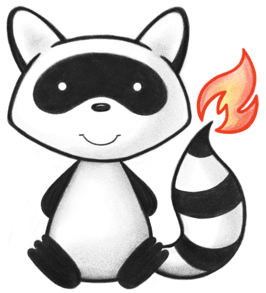
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A provider issued list of professional services and products which have been 051 * provided, or are to be provided, to a patient which is sent to an insurer for 052 * reimbursement. 053 */ 054@ResourceDef(name = "Claim", profile = "http://hl7.org/fhir/StructureDefinition/Claim") 055public class Claim extends DomainResource { 056 057 public enum ClaimStatus { 058 /** 059 * The instance is currently in-force. 060 */ 061 ACTIVE, 062 /** 063 * The instance is withdrawn, rescinded or reversed. 064 */ 065 CANCELLED, 066 /** 067 * A new instance the contents of which is not complete. 068 */ 069 DRAFT, 070 /** 071 * The instance was entered in error. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 079 public static ClaimStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("active".equals(codeString)) 083 return ACTIVE; 084 if ("cancelled".equals(codeString)) 085 return CANCELLED; 086 if ("draft".equals(codeString)) 087 return DRAFT; 088 if ("entered-in-error".equals(codeString)) 089 return ENTEREDINERROR; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown ClaimStatus code '" + codeString + "'"); 094 } 095 096 public String toCode() { 097 switch (this) { 098 case ACTIVE: 099 return "active"; 100 case CANCELLED: 101 return "cancelled"; 102 case DRAFT: 103 return "draft"; 104 case ENTEREDINERROR: 105 return "entered-in-error"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 switch (this) { 115 case ACTIVE: 116 return "http://hl7.org/fhir/fm-status"; 117 case CANCELLED: 118 return "http://hl7.org/fhir/fm-status"; 119 case DRAFT: 120 return "http://hl7.org/fhir/fm-status"; 121 case ENTEREDINERROR: 122 return "http://hl7.org/fhir/fm-status"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDefinition() { 131 switch (this) { 132 case ACTIVE: 133 return "The instance is currently in-force."; 134 case CANCELLED: 135 return "The instance is withdrawn, rescinded or reversed."; 136 case DRAFT: 137 return "A new instance the contents of which is not complete."; 138 case ENTEREDINERROR: 139 return "The instance was entered in error."; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDisplay() { 148 switch (this) { 149 case ACTIVE: 150 return "Active"; 151 case CANCELLED: 152 return "Cancelled"; 153 case DRAFT: 154 return "Draft"; 155 case ENTEREDINERROR: 156 return "Entered in Error"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 } 164 165 public static class ClaimStatusEnumFactory implements EnumFactory<ClaimStatus> { 166 public ClaimStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("active".equals(codeString)) 171 return ClaimStatus.ACTIVE; 172 if ("cancelled".equals(codeString)) 173 return ClaimStatus.CANCELLED; 174 if ("draft".equals(codeString)) 175 return ClaimStatus.DRAFT; 176 if ("entered-in-error".equals(codeString)) 177 return ClaimStatus.ENTEREDINERROR; 178 throw new IllegalArgumentException("Unknown ClaimStatus code '" + codeString + "'"); 179 } 180 181 public Enumeration<ClaimStatus> fromType(PrimitiveType<?> code) throws FHIRException { 182 if (code == null) 183 return null; 184 if (code.isEmpty()) 185 return new Enumeration<ClaimStatus>(this, ClaimStatus.NULL, code); 186 String codeString = code.asStringValue(); 187 if (codeString == null || "".equals(codeString)) 188 return new Enumeration<ClaimStatus>(this, ClaimStatus.NULL, code); 189 if ("active".equals(codeString)) 190 return new Enumeration<ClaimStatus>(this, ClaimStatus.ACTIVE, code); 191 if ("cancelled".equals(codeString)) 192 return new Enumeration<ClaimStatus>(this, ClaimStatus.CANCELLED, code); 193 if ("draft".equals(codeString)) 194 return new Enumeration<ClaimStatus>(this, ClaimStatus.DRAFT, code); 195 if ("entered-in-error".equals(codeString)) 196 return new Enumeration<ClaimStatus>(this, ClaimStatus.ENTEREDINERROR, code); 197 throw new FHIRException("Unknown ClaimStatus code '" + codeString + "'"); 198 } 199 200 public String toCode(ClaimStatus code) { 201 if (code == ClaimStatus.ACTIVE) 202 return "active"; 203 if (code == ClaimStatus.CANCELLED) 204 return "cancelled"; 205 if (code == ClaimStatus.DRAFT) 206 return "draft"; 207 if (code == ClaimStatus.ENTEREDINERROR) 208 return "entered-in-error"; 209 return "?"; 210 } 211 212 public String toSystem(ClaimStatus code) { 213 return code.getSystem(); 214 } 215 } 216 217 public enum Use { 218 /** 219 * The treatment is complete and this represents a Claim for the services. 220 */ 221 CLAIM, 222 /** 223 * The treatment is proposed and this represents a Pre-authorization for the 224 * services. 225 */ 226 PREAUTHORIZATION, 227 /** 228 * The treatment is proposed and this represents a Pre-determination for the 229 * services. 230 */ 231 PREDETERMINATION, 232 /** 233 * added to help the parsers with the generic types 234 */ 235 NULL; 236 237 public static Use fromCode(String codeString) throws FHIRException { 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("claim".equals(codeString)) 241 return CLAIM; 242 if ("preauthorization".equals(codeString)) 243 return PREAUTHORIZATION; 244 if ("predetermination".equals(codeString)) 245 return PREDETERMINATION; 246 if (Configuration.isAcceptInvalidEnums()) 247 return null; 248 else 249 throw new FHIRException("Unknown Use code '" + codeString + "'"); 250 } 251 252 public String toCode() { 253 switch (this) { 254 case CLAIM: 255 return "claim"; 256 case PREAUTHORIZATION: 257 return "preauthorization"; 258 case PREDETERMINATION: 259 return "predetermination"; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getSystem() { 268 switch (this) { 269 case CLAIM: 270 return "http://hl7.org/fhir/claim-use"; 271 case PREAUTHORIZATION: 272 return "http://hl7.org/fhir/claim-use"; 273 case PREDETERMINATION: 274 return "http://hl7.org/fhir/claim-use"; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getDefinition() { 283 switch (this) { 284 case CLAIM: 285 return "The treatment is complete and this represents a Claim for the services."; 286 case PREAUTHORIZATION: 287 return "The treatment is proposed and this represents a Pre-authorization for the services."; 288 case PREDETERMINATION: 289 return "The treatment is proposed and this represents a Pre-determination for the services."; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 297 public String getDisplay() { 298 switch (this) { 299 case CLAIM: 300 return "Claim"; 301 case PREAUTHORIZATION: 302 return "Preauthorization"; 303 case PREDETERMINATION: 304 return "Predetermination"; 305 case NULL: 306 return null; 307 default: 308 return "?"; 309 } 310 } 311 } 312 313 public static class UseEnumFactory implements EnumFactory<Use> { 314 public Use fromCode(String codeString) throws IllegalArgumentException { 315 if (codeString == null || "".equals(codeString)) 316 if (codeString == null || "".equals(codeString)) 317 return null; 318 if ("claim".equals(codeString)) 319 return Use.CLAIM; 320 if ("preauthorization".equals(codeString)) 321 return Use.PREAUTHORIZATION; 322 if ("predetermination".equals(codeString)) 323 return Use.PREDETERMINATION; 324 throw new IllegalArgumentException("Unknown Use code '" + codeString + "'"); 325 } 326 327 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 328 if (code == null) 329 return null; 330 if (code.isEmpty()) 331 return new Enumeration<Use>(this, Use.NULL, code); 332 String codeString = code.asStringValue(); 333 if (codeString == null || "".equals(codeString)) 334 return new Enumeration<Use>(this, Use.NULL, code); 335 if ("claim".equals(codeString)) 336 return new Enumeration<Use>(this, Use.CLAIM, code); 337 if ("preauthorization".equals(codeString)) 338 return new Enumeration<Use>(this, Use.PREAUTHORIZATION, code); 339 if ("predetermination".equals(codeString)) 340 return new Enumeration<Use>(this, Use.PREDETERMINATION, code); 341 throw new FHIRException("Unknown Use code '" + codeString + "'"); 342 } 343 344 public String toCode(Use code) { 345 if (code == Use.CLAIM) 346 return "claim"; 347 if (code == Use.PREAUTHORIZATION) 348 return "preauthorization"; 349 if (code == Use.PREDETERMINATION) 350 return "predetermination"; 351 return "?"; 352 } 353 354 public String toSystem(Use code) { 355 return code.getSystem(); 356 } 357 } 358 359 @Block() 360 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 361 /** 362 * Reference to a related claim. 363 */ 364 @Child(name = "claim", type = { Claim.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 365 @Description(shortDefinition = "Reference to the related claim", formalDefinition = "Reference to a related claim.") 366 protected Reference claim; 367 368 /** 369 * The actual object that is the target of the reference (Reference to a related 370 * claim.) 371 */ 372 protected Claim claimTarget; 373 374 /** 375 * A code to convey how the claims are related. 376 */ 377 @Child(name = "relationship", type = { 378 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 379 @Description(shortDefinition = "How the reference claim is related", formalDefinition = "A code to convey how the claims are related.") 380 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/related-claim-relationship") 381 protected CodeableConcept relationship; 382 383 /** 384 * An alternate organizational reference to the case or file to which this 385 * particular claim pertains. 386 */ 387 @Child(name = "reference", type = { 388 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 389 @Description(shortDefinition = "File or case reference", formalDefinition = "An alternate organizational reference to the case or file to which this particular claim pertains.") 390 protected Identifier reference; 391 392 private static final long serialVersionUID = -379338905L; 393 394 /** 395 * Constructor 396 */ 397 public RelatedClaimComponent() { 398 super(); 399 } 400 401 /** 402 * @return {@link #claim} (Reference to a related claim.) 403 */ 404 public Reference getClaim() { 405 if (this.claim == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 408 else if (Configuration.doAutoCreate()) 409 this.claim = new Reference(); // cc 410 return this.claim; 411 } 412 413 public boolean hasClaim() { 414 return this.claim != null && !this.claim.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #claim} (Reference to a related claim.) 419 */ 420 public RelatedClaimComponent setClaim(Reference value) { 421 this.claim = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #claim} The actual object that is the target of the reference. 427 * The reference library doesn't populate this, but you can use it to 428 * hold the resource if you resolve it. (Reference to a related claim.) 429 */ 430 public Claim getClaimTarget() { 431 if (this.claimTarget == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 434 else if (Configuration.doAutoCreate()) 435 this.claimTarget = new Claim(); // aa 436 return this.claimTarget; 437 } 438 439 /** 440 * @param value {@link #claim} The actual object that is the target of the 441 * reference. The reference library doesn't use these, but you can 442 * use it to hold the resource if you resolve it. (Reference to a 443 * related claim.) 444 */ 445 public RelatedClaimComponent setClaimTarget(Claim value) { 446 this.claimTarget = value; 447 return this; 448 } 449 450 /** 451 * @return {@link #relationship} (A code to convey how the claims are related.) 452 */ 453 public CodeableConcept getRelationship() { 454 if (this.relationship == null) 455 if (Configuration.errorOnAutoCreate()) 456 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 457 else if (Configuration.doAutoCreate()) 458 this.relationship = new CodeableConcept(); // cc 459 return this.relationship; 460 } 461 462 public boolean hasRelationship() { 463 return this.relationship != null && !this.relationship.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #relationship} (A code to convey how the claims are 468 * related.) 469 */ 470 public RelatedClaimComponent setRelationship(CodeableConcept value) { 471 this.relationship = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #reference} (An alternate organizational reference to the case 477 * or file to which this particular claim pertains.) 478 */ 479 public Identifier getReference() { 480 if (this.reference == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 483 else if (Configuration.doAutoCreate()) 484 this.reference = new Identifier(); // cc 485 return this.reference; 486 } 487 488 public boolean hasReference() { 489 return this.reference != null && !this.reference.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #reference} (An alternate organizational reference to the 494 * case or file to which this particular claim pertains.) 495 */ 496 public RelatedClaimComponent setReference(Identifier value) { 497 this.reference = value; 498 return this; 499 } 500 501 protected void listChildren(List<Property> children) { 502 super.listChildren(children); 503 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 504 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, 505 relationship)); 506 children.add(new Property("reference", "Identifier", 507 "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, 508 reference)); 509 } 510 511 @Override 512 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 513 switch (_hash) { 514 case 94742588: 515 /* claim */ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 516 case -261851592: 517 /* relationship */ return new Property("relationship", "CodeableConcept", 518 "A code to convey how the claims are related.", 0, 1, relationship); 519 case -925155509: 520 /* reference */ return new Property("reference", "Identifier", 521 "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, 522 reference); 523 default: 524 return super.getNamedProperty(_hash, _name, _checkValid); 525 } 526 527 } 528 529 @Override 530 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 531 switch (hash) { 532 case 94742588: 533 /* claim */ return this.claim == null ? new Base[0] : new Base[] { this.claim }; // Reference 534 case -261851592: 535 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 536 case -925155509: 537 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Identifier 538 default: 539 return super.getProperty(hash, name, checkValid); 540 } 541 542 } 543 544 @Override 545 public Base setProperty(int hash, String name, Base value) throws FHIRException { 546 switch (hash) { 547 case 94742588: // claim 548 this.claim = castToReference(value); // Reference 549 return value; 550 case -261851592: // relationship 551 this.relationship = castToCodeableConcept(value); // CodeableConcept 552 return value; 553 case -925155509: // reference 554 this.reference = castToIdentifier(value); // Identifier 555 return value; 556 default: 557 return super.setProperty(hash, name, value); 558 } 559 560 } 561 562 @Override 563 public Base setProperty(String name, Base value) throws FHIRException { 564 if (name.equals("claim")) { 565 this.claim = castToReference(value); // Reference 566 } else if (name.equals("relationship")) { 567 this.relationship = castToCodeableConcept(value); // CodeableConcept 568 } else if (name.equals("reference")) { 569 this.reference = castToIdentifier(value); // Identifier 570 } else 571 return super.setProperty(name, value); 572 return value; 573 } 574 575 @Override 576 public void removeChild(String name, Base value) throws FHIRException { 577 if (name.equals("claim")) { 578 this.claim = null; 579 } else if (name.equals("relationship")) { 580 this.relationship = null; 581 } else if (name.equals("reference")) { 582 this.reference = null; 583 } else 584 super.removeChild(name, value); 585 586 } 587 588 @Override 589 public Base makeProperty(int hash, String name) throws FHIRException { 590 switch (hash) { 591 case 94742588: 592 return getClaim(); 593 case -261851592: 594 return getRelationship(); 595 case -925155509: 596 return getReference(); 597 default: 598 return super.makeProperty(hash, name); 599 } 600 601 } 602 603 @Override 604 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 605 switch (hash) { 606 case 94742588: 607 /* claim */ return new String[] { "Reference" }; 608 case -261851592: 609 /* relationship */ return new String[] { "CodeableConcept" }; 610 case -925155509: 611 /* reference */ return new String[] { "Identifier" }; 612 default: 613 return super.getTypesForProperty(hash, name); 614 } 615 616 } 617 618 @Override 619 public Base addChild(String name) throws FHIRException { 620 if (name.equals("claim")) { 621 this.claim = new Reference(); 622 return this.claim; 623 } else if (name.equals("relationship")) { 624 this.relationship = new CodeableConcept(); 625 return this.relationship; 626 } else if (name.equals("reference")) { 627 this.reference = new Identifier(); 628 return this.reference; 629 } else 630 return super.addChild(name); 631 } 632 633 public RelatedClaimComponent copy() { 634 RelatedClaimComponent dst = new RelatedClaimComponent(); 635 copyValues(dst); 636 return dst; 637 } 638 639 public void copyValues(RelatedClaimComponent dst) { 640 super.copyValues(dst); 641 dst.claim = claim == null ? null : claim.copy(); 642 dst.relationship = relationship == null ? null : relationship.copy(); 643 dst.reference = reference == null ? null : reference.copy(); 644 } 645 646 @Override 647 public boolean equalsDeep(Base other_) { 648 if (!super.equalsDeep(other_)) 649 return false; 650 if (!(other_ instanceof RelatedClaimComponent)) 651 return false; 652 RelatedClaimComponent o = (RelatedClaimComponent) other_; 653 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) 654 && compareDeep(reference, o.reference, true); 655 } 656 657 @Override 658 public boolean equalsShallow(Base other_) { 659 if (!super.equalsShallow(other_)) 660 return false; 661 if (!(other_ instanceof RelatedClaimComponent)) 662 return false; 663 RelatedClaimComponent o = (RelatedClaimComponent) other_; 664 return true; 665 } 666 667 public boolean isEmpty() { 668 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference); 669 } 670 671 public String fhirType() { 672 return "Claim.related"; 673 674 } 675 676 } 677 678 @Block() 679 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 680 /** 681 * Type of Party to be reimbursed: subscriber, provider, other. 682 */ 683 @Child(name = "type", type = { 684 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 685 @Description(shortDefinition = "Category of recipient", formalDefinition = "Type of Party to be reimbursed: subscriber, provider, other.") 686 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payeetype") 687 protected CodeableConcept type; 688 689 /** 690 * Reference to the individual or organization to whom any payment will be made. 691 */ 692 @Child(name = "party", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 693 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 694 @Description(shortDefinition = "Recipient reference", formalDefinition = "Reference to the individual or organization to whom any payment will be made.") 695 protected Reference party; 696 697 /** 698 * The actual object that is the target of the reference (Reference to the 699 * individual or organization to whom any payment will be made.) 700 */ 701 protected Resource partyTarget; 702 703 private static final long serialVersionUID = 1609484699L; 704 705 /** 706 * Constructor 707 */ 708 public PayeeComponent() { 709 super(); 710 } 711 712 /** 713 * Constructor 714 */ 715 public PayeeComponent(CodeableConcept type) { 716 super(); 717 this.type = type; 718 } 719 720 /** 721 * @return {@link #type} (Type of Party to be reimbursed: subscriber, provider, 722 * other.) 723 */ 724 public CodeableConcept getType() { 725 if (this.type == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create PayeeComponent.type"); 728 else if (Configuration.doAutoCreate()) 729 this.type = new CodeableConcept(); // cc 730 return this.type; 731 } 732 733 public boolean hasType() { 734 return this.type != null && !this.type.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #type} (Type of Party to be reimbursed: subscriber, 739 * provider, other.) 740 */ 741 public PayeeComponent setType(CodeableConcept value) { 742 this.type = value; 743 return this; 744 } 745 746 /** 747 * @return {@link #party} (Reference to the individual or organization to whom 748 * any payment will be made.) 749 */ 750 public Reference getParty() { 751 if (this.party == null) 752 if (Configuration.errorOnAutoCreate()) 753 throw new Error("Attempt to auto-create PayeeComponent.party"); 754 else if (Configuration.doAutoCreate()) 755 this.party = new Reference(); // cc 756 return this.party; 757 } 758 759 public boolean hasParty() { 760 return this.party != null && !this.party.isEmpty(); 761 } 762 763 /** 764 * @param value {@link #party} (Reference to the individual or organization to 765 * whom any payment will be made.) 766 */ 767 public PayeeComponent setParty(Reference value) { 768 this.party = value; 769 return this; 770 } 771 772 /** 773 * @return {@link #party} The actual object that is the target of the reference. 774 * The reference library doesn't populate this, but you can use it to 775 * hold the resource if you resolve it. (Reference to the individual or 776 * organization to whom any payment will be made.) 777 */ 778 public Resource getPartyTarget() { 779 return this.partyTarget; 780 } 781 782 /** 783 * @param value {@link #party} The actual object that is the target of the 784 * reference. The reference library doesn't use these, but you can 785 * use it to hold the resource if you resolve it. (Reference to the 786 * individual or organization to whom any payment will be made.) 787 */ 788 public PayeeComponent setPartyTarget(Resource value) { 789 this.partyTarget = value; 790 return this; 791 } 792 793 protected void listChildren(List<Property> children) { 794 super.listChildren(children); 795 children.add(new Property("type", "CodeableConcept", 796 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type)); 797 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", 798 "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 799 } 800 801 @Override 802 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 803 switch (_hash) { 804 case 3575610: 805 /* type */ return new Property("type", "CodeableConcept", 806 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type); 807 case 106437350: 808 /* party */ return new Property("party", 809 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", 810 "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 811 default: 812 return super.getNamedProperty(_hash, _name, _checkValid); 813 } 814 815 } 816 817 @Override 818 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 819 switch (hash) { 820 case 3575610: 821 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 822 case 106437350: 823 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 824 default: 825 return super.getProperty(hash, name, checkValid); 826 } 827 828 } 829 830 @Override 831 public Base setProperty(int hash, String name, Base value) throws FHIRException { 832 switch (hash) { 833 case 3575610: // type 834 this.type = castToCodeableConcept(value); // CodeableConcept 835 return value; 836 case 106437350: // party 837 this.party = castToReference(value); // Reference 838 return value; 839 default: 840 return super.setProperty(hash, name, value); 841 } 842 843 } 844 845 @Override 846 public Base setProperty(String name, Base value) throws FHIRException { 847 if (name.equals("type")) { 848 this.type = castToCodeableConcept(value); // CodeableConcept 849 } else if (name.equals("party")) { 850 this.party = castToReference(value); // Reference 851 } else 852 return super.setProperty(name, value); 853 return value; 854 } 855 856 @Override 857 public void removeChild(String name, Base value) throws FHIRException { 858 if (name.equals("type")) { 859 this.type = null; 860 } else if (name.equals("party")) { 861 this.party = null; 862 } else 863 super.removeChild(name, value); 864 865 } 866 867 @Override 868 public Base makeProperty(int hash, String name) throws FHIRException { 869 switch (hash) { 870 case 3575610: 871 return getType(); 872 case 106437350: 873 return getParty(); 874 default: 875 return super.makeProperty(hash, name); 876 } 877 878 } 879 880 @Override 881 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 882 switch (hash) { 883 case 3575610: 884 /* type */ return new String[] { "CodeableConcept" }; 885 case 106437350: 886 /* party */ return new String[] { "Reference" }; 887 default: 888 return super.getTypesForProperty(hash, name); 889 } 890 891 } 892 893 @Override 894 public Base addChild(String name) throws FHIRException { 895 if (name.equals("type")) { 896 this.type = new CodeableConcept(); 897 return this.type; 898 } else if (name.equals("party")) { 899 this.party = new Reference(); 900 return this.party; 901 } else 902 return super.addChild(name); 903 } 904 905 public PayeeComponent copy() { 906 PayeeComponent dst = new PayeeComponent(); 907 copyValues(dst); 908 return dst; 909 } 910 911 public void copyValues(PayeeComponent dst) { 912 super.copyValues(dst); 913 dst.type = type == null ? null : type.copy(); 914 dst.party = party == null ? null : party.copy(); 915 } 916 917 @Override 918 public boolean equalsDeep(Base other_) { 919 if (!super.equalsDeep(other_)) 920 return false; 921 if (!(other_ instanceof PayeeComponent)) 922 return false; 923 PayeeComponent o = (PayeeComponent) other_; 924 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 925 } 926 927 @Override 928 public boolean equalsShallow(Base other_) { 929 if (!super.equalsShallow(other_)) 930 return false; 931 if (!(other_ instanceof PayeeComponent)) 932 return false; 933 PayeeComponent o = (PayeeComponent) other_; 934 return true; 935 } 936 937 public boolean isEmpty() { 938 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 939 } 940 941 public String fhirType() { 942 return "Claim.payee"; 943 944 } 945 946 } 947 948 @Block() 949 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 950 /** 951 * A number to uniquely identify care team entries. 952 */ 953 @Child(name = "sequence", type = { 954 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 955 @Description(shortDefinition = "Order of care team", formalDefinition = "A number to uniquely identify care team entries.") 956 protected PositiveIntType sequence; 957 958 /** 959 * Member of the team who provided the product or service. 960 */ 961 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 962 Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 963 @Description(shortDefinition = "Practitioner or organization", formalDefinition = "Member of the team who provided the product or service.") 964 protected Reference provider; 965 966 /** 967 * The actual object that is the target of the reference (Member of the team who 968 * provided the product or service.) 969 */ 970 protected Resource providerTarget; 971 972 /** 973 * The party who is billing and/or responsible for the claimed products or 974 * services. 975 */ 976 @Child(name = "responsible", type = { 977 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 978 @Description(shortDefinition = "Indicator of the lead practitioner", formalDefinition = "The party who is billing and/or responsible for the claimed products or services.") 979 protected BooleanType responsible; 980 981 /** 982 * The lead, assisting or supervising practitioner and their discipline if a 983 * multidisciplinary team. 984 */ 985 @Child(name = "role", type = { 986 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 987 @Description(shortDefinition = "Function within the team", formalDefinition = "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.") 988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-careteamrole") 989 protected CodeableConcept role; 990 991 /** 992 * The qualification of the practitioner which is applicable for this service. 993 */ 994 @Child(name = "qualification", type = { 995 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 996 @Description(shortDefinition = "Practitioner credential or specialization", formalDefinition = "The qualification of the practitioner which is applicable for this service.") 997 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provider-qualification") 998 protected CodeableConcept qualification; 999 1000 private static final long serialVersionUID = 1758966968L; 1001 1002 /** 1003 * Constructor 1004 */ 1005 public CareTeamComponent() { 1006 super(); 1007 } 1008 1009 /** 1010 * Constructor 1011 */ 1012 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 1013 super(); 1014 this.sequence = sequence; 1015 this.provider = provider; 1016 } 1017 1018 /** 1019 * @return {@link #sequence} (A number to uniquely identify care team entries.). 1020 * This is the underlying object with id, value and extensions. The 1021 * accessor "getSequence" gives direct access to the value 1022 */ 1023 public PositiveIntType getSequenceElement() { 1024 if (this.sequence == null) 1025 if (Configuration.errorOnAutoCreate()) 1026 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 1027 else if (Configuration.doAutoCreate()) 1028 this.sequence = new PositiveIntType(); // bb 1029 return this.sequence; 1030 } 1031 1032 public boolean hasSequenceElement() { 1033 return this.sequence != null && !this.sequence.isEmpty(); 1034 } 1035 1036 public boolean hasSequence() { 1037 return this.sequence != null && !this.sequence.isEmpty(); 1038 } 1039 1040 /** 1041 * @param value {@link #sequence} (A number to uniquely identify care team 1042 * entries.). This is the underlying object with id, value and 1043 * extensions. The accessor "getSequence" gives direct access to 1044 * the value 1045 */ 1046 public CareTeamComponent setSequenceElement(PositiveIntType value) { 1047 this.sequence = value; 1048 return this; 1049 } 1050 1051 /** 1052 * @return A number to uniquely identify care team entries. 1053 */ 1054 public int getSequence() { 1055 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1056 } 1057 1058 /** 1059 * @param value A number to uniquely identify care team entries. 1060 */ 1061 public CareTeamComponent setSequence(int value) { 1062 if (this.sequence == null) 1063 this.sequence = new PositiveIntType(); 1064 this.sequence.setValue(value); 1065 return this; 1066 } 1067 1068 /** 1069 * @return {@link #provider} (Member of the team who provided the product or 1070 * service.) 1071 */ 1072 public Reference getProvider() { 1073 if (this.provider == null) 1074 if (Configuration.errorOnAutoCreate()) 1075 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 1076 else if (Configuration.doAutoCreate()) 1077 this.provider = new Reference(); // cc 1078 return this.provider; 1079 } 1080 1081 public boolean hasProvider() { 1082 return this.provider != null && !this.provider.isEmpty(); 1083 } 1084 1085 /** 1086 * @param value {@link #provider} (Member of the team who provided the product 1087 * or service.) 1088 */ 1089 public CareTeamComponent setProvider(Reference value) { 1090 this.provider = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return {@link #provider} The actual object that is the target of the 1096 * reference. The reference library doesn't populate this, but you can 1097 * use it to hold the resource if you resolve it. (Member of the team 1098 * who provided the product or service.) 1099 */ 1100 public Resource getProviderTarget() { 1101 return this.providerTarget; 1102 } 1103 1104 /** 1105 * @param value {@link #provider} The actual object that is the target of the 1106 * reference. The reference library doesn't use these, but you can 1107 * use it to hold the resource if you resolve it. (Member of the 1108 * team who provided the product or service.) 1109 */ 1110 public CareTeamComponent setProviderTarget(Resource value) { 1111 this.providerTarget = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return {@link #responsible} (The party who is billing and/or responsible for 1117 * the claimed products or services.). This is the underlying object 1118 * with id, value and extensions. The accessor "getResponsible" gives 1119 * direct access to the value 1120 */ 1121 public BooleanType getResponsibleElement() { 1122 if (this.responsible == null) 1123 if (Configuration.errorOnAutoCreate()) 1124 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1125 else if (Configuration.doAutoCreate()) 1126 this.responsible = new BooleanType(); // bb 1127 return this.responsible; 1128 } 1129 1130 public boolean hasResponsibleElement() { 1131 return this.responsible != null && !this.responsible.isEmpty(); 1132 } 1133 1134 public boolean hasResponsible() { 1135 return this.responsible != null && !this.responsible.isEmpty(); 1136 } 1137 1138 /** 1139 * @param value {@link #responsible} (The party who is billing and/or 1140 * responsible for the claimed products or services.). This is the 1141 * underlying object with id, value and extensions. The accessor 1142 * "getResponsible" gives direct access to the value 1143 */ 1144 public CareTeamComponent setResponsibleElement(BooleanType value) { 1145 this.responsible = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return The party who is billing and/or responsible for the claimed products 1151 * or services. 1152 */ 1153 public boolean getResponsible() { 1154 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1155 } 1156 1157 /** 1158 * @param value The party who is billing and/or responsible for the claimed 1159 * products or services. 1160 */ 1161 public CareTeamComponent setResponsible(boolean value) { 1162 if (this.responsible == null) 1163 this.responsible = new BooleanType(); 1164 this.responsible.setValue(value); 1165 return this; 1166 } 1167 1168 /** 1169 * @return {@link #role} (The lead, assisting or supervising practitioner and 1170 * their discipline if a multidisciplinary team.) 1171 */ 1172 public CodeableConcept getRole() { 1173 if (this.role == null) 1174 if (Configuration.errorOnAutoCreate()) 1175 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1176 else if (Configuration.doAutoCreate()) 1177 this.role = new CodeableConcept(); // cc 1178 return this.role; 1179 } 1180 1181 public boolean hasRole() { 1182 return this.role != null && !this.role.isEmpty(); 1183 } 1184 1185 /** 1186 * @param value {@link #role} (The lead, assisting or supervising practitioner 1187 * and their discipline if a multidisciplinary team.) 1188 */ 1189 public CareTeamComponent setRole(CodeableConcept value) { 1190 this.role = value; 1191 return this; 1192 } 1193 1194 /** 1195 * @return {@link #qualification} (The qualification of the practitioner which 1196 * is applicable for this service.) 1197 */ 1198 public CodeableConcept getQualification() { 1199 if (this.qualification == null) 1200 if (Configuration.errorOnAutoCreate()) 1201 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1202 else if (Configuration.doAutoCreate()) 1203 this.qualification = new CodeableConcept(); // cc 1204 return this.qualification; 1205 } 1206 1207 public boolean hasQualification() { 1208 return this.qualification != null && !this.qualification.isEmpty(); 1209 } 1210 1211 /** 1212 * @param value {@link #qualification} (The qualification of the practitioner 1213 * which is applicable for this service.) 1214 */ 1215 public CareTeamComponent setQualification(CodeableConcept value) { 1216 this.qualification = value; 1217 return this; 1218 } 1219 1220 protected void listChildren(List<Property> children) { 1221 super.listChildren(children); 1222 children.add( 1223 new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 1224 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 1225 "Member of the team who provided the product or service.", 0, 1, provider)); 1226 children.add(new Property("responsible", "boolean", 1227 "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 1228 children.add(new Property("role", "CodeableConcept", 1229 "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, 1230 role)); 1231 children.add(new Property("qualification", "CodeableConcept", 1232 "The qualification of the practitioner which is applicable for this service.", 0, 1, qualification)); 1233 } 1234 1235 @Override 1236 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1237 switch (_hash) { 1238 case 1349547969: 1239 /* sequence */ return new Property("sequence", "positiveInt", 1240 "A number to uniquely identify care team entries.", 0, 1, sequence); 1241 case -987494927: 1242 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 1243 "Member of the team who provided the product or service.", 0, 1, provider); 1244 case 1847674614: 1245 /* responsible */ return new Property("responsible", "boolean", 1246 "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 1247 case 3506294: 1248 /* role */ return new Property("role", "CodeableConcept", 1249 "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, 1250 role); 1251 case -631333393: 1252 /* qualification */ return new Property("qualification", "CodeableConcept", 1253 "The qualification of the practitioner which is applicable for this service.", 0, 1, qualification); 1254 default: 1255 return super.getNamedProperty(_hash, _name, _checkValid); 1256 } 1257 1258 } 1259 1260 @Override 1261 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1262 switch (hash) { 1263 case 1349547969: 1264 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 1265 case -987494927: 1266 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 1267 case 1847674614: 1268 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // BooleanType 1269 case 3506294: 1270 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 1271 case -631333393: 1272 /* qualification */ return this.qualification == null ? new Base[0] : new Base[] { this.qualification }; // CodeableConcept 1273 default: 1274 return super.getProperty(hash, name, checkValid); 1275 } 1276 1277 } 1278 1279 @Override 1280 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1281 switch (hash) { 1282 case 1349547969: // sequence 1283 this.sequence = castToPositiveInt(value); // PositiveIntType 1284 return value; 1285 case -987494927: // provider 1286 this.provider = castToReference(value); // Reference 1287 return value; 1288 case 1847674614: // responsible 1289 this.responsible = castToBoolean(value); // BooleanType 1290 return value; 1291 case 3506294: // role 1292 this.role = castToCodeableConcept(value); // CodeableConcept 1293 return value; 1294 case -631333393: // qualification 1295 this.qualification = castToCodeableConcept(value); // CodeableConcept 1296 return value; 1297 default: 1298 return super.setProperty(hash, name, value); 1299 } 1300 1301 } 1302 1303 @Override 1304 public Base setProperty(String name, Base value) throws FHIRException { 1305 if (name.equals("sequence")) { 1306 this.sequence = castToPositiveInt(value); // PositiveIntType 1307 } else if (name.equals("provider")) { 1308 this.provider = castToReference(value); // Reference 1309 } else if (name.equals("responsible")) { 1310 this.responsible = castToBoolean(value); // BooleanType 1311 } else if (name.equals("role")) { 1312 this.role = castToCodeableConcept(value); // CodeableConcept 1313 } else if (name.equals("qualification")) { 1314 this.qualification = castToCodeableConcept(value); // CodeableConcept 1315 } else 1316 return super.setProperty(name, value); 1317 return value; 1318 } 1319 1320 @Override 1321 public void removeChild(String name, Base value) throws FHIRException { 1322 if (name.equals("sequence")) { 1323 this.sequence = null; 1324 } else if (name.equals("provider")) { 1325 this.provider = null; 1326 } else if (name.equals("responsible")) { 1327 this.responsible = null; 1328 } else if (name.equals("role")) { 1329 this.role = null; 1330 } else if (name.equals("qualification")) { 1331 this.qualification = null; 1332 } else 1333 super.removeChild(name, value); 1334 1335 } 1336 1337 @Override 1338 public Base makeProperty(int hash, String name) throws FHIRException { 1339 switch (hash) { 1340 case 1349547969: 1341 return getSequenceElement(); 1342 case -987494927: 1343 return getProvider(); 1344 case 1847674614: 1345 return getResponsibleElement(); 1346 case 3506294: 1347 return getRole(); 1348 case -631333393: 1349 return getQualification(); 1350 default: 1351 return super.makeProperty(hash, name); 1352 } 1353 1354 } 1355 1356 @Override 1357 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1358 switch (hash) { 1359 case 1349547969: 1360 /* sequence */ return new String[] { "positiveInt" }; 1361 case -987494927: 1362 /* provider */ return new String[] { "Reference" }; 1363 case 1847674614: 1364 /* responsible */ return new String[] { "boolean" }; 1365 case 3506294: 1366 /* role */ return new String[] { "CodeableConcept" }; 1367 case -631333393: 1368 /* qualification */ return new String[] { "CodeableConcept" }; 1369 default: 1370 return super.getTypesForProperty(hash, name); 1371 } 1372 1373 } 1374 1375 @Override 1376 public Base addChild(String name) throws FHIRException { 1377 if (name.equals("sequence")) { 1378 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1379 } else if (name.equals("provider")) { 1380 this.provider = new Reference(); 1381 return this.provider; 1382 } else if (name.equals("responsible")) { 1383 throw new FHIRException("Cannot call addChild on a singleton property Claim.responsible"); 1384 } else if (name.equals("role")) { 1385 this.role = new CodeableConcept(); 1386 return this.role; 1387 } else if (name.equals("qualification")) { 1388 this.qualification = new CodeableConcept(); 1389 return this.qualification; 1390 } else 1391 return super.addChild(name); 1392 } 1393 1394 public CareTeamComponent copy() { 1395 CareTeamComponent dst = new CareTeamComponent(); 1396 copyValues(dst); 1397 return dst; 1398 } 1399 1400 public void copyValues(CareTeamComponent dst) { 1401 super.copyValues(dst); 1402 dst.sequence = sequence == null ? null : sequence.copy(); 1403 dst.provider = provider == null ? null : provider.copy(); 1404 dst.responsible = responsible == null ? null : responsible.copy(); 1405 dst.role = role == null ? null : role.copy(); 1406 dst.qualification = qualification == null ? null : qualification.copy(); 1407 } 1408 1409 @Override 1410 public boolean equalsDeep(Base other_) { 1411 if (!super.equalsDeep(other_)) 1412 return false; 1413 if (!(other_ instanceof CareTeamComponent)) 1414 return false; 1415 CareTeamComponent o = (CareTeamComponent) other_; 1416 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) 1417 && compareDeep(responsible, o.responsible, true) && compareDeep(role, o.role, true) 1418 && compareDeep(qualification, o.qualification, true); 1419 } 1420 1421 @Override 1422 public boolean equalsShallow(Base other_) { 1423 if (!super.equalsShallow(other_)) 1424 return false; 1425 if (!(other_ instanceof CareTeamComponent)) 1426 return false; 1427 CareTeamComponent o = (CareTeamComponent) other_; 1428 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true); 1429 } 1430 1431 public boolean isEmpty() { 1432 return super.isEmpty() 1433 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible, role, qualification); 1434 } 1435 1436 public String fhirType() { 1437 return "Claim.careTeam"; 1438 1439 } 1440 1441 } 1442 1443 @Block() 1444 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1445 /** 1446 * A number to uniquely identify supporting information entries. 1447 */ 1448 @Child(name = "sequence", type = { 1449 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1450 @Description(shortDefinition = "Information instance identifier", formalDefinition = "A number to uniquely identify supporting information entries.") 1451 protected PositiveIntType sequence; 1452 1453 /** 1454 * The general class of the information supplied: information; exception; 1455 * accident, employment; onset, etc. 1456 */ 1457 @Child(name = "category", type = { 1458 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1459 @Description(shortDefinition = "Classification of the supplied information", formalDefinition = "The general class of the information supplied: information; exception; accident, employment; onset, etc.") 1460 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-informationcategory") 1461 protected CodeableConcept category; 1462 1463 /** 1464 * System and code pertaining to the specific information regarding special 1465 * conditions relating to the setting, treatment or patient for which care is 1466 * sought. 1467 */ 1468 @Child(name = "code", type = { 1469 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1470 @Description(shortDefinition = "Type of information", formalDefinition = "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.") 1471 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-exception") 1472 protected CodeableConcept code; 1473 1474 /** 1475 * The date when or period to which this information refers. 1476 */ 1477 @Child(name = "timing", type = { DateType.class, 1478 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1479 @Description(shortDefinition = "When it occurred", formalDefinition = "The date when or period to which this information refers.") 1480 protected Type timing; 1481 1482 /** 1483 * Additional data or information such as resources, documents, images etc. 1484 * including references to the data or the actual inclusion of the data. 1485 */ 1486 @Child(name = "value", type = { BooleanType.class, StringType.class, Quantity.class, Attachment.class, 1487 Reference.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1488 @Description(shortDefinition = "Data to be provided", formalDefinition = "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.") 1489 protected Type value; 1490 1491 /** 1492 * Provides the reason in the situation where a reason code is required in 1493 * addition to the content. 1494 */ 1495 @Child(name = "reason", type = { 1496 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1497 @Description(shortDefinition = "Explanation for the information", formalDefinition = "Provides the reason in the situation where a reason code is required in addition to the content.") 1498 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1499 protected CodeableConcept reason; 1500 1501 private static final long serialVersionUID = -518630232L; 1502 1503 /** 1504 * Constructor 1505 */ 1506 public SupportingInformationComponent() { 1507 super(); 1508 } 1509 1510 /** 1511 * Constructor 1512 */ 1513 public SupportingInformationComponent(PositiveIntType sequence, CodeableConcept category) { 1514 super(); 1515 this.sequence = sequence; 1516 this.category = category; 1517 } 1518 1519 /** 1520 * @return {@link #sequence} (A number to uniquely identify supporting 1521 * information entries.). This is the underlying object with id, value 1522 * and extensions. The accessor "getSequence" gives direct access to the 1523 * value 1524 */ 1525 public PositiveIntType getSequenceElement() { 1526 if (this.sequence == null) 1527 if (Configuration.errorOnAutoCreate()) 1528 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1529 else if (Configuration.doAutoCreate()) 1530 this.sequence = new PositiveIntType(); // bb 1531 return this.sequence; 1532 } 1533 1534 public boolean hasSequenceElement() { 1535 return this.sequence != null && !this.sequence.isEmpty(); 1536 } 1537 1538 public boolean hasSequence() { 1539 return this.sequence != null && !this.sequence.isEmpty(); 1540 } 1541 1542 /** 1543 * @param value {@link #sequence} (A number to uniquely identify supporting 1544 * information entries.). This is the underlying object with id, 1545 * value and extensions. The accessor "getSequence" gives direct 1546 * access to the value 1547 */ 1548 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1549 this.sequence = value; 1550 return this; 1551 } 1552 1553 /** 1554 * @return A number to uniquely identify supporting information entries. 1555 */ 1556 public int getSequence() { 1557 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1558 } 1559 1560 /** 1561 * @param value A number to uniquely identify supporting information entries. 1562 */ 1563 public SupportingInformationComponent setSequence(int value) { 1564 if (this.sequence == null) 1565 this.sequence = new PositiveIntType(); 1566 this.sequence.setValue(value); 1567 return this; 1568 } 1569 1570 /** 1571 * @return {@link #category} (The general class of the information supplied: 1572 * information; exception; accident, employment; onset, etc.) 1573 */ 1574 public CodeableConcept getCategory() { 1575 if (this.category == null) 1576 if (Configuration.errorOnAutoCreate()) 1577 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1578 else if (Configuration.doAutoCreate()) 1579 this.category = new CodeableConcept(); // cc 1580 return this.category; 1581 } 1582 1583 public boolean hasCategory() { 1584 return this.category != null && !this.category.isEmpty(); 1585 } 1586 1587 /** 1588 * @param value {@link #category} (The general class of the information 1589 * supplied: information; exception; accident, employment; onset, 1590 * etc.) 1591 */ 1592 public SupportingInformationComponent setCategory(CodeableConcept value) { 1593 this.category = value; 1594 return this; 1595 } 1596 1597 /** 1598 * @return {@link #code} (System and code pertaining to the specific information 1599 * regarding special conditions relating to the setting, treatment or 1600 * patient for which care is sought.) 