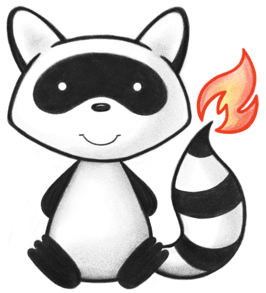
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A provider issued list of professional services and products which have been 051 * provided, or are to be provided, to a patient which is sent to an insurer for 052 * reimbursement. 053 */ 054@ResourceDef(name = "Claim", profile = "http://hl7.org/fhir/StructureDefinition/Claim") 055public class Claim extends DomainResource { 056 057 public enum ClaimStatus { 058 /** 059 * The instance is currently in-force. 060 */ 061 ACTIVE, 062 /** 063 * The instance is withdrawn, rescinded or reversed. 064 */ 065 CANCELLED, 066 /** 067 * A new instance the contents of which is not complete. 068 */ 069 DRAFT, 070 /** 071 * The instance was entered in error. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 079 public static ClaimStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("active".equals(codeString)) 083 return ACTIVE; 084 if ("cancelled".equals(codeString)) 085 return CANCELLED; 086 if ("draft".equals(codeString)) 087 return DRAFT; 088 if ("entered-in-error".equals(codeString)) 089 return ENTEREDINERROR; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown ClaimStatus code '" + codeString + "'"); 094 } 095 096 public String toCode() { 097 switch (this) { 098 case ACTIVE: 099 return "active"; 100 case CANCELLED: 101 return "cancelled"; 102 case DRAFT: 103 return "draft"; 104 case ENTEREDINERROR: 105 return "entered-in-error"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 switch (this) { 115 case ACTIVE: 116 return "http://hl7.org/fhir/fm-status"; 117 case CANCELLED: 118 return "http://hl7.org/fhir/fm-status"; 119 case DRAFT: 120 return "http://hl7.org/fhir/fm-status"; 121 case ENTEREDINERROR: 122 return "http://hl7.org/fhir/fm-status"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDefinition() { 131 switch (this) { 132 case ACTIVE: 133 return "The instance is currently in-force."; 134 case CANCELLED: 135 return "The instance is withdrawn, rescinded or reversed."; 136 case DRAFT: 137 return "A new instance the contents of which is not complete."; 138 case ENTEREDINERROR: 139 return "The instance was entered in error."; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDisplay() { 148 switch (this) { 149 case ACTIVE: 150 return "Active"; 151 case CANCELLED: 152 return "Cancelled"; 153 case DRAFT: 154 return "Draft"; 155 case ENTEREDINERROR: 156 return "Entered in Error"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 } 164 165 public static class ClaimStatusEnumFactory implements EnumFactory<ClaimStatus> { 166 public ClaimStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("active".equals(codeString)) 171 return ClaimStatus.ACTIVE; 172 if ("cancelled".equals(codeString)) 173 return ClaimStatus.CANCELLED; 174 if ("draft".equals(codeString)) 175 return ClaimStatus.DRAFT; 176 if ("entered-in-error".equals(codeString)) 177 return ClaimStatus.ENTEREDINERROR; 178 throw new IllegalArgumentException("Unknown ClaimStatus code '" + codeString + "'"); 179 } 180 181 public Enumeration<ClaimStatus> fromType(PrimitiveType<?> code) throws FHIRException { 182 if (code == null) 183 return null; 184 if (code.isEmpty()) 185 return new Enumeration<ClaimStatus>(this, ClaimStatus.NULL, code); 186 String codeString = code.asStringValue(); 187 if (codeString == null || "".equals(codeString)) 188 return new Enumeration<ClaimStatus>(this, ClaimStatus.NULL, code); 189 if ("active".equals(codeString)) 190 return new Enumeration<ClaimStatus>(this, ClaimStatus.ACTIVE, code); 191 if ("cancelled".equals(codeString)) 192 return new Enumeration<ClaimStatus>(this, ClaimStatus.CANCELLED, code); 193 if ("draft".equals(codeString)) 194 return new Enumeration<ClaimStatus>(this, ClaimStatus.DRAFT, code); 195 if ("entered-in-error".equals(codeString)) 196 return new Enumeration<ClaimStatus>(this, ClaimStatus.ENTEREDINERROR, code); 197 throw new FHIRException("Unknown ClaimStatus code '" + codeString + "'"); 198 } 199 200 public String toCode(ClaimStatus code) { 201 if (code == ClaimStatus.NULL) 202 return null; 203 if (code == ClaimStatus.ACTIVE) 204 return "active"; 205 if (code == ClaimStatus.CANCELLED) 206 return "cancelled"; 207 if (code == ClaimStatus.DRAFT) 208 return "draft"; 209 if (code == ClaimStatus.ENTEREDINERROR) 210 return "entered-in-error"; 211 return "?"; 212 } 213 214 public String toSystem(ClaimStatus code) { 215 return code.getSystem(); 216 } 217 } 218 219 public enum Use { 220 /** 221 * The treatment is complete and this represents a Claim for the services. 222 */ 223 CLAIM, 224 /** 225 * The treatment is proposed and this represents a Pre-authorization for the 226 * services. 227 */ 228 PREAUTHORIZATION, 229 /** 230 * The treatment is proposed and this represents a Pre-determination for the 231 * services. 232 */ 233 PREDETERMINATION, 234 /** 235 * added to help the parsers with the generic types 236 */ 237 NULL; 238 239 public static Use fromCode(String codeString) throws FHIRException { 240 if (codeString == null || "".equals(codeString)) 241 return null; 242 if ("claim".equals(codeString)) 243 return CLAIM; 244 if ("preauthorization".equals(codeString)) 245 return PREAUTHORIZATION; 246 if ("predetermination".equals(codeString)) 247 return PREDETERMINATION; 248 if (Configuration.isAcceptInvalidEnums()) 249 return null; 250 else 251 throw new FHIRException("Unknown Use code '" + codeString + "'"); 252 } 253 254 public String toCode() { 255 switch (this) { 256 case CLAIM: 257 return "claim"; 258 case PREAUTHORIZATION: 259 return "preauthorization"; 260 case PREDETERMINATION: 261 return "predetermination"; 262 case NULL: 263 return null; 264 default: 265 return "?"; 266 } 267 } 268 269 public String getSystem() { 270 switch (this) { 271 case CLAIM: 272 return "http://hl7.org/fhir/claim-use"; 273 case PREAUTHORIZATION: 274 return "http://hl7.org/fhir/claim-use"; 275 case PREDETERMINATION: 276 return "http://hl7.org/fhir/claim-use"; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getDefinition() { 285 switch (this) { 286 case CLAIM: 287 return "The treatment is complete and this represents a Claim for the services."; 288 case PREAUTHORIZATION: 289 return "The treatment is proposed and this represents a Pre-authorization for the services."; 290 case PREDETERMINATION: 291 return "The treatment is proposed and this represents a Pre-determination for the services."; 292 case NULL: 293 return null; 294 default: 295 return "?"; 296 } 297 } 298 299 public String getDisplay() { 300 switch (this) { 301 case CLAIM: 302 return "Claim"; 303 case PREAUTHORIZATION: 304 return "Preauthorization"; 305 case PREDETERMINATION: 306 return "Predetermination"; 307 case NULL: 308 return null; 309 default: 310 return "?"; 311 } 312 } 313 } 314 315 public static class UseEnumFactory implements EnumFactory<Use> { 316 public Use fromCode(String codeString) throws IllegalArgumentException { 317 if (codeString == null || "".equals(codeString)) 318 if (codeString == null || "".equals(codeString)) 319 return null; 320 if ("claim".equals(codeString)) 321 return Use.CLAIM; 322 if ("preauthorization".equals(codeString)) 323 return Use.PREAUTHORIZATION; 324 if ("predetermination".equals(codeString)) 325 return Use.PREDETERMINATION; 326 throw new IllegalArgumentException("Unknown Use code '" + codeString + "'"); 327 } 328 329 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 330 if (code == null) 331 return null; 332 if (code.isEmpty()) 333 return new Enumeration<Use>(this, Use.NULL, code); 334 String codeString = code.asStringValue(); 335 if (codeString == null || "".equals(codeString)) 336 return new Enumeration<Use>(this, Use.NULL, code); 337 if ("claim".equals(codeString)) 338 return new Enumeration<Use>(this, Use.CLAIM, code); 339 if ("preauthorization".equals(codeString)) 340 return new Enumeration<Use>(this, Use.PREAUTHORIZATION, code); 341 if ("predetermination".equals(codeString)) 342 return new Enumeration<Use>(this, Use.PREDETERMINATION, code); 343 throw new FHIRException("Unknown Use code '" + codeString + "'"); 344 } 345 346 public String toCode(Use code) { 347 if (code == Use.NULL) 348 return null; 349 if (code == Use.CLAIM) 350 return "claim"; 351 if (code == Use.PREAUTHORIZATION) 352 return "preauthorization"; 353 if (code == Use.PREDETERMINATION) 354 return "predetermination"; 355 return "?"; 356 } 357 358 public String toSystem(Use code) { 359 return code.getSystem(); 360 } 361 } 362 363 @Block() 364 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 365 /** 366 * Reference to a related claim. 367 */ 368 @Child(name = "claim", type = { Claim.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 369 @Description(shortDefinition = "Reference to the related claim", formalDefinition = "Reference to a related claim.") 370 protected Reference claim; 371 372 /** 373 * The actual object that is the target of the reference (Reference to a related 374 * claim.) 375 */ 376 protected Claim claimTarget; 377 378 /** 379 * A code to convey how the claims are related. 380 */ 381 @Child(name = "relationship", type = { 382 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 383 @Description(shortDefinition = "How the reference claim is related", formalDefinition = "A code to convey how the claims are related.") 384 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/related-claim-relationship") 385 protected CodeableConcept relationship; 386 387 /** 388 * An alternate organizational reference to the case or file to which this 389 * particular claim pertains. 390 */ 391 @Child(name = "reference", type = { 392 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 393 @Description(shortDefinition = "File or case reference", formalDefinition = "An alternate organizational reference to the case or file to which this particular claim pertains.") 394 protected Identifier reference; 395 396 private static final long serialVersionUID = -379338905L; 397 398 /** 399 * Constructor 400 */ 401 public RelatedClaimComponent() { 402 super(); 403 } 404 405 /** 406 * @return {@link #claim} (Reference to a related claim.) 407 */ 408 public Reference getClaim() { 409 if (this.claim == null) 410 if (Configuration.errorOnAutoCreate()) 411 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 412 else if (Configuration.doAutoCreate()) 413 this.claim = new Reference(); // cc 414 return this.claim; 415 } 416 417 public boolean hasClaim() { 418 return this.claim != null && !this.claim.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #claim} (Reference to a related claim.) 423 */ 424 public RelatedClaimComponent setClaim(Reference value) { 425 this.claim = value; 426 return this; 427 } 428 429 /** 430 * @return {@link #claim} The actual object that is the target of the reference. 431 * The reference library doesn't populate this, but you can use it to 432 * hold the resource if you resolve it. (Reference to a related claim.) 433 */ 434 public Claim getClaimTarget() { 435 if (this.claimTarget == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 438 else if (Configuration.doAutoCreate()) 439 this.claimTarget = new Claim(); // aa 440 return this.claimTarget; 441 } 442 443 /** 444 * @param value {@link #claim} The actual object that is the target of the 445 * reference. The reference library doesn't use these, but you can 446 * use it to hold the resource if you resolve it. (Reference to a 447 * related claim.) 448 */ 449 public RelatedClaimComponent setClaimTarget(Claim value) { 450 this.claimTarget = value; 451 return this; 452 } 453 454 /** 455 * @return {@link #relationship} (A code to convey how the claims are related.) 456 */ 457 public CodeableConcept getRelationship() { 458 if (this.relationship == null) 459 if (Configuration.errorOnAutoCreate()) 460 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 461 else if (Configuration.doAutoCreate()) 462 this.relationship = new CodeableConcept(); // cc 463 return this.relationship; 464 } 465 466 public boolean hasRelationship() { 467 return this.relationship != null && !this.relationship.isEmpty(); 468 } 469 470 /** 471 * @param value {@link #relationship} (A code to convey how the claims are 472 * related.) 473 */ 474 public RelatedClaimComponent setRelationship(CodeableConcept value) { 475 this.relationship = value; 476 return this; 477 } 478 479 /** 480 * @return {@link #reference} (An alternate organizational reference to the case 481 * or file to which this particular claim pertains.) 482 */ 483 public Identifier getReference() { 484 if (this.reference == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 487 else if (Configuration.doAutoCreate()) 488 this.reference = new Identifier(); // cc 489 return this.reference; 490 } 491 492 public boolean hasReference() { 493 return this.reference != null && !this.reference.isEmpty(); 494 } 495 496 /** 497 * @param value {@link #reference} (An alternate organizational reference to the 498 * case or file to which this particular claim pertains.) 499 */ 500 public RelatedClaimComponent setReference(Identifier value) { 501 this.reference = value; 502 return this; 503 } 504 505 protected void listChildren(List<Property> children) { 506 super.listChildren(children); 507 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 508 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, 509 relationship)); 510 children.add(new Property("reference", "Identifier", 511 "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, 512 reference)); 513 } 514 515 @Override 516 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 517 switch (_hash) { 518 case 94742588: 519 /* claim */ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 520 case -261851592: 521 /* relationship */ return new Property("relationship", "CodeableConcept", 522 "A code to convey how the claims are related.", 0, 1, relationship); 523 case -925155509: 524 /* reference */ return new Property("reference", "Identifier", 525 "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, 526 reference); 527 default: 528 return super.getNamedProperty(_hash, _name, _checkValid); 529 } 530 531 } 532 533 @Override 534 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 535 switch (hash) { 536 case 94742588: 537 /* claim */ return this.claim == null ? new Base[0] : new Base[] { this.claim }; // Reference 538 case -261851592: 539 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 540 case -925155509: 541 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Identifier 542 default: 543 return super.getProperty(hash, name, checkValid); 544 } 545 546 } 547 548 @Override 549 public Base setProperty(int hash, String name, Base value) throws FHIRException { 550 switch (hash) { 551 case 94742588: // claim 552 this.claim = castToReference(value); // Reference 553 return value; 554 case -261851592: // relationship 555 this.relationship = castToCodeableConcept(value); // CodeableConcept 556 return value; 557 case -925155509: // reference 558 this.reference = castToIdentifier(value); // Identifier 559 return value; 560 default: 561 return super.setProperty(hash, name, value); 562 } 563 564 } 565 566 @Override 567 public Base setProperty(String name, Base value) throws FHIRException { 568 if (name.equals("claim")) { 569 this.claim = castToReference(value); // Reference 570 } else if (name.equals("relationship")) { 571 this.relationship = castToCodeableConcept(value); // CodeableConcept 572 } else if (name.equals("reference")) { 573 this.reference = castToIdentifier(value); // Identifier 574 } else 575 return super.setProperty(name, value); 576 return value; 577 } 578 579 @Override 580 public void removeChild(String name, Base value) throws FHIRException { 581 if (name.equals("claim")) { 582 this.claim = null; 583 } else if (name.equals("relationship")) { 584 this.relationship = null; 585 } else if (name.equals("reference")) { 586 this.reference = null; 587 } else 588 super.removeChild(name, value); 589 590 } 591 592 @Override 593 public Base makeProperty(int hash, String name) throws FHIRException { 594 switch (hash) { 595 case 94742588: 596 return getClaim(); 597 case -261851592: 598 return getRelationship(); 599 case -925155509: 600 return getReference(); 601 default: 602 return super.makeProperty(hash, name); 603 } 604 605 } 606 607 @Override 608 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 609 switch (hash) { 610 case 94742588: 611 /* claim */ return new String[] { "Reference" }; 612 case -261851592: 613 /* relationship */ return new String[] { "CodeableConcept" }; 614 case -925155509: 615 /* reference */ return new String[] { "Identifier" }; 616 default: 617 return super.getTypesForProperty(hash, name); 618 } 619 620 } 621 622 @Override 623 public Base addChild(String name) throws FHIRException { 624 if (name.equals("claim")) { 625 this.claim = new Reference(); 626 return this.claim; 627 } else if (name.equals("relationship")) { 628 this.relationship = new CodeableConcept(); 629 return this.relationship; 630 } else if (name.equals("reference")) { 631 this.reference = new Identifier(); 632 return this.reference; 633 } else 634 return super.addChild(name); 635 } 636 637 public RelatedClaimComponent copy() { 638 RelatedClaimComponent dst = new RelatedClaimComponent(); 639 copyValues(dst); 640 return dst; 641 } 642 643 public void copyValues(RelatedClaimComponent dst) { 644 super.copyValues(dst); 645 dst.claim = claim == null ? null : claim.copy(); 646 dst.relationship = relationship == null ? null : relationship.copy(); 647 dst.reference = reference == null ? null : reference.copy(); 648 } 649 650 @Override 651 public boolean equalsDeep(Base other_) { 652 if (!super.equalsDeep(other_)) 653 return false; 654 if (!(other_ instanceof RelatedClaimComponent)) 655 return false; 656 RelatedClaimComponent o = (RelatedClaimComponent) other_; 657 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) 658 && compareDeep(reference, o.reference, true); 659 } 660 661 @Override 662 public boolean equalsShallow(Base other_) { 663 if (!super.equalsShallow(other_)) 664 return false; 665 if (!(other_ instanceof RelatedClaimComponent)) 666 return false; 667 RelatedClaimComponent o = (RelatedClaimComponent) other_; 668 return true; 669 } 670 671 public boolean isEmpty() { 672 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference); 673 } 674 675 public String fhirType() { 676 return "Claim.related"; 677 678 } 679 680 } 681 682 @Block() 683 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 684 /** 685 * Type of Party to be reimbursed: subscriber, provider, other. 686 */ 687 @Child(name = "type", type = { 688 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 689 @Description(shortDefinition = "Category of recipient", formalDefinition = "Type of Party to be reimbursed: subscriber, provider, other.") 690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payeetype") 691 protected CodeableConcept type; 692 693 /** 694 * Reference to the individual or organization to whom any payment will be made. 695 */ 696 @Child(name = "party", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 697 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 698 @Description(shortDefinition = "Recipient reference", formalDefinition = "Reference to the individual or organization to whom any payment will be made.") 699 protected Reference party; 700 701 /** 702 * The actual object that is the target of the reference (Reference to the 703 * individual or organization to whom any payment will be made.) 704 */ 705 protected Resource partyTarget; 706 707 private static final long serialVersionUID = 1609484699L; 708 709 /** 710 * Constructor 711 */ 712 public PayeeComponent() { 713 super(); 714 } 715 716 /** 717 * Constructor 718 */ 719 public PayeeComponent(CodeableConcept type) { 720 super(); 721 this.type = type; 722 } 723 724 /** 725 * @return {@link #type} (Type of Party to be reimbursed: subscriber, provider, 726 * other.) 727 */ 728 public CodeableConcept getType() { 729 if (this.type == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create PayeeComponent.type"); 732 else if (Configuration.doAutoCreate()) 733 this.type = new CodeableConcept(); // cc 734 return this.type; 735 } 736 737 public boolean hasType() { 738 return this.type != null && !this.type.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #type} (Type of Party to be reimbursed: subscriber, 743 * provider, other.) 744 */ 745 public PayeeComponent setType(CodeableConcept value) { 746 this.type = value; 747 return this; 748 } 749 750 /** 751 * @return {@link #party} (Reference to the individual or organization to whom 752 * any payment will be made.) 753 */ 754 public Reference getParty() { 755 if (this.party == null) 756 if (Configuration.errorOnAutoCreate()) 757 throw new Error("Attempt to auto-create PayeeComponent.party"); 758 else if (Configuration.doAutoCreate()) 759 this.party = new Reference(); // cc 760 return this.party; 761 } 762 763 public boolean hasParty() { 764 return this.party != null && !this.party.isEmpty(); 765 } 766 767 /** 768 * @param value {@link #party} (Reference to the individual or organization to 769 * whom any payment will be made.) 770 */ 771 public PayeeComponent setParty(Reference value) { 772 this.party = value; 773 return this; 774 } 775 776 /** 777 * @return {@link #party} The actual object that is the target of the reference. 778 * The reference library doesn't populate this, but you can use it to 779 * hold the resource if you resolve it. (Reference to the individual or 780 * organization to whom any payment will be made.) 781 */ 782 public Resource getPartyTarget() { 783 return this.partyTarget; 784 } 785 786 /** 787 * @param value {@link #party} The actual object that is the target of the 788 * reference. The reference library doesn't use these, but you can 789 * use it to hold the resource if you resolve it. (Reference to the 790 * individual or organization to whom any payment will be made.) 791 */ 792 public PayeeComponent setPartyTarget(Resource value) { 793 this.partyTarget = value; 794 return this; 795 } 796 797 protected void listChildren(List<Property> children) { 798 super.listChildren(children); 799 children.add(new Property("type", "CodeableConcept", 800 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type)); 801 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", 802 "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 803 } 804 805 @Override 806 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 807 switch (_hash) { 808 case 3575610: 809 /* type */ return new Property("type", "CodeableConcept", 810 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type); 811 case 106437350: 812 /* party */ return new Property("party", 813 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", 814 "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 815 default: 816 return super.getNamedProperty(_hash, _name, _checkValid); 817 } 818 819 } 820 821 @Override 822 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 823 switch (hash) { 824 case 3575610: 825 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 826 case 106437350: 827 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 828 default: 829 return super.getProperty(hash, name, checkValid); 830 } 831 832 } 833 834 @Override 835 public Base setProperty(int hash, String name, Base value) throws FHIRException { 836 switch (hash) { 837 case 3575610: // type 838 this.type = castToCodeableConcept(value); // CodeableConcept 839 return value; 840 case 106437350: // party 841 this.party = castToReference(value); // Reference 842 return value; 843 default: 844 return super.setProperty(hash, name, value); 845 } 846 847 } 848 849 @Override 850 public Base setProperty(String name, Base value) throws FHIRException { 851 if (name.equals("type")) { 852 this.type = castToCodeableConcept(value); // CodeableConcept 853 } else if (name.equals("party")) { 854 this.party = castToReference(value); // Reference 855 } else 856 return super.setProperty(name, value); 857 return value; 858 } 859 860 @Override 861 public void removeChild(String name, Base value) throws FHIRException { 862 if (name.equals("type")) { 863 this.type = null; 864 } else if (name.equals("party")) { 865 this.party = null; 866 } else 867 super.removeChild(name, value); 868 869 } 870 871 @Override 872 public Base makeProperty(int hash, String name) throws FHIRException { 873 switch (hash) { 874 case 3575610: 875 return getType(); 876 case 106437350: 877 return getParty(); 878 default: 879 return super.makeProperty(hash, name); 880 } 881 882 } 883 884 @Override 885 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case 3575610: 888 /* type */ return new String[] { "CodeableConcept" }; 889 case 106437350: 890 /* party */ return new String[] { "Reference" }; 891 default: 892 return super.getTypesForProperty(hash, name); 893 } 894 895 } 896 897 @Override 898 public Base addChild(String name) throws FHIRException { 899 if (name.equals("type")) { 900 this.type = new CodeableConcept(); 901 return this.type; 902 } else if (name.equals("party")) { 903 this.party = new Reference(); 904 return this.party; 905 } else 906 return super.addChild(name); 907 } 908 909 public PayeeComponent copy() { 910 PayeeComponent dst = new PayeeComponent(); 911 copyValues(dst); 912 return dst; 913 } 914 915 public void copyValues(PayeeComponent dst) { 916 super.copyValues(dst); 917 dst.type = type == null ? null : type.copy(); 918 dst.party = party == null ? null : party.copy(); 919 } 920 921 @Override 922 public boolean equalsDeep(Base other_) { 923 if (!super.equalsDeep(other_)) 924 return false; 925 if (!(other_ instanceof PayeeComponent)) 926 return false; 927 PayeeComponent o = (PayeeComponent) other_; 928 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 929 } 930 931 @Override 932 public boolean equalsShallow(Base other_) { 933 if (!super.equalsShallow(other_)) 934 return false; 935 if (!(other_ instanceof PayeeComponent)) 936 return false; 937 PayeeComponent o = (PayeeComponent) other_; 938 return true; 939 } 940 941 public boolean isEmpty() { 942 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 943 } 944 945 public String fhirType() { 946 return "Claim.payee"; 947 948 } 949 950 } 951 952 @Block() 953 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 954 /** 955 * A number to uniquely identify care team entries. 956 */ 957 @Child(name = "sequence", type = { 958 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 959 @Description(shortDefinition = "Order of care team", formalDefinition = "A number to uniquely identify care team entries.") 960 protected PositiveIntType sequence; 961 962 /** 963 * Member of the team who provided the product or service. 964 */ 965 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 966 Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 967 @Description(shortDefinition = "Practitioner or organization", formalDefinition = "Member of the team who provided the product or service.") 968 protected Reference provider; 969 970 /** 971 * The actual object that is the target of the reference (Member of the team who 972 * provided the product or service.) 973 */ 974 protected Resource providerTarget; 975 976 /** 977 * The party who is billing and/or responsible for the claimed products or 978 * services. 979 */ 980 @Child(name = "responsible", type = { 981 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 982 @Description(shortDefinition = "Indicator of the lead practitioner", formalDefinition = "The party who is billing and/or responsible for the claimed products or services.") 983 protected BooleanType responsible; 984 985 /** 986 * The lead, assisting or supervising practitioner and their discipline if a 987 * multidisciplinary team. 988 */ 989 @Child(name = "role", type = { 990 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 991 @Description(shortDefinition = "Function within the team", formalDefinition = "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.") 992 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-careteamrole") 993 protected CodeableConcept role; 994 995 /** 996 * The qualification of the practitioner which is applicable for this service. 997 */ 998 @Child(name = "qualification", type = { 999 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1000 @Description(shortDefinition = "Practitioner credential or specialization", formalDefinition = "The qualification of the practitioner which is applicable for this service.") 1001 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provider-qualification") 1002 protected CodeableConcept qualification; 1003 1004 private static final long serialVersionUID = 1758966968L; 1005 1006 /** 1007 * Constructor 1008 */ 1009 public CareTeamComponent() { 1010 super(); 1011 } 1012 1013 /** 1014 * Constructor 1015 */ 1016 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 1017 super(); 1018 this.sequence = sequence; 1019 this.provider = provider; 1020 } 1021 1022 /** 1023 * @return {@link #sequence} (A number to uniquely identify care team entries.). 1024 * This is the underlying object with id, value and extensions. The 1025 * accessor "getSequence" gives direct access to the value 1026 */ 1027 public PositiveIntType getSequenceElement() { 1028 if (this.sequence == null) 1029 if (Configuration.errorOnAutoCreate()) 1030 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 1031 else if (Configuration.doAutoCreate()) 1032 this.sequence = new PositiveIntType(); // bb 1033 return this.sequence; 1034 } 1035 1036 public boolean hasSequenceElement() { 1037 return this.sequence != null && !this.sequence.isEmpty(); 1038 } 1039 1040 public boolean hasSequence() { 1041 return this.sequence != null && !this.sequence.isEmpty(); 1042 } 1043 1044 /** 1045 * @param value {@link #sequence} (A number to uniquely identify care team 1046 * entries.). This is the underlying object with id, value and 1047 * extensions. The accessor "getSequence" gives direct access to 1048 * the value 1049 */ 1050 public CareTeamComponent setSequenceElement(PositiveIntType value) { 1051 this.sequence = value; 1052 return this; 1053 } 1054 1055 /** 1056 * @return A number to uniquely identify care team entries. 1057 */ 1058 public int getSequence() { 1059 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1060 } 1061 1062 /** 1063 * @param value A number to uniquely identify care team entries. 1064 */ 1065 public CareTeamComponent setSequence(int value) { 1066 if (this.sequence == null) 1067 this.sequence = new PositiveIntType(); 1068 this.sequence.setValue(value); 1069 return this; 1070 } 1071 1072 /** 1073 * @return {@link #provider} (Member of the team who provided the product or 1074 * service.) 1075 */ 1076 public Reference getProvider() { 1077 if (this.provider == null) 1078 if (Configuration.errorOnAutoCreate()) 1079 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 1080 else if (Configuration.doAutoCreate()) 1081 this.provider = new Reference(); // cc 1082 return this.provider; 1083 } 1084 1085 public boolean hasProvider() { 1086 return this.provider != null && !this.provider.isEmpty(); 1087 } 1088 1089 /** 1090 * @param value {@link #provider} (Member of the team who provided the product 1091 * or service.) 1092 */ 1093 public CareTeamComponent setProvider(Reference value) { 1094 this.provider = value; 1095 return this; 1096 } 1097 1098 /** 1099 * @return {@link #provider} The actual object that is the target of the 1100 * reference. The reference library doesn't populate this, but you can 1101 * use it to hold the resource if you resolve it. (Member of the team 1102 * who provided the product or service.) 