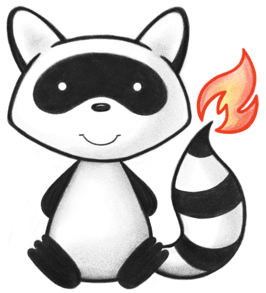
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * The ChargeItemDefinition resource provides the properties that apply to the 054 * (billing) codes necessary to calculate costs and prices. The properties may 055 * differ largely depending on type and realm, therefore this resource gives 056 * only a rough structure and requires profiling for each type of billing code 057 * system. 058 */ 059@ResourceDef(name = "ChargeItemDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ChargeItemDefinition") 060@ChildOrder(names = { "url", "identifier", "version", "title", "derivedFromUri", "partOf", "replaces", "status", 061 "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "copyright", 062 "approvalDate", "lastReviewDate", "effectivePeriod", "code", "instance", "applicability", "propertyGroup" }) 063public class ChargeItemDefinition extends MetadataResource { 064 065 public enum ChargeItemDefinitionPriceComponentType { 066 /** 067 * the amount is the base price used for calculating the total price before 068 * applying surcharges, discount or taxes. 069 */ 070 BASE, 071 /** 072 * the amount is a surcharge applied on the base price. 073 */ 074 SURCHARGE, 075 /** 076 * the amount is a deduction applied on the base price. 077 */ 078 DEDUCTION, 079 /** 080 * the amount is a discount applied on the base price. 081 */ 082 DISCOUNT, 083 /** 084 * the amount is the tax component of the total price. 085 */ 086 TAX, 087 /** 088 * the amount is of informational character, it has not been applied in the 089 * calculation of the total price. 090 */ 091 INFORMATIONAL, 092 /** 093 * added to help the parsers with the generic types 094 */ 095 NULL; 096 097 public static ChargeItemDefinitionPriceComponentType fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("base".equals(codeString)) 101 return BASE; 102 if ("surcharge".equals(codeString)) 103 return SURCHARGE; 104 if ("deduction".equals(codeString)) 105 return DEDUCTION; 106 if ("discount".equals(codeString)) 107 return DISCOUNT; 108 if ("tax".equals(codeString)) 109 return TAX; 110 if ("informational".equals(codeString)) 111 return INFORMATIONAL; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ChargeItemDefinitionPriceComponentType code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case BASE: 121 return "base"; 122 case SURCHARGE: 123 return "surcharge"; 124 case DEDUCTION: 125 return "deduction"; 126 case DISCOUNT: 127 return "discount"; 128 case TAX: 129 return "tax"; 130 case INFORMATIONAL: 131 return "informational"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getSystem() { 140 switch (this) { 141 case BASE: 142 return "http://hl7.org/fhir/invoice-priceComponentType"; 143 case SURCHARGE: 144 return "http://hl7.org/fhir/invoice-priceComponentType"; 145 case DEDUCTION: 146 return "http://hl7.org/fhir/invoice-priceComponentType"; 147 case DISCOUNT: 148 return "http://hl7.org/fhir/invoice-priceComponentType"; 149 case TAX: 150 return "http://hl7.org/fhir/invoice-priceComponentType"; 151 case INFORMATIONAL: 152 return "http://hl7.org/fhir/invoice-priceComponentType"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDefinition() { 161 switch (this) { 162 case BASE: 163 return "the amount is the base price used for calculating the total price before applying surcharges, discount or taxes."; 164 case SURCHARGE: 165 return "the amount is a surcharge applied on the base price."; 166 case DEDUCTION: 167 return "the amount is a deduction applied on the base price."; 168 case DISCOUNT: 169 return "the amount is a discount applied on the base price."; 170 case TAX: 171 return "the amount is the tax component of the total price."; 172 case INFORMATIONAL: 173 return "the amount is of informational character, it has not been applied in the calculation of the total price."; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDisplay() { 182 switch (this) { 183 case BASE: 184 return "base price"; 185 case SURCHARGE: 186 return "surcharge"; 187 case DEDUCTION: 188 return "deduction"; 189 case DISCOUNT: 190 return "discount"; 191 case TAX: 192 return "tax"; 193 case INFORMATIONAL: 194 return "informational"; 195 case NULL: 196 return null; 197 default: 198 return "?"; 199 } 200 } 201 } 202 203 public static class ChargeItemDefinitionPriceComponentTypeEnumFactory 204 implements EnumFactory<ChargeItemDefinitionPriceComponentType> { 205 public ChargeItemDefinitionPriceComponentType fromCode(String codeString) throws IllegalArgumentException { 206 if (codeString == null || "".equals(codeString)) 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("base".equals(codeString)) 210 return ChargeItemDefinitionPriceComponentType.BASE; 211 if ("surcharge".equals(codeString)) 212 return ChargeItemDefinitionPriceComponentType.SURCHARGE; 213 if ("deduction".equals(codeString)) 214 return ChargeItemDefinitionPriceComponentType.DEDUCTION; 215 if ("discount".equals(codeString)) 216 return ChargeItemDefinitionPriceComponentType.DISCOUNT; 217 if ("tax".equals(codeString)) 218 return ChargeItemDefinitionPriceComponentType.TAX; 219 if ("informational".equals(codeString)) 220 return ChargeItemDefinitionPriceComponentType.INFORMATIONAL; 221 throw new IllegalArgumentException("Unknown ChargeItemDefinitionPriceComponentType code '" + codeString + "'"); 222 } 223 224 public Enumeration<ChargeItemDefinitionPriceComponentType> fromType(PrimitiveType<?> code) throws FHIRException { 225 if (code == null) 226 return null; 227 if (code.isEmpty()) 228 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 229 ChargeItemDefinitionPriceComponentType.NULL, code); 230 String codeString = code.asStringValue(); 231 if (codeString == null || "".equals(codeString)) 232 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 233 ChargeItemDefinitionPriceComponentType.NULL, code); 234 if ("base".equals(codeString)) 235 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 236 ChargeItemDefinitionPriceComponentType.BASE, code); 237 if ("surcharge".equals(codeString)) 238 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 239 ChargeItemDefinitionPriceComponentType.SURCHARGE, code); 240 if ("deduction".equals(codeString)) 241 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 242 ChargeItemDefinitionPriceComponentType.DEDUCTION, code); 243 if ("discount".equals(codeString)) 244 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 245 ChargeItemDefinitionPriceComponentType.DISCOUNT, code); 246 if ("tax".equals(codeString)) 247 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, ChargeItemDefinitionPriceComponentType.TAX, 248 code); 249 if ("informational".equals(codeString)) 250 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 251 ChargeItemDefinitionPriceComponentType.INFORMATIONAL, code); 252 throw new FHIRException("Unknown ChargeItemDefinitionPriceComponentType code '" + codeString + "'"); 253 } 254 255 public String toCode(ChargeItemDefinitionPriceComponentType code) { 256 if (code == ChargeItemDefinitionPriceComponentType.BASE) 257 return "base"; 258 if (code == ChargeItemDefinitionPriceComponentType.SURCHARGE) 259 return "surcharge"; 260 if (code == ChargeItemDefinitionPriceComponentType.DEDUCTION) 261 return "deduction"; 262 if (code == ChargeItemDefinitionPriceComponentType.DISCOUNT) 263 return "discount"; 264 if (code == ChargeItemDefinitionPriceComponentType.TAX) 265 return "tax"; 266 if (code == ChargeItemDefinitionPriceComponentType.INFORMATIONAL) 267 return "informational"; 268 return "?"; 269 } 270 271 public String toSystem(ChargeItemDefinitionPriceComponentType code) { 272 return code.getSystem(); 273 } 274 } 275 276 @Block() 277 public static class ChargeItemDefinitionApplicabilityComponent extends BackboneElement 278 implements IBaseBackboneElement { 279 /** 280 * A brief, natural language description of the condition that effectively 281 * communicates the intended semantics. 282 */ 283 @Child(name = "description", type = { 284 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 285 @Description(shortDefinition = "Natural language description of the condition", formalDefinition = "A brief, natural language description of the condition that effectively communicates the intended semantics.") 286 protected StringType description; 287 288 /** 289 * The media type of the language for the expression, e.g. "text/cql" for 290 * Clinical Query Language expressions or "text/fhirpath" for FHIRPath 291 * expressions. 292 */ 293 @Child(name = "language", type = { 294 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 295 @Description(shortDefinition = "Language of the expression", formalDefinition = "The media type of the language for the expression, e.g. \"text/cql\" for Clinical Query Language expressions or \"text/fhirpath\" for FHIRPath expressions.") 296 protected StringType language; 297 298 /** 299 * An expression that returns true or false, indicating whether the condition is 300 * satisfied. When using FHIRPath expressions, the %context environment variable 301 * must be replaced at runtime with the ChargeItem resource to which this 302 * definition is applied. 303 */ 304 @Child(name = "expression", type = { 305 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 306 @Description(shortDefinition = "Boolean-valued expression", formalDefinition = "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.") 307 protected StringType expression; 308 309 private static final long serialVersionUID = 1354288281L; 310 311 /** 312 * Constructor 313 */ 314 public ChargeItemDefinitionApplicabilityComponent() { 315 super(); 316 } 317 318 /** 319 * @return {@link #description} (A brief, natural language description of the 320 * condition that effectively communicates the intended semantics.). 321 * This is the underlying object with id, value and extensions. The 322 * accessor "getDescription" gives direct access to the value 323 */ 324 public StringType getDescriptionElement() { 325 if (this.description == null) 326 if (Configuration.errorOnAutoCreate()) 327 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.description"); 328 else if (Configuration.doAutoCreate()) 329 this.description = new StringType(); // bb 330 return this.description; 331 } 332 333 public boolean hasDescriptionElement() { 334 return this.description != null && !this.description.isEmpty(); 335 } 336 337 public boolean hasDescription() { 338 return this.description != null && !this.description.isEmpty(); 339 } 340 341 /** 342 * @param value {@link #description} (A brief, natural language description of 343 * the condition that effectively communicates the intended 344 * semantics.). This is the underlying object with id, value and 345 * extensions. The accessor "getDescription" gives direct access to 346 * the value 347 */ 348 public ChargeItemDefinitionApplicabilityComponent setDescriptionElement(StringType value) { 349 this.description = value; 350 return this; 351 } 352 353 /** 354 * @return A brief, natural language description of the condition that 355 * effectively communicates the intended semantics. 356 */ 357 public String getDescription() { 358 return this.description == null ? null : this.description.getValue(); 359 } 360 361 /** 362 * @param value A brief, natural language description of the condition that 363 * effectively communicates the intended semantics. 364 */ 365 public ChargeItemDefinitionApplicabilityComponent setDescription(String value) { 366 if (Utilities.noString(value)) 367 this.description = null; 368 else { 369 if (this.description == null) 370 this.description = new StringType(); 371 this.description.setValue(value); 372 } 373 return this; 374 } 375 376 /** 377 * @return {@link #language} (The media type of the language for the expression, 378 * e.g. "text/cql" for Clinical Query Language expressions or 379 * "text/fhirpath" for FHIRPath expressions.). This is the underlying 380 * object with id, value and extensions. The accessor "getLanguage" 381 * gives direct access to the value 382 */ 383 public StringType getLanguageElement() { 384 if (this.language == null) 385 if (Configuration.errorOnAutoCreate()) 386 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.language"); 387 else if (Configuration.doAutoCreate()) 388 this.language = new StringType(); // bb 389 return this.language; 390 } 391 392 public boolean hasLanguageElement() { 393 return this.language != null && !this.language.isEmpty(); 394 } 395 396 public boolean hasLanguage() { 397 return this.language != null && !this.language.isEmpty(); 398 } 399 400 /** 401 * @param value {@link #language} (The media type of the language for the 402 * expression, e.g. "text/cql" for Clinical Query Language 403 * expressions or "text/fhirpath" for FHIRPath expressions.). This 404 * is the underlying object with id, value and extensions. The 405 * accessor "getLanguage" gives direct access to the value 406 */ 407 public ChargeItemDefinitionApplicabilityComponent setLanguageElement(StringType value) { 408 this.language = value; 409 return this; 410 } 411 412 /** 413 * @return The media type of the language for the expression, e.g. "text/cql" 414 * for Clinical Query Language expressions or "text/fhirpath" for 415 * FHIRPath expressions. 416 */ 417 public String getLanguage() { 418 return this.language == null ? null : this.language.getValue(); 419 } 420 421 /** 422 * @param value The media type of the language for the expression, e.g. 423 * "text/cql" for Clinical Query Language expressions or 424 * "text/fhirpath" for FHIRPath expressions. 425 */ 426 public ChargeItemDefinitionApplicabilityComponent setLanguage(String value) { 427 if (Utilities.noString(value)) 428 this.language = null; 429 else { 430 if (this.language == null) 431 this.language = new StringType(); 432 this.language.setValue(value); 433 } 434 return this; 435 } 436 437 /** 438 * @return {@link #expression} (An expression that returns true or false, 439 * indicating whether the condition is satisfied. When using FHIRPath 440 * expressions, the %context environment variable must be replaced at 441 * runtime with the ChargeItem resource to which this definition is 442 * applied.). This is the underlying object with id, value and 443 * extensions. The accessor "getExpression" gives direct access to the 444 * value 445 */ 446 public StringType getExpressionElement() { 447 if (this.expression == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.expression"); 450 else if (Configuration.doAutoCreate()) 451 this.expression = new StringType(); // bb 452 return this.expression; 453 } 454 455 public boolean hasExpressionElement() { 456 return this.expression != null && !this.expression.isEmpty(); 457 } 458 459 public boolean hasExpression() { 460 return this.expression != null && !this.expression.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #expression} (An expression that returns true or false, 465 * indicating whether the condition is satisfied. When using 466 * FHIRPath expressions, the %context environment variable must be 467 * replaced at runtime with the ChargeItem resource to which this 468 * definition is applied.). This is the underlying object with id, 469 * value and extensions. The accessor "getExpression" gives direct 470 * access to the value 471 */ 472 public ChargeItemDefinitionApplicabilityComponent setExpressionElement(StringType value) { 473 this.expression = value; 474 return this; 475 } 476 477 /** 478 * @return An expression that returns true or false, indicating whether the 479 * condition is satisfied. When using FHIRPath expressions, the %context 480 * environment variable must be replaced at runtime with the ChargeItem 481 * resource to which this definition is applied. 482 */ 483 public String getExpression() { 484 return this.expression == null ? null : this.expression.getValue(); 485 } 486 487 /** 488 * @param value An expression that returns true or false, indicating whether the 489 * condition is satisfied. When using FHIRPath expressions, the 490 * %context environment variable must be replaced at runtime with 491 * the ChargeItem resource to which this definition is applied. 