1601 */ 1602 public CodeableConcept getCode() { 1603 if (this.code == null) 1604 if (Configuration.errorOnAutoCreate()) 1605 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1606 else if (Configuration.doAutoCreate()) 1607 this.code = new CodeableConcept(); // cc 1608 return this.code; 1609 } 1610 1611 public boolean hasCode() { 1612 return this.code != null && !this.code.isEmpty(); 1613 } 1614 1615 /** 1616 * @param value {@link #code} (System and code pertaining to the specific 1617 * information regarding special conditions relating to the 1618 * setting, treatment or patient for which care is sought.) 1619 */ 1620 public SupportingInformationComponent setCode(CodeableConcept value) { 1621 this.code = value; 1622 return this; 1623 } 1624 1625 /** 1626 * @return {@link #timing} (The date when or period to which this information 1627 * refers.) 1628 */ 1629 public Type getTiming() { 1630 return this.timing; 1631 } 1632 1633 /** 1634 * @return {@link #timing} (The date when or period to which this information 1635 * refers.) 1636 */ 1637 public DateType getTimingDateType() throws FHIRException { 1638 if (this.timing == null) 1639 this.timing = new DateType(); 1640 if (!(this.timing instanceof DateType)) 1641 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.timing.getClass().getName() 1642 + " was encountered"); 1643 return (DateType) this.timing; 1644 } 1645 1646 public boolean hasTimingDateType() { 1647 return this != null && this.timing instanceof DateType; 1648 } 1649 1650 /** 1651 * @return {@link #timing} (The date when or period to which this information 1652 * refers.) 1653 */ 1654 public Period getTimingPeriod() throws FHIRException { 1655 if (this.timing == null) 1656 this.timing = new Period(); 1657 if (!(this.timing instanceof Period)) 1658 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 1659 + " was encountered"); 1660 return (Period) this.timing; 1661 } 1662 1663 public boolean hasTimingPeriod() { 1664 return this != null && this.timing instanceof Period; 1665 } 1666 1667 public boolean hasTiming() { 1668 return this.timing != null && !this.timing.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #timing} (The date when or period to which this 1673 * information refers.) 1674 */ 1675 public SupportingInformationComponent setTiming(Type value) { 1676 if (value != null && !(value instanceof DateType || value instanceof Period)) 1677 throw new Error("Not the right type for Claim.supportingInfo.timing[x]: " + value.fhirType()); 1678 this.timing = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return {@link #value} (Additional data or information such as resources, 1684 * documents, images etc. including references to the data or the actual 1685 * inclusion of the data.) 1686 */ 1687 public Type getValue() { 1688 return this.value; 1689 } 1690 1691 /** 1692 * @return {@link #value} (Additional data or information such as resources, 1693 * documents, images etc. including references to the data or the actual 1694 * inclusion of the data.) 1695 */ 1696 public BooleanType getValueBooleanType() throws FHIRException { 1697 if (this.value == null) 1698 this.value = new BooleanType(); 1699 if (!(this.value instanceof BooleanType)) 1700 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1701 + this.value.getClass().getName() + " was encountered"); 1702 return (BooleanType) this.value; 1703 } 1704 1705 public boolean hasValueBooleanType() { 1706 return this != null && this.value instanceof BooleanType; 1707 } 1708 1709 /** 1710 * @return {@link #value} (Additional data or information such as resources, 1711 * documents, images etc. including references to the data or the actual 1712 * inclusion of the data.) 1713 */ 1714 public StringType getValueStringType() throws FHIRException { 1715 if (this.value == null) 1716 this.value = new StringType(); 1717 if (!(this.value instanceof StringType)) 1718 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1719 + this.value.getClass().getName() + " was encountered"); 1720 return (StringType) this.value; 1721 } 1722 1723 public boolean hasValueStringType() { 1724 return this != null && this.value instanceof StringType; 1725 } 1726 1727 /** 1728 * @return {@link #value} (Additional data or information such as resources, 1729 * documents, images etc. including references to the data or the actual 1730 * inclusion of the data.) 1731 */ 1732 public Quantity getValueQuantity() throws FHIRException { 1733 if (this.value == null) 1734 this.value = new Quantity(); 1735 if (!(this.value instanceof Quantity)) 1736 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1737 + " was encountered"); 1738 return (Quantity) this.value; 1739 } 1740 1741 public boolean hasValueQuantity() { 1742 return this != null && this.value instanceof Quantity; 1743 } 1744 1745 /** 1746 * @return {@link #value} (Additional data or information such as resources, 1747 * documents, images etc. including references to the data or the actual 1748 * inclusion of the data.) 1749 */ 1750 public Attachment getValueAttachment() throws FHIRException { 1751 if (this.value == null) 1752 this.value = new Attachment(); 1753 if (!(this.value instanceof Attachment)) 1754 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 1755 + this.value.getClass().getName() + " was encountered"); 1756 return (Attachment) this.value; 1757 } 1758 1759 public boolean hasValueAttachment() { 1760 return this != null && this.value instanceof Attachment; 1761 } 1762 1763 /** 1764 * @return {@link #value} (Additional data or information such as resources, 1765 * documents, images etc. including references to the data or the actual 1766 * inclusion of the data.) 1767 */ 1768 public Reference getValueReference() throws FHIRException { 1769 if (this.value == null) 1770 this.value = new Reference(); 1771 if (!(this.value instanceof Reference)) 1772 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 1773 + " was encountered"); 1774 return (Reference) this.value; 1775 } 1776 1777 public boolean hasValueReference() { 1778 return this != null && this.value instanceof Reference; 1779 } 1780 1781 public boolean hasValue() { 1782 return this.value != null && !this.value.isEmpty(); 1783 } 1784 1785 /** 1786 * @param value {@link #value} (Additional data or information such as 1787 * resources, documents, images etc. including references to the 1788 * data or the actual inclusion of the data.) 1789 */ 1790 public SupportingInformationComponent setValue(Type value) { 1791 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity 1792 || value instanceof Attachment || value instanceof Reference)) 1793 throw new Error("Not the right type for Claim.supportingInfo.value[x]: " + value.fhirType()); 1794 this.value = value; 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #reason} (Provides the reason in the situation where a reason 1800 * code is required in addition to the content.) 1801 */ 1802 public CodeableConcept getReason() { 1803 if (this.reason == null) 1804 if (Configuration.errorOnAutoCreate()) 1805 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1806 else if (Configuration.doAutoCreate()) 1807 this.reason = new CodeableConcept(); // cc 1808 return this.reason; 1809 } 1810 1811 public boolean hasReason() { 1812 return this.reason != null && !this.reason.isEmpty(); 1813 } 1814 1815 /** 1816 * @param value {@link #reason} (Provides the reason in the situation where a 1817 * reason code is required in addition to the content.) 1818 */ 1819 public SupportingInformationComponent setReason(CodeableConcept value) { 1820 this.reason = value; 1821 return this; 1822 } 1823 1824 protected void listChildren(List<Property> children) { 1825 super.listChildren(children); 1826 children.add(new Property("sequence", "positiveInt", 1827 "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1828 children.add(new Property("category", "CodeableConcept", 1829 "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1830 1, category)); 1831 children.add(new Property("code", "CodeableConcept", 1832 "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 1833 0, 1, code)); 1834 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 1835 0, 1, timing)); 1836 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1837 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1838 0, 1, value)); 1839 children.add(new Property("reason", "CodeableConcept", 1840 "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, 1841 reason)); 1842 } 1843 1844 @Override 1845 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1846 switch (_hash) { 1847 case 1349547969: 1848 /* sequence */ return new Property("sequence", "positiveInt", 1849 "A number to uniquely identify supporting information entries.", 0, 1, sequence); 1850 case 50511102: 1851 /* category */ return new Property("category", "CodeableConcept", 1852 "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 1853 0, 1, category); 1854 case 3059181: 1855 /* code */ return new Property("code", "CodeableConcept", 1856 "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 1857 0, 1, code); 1858 case 164632566: 1859 /* timing[x] */ return new Property("timing[x]", "date|Period", 1860 "The date when or period to which this information refers.", 0, 1, timing); 1861 case -873664438: 1862 /* timing */ return new Property("timing[x]", "date|Period", 1863 "The date when or period to which this information refers.", 0, 1, timing); 1864 case 807935768: 1865 /* timingDate */ return new Property("timing[x]", "date|Period", 1866 "The date when or period to which this information refers.", 0, 1, timing); 1867 case -615615829: 1868 /* timingPeriod */ return new Property("timing[x]", "date|Period", 1869 "The date when or period to which this information refers.", 0, 1, timing); 1870 case -1410166417: 1871 /* value[x] */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1872 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1873 0, 1, value); 1874 case 111972721: 1875 /* value */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1876 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1877 0, 1, value); 1878 case 733421943: 1879 /* valueBoolean */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1880 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1881 0, 1, value); 1882 case -1424603934: 1883 /* valueString */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1884 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1885 0, 1, value); 1886 case -2029823716: 1887 /* valueQuantity */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1888 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1889 0, 1, value); 1890 case -475566732: 1891 /* valueAttachment */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1892 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1893 0, 1, value); 1894 case 1755241690: 1895 /* valueReference */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1896 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1897 0, 1, value); 1898 case -934964668: 1899 /* reason */ return new Property("reason", "CodeableConcept", 1900 "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, 1901 reason); 1902 default: 1903 return super.getNamedProperty(_hash, _name, _checkValid); 1904 } 1905 1906 } 1907 1908 @Override 1909 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1910 switch (hash) { 1911 case 1349547969: 1912 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 1913 case 50511102: 1914 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1915 case 3059181: 1916 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1917 case -873664438: 1918 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 1919 case 111972721: 1920 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1921 case -934964668: 1922 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 1923 default: 1924 return super.getProperty(hash, name, checkValid); 1925 } 1926 1927 } 1928 1929 @Override 1930 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1931 switch (hash) { 1932 case 1349547969: // sequence 1933 this.sequence = castToPositiveInt(value); // PositiveIntType 1934 return value; 1935 case 50511102: // category 1936 this.category = castToCodeableConcept(value); // CodeableConcept 1937 return value; 1938 case 3059181: // code 1939 this.code = castToCodeableConcept(value); // CodeableConcept 1940 return value; 1941 case -873664438: // timing 1942 this.timing = castToType(value); // Type 1943 return value; 1944 case 111972721: // value 1945 this.value = castToType(value); // Type 1946 return value; 1947 case -934964668: // reason 1948 this.reason = castToCodeableConcept(value); // CodeableConcept 1949 return value; 1950 default: 1951 return super.setProperty(hash, name, value); 1952 } 1953 1954 } 1955 1956 @Override 1957 public Base setProperty(String name, Base value) throws FHIRException { 1958 if (name.equals("sequence")) { 1959 this.sequence = castToPositiveInt(value); // PositiveIntType 1960 } else if (name.equals("category")) { 1961 this.category = castToCodeableConcept(value); // CodeableConcept 1962 } else if (name.equals("code")) { 1963 this.code = castToCodeableConcept(value); // CodeableConcept 1964 } else if (name.equals("timing[x]")) { 1965 this.timing = castToType(value); // Type 1966 } else if (name.equals("value[x]")) { 1967 this.value = castToType(value); // Type 1968 } else if (name.equals("reason")) { 1969 this.reason = castToCodeableConcept(value); // CodeableConcept 1970 } else 1971 return super.setProperty(name, value); 1972 return value; 1973 } 1974 1975 @Override 1976 public void removeChild(String name, Base value) throws FHIRException { 1977 if (name.equals("sequence")) { 1978 this.sequence = null; 1979 } else if (name.equals("category")) { 1980 this.category = null; 1981 } else if (name.equals("code")) { 1982 this.code = null; 1983 } else if (name.equals("timing[x]")) { 1984 this.timing = null; 1985 } else if (name.equals("value[x]")) { 1986 this.value = null; 1987 } else if (name.equals("reason")) { 1988 this.reason = null; 1989 } else 1990 super.removeChild(name, value); 1991 1992 } 1993 1994 @Override 1995 public Base makeProperty(int hash, String name) throws FHIRException { 1996 switch (hash) { 1997 case 1349547969: 1998 return getSequenceElement(); 1999 case 50511102: 2000 return getCategory(); 2001 case 3059181: 2002 return getCode(); 2003 case 164632566: 2004 return getTiming(); 2005 case -873664438: 2006 return getTiming(); 2007 case -1410166417: 2008 return getValue(); 2009 case 111972721: 2010 return getValue(); 2011 case -934964668: 2012 return getReason(); 2013 default: 2014 return super.makeProperty(hash, name); 2015 } 2016 2017 } 2018 2019 @Override 2020 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2021 switch (hash) { 2022 case 1349547969: 2023 /* sequence */ return new String[] { "positiveInt" }; 2024 case 50511102: 2025 /* category */ return new String[] { "CodeableConcept" }; 2026 case 3059181: 2027 /* code */ return new String[] { "CodeableConcept" }; 2028 case -873664438: 2029 /* timing */ return new String[] { "date", "Period" }; 2030 case 111972721: 2031 /* value */ return new String[] { "boolean", "string", "Quantity", "Attachment", "Reference" }; 2032 case -934964668: 2033 /* reason */ return new String[] { "CodeableConcept" }; 2034 default: 2035 return super.getTypesForProperty(hash, name); 2036 } 2037 2038 } 2039 2040 @Override 2041 public Base addChild(String name) throws FHIRException { 2042 if (name.equals("sequence")) { 2043 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2044 } else if (name.equals("category")) { 2045 this.category = new CodeableConcept(); 2046 return this.category; 2047 } else if (name.equals("code")) { 2048 this.code = new CodeableConcept(); 2049 return this.code; 2050 } else if (name.equals("timingDate")) { 2051 this.timing = new DateType(); 2052 return this.timing; 2053 } else if (name.equals("timingPeriod")) { 2054 this.timing = new Period(); 2055 return this.timing; 2056 } else if (name.equals("valueBoolean")) { 2057 this.value = new BooleanType(); 2058 return this.value; 2059 } else if (name.equals("valueString")) { 2060 this.value = new StringType(); 2061 return this.value; 2062 } else if (name.equals("valueQuantity")) { 2063 this.value = new Quantity(); 2064 return this.value; 2065 } else if (name.equals("valueAttachment")) { 2066 this.value = new Attachment(); 2067 return this.value; 2068 } else if (name.equals("valueReference")) { 2069 this.value = new Reference(); 2070 return this.value; 2071 } else if (name.equals("reason")) { 2072 this.reason = new CodeableConcept(); 2073 return this.reason; 2074 } else 2075 return super.addChild(name); 2076 } 2077 2078 public SupportingInformationComponent copy() { 2079 SupportingInformationComponent dst = new SupportingInformationComponent(); 2080 copyValues(dst); 2081 return dst; 2082 } 2083 2084 public void copyValues(SupportingInformationComponent dst) { 2085 super.copyValues(dst); 2086 dst.sequence = sequence == null ? null : sequence.copy(); 2087 dst.category = category == null ? null : category.copy(); 2088 dst.code = code == null ? null : code.copy(); 2089 dst.timing = timing == null ? null : timing.copy(); 2090 dst.value = value == null ? null : value.copy(); 2091 dst.reason = reason == null ? null : reason.copy(); 2092 } 2093 2094 @Override 2095 public boolean equalsDeep(Base other_) { 2096 if (!super.equalsDeep(other_)) 2097 return false; 2098 if (!(other_ instanceof SupportingInformationComponent)) 2099 return false; 2100 SupportingInformationComponent o = (SupportingInformationComponent) other_; 2101 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) 2102 && compareDeep(code, o.code, true) && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) 2103 && compareDeep(reason, o.reason, true); 2104 } 2105 2106 @Override 2107 public boolean equalsShallow(Base other_) { 2108 if (!super.equalsShallow(other_)) 2109 return false; 2110 if (!(other_ instanceof SupportingInformationComponent)) 2111 return false; 2112 SupportingInformationComponent o = (SupportingInformationComponent) other_; 2113 return compareValues(sequence, o.sequence, true); 2114 } 2115 2116 public boolean isEmpty() { 2117 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code, timing, value, reason); 2118 } 2119 2120 public String fhirType() { 2121 return "Claim.supportingInfo"; 2122 2123 } 2124 2125 } 2126 2127 @Block() 2128 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 2129 /** 2130 * A number to uniquely identify diagnosis entries. 2131 */ 2132 @Child(name = "sequence", type = { 2133 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2134 @Description(shortDefinition = "Diagnosis instance identifier", formalDefinition = "A number to uniquely identify diagnosis entries.") 2135 protected PositiveIntType sequence; 2136 2137 /** 2138 * The nature of illness or problem in a coded form or as a reference to an 2139 * external defined Condition. 2140 */ 2141 @Child(name = "diagnosis", type = { CodeableConcept.class, 2142 Condition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2143 @Description(shortDefinition = "Nature of illness or problem", formalDefinition = "The nature of illness or problem in a coded form or as a reference to an external defined Condition.") 2144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10") 2145 protected Type diagnosis; 2146 2147 /** 2148 * When the condition was observed or the relative ranking. 2149 */ 2150 @Child(name = "type", type = { 2151 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2152 @Description(shortDefinition = "Timing or nature of the diagnosis", formalDefinition = "When the condition was observed or the relative ranking.") 2153 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosistype") 2154 protected List<CodeableConcept> type; 2155 2156 /** 2157 * Indication of whether the diagnosis was present on admission to a facility. 2158 */ 2159 @Child(name = "onAdmission", type = { 2160 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2161 @Description(shortDefinition = "Present on admission", formalDefinition = "Indication of whether the diagnosis was present on admission to a facility.") 2162 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 2163 protected CodeableConcept onAdmission; 2164 2165 /** 2166 * A package billing code or bundle code used to group products and services to 2167 * a particular health condition (such as heart attack) which is based on a 2168 * predetermined grouping code system. 2169 */ 2170 @Child(name = "packageCode", type = { 2171 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2172 @Description(shortDefinition = "Package billing code", formalDefinition = "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.") 2173 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 2174 protected CodeableConcept packageCode; 2175 2176 private static final long serialVersionUID = 2120593974L; 2177 2178 /** 2179 * Constructor 2180 */ 2181 public DiagnosisComponent() { 2182 super(); 2183 } 2184 2185 /** 2186 * Constructor 2187 */ 2188 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 2189 super(); 2190 this.sequence = sequence; 2191 this.diagnosis = diagnosis; 2192 } 2193 2194 /** 2195 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). 2196 * This is the underlying object with id, value and extensions. The 2197 * accessor "getSequence" gives direct access to the value 2198 */ 2199 public PositiveIntType getSequenceElement() { 2200 if (this.sequence == null) 2201 if (Configuration.errorOnAutoCreate()) 2202 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 2203 else if (Configuration.doAutoCreate()) 2204 this.sequence = new PositiveIntType(); // bb 2205 return this.sequence; 2206 } 2207 2208 public boolean hasSequenceElement() { 2209 return this.sequence != null && !this.sequence.isEmpty(); 2210 } 2211 2212 public boolean hasSequence() { 2213 return this.sequence != null && !this.sequence.isEmpty(); 2214 } 2215 2216 /** 2217 * @param value {@link #sequence} (A number to uniquely identify diagnosis 2218 * entries.). This is the underlying object with id, value and 2219 * extensions. The accessor "getSequence" gives direct access to 2220 * the value 2221 */ 2222 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 2223 this.sequence = value; 2224 return this; 2225 } 2226 2227 /** 2228 * @return A number to uniquely identify diagnosis entries. 2229 */ 2230 public int getSequence() { 2231 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2232 } 2233 2234 /** 2235 * @param value A number to uniquely identify diagnosis entries. 2236 */ 2237 public DiagnosisComponent setSequence(int value) { 2238 if (this.sequence == null) 2239 this.sequence = new PositiveIntType(); 2240 this.sequence.setValue(value); 2241 return this; 2242 } 2243 2244 /** 2245 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2246 * or as a reference to an external defined Condition.) 2247 */ 2248 public Type getDiagnosis() { 2249 return this.diagnosis; 2250 } 2251 2252 /** 2253 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2254 * or as a reference to an external defined Condition.) 2255 */ 2256 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 2257 if (this.diagnosis == null) 2258 this.diagnosis = new CodeableConcept(); 2259 if (!(this.diagnosis instanceof CodeableConcept)) 2260 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2261 + this.diagnosis.getClass().getName() + " was encountered"); 2262 return (CodeableConcept) this.diagnosis; 2263 } 2264 2265 public boolean hasDiagnosisCodeableConcept() { 2266 return this != null && this.diagnosis instanceof CodeableConcept; 2267 } 2268 2269 /** 2270 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2271 * or as a reference to an external defined Condition.) 2272 */ 2273 public Reference getDiagnosisReference() throws FHIRException { 2274 if (this.diagnosis == null) 2275 this.diagnosis = new Reference(); 2276 if (!(this.diagnosis instanceof Reference)) 2277 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2278 + this.diagnosis.getClass().getName() + " was encountered"); 2279 return (Reference) this.diagnosis; 2280 } 2281 2282 public boolean hasDiagnosisReference() { 2283 return this != null && this.diagnosis instanceof Reference; 2284 } 2285 2286 public boolean hasDiagnosis() { 2287 return this.diagnosis != null && !this.diagnosis.isEmpty(); 2288 } 2289 2290 /** 2291 * @param value {@link #diagnosis} (The nature of illness or problem in a coded 2292 * form or as a reference to an external defined Condition.) 2293 */ 2294 public DiagnosisComponent setDiagnosis(Type value) { 2295 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2296 throw new Error("Not the right type for Claim.diagnosis.diagnosis[x]: " + value.fhirType()); 2297 this.diagnosis = value; 2298 return this; 2299 } 2300 2301 /** 2302 * @return {@link #type} (When the condition was observed or the relative 2303 * ranking.) 2304 */ 2305 public List<CodeableConcept> getType() { 2306 if (this.type == null) 2307 this.type = new ArrayList<CodeableConcept>(); 2308 return this.type; 2309 } 2310 2311 /** 2312 * @return Returns a reference to <code>this</code> for easy method chaining 2313 */ 2314 public DiagnosisComponent setType(List<CodeableConcept> theType) { 2315 this.type = theType; 2316 return this; 2317 } 2318 2319 public boolean hasType() { 2320 if (this.type == null) 2321 return false; 2322 for (CodeableConcept item : this.type) 2323 if (!item.isEmpty()) 2324 return true; 2325 return false; 2326 } 2327 2328 public CodeableConcept addType() { // 3 2329 CodeableConcept t = new CodeableConcept(); 2330 if (this.type == null) 2331 this.type = new ArrayList<CodeableConcept>(); 2332 this.type.add(t); 2333 return t; 2334 } 2335 2336 public DiagnosisComponent addType(CodeableConcept t) { // 3 2337 if (t == null) 2338 return this; 2339 if (this.type == null) 2340 this.type = new ArrayList<CodeableConcept>(); 2341 this.type.add(t); 2342 return this; 2343 } 2344 2345 /** 2346 * @return The first repetition of repeating field {@link #type}, creating it if 2347 * it does not already exist 2348 */ 2349 public CodeableConcept getTypeFirstRep() { 2350 if (getType().isEmpty()) { 2351 addType(); 2352 } 2353 return getType().get(0); 2354 } 2355 2356 /** 2357 * @return {@link #onAdmission} (Indication of whether the diagnosis was present 2358 * on admission to a facility.) 2359 */ 2360 public CodeableConcept getOnAdmission() { 2361 if (this.onAdmission == null) 2362 if (Configuration.errorOnAutoCreate()) 2363 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 2364 else if (Configuration.doAutoCreate()) 2365 this.onAdmission = new CodeableConcept(); // cc 2366 return this.onAdmission; 2367 } 2368 2369 public boolean hasOnAdmission() { 2370 return this.onAdmission != null && !this.onAdmission.isEmpty(); 2371 } 2372 2373 /** 2374 * @param value {@link #onAdmission} (Indication of whether the diagnosis was 2375 * present on admission to a facility.) 2376 */ 2377 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 2378 this.onAdmission = value; 2379 return this; 2380 } 2381 2382 /** 2383 * @return {@link #packageCode} (A package billing code or bundle code used to 2384 * group products and services to a particular health condition (such as 2385 * heart attack) which is based on a predetermined grouping code 2386 * system.) 2387 */ 2388 public CodeableConcept getPackageCode() { 2389 if (this.packageCode == null) 2390 if (Configuration.errorOnAutoCreate()) 2391 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 2392 else if (Configuration.doAutoCreate()) 2393 this.packageCode = new CodeableConcept(); // cc 2394 return this.packageCode; 2395 } 2396 2397 public boolean hasPackageCode() { 2398 return this.packageCode != null && !this.packageCode.isEmpty(); 2399 } 2400 2401 /** 2402 * @param value {@link #packageCode} (A package billing code or bundle code used 2403 * to group products and services to a particular health condition 2404 * (such as heart attack) which is based on a predetermined 2405 * grouping code system.) 2406 */ 2407 public DiagnosisComponent setPackageCode(CodeableConcept value) { 2408 this.packageCode = value; 2409 return this; 2410 } 2411 2412 protected void listChildren(List<Property> children) { 2413 super.listChildren(children); 2414 children.add( 2415 new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 2416 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2417 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, 2418 diagnosis)); 2419 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 2420 0, java.lang.Integer.MAX_VALUE, type)); 2421 children.add(new Property("onAdmission", "CodeableConcept", 2422 "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 2423 children.add(new Property("packageCode", "CodeableConcept", 2424 "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 2425 0, 1, packageCode)); 2426 } 2427 2428 @Override 2429 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2430 switch (_hash) { 2431 case 1349547969: 2432 /* sequence */ return new Property("sequence", "positiveInt", 2433 "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 2434 case -1487009809: 2435 /* diagnosis[x] */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2436 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2437 1, diagnosis); 2438 case 1196993265: 2439 /* diagnosis */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2440 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2441 1, diagnosis); 2442 case 277781616: 2443 /* diagnosisCodeableConcept */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2444 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2445 1, diagnosis); 2446 case 2050454362: 2447 /* diagnosisReference */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2448 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2449 1, diagnosis); 2450 case 3575610: 2451 /* type */ return new Property("type", "CodeableConcept", 2452 "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2453 case -3386134: 2454 /* onAdmission */ return new Property("onAdmission", "CodeableConcept", 2455 "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 2456 case 908444499: 2457 /* packageCode */ return new Property("packageCode", "CodeableConcept", 2458 "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 2459 0, 1, packageCode); 2460 default: 2461 return super.getNamedProperty(_hash, _name, _checkValid); 2462 } 2463 2464 } 2465 2466 @Override 2467 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2468 switch (hash) { 2469 case 1349547969: 2470 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 2471 case 1196993265: 2472 /* diagnosis */ return this.diagnosis == null ? new Base[0] : new Base[] { this.diagnosis }; // Type 2473 case 3575610: 2474 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2475 case -3386134: 2476 /* onAdmission */ return this.onAdmission == null ? new Base[0] : new Base[] { this.onAdmission }; // CodeableConcept 2477 case 908444499: 2478 /* packageCode */ return this.packageCode == null ? new Base[0] : new Base[] { this.packageCode }; // CodeableConcept 2479 default: 2480 return super.getProperty(hash, name, checkValid); 2481 } 2482 2483 } 2484 2485 @Override 2486 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2487 switch (hash) { 2488 case 1349547969: // sequence 2489 this.sequence = castToPositiveInt(value); // PositiveIntType 2490 return value; 2491 case 1196993265: // diagnosis 2492 this.diagnosis = castToType(value); // Type 2493 return value; 2494 case 3575610: // type 2495 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2496 return value; 2497 case -3386134: // onAdmission 2498 this.onAdmission = castToCodeableConcept(value); // CodeableConcept 2499 return value; 2500 case 908444499: // packageCode 2501 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2502 return value; 2503 default: 2504 return super.setProperty(hash, name, value); 2505 } 2506 2507 } 2508 2509 @Override 2510 public Base setProperty(String name, Base value) throws FHIRException { 2511 if (name.equals("sequence")) { 2512 this.sequence = castToPositiveInt(value); // PositiveIntType 2513 } else if (name.equals("diagnosis[x]")) { 2514 this.diagnosis = castToType(value); // Type 2515 } else if (name.equals("type")) { 2516 this.getType().add(castToCodeableConcept(value)); 2517 } else if (name.equals("onAdmission")) { 2518 this.onAdmission = castToCodeableConcept(value); // CodeableConcept 2519 } else if (name.equals("packageCode")) { 2520 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2521 } else 2522 return super.setProperty(name, value); 2523 return value; 2524 } 2525 2526 @Override 2527 public void removeChild(String name, Base value) throws FHIRException { 2528 if (name.equals("sequence")) { 2529 this.sequence = null; 2530 } else if (name.equals("diagnosis[x]")) { 2531 this.diagnosis = null; 2532 } else if (name.equals("type")) { 2533 this.getType().remove(castToCodeableConcept(value)); 2534 } else if (name.equals("onAdmission")) { 2535 this.onAdmission = null; 2536 } else if (name.equals("packageCode")) { 2537 this.packageCode = null; 2538 } else 2539 super.removeChild(name, value); 2540 2541 } 2542 2543 @Override 2544 public Base makeProperty(int hash, String name) throws FHIRException { 2545 switch (hash) { 2546 case 1349547969: 2547 return getSequenceElement(); 2548 case -1487009809: 2549 return getDiagnosis(); 2550 case 1196993265: 2551 return getDiagnosis(); 2552 case 3575610: 2553 return addType(); 2554 case -3386134: 2555 return getOnAdmission(); 2556 case 908444499: 2557 return getPackageCode(); 2558 default: 2559 return super.makeProperty(hash, name); 2560 } 2561 2562 } 2563 2564 @Override 2565 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2566 switch (hash) { 2567 case 1349547969: 2568 /* sequence */ return new String[] { "positiveInt" }; 2569 case 1196993265: 2570 /* diagnosis */ return new String[] { "CodeableConcept", "Reference" }; 2571 case 3575610: 2572 /* type */ return new String[] { "CodeableConcept" }; 2573 case -3386134: 2574 /* onAdmission */ return new String[] { "CodeableConcept" }; 2575 case 908444499: 2576 /* packageCode */ return new String[] { "CodeableConcept" }; 2577 default: 2578 return super.getTypesForProperty(hash, name); 2579 } 2580 2581 } 2582 2583 @Override 2584 public Base addChild(String name) throws FHIRException { 2585 if (name.equals("sequence")) { 2586 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2587 } else if (name.equals("diagnosisCodeableConcept")) { 2588 this.diagnosis = new CodeableConcept(); 2589 return this.diagnosis; 2590 } else if (name.equals("diagnosisReference")) { 2591 this.diagnosis = new Reference(); 2592 return this.diagnosis; 2593 } else if (name.equals("type")) { 2594 return addType(); 2595 } else if (name.equals("onAdmission")) { 2596 this.onAdmission = new CodeableConcept(); 2597 return this.onAdmission; 2598 } else if (name.equals("packageCode")) { 2599 this.packageCode = new CodeableConcept(); 2600 return this.packageCode; 2601 } else 2602 return super.addChild(name); 2603 } 2604 2605 public DiagnosisComponent copy() { 2606 DiagnosisComponent dst = new DiagnosisComponent(); 2607 copyValues(dst); 2608 return dst; 2609 } 2610 2611 public void copyValues(DiagnosisComponent dst) { 2612 super.copyValues(dst); 2613 dst.sequence = sequence == null ? null : sequence.copy(); 2614 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2615 if (type != null) { 2616 dst.type = new ArrayList<CodeableConcept>(); 2617 for (CodeableConcept i : type) 2618 dst.type.add(i.copy()); 2619 } 2620 ; 2621 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2622 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2623 } 2624 2625 @Override 2626 public boolean equalsDeep(Base other_) { 2627 if (!super.equalsDeep(other_)) 2628 return false; 2629 if (!(other_ instanceof DiagnosisComponent)) 2630 return false; 2631 DiagnosisComponent o = (DiagnosisComponent) other_; 2632 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) 2633 && compareDeep(type, o.type, true) && compareDeep(onAdmission, o.onAdmission, true) 2634 && compareDeep(packageCode, o.packageCode, true); 2635 } 2636 2637 @Override 2638 public boolean equalsShallow(Base other_) { 2639 if (!super.equalsShallow(other_)) 2640 return false; 2641 if (!(other_ instanceof DiagnosisComponent)) 2642 return false; 2643 DiagnosisComponent o = (DiagnosisComponent) other_; 2644 return compareValues(sequence, o.sequence, true); 2645 } 2646 2647 public boolean isEmpty() { 2648 return super.isEmpty() 2649 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type, onAdmission, packageCode); 2650 } 2651 2652 public String fhirType() { 2653 return "Claim.diagnosis"; 2654 2655 } 2656 2657 } 2658 2659 @Block() 2660 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2661 /** 2662 * A number to uniquely identify procedure entries. 2663 */ 2664 @Child(name = "sequence", type = { 2665 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2666 @Description(shortDefinition = "Procedure instance identifier", formalDefinition = "A number to uniquely identify procedure entries.") 2667 protected PositiveIntType sequence; 2668 2669 /** 2670 * When the condition was observed or the relative ranking. 2671 */ 2672 @Child(name = "type", type = { 2673 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2674 @Description(shortDefinition = "Category of Procedure", formalDefinition = "When the condition was observed or the relative ranking.") 2675 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-procedure-type") 2676 protected List<CodeableConcept> type; 2677 2678 /** 2679 * Date and optionally time the procedure was performed. 2680 */ 2681 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2682 @Description(shortDefinition = "When the procedure was performed", formalDefinition = "Date and optionally time the procedure was performed.") 2683 protected DateTimeType date; 2684 2685 /** 2686 * The code or reference to a Procedure resource which identifies the clinical 2687 * intervention performed. 2688 */ 2689 @Child(name = "procedure", type = { CodeableConcept.class, 2690 Procedure.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 2691 @Description(shortDefinition = "Specific clinical procedure", formalDefinition = "The code or reference to a Procedure resource which identifies the clinical intervention performed.") 2692 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10-procedures") 2693 protected Type procedure; 2694 2695 /** 2696 * Unique Device Identifiers associated with this line item. 2697 */ 2698 @Child(name = "udi", type = { 2699 Device.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2700 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 2701 protected List<Reference> udi; 2702 /** 2703 * The actual objects that are the target of the reference (Unique Device 2704 * Identifiers associated with this line item.) 2705 */ 2706 protected List<Device> udiTarget; 2707 2708 private static final long serialVersionUID = 935341852L; 2709 2710 /** 2711 * Constructor 2712 */ 2713 public ProcedureComponent() { 2714 super(); 2715 } 2716 2717 /** 2718 * Constructor 2719 */ 2720 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2721 super(); 2722 this.sequence = sequence; 2723 this.procedure = procedure; 2724 } 2725 2726 /** 2727 * @return {@link #sequence} (A number to uniquely identify procedure entries.). 2728 * This is the underlying object with id, value and extensions. The 2729 * accessor "getSequence" gives direct access to the value 2730 */ 2731 public PositiveIntType getSequenceElement() { 2732 if (this.sequence == null) 2733 if (Configuration.errorOnAutoCreate()) 2734 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2735 else if (Configuration.doAutoCreate()) 2736 this.sequence = new PositiveIntType(); // bb 2737 return this.sequence; 2738 } 2739 2740 public boolean hasSequenceElement() { 2741 return this.sequence != null && !this.sequence.isEmpty(); 2742 } 2743 2744 public boolean hasSequence() { 2745 return this.sequence != null && !this.sequence.isEmpty(); 2746 } 2747 2748 /** 2749 * @param value {@link #sequence} (A number to uniquely identify procedure 2750 * entries.). This is the underlying object with id, value and 2751 * extensions. The accessor "getSequence" gives direct access to 2752 * the value 2753 */ 2754 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2755 this.sequence = value; 2756 return this; 2757 } 2758 2759 /** 2760 * @return A number to uniquely identify procedure entries. 2761 */ 2762 public int getSequence() { 2763 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2764 } 2765 2766 /** 2767 * @param value A number to uniquely identify procedure entries. 2768 */ 2769 public ProcedureComponent setSequence(int value) { 2770 if (this.sequence == null) 2771 this.sequence = new PositiveIntType(); 2772 this.sequence.setValue(value); 2773 return this; 2774 } 2775 2776 /** 2777 * @return {@link #type} (When the condition was observed or the relative 2778 * ranking.) 2779 */ 2780 public List<CodeableConcept> getType() { 2781 if (this.type == null) 2782 this.type = new ArrayList<CodeableConcept>(); 2783 return this.type; 2784 } 2785 2786 /** 2787 * @return Returns a reference to <code>this</code> for easy method chaining 2788 */ 2789 public ProcedureComponent setType(List<CodeableConcept> theType) { 2790 this.type = theType; 2791 return this; 2792 } 2793 2794 public boolean hasType() { 2795 if (this.type == null) 2796 return false; 2797 for (CodeableConcept item : this.type) 2798 if (!item.isEmpty()) 2799 return true; 2800 return false; 2801 } 2802 2803 public CodeableConcept addType() { // 3 2804 CodeableConcept t = new CodeableConcept(); 2805 if (this.type == null) 2806 this.type = new ArrayList<CodeableConcept>(); 2807 this.type.add(t); 2808 return t; 2809 } 2810 2811 public ProcedureComponent addType(CodeableConcept t) { // 3 2812 if (t == null) 2813 return this; 2814 if (this.type == null) 2815 this.type = new ArrayList<CodeableConcept>(); 2816 this.type.add(t); 2817 return this; 2818 } 2819 2820 /** 2821 * @return The first repetition of repeating field {@link #type}, creating it if 2822 * it does not already exist 2823 */ 2824 public CodeableConcept getTypeFirstRep() { 2825 if (getType().isEmpty()) { 2826 addType(); 2827 } 2828 return getType().get(0); 2829 } 2830 2831 /** 2832 * @return {@link #date} (Date and optionally time the procedure was 2833 * performed.). This is the underlying object with id, value and 2834 * extensions. The accessor "getDate" gives direct access to the value 2835 */ 2836 public DateTimeType getDateElement() { 2837 if (this.date == null) 2838 if (Configuration.errorOnAutoCreate()) 2839 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2840 else if (Configuration.doAutoCreate()) 2841 this.date = new DateTimeType(); // bb 2842 return this.date; 2843 } 2844 2845 public boolean hasDateElement() { 2846 return this.date != null && !this.date.isEmpty(); 2847 } 2848 2849 public boolean hasDate() { 2850 return this.date != null && !this.date.isEmpty(); 2851 } 2852 2853 /** 2854 * @param value {@link #date} (Date and optionally time the procedure was 2855 * performed.). This is the underlying object with id, value and 2856 * extensions. The accessor "getDate" gives direct access to the 2857 * value 2858 */ 2859 public ProcedureComponent setDateElement(DateTimeType value) { 2860 this.date = value; 2861 return this; 2862 } 2863 2864 /** 2865 * @return Date and optionally time the procedure was performed. 2866 */ 2867 public Date getDate() { 2868 return this.date == null ? null : this.date.getValue(); 2869 } 2870 2871 /** 2872 * @param value Date and optionally time the procedure was performed. 2873 */ 2874 public ProcedureComponent setDate(Date value) { 2875 if (value == null) 2876 this.date = null; 2877 else { 2878 if (this.date == null) 2879 this.date = new DateTimeType(); 2880 this.date.setValue(value); 2881 } 2882 return this; 2883 } 2884 2885 /** 2886 * @return {@link #procedure} (The code or reference to a Procedure resource 2887 * which identifies the clinical intervention performed.) 2888 */ 2889 public Type getProcedure() { 2890 return this.procedure; 2891 } 2892 2893 /** 2894 * @return {@link #procedure} (The code or reference to a Procedure resource 2895 * which identifies the clinical intervention performed.) 2896 */ 2897 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2898 if (this.procedure == null) 2899 this.procedure = new CodeableConcept(); 2900 if (!(this.procedure instanceof CodeableConcept)) 2901 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2902 + this.procedure.getClass().getName() + " was encountered"); 2903 return (CodeableConcept) this.procedure; 2904 } 2905 2906 public boolean hasProcedureCodeableConcept() { 2907 return this != null && this.procedure instanceof CodeableConcept; 2908 } 2909 2910 /** 2911 * @return {@link #procedure} (The code or reference to a Procedure resource 2912 * which identifies the clinical intervention performed.) 2913 */ 2914 public Reference getProcedureReference() throws FHIRException { 2915 if (this.procedure == null) 2916 this.procedure = new Reference(); 2917 if (!(this.procedure instanceof Reference)) 2918 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2919 + this.procedure.getClass().getName() + " was encountered"); 2920 return (Reference) this.procedure; 2921 } 2922 2923 public boolean hasProcedureReference() { 2924 return this != null && this.procedure instanceof Reference; 2925 } 2926 2927 public boolean hasProcedure() { 2928 return this.procedure != null && !this.procedure.isEmpty(); 2929 } 2930 2931 /** 2932 * @param value {@link #procedure} (The code or reference to a Procedure 2933 * resource which identifies the clinical intervention performed.) 