1103 */ 1104 public Resource getProviderTarget() { 1105 return this.providerTarget; 1106 } 1107 1108 /** 1109 * @param value {@link #provider} The actual object that is the target of the 1110 * reference. The reference library doesn't use these, but you can 1111 * use it to hold the resource if you resolve it. (Member of the 1112 * team who provided the product or service.) 1113 */ 1114 public CareTeamComponent setProviderTarget(Resource value) { 1115 this.providerTarget = value; 1116 return this; 1117 } 1118 1119 /** 1120 * @return {@link #responsible} (The party who is billing and/or responsible for 1121 * the claimed products or services.). This is the underlying object 1122 * with id, value and extensions. The accessor "getResponsible" gives 1123 * direct access to the value 1124 */ 1125 public BooleanType getResponsibleElement() { 1126 if (this.responsible == null) 1127 if (Configuration.errorOnAutoCreate()) 1128 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1129 else if (Configuration.doAutoCreate()) 1130 this.responsible = new BooleanType(); // bb 1131 return this.responsible; 1132 } 1133 1134 public boolean hasResponsibleElement() { 1135 return this.responsible != null && !this.responsible.isEmpty(); 1136 } 1137 1138 public boolean hasResponsible() { 1139 return this.responsible != null && !this.responsible.isEmpty(); 1140 } 1141 1142 /** 1143 * @param value {@link #responsible} (The party who is billing and/or 1144 * responsible for the claimed products or services.). This is the 1145 * underlying object with id, value and extensions. The accessor 1146 * "getResponsible" gives direct access to the value 1147 */ 1148 public CareTeamComponent setResponsibleElement(BooleanType value) { 1149 this.responsible = value; 1150 return this; 1151 } 1152 1153 /** 1154 * @return The party who is billing and/or responsible for the claimed products 1155 * or services. 1156 */ 1157 public boolean getResponsible() { 1158 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1159 } 1160 1161 /** 1162 * @param value The party who is billing and/or responsible for the claimed 1163 * products or services. 1164 */ 1165 public CareTeamComponent setResponsible(boolean value) { 1166 if (this.responsible == null) 1167 this.responsible = new BooleanType(); 1168 this.responsible.setValue(value); 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #role} (The lead, assisting or supervising practitioner and 1174 * their discipline if a multidisciplinary team.) 1175 */ 1176 public CodeableConcept getRole() { 1177 if (this.role == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1180 else if (Configuration.doAutoCreate()) 1181 this.role = new CodeableConcept(); // cc 1182 return this.role; 1183 } 1184 1185 public boolean hasRole() { 1186 return this.role != null && !this.role.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #role} (The lead, assisting or supervising practitioner 1191 * and their discipline if a multidisciplinary team.) 1192 */ 1193 public CareTeamComponent setRole(CodeableConcept value) { 1194 this.role = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return {@link #qualification} (The qualification of the practitioner which 1200 * is applicable for this service.) 1201 */ 1202 public CodeableConcept getQualification() { 1203 if (this.qualification == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1206 else if (Configuration.doAutoCreate()) 1207 this.qualification = new CodeableConcept(); // cc 1208 return this.qualification; 1209 } 1210 1211 public boolean hasQualification() { 1212 return this.qualification != null && !this.qualification.isEmpty(); 1213 } 1214 1215 /** 1216 * @param value {@link #qualification} (The qualification of the practitioner 1217 * which is applicable for this service.) 1218 */ 1219 public CareTeamComponent setQualification(CodeableConcept value) { 1220 this.qualification = value; 1221 return this; 1222 } 1223 1224 protected void listChildren(List<Property> children) { 1225 super.listChildren(children); 1226 children.add( 1227 new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 1228 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 1229 "Member of the team who provided the product or service.", 0, 1, provider)); 1230 children.add(new Property("responsible", "boolean", 1231 "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 1232 children.add(new Property("role", "CodeableConcept", 1233 "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, 1234 role)); 1235 children.add(new Property("qualification", "CodeableConcept", 1236 "The qualification of the practitioner which is applicable for this service.", 0, 1, qualification)); 1237 } 1238 1239 @Override 1240 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1241 switch (_hash) { 1242 case 1349547969: 1243 /* sequence */ return new Property("sequence", "positiveInt", 1244 "A number to uniquely identify care team entries.", 0, 1, sequence); 1245 case -987494927: 1246 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 1247 "Member of the team who provided the product or service.", 0, 1, provider); 1248 case 1847674614: 1249 /* responsible */ return new Property("responsible", "boolean", 1250 "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 1251 case 3506294: 1252 /* role */ return new Property("role", "CodeableConcept", 1253 "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, 1254 role); 1255 case -631333393: 1256 /* qualification */ return new Property("qualification", "CodeableConcept", 1257 "The qualification of the practitioner which is applicable for this service.", 0, 1, qualification); 1258 default: 1259 return super.getNamedProperty(_hash, _name, _checkValid); 1260 } 1261 1262 } 1263 1264 @Override 1265 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1266 switch (hash) { 1267 case 1349547969: 1268 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 1269 case -987494927: 1270 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 1271 case 1847674614: 1272 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // BooleanType 1273 case 3506294: 1274 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 1275 case -631333393: 1276 /* qualification */ return this.qualification == null ? new Base[0] : new Base[] { this.qualification }; // CodeableConcept 1277 default: 1278 return super.getProperty(hash, name, checkValid); 1279 } 1280 1281 } 1282 1283 @Override 1284 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1285 switch (hash) { 1286 case 1349547969: // sequence 1287 this.sequence = castToPositiveInt(value); // PositiveIntType 1288 return value; 1289 case -987494927: // provider 1290 this.provider = castToReference(value); // Reference 1291 return value; 1292 case 1847674614: // responsible 1293 this.responsible = castToBoolean(value); // BooleanType 1294 return value; 1295 case 3506294: // role 1296 this.role = castToCodeableConcept(value); // CodeableConcept 1297 return value; 1298 case -631333393: // qualification 1299 this.qualification = castToCodeableConcept(value); // CodeableConcept 1300 return value; 1301 default: 1302 return super.setProperty(hash, name, value); 1303 } 1304 1305 } 1306 1307 @Override 1308 public Base setProperty(String name, Base value) throws FHIRException { 1309 if (name.equals("sequence")) { 1310 this.sequence = castToPositiveInt(value); // PositiveIntType 1311 } else if (name.equals("provider")) { 1312 this.provider = castToReference(value); // Reference 1313 } else if (name.equals("responsible")) { 1314 this.responsible = castToBoolean(value); // BooleanType 1315 } else if (name.equals("role")) { 1316 this.role = castToCodeableConcept(value); // CodeableConcept 1317 } else if (name.equals("qualification")) { 1318 this.qualification = castToCodeableConcept(value); // CodeableConcept 1319 } else 1320 return super.setProperty(name, value); 1321 return value; 1322 } 1323 1324 @Override 1325 public void removeChild(String name, Base value) throws FHIRException { 1326 if (name.equals("sequence")) { 1327 this.sequence = null; 1328 } else if (name.equals("provider")) { 1329 this.provider = null; 1330 } else if (name.equals("responsible")) { 1331 this.responsible = null; 1332 } else if (name.equals("role")) { 1333 this.role = null; 1334 } else if (name.equals("qualification")) { 1335 this.qualification = null; 1336 } else 1337 super.removeChild(name, value); 1338 1339 } 1340 1341 @Override 1342 public Base makeProperty(int hash, String name) throws FHIRException { 1343 switch (hash) { 1344 case 1349547969: 1345 return getSequenceElement(); 1346 case -987494927: 1347 return getProvider(); 1348 case 1847674614: 1349 return getResponsibleElement(); 1350 case 3506294: 1351 return getRole(); 1352 case -631333393: 1353 return getQualification(); 1354 default: 1355 return super.makeProperty(hash, name); 1356 } 1357 1358 } 1359 1360 @Override 1361 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1362 switch (hash) { 1363 case 1349547969: 1364 /* sequence */ return new String[] { "positiveInt" }; 1365 case -987494927: 1366 /* provider */ return new String[] { "Reference" }; 1367 case 1847674614: 1368 /* responsible */ return new String[] { "boolean" }; 1369 case 3506294: 1370 /* role */ return new String[] { "CodeableConcept" }; 1371 case -631333393: 1372 /* qualification */ return new String[] { "CodeableConcept" }; 1373 default: 1374 return super.getTypesForProperty(hash, name); 1375 } 1376 1377 } 1378 1379 @Override 1380 public Base addChild(String name) throws FHIRException { 1381 if (name.equals("sequence")) { 1382 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1383 } else if (name.equals("provider")) { 1384 this.provider = new Reference(); 1385 return this.provider; 1386 } else if (name.equals("responsible")) { 1387 throw new FHIRException("Cannot call addChild on a singleton property Claim.responsible"); 1388 } else if (name.equals("role")) { 1389 this.role = new CodeableConcept(); 1390 return this.role; 1391 } else if (name.equals("qualification")) { 1392 this.qualification = new CodeableConcept(); 1393 return this.qualification; 1394 } else 1395 return super.addChild(name); 1396 } 1397 1398 public CareTeamComponent copy() { 1399 CareTeamComponent dst = new CareTeamComponent(); 1400 copyValues(dst); 1401 return dst; 1402 } 1403 1404 public void copyValues(CareTeamComponent dst) { 1405 super.copyValues(dst); 1406 dst.sequence = sequence == null ? null : sequence.copy(); 1407 dst.provider = provider == null ? null : provider.copy(); 1408 dst.responsible = responsible == null ? null : responsible.copy(); 1409 dst.role = role == null ? null : role.copy(); 1410 dst.qualification = qualification == null ? null : qualification.copy(); 1411 } 1412 1413 @Override 1414 public boolean equalsDeep(Base other_) { 1415 if (!super.equalsDeep(other_)) 1416 return false; 1417 if (!(other_ instanceof CareTeamComponent)) 1418 return false; 1419 CareTeamComponent o = (CareTeamComponent) other_; 1420 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) 1421 && compareDeep(responsible, o.responsible, true) && compareDeep(role, o.role, true) 1422 && compareDeep(qualification, o.qualification, true); 1423 } 1424 1425 @Override 1426 public boolean equalsShallow(Base other_) { 1427 if (!super.equalsShallow(other_)) 1428 return false; 1429 if (!(other_ instanceof CareTeamComponent)) 1430 return false; 1431 CareTeamComponent o = (CareTeamComponent) other_; 1432 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true); 1433 } 1434 1435 public boolean isEmpty() { 1436 return super.isEmpty() 1437 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible, role, qualification); 1438 } 1439 1440 public String fhirType() { 1441 return "Claim.careTeam"; 1442 1443 } 1444 1445 } 1446 1447 @Block() 1448 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1449 /** 1450 * A number to uniquely identify supporting information entries. 1451 */ 1452 @Child(name = "sequence", type = { 1453 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1454 @Description(shortDefinition = "Information instance identifier", formalDefinition = "A number to uniquely identify supporting information entries.") 1455 protected PositiveIntType sequence; 1456 1457 /** 1458 * The general class of the information supplied: information; exception; 1459 * accident, employment; onset, etc. 1460 */ 1461 @Child(name = "category", type = { 1462 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1463 @Description(shortDefinition = "Classification of the supplied information", formalDefinition = "The general class of the information supplied: information; exception; accident, employment; onset, etc.") 1464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-informationcategory") 1465 protected CodeableConcept category; 1466 1467 /** 1468 * System and code pertaining to the specific information regarding special 1469 * conditions relating to the setting, treatment or patient for which care is 1470 * sought. 1471 */ 1472 @Child(name = "code", type = { 1473 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1474 @Description(shortDefinition = "Type of information", formalDefinition = "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.") 1475 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-exception") 1476 protected CodeableConcept code; 1477 1478 /** 1479 * The date when or period to which this information refers. 1480 */ 1481 @Child(name = "timing", type = { DateType.class, 1482 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1483 @Description(shortDefinition = "When it occurred", formalDefinition = "The date when or period to which this information refers.") 1484 protected Type timing; 1485 1486 /** 1487 * Additional data or information such as resources, documents, images etc. 1488 * including references to the data or the actual inclusion of the data. 1489 */ 1490 @Child(name = "value", type = { BooleanType.class, StringType.class, Quantity.class, Attachment.class, 1491 Reference.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1492 @Description(shortDefinition = "Data to be provided", formalDefinition = "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.") 1493 protected Type value; 1494 1495 /** 1496 * Provides the reason in the situation where a reason code is required in 1497 * addition to the content. 1498 */ 1499 @Child(name = "reason", type = { 1500 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1501 @Description(shortDefinition = "Explanation for the information", formalDefinition = "Provides the reason in the situation where a reason code is required in addition to the content.") 1502 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1503 protected CodeableConcept reason; 1504 1505 private static final long serialVersionUID = -518630232L; 1506 1507 /** 1508 * Constructor 1509 */ 1510 public SupportingInformationComponent() { 1511 super(); 1512 } 1513 1514 /** 1515 * Constructor 1516 */ 1517 public SupportingInformationComponent(PositiveIntType sequence, CodeableConcept category) { 1518 super(); 1519 this.sequence = sequence; 1520 this.category = category; 1521 } 1522 1523 /** 1524 * @return {@link #sequence} (A number to uniquely identify supporting 1525 * information entries.). This is the underlying object with id, value 1526 * and extensions. The accessor "getSequence" gives direct access to the 1527 * value 1528 */ 1529 public PositiveIntType getSequenceElement() { 1530 if (this.sequence == null) 1531 if (Configuration.errorOnAutoCreate()) 1532 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1533 else if (Configuration.doAutoCreate()) 1534 this.sequence = new PositiveIntType(); // bb 1535 return this.sequence; 1536 } 1537 1538 public boolean hasSequenceElement() { 1539 return this.sequence != null && !this.sequence.isEmpty(); 1540 } 1541 1542 public boolean hasSequence() { 1543 return this.sequence != null && !this.sequence.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #sequence} (A number to uniquely identify supporting 1548 * information entries.). This is the underlying object with id, 1549 * value and extensions. The accessor "getSequence" gives direct 1550 * access to the value 1551 */ 1552 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1553 this.sequence = value; 1554 return this; 1555 } 1556 1557 /** 1558 * @return A number to uniquely identify supporting information entries. 1559 */ 1560 public int getSequence() { 1561 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1562 } 1563 1564 /** 1565 * @param value A number to uniquely identify supporting information entries. 1566 */ 1567 public SupportingInformationComponent setSequence(int value) { 1568 if (this.sequence == null) 1569 this.sequence = new PositiveIntType(); 1570 this.sequence.setValue(value); 1571 return this; 1572 } 1573 1574 /** 1575 * @return {@link #category} (The general class of the information supplied: 1576 * information; exception; accident, employment; onset, etc.) 1577 */ 1578 public CodeableConcept getCategory() { 1579 if (this.category == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1582 else if (Configuration.doAutoCreate()) 1583 this.category = new CodeableConcept(); // cc 1584 return this.category; 1585 } 1586 1587 public boolean hasCategory() { 1588 return this.category != null && !this.category.isEmpty(); 1589 } 1590 1591 /** 1592 * @param value {@link #category} (The general class of the information 1593 * supplied: information; exception; accident, employment; onset, 1594 * etc.) 1595 */ 1596 public SupportingInformationComponent setCategory(CodeableConcept value) { 1597 this.category = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #code} (System and code pertaining to the specific information 1603 * regarding special conditions relating to the setting, treatment or 1604 * patient for which care is sought.) 1605 */ 1606 public CodeableConcept getCode() { 1607 if (this.code == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1610 else if (Configuration.doAutoCreate()) 1611 this.code = new CodeableConcept(); // cc 1612 return this.code; 1613 } 1614 1615 public boolean hasCode() { 1616 return this.code != null && !this.code.isEmpty(); 1617 } 1618 1619 /** 1620 * @param value {@link #code} (System and code pertaining to the specific 1621 * information regarding special conditions relating to the 1622 * setting, treatment or patient for which care is sought.) 1623 */ 1624 public SupportingInformationComponent setCode(CodeableConcept value) { 1625 this.code = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #timing} (The date when or period to which this information 1631 * refers.) 1632 */ 1633 public Type getTiming() { 1634 return this.timing; 1635 } 1636 1637 /** 1638 * @return {@link #timing} (The date when or period to which this information 1639 * refers.) 1640 */ 1641 public DateType getTimingDateType() throws FHIRException { 1642 if (this.timing == null) 1643 this.timing = new DateType(); 1644 if (!(this.timing instanceof DateType)) 1645 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.timing.getClass().getName() 1646 + " was encountered"); 1647 return (DateType) this.timing; 1648 } 1649 1650 public boolean hasTimingDateType() { 1651 return this != null && this.timing instanceof DateType; 1652 } 1653 1654 /** 1655 * @return {@link #timing} (The date when or period to which this information 1656 * refers.) 1657 */ 1658 public Period getTimingPeriod() throws FHIRException { 1659 if (this.timing == null) 1660 this.timing = new Period(); 1661 if (!(this.timing instanceof Period)) 1662 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 1663 + " was encountered"); 1664 return (Period) this.timing; 1665 } 1666 1667 public boolean hasTimingPeriod() { 1668 return this != null && this.timing instanceof Period; 1669 } 1670 1671 public boolean hasTiming() { 1672 return this.timing != null && !this.timing.isEmpty(); 1673 } 1674 1675 /** 1676 * @param value {@link #timing} (The date when or period to which this 1677 * information refers.) 1678 */ 1679 public SupportingInformationComponent setTiming(Type value) { 1680 if (value != null && !(value instanceof DateType || value instanceof Period)) 1681 throw new Error("Not the right type for Claim.supportingInfo.timing[x]: " + value.fhirType()); 1682 this.timing = value; 1683 return this; 1684 } 1685 1686 /** 1687 * @return {@link #value} (Additional data or information such as resources, 1688 * documents, images etc. including references to the data or the actual 1689 * inclusion of the data.) 1690 */ 1691 public Type getValue() { 1692 return this.value; 1693 } 1694 1695 /** 1696 * @return {@link #value} (Additional data or information such as resources, 1697 * documents, images etc. including references to the data or the actual 1698 * inclusion of the data.) 1699 */ 1700 public BooleanType getValueBooleanType() throws FHIRException { 1701 if (this.value == null) 1702 this.value = new BooleanType(); 1703 if (!(this.value instanceof BooleanType)) 1704 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1705 + this.value.getClass().getName() + " was encountered"); 1706 return (BooleanType) this.value; 1707 } 1708 1709 public boolean hasValueBooleanType() { 1710 return this != null && this.value instanceof BooleanType; 1711 } 1712 1713 /** 1714 * @return {@link #value} (Additional data or information such as resources, 1715 * documents, images etc. including references to the data or the actual 1716 * inclusion of the data.) 1717 */ 1718 public StringType getValueStringType() throws FHIRException { 1719 if (this.value == null) 1720 this.value = new StringType(); 1721 if (!(this.value instanceof StringType)) 1722 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1723 + this.value.getClass().getName() + " was encountered"); 1724 return (StringType) this.value; 1725 } 1726 1727 public boolean hasValueStringType() { 1728 return this != null && this.value instanceof StringType; 1729 } 1730 1731 /** 1732 * @return {@link #value} (Additional data or information such as resources, 1733 * documents, images etc. including references to the data or the actual 1734 * inclusion of the data.) 1735 */ 1736 public Quantity getValueQuantity() throws FHIRException { 1737 if (this.value == null) 1738 this.value = new Quantity(); 1739 if (!(this.value instanceof Quantity)) 1740 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1741 + " was encountered"); 1742 return (Quantity) this.value; 1743 } 1744 1745 public boolean hasValueQuantity() { 1746 return this != null && this.value instanceof Quantity; 1747 } 1748 1749 /** 1750 * @return {@link #value} (Additional data or information such as resources, 1751 * documents, images etc. including references to the data or the actual 1752 * inclusion of the data.) 1753 */ 1754 public Attachment getValueAttachment() throws FHIRException { 1755 if (this.value == null) 1756 this.value = new Attachment(); 1757 if (!(this.value instanceof Attachment)) 1758 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 1759 + this.value.getClass().getName() + " was encountered"); 1760 return (Attachment) this.value; 1761 } 1762 1763 public boolean hasValueAttachment() { 1764 return this != null && this.value instanceof Attachment; 1765 } 1766 1767 /** 1768 * @return {@link #value} (Additional data or information such as resources, 1769 * documents, images etc. including references to the data or the actual 1770 * inclusion of the data.) 1771 */ 1772 public Reference getValueReference() throws FHIRException { 1773 if (this.value == null) 1774 this.value = new Reference(); 1775 if (!(this.value instanceof Reference)) 1776 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 1777 + " was encountered"); 1778 return (Reference) this.value; 1779 } 1780 1781 public boolean hasValueReference() { 1782 return this != null && this.value instanceof Reference; 1783 } 1784 1785 public boolean hasValue() { 1786 return this.value != null && !this.value.isEmpty(); 1787 } 1788 1789 /** 1790 * @param value {@link #value} (Additional data or information such as 1791 * resources, documents, images etc. including references to the 1792 * data or the actual inclusion of the data.) 1793 */ 1794 public SupportingInformationComponent setValue(Type value) { 1795 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity 1796 || value instanceof Attachment || value instanceof Reference)) 1797 throw new Error("Not the right type for Claim.supportingInfo.value[x]: " + value.fhirType()); 1798 this.value = value; 1799 return this; 1800 } 1801 1802 /** 1803 * @return {@link #reason} (Provides the reason in the situation where a reason 1804 * code is required in addition to the content.) 1805 */ 1806 public CodeableConcept getReason() { 1807 if (this.reason == null) 1808 if (Configuration.errorOnAutoCreate()) 1809 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1810 else if (Configuration.doAutoCreate()) 1811 this.reason = new CodeableConcept(); // cc 1812 return this.reason; 1813 } 1814 1815 public boolean hasReason() { 1816 return this.reason != null && !this.reason.isEmpty(); 1817 } 1818 1819 /** 1820 * @param value {@link #reason} (Provides the reason in the situation where a 1821 * reason code is required in addition to the content.) 1822 */ 1823 public SupportingInformationComponent setReason(CodeableConcept value) { 1824 this.reason = value; 1825 return this; 1826 } 1827 1828 protected void listChildren(List<Property> children) { 1829 super.listChildren(children); 1830 children.add(new Property("sequence", "positiveInt", 1831 "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1832 children.add(new Property("category", "CodeableConcept", 1833 "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1834 1, category)); 1835 children.add(new Property("code", "CodeableConcept", 1836 "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 1837 0, 1, code)); 1838 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 1839 0, 1, timing)); 1840 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1841 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1842 0, 1, value)); 1843 children.add(new Property("reason", "CodeableConcept", 1844 "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, 1845 reason)); 1846 } 1847 1848 @Override 1849 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1850 switch (_hash) { 1851 case 1349547969: 1852 /* sequence */ return new Property("sequence", "positiveInt", 1853 "A number to uniquely identify supporting information entries.", 0, 1, sequence); 1854 case 50511102: 1855 /* category */ return new Property("category", "CodeableConcept", 1856 "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 1857 0, 1, category); 1858 case 3059181: 1859 /* code */ return new Property("code", "CodeableConcept", 1860 "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 1861 0, 1, code); 1862 case 164632566: 1863 /* timing[x] */ return new Property("timing[x]", "date|Period", 1864 "The date when or period to which this information refers.", 0, 1, timing); 1865 case -873664438: 1866 /* timing */ return new Property("timing[x]", "date|Period", 1867 "The date when or period to which this information refers.", 0, 1, timing); 1868 case 807935768: 1869 /* timingDate */ return new Property("timing[x]", "date|Period", 1870 "The date when or period to which this information refers.", 0, 1, timing); 1871 case -615615829: 1872 /* timingPeriod */ return new Property("timing[x]", "date|Period", 1873 "The date when or period to which this information refers.", 0, 1, timing); 1874 case -1410166417: 1875 /* value[x] */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1876 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1877 0, 1, value); 1878 case 111972721: 1879 /* value */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1880 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1881 0, 1, value); 1882 case 733421943: 1883 /* valueBoolean */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1884 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1885 0, 1, value); 1886 case -1424603934: 1887 /* valueString */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1888 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1889 0, 1, value); 1890 case -2029823716: 1891 /* valueQuantity */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1892 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1893 0, 1, value); 1894 case -475566732: 1895 /* valueAttachment */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1896 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1897 0, 1, value); 1898 case 1755241690: 1899 /* valueReference */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1900 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1901 0, 1, value); 1902 case -934964668: 1903 /* reason */ return new Property("reason", "CodeableConcept", 1904 "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, 1905 reason); 1906 default: 1907 return super.getNamedProperty(_hash, _name, _checkValid); 1908 } 1909 1910 } 1911 1912 @Override 1913 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1914 switch (hash) { 1915 case 1349547969: 1916 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 1917 case 50511102: 1918 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1919 case 3059181: 1920 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1921 case -873664438: 1922 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 1923 case 111972721: 1924 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1925 case -934964668: 1926 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 1927 default: 1928 return super.getProperty(hash, name, checkValid); 1929 } 1930 1931 } 1932 1933 @Override 1934 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1935 switch (hash) { 1936 case 1349547969: // sequence 1937 this.sequence = castToPositiveInt(value); // PositiveIntType 1938 return value; 1939 case 50511102: // category 1940 this.category = castToCodeableConcept(value); // CodeableConcept 1941 return value; 1942 case 3059181: // code 1943 this.code = castToCodeableConcept(value); // CodeableConcept 1944 return value; 1945 case -873664438: // timing 1946 this.timing = castToType(value); // Type 1947 return value; 1948 case 111972721: // value 1949 this.value = castToType(value); // Type 1950 return value; 1951 case -934964668: // reason 1952 this.reason = castToCodeableConcept(value); // CodeableConcept 1953 return value; 1954 default: 1955 return super.setProperty(hash, name, value); 1956 } 1957 1958 } 1959 1960 @Override 1961 public Base setProperty(String name, Base value) throws FHIRException { 1962 if (name.equals("sequence")) { 1963 this.sequence = castToPositiveInt(value); // PositiveIntType 1964 } else if (name.equals("category")) { 1965 this.category = castToCodeableConcept(value); // CodeableConcept 1966 } else if (name.equals("code")) { 1967 this.code = castToCodeableConcept(value); // CodeableConcept 1968 } else if (name.equals("timing[x]")) { 1969 this.timing = castToType(value); // Type 1970 } else if (name.equals("value[x]")) { 1971 this.value = castToType(value); // Type 1972 } else if (name.equals("reason")) { 1973 this.reason = castToCodeableConcept(value); // CodeableConcept 1974 } else 1975 return super.setProperty(name, value); 1976 return value; 1977 } 1978 1979 @Override 1980 public void removeChild(String name, Base value) throws FHIRException { 1981 if (name.equals("sequence")) { 1982 this.sequence = null; 1983 } else if (name.equals("category")) { 1984 this.category = null; 1985 } else if (name.equals("code")) { 1986 this.code = null; 1987 } else if (name.equals("timing[x]")) { 1988 this.timing = null; 1989 } else if (name.equals("value[x]")) { 1990 this.value = null; 1991 } else if (name.equals("reason")) { 1992 this.reason = null; 1993 } else 1994 super.removeChild(name, value); 1995 1996 } 1997 1998 @Override 1999 public Base makeProperty(int hash, String name) throws FHIRException { 2000 switch (hash) { 2001 case 1349547969: 2002 return getSequenceElement(); 2003 case 50511102: 2004 return getCategory(); 2005 case 3059181: 2006 return getCode(); 2007 case 164632566: 2008 return getTiming(); 2009 case -873664438: 2010 return getTiming(); 2011 case -1410166417: 2012 return getValue(); 2013 case 111972721: 2014 return getValue(); 2015 case -934964668: 2016 return getReason(); 2017 default: 2018 return super.makeProperty(hash, name); 2019 } 2020 2021 } 2022 2023 @Override 2024 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2025 switch (hash) { 2026 case 1349547969: 2027 /* sequence */ return new String[] { "positiveInt" }; 2028 case 50511102: 2029 /* category */ return new String[] { "CodeableConcept" }; 2030 case 3059181: 2031 /* code */ return new String[] { "CodeableConcept" }; 2032 case -873664438: 2033 /* timing */ return new String[] { "date", "Period" }; 2034 case 111972721: 2035 /* value */ return new String[] { "boolean", "string", "Quantity", "Attachment", "Reference" }; 2036 case -934964668: 2037 /* reason */ return new String[] { "CodeableConcept" }; 2038 default: 2039 return super.getTypesForProperty(hash, name); 2040 } 2041 2042 } 2043 2044 @Override 2045 public Base addChild(String name) throws FHIRException { 2046 if (name.equals("sequence")) { 2047 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2048 } else if (name.equals("category")) { 2049 this.category = new CodeableConcept(); 2050 return this.category; 2051 } else if (name.equals("code")) { 2052 this.code = new CodeableConcept(); 2053 return this.code; 2054 } else if (name.equals("timingDate")) { 2055 this.timing = new DateType(); 2056 return this.timing; 2057 } else if (name.equals("timingPeriod")) { 2058 this.timing = new Period(); 2059 return this.timing; 2060 } else if (name.equals("valueBoolean")) { 2061 this.value = new BooleanType(); 2062 return this.value; 2063 } else if (name.equals("valueString")) { 2064 this.value = new StringType(); 2065 return this.value; 2066 } else if (name.equals("valueQuantity")) { 2067 this.value = new Quantity(); 2068 return this.value; 2069 } else if (name.equals("valueAttachment")) { 2070 this.value = new Attachment(); 2071 return this.value; 2072 } else if (name.equals("valueReference")) { 2073 this.value = new Reference(); 2074 return this.value; 2075 } else if (name.equals("reason")) { 2076 this.reason = new CodeableConcept(); 2077 return this.reason; 2078 } else 2079 return super.addChild(name); 2080 } 2081 2082 public SupportingInformationComponent copy() { 2083 SupportingInformationComponent dst = new SupportingInformationComponent(); 2084 copyValues(dst); 2085 return dst; 2086 } 2087 2088 public void copyValues(SupportingInformationComponent dst) { 2089 super.copyValues(dst); 2090 dst.sequence = sequence == null ? null : sequence.copy(); 2091 dst.category = category == null ? null : category.copy(); 2092 dst.code = code == null ? null : code.copy(); 2093 dst.timing = timing == null ? null : timing.copy(); 2094 dst.value = value == null ? null : value.copy(); 2095 dst.reason = reason == null ? null : reason.copy(); 2096 } 2097 2098 @Override 2099 public boolean equalsDeep(Base other_) { 2100 if (!super.equalsDeep(other_)) 2101 return false; 2102 if (!