492 */ 493 public ChargeItemDefinitionApplicabilityComponent setExpression(String value) { 494 if (Utilities.noString(value)) 495 this.expression = null; 496 else { 497 if (this.expression == null) 498 this.expression = new StringType(); 499 this.expression.setValue(value); 500 } 501 return this; 502 } 503 504 protected void listChildren(List<Property> children) { 505 super.listChildren(children); 506 children.add(new Property("description", "string", 507 "A brief, natural language description of the condition that effectively communicates the intended semantics.", 508 0, 1, description)); 509 children.add(new Property("language", "string", 510 "The media type of the language for the expression, e.g. \"text/cql\" for Clinical Query Language expressions or \"text/fhirpath\" for FHIRPath expressions.", 511 0, 1, language)); 512 children.add(new Property("expression", "string", 513 "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 514 0, 1, expression)); 515 } 516 517 @Override 518 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 519 switch (_hash) { 520 case -1724546052: 521 /* description */ return new Property("description", "string", 522 "A brief, natural language description of the condition that effectively communicates the intended semantics.", 523 0, 1, description); 524 case -1613589672: 525 /* language */ return new Property("language", "string", 526 "The media type of the language for the expression, e.g. \"text/cql\" for Clinical Query Language expressions or \"text/fhirpath\" for FHIRPath expressions.", 527 0, 1, language); 528 case -1795452264: 529 /* expression */ return new Property("expression", "string", 530 "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 531 0, 1, expression); 532 default: 533 return super.getNamedProperty(_hash, _name, _checkValid); 534 } 535 536 } 537 538 @Override 539 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 540 switch (hash) { 541 case -1724546052: 542 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 543 case -1613589672: 544 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // StringType 545 case -1795452264: 546 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 547 default: 548 return super.getProperty(hash, name, checkValid); 549 } 550 551 } 552 553 @Override 554 public Base setProperty(int hash, String name, Base value) throws FHIRException { 555 switch (hash) { 556 case -1724546052: // description 557 this.description = castToString(value); // StringType 558 return value; 559 case -1613589672: // language 560 this.language = castToString(value); // StringType 561 return value; 562 case -1795452264: // expression 563 this.expression = castToString(value); // StringType 564 return value; 565 default: 566 return super.setProperty(hash, name, value); 567 } 568 569 } 570 571 @Override 572 public Base setProperty(String name, Base value) throws FHIRException { 573 if (name.equals("description")) { 574 this.description = castToString(value); // StringType 575 } else if (name.equals("language")) { 576 this.language = castToString(value); // StringType 577 } else if (name.equals("expression")) { 578 this.expression = castToString(value); // StringType 579 } else 580 return super.setProperty(name, value); 581 return value; 582 } 583 584 @Override 585 public Base makeProperty(int hash, String name) throws FHIRException { 586 switch (hash) { 587 case -1724546052: 588 return getDescriptionElement(); 589 case -1613589672: 590 return getLanguageElement(); 591 case -1795452264: 592 return getExpressionElement(); 593 default: 594 return super.makeProperty(hash, name); 595 } 596 597 } 598 599 @Override 600 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case -1724546052: 603 /* description */ return new String[] { "string" }; 604 case -1613589672: 605 /* language */ return new String[] { "string" }; 606 case -1795452264: 607 /* expression */ return new String[] { "string" }; 608 default: 609 return super.getTypesForProperty(hash, name); 610 } 611 612 } 613 614 @Override 615 public Base addChild(String name) throws FHIRException { 616 if (name.equals("description")) { 617 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.description"); 618 } else if (name.equals("language")) { 619 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.language"); 620 } else if (name.equals("expression")) { 621 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.expression"); 622 } else 623 return super.addChild(name); 624 } 625 626 public ChargeItemDefinitionApplicabilityComponent copy() { 627 ChargeItemDefinitionApplicabilityComponent dst = new ChargeItemDefinitionApplicabilityComponent(); 628 copyValues(dst); 629 return dst; 630 } 631 632 public void copyValues(ChargeItemDefinitionApplicabilityComponent dst) { 633 super.copyValues(dst); 634 dst.description = description == null ? null : description.copy(); 635 dst.language = language == null ? null : language.copy(); 636 dst.expression = expression == null ? null : expression.copy(); 637 } 638 639 @Override 640 public boolean equalsDeep(Base other_) { 641 if (!super.equalsDeep(other_)) 642 return false; 643 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 644 return false; 645 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 646 return compareDeep(description, o.description, true) && compareDeep(language, o.language, true) 647 && compareDeep(expression, o.expression, true); 648 } 649 650 @Override 651 public boolean equalsShallow(Base other_) { 652 if (!super.equalsShallow(other_)) 653 return false; 654 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 655 return false; 656 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 657 return compareValues(description, o.description, true) && compareValues(language, o.language, true) 658 && compareValues(expression, o.expression, true); 659 } 660 661 public boolean isEmpty() { 662 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, language, expression); 663 } 664 665 public String fhirType() { 666 return "ChargeItemDefinition.applicability"; 667 668 } 669 670 } 671 672 @Block() 673 public static class ChargeItemDefinitionPropertyGroupComponent extends BackboneElement 674 implements IBaseBackboneElement { 675 /** 676 * Expressions that describe applicability criteria for the priceComponent. 677 */ 678 @Child(name = "applicability", type = { 679 ChargeItemDefinitionApplicabilityComponent.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 680 @Description(shortDefinition = "Conditions under which the priceComponent is applicable", formalDefinition = "Expressions that describe applicability criteria for the priceComponent.") 681 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 682 683 /** 684 * The price for a ChargeItem may be calculated as a base price with 685 * surcharges/deductions that apply in certain conditions. A 686 * ChargeItemDefinition resource that defines the prices, factors and conditions 687 * that apply to a billing code is currently under development. The 688 * priceComponent element can be used to offer transparency to the recipient of 689 * the Invoice of how the prices have been calculated. 690 */ 691 @Child(name = "priceComponent", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 692 @Description(shortDefinition = "Components of total line item price", formalDefinition = "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.") 693 protected List<ChargeItemDefinitionPropertyGroupPriceComponentComponent> priceComponent; 694 695 private static final long serialVersionUID = 1723436176L; 696 697 /** 698 * Constructor 699 */ 700 public ChargeItemDefinitionPropertyGroupComponent() { 701 super(); 702 } 703 704 /** 705 * @return {@link #applicability} (Expressions that describe applicability 706 * criteria for the priceComponent.) 707 */ 708 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 709 if (this.applicability == null) 710 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 711 return this.applicability; 712 } 713 714 /** 715 * @return Returns a reference to <code>this</code> for easy method chaining 716 */ 717 public ChargeItemDefinitionPropertyGroupComponent setApplicability( 718 List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 719 this.applicability = theApplicability; 720 return this; 721 } 722 723 public boolean hasApplicability() { 724 if (this.applicability == null) 725 return false; 726 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 727 if (!item.isEmpty()) 728 return true; 729 return false; 730 } 731 732 public ChargeItemDefinitionApplicabilityComponent addApplicability() { // 3 733 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 734 if (this.applicability == null) 735 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 736 this.applicability.add(t); 737 return t; 738 } 739 740 public ChargeItemDefinitionPropertyGroupComponent addApplicability(ChargeItemDefinitionApplicabilityComponent t) { // 3 741 if (t == null) 742 return this; 743 if (this.applicability == null) 744 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 745 this.applicability.add(t); 746 return this; 747 } 748 749 /** 750 * @return The first repetition of repeating field {@link #applicability}, 751 * creating it if it does not already exist 752 */ 753 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 754 if (getApplicability().isEmpty()) { 755 addApplicability(); 756 } 757 return getApplicability().get(0); 758 } 759 760 /** 761 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated 762 * as a base price with surcharges/deductions that apply in certain 763 * conditions. A ChargeItemDefinition resource that defines the prices, 764 * factors and conditions that apply to a billing code is currently 765 * under development. The priceComponent element can be used to offer 766 * transparency to the recipient of the Invoice of how the prices have 767 * been calculated.) 768 */ 769 public List<ChargeItemDefinitionPropertyGroupPriceComponentComponent> getPriceComponent() { 770 if (this.priceComponent == null) 771 this.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 772 return this.priceComponent; 773 } 774 775 /** 776 * @return Returns a reference to <code>this</code> for easy method chaining 777 */ 778 public ChargeItemDefinitionPropertyGroupComponent setPriceComponent( 779 List<ChargeItemDefinitionPropertyGroupPriceComponentComponent> thePriceComponent) { 780 this.priceComponent = thePriceComponent; 781 return this; 782 } 783 784 public boolean hasPriceComponent() { 785 if (this.priceComponent == null) 786 return false; 787 for (ChargeItemDefinitionPropertyGroupPriceComponentComponent item : this.priceComponent) 788 if (!item.isEmpty()) 789 return true; 790 return false; 791 } 792 793 public ChargeItemDefinitionPropertyGroupPriceComponentComponent addPriceComponent() { // 3 794 ChargeItemDefinitionPropertyGroupPriceComponentComponent t = new ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 795 if (this.priceComponent == null) 796 this.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 797 this.priceComponent.add(t); 798 return t; 799 } 800 801 public ChargeItemDefinitionPropertyGroupComponent addPriceComponent( 802 ChargeItemDefinitionPropertyGroupPriceComponentComponent t) { // 3 803 if (t == null) 804 return this; 805 if (this.priceComponent == null) 806 this.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 807 this.priceComponent.add(t); 808 return this; 809 } 810 811 /** 812 * @return The first repetition of repeating field {@link #priceComponent}, 813 * creating it if it does not already exist 814 */ 815 public ChargeItemDefinitionPropertyGroupPriceComponentComponent getPriceComponentFirstRep() { 816 if (getPriceComponent().isEmpty()) { 817 addPriceComponent(); 818 } 819 return getPriceComponent().get(0); 820 } 821 822 protected void listChildren(List<Property> children) { 823 super.listChildren(children); 824 children.add(new Property("applicability", "@ChargeItemDefinition.applicability", 825 "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, 826 applicability)); 827 children.add(new Property("priceComponent", "", 828 "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 829 0, java.lang.Integer.MAX_VALUE, priceComponent)); 830 } 831 832 @Override 833 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 834 switch (_hash) { 835 case -1526770491: 836 /* applicability */ return new Property("applicability", "@ChargeItemDefinition.applicability", 837 "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, 838 applicability); 839 case 1219095988: 840 /* priceComponent */ return new Property("priceComponent", "", 841 "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 842 0, java.lang.Integer.MAX_VALUE, priceComponent); 843 default: 844 return super.getNamedProperty(_hash, _name, _checkValid); 845 } 846 847 } 848 849 @Override 850 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 851 switch (hash) { 852 case -1526770491: 853 /* applicability */ return this.applicability == null ? new Base[0] 854 : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 855 case 1219095988: 856 /* priceComponent */ return this.priceComponent == null ? new Base[0] 857 : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // ChargeItemDefinitionPropertyGroupPriceComponentComponent 858 default: 859 return super.getProperty(hash, name, checkValid); 860 } 861 862 } 863 864 @Override 865 public Base setProperty(int hash, String name, Base value) throws FHIRException { 866 switch (hash) { 867 case -1526770491: // applicability 868 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 869 return value; 870 case 1219095988: // priceComponent 871 this.getPriceComponent().add((ChargeItemDefinitionPropertyGroupPriceComponentComponent) value); // ChargeItemDefinitionPropertyGroupPriceComponentComponent 872 return value; 873 default: 874 return super.setProperty(hash, name, value); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(String name, Base value) throws FHIRException { 881 if (name.equals("applicability")) { 882 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 883 } else if (name.equals("priceComponent")) { 884 this.getPriceComponent().add((ChargeItemDefinitionPropertyGroupPriceComponentComponent) value); 885 } else 886 return super.setProperty(name, value); 887 return value; 888 } 889 890 @Override 891 public Base makeProperty(int hash, String name) throws FHIRException { 892 switch (hash) { 893 case -1526770491: 894 return addApplicability(); 895 case 1219095988: 896 return addPriceComponent(); 897 default: 898 return super.makeProperty(hash, name); 899 } 900 901 } 902 903 @Override 904 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 905 switch (hash) { 906 case -1526770491: 907 /* applicability */ return new String[] { "@ChargeItemDefinition.applicability" }; 908 case 1219095988: 909 /* priceComponent */ return new String[] {}; 910 default: 911 return super.getTypesForProperty(hash, name); 912 } 913 914 } 915 916 @Override 917 public Base addChild(String name) throws FHIRException { 918 if (name.equals("applicability")) { 919 return addApplicability(); 920 } else if (name.equals("priceComponent")) { 921 return addPriceComponent(); 922 } else 923 return super.addChild(name); 924 } 925 926 public ChargeItemDefinitionPropertyGroupComponent copy() { 927 ChargeItemDefinitionPropertyGroupComponent dst = new ChargeItemDefinitionPropertyGroupComponent(); 928 copyValues(dst); 929 return dst; 930 } 931 932 public void copyValues(ChargeItemDefinitionPropertyGroupComponent dst) { 933 super.copyValues(dst); 934 if (applicability != null) { 935 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 936 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 937 dst.applicability.add(i.copy()); 938 } 939 ; 940 if (priceComponent != null) { 941 dst.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 942 for (ChargeItemDefinitionPropertyGroupPriceComponentComponent i : priceComponent) 943 dst.priceComponent.add(i.copy()); 944 } 945 ; 946 } 947 948 @Override 949 public boolean equalsDeep(Base other_) { 950 if (!super.equalsDeep(other_)) 951 return false; 952 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 953 return false; 954 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 955 return compareDeep(applicability, o.applicability, true) && compareDeep(priceComponent, o.priceComponent, true); 956 } 957 958 @Override 959 public boolean equalsShallow(Base other_) { 960 if (!super.equalsShallow(other_)) 961 return false; 962 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 963 return false; 964 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 965 return true; 966 } 967 968 public boolean isEmpty() { 969 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(applicability, priceComponent); 970 } 971 972 public String fhirType() { 973 return "ChargeItemDefinition.propertyGroup"; 974 975 } 976 977 } 978 979 @Block() 980 public static class ChargeItemDefinitionPropertyGroupPriceComponentComponent extends BackboneElement 981 implements IBaseBackboneElement { 982 /** 983 * This code identifies the type of the component. 984 */ 985 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 986 @Description(shortDefinition = "base | surcharge | deduction | discount | tax | informational", formalDefinition = "This code identifies the type of the component.") 987 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/invoice-priceComponentType") 988 protected Enumeration<ChargeItemDefinitionPriceComponentType> type; 989 990 /** 991 * A code that identifies the component. Codes may be used to differentiate 992 * between kinds of taxes, surcharges, discounts etc. 993 */ 994 @Child(name = "code", type = { 995 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 996 @Description(shortDefinition = "Code identifying the specific component", formalDefinition = "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.") 997 protected CodeableConcept code; 998 999 /** 1000 * The factor that has been applied on the base price for calculating this 1001 * component. 1002 */ 1003 @Child(name = "factor", type = { 1004 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1005 @Description(shortDefinition = "Factor used for calculating this component", formalDefinition = "The factor that has been applied on the base price for calculating this component.") 1006 protected DecimalType factor; 1007 1008 /** 1009 * The amount calculated for this component. 1010 */ 1011 @Child(name = "amount", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1012 @Description(shortDefinition = "Monetary amount associated with this component", formalDefinition = "The amount calculated for this component.") 1013 protected Money amount; 1014 1015 private static final long serialVersionUID = -841451335L; 1016 1017 /** 1018 * Constructor 1019 */ 1020 public ChargeItemDefinitionPropertyGroupPriceComponentComponent() { 1021 super(); 1022 } 1023 1024 /** 1025 * Constructor 1026 */ 1027 public ChargeItemDefinitionPropertyGroupPriceComponentComponent( 1028 Enumeration<ChargeItemDefinitionPriceComponentType> type) { 1029 super(); 1030 this.type = type; 1031 } 1032 1033 /** 1034 * @return {@link #type} (This code identifies the type of the component.). This 1035 * is the underlying object with id, value and extensions. The accessor 1036 * "getType" gives direct access to the value 1037 */ 1038 public Enumeration<ChargeItemDefinitionPriceComponentType> getTypeElement() { 1039 if (this.type == null) 1040 if (Configuration.errorOnAutoCreate()) 1041 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.type"); 1042 else if (Configuration.doAutoCreate()) 1043 this.type = new Enumeration<ChargeItemDefinitionPriceComponentType>( 1044 new ChargeItemDefinitionPriceComponentTypeEnumFactory()); // bb 1045 return this.type; 1046 } 1047 1048 public boolean hasTypeElement() { 1049 return this.type != null && !this.type.isEmpty(); 1050 } 1051 1052 public boolean hasType() { 1053 return this.type != null && !this.type.isEmpty(); 1054 } 1055 1056 /** 1057 * @param value {@link #type} (This code identifies the type of the component.). 