2934 */ 2935 public ProcedureComponent setProcedure(Type value) { 2936 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2937 throw new Error("Not the right type for Claim.procedure.procedure[x]: " + value.fhirType()); 2938 this.procedure = value; 2939 return this; 2940 } 2941 2942 /** 2943 * @return {@link #udi} (Unique Device Identifiers associated with this line 2944 * item.) 2945 */ 2946 public List<Reference> getUdi() { 2947 if (this.udi == null) 2948 this.udi = new ArrayList<Reference>(); 2949 return this.udi; 2950 } 2951 2952 /** 2953 * @return Returns a reference to <code>this</code> for easy method chaining 2954 */ 2955 public ProcedureComponent setUdi(List<Reference> theUdi) { 2956 this.udi = theUdi; 2957 return this; 2958 } 2959 2960 public boolean hasUdi() { 2961 if (this.udi == null) 2962 return false; 2963 for (Reference item : this.udi) 2964 if (!item.isEmpty()) 2965 return true; 2966 return false; 2967 } 2968 2969 public Reference addUdi() { // 3 2970 Reference t = new Reference(); 2971 if (this.udi == null) 2972 this.udi = new ArrayList<Reference>(); 2973 this.udi.add(t); 2974 return t; 2975 } 2976 2977 public ProcedureComponent addUdi(Reference t) { // 3 2978 if (t == null) 2979 return this; 2980 if (this.udi == null) 2981 this.udi = new ArrayList<Reference>(); 2982 this.udi.add(t); 2983 return this; 2984 } 2985 2986 /** 2987 * @return The first repetition of repeating field {@link #udi}, creating it if 2988 * it does not already exist 2989 */ 2990 public Reference getUdiFirstRep() { 2991 if (getUdi().isEmpty()) { 2992 addUdi(); 2993 } 2994 return getUdi().get(0); 2995 } 2996 2997 /** 2998 * @deprecated Use Reference#setResource(IBaseResource) instead 2999 */ 3000 @Deprecated 3001 public List<Device> getUdiTarget() { 3002 if (this.udiTarget == null) 3003 this.udiTarget = new ArrayList<Device>(); 3004 return this.udiTarget; 3005 } 3006 3007 /** 3008 * @deprecated Use Reference#setResource(IBaseResource) instead 3009 */ 3010 @Deprecated 3011 public Device addUdiTarget() { 3012 Device r = new Device(); 3013 if (this.udiTarget == null) 3014 this.udiTarget = new ArrayList<Device>(); 3015 this.udiTarget.add(r); 3016 return r; 3017 } 3018 3019 protected void listChildren(List<Property> children) { 3020 super.listChildren(children); 3021 children.add( 3022 new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 3023 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 3024 0, java.lang.Integer.MAX_VALUE, type)); 3025 children 3026 .add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 3027 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3028 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3029 procedure)); 3030 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 3031 0, java.lang.Integer.MAX_VALUE, udi)); 3032 } 3033 3034 @Override 3035 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3036 switch (_hash) { 3037 case 1349547969: 3038 /* sequence */ return new Property("sequence", "positiveInt", 3039 "A number to uniquely identify procedure entries.", 0, 1, sequence); 3040 case 3575610: 3041 /* type */ return new Property("type", "CodeableConcept", 3042 "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 3043 case 3076014: 3044 /* date */ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 3045 1, date); 3046 case 1640074445: 3047 /* procedure[x] */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3048 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3049 procedure); 3050 case -1095204141: 3051 /* procedure */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3052 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3053 procedure); 3054 case -1284783026: 3055 /* procedureCodeableConcept */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3056 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3057 procedure); 3058 case 881809848: 3059 /* procedureReference */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3060 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3061 procedure); 3062 case 115642: 3063 /* udi */ return new Property("udi", "Reference(Device)", 3064 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 3065 default: 3066 return super.getNamedProperty(_hash, _name, _checkValid); 3067 } 3068 3069 } 3070 3071 @Override 3072 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3073 switch (hash) { 3074 case 1349547969: 3075 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 3076 case 3575610: 3077 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3078 case 3076014: 3079 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3080 case -1095204141: 3081 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // Type 3082 case 115642: 3083 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 3084 default: 3085 return super.getProperty(hash, name, checkValid); 3086 } 3087 3088 } 3089 3090 @Override 3091 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3092 switch (hash) { 3093 case 1349547969: // sequence 3094 this.sequence = castToPositiveInt(value); // PositiveIntType 3095 return value; 3096 case 3575610: // type 3097 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3098 return value; 3099 case 3076014: // date 3100 this.date = castToDateTime(value); // DateTimeType 3101 return value; 3102 case -1095204141: // procedure 3103 this.procedure = castToType(value); // Type 3104 return value; 3105 case 115642: // udi 3106 this.getUdi().add(castToReference(value)); // Reference 3107 return value; 3108 default: 3109 return super.setProperty(hash, name, value); 3110 } 3111 3112 } 3113 3114 @Override 3115 public Base setProperty(String name, Base value) throws FHIRException { 3116 if (name.equals("sequence")) { 3117 this.sequence = castToPositiveInt(value); // PositiveIntType 3118 } else if (name.equals("type")) { 3119 this.getType().add(castToCodeableConcept(value)); 3120 } else if (name.equals("date")) { 3121 this.date = castToDateTime(value); // DateTimeType 3122 } else if (name.equals("procedure[x]")) { 3123 this.procedure = castToType(value); // Type 3124 } else if (name.equals("udi")) { 3125 this.getUdi().add(castToReference(value)); 3126 } else 3127 return super.setProperty(name, value); 3128 return value; 3129 } 3130 3131 @Override 3132 public void removeChild(String name, Base value) throws FHIRException { 3133 if (name.equals("sequence")) { 3134 this.sequence = null; 3135 } else if (name.equals("type")) { 3136 this.getType().remove(castToCodeableConcept(value)); 3137 } else if (name.equals("date")) { 3138 this.date = null; 3139 } else if (name.equals("procedure[x]")) { 3140 this.procedure = null; 3141 } else if (name.equals("udi")) { 3142 this.getUdi().remove(castToReference(value)); 3143 } else 3144 super.removeChild(name, value); 3145 3146 } 3147 3148 @Override 3149 public Base makeProperty(int hash, String name) throws FHIRException { 3150 switch (hash) { 3151 case 1349547969: 3152 return getSequenceElement(); 3153 case 3575610: 3154 return addType(); 3155 case 3076014: 3156 return getDateElement(); 3157 case 1640074445: 3158 return getProcedure(); 3159 case -1095204141: 3160 return getProcedure(); 3161 case 115642: 3162 return addUdi(); 3163 default: 3164 return super.makeProperty(hash, name); 3165 } 3166 3167 } 3168 3169 @Override 3170 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3171 switch (hash) { 3172 case 1349547969: 3173 /* sequence */ return new String[] { "positiveInt" }; 3174 case 3575610: 3175 /* type */ return new String[] { "CodeableConcept" }; 3176 case 3076014: 3177 /* date */ return new String[] { "dateTime" }; 3178 case -1095204141: 3179 /* procedure */ return new String[] { "CodeableConcept", "Reference" }; 3180 case 115642: 3181 /* udi */ return new String[] { "Reference" }; 3182 default: 3183 return super.getTypesForProperty(hash, name); 3184 } 3185 3186 } 3187 3188 @Override 3189 public Base addChild(String name) throws FHIRException { 3190 if (name.equals("sequence")) { 3191 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 3192 } else if (name.equals("type")) { 3193 return addType(); 3194 } else if (name.equals("date")) { 3195 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 3196 } else if (name.equals("procedureCodeableConcept")) { 3197 this.procedure = new CodeableConcept(); 3198 return this.procedure; 3199 } else if (name.equals("procedureReference")) { 3200 this.procedure = new Reference(); 3201 return this.procedure; 3202 } else if (name.equals("udi")) { 3203 return addUdi(); 3204 } else 3205 return super.addChild(name); 3206 } 3207 3208 public ProcedureComponent copy() { 3209 ProcedureComponent dst = new ProcedureComponent(); 3210 copyValues(dst); 3211 return dst; 3212 } 3213 3214 public void copyValues(ProcedureComponent dst) { 3215 super.copyValues(dst); 3216 dst.sequence = sequence == null ? null : sequence.copy(); 3217 if (type != null) { 3218 dst.type = new ArrayList<CodeableConcept>(); 3219 for (CodeableConcept i : type) 3220 dst.type.add(i.copy()); 3221 } 3222 ; 3223 dst.date = date == null ? null : date.copy(); 3224 dst.procedure = procedure == null ? null : procedure.copy(); 3225 if (udi != null) { 3226 dst.udi = new ArrayList<Reference>(); 3227 for (Reference i : udi) 3228 dst.udi.add(i.copy()); 3229 } 3230 ; 3231 } 3232 3233 @Override 3234 public boolean equalsDeep(Base other_) { 3235 if (!super.equalsDeep(other_)) 3236 return false; 3237 if (!(other_ instanceof ProcedureComponent)) 3238 return false; 3239 ProcedureComponent o = (ProcedureComponent) other_; 3240 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) 3241 && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 3242 && compareDeep(udi, o.udi, true); 3243 } 3244 3245 @Override 3246 public boolean equalsShallow(Base other_) { 3247 if (!super.equalsShallow(other_)) 3248 return false; 3249 if (!(other_ instanceof ProcedureComponent)) 3250 return false; 3251 ProcedureComponent o = (ProcedureComponent) other_; 3252 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 3253 } 3254 3255 public boolean isEmpty() { 3256 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure, udi); 3257 } 3258 3259 public String fhirType() { 3260 return "Claim.procedure"; 3261 3262 } 3263 3264 } 3265 3266 @Block() 3267 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 3268 /** 3269 * A number to uniquely identify insurance entries and provide a sequence of 3270 * coverages to convey coordination of benefit order. 3271 */ 3272 @Child(name = "sequence", type = { 3273 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3274 @Description(shortDefinition = "Insurance instance identifier", formalDefinition = "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.") 3275 protected PositiveIntType sequence; 3276 3277 /** 3278 * A flag to indicate that this Coverage is to be used for adjudication of this 3279 * claim when set to true. 3280 */ 3281 @Child(name = "focal", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3282 @Description(shortDefinition = "Coverage to be used for adjudication", formalDefinition = "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.") 3283 protected BooleanType focal; 3284 3285 /** 3286 * The business identifier to be used when the claim is sent for adjudication 3287 * against this insurance policy. 3288 */ 3289 @Child(name = "identifier", type = { 3290 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3291 @Description(shortDefinition = "Pre-assigned Claim number", formalDefinition = "The business identifier to be used when the claim is sent for adjudication against this insurance policy.") 3292 protected Identifier identifier; 3293 3294 /** 3295 * Reference to the insurance card level information contained in the Coverage 3296 * resource. The coverage issuing insurer will use these details to locate the 3297 * patient's actual coverage within the insurer's information system. 3298 */ 3299 @Child(name = "coverage", type = { Coverage.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3300 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 3301 protected Reference coverage; 3302 3303 /** 3304 * The actual object that is the target of the reference (Reference to the 3305 * insurance card level information contained in the Coverage resource. The 3306 * coverage issuing insurer will use these details to locate the patient's 3307 * actual coverage within the insurer's information system.) 3308 */ 3309 protected Coverage coverageTarget; 3310 3311 /** 3312 * A business agreement number established between the provider and the insurer 3313 * for special business processing purposes. 3314 */ 3315 @Child(name = "businessArrangement", type = { 3316 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3317 @Description(shortDefinition = "Additional provider contract number", formalDefinition = "A business agreement number established between the provider and the insurer for special business processing purposes.") 3318 protected StringType businessArrangement; 3319 3320 /** 3321 * Reference numbers previously provided by the insurer to the provider to be 3322 * quoted on subsequent claims containing services or products related to the 3323 * prior authorization. 3324 */ 3325 @Child(name = "preAuthRef", type = { 3326 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3327 @Description(shortDefinition = "Prior authorization reference number", formalDefinition = "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.") 3328 protected List<StringType> preAuthRef; 3329 3330 /** 3331 * The result of the adjudication of the line items for the Coverage specified 3332 * in this insurance. 3333 */ 3334 @Child(name = "claimResponse", type = { 3335 ClaimResponse.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3336 @Description(shortDefinition = "Adjudication results", formalDefinition = "The result of the adjudication of the line items for the Coverage specified in this insurance.") 3337 protected Reference claimResponse; 3338 3339 /** 3340 * The actual object that is the target of the reference (The result of the 3341 * adjudication of the line items for the Coverage specified in this insurance.) 3342 */ 3343 protected ClaimResponse claimResponseTarget; 3344 3345 private static final long serialVersionUID = -1711744215L; 3346 3347 /** 3348 * Constructor 3349 */ 3350 public InsuranceComponent() { 3351 super(); 3352 } 3353 3354 /** 3355 * Constructor 3356 */ 3357 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 3358 super(); 3359 this.sequence = sequence; 3360 this.focal = focal; 3361 this.coverage = coverage; 3362 } 3363 3364 /** 3365 * @return {@link #sequence} (A number to uniquely identify insurance entries 3366 * and provide a sequence of coverages to convey coordination of benefit 3367 * order.). This is the underlying object with id, value and extensions. 3368 * The accessor "getSequence" gives direct access to the value 3369 */ 3370 public PositiveIntType getSequenceElement() { 3371 if (this.sequence == null) 3372 if (Configuration.errorOnAutoCreate()) 3373 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 3374 else if (Configuration.doAutoCreate()) 3375 this.sequence = new PositiveIntType(); // bb 3376 return this.sequence; 3377 } 3378 3379 public boolean hasSequenceElement() { 3380 return this.sequence != null && !this.sequence.isEmpty(); 3381 } 3382 3383 public boolean hasSequence() { 3384 return this.sequence != null && !this.sequence.isEmpty(); 3385 } 3386 3387 /** 3388 * @param value {@link #sequence} (A number to uniquely identify insurance 3389 * entries and provide a sequence of coverages to convey 3390 * coordination of benefit order.). This is the underlying object 3391 * with id, value and extensions. The accessor "getSequence" gives 3392 * direct access to the value 3393 */ 3394 public InsuranceComponent setSequenceElement(PositiveIntType value) { 3395 this.sequence = value; 3396 return this; 3397 } 3398 3399 /** 3400 * @return A number to uniquely identify insurance entries and provide a 3401 * sequence of coverages to convey coordination of benefit order. 3402 */ 3403 public int getSequence() { 3404 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3405 } 3406 3407 /** 3408 * @param value A number to uniquely identify insurance entries and provide a 3409 * sequence of coverages to convey coordination of benefit order. 3410 */ 3411 public InsuranceComponent setSequence(int value) { 3412 if (this.sequence == null) 3413 this.sequence = new PositiveIntType(); 3414 this.sequence.setValue(value); 3415 return this; 3416 } 3417 3418 /** 3419 * @return {@link #focal} (A flag to indicate that this Coverage is to be used 3420 * for adjudication of this claim when set to true.). This is the 3421 * underlying object with id, value and extensions. The accessor 3422 * "getFocal" gives direct access to the value 3423 */ 3424 public BooleanType getFocalElement() { 3425 if (this.focal == null) 3426 if (Configuration.errorOnAutoCreate()) 3427 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 3428 else if (Configuration.doAutoCreate()) 3429 this.focal = new BooleanType(); // bb 3430 return this.focal; 3431 } 3432 3433 public boolean hasFocalElement() { 3434 return this.focal != null && !this.focal.isEmpty(); 3435 } 3436 3437 public boolean hasFocal() { 3438 return this.focal != null && !this.focal.isEmpty(); 3439 } 3440 3441 /** 3442 * @param value {@link #focal} (A flag to indicate that this Coverage is to be 3443 * used for adjudication of this claim when set to true.). This is 3444 * the underlying object with id, value and extensions. The 3445 * accessor "getFocal" gives direct access to the value 3446 */ 3447 public InsuranceComponent setFocalElement(BooleanType value) { 3448 this.focal = value; 3449 return this; 3450 } 3451 3452 /** 3453 * @return A flag to indicate that this Coverage is to be used for adjudication 3454 * of this claim when set to true. 3455 */ 3456 public boolean getFocal() { 3457 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 3458 } 3459 3460 /** 3461 * @param value A flag to indicate that this Coverage is to be used for 3462 * adjudication of this claim when set to true. 3463 */ 3464 public InsuranceComponent setFocal(boolean value) { 3465 if (this.focal == null) 3466 this.focal = new BooleanType(); 3467 this.focal.setValue(value); 3468 return this; 3469 } 3470 3471 /** 3472 * @return {@link #identifier} (The business identifier to be used when the 3473 * claim is sent for adjudication against this insurance policy.) 3474 */ 3475 public Identifier getIdentifier() { 3476 if (this.identifier == null) 3477 if (Configuration.errorOnAutoCreate()) 3478 throw new Error("Attempt to auto-create InsuranceComponent.identifier"); 3479 else if (Configuration.doAutoCreate()) 3480 this.identifier = new Identifier(); // cc 3481 return this.identifier; 3482 } 3483 3484 public boolean hasIdentifier() { 3485 return this.identifier != null && !this.identifier.isEmpty(); 3486 } 3487 3488 /** 3489 * @param value {@link #identifier} (The business identifier to be used when the 3490 * claim is sent for adjudication against this insurance policy.) 3491 */ 3492 public InsuranceComponent setIdentifier(Identifier value) { 3493 this.identifier = value; 3494 return this; 3495 } 3496 3497 /** 3498 * @return {@link #coverage} (Reference to the insurance card level information 3499 * contained in the Coverage resource. The coverage issuing insurer will 3500 * use these details to locate the patient's actual coverage within the 3501 * insurer's information system.) 3502 */ 3503 public Reference getCoverage() { 3504 if (this.coverage == null) 3505 if (Configuration.errorOnAutoCreate()) 3506 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 3507 else if (Configuration.doAutoCreate()) 3508 this.coverage = new Reference(); // cc 3509 return this.coverage; 3510 } 3511 3512 public boolean hasCoverage() { 3513 return this.coverage != null && !this.coverage.isEmpty(); 3514 } 3515 3516 /** 3517 * @param value {@link #coverage} (Reference to the insurance card level 3518 * information contained in the Coverage resource. The coverage 3519 * issuing insurer will use these details to locate the patient's 3520 * actual coverage within the insurer's information system.) 3521 */ 3522 public InsuranceComponent setCoverage(Reference value) { 3523 this.coverage = value; 3524 return this; 3525 } 3526 3527 /** 3528 * @return {@link #coverage} The actual object that is the target of the 3529 * reference. The reference library doesn't populate this, but you can 3530 * use it to hold the resource if you resolve it. (Reference to the 3531 * insurance card level information contained in the Coverage resource. 3532 * The coverage issuing insurer will use these details to locate the 3533 * patient's actual coverage within the insurer's information system.) 3534 */ 3535 public Coverage getCoverageTarget() { 3536 if (this.coverageTarget == null) 3537 if (Configuration.errorOnAutoCreate()) 3538 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 3539 else if (Configuration.doAutoCreate()) 3540 this.coverageTarget = new Coverage(); // aa 3541 return this.coverageTarget; 3542 } 3543 3544 /** 3545 * @param value {@link #coverage} The actual object that is the target of the 3546 * reference. The reference library doesn't use these, but you can 3547 * use it to hold the resource if you resolve it. (Reference to the 3548 * insurance card level information contained in the Coverage 3549 * resource. The coverage issuing insurer will use these details to 3550 * locate the patient's actual coverage within the insurer's 3551 * information system.) 3552 */ 3553 public InsuranceComponent setCoverageTarget(Coverage value) { 3554 this.coverageTarget = value; 3555 return this; 3556 } 3557 3558 /** 3559 * @return {@link #businessArrangement} (A business agreement number established 3560 * between the provider and the insurer for special business processing 3561 * purposes.). This is the underlying object with id, value and 3562 * extensions. The accessor "getBusinessArrangement" gives direct access 3563 * to the value 3564 */ 3565 public StringType getBusinessArrangementElement() { 3566 if (this.businessArrangement == null) 3567 if (Configuration.errorOnAutoCreate()) 3568 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 3569 else if (Configuration.doAutoCreate()) 3570 this.businessArrangement = new StringType(); // bb 3571 return this.businessArrangement; 3572 } 3573 3574 public boolean hasBusinessArrangementElement() { 3575 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 3576 } 3577 3578 public boolean hasBusinessArrangement() { 3579 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 3580 } 3581 3582 /** 3583 * @param value {@link #businessArrangement} (A business agreement number 3584 * established between the provider and the insurer for special 3585 * business processing purposes.). This is the underlying object 3586 * with id, value and extensions. The accessor 3587 * "getBusinessArrangement" gives direct access to the value 3588 */ 3589 public InsuranceComponent setBusinessArrangementElement(StringType value) { 3590 this.businessArrangement = value; 3591 return this; 3592 } 3593 3594 /** 3595 * @return A business agreement number established between the provider and the 3596 * insurer for special business processing purposes. 3597 */ 3598 public String getBusinessArrangement() { 3599 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 3600 } 3601 3602 /** 3603 * @param value A business agreement number established between the provider and 3604 * the insurer for special business processing purposes. 3605 */ 3606 public InsuranceComponent setBusinessArrangement(String value) { 3607 if (Utilities.noString(value)) 3608 this.businessArrangement = null; 3609 else { 3610 if (this.businessArrangement == null) 3611 this.businessArrangement = new StringType(); 3612 this.businessArrangement.setValue(value); 3613 } 3614 return this; 3615 } 3616 3617 /** 3618 * @return {@link #preAuthRef} (Reference numbers previously provided by the 3619 * insurer to the provider to be quoted on subsequent claims containing 3620 * services or products related to the prior authorization.) 3621 */ 3622 public List<StringType> getPreAuthRef() { 3623 if (this.preAuthRef == null) 3624 this.preAuthRef = new ArrayList<StringType>(); 3625 return this.preAuthRef; 3626 } 3627 3628 /** 3629 * @return Returns a reference to <code>this</code> for easy method chaining 3630 */ 3631 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 3632 this.preAuthRef = thePreAuthRef; 3633 return this; 3634 } 3635 3636 public boolean hasPreAuthRef() { 3637 if (this.preAuthRef == null) 3638 return false; 3639 for (StringType item : this.preAuthRef) 3640 if (!item.isEmpty()) 3641 return true; 3642 return false; 3643 } 3644 3645 /** 3646 * @return {@link #preAuthRef} (Reference numbers previously provided by the 3647 * insurer to the provider to be quoted on subsequent claims containing 3648 * services or products related to the prior authorization.) 3649 */ 3650 public StringType addPreAuthRefElement() {// 2 3651 StringType t = new StringType(); 3652 if (this.preAuthRef == null) 3653 this.preAuthRef = new ArrayList<StringType>(); 3654 this.preAuthRef.add(t); 3655 return t; 3656 } 3657 3658 /** 3659 * @param value {@link #preAuthRef} (Reference numbers previously provided by 3660 * the insurer to the provider to be quoted on subsequent claims 3661 * containing services or products related to the prior 3662 * authorization.) 3663 */ 3664 public InsuranceComponent addPreAuthRef(String value) { // 1 3665 StringType t = new StringType(); 3666 t.setValue(value); 3667 if (this.preAuthRef == null) 3668 this.preAuthRef = new ArrayList<StringType>(); 3669 this.preAuthRef.add(t); 3670 return this; 3671 } 3672 3673 /** 3674 * @param value {@link #preAuthRef} (Reference numbers previously provided by 3675 * the insurer to the provider to be quoted on subsequent claims 3676 * containing services or products related to the prior 3677 * authorization.) 3678 */ 3679 public boolean hasPreAuthRef(String value) { 3680 if (this.preAuthRef == null) 3681 return false; 3682 for (StringType v : this.preAuthRef) 3683 if (v.getValue().equals(value)) // string 3684 return true; 3685 return false; 3686 } 3687 3688 /** 3689 * @return {@link #claimResponse} (The result of the adjudication of the line 3690 * items for the Coverage specified in this insurance.) 3691 */ 3692 public Reference getClaimResponse() { 3693 if (this.claimResponse == null) 3694 if (Configuration.errorOnAutoCreate()) 3695 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 3696 else if (Configuration.doAutoCreate()) 3697 this.claimResponse = new Reference(); // cc 3698 return this.claimResponse; 3699 } 3700 3701 public boolean hasClaimResponse() { 3702 return this.claimResponse != null && !this.claimResponse.isEmpty(); 3703 } 3704 3705 /** 3706 * @param value {@link #claimResponse} (The result of the adjudication of the 3707 * line items for the Coverage specified in this insurance.) 3708 */ 3709 public InsuranceComponent setClaimResponse(Reference value) { 3710 this.claimResponse = value; 3711 return this; 3712 } 3713 3714 /** 3715 * @return {@link #claimResponse} The actual object that is the target of the 3716 * reference. The reference library doesn't populate this, but you can 3717 * use it to hold the resource if you resolve it. (The result of the 3718 * adjudication of the line items for the Coverage specified in this 3719 * insurance.) 3720 */ 3721 public ClaimResponse getClaimResponseTarget() { 3722 if (this.claimResponseTarget == null) 3723 if (Configuration.errorOnAutoCreate()) 3724 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 3725 else if (Configuration.doAutoCreate()) 3726 this.claimResponseTarget = new ClaimResponse(); // aa 3727 return this.claimResponseTarget; 3728 } 3729 3730 /** 3731 * @param value {@link #claimResponse} The actual object that is the target of 3732 * the reference. The reference library doesn't use these, but you 3733 * can use it to hold the resource if you resolve it. (The result 3734 * of the adjudication of the line items for the Coverage specified 3735 * in this insurance.) 3736 */ 3737 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 3738 this.claimResponseTarget = value; 3739 return this; 3740 } 3741 3742 protected void listChildren(List<Property> children) { 3743 super.listChildren(children); 3744 children.add(new Property("sequence", "positiveInt", 3745 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 3746 0, 1, sequence)); 3747 children.add(new Property("focal", "boolean", 3748 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, 3749 focal)); 3750 children.add(new Property("identifier", "Identifier", 3751 "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 3752 0, 1, identifier)); 3753 children.add(new Property("coverage", "Reference(Coverage)", 3754 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 3755 0, 1, coverage)); 3756 children.add(new Property("businessArrangement", "string", 3757 "A business agreement number established between the provider and the insurer for special business processing purposes.", 3758 0, 1, businessArrangement)); 3759 children.add(new Property("preAuthRef", "string", 3760 "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 3761 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 3762 children.add(new Property("claimResponse", "Reference(ClaimResponse)", 3763 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 3764 claimResponse)); 3765 } 3766 3767 @Override 3768 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3769 switch (_hash) { 3770 case 1349547969: 3771 /* sequence */ return new Property("sequence", "positiveInt", 3772 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 3773 0, 1, sequence); 3774 case 97604197: 3775 /* focal */ return new Property("focal", "boolean", 3776 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 3777 1, focal); 3778 case -1618432855: 3779 /* identifier */ return new Property("identifier", "Identifier", 3780 "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 3781 0, 1, identifier); 3782 case -351767064: 3783 /* coverage */ return new Property("coverage", "Reference(Coverage)", 3784 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 3785 0, 1, coverage); 3786 case 259920682: 3787 /* businessArrangement */ return new Property("businessArrangement", "string", 3788 "A business agreement number established between the provider and the insurer for special business processing purposes.", 3789 0, 1, businessArrangement); 3790 case 522246568: 3791 /* preAuthRef */ return new Property("preAuthRef", "string", 3792 "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 3793 0, java.lang.Integer.MAX_VALUE, preAuthRef); 3794 case 689513629: 3795 /* claimResponse */ return new Property("claimResponse", "Reference(ClaimResponse)", 3796 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 3797 claimResponse); 3798 default: 3799 return super.getNamedProperty(_hash, _name, _checkValid); 3800 } 3801 3802 } 3803 3804 @Override 3805 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3806 switch (hash) { 3807 case 1349547969: 3808 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 3809 case 97604197: 3810 /* focal */ return this.focal == null ? new Base[0] : new Base[] { this.focal }; // BooleanType 3811 case -1618432855: 3812 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 3813 case -351767064: 3814 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 3815 case 259920682: 3816 /* businessArrangement */ return this.businessArrangement == null ? new Base[0] 3817 : new Base[] { this.businessArrangement }; // StringType 3818 case 522246568: 3819 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] 3820 : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 3821 case 689513629: 3822 /* claimResponse */ return this.claimResponse == null ? new Base[0] : new Base[] { this.claimResponse }; // Reference 3823 default: 3824 return super.getProperty(hash, name, checkValid); 3825 } 3826 3827 } 3828 3829 @Override 3830 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3831 switch (hash) { 3832 case 1349547969: // sequence 3833 this.sequence = castToPositiveInt(value); // PositiveIntType 3834 return value; 3835 case 97604197: // focal 3836 this.focal = castToBoolean(value); // BooleanType 3837 return value; 3838 case -1618432855: // identifier 3839 this.identifier = castToIdentifier(value); // Identifier 3840 return value; 3841 case -351767064: // coverage 3842 this.coverage = castToReference(value); // Reference 3843 return value; 3844 case 259920682: // businessArrangement 3845 this.businessArrangement = castToString(value); // StringType 3846 return value; 3847 case 522246568: // preAuthRef 3848 this.getPreAuthRef().add(castToString(value)); // StringType 3849 return value; 3850 case 689513629: // claimResponse 3851 this.claimResponse = castToReference(value); // Reference 3852 return value; 3853 default: 3854 return super.setProperty(hash, name, value); 3855 } 3856 3857 } 3858 3859 @Override 3860 public Base setProperty(String name, Base value) throws FHIRException { 3861 if (name.equals("sequence")) { 3862 this.sequence = castToPositiveInt(value); // PositiveIntType 3863 } else if (name.equals("focal")) { 3864 this.focal = castToBoolean(value); // BooleanType 3865 } else if (name.equals("identifier")) { 3866 this.identifier = castToIdentifier(value); // Identifier 3867 } else if (name.equals("coverage")) { 3868 this.coverage = castToReference(value); // Reference 3869 } else if (name.equals("businessArrangement")) { 3870 this.businessArrangement = castToString(value); // StringType 3871 } else if (name.equals("preAuthRef")) { 3872 this.getPreAuthRef().add(castToString(value)); 3873 } else if (name.equals("claimResponse")) { 3874 this.claimResponse = castToReference(value); // Reference 3875 } else 3876 return super.setProperty(name, value); 3877 return value; 3878 } 3879 3880 @Override 3881 public void removeChild(String name, Base value) throws FHIRException { 3882 if (name.equals("sequence")) { 3883 this.sequence = null; 3884 } else if (name.equals("focal")) { 3885 this.focal = null; 3886 } else if (name.equals("identifier")) { 3887 this.identifier = null; 3888 } else if (name.equals("coverage")) { 3889 this.coverage = null; 3890 } else if (name.equals("businessArrangement")) { 3891 this.businessArrangement = null; 3892 } else if (name.equals("preAuthRef")) { 3893 this.getPreAuthRef().remove(castToString(value)); 3894 } else if (name.equals("claimResponse")) { 3895 this.claimResponse = null; 3896 } else 3897 super.removeChild(name, value); 3898 3899 } 3900 3901 @Override 3902 public Base makeProperty(int hash, String name) throws FHIRException { 3903 switch (hash) { 3904 case 1349547969: 3905 return getSequenceElement(); 3906 case 97604197: 3907 return getFocalElement(); 3908 case -1618432855: 3909 return getIdentifier(); 3910 case -351767064: 3911 return getCoverage(); 3912 case 259920682: 3913 return getBusinessArrangementElement(); 3914 case 522246568: 3915 return addPreAuthRefElement(); 3916 case 689513629: 3917 return getClaimResponse(); 3918 default: 3919 return super.makeProperty(hash, name); 3920 } 3921 3922 } 3923 3924 @Override 3925 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3926 switch (hash) { 3927 case 1349547969: 3928 /* sequence */ return new String[] { "positiveInt" }; 3929 case 97604197: 3930 /* focal */ return new String[] { "boolean" }; 3931 case -1618432855: 3932 /* identifier */ return new String[] { "Identifier" }; 3933 case -351767064: 3934 /* coverage */ return new String[] { "Reference" }; 3935 case 259920682: 3936 /* businessArrangement */ return new String[] { "string" }; 3937 case 522246568: 3938 /* preAuthRef */ return new String[] { "string" }; 3939 case 689513629: 3940 /* claimResponse */ return new String[] { "Reference" }; 3941 default: 3942 return super.getTypesForProperty(hash, name); 3943 } 3944 3945 } 3946 3947 @Override 3948 public Base addChild(String name) throws FHIRException { 3949 if (name.equals("sequence")) { 3950 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 3951 } else if (name.equals("focal")) { 3952 throw new FHIRException("Cannot call addChild on a singleton property Claim.focal"); 3953 } else if (name.equals("identifier")) { 3954 this.identifier = new Identifier(); 3955 return this.identifier; 3956 } else if (name.equals("coverage")) { 3957 this.coverage = new Reference(); 3958 return this.coverage; 3959 } else if (name.equals("businessArrangement")) { 3960 throw new FHIRException("Cannot call addChild on a singleton property Claim.businessArrangement"); 3961 } else if (name.equals("preAuthRef")) { 3962 throw new FHIRException("Cannot call addChild on a singleton property Claim.preAuthRef"); 3963 } else if (name.equals("claimResponse")) { 3964 this.claimResponse = new Reference(); 3965 return this.claimResponse; 3966 } else 3967 return super.addChild(name); 3968 } 3969 3970 public InsuranceComponent copy() { 3971 InsuranceComponent dst = new InsuranceComponent(); 3972 copyValues(dst); 3973 return dst; 3974 } 3975 3976 public void copyValues(InsuranceComponent dst) { 3977 super.copyValues(dst); 3978 dst.sequence = sequence == null ? null : sequence.copy(); 3979 dst.focal = focal == null ? null : focal.copy(); 3980 dst.identifier = identifier == null ? null : identifier.copy(); 3981 dst.coverage = coverage == null ? null : coverage.copy(); 3982 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3983 if (preAuthRef != null) { 3984 dst.preAuthRef = new ArrayList<StringType>(); 3985 for (StringType i : preAuthRef) 3986 dst.preAuthRef.add(i.copy()); 3987 } 3988 ; 3989 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3990 } 3991 3992 @Override 3993 public boolean equalsDeep(Base other_) { 3994 if (!super.equalsDeep(other_)) 3995 return false; 3996 if (!(other_ instanceof InsuranceComponent)) 3997 return false; 3998 InsuranceComponent o = (InsuranceComponent) other_; 3999 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) 4000 && compareDeep(identifier, o.identifier, true) && compareDeep(coverage, o.coverage, true) 4001 && compareDeep(businessArrangement, o.businessArrangement, true) 4002 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(claimResponse, o.claimResponse, true); 4003 } 4004 4005 @Override 4006 public boolean equalsShallow(Base other_) { 4007 if (!super.equalsShallow(other_)) 4008 return false; 4009 if (!(other_ instanceof InsuranceComponent)) 4010 return false; 4011 InsuranceComponent o = (InsuranceComponent) other_; 4012 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) 4013 && compareValues(businessArrangement, o.businessArrangement, true) 4014 && compareValues(preAuthRef, o.preAuthRef, true); 4015 } 4016 4017 public boolean isEmpty() { 4018 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, identifier, coverage, 4019 businessArrangement, preAuthRef, claimResponse); 4020 } 4021 4022 public String fhirType() { 4023 return "Claim.insurance"; 4024 4025 } 4026 4027 } 4028 4029 @Block() 4030 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 4031 /** 4032 * Date of an accident event related to the products and services contained in 4033 * the claim. 4034 */ 4035 @Child(name = "date", type = { DateType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4036 @Description(shortDefinition = "When the incident occurred", formalDefinition = "Date of an accident event related to the products and services contained in the claim.") 4037 protected DateType date; 4038 4039 /** 4040 * The type or context of the accident event for the purposes of selection of 4041 * potential insurance coverages and determination of coordination between 4042 * insurers. 4043 */ 4044 @Child(name = "type", type = { 4045 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4046 @Description(shortDefinition = "The nature of the accident", formalDefinition = "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.") 4047 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 4048 protected CodeableConcept type; 4049 4050 /** 4051 * The physical location of the accident event. 4052 */ 4053 @Child(name = "location", type = { Address.class, 4054 Location.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4055 @Description(shortDefinition = "Where the event occurred", formalDefinition = "The physical location of the accident event.") 4056 protected Type location; 4057 4058 private static final long serialVersionUID = 622904984L; 4059 4060 /** 4061 * Constructor 4062 */ 4063 public AccidentComponent() { 4064 super(); 4065 } 4066 4067 /** 4068 * Constructor 4069 */ 4070 public AccidentComponent(DateType date) { 4071 super(); 4072 this.date = date; 4073 } 4074 4075 /** 4076 * @return {@link #date} (Date of an accident event related to the products and 4077 * services contained in the claim.). This is the underlying object with 4078 * id, value and extensions. The accessor "getDate" gives direct access 4079 * to the value 4080 */ 4081 public DateType getDateElement() { 4082 if (this.date == null) 4083 if (Configuration.errorOnAutoCreate()) 4084 throw new Error("Attempt to auto-create AccidentComponent.date"); 4085 else if (Configuration.doAutoCreate()) 4086 this.date = new DateType(); // bb 4087 return this.date; 4088 } 4089 4090 public boolean hasDateElement() { 4091 return this.date != null && !this.date.isEmpty(); 4092 } 4093 4094 public boolean hasDate() { 4095 return this.date != null && !this.date.isEmpty(); 4096 } 4097 4098 /** 4099 * @param value {@link #date} (Date of an accident event related to the products 4100 * and services contained in the claim.). This is the underlying 4101 * object with id, value and extensions. The accessor "getDate" 4102 * gives direct access to the value 4103 */ 4104 public AccidentComponent setDateElement(DateType value) { 4105 this.date = value; 4106 return this; 4107 } 4108 4109 /** 4110 * @return Date of an accident event related to the products and services 4111 * contained in the claim. 4112 */ 4113 public Date getDate() { 4114 return this.date == null ? null : this.date.getValue(); 4115 } 4116 4117 /** 4118 * @param value Date of an accident event related to the products and services 4119 * contained in the claim. 4120 */ 4121 public AccidentComponent setDate(Date value) { 4122 if (this.date == null) 4123 this.date = new DateType(); 4124 this.date.setValue(value); 4125 return this; 4126 } 4127 4128 /** 4129 * @return {@link #type} (The type or context of the accident event for the 4130 * purposes of selection of potential insurance coverages and 4131 * determination of coordination between insurers.) 4132 */ 4133 public CodeableConcept getType() { 4134 if (this.type == null) 4135 if (Configuration.errorOnAutoCreate()) 4136 throw new Error("Attempt to auto-create AccidentComponent.type"); 4137 else if (Configuration.doAutoCreate()) 4138 this.type = new CodeableConcept(); // cc 4139 return this.type; 4140 } 4141 4142 public boolean hasType() { 4143 return this.type != null && !this.type.isEmpty(); 4144 } 4145 4146 /** 4147 * @param value {@link #type} (The type or context of the accident event for the 4148 * purposes of selection of potential insurance coverages and 4149 * determination of coordination between insurers.) 4150 */ 4151 public AccidentComponent setType(CodeableConcept value) { 4152 this.type = value; 4153 return this; 4154 } 4155 4156 /** 4157 * @return {@link #location} (The physical location of the accident event.) 4158 */ 4159 public Type getLocation() { 4160 return this.location; 4161 } 4162 4163 /** 4164 * @return {@link #location} (The physical location of the accident event.) 4165 */ 4166 public Address getLocationAddress() throws FHIRException { 4167 if (this.location == null) 4168 this.location = new Address(); 4169 if (!(this.location instanceof Address)) 4170 throw new FHIRException("Type mismatch: the type Address was expected, but " 4171 + this.location.getClass().getName() + " was encountered"); 4172 return (Address) this.location; 4173 } 4174 4175 public boolean hasLocationAddress() { 4176 return this != null && this.location instanceof Address; 4177 } 4178 4179 /** 4180 * @return {@link #location} (The physical location of the accident event.) 4181 */ 4182 public Reference getLocationReference() throws FHIRException { 4183 if (this.location == null) 4184 this.location = new Reference(); 4185 if (!(this.location instanceof Reference)) 4186 throw new FHIRException("Type mismatch: the type Reference was expected, but " 4187 + this.location.getClass().getName() + " was encountered"); 4188 return (Reference) this.location; 4189 } 4190 4191 public boolean hasLocationReference() { 4192 return this != null && this.location instanceof Reference; 4193 } 4194 4195 public boolean hasLocation() { 4196 return this.location != null && !this.location.isEmpty(); 4197 } 4198 4199 /** 4200 * @param value {@link #location} (The physical location of the accident event.) 4201 */ 4202 public AccidentComponent setLocation(Type value) { 4203 if (value != null && !(value instanceof Address || value instanceof Reference)) 4204 throw new Error("Not the right type for Claim.accident.location[x]: " + value.fhirType()); 4205 this.location = value; 4206 return this; 4207 } 4208 4209 protected void listChildren(List<Property> children) { 4210 super.listChildren(children); 4211 children.add(new Property("date", "date", 4212 "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 4213 children.add(new Property("type", "CodeableConcept", 4214 "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 4215 0, 1, type)); 4216 children.add(new Property("location[x]", "Address|Reference(Location)", 4217 "The physical location of the accident event.", 0, 1, location)); 4218 } 4219 4220 @Override 4221 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4222 switch (_hash) { 4223 case 3076014: 4224 /* date */ return new Property("date", "date", 4225 "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 4226 case 3575610: 4227 /* type */ return new Property("type", "CodeableConcept", 4228 "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 4229 0, 1, type); 4230 case 552316075: 4231 /* location[x] */ return new Property("location[x]", "Address|Reference(Location)", 4232 "The physical location of the accident event.", 0, 1, location); 4233 case 1901043637: 4234 /* location */ return new Property("location[x]", "Address|Reference(Location)", 4235 "The physical location of the accident event.", 0, 1, location); 4236 case -1280020865: 4237 /* locationAddress */ return new Property("location[x]", "Address|Reference(Location)", 4238 "The physical location of the accident event.", 0, 1, location); 4239 case 755866390: 4240 /* locationReference */ return new Property("location[x]", "Address|Reference(Location)", 4241 "The physical location of the accident event.", 0, 1, location); 4242 default: 4243 return super.getNamedProperty(_hash, _name, _checkValid); 4244 } 4245 4246 } 4247 4248 @Override 4249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4250 switch (hash) { 4251 case 3076014: 4252 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 4253 case 3575610: 4254 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4255 case 1901043637: 4256 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 4257 default: 4258 return super.getProperty(hash, name, checkValid); 4259 } 4260 4261 } 4262 4263 @Override 4264 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4265 switch (hash) { 4266 case 3076014: // date 4267 this.date = castToDate(value); // DateType 4268 return value; 4269 case 3575610: // type 4270 this.type = castToCodeableConcept(value); // CodeableConcept 4271 return value; 4272 case 1901043637: // location 4273 this.location = castToType(value); // Type 4274 return value; 4275 default: 4276 return super.setProperty(hash, name, value); 4277 } 4278 4279 } 4280 4281 @Override 4282 public Base setProperty(String name, Base value) throws FHIRException { 4283 if (name.equals("date")) { 4284 this.date = castToDate(value); // DateType 4285 } else if (name.equals("type")) { 4286 this.type = castToCodeableConcept(value); // CodeableConcept 4287 } else if (name.equals("location[x]")) { 4288 this.location = castToType(value); // Type 4289 } else 4290 return super.setProperty(name, value); 4291 return value; 4292 } 4293 4294 @Override 4295 public void removeChild(String name, Base value) throws FHIRException { 4296 if (name.equals("date")) { 4297 this.date = null; 4298 } else if (name.equals("type")) { 4299 this.type = null; 4300 } else if (name.equals("location[x]")) { 4301 this.location = null; 4302 } else 4303 super.removeChild(name, value); 4304 4305 } 4306 4307 @Override 4308 public Base makeProperty(int hash, String name) throws FHIRException { 4309 switch (hash) { 4310 case 3076014: 4311 return getDateElement(); 4312 case 3575610: 4313 return getType(); 4314 case 552316075: 4315 return getLocation(); 4316 case 1901043637: 4317 return getLocation(); 4318 default: 4319 return super.makeProperty(hash, name); 4320 } 4321 4322 } 4323 4324 @Override 4325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4326 switch (hash) { 4327 case 3076014: 4328 /* date */ return new String[] { "date" }; 4329 case 3575610: 4330 /* type */ return new String[] { "CodeableConcept" }; 4331 case 1901043637: 4332 /* location */ return new String[] { "Address", "Reference" }; 4333 default: 4334 return super.getTypesForProperty(hash, name); 4335 } 4336 4337 } 4338 4339 @Override 4340 public Base addChild(String name) throws FHIRException { 4341 if (name.equals("date")) { 4342 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 4343 } else if (name.equals("type")) { 4344 this.type = new CodeableConcept(); 4345 return this.type; 4346 } else if (name.equals("locationAddress")) { 4347 this.location = new Address(); 4348 return this.location; 4349 } else if (name.equals("locationReference")) { 4350 this.location = new Reference(); 4351 return this.location; 4352 } else 4353 return super.addChild(name); 4354 } 4355 4356 public AccidentComponent copy() { 4357 AccidentComponent dst = new AccidentComponent(); 4358 copyValues(dst); 4359 return dst; 4360 } 4361 4362 public void copyValues(AccidentComponent dst) { 4363 super.copyValues(dst); 4364 dst.date = date == null ? null : date.copy(); 4365 dst.type = type == null ? null : type.copy(); 4366 dst.location = location == null ? null : location.copy(); 4367 } 4368 4369 @Override 4370 public boolean equalsDeep(Base other_) { 4371 if (!super.equalsDeep(other_)) 4372 return false; 4373 if (!(other_ instanceof AccidentComponent)) 4374 return false; 4375 AccidentComponent o = (AccidentComponent) other_; 4376 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) 4377 && compareDeep(location, o.location, true); 4378 } 4379 4380 @Override 4381 public boolean equalsShallow(Base other_) { 4382 if (!super.equalsShallow(other_)) 4383 return false; 4384 if (!(other_ instanceof AccidentComponent)) 4385 return false; 4386 AccidentComponent o = (AccidentComponent) other_; 4387 return compareValues(date, o.date, true); 4388 } 4389 4390 public boolean isEmpty() { 4391 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 4392 } 4393 4394 public String fhirType() { 4395 return "Claim.accident"; 4396 4397 } 4398 4399 } 4400 4401 @Block() 4402 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 4403 /** 4404 * A number to uniquely identify item entries. 4405 */ 4406 @Child(name = "sequence", type = { 4407 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4408 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 4409 protected PositiveIntType sequence; 4410 4411 /** 4412 * CareTeam members related to this service or product. 