(other_ instanceof SupportingInformationComponent)) 2103 return false; 2104 SupportingInformationComponent o = (SupportingInformationComponent) other_; 2105 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) 2106 && compareDeep(code, o.code, true) && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) 2107 && compareDeep(reason, o.reason, true); 2108 } 2109 2110 @Override 2111 public boolean equalsShallow(Base other_) { 2112 if (!super.equalsShallow(other_)) 2113 return false; 2114 if (!(other_ instanceof SupportingInformationComponent)) 2115 return false; 2116 SupportingInformationComponent o = (SupportingInformationComponent) other_; 2117 return compareValues(sequence, o.sequence, true); 2118 } 2119 2120 public boolean isEmpty() { 2121 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code, timing, value, reason); 2122 } 2123 2124 public String fhirType() { 2125 return "Claim.supportingInfo"; 2126 2127 } 2128 2129 } 2130 2131 @Block() 2132 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 2133 /** 2134 * A number to uniquely identify diagnosis entries. 2135 */ 2136 @Child(name = "sequence", type = { 2137 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2138 @Description(shortDefinition = "Diagnosis instance identifier", formalDefinition = "A number to uniquely identify diagnosis entries.") 2139 protected PositiveIntType sequence; 2140 2141 /** 2142 * The nature of illness or problem in a coded form or as a reference to an 2143 * external defined Condition. 2144 */ 2145 @Child(name = "diagnosis", type = { CodeableConcept.class, 2146 Condition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2147 @Description(shortDefinition = "Nature of illness or problem", formalDefinition = "The nature of illness or problem in a coded form or as a reference to an external defined Condition.") 2148 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10") 2149 protected Type diagnosis; 2150 2151 /** 2152 * When the condition was observed or the relative ranking. 2153 */ 2154 @Child(name = "type", type = { 2155 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2156 @Description(shortDefinition = "Timing or nature of the diagnosis", formalDefinition = "When the condition was observed or the relative ranking.") 2157 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosistype") 2158 protected List<CodeableConcept> type; 2159 2160 /** 2161 * Indication of whether the diagnosis was present on admission to a facility. 2162 */ 2163 @Child(name = "onAdmission", type = { 2164 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2165 @Description(shortDefinition = "Present on admission", formalDefinition = "Indication of whether the diagnosis was present on admission to a facility.") 2166 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 2167 protected CodeableConcept onAdmission; 2168 2169 /** 2170 * A package billing code or bundle code used to group products and services to 2171 * a particular health condition (such as heart attack) which is based on a 2172 * predetermined grouping code system. 2173 */ 2174 @Child(name = "packageCode", type = { 2175 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2176 @Description(shortDefinition = "Package billing code", formalDefinition = "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.") 2177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 2178 protected CodeableConcept packageCode; 2179 2180 private static final long serialVersionUID = 2120593974L; 2181 2182 /** 2183 * Constructor 2184 */ 2185 public DiagnosisComponent() { 2186 super(); 2187 } 2188 2189 /** 2190 * Constructor 2191 */ 2192 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 2193 super(); 2194 this.sequence = sequence; 2195 this.diagnosis = diagnosis; 2196 } 2197 2198 /** 2199 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). 2200 * This is the underlying object with id, value and extensions. The 2201 * accessor "getSequence" gives direct access to the value 2202 */ 2203 public PositiveIntType getSequenceElement() { 2204 if (this.sequence == null) 2205 if (Configuration.errorOnAutoCreate()) 2206 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 2207 else if (Configuration.doAutoCreate()) 2208 this.sequence = new PositiveIntType(); // bb 2209 return this.sequence; 2210 } 2211 2212 public boolean hasSequenceElement() { 2213 return this.sequence != null && !this.sequence.isEmpty(); 2214 } 2215 2216 public boolean hasSequence() { 2217 return this.sequence != null && !this.sequence.isEmpty(); 2218 } 2219 2220 /** 2221 * @param value {@link #sequence} (A number to uniquely identify diagnosis 2222 * entries.). This is the underlying object with id, value and 2223 * extensions. The accessor "getSequence" gives direct access to 2224 * the value 2225 */ 2226 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 2227 this.sequence = value; 2228 return this; 2229 } 2230 2231 /** 2232 * @return A number to uniquely identify diagnosis entries. 2233 */ 2234 public int getSequence() { 2235 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2236 } 2237 2238 /** 2239 * @param value A number to uniquely identify diagnosis entries. 2240 */ 2241 public DiagnosisComponent setSequence(int value) { 2242 if (this.sequence == null) 2243 this.sequence = new PositiveIntType(); 2244 this.sequence.setValue(value); 2245 return this; 2246 } 2247 2248 /** 2249 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2250 * or as a reference to an external defined Condition.) 2251 */ 2252 public Type getDiagnosis() { 2253 return this.diagnosis; 2254 } 2255 2256 /** 2257 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2258 * or as a reference to an external defined Condition.) 2259 */ 2260 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 2261 if (this.diagnosis == null) 2262 this.diagnosis = new CodeableConcept(); 2263 if (!(this.diagnosis instanceof CodeableConcept)) 2264 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2265 + this.diagnosis.getClass().getName() + " was encountered"); 2266 return (CodeableConcept) this.diagnosis; 2267 } 2268 2269 public boolean hasDiagnosisCodeableConcept() { 2270 return this != null && this.diagnosis instanceof CodeableConcept; 2271 } 2272 2273 /** 2274 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2275 * or as a reference to an external defined Condition.) 2276 */ 2277 public Reference getDiagnosisReference() throws FHIRException { 2278 if (this.diagnosis == null) 2279 this.diagnosis = new Reference(); 2280 if (!(this.diagnosis instanceof Reference)) 2281 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2282 + this.diagnosis.getClass().getName() + " was encountered"); 2283 return (Reference) this.diagnosis; 2284 } 2285 2286 public boolean hasDiagnosisReference() { 2287 return this != null && this.diagnosis instanceof Reference; 2288 } 2289 2290 public boolean hasDiagnosis() { 2291 return this.diagnosis != null && !this.diagnosis.isEmpty(); 2292 } 2293 2294 /** 2295 * @param value {@link #diagnosis} (The nature of illness or problem in a coded 2296 * form or as a reference to an external defined Condition.) 2297 */ 2298 public DiagnosisComponent setDiagnosis(Type value) { 2299 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2300 throw new Error("Not the right type for Claim.diagnosis.diagnosis[x]: " + value.fhirType()); 2301 this.diagnosis = value; 2302 return this; 2303 } 2304 2305 /** 2306 * @return {@link #type} (When the condition was observed or the relative 2307 * ranking.) 2308 */ 2309 public List<CodeableConcept> getType() { 2310 if (this.type == null) 2311 this.type = new ArrayList<CodeableConcept>(); 2312 return this.type; 2313 } 2314 2315 /** 2316 * @return Returns a reference to <code>this</code> for easy method chaining 2317 */ 2318 public DiagnosisComponent setType(List<CodeableConcept> theType) { 2319 this.type = theType; 2320 return this; 2321 } 2322 2323 public boolean hasType() { 2324 if (this.type == null) 2325 return false; 2326 for (CodeableConcept item : this.type) 2327 if (!item.isEmpty()) 2328 return true; 2329 return false; 2330 } 2331 2332 public CodeableConcept addType() { // 3 2333 CodeableConcept t = new CodeableConcept(); 2334 if (this.type == null) 2335 this.type = new ArrayList<CodeableConcept>(); 2336 this.type.add(t); 2337 return t; 2338 } 2339 2340 public DiagnosisComponent addType(CodeableConcept t) { // 3 2341 if (t == null) 2342 return this; 2343 if (this.type == null) 2344 this.type = new ArrayList<CodeableConcept>(); 2345 this.type.add(t); 2346 return this; 2347 } 2348 2349 /** 2350 * @return The first repetition of repeating field {@link #type}, creating it if 2351 * it does not already exist 2352 */ 2353 public CodeableConcept getTypeFirstRep() { 2354 if (getType().isEmpty()) { 2355 addType(); 2356 } 2357 return getType().get(0); 2358 } 2359 2360 /** 2361 * @return {@link #onAdmission} (Indication of whether the diagnosis was present 2362 * on admission to a facility.) 2363 */ 2364 public CodeableConcept getOnAdmission() { 2365 if (this.onAdmission == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 2368 else if (Configuration.doAutoCreate()) 2369 this.onAdmission = new CodeableConcept(); // cc 2370 return this.onAdmission; 2371 } 2372 2373 public boolean hasOnAdmission() { 2374 return this.onAdmission != null && !this.onAdmission.isEmpty(); 2375 } 2376 2377 /** 2378 * @param value {@link #onAdmission} (Indication of whether the diagnosis was 2379 * present on admission to a facility.) 2380 */ 2381 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 2382 this.onAdmission = value; 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #packageCode} (A package billing code or bundle code used to 2388 * group products and services to a particular health condition (such as 2389 * heart attack) which is based on a predetermined grouping code 2390 * system.) 2391 */ 2392 public CodeableConcept getPackageCode() { 2393 if (this.packageCode == null) 2394 if (Configuration.errorOnAutoCreate()) 2395 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 2396 else if (Configuration.doAutoCreate()) 2397 this.packageCode = new CodeableConcept(); // cc 2398 return this.packageCode; 2399 } 2400 2401 public boolean hasPackageCode() { 2402 return this.packageCode != null && !this.packageCode.isEmpty(); 2403 } 2404 2405 /** 2406 * @param value {@link #packageCode} (A package billing code or bundle code used 2407 * to group products and services to a particular health condition 2408 * (such as heart attack) which is based on a predetermined 2409 * grouping code system.) 2410 */ 2411 public DiagnosisComponent setPackageCode(CodeableConcept value) { 2412 this.packageCode = value; 2413 return this; 2414 } 2415 2416 protected void listChildren(List<Property> children) { 2417 super.listChildren(children); 2418 children.add( 2419 new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 2420 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2421 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, 2422 diagnosis)); 2423 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 2424 0, java.lang.Integer.MAX_VALUE, type)); 2425 children.add(new Property("onAdmission", "CodeableConcept", 2426 "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 2427 children.add(new Property("packageCode", "CodeableConcept", 2428 "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 2429 0, 1, packageCode)); 2430 } 2431 2432 @Override 2433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2434 switch (_hash) { 2435 case 1349547969: 2436 /* sequence */ return new Property("sequence", "positiveInt", 2437 "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 2438 case -1487009809: 2439 /* diagnosis[x] */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2440 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2441 1, diagnosis); 2442 case 1196993265: 2443 /* diagnosis */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2444 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2445 1, diagnosis); 2446 case 277781616: 2447 /* diagnosisCodeableConcept */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2448 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2449 1, diagnosis); 2450 case 2050454362: 2451 /* diagnosisReference */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2452 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2453 1, diagnosis); 2454 case 3575610: 2455 /* type */ return new Property("type", "CodeableConcept", 2456 "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2457 case -3386134: 2458 /* onAdmission */ return new Property("onAdmission", "CodeableConcept", 2459 "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 2460 case 908444499: 2461 /* packageCode */ return new Property("packageCode", "CodeableConcept", 2462 "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 2463 0, 1, packageCode); 2464 default: 2465 return super.getNamedProperty(_hash, _name, _checkValid); 2466 } 2467 2468 } 2469 2470 @Override 2471 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2472 switch (hash) { 2473 case 1349547969: 2474 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 2475 case 1196993265: 2476 /* diagnosis */ return this.diagnosis == null ? new Base[0] : new Base[] { this.diagnosis }; // Type 2477 case 3575610: 2478 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2479 case -3386134: 2480 /* onAdmission */ return this.onAdmission == null ? new Base[0] : new Base[] { this.onAdmission }; // CodeableConcept 2481 case 908444499: 2482 /* packageCode */ return this.packageCode == null ? new Base[0] : new Base[] { this.packageCode }; // CodeableConcept 2483 default: 2484 return super.getProperty(hash, name, checkValid); 2485 } 2486 2487 } 2488 2489 @Override 2490 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2491 switch (hash) { 2492 case 1349547969: // sequence 2493 this.sequence = castToPositiveInt(value); // PositiveIntType 2494 return value; 2495 case 1196993265: // diagnosis 2496 this.diagnosis = castToType(value); // Type 2497 return value; 2498 case 3575610: // type 2499 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2500 return value; 2501 case -3386134: // onAdmission 2502 this.onAdmission = castToCodeableConcept(value); // CodeableConcept 2503 return value; 2504 case 908444499: // packageCode 2505 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2506 return value; 2507 default: 2508 return super.setProperty(hash, name, value); 2509 } 2510 2511 } 2512 2513 @Override 2514 public Base setProperty(String name, Base value) throws FHIRException { 2515 if (name.equals("sequence")) { 2516 this.sequence = castToPositiveInt(value); // PositiveIntType 2517 } else if (name.equals("diagnosis[x]")) { 2518 this.diagnosis = castToType(value); // Type 2519 } else if (name.equals("type")) { 2520 this.getType().add(castToCodeableConcept(value)); 2521 } else if (name.equals("onAdmission")) { 2522 this.onAdmission = castToCodeableConcept(value); // CodeableConcept 2523 } else if (name.equals("packageCode")) { 2524 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2525 } else 2526 return super.setProperty(name, value); 2527 return value; 2528 } 2529 2530 @Override 2531 public void removeChild(String name, Base value) throws FHIRException { 2532 if (name.equals("sequence")) { 2533 this.sequence = null; 2534 } else if (name.equals("diagnosis[x]")) { 2535 this.diagnosis = null; 2536 } else if (name.equals("type")) { 2537 this.getType().remove(castToCodeableConcept(value)); 2538 } else if (name.equals("onAdmission")) { 2539 this.onAdmission = null; 2540 } else if (name.equals("packageCode")) { 2541 this.packageCode = null; 2542 } else 2543 super.removeChild(name, value); 2544 2545 } 2546 2547 @Override 2548 public Base makeProperty(int hash, String name) throws FHIRException { 2549 switch (hash) { 2550 case 1349547969: 2551 return getSequenceElement(); 2552 case -1487009809: 2553 return getDiagnosis(); 2554 case 1196993265: 2555 return getDiagnosis(); 2556 case 3575610: 2557 return addType(); 2558 case -3386134: 2559 return getOnAdmission(); 2560 case 908444499: 2561 return getPackageCode(); 2562 default: 2563 return super.makeProperty(hash, name); 2564 } 2565 2566 } 2567 2568 @Override 2569 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2570 switch (hash) { 2571 case 1349547969: 2572 /* sequence */ return new String[] { "positiveInt" }; 2573 case 1196993265: 2574 /* diagnosis */ return new String[] { "CodeableConcept", "Reference" }; 2575 case 3575610: 2576 /* type */ return new String[] { "CodeableConcept" }; 2577 case -3386134: 2578 /* onAdmission */ return new String[] { "CodeableConcept" }; 2579 case 908444499: 2580 /* packageCode */ return new String[] { "CodeableConcept" }; 2581 default: 2582 return super.getTypesForProperty(hash, name); 2583 } 2584 2585 } 2586 2587 @Override 2588 public Base addChild(String name) throws FHIRException { 2589 if (name.equals("sequence")) { 2590 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2591 } else if (name.equals("diagnosisCodeableConcept")) { 2592 this.diagnosis = new CodeableConcept(); 2593 return this.diagnosis; 2594 } else if (name.equals("diagnosisReference")) { 2595 this.diagnosis = new Reference(); 2596 return this.diagnosis; 2597 } else if (name.equals("type")) { 2598 return addType(); 2599 } else if (name.equals("onAdmission")) { 2600 this.onAdmission = new CodeableConcept(); 2601 return this.onAdmission; 2602 } else if (name.equals("packageCode")) { 2603 this.packageCode = new CodeableConcept(); 2604 return this.packageCode; 2605 } else 2606 return super.addChild(name); 2607 } 2608 2609 public DiagnosisComponent copy() { 2610 DiagnosisComponent dst = new DiagnosisComponent(); 2611 copyValues(dst); 2612 return dst; 2613 } 2614 2615 public void copyValues(DiagnosisComponent dst) { 2616 super.copyValues(dst); 2617 dst.sequence = sequence == null ? null : sequence.copy(); 2618 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2619 if (type != null) { 2620 dst.type = new ArrayList<CodeableConcept>(); 2621 for (CodeableConcept i : type) 2622 dst.type.add(i.copy()); 2623 } 2624 ; 2625 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2626 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2627 } 2628 2629 @Override 2630 public boolean equalsDeep(Base other_) { 2631 if (!super.equalsDeep(other_)) 2632 return false; 2633 if (!(other_ instanceof DiagnosisComponent)) 2634 return false; 2635 DiagnosisComponent o = (DiagnosisComponent) other_; 2636 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) 2637 && compareDeep(type, o.type, true) && compareDeep(onAdmission, o.onAdmission, true) 2638 && compareDeep(packageCode, o.packageCode, true); 2639 } 2640 2641 @Override 2642 public boolean equalsShallow(Base other_) { 2643 if (!super.equalsShallow(other_)) 2644 return false; 2645 if (!(other_ instanceof DiagnosisComponent)) 2646 return false; 2647 DiagnosisComponent o = (DiagnosisComponent) other_; 2648 return compareValues(sequence, o.sequence, true); 2649 } 2650 2651 public boolean isEmpty() { 2652 return super.isEmpty() 2653 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type, onAdmission, packageCode); 2654 } 2655 2656 public String fhirType() { 2657 return "Claim.diagnosis"; 2658 2659 } 2660 2661 } 2662 2663 @Block() 2664 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2665 /** 2666 * A number to uniquely identify procedure entries. 2667 */ 2668 @Child(name = "sequence", type = { 2669 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2670 @Description(shortDefinition = "Procedure instance identifier", formalDefinition = "A number to uniquely identify procedure entries.") 2671 protected PositiveIntType sequence; 2672 2673 /** 2674 * When the condition was observed or the relative ranking. 2675 */ 2676 @Child(name = "type", type = { 2677 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2678 @Description(shortDefinition = "Category of Procedure", formalDefinition = "When the condition was observed or the relative ranking.") 2679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-procedure-type") 2680 protected List<CodeableConcept> type; 2681 2682 /** 2683 * Date and optionally time the procedure was performed. 2684 */ 2685 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2686 @Description(shortDefinition = "When the procedure was performed", formalDefinition = "Date and optionally time the procedure was performed.") 2687 protected DateTimeType date; 2688 2689 /** 2690 * The code or reference to a Procedure resource which identifies the clinical 2691 * intervention performed. 2692 */ 2693 @Child(name = "procedure", type = { CodeableConcept.class, 2694 Procedure.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 2695 @Description(shortDefinition = "Specific clinical procedure", formalDefinition = "The code or reference to a Procedure resource which identifies the clinical intervention performed.") 2696 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10-procedures") 2697 protected Type procedure; 2698 2699 /** 2700 * Unique Device Identifiers associated with this line item. 2701 */ 2702 @Child(name = "udi", type = { 2703 Device.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2704 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 2705 protected List<Reference> udi; 2706 /** 2707 * The actual objects that are the target of the reference (Unique Device 2708 * Identifiers associated with this line item.) 2709 */ 2710 protected List<Device> udiTarget; 2711 2712 private static final long serialVersionUID = 935341852L; 2713 2714 /** 2715 * Constructor 2716 */ 2717 public ProcedureComponent() { 2718 super(); 2719 } 2720 2721 /** 2722 * Constructor 2723 */ 2724 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2725 super(); 2726 this.sequence = sequence; 2727 this.procedure = procedure; 2728 } 2729 2730 /** 2731 * @return {@link #sequence} (A number to uniquely identify procedure entries.). 2732 * This is the underlying object with id, value and extensions. The 2733 * accessor "getSequence" gives direct access to the value 2734 */ 2735 public PositiveIntType getSequenceElement() { 2736 if (this.sequence == null) 2737 if (Configuration.errorOnAutoCreate()) 2738 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2739 else if (Configuration.doAutoCreate()) 2740 this.sequence = new PositiveIntType(); // bb 2741 return this.sequence; 2742 } 2743 2744 public boolean hasSequenceElement() { 2745 return this.sequence != null && !this.sequence.isEmpty(); 2746 } 2747 2748 public boolean hasSequence() { 2749 return this.sequence != null && !this.sequence.isEmpty(); 2750 } 2751 2752 /** 2753 * @param value {@link #sequence} (A number to uniquely identify procedure 2754 * entries.). This is the underlying object with id, value and 2755 * extensions. The accessor "getSequence" gives direct access to 2756 * the value 2757 */ 2758 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2759 this.sequence = value; 2760 return this; 2761 } 2762 2763 /** 2764 * @return A number to uniquely identify procedure entries. 2765 */ 2766 public int getSequence() { 2767 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2768 } 2769 2770 /** 2771 * @param value A number to uniquely identify procedure entries. 2772 */ 2773 public ProcedureComponent setSequence(int value) { 2774 if (this.sequence == null) 2775 this.sequence = new PositiveIntType(); 2776 this.sequence.setValue(value); 2777 return this; 2778 } 2779 2780 /** 2781 * @return {@link #type} (When the condition was observed or the relative 2782 * ranking.) 2783 */ 2784 public List<CodeableConcept> getType() { 2785 if (this.type == null) 2786 this.type = new ArrayList<CodeableConcept>(); 2787 return this.type; 2788 } 2789 2790 /** 2791 * @return Returns a reference to <code>this</code> for easy method chaining 2792 */ 2793 public ProcedureComponent setType(List<CodeableConcept> theType) { 2794 this.type = theType; 2795 return this; 2796 } 2797 2798 public boolean hasType() { 2799 if (this.type == null) 2800 return false; 2801 for (CodeableConcept item : this.type) 2802 if (!item.isEmpty()) 2803 return true; 2804 return false; 2805 } 2806 2807 public CodeableConcept addType() { // 3 2808 CodeableConcept t = new CodeableConcept(); 2809 if (this.type == null) 2810 this.type = new ArrayList<CodeableConcept>(); 2811 this.type.add(t); 2812 return t; 2813 } 2814 2815 public ProcedureComponent addType(CodeableConcept t) { // 3 2816 if (t == null) 2817 return this; 2818 if (this.type == null) 2819 this.type = new ArrayList<CodeableConcept>(); 2820 this.type.add(t); 2821 return this; 2822 } 2823 2824 /** 2825 * @return The first repetition of repeating field {@link #type}, creating it if 2826 * it does not already exist 2827 */ 2828 public CodeableConcept getTypeFirstRep() { 2829 if (getType().isEmpty()) { 2830 addType(); 2831 } 2832 return getType().get(0); 2833 } 2834 2835 /** 2836 * @return {@link #date} (Date and optionally time the procedure was 2837 * performed.). This is the underlying object with id, value and 2838 * extensions. The accessor "getDate" gives direct access to the value 2839 */ 2840 public DateTimeType getDateElement() { 2841 if (this.date == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2844 else if (Configuration.doAutoCreate()) 2845 this.date = new DateTimeType(); // bb 2846 return this.date; 2847 } 2848 2849 public boolean hasDateElement() { 2850 return this.date != null && !this.date.isEmpty(); 2851 } 2852 2853 public boolean hasDate() { 2854 return this.date != null && !this.date.isEmpty(); 2855 } 2856 2857 /** 2858 * @param value {@link #date} (Date and optionally time the procedure was 2859 * performed.). This is the underlying object with id, value and 2860 * extensions. The accessor "getDate" gives direct access to the 2861 * value 2862 */ 2863 public ProcedureComponent setDateElement(DateTimeType value) { 2864 this.date = value; 2865 return this; 2866 } 2867 2868 /** 2869 * @return Date and optionally time the procedure was performed. 2870 */ 2871 public Date getDate() { 2872 return this.date == null ? null : this.date.getValue(); 2873 } 2874 2875 /** 2876 * @param value Date and optionally time the procedure was performed. 2877 */ 2878 public ProcedureComponent setDate(Date value) { 2879 if (value == null) 2880 this.date = null; 2881 else { 2882 if (this.date == null) 2883 this.date = new DateTimeType(); 2884 this.date.setValue(value); 2885 } 2886 return this; 2887 } 2888 2889 /** 2890 * @return {@link #procedure} (The code or reference to a Procedure resource 2891 * which identifies the clinical intervention performed.) 2892 */ 2893 public Type getProcedure() { 2894 return this.procedure; 2895 } 2896 2897 /** 2898 * @return {@link #procedure} (The code or reference to a Procedure resource 2899 * which identifies the clinical intervention performed.) 2900 */ 2901 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2902 if (this.procedure == null) 2903 this.procedure = new CodeableConcept(); 2904 if (!(this.procedure instanceof CodeableConcept)) 2905 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2906 + this.procedure.getClass().getName() + " was encountered"); 2907 return (CodeableConcept) this.procedure; 2908 } 2909 2910 public boolean hasProcedureCodeableConcept() { 2911 return this != null && this.procedure instanceof CodeableConcept; 2912 } 2913 2914 /** 2915 * @return {@link #procedure} (The code or reference to a Procedure resource 2916 * which identifies the clinical intervention performed.) 