1058 * This is the underlying object with id, value and extensions. The 1059 * accessor "getType" gives direct access to the value 1060 */ 1061 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setTypeElement( 1062 Enumeration<ChargeItemDefinitionPriceComponentType> value) { 1063 this.type = value; 1064 return this; 1065 } 1066 1067 /** 1068 * @return This code identifies the type of the component. 1069 */ 1070 public ChargeItemDefinitionPriceComponentType getType() { 1071 return this.type == null ? null : this.type.getValue(); 1072 } 1073 1074 /** 1075 * @param value This code identifies the type of the component. 1076 */ 1077 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setType( 1078 ChargeItemDefinitionPriceComponentType value) { 1079 if (this.type == null) 1080 this.type = new Enumeration<ChargeItemDefinitionPriceComponentType>( 1081 new ChargeItemDefinitionPriceComponentTypeEnumFactory()); 1082 this.type.setValue(value); 1083 return this; 1084 } 1085 1086 /** 1087 * @return {@link #code} (A code that identifies the component. Codes may be 1088 * used to differentiate between kinds of taxes, surcharges, discounts 1089 * etc.) 1090 */ 1091 public CodeableConcept getCode() { 1092 if (this.code == null) 1093 if (Configuration.errorOnAutoCreate()) 1094 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.code"); 1095 else if (Configuration.doAutoCreate()) 1096 this.code = new CodeableConcept(); // cc 1097 return this.code; 1098 } 1099 1100 public boolean hasCode() { 1101 return this.code != null && !this.code.isEmpty(); 1102 } 1103 1104 /** 1105 * @param value {@link #code} (A code that identifies the component. Codes may 1106 * be used to differentiate between kinds of taxes, surcharges, 1107 * discounts etc.) 1108 */ 1109 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setCode(CodeableConcept value) { 1110 this.code = value; 1111 return this; 1112 } 1113 1114 /** 1115 * @return {@link #factor} (The factor that has been applied on the base price 1116 * for calculating this component.). This is the underlying object with 1117 * id, value and extensions. The accessor "getFactor" gives direct 1118 * access to the value 1119 */ 1120 public DecimalType getFactorElement() { 1121 if (this.factor == null) 1122 if (Configuration.errorOnAutoCreate()) 1123 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.factor"); 1124 else if (Configuration.doAutoCreate()) 1125 this.factor = new DecimalType(); // bb 1126 return this.factor; 1127 } 1128 1129 public boolean hasFactorElement() { 1130 return this.factor != null && !this.factor.isEmpty(); 1131 } 1132 1133 public boolean hasFactor() { 1134 return this.factor != null && !this.factor.isEmpty(); 1135 } 1136 1137 /** 1138 * @param value {@link #factor} (The factor that has been applied on the base 1139 * price for calculating this component.). This is the underlying 1140 * object with id, value and extensions. The accessor "getFactor" 1141 * gives direct access to the value 1142 */ 1143 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactorElement(DecimalType value) { 1144 this.factor = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return The factor that has been applied on the base price for calculating 1150 * this component. 1151 */ 1152 public BigDecimal getFactor() { 1153 return this.factor == null ? null : this.factor.getValue(); 1154 } 1155 1156 /** 1157 * @param value The factor that has been applied on the base price for 1158 * calculating this component. 1159 */ 1160 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactor(BigDecimal value) { 1161 if (value == null) 1162 this.factor = null; 1163 else { 1164 if (this.factor == null) 1165 this.factor = new DecimalType(); 1166 this.factor.setValue(value); 1167 } 1168 return this; 1169 } 1170 1171 /** 1172 * @param value The factor that has been applied on the base price for 1173 * calculating this component. 1174 */ 1175 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactor(long value) { 1176 this.factor = new DecimalType(); 1177 this.factor.setValue(value); 1178 return this; 1179 } 1180 1181 /** 1182 * @param value The factor that has been applied on the base price for 1183 * calculating this component. 1184 */ 1185 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactor(double value) { 1186 this.factor = new DecimalType(); 1187 this.factor.setValue(value); 1188 return this; 1189 } 1190 1191 /** 1192 * @return {@link #amount} (The amount calculated for this component.) 1193 */ 1194 public Money getAmount() { 1195 if (this.amount == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.amount"); 1198 else if (Configuration.doAutoCreate()) 1199 this.amount = new Money(); // cc 1200 return this.amount; 1201 } 1202 1203 public boolean hasAmount() { 1204 return this.amount != null && !this.amount.isEmpty(); 1205 } 1206 1207 /** 1208 * @param value {@link #amount} (The amount calculated for this component.) 1209 */ 1210 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setAmount(Money value) { 1211 this.amount = value; 1212 return this; 1213 } 1214 1215 protected void listChildren(List<Property> children) { 1216 super.listChildren(children); 1217 children.add(new Property("type", "code", "This code identifies the type of the component.", 0, 1, type)); 1218 children.add(new Property("code", "CodeableConcept", 1219 "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 1220 0, 1, code)); 1221 children.add(new Property("factor", "decimal", 1222 "The factor that has been applied on the base price for calculating this component.", 0, 1, factor)); 1223 children.add(new Property("amount", "Money", "The amount calculated for this component.", 0, 1, amount)); 1224 } 1225 1226 @Override 1227 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1228 switch (_hash) { 1229 case 3575610: 1230 /* type */ return new Property("type", "code", "This code identifies the type of the component.", 0, 1, type); 1231 case 3059181: 1232 /* code */ return new Property("code", "CodeableConcept", 1233 "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 1234 0, 1, code); 1235 case -1282148017: 1236 /* factor */ return new Property("factor", "decimal", 1237 "The factor that has been applied on the base price for calculating this component.", 0, 1, factor); 1238 case -1413853096: 1239 /* amount */ return new Property("amount", "Money", "The amount calculated for this component.", 0, 1, amount); 1240 default: 1241 return super.getNamedProperty(_hash, _name, _checkValid); 1242 } 1243 1244 } 1245 1246 @Override 1247 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1248 switch (hash) { 1249 case 3575610: 1250 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ChargeItemDefinitionPriceComponentType> 1251 case 3059181: 1252 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1253 case -1282148017: 1254 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 1255 case -1413853096: 1256 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 1257 default: 1258 return super.getProperty(hash, name, checkValid); 1259 } 1260 1261 } 1262 1263 @Override 1264 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1265 switch (hash) { 1266 case 3575610: // type 1267 value = new ChargeItemDefinitionPriceComponentTypeEnumFactory().fromType(castToCode(value)); 1268 this.type = (Enumeration) value; // Enumeration<ChargeItemDefinitionPriceComponentType> 1269 return value; 1270 case 3059181: // code 1271 this.code = castToCodeableConcept(value); // CodeableConcept 1272 return value; 1273 case -1282148017: // factor 1274 this.factor = castToDecimal(value); // DecimalType 1275 return value; 1276 case -1413853096: // amount 1277 this.amount = castToMoney(value); // Money 1278 return value; 1279 default: 1280 return super.setProperty(hash, name, value); 1281 } 1282 1283 } 1284 1285 @Override 1286 public Base setProperty(String name, Base value) throws FHIRException { 1287 if (name.equals("type")) { 1288 value = new ChargeItemDefinitionPriceComponentTypeEnumFactory().fromType(castToCode(value)); 1289 this.type = (Enumeration) value; // Enumeration<ChargeItemDefinitionPriceComponentType> 1290 } else if (name.equals("code")) { 1291 this.code = castToCodeableConcept(value); // CodeableConcept 1292 } else if (name.equals("factor")) { 1293 this.factor = castToDecimal(value); // DecimalType 1294 } else if (name.equals("amount")) { 1295 this.amount = castToMoney(value); // Money 1296 } else 1297 return super.setProperty(name, value); 1298 return value; 1299 } 1300 1301 @Override 1302 public Base makeProperty(int hash, String name) throws FHIRException { 1303 switch (hash) { 1304 case 3575610: 1305 return getTypeElement(); 1306 case 3059181: 1307 return getCode(); 1308 case -1282148017: 1309 return getFactorElement(); 1310 case -1413853096: 1311 return getAmount(); 1312 default: 1313 return super.makeProperty(hash, name); 1314 } 1315 1316 } 1317 1318 @Override 1319 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1320 switch (hash) { 1321 case 3575610: 1322 /* type */ return new String[] { "code" }; 1323 case 3059181: 1324 /* code */ return new String[] { "CodeableConcept" }; 1325 case -1282148017: 1326 /* factor */ return new String[] { "decimal" }; 1327 case -1413853096: 1328 /* amount */ return new String[] { "Money" }; 1329 default: 1330 return super.getTypesForProperty(hash, name); 1331 } 1332 1333 } 1334 1335 @Override 1336 public Base addChild(String name) throws FHIRException { 1337 if (name.equals("type")) { 1338 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.type"); 1339 } else if (name.equals("code")) { 1340 this.code = new CodeableConcept(); 1341 return this.code; 1342 } else if (name.equals("factor")) { 1343 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.factor"); 1344 } else if (name.equals("amount")) { 1345 this.amount = new Money(); 1346 return this.amount; 1347 } else 1348 return super.addChild(name); 1349 } 1350 1351 public ChargeItemDefinitionPropertyGroupPriceComponentComponent copy() { 1352 ChargeItemDefinitionPropertyGroupPriceComponentComponent dst = new ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 1353 copyValues(dst); 1354 return dst; 1355 } 1356 1357 public void copyValues(ChargeItemDefinitionPropertyGroupPriceComponentComponent dst) { 1358 super.copyValues(dst); 1359 dst.type = type == null ? null : type.copy(); 1360 dst.code = code == null ? null : code.copy(); 1361 dst.factor = factor == null ? null : factor.copy(); 1362 dst.amount = amount == null ? null : amount.copy(); 1363 } 1364 1365 @Override 1366 public boolean equalsDeep(Base other_) { 1367 if (!super.equalsDeep(other_)) 1368 return false; 1369 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupPriceComponentComponent)) 1370 return false; 1371 ChargeItemDefinitionPropertyGroupPriceComponentComponent o = (ChargeItemDefinitionPropertyGroupPriceComponentComponent) other_; 1372 return compareDeep(type, o.type, true) && compareDeep(code, o.code, true) && compareDeep(factor, o.factor, true) 1373 && compareDeep(amount, o.amount, true); 1374 } 1375 1376 @Override 1377 public boolean equalsShallow(Base other_) { 1378 if (!super.equalsShallow(other_)) 1379 return false; 1380 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupPriceComponentComponent)) 1381 return false; 1382 ChargeItemDefinitionPropertyGroupPriceComponentComponent o = (ChargeItemDefinitionPropertyGroupPriceComponentComponent) other_; 1383 return compareValues(type, o.type, true) && compareValues(factor, o.factor, true); 1384 } 1385 1386 public boolean isEmpty() { 1387 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, code, factor, amount); 1388 } 1389 1390 public String fhirType() { 1391 return "ChargeItemDefinition.propertyGroup.priceComponent"; 1392 1393 } 1394 1395 } 1396 1397 /** 1398 * A formal identifier that is used to identify this charge item definition when 1399 * it is represented in other formats, or referenced in a specification, model, 1400 * design or an instance. 1401 */ 1402 @Child(name = "identifier", type = { 1403 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1404 @Description(shortDefinition = "Additional identifier for the charge item definition", formalDefinition = "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1405 protected List<Identifier> identifier; 1406 1407 /** 1408 * The URL pointing to an externally-defined charge item definition that is 1409 * adhered to in whole or in part by this definition. 1410 */ 1411 @Child(name = "derivedFromUri", type = { 1412 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1413 @Description(shortDefinition = "Underlying externally-defined charge item definition", formalDefinition = "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.") 1414 protected List<UriType> derivedFromUri; 1415 1416 /** 1417 * A larger definition of which this particular definition is a component or 1418 * step. 1419 */ 1420 @Child(name = "partOf", type = { 1421 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1422 @Description(shortDefinition = "A larger definition of which this particular definition is a component or step", formalDefinition = "A larger definition of which this particular definition is a component or step.") 1423 protected List<CanonicalType> partOf; 1424 1425 /** 1426 * As new versions of a protocol or guideline are defined, allows identification 1427 * of what versions are replaced by a new instance. 1428 */ 1429 @Child(name = "replaces", type = { 1430 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1431 @Description(shortDefinition = "Completed or terminated request(s) whose function is taken by this new request", formalDefinition = "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.") 1432 protected List<CanonicalType> replaces; 1433 1434 /** 1435 * A copyright statement relating to the charge item definition and/or its 1436 * contents. Copyright statements are generally legal restrictions on the use 1437 * and publishing of the charge item definition. 1438 */ 1439 @Child(name = "copyright", type = { 1440 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1441 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.") 1442 protected MarkdownType copyright; 1443 1444 /** 1445 * The date on which the resource content was approved by the publisher. 1446 * Approval happens once when the content is officially approved for usage. 1447 */ 1448 @Child(name = "approvalDate", type = { 1449 DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1450 @Description(shortDefinition = "When the charge item definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1451 protected DateType approvalDate; 1452 1453 /** 1454 * The date on which the resource content was last reviewed. Review happens 1455 * periodically after approval but does not change the original approval date. 1456 */ 1457 @Child(name = "lastReviewDate", type = { 1458 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1459 @Description(shortDefinition = "When the charge item definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1460 protected DateType lastReviewDate; 1461 1462 /** 1463 * The period during which the charge item definition content was or is planned 1464 * to be in active use. 1465 */ 1466 @Child(name = "effectivePeriod", type = { 1467 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1468 @Description(shortDefinition = "When the charge item definition is expected to be used", formalDefinition = "The period during which the charge item definition content was or is planned to be in active use.") 1469 protected Period effectivePeriod; 1470 1471 /** 1472 * The defined billing details in this resource pertain to the given billing 1473 * code. 1474 */ 1475 @Child(name = "code", type = { CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1476 @Description(shortDefinition = "Billing codes or product types this definition applies to", formalDefinition = "The defined billing details in this resource pertain to the given billing code.") 1477 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 1478 protected CodeableConcept code; 1479 1480 /** 1481 * The defined billing details in this resource pertain to the given product 1482 * instance(s). 1483 */ 1484 @Child(name = "instance", type = { Medication.class, Substance.class, 1485 Device.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1486 @Description(shortDefinition = "Instances this definition applies to", formalDefinition = "The defined billing details in this resource pertain to the given product instance(s).") 1487 protected List<Reference> instance; 1488 /** 1489 * The actual objects that are the target of the reference (The defined billing 1490 * details in this resource pertain to the given product instance(s).) 