4413 */ 4414 @Child(name = "careTeamSequence", type = { 4415 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4416 @Description(shortDefinition = "Applicable careTeam members", formalDefinition = "CareTeam members related to this service or product.") 4417 protected List<PositiveIntType> careTeamSequence; 4418 4419 /** 4420 * Diagnosis applicable for this service or product. 4421 */ 4422 @Child(name = "diagnosisSequence", type = { 4423 PositiveIntType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4424 @Description(shortDefinition = "Applicable diagnoses", formalDefinition = "Diagnosis applicable for this service or product.") 4425 protected List<PositiveIntType> diagnosisSequence; 4426 4427 /** 4428 * Procedures applicable for this service or product. 4429 */ 4430 @Child(name = "procedureSequence", type = { 4431 PositiveIntType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4432 @Description(shortDefinition = "Applicable procedures", formalDefinition = "Procedures applicable for this service or product.") 4433 protected List<PositiveIntType> procedureSequence; 4434 4435 /** 4436 * Exceptions, special conditions and supporting information applicable for this 4437 * service or product. 4438 */ 4439 @Child(name = "informationSequence", type = { 4440 PositiveIntType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4441 @Description(shortDefinition = "Applicable exception and supporting information", formalDefinition = "Exceptions, special conditions and supporting information applicable for this service or product.") 4442 protected List<PositiveIntType> informationSequence; 4443 4444 /** 4445 * The type of revenue or cost center providing the product and/or service. 4446 */ 4447 @Child(name = "revenue", type = { 4448 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4449 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 4450 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 4451 protected CodeableConcept revenue; 4452 4453 /** 4454 * Code to identify the general type of benefits under which products and 4455 * services are provided. 4456 */ 4457 @Child(name = "category", type = { 4458 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4459 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 4460 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 4461 protected CodeableConcept category; 4462 4463 /** 4464 * When the value is a group code then this item collects a set of related claim 4465 * details, otherwise this contains the product, service, drug or other billing 4466 * code for the item. 4467 */ 4468 @Child(name = "productOrService", type = { 4469 CodeableConcept.class }, order = 8, min = 1, max = 1, modifier = false, summary = false) 4470 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4471 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4472 protected CodeableConcept productOrService; 4473 4474 /** 4475 * Item typification or modifiers codes to convey additional context for the 4476 * product or service. 4477 */ 4478 @Child(name = "modifier", type = { 4479 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4480 @Description(shortDefinition = "Product or service billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4482 protected List<CodeableConcept> modifier; 4483 4484 /** 4485 * Identifies the program under which this may be recovered. 4486 */ 4487 @Child(name = "programCode", type = { 4488 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4489 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 4490 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 4491 protected List<CodeableConcept> programCode; 4492 4493 /** 4494 * The date or dates when the service or product was supplied, performed or 4495 * completed. 4496 */ 4497 @Child(name = "serviced", type = { DateType.class, 4498 Period.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4499 @Description(shortDefinition = "Date or dates of service or product delivery", formalDefinition = "The date or dates when the service or product was supplied, performed or completed.") 4500 protected Type serviced; 4501 4502 /** 4503 * Where the product or service was provided. 4504 */ 4505 @Child(name = "location", type = { CodeableConcept.class, Address.class, 4506 Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 4507 @Description(shortDefinition = "Place of service or where product was supplied", formalDefinition = "Where the product or service was provided.") 4508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-place") 4509 protected Type location; 4510 4511 /** 4512 * The number of repetitions of a service or product. 4513 */ 4514 @Child(name = "quantity", type = { 4515 Quantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4516 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4517 protected Quantity quantity; 4518 4519 /** 4520 * If the item is not a group then this is the fee for the product or service, 4521 * otherwise this is the total of the fees for the details of the group. 4522 */ 4523 @Child(name = "unitPrice", type = { Money.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 4524 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 4525 protected Money unitPrice; 4526 4527 /** 4528 * A real number that represents a multiplier used in determining the overall 4529 * value of services delivered and/or goods received. The concept of a Factor 4530 * allows for a discount or surcharge multiplier to be applied to a monetary 4531 * amount. 4532 */ 4533 @Child(name = "factor", type = { 4534 DecimalType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 4535 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 4536 protected DecimalType factor; 4537 4538 /** 4539 * The quantity times the unit price for an additional service or product or 4540 * charge. 4541 */ 4542 @Child(name = "net", type = { Money.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 4543 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 4544 protected Money net; 4545 4546 /** 4547 * Unique Device Identifiers associated with this line item. 4548 */ 4549 @Child(name = "udi", type = { 4550 Device.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4551 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 4552 protected List<Reference> udi; 4553 /** 4554 * The actual objects that are the target of the reference (Unique Device 4555 * Identifiers associated with this line item.) 4556 */ 4557 protected List<Device> udiTarget; 4558 4559 /** 4560 * Physical service site on the patient (limb, tooth, etc.). 4561 */ 4562 @Child(name = "bodySite", type = { 4563 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 4564 @Description(shortDefinition = "Anatomical location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 4565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/tooth") 4566 protected CodeableConcept bodySite; 4567 4568 /** 4569 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 4570 */ 4571 @Child(name = "subSite", type = { 4572 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4573 @Description(shortDefinition = "Anatomical sub-location", formalDefinition = "A region or surface of the bodySite, e.g. limb region or tooth surface(s).") 4574 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/surface") 4575 protected List<CodeableConcept> subSite; 4576 4577 /** 4578 * The Encounters during which this Claim was created or to which the creation 4579 * of this record is tightly associated. 4580 */ 4581 @Child(name = "encounter", type = { 4582 Encounter.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4583 @Description(shortDefinition = "Encounters related to this billed item", formalDefinition = "The Encounters during which this Claim was created or to which the creation of this record is tightly associated.") 4584 protected List<Reference> encounter; 4585 /** 4586 * The actual objects that are the target of the reference (The Encounters 4587 * during which this Claim was created or to which the creation of this record 4588 * is tightly associated.) 4589 */ 4590 protected List<Encounter> encounterTarget; 4591 4592 /** 4593 * A claim detail line. Either a simple (a product or service) or a 'group' of 4594 * sub-details which are simple items. 4595 */ 4596 @Child(name = "detail", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4597 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 4598 protected List<DetailComponent> detail; 4599 4600 private static final long serialVersionUID = -329028323L; 4601 4602 /** 4603 * Constructor 4604 */ 4605 public ItemComponent() { 4606 super(); 4607 } 4608 4609 /** 4610 * Constructor 4611 */ 4612 public ItemComponent(PositiveIntType sequence, CodeableConcept productOrService) { 4613 super(); 4614 this.sequence = sequence; 4615 this.productOrService = productOrService; 4616 } 4617 4618 /** 4619 * @return {@link #sequence} (A number to uniquely identify item entries.). This 4620 * is the underlying object with id, value and extensions. The accessor 4621 * "getSequence" gives direct access to the value 4622 */ 4623 public PositiveIntType getSequenceElement() { 4624 if (this.sequence == null) 4625 if (Configuration.errorOnAutoCreate()) 4626 throw new Error("Attempt to auto-create ItemComponent.sequence"); 4627 else if (Configuration.doAutoCreate()) 4628 this.sequence = new PositiveIntType(); // bb 4629 return this.sequence; 4630 } 4631 4632 public boolean hasSequenceElement() { 4633 return this.sequence != null && !this.sequence.isEmpty(); 4634 } 4635 4636 public boolean hasSequence() { 4637 return this.sequence != null && !this.sequence.isEmpty(); 4638 } 4639 4640 /** 4641 * @param value {@link #sequence} (A number to uniquely identify item entries.). 4642 * This is the underlying object with id, value and extensions. The 4643 * accessor "getSequence" gives direct access to the value 4644 */ 4645 public ItemComponent setSequenceElement(PositiveIntType value) { 4646 this.sequence = value; 4647 return this; 4648 } 4649 4650 /** 4651 * @return A number to uniquely identify item entries. 4652 */ 4653 public int getSequence() { 4654 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 4655 } 4656 4657 /** 4658 * @param value A number to uniquely identify item entries. 4659 */ 4660 public ItemComponent setSequence(int value) { 4661 if (this.sequence == null) 4662 this.sequence = new PositiveIntType(); 4663 this.sequence.setValue(value); 4664 return this; 4665 } 4666 4667 /** 4668 * @return {@link #careTeamSequence} (CareTeam members related to this service 4669 * or product.) 4670 */ 4671 public List<PositiveIntType> getCareTeamSequence() { 4672 if (this.careTeamSequence == null) 4673 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4674 return this.careTeamSequence; 4675 } 4676 4677 /** 4678 * @return Returns a reference to <code>this</code> for easy method chaining 4679 */ 4680 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 4681 this.careTeamSequence = theCareTeamSequence; 4682 return this; 4683 } 4684 4685 public boolean hasCareTeamSequence() { 4686 if (this.careTeamSequence == null) 4687 return false; 4688 for (PositiveIntType item : this.careTeamSequence) 4689 if (!item.isEmpty()) 4690 return true; 4691 return false; 4692 } 4693 4694 /** 4695 * @return {@link #careTeamSequence} (CareTeam members related to this service 4696 * or product.) 4697 */ 4698 public PositiveIntType addCareTeamSequenceElement() {// 2 4699 PositiveIntType t = new PositiveIntType(); 4700 if (this.careTeamSequence == null) 4701 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4702 this.careTeamSequence.add(t); 4703 return t; 4704 } 4705 4706 /** 4707 * @param value {@link #careTeamSequence} (CareTeam members related to this 4708 * service or product.) 4709 */ 4710 public ItemComponent addCareTeamSequence(int value) { // 1 4711 PositiveIntType t = new PositiveIntType(); 4712 t.setValue(value); 4713 if (this.careTeamSequence == null) 4714 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4715 this.careTeamSequence.add(t); 4716 return this; 4717 } 4718 4719 /** 4720 * @param value {@link #careTeamSequence} (CareTeam members related to this 4721 * service or product.) 4722 */ 4723 public boolean hasCareTeamSequence(int value) { 4724 if (this.careTeamSequence == null) 4725 return false; 4726 for (PositiveIntType v : this.careTeamSequence) 4727 if (v.getValue().equals(value)) // positiveInt 4728 return true; 4729 return false; 4730 } 4731 4732 /** 4733 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or 4734 * product.) 4735 */ 4736 public List<PositiveIntType> getDiagnosisSequence() { 4737 if (this.diagnosisSequence == null) 4738 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4739 return this.diagnosisSequence; 4740 } 4741 4742 /** 4743 * @return Returns a reference to <code>this</code> for easy method chaining 4744 */ 4745 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 4746 this.diagnosisSequence = theDiagnosisSequence; 4747 return this; 4748 } 4749 4750 public boolean hasDiagnosisSequence() { 4751 if (this.diagnosisSequence == null) 4752 return false; 4753 for (PositiveIntType item : this.diagnosisSequence) 4754 if (!item.isEmpty()) 4755 return true; 4756 return false; 4757 } 4758 4759 /** 4760 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or 4761 * product.) 4762 */ 4763 public PositiveIntType addDiagnosisSequenceElement() {// 2 4764 PositiveIntType t = new PositiveIntType(); 4765 if (this.diagnosisSequence == null) 4766 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4767 this.diagnosisSequence.add(t); 4768 return t; 4769 } 4770 4771 /** 4772 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this 4773 * service or product.) 4774 */ 4775 public ItemComponent addDiagnosisSequence(int value) { // 1 4776 PositiveIntType t = new PositiveIntType(); 4777 t.setValue(value); 4778 if (this.diagnosisSequence == null) 4779 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4780 this.diagnosisSequence.add(t); 4781 return this; 4782 } 4783 4784 /** 4785 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this 4786 * service or product.) 4787 */ 4788 public boolean hasDiagnosisSequence(int value) { 4789 if (this.diagnosisSequence == null) 4790 return false; 4791 for (PositiveIntType v : this.diagnosisSequence) 4792 if (v.getValue().equals(value)) // positiveInt 4793 return true; 4794 return false; 4795 } 4796 4797 /** 4798 * @return {@link #procedureSequence} (Procedures applicable for this service or 4799 * product.) 4800 */ 4801 public List<PositiveIntType> getProcedureSequence() { 4802 if (this.procedureSequence == null) 4803 this.procedureSequence = new ArrayList<PositiveIntType>(); 4804 return this.procedureSequence; 4805 } 4806 4807 /** 4808 * @return Returns a reference to <code>this</code> for easy method chaining 4809 */ 4810 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 4811 this.procedureSequence = theProcedureSequence; 4812 return this; 4813 } 4814 4815 public boolean hasProcedureSequence() { 4816 if (this.procedureSequence == null) 4817 return false; 4818 for (PositiveIntType item : this.procedureSequence) 4819 if (!item.isEmpty()) 4820 return true; 4821 return false; 4822 } 4823 4824 /** 4825 * @return {@link #procedureSequence} (Procedures applicable for this service or 4826 * product.) 4827 */ 4828 public PositiveIntType addProcedureSequenceElement() {// 2 4829 PositiveIntType t = new PositiveIntType(); 4830 if (this.procedureSequence == null) 4831 this.procedureSequence = new ArrayList<PositiveIntType>(); 4832 this.procedureSequence.add(t); 4833 return t; 4834 } 4835 4836 /** 4837 * @param value {@link #procedureSequence} (Procedures applicable for this 4838 * service or product.) 4839 */ 4840 public ItemComponent addProcedureSequence(int value) { // 1 4841 PositiveIntType t = new PositiveIntType(); 4842 t.setValue(value); 4843 if (this.procedureSequence == null) 4844 this.procedureSequence = new ArrayList<PositiveIntType>(); 4845 this.procedureSequence.add(t); 4846 return this; 4847 } 4848 4849 /** 4850 * @param value {@link #procedureSequence} (Procedures applicable for this 4851 * service or product.) 4852 */ 4853 public boolean hasProcedureSequence(int value) { 4854 if (this.procedureSequence == null) 4855 return false; 4856 for (PositiveIntType v : this.procedureSequence) 4857 if (v.getValue().equals(value)) // positiveInt 4858 return true; 4859 return false; 4860 } 4861 4862 /** 4863 * @return {@link #informationSequence} (Exceptions, special conditions and 4864 * supporting information applicable for this service or product.) 4865 */ 4866 public List<PositiveIntType> getInformationSequence() { 4867 if (this.informationSequence == null) 4868 this.informationSequence = new ArrayList<PositiveIntType>(); 4869 return this.informationSequence; 4870 } 4871 4872 /** 4873 * @return Returns a reference to <code>this</code> for easy method chaining 4874 */ 4875 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 4876 this.informationSequence = theInformationSequence; 4877 return this; 4878 } 4879 4880 public boolean hasInformationSequence() { 4881 if (this.informationSequence == null) 4882 return false; 4883 for (PositiveIntType item : this.informationSequence) 4884 if (!item.isEmpty()) 4885 return true; 4886 return false; 4887 } 4888 4889 /** 4890 * @return {@link #informationSequence} (Exceptions, special conditions and 4891 * supporting information applicable for this service or product.) 4892 */ 4893 public PositiveIntType addInformationSequenceElement() {// 2 4894 PositiveIntType t = new PositiveIntType(); 4895 if (this.informationSequence == null) 4896 this.informationSequence = new ArrayList<PositiveIntType>(); 4897 this.informationSequence.add(t); 4898 return t; 4899 } 4900 4901 /** 4902 * @param value {@link #informationSequence} (Exceptions, special conditions and 4903 * supporting information applicable for this service or product.) 4904 */ 4905 public ItemComponent addInformationSequence(int value) { // 1 4906 PositiveIntType t = new PositiveIntType(); 4907 t.setValue(value); 4908 if (this.informationSequence == null) 4909 this.informationSequence = new ArrayList<PositiveIntType>(); 4910 this.informationSequence.add(t); 4911 return this; 4912 } 4913 4914 /** 4915 * @param value {@link #informationSequence} (Exceptions, special conditions and 4916 * supporting information applicable for this service or product.) 4917 */ 4918 public boolean hasInformationSequence(int value) { 4919 if (this.informationSequence == null) 4920 return false; 4921 for (PositiveIntType v : this.informationSequence) 4922 if (v.getValue().equals(value)) // positiveInt 4923 return true; 4924 return false; 4925 } 4926 4927 /** 4928 * @return {@link #revenue} (The type of revenue or cost center providing the 4929 * product and/or service.) 4930 */ 4931 public CodeableConcept getRevenue() { 4932 if (this.revenue == null) 4933 if (Configuration.errorOnAutoCreate()) 4934 throw new Error("Attempt to auto-create ItemComponent.revenue"); 4935 else if (Configuration.doAutoCreate()) 4936 this.revenue = new CodeableConcept(); // cc 4937 return this.revenue; 4938 } 4939 4940 public boolean hasRevenue() { 4941 return this.revenue != null && !this.revenue.isEmpty(); 4942 } 4943 4944 /** 4945 * @param value {@link #revenue} (The type of revenue or cost center providing 4946 * the product and/or service.) 4947 */ 4948 public ItemComponent setRevenue(CodeableConcept value) { 4949 this.revenue = value; 4950 return this; 4951 } 4952 4953 /** 4954 * @return {@link #category} (Code to identify the general type of benefits 4955 * under which products and services are provided.) 4956 */ 4957 public CodeableConcept getCategory() { 4958 if (this.category == null) 4959 if (Configuration.errorOnAutoCreate()) 4960 throw new Error("Attempt to auto-create ItemComponent.category"); 4961 else if (Configuration.doAutoCreate()) 4962 this.category = new CodeableConcept(); // cc 4963 return this.category; 4964 } 4965 4966 public boolean hasCategory() { 4967 return this.category != null && !this.category.isEmpty(); 4968 } 4969 4970 /** 4971 * @param value {@link #category} (Code to identify the general type of benefits 4972 * under which products and services are provided.) 4973 */ 4974 public ItemComponent setCategory(CodeableConcept value) { 4975 this.category = value; 4976 return this; 4977 } 4978 4979 /** 4980 * @return {@link #productOrService} (When the value is a group code then this 4981 * item collects a set of related claim details, otherwise this contains 4982 * the product, service, drug or other billing code for the item.) 4983 */ 4984 public CodeableConcept getProductOrService() { 4985 if (this.productOrService == null) 4986 if (Configuration.errorOnAutoCreate()) 4987 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 4988 else if (Configuration.doAutoCreate()) 4989 this.productOrService = new CodeableConcept(); // cc 4990 return this.productOrService; 4991 } 4992 4993 public boolean hasProductOrService() { 4994 return this.productOrService != null && !this.productOrService.isEmpty(); 4995 } 4996 4997 /** 4998 * @param value {@link #productOrService} (When the value is a group code then 4999 * this item collects a set of related claim details, otherwise 5000 * this contains the product, service, drug or other billing code 5001 * for the item.) 5002 */ 5003 public ItemComponent setProductOrService(CodeableConcept value) { 5004 this.productOrService = value; 5005 return this; 5006 } 5007 5008 /** 5009 * @return {@link #modifier} (Item typification or modifiers codes to convey 5010 * additional context for the product or service.) 5011 */ 5012 public List<CodeableConcept> getModifier() { 5013 if (this.modifier == null) 5014 this.modifier = new ArrayList<CodeableConcept>(); 5015 return this.modifier; 5016 } 5017 5018 /** 5019 * @return Returns a reference to <code>this</code> for easy method chaining 5020 */ 5021 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 5022 this.modifier = theModifier; 5023 return this; 5024 } 5025 5026 public boolean hasModifier() { 5027 if (this.modifier == null) 5028 return false; 5029 for (CodeableConcept item : this.modifier) 5030 if (!item.isEmpty()) 5031 return true; 5032 return false; 5033 } 5034 5035 public CodeableConcept addModifier() { // 3 5036 CodeableConcept t = new CodeableConcept(); 5037 if (this.modifier == null) 5038 this.modifier = new ArrayList<CodeableConcept>(); 5039 this.modifier.add(t); 5040 return t; 5041 } 5042 5043 public ItemComponent addModifier(CodeableConcept t) { // 3 5044 if (t == null) 5045 return this; 5046 if (this.modifier == null) 5047 this.modifier = new ArrayList<CodeableConcept>(); 5048 this.modifier.add(t); 5049 return this; 5050 } 5051 5052 /** 5053 * @return The first repetition of repeating field {@link #modifier}, creating 5054 * it if it does not already exist 5055 */ 5056 public CodeableConcept getModifierFirstRep() { 5057 if (getModifier().isEmpty()) { 5058 addModifier(); 5059 } 5060 return getModifier().get(0); 5061 } 5062 5063 /** 5064 * @return {@link #programCode} (Identifies the program under which this may be 5065 * recovered.) 5066 */ 5067 public List<CodeableConcept> getProgramCode() { 5068 if (this.programCode == null) 5069 this.programCode = new ArrayList<CodeableConcept>(); 5070 return this.programCode; 5071 } 5072 5073 /** 5074 * @return Returns a reference to <code>this</code> for easy method chaining 5075 */ 5076 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 5077 this.programCode = theProgramCode; 5078 return this; 5079 } 5080 5081 public boolean hasProgramCode() { 5082 if (this.programCode == null) 5083 return false; 5084 for (CodeableConcept item : this.programCode) 5085 if (!item.isEmpty()) 5086 return true; 5087 return false; 5088 } 5089 5090 public CodeableConcept addProgramCode() { // 3 5091 CodeableConcept t = new CodeableConcept(); 5092 if (this.programCode == null) 5093 this.programCode = new ArrayList<CodeableConcept>(); 5094 this.programCode.add(t); 5095 return t; 5096 } 5097 5098 public ItemComponent addProgramCode(CodeableConcept t) { // 3 5099 if (t == null) 5100 return this; 5101 if (this.programCode == null) 5102 this.programCode = new ArrayList<CodeableConcept>(); 5103 this.programCode.add(t); 5104 return this; 5105 } 5106 5107 /** 5108 * @return The first repetition of repeating field {@link #programCode}, 5109 * creating it if it does not already exist 5110 */ 5111 public CodeableConcept getProgramCodeFirstRep() { 5112 if (getProgramCode().isEmpty()) { 5113 addProgramCode(); 5114 } 5115 return getProgramCode().get(0); 5116 } 5117 5118 /** 5119 * @return {@link #serviced} (The date or dates when the service or product was 5120 * supplied, performed or completed.) 5121 */ 5122 public Type getServiced() { 5123 return this.serviced; 5124 } 5125 5126 /** 5127 * @return {@link #serviced} (The date or dates when the service or product was 5128 * supplied, performed or completed.) 5129 */ 5130 public DateType getServicedDateType() throws FHIRException { 5131 if (this.serviced == null) 5132 this.serviced = new DateType(); 5133 if (!(this.serviced instanceof DateType)) 5134 throw new FHIRException("Type mismatch: the type DateType was expected, but " 5135 + this.serviced.getClass().getName() + " was encountered"); 5136 return (DateType) this.serviced; 5137 } 5138 5139 public boolean hasServicedDateType() { 5140 return this != null && this.serviced instanceof DateType; 5141 } 5142 5143 /** 5144 * @return {@link #serviced} (The date or dates when the service or product was 5145 * supplied, performed or completed.) 5146 */ 5147 public Period getServicedPeriod() throws FHIRException { 5148 if (this.serviced == null) 5149 this.serviced = new Period(); 5150 if (!(this.serviced instanceof Period)) 5151 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 5152 + " was encountered"); 5153 return (Period) this.serviced; 5154 } 5155 5156 public boolean hasServicedPeriod() { 5157 return this != null && this.serviced instanceof Period; 5158 } 5159 5160 public boolean hasServiced() { 5161 return this.serviced != null && !this.serviced.isEmpty(); 5162 } 5163 5164 /** 5165 * @param value {@link #serviced} (The date or dates when the service or product 5166 * was supplied, performed or completed.) 5167 */ 5168 public ItemComponent setServiced(Type value) { 5169 if (value != null && !(value instanceof DateType || value instanceof Period)) 5170 throw new Error("Not the right type for Claim.item.serviced[x]: " + value.fhirType()); 5171 this.serviced = value; 5172 return this; 5173 } 5174 5175 /** 5176 * @return {@link #location} (Where the product or service was provided.) 5177 */ 5178 public Type getLocation() { 5179 return this.location; 5180 } 5181 5182 /** 5183 * @return {@link #location} (Where the product or service was provided.) 5184 */ 5185 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 5186 if (this.location == null) 5187 this.location = new CodeableConcept(); 5188 if (!(this.location instanceof CodeableConcept)) 5189 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 5190 + this.location.getClass().getName() + " was encountered"); 5191 return (CodeableConcept) this.location; 5192 } 5193 5194 public boolean hasLocationCodeableConcept() { 5195 return this != null && this.location instanceof CodeableConcept; 5196 } 5197 5198 /** 5199 * @return {@link #location} (Where the product or service was provided.) 5200 */ 5201 public Address getLocationAddress() throws FHIRException { 5202 if (this.location == null) 5203 this.location = new Address(); 5204 if (!(this.location instanceof Address)) 5205 throw new FHIRException("Type mismatch: the type Address was expected, but " 5206 + this.location.getClass().getName() + " was encountered"); 5207 return (Address) this.location; 5208 } 5209 5210 public boolean hasLocationAddress() { 5211 return this != null && this.location instanceof Address; 5212 } 5213 5214 /** 5215 * @return {@link #location} (Where the product or service was provided.) 5216 */ 5217 public Reference getLocationReference() throws FHIRException { 5218 if (this.location == null) 5219 this.location = new Reference(); 5220 if (!(this.location instanceof Reference)) 5221 throw new FHIRException("Type mismatch: the type Reference was expected, but " 5222 + this.location.getClass().getName() + " was encountered"); 5223 return (Reference) this.location; 5224 } 5225 5226 public boolean hasLocationReference() { 5227 return this != null && this.location instanceof Reference; 5228 } 5229 5230 public boolean hasLocation() { 5231 return this.location != null && !this.location.isEmpty(); 5232 } 5233 5234 /** 5235 * @param value {@link #location} (Where the product or service was provided.) 5236 */ 5237 public ItemComponent setLocation(Type value) { 5238 if (value != null 5239 && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 5240 throw new Error("Not the right type for Claim.item.location[x]: " + value.fhirType()); 5241 this.location = value; 5242 return this; 5243 } 5244 5245 /** 5246 * @return {@link #quantity} (The number of repetitions of a service or 5247 * product.) 5248 */ 5249 public Quantity getQuantity() { 5250 if (this.quantity == null) 5251 if (Configuration.errorOnAutoCreate()) 5252 throw new Error("Attempt to auto-create ItemComponent.quantity"); 5253 else if (Configuration.doAutoCreate()) 5254 this.quantity = new Quantity(); // cc 5255 return this.quantity; 5256 } 5257 5258 public boolean hasQuantity() { 5259 return this.quantity != null && !this.quantity.isEmpty(); 5260 } 5261 5262 /** 5263 * @param value {@link #quantity} (The number of repetitions of a service or 5264 * product.) 5265 */ 5266 public ItemComponent setQuantity(Quantity value) { 5267 this.quantity = value; 5268 return this; 5269 } 5270 5271 /** 5272 * @return {@link #unitPrice} (If the item is not a group then this is the fee 5273 * for the product or service, otherwise this is the total of the fees 5274 * for the details of the group.) 5275 */ 5276 public Money getUnitPrice() { 5277 if (this.unitPrice == null) 5278 if (Configuration.errorOnAutoCreate()) 5279 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 5280 else if (Configuration.doAutoCreate()) 5281 this.unitPrice = new Money(); // cc 5282 return this.unitPrice; 5283 } 5284 5285 public boolean hasUnitPrice() { 5286 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5287 } 5288 5289 /** 5290 * @param value {@link #unitPrice} (If the item is not a group then this is the 5291 * fee for the product or service, otherwise this is the total of 5292 * the fees for the details of the group.) 5293 */ 5294 public ItemComponent setUnitPrice(Money value) { 5295 this.unitPrice = value; 5296 return this; 5297 } 5298 5299 /** 5300 * @return {@link #factor} (A real number that represents a multiplier used in 5301 * determining the overall value of services delivered and/or goods 5302 * received. The concept of a Factor allows for a discount or surcharge 5303 * multiplier to be applied to a monetary amount.). This is the 5304 * underlying object with id, value and extensions. The accessor 5305 * "getFactor" gives direct access to the value 5306 */ 5307 public DecimalType getFactorElement() { 5308 if (this.factor == null) 5309 if (Configuration.errorOnAutoCreate()) 5310 throw new Error("Attempt to auto-create ItemComponent.factor"); 5311 else if (Configuration.doAutoCreate()) 5312 this.factor = new DecimalType(); // bb 5313 return this.factor; 5314 } 5315 5316 public boolean hasFactorElement() { 5317 return this.factor != null && !this.factor.isEmpty(); 5318 } 5319 5320 public boolean hasFactor() { 5321 return this.factor != null && !this.factor.isEmpty(); 5322 } 5323 5324 /** 5325 * @param value {@link #factor} (A real number that represents a multiplier used 5326 * in determining the overall value of services delivered and/or 5327 * goods received. The concept of a Factor allows for a discount or 5328 * surcharge multiplier to be applied to a monetary amount.). This 5329 * is the underlying object with id, value and extensions. The 5330 * accessor "getFactor" gives direct access to the value 5331 */ 5332 public ItemComponent setFactorElement(DecimalType value) { 5333 this.factor = value; 5334 return this; 5335 } 5336 5337 /** 5338 * @return A real number that represents a multiplier used in determining the 5339 * overall value of services delivered and/or goods received. The 5340 * concept of a Factor allows for a discount or surcharge multiplier to 5341 * be applied to a monetary amount. 5342 */ 5343 public BigDecimal getFactor() { 5344 return this.factor == null ? null : this.factor.getValue(); 5345 } 5346 5347 /** 5348 * @param value A real number that represents a multiplier used in determining 5349 * the overall value of services delivered and/or goods received. 5350 * The concept of a Factor allows for a discount or surcharge 5351 * multiplier to be applied to a monetary amount. 5352 */ 5353 public ItemComponent setFactor(BigDecimal value) { 5354 if (value == null) 5355 this.factor = null; 5356 else { 5357 if (this.factor == null) 5358 this.factor = new DecimalType(); 5359 this.factor.setValue(value); 5360 } 5361 return this; 5362 } 5363 5364 /** 5365 * @param value A real number that represents a multiplier used in determining 5366 * the overall value of services delivered and/or goods received. 5367 * The concept of a Factor allows for a discount or surcharge 5368 * multiplier to be applied to a monetary amount. 5369 */ 5370 public ItemComponent setFactor(long value) { 5371 this.factor = new DecimalType(); 5372 this.factor.setValue(value); 5373 return this; 5374 } 5375 5376 /** 5377 * @param value A real number that represents a multiplier used in determining 5378 * the overall value of services delivered and/or goods received. 5379 * The concept of a Factor allows for a discount or surcharge 5380 * multiplier to be applied to a monetary amount. 5381 */ 5382 public ItemComponent setFactor(double value) { 5383 this.factor = new DecimalType(); 5384 this.factor.setValue(value); 5385 return this; 5386 } 5387 5388 /** 5389 * @return {@link #net} (The quantity times the unit price for an additional 5390 * service or product or charge.) 5391 */ 5392 public Money getNet() { 5393 if (this.net == null) 5394 if (Configuration.errorOnAutoCreate()) 5395 throw new Error("Attempt to auto-create ItemComponent.net"); 5396 else if (Configuration.doAutoCreate()) 5397 this.net = new Money(); // cc 5398 return this.net; 5399 } 5400 5401 public boolean hasNet() { 5402 return this.net != null && !this.net.isEmpty(); 5403 } 5404 5405 /** 5406 * @param value {@link #net} (The quantity times the unit price for an 5407 * additional service or product or charge.) 5408 */ 5409 public ItemComponent setNet(Money value) { 5410 this.net = value; 5411 return this; 5412 } 5413 5414 /** 5415 * @return {@link #udi} (Unique Device Identifiers associated with this line 5416 * item.) 5417 */ 5418 public List<Reference> getUdi() { 5419 if (this.udi == null) 5420 this.udi = new ArrayList<Reference>(); 5421 return this.udi; 5422 } 5423 5424 /** 5425 * @return Returns a reference to <code>this</code> for easy method chaining 5426 */ 5427 public ItemComponent setUdi(List<Reference> theUdi) { 5428 this.udi = theUdi; 5429 return this; 5430 } 5431 5432 public boolean hasUdi() { 5433 if (this.udi == null) 5434 return false; 5435 for (Reference item : this.udi) 5436 if (!item.isEmpty()) 5437 return true; 5438 return false; 5439 } 5440 5441 public Reference addUdi() { // 3 5442 Reference t = new Reference(); 5443 if (this.udi == null) 5444 this.udi = new ArrayList<Reference>(); 5445 this.udi.add(t); 5446 return t; 5447 } 5448 5449 public ItemComponent addUdi(Reference t) { // 3 5450 if (t == null) 5451 return this; 5452 if (this.udi == null) 5453 this.udi = new ArrayList<Reference>(); 5454 this.udi.add(t); 5455 return this; 5456 } 5457 5458 /** 5459 * @return The first repetition of repeating field {@link #udi}, creating it if 5460 * it does not already exist 5461 */ 5462 public Reference getUdiFirstRep() { 5463 if (getUdi().isEmpty()) { 5464 addUdi(); 5465 } 5466 return getUdi().get(0); 5467 } 5468 5469 /** 5470 * @deprecated Use Reference#setResource(IBaseResource) instead 5471 */ 5472 @Deprecated 5473 public List<Device> getUdiTarget() { 5474 if (this.udiTarget == null) 5475 this.udiTarget = new ArrayList<Device>(); 5476 return this.udiTarget; 5477 } 5478 5479 /** 5480 * @deprecated Use Reference#setResource(IBaseResource) instead 5481 */ 5482 @Deprecated 5483 public Device addUdiTarget() { 5484 Device r = new Device(); 5485 if (this.udiTarget == null) 5486 this.udiTarget = new ArrayList<Device>(); 5487 this.udiTarget.add(r); 5488 return r; 5489 } 5490 5491 /** 5492 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 5493 * etc.).) 5494 */ 5495 public CodeableConcept getBodySite() { 5496 if (this.bodySite == null) 5497 if (Configuration.errorOnAutoCreate()) 5498 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 5499 else if (Configuration.doAutoCreate()) 5500 this.bodySite = new CodeableConcept(); // cc 5501 return this.bodySite; 5502 } 5503 5504 public boolean hasBodySite() { 5505 return this.bodySite != null && !this.bodySite.isEmpty(); 5506 } 5507 5508 /** 5509 * @param value {@link #bodySite} (Physical service site on the patient (limb, 5510 * tooth, etc.).) 5511 */ 5512 public ItemComponent setBodySite(CodeableConcept value) { 5513 this.bodySite = value; 5514 return this; 5515 } 5516 5517 /** 5518 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb 5519 * region or tooth surface(s).) 5520 */ 5521 public List<CodeableConcept> getSubSite() { 5522 if (this.subSite == null) 5523 this.subSite = new ArrayList<CodeableConcept>(); 5524 return this.subSite; 5525 } 5526 5527 /** 5528 * @return Returns a reference to <code>this</code> for easy method chaining 5529 */ 5530 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 5531 this.subSite = theSubSite; 5532 return this; 5533 } 5534 5535 public boolean hasSubSite() { 5536 if (this.subSite == null) 5537 return false; 5538 for (CodeableConcept item : this.subSite) 5539 if (!item.isEmpty()) 5540 return true; 5541 return false; 5542 } 5543 5544 public CodeableConcept addSubSite() { // 3 5545 CodeableConcept t = new CodeableConcept(); 5546 if (this.subSite == null) 5547 this.subSite = new ArrayList<CodeableConcept>(); 5548 this.subSite.add(t); 5549 return t; 5550 } 5551 5552 public ItemComponent addSubSite(CodeableConcept t) { // 3 5553 if (t == null) 5554 return this; 5555 if (this.subSite == null) 5556 this.subSite = new ArrayList<CodeableConcept>(); 5557 this.subSite.add(t); 5558 return this; 5559 } 5560 5561 /** 5562 * @return The first repetition of repeating field {@link #subSite}, creating it 5563 * if it does not already exist 5564 */ 5565 public CodeableConcept getSubSiteFirstRep() { 5566 if (getSubSite().isEmpty()) { 5567 addSubSite(); 5568 } 5569 return getSubSite().get(0); 5570 } 5571 5572 /** 5573 * @return {@link #encounter} (The Encounters during which this Claim was 5574 * created or to which the creation of this record is tightly 5575 * associated.) 5576 */ 5577 public List<Reference> getEncounter() { 5578 if (this.encounter == null) 5579 this.encounter = new ArrayList<Reference>(); 5580 return this.encounter; 5581 } 5582 5583 /** 5584 * @return Returns a reference to <code>this</code> for easy method chaining 5585 */ 5586 public ItemComponent setEncounter(List<Reference> theEncounter) { 5587 this.encounter = theEncounter; 5588 return this; 5589 } 5590 5591 public boolean hasEncounter() { 5592 if (this.encounter == null) 5593 return false; 5594 for (Reference item : this.encounter) 5595 if (!item.isEmpty()) 5596 return true; 5597 return false; 5598 } 5599 5600 public Reference addEncounter() { // 3 5601 Reference t = new Reference(); 5602 if (this.encounter == null) 5603 this.encounter = new ArrayList<Reference>(); 5604 this.encounter.add(t); 5605 return t; 5606 } 5607 5608 public ItemComponent addEncounter(Reference t) { // 3 5609 if (t == null) 5610 return this; 5611 if (this.encounter == null) 5612 this.encounter = new ArrayList<Reference>(); 5613 this.encounter.add(t); 5614 return this; 5615 } 5616 5617 /** 5618 * @return The first repetition of repeating field {@link #encounter}, creating 5619 * it if it does not already exist 5620 */ 5621 public Reference getEncounterFirstRep() { 5622 if (getEncounter().isEmpty()) { 5623 addEncounter(); 5624 } 5625 return getEncounter().get(0); 5626 } 5627 5628 /** 5629 * @deprecated Use Reference#setResource(IBaseResource) instead 5630 */ 5631 @Deprecated 5632 public List<Encounter> getEncounterTarget() { 5633 if (this.encounterTarget == null) 5634 this.encounterTarget = new ArrayList<Encounter>(); 5635 return this.encounterTarget; 5636 } 5637 5638 /** 5639 * @deprecated Use Reference#setResource(IBaseResource) instead 5640 */ 5641 @Deprecated 5642 public Encounter addEncounterTarget() { 5643 Encounter r = new Encounter(); 5644 if (this.encounterTarget == null) 5645 this.encounterTarget = new ArrayList<Encounter>(); 5646 this.encounterTarget.add(r); 5647 return r; 5648 } 5649 5650 /** 5651 * @return {@link #detail} (A claim detail line. Either a simple (a product or 5652 * service) or a 'group' of sub-details which are simple items.) 5653 */ 5654 public List<DetailComponent> getDetail() { 5655 if (this.detail == null) 5656 this.detail = new ArrayList<DetailComponent>(); 5657 return this.detail; 5658 } 5659 5660 /** 5661 * @return Returns a reference to <code>this</code> for easy method chaining 5662 */ 5663 public ItemComponent setDetail(List<DetailComponent> theDetail) { 5664 this.detail = theDetail; 5665 return this; 5666 } 5667 5668 public boolean hasDetail() { 5669 if (this.detail == null) 5670 return false; 5671 for (DetailComponent item : this.detail) 5672 if (!item.isEmpty()) 5673 return true; 5674 return false; 5675 } 5676 5677 public DetailComponent addDetail() { // 3 5678 DetailComponent t = new DetailComponent(); 5679 if (this.detail == null) 5680 this.detail = new ArrayList<DetailComponent>(); 5681 this.detail.add(t); 5682 return t; 5683 } 5684 5685 public ItemComponent addDetail(DetailComponent t) { // 3 5686 if (t == null) 5687 return this; 5688 if (this.detail == null) 5689 this.detail = new ArrayList<DetailComponent>(); 5690 this.detail.add(t); 5691 return this; 5692 } 5693 5694 /** 5695 * @return The first repetition of repeating field {@link #detail}, creating it 5696 * if it does not already exist 5697 */ 5698 public DetailComponent getDetailFirstRep() { 5699 if (getDetail().isEmpty()) { 5700 addDetail(); 5701 } 5702 return getDetail().get(0); 5703 } 5704 5705 protected void listChildren(List<Property> children) { 5706 super.listChildren(children); 5707 children 5708 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 5709 children.add(new Property("careTeamSequence", "positiveInt", 5710 "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 5711 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnosis applicable for this service or product.", 5712 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 5713 children.add(new Property("procedureSequence", "positiveInt", 5714 "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 5715 children.add(new Property("informationSequence", "positiveInt", 5716 "Exceptions, special conditions and supporting information applicable for this service or product.", 0, 5717 java.lang.Integer.MAX_VALUE, informationSequence)); 5718 children.add(new Property("revenue", "CodeableConcept", 5719 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 5720 children.add(new Property("category", "CodeableConcept", 5721 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 5722 category)); 5723 children.add(new Property("productOrService", "CodeableConcept", 5724 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5725 0, 1, productOrService)); 5726 children.add(new Property("modifier", "CodeableConcept", 5727 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5728 java.lang.Integer.MAX_VALUE, modifier)); 5729 children.add(new Property("programCode", "CodeableConcept", 5730 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5731 children.add(new Property("serviced[x]", "date|Period", 5732 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 5733 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5734 "Where the product or service was provided.", 0, 1, location)); 5735 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 5736 1, quantity)); 5737 children.add(new Property("unitPrice", "Money", 5738 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5739 0, 1, unitPrice)); 5740 children.add(new Property("factor", "decimal", 5741 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5742 0, 1, factor)); 5743 children.add(new Property("net", "Money", 5744 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 5745 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 5746 0, java.lang.Integer.MAX_VALUE, udi)); 5747 children.add(new Property("bodySite", "CodeableConcept", 5748 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite)); 5749 children.add(new Property("subSite", "CodeableConcept", 5750 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, 5751 subSite)); 5752 children.add(new Property("encounter", "Reference(Encounter)", 5753 "The Encounters during which this Claim was created or to which the creation of this record is tightly associated.", 5754 0, java.lang.Integer.MAX_VALUE, encounter)); 5755 children.add(new Property("detail", "", 5756 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 5757 0, java.lang.Integer.MAX_VALUE, detail)); 5758 } 5759 5760 @Override 5761 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5762 switch (_hash) { 5763 case 1349547969: 5764 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 5765 1, sequence); 5766 case 1070083823: 5767 /* careTeamSequence */ return new Property("careTeamSequence", "positiveInt", 5768 "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 5769 case -909769262: 5770 /* diagnosisSequence */ return new Property("diagnosisSequence", "positiveInt", 5771 "Diagnosis applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 5772 case -808920140: 5773 /* procedureSequence */ return new Property("procedureSequence", "positiveInt", 5774 "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 5775 case -702585587: 5776 /* informationSequence */ return new Property("informationSequence", "positiveInt", 5777 "Exceptions, special conditions and supporting information applicable for this service or product.", 0, 5778 java.lang.Integer.MAX_VALUE, informationSequence); 5779 case 1099842588: 5780 /* revenue */ return new Property("revenue", "CodeableConcept", 5781 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 5782 case 50511102: 5783 /* category */ return new Property("category", "CodeableConcept", 5784 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 5785 category); 5786 case 1957227299: 5787 /* productOrService */ return new Property("productOrService", "CodeableConcept", 5788 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5789 0, 1, productOrService); 5790 case -615513385: 5791 /* modifier */ return new Property("modifier", "CodeableConcept", 5792 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5793 java.lang.Integer.MAX_VALUE, modifier); 5794 case 1010065041: 5795 /* programCode */ return new Property("programCode", "CodeableConcept", 5796 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 5797 case -1927922223: 5798 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 5799 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5800 case 1379209295: 5801 /* serviced */ return new Property("serviced[x]", "date|Period", 5802 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5803 case 363246749: 5804 /* servicedDate */ return new Property("serviced[x]", "date|Period", 5805 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5806 case 1534966512: 5807 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 5808 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5809 case 552316075: 5810 /* location[x] */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5811 "Where the product or service was provided.", 0, 1, location); 5812 case 1901043637: 5813 /* location */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5814 "Where the product or service was provided.", 0, 1, location); 5815 case -1224800468: 5816 /* locationCodeableConcept */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5817 "Where the product or service was provided.", 0, 1, location); 5818 case -1280020865: 5819 /* locationAddress */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5820 "Where the product or service was provided.", 0, 1, location); 5821 case 755866390: 5822 /* locationReference */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5823 "Where the product or service was provided.", 0, 1, location); 5824 case -1285004149: 5825 /* quantity */ return new Property("quantity", "SimpleQuantity", 5826 "The number of repetitions of a