2917 */ 2918 public Reference getProcedureReference() throws FHIRException { 2919 if (this.procedure == null) 2920 this.procedure = new Reference(); 2921 if (!(this.procedure instanceof Reference)) 2922 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2923 + this.procedure.getClass().getName() + " was encountered"); 2924 return (Reference) this.procedure; 2925 } 2926 2927 public boolean hasProcedureReference() { 2928 return this != null && this.procedure instanceof Reference; 2929 } 2930 2931 public boolean hasProcedure() { 2932 return this.procedure != null && !this.procedure.isEmpty(); 2933 } 2934 2935 /** 2936 * @param value {@link #procedure} (The code or reference to a Procedure 2937 * resource which identifies the clinical intervention performed.) 2938 */ 2939 public ProcedureComponent setProcedure(Type value) { 2940 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2941 throw new Error("Not the right type for Claim.procedure.procedure[x]: " + value.fhirType()); 2942 this.procedure = value; 2943 return this; 2944 } 2945 2946 /** 2947 * @return {@link #udi} (Unique Device Identifiers associated with this line 2948 * item.) 2949 */ 2950 public List<Reference> getUdi() { 2951 if (this.udi == null) 2952 this.udi = new ArrayList<Reference>(); 2953 return this.udi; 2954 } 2955 2956 /** 2957 * @return Returns a reference to <code>this</code> for easy method chaining 2958 */ 2959 public ProcedureComponent setUdi(List<Reference> theUdi) { 2960 this.udi = theUdi; 2961 return this; 2962 } 2963 2964 public boolean hasUdi() { 2965 if (this.udi == null) 2966 return false; 2967 for (Reference item : this.udi) 2968 if (!item.isEmpty()) 2969 return true; 2970 return false; 2971 } 2972 2973 public Reference addUdi() { // 3 2974 Reference t = new Reference(); 2975 if (this.udi == null) 2976 this.udi = new ArrayList<Reference>(); 2977 this.udi.add(t); 2978 return t; 2979 } 2980 2981 public ProcedureComponent addUdi(Reference t) { // 3 2982 if (t == null) 2983 return this; 2984 if (this.udi == null) 2985 this.udi = new ArrayList<Reference>(); 2986 this.udi.add(t); 2987 return this; 2988 } 2989 2990 /** 2991 * @return The first repetition of repeating field {@link #udi}, creating it if 2992 * it does not already exist 2993 */ 2994 public Reference getUdiFirstRep() { 2995 if (getUdi().isEmpty()) { 2996 addUdi(); 2997 } 2998 return getUdi().get(0); 2999 } 3000 3001 /** 3002 * @deprecated Use Reference#setResource(IBaseResource) instead 3003 */ 3004 @Deprecated 3005 public List<Device> getUdiTarget() { 3006 if (this.udiTarget == null) 3007 this.udiTarget = new ArrayList<Device>(); 3008 return this.udiTarget; 3009 } 3010 3011 /** 3012 * @deprecated Use Reference#setResource(IBaseResource) instead 3013 */ 3014 @Deprecated 3015 public Device addUdiTarget() { 3016 Device r = new Device(); 3017 if (this.udiTarget == null) 3018 this.udiTarget = new ArrayList<Device>(); 3019 this.udiTarget.add(r); 3020 return r; 3021 } 3022 3023 protected void listChildren(List<Property> children) { 3024 super.listChildren(children); 3025 children.add( 3026 new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 3027 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 3028 0, java.lang.Integer.MAX_VALUE, type)); 3029 children 3030 .add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 3031 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3032 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3033 procedure)); 3034 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 3035 0, java.lang.Integer.MAX_VALUE, udi)); 3036 } 3037 3038 @Override 3039 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3040 switch (_hash) { 3041 case 1349547969: 3042 /* sequence */ return new Property("sequence", "positiveInt", 3043 "A number to uniquely identify procedure entries.", 0, 1, sequence); 3044 case 3575610: 3045 /* type */ return new Property("type", "CodeableConcept", 3046 "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 3047 case 3076014: 3048 /* date */ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 3049 1, date); 3050 case 1640074445: 3051 /* procedure[x] */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3052 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3053 procedure); 3054 case -1095204141: 3055 /* procedure */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3056 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3057 procedure); 3058 case -1284783026: 3059 /* procedureCodeableConcept */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3060 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3061 procedure); 3062 case 881809848: 3063 /* procedureReference */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3064 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3065 procedure); 3066 case 115642: 3067 /* udi */ return new Property("udi", "Reference(Device)", 3068 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 3069 default: 3070 return super.getNamedProperty(_hash, _name, _checkValid); 3071 } 3072 3073 } 3074 3075 @Override 3076 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3077 switch (hash) { 3078 case 1349547969: 3079 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 3080 case 3575610: 3081 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3082 case 3076014: 3083 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3084 case -1095204141: 3085 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // Type 3086 case 115642: 3087 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 3088 default: 3089 return super.getProperty(hash, name, checkValid); 3090 } 3091 3092 } 3093 3094 @Override 3095 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3096 switch (hash) { 3097 case 1349547969: // sequence 3098 this.sequence = castToPositiveInt(value); // PositiveIntType 3099 return value; 3100 case 3575610: // type 3101 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3102 return value; 3103 case 3076014: // date 3104 this.date = castToDateTime(value); // DateTimeType 3105 return value; 3106 case -1095204141: // procedure 3107 this.procedure = castToType(value); // Type 3108 return value; 3109 case 115642: // udi 3110 this.getUdi().add(castToReference(value)); // Reference 3111 return value; 3112 default: 3113 return super.setProperty(hash, name, value); 3114 } 3115 3116 } 3117 3118 @Override 3119 public Base setProperty(String name, Base value) throws FHIRException { 3120 if (name.equals("sequence")) { 3121 this.sequence = castToPositiveInt(value); // PositiveIntType 3122 } else if (name.equals("type")) { 3123 this.getType().add(castToCodeableConcept(value)); 3124 } else if (name.equals("date")) { 3125 this.date = castToDateTime(value); // DateTimeType 3126 } else if (name.equals("procedure[x]")) { 3127 this.procedure = castToType(value); // Type 3128 } else if (name.equals("udi")) { 3129 this.getUdi().add(castToReference(value)); 3130 } else 3131 return super.setProperty(name, value); 3132 return value; 3133 } 3134 3135 @Override 3136 public void removeChild(String name, Base value) throws FHIRException { 3137 if (name.equals("sequence")) { 3138 this.sequence = null; 3139 } else if (name.equals("type")) { 3140 this.getType().remove(castToCodeableConcept(value)); 3141 } else if (name.equals("date")) { 3142 this.date = null; 3143 } else if (name.equals("procedure[x]")) { 3144 this.procedure = null; 3145 } else if (name.equals("udi")) { 3146 this.getUdi().remove(castToReference(value)); 3147 } else 3148 super.removeChild(name, value); 3149 3150 } 3151 3152 @Override 3153 public Base makeProperty(int hash, String name) throws FHIRException { 3154 switch (hash) { 3155 case 1349547969: 3156 return getSequenceElement(); 3157 case 3575610: 3158 return addType(); 3159 case 3076014: 3160 return getDateElement(); 3161 case 1640074445: 3162 return getProcedure(); 3163 case -1095204141: 3164 return getProcedure(); 3165 case 115642: 3166 return addUdi(); 3167 default: 3168 return super.makeProperty(hash, name); 3169 } 3170 3171 } 3172 3173 @Override 3174 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3175 switch (hash) { 3176 case 1349547969: 3177 /* sequence */ return new String[] { "positiveInt" }; 3178 case 3575610: 3179 /* type */ return new String[] { "CodeableConcept" }; 3180 case 3076014: 3181 /* date */ return new String[] { "dateTime" }; 3182 case -1095204141: 3183 /* procedure */ return new String[] { "CodeableConcept", "Reference" }; 3184 case 115642: 3185 /* udi */ return new String[] { "Reference" }; 3186 default: 3187 return super.getTypesForProperty(hash, name); 3188 } 3189 3190 } 3191 3192 @Override 3193 public Base addChild(String name) throws FHIRException { 3194 if (name.equals("sequence")) { 3195 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 3196 } else if (name.equals("type")) { 3197 return addType(); 3198 } else if (name.equals("date")) { 3199 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 3200 } else if (name.equals("procedureCodeableConcept")) { 3201 this.procedure = new CodeableConcept(); 3202 return this.procedure; 3203 } else if (name.equals("procedureReference")) { 3204 this.procedure = new Reference(); 3205 return this.procedure; 3206 } else if (name.equals("udi")) { 3207 return addUdi(); 3208 } else 3209 return super.addChild(name); 3210 } 3211 3212 public ProcedureComponent copy() { 3213 ProcedureComponent dst = new ProcedureComponent(); 3214 copyValues(dst); 3215 return dst; 3216 } 3217 3218 public void copyValues(ProcedureComponent dst) { 3219 super.copyValues(dst); 3220 dst.sequence = sequence == null ? null : sequence.copy(); 3221 if (type != null) { 3222 dst.type = new ArrayList<CodeableConcept>(); 3223 for (CodeableConcept i : type) 3224 dst.type.add(i.copy()); 3225 } 3226 ; 3227 dst.date = date == null ? null : date.copy(); 3228 dst.procedure = procedure == null ? null : procedure.copy(); 3229 if (udi != null) { 3230 dst.udi = new ArrayList<Reference>(); 3231 for (Reference i : udi) 3232 dst.udi.add(i.copy()); 3233 } 3234 ; 3235 } 3236 3237 @Override 3238 public boolean equalsDeep(Base other_) { 3239 if (!super.equalsDeep(other_)) 3240 return false; 3241 if (!(other_ instanceof ProcedureComponent)) 3242 return false; 3243 ProcedureComponent o = (ProcedureComponent) other_; 3244 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) 3245 && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 3246 && compareDeep(udi, o.udi, true); 3247 } 3248 3249 @Override 3250 public boolean equalsShallow(Base other_) { 3251 if (!super.equalsShallow(other_)) 3252 return false; 3253 if (!(other_ instanceof ProcedureComponent)) 3254 return false; 3255 ProcedureComponent o = (ProcedureComponent) other_; 3256 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 3257 } 3258 3259 public boolean isEmpty() { 3260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure, udi); 3261 } 3262 3263 public String fhirType() { 3264 return "Claim.procedure"; 3265 3266 } 3267 3268 } 3269 3270 @Block() 3271 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 3272 /** 3273 * A number to uniquely identify insurance entries and provide a sequence of 3274 * coverages to convey coordination of benefit order. 3275 */ 3276 @Child(name = "sequence", type = { 3277 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3278 @Description(shortDefinition = "Insurance instance identifier", formalDefinition = "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.") 3279 protected PositiveIntType sequence; 3280 3281 /** 3282 * A flag to indicate that this Coverage is to be used for adjudication of this 3283 * claim when set to true. 3284 */ 3285 @Child(name = "focal", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3286 @Description(shortDefinition = "Coverage to be used for adjudication", formalDefinition = "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.") 3287 protected BooleanType focal; 3288 3289 /** 3290 * The business identifier to be used when the claim is sent for adjudication 3291 * against this insurance policy. 3292 */ 3293 @Child(name = "identifier", type = { 3294 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3295 @Description(shortDefinition = "Pre-assigned Claim number", formalDefinition = "The business identifier to be used when the claim is sent for adjudication against this insurance policy.") 3296 protected Identifier identifier; 3297 3298 /** 3299 * Reference to the insurance card level information contained in the Coverage 3300 * resource. The coverage issuing insurer will use these details to locate the 3301 * patient's actual coverage within the insurer's information system. 3302 */ 3303 @Child(name = "coverage", type = { Coverage.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3304 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 3305 protected Reference coverage; 3306 3307 /** 3308 * The actual object that is the target of the reference (Reference to the 3309 * insurance card level information contained in the Coverage resource. The 3310 * coverage issuing insurer will use these details to locate the patient's 3311 * actual coverage within the insurer's information system.) 3312 */ 3313 protected Coverage coverageTarget; 3314 3315 /** 3316 * A business agreement number established between the provider and the insurer 3317 * for special business processing purposes. 3318 */ 3319 @Child(name = "businessArrangement", type = { 3320 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3321 @Description(shortDefinition = "Additional provider contract number", formalDefinition = "A business agreement number established between the provider and the insurer for special business processing purposes.") 3322 protected StringType businessArrangement; 3323 3324 /** 3325 * Reference numbers previously provided by the insurer to the provider to be 3326 * quoted on subsequent claims containing services or products related to the 3327 * prior authorization. 3328 */ 3329 @Child(name = "preAuthRef", type = { 3330 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3331 @Description(shortDefinition = "Prior authorization reference number", formalDefinition = "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.") 3332 protected List<StringType> preAuthRef; 3333 3334 /** 3335 * The result of the adjudication of the line items for the Coverage specified 3336 * in this insurance. 3337 */ 3338 @Child(name = "claimResponse", type = { 3339 ClaimResponse.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3340 @Description(shortDefinition = "Adjudication results", formalDefinition = "The result of the adjudication of the line items for the Coverage specified in this insurance.") 3341 protected Reference claimResponse; 3342 3343 /** 3344 * The actual object that is the target of the reference (The result of the 3345 * adjudication of the line items for the Coverage specified in this insurance.) 3346 */ 3347 protected ClaimResponse claimResponseTarget; 3348 3349 private static final long serialVersionUID = -1711744215L; 3350 3351 /** 3352 * Constructor 3353 */ 3354 public InsuranceComponent() { 3355 super(); 3356 } 3357 3358 /** 3359 * Constructor 3360 */ 3361 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 3362 super(); 3363 this.sequence = sequence; 3364 this.focal = focal; 3365 this.coverage = coverage; 3366 } 3367 3368 /** 3369 * @return {@link #sequence} (A number to uniquely identify insurance entries 3370 * and provide a sequence of coverages to convey coordination of benefit 3371 * order.). This is the underlying object with id, value and extensions. 3372 * The accessor "getSequence" gives direct access to the value 3373 */ 3374 public PositiveIntType getSequenceElement() { 3375 if (this.sequence == null) 3376 if (Configuration.errorOnAutoCreate()) 3377 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 3378 else if (Configuration.doAutoCreate()) 3379 this.sequence = new PositiveIntType(); // bb 3380 return this.sequence; 3381 } 3382 3383 public boolean hasSequenceElement() { 3384 return this.sequence != null && !this.sequence.isEmpty(); 3385 } 3386 3387 public boolean hasSequence() { 3388 return this.sequence != null && !this.sequence.isEmpty(); 3389 } 3390 3391 /** 3392 * @param value {@link #sequence} (A number to uniquely identify insurance 3393 * entries and provide a sequence of coverages to convey 3394 * coordination of benefit order.). This is the underlying object 3395 * with id, value and extensions. The accessor "getSequence" gives 3396 * direct access to the value 3397 */ 3398 public InsuranceComponent setSequenceElement(PositiveIntType value) { 3399 this.sequence = value; 3400 return this; 3401 } 3402 3403 /** 3404 * @return A number to uniquely identify insurance entries and provide a 3405 * sequence of coverages to convey coordination of benefit order. 3406 */ 3407 public int getSequence() { 3408 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3409 } 3410 3411 /** 3412 * @param value A number to uniquely identify insurance entries and provide a 3413 * sequence of coverages to convey coordination of benefit order. 3414 */ 3415 public InsuranceComponent setSequence(int value) { 3416 if (this.sequence == null) 3417 this.sequence = new PositiveIntType(); 3418 this.sequence.setValue(value); 3419 return this; 3420 } 3421 3422 /** 3423 * @return {@link #focal} (A flag to indicate that this Coverage is to be used 3424 * for adjudication of this claim when set to true.). This is the 3425 * underlying object with id, value and extensions. The accessor 3426 * "getFocal" gives direct access to the value 3427 */ 3428 public BooleanType getFocalElement() { 3429 if (this.focal == null) 3430 if (Configuration.errorOnAutoCreate()) 3431 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 3432 else if (Configuration.doAutoCreate()) 3433 this.focal = new BooleanType(); // bb 3434 return this.focal; 3435 } 3436 3437 public boolean hasFocalElement() { 3438 return this.focal != null && !this.focal.isEmpty(); 3439 } 3440 3441 public boolean hasFocal() { 3442 return this.focal != null && !this.focal.isEmpty(); 3443 } 3444 3445 /** 3446 * @param value {@link #focal} (A flag to indicate that this Coverage is to be 3447 * used for adjudication of this claim when set to true.). This is 3448 * the underlying object with id, value and extensions. The 3449 * accessor "getFocal" gives direct access to the value 3450 */ 3451 public InsuranceComponent setFocalElement(BooleanType value) { 3452 this.focal = value; 3453 return this; 3454 } 3455 3456 /** 3457 * @return A flag to indicate that this Coverage is to be used for adjudication 3458 * of this claim when set to true. 3459 */ 3460 public boolean getFocal() { 3461 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 3462 } 3463 3464 /** 3465 * @param value A flag to indicate that this Coverage is to be used for 3466 * adjudication of this claim when set to true. 3467 */ 3468 public InsuranceComponent setFocal(boolean value) { 3469 if (this.focal == null) 3470 this.focal = new BooleanType(); 3471 this.focal.setValue(value); 3472 return this; 3473 } 3474 3475 /** 3476 * @return {@link #identifier} (The business identifier to be used when the 3477 * claim is sent for adjudication against this insurance policy.) 3478 */ 3479 public Identifier getIdentifier() { 3480 if (this.identifier == null) 3481 if (Configuration.errorOnAutoCreate()) 3482 throw new Error("Attempt to auto-create InsuranceComponent.identifier"); 3483 else if (Configuration.doAutoCreate()) 3484 this.identifier = new Identifier(); // cc 3485 return this.identifier; 3486 } 3487 3488 public boolean hasIdentifier() { 3489 return this.identifier != null && !this.identifier.isEmpty(); 3490 } 3491 3492 /** 3493 * @param value {@link #identifier} (The business identifier to be used when the 3494 * claim is sent for adjudication against this insurance policy.) 3495 */ 3496 public InsuranceComponent setIdentifier(Identifier value) { 3497 this.identifier = value; 3498 return this; 3499 } 3500 3501 /** 3502 * @return {@link #coverage} (Reference to the insurance card level information 3503 * contained in the Coverage resource. The coverage issuing insurer will 3504 * use these details to locate the patient's actual coverage within the 3505 * insurer's information system.) 3506 */ 3507 public Reference getCoverage() { 3508 if (this.coverage == null) 3509 if (Configuration.errorOnAutoCreate()) 3510 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 3511 else if (Configuration.doAutoCreate()) 3512 this.coverage = new Reference(); // cc 3513 return this.coverage; 3514 } 3515 3516 public boolean hasCoverage() { 3517 return this.coverage != null && !this.coverage.isEmpty(); 3518 } 3519 3520 /** 3521 * @param value {@link #coverage} (Reference to the insurance card level 3522 * information contained in the Coverage resource. The coverage 3523 * issuing insurer will use these details to locate the patient's 3524 * actual coverage within the insurer's information system.) 3525 */ 3526 public InsuranceComponent setCoverage(Reference value) { 3527 this.coverage = value; 3528 return this; 3529 } 3530 3531 /** 3532 * @return {@link #coverage} The actual object that is the target of the 3533 * reference. The reference library doesn't populate this, but you can 3534 * use it to hold the resource if you resolve it. (Reference to the 3535 * insurance card level information contained in the Coverage resource. 3536 * The coverage issuing insurer will use these details to locate the 3537 * patient's actual coverage within the insurer's information system.) 3538 */ 3539 public Coverage getCoverageTarget() { 3540 if (this.coverageTarget == null) 3541 if (Configuration.errorOnAutoCreate()) 3542 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 3543 else if (Configuration.doAutoCreate()) 3544 this.coverageTarget = new Coverage(); // aa 3545 return this.coverageTarget; 3546 } 3547 3548 /** 3549 * @param value {@link #coverage} The actual object that is the target of the 3550 * reference. The reference library doesn't use these, but you can 3551 * use it to hold the resource if you resolve it. (Reference to the 3552 * insurance card level information contained in the Coverage 3553 * resource. The coverage issuing insurer will use these details to 3554 * locate the patient's actual coverage within the insurer's 3555 * information system.) 3556 */ 3557 public InsuranceComponent setCoverageTarget(Coverage value) { 3558 this.coverageTarget = value; 3559 return this; 3560 } 3561 3562 /** 3563 * @return {@link #businessArrangement} (A business agreement number established 3564 * between the provider and the insurer for special business processing 3565 * purposes.). This is the underlying object with id, value and 3566 * extensions. The accessor "getBusinessArrangement" gives direct access 3567 * to the value 3568 */ 3569 public StringType getBusinessArrangementElement() { 3570 if (this.businessArrangement == null) 3571 if (Configuration.errorOnAutoCreate()) 3572 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 3573 else if (Configuration.doAutoCreate()) 3574 this.businessArrangement = new StringType(); // bb 3575 return this.businessArrangement; 3576 } 3577 3578 public boolean hasBusinessArrangementElement() { 3579 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 3580 } 3581 3582 public boolean hasBusinessArrangement() { 3583 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 3584 } 3585 3586 /** 3587 * @param value {@link #businessArrangement} (A business agreement number 3588 * established between the provider and the insurer for special 3589 * business processing purposes.). This is the underlying object 3590 * with id, value and extensions. The accessor 3591 * "getBusinessArrangement" gives direct access to the value 3592 */ 3593 public InsuranceComponent setBusinessArrangementElement(StringType value) { 3594 this.businessArrangement = value; 3595 return this; 3596 } 3597 3598 /** 3599 * @return A business agreement number established between the provider and the 3600 * insurer for special business processing purposes. 3601 */ 3602 public String getBusinessArrangement() { 3603 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 3604 } 3605 3606 /** 3607 * @param value A business agreement number established between the provider and 3608 * the insurer for special business processing purposes. 3609 */ 3610 public InsuranceComponent setBusinessArrangement(String value) { 3611 if (Utilities.noString(value)) 3612 this.businessArrangement = null; 3613 else { 3614 if (this.businessArrangement == null) 3615 this.businessArrangement = new StringType(); 3616 this.businessArrangement.setValue(value); 3617 } 3618 return this; 3619 } 3620 3621 /** 3622 * @return {@link #preAuthRef} (Reference numbers previously provided by the 3623 * insurer to the provider to be quoted on subsequent claims containing 3624 * services or products related to the prior authorization.) 3625 */ 3626 public List<StringType> getPreAuthRef() { 3627 if (this.preAuthRef == null) 3628 this.preAuthRef = new ArrayList<StringType>(); 3629 return this.preAuthRef; 3630 } 3631 3632 /** 3633 * @return Returns a reference to <code>this</code> for easy method chaining 3634 */ 3635 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 3636 this.preAuthRef = thePreAuthRef; 3637 return this; 3638 } 3639 3640 public boolean hasPreAuthRef() { 3641 if (this.preAuthRef == null) 3642 return false; 3643 for (StringType item : this.preAuthRef) 3644 if (!item.isEmpty()) 3645 return true; 3646 return false; 3647 } 3648 3649 /** 3650 * @return {@link #preAuthRef} (Reference numbers previously provided by the 3651 * insurer to the provider to be quoted on subsequent claims containing 3652 * services or products related to the prior authorization.) 3653 */ 3654 public StringType addPreAuthRefElement() {// 2 3655 StringType t = new StringType(); 3656 if (this.preAuthRef == null) 3657 this.preAuthRef = new ArrayList<StringType>(); 3658 this.preAuthRef.add(t); 3659 return t; 3660 } 3661 3662 /** 3663 * @param value {@link #preAuthRef} (Reference numbers previously provided by 3664 * the insurer to the provider to be quoted on subsequent claims 3665 * containing services or products related to the prior 3666 * authorization.) 3667 */ 3668 public InsuranceComponent addPreAuthRef(String value) { // 1 3669 StringType t = new StringType(); 3670 t.setValue(value); 3671 if (this.preAuthRef == null) 3672 this.preAuthRef = new ArrayList<StringType>(); 3673 this.preAuthRef.add(t); 3674 return this; 3675 } 3676 3677 /** 3678 * @param value {@link #preAuthRef} (Reference numbers previously provided by 3679 * the insurer to the provider to be quoted on subsequent claims 3680 * containing services or products related to the prior 3681 * authorization.) 3682 */ 3683 public boolean hasPreAuthRef(String value) { 3684 if (this.preAuthRef == null) 3685 return false; 3686 for (StringType v : this.preAuthRef) 3687 if (v.getValue().equals(value)) // string 3688 return true; 3689 return false; 3690 } 3691 3692 /** 3693 * @return {@link #claimResponse} (The result of the adjudication of the line 3694 * items for the Coverage specified in this insurance.) 3695 */ 3696 public Reference getClaimResponse() { 3697 if (this.claimResponse == null) 3698 if (Configuration.errorOnAutoCreate()) 3699 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 3700 else if (Configuration.doAutoCreate()) 3701 this.claimResponse = new Reference(); // cc 3702 return this.claimResponse; 3703 } 3704 3705 public boolean hasClaimResponse() { 3706 return this.claimResponse != null && !this.claimResponse.isEmpty(); 3707 } 3708 3709 /** 3710 * @param value {@link #claimResponse} (The result of the adjudication of the 3711 * line items for the Coverage specified in this insurance.) 3712 */ 3713 public InsuranceComponent setClaimResponse(Reference value) { 3714 this.claimResponse = value; 3715 return this; 3716 } 3717 3718 /** 3719 * @return {@link #claimResponse} The actual object that is the target of the 3720 * reference. The reference library doesn't populate this, but you can 3721 * use it to hold the resource if you resolve it. (The result of the 3722 * adjudication of the line items for the Coverage specified in this 3723 * insurance.) 3724 */ 3725 public ClaimResponse getClaimResponseTarget() { 3726 if (this.claimResponseTarget == null) 3727 if (Configuration.errorOnAutoCreate()) 3728 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 3729 else if (Configuration.doAutoCreate()) 3730 this.claimResponseTarget = new ClaimResponse(); // aa 3731 return this.claimResponseTarget; 3732 } 3733 3734 /** 3735 * @param value {@link #claimResponse} The actual object that is the target of 3736 * the reference. The reference library doesn't use these, but you 3737 * can use it to hold the resource if you resolve it. (The result 3738 * of the adjudication of the line items for the Coverage specified 3739 * in this insurance.) 