1491 */ 1492 protected List<Resource> instanceTarget; 1493 1494 /** 1495 * Expressions that describe applicability criteria for the billing code. 1496 */ 1497 @Child(name = "applicability", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1498 @Description(shortDefinition = "Whether or not the billing code is applicable", formalDefinition = "Expressions that describe applicability criteria for the billing code.") 1499 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 1500 1501 /** 1502 * Group of properties which are applicable under the same conditions. If no 1503 * applicability rules are established for the group, then all properties always 1504 * apply. 1505 */ 1506 @Child(name = "propertyGroup", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1507 @Description(shortDefinition = "Group of properties which are applicable under the same conditions", formalDefinition = "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.") 1508 protected List<ChargeItemDefinitionPropertyGroupComponent> propertyGroup; 1509 1510 private static final long serialVersionUID = -583681330L; 1511 1512 /** 1513 * Constructor 1514 */ 1515 public ChargeItemDefinition() { 1516 super(); 1517 } 1518 1519 /** 1520 * Constructor 1521 */ 1522 public ChargeItemDefinition(UriType url, Enumeration<PublicationStatus> status) { 1523 super(); 1524 this.url = url; 1525 this.status = status; 1526 } 1527 1528 /** 1529 * @return {@link #url} (An absolute URI that is used to identify this charge 1530 * item definition when it is referenced in a specification, model, 1531 * design or an instance; also called its canonical identifier. This 1532 * SHOULD be globally unique and SHOULD be a literal address at which at 1533 * which an authoritative instance of this charge item definition is (or 1534 * will be) published. This URL can be the target of a canonical 1535 * reference. It SHALL remain the same when the charge item definition 1536 * is stored on different servers.). This is the underlying object with 1537 * id, value and extensions. The accessor "getUrl" gives direct access 1538 * to the value 1539 */ 1540 public UriType getUrlElement() { 1541 if (this.url == null) 1542 if (Configuration.errorOnAutoCreate()) 1543 throw new Error("Attempt to auto-create ChargeItemDefinition.url"); 1544 else if (Configuration.doAutoCreate()) 1545 this.url = new UriType(); // bb 1546 return this.url; 1547 } 1548 1549 public boolean hasUrlElement() { 1550 return this.url != null && !this.url.isEmpty(); 1551 } 1552 1553 public boolean hasUrl() { 1554 return this.url != null && !this.url.isEmpty(); 1555 } 1556 1557 /** 1558 * @param value {@link #url} (An absolute URI that is used to identify this 1559 * charge item definition when it is referenced in a specification, 1560 * model, design or an instance; also called its canonical 1561 * identifier. This SHOULD be globally unique and SHOULD be a 1562 * literal address at which at which an authoritative instance of 1563 * this charge item definition is (or will be) published. This URL 1564 * can be the target of a canonical reference. It SHALL remain the 1565 * same when the charge item definition is stored on different 1566 * servers.). This is the underlying object with id, value and 1567 * extensions. The accessor "getUrl" gives direct access to the 1568 * value 1569 */ 1570 public ChargeItemDefinition setUrlElement(UriType value) { 1571 this.url = value; 1572 return this; 1573 } 1574 1575 /** 1576 * @return An absolute URI that is used to identify this charge item definition 1577 * when it is referenced in a specification, model, design or an 1578 * instance; also called its canonical identifier. This SHOULD be 1579 * globally unique and SHOULD be a literal address at which at which an 1580 * authoritative instance of this charge item definition is (or will be) 1581 * published. This URL can be the target of a canonical reference. It 1582 * SHALL remain the same when the charge item definition is stored on 1583 * different servers. 1584 */ 1585 public String getUrl() { 1586 return this.url == null ? null : this.url.getValue(); 1587 } 1588 1589 /** 1590 * @param value An absolute URI that is used to identify this charge item 1591 * definition when it is referenced in a specification, model, 1592 * design or an instance; also called its canonical identifier. 1593 * This SHOULD be globally unique and SHOULD be a literal address 1594 * at which at which an authoritative instance of this charge item 1595 * definition is (or will be) published. This URL can be the target 1596 * of a canonical reference. It SHALL remain the same when the 1597 * charge item definition is stored on different servers. 1598 */ 1599 public ChargeItemDefinition setUrl(String value) { 1600 if (this.url == null) 1601 this.url = new UriType(); 1602 this.url.setValue(value); 1603 return this; 1604 } 1605 1606 /** 1607 * @return {@link #identifier} (A formal identifier that is used to identify 1608 * this charge item definition when it is represented in other formats, 1609 * or referenced in a specification, model, design or an instance.) 1610 */ 1611 public List<Identifier> getIdentifier() { 1612 if (this.identifier == null) 1613 this.identifier = new ArrayList<Identifier>(); 1614 return this.identifier; 1615 } 1616 1617 /** 1618 * @return Returns a reference to <code>this</code> for easy method chaining 1619 */ 1620 public ChargeItemDefinition setIdentifier(List<Identifier> theIdentifier) { 1621 this.identifier = theIdentifier; 1622 return this; 1623 } 1624 1625 public boolean hasIdentifier() { 1626 if (this.identifier == null) 1627 return false; 1628 for (Identifier item : this.identifier) 1629 if (!item.isEmpty()) 1630 return true; 1631 return false; 1632 } 1633 1634 public Identifier addIdentifier() { // 3 1635 Identifier t = new Identifier(); 1636 if (this.identifier == null) 1637 this.identifier = new ArrayList<Identifier>(); 1638 this.identifier.add(t); 1639 return t; 1640 } 1641 1642 public ChargeItemDefinition addIdentifier(Identifier t) { // 3 1643 if (t == null) 1644 return this; 1645 if (this.identifier == null) 1646 this.identifier = new ArrayList<Identifier>(); 1647 this.identifier.add(t); 1648 return this; 1649 } 1650 1651 /** 1652 * @return The first repetition of repeating field {@link #identifier}, creating 1653 * it if it does not already exist 1654 */ 1655 public Identifier getIdentifierFirstRep() { 1656 if (getIdentifier().isEmpty()) { 1657 addIdentifier(); 1658 } 1659 return getIdentifier().get(0); 1660 } 1661 1662 /** 1663 * @return {@link #version} (The identifier that is used to identify this 1664 * version of the charge item definition when it is referenced in a 1665 * specification, model, design or instance. This is an arbitrary value 1666 * managed by the charge item definition author and is not expected to 1667 * be globally unique. For example, it might be a timestamp (e.g. 1668 * yyyymmdd) if a managed version is not available. There is also no 1669 * expectation that versions can be placed in a lexicographical 1670 * sequence. To provide a version consistent with the Decision Support 1671 * Service specification, use the format Major.Minor.Revision (e.g. 1672 * 1.0.0). For more information on versioning knowledge assets, refer to 1673 * the Decision Support Service specification. Note that a version is 1674 * required for non-experimental active assets.). This is the underlying 1675 * object with id, value and extensions. The accessor "getVersion" gives 1676 * direct access to the value 1677 */ 1678 public StringType getVersionElement() { 1679 if (this.version == null) 1680 if (Configuration.errorOnAutoCreate()) 1681 throw new Error("Attempt to auto-create ChargeItemDefinition.version"); 1682 else if (Configuration.doAutoCreate()) 1683 this.version = new StringType(); // bb 1684 return this.version; 1685 } 1686 1687 public boolean hasVersionElement() { 1688 return this.version != null && !this.version.isEmpty(); 1689 } 1690 1691 public boolean hasVersion() { 1692 return this.version != null && !this.version.isEmpty(); 1693 } 1694 1695 /** 1696 * @param value {@link #version} (The identifier that is used to identify this 1697 * version of the charge item definition when it is referenced in a 1698 * specification, model, design or instance. This is an arbitrary 1699 * value managed by the charge item definition author and is not 1700 * expected to be globally unique. For example, it might be a 1701 * timestamp (e.g. yyyymmdd) if a managed version is not available. 1702 * There is also no expectation that versions can be placed in a 1703 * lexicographical sequence. To provide a version consistent with 1704 * the Decision Support Service specification, use the format 1705 * Major.Minor.Revision (e.g. 1.0.0). For more information on 1706 * versioning knowledge assets, refer to the Decision Support 1707 * Service specification. Note that a version is required for 1708 * non-experimental active assets.). This is the underlying object 1709 * with id, value and extensions. The accessor "getVersion" gives 1710 * direct access to the value 1711 */ 1712 public ChargeItemDefinition setVersionElement(StringType value) { 1713 this.version = value; 1714 return this; 1715 } 1716 1717 /** 1718 * @return The identifier that is used to identify this version of the charge 1719 * item definition when it is referenced in a specification, model, 1720 * design or instance. This is an arbitrary value managed by the charge 1721 * item definition author and is not expected to be globally unique. For 1722 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 1723 * is not available. There is also no expectation that versions can be 1724 * placed in a lexicographical sequence. To provide a version consistent 1725 * with the Decision Support Service specification, use the format 1726 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 1727 * knowledge assets, refer to the Decision Support Service 1728 * specification. Note that a version is required for non-experimental 1729 * active assets. 1730 */ 1731 public String getVersion() { 1732 return this.version == null ? null : this.version.getValue(); 1733 } 1734 1735 /** 1736 * @param value The identifier that is used to identify this version of the 1737 * charge item definition when it is referenced in a specification, 1738 * model, design or instance. This is an arbitrary value managed by 1739 * the charge item definition author and is not expected to be 1740 * globally unique. For example, it might be a timestamp (e.g. 1741 * yyyymmdd) if a managed version is not available. There is also 1742 * no expectation that versions can be placed in a lexicographical 1743 * sequence. To provide a version consistent with the Decision 1744 * Support Service specification, use the format 1745 * Major.Minor.Revision (e.g. 1.0.0). For more information on 1746 * versioning knowledge assets, refer to the Decision Support 1747 * Service specification. Note that a version is required for 1748 * non-experimental active assets. 1749 */ 1750 public ChargeItemDefinition setVersion(String value) { 1751 if (Utilities.noString(value)) 1752 this.version = null; 1753 else { 1754 if (this.version == null) 1755 this.version = new StringType(); 1756 this.version.setValue(value); 1757 } 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #title} (A short, descriptive, user-friendly title for the 1763 * charge item definition.). This is the underlying object with id, 1764 * value and extensions. The accessor "getTitle" gives direct access to 1765 * the value 1766 */ 1767 public StringType getTitleElement() { 1768 if (this.title == null) 1769 if (Configuration.errorOnAutoCreate()) 1770 throw new Error("Attempt to auto-create ChargeItemDefinition.title"); 1771 else if (Configuration.doAutoCreate()) 1772 this.title = new StringType(); // bb 1773 return this.title; 1774 } 1775 1776 public boolean hasTitleElement() { 1777 return this.title != null && !this.title.isEmpty(); 1778 } 1779 1780 public boolean hasTitle() { 1781 return this.title != null && !this.title.isEmpty(); 1782 } 1783 1784 /** 1785 * @param value {@link #title} (A short, descriptive, user-friendly title for 1786 * the charge item definition.). This is the underlying object with 1787 * id, value and extensions. The accessor "getTitle" gives direct 1788 * access to the value 1789 */ 1790 public ChargeItemDefinition setTitleElement(StringType value) { 1791 this.title = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return A short, descriptive, user-friendly title for the charge item 1797 * definition. 1798 */ 1799 public String getTitle() { 1800 return this.title == null ? null : this.title.getValue(); 1801 } 1802 1803 /** 1804 * @param value A short, descriptive, user-friendly title for the charge item 1805 * definition. 1806 */ 1807 public ChargeItemDefinition setTitle(String value) { 1808 if (Utilities.noString(value)) 1809 this.title = null; 1810 else { 1811 if (this.title == null) 1812 this.title = new StringType(); 1813 this.title.setValue(value); 1814 } 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined 1820 * charge item definition that is adhered to in whole or in part by this 1821 * definition.) 1822 */ 1823 public List<UriType> getDerivedFromUri() { 1824 if (this.derivedFromUri == null) 1825 this.derivedFromUri = new ArrayList<UriType>(); 1826 return this.derivedFromUri; 1827 } 1828 1829 /** 1830 * @return Returns a reference to <code>this</code> for easy method chaining 1831 */ 1832 public ChargeItemDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 1833 this.derivedFromUri = theDerivedFromUri; 1834 return this; 1835 } 1836 1837 public boolean hasDerivedFromUri() { 1838 if (this.derivedFromUri == null) 1839 return false; 1840 for (UriType item : this.derivedFromUri) 1841 if (!item.isEmpty()) 1842 return true; 1843 return false; 1844 } 1845 1846 /** 1847 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined 1848 * charge item definition that is adhered to in whole or in part by this 1849 * definition.) 1850 */ 1851 public UriType addDerivedFromUriElement() {// 2 1852 UriType t = new UriType(); 1853 if (this.derivedFromUri == null) 1854 this.derivedFromUri = new ArrayList<UriType>(); 1855 this.derivedFromUri.add(t); 1856 return t; 1857 } 1858 1859 /** 1860 * @param value {@link #derivedFromUri} (The URL pointing to an 1861 * externally-defined charge item definition that is adhered to in 1862 * whole or in part by this definition.) 1863 */ 1864 public ChargeItemDefinition addDerivedFromUri(String value) { // 1 1865 UriType t = new UriType(); 1866 t.setValue(value); 1867 if (this.derivedFromUri == null) 1868 this.derivedFromUri = new ArrayList<UriType>(); 1869 this.derivedFromUri.add(t); 1870 return this; 1871 } 1872 1873 /** 1874 * @param value {@link #derivedFromUri} (The URL pointing to an 1875 * externally-defined charge item definition that is adhered to in 1876 * whole or in part by this definition.) 1877 */ 1878 public boolean hasDerivedFromUri(String value) { 1879 if (this.derivedFromUri == null) 1880 return false; 1881 for (UriType v : this.derivedFromUri) 1882 if (v.getValue().equals(value)) // uri 1883 return true; 1884 return false; 1885 } 1886 1887 /** 1888 * @return {@link #partOf} (A larger definition of which this particular 1889 * definition is a component or step.) 1890 */ 1891 public List<CanonicalType> getPartOf() { 1892 if (this.partOf == null) 1893 this.partOf = new ArrayList<CanonicalType>(); 1894 return this.partOf; 1895 } 1896 1897 /** 1898 * @return Returns a reference to <code>this</code> for easy method chaining 1899 */ 1900 public ChargeItemDefinition setPartOf(List<CanonicalType> thePartOf) { 1901 this.partOf = thePartOf; 1902 return this; 1903 } 1904 1905 public boolean hasPartOf() { 1906 if (this.partOf == null) 1907 return false; 1908 for (CanonicalType item : this.partOf) 1909 if (!item.isEmpty()) 1910 return true; 1911 return false; 1912 } 1913 1914 /** 1915 * @return {@link #partOf} (A larger definition of which this particular 1916 * definition is a component or step.) 1917 */ 1918 public CanonicalType addPartOfElement() {// 2 1919 CanonicalType t = new CanonicalType(); 1920 if (this.partOf == null) 1921 this.partOf = new ArrayList<CanonicalType>(); 1922 this.partOf.add(t); 1923 return t; 1924 } 1925 1926 /** 1927 * @param value {@link #partOf} (A larger definition of which this particular 1928 * definition is a component or step.) 1929 */ 1930 public ChargeItemDefinition addPartOf(String value) { // 1 1931 CanonicalType t = new CanonicalType(); 1932 t.setValue(value); 1933 if (this.partOf == null) 1934 this.partOf = new ArrayList<CanonicalType>(); 1935 this.partOf.add(t); 1936 return this; 1937 } 1938 1939 /** 1940 * @param value {@link #partOf} (A larger definition of which this particular 1941 * definition is a component or step.) 1942 */ 1943 public boolean hasPartOf(String value) { 1944 if (this.partOf == null) 1945 return false; 1946 for (CanonicalType v : this.partOf) 1947 if (v.getValue().equals(value)) // canonical(ChargeItemDefinition) 1948 return true; 1949 return false; 1950 } 1951 1952 /** 1953 * @return {@link #replaces} (As new versions of a protocol or guideline are 1954 * defined, allows identification of what versions are replaced by a new 1955 * instance.) 1956 */ 1957 public List<CanonicalType> getReplaces() { 1958 if (this.replaces == null) 1959 this.replaces = new ArrayList<CanonicalType>(); 1960 return this.replaces; 1961 } 1962 1963 /** 1964 * @return Returns a reference to <code>this</code> for easy method chaining 1965 */ 1966 public ChargeItemDefinition setReplaces(List<CanonicalType> theReplaces) { 1967 this.replaces = theReplaces; 1968 return this; 1969 } 1970 1971 public boolean hasReplaces() { 1972 if (this.replaces == null) 1973 return false; 1974 for (CanonicalType item : this.replaces) 1975 if (!item.isEmpty()) 1976 return true; 1977 return false; 1978 } 1979 1980 /** 1981 * @return {@link #replaces} (As new versions of a protocol or guideline are 1982 * defined, allows identification of what versions are replaced by a new 1983 * instance.) 