service or product.", 0, 1, quantity); 5827 case -486196699: 5828 /* unitPrice */ return new Property("unitPrice", "Money", 5829 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5830 0, 1, unitPrice); 5831 case -1282148017: 5832 /* factor */ return new Property("factor", "decimal", 5833 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5834 0, 1, factor); 5835 case 108957: 5836 /* net */ return new Property("net", "Money", 5837 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 5838 case 115642: 5839 /* udi */ return new Property("udi", "Reference(Device)", 5840 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5841 case 1702620169: 5842 /* bodySite */ return new Property("bodySite", "CodeableConcept", 5843 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite); 5844 case -1868566105: 5845 /* subSite */ return new Property("subSite", "CodeableConcept", 5846 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, 5847 java.lang.Integer.MAX_VALUE, subSite); 5848 case 1524132147: 5849 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5850 "The Encounters during which this Claim was created or to which the creation of this record is tightly associated.", 5851 0, java.lang.Integer.MAX_VALUE, encounter); 5852 case -1335224239: 5853 /* detail */ return new Property("detail", "", 5854 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 5855 0, java.lang.Integer.MAX_VALUE, detail); 5856 default: 5857 return super.getNamedProperty(_hash, _name, _checkValid); 5858 } 5859 5860 } 5861 5862 @Override 5863 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5864 switch (hash) { 5865 case 1349547969: 5866 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 5867 case 1070083823: 5868 /* careTeamSequence */ return this.careTeamSequence == null ? new Base[0] 5869 : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 5870 case -909769262: 5871 /* diagnosisSequence */ return this.diagnosisSequence == null ? new Base[0] 5872 : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 5873 case -808920140: 5874 /* procedureSequence */ return this.procedureSequence == null ? new Base[0] 5875 : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 5876 case -702585587: 5877 /* informationSequence */ return this.informationSequence == null ? new Base[0] 5878 : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 5879 case 1099842588: 5880 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 5881 case 50511102: 5882 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 5883 case 1957227299: 5884 /* productOrService */ return this.productOrService == null ? new Base[0] 5885 : new Base[] { this.productOrService }; // CodeableConcept 5886 case -615513385: 5887 /* modifier */ return this.modifier == null ? new Base[0] 5888 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5889 case 1010065041: 5890 /* programCode */ return this.programCode == null ? new Base[0] 5891 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5892 case 1379209295: 5893 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 5894 case 1901043637: 5895 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 5896 case -1285004149: 5897 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5898 case -486196699: 5899 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 5900 case -1282148017: 5901 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 5902 case 108957: 5903 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 5904 case 115642: 5905 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5906 case 1702620169: 5907 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 5908 case -1868566105: 5909 /* subSite */ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5910 case 1524132147: 5911 /* encounter */ return this.encounter == null ? new Base[0] 5912 : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 5913 case -1335224239: 5914 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 5915 default: 5916 return super.getProperty(hash, name, checkValid); 5917 } 5918 5919 } 5920 5921 @Override 5922 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5923 switch (hash) { 5924 case 1349547969: // sequence 5925 this.sequence = castToPositiveInt(value); // PositiveIntType 5926 return value; 5927 case 1070083823: // careTeamSequence 5928 this.getCareTeamSequence().add(castToPositiveInt(value)); // PositiveIntType 5929 return value; 5930 case -909769262: // diagnosisSequence 5931 this.getDiagnosisSequence().add(castToPositiveInt(value)); // PositiveIntType 5932 return value; 5933 case -808920140: // procedureSequence 5934 this.getProcedureSequence().add(castToPositiveInt(value)); // PositiveIntType 5935 return value; 5936 case -702585587: // informationSequence 5937 this.getInformationSequence().add(castToPositiveInt(value)); // PositiveIntType 5938 return value; 5939 case 1099842588: // revenue 5940 this.revenue = castToCodeableConcept(value); // CodeableConcept 5941 return value; 5942 case 50511102: // category 5943 this.category = castToCodeableConcept(value); // CodeableConcept 5944 return value; 5945 case 1957227299: // productOrService 5946 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5947 return value; 5948 case -615513385: // modifier 5949 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5950 return value; 5951 case 1010065041: // programCode 5952 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5953 return value; 5954 case 1379209295: // serviced 5955 this.serviced = castToType(value); // Type 5956 return value; 5957 case 1901043637: // location 5958 this.location = castToType(value); // Type 5959 return value; 5960 case -1285004149: // quantity 5961 this.quantity = castToQuantity(value); // Quantity 5962 return value; 5963 case -486196699: // unitPrice 5964 this.unitPrice = castToMoney(value); // Money 5965 return value; 5966 case -1282148017: // factor 5967 this.factor = castToDecimal(value); // DecimalType 5968 return value; 5969 case 108957: // net 5970 this.net = castToMoney(value); // Money 5971 return value; 5972 case 115642: // udi 5973 this.getUdi().add(castToReference(value)); // Reference 5974 return value; 5975 case 1702620169: // bodySite 5976 this.bodySite = castToCodeableConcept(value); // CodeableConcept 5977 return value; 5978 case -1868566105: // subSite 5979 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 5980 return value; 5981 case 1524132147: // encounter 5982 this.getEncounter().add(castToReference(value)); // Reference 5983 return value; 5984 case -1335224239: // detail 5985 this.getDetail().add((DetailComponent) value); // DetailComponent 5986 return value; 5987 default: 5988 return super.setProperty(hash, name, value); 5989 } 5990 5991 } 5992 5993 @Override 5994 public Base setProperty(String name, Base value) throws FHIRException { 5995 if (name.equals("sequence")) { 5996 this.sequence = castToPositiveInt(value); // PositiveIntType 5997 } else if (name.equals("careTeamSequence")) { 5998 this.getCareTeamSequence().add(castToPositiveInt(value)); 5999 } else if (name.equals("diagnosisSequence")) { 6000 this.getDiagnosisSequence().add(castToPositiveInt(value)); 6001 } else if (name.equals("procedureSequence")) { 6002 this.getProcedureSequence().add(castToPositiveInt(value)); 6003 } else if (name.equals("informationSequence")) { 6004 this.getInformationSequence().add(castToPositiveInt(value)); 6005 } else if (name.equals("revenue")) { 6006 this.revenue = castToCodeableConcept(value); // CodeableConcept 6007 } else if (name.equals("category")) { 6008 this.category = castToCodeableConcept(value); // CodeableConcept 6009 } else if (name.equals("productOrService")) { 6010 this.productOrService = castToCodeableConcept(value); // CodeableConcept 6011 } else if (name.equals("modifier")) { 6012 this.getModifier().add(castToCodeableConcept(value)); 6013 } else if (name.equals("programCode")) { 6014 this.getProgramCode().add(castToCodeableConcept(value)); 6015 } else if (name.equals("serviced[x]")) { 6016 this.serviced = castToType(value); // Type 6017 } else if (name.equals("location[x]")) { 6018 this.location = castToType(value); // Type 6019 } else if (name.equals("quantity")) { 6020 this.quantity = castToQuantity(value); // Quantity 6021 } else if (name.equals("unitPrice")) { 6022 this.unitPrice = castToMoney(value); // Money 6023 } else if (name.equals("factor")) { 6024 this.factor = castToDecimal(value); // DecimalType 6025 } else if (name.equals("net")) { 6026 this.net = castToMoney(value); // Money 6027 } else if (name.equals("udi")) { 6028 this.getUdi().add(castToReference(value)); 6029 } else if (name.equals("bodySite")) { 6030 this.bodySite = castToCodeableConcept(value); // CodeableConcept 6031 } else if (name.equals("subSite")) { 6032 this.getSubSite().add(castToCodeableConcept(value)); 6033 } else if (name.equals("encounter")) { 6034 this.getEncounter().add(castToReference(value)); 6035 } else if (name.equals("detail")) { 6036 this.getDetail().add((DetailComponent) value); 6037 } else 6038 return super.setProperty(name, value); 6039 return value; 6040 } 6041 6042 @Override 6043 public void removeChild(String name, Base value) throws FHIRException { 6044 if (name.equals("sequence")) { 6045 this.sequence = null; 6046 } else if (name.equals("careTeamSequence")) { 6047 this.getCareTeamSequence().remove(castToPositiveInt(value)); 6048 } else if (name.equals("diagnosisSequence")) { 6049 this.getDiagnosisSequence().remove(castToPositiveInt(value)); 6050 } else if (name.equals("procedureSequence")) { 6051 this.getProcedureSequence().remove(castToPositiveInt(value)); 6052 } else if (name.equals("informationSequence")) { 6053 this.getInformationSequence().remove(castToPositiveInt(value)); 6054 } else if (name.equals("revenue")) { 6055 this.revenue = null; 6056 } else if (name.equals("category")) { 6057 this.category = null; 6058 } else if (name.equals("productOrService")) { 6059 this.productOrService = null; 6060 } else if (name.equals("modifier")) { 6061 this.getModifier().remove(castToCodeableConcept(value)); 6062 } else if (name.equals("programCode")) { 6063 this.getProgramCode().remove(castToCodeableConcept(value)); 6064 } else if (name.equals("serviced[x]")) { 6065 this.serviced = null; 6066 } else if (name.equals("location[x]")) { 6067 this.location = null; 6068 } else if (name.equals("quantity")) { 6069 this.quantity = null; 6070 } else if (name.equals("unitPrice")) { 6071 this.unitPrice = null; 6072 } else if (name.equals("factor")) { 6073 this.factor = null; 6074 } else if (name.equals("net")) { 6075 this.net = null; 6076 } else if (name.equals("udi")) { 6077 this.getUdi().remove(castToReference(value)); 6078 } else if (name.equals("bodySite")) { 6079 this.bodySite = null; 6080 } else if (name.equals("subSite")) { 6081 this.getSubSite().remove(castToCodeableConcept(value)); 6082 } else if (name.equals("encounter")) { 6083 this.getEncounter().remove(castToReference(value)); 6084 } else if (name.equals("detail")) { 6085 this.getDetail().remove((DetailComponent) value); 6086 } else 6087 super.removeChild(name, value); 6088 6089 } 6090 6091 @Override 6092 public Base makeProperty(int hash, String name) throws FHIRException { 6093 switch (hash) { 6094 case 1349547969: 6095 return getSequenceElement(); 6096 case 1070083823: 6097 return addCareTeamSequenceElement(); 6098 case -909769262: 6099 return addDiagnosisSequenceElement(); 6100 case -808920140: 6101 return addProcedureSequenceElement(); 6102 case -702585587: 6103 return addInformationSequenceElement(); 6104 case 1099842588: 6105 return getRevenue(); 6106 case 50511102: 6107 return getCategory(); 6108 case 1957227299: 6109 return getProductOrService(); 6110 case -615513385: 6111 return addModifier(); 6112 case 1010065041: 6113 return addProgramCode(); 6114 case -1927922223: 6115 return getServiced(); 6116 case 1379209295: 6117 return getServiced(); 6118 case 552316075: 6119 return getLocation(); 6120 case 1901043637: 6121 return getLocation(); 6122 case -1285004149: 6123 return getQuantity(); 6124 case -486196699: 6125 return getUnitPrice(); 6126 case -1282148017: 6127 return getFactorElement(); 6128 case 108957: 6129 return getNet(); 6130 case 115642: 6131 return addUdi(); 6132 case 1702620169: 6133 return getBodySite(); 6134 case -1868566105: 6135 return addSubSite(); 6136 case 1524132147: 6137 return addEncounter(); 6138 case -1335224239: 6139 return addDetail(); 6140 default: 6141 return super.makeProperty(hash, name); 6142 } 6143 6144 } 6145 6146 @Override 6147 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6148 switch (hash) { 6149 case 1349547969: 6150 /* sequence */ return new String[] { "positiveInt" }; 6151 case 1070083823: 6152 /* careTeamSequence */ return new String[] { "positiveInt" }; 6153 case -909769262: 6154 /* diagnosisSequence */ return new String[] { "positiveInt" }; 6155 case -808920140: 6156 /* procedureSequence */ return new String[] { "positiveInt" }; 6157 case -702585587: 6158 /* informationSequence */ return new String[] { "positiveInt" }; 6159 case 1099842588: 6160 /* revenue */ return new String[] { "CodeableConcept" }; 6161 case 50511102: 6162 /* category */ return new String[] { "CodeableConcept" }; 6163 case 1957227299: 6164 /* productOrService */ return new String[] { "CodeableConcept" }; 6165 case -615513385: 6166 /* modifier */ return new String[] { "CodeableConcept" }; 6167 case 1010065041: 6168 /* programCode */ return new String[] { "CodeableConcept" }; 6169 case 1379209295: 6170 /* serviced */ return new String[] { "date", "Period" }; 6171 case 1901043637: 6172 /* location */ return new String[] { "CodeableConcept", "Address", "Reference" }; 6173 case -1285004149: 6174 /* quantity */ return new String[] { "SimpleQuantity" }; 6175 case -486196699: 6176 /* unitPrice */ return new String[] { "Money" }; 6177 case -1282148017: 6178 /* factor */ return new String[] { "decimal" }; 6179 case 108957: 6180 /* net */ return new String[] { "Money" }; 6181 case 115642: 6182 /* udi */ return new String[] { "Reference" }; 6183 case 1702620169: 6184 /* bodySite */ return new String[] { "CodeableConcept" }; 6185 case -1868566105: 6186 /* subSite */ return new String[] { "CodeableConcept" }; 6187 case 1524132147: 6188 /* encounter */ return new String[] { "Reference" }; 6189 case -1335224239: 6190 /* detail */ return new String[] {}; 6191 default: 6192 return super.getTypesForProperty(hash, name); 6193 } 6194 6195 } 6196 6197 @Override 6198 public Base addChild(String name) throws FHIRException { 6199 if (name.equals("sequence")) { 6200 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 6201 } else if (name.equals("careTeamSequence")) { 6202 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeamSequence"); 6203 } else if (name.equals("diagnosisSequence")) { 6204 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosisSequence"); 6205 } else if (name.equals("procedureSequence")) { 6206 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedureSequence"); 6207 } else if (name.equals("informationSequence")) { 6208 throw new FHIRException("Cannot call addChild on a singleton property Claim.informationSequence"); 6209 } else if (name.equals("revenue")) { 6210 this.revenue = new CodeableConcept(); 6211 return this.revenue; 6212 } else if (name.equals("category")) { 6213 this.category = new CodeableConcept(); 6214 return this.category; 6215 } else if (name.equals("productOrService")) { 6216 this.productOrService = new CodeableConcept(); 6217 return this.productOrService; 6218 } else if (name.equals("modifier")) { 6219 return addModifier(); 6220 } else if (name.equals("programCode")) { 6221 return addProgramCode(); 6222 } else if (name.equals("servicedDate")) { 6223 this.serviced = new DateType(); 6224 return this.serviced; 6225 } else if (name.equals("servicedPeriod")) { 6226 this.serviced = new Period(); 6227 return this.serviced; 6228 } else if (name.equals("locationCodeableConcept")) { 6229 this.location = new CodeableConcept(); 6230 return this.location; 6231 } else if (name.equals("locationAddress")) { 6232 this.location = new Address(); 6233 return this.location; 6234 } else if (name.equals("locationReference")) { 6235 this.location = new Reference(); 6236 return this.location; 6237 } else if (name.equals("quantity")) { 6238 this.quantity = new Quantity(); 6239 return this.quantity; 6240 } else if (name.equals("unitPrice")) { 6241 this.unitPrice = new Money(); 6242 return this.unitPrice; 6243 } else if (name.equals("factor")) { 6244 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 6245 } else if (name.equals("net")) { 6246 this.net = new Money(); 6247 return this.net; 6248 } else if (name.equals("udi")) { 6249 return addUdi(); 6250 } else if (name.equals("bodySite")) { 6251 this.bodySite = new CodeableConcept(); 6252 return this.bodySite; 6253 } else if (name.equals("subSite")) { 6254 return addSubSite(); 6255 } else if (name.equals("encounter")) { 6256 return addEncounter(); 6257 } else if (name.equals("detail")) { 6258 return addDetail(); 6259 } else 6260 return super.addChild(name); 6261 } 6262 6263 public ItemComponent copy() { 6264 ItemComponent dst = new ItemComponent(); 6265 copyValues(dst); 6266 return dst; 6267 } 6268 6269 public void copyValues(ItemComponent dst) { 6270 super.copyValues(dst); 6271 dst.sequence = sequence == null ? null : sequence.copy(); 6272 if (careTeamSequence != null) { 6273 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 6274 for (PositiveIntType i : careTeamSequence) 6275 dst.careTeamSequence.add(i.copy()); 6276 } 6277 ; 6278 if (diagnosisSequence != null) { 6279 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 6280 for (PositiveIntType i : diagnosisSequence) 6281 dst.diagnosisSequence.add(i.copy()); 6282 } 6283 ; 6284 if (procedureSequence != null) { 6285 dst.procedureSequence = new ArrayList<PositiveIntType>(); 6286 for (PositiveIntType i : procedureSequence) 6287 dst.procedureSequence.add(i.copy()); 6288 } 6289 ; 6290 if (informationSequence != null) { 6291 dst.informationSequence = new ArrayList<PositiveIntType>(); 6292 for (PositiveIntType i : informationSequence) 6293 dst.informationSequence.add(i.copy()); 6294 } 6295 ; 6296 dst.revenue = revenue == null ? null : revenue.copy(); 6297 dst.category = category == null ? null : category.copy(); 6298 dst.productOrService = productOrService == null ? null : productOrService.copy(); 6299 if (modifier != null) { 6300 dst.modifier = new ArrayList<CodeableConcept>(); 6301 for (CodeableConcept i : modifier) 6302 dst.modifier.add(i.copy()); 6303 } 6304 ; 6305 if (programCode != null) { 6306 dst.programCode = new ArrayList<CodeableConcept>(); 6307 for (CodeableConcept i : programCode) 6308 dst.programCode.add(i.copy()); 6309 } 6310 ; 6311 dst.serviced = serviced == null ? null : serviced.copy(); 6312 dst.location = location == null ? null : location.copy(); 6313 dst.quantity = quantity == null ? null : quantity.copy(); 6314 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6315 dst.factor = factor == null ? null : factor.copy(); 6316 dst.net = net == null ? null : net.copy(); 6317 if (udi != null) { 6318 dst.udi = new ArrayList<Reference>(); 6319 for (Reference i : udi) 6320 dst.udi.add(i.copy()); 6321 } 6322 ; 6323 dst.bodySite = bodySite == null ? null : bodySite.copy(); 6324 if (subSite != null) { 6325 dst.subSite = new ArrayList<CodeableConcept>(); 6326 for (CodeableConcept i : subSite) 6327 dst.subSite.add(i.copy()); 6328 } 6329 ; 6330 if (encounter != null) { 6331 dst.encounter = new ArrayList<Reference>(); 6332 for (Reference i : encounter) 6333 dst.encounter.add(i.copy()); 6334 } 6335 ; 6336 if (detail != null) { 6337 dst.detail = new ArrayList<DetailComponent>(); 6338 for (DetailComponent i : detail) 6339 dst.detail.add(i.copy()); 6340 } 6341 ; 6342 } 6343 6344 @Override 6345 public boolean equalsDeep(Base other_) { 6346 if (!super.equalsDeep(other_)) 6347 return false; 6348 if (!(other_ instanceof ItemComponent)) 6349 return false; 6350 ItemComponent o = (ItemComponent) other_; 6351 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamSequence, o.careTeamSequence, true) 6352 && compareDeep(diagnosisSequence, o.diagnosisSequence, true) 6353 && compareDeep(procedureSequence, o.procedureSequence, true) 6354 && compareDeep(informationSequence, o.informationSequence, true) && compareDeep(revenue, o.revenue, true) 6355 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 6356 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 6357 && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 6358 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 6359 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6360 && compareDeep(bodySite, o.bodySite, true) && compareDeep(subSite, o.subSite, true) 6361 && compareDeep(encounter, o.encounter, true) && compareDeep(detail, o.detail, true); 6362 } 6363 6364 @Override 6365 public boolean equalsShallow(Base other_) { 6366 if (!super.equalsShallow(other_)) 6367 return false; 6368 if (!(other_ instanceof ItemComponent)) 6369 return false; 6370 ItemComponent o = (ItemComponent) other_; 6371 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 6372 && compareValues(diagnosisSequence, o.diagnosisSequence, true) 6373 && compareValues(procedureSequence, o.procedureSequence, true) 6374 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true); 6375 } 6376 6377 public boolean isEmpty() { 6378 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamSequence, diagnosisSequence, 6379 procedureSequence, informationSequence, revenue, category, productOrService, modifier, programCode, serviced, 6380 location, quantity, unitPrice, factor, net, udi, bodySite, subSite, encounter, detail); 6381 } 6382 6383 public String fhirType() { 6384 return "Claim.item"; 6385 6386 } 6387 6388 } 6389 6390 @Block() 6391 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 6392 /** 6393 * A number to uniquely identify item entries. 6394 */ 6395 @Child(name = "sequence", type = { 6396 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6397 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 6398 protected PositiveIntType sequence; 6399 6400 /** 6401 * The type of revenue or cost center providing the product and/or service. 6402 */ 6403 @Child(name = "revenue", type = { 6404 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6405 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 6406 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 6407 protected CodeableConcept revenue; 6408 6409 /** 6410 * Code to identify the general type of benefits under which products and 6411 * services are provided. 6412 */ 6413 @Child(name = "category", type = { 6414 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6415 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 6416 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6417 protected CodeableConcept category; 6418 6419 /** 6420 * When the value is a group code then this item collects a set of related claim 6421 * details, otherwise this contains the product, service, drug or other billing 6422 * code for the item. 6423 */ 6424 @Child(name = "productOrService", type = { 6425 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 6426 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 6427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 6428 protected CodeableConcept productOrService; 6429 6430 /** 6431 * Item typification or modifiers codes to convey additional context for the 6432 * product or service. 6433 */ 6434 @Child(name = "modifier", type = { 6435 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6436 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 6437 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 6438 protected List<CodeableConcept> modifier; 6439 6440 /** 6441 * Identifies the program under which this may be recovered. 6442 */ 6443 @Child(name = "programCode", type = { 6444 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6445 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 6446 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 6447 protected List<CodeableConcept> programCode; 6448 6449 /** 6450 * The number of repetitions of a service or product. 6451 */ 6452 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6453 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 6454 protected Quantity quantity; 6455 6456 /** 6457 * If the item is not a group then this is the fee for the product or service, 6458 * otherwise this is the total of the fees for the details of the group. 6459 */ 6460 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6461 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 6462 protected Money unitPrice; 6463 6464 /** 6465 * A real number that represents a multiplier used in determining the overall 6466 * value of services delivered and/or goods received. The concept of a Factor 6467 * allows for a discount or surcharge multiplier to be applied to a monetary 6468 * amount. 6469 */ 6470 @Child(name = "factor", type = { 6471 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6472 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 6473 protected DecimalType factor; 6474 6475 /** 6476 * The quantity times the unit price for an additional service or product or 6477 * charge. 6478 */ 6479 @Child(name = "net", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6480 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 6481 protected Money net; 6482 6483 /** 6484 * Unique Device Identifiers associated with this line item. 6485 */ 6486 @Child(name = "udi", type = { 6487 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6488 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 6489 protected List<Reference> udi; 6490 /** 6491 * The actual objects that are the target of the reference (Unique Device 6492 * Identifiers associated with this line item.) 6493 */ 6494 protected List<Device> udiTarget; 6495 6496 /** 6497 * A claim detail line. Either a simple (a product or service) or a 'group' of 6498 * sub-details which are simple items. 6499 */ 6500 @Child(name = "subDetail", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6501 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 6502 protected List<SubDetailComponent> subDetail; 6503 6504 private static final long serialVersionUID = -1245004924L; 6505 6506 /** 6507 * Constructor 6508 */ 6509 public DetailComponent() { 6510 super(); 6511 } 6512 6513 /** 6514 * Constructor 6515 */ 6516 public DetailComponent(PositiveIntType sequence, CodeableConcept productOrService) { 6517 super(); 6518 this.sequence = sequence; 6519 this.productOrService = productOrService; 6520 } 6521 6522 /** 6523 * @return {@link #sequence} (A number to uniquely identify item entries.). This 6524 * is the underlying object with id, value and extensions. The accessor 6525 * "getSequence" gives direct access to the value 6526 */ 6527 public PositiveIntType getSequenceElement() { 6528 if (this.sequence == null) 6529 if (Configuration.errorOnAutoCreate()) 6530 throw new Error("Attempt to auto-create DetailComponent.sequence"); 6531 else if (Configuration.doAutoCreate()) 6532 this.sequence = new PositiveIntType(); // bb 6533 return this.sequence; 6534 } 6535 6536 public boolean hasSequenceElement() { 6537 return this.sequence != null && !this.sequence.isEmpty(); 6538 } 6539 6540 public boolean hasSequence() { 6541 return this.sequence != null && !this.sequence.isEmpty(); 6542 } 6543 6544 /** 6545 * @param value {@link #sequence} (A number to uniquely identify item entries.). 6546 * This is the underlying object with id, value and extensions. The 6547 * accessor "getSequence" gives direct access to the value 6548 */ 6549 public DetailComponent setSequenceElement(PositiveIntType value) { 6550 this.sequence = value; 6551 return this; 6552 } 6553 6554 /** 6555 * @return A number to uniquely identify item entries. 6556 */ 6557 public int getSequence() { 6558 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6559 } 6560 6561 /** 6562 * @param value A number to uniquely identify item entries. 6563 */ 6564 public DetailComponent setSequence(int value) { 6565 if (this.sequence == null) 6566 this.sequence = new PositiveIntType(); 6567 this.sequence.setValue(value); 6568 return this; 6569 } 6570 6571 /** 6572 * @return {@link #revenue} (The type of revenue or cost center providing the 6573 * product and/or service.) 6574 */ 6575 public CodeableConcept getRevenue() { 6576 if (this.revenue == null) 6577 if (Configuration.errorOnAutoCreate()) 6578 throw new Error("Attempt to auto-create DetailComponent.revenue"); 6579 else if (Configuration.doAutoCreate()) 6580 this.revenue = new CodeableConcept(); // cc 6581 return this.revenue; 6582 } 6583 6584 public boolean hasRevenue() { 6585 return this.revenue != null && !this.revenue.isEmpty(); 6586 } 6587 6588 /** 6589 * @param value {@link #revenue} (The type of revenue or cost center providing 6590 * the product and/or service.) 6591 */ 6592 public DetailComponent setRevenue(CodeableConcept value) { 6593 this.revenue = value; 6594 return this; 6595 } 6596 6597 /** 6598 * @return {@link #category} (Code to identify the general type of benefits 6599 * under which products and services are provided.) 6600 */ 6601 public CodeableConcept getCategory() { 6602 if (this.category == null) 6603 if (Configuration.errorOnAutoCreate()) 6604 throw new Error("Attempt to auto-create DetailComponent.category"); 6605 else if (Configuration.doAutoCreate()) 6606 this.category = new CodeableConcept(); // cc 6607 return this.category; 6608 } 6609 6610 public boolean hasCategory() { 6611 return this.category != null && !this.category.isEmpty(); 6612 } 6613 6614 /** 6615 * @param value {@link #category} (Code to identify the general type of benefits 6616 * under which products and services are provided.) 6617 */ 6618 public DetailComponent setCategory(CodeableConcept value) { 6619 this.category = value; 6620 return this; 6621 } 6622 6623 /** 6624 * @return {@link #productOrService} (When the value is a group code then this 6625 * item collects a set of related claim details, otherwise this contains 6626 * the product, service, drug or other billing code for the item.) 6627 */ 6628 public CodeableConcept getProductOrService() { 6629 if (this.productOrService == null) 6630 if (Configuration.errorOnAutoCreate()) 6631 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 6632 else if (Configuration.doAutoCreate()) 6633 this.productOrService = new CodeableConcept(); // cc 6634 return this.productOrService; 6635 } 6636 6637 public boolean hasProductOrService() { 6638 return this.productOrService != null && !this.productOrService.isEmpty(); 6639 } 6640 6641 /** 6642 * @param value {@link #productOrService} (When the value is a group code then 6643 * this item collects a set of related claim details, otherwise 6644 * this contains the product, service, drug or other billing code 6645 * for the item.) 6646 */ 6647 public DetailComponent setProductOrService(CodeableConcept value) { 6648 this.productOrService = value; 6649 return this; 6650 } 6651 6652 /** 6653 * @return {@link #modifier} (Item typification or modifiers codes to convey 6654 * additional context for the product or service.) 6655 */ 6656 public List<CodeableConcept> getModifier() { 6657 if (this.modifier == null) 6658 this.modifier = new ArrayList<CodeableConcept>(); 6659 return this.modifier; 6660 } 6661 6662 /** 6663 * @return Returns a reference to <code>this</code> for easy method chaining 6664 */ 6665 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 6666 this.modifier = theModifier; 6667 return this; 6668 } 6669 6670 public boolean hasModifier() { 6671 if (this.modifier == null) 6672 return false; 6673 for (CodeableConcept item : this.modifier) 6674 if (!item.isEmpty()) 6675 return true; 6676 return false; 6677 } 6678 6679 public CodeableConcept addModifier() { // 3 6680 CodeableConcept t = new CodeableConcept(); 6681 if (this.modifier == null) 6682 this.modifier = new ArrayList<CodeableConcept>(); 6683 this.modifier.add(t); 6684 return t; 6685 } 6686 6687 public DetailComponent addModifier(CodeableConcept t) { // 3 6688 if (t == null) 6689 return this; 6690 if (this.modifier == null) 6691 this.modifier = new ArrayList<CodeableConcept>(); 6692 this.modifier.add(t); 6693 return this; 6694 } 6695 6696 /** 6697 * @return The first repetition of repeating field {@link #modifier}, creating 6698 * it if it does not already exist 6699 */ 6700 public CodeableConcept getModifierFirstRep() { 6701 if (getModifier().isEmpty()) { 6702 addModifier(); 6703 } 6704 return getModifier().get(0); 6705 } 6706 6707 /** 6708 * @return {@link #programCode} (Identifies the program under which this may be 6709 * recovered.) 6710 */ 6711 public List<CodeableConcept> getProgramCode() { 6712 if (this.programCode == null) 6713 this.programCode = new ArrayList<CodeableConcept>(); 6714 return this.programCode; 6715 } 6716 6717 /** 6718 * @return Returns a reference to <code>this</code> for easy method chaining 6719 */ 6720 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6721 this.programCode = theProgramCode; 6722 return this; 6723 } 6724 6725 public boolean hasProgramCode() { 6726 if (this.programCode == null) 6727 return false; 6728 for (CodeableConcept item : this.programCode) 6729 if (!item.isEmpty()) 6730 return true; 6731 return false; 6732 } 6733 6734 public CodeableConcept addProgramCode() { // 3 6735 CodeableConcept t = new CodeableConcept(); 6736 if (this.programCode == null) 6737 this.programCode = new ArrayList<CodeableConcept>(); 6738 this.programCode.add(t); 6739 return t; 6740 } 6741 6742 public DetailComponent addProgramCode(CodeableConcept t) { // 3 6743 if (t == null) 6744 return this; 6745 if (this.programCode == null) 6746 this.programCode = new ArrayList<CodeableConcept>(); 6747 this.programCode.add(t); 6748 return this; 6749 } 6750 6751 /** 6752 * @return The first repetition of repeating field {@link #programCode}, 6753 * creating it if it does not already exist 6754 */ 6755 public CodeableConcept getProgramCodeFirstRep() { 6756 if (getProgramCode().isEmpty()) { 6757 addProgramCode(); 6758 } 6759 return getProgramCode().get(0); 6760 } 6761 6762 /** 6763 * @return {@link #quantity} (The number of repetitions of a service or 6764 * product.) 6765 */ 6766 public Quantity getQuantity() { 6767 if (this.quantity == null) 6768 if (Configuration.errorOnAutoCreate()) 6769 throw new Error("Attempt to auto-create DetailComponent.quantity"); 6770 else if (Configuration.doAutoCreate()) 6771 this.quantity = new Quantity(); // cc 6772 return this.quantity; 6773 } 6774 6775 public boolean hasQuantity() { 6776 return this.quantity != null && !this.quantity.isEmpty(); 6777 } 6778 6779 /** 6780 * @param value {@link #quantity} (The number of repetitions of a service or 6781 * product.) 6782 */ 6783 public DetailComponent setQuantity(Quantity value) { 6784 this.quantity = value; 6785 return this; 6786 } 6787 6788 /** 6789 * @return {@link #unitPrice} (If the item is not a group then this is the fee 6790 * for the product or service, otherwise this is the total of the fees 6791 * for the details of the group.) 6792 */ 6793 public Money getUnitPrice() { 6794 if (this.unitPrice == null) 6795 if (Configuration.errorOnAutoCreate()) 6796 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 6797 else if (Configuration.doAutoCreate()) 6798 this.unitPrice = new Money(); // cc 6799 return this.unitPrice; 6800 } 6801 6802 public boolean hasUnitPrice() { 6803 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6804 } 6805 6806 /** 6807 * @param value {@link #unitPrice} (If the item is not a group then this is the 6808 * fee for the product or service, otherwise this is the total of 6809 * the fees for the details of the group.) 6810 */ 6811 public DetailComponent setUnitPrice(Money value) { 6812 this.unitPrice = value; 6813 return this; 6814 } 6815 6816 /** 6817 * @return {@link #factor} (A real number that represents a multiplier used in 6818 * determining the overall value of services delivered and/or goods 6819 * received. The concept of a Factor allows for a discount or surcharge 6820 * multiplier to be applied to a monetary amount.). This is the 6821 * underlying object with id, value and extensions. The accessor 6822 * "getFactor" gives direct access to the value 6823 */ 6824 public DecimalType getFactorElement() { 6825 if (this.factor == null) 6826 if (Configuration.errorOnAutoCreate()) 6827 throw new Error("Attempt to auto-create DetailComponent.factor"); 6828 else if (Configuration.doAutoCreate()) 6829 this.factor = new DecimalType(); // bb 6830 return this.factor; 6831 } 6832 6833 public boolean hasFactorElement() { 6834 return this.factor != null && !this.factor.isEmpty(); 6835 } 6836 6837 public boolean hasFactor() { 6838 return this.factor != null && !this.factor.isEmpty(); 6839 } 6840 6841 /** 6842 * @param value {@link #factor} (A real number that represents a multiplier used 6843 * in determining the overall value of services delivered and/or 6844 * goods received. The concept of a Factor allows for a discount or 6845 * surcharge multiplier to be applied to a monetary amount.). This 6846 * is the underlying object with id, value and extensions. The 6847 * accessor "getFactor" gives direct access to the value 6848 */ 6849 public DetailComponent setFactorElement(DecimalType value) { 6850 this.factor = value; 6851 return this; 6852 } 6853 6854 /** 6855 * @return A real number that represents a multiplier used in determining the 6856 * overall value of services delivered and/or goods received. The 6857 * concept of a Factor allows for a discount or surcharge multiplier to 6858 * be applied to a monetary amount. 6859 */ 6860 public BigDecimal getFactor() { 6861 return this.factor == null ? null : this.factor.getValue(); 6862 } 6863 6864 /** 6865 * @param value A real number that represents a multiplier used in determining 6866 * the overall value of services delivered and/or goods received. 6867 * The concept of a Factor allows for a discount or surcharge 6868 * multiplier to be applied to a monetary amount. 6869 */ 6870 public DetailComponent setFactor(BigDecimal value) { 6871 if (value == null) 6872 this.factor = null; 6873 else { 6874 if (this.factor == null) 6875 this.factor = new DecimalType(); 6876 this.factor.setValue(value); 6877 } 6878 return this; 6879 } 6880 6881 /** 6882 * @param value A real number that represents a multiplier used in determining 6883 * the overall value of services delivered and/or goods received. 6884 * The concept of a Factor allows for a discount or surcharge 6885 * multiplier to be applied to a monetary amount. 6886 */ 6887 public DetailComponent setFactor(long value) { 6888 this.factor = new DecimalType(); 6889 this.factor.setValue(value); 6890 return this; 6891 } 6892 6893 /** 6894 * @param value A real number that represents a multiplier used in determining 6895 * the overall value of services delivered and/or goods received. 6896 * The concept of a Factor allows for a discount or surcharge 6897 * multiplier to be applied to a monetary amount. 6898 */ 6899 public DetailComponent setFactor(double value) { 6900 this.factor = new DecimalType(); 6901 this.factor.setValue(value); 6902 return this; 6903 } 6904 6905 /** 6906 * @return {@link #net} (The quantity times the unit price for an additional 6907 * service or product or charge.) 6908 */ 6909 public Money getNet() { 6910 if (this.net == null) 6911 if (Configuration.errorOnAutoCreate()) 6912 throw new Error("Attempt to auto-create DetailComponent.net"); 6913 else if (Configuration.doAutoCreate()) 6914 this.net = new Money(); // cc 6915 return this.net; 6916 } 6917 6918 public boolean hasNet() { 6919 return this.net != null && !this.net.isEmpty(); 6920 } 6921 6922 /** 6923 * @param value {@link #net} (The quantity times the unit price for an 6924 * additional service or product or charge.) 6925 */ 6926 public DetailComponent setNet(Money value) { 6927 this.net = value; 6928 return this; 6929 } 6930 6931 /** 6932 * @return {@link #udi} (Unique Device Identifiers associated with this line 6933 * item.) 6934 */ 6935 public List<Reference> getUdi() { 6936 if (this.udi == null) 6937 this.udi = new ArrayList<Reference>(); 6938 return this.udi; 6939 } 6940 6941 /** 6942 * @return Returns a reference to <code>this</code> for easy method chaining 6943 */ 6944 public DetailComponent setUdi(List<Reference> theUdi) { 6945 this.udi = theUdi; 6946 return this; 6947 } 6948 6949 public boolean hasUdi() { 6950 if (this.udi == null) 6951 return false; 6952 for (Reference item : this.udi) 6953 if (!item.isEmpty()) 6954 return true; 6955 return false; 6956 } 6957 6958 public Reference addUdi() { // 3 6959 Reference t = new Reference(); 6960 if (this.udi == null) 6961 this.udi = new ArrayList<Reference>(); 6962 this.udi.add(t); 6963 return t; 6964 } 6965 6966 public DetailComponent addUdi(Reference t) { // 3 6967 if (t == null) 6968 return this; 6969 if (this.udi == null) 6970 this.udi = new ArrayList<Reference>(); 6971 this.udi.add(t); 6972 return this; 6973 } 6974 6975 /** 6976 * @return The first repetition of repeating field {@link #udi}, creating it if 6977 * it does not already exist 6978 */ 6979 public Reference getUdiFirstRep() { 6980 if (getUdi().isEmpty()) { 6981 addUdi(); 6982 } 6983 return getUdi().get(0); 6984 } 6985 6986 /** 6987 * @deprecated Use Reference#setResource(IBaseResource) instead 6988 */ 6989 @Deprecated 6990 public List<Device> getUdiTarget() { 6991 if (this.udiTarget == null) 6992 this.udiTarget = new ArrayList<Device>(); 6993 return this.udiTarget; 6994 } 6995 6996 /** 6997 * @deprecated Use Reference#setResource(IBaseResource) instead 6998 */ 6999 @Deprecated 7000 public Device addUdiTarget() { 7001 Device r = new Device(); 7002 if (this.udiTarget == null) 7003 this.udiTarget = new ArrayList<Device>(); 7004 this.udiTarget.add(r); 7005 return r; 7006 } 7007 7008 /** 7009 * @return {@link #subDetail} (A claim detail line. Either a simple (a product 7010 * or service) or a 'group' of sub-details which are simple items.) 