3740 */ 3741 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 3742 this.claimResponseTarget = value; 3743 return this; 3744 } 3745 3746 protected void listChildren(List<Property> children) { 3747 super.listChildren(children); 3748 children.add(new Property("sequence", "positiveInt", 3749 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 3750 0, 1, sequence)); 3751 children.add(new Property("focal", "boolean", 3752 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, 3753 focal)); 3754 children.add(new Property("identifier", "Identifier", 3755 "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 3756 0, 1, identifier)); 3757 children.add(new Property("coverage", "Reference(Coverage)", 3758 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 3759 0, 1, coverage)); 3760 children.add(new Property("businessArrangement", "string", 3761 "A business agreement number established between the provider and the insurer for special business processing purposes.", 3762 0, 1, businessArrangement)); 3763 children.add(new Property("preAuthRef", "string", 3764 "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 3765 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 3766 children.add(new Property("claimResponse", "Reference(ClaimResponse)", 3767 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 3768 claimResponse)); 3769 } 3770 3771 @Override 3772 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3773 switch (_hash) { 3774 case 1349547969: 3775 /* sequence */ return new Property("sequence", "positiveInt", 3776 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 3777 0, 1, sequence); 3778 case 97604197: 3779 /* focal */ return new Property("focal", "boolean", 3780 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 3781 1, focal); 3782 case -1618432855: 3783 /* identifier */ return new Property("identifier", "Identifier", 3784 "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 3785 0, 1, identifier); 3786 case -351767064: 3787 /* coverage */ return new Property("coverage", "Reference(Coverage)", 3788 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 3789 0, 1, coverage); 3790 case 259920682: 3791 /* businessArrangement */ return new Property("businessArrangement", "string", 3792 "A business agreement number established between the provider and the insurer for special business processing purposes.", 3793 0, 1, businessArrangement); 3794 case 522246568: 3795 /* preAuthRef */ return new Property("preAuthRef", "string", 3796 "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 3797 0, java.lang.Integer.MAX_VALUE, preAuthRef); 3798 case 689513629: 3799 /* claimResponse */ return new Property("claimResponse", "Reference(ClaimResponse)", 3800 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 3801 claimResponse); 3802 default: 3803 return super.getNamedProperty(_hash, _name, _checkValid); 3804 } 3805 3806 } 3807 3808 @Override 3809 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3810 switch (hash) { 3811 case 1349547969: 3812 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 3813 case 97604197: 3814 /* focal */ return this.focal == null ? new Base[0] : new Base[] { this.focal }; // BooleanType 3815 case -1618432855: 3816 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 3817 case -351767064: 3818 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 3819 case 259920682: 3820 /* businessArrangement */ return this.businessArrangement == null ? new Base[0] 3821 : new Base[] { this.businessArrangement }; // StringType 3822 case 522246568: 3823 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] 3824 : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 3825 case 689513629: 3826 /* claimResponse */ return this.claimResponse == null ? new Base[0] : new Base[] { this.claimResponse }; // Reference 3827 default: 3828 return super.getProperty(hash, name, checkValid); 3829 } 3830 3831 } 3832 3833 @Override 3834 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3835 switch (hash) { 3836 case 1349547969: // sequence 3837 this.sequence = castToPositiveInt(value); // PositiveIntType 3838 return value; 3839 case 97604197: // focal 3840 this.focal = castToBoolean(value); // BooleanType 3841 return value; 3842 case -1618432855: // identifier 3843 this.identifier = castToIdentifier(value); // Identifier 3844 return value; 3845 case -351767064: // coverage 3846 this.coverage = castToReference(value); // Reference 3847 return value; 3848 case 259920682: // businessArrangement 3849 this.businessArrangement = castToString(value); // StringType 3850 return value; 3851 case 522246568: // preAuthRef 3852 this.getPreAuthRef().add(castToString(value)); // StringType 3853 return value; 3854 case 689513629: // claimResponse 3855 this.claimResponse = castToReference(value); // Reference 3856 return value; 3857 default: 3858 return super.setProperty(hash, name, value); 3859 } 3860 3861 } 3862 3863 @Override 3864 public Base setProperty(String name, Base value) throws FHIRException { 3865 if (name.equals("sequence")) { 3866 this.sequence = castToPositiveInt(value); // PositiveIntType 3867 } else if (name.equals("focal")) { 3868 this.focal = castToBoolean(value); // BooleanType 3869 } else if (name.equals("identifier")) { 3870 this.identifier = castToIdentifier(value); // Identifier 3871 } else if (name.equals("coverage")) { 3872 this.coverage = castToReference(value); // Reference 3873 } else if (name.equals("businessArrangement")) { 3874 this.businessArrangement = castToString(value); // StringType 3875 } else if (name.equals("preAuthRef")) { 3876 this.getPreAuthRef().add(castToString(value)); 3877 } else if (name.equals("claimResponse")) { 3878 this.claimResponse = castToReference(value); // Reference 3879 } else 3880 return super.setProperty(name, value); 3881 return value; 3882 } 3883 3884 @Override 3885 public void removeChild(String name, Base value) throws FHIRException { 3886 if (name.equals("sequence")) { 3887 this.sequence = null; 3888 } else if (name.equals("focal")) { 3889 this.focal = null; 3890 } else if (name.equals("identifier")) { 3891 this.identifier = null; 3892 } else if (name.equals("coverage")) { 3893 this.coverage = null; 3894 } else if (name.equals("businessArrangement")) { 3895 this.businessArrangement = null; 3896 } else if (name.equals("preAuthRef")) { 3897 this.getPreAuthRef().remove(castToString(value)); 3898 } else if (name.equals("claimResponse")) { 3899 this.claimResponse = null; 3900 } else 3901 super.removeChild(name, value); 3902 3903 } 3904 3905 @Override 3906 public Base makeProperty(int hash, String name) throws FHIRException { 3907 switch (hash) { 3908 case 1349547969: 3909 return getSequenceElement(); 3910 case 97604197: 3911 return getFocalElement(); 3912 case -1618432855: 3913 return getIdentifier(); 3914 case -351767064: 3915 return getCoverage(); 3916 case 259920682: 3917 return getBusinessArrangementElement(); 3918 case 522246568: 3919 return addPreAuthRefElement(); 3920 case 689513629: 3921 return getClaimResponse(); 3922 default: 3923 return super.makeProperty(hash, name); 3924 } 3925 3926 } 3927 3928 @Override 3929 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3930 switch (hash) { 3931 case 1349547969: 3932 /* sequence */ return new String[] { "positiveInt" }; 3933 case 97604197: 3934 /* focal */ return new String[] { "boolean" }; 3935 case -1618432855: 3936 /* identifier */ return new String[] { "Identifier" }; 3937 case -351767064: 3938 /* coverage */ return new String[] { "Reference" }; 3939 case 259920682: 3940 /* businessArrangement */ return new String[] { "string" }; 3941 case 522246568: 3942 /* preAuthRef */ return new String[] { "string" }; 3943 case 689513629: 3944 /* claimResponse */ return new String[] { "Reference" }; 3945 default: 3946 return super.getTypesForProperty(hash, name); 3947 } 3948 3949 } 3950 3951 @Override 3952 public Base addChild(String name) throws FHIRException { 3953 if (name.equals("sequence")) { 3954 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 3955 } else if (name.equals("focal")) { 3956 throw new FHIRException("Cannot call addChild on a singleton property Claim.focal"); 3957 } else if (name.equals("identifier")) { 3958 this.identifier = new Identifier(); 3959 return this.identifier; 3960 } else if (name.equals("coverage")) { 3961 this.coverage = new Reference(); 3962 return this.coverage; 3963 } else if (name.equals("businessArrangement")) { 3964 throw new FHIRException("Cannot call addChild on a singleton property Claim.businessArrangement"); 3965 } else if (name.equals("preAuthRef")) { 3966 throw new FHIRException("Cannot call addChild on a singleton property Claim.preAuthRef"); 3967 } else if (name.equals("claimResponse")) { 3968 this.claimResponse = new Reference(); 3969 return this.claimResponse; 3970 } else 3971 return super.addChild(name); 3972 } 3973 3974 public InsuranceComponent copy() { 3975 InsuranceComponent dst = new InsuranceComponent(); 3976 copyValues(dst); 3977 return dst; 3978 } 3979 3980 public void copyValues(InsuranceComponent dst) { 3981 super.copyValues(dst); 3982 dst.sequence = sequence == null ? null : sequence.copy(); 3983 dst.focal = focal == null ? null : focal.copy(); 3984 dst.identifier = identifier == null ? null : identifier.copy(); 3985 dst.coverage = coverage == null ? null : coverage.copy(); 3986 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3987 if (preAuthRef != null) { 3988 dst.preAuthRef = new ArrayList<StringType>(); 3989 for (StringType i : preAuthRef) 3990 dst.preAuthRef.add(i.copy()); 3991 } 3992 ; 3993 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3994 } 3995 3996 @Override 3997 public boolean equalsDeep(Base other_) { 3998 if (!super.equalsDeep(other_)) 3999 return false; 4000 if (!(other_ instanceof InsuranceComponent)) 4001 return false; 4002 InsuranceComponent o = (InsuranceComponent) other_; 4003 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) 4004 && compareDeep(identifier, o.identifier, true) && compareDeep(coverage, o.coverage, true) 4005 && compareDeep(businessArrangement, o.businessArrangement, true) 4006 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(claimResponse, o.claimResponse, true); 4007 } 4008 4009 @Override 4010 public boolean equalsShallow(Base other_) { 4011 if (!super.equalsShallow(other_)) 4012 return false; 4013 if (!(other_ instanceof InsuranceComponent)) 4014 return false; 4015 InsuranceComponent o = (InsuranceComponent) other_; 4016 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) 4017 && compareValues(businessArrangement, o.businessArrangement, true) 4018 && compareValues(preAuthRef, o.preAuthRef, true); 4019 } 4020 4021 public boolean isEmpty() { 4022 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, identifier, coverage, 4023 businessArrangement, preAuthRef, claimResponse); 4024 } 4025 4026 public String fhirType() { 4027 return "Claim.insurance"; 4028 4029 } 4030 4031 } 4032 4033 @Block() 4034 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 4035 /** 4036 * Date of an accident event related to the products and services contained in 4037 * the claim. 4038 */ 4039 @Child(name = "date", type = { DateType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4040 @Description(shortDefinition = "When the incident occurred", formalDefinition = "Date of an accident event related to the products and services contained in the claim.") 4041 protected DateType date; 4042 4043 /** 4044 * The type or context of the accident event for the purposes of selection of 4045 * potential insurance coverages and determination of coordination between 4046 * insurers. 4047 */ 4048 @Child(name = "type", type = { 4049 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4050 @Description(shortDefinition = "The nature of the accident", formalDefinition = "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.") 4051 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 4052 protected CodeableConcept type; 4053 4054 /** 4055 * The physical location of the accident event. 4056 */ 4057 @Child(name = "location", type = { Address.class, 4058 Location.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4059 @Description(shortDefinition = "Where the event occurred", formalDefinition = "The physical location of the accident event.") 4060 protected Type location; 4061 4062 private static final long serialVersionUID = 622904984L; 4063 4064 /** 4065 * Constructor 4066 */ 4067 public AccidentComponent() { 4068 super(); 4069 } 4070 4071 /** 4072 * Constructor 4073 */ 4074 public AccidentComponent(DateType date) { 4075 super(); 4076 this.date = date; 4077 } 4078 4079 /** 4080 * @return {@link #date} (Date of an accident event related to the products and 4081 * services contained in the claim.). This is the underlying object with 4082 * id, value and extensions. The accessor "getDate" gives direct access 4083 * to the value 4084 */ 4085 public DateType getDateElement() { 4086 if (this.date == null) 4087 if (Configuration.errorOnAutoCreate()) 4088 throw new Error("Attempt to auto-create AccidentComponent.date"); 4089 else if (Configuration.doAutoCreate()) 4090 this.date = new DateType(); // bb 4091 return this.date; 4092 } 4093 4094 public boolean hasDateElement() { 4095 return this.date != null && !this.date.isEmpty(); 4096 } 4097 4098 public boolean hasDate() { 4099 return this.date != null && !this.date.isEmpty(); 4100 } 4101 4102 /** 4103 * @param value {@link #date} (Date of an accident event related to the products 4104 * and services contained in the claim.). This is the underlying 4105 * object with id, value and extensions. The accessor "getDate" 4106 * gives direct access to the value 4107 */ 4108 public AccidentComponent setDateElement(DateType value) { 4109 this.date = value; 4110 return this; 4111 } 4112 4113 /** 4114 * @return Date of an accident event related to the products and services 4115 * contained in the claim. 4116 */ 4117 public Date getDate() { 4118 return this.date == null ? null : this.date.getValue(); 4119 } 4120 4121 /** 4122 * @param value Date of an accident event related to the products and services 4123 * contained in the claim. 4124 */ 4125 public AccidentComponent setDate(Date value) { 4126 if (this.date == null) 4127 this.date = new DateType(); 4128 this.date.setValue(value); 4129 return this; 4130 } 4131 4132 /** 4133 * @return {@link #type} (The type or context of the accident event for the 4134 * purposes of selection of potential insurance coverages and 4135 * determination of coordination between insurers.) 4136 */ 4137 public CodeableConcept getType() { 4138 if (this.type == null) 4139 if (Configuration.errorOnAutoCreate()) 4140 throw new Error("Attempt to auto-create AccidentComponent.type"); 4141 else if (Configuration.doAutoCreate()) 4142 this.type = new CodeableConcept(); // cc 4143 return this.type; 4144 } 4145 4146 public boolean hasType() { 4147 return this.type != null && !this.type.isEmpty(); 4148 } 4149 4150 /** 4151 * @param value {@link #type} (The type or context of the accident event for the 4152 * purposes of selection of potential insurance coverages and 4153 * determination of coordination between insurers.) 4154 */ 4155 public AccidentComponent setType(CodeableConcept value) { 4156 this.type = value; 4157 return this; 4158 } 4159 4160 /** 4161 * @return {@link #location} (The physical location of the accident event.) 4162 */ 4163 public Type getLocation() { 4164 return this.location; 4165 } 4166 4167 /** 4168 * @return {@link #location} (The physical location of the accident event.) 4169 */ 4170 public Address getLocationAddress() throws FHIRException { 4171 if (this.location == null) 4172 this.location = new Address(); 4173 if (!(this.location instanceof Address)) 4174 throw new FHIRException("Type mismatch: the type Address was expected, but " 4175 + this.location.getClass().getName() + " was encountered"); 4176 return (Address) this.location; 4177 } 4178 4179 public boolean hasLocationAddress() { 4180 return this != null && this.location instanceof Address; 4181 } 4182 4183 /** 4184 * @return {@link #location} (The physical location of the accident event.) 4185 */ 4186 public Reference getLocationReference() throws FHIRException { 4187 if (this.location == null) 4188 this.location = new Reference(); 4189 if (!(this.location instanceof Reference)) 4190 throw new FHIRException("Type mismatch: the type Reference was expected, but " 4191 + this.location.getClass().getName() + " was encountered"); 4192 return (Reference) this.location; 4193 } 4194 4195 public boolean hasLocationReference() { 4196 return this != null && this.location instanceof Reference; 4197 } 4198 4199 public boolean hasLocation() { 4200 return this.location != null && !this.location.isEmpty(); 4201 } 4202 4203 /** 4204 * @param value {@link #location} (The physical location of the accident event.) 4205 */ 4206 public AccidentComponent setLocation(Type value) { 4207 if (value != null && !(value instanceof Address || value instanceof Reference)) 4208 throw new Error("Not the right type for Claim.accident.location[x]: " + value.fhirType()); 4209 this.location = value; 4210 return this; 4211 } 4212 4213 protected void listChildren(List<Property> children) { 4214 super.listChildren(children); 4215 children.add(new Property("date", "date", 4216 "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 4217 children.add(new Property("type", "CodeableConcept", 4218 "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 4219 0, 1, type)); 4220 children.add(new Property("location[x]", "Address|Reference(Location)", 4221 "The physical location of the accident event.", 0, 1, location)); 4222 } 4223 4224 @Override 4225 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4226 switch (_hash) { 4227 case 3076014: 4228 /* date */ return new Property("date", "date", 4229 "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 4230 case 3575610: 4231 /* type */ return new Property("type", "CodeableConcept", 4232 "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 4233 0, 1, type); 4234 case 552316075: 4235 /* location[x] */ return new Property("location[x]", "Address|Reference(Location)", 4236 "The physical location of the accident event.", 0, 1, location); 4237 case 1901043637: 4238 /* location */ return new Property("location[x]", "Address|Reference(Location)", 4239 "The physical location of the accident event.", 0, 1, location); 4240 case -1280020865: 4241 /* locationAddress */ return new Property("location[x]", "Address|Reference(Location)", 4242 "The physical location of the accident event.", 0, 1, location); 4243 case 755866390: 4244 /* locationReference */ return new Property("location[x]", "Address|Reference(Location)", 4245 "The physical location of the accident event.", 0, 1, location); 4246 default: 4247 return super.getNamedProperty(_hash, _name, _checkValid); 4248 } 4249 4250 } 4251 4252 @Override 4253 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4254 switch (hash) { 4255 case 3076014: 4256 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 4257 case 3575610: 4258 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4259 case 1901043637: 4260 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 4261 default: 4262 return super.getProperty(hash, name, checkValid); 4263 } 4264 4265 } 4266 4267 @Override 4268 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4269 switch (hash) { 4270 case 3076014: // date 4271 this.date = castToDate(value); // DateType 4272 return value; 4273 case 3575610: // type 4274 this.type = castToCodeableConcept(value); // CodeableConcept 4275 return value; 4276 case 1901043637: // location 4277 this.location = castToType(value); // Type 4278 return value; 4279 default: 4280 return super.setProperty(hash, name, value); 4281 } 4282 4283 } 4284 4285 @Override 4286 public Base setProperty(String name, Base value) throws FHIRException { 4287 if (name.equals("date")) { 4288 this.date = castToDate(value); // DateType 4289 } else if (name.equals("type")) { 4290 this.type = castToCodeableConcept(value); // CodeableConcept 4291 } else if (name.equals("location[x]")) { 4292 this.location = castToType(value); // Type 4293 } else 4294 return super.setProperty(name, value); 4295 return value; 4296 } 4297 4298 @Override 4299 public void removeChild(String name, Base value) throws FHIRException { 4300 if (name.equals("date")) { 4301 this.date = null; 4302 } else if (name.equals("type")) { 4303 this.type = null; 4304 } else if (name.equals("location[x]")) { 4305 this.location = null; 4306 } else 4307 super.removeChild(name, value); 4308 4309 } 4310 4311 @Override 4312 public Base makeProperty(int hash, String name) throws FHIRException { 4313 switch (hash) { 4314 case 3076014: 4315 return getDateElement(); 4316 case 3575610: 4317 return getType(); 4318 case 552316075: 4319 return getLocation(); 4320 case 1901043637: 4321 return getLocation(); 4322 default: 4323 return super.makeProperty(hash, name); 4324 } 4325 4326 } 4327 4328 @Override 4329 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4330 switch (hash) { 4331 case 3076014: 4332 /* date */ return new String[] { "date" }; 4333 case 3575610: 4334 /* type */ return new String[] { "CodeableConcept" }; 4335 case 1901043637: 4336 /* location */ return new String[] { "Address", "Reference" }; 4337 default: 4338 return super.getTypesForProperty(hash, name); 4339 } 4340 4341 } 4342 4343 @Override 4344 public Base addChild(String name) throws FHIRException { 4345 if (name.equals("date")) { 4346 throw new FHIRException("Cannot call addChild on a singleton property Claim.date"); 4347 } else if (name.equals("type")) { 4348 this.type = new CodeableConcept(); 4349 return this.type; 4350 } else if (name.equals("locationAddress")) { 4351 this.location = new Address(); 4352 return this.location; 4353 } else if (name.equals("locationReference")) { 4354 this.location = new Reference(); 4355 return this.location; 4356 } else 4357 return super.addChild(name); 4358 } 4359 4360 public AccidentComponent copy() { 4361 AccidentComponent dst = new AccidentComponent(); 4362 copyValues(dst); 4363 return dst; 4364 } 4365 4366 public void copyValues(AccidentComponent dst) { 4367 super.copyValues(dst); 4368 dst.date = date == null ? null : date.copy(); 4369 dst.type = type == null ? null : type.copy(); 4370 dst.location = location == null ? null : location.copy(); 4371 } 4372 4373 @Override 4374 public boolean equalsDeep(Base other_) { 4375 if (!super.equalsDeep(other_)) 4376 return false; 4377 if (!(other_ instanceof AccidentComponent)) 4378 return false; 4379 AccidentComponent o = (AccidentComponent) other_; 4380 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) 4381 && compareDeep(location, o.location, true); 4382 } 4383 4384 @Override 4385 public boolean equalsShallow(Base other_) { 4386 if (!super.equalsShallow(other_)) 4387 return false; 4388 if (!(other_ instanceof AccidentComponent)) 4389 return false; 4390 AccidentComponent o = (AccidentComponent) other_; 4391 return compareValues(date, o.date, true); 4392 } 4393 4394 public boolean isEmpty() { 4395 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 4396 } 4397 4398 public String fhirType() { 4399 return "Claim.accident"; 4400 4401 } 4402 4403 } 4404 4405 @Block() 4406 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 4407 /** 4408 * A number to uniquely identify item entries. 4409 */ 4410 @Child(name = "sequence", type = { 4411 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4412 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 4413 protected PositiveIntType sequence; 4414 4415 /** 4416 * CareTeam members related to this service or product. 4417 */ 4418 @Child(name = "careTeamSequence", type = { 4419 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4420 @Description(shortDefinition = "Applicable careTeam members", formalDefinition = "CareTeam members related to this service or product.") 4421 protected List<PositiveIntType> careTeamSequence; 4422 4423 /** 4424 * Diagnosis applicable for this service or product. 4425 */ 4426 @Child(name = "diagnosisSequence", type = { 4427 PositiveIntType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4428 @Description(shortDefinition = "Applicable diagnoses", formalDefinition = "Diagnosis applicable for this service or product.") 4429 protected List<PositiveIntType> diagnosisSequence; 4430 4431 /** 4432 * Procedures applicable for this service or product. 4433 */ 4434 @Child(name = "procedureSequence", type = { 4435 PositiveIntType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4436 @Description(shortDefinition = "Applicable procedures", formalDefinition = "Procedures applicable for this service or product.") 4437 protected List<PositiveIntType> procedureSequence; 4438 4439 /** 4440 * Exceptions, special conditions and supporting information applicable for this 4441 * service or product. 4442 */ 4443 @Child(name = "informationSequence", type = { 4444 PositiveIntType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4445 @Description(shortDefinition = "Applicable exception and supporting information", formalDefinition = "Exceptions, special conditions and supporting information applicable for this service or product.") 4446 protected List<PositiveIntType> informationSequence; 4447 4448 /** 4449 * The type of revenue or cost center providing the product and/or service. 4450 */ 4451 @Child(name = "revenue", type = { 4452 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4453 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 4454 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 4455 protected CodeableConcept revenue; 4456 4457 /** 4458 * Code to identify the general type of benefits under which products and 4459 * services are provided. 4460 */ 4461 @Child(name = "category", type = { 4462 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4463 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 4464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 4465 protected CodeableConcept category; 4466 4467 /** 4468 * When the value is a group code then this item collects a set of related claim 4469 * details, otherwise this contains the product, service, drug or other billing 4470 * code for the item. 4471 */ 4472 @Child(name = "productOrService", type = { 4473 CodeableConcept.class }, order = 8, min = 1, max = 1, modifier = false, summary = false) 4474 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4475 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4476 protected CodeableConcept productOrService; 4477 4478 /** 4479 * Item typification or modifiers codes to convey additional context for the 4480 * product or service. 4481 */ 4482 @Child(name = "modifier", type = { 4483 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4484 @Description(shortDefinition = "Product or service billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4486 protected List<CodeableConcept> modifier; 4487 4488 /** 4489 * Identifies the program under which this may be recovered. 4490 */ 4491 @Child(name = "programCode", type = { 4492 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4493 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 4494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 4495 protected List<CodeableConcept> programCode; 4496 4497 /** 4498 * The date or dates when the service or product was supplied, performed or 4499 * completed. 4500 */ 4501 @Child(name = "serviced", type = { DateType.class, 4502 Period.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4503 @Description(shortDefinition = "Date or dates of service or product delivery", formalDefinition = "The date or dates when the service or product was supplied, performed or completed.") 4504 protected Type serviced; 4505 4506 /** 4507 * Where the product or service was provided. 4508 */ 4509 @Child(name = "location", type = { CodeableConcept.class, Address.class, 4510 Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 4511 @Description(shortDefinition = "Place of service or where product was supplied", formalDefinition = "Where the product or service was provided.") 4512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-place") 4513 protected Type location; 4514 4515 /** 4516 * The number of repetitions of a service or product. 4517 */ 4518 @Child(name = "quantity", type = { 4519 Quantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4520 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4521 protected Quantity quantity; 4522 4523 /** 4524 * If the item is not a group then this is the fee for the product or service, 4525 * otherwise this is the total of the fees for the details of the group. 4526 */ 4527 @Child(name = "unitPrice", type = { Money.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 4528 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 4529 protected Money unitPrice; 4530 4531 /** 4532 * A real number that represents a multiplier used in determining the overall 4533 * value of services delivered and/or goods received. The concept of a Factor 4534 * allows for a discount or surcharge multiplier to be applied to a monetary 4535 * amount. 4536 */ 4537 @Child(name = "factor", type = { 4538 DecimalType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 4539 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 4540 protected DecimalType factor; 4541 4542 /** 4543 * The quantity times the unit price for an additional service or product or 4544 * charge. 4545 */ 4546 @Child(name = "net", type = { Money.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 4547 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 4548 protected Money net; 4549 4550 /** 4551 * Unique Device Identifiers associated with this line item. 4552 */ 4553 @Child(name = "udi", type = { 4554 Device.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4555 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 4556 protected List<Reference> udi; 4557 /** 4558 * The actual objects that are the target of the reference (Unique Device 4559 * Identifiers associated with this line item.) 4560 */ 4561 protected List<Device> udiTarget; 4562 4563 /** 4564 * Physical service site on the patient (limb, tooth, etc.). 4565 */ 4566 @Child(name = "bodySite", type = { 4567 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 4568 @Description(shortDefinition = "Anatomical location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 4569 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/tooth") 4570 protected CodeableConcept bodySite; 4571 4572 /** 4573 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 4574 */ 4575 @Child(name = "subSite", type = { 4576 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4577 @Description(shortDefinition = "Anatomical sub-location", formalDefinition = "A region or surface of the bodySite, e.g. limb region or tooth surface(s).") 4578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/surface") 4579 protected List<CodeableConcept> subSite; 4580 4581 /** 4582 * The Encounters during which this Claim was created or to which the creation 4583 * of this record is tightly associated. 4584 */ 4585 @Child(name = "encounter", type = { 4586 Encounter.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4587 @Description(shortDefinition = "Encounters related to this billed item", formalDefinition = "The Encounters during which this Claim was created or to which the creation of this record is tightly associated.") 4588 protected List<Reference> encounter; 4589 /** 4590 * The actual objects that are the target of the reference (The Encounters 4591 * during which this Claim was created or to which the creation of this record 4592 * is tightly associated.) 4593 */ 4594 protected List<Encounter> encounterTarget; 4595 4596 /** 4597 * A claim detail line. Either a simple (a product or service) or a 'group' of 4598 * sub-details which are simple items. 4599 */ 4600 @Child(name = "detail", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4601 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 4602 protected List<DetailComponent> detail; 4603 4604 private static final long serialVersionUID = -329028323L; 4605 4606 /** 4607 * Constructor 4608 */ 4609 public ItemComponent() { 4610 super(); 4611 } 4612 4613 /** 4614 * Constructor 4615 */ 4616 public ItemComponent(PositiveIntType sequence, CodeableConcept productOrService) { 4617 super(); 4618 this.sequence = sequence; 4619 this.productOrService = productOrService; 4620 } 4621 4622 /** 4623 * @return {@link #sequence} (A number to uniquely identify item entries.). This 4624 * is the underlying object with id, value and extensions. The accessor 4625 * "getSequence" gives direct access to the value 4626 */ 4627 public PositiveIntType getSequenceElement() { 4628 if (this.sequence == null) 4629 if (Configuration.errorOnAutoCreate()) 4630 throw new Error("Attempt to auto-create ItemComponent.sequence"); 4631 else if (Configuration.doAutoCreate()) 4632 this.sequence = new PositiveIntType(); // bb 4633 return this.sequence; 4634 } 4635 4636 public boolean hasSequenceElement() { 4637 return this.sequence != null && !this.sequence.isEmpty(); 4638 } 4639 4640 public boolean hasSequence() { 4641 return this.sequence != null && !this.sequence.isEmpty(); 4642 } 4643 4644 /** 4645 * @param value {@link #sequence} (A number to uniquely identify item entries.). 4646 * This is the underlying object with id, value and extensions. The 4647 * accessor "getSequence" gives direct access to the value 4648 */ 4649 public ItemComponent setSequenceElement(PositiveIntType value) { 4650 this.sequence = value; 4651 return this; 4652 } 4653 4654 /** 4655 * @return A number to uniquely identify item entries. 4656 */ 4657 public int getSequence() { 4658 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 4659 } 4660 4661 /** 4662 * @param value A number to uniquely identify item entries. 