463 * </p> 3464 */ 3465 @SearchParamDefinition(name = "performer-actor", path = "ChargeItem.performer.actor", description = "Individual who was performing", type = "reference", providesMembershipIn = { 3466 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3467 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3468 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 3469 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3470 public static final String SP_PERFORMER_ACTOR = "performer-actor"; 3471 /** 3472 * <b>Fluent Client</b> search parameter constant for <b>performer-actor</b> 3473 * <p> 3474 * Description: <b>Individual who was performing</b><br> 3475 * Type: <b>reference</b><br> 3476 * Path: <b>ChargeItem.performer.actor</b><br> 3477 * </p> 3478 */ 3479 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER_ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3480 SP_PERFORMER_ACTOR); 3481 3482 /** 3483 * Constant for fluent queries to be used to add include statements. Specifies 3484 * the path value of "<b>ChargeItem:performer-actor</b>". 3485 */ 3486 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER_ACTOR = new ca.uhn.fhir.model.api.Include( 3487 "ChargeItem:performer-actor").toLocked(); 3488 3489 /** 3490 * Search parameter: <b>account</b> 3491 * <p> 3492 * Description: <b>Account to place this charge</b><br> 3493 * Type: <b>reference</b><br> 3494 * Path: <b>ChargeItem.account</b><br> 3495 * </p> 3496 */ 3497 @SearchParamDefinition(name = "account", path = "ChargeItem.account", description = "Account to place this charge", type = "reference", target = { 3498 Account.class }) 3499 public static final String SP_ACCOUNT = "account"; 3500 /** 3501 * <b>Fluent Client</b> search parameter constant for <b>account</b> 3502 * <p> 3503 * Description: <b>Account to place this charge</b><br> 3504 * Type: <b>reference</b><br> 3505 * Path: <b>ChargeItem.account</b><br> 3506 * </p> 3507 */ 3508 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3509 SP_ACCOUNT); 3510 3511 /** 3512 * Constant for fluent queries to be used to add include statements. Specifies 3513 * the path value of "<b>ChargeItem:account</b>". 3514 */ 3515 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include( 3516 "ChargeItem:account").toLocked(); 3517 3518 /** 3519 * Search parameter: <b>requesting-organization</b> 3520 * <p> 3521 * Description: <b>Organization requesting the charged service</b><br> 3522 * Type: <b>reference</b><br> 3523 * Path: <b>ChargeItem.requestingOrganization</b><br> 3524 * </p> 3525 */ 3526 @SearchParamDefinition(name = "requesting-organization", path = "ChargeItem.requestingOrganization", description = "Organization requesting the charged service", type = "reference", target = { 3527 Organization.class }) 3528 public static final String SP_REQUESTING_ORGANIZATION = "requesting-organization"; 3529 /** 3530 * <b>Fluent Client</b> search parameter constant for 3531 * <b>requesting-organization</b> 3532 * <p> 3533 * Description: <b>Organization requesting the charged service</b><br> 3534 * Type: <b>reference</b><br> 3535 * Path: <b>ChargeItem.requestingOrganization</b><br> 3536 * </p> 3537 */ 3538 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3539 SP_REQUESTING_ORGANIZATION); 3540 3541 /** 3542 * Constant for fluent queries to be used to add include statements. Specifies 3543 * the path value of "<b>ChargeItem:requesting-organization</b>". 3544 */ 3545 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTING_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3546 "ChargeItem:requesting-organization").toLocked(); 3547 3548}