1984 */ 1985 public CanonicalType addReplacesElement() {// 2 1986 CanonicalType t = new CanonicalType(); 1987 if (this.replaces == null) 1988 this.replaces = new ArrayList<CanonicalType>(); 1989 this.replaces.add(t); 1990 return t; 1991 } 1992 1993 /** 1994 * @param value {@link #replaces} (As new versions of a protocol or guideline 1995 * are defined, allows identification of what versions are replaced 1996 * by a new instance.) 1997 */ 1998 public ChargeItemDefinition addReplaces(String value) { // 1 1999 CanonicalType t = new CanonicalType(); 2000 t.setValue(value); 2001 if (this.replaces == null) 2002 this.replaces = new ArrayList<CanonicalType>(); 2003 this.replaces.add(t); 2004 return this; 2005 } 2006 2007 /** 2008 * @param value {@link #replaces} (As new versions of a protocol or guideline 2009 * are defined, allows identification of what versions are replaced 2010 * by a new instance.) 2011 */ 2012 public boolean hasReplaces(String value) { 2013 if (this.replaces == null) 2014 return false; 2015 for (CanonicalType v : this.replaces) 2016 if (v.getValue().equals(value)) // canonical(ChargeItemDefinition) 2017 return true; 2018 return false; 2019 } 2020 2021 /** 2022 * @return {@link #status} (The current state of the ChargeItemDefinition.). 2023 * This is the underlying object with id, value and extensions. The 2024 * accessor "getStatus" gives direct access to the value 2025 */ 2026 public Enumeration<PublicationStatus> getStatusElement() { 2027 if (this.status == null) 2028 if (Configuration.errorOnAutoCreate()) 2029 throw new Error("Attempt to auto-create ChargeItemDefinition.status"); 2030 else if (Configuration.doAutoCreate()) 2031 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2032 return this.status; 2033 } 2034 2035 public boolean hasStatusElement() { 2036 return this.status != null && !this.status.isEmpty(); 2037 } 2038 2039 public boolean hasStatus() { 2040 return this.status != null && !this.status.isEmpty(); 2041 } 2042 2043 /** 2044 * @param value {@link #status} (The current state of the 2045 * ChargeItemDefinition.). This is the underlying object with id, 2046 * value and extensions. The accessor "getStatus" gives direct 2047 * access to the value 2048 */ 2049 public ChargeItemDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2050 this.status = value; 2051 return this; 2052 } 2053 2054 /** 2055 * @return The current state of the ChargeItemDefinition. 2056 */ 2057 public PublicationStatus getStatus() { 2058 return this.status == null ? null : this.status.getValue(); 2059 } 2060 2061 /** 2062 * @param value The current state of the ChargeItemDefinition. 2063 */ 2064 public ChargeItemDefinition setStatus(PublicationStatus value) { 2065 if (this.status == null) 2066 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2067 this.status.setValue(value); 2068 return this; 2069 } 2070 2071 /** 2072 * @return {@link #experimental} (A Boolean value to indicate that this charge 2073 * item definition is authored for testing purposes (or 2074 * education/evaluation/marketing) and is not intended to be used for 2075 * genuine usage.). This is the underlying object with id, value and 2076 * extensions. The accessor "getExperimental" gives direct access to the 2077 * value 2078 */ 2079 public BooleanType getExperimentalElement() { 2080 if (this.experimental == null) 2081 if (Configuration.errorOnAutoCreate()) 2082 throw new Error("Attempt to auto-create ChargeItemDefinition.experimental"); 2083 else if (Configuration.doAutoCreate()) 2084 this.experimental = new BooleanType(); // bb 2085 return this.experimental; 2086 } 2087 2088 public boolean hasExperimentalElement() { 2089 return this.experimental != null && !this.experimental.isEmpty(); 2090 } 2091 2092 public boolean hasExperimental() { 2093 return this.experimental != null && !this.experimental.isEmpty(); 2094 } 2095 2096 /** 2097 * @param value {@link #experimental} (A Boolean value to indicate that this 2098 * charge item definition is authored for testing purposes (or 2099 * education/evaluation/marketing) and is not intended to be used 2100 * for genuine usage.). This is the underlying object with id, 2101 * value and extensions. The accessor "getExperimental" gives 2102 * direct access to the value 2103 */ 2104 public ChargeItemDefinition setExperimentalElement(BooleanType value) { 2105 this.experimental = value; 2106 return this; 2107 } 2108 2109 /** 2110 * @return A Boolean value to indicate that this charge item definition is 2111 * authored for testing purposes (or education/evaluation/marketing) and 2112 * is not intended to be used for genuine usage. 2113 */ 2114 public boolean getExperimental() { 2115 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2116 } 2117 2118 /** 2119 * @param value A Boolean value to indicate that this charge item definition is 2120 * authored for testing purposes (or 2121 * education/evaluation/marketing) and is not intended to be used 2122 * for genuine usage. 2123 */ 2124 public ChargeItemDefinition setExperimental(boolean value) { 2125 if (this.experimental == null) 2126 this.experimental = new BooleanType(); 2127 this.experimental.setValue(value); 2128 return this; 2129 } 2130 2131 /** 2132 * @return {@link #date} (The date (and optionally time) when the charge item 2133 * definition was published. The date must change when the business 2134 * version changes and it must change if the status code changes. In 2135 * addition, it should change when the substantive content of the charge 2136 * item definition changes.). This is the underlying object with id, 2137 * value and extensions. The accessor "getDate" gives direct access to 2138 * the value 2139 */ 2140 public DateTimeType getDateElement() { 2141 if (this.date == null) 2142 if (Configuration.errorOnAutoCreate()) 2143 throw new Error("Attempt to auto-create ChargeItemDefinition.date"); 2144 else if (Configuration.doAutoCreate()) 2145 this.date = new DateTimeType(); // bb 2146 return this.date; 2147 } 2148 2149 public boolean hasDateElement() { 2150 return this.date != null && !this.date.isEmpty(); 2151 } 2152 2153 public boolean hasDate() { 2154 return this.date != null && !this.date.isEmpty(); 2155 } 2156 2157 /** 2158 * @param value {@link #date} (The date (and optionally time) when the charge 2159 * item definition was published. The date must change when the 2160 * business version changes and it must change if the status code 2161 * changes. In addition, it should change when the substantive 2162 * content of the charge item definition changes.). This is the 2163 * underlying object with id, value and extensions. The accessor 2164 * "getDate" gives direct access to the value 2165 */ 2166 public ChargeItemDefinition setDateElement(DateTimeType value) { 2167 this.date = value; 2168 return this; 2169 } 2170 2171 /** 2172 * @return The date (and optionally time) when the charge item definition was 2173 * published. The date must change when the business version changes and 2174 * it must change if the status code changes. In addition, it should 2175 * change when the substantive content of the charge item definition 2176 * changes. 2177 */ 2178 public Date getDate() { 2179 return this.date == null ? null : this.date.getValue(); 2180 } 2181 2182 /** 2183 * @param value The date (and optionally time) when the charge item definition 2184 * was published. The date must change when the business version 2185 * changes and it must change if the status code changes. In 2186 * addition, it should change when the substantive content of the 2187 * charge item definition changes. 2188 */ 2189 public ChargeItemDefinition setDate(Date value) { 2190 if (value == null) 2191 this.date = null; 2192 else { 2193 if (this.date == null) 2194 this.date = new DateTimeType(); 2195 this.date.setValue(value); 2196 } 2197 return this; 2198 } 2199 2200 /** 2201 * @return {@link #publisher} (The name of the organization or individual that 2202 * published the charge item definition.). This is the underlying object 2203 * with id, value and extensions. The accessor "getPublisher" gives 2204 * direct access to the value 2205 */ 2206 public StringType getPublisherElement() { 2207 if (this.publisher == null) 2208 if (Configuration.errorOnAutoCreate()) 2209 throw new Error("Attempt to auto-create ChargeItemDefinition.publisher"); 2210 else if (Configuration.doAutoCreate()) 2211 this.publisher = new StringType(); // bb 2212 return this.publisher; 2213 } 2214 2215 public boolean hasPublisherElement() { 2216 return this.publisher != null && !this.publisher.isEmpty(); 2217 } 2218 2219 public boolean hasPublisher() { 2220 return this.publisher != null && !this.publisher.isEmpty(); 2221 } 2222 2223 /** 2224 * @param value {@link #publisher} (The name of the organization or individual 2225 * that published the charge item definition.). This is the 2226 * underlying object with id, value and extensions. The accessor 2227 * "getPublisher" gives direct access to the value 2228 */ 2229 public ChargeItemDefinition setPublisherElement(StringType value) { 2230 this.publisher = value; 2231 return this; 2232 } 2233 2234 /** 2235 * @return The name of the organization or individual that published the charge 2236 * item definition. 2237 */ 2238 public String getPublisher() { 2239 return this.publisher == null ? null : this.publisher.getValue(); 2240 } 2241 2242 /** 2243 * @param value The name of the organization or individual that published the 2244 * charge item definition. 2245 */ 2246 public ChargeItemDefinition setPublisher(String value) { 2247 if (Utilities.noString(value)) 2248 this.publisher = null; 2249 else { 2250 if (this.publisher == null) 2251 this.publisher = new StringType(); 2252 this.publisher.setValue(value); 2253 } 2254 return this; 2255 } 2256 2257 /** 2258 * @return {@link #contact} (Contact details to assist a user in finding and 2259 * communicating with the publisher.) 2260 */ 2261 public List<ContactDetail> getContact() { 2262 if (this.contact == null) 2263 this.contact = new ArrayList<ContactDetail>(); 2264 return this.contact; 2265 } 2266 2267 /** 2268 * @return Returns a reference to <code>this</code> for easy method chaining 2269 */ 2270 public ChargeItemDefinition setContact(List<ContactDetail> theContact) { 2271 this.contact = theContact; 2272 return this; 2273 } 2274 2275 public boolean hasContact() { 2276 if (this.contact == null) 2277 return false; 2278 for (ContactDetail item : this.contact) 2279 if (!item.isEmpty()) 2280 return true; 2281 return false; 2282 } 2283 2284 public ContactDetail addContact() { // 3 2285 ContactDetail t = new ContactDetail(); 2286 if (this.contact == null) 2287 this.contact = new ArrayList<ContactDetail>(); 2288 this.contact.add(t); 2289 return t; 2290 } 2291 2292 public ChargeItemDefinition addContact(ContactDetail t) { // 3 2293 if (t == null) 2294 return this; 2295 if (this.contact == null) 2296 this.contact = new ArrayList<ContactDetail>(); 2297 this.contact.add(t); 2298 return this; 2299 } 2300 2301 /** 2302 * @return The first repetition of repeating field {@link #contact}, creating it 2303 * if it does not already exist 2304 */ 2305 public ContactDetail getContactFirstRep() { 2306 if (getContact().isEmpty()) { 2307 addContact(); 2308 } 2309 return getContact().get(0); 2310 } 2311 2312 /** 2313 * @return {@link #description} (A free text natural language description of the 2314 * charge item definition from a consumer's perspective.). This is the 2315 * underlying object with id, value and extensions. The accessor 2316 * "getDescription" gives direct access to the value 2317 */ 2318 public MarkdownType getDescriptionElement() { 2319 if (this.description == null) 2320 if (Configuration.errorOnAutoCreate()) 2321 throw new Error("Attempt to auto-create ChargeItemDefinition.description"); 2322 else if (Configuration.doAutoCreate()) 2323 this.description = new MarkdownType(); // bb 2324 return this.description; 2325 } 2326 2327 public boolean hasDescriptionElement() { 2328 return this.description != null && !this.description.isEmpty(); 2329 } 2330 2331 public boolean hasDescription() { 2332 return this.description != null && !this.description.isEmpty(); 2333 } 2334 2335 /** 2336 * @param value {@link #description} (A free text natural language description 2337 * of the charge item definition from a consumer's perspective.). 2338 * This is the underlying object with id, value and extensions. The 2339 * accessor "getDescription" gives direct access to the value 2340 */ 2341 public ChargeItemDefinition setDescriptionElement(MarkdownType value) { 2342 this.description = value; 2343 return this; 2344 } 2345 2346 /** 2347 * @return A free text natural language description of the charge item 2348 * definition from a consumer's perspective. 2349 */ 2350 public String getDescription() { 2351 return this.description == null ? null : this.description.getValue(); 2352 } 2353 2354 /** 2355 * @param value A free text natural language description of the charge item 2356 * definition from a consumer's perspective. 2357 */ 2358 public ChargeItemDefinition setDescription(String value) { 2359 if (value == null) 2360 this.description = null; 2361 else { 2362 if (this.description == null) 2363 this.description = new MarkdownType(); 2364 this.description.setValue(value); 2365 } 2366 return this; 2367 } 2368 2369 /** 2370 * @return {@link #useContext} (The content was developed with a focus and 2371 * intent of supporting the contexts that are listed. These contexts may 2372 * be general categories (gender, age, ...) or may be references to 2373 * specific programs (insurance plans, studies, ...) and may be used to 2374 * assist with indexing and searching for appropriate charge item 2375 * definition instances.) 2376 */ 2377 public List<UsageContext> getUseContext() { 2378 if (this.useContext == null) 2379 this.useContext = new ArrayList<UsageContext>(); 2380 return this.useContext; 2381 } 2382 2383 /** 2384 * @return Returns a reference to <code>this</code> for easy method chaining 2385 */ 2386 public ChargeItemDefinition setUseContext(List<UsageContext> theUseContext) { 2387 this.useContext = theUseContext; 2388 return this; 2389 } 2390 2391 public boolean hasUseContext() { 2392 if (this.useContext == null) 2393 return false; 2394 for (UsageContext item : this.useContext) 2395 if (!item.isEmpty()) 2396 return true; 2397 return false; 2398 } 2399 2400 public UsageContext addUseContext() { // 3 2401 UsageContext t = new UsageContext(); 2402 if (this.useContext == null) 2403 this.useContext = new ArrayList<UsageContext>(); 2404 this.useContext.add(t); 2405 return t; 2406 } 2407 2408 public ChargeItemDefinition addUseContext(UsageContext t) { // 3 2409 if (t == null) 2410 return this; 2411 if (this.useContext == null) 2412 this.useContext = new ArrayList<UsageContext>(); 2413 this.useContext.add(t); 2414 return this; 2415 } 2416 2417 /** 2418 * @return The first repetition of repeating field {@link #useContext}, creating 2419 * it if it does not already exist 2420 */ 2421 public UsageContext getUseContextFirstRep() { 2422 if (getUseContext().isEmpty()) { 2423 addUseContext(); 2424 } 2425 return getUseContext().get(0); 2426 } 2427 2428 /** 2429 * @return {@link #jurisdiction} (A legal or geographic region in which the 2430 * charge item definition is intended to be used.) 2431 */ 2432 public List<CodeableConcept> getJurisdiction() { 2433 if (this.jurisdiction == null) 2434 this.jurisdiction = new ArrayList<CodeableConcept>(); 2435 return this.jurisdiction; 2436 } 2437 2438 /** 2439 * @return Returns a reference to <code>this</code> for easy method chaining 2440 */ 2441 public ChargeItemDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2442 this.jurisdiction = theJurisdiction; 2443 return this; 2444 } 2445 2446 public boolean hasJurisdiction() { 2447 if (this.jurisdiction == null) 2448 return false; 2449 for (CodeableConcept item : this.jurisdiction) 2450 if (!item.isEmpty()) 2451 return true; 2452 return false; 2453 } 2454 2455 public CodeableConcept addJurisdiction() { // 3 2456 CodeableConcept t = new CodeableConcept(); 2457 if (this.jurisdiction == null) 2458 this.jurisdiction = new ArrayList<CodeableConcept>(); 2459 this.jurisdiction.add(t); 2460 return t; 2461 } 2462 2463 public ChargeItemDefinition addJurisdiction(CodeableConcept t) { // 3 2464 if (t == null) 2465 return this; 2466 if (this.jurisdiction == null) 2467 this.jurisdiction = new ArrayList<CodeableConcept>(); 2468 this.jurisdiction.add(t); 2469 return this; 2470 } 2471 2472 /** 2473 * @return The first repetition of repeating field {@link #jurisdiction}, 2474 * creating it if it does not already exist 2475 */ 2476 public CodeableConcept getJurisdictionFirstRep() { 2477 if (getJurisdiction().isEmpty()) { 2478 addJurisdiction(); 2479 } 2480 return getJurisdiction().get(0); 2481 } 2482 2483 /** 2484 * @return {@link #copyright} (A copyright statement relating to the charge item 2485 * definition and/or its contents. Copyright statements are generally 2486 * legal restrictions on the use and publishing of the charge item 2487 * definition.). This is the underlying object with id, value and 2488 * extensions. The accessor "getCopyright" gives direct access to the 2489 * value 2490 */ 2491 public MarkdownType getCopyrightElement() { 2492 if (this.copyright == null) 2493 if (Configuration.errorOnAutoCreate()) 2494 throw new Error("Attempt to auto-create ChargeItemDefinition.copyright"); 2495 else if (Configuration.doAutoCreate()) 2496 this.copyright = new MarkdownType(); // bb 2497 return this.copyright; 2498 } 2499 2500 public boolean hasCopyrightElement() { 2501 return this.copyright != null && !this.copyright.isEmpty(); 2502 } 2503 2504 public boolean hasCopyright() { 2505 return this.copyright != null && !this.copyright.isEmpty(); 2506 } 2507 2508 /** 2509 * @param value {@link #copyright} (A copyright statement relating to the charge 2510 * item definition and/or its contents. Copyright statements are 2511 * generally legal restrictions on the use and publishing of the 2512 * charge item definition.). This is the underlying object with id, 2513 * value and extensions. The accessor "getCopyright" gives direct 2514 * access to the value 2515 */ 2516 public ChargeItemDefinition setCopyrightElement(MarkdownType value) { 2517 this.copyright = value; 2518 return this; 2519 } 2520 2521 /** 2522 * @return A copyright statement relating to the charge item definition and/or 2523 * its contents. Copyright statements are generally legal restrictions 2524 * on the use and publishing of the charge item definition. 