7011 */ 7012 public List<SubDetailComponent> getSubDetail() { 7013 if (this.subDetail == null) 7014 this.subDetail = new ArrayList<SubDetailComponent>(); 7015 return this.subDetail; 7016 } 7017 7018 /** 7019 * @return Returns a reference to <code>this</code> for easy method chaining 7020 */ 7021 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 7022 this.subDetail = theSubDetail; 7023 return this; 7024 } 7025 7026 public boolean hasSubDetail() { 7027 if (this.subDetail == null) 7028 return false; 7029 for (SubDetailComponent item : this.subDetail) 7030 if (!item.isEmpty()) 7031 return true; 7032 return false; 7033 } 7034 7035 public SubDetailComponent addSubDetail() { // 3 7036 SubDetailComponent t = new SubDetailComponent(); 7037 if (this.subDetail == null) 7038 this.subDetail = new ArrayList<SubDetailComponent>(); 7039 this.subDetail.add(t); 7040 return t; 7041 } 7042 7043 public DetailComponent addSubDetail(SubDetailComponent t) { // 3 7044 if (t == null) 7045 return this; 7046 if (this.subDetail == null) 7047 this.subDetail = new ArrayList<SubDetailComponent>(); 7048 this.subDetail.add(t); 7049 return this; 7050 } 7051 7052 /** 7053 * @return The first repetition of repeating field {@link #subDetail}, creating 7054 * it if it does not already exist 7055 */ 7056 public SubDetailComponent getSubDetailFirstRep() { 7057 if (getSubDetail().isEmpty()) { 7058 addSubDetail(); 7059 } 7060 return getSubDetail().get(0); 7061 } 7062 7063 protected void listChildren(List<Property> children) { 7064 super.listChildren(children); 7065 children 7066 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 7067 children.add(new Property("revenue", "CodeableConcept", 7068 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7069 children.add(new Property("category", "CodeableConcept", 7070 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 7071 category)); 7072 children.add(new Property("productOrService", "CodeableConcept", 7073 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 7074 0, 1, productOrService)); 7075 children.add(new Property("modifier", "CodeableConcept", 7076 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 7077 java.lang.Integer.MAX_VALUE, modifier)); 7078 children.add(new Property("programCode", "CodeableConcept", 7079 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7080 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 7081 1, quantity)); 7082 children.add(new Property("unitPrice", "Money", 7083 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 7084 0, 1, unitPrice)); 7085 children.add(new Property("factor", "decimal", 7086 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7087 0, 1, factor)); 7088 children.add(new Property("net", "Money", 7089 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 7090 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 7091 0, java.lang.Integer.MAX_VALUE, udi)); 7092 children.add(new Property("subDetail", "", 7093 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 7094 0, java.lang.Integer.MAX_VALUE, subDetail)); 7095 } 7096 7097 @Override 7098 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7099 switch (_hash) { 7100 case 1349547969: 7101 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 7102 1, sequence); 7103 case 1099842588: 7104 /* revenue */ return new Property("revenue", "CodeableConcept", 7105 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7106 case 50511102: 7107 /* category */ return new Property("category", "CodeableConcept", 7108 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 7109 category); 7110 case 1957227299: 7111 /* productOrService */ return new Property("productOrService", "CodeableConcept", 7112 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 7113 0, 1, productOrService); 7114 case -615513385: 7115 /* modifier */ return new Property("modifier", "CodeableConcept", 7116 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 7117 java.lang.Integer.MAX_VALUE, modifier); 7118 case 1010065041: 7119 /* programCode */ return new Property("programCode", "CodeableConcept", 7120 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7121 case -1285004149: 7122 /* quantity */ return new Property("quantity", "SimpleQuantity", 7123 "The number of repetitions of a service or product.", 0, 1, quantity); 7124 case -486196699: 7125 /* unitPrice */ return new Property("unitPrice", "Money", 7126 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 7127 0, 1, unitPrice); 7128 case -1282148017: 7129 /* factor */ return new Property("factor", "decimal", 7130 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7131 0, 1, factor); 7132 case 108957: 7133 /* net */ return new Property("net", "Money", 7134 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 7135 case 115642: 7136 /* udi */ return new Property("udi", "Reference(Device)", 7137 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7138 case -828829007: 7139 /* subDetail */ return new Property("subDetail", "", 7140 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 7141 0, java.lang.Integer.MAX_VALUE, subDetail); 7142 default: 7143 return super.getNamedProperty(_hash, _name, _checkValid); 7144 } 7145 7146 } 7147 7148 @Override 7149 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7150 switch (hash) { 7151 case 1349547969: 7152 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 7153 case 1099842588: 7154 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 7155 case 50511102: 7156 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 7157 case 1957227299: 7158 /* productOrService */ return this.productOrService == null ? new Base[0] 7159 : new Base[] { this.productOrService }; // CodeableConcept 7160 case -615513385: 7161 /* modifier */ return this.modifier == null ? new Base[0] 7162 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7163 case 1010065041: 7164 /* programCode */ return this.programCode == null ? new Base[0] 7165 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7166 case -1285004149: 7167 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7168 case -486196699: 7169 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 7170 case -1282148017: 7171 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 7172 case 108957: 7173 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 7174 case 115642: 7175 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7176 case -828829007: 7177 /* subDetail */ return this.subDetail == null ? new Base[0] 7178 : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 7179 default: 7180 return super.getProperty(hash, name, checkValid); 7181 } 7182 7183 } 7184 7185 @Override 7186 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7187 switch (hash) { 7188 case 1349547969: // sequence 7189 this.sequence = castToPositiveInt(value); // PositiveIntType 7190 return value; 7191 case 1099842588: // revenue 7192 this.revenue = castToCodeableConcept(value); // CodeableConcept 7193 return value; 7194 case 50511102: // category 7195 this.category = castToCodeableConcept(value); // CodeableConcept 7196 return value; 7197 case 1957227299: // productOrService 7198 this.productOrService = castToCodeableConcept(value); // CodeableConcept 7199 return value; 7200 case -615513385: // modifier 7201 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7202 return value; 7203 case 1010065041: // programCode 7204 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 7205 return value; 7206 case -1285004149: // quantity 7207 this.quantity = castToQuantity(value); // Quantity 7208 return value; 7209 case -486196699: // unitPrice 7210 this.unitPrice = castToMoney(value); // Money 7211 return value; 7212 case -1282148017: // factor 7213 this.factor = castToDecimal(value); // DecimalType 7214 return value; 7215 case 108957: // net 7216 this.net = castToMoney(value); // Money 7217 return value; 7218 case 115642: // udi 7219 this.getUdi().add(castToReference(value)); // Reference 7220 return value; 7221 case -828829007: // subDetail 7222 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 7223 return value; 7224 default: 7225 return super.setProperty(hash, name, value); 7226 } 7227 7228 } 7229 7230 @Override 7231 public Base setProperty(String name, Base value) throws FHIRException { 7232 if (name.equals("sequence")) { 7233 this.sequence = castToPositiveInt(value); // PositiveIntType 7234 } else if (name.equals("revenue")) { 7235 this.revenue = castToCodeableConcept(value); // CodeableConcept 7236 } else if (name.equals("category")) { 7237 this.category = castToCodeableConcept(value); // CodeableConcept 7238 } else if (name.equals("productOrService")) { 7239 this.productOrService = castToCodeableConcept(value); // CodeableConcept 7240 } else if (name.equals("modifier")) { 7241 this.getModifier().add(castToCodeableConcept(value)); 7242 } else if (name.equals("programCode")) { 7243 this.getProgramCode().add(castToCodeableConcept(value)); 7244 } else if (name.equals("quantity")) { 7245 this.quantity = castToQuantity(value); // Quantity 7246 } else if (name.equals("unitPrice")) { 7247 this.unitPrice = castToMoney(value); // Money 7248 } else if (name.equals("factor")) { 7249 this.factor = castToDecimal(value); // DecimalType 7250 } else if (name.equals("net")) { 7251 this.net = castToMoney(value); // Money 7252 } else if (name.equals("udi")) { 7253 this.getUdi().add(castToReference(value)); 7254 } else if (name.equals("subDetail")) { 7255 this.getSubDetail().add((SubDetailComponent) value); 7256 } else 7257 return super.setProperty(name, value); 7258 return value; 7259 } 7260 7261 @Override 7262 public void removeChild(String name, Base value) throws FHIRException { 7263 if (name.equals("sequence")) { 7264 this.sequence = null; 7265 } else if (name.equals("revenue")) { 7266 this.revenue = null; 7267 } else if (name.equals("category")) { 7268 this.category = null; 7269 } else if (name.equals("productOrService")) { 7270 this.productOrService = null; 7271 } else if (name.equals("modifier")) { 7272 this.getModifier().remove(castToCodeableConcept(value)); 7273 } else if (name.equals("programCode")) { 7274 this.getProgramCode().remove(castToCodeableConcept(value)); 7275 } else if (name.equals("quantity")) { 7276 this.quantity = null; 7277 } else if (name.equals("unitPrice")) { 7278 this.unitPrice = null; 7279 } else if (name.equals("factor")) { 7280 this.factor = null; 7281 } else if (name.equals("net")) { 7282 this.net = null; 7283 } else if (name.equals("udi")) { 7284 this.getUdi().remove(castToReference(value)); 7285 } else if (name.equals("subDetail")) { 7286 this.getSubDetail().remove((SubDetailComponent) value); 7287 } else 7288 super.removeChild(name, value); 7289 7290 } 7291 7292 @Override 7293 public Base makeProperty(int hash, String name) throws FHIRException { 7294 switch (hash) { 7295 case 1349547969: 7296 return getSequenceElement(); 7297 case 1099842588: 7298 return getRevenue(); 7299 case 50511102: 7300 return getCategory(); 7301 case 1957227299: 7302 return getProductOrService(); 7303 case -615513385: 7304 return addModifier(); 7305 case 1010065041: 7306 return addProgramCode(); 7307 case -1285004149: 7308 return getQuantity(); 7309 case -486196699: 7310 return getUnitPrice(); 7311 case -1282148017: 7312 return getFactorElement(); 7313 case 108957: 7314 return getNet(); 7315 case 115642: 7316 return addUdi(); 7317 case -828829007: 7318 return addSubDetail(); 7319 default: 7320 return super.makeProperty(hash, name); 7321 } 7322 7323 } 7324 7325 @Override 7326 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7327 switch (hash) { 7328 case 1349547969: 7329 /* sequence */ return new String[] { "positiveInt" }; 7330 case 1099842588: 7331 /* revenue */ return new String[] { "CodeableConcept" }; 7332 case 50511102: 7333 /* category */ return new String[] { "CodeableConcept" }; 7334 case 1957227299: 7335 /* productOrService */ return new String[] { "CodeableConcept" }; 7336 case -615513385: 7337 /* modifier */ return new String[] { "CodeableConcept" }; 7338 case 1010065041: 7339 /* programCode */ return new String[] { "CodeableConcept" }; 7340 case -1285004149: 7341 /* quantity */ return new String[] { "SimpleQuantity" }; 7342 case -486196699: 7343 /* unitPrice */ return new String[] { "Money" }; 7344 case -1282148017: 7345 /* factor */ return new String[] { "decimal" }; 7346 case 108957: 7347 /* net */ return new String[] { "Money" }; 7348 case 115642: 7349 /* udi */ return new String[] { "Reference" }; 7350 case -828829007: 7351 /* subDetail */ return new String[] {}; 7352 default: 7353 return super.getTypesForProperty(hash, name); 7354 } 7355 7356 } 7357 7358 @Override 7359 public Base addChild(String name) throws FHIRException { 7360 if (name.equals("sequence")) { 7361 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 7362 } else if (name.equals("revenue")) { 7363 this.revenue = new CodeableConcept(); 7364 return this.revenue; 7365 } else if (name.equals("category")) { 7366 this.category = new CodeableConcept(); 7367 return this.category; 7368 } else if (name.equals("productOrService")) { 7369 this.productOrService = new CodeableConcept(); 7370 return this.productOrService; 7371 } else if (name.equals("modifier")) { 7372 return addModifier(); 7373 } else if (name.equals("programCode")) { 7374 return addProgramCode(); 7375 } else if (name.equals("quantity")) { 7376 this.quantity = new Quantity(); 7377 return this.quantity; 7378 } else if (name.equals("unitPrice")) { 7379 this.unitPrice = new Money(); 7380 return this.unitPrice; 7381 } else if (name.equals("factor")) { 7382 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 7383 } else if (name.equals("net")) { 7384 this.net = new Money(); 7385 return this.net; 7386 } else if (name.equals("udi")) { 7387 return addUdi(); 7388 } else if (name.equals("subDetail")) { 7389 return addSubDetail(); 7390 } else 7391 return super.addChild(name); 7392 } 7393 7394 public DetailComponent copy() { 7395 DetailComponent dst = new DetailComponent(); 7396 copyValues(dst); 7397 return dst; 7398 } 7399 7400 public void copyValues(DetailComponent dst) { 7401 super.copyValues(dst); 7402 dst.sequence = sequence == null ? null : sequence.copy(); 7403 dst.revenue = revenue == null ? null : revenue.copy(); 7404 dst.category = category == null ? null : category.copy(); 7405 dst.productOrService = productOrService == null ? null : productOrService.copy(); 7406 if (modifier != null) { 7407 dst.modifier = new ArrayList<CodeableConcept>(); 7408 for (CodeableConcept i : modifier) 7409 dst.modifier.add(i.copy()); 7410 } 7411 ; 7412 if (programCode != null) { 7413 dst.programCode = new ArrayList<CodeableConcept>(); 7414 for (CodeableConcept i : programCode) 7415 dst.programCode.add(i.copy()); 7416 } 7417 ; 7418 dst.quantity = quantity == null ? null : quantity.copy(); 7419 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7420 dst.factor = factor == null ? null : factor.copy(); 7421 dst.net = net == null ? null : net.copy(); 7422 if (udi != null) { 7423 dst.udi = new ArrayList<Reference>(); 7424 for (Reference i : udi) 7425 dst.udi.add(i.copy()); 7426 } 7427 ; 7428 if (subDetail != null) { 7429 dst.subDetail = new ArrayList<SubDetailComponent>(); 7430 for (SubDetailComponent i : subDetail) 7431 dst.subDetail.add(i.copy()); 7432 } 7433 ; 7434 } 7435 7436 @Override 7437 public boolean equalsDeep(Base other_) { 7438 if (!super.equalsDeep(other_)) 7439 return false; 7440 if (!(other_ instanceof DetailComponent)) 7441 return false; 7442 DetailComponent o = (DetailComponent) other_; 7443 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) 7444 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 7445 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 7446 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 7447 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7448 && compareDeep(subDetail, o.subDetail, true); 7449 } 7450 7451 @Override 7452 public boolean equalsShallow(Base other_) { 7453 if (!super.equalsShallow(other_)) 7454 return false; 7455 if (!(other_ instanceof DetailComponent)) 7456 return false; 7457 DetailComponent o = (DetailComponent) other_; 7458 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 7459 } 7460 7461 public boolean isEmpty() { 7462 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category, productOrService, 7463 modifier, programCode, quantity, unitPrice, factor, net, udi, subDetail); 7464 } 7465 7466 public String fhirType() { 7467 return "Claim.item.detail"; 7468 7469 } 7470 7471 } 7472 7473 @Block() 7474 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 7475 /** 7476 * A number to uniquely identify item entries. 7477 */ 7478 @Child(name = "sequence", type = { 7479 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7480 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 7481 protected PositiveIntType sequence; 7482 7483 /** 7484 * The type of revenue or cost center providing the product and/or service. 7485 */ 7486 @Child(name = "revenue", type = { 7487 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7488 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 7489 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 7490 protected CodeableConcept revenue; 7491 7492 /** 7493 * Code to identify the general type of benefits under which products and 7494 * services are provided. 7495 */ 7496 @Child(name = "category", type = { 7497 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 7498 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 7499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 7500 protected CodeableConcept category; 7501 7502 /** 7503 * When the value is a group code then this item collects a set of related claim 7504 * details, otherwise this contains the product, service, drug or other billing 7505 * code for the item. 7506 */ 7507 @Child(name = "productOrService", type = { 7508 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 7509 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 7510 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 7511 protected CodeableConcept productOrService; 7512 7513 /** 7514 * Item typification or modifiers codes to convey additional context for the 7515 * product or service. 7516 */ 7517 @Child(name = "modifier", type = { 7518 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7519 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 7520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 7521 protected List<CodeableConcept> modifier; 7522 7523 /** 7524 * Identifies the program under which this may be recovered. 7525 */ 7526 @Child(name = "programCode", type = { 7527 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7528 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 7529 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 7530 protected List<CodeableConcept> programCode; 7531 7532 /** 7533 * The number of repetitions of a service or product. 7534 */ 7535 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 7536 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 7537 protected Quantity quantity; 7538 7539 /** 7540 * If the item is not a group then this is the fee for the product or service, 7541 * otherwise this is the total of the fees for the details of the group. 7542 */ 7543 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 7544 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 7545 protected Money unitPrice; 7546 7547 /** 7548 * A real number that represents a multiplier used in determining the overall 7549 * value of services delivered and/or goods received. The concept of a Factor 7550 * allows for a discount or surcharge multiplier to be applied to a monetary 7551 * amount. 7552 */ 7553 @Child(name = "factor", type = { 7554 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 7555 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 7556 protected DecimalType factor; 7557 7558 /** 7559 * The quantity times the unit price for an additional service or product or 7560 * charge. 7561 */ 7562 @Child(name = "net", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 7563 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 7564 protected Money net; 7565 7566 /** 7567 * Unique Device Identifiers associated with this line item. 7568 */ 7569 @Child(name = "udi", type = { 7570 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7571 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 7572 protected List<Reference> udi; 7573 /** 7574 * The actual objects that are the target of the reference (Unique Device 7575 * Identifiers associated with this line item.) 7576 */ 7577 protected List<Device> udiTarget; 7578 7579 private static final long serialVersionUID = 1133026301L; 7580 7581 /** 7582 * Constructor 7583 */ 7584 public SubDetailComponent() { 7585 super(); 7586 } 7587 7588 /** 7589 * Constructor 7590 */ 7591 public SubDetailComponent(PositiveIntType sequence, CodeableConcept productOrService) { 7592 super(); 7593 this.sequence = sequence; 7594 this.productOrService = productOrService; 7595 } 7596 7597 /** 7598 * @return {@link #sequence} (A number to uniquely identify item entries.). This 7599 * is the underlying object with id, value and extensions. The accessor 7600 * "getSequence" gives direct access to the value 7601 */ 7602 public PositiveIntType getSequenceElement() { 7603 if (this.sequence == null) 7604 if (Configuration.errorOnAutoCreate()) 7605 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 7606 else if (Configuration.doAutoCreate()) 7607 this.sequence = new PositiveIntType(); // bb 7608 return this.sequence; 7609 } 7610 7611 public boolean hasSequenceElement() { 7612 return this.sequence != null && !this.sequence.isEmpty(); 7613 } 7614 7615 public boolean hasSequence() { 7616 return this.sequence != null && !this.sequence.isEmpty(); 7617 } 7618 7619 /** 7620 * @param value {@link #sequence} (A number to uniquely identify item entries.). 7621 * This is the underlying object with id, value and extensions. The 7622 * accessor "getSequence" gives direct access to the value 7623 */ 7624 public SubDetailComponent setSequenceElement(PositiveIntType value) { 7625 this.sequence = value; 7626 return this; 7627 } 7628 7629 /** 7630 * @return A number to uniquely identify item entries. 7631 */ 7632 public int getSequence() { 7633 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7634 } 7635 7636 /** 7637 * @param value A number to uniquely identify item entries. 7638 */ 7639 public SubDetailComponent setSequence(int value) { 7640 if (this.sequence == null) 7641 this.sequence = new PositiveIntType(); 7642 this.sequence.setValue(value); 7643 return this; 7644 } 7645 7646 /** 7647 * @return {@link #revenue} (The type of revenue or cost center providing the 7648 * product and/or service.) 7649 */ 7650 public CodeableConcept getRevenue() { 7651 if (this.revenue == null) 7652 if (Configuration.errorOnAutoCreate()) 7653 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 7654 else if (Configuration.doAutoCreate()) 7655 this.revenue = new CodeableConcept(); // cc 7656 return this.revenue; 7657 } 7658 7659 public boolean hasRevenue() { 7660 return this.revenue != null && !this.revenue.isEmpty(); 7661 } 7662 7663 /** 7664 * @param value {@link #revenue} (The type of revenue or cost center providing 7665 * the product and/or service.) 7666 */ 7667 public SubDetailComponent setRevenue(CodeableConcept value) { 7668 this.revenue = value; 7669 return this; 7670 } 7671 7672 /** 7673 * @return {@link #category} (Code to identify the general type of benefits 7674 * under which products and services are provided.) 7675 */ 7676 public CodeableConcept getCategory() { 7677 if (this.category == null) 7678 if (Configuration.errorOnAutoCreate()) 7679 throw new Error("Attempt to auto-create SubDetailComponent.category"); 7680 else if (Configuration.doAutoCreate()) 7681 this.category = new CodeableConcept(); // cc 7682 return this.category; 7683 } 7684 7685 public boolean hasCategory() { 7686 return this.category != null && !this.category.isEmpty(); 7687 } 7688 7689 /** 7690 * @param value {@link #category} (Code to identify the general type of benefits 7691 * under which products and services are provided.) 7692 */ 7693 public SubDetailComponent setCategory(CodeableConcept value) { 7694 this.category = value; 7695 return this; 7696 } 7697 7698 /** 7699 * @return {@link #productOrService} (When the value is a group code then this 7700 * item collects a set of related claim details, otherwise this contains 7701 * the product, service, drug or other billing code for the item.) 7702 */ 7703 public CodeableConcept getProductOrService() { 7704 if (this.productOrService == null) 7705 if (Configuration.errorOnAutoCreate()) 7706 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 7707 else if (Configuration.doAutoCreate()) 7708 this.productOrService = new CodeableConcept(); // cc 7709 return this.productOrService; 7710 } 7711 7712 public boolean hasProductOrService() { 7713 return this.productOrService != null && !this.productOrService.isEmpty(); 7714 } 7715 7716 /** 7717 * @param value {@link #productOrService} (When the value is a group code then 7718 * this item collects a set of related claim details, otherwise 7719 * this contains the product, service, drug or other billing code 7720 * for the item.) 7721 */ 7722 public SubDetailComponent setProductOrService(CodeableConcept value) { 7723 this.productOrService = value; 7724 return this; 7725 } 7726 7727 /** 7728 * @return {@link #modifier} (Item typification or modifiers codes to convey 7729 * additional context for the product or service.) 7730 */ 7731 public List<CodeableConcept> getModifier() { 7732 if (this.modifier == null) 7733 this.modifier = new ArrayList<CodeableConcept>(); 7734 return this.modifier; 7735 } 7736 7737 /** 7738 * @return Returns a reference to <code>this</code> for easy method chaining 7739 */ 7740 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 7741 this.modifier = theModifier; 7742 return this; 7743 } 7744 7745 public boolean hasModifier() { 7746 if (this.modifier == null) 7747 return false; 7748 for (CodeableConcept item : this.modifier) 7749 if (!item.isEmpty()) 7750 return true; 7751 return false; 7752 } 7753 7754 public CodeableConcept addModifier() { // 3 7755 CodeableConcept t = new CodeableConcept(); 7756 if (this.modifier == null) 7757 this.modifier = new ArrayList<CodeableConcept>(); 7758 this.modifier.add(t); 7759 return t; 7760 } 7761 7762 public SubDetailComponent addModifier(CodeableConcept t) { // 3 7763 if (t == null) 7764 return this; 7765 if (this.modifier == null) 7766 this.modifier = new ArrayList<CodeableConcept>(); 7767 this.modifier.add(t); 7768 return this; 7769 } 7770 7771 /** 7772 * @return The first repetition of repeating field {@link #modifier}, creating 7773 * it if it does not already exist 7774 */ 7775 public CodeableConcept getModifierFirstRep() { 7776 if (getModifier().isEmpty()) { 7777 addModifier(); 7778 } 7779 return getModifier().get(0); 7780 } 7781 7782 /** 7783 * @return {@link #programCode} (Identifies the program under which this may be 7784 * recovered.) 7785 */ 7786 public List<CodeableConcept> getProgramCode() { 7787 if (this.programCode == null) 7788 this.programCode = new ArrayList<CodeableConcept>(); 7789 return this.programCode; 7790 } 7791 7792 /** 7793 * @return Returns a reference to <code>this</code> for easy method chaining 7794 */ 7795 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 7796 this.programCode = theProgramCode; 7797 return this; 7798 } 7799 7800 public boolean hasProgramCode() { 7801 if (this.programCode == null) 7802 return false; 7803 for (CodeableConcept item : this.programCode) 7804 if (!item.isEmpty()) 7805 return true; 7806 return false; 7807 } 7808 7809 public CodeableConcept addProgramCode() { // 3 7810 CodeableConcept t = new CodeableConcept(); 7811 if (this.programCode == null) 7812 this.programCode = new ArrayList<CodeableConcept>(); 7813 this.programCode.add(t); 7814 return t; 7815 } 7816 7817 public SubDetailComponent addProgramCode(CodeableConcept t) { // 3 7818 if (t == null) 7819 return this; 7820 if (this.programCode == null) 7821 this.programCode = new ArrayList<CodeableConcept>(); 7822 this.programCode.add(t); 7823 return this; 7824 } 7825 7826 /** 7827 * @return The first repetition of repeating field {@link #programCode}, 7828 * creating it if it does not already exist 7829 */ 7830 public CodeableConcept getProgramCodeFirstRep() { 7831 if (getProgramCode().isEmpty()) { 7832 addProgramCode(); 7833 } 7834 return getProgramCode().get(0); 7835 } 7836 7837 /** 7838 * @return {@link #quantity} (The number of repetitions of a service or 7839 * product.) 7840 */ 7841 public Quantity getQuantity() { 7842 if (this.quantity == null) 7843 if (Configuration.errorOnAutoCreate()) 7844 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 7845 else if (Configuration.doAutoCreate()) 7846 this.quantity = new Quantity(); // cc 7847 return this.quantity; 7848 } 7849 7850 public boolean hasQuantity() { 7851 return this.quantity != null && !this.quantity.isEmpty(); 7852 } 7853 7854 /** 7855 * @param value {@link #quantity} (The number of repetitions of a service or 7856 * product.) 7857 */ 7858 public SubDetailComponent setQuantity(Quantity value) { 7859 this.quantity = value; 7860 return this; 7861 } 7862 7863 /** 7864 * @return {@link #unitPrice} (If the item is not a group then this is the fee 7865 * for the product or service, otherwise this is the total of the fees 7866 * for the details of the group.) 7867 */ 7868 public Money getUnitPrice() { 7869 if (this.unitPrice == null) 7870 if (Configuration.errorOnAutoCreate()) 7871 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 7872 else if (Configuration.doAutoCreate()) 7873 this.unitPrice = new Money(); // cc 7874 return this.unitPrice; 7875 } 7876 7877 public boolean hasUnitPrice() { 7878 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7879 } 7880 7881 /** 7882 * @param value {@link #unitPrice} (If the item is not a group then this is the 7883 * fee for the product or service, otherwise this is the total of 7884 * the fees for the details of the group.) 7885 */ 7886 public SubDetailComponent setUnitPrice(Money value) { 7887 this.unitPrice = value; 7888 return this; 7889 } 7890 7891 /** 7892 * @return {@link #factor} (A real number that represents a multiplier used in 7893 * determining the overall value of services delivered and/or goods 7894 * received. The concept of a Factor allows for a discount or surcharge 7895 * multiplier to be applied to a monetary amount.). This is the 7896 * underlying object with id, value and extensions. The accessor 7897 * "getFactor" gives direct access to the value 7898 */ 7899 public DecimalType getFactorElement() { 7900 if (this.factor == null) 7901 if (Configuration.errorOnAutoCreate()) 7902 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 7903 else if (Configuration.doAutoCreate()) 7904 this.factor = new DecimalType(); // bb 7905 return this.factor; 7906 } 7907 7908 public boolean hasFactorElement() { 7909 return this.factor != null && !this.factor.isEmpty(); 7910 } 7911 7912 public boolean hasFactor() { 7913 return this.factor != null && !this.factor.isEmpty(); 7914 } 7915 7916 /** 7917 * @param value {@link #factor} (A real number that represents a multiplier used 7918 * in determining the overall value of services delivered and/or 7919 * goods received. The concept of a Factor allows for a discount or 7920 * surcharge multiplier to be applied to a monetary amount.). This 7921 * is the underlying object with id, value and extensions. The 7922 * accessor "getFactor" gives direct access to the value 7923 */ 7924 public SubDetailComponent setFactorElement(DecimalType value) { 7925 this.factor = value; 7926 return this; 7927 } 7928 7929 /** 7930 * @return A real number that represents a multiplier used in determining the 7931 * overall value of services delivered and/or goods received. The 7932 * concept of a Factor allows for a discount or surcharge multiplier to 7933 * be applied to a monetary amount. 7934 */ 7935 public BigDecimal getFactor() { 7936 return this.factor == null ? null : this.factor.getValue(); 7937 } 7938 7939 /** 7940 * @param value A real number that represents a multiplier used in determining 7941 * the overall value of services delivered and/or goods received. 7942 * The concept of a Factor allows for a discount or surcharge 7943 * multiplier to be applied to a monetary amount. 7944 */ 7945 public SubDetailComponent setFactor(BigDecimal value) { 7946 if (value == null) 7947 this.factor = null; 7948 else { 7949 if (this.factor == null) 7950 this.factor = new DecimalType(); 7951 this.factor.setValue(value); 7952 } 7953 return this; 7954 } 7955 7956 /** 7957 * @param value A real number that represents a multiplier used in determining 7958 * the overall value of services delivered and/or goods received. 7959 * The concept of a Factor allows for a discount or surcharge 7960 * multiplier to be applied to a monetary amount. 7961 */ 7962 public SubDetailComponent setFactor(long value) { 7963 this.factor = new DecimalType(); 7964 this.factor.setValue(value); 7965 return this; 7966 } 7967 7968 /** 7969 * @param value A real number that represents a multiplier used in determining 7970 * the overall value of services delivered and/or goods received. 7971 * The concept of a Factor allows for a discount or surcharge 7972 * multiplier to be applied to a monetary amount. 7973 */ 7974 public SubDetailComponent setFactor(double value) { 7975 this.factor = new DecimalType(); 7976 this.factor.setValue(value); 7977 return this; 7978 } 7979 7980 /** 7981 * @return {@link #net} (The quantity times the unit price for an additional 7982 * service or product or charge.) 7983 */ 7984 public Money getNet() { 7985 if (this.net == null) 7986 if (Configuration.errorOnAutoCreate()) 7987 throw new Error("Attempt to auto-create SubDetailComponent.net"); 7988 else if (Configuration.doAutoCreate()) 7989 this.net = new Money(); // cc 7990 return this.net; 7991 } 7992 7993 public boolean hasNet() { 7994 return this.net != null && !this.net.isEmpty(); 7995 } 7996 7997 /** 7998 * @param value {@link #net} (The quantity times the unit price for an 7999 * additional service or product or charge.) 8000 */ 8001 public SubDetailComponent setNet(Money value) { 8002 this.net = value; 8003 return this; 8004 } 8005 8006 /** 8007 * @return {@link #udi} (Unique Device Identifiers associated with this line 8008 * item.) 8009 */ 8010 public List<Reference> getUdi() { 8011 if (this.udi == null) 8012 this.udi = new ArrayList<Reference>(); 8013 return this.udi; 8014 } 8015 8016 /** 8017 * @return Returns a reference to <code>this</code> for easy method chaining 8018 */ 8019 public SubDetailComponent setUdi(List<Reference> theUdi) { 8020 this.udi = theUdi; 8021 return this; 8022 } 8023 8024 public boolean hasUdi() { 8025 if (this.udi == null) 8026 return false; 8027 for (Reference item : this.udi) 8028 if (!item.isEmpty()) 8029 return true; 8030 return false; 8031 } 8032 8033 public Reference addUdi() { // 3 8034 Reference t = new Reference(); 8035 if (this.udi == null) 8036 this.udi = new ArrayList<Reference>(); 8037 this.udi.add(t); 8038 return t; 8039 } 8040 8041 public SubDetailComponent addUdi(Reference t) { // 3 8042 if (t == null) 8043 return this; 8044 if (this.udi == null) 8045 this.udi = new ArrayList<Reference>(); 8046 this.udi.add(t); 8047 return this; 8048 } 8049 8050 /** 8051 * @return The first repetition of repeating field {@link #udi}, creating it if 8052 * it does not already exist 8053 */ 8054 public Reference getUdiFirstRep() { 8055 if (getUdi().isEmpty()) { 8056 addUdi(); 8057 } 8058 return getUdi().get(0); 8059 } 8060 8061 /** 8062 * @deprecated Use Reference#setResource(IBaseResource) instead 8063 */ 8064 @Deprecated 8065 public List<Device> getUdiTarget() { 8066 if (this.udiTarget == null) 8067 this.udiTarget = new ArrayList<Device>(); 8068 return this.udiTarget; 8069 } 8070 8071 /** 8072 * @deprecated Use Reference#setResource(IBaseResource) instead 8073 */ 8074 @Deprecated 8075 public Device addUdiTarget() { 8076 Device r = new Device(); 8077 if (this.udiTarget == null) 8078 this.udiTarget = new ArrayList<Device>(); 8079 this.udiTarget.add(r); 8080 return r; 8081 } 8082 8083 protected void listChildren(List<Property> children) { 8084 super.listChildren(children); 8085 children 8086 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 8087 children.add(new Property("revenue", "CodeableConcept", 8088 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 8089 children.add(new Property("category", "CodeableConcept", 8090 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 8091 category)); 8092 children.add(new Property("productOrService", "CodeableConcept", 8093 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 8094 0, 1, productOrService)); 8095 children.add(new Property("modifier", "CodeableConcept", 8096 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 8097 java.lang.Integer.MAX_VALUE, modifier)); 8098 children.add(new Property("programCode", "CodeableConcept", 8099 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 8100 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 8101 1, quantity)); 8102 children.add(new Property("unitPrice", "Money", 8103 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 8104 0, 1, unitPrice)); 8105 children.add(new Property("factor", "decimal", 8106 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 8107 0, 1, factor)); 8108 children.add(new Property("net", "Money", 8109 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 8110 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 8111 0, java.lang.Integer.MAX_VALUE, udi)); 8112 } 8113 8114 @Override 8115 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8116 switch (_hash) { 8117 case 1349547969: 8118 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 8119 1, sequence); 8120 case 1099842588: 8121 /* revenue */ return new Property("revenue", "CodeableConcept", 8122 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 8123 case 50511102: 8124 /* category */ return new Property("category", "CodeableConcept", 8125 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 8126 category); 8127 case 1957227299: 8128 /* productOrService */ return new Property("productOrService", "CodeableConcept", 8129 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 8130 0, 1, productOrService); 8131 case -615513385: 8132 /* modifier */ return new Property("modifier", "CodeableConcept", 8133 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 8134 java.lang.Integer.MAX_VALUE, modifier); 8135 case 1010065041: 8136 /* programCode */ return new Property("programCode", "CodeableConcept", 8137 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 8138 case -1285004149: 8139 /* quantity */ return new Property("quantity", "SimpleQuantity", 8140 "The number of repetitions of a service or product.", 0, 1, quantity); 8141 case -486196699: 8142 /* unitPrice */ return new Property("unitPrice", "Money", 8143 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 8144 0, 1, unitPrice); 8145 case -1282148017: 8146 /* factor */ return new Property("factor", "decimal", 8147 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 8148 0, 1, factor); 8149 case 108957: 8150 /* net */ return new Property("net", "Money", 8151 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 8152 case 115642: 8153 /* udi */ return new Property("udi", "Reference(Device)", 8154 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 8155 default: 8156 return super.getNamedProperty(_hash, _name, _checkValid); 8157 } 8158 8159 } 8160 8161 @Override 8162 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8163 switch (hash) { 8164 case 1349547969: 8165 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 8166 case 1099842588: 8167 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 8168 case 50511102: 8169 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 8170 case 1957227299: 8171 /* productOrService */ return this.productOrService == null ? new Base[0] 8172 : new Base[] { this.productOrService }; // CodeableConcept 8173 case -615513385: 8174 /* modifier */ return this.modifier == null ? new Base[0] 8175 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8176 case 1010065041: 8177 /* programCode */ return this.programCode == null ? new Base[0] 8178 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 8179 case -1285004149: 8180 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 8181 case -486196699: 8182 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 8183 case -1282148017: 8184 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 8185 case 108957: 8186 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 8187 case 115642: 8188 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 8189 default: 8190 return super.getProperty(hash, name, checkValid); 8191 } 8192 8193 } 8194 8195 @Override 8196 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8197 switch (hash) { 8198 case 1349547969: // sequence 8199 this.sequence = castToPositiveInt(value); // PositiveIntType 8200 return value; 8201 case 1099842588: // revenue 8202 this.revenue = castToCodeableConcept(value); // CodeableConcept 8203 return value; 8204 case 50511102: // category 8205 this.category = castToCodeableConcept(value); // CodeableConcept 8206 return value; 8207 case 1957227299: // productOrService 8208 this.productOrService = castToCodeableConcept(value); // CodeableConcept 8209 return value; 8210 case -615513385: // modifier 8211 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 8212 return value; 8213 case 1010065041: // programCode 8214 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 8215 return value; 8216 case -1285004149: // quantity 8217 this.quantity = castToQuantity(value); // Quantity 8218 return value; 8219 case -486196699: // unitPrice 8220 this.unitPrice = castToMoney(value); // Money 8221 return value; 8222 case -1282148017: // factor 8223 this.factor = castToDecimal(value); // DecimalType 8224 return value; 8225 case 108957: // net 8226 this.net = castToMoney(value); // Money 8227 return value; 8228 case 115642: // udi 8229 this.getUdi().add(castToReference(value)); // Reference 8230 return value; 8231 default: 8232 return super.setProperty(hash, name, value); 8233 } 8234 8235 } 8236 8237 @Override 8238 public Base setProperty(String name, Base value) throws FHIRException { 8239 if (name.equals("sequence")) { 8240 this.sequence = castToPositiveInt(value); // PositiveIntType 8241 } else if (name.equals("revenue")) { 8242 this.revenue = castToCodeableConcept(value); // CodeableConcept 8243 } else if (name.equals("category")) { 8244 this.category = castToCodeableConcept(value); // CodeableConcept 8245 } else if (name.equals("productOrService")) { 8246 this.productOrService = castToCodeableConcept(value); // CodeableConcept 8247 } else if (name.equals("modifier")) { 8248 this.getModifier().add(castToCodeableConcept(value)); 8249 } else if (name.equals("programCode")) { 8250 this.getProgramCode().add(castToCodeableConcept(value)); 8251 } else if (name.equals("quantity")) { 8252 this.quantity = castToQuantity(value); // Quantity 8253 } else if (name.equals("unitPrice")) { 8254 this.unitPrice = castToMoney(value); // Money 8255 } else if (name.equals("factor")) { 8256 this.factor = castToDecimal(value); // DecimalType 8257 } else if (name.equals("net")) { 8258 this.net = castToMoney(value); // Money 8259 } else if (name.equals("udi")) { 8260 this.getUdi().add(castToReference(value)); 8261 } else 8262 return super.setProperty(name, value); 8263 return value; 8264 } 8265 8266 @Override 8267 public void removeChild(String name, Base value) throws FHIRException { 8268 if (name.equals("sequence")) { 8269 this.sequence = null; 8270 } else if (name.equals("revenue")) { 8271 this.revenue = null; 8272 } else if (name.equals("category")) { 8273 this.category = null; 8274 } else if (name.equals("productOrService")) { 8275 this.productOrService = null; 8276 } else if (name.equals("modifier")) { 8277 this.getModifier().remove(castToCodeableConcept(value)); 8278 } else if (name.equals("programCode")) { 8279 this.getProgramCode().remove(castToCodeableConcept(value)); 8280 } else if (name.equals("quantity")) { 8281 this.quantity = null; 8282 } else if (name.equals("unitPrice")) { 8283 this.unitPrice = null; 8284 } else if (name.equals("factor")) { 8285 this.factor = null; 8286 } else if (name.equals("net")) { 8287 this.net = null; 8288 } else if (name.equals("udi")) { 8289 this.getUdi().remove(castToReference(value)); 8290 } else 8291 super.removeChild(name, value); 8292 8293 } 8294 8295 @Override 8296 public Base makeProperty(int hash, String name) throws FHIRException { 8297 switch (hash) { 8298 case 1349547969: 8299 return getSequenceElement(); 8300 case 1099842588: 8301 return getRevenue(); 8302 case 50511102: 8303 return getCategory(); 8304 case 1957227299: 8305 return getProductOrService(); 8306 case -615513385: 8307 return addModifier(); 8308 case 1010065041: 8309 return addProgramCode(); 8310 case -1285004149: 8311 return getQuantity(); 8312 case -486196699: 8313 return getUnitPrice(); 8314 case -1282148017: 8315 return getFactorElement(); 8316 case 108957: 8317 return getNet(); 8318 case 115642: 8319 return addUdi(); 8320 default: 8321 return super.makeProperty(hash, name); 8322 } 8323 8324 } 8325 8326 @Override 8327 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8328 switch (hash) { 8329 case 1349547969: 8330 /* sequence */ return new String[] { "positiveInt" }; 8331 case 1099842588: 8332 /* revenue */ return new String[] { "CodeableConcept" }; 8333 case 50511102: 8334 /* category */ return new String[] { "CodeableConcept" }; 8335 case 1957227299: 8336 /* productOrService */ return new String[] { "CodeableConcept" }; 8337 case -615513385: 8338 /* modifier */ return new String[] { "CodeableConcept" }; 8339 case 1010065041: 8340 /* programCode */ return new String[] { "CodeableConcept" }; 8341 case -1285004149: 8342 /* quantity */ return new String[] { "SimpleQuantity" }; 8343 case -486196699: 8344 /* unitPrice */ return new String[] { "Money" }; 8345 case -1282148017: 8346 /* factor */ return new String[] { "decimal" }; 8347 case 108957: 8348 /* net */ return new String[] { "Money" }; 8349 case 115642: 8350 /* udi */ return new String[] { "Reference" }; 8351 default: 8352 return super.getTypesForProperty(hash, name); 8353 } 8354 8355 } 8356 8357 @Override 8358 public Base addChild(String name) throws FHIRException { 8359 if (name.equals("sequence")) { 8360 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 8361 } else if (name.equals("revenue")) { 8362 this.revenue = new CodeableConcept(); 8363 return this.revenue; 8364 } else if (name.equals("category")) { 8365 this.category = new CodeableConcept(); 8366 return this.category; 8367 } else if (name.equals("productOrService")) { 8368 this.productOrService = new CodeableConcept(); 8369 return this.productOrService; 8370 } else if (name.equals("modifier")) { 8371 return addModifier(); 8372 } else if (name.equals("programCode")) { 8373 return addProgramCode(); 8374 } else if (name.equals("quantity")) { 8375 this.quantity = new Quantity(); 8376 return this.quantity; 8377 } else if (name.equals("unitPrice")) { 8378 this.unitPrice = new Money(); 8379 return this.unitPrice; 8380 } else if (name.equals("factor")) { 8381 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 8382 } else if (name.equals("net")) { 8383 this.net = new Money(); 8384 return this.net; 8385 } else if (name.equals("udi")) { 8386 return addUdi(); 8387 } else 8388 return super.addChild(name); 8389 } 8390 8391 public SubDetailComponent copy() { 8392 SubDetailComponent dst = new SubDetailComponent(); 8393 copyValues(dst); 8394 return dst; 8395 } 8396 8397 public void copyValues(SubDetailComponent dst) { 8398 super.copyValues(dst); 8399 dst.sequence = sequence == null ? null : sequence.copy(); 8400 dst.revenue = revenue == null ? null : revenue.copy(); 8401 dst.category = category == null ? null : category.copy(); 8402 dst.productOrService = productOrService == null ? null : productOrService.copy(); 8403 if (modifier != null) { 8404 dst.modifier = new ArrayList<CodeableConcept>(); 8405 for (CodeableConcept i : modifier) 8406 dst.modifier.add(i.copy()); 8407 } 8408 ; 8409 if (programCode != null) { 8410 dst.programCode = new ArrayList<CodeableConcept>(); 8411 for (CodeableConcept i : programCode) 8412 dst.programCode.add(i.copy()); 8413 } 8414 ; 8415 dst.quantity = quantity == null ? null : quantity.copy(); 8416 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 8417 dst.factor = factor == null ? null : factor.copy(); 8418 dst.net = net == null ? null : net.copy(); 8419 if (udi != null) { 8420 dst.udi = new ArrayList<Reference>(); 8421 for (Reference i : udi) 8422 dst.udi.add(i.copy()); 8423 } 8424 ; 8425 } 8426 8427 @Override 8428 public boolean equalsDeep(Base other_) { 8429 if (!super.equalsDeep(other_)) 8430 return false; 8431 if (!(other_ instanceof SubDetailComponent)) 8432 return false; 8433 SubDetailComponent o = (SubDetailComponent) other_; 8434 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) 8435 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 8436 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 8437 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 8438 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true); 8439 } 8440 8441 @Override 8442 public boolean equalsShallow(Base other_) { 8443 if (!super.equalsShallow(other_)) 8444 return false; 8445 if (!(other_ instanceof SubDetailComponent)) 8446 return false; 8447 SubDetailComponent o = (SubDetailComponent) other_; 8448 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 8449 } 8450 8451 public boolean isEmpty() { 8452 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category, productOrService, 8453 modifier, programCode, quantity, unitPrice, factor, net, udi); 8454 } 8455 8456 public String fhirType() { 8457 return "Claim.item.detail.subDetail"; 8458 8459 } 8460 8461 } 8462 8463 /** 8464 * A unique identifier assigned to this claim. 8465 */ 8466 @Child(name = "identifier", type = { 8467 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8468 @Description(shortDefinition = "Business Identifier for claim", formalDefinition = "A unique identifier assigned to this claim.") 8469 protected List<Identifier> identifier; 8470 8471 /** 8472 * The status of the resource instance. 8473 */ 8474 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 8475 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 8476 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 8477 protected Enumeration<ClaimStatus> status; 8478 8479 /** 8480 * The category of claim, e.g. oral, pharmacy, vision, institutional, 8481 * professional. 8482 */ 8483 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 8484 @Description(shortDefinition = "Category or discipline", formalDefinition = "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.") 8485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-type") 8486 protected CodeableConcept type; 8487 8488 /** 8489 * A finer grained suite of claim type codes which may convey additional 8490 * information such as Inpatient vs Outpatient and/or a specialty service. 8491 */ 8492 @Child(name = "subType", type = { 8493 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 8494 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 8495 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-subtype") 8496 protected CodeableConcept subType; 8497 8498 /** 8499 * A code to indicate whether the nature of the request is: to request 8500 * adjudication of products and services previously rendered; or requesting 8501 * authorization and adjudication for provision in the future; or requesting the 8502 * non-binding adjudication of the listed products and services which could be 8503 * provided in the future. 8504 */ 8505 @Child(name = "use", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 8506 @Description(shortDefinition = "claim | preauthorization | predetermination", formalDefinition = "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.") 8507 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-use") 8508 protected Enumeration<Use> use; 8509 8510 /** 8511 * The party to whom the professional services and/or products have been 8512 * supplied or are being considered and for whom actual or forecast 8513 * reimbursement is sought. 8514 */ 8515 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 8516 @Description(shortDefinition = "The recipient of the products and services", formalDefinition = "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.") 8517 protected Reference patient; 8518 8519 /** 8520 * The actual object that is the target of the reference (The party to whom the 8521 * professional services and/or products have been supplied or are being 8522 * considered and for whom actual or forecast reimbursement is sought.) 8523 */ 8524 protected Patient patientTarget; 8525 8526 /** 8527 * The period for which charges are being submitted. 8528 */ 8529 @Child(name = "billablePeriod", type = { 8530 Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 8531 @Description(shortDefinition = "Relevant time frame for the claim", formalDefinition = "The period for which charges are being submitted.") 8532 protected Period billablePeriod; 8533 8534 /** 8535 * The date this resource was created. 8536 */ 8537 @Child(name = "created", type = { DateTimeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 8538 @Description(shortDefinition = "Resource creation date", formalDefinition = "The date this resource was created.") 8539 protected DateTimeType created; 8540 8541 /** 8542 * Individual who created the claim, predetermination or preauthorization. 8543 */ 8544 @Child(name = "enterer", type = { Practitioner.class, 8545 PractitionerRole.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 8546 @Description(shortDefinition = "Author of the claim", formalDefinition = "Individual who created the claim, predetermination or preauthorization.") 8547 protected Reference enterer; 8548 8549 /** 8550 * The actual object that is the target of the reference (Individual who created 8551 * the claim, predetermination or preauthorization.) 8552 */ 8553 protected Resource entererTarget; 8554 8555 /** 8556 * The Insurer who is target of the request. 8557 */ 8558 @Child(name = "insurer", type = { Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 8559 @Description(shortDefinition = "Target", formalDefinition = "The Insurer who is target of the request.") 8560 protected Reference insurer; 8561 8562 /** 8563 * The actual object that is the target of the reference (The Insurer who is 8564 * target of the request.) 8565 */ 8566 protected Organization insurerTarget; 8567 8568 /** 8569 * The provider which is responsible for the claim, predetermination or 8570 * preauthorization. 8571 */ 8572 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 8573 Organization.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 8574 @Description(shortDefinition = "Party responsible for the claim", formalDefinition = "The provider which is responsible for the claim, predetermination or preauthorization.") 8575 protected Reference provider; 8576 8577 /** 8578 * The actual object that is the target of the reference (The provider which is 8579 * responsible for the claim, predetermination or preauthorization.) 8580 */ 8581 protected Resource providerTarget; 8582 8583 /** 8584 * The provider-required urgency of processing the request. Typical values 8585 * include: stat, routine deferred. 8586 */ 8587 @Child(name = "priority", type = { 8588 CodeableConcept.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 8589 @Description(shortDefinition = "Desired processing ugency", formalDefinition = "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.") 