4663 */ 4664 public ItemComponent setSequence(int value) { 4665 if (this.sequence == null) 4666 this.sequence = new PositiveIntType(); 4667 this.sequence.setValue(value); 4668 return this; 4669 } 4670 4671 /** 4672 * @return {@link #careTeamSequence} (CareTeam members related to this service 4673 * or product.) 4674 */ 4675 public List<PositiveIntType> getCareTeamSequence() { 4676 if (this.careTeamSequence == null) 4677 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4678 return this.careTeamSequence; 4679 } 4680 4681 /** 4682 * @return Returns a reference to <code>this</code> for easy method chaining 4683 */ 4684 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 4685 this.careTeamSequence = theCareTeamSequence; 4686 return this; 4687 } 4688 4689 public boolean hasCareTeamSequence() { 4690 if (this.careTeamSequence == null) 4691 return false; 4692 for (PositiveIntType item : this.careTeamSequence) 4693 if (!item.isEmpty()) 4694 return true; 4695 return false; 4696 } 4697 4698 /** 4699 * @return {@link #careTeamSequence} (CareTeam members related to this service 4700 * or product.) 4701 */ 4702 public PositiveIntType addCareTeamSequenceElement() {// 2 4703 PositiveIntType t = new PositiveIntType(); 4704 if (this.careTeamSequence == null) 4705 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4706 this.careTeamSequence.add(t); 4707 return t; 4708 } 4709 4710 /** 4711 * @param value {@link #careTeamSequence} (CareTeam members related to this 4712 * service or product.) 4713 */ 4714 public ItemComponent addCareTeamSequence(int value) { // 1 4715 PositiveIntType t = new PositiveIntType(); 4716 t.setValue(value); 4717 if (this.careTeamSequence == null) 4718 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4719 this.careTeamSequence.add(t); 4720 return this; 4721 } 4722 4723 /** 4724 * @param value {@link #careTeamSequence} (CareTeam members related to this 4725 * service or product.) 4726 */ 4727 public boolean hasCareTeamSequence(int value) { 4728 if (this.careTeamSequence == null) 4729 return false; 4730 for (PositiveIntType v : this.careTeamSequence) 4731 if (v.getValue().equals(value)) // positiveInt 4732 return true; 4733 return false; 4734 } 4735 4736 /** 4737 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or 4738 * product.) 4739 */ 4740 public List<PositiveIntType> getDiagnosisSequence() { 4741 if (this.diagnosisSequence == null) 4742 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4743 return this.diagnosisSequence; 4744 } 4745 4746 /** 4747 * @return Returns a reference to <code>this</code> for easy method chaining 4748 */ 4749 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 4750 this.diagnosisSequence = theDiagnosisSequence; 4751 return this; 4752 } 4753 4754 public boolean hasDiagnosisSequence() { 4755 if (this.diagnosisSequence == null) 4756 return false; 4757 for (PositiveIntType item : this.diagnosisSequence) 4758 if (!item.isEmpty()) 4759 return true; 4760 return false; 4761 } 4762 4763 /** 4764 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or 4765 * product.) 4766 */ 4767 public PositiveIntType addDiagnosisSequenceElement() {// 2 4768 PositiveIntType t = new PositiveIntType(); 4769 if (this.diagnosisSequence == null) 4770 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4771 this.diagnosisSequence.add(t); 4772 return t; 4773 } 4774 4775 /** 4776 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this 4777 * service or product.) 4778 */ 4779 public ItemComponent addDiagnosisSequence(int value) { // 1 4780 PositiveIntType t = new PositiveIntType(); 4781 t.setValue(value); 4782 if (this.diagnosisSequence == null) 4783 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4784 this.diagnosisSequence.add(t); 4785 return this; 4786 } 4787 4788 /** 4789 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this 4790 * service or product.) 4791 */ 4792 public boolean hasDiagnosisSequence(int value) { 4793 if (this.diagnosisSequence == null) 4794 return false; 4795 for (PositiveIntType v : this.diagnosisSequence) 4796 if (v.getValue().equals(value)) // positiveInt 4797 return true; 4798 return false; 4799 } 4800 4801 /** 4802 * @return {@link #procedureSequence} (Procedures applicable for this service or 4803 * product.) 4804 */ 4805 public List<PositiveIntType> getProcedureSequence() { 4806 if (this.procedureSequence == null) 4807 this.procedureSequence = new ArrayList<PositiveIntType>(); 4808 return this.procedureSequence; 4809 } 4810 4811 /** 4812 * @return Returns a reference to <code>this</code> for easy method chaining 4813 */ 4814 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 4815 this.procedureSequence = theProcedureSequence; 4816 return this; 4817 } 4818 4819 public boolean hasProcedureSequence() { 4820 if (this.procedureSequence == null) 4821 return false; 4822 for (PositiveIntType item : this.procedureSequence) 4823 if (!item.isEmpty()) 4824 return true; 4825 return false; 4826 } 4827 4828 /** 4829 * @return {@link #procedureSequence} (Procedures applicable for this service or 4830 * product.) 4831 */ 4832 public PositiveIntType addProcedureSequenceElement() {// 2 4833 PositiveIntType t = new PositiveIntType(); 4834 if (this.procedureSequence == null) 4835 this.procedureSequence = new ArrayList<PositiveIntType>(); 4836 this.procedureSequence.add(t); 4837 return t; 4838 } 4839 4840 /** 4841 * @param value {@link #procedureSequence} (Procedures applicable for this 4842 * service or product.) 4843 */ 4844 public ItemComponent addProcedureSequence(int value) { // 1 4845 PositiveIntType t = new PositiveIntType(); 4846 t.setValue(value); 4847 if (this.procedureSequence == null) 4848 this.procedureSequence = new ArrayList<PositiveIntType>(); 4849 this.procedureSequence.add(t); 4850 return this; 4851 } 4852 4853 /** 4854 * @param value {@link #procedureSequence} (Procedures applicable for this 4855 * service or product.) 4856 */ 4857 public boolean hasProcedureSequence(int value) { 4858 if (this.procedureSequence == null) 4859 return false; 4860 for (PositiveIntType v : this.procedureSequence) 4861 if (v.getValue().equals(value)) // positiveInt 4862 return true; 4863 return false; 4864 } 4865 4866 /** 4867 * @return {@link #informationSequence} (Exceptions, special conditions and 4868 * supporting information applicable for this service or product.) 4869 */ 4870 public List<PositiveIntType> getInformationSequence() { 4871 if (this.informationSequence == null) 4872 this.informationSequence = new ArrayList<PositiveIntType>(); 4873 return this.informationSequence; 4874 } 4875 4876 /** 4877 * @return Returns a reference to <code>this</code> for easy method chaining 4878 */ 4879 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 4880 this.informationSequence = theInformationSequence; 4881 return this; 4882 } 4883 4884 public boolean hasInformationSequence() { 4885 if (this.informationSequence == null) 4886 return false; 4887 for (PositiveIntType item : this.informationSequence) 4888 if (!item.isEmpty()) 4889 return true; 4890 return false; 4891 } 4892 4893 /** 4894 * @return {@link #informationSequence} (Exceptions, special conditions and 4895 * supporting information applicable for this service or product.) 4896 */ 4897 public PositiveIntType addInformationSequenceElement() {// 2 4898 PositiveIntType t = new PositiveIntType(); 4899 if (this.informationSequence == null) 4900 this.informationSequence = new ArrayList<PositiveIntType>(); 4901 this.informationSequence.add(t); 4902 return t; 4903 } 4904 4905 /** 4906 * @param value {@link #informationSequence} (Exceptions, special conditions and 4907 * supporting information applicable for this service or product.) 4908 */ 4909 public ItemComponent addInformationSequence(int value) { // 1 4910 PositiveIntType t = new PositiveIntType(); 4911 t.setValue(value); 4912 if (this.informationSequence == null) 4913 this.informationSequence = new ArrayList<PositiveIntType>(); 4914 this.informationSequence.add(t); 4915 return this; 4916 } 4917 4918 /** 4919 * @param value {@link #informationSequence} (Exceptions, special conditions and 4920 * supporting information applicable for this service or product.) 4921 */ 4922 public boolean hasInformationSequence(int value) { 4923 if (this.informationSequence == null) 4924 return false; 4925 for (PositiveIntType v : this.informationSequence) 4926 if (v.getValue().equals(value)) // positiveInt 4927 return true; 4928 return false; 4929 } 4930 4931 /** 4932 * @return {@link #revenue} (The type of revenue or cost center providing the 4933 * product and/or service.) 4934 */ 4935 public CodeableConcept getRevenue() { 4936 if (this.revenue == null) 4937 if (Configuration.errorOnAutoCreate()) 4938 throw new Error("Attempt to auto-create ItemComponent.revenue"); 4939 else if (Configuration.doAutoCreate()) 4940 this.revenue = new CodeableConcept(); // cc 4941 return this.revenue; 4942 } 4943 4944 public boolean hasRevenue() { 4945 return this.revenue != null && !this.revenue.isEmpty(); 4946 } 4947 4948 /** 4949 * @param value {@link #revenue} (The type of revenue or cost center providing 4950 * the product and/or service.) 4951 */ 4952 public ItemComponent setRevenue(CodeableConcept value) { 4953 this.revenue = value; 4954 return this; 4955 } 4956 4957 /** 4958 * @return {@link #category} (Code to identify the general type of benefits 4959 * under which products and services are provided.) 4960 */ 4961 public CodeableConcept getCategory() { 4962 if (this.category == null) 4963 if (Configuration.errorOnAutoCreate()) 4964 throw new Error("Attempt to auto-create ItemComponent.category"); 4965 else if (Configuration.doAutoCreate()) 4966 this.category = new CodeableConcept(); // cc 4967 return this.category; 4968 } 4969 4970 public boolean hasCategory() { 4971 return this.category != null && !this.category.isEmpty(); 4972 } 4973 4974 /** 4975 * @param value {@link #category} (Code to identify the general type of benefits 4976 * under which products and services are provided.) 4977 */ 4978 public ItemComponent setCategory(CodeableConcept value) { 4979 this.category = value; 4980 return this; 4981 } 4982 4983 /** 4984 * @return {@link #productOrService} (When the value is a group code then this 4985 * item collects a set of related claim details, otherwise this contains 4986 * the product, service, drug or other billing code for the item.) 4987 */ 4988 public CodeableConcept getProductOrService() { 4989 if (this.productOrService == null) 4990 if (Configuration.errorOnAutoCreate()) 4991 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 4992 else if (Configuration.doAutoCreate()) 4993 this.productOrService = new CodeableConcept(); // cc 4994 return this.productOrService; 4995 } 4996 4997 public boolean hasProductOrService() { 4998 return this.productOrService != null && !this.productOrService.isEmpty(); 4999 } 5000 5001 /** 5002 * @param value {@link #productOrService} (When the value is a group code then 5003 * this item collects a set of related claim details, otherwise 5004 * this contains the product, service, drug or other billing code 5005 * for the item.) 5006 */ 5007 public ItemComponent setProductOrService(CodeableConcept value) { 5008 this.productOrService = value; 5009 return this; 5010 } 5011 5012 /** 5013 * @return {@link #modifier} (Item typification or modifiers codes to convey 5014 * additional context for the product or service.) 5015 */ 5016 public List<CodeableConcept> getModifier() { 5017 if (this.modifier == null) 5018 this.modifier = new ArrayList<CodeableConcept>(); 5019 return this.modifier; 5020 } 5021 5022 /** 5023 * @return Returns a reference to <code>this</code> for easy method chaining 5024 */ 5025 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 5026 this.modifier = theModifier; 5027 return this; 5028 } 5029 5030 public boolean hasModifier() { 5031 if (this.modifier == null) 5032 return false; 5033 for (CodeableConcept item : this.modifier) 5034 if (!item.isEmpty()) 5035 return true; 5036 return false; 5037 } 5038 5039 public CodeableConcept addModifier() { // 3 5040 CodeableConcept t = new CodeableConcept(); 5041 if (this.modifier == null) 5042 this.modifier = new ArrayList<CodeableConcept>(); 5043 this.modifier.add(t); 5044 return t; 5045 } 5046 5047 public ItemComponent addModifier(CodeableConcept t) { // 3 5048 if (t == null) 5049 return this; 5050 if (this.modifier == null) 5051 this.modifier = new ArrayList<CodeableConcept>(); 5052 this.modifier.add(t); 5053 return this; 5054 } 5055 5056 /** 5057 * @return The first repetition of repeating field {@link #modifier}, creating 5058 * it if it does not already exist 5059 */ 5060 public CodeableConcept getModifierFirstRep() { 5061 if (getModifier().isEmpty()) { 5062 addModifier(); 5063 } 5064 return getModifier().get(0); 5065 } 5066 5067 /** 5068 * @return {@link #programCode} (Identifies the program under which this may be 5069 * recovered.) 5070 */ 5071 public List<CodeableConcept> getProgramCode() { 5072 if (this.programCode == null) 5073 this.programCode = new ArrayList<CodeableConcept>(); 5074 return this.programCode; 5075 } 5076 5077 /** 5078 * @return Returns a reference to <code>this</code> for easy method chaining 5079 */ 5080 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 5081 this.programCode = theProgramCode; 5082 return this; 5083 } 5084 5085 public boolean hasProgramCode() { 5086 if (this.programCode == null) 5087 return false; 5088 for (CodeableConcept item : this.programCode) 5089 if (!item.isEmpty()) 5090 return true; 5091 return false; 5092 } 5093 5094 public CodeableConcept addProgramCode() { // 3 5095 CodeableConcept t = new CodeableConcept(); 5096 if (this.programCode == null) 5097 this.programCode = new ArrayList<CodeableConcept>(); 5098 this.programCode.add(t); 5099 return t; 5100 } 5101 5102 public ItemComponent addProgramCode(CodeableConcept t) { // 3 5103 if (t == null) 5104 return this; 5105 if (this.programCode == null) 5106 this.programCode = new ArrayList<CodeableConcept>(); 5107 this.programCode.add(t); 5108 return this; 5109 } 5110 5111 /** 5112 * @return The first repetition of repeating field {@link #programCode}, 5113 * creating it if it does not already exist 5114 */ 5115 public CodeableConcept getProgramCodeFirstRep() { 5116 if (getProgramCode().isEmpty()) { 5117 addProgramCode(); 5118 } 5119 return getProgramCode().get(0); 5120 } 5121 5122 /** 5123 * @return {@link #serviced} (The date or dates when the service or product was 5124 * supplied, performed or completed.) 5125 */ 5126 public Type getServiced() { 5127 return this.serviced; 5128 } 5129 5130 /** 5131 * @return {@link #serviced} (The date or dates when the service or product was 5132 * supplied, performed or completed.) 5133 */ 5134 public DateType getServicedDateType() throws FHIRException { 5135 if (this.serviced == null) 5136 this.serviced = new DateType(); 5137 if (!(this.serviced instanceof DateType)) 5138 throw new FHIRException("Type mismatch: the type DateType was expected, but " 5139 + this.serviced.getClass().getName() + " was encountered"); 5140 return (DateType) this.serviced; 5141 } 5142 5143 public boolean hasServicedDateType() { 5144 return this != null && this.serviced instanceof DateType; 5145 } 5146 5147 /** 5148 * @return {@link #serviced} (The date or dates when the service or product was 5149 * supplied, performed or completed.) 5150 */ 5151 public Period getServicedPeriod() throws FHIRException { 5152 if (this.serviced == null) 5153 this.serviced = new Period(); 5154 if (!(this.serviced instanceof Period)) 5155 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 5156 + " was encountered"); 5157 return (Period) this.serviced; 5158 } 5159 5160 public boolean hasServicedPeriod() { 5161 return this != null && this.serviced instanceof Period; 5162 } 5163 5164 public boolean hasServiced() { 5165 return this.serviced != null && !this.serviced.isEmpty(); 5166 } 5167 5168 /** 5169 * @param value {@link #serviced} (The date or dates when the service or product 5170 * was supplied, performed or completed.) 5171 */ 5172 public ItemComponent setServiced(Type value) { 5173 if (value != null && !(value instanceof DateType || value instanceof Period)) 5174 throw new Error("Not the right type for Claim.item.serviced[x]: " + value.fhirType()); 5175 this.serviced = value; 5176 return this; 5177 } 5178 5179 /** 5180 * @return {@link #location} (Where the product or service was provided.) 5181 */ 5182 public Type getLocation() { 5183 return this.location; 5184 } 5185 5186 /** 5187 * @return {@link #location} (Where the product or service was provided.) 5188 */ 5189 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 5190 if (this.location == null) 5191 this.location = new CodeableConcept(); 5192 if (!(this.location instanceof CodeableConcept)) 5193 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 5194 + this.location.getClass().getName() + " was encountered"); 5195 return (CodeableConcept) this.location; 5196 } 5197 5198 public boolean hasLocationCodeableConcept() { 5199 return this != null && this.location instanceof CodeableConcept; 5200 } 5201 5202 /** 5203 * @return {@link #location} (Where the product or service was provided.) 5204 */ 5205 public Address getLocationAddress() throws FHIRException { 5206 if (this.location == null) 5207 this.location = new Address(); 5208 if (!(this.location instanceof Address)) 5209 throw new FHIRException("Type mismatch: the type Address was expected, but " 5210 + this.location.getClass().getName() + " was encountered"); 5211 return (Address) this.location; 5212 } 5213 5214 public boolean hasLocationAddress() { 5215 return this != null && this.location instanceof Address; 5216 } 5217 5218 /** 5219 * @return {@link #location} (Where the product or service was provided.) 5220 */ 5221 public Reference getLocationReference() throws FHIRException { 5222 if (this.location == null) 5223 this.location = new Reference(); 5224 if (!(this.location instanceof Reference)) 5225 throw new FHIRException("Type mismatch: the type Reference was expected, but " 5226 + this.location.getClass().getName() + " was encountered"); 5227 return (Reference) this.location; 5228 } 5229 5230 public boolean hasLocationReference() { 5231 return this != null && this.location instanceof Reference; 5232 } 5233 5234 public boolean hasLocation() { 5235 return this.location != null && !this.location.isEmpty(); 5236 } 5237 5238 /** 5239 * @param value {@link #location} (Where the product or service was provided.) 5240 */ 5241 public ItemComponent setLocation(Type value) { 5242 if (value != null 5243 && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 5244 throw new Error("Not the right type for Claim.item.location[x]: " + value.fhirType()); 5245 this.location = value; 5246 return this; 5247 } 5248 5249 /** 5250 * @return {@link #quantity} (The number of repetitions of a service or 5251 * product.) 5252 */ 5253 public Quantity getQuantity() { 5254 if (this.quantity == null) 5255 if (Configuration.errorOnAutoCreate()) 5256 throw new Error("Attempt to auto-create ItemComponent.quantity"); 5257 else if (Configuration.doAutoCreate()) 5258 this.quantity = new Quantity(); // cc 5259 return this.quantity; 5260 } 5261 5262 public boolean hasQuantity() { 5263 return this.quantity != null && !this.quantity.isEmpty(); 5264 } 5265 5266 /** 5267 * @param value {@link #quantity} (The number of repetitions of a service or 5268 * product.) 5269 */ 5270 public ItemComponent setQuantity(Quantity value) { 5271 this.quantity = value; 5272 return this; 5273 } 5274 5275 /** 5276 * @return {@link #unitPrice} (If the item is not a group then this is the fee 5277 * for the product or service, otherwise this is the total of the fees 5278 * for the details of the group.) 5279 */ 5280 public Money getUnitPrice() { 5281 if (this.unitPrice == null) 5282 if (Configuration.errorOnAutoCreate()) 5283 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 5284 else if (Configuration.doAutoCreate()) 5285 this.unitPrice = new Money(); // cc 5286 return this.unitPrice; 5287 } 5288 5289 public boolean hasUnitPrice() { 5290 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5291 } 5292 5293 /** 5294 * @param value {@link #unitPrice} (If the item is not a group then this is the 5295 * fee for the product or service, otherwise this is the total of 5296 * the fees for the details of the group.) 5297 */ 5298 public ItemComponent setUnitPrice(Money value) { 5299 this.unitPrice = value; 5300 return this; 5301 } 5302 5303 /** 5304 * @return {@link #factor} (A real number that represents a multiplier used in 5305 * determining the overall value of services delivered and/or goods 5306 * received. The concept of a Factor allows for a discount or surcharge 5307 * multiplier to be applied to a monetary amount.). This is the 5308 * underlying object with id, value and extensions. The accessor 5309 * "getFactor" gives direct access to the value 5310 */ 5311 public DecimalType getFactorElement() { 5312 if (this.factor == null) 5313 if (Configuration.errorOnAutoCreate()) 5314 throw new Error("Attempt to auto-create ItemComponent.factor"); 5315 else if (Configuration.doAutoCreate()) 5316 this.factor = new DecimalType(); // bb 5317 return this.factor; 5318 } 5319 5320 public boolean hasFactorElement() { 5321 return this.factor != null && !this.factor.isEmpty(); 5322 } 5323 5324 public boolean hasFactor() { 5325 return this.factor != null && !this.factor.isEmpty(); 5326 } 5327 5328 /** 5329 * @param value {@link #factor} (A real number that represents a multiplier used 5330 * in determining the overall value of services delivered and/or 5331 * goods received. The concept of a Factor allows for a discount or 5332 * surcharge multiplier to be applied to a monetary amount.). This 5333 * is the underlying object with id, value and extensions. The 5334 * accessor "getFactor" gives direct access to the value 5335 */ 5336 public ItemComponent setFactorElement(DecimalType value) { 5337 this.factor = value; 5338 return this; 5339 } 5340 5341 /** 5342 * @return A real number that represents a multiplier used in determining the 5343 * overall value of services delivered and/or goods received. The 5344 * concept of a Factor allows for a discount or surcharge multiplier to 5345 * be applied to a monetary amount. 5346 */ 5347 public BigDecimal getFactor() { 5348 return this.factor == null ? null : this.factor.getValue(); 5349 } 5350 5351 /** 5352 * @param value A real number that represents a multiplier used in determining 5353 * the overall value of services delivered and/or goods received. 5354 * The concept of a Factor allows for a discount or surcharge 5355 * multiplier to be applied to a monetary amount. 5356 */ 5357 public ItemComponent setFactor(BigDecimal value) { 5358 if (value == null) 5359 this.factor = null; 5360 else { 5361 if (this.factor == null) 5362 this.factor = new DecimalType(); 5363 this.factor.setValue(value); 5364 } 5365 return this; 5366 } 5367 5368 /** 5369 * @param value A real number that represents a multiplier used in determining 5370 * the overall value of services delivered and/or goods received. 5371 * The concept of a Factor allows for a discount or surcharge 5372 * multiplier to be applied to a monetary amount. 5373 */ 5374 public ItemComponent setFactor(long value) { 5375 this.factor = new DecimalType(); 5376 this.factor.setValue(value); 5377 return this; 5378 } 5379 5380 /** 5381 * @param value A real number that represents a multiplier used in determining 5382 * the overall value of services delivered and/or goods received. 5383 * The concept of a Factor allows for a discount or surcharge 5384 * multiplier to be applied to a monetary amount. 5385 */ 5386 public ItemComponent setFactor(double value) { 5387 this.factor = new DecimalType(); 5388 this.factor.setValue(value); 5389 return this; 5390 } 5391 5392 /** 5393 * @return {@link #net} (The quantity times the unit price for an additional 5394 * service or product or charge.) 5395 */ 5396 public Money getNet() { 5397 if (this.net == null) 5398 if (Configuration.errorOnAutoCreate()) 5399 throw new Error("Attempt to auto-create ItemComponent.net"); 5400 else if (Configuration.doAutoCreate()) 5401 this.net = new Money(); // cc 5402 return this.net; 5403 } 5404 5405 public boolean hasNet() { 5406 return this.net != null && !this.net.isEmpty(); 5407 } 5408 5409 /** 5410 * @param value {@link #net} (The quantity times the unit price for an 5411 * additional service or product or charge.) 5412 */ 5413 public ItemComponent setNet(Money value) { 5414 this.net = value; 5415 return this; 5416 } 5417 5418 /** 5419 * @return {@link #udi} (Unique Device Identifiers associated with this line 5420 * item.) 5421 */ 5422 public List<Reference> getUdi() { 5423 if (this.udi == null) 5424 this.udi = new ArrayList<Reference>(); 5425 return this.udi; 5426 } 5427 5428 /** 5429 * @return Returns a reference to <code>this</code> for easy method chaining 5430 */ 5431 public ItemComponent setUdi(List<Reference> theUdi) { 5432 this.udi = theUdi; 5433 return this; 5434 } 5435 5436 public boolean hasUdi() { 5437 if (this.udi == null) 5438 return false; 5439 for (Reference item : this.udi) 5440 if (!item.isEmpty()) 5441 return true; 5442 return false; 5443 } 5444 5445 public Reference addUdi() { // 3 5446 Reference t = new Reference(); 5447 if (this.udi == null) 5448 this.udi = new ArrayList<Reference>(); 5449 this.udi.add(t); 5450 return t; 5451 } 5452 5453 public ItemComponent addUdi(Reference t) { // 3 5454 if (t == null) 5455 return this; 5456 if (this.udi == null) 5457 this.udi = new ArrayList<Reference>(); 5458 this.udi.add(t); 5459 return this; 5460 } 5461 5462 /** 5463 * @return The first repetition of repeating field {@link #udi}, creating it if 5464 * it does not already exist 5465 */ 5466 public Reference getUdiFirstRep() { 5467 if (getUdi().isEmpty()) { 5468 addUdi(); 5469 } 5470 return getUdi().get(0); 5471 } 5472 5473 /** 5474 * @deprecated Use Reference#setResource(IBaseResource) instead 5475 */ 5476 @Deprecated 5477 public List<Device> getUdiTarget() { 5478 if (this.udiTarget == null) 5479 this.udiTarget = new ArrayList<Device>(); 5480 return this.udiTarget; 5481 } 5482 5483 /** 5484 * @deprecated Use Reference#setResource(IBaseResource) instead 5485 */ 5486 @Deprecated 5487 public Device addUdiTarget() { 5488 Device r = new Device(); 5489 if (this.udiTarget == null) 5490 this.udiTarget = new ArrayList<Device>(); 5491 this.udiTarget.add(r); 5492 return r; 5493 } 5494 5495 /** 5496 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 5497 * etc.).) 5498 */ 5499 public CodeableConcept getBodySite() { 5500 if (this.bodySite == null) 5501 if (Configuration.errorOnAutoCreate()) 5502 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 5503 else if (Configuration.doAutoCreate()) 5504 this.bodySite = new CodeableConcept(); // cc 5505 return this.bodySite; 5506 } 5507 5508 public boolean hasBodySite() { 5509 return this.bodySite != null && !this.bodySite.isEmpty(); 5510 } 5511 5512 /** 5513 * @param value {@link #bodySite} (Physical service site on the patient (limb, 5514 * tooth, etc.).) 5515 */ 5516 public ItemComponent setBodySite(CodeableConcept value) { 5517 this.bodySite = value; 5518 return this; 5519 } 5520 5521 /** 5522 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb 5523 * region or tooth surface(s).) 5524 */ 5525 public List<CodeableConcept> getSubSite() { 5526 if (this.subSite == null) 5527 this.subSite = new ArrayList<CodeableConcept>(); 5528 return this.subSite; 5529 } 5530 5531 /** 5532 * @return Returns a reference to <code>this</code> for easy method chaining 5533 */ 5534 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 5535 this.subSite = theSubSite; 5536 return this; 5537 } 5538 5539 public boolean hasSubSite() { 5540 if (this.subSite == null) 5541 return false; 5542 for (CodeableConcept item : this.subSite) 5543 if (!item.isEmpty()) 5544 return true; 5545 return false; 5546 } 5547 5548 public CodeableConcept addSubSite() { // 3 5549 CodeableConcept t = new CodeableConcept(); 5550 if (this.subSite == null) 5551 this.subSite = new ArrayList<CodeableConcept>(); 5552 this.subSite.add(t); 5553 return t; 5554 } 5555 5556 public ItemComponent addSubSite(CodeableConcept t) { // 3 5557 if (t == null) 5558 return this; 5559 if (this.subSite == null) 5560 this.subSite = new ArrayList<CodeableConcept>(); 5561 this.subSite.add(t); 5562 return this; 5563 } 5564 5565 /** 5566 * @return The first repetition of repeating field {@link #subSite}, creating it 5567 * if it does not already exist 5568 */ 5569 public CodeableConcept getSubSiteFirstRep() { 5570 if (getSubSite().isEmpty()) { 5571 addSubSite(); 5572 } 5573 return getSubSite().get(0); 5574 } 5575 5576 /** 5577 * @return {@link #encounter} (The Encounters during which this Claim was 5578 * created or to which the creation of this record is tightly 5579 * associated.) 5580 */ 5581 public List<Reference> getEncounter() { 5582 if (this.encounter == null) 5583 this.encounter = new ArrayList<Reference>(); 5584 return this.encounter; 5585 } 5586 5587 /** 5588 * @return Returns a reference to <code>this</code> for easy method chaining 5589 */ 5590 public ItemComponent setEncounter(List<Reference> theEncounter) { 5591 this.encounter = theEncounter; 5592 return this; 5593 } 5594 5595 public boolean hasEncounter() { 5596 if (this.encounter == null) 5597 return false; 5598 for (Reference item : this.encounter) 5599 if (!item.isEmpty()) 5600 return true; 5601 return false; 5602 } 5603 5604 public Reference addEncounter() { // 3 5605 Reference t = new Reference(); 5606 if (this.encounter == null) 5607 this.encounter = new ArrayList<Reference>(); 5608 this.encounter.add(t); 5609 return t; 5610 } 5611 5612 public ItemComponent addEncounter(Reference t) { // 3 5613 if (t == null) 5614 return this; 5615 if (this.encounter == null) 5616 this.encounter = new ArrayList<Reference>(); 5617 this.encounter.add(t); 5618 return this; 5619 } 5620 5621 /** 5622 * @return The first repetition of repeating field {@link #encounter}, creating 5623 * it if it does not already exist 5624 */ 5625 public Reference getEncounterFirstRep() { 5626 if (getEncounter().isEmpty()) { 5627 addEncounter(); 5628 } 5629 return getEncounter().get(0); 5630 } 5631 5632 /** 5633 * @deprecated Use Reference#setResource(IBaseResource) instead 5634 */ 5635 @Deprecated 5636 public List<Encounter> getEncounterTarget() { 5637 if (this.encounterTarget == null) 5638 this.encounterTarget = new ArrayList<Encounter>(); 5639 return this.encounterTarget; 5640 } 5641 5642 /** 5643 * @deprecated Use Reference#setResource(IBaseResource) instead 5644 */ 5645 @Deprecated 5646 public Encounter addEncounterTarget() { 5647 Encounter r = new Encounter(); 5648 if (this.encounterTarget == null) 5649 this.encounterTarget = new ArrayList<Encounter>(); 5650 this.encounterTarget.add(r); 5651 return r; 5652 } 5653 5654 /** 5655 * @return {@link #detail} (A claim detail line. Either a simple (a product or 5656 * service) or a 'group' of sub-details which are simple items.) 