2525 */ 2526 public String getCopyright() { 2527 return this.copyright == null ? null : this.copyright.getValue(); 2528 } 2529 2530 /** 2531 * @param value A copyright statement relating to the charge item definition 2532 * and/or its contents. Copyright statements are generally legal 2533 * restrictions on the use and publishing of the charge item 2534 * definition. 2535 */ 2536 public ChargeItemDefinition setCopyright(String value) { 2537 if (value == null) 2538 this.copyright = null; 2539 else { 2540 if (this.copyright == null) 2541 this.copyright = new MarkdownType(); 2542 this.copyright.setValue(value); 2543 } 2544 return this; 2545 } 2546 2547 /** 2548 * @return {@link #approvalDate} (The date on which the resource content was 2549 * approved by the publisher. Approval happens once when the content is 2550 * officially approved for usage.). This is the underlying object with 2551 * id, value and extensions. The accessor "getApprovalDate" gives direct 2552 * access to the value 2553 */ 2554 public DateType getApprovalDateElement() { 2555 if (this.approvalDate == null) 2556 if (Configuration.errorOnAutoCreate()) 2557 throw new Error("Attempt to auto-create ChargeItemDefinition.approvalDate"); 2558 else if (Configuration.doAutoCreate()) 2559 this.approvalDate = new DateType(); // bb 2560 return this.approvalDate; 2561 } 2562 2563 public boolean hasApprovalDateElement() { 2564 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2565 } 2566 2567 public boolean hasApprovalDate() { 2568 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2569 } 2570 2571 /** 2572 * @param value {@link #approvalDate} (The date on which the resource content 2573 * was approved by the publisher. Approval happens once when the 2574 * content is officially approved for usage.). This is the 2575 * underlying object with id, value and extensions. The accessor 2576 * "getApprovalDate" gives direct access to the value 2577 */ 2578 public ChargeItemDefinition setApprovalDateElement(DateType value) { 2579 this.approvalDate = value; 2580 return this; 2581 } 2582 2583 /** 2584 * @return The date on which the resource content was approved by the publisher. 2585 * Approval happens once when the content is officially approved for 2586 * usage. 2587 */ 2588 public Date getApprovalDate() { 2589 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2590 } 2591 2592 /** 2593 * @param value The date on which the resource content was approved by the 2594 * publisher. Approval happens once when the content is officially 2595 * approved for usage. 2596 */ 2597 public ChargeItemDefinition setApprovalDate(Date value) { 2598 if (value == null) 2599 this.approvalDate = null; 2600 else { 2601 if (this.approvalDate == null) 2602 this.approvalDate = new DateType(); 2603 this.approvalDate.setValue(value); 2604 } 2605 return this; 2606 } 2607 2608 /** 2609 * @return {@link #lastReviewDate} (The date on which the resource content was 2610 * last reviewed. Review happens periodically after approval but does 2611 * not change the original approval date.). This is the underlying 2612 * object with id, value and extensions. The accessor 2613 * "getLastReviewDate" gives direct access to the value 2614 */ 2615 public DateType getLastReviewDateElement() { 2616 if (this.lastReviewDate == null) 2617 if (Configuration.errorOnAutoCreate()) 2618 throw new Error("Attempt to auto-create ChargeItemDefinition.lastReviewDate"); 2619 else if (Configuration.doAutoCreate()) 2620 this.lastReviewDate = new DateType(); // bb 2621 return this.lastReviewDate; 2622 } 2623 2624 public boolean hasLastReviewDateElement() { 2625 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2626 } 2627 2628 public boolean hasLastReviewDate() { 2629 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2630 } 2631 2632 /** 2633 * @param value {@link #lastReviewDate} (The date on which the resource content 2634 * was last reviewed. Review happens periodically after approval 2635 * but does not change the original approval date.). This is the 2636 * underlying object with id, value and extensions. The accessor 2637 * "getLastReviewDate" gives direct access to the value 2638 */ 2639 public ChargeItemDefinition setLastReviewDateElement(DateType value) { 2640 this.lastReviewDate = value; 2641 return this; 2642 } 2643 2644 /** 2645 * @return The date on which the resource content was last reviewed. Review 2646 * happens periodically after approval but does not change the original 2647 * approval date. 2648 */ 2649 public Date getLastReviewDate() { 2650 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2651 } 2652 2653 /** 2654 * @param value The date on which the resource content was last reviewed. Review 2655 * happens periodically after approval but does not change the 2656 * original approval date. 2657 */ 2658 public ChargeItemDefinition setLastReviewDate(Date value) { 2659 if (value == null) 2660 this.lastReviewDate = null; 2661 else { 2662 if (this.lastReviewDate == null) 2663 this.lastReviewDate = new DateType(); 2664 this.lastReviewDate.setValue(value); 2665 } 2666 return this; 2667 } 2668 2669 /** 2670 * @return {@link #effectivePeriod} (The period during which the charge item 2671 * definition content was or is planned to be in active use.) 2672 */ 2673 public Period getEffectivePeriod() { 2674 if (this.effectivePeriod == null) 2675 if (Configuration.errorOnAutoCreate()) 2676 throw new Error("Attempt to auto-create ChargeItemDefinition.effectivePeriod"); 2677 else if (Configuration.doAutoCreate()) 2678 this.effectivePeriod = new Period(); // cc 2679 return this.effectivePeriod; 2680 } 2681 2682 public boolean hasEffectivePeriod() { 2683 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2684 } 2685 2686 /** 2687 * @param value {@link #effectivePeriod} (The period during which the charge 2688 * item definition content was or is planned to be in active use.) 2689 */ 2690 public ChargeItemDefinition setEffectivePeriod(Period value) { 2691 this.effectivePeriod = value; 2692 return this; 2693 } 2694 2695 /** 2696 * @return {@link #code} (The defined billing details in this resource pertain 2697 * to the given billing code.) 2698 */ 2699 public CodeableConcept getCode() { 2700 if (this.code == null) 2701 if (Configuration.errorOnAutoCreate()) 2702 throw new Error("Attempt to auto-create ChargeItemDefinition.code"); 2703 else if (Configuration.doAutoCreate()) 2704 this.code = new CodeableConcept(); // cc 2705 return this.code; 2706 } 2707 2708 public boolean hasCode() { 2709 return this.code != null && !this.code.isEmpty(); 2710 } 2711 2712 /** 2713 * @param value {@link #code} (The defined billing details in this resource 2714 * pertain to the given billing code.) 2715 */ 2716 public ChargeItemDefinition setCode(CodeableConcept value) { 2717 this.code = value; 2718 return this; 2719 } 2720 2721 /** 2722 * @return {@link #instance} (The defined billing details in this resource 2723 * pertain to the given product instance(s).) 2724 */ 2725 public List<Reference> getInstance() { 2726 if (this.instance == null) 2727 this.instance = new ArrayList<Reference>(); 2728 return this.instance; 2729 } 2730 2731 /** 2732 * @return Returns a reference to <code>this</code> for easy method chaining 2733 */ 2734 public ChargeItemDefinition setInstance(List<Reference> theInstance) { 2735 this.instance = theInstance; 2736 return this; 2737 } 2738 2739 public boolean hasInstance() { 2740 if (this.instance == null) 2741 return false; 2742 for (Reference item : this.instance) 2743 if (!item.isEmpty()) 2744 return true; 2745 return false; 2746 } 2747 2748 public Reference addInstance() { // 3 2749 Reference t = new Reference(); 2750 if (this.instance == null) 2751 this.instance = new ArrayList<Reference>(); 2752 this.instance.add(t); 2753 return t; 2754 } 2755 2756 public ChargeItemDefinition addInstance(Reference t) { // 3 2757 if (t == null) 2758 return this; 2759 if (this.instance == null) 2760 this.instance = new ArrayList<Reference>(); 2761 this.instance.add(t); 2762 return this; 2763 } 2764 2765 /** 2766 * @return The first repetition of repeating field {@link #instance}, creating 2767 * it if it does not already exist 2768 */ 2769 public Reference getInstanceFirstRep() { 2770 if (getInstance().isEmpty()) { 2771 addInstance(); 2772 } 2773 return getInstance().get(0); 2774 } 2775 2776 /** 2777 * @deprecated Use Reference#setResource(IBaseResource) instead 2778 */ 2779 @Deprecated 2780 public List<Resource> getInstanceTarget() { 2781 if (this.instanceTarget == null) 2782 this.instanceTarget = new ArrayList<Resource>(); 2783 return this.instanceTarget; 2784 } 2785 2786 /** 2787 * @return {@link #applicability} (Expressions that describe applicability 2788 * criteria for the billing code.) 2789 */ 2790 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 2791 if (this.applicability == null) 2792 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2793 return this.applicability; 2794 } 2795 2796 /** 2797 * @return Returns a reference to <code>this</code> for easy method chaining 2798 */ 2799 public ChargeItemDefinition setApplicability(List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 2800 this.applicability = theApplicability; 2801 return this; 2802 } 2803 2804 public boolean hasApplicability() { 2805 if (this.applicability == null) 2806 return false; 2807 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 2808 if (!item.isEmpty()) 2809 return true; 2810 return false; 2811 } 2812 2813 public ChargeItemDefinitionApplicabilityComponent addApplicability() { // 3 2814 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 2815 if (this.applicability == null) 2816 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2817 this.applicability.add(t); 2818 return t; 2819 } 2820 2821 public ChargeItemDefinition addApplicability(ChargeItemDefinitionApplicabilityComponent t) { // 3 2822 if (t == null) 2823 return this; 2824 if (this.applicability == null) 2825 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2826 this.applicability.add(t); 2827 return this; 2828 } 2829 2830 /** 2831 * @return The first repetition of repeating field {@link #applicability}, 2832 * creating it if it does not already exist 2833 */ 2834 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 2835 if (getApplicability().isEmpty()) { 2836 addApplicability(); 2837 } 2838 return getApplicability().get(0); 2839 } 2840 2841 /** 2842 * @return {@link #propertyGroup} (Group of properties which are applicable 2843 * under the same conditions. If no applicability rules are established 2844 * for the group, then all properties always apply.) 2845 */ 2846 public List<ChargeItemDefinitionPropertyGroupComponent> getPropertyGroup() { 2847 if (this.propertyGroup == null) 2848 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2849 return this.propertyGroup; 2850 } 2851 2852 /** 2853 * @return Returns a reference to <code>this</code> for easy method chaining 2854 */ 2855 public ChargeItemDefinition setPropertyGroup(List<ChargeItemDefinitionPropertyGroupComponent> thePropertyGroup) { 2856 this.propertyGroup = thePropertyGroup; 2857 return this; 2858 } 2859 2860 public boolean hasPropertyGroup() { 2861 if (this.propertyGroup == null) 2862 return false; 2863 for (ChargeItemDefinitionPropertyGroupComponent item : this.propertyGroup) 2864 if (!item.isEmpty()) 2865 return true; 2866 return false; 2867 } 2868 2869 public ChargeItemDefinitionPropertyGroupComponent addPropertyGroup() { // 3 2870 ChargeItemDefinitionPropertyGroupComponent t = new ChargeItemDefinitionPropertyGroupComponent(); 2871 if (this.propertyGroup == null) 2872 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2873 this.propertyGroup.add(t); 2874 return t; 2875 } 2876 2877 public ChargeItemDefinition addPropertyGroup(ChargeItemDefinitionPropertyGroupComponent t) { // 3 2878 if (t == null) 2879 return this; 2880 if (this.propertyGroup == null) 2881 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2882 this.propertyGroup.add(t); 2883 return this; 2884 } 2885 2886 /** 2887 * @return The first repetition of repeating field {@link #propertyGroup}, 2888 * creating it if it does not already exist 2889 */ 2890 public ChargeItemDefinitionPropertyGroupComponent getPropertyGroupFirstRep() { 2891 if (getPropertyGroup().isEmpty()) { 2892 addPropertyGroup(); 2893 } 2894 return getPropertyGroup().get(0); 2895 } 2896 2897 protected void listChildren(List<Property> children) { 2898 super.listChildren(children); 2899 children.add(new Property("url", "uri", 2900 "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 2901 0, 1, url)); 2902 children.add(new Property("identifier", "Identifier", 2903 "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2904 0, java.lang.Integer.MAX_VALUE, identifier)); 2905 children.add(new Property("version", "string", 2906 "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 2907 0, 1, version)); 2908 children.add(new Property("title", "string", 2909 "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title)); 2910 children.add(new Property("derivedFromUri", "uri", 2911 "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 2912 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 2913 children.add(new Property("partOf", "canonical(ChargeItemDefinition)", 2914 "A larger definition of which this particular definition is a component or step.", 0, 2915 java.lang.Integer.MAX_VALUE, partOf)); 2916 children.add(new Property("replaces", "canonical(ChargeItemDefinition)", 2917 "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 2918 0, java.lang.Integer.MAX_VALUE, replaces)); 2919 children.add(new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, status)); 2920 children.add(new Property("experimental", "boolean", 2921 "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2922 0, 1, experimental)); 2923 children.add(new Property("date", "dateTime", 2924 "The date (and optionally time) when the charge item definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 2925 0, 1, date)); 2926 children.add(new Property("publisher", "string", 2927 "The name of the organization or individual that published the charge item definition.", 0, 1, publisher)); 2928 children.add(new Property("contact", "ContactDetail", 2929 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2930 java.lang.Integer.MAX_VALUE, contact)); 2931 children.add(new Property("description", "markdown", 2932 "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, 2933 description)); 2934 children.add(new Property("useContext", "UsageContext", 2935 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 2936 0, java.lang.Integer.MAX_VALUE, useContext)); 2937 children.add(new Property("jurisdiction", "CodeableConcept", 2938 "A legal or geographic region in which the charge item definition is intended to be used.", 0, 2939 java.lang.Integer.MAX_VALUE, jurisdiction)); 2940 children.add(new Property("copyright", "markdown", 2941 "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 2942 0, 1, copyright)); 2943 children.add(new Property("approvalDate", "date", 2944 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2945 0, 1, approvalDate)); 2946 children.add(new Property("lastReviewDate", "date", 2947 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2948 0, 1, lastReviewDate)); 2949 children.add(new Property("effectivePeriod", "Period", 2950 "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, 2951 effectivePeriod)); 2952 children.add(new Property("code", "CodeableConcept", 2953 "The defined billing details in this resource pertain to the given billing code.", 0, 1, code)); 2954 children.add(new Property("instance", "Reference(Medication|Substance|Device)", 2955 "The defined billing details in this resource pertain to the given product instance(s).", 0, 2956 java.lang.Integer.MAX_VALUE, instance)); 2957 children 2958 .add(new Property("applicability", "", "Expressions that describe applicability criteria for the billing code.", 2959 0, java.lang.Integer.MAX_VALUE, applicability)); 2960 children.add(new Property("propertyGroup", "", 2961 "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 2962 0, java.lang.Integer.MAX_VALUE, propertyGroup)); 2963 } 2964 2965 @Override 2966 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2967 switch (_hash) { 2968 case 116079: 2969 /* url */ return new Property("url", "uri", 2970 "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 2971 0, 1, url); 2972 case -1618432855: 2973 /* identifier */ return new Property("identifier", "Identifier", 2974 "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2975 0, java.lang.Integer.MAX_VALUE, identifier); 2976 case 351608024: 2977 /* version */ return new Property("version", "string", 2978 "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 2979 0, 1, version); 2980 case 