8590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/process-priority") 8591 protected CodeableConcept priority; 8592 8593 /** 8594 * A code to indicate whether and for whom funds are to be reserved for future 8595 * claims. 8596 */ 8597 @Child(name = "fundsReserve", type = { 8598 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 8599 @Description(shortDefinition = "For whom to reserve funds", formalDefinition = "A code to indicate whether and for whom funds are to be reserved for future claims.") 8600 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fundsreserve") 8601 protected CodeableConcept fundsReserve; 8602 8603 /** 8604 * Other claims which are related to this claim such as prior submissions or 8605 * claims for related services or for the same event. 8606 */ 8607 @Child(name = "related", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8608 @Description(shortDefinition = "Prior or corollary claims", formalDefinition = "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.") 8609 protected List<RelatedClaimComponent> related; 8610 8611 /** 8612 * Prescription to support the dispensing of pharmacy, device or vision 8613 * products. 8614 */ 8615 @Child(name = "prescription", type = { DeviceRequest.class, MedicationRequest.class, 8616 VisionPrescription.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 8617 @Description(shortDefinition = "Prescription authorizing services and products", formalDefinition = "Prescription to support the dispensing of pharmacy, device or vision products.") 8618 protected Reference prescription; 8619 8620 /** 8621 * The actual object that is the target of the reference (Prescription to 8622 * support the dispensing of pharmacy, device or vision products.) 8623 */ 8624 protected Resource prescriptionTarget; 8625 8626 /** 8627 * Original prescription which has been superseded by this prescription to 8628 * support the dispensing of pharmacy services, medications or products. 8629 */ 8630 @Child(name = "originalPrescription", type = { DeviceRequest.class, MedicationRequest.class, 8631 VisionPrescription.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 8632 @Description(shortDefinition = "Original prescription if superseded by fulfiller", formalDefinition = "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.") 8633 protected Reference originalPrescription; 8634 8635 /** 8636 * The actual object that is the target of the reference (Original prescription 8637 * which has been superseded by this prescription to support the dispensing of 8638 * pharmacy services, medications or products.) 8639 */ 8640 protected Resource originalPrescriptionTarget; 8641 8642 /** 8643 * The party to be reimbursed for cost of the products and services according to 8644 * the terms of the policy. 8645 */ 8646 @Child(name = "payee", type = {}, order = 16, min = 0, max = 1, modifier = false, summary = false) 8647 @Description(shortDefinition = "Recipient of benefits payable", formalDefinition = "The party to be reimbursed for cost of the products and services according to the terms of the policy.") 8648 protected PayeeComponent payee; 8649 8650 /** 8651 * A reference to a referral resource. 8652 */ 8653 @Child(name = "referral", type = { 8654 ServiceRequest.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 8655 @Description(shortDefinition = "Treatment referral", formalDefinition = "A reference to a referral resource.") 8656 protected Reference referral; 8657 8658 /** 8659 * The actual object that is the target of the reference (A reference to a 8660 * referral resource.) 8661 */ 8662 protected ServiceRequest referralTarget; 8663 8664 /** 8665 * Facility where the services were provided. 8666 */ 8667 @Child(name = "facility", type = { Location.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 8668 @Description(shortDefinition = "Servicing facility", formalDefinition = "Facility where the services were provided.") 8669 protected Reference facility; 8670 8671 /** 8672 * The actual object that is the target of the reference (Facility where the 8673 * services were provided.) 8674 */ 8675 protected Location facilityTarget; 8676 8677 /** 8678 * The members of the team who provided the products and services. 8679 */ 8680 @Child(name = "careTeam", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8681 @Description(shortDefinition = "Members of the care team", formalDefinition = "The members of the team who provided the products and services.") 8682 protected List<CareTeamComponent> careTeam; 8683 8684 /** 8685 * Additional information codes regarding exceptions, special considerations, 8686 * the condition, situation, prior or concurrent issues. 8687 */ 8688 @Child(name = "supportingInfo", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8689 @Description(shortDefinition = "Supporting information", formalDefinition = "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.") 8690 protected List<SupportingInformationComponent> supportingInfo; 8691 8692 /** 8693 * Information about diagnoses relevant to the claim items. 8694 */ 8695 @Child(name = "diagnosis", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8696 @Description(shortDefinition = "Pertinent diagnosis information", formalDefinition = "Information about diagnoses relevant to the claim items.") 8697 protected List<DiagnosisComponent> diagnosis; 8698 8699 /** 8700 * Procedures performed on the patient relevant to the billing items with the 8701 * claim. 8702 */ 8703 @Child(name = "procedure", type = {}, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8704 @Description(shortDefinition = "Clinical procedures performed", formalDefinition = "Procedures performed on the patient relevant to the billing items with the claim.") 8705 protected List<ProcedureComponent> procedure; 8706 8707 /** 8708 * Financial instruments for reimbursement for the health care products and 8709 * services specified on the claim. 8710 */ 8711 @Child(name = "insurance", type = {}, order = 23, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8712 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services specified on the claim.") 8713 protected List<InsuranceComponent> insurance; 8714 8715 /** 8716 * Details of an accident which resulted in injuries which required the products 8717 * and services listed in the claim. 8718 */ 8719 @Child(name = "accident", type = {}, order = 24, min = 0, max = 1, modifier = false, summary = false) 8720 @Description(shortDefinition = "Details of the event", formalDefinition = "Details of an accident which resulted in injuries which required the products and services listed in the claim.") 8721 protected AccidentComponent accident; 8722 8723 /** 8724 * A claim line. Either a simple product or service or a 'group' of details 8725 * which can each be a simple items or groups of sub-details. 8726 */ 8727 @Child(name = "item", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8728 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.") 8729 protected List<ItemComponent> item; 8730 8731 /** 8732 * The total value of the all the items in the claim. 8733 */ 8734 @Child(name = "total", type = { Money.class }, order = 26, min = 0, max = 1, modifier = false, summary = false) 8735 @Description(shortDefinition = "Total claim cost", formalDefinition = "The total value of the all the items in the claim.") 8736 protected Money total; 8737 8738 private static final long serialVersionUID = -80376108L; 8739 8740 /** 8741 * Constructor 8742 */ 8743 public Claim() { 8744 super(); 8745 } 8746 8747 /** 8748 * Constructor 8749 */ 8750 public Claim(Enumeration<ClaimStatus> status, CodeableConcept type, Enumeration<Use> use, Reference patient, 8751 DateTimeType created, Reference provider, CodeableConcept priority) { 8752 super(); 8753 this.status = status; 8754 this.type = type; 8755 this.use = use; 8756 this.patient = patient; 8757 this.created = created; 8758 this.provider = provider; 8759 this.priority = priority; 8760 } 8761 8762 /** 8763 * @return {@link #identifier} (A unique identifier assigned to this claim.) 8764 */ 8765 public List<Identifier> getIdentifier() { 8766 if (this.identifier == null) 8767 this.identifier = new ArrayList<Identifier>(); 8768 return this.identifier; 8769 } 8770 8771 /** 8772 * @return Returns a reference to <code>this</code> for easy method chaining 8773 */ 8774 public Claim setIdentifier(List<Identifier> theIdentifier) { 8775 this.identifier = theIdentifier; 8776 return this; 8777 } 8778 8779 public boolean hasIdentifier() { 8780 if (this.identifier == null) 8781 return false; 8782 for (Identifier item : this.identifier) 8783 if (!item.isEmpty()) 8784 return true; 8785 return false; 8786 } 8787 8788 public Identifier addIdentifier() { // 3 8789 Identifier t = new Identifier(); 8790 if (this.identifier == null) 8791 this.identifier = new ArrayList<Identifier>(); 8792 this.identifier.add(t); 8793 return t; 8794 } 8795 8796 public Claim addIdentifier(Identifier t) { // 3 8797 if (t == null) 8798 return this; 8799 if (this.identifier == null) 8800 this.identifier = new ArrayList<Identifier>(); 8801 this.identifier.add(t); 8802 return this; 8803 } 8804 8805 /** 8806 * @return The first repetition of repeating field {@link #identifier}, creating 8807 * it if it does not already exist 8808 */ 8809 public Identifier getIdentifierFirstRep() { 8810 if (getIdentifier().isEmpty()) { 8811 addIdentifier(); 8812 } 8813 return getIdentifier().get(0); 8814 } 8815 8816 /** 8817 * @return {@link #status} (The status of the resource instance.). This is the 8818 * underlying object with id, value and extensions. The accessor 8819 * "getStatus" gives direct access to the value 8820 */ 8821 public Enumeration<ClaimStatus> getStatusElement() { 8822 if (this.status == null) 8823 if (Configuration.errorOnAutoCreate()) 8824 throw new Error("Attempt to auto-create Claim.status"); 8825 else if (Configuration.doAutoCreate()) 8826 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); // bb 8827 return this.status; 8828 } 8829 8830 public boolean hasStatusElement() { 8831 return this.status != null && !this.status.isEmpty(); 8832 } 8833 8834 public boolean hasStatus() { 8835 return this.status != null && !this.status.isEmpty(); 8836 } 8837 8838 /** 8839 * @param value {@link #status} (The status of the resource instance.). This is 8840 * the underlying object with id, value and extensions. The 8841 * accessor "getStatus" gives direct access to the value 8842 */ 8843 public Claim setStatusElement(Enumeration<ClaimStatus> value) { 8844 this.status = value; 8845 return this; 8846 } 8847 8848 /** 8849 * @return The status of the resource instance. 8850 */ 8851 public ClaimStatus getStatus() { 8852 return this.status == null ? null : this.status.getValue(); 8853 } 8854 8855 /** 8856 * @param value The status of the resource instance. 8857 */ 8858 public Claim setStatus(ClaimStatus value) { 8859 if (this.status == null) 8860 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); 8861 this.status.setValue(value); 8862 return this; 8863 } 8864 8865 /** 8866 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, 8867 * institutional, professional.) 8868 */ 8869 public CodeableConcept getType() { 8870 if (this.type == null) 8871 if (Configuration.errorOnAutoCreate()) 8872 throw new Error("Attempt to auto-create Claim.type"); 8873 else if (Configuration.doAutoCreate()) 8874 this.type = new CodeableConcept(); // cc 8875 return this.type; 8876 } 8877 8878 public boolean hasType() { 8879 return this.type != null && !this.type.isEmpty(); 8880 } 8881 8882 /** 8883 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, 8884 * vision, institutional, professional.) 8885 */ 8886 public Claim setType(CodeableConcept value) { 8887 this.type = value; 8888 return this; 8889 } 8890 8891 /** 8892 * @return {@link #subType} (A finer grained suite of claim type codes which may 8893 * convey additional information such as Inpatient vs Outpatient and/or 8894 * a specialty service.) 8895 */ 8896 public CodeableConcept getSubType() { 8897 if (this.subType == null) 8898 if (Configuration.errorOnAutoCreate()) 8899 throw new Error("Attempt to auto-create Claim.subType"); 8900 else if (Configuration.doAutoCreate()) 8901 this.subType = new CodeableConcept(); // cc 8902 return this.subType; 8903 } 8904 8905 public boolean hasSubType() { 8906 return this.subType != null && !this.subType.isEmpty(); 8907 } 8908 8909 /** 8910 * @param value {@link #subType} (A finer grained suite of claim type codes 8911 * which may convey additional information such as Inpatient vs 8912 * Outpatient and/or a specialty service.) 8913 */ 8914 public Claim setSubType(CodeableConcept value) { 8915 this.subType = value; 8916 return this; 8917 } 8918 8919 /** 8920 * @return {@link #use} (A code to indicate whether the nature of the request 8921 * is: to request adjudication of products and services previously 8922 * rendered; or requesting authorization and adjudication for provision 8923 * in the future; or requesting the non-binding adjudication of the 8924 * listed products and services which could be provided in the future.). 8925 * This is the underlying object with id, value and extensions. The 8926 * accessor "getUse" gives direct access to the value 8927 */ 8928 public Enumeration<Use> getUseElement() { 8929 if (this.use == null) 8930 if (Configuration.errorOnAutoCreate()) 8931 throw new Error("Attempt to auto-create Claim.use"); 8932 else if (Configuration.doAutoCreate()) 8933 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 8934 return this.use; 8935 } 8936 8937 public boolean hasUseElement() { 8938 return this.use != null && !this.use.isEmpty(); 8939 } 8940 8941 public boolean hasUse() { 8942 return this.use != null && !this.use.isEmpty(); 8943 } 8944 8945 /** 8946 * @param value {@link #use} (A code to indicate whether the nature of the 8947 * request is: to request adjudication of products and services 8948 * previously rendered; or requesting authorization and 8949 * adjudication for provision in the future; or requesting the 8950 * non-binding adjudication of the listed products and services 8951 * which could be provided in the future.). This is the underlying 8952 * object with id, value and extensions. The accessor "getUse" 8953 * gives direct access to the value 8954 */ 8955 public Claim setUseElement(Enumeration<Use> value) { 8956 this.use = value; 8957 return this; 8958 } 8959 8960 /** 8961 * @return A code to indicate whether the nature of the request is: to request 8962 * adjudication of products and services previously rendered; or 8963 * requesting authorization and adjudication for provision in the 8964 * future; or requesting the non-binding adjudication of the listed 8965 * products and services which could be provided in the future. 8966 */ 8967 public Use getUse() { 8968 return this.use == null ? null : this.use.getValue(); 8969 } 8970 8971 /** 8972 * @param value A code to indicate whether the nature of the request is: to 8973 * request adjudication of products and services previously 8974 * rendered; or requesting authorization and adjudication for 8975 * provision in the future; or requesting the non-binding 8976 * adjudication of the listed products and services which could be 8977 * provided in the future. 8978 */ 8979 public Claim setUse(Use value) { 8980 if (this.use == null) 8981 this.use = new Enumeration<Use>(new UseEnumFactory()); 8982 this.use.setValue(value); 8983 return this; 8984 } 8985 8986 /** 8987 * @return {@link #patient} (The party to whom the professional services and/or 8988 * products have been supplied or are being considered and for whom 8989 * actual or forecast reimbursement is sought.) 8990 */ 8991 public Reference getPatient() { 8992 if (this.patient == null) 8993 if (Configuration.errorOnAutoCreate()) 8994 throw new Error("Attempt to auto-create Claim.patient"); 8995 else if (Configuration.doAutoCreate()) 8996 this.patient = new Reference(); // cc 8997 return this.patient; 8998 } 8999 9000 public boolean hasPatient() { 9001 return this.patient != null && !this.patient.isEmpty(); 9002 } 9003 9004 /** 9005 * @param value {@link #patient} (The party to whom the professional services 9006 * and/or products have been supplied or are being considered and 9007 * for whom actual or forecast reimbursement is sought.) 9008 */ 9009 public Claim setPatient(Reference value) { 9010 this.patient = value; 9011 return this; 9012 } 9013 9014 /** 9015 * @return {@link #patient} The actual object that is the target of the 9016 * reference. The reference library doesn't populate this, but you can 9017 * use it to hold the resource if you resolve it. (The party to whom the 9018 * professional services and/or products have been supplied or are being 9019 * considered and for whom actual or forecast reimbursement is sought.) 9020 */ 9021 public Patient getPatientTarget() { 9022 if (this.patientTarget == null) 9023 if (Configuration.errorOnAutoCreate()) 9024 throw new Error("Attempt to auto-create Claim.patient"); 9025 else if (Configuration.doAutoCreate()) 9026 this.patientTarget = new Patient(); // aa 9027 return this.patientTarget; 9028 } 9029 9030 /** 9031 * @param value {@link #patient} The actual object that is the target of the 9032 * reference. The reference library doesn't use these, but you can 9033 * use it to hold the resource if you resolve it. (The party to 9034 * whom the professional services and/or products have been 9035 * supplied or are being considered and for whom actual or forecast 9036 * reimbursement is sought.) 9037 */ 9038 public Claim setPatientTarget(Patient value) { 9039 this.patientTarget = value; 9040 return this; 9041 } 9042 9043 /** 9044 * @return {@link #billablePeriod} (The period for which charges are being 9045 * submitted.) 9046 */ 9047 public Period getBillablePeriod() { 9048 if (this.billablePeriod == null) 9049 if (Configuration.errorOnAutoCreate()) 9050 throw new Error("Attempt to auto-create Claim.billablePeriod"); 9051 else if (Configuration.doAutoCreate()) 9052 this.billablePeriod = new Period(); // cc 9053 return this.billablePeriod; 9054 } 9055 9056 public boolean hasBillablePeriod() { 9057 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 9058 } 9059 9060 /** 9061 * @param value {@link #billablePeriod} (The period for which charges are being 9062 * submitted.) 9063 */ 9064 public Claim setBillablePeriod(Period value) { 9065 this.billablePeriod = value; 9066 return this; 9067 } 9068 9069 /** 9070 * @return {@link #created} (The date this resource was created.). This is the 9071 * underlying object with id, value and extensions. The accessor 9072 * "getCreated" gives direct access to the value 9073 */ 9074 public DateTimeType getCreatedElement() { 9075 if (this.created == null) 9076 if (Configuration.errorOnAutoCreate()) 9077 throw new Error("Attempt to auto-create Claim.created"); 9078 else if (Configuration.doAutoCreate()) 9079 this.created = new DateTimeType(); // bb 9080 return this.created; 9081 } 9082 9083 public boolean hasCreatedElement() { 9084 return this.created != null && !this.created.isEmpty(); 9085 } 9086 9087 public boolean hasCreated() { 9088 return this.created != null && !this.created.isEmpty(); 9089 } 9090 9091 /** 9092 * @param value {@link #created} (The date this resource was created.). This is 9093 * the underlying object with id, value and extensions. The 9094 * accessor "getCreated" gives direct access to the value 9095 */ 9096 public Claim setCreatedElement(DateTimeType value) { 9097 this.created = value; 9098 return this; 9099 } 9100 9101 /** 9102 * @return The date this resource was created. 9103 */ 9104 public Date getCreated() { 9105 return this.created == null ? null : this.created.getValue(); 9106 } 9107 9108 /** 9109 * @param value The date this resource was created. 9110 */ 9111 public Claim setCreated(Date value) { 9112 if (this.created == null) 9113 this.created = new DateTimeType(); 9114 this.created.setValue(value); 9115 return this; 9116 } 9117 9118 /** 9119 * @return {@link #enterer} (Individual who created the claim, predetermination 9120 * or preauthorization.) 9121 */ 9122 public Reference getEnterer() { 9123 if (this.enterer == null) 9124 if (Configuration.errorOnAutoCreate()) 9125 throw new Error("Attempt to auto-create Claim.enterer"); 9126 else if (Configuration.doAutoCreate()) 9127 this.enterer = new Reference(); // cc 9128 return this.enterer; 9129 } 9130 9131 public boolean hasEnterer() { 9132 return this.enterer != null && !this.enterer.isEmpty(); 9133 } 9134 9135 /** 9136 * @param value {@link #enterer} (Individual who created the claim, 9137 * predetermination or preauthorization.) 9138 */ 9139 public Claim setEnterer(Reference value) { 9140 this.enterer = value; 9141 return this; 9142 } 9143 9144 /** 9145 * @return {@link #enterer} The actual object that is the target of the 9146 * reference. The reference library doesn't populate this, but you can 9147 * use it to hold the resource if you resolve it. (Individual who 9148 * created the claim, predetermination or preauthorization.) 9149 */ 9150 public Resource getEntererTarget() { 9151 return this.entererTarget; 9152 } 9153 9154 /** 9155 * @param value {@link #enterer} The actual object that is the target of the 9156 * reference. The reference library doesn't use these, but you can 9157 * use it to hold the resource if you resolve it. (Individual who 9158 * created the claim, predetermination or preauthorization.) 9159 */ 9160 public Claim setEntererTarget(Resource value) { 9161 this.entererTarget = value; 9162 return this; 9163 } 9164 9165 /** 9166 * @return {@link #insurer} (The Insurer who is target of the request.) 9167 */ 9168 public Reference getInsurer() { 9169 if (this.insurer == null) 9170 if (Configuration.errorOnAutoCreate()) 9171 throw new Error("Attempt to auto-create Claim.insurer"); 9172 else if (Configuration.doAutoCreate()) 9173 this.insurer = new Reference(); // cc 9174 return this.insurer; 9175 } 9176 9177 public boolean hasInsurer() { 9178 return this.insurer != null && !this.insurer.isEmpty(); 9179 } 9180 9181 /** 9182 * @param value {@link #insurer} (The Insurer who is target of the request.) 9183 */ 9184 public Claim setInsurer(Reference value) { 9185 this.insurer = value; 9186 return this; 9187 } 9188 9189 /** 9190 * @return {@link #insurer} The actual object that is the target of the 9191 * reference. The reference library doesn't populate this, but you can 9192 * use it to hold the resource if you resolve it. (The Insurer who is 9193 * target of the request.) 9194 */ 9195 public Organization getInsurerTarget() { 9196 if (this.insurerTarget == null) 9197 if (Configuration.errorOnAutoCreate()) 9198 throw new Error("Attempt to auto-create Claim.insurer"); 9199 else if (Configuration.doAutoCreate()) 9200 this.insurerTarget = new Organization(); // aa 9201 return this.insurerTarget; 9202 } 9203 9204 /** 9205 * @param value {@link #insurer} The actual object that is the target of the 9206 * reference. The reference library doesn't use these, but you can 9207 * use it to hold the resource if you resolve it. (The Insurer who 9208 * is target of the request.) 9209 */ 9210 public Claim setInsurerTarget(Organization value) { 9211 this.insurerTarget = value; 9212 return this; 9213 } 9214 9215 /** 9216 * @return {@link #provider} (The provider which is responsible for the claim, 9217 * predetermination or preauthorization.) 9218 */ 9219 public Reference getProvider() { 9220 if (this.provider == null) 9221 if (Configuration.errorOnAutoCreate()) 9222 throw new Error("Attempt to auto-create Claim.provider"); 9223 else if (Configuration.doAutoCreate()) 9224 this.provider = new Reference(); // cc 9225 return this.provider; 9226 } 9227 9228 public boolean hasProvider() { 9229 return this.provider != null && !this.provider.isEmpty(); 9230 } 9231 9232 /** 9233 * @param value {@link #provider} (The provider which is responsible for the 9234 * claim, predetermination or preauthorization.) 9235 */ 9236 public Claim setProvider(Reference value) { 9237 this.provider = value; 9238 return this; 9239 } 9240 9241 /** 9242 * @return {@link #provider} The actual object that is the target of the 9243 * reference. The reference library doesn't populate this, but you can 9244 * use it to hold the resource if you resolve it. (The provider which is 9245 * responsible for the claim, predetermination or preauthorization.) 9246 */ 9247 public Resource getProviderTarget() { 9248 return this.providerTarget; 9249 } 9250 9251 /** 9252 * @param value {@link #provider} The actual object that is the target of the 9253 * reference. The reference library doesn't use these, but you can 9254 * use it to hold the resource if you resolve it. (The provider 9255 * which is responsible for the claim, predetermination or 9256 * preauthorization.) 9257 */ 9258 public Claim setProviderTarget(Resource value) { 9259 this.providerTarget = value; 9260 return this; 9261 } 9262 9263 /** 9264 * @return {@link #priority} (The provider-required urgency of processing the 9265 * request. Typical values include: stat, routine deferred.) 9266 */ 9267 public CodeableConcept getPriority() { 9268 if (this.priority == null) 9269 if (Configuration.errorOnAutoCreate()) 9270 throw new Error("Attempt to auto-create Claim.priority"); 9271 else if (Configuration.doAutoCreate()) 9272 this.priority = new CodeableConcept(); // cc 9273 return this.priority; 9274 } 9275 9276 public boolean hasPriority() { 9277 return this.priority != null && !this.priority.isEmpty(); 9278 } 9279 9280 /** 9281 * @param value {@link #priority} (The provider-required urgency of processing 9282 * the request. Typical values include: stat, routine deferred.) 9283 */ 9284 public Claim setPriority(CodeableConcept value) { 9285 this.priority = value; 9286 return this; 9287 } 9288 9289 /** 9290 * @return {@link #fundsReserve} (A code to indicate whether and for whom funds 9291 * are to be reserved for future claims.) 9292 */ 9293 public CodeableConcept getFundsReserve() { 9294 if (this.fundsReserve == null) 9295 if (Configuration.errorOnAutoCreate()) 9296 throw new Error("Attempt to auto-create Claim.fundsReserve"); 9297 else if (Configuration.doAutoCreate()) 9298 this.fundsReserve = new CodeableConcept(); // cc 9299 return this.fundsReserve; 9300 } 9301 9302 public boolean hasFundsReserve() { 9303 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 9304 } 9305 9306 /** 9307 * @param value {@link #fundsReserve} (A code to indicate whether and for whom 9308 * funds are to be reserved for future claims.) 9309 */ 9310 public Claim setFundsReserve(CodeableConcept value) { 9311 this.fundsReserve = value; 9312 return this; 9313 } 9314 9315 /** 9316 * @return {@link #related} (Other claims which are related to this claim such 9317 * as prior submissions or claims for related services or for the same 9318 * event.) 9319 */ 9320 public List<RelatedClaimComponent> getRelated() { 9321 if (this.related == null) 9322 this.related = new ArrayList<RelatedClaimComponent>(); 9323 return this.related; 9324 } 9325 9326 /** 9327 * @return Returns a reference to <code>this</code> for easy method chaining 9328 */ 9329 public Claim setRelated(List<RelatedClaimComponent> theRelated) { 9330 this.related = theRelated; 9331 return this; 9332 } 9333 9334 public boolean hasRelated() { 9335 if (this.related == null) 9336 return false; 9337 for (RelatedClaimComponent item : this.related) 9338 if (!item.isEmpty()) 9339 return true; 9340 return false; 9341 } 9342 9343 public RelatedClaimComponent addRelated() { // 3 9344 RelatedClaimComponent t = new RelatedClaimComponent(); 9345 if (this.related == null) 9346 this.related = new ArrayList<RelatedClaimComponent>(); 9347 this.related.add(t); 9348 return t; 9349 } 9350 9351 public Claim addRelated(RelatedClaimComponent t) { // 3 9352 if (t == null) 9353 return this; 9354 if (this.related == null) 9355 this.related = new ArrayList<RelatedClaimComponent>(); 9356 this.related.add(t); 9357 return this; 9358 } 9359 9360 /** 9361 * @return The first repetition of repeating field {@link #related}, creating it 9362 * if it does not already exist 9363 */ 9364 public RelatedClaimComponent getRelatedFirstRep() { 9365 if (getRelated().isEmpty()) { 9366 addRelated(); 9367 } 9368 return getRelated().get(0); 9369 } 9370 9371 /** 9372 * @return {@link #prescription} (Prescription to support the dispensing of 9373 * pharmacy, device or vision products.) 9374 */ 9375 public Reference getPrescription() { 9376 if (this.prescription == null) 9377 if (Configuration.errorOnAutoCreate()) 9378 throw new Error("Attempt to auto-create Claim.prescription"); 9379 else if (Configuration.doAutoCreate()) 9380 this.prescription = new Reference(); // cc 9381 return this.prescription; 9382 } 9383 9384 public boolean hasPrescription() { 9385 return this.prescription != null && !this.prescription.isEmpty(); 9386 } 9387 9388 /** 9389 * @param value {@link #prescription} (Prescription to support the dispensing of 9390 * pharmacy, device or vision products.) 9391 */ 9392 public Claim setPrescription(Reference value) { 9393 this.prescription = value; 9394 return this; 9395 } 9396 9397 /** 9398 * @return {@link #prescription} The actual object that is the target of the 9399 * reference. The reference library doesn't populate this, but you can 9400 * use it to hold the resource if you resolve it. (Prescription to 9401 * support the dispensing of pharmacy, device or vision products.) 9402 */ 9403 public Resource getPrescriptionTarget() { 9404 return this.prescriptionTarget; 9405 } 9406 9407 /** 9408 * @param value {@link #prescription} The actual object that is the target of 9409 * the reference. The reference library doesn't use these, but you 9410 * can use it to hold the resource if you resolve it. (Prescription 9411 * to support the dispensing of pharmacy, device or vision 9412 * products.) 9413 */ 9414 public Claim setPrescriptionTarget(Resource value) { 9415 this.prescriptionTarget = value; 9416 return this; 9417 } 9418 9419 /** 9420 * @return {@link #originalPrescription} (Original prescription which has been 9421 * superseded by this prescription to support the dispensing of pharmacy 9422 * services, medications or products.) 9423 */ 9424 public Reference getOriginalPrescription() { 9425 if (this.originalPrescription == null) 9426 if (Configuration.errorOnAutoCreate()) 9427 throw new Error("Attempt to auto-create Claim.originalPrescription"); 9428 else if (Configuration.doAutoCreate()) 9429 this.originalPrescription = new Reference(); // cc 9430 return this.originalPrescription; 9431 } 9432 9433 public boolean hasOriginalPrescription() { 9434 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 9435 } 9436 9437 /** 9438 * @param value {@link #originalPrescription} (Original prescription which has 9439 * been superseded by this prescription to support the dispensing 9440 * of pharmacy services, medications or products.) 9441 */ 9442 public Claim setOriginalPrescription(Reference value) { 9443 this.originalPrescription = value; 9444 return this; 9445 } 9446 9447 /** 9448 * @return {@link #originalPrescription} The actual object that is the target of 9449 * the reference. The reference library doesn't populate this, but you 9450 * can use it to hold the resource if you resolve it. (Original 9451 * prescription which has been superseded by this prescription to 9452 * support the dispensing of pharmacy services, medications or 9453 * products.) 9454 */ 9455 public Resource getOriginalPrescriptionTarget() { 9456 return this.originalPrescriptionTarget; 9457 } 9458 9459 /** 9460 * @param value {@link #originalPrescription} The actual object that is the 9461 * target of the reference. The reference library doesn't use 9462 * these, but you can use it to hold the resource if you resolve 9463 * it. (Original prescription which has been superseded by this 9464 * prescription to support the dispensing of pharmacy services, 9465 * medications or products.) 9466 */ 9467 public Claim setOriginalPrescriptionTarget(Resource value) { 9468 this.originalPrescriptionTarget = value; 9469 return this; 9470 } 9471 9472 /** 9473 * @return {@link #payee} (The party to be reimbursed for cost of the products 9474 * and services according to the terms of the policy.) 9475 */ 9476 public PayeeComponent getPayee() { 9477 if (this.payee == null) 9478 if (Configuration.errorOnAutoCreate()) 9479 throw new Error("Attempt to auto-create Claim.payee"); 9480 else if (Configuration.doAutoCreate()) 9481 this.payee = new PayeeComponent(); // cc 9482 return this.payee; 9483 } 9484 9485 public boolean hasPayee() { 9486 return this.payee != null && !this.payee.isEmpty(); 9487 } 9488 9489 /** 9490 * @param value {@link #payee} (The party to be reimbursed for cost of the 9491 * products and services according to the terms of the policy.) 9492 */ 9493 public Claim setPayee(PayeeComponent value) { 9494 this.payee = value; 9495 return this; 9496 } 9497 9498 /** 9499 * @return {@link #referral} (A reference to a referral resource.) 9500 */ 9501 public Reference getReferral() { 9502 if (this.referral == null) 9503 if (Configuration.errorOnAutoCreate()) 9504 throw new Error("Attempt to auto-create Claim.referral"); 9505 else if (Configuration.doAutoCreate()) 9506 this.referral = new Reference(); // cc 9507 return this.referral; 9508 } 9509 9510 public boolean hasReferral() { 9511 return this.referral != null && !this.referral.isEmpty(); 9512 } 9513 9514 /** 9515 * @param value {@link #referral} (A reference to a referral resource.) 9516 */ 9517 public Claim setReferral(Reference value) { 9518 this.referral = value; 9519 return this; 9520 } 9521 9522 /** 9523 * @return {@link #referral} The actual object that is the target of the 9524 * reference. The reference library doesn't populate this, but you can 9525 * use it to hold the resource if you resolve it. (A reference to a 9526 * referral resource.) 9527 */ 9528 public ServiceRequest getReferralTarget() { 9529 if (this.referralTarget == null) 9530 if (Configuration.errorOnAutoCreate()) 9531 throw new Error("Attempt to auto-create Claim.referral"); 9532 else if (Configuration.doAutoCreate()) 9533 this.referralTarget = new ServiceRequest(); // aa 9534 return this.referralTarget; 9535 } 9536 9537 /** 9538 * @param value {@link #referral} The actual object that is the target of the 9539 * reference. The reference library doesn't use these, but you can 9540 * use it to hold the resource if you resolve it. (A reference to a 9541 * referral resource.) 9542 */ 9543 public Claim setReferralTarget(ServiceRequest value) { 9544 this.referralTarget = value; 9545 return this; 9546 } 9547 9548 /** 9549 * @return {@link #facility} (Facility where the services were provided.) 9550 */ 9551 public Reference getFacility() { 9552 if (this.facility == null) 9553 if (Configuration.errorOnAutoCreate()) 9554 throw new Error("Attempt to auto-create Claim.facility"); 9555 else if (Configuration.doAutoCreate()) 9556 this.facility = new Reference(); // cc 9557 return this.facility; 9558 } 9559 9560 public boolean hasFacility() { 9561 return this.facility != null && !this.facility.isEmpty(); 9562 } 9563 9564 /** 9565 * @param value {@link #facility} (Facility where the services were provided.) 9566 */ 9567 public Claim setFacility(Reference value) { 9568 this.facility = value; 9569 return this; 9570 } 9571 9572 /** 9573 * @return {@link #facility} The actual object that is the target of the 9574 * reference. The reference library doesn't populate this, but you can 9575 * use it to hold the resource if you resolve it. (Facility where the 9576 * services were provided.) 9577 */ 9578 public Location getFacilityTarget() { 9579 if (this.facilityTarget == null) 9580 if (Configuration.errorOnAutoCreate()) 9581 throw new Error("Attempt to auto-create Claim.facility"); 9582 else if (Configuration.doAutoCreate()) 9583 this.facilityTarget = new Location(); // aa 9584 return this.facilityTarget; 9585 } 9586 9587 /** 9588 * @param value {@link #facility} The actual object that is the target of the 9589 * reference. The reference library doesn't use these, but you can 9590 * use it to hold the resource if you resolve it. (Facility where 9591 * the services were provided.) 9592 */ 9593 public Claim setFacilityTarget(Location value) { 9594 this.facilityTarget = value; 9595 return this; 9596 } 9597 9598 /** 9599 * @return {@link #careTeam} (The members of the team who provided the products 9600 * and services.) 9601 */ 9602 public List<CareTeamComponent> getCareTeam() { 9603 if (this.careTeam == null) 9604 this.careTeam = new ArrayList<CareTeamComponent>(); 9605 return this.careTeam; 9606 } 9607 9608 /** 9609 * @return Returns a reference to <code>this</code> for easy method chaining 9610 */ 9611 public Claim setCareTeam(List<CareTeamComponent> theCareTeam) { 9612 this.careTeam = theCareTeam; 9613 return this; 9614 } 9615 9616 public boolean hasCareTeam() { 9617 if (this.careTeam == null) 9618 return false; 9619 for (CareTeamComponent item : this.careTeam) 9620 if (!item.isEmpty()) 9621 return true; 9622 return false; 9623 } 9624 9625 public CareTeamComponent addCareTeam() { // 3 9626 CareTeamComponent t = new CareTeamComponent(); 9627 if (this.careTeam == null) 9628 this.careTeam = new ArrayList<CareTeamComponent>(); 9629 this.careTeam.add(t); 9630 return t; 9631 } 9632 9633 public Claim addCareTeam(CareTeamComponent t) { // 3 9634 if (t == null) 9635 return this; 9636 if (this.careTeam == null) 9637 this.careTeam = new ArrayList<CareTeamComponent>(); 9638 this.careTeam.add(t); 9639 return this; 9640 } 9641 9642 /** 9643 * @return The first repetition of repeating field {@link #careTeam}, creating 9644 * it if it does not already exist 9645 */ 9646 public CareTeamComponent getCareTeamFirstRep() { 9647 if (getCareTeam().isEmpty()) { 9648 addCareTeam(); 9649 } 9650 return getCareTeam().get(0); 9651 } 9652 9653 /** 9654 * @return {@link #supportingInfo} (Additional information codes regarding 9655 * exceptions, special considerations, the condition, situation, prior 9656 * or concurrent issues.) 9657 */ 9658 public List<SupportingInformationComponent> getSupportingInfo() { 9659 if (this.supportingInfo == null) 9660 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9661 return this.supportingInfo; 9662 } 9663 9664 /** 9665 * @return Returns a reference to <code>this</code> for easy method chaining 9666 */ 9667 public Claim setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 9668 this.supportingInfo = theSupportingInfo; 9669 return this; 9670 } 9671 9672 public boolean hasSupportingInfo() { 9673 if (this.supportingInfo == null) 9674 return false; 9675 for (SupportingInformationComponent item : this.supportingInfo) 9676 if (!item.isEmpty()) 9677 return true; 9678 return false; 9679 } 9680 9681 public SupportingInformationComponent addSupportingInfo() { // 3 9682 SupportingInformationComponent t = new SupportingInformationComponent(); 9683 if (this.supportingInfo == null) 9684 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9685 this.supportingInfo.add(t); 9686 return t; 9687 } 9688 9689 public Claim addSupportingInfo(SupportingInformationComponent t) { // 3 9690 if (t == null) 9691 return this; 9692 if (this.supportingInfo == null) 9693 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9694 this.supportingInfo.add(t); 9695 return this; 9696 } 9697 9698 /** 9699 * @return The first repetition of repeating field {@link #supportingInfo}, 9700 * creating it if it does not already exist 9701 */ 9702 public SupportingInformationComponent getSupportingInfoFirstRep() { 9703 if (getSupportingInfo().isEmpty()) { 9704 addSupportingInfo(); 9705 } 9706 return getSupportingInfo().get(0); 9707 } 9708 9709 /** 9710 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim 9711 * items.) 9712 */ 9713 public List<DiagnosisComponent> getDiagnosis() { 9714 if (this.diagnosis == null) 9715 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9716 return this.diagnosis; 9717 } 9718 9719 /** 9720 * @return Returns a reference to <code>this</code> for easy method chaining 9721 */ 9722 public Claim setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 9723 this.diagnosis = theDiagnosis; 9724 return this; 9725 } 9726 9727 public boolean hasDiagnosis() { 9728 if (this.diagnosis == null) 9729 return false; 9730 for (DiagnosisComponent item : this.diagnosis) 9731 if (!item.isEmpty()) 9732 return true; 9733 return false; 9734 } 9735 9736 public DiagnosisComponent addDiagnosis() { // 3 9737 DiagnosisComponent t = new DiagnosisComponent(); 9738 if (this.diagnosis == null) 9739 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9740 this.diagnosis.add(t); 9741 return t; 9742 } 9743 9744 public Claim addDiagnosis(DiagnosisComponent t) { // 3 9745 if (t == null) 9746 return this; 9747 if (this.diagnosis == null) 9748 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9749 this.diagnosis.add(t); 9750 return this; 9751 } 9752 9753 /** 9754 * @return The first repetition of repeating field {@link #diagnosis}, creating 9755 * it if it does not already exist 9756 */ 9757 public DiagnosisComponent getDiagnosisFirstRep() { 9758 if (getDiagnosis().isEmpty()) { 9759 addDiagnosis(); 9760 } 9761 return getDiagnosis().get(0); 9762 } 9763 9764 /** 9765 * @return {@link #procedure} (Procedures performed on the patient relevant to 9766 * the billing items with the claim.) 9767 */ 9768 public List<ProcedureComponent> getProcedure() { 9769 if (this.procedure == null) 9770 this.procedure = new ArrayList<ProcedureComponent>(); 9771 return this.procedure; 9772 } 9773 9774 /** 9775 * @return Returns a reference to <code>this</code> for easy method chaining 9776 */ 9777 public Claim setProcedure(List<ProcedureComponent> theProcedure) { 9778 this.procedure = theProcedure; 9779 return this; 9780 } 9781 9782 public boolean hasProcedure() { 9783 if (this.procedure == null) 9784 return false; 9785 for (ProcedureComponent item : this.procedure) 9786 if (!item.isEmpty()) 9787 return true; 9788 return false; 9789 } 9790 9791 public ProcedureComponent addProcedure() { // 3 9792 ProcedureComponent t = new ProcedureComponent(); 9793 if (this.procedure == null) 9794 this.procedure = new ArrayList<ProcedureComponent>(); 9795 this.procedure.add(t); 9796 return t; 9797 } 9798 9799 public Claim addProcedure(ProcedureComponent t) { // 3 9800 if (t == null) 9801 return this; 9802 if (this.procedure == null) 9803 this.procedure = new ArrayList<ProcedureComponent>(); 9804 this.procedure.add(t); 9805 return this; 9806 } 9807 9808 /** 9809 * @return The first repetition of repeating field {@link #procedure}, creating 9810 * it if it does not already exist 9811 */ 9812 public ProcedureComponent getProcedureFirstRep() { 9813 if (getProcedure().isEmpty()) { 9814 addProcedure(); 9815 } 9816 return getProcedure().get(0); 9817 } 9818 9819 /** 9820 * @return {@link #insurance} (Financial instruments for reimbursement for the 9821 * health care products and services specified on the claim.) 9822 */ 9823 public List<InsuranceComponent> getInsurance() { 9824 if (this.insurance == null) 9825 this.insurance = new ArrayList<InsuranceComponent>(); 9826 return this.insurance; 9827 } 9828 9829 /** 9830 * @return Returns a reference to <code>this</code> for easy method chaining 9831 */ 9832 public Claim setInsurance(List<InsuranceComponent> theInsurance) { 9833 this.insurance = theInsurance; 9834 return this; 9835 } 9836 9837 public boolean hasInsurance() { 9838 if (this.insurance == null) 9839 return false; 9840 for (InsuranceComponent item : this.insurance) 9841 if (!item.isEmpty()) 9842 return true; 9843 return false; 9844 } 9845 9846 public InsuranceComponent addInsurance() { // 3 9847 InsuranceComponent t = new InsuranceComponent(); 9848 if (this.insurance == null) 9849 this.insurance = new ArrayList<InsuranceComponent>(); 9850 this.insurance.add(t); 9851 return t; 9852 } 9853 9854 public Claim addInsurance(InsuranceComponent t) { // 3 9855 if (t == null) 9856 return this; 9857 if (this.insurance == null) 9858 this.insurance = new ArrayList<InsuranceComponent>(); 9859 this.insurance.add(t); 9860 return this; 9861 } 9862 9863 /** 9864 * @return The first repetition of repeating field {@link #insurance}, creating 9865 * it if it does not already exist 9866 */ 9867 public InsuranceComponent getInsuranceFirstRep() { 9868 if (getInsurance().isEmpty()) { 9869 addInsurance(); 9870 } 9871 return getInsurance().get(0); 9872 } 9873 9874 /** 9875 * @return {@link #accident} (Details of an accident which resulted in injuries 9876 * which required the products and services listed in the claim.) 9877 */ 9878 public AccidentComponent getAccident() { 9879 if (this.accident == null) 9880 if (Configuration.errorOnAutoCreate()) 9881 throw new Error("Attempt to auto-create Claim.accident"); 9882 else if (Configuration.doAutoCreate()) 9883 this.accident = new AccidentComponent(); // cc 9884 return this.accident; 9885 } 9886 9887 public boolean hasAccident() { 9888 return this.accident != null && !this.accident.isEmpty(); 9889 } 9890 9891 /** 9892 * @param value {@link #accident} (Details of an accident which resulted in 9893 * injuries which required the products and services listed in the 9894 * claim.) 9895 */ 9896 public Claim setAccident(AccidentComponent value) { 9897 this.accident = value; 9898 return this; 9899 } 9900 9901 /** 9902 * @return {@link #item} (A claim line. Either a simple product or service or a 9903 * 'group' of details which can each be a simple items or groups of 9904 * sub-details.) 9905 */ 9906 public List<ItemComponent> getItem() { 9907 if (this.item == null) 9908 this.item = new ArrayList<ItemComponent>(); 9909 return this.item; 9910 } 9911 9912 /** 9913 * @return Returns a reference to <code>this</code> for easy method chaining 9914 */ 9915 public Claim setItem(List<ItemComponent> theItem) { 9916 this.item = theItem; 9917 return this; 9918 } 9919 9920 public boolean hasItem() { 9921 if (this.item == null) 9922 return false; 9923 for (ItemComponent item : this.item) 9924 if (!item.isEmpty()) 9925 return true; 9926 return false; 9927 } 9928 9929 public ItemComponent addItem() { // 3 9930 ItemComponent t = new ItemComponent(); 9931 if (this.item == null) 9932 this.item = new ArrayList<ItemComponent>(); 9933 this.item.add(t); 9934 return t; 9935 } 9936 9937 public Claim addItem(ItemComponent t) { // 3 9938 if (t == null) 9939 return this; 9940 if (this.item == null) 9941 this.item = new ArrayList<ItemComponent>(); 9942 this.item.add(t); 9943 return this; 9944 } 9945 9946 /** 9947 * @return The first repetition of repeating field {@link #item}, creating it if 9948 * it does not already exist 9949 */ 9950 public ItemComponent getItemFirstRep() { 9951 if (getItem().isEmpty()) { 9952 addItem(); 9953 } 9954 return getItem().get(0); 9955 } 9956 9957 /** 9958 * @return {@link #total} (The total value of the all the items in the claim.) 9959 */ 9960 public Money getTotal() { 9961 if (this.total == null) 9962 if (Configuration.errorOnAutoCreate()) 9963 throw new Error("Attempt to auto-create Claim.total"); 9964 else if (Configuration.doAutoCreate()) 9965 this.total = new Money(); // cc 9966 return this.total; 9967 } 9968 9969 public boolean hasTotal() { 9970 return this.total != null && !this.total.isEmpty(); 9971 } 9972 9973 /** 9974 * @param value {@link #total} (The total value of the all the items in the 9975 * claim.) 