5657 */ 5658 public List<DetailComponent> getDetail() { 5659 if (this.detail == null) 5660 this.detail = new ArrayList<DetailComponent>(); 5661 return this.detail; 5662 } 5663 5664 /** 5665 * @return Returns a reference to <code>this</code> for easy method chaining 5666 */ 5667 public ItemComponent setDetail(List<DetailComponent> theDetail) { 5668 this.detail = theDetail; 5669 return this; 5670 } 5671 5672 public boolean hasDetail() { 5673 if (this.detail == null) 5674 return false; 5675 for (DetailComponent item : this.detail) 5676 if (!item.isEmpty()) 5677 return true; 5678 return false; 5679 } 5680 5681 public DetailComponent addDetail() { // 3 5682 DetailComponent t = new DetailComponent(); 5683 if (this.detail == null) 5684 this.detail = new ArrayList<DetailComponent>(); 5685 this.detail.add(t); 5686 return t; 5687 } 5688 5689 public ItemComponent addDetail(DetailComponent t) { // 3 5690 if (t == null) 5691 return this; 5692 if (this.detail == null) 5693 this.detail = new ArrayList<DetailComponent>(); 5694 this.detail.add(t); 5695 return this; 5696 } 5697 5698 /** 5699 * @return The first repetition of repeating field {@link #detail}, creating it 5700 * if it does not already exist 5701 */ 5702 public DetailComponent getDetailFirstRep() { 5703 if (getDetail().isEmpty()) { 5704 addDetail(); 5705 } 5706 return getDetail().get(0); 5707 } 5708 5709 protected void listChildren(List<Property> children) { 5710 super.listChildren(children); 5711 children 5712 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 5713 children.add(new Property("careTeamSequence", "positiveInt", 5714 "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 5715 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnosis applicable for this service or product.", 5716 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 5717 children.add(new Property("procedureSequence", "positiveInt", 5718 "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 5719 children.add(new Property("informationSequence", "positiveInt", 5720 "Exceptions, special conditions and supporting information applicable for this service or product.", 0, 5721 java.lang.Integer.MAX_VALUE, informationSequence)); 5722 children.add(new Property("revenue", "CodeableConcept", 5723 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 5724 children.add(new Property("category", "CodeableConcept", 5725 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 5726 category)); 5727 children.add(new Property("productOrService", "CodeableConcept", 5728 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5729 0, 1, productOrService)); 5730 children.add(new Property("modifier", "CodeableConcept", 5731 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5732 java.lang.Integer.MAX_VALUE, modifier)); 5733 children.add(new Property("programCode", "CodeableConcept", 5734 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5735 children.add(new Property("serviced[x]", "date|Period", 5736 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 5737 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5738 "Where the product or service was provided.", 0, 1, location)); 5739 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 5740 1, quantity)); 5741 children.add(new Property("unitPrice", "Money", 5742 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5743 0, 1, unitPrice)); 5744 children.add(new Property("factor", "decimal", 5745 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5746 0, 1, factor)); 5747 children.add(new Property("net", "Money", 5748 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 5749 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 5750 0, java.lang.Integer.MAX_VALUE, udi)); 5751 children.add(new Property("bodySite", "CodeableConcept", 5752 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite)); 5753 children.add(new Property("subSite", "CodeableConcept", 5754 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, 5755 subSite)); 5756 children.add(new Property("encounter", "Reference(Encounter)", 5757 "The Encounters during which this Claim was created or to which the creation of this record is tightly associated.", 5758 0, java.lang.Integer.MAX_VALUE, encounter)); 5759 children.add(new Property("detail", "", 5760 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 5761 0, java.lang.Integer.MAX_VALUE, detail)); 5762 } 5763 5764 @Override 5765 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5766 switch (_hash) { 5767 case 1349547969: 5768 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 5769 1, sequence); 5770 case 1070083823: 5771 /* careTeamSequence */ return new Property("careTeamSequence", "positiveInt", 5772 "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 5773 case -909769262: 5774 /* diagnosisSequence */ return new Property("diagnosisSequence", "positiveInt", 5775 "Diagnosis applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 5776 case -808920140: 5777 /* procedureSequence */ return new Property("procedureSequence", "positiveInt", 5778 "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 5779 case -702585587: 5780 /* informationSequence */ return new Property("informationSequence", "positiveInt", 5781 "Exceptions, special conditions and supporting information applicable for this service or product.", 0, 5782 java.lang.Integer.MAX_VALUE, informationSequence); 5783 case 1099842588: 5784 /* revenue */ return new Property("revenue", "CodeableConcept", 5785 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 5786 case 50511102: 5787 /* category */ return new Property("category", "CodeableConcept", 5788 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 5789 category); 5790 case 1957227299: 5791 /* productOrService */ return new Property("productOrService", "CodeableConcept", 5792 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5793 0, 1, productOrService); 5794 case -615513385: 5795 /* modifier */ return new Property("modifier", "CodeableConcept", 5796 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5797 java.lang.Integer.MAX_VALUE, modifier); 5798 case 1010065041: 5799 /* programCode */ return new Property("programCode", "CodeableConcept", 5800 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 5801 case -1927922223: 5802 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 5803 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5804 case 1379209295: 5805 /* serviced */ return new Property("serviced[x]", "date|Period", 5806 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5807 case 363246749: 5808 /* servicedDate */ return new Property("serviced[x]", "date|Period", 5809 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5810 case 1534966512: 5811 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 5812 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5813 case 552316075: 5814 /* location[x] */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5815 "Where the product or service was provided.", 0, 1, location); 5816 case 1901043637: 5817 /* location */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5818 "Where the product or service was provided.", 0, 1, location); 5819 case -1224800468: 5820 /* locationCodeableConcept */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5821 "Where the product or service was provided.", 0, 1, location); 5822 case -1280020865: 5823 /* locationAddress */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5824 "Where the product or service was provided.", 0, 1, location); 5825 case 755866390: 5826 /* locationReference */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5827 "Where the product or service was provided.", 0, 1, location); 5828 case -1285004149: 5829 /* quantity */ return new Property("quantity", "SimpleQuantity", 5830 "The number of repetitions of a service or product.", 0, 1, quantity); 5831 case -486196699: 5832 /* unitPrice */ return new Property("unitPrice", "Money", 5833 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5834 0, 1, unitPrice); 5835 case -1282148017: 5836 /* factor */ return new Property("factor", "decimal", 5837 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5838 0, 1, factor); 5839 case 108957: 5840 /* net */ return new Property("net", "Money", 5841 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 5842 case 115642: 5843 /* udi */ return new Property("udi", "Reference(Device)", 5844 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5845 case 1702620169: 5846 /* bodySite */ return new Property("bodySite", "CodeableConcept", 5847 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite); 5848 case -1868566105: 5849 /* subSite */ return new Property("subSite", "CodeableConcept", 5850 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, 5851 java.lang.Integer.MAX_VALUE, subSite); 5852 case 1524132147: 5853 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5854 "The Encounters during which this Claim was created or to which the creation of this record is tightly associated.", 5855 0, java.lang.Integer.MAX_VALUE, encounter); 5856 case -1335224239: 5857 /* detail */ return new Property("detail", "", 5858 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 5859 0, java.lang.Integer.MAX_VALUE, detail); 5860 default: 5861 return super.getNamedProperty(_hash, _name, _checkValid); 5862 } 5863 5864 } 5865 5866 @Override 5867 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5868 switch (hash) { 5869 case 1349547969: 5870 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 5871 case 1070083823: 5872 /* careTeamSequence */ return this.careTeamSequence == null ? new Base[0] 5873 : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 5874 case -909769262: 5875 /* diagnosisSequence */ return this.diagnosisSequence == null ? new Base[0] 5876 : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 5877 case -808920140: 5878 /* procedureSequence */ return this.procedureSequence == null ? new Base[0] 5879 : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 5880 case -702585587: 5881 /* informationSequence */ return this.informationSequence == null ? new Base[0] 5882 : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 5883 case 1099842588: 5884 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 5885 case 50511102: 5886 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 5887 case 1957227299: 5888 /* productOrService */ return this.productOrService == null ? new Base[0] 5889 : new Base[] { this.productOrService }; // CodeableConcept 5890 case -615513385: 5891 /* modifier */ return this.modifier == null ? new Base[0] 5892 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5893 case 1010065041: 5894 /* programCode */ return this.programCode == null ? new Base[0] 5895 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5896 case 1379209295: 5897 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 5898 case 1901043637: 5899 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 5900 case -1285004149: 5901 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5902 case -486196699: 5903 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 5904 case -1282148017: 5905 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 5906 case 108957: 5907 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 5908 case 115642: 5909 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5910 case 1702620169: 5911 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 5912 case -1868566105: 5913 /* subSite */ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5914 case 1524132147: 5915 /* encounter */ return this.encounter == null ? new Base[0] 5916 : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 5917 case -1335224239: 5918 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 5919 default: 5920 return super.getProperty(hash, name, checkValid); 5921 } 5922 5923 } 5924 5925 @Override 5926 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5927 switch (hash) { 5928 case 1349547969: // sequence 5929 this.sequence = castToPositiveInt(value); // PositiveIntType 5930 return value; 5931 case 1070083823: // careTeamSequence 5932 this.getCareTeamSequence().add(castToPositiveInt(value)); // PositiveIntType 5933 return value; 5934 case -909769262: // diagnosisSequence 5935 this.getDiagnosisSequence().add(castToPositiveInt(value)); // PositiveIntType 5936 return value; 5937 case -808920140: // procedureSequence 5938 this.getProcedureSequence().add(castToPositiveInt(value)); // PositiveIntType 5939 return value; 5940 case -702585587: // informationSequence 5941 this.getInformationSequence().add(castToPositiveInt(value)); // PositiveIntType 5942 return value; 5943 case 1099842588: // revenue 5944 this.revenue = castToCodeableConcept(value); // CodeableConcept 5945 return value; 5946 case 50511102: // category 5947 this.category = castToCodeableConcept(value); // CodeableConcept 5948 return value; 5949 case 1957227299: // productOrService 5950 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5951 return value; 5952 case -615513385: // modifier 5953 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5954 return value; 5955 case 1010065041: // programCode 5956 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5957 return value; 5958 case 1379209295: // serviced 5959 this.serviced = castToType(value); // Type 5960 return value; 5961 case 1901043637: // location 5962 this.location = castToType(value); // Type 5963 return value; 5964 case -1285004149: // quantity 5965 this.quantity = castToQuantity(value); // Quantity 5966 return value; 5967 case -486196699: // unitPrice 5968 this.unitPrice = castToMoney(value); // Money 5969 return value; 5970 case -1282148017: // factor 5971 this.factor = castToDecimal(value); // DecimalType 5972 return value; 5973 case 108957: // net 5974 this.net = castToMoney(value); // Money 5975 return value; 5976 case 115642: // udi 5977 this.getUdi().add(castToReference(value)); // Reference 5978 return value; 5979 case 1702620169: // bodySite 5980 this.bodySite = castToCodeableConcept(value); // CodeableConcept 5981 return value; 5982 case -1868566105: // subSite 5983 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 5984 return value; 5985 case 1524132147: // encounter 5986 this.getEncounter().add(castToReference(value)); // Reference 5987 return value; 5988 case -1335224239: // detail 5989 this.getDetail().add((DetailComponent) value); // DetailComponent 5990 return value; 5991 default: 5992 return super.setProperty(hash, name, value); 5993 } 5994 5995 } 5996 5997 @Override 5998 public Base setProperty(String name, Base value) throws FHIRException { 5999 if (name.equals("sequence")) { 6000 this.sequence = castToPositiveInt(value); // PositiveIntType 6001 } else if (name.equals("careTeamSequence")) { 6002 this.getCareTeamSequence().add(castToPositiveInt(value)); 6003 } else if (name.equals("diagnosisSequence")) { 6004 this.getDiagnosisSequence().add(castToPositiveInt(value)); 6005 } else if (name.equals("procedureSequence")) { 6006 this.getProcedureSequence().add(castToPositiveInt(value)); 6007 } else if (name.equals("informationSequence")) { 6008 this.getInformationSequence().add(castToPositiveInt(value)); 6009 } else if (name.equals("revenue")) { 6010 this.revenue = castToCodeableConcept(value); // CodeableConcept 6011 } else if (name.equals("category")) { 6012 this.category = castToCodeableConcept(value); // CodeableConcept 6013 } else if (name.equals("productOrService")) { 6014 this.productOrService = castToCodeableConcept(value); // CodeableConcept 6015 } else if (name.equals("modifier")) { 6016 this.getModifier().add(castToCodeableConcept(value)); 6017 } else if (name.equals("programCode")) { 6018 this.getProgramCode().add(castToCodeableConcept(value)); 6019 } else if (name.equals("serviced[x]")) { 6020 this.serviced = castToType(value); // Type 6021 } else if (name.equals("location[x]")) { 6022 this.location = castToType(value); // Type 6023 } else if (name.equals("quantity")) { 6024 this.quantity = castToQuantity(value); // Quantity 6025 } else if (name.equals("unitPrice")) { 6026 this.unitPrice = castToMoney(value); // Money 6027 } else if (name.equals("factor")) { 6028 this.factor = castToDecimal(value); // DecimalType 6029 } else if (name.equals("net")) { 6030 this.net = castToMoney(value); // Money 6031 } else if (name.equals("udi")) { 6032 this.getUdi().add(castToReference(value)); 6033 } else if (name.equals("bodySite")) { 6034 this.bodySite = castToCodeableConcept(value); // CodeableConcept 6035 } else if (name.equals("subSite")) { 6036 this.getSubSite().add(castToCodeableConcept(value)); 6037 } else if (name.equals("encounter")) { 6038 this.getEncounter().add(castToReference(value)); 6039 } else if (name.equals("detail")) { 6040 this.getDetail().add((DetailComponent) value); 6041 } else 6042 return super.setProperty(name, value); 6043 return value; 6044 } 6045 6046 @Override 6047 public void removeChild(String name, Base value) throws FHIRException { 6048 if (name.equals("sequence")) { 6049 this.sequence = null; 6050 } else if (name.equals("careTeamSequence")) { 6051 this.getCareTeamSequence().remove(castToPositiveInt(value)); 6052 } else if (name.equals("diagnosisSequence")) { 6053 this.getDiagnosisSequence().remove(castToPositiveInt(value)); 6054 } else if (name.equals("procedureSequence")) { 6055 this.getProcedureSequence().remove(castToPositiveInt(value)); 6056 } else if (name.equals("informationSequence")) { 6057 this.getInformationSequence().remove(castToPositiveInt(value)); 6058 } else if (name.equals("revenue")) { 6059 this.revenue = null; 6060 } else if (name.equals("category")) { 6061 this.category = null; 6062 } else if (name.equals("productOrService")) { 6063 this.productOrService = null; 6064 } else if (name.equals("modifier")) { 6065 this.getModifier().remove(castToCodeableConcept(value)); 6066 } else if (name.equals("programCode")) { 6067 this.getProgramCode().remove(castToCodeableConcept(value)); 6068 } else if (name.equals("serviced[x]")) { 6069 this.serviced = null; 6070 } else if (name.equals("location[x]")) { 6071 this.location = null; 6072 } else if (name.equals("quantity")) { 6073 this.quantity = null; 6074 } else if (name.equals("unitPrice")) { 6075 this.unitPrice = null; 6076 } else if (name.equals("factor")) { 6077 this.factor = null; 6078 } else if (name.equals("net")) { 6079 this.net = null; 6080 } else if (name.equals("udi")) { 6081 this.getUdi().remove(castToReference(value)); 6082 } else if (name.equals("bodySite")) { 6083 this.bodySite = null; 6084 } else if (name.equals("subSite")) { 6085 this.getSubSite().remove(castToCodeableConcept(value)); 6086 } else if (name.equals("encounter")) { 6087 this.getEncounter().remove(castToReference(value)); 6088 } else if (name.equals("detail")) { 6089 this.getDetail().remove((DetailComponent) value); 6090 } else 6091 super.removeChild(name, value); 6092 6093 } 6094 6095 @Override 6096 public Base makeProperty(int hash, String name) throws FHIRException { 6097 switch (hash) { 6098 case 1349547969: 6099 return getSequenceElement(); 6100 case 1070083823: 6101 return addCareTeamSequenceElement(); 6102 case -909769262: 6103 return addDiagnosisSequenceElement(); 6104 case -808920140: 6105 return addProcedureSequenceElement(); 6106 case -702585587: 6107 return addInformationSequenceElement(); 6108 case 1099842588: 6109 return getRevenue(); 6110 case 50511102: 6111 return getCategory(); 6112 case 1957227299: 6113 return getProductOrService(); 6114 case -615513385: 6115 return addModifier(); 6116 case 1010065041: 6117 return addProgramCode(); 6118 case -1927922223: 6119 return getServiced(); 6120 case 1379209295: 6121 return getServiced(); 6122 case 552316075: 6123 return getLocation(); 6124 case 1901043637: 6125 return getLocation(); 6126 case -1285004149: 6127 return getQuantity(); 6128 case -486196699: 6129 return getUnitPrice(); 6130 case -1282148017: 6131 return getFactorElement(); 6132 case 108957: 6133 return getNet(); 6134 case 115642: 6135 return addUdi(); 6136 case 1702620169: 6137 return getBodySite(); 6138 case -1868566105: 6139 return addSubSite(); 6140 case 1524132147: 6141 return addEncounter(); 6142 case -1335224239: 6143 return addDetail(); 6144 default: 6145 return super.makeProperty(hash, name); 6146 } 6147 6148 } 6149 6150 @Override 6151 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6152 switch (hash) { 6153 case 1349547969: 6154 /* sequence */ return new String[] { "positiveInt" }; 6155 case 1070083823: 6156 /* careTeamSequence */ return new String[] { "positiveInt" }; 6157 case -909769262: 6158 /* diagnosisSequence */ return new String[] { "positiveInt" }; 6159 case -808920140: 6160 /* procedureSequence */ return new String[] { "positiveInt" }; 6161 case -702585587: 6162 /* informationSequence */ return new String[] { "positiveInt" }; 6163 case 1099842588: 6164 /* revenue */ return new String[] { "CodeableConcept" }; 6165 case 50511102: 6166 /* category */ return new String[] { "CodeableConcept" }; 6167 case 1957227299: 6168 /* productOrService */ return new String[] { "CodeableConcept" }; 6169 case -615513385: 6170 /* modifier */ return new String[] { "CodeableConcept" }; 6171 case 1010065041: 6172 /* programCode */ return new String[] { "CodeableConcept" }; 6173 case 1379209295: 6174 /* serviced */ return new String[] { "date", "Period" }; 6175 case 1901043637: 6176 /* location */ return new String[] { "CodeableConcept", "Address", "Reference" }; 6177 case -1285004149: 6178 /* quantity */ return new String[] { "SimpleQuantity" }; 6179 case -486196699: 6180 /* unitPrice */ return new String[] { "Money" }; 6181 case -1282148017: 6182 /* factor */ return new String[] { "decimal" }; 6183 case 108957: 6184 /* net */ return new String[] { "Money" }; 6185 case 115642: 6186 /* udi */ return new String[] { "Reference" }; 6187 case 1702620169: 6188 /* bodySite */ return new String[] { "CodeableConcept" }; 6189 case -1868566105: 6190 /* subSite */ return new String[] { "CodeableConcept" }; 6191 case 1524132147: 6192 /* encounter */ return new String[] { "Reference" }; 6193 case -1335224239: 6194 /* detail */ return new String[] {}; 6195 default: 6196 return super.getTypesForProperty(hash, name); 6197 } 6198 6199 } 6200 6201 @Override 6202 public Base addChild(String name) throws FHIRException { 6203 if (name.equals("sequence")) { 6204 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 6205 } else if (name.equals("careTeamSequence")) { 6206 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeamSequence"); 6207 } else if (name.equals("diagnosisSequence")) { 6208 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosisSequence"); 6209 } else if (name.equals("procedureSequence")) { 6210 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedureSequence"); 6211 } else if (name.equals("informationSequence")) { 6212 throw new FHIRException("Cannot call addChild on a singleton property Claim.informationSequence"); 6213 } else if (name.equals("revenue")) { 6214 this.revenue = new CodeableConcept(); 6215 return this.revenue; 6216 } else if (name.equals("category")) { 6217 this.category = new CodeableConcept(); 6218 return this.category; 6219 } else if (name.equals("productOrService")) { 6220 this.productOrService = new CodeableConcept(); 6221 return this.productOrService; 6222 } else if (name.equals("modifier")) { 6223 return addModifier(); 6224 } else if (name.equals("programCode")) { 6225 return addProgramCode(); 6226 } else if (name.equals("servicedDate")) { 6227 this.serviced = new DateType(); 6228 return this.serviced; 6229 } else if (name.equals("servicedPeriod")) { 6230 this.serviced = new Period(); 6231 return this.serviced; 6232 } else if (name.equals("locationCodeableConcept")) { 6233 this.location = new CodeableConcept(); 6234 return this.location; 6235 } else if (name.equals("locationAddress")) { 6236 this.location = new Address(); 6237 return this.location; 6238 } else if (name.equals("locationReference")) { 6239 this.location = new Reference(); 6240 return this.location; 6241 } else if (name.equals("quantity")) { 6242 this.quantity = new Quantity(); 6243 return this.quantity; 6244 } else if (name.equals("unitPrice")) { 6245 this.unitPrice = new Money(); 6246 return this.unitPrice; 6247 } else if (name.equals("factor")) { 6248 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 6249 } else if (name.equals("net")) { 6250 this.net = new Money(); 6251 return this.net; 6252 } else if (name.equals("udi")) { 6253 return addUdi(); 6254 } else if (name.equals("bodySite")) { 6255 this.bodySite = new CodeableConcept(); 6256 return this.bodySite; 6257 } else if (name.equals("subSite")) { 6258 return addSubSite(); 6259 } else if (name.equals("encounter")) { 6260 return addEncounter(); 6261 } else if (name.equals("detail")) { 6262 return addDetail(); 6263 } else 6264 return super.addChild(name); 6265 } 6266 6267 public ItemComponent copy() { 6268 ItemComponent dst = new ItemComponent(); 6269 copyValues(dst); 6270 return dst; 6271 } 6272 6273 public void copyValues(ItemComponent dst) { 6274 super.copyValues(dst); 6275 dst.sequence = sequence == null ? null : sequence.copy(); 6276 if (careTeamSequence != null) { 6277 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 6278 for (PositiveIntType i : careTeamSequence) 6279 dst.careTeamSequence.add(i.copy()); 6280 } 6281 ; 6282 if (diagnosisSequence != null) { 6283 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 6284 for (PositiveIntType i : diagnosisSequence) 6285 dst.diagnosisSequence.add(i.copy()); 6286 } 6287 ; 6288 if (procedureSequence != null) { 6289 dst.procedureSequence = new ArrayList<PositiveIntType>(); 6290 for (PositiveIntType i : procedureSequence) 6291 dst.procedureSequence.add(i.copy()); 6292 } 6293 ; 6294 if (informationSequence != null) { 6295 dst.informationSequence = new ArrayList<PositiveIntType>(); 6296 for (PositiveIntType i : informationSequence) 6297 dst.informationSequence.add(i.copy()); 6298 } 6299 ; 6300 dst.revenue = revenue == null ? null : revenue.copy(); 6301 dst.category = category == null ? null : category.copy(); 6302 dst.productOrService = productOrService == null ? null : productOrService.copy(); 6303 if (modifier != null) { 6304 dst.modifier = new ArrayList<CodeableConcept>(); 6305 for (CodeableConcept i : modifier) 6306 dst.modifier.add(i.copy()); 6307 } 6308 ; 6309 if (programCode != null) { 6310 dst.programCode = new ArrayList<CodeableConcept>(); 6311 for (CodeableConcept i : programCode) 6312 dst.programCode.add(i.copy()); 6313 } 6314 ; 6315 dst.serviced = serviced == null ? null : serviced.copy(); 6316 dst.location = location == null ? null : location.copy(); 6317 dst.quantity = quantity == null ? null : quantity.copy(); 6318 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6319 dst.factor = factor == null ? null : factor.copy(); 6320 dst.net = net == null ? null : net.copy(); 6321 if (udi != null) { 6322 dst.udi = new ArrayList<Reference>(); 6323 for (Reference i : udi) 6324 dst.udi.add(i.copy()); 6325 } 6326 ; 6327 dst.bodySite = bodySite == null ? null : bodySite.copy(); 6328 if (subSite != null) { 6329 dst.subSite = new ArrayList<CodeableConcept>(); 6330 for (CodeableConcept i : subSite) 6331 dst.subSite.add(i.copy()); 6332 } 6333 ; 6334 if (encounter != null) { 6335 dst.encounter = new ArrayList<Reference>(); 6336 for (Reference i : encounter) 6337 dst.encounter.add(i.copy()); 6338 } 6339 ; 6340 if (detail != null) { 6341 dst.detail = new ArrayList<DetailComponent>(); 6342 for (DetailComponent i : detail) 6343 dst.detail.add(i.copy()); 6344 } 6345 ; 6346 } 6347 6348 @Override 6349 public boolean equalsDeep(Base other_) { 6350 if (!super.equalsDeep(other_)) 6351 return false; 6352 if (!(other_ instanceof ItemComponent)) 6353 return false; 6354 ItemComponent o = (ItemComponent) other_; 6355 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamSequence, o.careTeamSequence, true) 6356 && compareDeep(diagnosisSequence, o.diagnosisSequence, true) 6357 && compareDeep(procedureSequence, o.procedureSequence, true) 6358 && compareDeep(informationSequence, o.informationSequence, true) && compareDeep(revenue, o.revenue, true) 6359 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 6360 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 6361 && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 6362 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 6363 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6364 && compareDeep(bodySite, o.bodySite, true) && compareDeep(subSite, o.subSite, true) 6365 && compareDeep(encounter, o.encounter, true) && compareDeep(detail, o.detail, true); 6366 } 6367 6368 @Override 6369 public boolean equalsShallow(Base other_) { 6370 if (!super.equalsShallow(other_)) 6371 return false; 6372 if (!(other_ instanceof ItemComponent)) 6373 return false; 6374 ItemComponent o = (ItemComponent) other_; 6375 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 6376 && compareValues(diagnosisSequence, o.diagnosisSequence, true) 6377 && compareValues(procedureSequence, o.procedureSequence, true) 6378 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true); 6379 } 6380 6381 public boolean isEmpty() { 6382 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamSequence, diagnosisSequence, 6383 procedureSequence, informationSequence, revenue, category, productOrService, modifier, programCode, serviced, 6384 location, quantity, unitPrice, factor, net, udi, bodySite, subSite, encounter, detail); 6385 } 6386 6387 public String fhirType() { 6388 return "Claim.item"; 6389 6390 } 6391 6392 } 6393 6394 @Block() 6395 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 6396 /** 6397 * A number to uniquely identify item entries. 6398 */ 6399 @Child(name = "sequence", type = { 6400 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6401 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 6402 protected PositiveIntType sequence; 6403 6404 /** 6405 * The type of revenue or cost center providing the product and/or service. 6406 */ 6407 @Child(name = "revenue", type = { 6408 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6409 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 6410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 6411 protected CodeableConcept revenue; 6412 6413 /** 6414 * Code to identify the general type of benefits under which products and 6415 * services are provided. 6416 */ 6417 @Child(name = "category", type = { 6418 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6419 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 6420 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6421 protected CodeableConcept category; 6422 6423 /** 6424 * When the value is a group code then this item collects a set of related claim 6425 * details, otherwise this contains the product, service, drug or other billing 6426 * code for the item. 6427 */ 6428 @Child(name = "productOrService", type = { 6429 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 6430 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 6431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 6432 protected CodeableConcept productOrService; 6433 6434 /** 6435 * Item typification or modifiers codes to convey additional context for the 6436 * product or service. 6437 */ 6438 @Child(name = "modifier", type = { 6439 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6440 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 6441 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 6442 protected List<CodeableConcept> modifier; 6443 6444 /** 6445 * Identifies the program under which this may be recovered. 6446 */ 6447 @Child(name = "programCode", type = { 6448 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6449 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 6450 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 6451 protected List<CodeableConcept> programCode; 6452 6453 /** 6454 * The number of repetitions of a service or product. 