110371416: 2981 /* title */ return new Property("title", "string", 2982 "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title); 2983 case -1076333435: 2984 /* derivedFromUri */ return new Property("derivedFromUri", "uri", 2985 "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 2986 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 2987 case -995410646: 2988 /* partOf */ return new Property("partOf", "canonical(ChargeItemDefinition)", 2989 "A larger definition of which this particular definition is a component or step.", 0, 2990 java.lang.Integer.MAX_VALUE, partOf); 2991 case -430332865: 2992 /* replaces */ return new Property("replaces", "canonical(ChargeItemDefinition)", 2993 "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 2994 0, java.lang.Integer.MAX_VALUE, replaces); 2995 case -892481550: 2996 /* status */ return new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, 2997 status); 2998 case -404562712: 2999 /* experimental */ return new Property("experimental", "boolean", 3000 "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3001 0, 1, experimental); 3002 case 3076014: 3003 /* date */ return new Property("date", "dateTime", 3004 "The date (and optionally time) when the charge item definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 3005 0, 1, date); 3006 case 1447404028: 3007 /* publisher */ return new Property("publisher", "string", 3008 "The name of the organization or individual that published the charge item definition.", 0, 1, publisher); 3009 case 951526432: 3010 /* contact */ return new Property("contact", "ContactDetail", 3011 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3012 java.lang.Integer.MAX_VALUE, contact); 3013 case -1724546052: 3014 /* description */ return new Property("description", "markdown", 3015 "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, 3016 description); 3017 case -669707736: 3018 /* useContext */ return new Property("useContext", "UsageContext", 3019 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 3020 0, java.lang.Integer.MAX_VALUE, useContext); 3021 case -507075711: 3022 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3023 "A legal or geographic region in which the charge item definition is intended to be used.", 0, 3024 java.lang.Integer.MAX_VALUE, jurisdiction); 3025 case 1522889671: 3026 /* copyright */ return new Property("copyright", "markdown", 3027 "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 3028 0, 1, copyright); 3029 case 223539345: 3030 /* approvalDate */ return new Property("approvalDate", "date", 3031 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 3032 0, 1, approvalDate); 3033 case -1687512484: 3034 /* lastReviewDate */ return new Property("lastReviewDate", "date", 3035 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 3036 0, 1, lastReviewDate); 3037 case -403934648: 3038 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 3039 "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, 3040 effectivePeriod); 3041 case 3059181: 3042 /* code */ return new Property("code", "CodeableConcept", 3043 "The defined billing details in this resource pertain to the given billing code.", 0, 1, code); 3044 case 555127957: 3045 /* instance */ return new Property("instance", "Reference(Medication|Substance|Device)", 3046 "The defined billing details in this resource pertain to the given product instance(s).", 0, 3047 java.lang.Integer.MAX_VALUE, instance); 3048 case -1526770491: 3049 /* applicability */ return new Property("applicability", "", 3050 "Expressions that describe applicability criteria for the billing code.", 0, java.lang.Integer.MAX_VALUE, 3051 applicability); 3052 case -1041594966: 3053 /* propertyGroup */ return new Property("propertyGroup", "", 3054 "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 3055 0, java.lang.Integer.MAX_VALUE, propertyGroup); 3056 default: 3057 return super.getNamedProperty(_hash, _name, _checkValid); 3058 } 3059 3060 } 3061 3062 @Override 3063 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3064 switch (hash) { 3065 case 116079: 3066 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3067 case -1618432855: 3068 /* identifier */ return this.identifier == null ? new Base[0] 3069 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3070 case 351608024: 3071 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3072 case 110371416: 3073 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3074 case -1076333435: 3075 /* derivedFromUri */ return this.derivedFromUri == null ? new Base[0] 3076 : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 3077 case -995410646: 3078 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // CanonicalType 3079 case -430332865: 3080 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 3081 case -892481550: 3082 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3083 case -404562712: 3084 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 3085 case 3076014: 3086 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3087 case 1447404028: 3088 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3089 case 951526432: 3090 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3091 case -1724546052: 3092 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3093 case -669707736: 3094 /* useContext */ return this.useContext == null ? new Base[0] 3095 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3096 case -507075711: 3097 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3098 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3099 case 1522889671: 3100 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 3101 case 223539345: 3102 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 3103 case -1687512484: 3104 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 3105 case -403934648: 3106 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 3107 case 3059181: 3108 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3109 case 555127957: 3110 /* instance */ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // Reference 3111 case -1526770491: 3112 /* applicability */ return this.applicability == null ? new Base[0] 3113 : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 3114 case -1041594966: 3115 /* propertyGroup */ return this.propertyGroup == null ? new Base[0] 3116 : this.propertyGroup.toArray(new Base[this.propertyGroup.size()]); // ChargeItemDefinitionPropertyGroupComponent 3117 default: 3118 return super.getProperty(hash, name, checkValid); 3119 } 3120 3121 } 3122 3123 @Override 3124 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3125 switch (hash) { 3126 case 116079: // url 3127 this.url = castToUri(value); // UriType 3128 return value; 3129 case -1618432855: // identifier 3130 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3131 return value; 3132 case 351608024: // version 3133 this.version = castToString(value); // StringType 3134 return value; 3135 case 110371416: // title 3136 this.title = castToString(value); // StringType 3137 return value; 3138 case -1076333435: // derivedFromUri 3139 this.getDerivedFromUri().add(castToUri(value)); // UriType 3140 return value; 3141 case -995410646: // partOf 3142 this.getPartOf().add(castToCanonical(value)); // CanonicalType 3143 return value; 3144 case -430332865: // replaces 3145 this.getReplaces().add(castToCanonical(value)); // CanonicalType 3146 return value; 3147 case -892481550: // status 3148 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3149 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3150 return value; 3151 case -404562712: // experimental 3152 this.experimental = castToBoolean(value); // BooleanType 3153 return value; 3154 case 3076014: // date 3155 this.date = castToDateTime(value); // DateTimeType 3156 return value; 3157 case 1447404028: // publisher 3158 this.publisher = castToString(value); // StringType 3159 return value; 3160 case 951526432: // contact 3161 this.getContact().add(castToContactDetail(value)); // ContactDetail 3162 return value; 3163 case -1724546052: // description 3164 this.description = castToMarkdown(value); // MarkdownType 3165 return value; 3166 case -669707736: // useContext 3167 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3168 return value; 3169 case -507075711: // jurisdiction 3170 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3171 return value; 3172 case 1522889671: // copyright 3173 this.copyright = castToMarkdown(value); // MarkdownType 3174 return value; 3175 case 223539345: // approvalDate 3176 this.approvalDate = castToDate(value); // DateType 3177 return value; 3178 case -1687512484: // lastReviewDate 3179 this.lastReviewDate = castToDate(value); // DateType 3180 return value; 3181 case -403934648: // effectivePeriod 3182 this.effectivePeriod = castToPeriod(value); // Period 3183 return value; 3184 case 3059181: // code 3185 this.code = castToCodeableConcept(value); // CodeableConcept 3186 return value; 3187 case 555127957: // instance 3188 this.getInstance().add(castToReference(value)); // Reference 3189 return value; 3190 case -1526770491: // applicability 3191 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 3192 return value; 3193 case -1041594966: // propertyGroup 3194 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); // ChargeItemDefinitionPropertyGroupComponent 3195 return value; 3196 default: 3197 return super.setProperty(hash, name, value); 3198 } 3199 3200 } 3201 3202 @Override 3203 public Base setProperty(String name, Base value) throws FHIRException { 3204 if (name.equals("url")) { 3205 this.url = castToUri(value); // UriType 3206 } else if (name.equals("identifier")) { 3207 this.getIdentifier().add(castToIdentifier(value)); 3208 } else if (name.equals("version")) { 3209 this.version = castToString(value); // StringType 3210 } else if (name.equals("title")) { 3211 this.title = castToString(value); // StringType 3212 } else if (name.equals("derivedFromUri")) { 3213 this.getDerivedFromUri().add(castToUri(value)); 3214 } else if (name.equals("partOf")) { 3215 this.getPartOf().add(castToCanonical(value)); 3216 } else if (name.equals("replaces")) { 3217 this.getReplaces().add(castToCanonical(value)); 3218 } else if (name.equals("status")) { 3219 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3220 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3221 } else if (name.equals("experimental")) { 3222 this.experimental = castToBoolean(value); // BooleanType 3223 } else if (name.equals("date")) { 3224 this.date = castToDateTime(value); // DateTimeType 3225 } else if (name.equals("publisher")) { 3226 this.publisher = castToString(value); // StringType 3227 } else if (name.equals("contact")) { 3228 this.getContact().add(castToContactDetail(value)); 3229 } else if (name.equals("description")) { 3230 this.description = castToMarkdown(value); // MarkdownType 3231 } else if (name.equals("useContext")) { 3232 this.getUseContext().add(castToUsageContext(value)); 3233 } else if (name.equals("jurisdiction")) { 3234 this.getJurisdiction().add(castToCodeableConcept(value)); 3235 } else if (name.equals("copyright")) { 3236 this.copyright = castToMarkdown(value); // MarkdownType 3237 } else if (name.equals("approvalDate")) { 3238 this.approvalDate = castToDate(value); // DateType 3239 } else if (name.equals("lastReviewDate")) { 3240 this.lastReviewDate = castToDate(value); // DateType 3241 } else if (name.equals("effectivePeriod")) { 3242 this.effectivePeriod = castToPeriod(value); // Period 3243 } else if (name.equals("code")) { 3244 this.code = castToCodeableConcept(value); // CodeableConcept 3245 } else if (name.equals("instance")) { 3246 this.getInstance().add(castToReference(value)); 3247 } else if (name.equals("applicability")) { 3248 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 3249 } else if (name.equals("propertyGroup")) { 3250 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); 3251 } else 3252 return super.setProperty(name, value); 3253 return value; 3254 } 3255 3256 @Override 3257 public Base makeProperty(int hash, String name) throws FHIRException { 3258 switch (hash) { 3259 case 116079: 3260 return getUrlElement(); 3261 case -1618432855: 3262 return addIdentifier(); 3263 case 351608024: 3264 return getVersionElement(); 3265 case 110371416: 3266 return getTitleElement(); 3267 case -1076333435: 3268 return addDerivedFromUriElement(); 3269 case -995410646: 3270 return addPartOfElement(); 3271 case -430332865: 3272 return addReplacesElement(); 3273 case -892481550: 3274 return getStatusElement(); 3275 case -404562712: 3276 return getExperimentalElement(); 3277 case 3076014: 3278 return getDateElement(); 3279 case 1447404028: 3280 return getPublisherElement(); 3281 case 951526432: 3282 return addContact(); 3283 case -1724546052: 3284 return getDescriptionElement(); 3285 case -669707736: 3286 return addUseContext(); 3287 case -507075711: 3288 return addJurisdiction(); 3289 case 1522889671: 3290 return getCopyrightElement(); 3291 case 223539345: 3292 return getApprovalDateElement(); 3293 case -1687512484: 3294 return getLastReviewDateElement(); 3295 case -403934648: 3296 return getEffectivePeriod(); 3297 case 3059181: 3298 return getCode(); 3299 case 555127957: 3300 return addInstance(); 3301 case -1526770491: 3302 return addApplicability(); 3303 case -1041594966: 3304 return addPropertyGroup(); 3305 default: 3306 return super.makeProperty(hash, name); 3307 } 3308 3309 } 3310 3311 @Override 3312 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3313 switch (hash) { 3314 case 116079: 3315 /* url */ return new String[] { "uri" }; 3316 case -1618432855: 3317 /* identifier */ return new String[] { "Identifier" }; 3318 case 351608024: 3319 /* version */ return new String[] { "string" }; 3320 case 110371416: 3321 /* title */ return new String[] { "string" }; 3322 case -1076333435: 3323 /* derivedFromUri */ return new String[] { "uri" }; 3324 case -995410646: 3325 /* partOf */ return new String[] { "canonical" }; 3326 case -430332865: 3327 /* replaces */ return new String[] { "canonical" }; 3328 case -892481550: 3329 /* status */ return new String[] { "code" }; 3330 case -404562712: 3331 /* experimental */ return new String[] { "boolean" }; 3332 case 3076014: 3333 /* date */ return new String[] { "dateTime" }; 3334 case 1447404028: 3335 /* publisher */ return new String[] { "string" }; 3336 case 951526432: 3337 /* contact */ return new String[] { "ContactDetail" }; 3338 case -1724546052: 3339 /* description */ return new String[] { "markdown" }; 3340 case -669707736: 3341 /* useContext */ return new String[] { "UsageContext" }; 3342 case -507075711: 3343 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3344 case 1522889671: 3345 /* copyright */ return new String[] { "markdown" }; 3346 case 223539345: 3347 /* approvalDate */ return new String[] { "date" }; 3348 case -1687512484: 3349 /* lastReviewDate */ return new String[] { "date" }; 3350 case -403934648: 3351 /* effectivePeriod */ return new String[] { "Period" }; 3352 case 3059181: 3353 /* code */ return new String[] { "CodeableConcept" }; 3354 case 555127957: 3355 /* instance */ return new String[] { "Reference" }; 3356 case -1526770491: 3357 /* applicability */ return new String[] {}; 3358 case -1041594966: 3359 /* propertyGroup */ return new String[] {}; 3360 default: 3361 return super.getTypesForProperty(hash, name); 3362 } 3363 3364 } 3365 3366 @Override 3367 public Base addChild(String name) throws FHIRException { 3368 if (name.equals("url")) { 3369 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.url"); 3370 } else if (name.equals("identifier")) { 3371 return addIdentifier(); 3372 } else if (name.equals("version")) { 3373 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.version"); 3374 } else if (name.equals("title")) { 3375 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.title"); 3376 } else if (name.equals("derivedFromUri")) { 3377 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.derivedFromUri"); 3378 } else if (name.equals("partOf")) { 3379 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.partOf"); 3380 } else if (name.equals("replaces")) { 3381 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.replaces"); 3382 } else if (name.equals("status")) { 3383 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.status"); 3384 } else if (name.equals("experimental")) { 3385 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.experimental"); 3386 } else if (name.equals("date")) { 3387 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.date"); 3388 } else if (name.equals("publisher")) { 3389 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.publisher"); 3390 } else if (name.equals("contact")) { 3391 return addContact(); 3392 } else if (name.equals("description")) { 3393 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.description"); 3394 } else if (name.equals("useContext")) { 3395 return addUseContext(); 3396 } else if (name.equals("jurisdiction")) { 3397 return addJurisdiction(); 3398 } else if (name.equals("copyright")) { 3399 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.copyright"); 3400 } else if (name.equals("approvalDate")) { 3401 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.approvalDate"); 3402 } else if (name.equals("lastReviewDate")) { 3403 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.lastReviewDate"); 3404 } else if (name.equals("effectivePeriod")) { 3405 this.effectivePeriod = new Period(); 3406 return this.effectivePeriod; 3407 } else if (name.equals("code")) { 3408 this.code = new CodeableConcept(); 3409 return this.code; 3410 } else if (name.equals("instance")) { 3411 return addInstance(); 3412 } else if (name.equals("applicability")) { 3413 return addApplicability(); 