9976 */ 9977 public Claim setTotal(Money value) { 9978 this.total = value; 9979 return this; 9980 } 9981 9982 protected void listChildren(List<Property> children) { 9983 super.listChildren(children); 9984 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, 9985 java.lang.Integer.MAX_VALUE, identifier)); 9986 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 9987 children.add(new Property("type", "CodeableConcept", 9988 "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 9989 children.add(new Property("subType", "CodeableConcept", 9990 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9991 0, 1, subType)); 9992 children.add(new Property("use", "code", 9993 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 9994 0, 1, use)); 9995 children.add(new Property("patient", "Reference(Patient)", 9996 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 9997 0, 1, patient)); 9998 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, 9999 billablePeriod)); 10000 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 10001 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole)", 10002 "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 10003 children.add( 10004 new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 10005 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 10006 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 10007 children.add(new Property("priority", "CodeableConcept", 10008 "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.", 0, 10009 1, priority)); 10010 children.add(new Property("fundsReserve", "CodeableConcept", 10011 "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve)); 10012 children.add(new Property("related", "", 10013 "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 10014 0, java.lang.Integer.MAX_VALUE, related)); 10015 children.add(new Property("prescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10016 "Prescription to support the dispensing of pharmacy, device or vision products.", 0, 1, prescription)); 10017 children.add(new Property("originalPrescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10018 "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 10019 0, 1, originalPrescription)); 10020 children.add(new Property("payee", "", 10021 "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, 10022 payee)); 10023 children.add( 10024 new Property("referral", "Reference(ServiceRequest)", "A reference to a referral resource.", 0, 1, referral)); 10025 children.add( 10026 new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 10027 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, 10028 java.lang.Integer.MAX_VALUE, careTeam)); 10029 children.add(new Property("supportingInfo", "", 10030 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 10031 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 10032 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, 10033 java.lang.Integer.MAX_VALUE, diagnosis)); 10034 children.add(new Property("procedure", "", 10035 "Procedures performed on the patient relevant to the billing items with the claim.", 0, 10036 java.lang.Integer.MAX_VALUE, procedure)); 10037 children.add(new Property("insurance", "", 10038 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, 10039 java.lang.Integer.MAX_VALUE, insurance)); 10040 children.add(new Property("accident", "", 10041 "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 10042 0, 1, accident)); 10043 children.add(new Property("item", "", 10044 "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 10045 0, java.lang.Integer.MAX_VALUE, item)); 10046 children.add(new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, total)); 10047 } 10048 10049 @Override 10050 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10051 switch (_hash) { 10052 case -1618432855: 10053 /* identifier */ return new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, 10054 java.lang.Integer.MAX_VALUE, identifier); 10055 case -892481550: 10056 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 10057 case 3575610: 10058 /* type */ return new Property("type", "CodeableConcept", 10059 "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 10060 case -1868521062: 10061 /* subType */ return new Property("subType", "CodeableConcept", 10062 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 10063 0, 1, subType); 10064 case 116103: 10065 /* use */ return new Property("use", "code", 10066 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 10067 0, 1, use); 10068 case -791418107: 10069 /* patient */ return new Property("patient", "Reference(Patient)", 10070 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 10071 0, 1, patient); 10072 case -332066046: 10073 /* billablePeriod */ return new Property("billablePeriod", "Period", 10074 "The period for which charges are being submitted.", 0, 1, billablePeriod); 10075 case 1028554472: 10076 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 10077 case -1591951995: 10078 /* enterer */ return new Property("enterer", "Reference(Practitioner|PractitionerRole)", 10079 "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 10080 case 1957615864: 10081 /* insurer */ return new Property("insurer", "Reference(Organization)", 10082 "The Insurer who is target of the request.", 0, 1, insurer); 10083 case -987494927: 10084 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 10085 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 10086 case -1165461084: 10087 /* priority */ return new Property("priority", "CodeableConcept", 10088 "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.", 0, 10089 1, priority); 10090 case 1314609806: 10091 /* fundsReserve */ return new Property("fundsReserve", "CodeableConcept", 10092 "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve); 10093 case 1090493483: 10094 /* related */ return new Property("related", "", 10095 "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 10096 0, java.lang.Integer.MAX_VALUE, related); 10097 case 460301338: 10098 /* prescription */ return new Property("prescription", 10099 "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10100 "Prescription to support the dispensing of pharmacy, device or vision products.", 0, 1, prescription); 10101 case -1814015861: 10102 /* originalPrescription */ return new Property("originalPrescription", 10103 "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10104 "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 10105 0, 1, originalPrescription); 10106 case 106443592: 10107 /* payee */ return new Property("payee", "", 10108 "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 10109 1, payee); 10110 case -722568291: 10111 /* referral */ return new Property("referral", "Reference(ServiceRequest)", "A reference to a referral resource.", 10112 0, 1, referral); 10113 case 501116579: 10114 /* facility */ return new Property("facility", "Reference(Location)", 10115 "Facility where the services were provided.", 0, 1, facility); 10116 case -7323378: 10117 /* careTeam */ return new Property("careTeam", "", 10118 "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 10119 case 1922406657: 10120 /* supportingInfo */ return new Property("supportingInfo", "", 10121 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 10122 0, java.lang.Integer.MAX_VALUE, supportingInfo); 10123 case 1196993265: 10124 /* diagnosis */ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 10125 0, java.lang.Integer.MAX_VALUE, diagnosis); 10126 case -1095204141: 10127 /* procedure */ return new Property("procedure", "", 10128 "Procedures performed on the patient relevant to the billing items with the claim.", 0, 10129 java.lang.Integer.MAX_VALUE, procedure); 10130 case 73049818: 10131 /* insurance */ return new Property("insurance", "", 10132 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 10133 0, java.lang.Integer.MAX_VALUE, insurance); 10134 case -2143202801: 10135 /* accident */ return new Property("accident", "", 10136 "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 10137 0, 1, accident); 10138 case 3242771: 10139 /* item */ return new Property("item", "", 10140 "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 10141 0, java.lang.Integer.MAX_VALUE, item); 10142 case 110549828: 10143 /* total */ return new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, 10144 total); 10145 default: 10146 return super.getNamedProperty(_hash, _name, _checkValid); 10147 } 10148 10149 } 10150 10151 @Override 10152 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10153 switch (hash) { 10154 case -1618432855: 10155 /* identifier */ return this.identifier == null ? new Base[0] 10156 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 10157 case -892481550: 10158 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ClaimStatus> 10159 case 3575610: 10160 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 10161 case -1868521062: 10162 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 10163 case 116103: 10164 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<Use> 10165 case -791418107: 10166 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 10167 case -332066046: 10168 /* billablePeriod */ return this.billablePeriod == null ? new Base[0] : new Base[] { this.billablePeriod }; // Period 10169 case 1028554472: 10170 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 10171 case -1591951995: 10172 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 10173 case 1957615864: 10174 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 10175 case -987494927: 10176 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 10177 case -1165461084: 10178 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 10179 case 1314609806: 10180 /* fundsReserve */ return this.fundsReserve == null ? new Base[0] : new Base[] { this.fundsReserve }; // CodeableConcept 10181 case 1090493483: 10182 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 10183 case 460301338: 10184 /* prescription */ return this.prescription == null ? new Base[0] : new Base[] { this.prescription }; // Reference 10185 case -1814015861: 10186 /* originalPrescription */ return this.originalPrescription == null ? new Base[0] 10187 : new Base[] { this.originalPrescription }; // Reference 10188 case 106443592: 10189 /* payee */ return this.payee == null ? new Base[0] : new Base[] { this.payee }; // PayeeComponent 10190 case -722568291: 10191 /* referral */ return this.referral == null ? new Base[0] : new Base[] { this.referral }; // Reference 10192 case 501116579: 10193 /* facility */ return this.facility == null ? new Base[0] : new Base[] { this.facility }; // Reference 10194 case -7323378: 10195 /* careTeam */ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 10196 case 1922406657: 10197 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 10198 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 10199 case 1196993265: 10200 /* diagnosis */ return this.diagnosis == null ? new Base[0] 10201 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 10202 case -1095204141: 10203 /* procedure */ return this.procedure == null ? new Base[0] 10204 : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 10205 case 73049818: 10206 /* insurance */ return this.insurance == null ? new Base[0] 10207 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 10208 case -2143202801: 10209 /* accident */ return this.accident == null ? new Base[0] : new Base[] { this.accident }; // AccidentComponent 10210 case 3242771: 10211 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 10212 case 110549828: 10213 /* total */ return this.total == null ? new Base[0] : new Base[] { this.total }; // Money 10214 default: 10215 return super.getProperty(hash, name, checkValid); 10216 } 10217 10218 } 10219 10220 @Override 10221 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10222 switch (hash) { 10223 case -1618432855: // identifier 10224 this.getIdentifier().add(castToIdentifier(value)); // Identifier 10225 return value; 10226 case -892481550: // status 10227 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 10228 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 10229 return value; 10230 case 3575610: // type 10231 this.type = castToCodeableConcept(value); // CodeableConcept 10232 return value; 10233 case -1868521062: // subType 10234 this.subType = castToCodeableConcept(value); // CodeableConcept 10235 return value; 10236 case 116103: // use 10237 value = new UseEnumFactory().fromType(castToCode(value)); 10238 this.use = (Enumeration) value; // Enumeration<Use> 10239 return value; 10240 case -791418107: // patient 10241 this.patient = castToReference(value); // Reference 10242 return value; 10243 case -332066046: // billablePeriod 10244 this.billablePeriod = castToPeriod(value); // Period 10245 return value; 10246 case 1028554472: // created 10247 this.created = castToDateTime(value); // DateTimeType 10248 return value; 10249 case -1591951995: // enterer 10250 this.enterer = castToReference(value); // Reference 10251 return value; 10252 case 1957615864: // insurer 10253 this.insurer = castToReference(value); // Reference 10254 return value; 10255 case -987494927: // provider 10256 this.provider = castToReference(value); // Reference 10257 return value; 10258 case -1165461084: // priority 10259 this.priority = castToCodeableConcept(value); // CodeableConcept 10260 return value; 10261 case 1314609806: // fundsReserve 10262 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 10263 return value; 10264 case 1090493483: // related 10265 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 10266 return value; 10267 case 460301338: // prescription 10268 this.prescription = castToReference(value); // Reference 10269 return value; 10270 case -1814015861: // originalPrescription 10271 this.originalPrescription = castToReference(value); // Reference 10272 return value; 10273 case 106443592: // payee 10274 this.payee = (PayeeComponent) value; // PayeeComponent 10275 return value; 10276 case -722568291: // referral 10277 this.referral = castToReference(value); // Reference 10278 return value; 10279 case 501116579: // facility 10280 this.facility = castToReference(value); // Reference 10281 return value; 10282 case -7323378: // careTeam 10283 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 10284 return value; 10285 case 1922406657: // supportingInfo 10286 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 10287 return value; 10288 case 1196993265: // diagnosis 10289 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 10290 return value; 10291 case -1095204141: // procedure 10292 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 10293 return value; 10294 case 73049818: // insurance 10295 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 10296 return value; 10297 case -2143202801: // accident 10298 this.accident = (AccidentComponent) value; // AccidentComponent 10299 return value; 10300 case 3242771: // item 10301 this.getItem().add((ItemComponent) value); // ItemComponent 10302 return value; 10303 case 110549828: // total 10304 this.total = castToMoney(value); // Money 10305 return value; 10306 default: 10307 return super.setProperty(hash, name, value); 10308 } 10309 10310 } 10311 10312 @Override 10313 public Base setProperty(String name, Base value) throws FHIRException { 10314 if (name.equals("identifier")) { 10315 this.getIdentifier().add(castToIdentifier(value)); 10316 } else if (name.equals("status")) { 10317 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 10318 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 10319 } else if (name.equals("type")) { 10320 this.type = castToCodeableConcept(value); // CodeableConcept 10321 } else if (name.equals("subType")) { 10322 this.subType = castToCodeableConcept(value); // CodeableConcept 10323 } else if (name.equals("use")) { 10324 value = new UseEnumFactory().fromType(castToCode(value)); 10325 this.use = (Enumeration) value; // Enumeration<Use> 10326 } else if (name.equals("patient")) { 10327 this.patient = castToReference(value); // Reference 10328 } else if (name.equals("billablePeriod")) { 10329 this.billablePeriod = castToPeriod(value); // Period 10330 } else if (name.equals("created")) { 10331 this.created = castToDateTime(value); // DateTimeType 10332 } else if (name.equals("enterer")) { 10333 this.enterer = castToReference(value); // Reference 10334 } else if (name.equals("insurer")) { 10335 this.insurer = castToReference(value); // Reference 10336 } else if (name.equals("provider")) { 10337 this.provider = castToReference(value); // Reference 10338 } else if (name.equals("priority")) { 10339 this.priority = castToCodeableConcept(value); // CodeableConcept 10340 } else if (name.equals("fundsReserve")) { 10341 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 10342 } else if (name.equals("related")) { 10343 this.getRelated().add((RelatedClaimComponent) value); 10344 } else if (name.equals("prescription")) { 10345 this.prescription = castToReference(value); // Reference 10346 } else if (name.equals("originalPrescription")) { 10347 this.originalPrescription = castToReference(value); // Reference 10348 } else if (name.equals("payee")) { 10349 this.payee = (PayeeComponent) value; // PayeeComponent 10350 } else if (name.equals("referral")) { 10351 this.referral = castToReference(value); // Reference 10352 } else if (name.equals("facility")) { 10353 this.facility = castToReference(value); // Reference 10354 } else if (name.equals("careTeam")) { 10355 this.getCareTeam().add((CareTeamComponent) value); 10356 } else if (name.equals("supportingInfo")) { 10357 this.getSupportingInfo().add((SupportingInformationComponent) value); 10358 } else if (name.equals("diagnosis")) { 10359 this.getDiagnosis().add((DiagnosisComponent) value); 10360 } else if (name.equals("procedure")) { 10361 this.getProcedure().add((ProcedureComponent) value); 10362 } else if (name.equals("insurance")) { 10363 this.getInsurance().add((InsuranceComponent) value); 10364 } else if (name.equals("accident")) { 10365 this.accident = (AccidentComponent) value; // AccidentComponent 10366 } else if (name.equals("item")) { 10367 this.getItem().add((ItemComponent) value); 10368 } else if (name.equals("total")) { 10369 this.total = castToMoney(value); // Money 10370 } else 10371 return super.setProperty(name, value); 10372 return value; 10373 } 10374 10375 @Override 10376 public void removeChild(String name, Base value) throws FHIRException { 10377 if (name.equals("identifier")) { 10378 this.getIdentifier().remove(castToIdentifier(value)); 10379 } else if (name.equals("status")) { 10380 this.status = null; 10381 } else if (name.equals("type")) { 10382 this.type = null; 10383 } else if (name.equals("subType")) { 10384 this.subType = null; 10385 } else if (name.equals("use")) { 10386 this.use = null; 10387 } else if (name.equals("patient")) { 10388 this.patient = null; 10389 } else if (name.equals("billablePeriod")) { 10390 this.billablePeriod = null; 10391 } else if (name.equals("created")) { 10392 this.created = null; 10393 } else if (name.equals("enterer")) { 10394 this.enterer = null; 10395 } else if (name.equals("insurer")) { 10396 this.insurer = null; 10397 } else if (name.equals("provider")) { 10398 this.provider = null; 10399 } else if (name.equals("priority")) { 10400 this.priority = null; 10401 } else if (name.equals("fundsReserve")) { 10402 this.fundsReserve = null; 10403 } else if (name.equals("related")) { 10404 this.getRelated().remove((RelatedClaimComponent) value); 10405 } else if (name.equals("prescription")) { 10406 this.prescription = null; 10407 } else if (name.equals("originalPrescription")) { 10408 this.originalPrescription = null; 10409 } else if (name.equals("payee")) { 10410 this.payee = (PayeeComponent) value; // PayeeComponent 10411 } else if (name.equals("referral")) { 10412 this.referral = null; 10413 } else if (name.equals("facility")) { 10414 this.facility = null; 10415 } else if (name.equals("careTeam")) { 10416 this.getCareTeam().remove((CareTeamComponent) value); 10417 } else if (name.equals("supportingInfo")) { 10418 this.getSupportingInfo().remove((SupportingInformationComponent) value); 10419 } else if (name.equals("diagnosis")) { 10420 this.getDiagnosis().remove((DiagnosisComponent) value); 10421 } else if (name.equals("procedure")) { 10422 this.getProcedure().remove((ProcedureComponent) value); 10423 } else if (name.equals("insurance")) { 10424 this.getInsurance().remove((InsuranceComponent) value); 10425 } else if (name.equals("accident")) { 10426 this.accident = (AccidentComponent) value; // AccidentComponent 10427 } else if (name.equals("item")) { 10428 this.getItem().remove((ItemComponent) value); 10429 } else if (name.equals("total")) { 10430 this.total = null; 10431 } else 10432 super.removeChild(name, value); 10433 10434 } 10435 10436 @Override 10437 public Base makeProperty(int hash, String name) throws FHIRException { 10438 switch (hash) { 10439 case -1618432855: 10440 return addIdentifier(); 10441 case -892481550: 10442 return getStatusElement(); 10443 case 3575610: 10444 return getType(); 10445 case -1868521062: 10446 return getSubType(); 10447 case 116103: 10448 return getUseElement(); 10449 case -791418107: 10450 return getPatient(); 10451 case -332066046: 10452 return getBillablePeriod(); 10453 case 1028554472: 10454 return getCreatedElement(); 10455 case -1591951995: 10456 return getEnterer(); 10457 case 1957615864: 10458 return getInsurer(); 10459 case -987494927: 10460 return getProvider(); 10461 case -1165461084: 10462 return getPriority(); 10463 case 1314609806: 10464 return getFundsReserve(); 10465 case 1090493483: 10466 return addRelated(); 10467 case 460301338: 10468 return getPrescription(); 10469 case -1814015861: 10470 return getOriginalPrescription(); 10471 case 106443592: 10472 return getPayee(); 10473 case -722568291: 10474 return getReferral(); 10475 case 501116579: 10476 return getFacility(); 10477 case -7323378: 10478 return addCareTeam(); 10479 case 1922406657: 10480 return addSupportingInfo(); 10481 case 1196993265: 10482 return addDiagnosis(); 10483 case -1095204141: 10484 return addProcedure(); 10485 case 73049818: 10486 return addInsurance(); 10487 case -2143202801: 10488 return getAccident(); 10489 case 3242771: 10490 return addItem(); 10491 case 110549828: 10492 return getTotal(); 10493 default: 10494 return super.makeProperty(hash, name); 10495 } 10496 10497 } 10498 10499 @Override 10500 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10501 switch (hash) { 10502 case -1618432855: 10503 /* identifier */ return new String[] { "Identifier" }; 10504 case -892481550: 10505 /* status */ return new String[] { "code" }; 10506 case 3575610: 10507 /* type */ return new String[] { "CodeableConcept" }; 10508 case -1868521062: 10509 /* subType */ return new String[] { "CodeableConcept" }; 10510 case 116103: 10511 /* use */ return new String[] { "code" }; 10512 case -791418107: 10513 /* patient */ return new String[] { "Reference" }; 10514 case -332066046: 10515 /* billablePeriod */ return new String[] { "Period" }; 10516 case 1028554472: 10517 /* created */ return new String[] { "dateTime" }; 10518 case -1591951995: 10519 /* enterer */ return new String[] { "Reference" }; 10520 case 1957615864: 10521 /* insurer */ return new String[] { "Reference" }; 10522 case -987494927: 10523 /* provider */ return new String[] { "Reference" }; 10524 case -1165461084: 10525 /* priority */ return new String[] { "CodeableConcept" }; 10526 case 1314609806: 10527 /* fundsReserve */ return new String[] { "CodeableConcept" }; 10528 case 1090493483: 10529 /* related */ return new String[] {}; 10530 case 460301338: 10531 /* prescription */ return new String[] { "Reference" }; 10532 case -1814015861: 10533 /* originalPrescription */ return new String[] { "Reference" }; 10534 case 106443592: 10535 /* payee */ return new String[] {}; 10536 case -722568291: 10537 /* referral */ return new String[] { "Reference" }; 10538 case 501116579: 10539 /* facility */ return new String[] { "Reference" }; 10540 case -7323378: 10541 /* careTeam */ return new String[] {}; 10542 case 1922406657: 10543 /* supportingInfo */ return new String[] {}; 10544 case 1196993265: 10545 /* diagnosis */ return new String[] {}; 10546 case -1095204141: 10547 /* procedure */ return new String[] {}; 10548 case 73049818: 10549 /* insurance */ return new String[] {}; 10550 case -2143202801: 10551 /* accident */ return new String[] {}; 10552 case 3242771: 10553 /* item */ return new String[] {}; 10554 case 110549828: 10555 /* total */ return new String[] { "Money" }; 10556 default: 10557 return super.getTypesForProperty(hash, name); 10558 } 10559 10560 } 10561 10562 @Override 10563 public Base addChild(String name) throws FHIRException { 10564 if (name.equals("identifier")) { 10565 return addIdentifier(); 10566 } else if (name.equals("status")) { 10567 throw new FHIRException("Cannot call addChild on a singleton property Claim.status"); 10568 } else if (name.equals("type")) { 10569 this.type = new CodeableConcept(); 10570 return this.type; 10571 } else if (name.equals("subType")) { 10572 this.subType = new CodeableConcept(); 10573 return this.subType; 10574 } else if (name.equals("use")) { 10575 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 10576 } else if (name.equals("patient")) { 10577 this.patient = new Reference(); 10578 return this.patient; 10579 } else if (name.equals("billablePeriod")) { 10580 this.billablePeriod = new Period(); 10581 return this.billablePeriod; 10582 } else if (name.equals("created")) { 10583 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 10584 } else if (name.equals("enterer")) { 10585 this.enterer = new Reference(); 10586 return this.enterer; 10587 } else if (name.equals("insurer")) { 10588 this.insurer = new Reference(); 10589 return this.insurer; 10590 } else if (name.equals("provider")) { 10591 this.provider = new Reference(); 10592 return this.provider; 10593 } else if (name.equals("priority")) { 10594 this.priority = new CodeableConcept(); 10595 return this.priority; 10596 } else if (name.equals("fundsReserve")) { 10597 this.fundsReserve = new CodeableConcept(); 10598 return this.fundsReserve; 10599 } else if (name.equals("related")) { 10600 return addRelated(); 10601 } else if (name.equals("prescription")) { 10602 this.prescription = new Reference(); 10603 return this.prescription; 10604 } else if (name.equals("originalPrescription")) { 10605 this.originalPrescription = new Reference(); 10606 return this.originalPrescription; 10607 } else if (name.equals("payee")) { 10608 this.payee = new PayeeComponent(); 10609 return this.payee; 10610 } else if (name.equals("referral")) { 10611 this.referral = new Reference(); 10612 return this.referral; 10613 } else if (name.equals("facility")) { 10614 this.facility = new Reference(); 10615 return this.facility; 10616 } else if (name.equals("careTeam")) { 10617 return addCareTeam(); 10618 } else if (name.equals("supportingInfo")) { 10619 return addSupportingInfo(); 10620 } else if (name.equals("diagnosis")) { 10621 return addDiagnosis(); 10622 } else if (name.equals("procedure")) { 10623 return addProcedure(); 10624 } else if (name.equals("insurance")) { 10625 return addInsurance(); 10626 } else if (name.equals("accident")) { 10627 this.accident = new AccidentComponent(); 10628 return this.accident; 10629 } else if (name.equals("item")) { 10630 return addItem(); 10631 } else if (name.equals("total")) { 10632 this.total = new Money(); 10633 return this.total; 10634 } else 10635 return super.addChild(name); 10636 } 10637 10638 public String fhirType() { 10639 return "Claim"; 10640 10641 } 10642 10643 public Claim copy() { 10644 Claim dst = new Claim(); 10645 copyValues(dst); 10646 return dst; 10647 } 10648 10649 public void copyValues(Claim dst) { 10650 super.copyValues(dst); 10651 if (identifier != null) { 10652 dst.identifier = new ArrayList<Identifier>(); 10653 for (Identifier i : identifier) 10654 dst.identifier.add(i.copy()); 10655 } 10656 ; 10657 dst.status = status == null ? null : status.copy(); 10658 dst.type = type == null ? null : type.copy(); 10659 dst.subType = subType == null ? null : subType.copy(); 10660 dst.use = use == null ? null : use.copy(); 10661 dst.patient = patient == null ? null : patient.copy(); 10662 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 10663 dst.created = created == null ? null : created.copy(); 10664 dst.enterer = enterer == null ? null : enterer.copy(); 10665 dst.insurer = insurer == null ? null : insurer.copy(); 10666 dst.provider = provider == null ? null : provider.copy(); 10667 dst.priority = priority == null ? null : priority.copy(); 10668 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 10669 if (related != null) { 10670 dst.related = new ArrayList<RelatedClaimComponent>(); 10671 for (RelatedClaimComponent i : related) 10672 dst.related.add(i.copy()); 10673 } 10674 ; 10675 dst.prescription = prescription == null ? null : prescription.copy(); 10676 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 10677 dst.payee = payee == null ? null : payee.copy(); 10678 dst.referral = referral == null ? null : referral.copy(); 10679 dst.facility = facility == null ? null : facility.copy(); 10680 if (careTeam != null) { 10681 dst.careTeam = new ArrayList<CareTeamComponent>(); 10682 for (CareTeamComponent i : careTeam) 10683 dst.careTeam.add(i.copy()); 10684 } 10685 ; 10686 if (supportingInfo != null) { 10687 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 10688 for (SupportingInformationComponent i : supportingInfo) 10689 dst.supportingInfo.add(i.copy()); 10690 } 10691 ; 10692 if (diagnosis != null) { 10693 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 10694 for (DiagnosisComponent i : diagnosis) 10695 dst.diagnosis.add(i.copy()); 10696 } 10697 ; 10698 if (procedure != null) { 10699 dst.procedure = new ArrayList<ProcedureComponent>(); 10700 for (ProcedureComponent i : procedure) 10701 dst.procedure.add(i.copy()); 10702 } 10703 ; 10704 if (insurance != null) { 10705 dst.insurance = new ArrayList<InsuranceComponent>(); 10706 for (InsuranceComponent i : insurance) 10707 dst.insurance.add(i.copy()); 10708 } 10709 ; 10710 dst.accident = accident == null ? null : accident.copy(); 10711 if (item != null) { 10712 dst.item = new ArrayList<ItemComponent>(); 10713 for (ItemComponent i : item) 10714 dst.item.add(i.copy()); 10715 } 10716 ; 10717 dst.total = total == null ? null : total.copy(); 10718 } 10719 10720 protected Claim typedCopy() { 10721 return copy(); 10722 } 10723 10724 @Override 10725 public boolean equalsDeep(Base other_) { 10726 if (!super.equalsDeep(other_)) 10727 return false; 10728 if (!(other_ instanceof Claim)) 10729 return false; 10730 Claim o = (Claim) other_; 10731 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 10732 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) 10733 && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 10734 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) 10735 && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) 10736 && compareDeep(priority, o.priority, true) && compareDeep(fundsReserve, o.fundsReserve, true) 10737 && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) 10738 && compareDeep(originalPrescription, o.originalPrescription, true) && compareDeep(payee, o.payee, true) 10739 && compareDeep(referral, o.referral, true) && compareDeep(facility, o.facility, true) 10740 && compareDeep(careTeam, o.careTeam, true) && compareDeep(supportingInfo, o.supportingInfo, true) 10741 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) 10742 && compareDeep(insurance, o.insurance, true) && compareDeep(accident, o.accident, true) 10743 && compareDeep(item, o.item, true) && compareDeep(total, o.total, true); 10744 } 10745 10746 @Override 10747 public boolean equalsShallow(Base other_) { 10748 if (!super.equalsShallow(other_)) 10749 return false; 10750 if (!(other_ instanceof Claim)) 10751 return false; 10752 Claim o = (Claim) other_; 10753 return compareValues(status, o.status, true) && compareValues(use, o.use, true) 10754 && compareValues(created, o.created, true); 10755 } 10756 10757 public boolean isEmpty() { 10758 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, subType, use, patient, 10759 billablePeriod, created, enterer, insurer, provider, priority, fundsReserve, related, prescription, 10760 originalPrescription, payee, referral, facility, careTeam, supportingInfo, diagnosis, procedure, insurance, 10761 accident, item, total); 10762 } 10763 10764 @Override 10765 public ResourceType getResourceType() { 10766 return ResourceType.Claim; 10767 } 10768 10769 /** 10770 * Search parameter: <b>care-team</b> 10771 * <p> 10772 * Description: <b>Member of the CareTeam</b><br> 10773 * Type: <b>reference</b><br> 10774 * Path: <b>Claim.careTeam.provider</b><br> 10775 * </p> 10776 */ 10777 @SearchParamDefinition(name = "care-team", path = "Claim.careTeam.provider", description = "Member of the CareTeam", type = "reference", providesMembershipIn = { 10778 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 10779 Practitioner.class, PractitionerRole.class }) 10780 public static final String SP_CARE_TEAM = "care-team"; 10781 /** 10782 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 10783 * <p> 10784 * Description: <b>Member of the CareTeam</b><br> 10785 * Type: <b>reference</b><br> 10786 * Path: <b>Claim.careTeam.provider</b><br> 10787 * </p> 10788 */ 10789 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10790 SP_CARE_TEAM); 10791 10792 /** 10793 * Constant for fluent queries to be used to add include statements. Specifies 10794 * the path value of "<b>Claim:care-team</b>". 10795 */ 10796 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include( 10797 "Claim:care-team").toLocked(); 10798 10799 /** 10800 * Search parameter: <b>identifier</b> 10801 * <p> 10802 * Description: <b>The primary identifier of the financial resource</b><br> 10803 * Type: <b>token</b><br> 10804 * Path: <b>Claim.identifier</b><br> 10805 * </p> 10806 */ 10807 @SearchParamDefinition(name = "identifier", path = "Claim.identifier", description = "The primary identifier of the financial resource", type = "token") 10808 public static final String SP_IDENTIFIER = "identifier"; 10809 /** 10810 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10811 * <p> 10812 * Description: <b>The primary identifier of the financial resource</b><br> 10813 * Type: <b>token</b><br> 10814 * Path: <b>Claim.identifier</b><br> 10815 * </p> 10816 */ 10817 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10818 SP_IDENTIFIER); 10819 10820 /** 10821 * Search parameter: <b>use</b> 10822 * <p> 10823 * Description: <b>The kind of financial resource</b><br> 10824 * Type: <b>token</b><br> 10825 * Path: <b>Claim.use</b><br> 10826 * </p> 10827 */ 10828 @SearchParamDefinition(name = "use", path = "Claim.use", description = "The kind of financial resource", type = "token") 10829 public static final String SP_USE = "use"; 10830 /** 10831 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10832 * <p> 10833 * Description: <b>The kind of financial resource</b><br> 10834 * Type: <b>token</b><br> 10835 * Path: <b>Claim.use</b><br> 10836 * </p> 10837 */ 10838 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10839 SP_USE); 10840 10841 /** 10842 * Search parameter: <b>created</b> 10843 * <p> 10844 * Description: <b>The creation date for the Claim</b><br> 10845 * Type: <b>date</b><br> 10846 * Path: <b>Claim.created</b><br> 10847 * </p> 10848 */ 10849 @SearchParamDefinition(name = "created", path = "Claim.created", description = "The creation date for the Claim", type = "date") 10850 public static final String SP_CREATED = "created"; 10851 /** 10852 * <b>Fluent Client</b> search parameter constant for <b>created</b> 10853 * <p> 10854 * Description: <b>The creation date for the Claim</b><br> 10855 * Type: <b>date</b><br> 10856 * Path: <b>Claim.created</b><br> 10857 * </p> 10858 */ 10859 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 10860 SP_CREATED); 10861 10862 /** 10863 * Search parameter: <b>encounter</b> 10864 * <p> 10865 * Description: <b>Encounters associated with a billed line item</b><br> 10866 * Type: <b>reference</b><br> 10867 * Path: <b>Claim.item.encounter</b><br> 10868 * </p> 10869 */ 10870 @SearchParamDefinition(name = "encounter", path = "Claim.item.encounter", description = "Encounters associated with a billed line item", type = "reference", providesMembershipIn = { 10871 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 10872 public static final String SP_ENCOUNTER = "encounter"; 10873 /** 10874 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 10875 * <p> 10876 * Description: <b>Encounters associated with a billed line item</b><br> 10877 * Type: <b>reference</b><br> 10878 * Path: <b>Claim.item.encounter</b><br> 10879 * </p> 10880 */ 10881 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10882 SP_ENCOUNTER); 10883 10884 /** 10885 * Constant for fluent queries to be used to add include statements. Specifies 10886 * the path value of "<b>Claim:encounter</b>". 10887 */ 10888 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 10889 "Claim:encounter").toLocked(); 10890 10891 /** 10892 * Search parameter: <b>priority</b> 10893 * <p> 10894 * Description: <b>Processing priority requested</b><br> 10895 * Type: <b>token</b><br> 10896 * Path: <b>Claim.priority</b><br> 10897 * </p> 10898 */ 10899 @SearchParamDefinition(name = "priority", path = "Claim.priority", description = "Processing priority requested", type = "token") 10900 public static final String SP_PRIORITY = "priority"; 10901 /** 10902 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 10903 * <p> 10904 * Description: <b>Processing priority requested</b><br> 10905 * Type: <b>token</b><br> 10906 * Path: <b>Claim.priority</b><br> 10907 * </p> 10908 */ 10909 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10910 SP_PRIORITY); 10911 10912 /** 10913 * Search parameter: <b>payee</b> 10914 * <p> 10915 * Description: <b>The party receiving any payment for the Claim</b><br> 10916 * Type: <b>reference</b><br> 10917 * Path: <b>Claim.payee.party</b><br> 10918 * </p> 10919 */ 10920 @SearchParamDefinition(name = "payee", path = "Claim.payee.party", description = "The party receiving any payment for the Claim", type = "reference", providesMembershipIn = { 10921 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 10922 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 10923 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 10924 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 10925 public static final String SP_PAYEE = "payee"; 10926 /** 10927 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 10928 * <p> 10929 * Description: <b>The party receiving any payment for the Claim</b><br> 10930 * Type: <b>reference</b><br> 10931 * Path: <b>Claim.payee.party</b><br> 10932 * </p> 10933 */ 10934 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10935 SP_PAYEE); 10936 10937 /** 10938 * Constant for fluent queries to be used to add include statements. Specifies 10939 * the path value of "<b>Claim:payee</b>". 10940 */ 10941 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("Claim:payee") 10942 .toLocked(); 10943 10944 /** 10945 * Search parameter: <b>provider</b> 10946 * <p> 10947 * Description: <b>Provider responsible for the Claim</b><br> 10948 * Type: <b>reference</b><br> 10949 * Path: <b>Claim.provider</b><br> 10950 * </p> 10951 */ 10952 @SearchParamDefinition(name = "provider", path = "Claim.provider", description = "Provider responsible for the Claim", type = "reference", providesMembershipIn = { 10953 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 10954 Practitioner.class, PractitionerRole.class }) 10955 public static final String SP_PROVIDER = "provider"; 10956 /** 10957 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 10958 * <p> 10959 * Description: <b>Provider responsible for the Claim</b><br> 10960 * Type: <b>reference</b><br> 10961 * Path: <b>Claim.provider</b><br> 10962 * </p> 10963 */ 10964 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10965 SP_PROVIDER); 10966 10967 /** 10968 * Constant for fluent queries to be used to add include statements. Specifies 10969 * the path value of "<b>Claim:provider</b>". 10970 */ 10971 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include( 10972 "Claim:provider").toLocked(); 10973 10974 /** 10975 * Search parameter: <b>patient</b> 10976 * <p> 10977 * Description: <b>Patient receiving the products or services</b><br> 10978 * Type: <b>reference</b><br> 10979 * Path: <b>Claim.patient</b><br> 10980 * </p> 10981 */ 10982 @SearchParamDefinition(name = "patient", path = "Claim.patient", description = "Patient receiving the products or services", type = "reference", providesMembershipIn = { 10983 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 10984 public static final String SP_PATIENT = "patient"; 10985 /** 10986 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 10987 * <p> 10988 * Description: <b>Patient receiving the products or services</b><br> 10989 * Type: <b>reference</b><br> 10990 * Path: <b>Claim.patient</b><br> 10991 * </p> 10992 */ 10993 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10994 SP_PATIENT); 10995 10996 /** 10997 * Constant for fluent queries to be used to add include statements. Specifies 10998 * the path value of "<b>Claim:patient</b>". 10999 */ 11000 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Claim:patient") 11001 .toLocked(); 11002 11003 /** 11004 * Search parameter: <b>insurer</b> 11005 * <p> 11006 * Description: <b>The target payor/insurer for the Claim</b><br> 11007 * Type: <b>reference</b><br> 11008 * Path: <b>Claim.insurer</b><br> 11009 * </p> 11010 */ 11011 @SearchParamDefinition(name = "insurer", path = "Claim.insurer", description = "The target payor/insurer for the Claim", type = "reference", target = { 11012 Organization.class }) 11013 public static final String SP_INSURER = "insurer"; 11014 /** 11015 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 11016 * <p> 11017 * Description: <b>The target payor/insurer for the Claim</b><br> 11018 * Type: <b>reference</b><br> 11019 * Path: <b>Claim.insurer</b><br> 11020 * </p> 11021 */ 11022 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11023 SP_INSURER); 11024 11025 /** 11026 * Constant for fluent queries to be used to add include statements. Specifies 11027 * the path value of "<b>Claim:insurer</b>". 11028 */ 11029 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Claim:insurer") 11030 .toLocked(); 11031 11032 /** 11033 * Search parameter: <b>detail-udi</b> 11034 * <p> 11035 * Description: <b>UDI associated with a line item, detail product or 11036 * service</b><br> 11037 * Type: <b>reference</b><br> 11038 * Path: <b>Claim.item.detail.udi</b><br> 11039 * </p> 11040 */ 11041 @SearchParamDefinition(name = "detail-udi", path = "Claim.item.detail.udi", description = "UDI associated with a line item, detail product or service", type = "reference", providesMembershipIn = { 11042 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11043 public static final String SP_DETAIL_UDI = "detail-udi"; 11044 /** 11045 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 11046 * <p> 11047 * Description: <b>UDI associated with a line item, detail product or 11048 * service</b><br> 11049 * Type: <b>reference</b><br> 11050 * Path: <b>Claim.item.detail.udi</b><br> 11051 * </p> 11052 */ 11053 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11054 SP_DETAIL_UDI); 11055 11056 /** 11057 * Constant for fluent queries to be used to add include statements. Specifies 11058 * the path value of "<b>Claim:detail-udi</b>". 11059 */ 11060 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include( 11061 "Claim:detail-udi").toLocked(); 11062 11063 /** 11064 * Search parameter: <b>enterer</b> 11065 * <p> 11066 * Description: <b>The party responsible for the entry of the Claim</b><br> 11067 * Type: <b>reference</b><br> 11068 * Path: <b>Claim.enterer</b><br> 11069 * </p> 11070 */ 11071 @SearchParamDefinition(name = "enterer", path = "Claim.enterer", description = "The party responsible for the entry of the Claim", type = "reference", providesMembershipIn = { 11072 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 11073 PractitionerRole.class }) 11074 public static final String SP_ENTERER = "enterer"; 11075 /** 11076 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 11077 * <p> 11078 * Description: <b>The party responsible for the entry of the Claim</b><br> 11079 * Type: <b>reference</b><br> 11080 * Path: <b>Claim.enterer</b><br> 11081 * </p> 11082 */ 11083 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11084 SP_ENTERER); 11085 11086 /** 11087 * Constant for fluent queries to be used to add include statements. Specifies 11088 * the path value of "<b>Claim:enterer</b>". 11089 */ 11090 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("Claim:enterer") 11091 .toLocked(); 11092 11093 /** 11094 * Search parameter: <b>procedure-udi</b> 11095 * <p> 11096 * Description: <b>UDI associated with a procedure</b><br> 11097 * Type: <b>reference</b><br> 11098 * Path: <b>Claim.procedure.udi</b><br> 11099 * </p> 11100 */ 11101 @SearchParamDefinition(name = "procedure-udi", path = "Claim.procedure.udi", description = "UDI associated with a procedure", type = "reference", providesMembershipIn = { 11102 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11103 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 11104 /** 11105 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 11106 * <p> 11107 * Description: <b>UDI associated with a procedure</b><br> 11108 * Type: <b>reference</b><br> 11109 * Path: <b>Claim.procedure.udi</b><br> 11110 * </p> 11111 */ 11112 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11113 SP_PROCEDURE_UDI); 11114 11115 /** 11116 * Constant for fluent queries to be used to add include statements. Specifies 11117 * the path value of "<b>Claim:procedure-udi</b>". 11118 */ 11119 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include( 11120 "Claim:procedure-udi").toLocked(); 11121 11122 /** 11123 * Search parameter: <b>subdetail-udi</b> 11124 * <p> 11125 * Description: <b>UDI associated with a line item, detail, subdetail product or 11126 * service</b><br> 11127 * Type: <b>reference</b><br> 11128 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 11129 * </p> 11130 */ 11131 @SearchParamDefinition(name = "subdetail-udi", path = "Claim.item.detail.subDetail.udi", description = "UDI associated with a line item, detail, subdetail product or service", type = "reference", providesMembershipIn = { 11132 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11133 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 11134 /** 11135 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 11136 * <p> 11137 * Description: <b>UDI associated with a line item, detail, subdetail product or 11138 * service</b><br> 11139 * Type: <b>reference</b><br> 11140 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 11141 * </p> 11142 */ 11143 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11144 SP_SUBDETAIL_UDI); 11145 11146 /** 11147 * Constant for fluent queries to be used to add include statements. Specifies 11148 * the path value of "<b>Claim:subdetail-udi</b>". 11149 */ 11150 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include( 11151 "Claim:subdetail-udi").toLocked(); 11152 11153 /** 11154 * Search parameter: <b>facility</b> 11155 * <p> 11156 * Description: <b>Facility where the products or services have been or will be 11157 * provided</b><br> 11158 * Type: <b>reference</b><br> 11159 * Path: <b>Claim.facility</b><br> 11160 * </p> 11161 */ 11162 @SearchParamDefinition(name = "facility", path = "Claim.facility", description = "Facility where the products or services have been or will be provided", type = "reference", target = { 11163 Location.class }) 11164 public static final String SP_FACILITY = "facility"; 11165 /** 11166 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 11167 * <p> 11168 * Description: <b>Facility where the products or services have been or will be 11169 * provided</b><br> 11170 * Type: <b>reference</b><br> 11171 * Path: <b>Claim.facility</b><br> 11172 * </p> 11173 */ 11174 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11175 SP_FACILITY); 11176 11177 /** 11178 * Constant for fluent queries to be used to add include statements. Specifies 11179 * the path value of "<b>Claim:facility</b>". 11180 */ 11181 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include( 11182 "Claim:facility").toLocked(); 11183 11184 /** 11185 * Search parameter: <b>item-udi</b> 11186 * <p> 11187 * Description: <b>UDI associated with a line item product or service</b><br> 11188 * Type: <b>reference</b><br> 11189 * Path: <b>Claim.item.udi</b><br> 11190 * </p> 11191 */ 11192 @SearchParamDefinition(name = "item-udi", path = "Claim.item.udi", description = "UDI associated with a line item product or service", type = "reference", providesMembershipIn = { 11193 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11194 public static final String SP_ITEM_UDI = "item-udi"; 11195 /** 11196 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 11197 * <p> 11198 * Description: <b>UDI associated with a line item product or service</b><br> 11199 * Type: <b>reference</b><br> 11200 * Path: <b>Claim.item.udi</b><br> 11201 * </p> 11202 */ 11203 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11204 SP_ITEM_UDI); 11205 11206 /** 11207 * Constant for fluent queries to be used to add include statements. Specifies 11208 * the path value of "<b>Claim:item-udi</b>". 11209 */ 11210 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include( 11211 "Claim:item-udi").toLocked(); 11212 11213 /** 11214 * Search parameter: <b>status</b> 11215 * <p> 11216 * Description: <b>The status of the Claim instance.</b><br> 11217 * Type: <b>token</b><br> 11218 * Path: <b>Claim.status</b><br> 11219 * </p> 11220 */ 11221 @SearchParamDefinition(name = "status", path = "Claim.status", description = "The status of the Claim instance.", type = "token") 11222 public static final String SP_STATUS = "status"; 11223 /** 11224 * <b>Fluent Client</b> search parameter constant for <b>status</b> 11225 * <p> 11226 * Description: <b>The status of the Claim instance.</b><br> 11227 * Type: <b>token</b><br> 11228 * Path: <b>Claim.status</b><br> 11229 * </p> 11230 */ 11231 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11232 SP_STATUS); 11233 11234}