3414 } else if (name.equals("propertyGroup")) { 3415 return addPropertyGroup(); 3416 } else 3417 return super.addChild(name); 3418 } 3419 3420 public String fhirType() { 3421 return "ChargeItemDefinition"; 3422 3423 } 3424 3425 public ChargeItemDefinition copy() { 3426 ChargeItemDefinition dst = new ChargeItemDefinition(); 3427 copyValues(dst); 3428 return dst; 3429 } 3430 3431 public void copyValues(ChargeItemDefinition dst) { 3432 super.copyValues(dst); 3433 dst.url = url == null ? null : url.copy(); 3434 if (identifier != null) { 3435 dst.identifier = new ArrayList<Identifier>(); 3436 for (Identifier i : identifier) 3437 dst.identifier.add(i.copy()); 3438 } 3439 ; 3440 dst.version = version == null ? null : version.copy(); 3441 dst.title = title == null ? null : title.copy(); 3442 if (derivedFromUri != null) { 3443 dst.derivedFromUri = new ArrayList<UriType>(); 3444 for (UriType i : derivedFromUri) 3445 dst.derivedFromUri.add(i.copy()); 3446 } 3447 ; 3448 if (partOf != null) { 3449 dst.partOf = new ArrayList<CanonicalType>(); 3450 for (CanonicalType i : partOf) 3451 dst.partOf.add(i.copy()); 3452 } 3453 ; 3454 if (replaces != null) { 3455 dst.replaces = new ArrayList<CanonicalType>(); 3456 for (CanonicalType i : replaces) 3457 dst.replaces.add(i.copy()); 3458 } 3459 ; 3460 dst.status = status == null ? null : status.copy(); 3461 dst.experimental = experimental == null ? null : experimental.copy(); 3462 dst.date = date == null ? null : date.copy(); 3463 dst.publisher = publisher == null ? null : publisher.copy(); 3464 if (contact != null) { 3465 dst.contact = new ArrayList<ContactDetail>(); 3466 for (ContactDetail i : contact) 3467 dst.contact.add(i.copy()); 3468 } 3469 ; 3470 dst.description = description == null ? null : description.copy(); 3471 if (useContext != null) { 3472 dst.useContext = new ArrayList<UsageContext>(); 3473 for (UsageContext i : useContext) 3474 dst.useContext.add(i.copy()); 3475 } 3476 ; 3477 if (jurisdiction != null) { 3478 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3479 for (CodeableConcept i : jurisdiction) 3480 dst.jurisdiction.add(i.copy()); 3481 } 3482 ; 3483 dst.copyright = copyright == null ? null : copyright.copy(); 3484 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3485 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3486 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3487 dst.code = code == null ? null : code.copy(); 3488 if (instance != null) { 3489 dst.instance = new ArrayList<Reference>(); 3490 for (Reference i : instance) 3491 dst.instance.add(i.copy()); 3492 } 3493 ; 3494 if (applicability != null) { 3495 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 3496 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 3497 dst.applicability.add(i.copy()); 3498 } 3499 ; 3500 if (propertyGroup != null) { 3501 dst.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 3502 for (ChargeItemDefinitionPropertyGroupComponent i : propertyGroup) 3503 dst.propertyGroup.add(i.copy()); 3504 } 3505 ; 3506 } 3507 3508 protected ChargeItemDefinition typedCopy() { 3509 return copy(); 3510 } 3511 3512 @Override 3513 public boolean equalsDeep(Base other_) { 3514 if (!super.equalsDeep(other_)) 3515 return false; 3516 if (!(other_ instanceof ChargeItemDefinition)) 3517 return false; 3518 ChargeItemDefinition o = (ChargeItemDefinition) other_; 3519 return compareDeep(identifier, o.identifier, true) && compareDeep(derivedFromUri, o.derivedFromUri, true) 3520 && compareDeep(partOf, o.partOf, true) && compareDeep(replaces, o.replaces, true) 3521 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 3522 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 3523 && compareDeep(code, o.code, true) && compareDeep(instance, o.instance, true) 3524 && compareDeep(applicability, o.applicability, true) && compareDeep(propertyGroup, o.propertyGroup, true); 3525 } 3526 3527 @Override 3528 public boolean equalsShallow(Base other_) { 3529 if (!super.equalsShallow(other_)) 3530 return false; 3531 if (!(other_ instanceof ChargeItemDefinition)) 3532 return false; 3533 ChargeItemDefinition o = (ChargeItemDefinition) other_; 3534 return compareValues(derivedFromUri, o.derivedFromUri, true) && compareValues(copyright, o.copyright, true) 3535 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 3536 } 3537 3538 public boolean isEmpty() { 3539 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, derivedFromUri, partOf, replaces, 3540 copyright, approvalDate, lastReviewDate, effectivePeriod, code, instance, applicability, propertyGroup); 3541 } 3542 3543 @Override 3544 public ResourceType getResourceType() { 3545 return ResourceType.ChargeItemDefinition; 3546 } 3547 3548 /** 3549 * Search parameter: <b>date</b> 3550 * <p> 3551 * Description: <b>The charge item definition publication date</b><br> 3552 * Type: <b>date</b><br> 3553 * Path: <b>ChargeItemDefinition.date</b><br> 3554 * </p> 3555 */ 3556 @SearchParamDefinition(name = "date", path = "ChargeItemDefinition.date", description = "The charge item definition publication date", type = "date") 3557 public static final String SP_DATE = "date"; 3558 /** 3559 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3560 * <p> 3561 * Description: <b>The charge item definition publication date</b><br> 3562 * Type: <b>date</b><br> 3563 * Path: <b>ChargeItemDefinition.date</b><br> 3564 * </p> 3565 */ 3566 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3567 SP_DATE); 3568 3569 /** 3570 * Search parameter: <b>identifier</b> 3571 * <p> 3572 * Description: <b>External identifier for the charge item definition</b><br> 3573 * Type: <b>token</b><br> 3574 * Path: <b>ChargeItemDefinition.identifier</b><br> 3575 * </p> 3576 */ 3577 @SearchParamDefinition(name = "identifier", path = "ChargeItemDefinition.identifier", description = "External identifier for the charge item definition", type = "token") 3578 public static final String SP_IDENTIFIER = "identifier"; 3579 /** 3580 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3581 * <p> 3582 * Description: <b>External identifier for the charge item definition</b><br> 3583 * Type: <b>token</b><br> 3584 * Path: <b>ChargeItemDefinition.identifier</b><br> 3585 * </p> 3586 */ 3587 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3588 SP_IDENTIFIER); 3589 3590 /** 3591 * Search parameter: <b>context-type-value</b> 3592 * <p> 3593 * Description: <b>A use context type and value assigned to the charge item 3594 * definition</b><br> 3595 * Type: <b>composite</b><br> 3596 * Path: <b></b><br> 3597 * </p> 3598 */ 3599 @SearchParamDefinition(name = "context-type-value", path = "ChargeItemDefinition.useContext", description = "A use context type and value assigned to the charge item definition", type = "composite", compositeOf = { 3600 "context-type", "context" }) 3601 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3602 /** 3603 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3604 * <p> 3605 * Description: <b>A use context type and value assigned to the charge item 3606 * definition</b><br> 3607 * Type: <b>composite</b><br> 3608 * Path: <b></b><br> 3609 * </p> 3610 */ 3611 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3612 SP_CONTEXT_TYPE_VALUE); 3613 3614 /** 3615 * Search parameter: <b>jurisdiction</b> 3616 * <p> 3617 * Description: <b>Intended jurisdiction for the charge item definition</b><br> 3618 * Type: <b>token</b><br> 3619 * Path: <b>ChargeItemDefinition.jurisdiction</b><br> 3620 * </p> 3621 */ 3622 @SearchParamDefinition(name = "jurisdiction", path = "ChargeItemDefinition.jurisdiction", description = "Intended jurisdiction for the charge item definition", type = "token") 3623 public static final String SP_JURISDICTION = "jurisdiction"; 3624 /** 3625 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3626 * <p> 3627 * Description: <b>Intended jurisdiction for the charge item definition</b><br> 3628 * Type: <b>token</b><br> 3629 * Path: <b>ChargeItemDefinition.jurisdiction</b><br> 3630 * </p> 3631 */ 3632 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3633 SP_JURISDICTION); 3634 3635 /** 3636 * Search parameter: <b>description</b> 3637 * <p> 3638 * Description: <b>The description of the charge item definition</b><br> 3639 * Type: <b>string</b><br> 3640 * Path: <b>ChargeItemDefinition.description</b><br> 3641 * </p> 3642 */ 3643 @SearchParamDefinition(name = "description", path = "ChargeItemDefinition.description", description = "The description of the charge item definition", type = "string") 3644 public static final String SP_DESCRIPTION = "description"; 3645 /** 3646 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3647 * <p> 3648 * Description: <b>The description of the charge item definition</b><br> 3649 * Type: <b>string</b><br> 3650 * Path: <b>ChargeItemDefinition.description</b><br> 3651 * </p> 3652 */ 3653 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3654 SP_DESCRIPTION); 3655 3656 /** 3657 * Search parameter: <b>context-type</b> 3658 * <p> 3659 * Description: <b>A type of use context assigned to the charge item 3660 * definition</b><br> 3661 * Type: <b>token</b><br> 3662 * Path: <b>ChargeItemDefinition.useContext.code</b><br> 3663 * </p> 3664 */ 3665 @SearchParamDefinition(name = "context-type", path = "ChargeItemDefinition.useContext.code", description = "A type of use context assigned to the charge item definition", type = "token") 3666 public static final String SP_CONTEXT_TYPE = "context-type"; 3667 /** 3668 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3669 * <p> 3670 * Description: <b>A type of use context assigned to the charge item 3671 * definition</b><br> 3672 * Type: <b>token</b><br> 3673 * Path: <b>ChargeItemDefinition.useContext.code</b><br> 3674 * </p> 3675 */ 3676 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3677 SP_CONTEXT_TYPE); 3678 3679 /** 3680 * Search parameter: <b>title</b> 3681 * <p> 3682 * Description: <b>The human-friendly name of the charge item definition</b><br> 3683 * Type: <b>string</b><br> 3684 * Path: <b>ChargeItemDefinition.title</b><br> 3685 * </p> 3686 */ 3687 @SearchParamDefinition(name = "title", path = "ChargeItemDefinition.title", description = "The human-friendly name of the charge item definition", type = "string") 3688 public static final String SP_TITLE = "title"; 3689 /** 3690 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3691 * <p> 3692 * Description: <b>The human-friendly name of the charge item definition</b><br> 3693 * Type: <b>string</b><br> 3694 * Path: <b>ChargeItemDefinition.title</b><br> 3695 * </p> 3696 */ 3697 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3698 SP_TITLE); 3699 3700 /** 3701 * Search parameter: <b>version</b> 3702 * <p> 3703 * Description: <b>The business version of the charge item definition</b><br> 3704 * Type: <b>token</b><br> 3705 * Path: <b>ChargeItemDefinition.version</b><br> 3706 * </p> 3707 */ 3708 @SearchParamDefinition(name = "version", path = "ChargeItemDefinition.version", description = "The business version of the charge item definition", type = "token") 3709 public static final String SP_VERSION = "version"; 3710 /** 3711 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3712 * <p> 3713 * Description: <b>The business version of the charge item definition</b><br> 3714 * Type: <b>token</b><br> 3715 * Path: <b>ChargeItemDefinition.version</b><br> 3716 * </p> 3717 */ 3718 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3719 SP_VERSION); 3720 3721 /** 3722 * Search parameter: <b>url</b> 3723 * <p> 3724 * Description: <b>The uri that identifies the charge item definition</b><br> 3725 * Type: <b>uri</b><br> 3726 * Path: <b>ChargeItemDefinition.url</b><br> 3727 * </p> 3728 */ 3729 @SearchParamDefinition(name = "url", path = "ChargeItemDefinition.url", description = "The uri that identifies the charge item definition", type = "uri") 3730 public static final String SP_URL = "url"; 3731 /** 3732 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3733 * <p> 3734 * Description: <b>The uri that identifies the charge item definition</b><br> 3735 * Type: <b>uri</b><br> 3736 * Path: <b>ChargeItemDefinition.url</b><br> 3737 * </p> 3738 */ 3739 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3740 3741 /** 3742 * Search parameter: <b>context-quantity</b> 3743 * <p> 3744 * Description: <b>A quantity- or range-valued use context assigned to the 3745 * charge item definition</b><br> 3746 * Type: <b>quantity</b><br> 3747 * Path: <b>ChargeItemDefinition.useContext.valueQuantity, 3748 * ChargeItemDefinition.useContext.valueRange</b><br> 3749 * </p> 3750 */ 3751 @SearchParamDefinition(name = "context-quantity", path = "(ChargeItemDefinition.useContext.value as Quantity) | (ChargeItemDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the charge item definition", type = "quantity") 3752 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3753 /** 3754 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3755 * <p> 3756 * Description: <b>A quantity- or range-valued use context assigned to the 3757 * charge item definition</b><br> 3758 * Type: <b>quantity</b><br> 3759 * Path: <b>ChargeItemDefinition.useContext.valueQuantity, 3760 * ChargeItemDefinition.useContext.valueRange</b><br> 3761 * </p> 3762 */ 3763 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3764 SP_CONTEXT_QUANTITY); 3765 3766 /** 3767 * Search parameter: <b>effective</b> 3768 * <p> 3769 * Description: <b>The time during which the charge item definition is intended 3770 * to be in use</b><br> 3771 * Type: <b>date</b><br> 3772 * Path: <b>ChargeItemDefinition.effectivePeriod</b><br> 3773 * </p> 3774 */ 3775 @SearchParamDefinition(name = "effective", path = "ChargeItemDefinition.effectivePeriod", description = "The time during which the charge item definition is intended to be in use", type = "date") 3776 public static final String SP_EFFECTIVE = "effective"; 3777 /** 3778 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3779 * <p> 3780 * Description: <b>The time during which the charge item definition is intended 3781 * to be in use</b><br> 3782 * Type: <b>date</b><br> 3783 * Path: <b>ChargeItemDefinition.effectivePeriod</b><br> 3784 * </p> 3785 */ 3786 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3787 SP_EFFECTIVE); 3788 3789 /** 3790 * Search parameter: <b>context</b> 3791 * <p> 3792 * Description: <b>A use context assigned to the charge item definition</b><br> 3793 * Type: <b>token</b><br> 3794 * Path: <b>ChargeItemDefinition.useContext.valueCodeableConcept</b><br> 3795 * </p> 3796 */ 3797 @SearchParamDefinition(name = "context", path = "(ChargeItemDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the charge item definition", type = "token") 3798 public static final String SP_CONTEXT = "context"; 3799 /** 3800 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3801 * <p> 3802 * Description: <b>A use context assigned to the charge item definition</b><br> 3803 * Type: <b>token</b><br> 3804 * Path: <b>ChargeItemDefinition.useContext.valueCodeableConcept</b><br> 3805 * </p> 3806 */ 3807 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3808 SP_CONTEXT); 3809 3810 /** 3811 * Search parameter: <b>publisher</b> 3812 * <p> 3813 * Description: <b>Name of the publisher of the charge item definition</b><br> 3814 * Type: <b>string</b><br> 3815 * Path: <b>ChargeItemDefinition.publisher</b><br> 3816 * </p> 3817 */ 3818 @SearchParamDefinition(name = "publisher", path = "ChargeItemDefinition.publisher", description = "Name of the publisher of the charge item definition", type = "string") 3819 public static final String SP_PUBLISHER = "publisher"; 3820 /** 3821 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3822 * <p> 3823 * Description: <b>Name of the publisher of the charge item definition</b><br> 3824 * Type: <b>string</b><br> 3825 * Path: <b>ChargeItemDefinition.publisher</b><br> 3826 * </p> 3827 */ 3828 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3829 SP_PUBLISHER); 3830 3831 /** 3832 * Search parameter: <b>context-type-quantity</b> 3833 * <p> 3834 * Description: <b>A use context type and quantity- or range-based value 3835 * assigned to the charge item definition</b><br> 3836 * Type: <b>composite</b><br> 3837 * Path: <b></b><br> 3838 * </p> 3839 */ 3840 @SearchParamDefinition(name = "context-type-quantity", path = "ChargeItemDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the charge item definition", type = "composite", compositeOf = { 3841 "context-type", "context-quantity" }) 3842 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3843 /** 3844 * <b>Fluent Client</b> search parameter constant for 3845 * <b>context-type-quantity</b> 3846 * <p> 3847 * Description: <b>A use context type and quantity- or range-based value 3848 * assigned to the charge item definition</b><br> 3849 * Type: <b>composite</b><br> 3850 * Path: <b></b><br> 3851 * </p> 3852 */ 3853 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3854 SP_CONTEXT_TYPE_QUANTITY); 3855 3856 /** 3857 * Search parameter: <b>status</b> 3858 * <p> 3859 * Description: <b>The current status of the charge item definition</b><br> 3860 * Type: <b>token</b><br> 3861 * Path: <b>ChargeItemDefinition.status</b><br> 3862 * </p> 3863 */ 3864 @SearchParamDefinition(name = "status", path = "ChargeItemDefinition.status", description = "The current status of the charge item definition", type = "token") 3865 public static final String SP_STATUS = "status"; 3866 /** 3867 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3868 * <p> 3869 * Description: <b>The current status of the charge item definition</b><br> 3870 * Type: <b>token</b><br> 3871 * Path: <b>ChargeItemDefinition.status</b><br> 3872 * </p> 3873 */ 3874 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3875 SP_STATUS); 3876 3877}