6455 */ 6456 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6457 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 6458 protected Quantity quantity; 6459 6460 /** 6461 * If the item is not a group then this is the fee for the product or service, 6462 * otherwise this is the total of the fees for the details of the group. 6463 */ 6464 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6465 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 6466 protected Money unitPrice; 6467 6468 /** 6469 * A real number that represents a multiplier used in determining the overall 6470 * value of services delivered and/or goods received. The concept of a Factor 6471 * allows for a discount or surcharge multiplier to be applied to a monetary 6472 * amount. 6473 */ 6474 @Child(name = "factor", type = { 6475 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6476 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 6477 protected DecimalType factor; 6478 6479 /** 6480 * The quantity times the unit price for an additional service or product or 6481 * charge. 6482 */ 6483 @Child(name = "net", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6484 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 6485 protected Money net; 6486 6487 /** 6488 * Unique Device Identifiers associated with this line item. 6489 */ 6490 @Child(name = "udi", type = { 6491 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6492 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 6493 protected List<Reference> udi; 6494 /** 6495 * The actual objects that are the target of the reference (Unique Device 6496 * Identifiers associated with this line item.) 6497 */ 6498 protected List<Device> udiTarget; 6499 6500 /** 6501 * A claim detail line. Either a simple (a product or service) or a 'group' of 6502 * sub-details which are simple items. 6503 */ 6504 @Child(name = "subDetail", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6505 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 6506 protected List<SubDetailComponent> subDetail; 6507 6508 private static final long serialVersionUID = -1245004924L; 6509 6510 /** 6511 * Constructor 6512 */ 6513 public DetailComponent() { 6514 super(); 6515 } 6516 6517 /** 6518 * Constructor 6519 */ 6520 public DetailComponent(PositiveIntType sequence, CodeableConcept productOrService) { 6521 super(); 6522 this.sequence = sequence; 6523 this.productOrService = productOrService; 6524 } 6525 6526 /** 6527 * @return {@link #sequence} (A number to uniquely identify item entries.). This 6528 * is the underlying object with id, value and extensions. The accessor 6529 * "getSequence" gives direct access to the value 6530 */ 6531 public PositiveIntType getSequenceElement() { 6532 if (this.sequence == null) 6533 if (Configuration.errorOnAutoCreate()) 6534 throw new Error("Attempt to auto-create DetailComponent.sequence"); 6535 else if (Configuration.doAutoCreate()) 6536 this.sequence = new PositiveIntType(); // bb 6537 return this.sequence; 6538 } 6539 6540 public boolean hasSequenceElement() { 6541 return this.sequence != null && !this.sequence.isEmpty(); 6542 } 6543 6544 public boolean hasSequence() { 6545 return this.sequence != null && !this.sequence.isEmpty(); 6546 } 6547 6548 /** 6549 * @param value {@link #sequence} (A number to uniquely identify item entries.). 6550 * This is the underlying object with id, value and extensions. The 6551 * accessor "getSequence" gives direct access to the value 6552 */ 6553 public DetailComponent setSequenceElement(PositiveIntType value) { 6554 this.sequence = value; 6555 return this; 6556 } 6557 6558 /** 6559 * @return A number to uniquely identify item entries. 6560 */ 6561 public int getSequence() { 6562 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6563 } 6564 6565 /** 6566 * @param value A number to uniquely identify item entries. 6567 */ 6568 public DetailComponent setSequence(int value) { 6569 if (this.sequence == null) 6570 this.sequence = new PositiveIntType(); 6571 this.sequence.setValue(value); 6572 return this; 6573 } 6574 6575 /** 6576 * @return {@link #revenue} (The type of revenue or cost center providing the 6577 * product and/or service.) 6578 */ 6579 public CodeableConcept getRevenue() { 6580 if (this.revenue == null) 6581 if (Configuration.errorOnAutoCreate()) 6582 throw new Error("Attempt to auto-create DetailComponent.revenue"); 6583 else if (Configuration.doAutoCreate()) 6584 this.revenue = new CodeableConcept(); // cc 6585 return this.revenue; 6586 } 6587 6588 public boolean hasRevenue() { 6589 return this.revenue != null && !this.revenue.isEmpty(); 6590 } 6591 6592 /** 6593 * @param value {@link #revenue} (The type of revenue or cost center providing 6594 * the product and/or service.) 6595 */ 6596 public DetailComponent setRevenue(CodeableConcept value) { 6597 this.revenue = value; 6598 return this; 6599 } 6600 6601 /** 6602 * @return {@link #category} (Code to identify the general type of benefits 6603 * under which products and services are provided.) 6604 */ 6605 public CodeableConcept getCategory() { 6606 if (this.category == null) 6607 if (Configuration.errorOnAutoCreate()) 6608 throw new Error("Attempt to auto-create DetailComponent.category"); 6609 else if (Configuration.doAutoCreate()) 6610 this.category = new CodeableConcept(); // cc 6611 return this.category; 6612 } 6613 6614 public boolean hasCategory() { 6615 return this.category != null && !this.category.isEmpty(); 6616 } 6617 6618 /** 6619 * @param value {@link #category} (Code to identify the general type of benefits 6620 * under which products and services are provided.) 6621 */ 6622 public DetailComponent setCategory(CodeableConcept value) { 6623 this.category = value; 6624 return this; 6625 } 6626 6627 /** 6628 * @return {@link #productOrService} (When the value is a group code then this 6629 * item collects a set of related claim details, otherwise this contains 6630 * the product, service, drug or other billing code for the item.) 6631 */ 6632 public CodeableConcept getProductOrService() { 6633 if (this.productOrService == null) 6634 if (Configuration.errorOnAutoCreate()) 6635 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 6636 else if (Configuration.doAutoCreate()) 6637 this.productOrService = new CodeableConcept(); // cc 6638 return this.productOrService; 6639 } 6640 6641 public boolean hasProductOrService() { 6642 return this.productOrService != null && !this.productOrService.isEmpty(); 6643 } 6644 6645 /** 6646 * @param value {@link #productOrService} (When the value is a group code then 6647 * this item collects a set of related claim details, otherwise 6648 * this contains the product, service, drug or other billing code 6649 * for the item.) 6650 */ 6651 public DetailComponent setProductOrService(CodeableConcept value) { 6652 this.productOrService = value; 6653 return this; 6654 } 6655 6656 /** 6657 * @return {@link #modifier} (Item typification or modifiers codes to convey 6658 * additional context for the product or service.) 6659 */ 6660 public List<CodeableConcept> getModifier() { 6661 if (this.modifier == null) 6662 this.modifier = new ArrayList<CodeableConcept>(); 6663 return this.modifier; 6664 } 6665 6666 /** 6667 * @return Returns a reference to <code>this</code> for easy method chaining 6668 */ 6669 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 6670 this.modifier = theModifier; 6671 return this; 6672 } 6673 6674 public boolean hasModifier() { 6675 if (this.modifier == null) 6676 return false; 6677 for (CodeableConcept item : this.modifier) 6678 if (!item.isEmpty()) 6679 return true; 6680 return false; 6681 } 6682 6683 public CodeableConcept addModifier() { // 3 6684 CodeableConcept t = new CodeableConcept(); 6685 if (this.modifier == null) 6686 this.modifier = new ArrayList<CodeableConcept>(); 6687 this.modifier.add(t); 6688 return t; 6689 } 6690 6691 public DetailComponent addModifier(CodeableConcept t) { // 3 6692 if (t == null) 6693 return this; 6694 if (this.modifier == null) 6695 this.modifier = new ArrayList<CodeableConcept>(); 6696 this.modifier.add(t); 6697 return this; 6698 } 6699 6700 /** 6701 * @return The first repetition of repeating field {@link #modifier}, creating 6702 * it if it does not already exist 6703 */ 6704 public CodeableConcept getModifierFirstRep() { 6705 if (getModifier().isEmpty()) { 6706 addModifier(); 6707 } 6708 return getModifier().get(0); 6709 } 6710 6711 /** 6712 * @return {@link #programCode} (Identifies the program under which this may be 6713 * recovered.) 6714 */ 6715 public List<CodeableConcept> getProgramCode() { 6716 if (this.programCode == null) 6717 this.programCode = new ArrayList<CodeableConcept>(); 6718 return this.programCode; 6719 } 6720 6721 /** 6722 * @return Returns a reference to <code>this</code> for easy method chaining 6723 */ 6724 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6725 this.programCode = theProgramCode; 6726 return this; 6727 } 6728 6729 public boolean hasProgramCode() { 6730 if (this.programCode == null) 6731 return false; 6732 for (CodeableConcept item : this.programCode) 6733 if (!item.isEmpty()) 6734 return true; 6735 return false; 6736 } 6737 6738 public CodeableConcept addProgramCode() { // 3 6739 CodeableConcept t = new CodeableConcept(); 6740 if (this.programCode == null) 6741 this.programCode = new ArrayList<CodeableConcept>(); 6742 this.programCode.add(t); 6743 return t; 6744 } 6745 6746 public DetailComponent addProgramCode(CodeableConcept t) { // 3 6747 if (t == null) 6748 return this; 6749 if (this.programCode == null) 6750 this.programCode = new ArrayList<CodeableConcept>(); 6751 this.programCode.add(t); 6752 return this; 6753 } 6754 6755 /** 6756 * @return The first repetition of repeating field {@link #programCode}, 6757 * creating it if it does not already exist 6758 */ 6759 public CodeableConcept getProgramCodeFirstRep() { 6760 if (getProgramCode().isEmpty()) { 6761 addProgramCode(); 6762 } 6763 return getProgramCode().get(0); 6764 } 6765 6766 /** 6767 * @return {@link #quantity} (The number of repetitions of a service or 6768 * product.) 6769 */ 6770 public Quantity getQuantity() { 6771 if (this.quantity == null) 6772 if (Configuration.errorOnAutoCreate()) 6773 throw new Error("Attempt to auto-create DetailComponent.quantity"); 6774 else if (Configuration.doAutoCreate()) 6775 this.quantity = new Quantity(); // cc 6776 return this.quantity; 6777 } 6778 6779 public boolean hasQuantity() { 6780 return this.quantity != null && !this.quantity.isEmpty(); 6781 } 6782 6783 /** 6784 * @param value {@link #quantity} (The number of repetitions of a service or 6785 * product.) 6786 */ 6787 public DetailComponent setQuantity(Quantity value) { 6788 this.quantity = value; 6789 return this; 6790 } 6791 6792 /** 6793 * @return {@link #unitPrice} (If the item is not a group then this is the fee 6794 * for the product or service, otherwise this is the total of the fees 6795 * for the details of the group.) 6796 */ 6797 public Money getUnitPrice() { 6798 if (this.unitPrice == null) 6799 if (Configuration.errorOnAutoCreate()) 6800 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 6801 else if (Configuration.doAutoCreate()) 6802 this.unitPrice = new Money(); // cc 6803 return this.unitPrice; 6804 } 6805 6806 public boolean hasUnitPrice() { 6807 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6808 } 6809 6810 /** 6811 * @param value {@link #unitPrice} (If the item is not a group then this is the 6812 * fee for the product or service, otherwise this is the total of 6813 * the fees for the details of the group.) 6814 */ 6815 public DetailComponent setUnitPrice(Money value) { 6816 this.unitPrice = value; 6817 return this; 6818 } 6819 6820 /** 6821 * @return {@link #factor} (A real number that represents a multiplier used in 6822 * determining the overall value of services delivered and/or goods 6823 * received. The concept of a Factor allows for a discount or surcharge 6824 * multiplier to be applied to a monetary amount.). This is the 6825 * underlying object with id, value and extensions. The accessor 6826 * "getFactor" gives direct access to the value 6827 */ 6828 public DecimalType getFactorElement() { 6829 if (this.factor == null) 6830 if (Configuration.errorOnAutoCreate()) 6831 throw new Error("Attempt to auto-create DetailComponent.factor"); 6832 else if (Configuration.doAutoCreate()) 6833 this.factor = new DecimalType(); // bb 6834 return this.factor; 6835 } 6836 6837 public boolean hasFactorElement() { 6838 return this.factor != null && !this.factor.isEmpty(); 6839 } 6840 6841 public boolean hasFactor() { 6842 return this.factor != null && !this.factor.isEmpty(); 6843 } 6844 6845 /** 6846 * @param value {@link #factor} (A real number that represents a multiplier used 6847 * in determining the overall value of services delivered and/or 6848 * goods received. The concept of a Factor allows for a discount or 6849 * surcharge multiplier to be applied to a monetary amount.). This 6850 * is the underlying object with id, value and extensions. The 6851 * accessor "getFactor" gives direct access to the value 6852 */ 6853 public DetailComponent setFactorElement(DecimalType value) { 6854 this.factor = value; 6855 return this; 6856 } 6857 6858 /** 6859 * @return A real number that represents a multiplier used in determining the 6860 * overall value of services delivered and/or goods received. The 6861 * concept of a Factor allows for a discount or surcharge multiplier to 6862 * be applied to a monetary amount. 6863 */ 6864 public BigDecimal getFactor() { 6865 return this.factor == null ? null : this.factor.getValue(); 6866 } 6867 6868 /** 6869 * @param value A real number that represents a multiplier used in determining 6870 * the overall value of services delivered and/or goods received. 6871 * The concept of a Factor allows for a discount or surcharge 6872 * multiplier to be applied to a monetary amount. 6873 */ 6874 public DetailComponent setFactor(BigDecimal value) { 6875 if (value == null) 6876 this.factor = null; 6877 else { 6878 if (this.factor == null) 6879 this.factor = new DecimalType(); 6880 this.factor.setValue(value); 6881 } 6882 return this; 6883 } 6884 6885 /** 6886 * @param value A real number that represents a multiplier used in determining 6887 * the overall value of services delivered and/or goods received. 6888 * The concept of a Factor allows for a discount or surcharge 6889 * multiplier to be applied to a monetary amount. 6890 */ 6891 public DetailComponent setFactor(long value) { 6892 this.factor = new DecimalType(); 6893 this.factor.setValue(value); 6894 return this; 6895 } 6896 6897 /** 6898 * @param value A real number that represents a multiplier used in determining 6899 * the overall value of services delivered and/or goods received. 6900 * The concept of a Factor allows for a discount or surcharge 6901 * multiplier to be applied to a monetary amount. 6902 */ 6903 public DetailComponent setFactor(double value) { 6904 this.factor = new DecimalType(); 6905 this.factor.setValue(value); 6906 return this; 6907 } 6908 6909 /** 6910 * @return {@link #net} (The quantity times the unit price for an additional 6911 * service or product or charge.) 6912 */ 6913 public Money getNet() { 6914 if (this.net == null) 6915 if (Configuration.errorOnAutoCreate()) 6916 throw new Error("Attempt to auto-create DetailComponent.net"); 6917 else if (Configuration.doAutoCreate()) 6918 this.net = new Money(); // cc 6919 return this.net; 6920 } 6921 6922 public boolean hasNet() { 6923 return this.net != null && !this.net.isEmpty(); 6924 } 6925 6926 /** 6927 * @param value {@link #net} (The quantity times the unit price for an 6928 * additional service or product or charge.) 6929 */ 6930 public DetailComponent setNet(Money value) { 6931 this.net = value; 6932 return this; 6933 } 6934 6935 /** 6936 * @return {@link #udi} (Unique Device Identifiers associated with this line 6937 * item.) 6938 */ 6939 public List<Reference> getUdi() { 6940 if (this.udi == null) 6941 this.udi = new ArrayList<Reference>(); 6942 return this.udi; 6943 } 6944 6945 /** 6946 * @return Returns a reference to <code>this</code> for easy method chaining 6947 */ 6948 public DetailComponent setUdi(List<Reference> theUdi) { 6949 this.udi = theUdi; 6950 return this; 6951 } 6952 6953 public boolean hasUdi() { 6954 if (this.udi == null) 6955 return false; 6956 for (Reference item : this.udi) 6957 if (!item.isEmpty()) 6958 return true; 6959 return false; 6960 } 6961 6962 public Reference addUdi() { // 3 6963 Reference t = new Reference(); 6964 if (this.udi == null) 6965 this.udi = new ArrayList<Reference>(); 6966 this.udi.add(t); 6967 return t; 6968 } 6969 6970 public DetailComponent addUdi(Reference t) { // 3 6971 if (t == null) 6972 return this; 6973 if (this.udi == null) 6974 this.udi = new ArrayList<Reference>(); 6975 this.udi.add(t); 6976 return this; 6977 } 6978 6979 /** 6980 * @return The first repetition of repeating field {@link #udi}, creating it if 6981 * it does not already exist 6982 */ 6983 public Reference getUdiFirstRep() { 6984 if (getUdi().isEmpty()) { 6985 addUdi(); 6986 } 6987 return getUdi().get(0); 6988 } 6989 6990 /** 6991 * @deprecated Use Reference#setResource(IBaseResource) instead 6992 */ 6993 @Deprecated 6994 public List<Device> getUdiTarget() { 6995 if (this.udiTarget == null) 6996 this.udiTarget = new ArrayList<Device>(); 6997 return this.udiTarget; 6998 } 6999 7000 /** 7001 * @deprecated Use Reference#setResource(IBaseResource) instead 7002 */ 7003 @Deprecated 7004 public Device addUdiTarget() { 7005 Device r = new Device(); 7006 if (this.udiTarget == null) 7007 this.udiTarget = new ArrayList<Device>(); 7008 this.udiTarget.add(r); 7009 return r; 7010 } 7011 7012 /** 7013 * @return {@link #subDetail} (A claim detail line. Either a simple (a product 7014 * or service) or a 'group' of sub-details which are simple items.) 7015 */ 7016 public List<SubDetailComponent> getSubDetail() { 7017 if (this.subDetail == null) 7018 this.subDetail = new ArrayList<SubDetailComponent>(); 7019 return this.subDetail; 7020 } 7021 7022 /** 7023 * @return Returns a reference to <code>this</code> for easy method chaining 7024 */ 7025 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 7026 this.subDetail = theSubDetail; 7027 return this; 7028 } 7029 7030 public boolean hasSubDetail() { 7031 if (this.subDetail == null) 7032 return false; 7033 for (SubDetailComponent item : this.subDetail) 7034 if (!item.isEmpty()) 7035 return true; 7036 return false; 7037 } 7038 7039 public SubDetailComponent addSubDetail() { // 3 7040 SubDetailComponent t = new SubDetailComponent(); 7041 if (this.subDetail == null) 7042 this.subDetail = new ArrayList<SubDetailComponent>(); 7043 this.subDetail.add(t); 7044 return t; 7045 } 7046 7047 public DetailComponent addSubDetail(SubDetailComponent t) { // 3 7048 if (t == null) 7049 return this; 7050 if (this.subDetail == null) 7051 this.subDetail = new ArrayList<SubDetailComponent>(); 7052 this.subDetail.add(t); 7053 return this; 7054 } 7055 7056 /** 7057 * @return The first repetition of repeating field {@link #subDetail}, creating 7058 * it if it does not already exist 7059 */ 7060 public SubDetailComponent getSubDetailFirstRep() { 7061 if (getSubDetail().isEmpty()) { 7062 addSubDetail(); 7063 } 7064 return getSubDetail().get(0); 7065 } 7066 7067 protected void listChildren(List<Property> children) { 7068 super.listChildren(children); 7069 children 7070 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 7071 children.add(new Property("revenue", "CodeableConcept", 7072 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7073 children.add(new Property("category", "CodeableConcept", 7074 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 7075 category)); 7076 children.add(new Property("productOrService", "CodeableConcept", 7077 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 7078 0, 1, productOrService)); 7079 children.add(new Property("modifier", "CodeableConcept", 7080 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 7081 java.lang.Integer.MAX_VALUE, modifier)); 7082 children.add(new Property("programCode", "CodeableConcept", 7083 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7084 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 7085 1, quantity)); 7086 children.add(new Property("unitPrice", "Money", 7087 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 7088 0, 1, unitPrice)); 7089 children.add(new Property("factor", "decimal", 7090 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7091 0, 1, factor)); 7092 children.add(new Property("net", "Money", 7093 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 7094 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 7095 0, java.lang.Integer.MAX_VALUE, udi)); 7096 children.add(new Property("subDetail", "", 7097 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 7098 0, java.lang.Integer.MAX_VALUE, subDetail)); 7099 } 7100 7101 @Override 7102 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7103 switch (_hash) { 7104 case 1349547969: 7105 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 7106 1, sequence); 7107 case 1099842588: 7108 /* revenue */ return new Property("revenue", "CodeableConcept", 7109 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7110 case 50511102: 7111 /* category */ return new Property("category", "CodeableConcept", 7112 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 7113 category); 7114 case 1957227299: 7115 /* productOrService */ return new Property("productOrService", "CodeableConcept", 7116 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 7117 0, 1, productOrService); 7118 case -615513385: 7119 /* modifier */ return new Property("modifier", "CodeableConcept", 7120 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 7121 java.lang.Integer.MAX_VALUE, modifier); 7122 case 1010065041: 7123 /* programCode */ return new Property("programCode", "CodeableConcept", 7124 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7125 case -1285004149: 7126 /* quantity */ return new Property("quantity", "SimpleQuantity", 7127 "The number of repetitions of a service or product.", 0, 1, quantity); 7128 case -486196699: 7129 /* unitPrice */ return new Property("unitPrice", "Money", 7130 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 7131 0, 1, unitPrice); 7132 case -1282148017: 7133 /* factor */ return new Property("factor", "decimal", 7134 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7135 0, 1, factor); 7136 case 108957: 7137 /* net */ return new Property("net", "Money", 7138 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 7139 case 115642: 7140 /* udi */ return new Property("udi", "Reference(Device)", 7141 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7142 case -828829007: 7143 /* subDetail */ return new Property("subDetail", "", 7144 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 7145 0, java.lang.Integer.MAX_VALUE, subDetail); 7146 default: 7147 return super.getNamedProperty(_hash, _name, _checkValid); 7148 } 7149 7150 } 7151 7152 @Override 7153 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7154 switch (hash) { 7155 case 1349547969: 7156 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 7157 case 1099842588: 7158 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 7159 case 50511102: 7160 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 7161 case 1957227299: 7162 /* productOrService */ return this.productOrService == null ? new Base[0] 7163 : new Base[] { this.productOrService }; // CodeableConcept 7164 case -615513385: 7165 /* modifier */ return this.modifier == null ? new Base[0] 7166 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7167 case 1010065041: 7168 /* programCode */ return this.programCode == null ? new Base[0] 7169 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7170 case -1285004149: 7171 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7172 case -486196699: 7173 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 7174 case -1282148017: 7175 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 7176 case 108957: 7177 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 7178 case 115642: 7179 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7180 case -828829007: 7181 /* subDetail */ return this.subDetail == null ? new Base[0] 7182 : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 7183 default: 7184 return super.getProperty(hash, name, checkValid); 7185 } 7186 7187 } 7188 7189 @Override 7190 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7191 switch (hash) { 7192 case 1349547969: // sequence 7193 this.sequence = castToPositiveInt(value); // PositiveIntType 7194 return value; 7195 case 1099842588: // revenue 7196 this.revenue = castToCodeableConcept(value); // CodeableConcept 7197 return value; 7198 case 50511102: // category 7199 this.category = castToCodeableConcept(value); // CodeableConcept 7200 return value; 7201 case 1957227299: // productOrService 7202 this.productOrService = castToCodeableConcept(value); // CodeableConcept 7203 return value; 7204 case -615513385: // modifier 7205 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7206 return value; 7207 case 1010065041: // programCode 7208 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 7209 return value; 7210 case -1285004149: // quantity 7211 this.quantity = castToQuantity(value); // Quantity 7212 return value; 7213 case -486196699: // unitPrice 7214 this.unitPrice = castToMoney(value); // Money 7215 return value; 7216 case -1282148017: // factor 7217 this.factor = castToDecimal(value); // DecimalType 7218 return value; 7219 case 108957: // net 7220 this.net = castToMoney(value); // Money 7221 return value; 7222 case 115642: // udi 7223 this.getUdi().add(castToReference(value)); // Reference 7224 return value; 7225 case -828829007: // subDetail 7226 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 7227 return value; 7228 default: 7229 return super.setProperty(hash, name, value); 7230 } 7231 7232 } 7233 7234 @Override 7235 public Base setProperty(String name, Base value) throws FHIRException { 7236 if (name.equals("sequence")) { 7237 this.sequence = castToPositiveInt(value); // PositiveIntType 7238 } else if (name.equals("revenue")) { 7239 this.revenue = castToCodeableConcept(value); // CodeableConcept 7240 } else if (name.equals("category")) { 7241 this.category = castToCodeableConcept(value); // CodeableConcept 7242 } else if (name.equals("productOrService")) { 7243 this.productOrService = castToCodeableConcept(value); // CodeableConcept 7244 } else if (name.equals("modifier")) { 7245 this.getModifier().add(castToCodeableConcept(value)); 7246 } else if (name.equals("programCode")) { 7247 this.getProgramCode().add(castToCodeableConcept(value)); 7248 } else if (name.equals("quantity")) { 7249 this.quantity = castToQuantity(value); // Quantity 7250 } else if (name.equals("unitPrice")) { 7251 this.unitPrice = castToMoney(value); // Money 7252 } else if (name.equals("factor")) { 7253 this.factor = castToDecimal(value); // DecimalType 7254 } else if (name.equals("net")) { 7255 this.net = castToMoney(value); // Money 7256 } else if (name.equals("udi")) { 7257 this.getUdi().add(castToReference(value)); 7258 } else if (name.equals("subDetail")) { 7259 this.getSubDetail().add((SubDetailComponent) value); 7260 } else 7261 return super.setProperty(name, value); 7262 return value; 7263 } 7264 7265 @Override 7266 public void removeChild(String name, Base value) throws FHIRException { 7267 if (name.equals("sequence")) { 7268 this.sequence = null; 7269 } else if (name.equals("revenue")) { 7270 this.revenue = null; 7271 } else if (name.equals("category")) { 7272 this.category = null; 7273 } else if (name.equals("productOrService")) { 7274 this.productOrService = null; 7275 } else if (name.equals("modifier")) { 7276 this.getModifier().remove(castToCodeableConcept(value)); 7277 } else if (name.equals("programCode")) { 7278 this.getProgramCode().remove(castToCodeableConcept(value)); 7279 } else if (name.equals("quantity")) { 7280 this.quantity = null; 7281 } else if (name.equals("unitPrice")) { 7282 this.unitPrice = null; 7283 } else if (name.equals("factor")) { 7284 this.factor = null; 7285 } else if (name.equals("net")) { 7286 this.net = null; 7287 } else if (name.equals("udi")) { 7288 this.getUdi().remove(castToReference(value)); 7289 } else if (name.equals("subDetail")) { 7290 this.getSubDetail().remove((SubDetailComponent) value); 7291 } else 7292 super.removeChild(name, value); 7293 7294 } 7295 7296 @Override 7297 public Base makeProperty(int hash, String name) throws FHIRException { 7298 switch (hash) { 7299 case 1349547969: 7300 return getSequenceElement(); 7301 case 1099842588: 7302 return getRevenue(); 7303 case 50511102: 7304 return getCategory(); 7305 case 1957227299: 7306 return getProductOrService(); 7307 case -615513385: 7308 return addModifier(); 7309 case 1010065041: 7310 return addProgramCode(); 7311 case -1285004149: 7312 return getQuantity(); 7313 case -486196699: 7314 return getUnitPrice(); 7315 case -1282148017: 7316 return getFactorElement(); 7317 case 108957: 7318 return getNet(); 7319 case 115642: 7320 return addUdi(); 7321 case -828829007: 7322 return addSubDetail(); 7323 default: 7324 return super.makeProperty(hash, name); 7325 } 7326 7327 } 7328 7329 @Override 7330 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7331 switch (hash) { 7332 case 1349547969: 7333 /* sequence */ return new String[] { "positiveInt" }; 7334 case 1099842588: 7335 /* revenue */ return new String[] { "CodeableConcept" }; 7336 case 50511102: 7337 /* category */ return new String[] { "CodeableConcept" }; 7338 case 1957227299: 7339 /* productOrService */ return new String[] { "CodeableConcept" }; 7340 case -615513385: 7341 /* modifier */ return new String[] { "CodeableConcept" }; 7342 case 1010065041: 7343 /* programCode */ return new String[] { "CodeableConcept" }; 7344 case -1285004149: 7345 /* quantity */ return new String[] { "SimpleQuantity" }; 7346 case -486196699: 7347 /* unitPrice */ return new String[] { "Money" }; 7348 case -1282148017: 7349 /* factor */ return new String[] { "decimal" }; 7350 case 108957: 7351 /* net */ return new String[] { "Money" }; 7352 case 115642: 7353 /* udi */ return new String[] { "Reference" }; 7354 case -828829007: 7355 /* subDetail */ return new String[] {}; 7356 default: 7357 return super.getTypesForProperty(hash, name); 7358 } 7359 7360 } 7361 7362 @Override 7363 public Base addChild(String name) throws FHIRException { 7364 if (name.equals("sequence")) { 7365 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 7366 } else if (name.equals("revenue")) { 7367 this.revenue = new CodeableConcept(); 7368 return this.revenue; 7369 } else if (name.equals("category")) { 7370 this.category = new CodeableConcept(); 7371 return this.category; 7372 } else if (name.equals("productOrService")) { 7373 this.productOrService = new CodeableConcept(); 7374 return this.productOrService; 7375 } else if (name.equals("modifier")) { 7376 return addModifier(); 7377 } else if (name.equals("programCode")) { 7378 return addProgramCode(); 7379 } else if (name.equals("quantity")) { 7380 this.quantity = new Quantity(); 7381 return this.quantity; 7382 } else if (name.equals("unitPrice")) { 7383 this.unitPrice = new Money(); 7384 return this.unitPrice; 7385 } else if (name.equals("factor")) { 7386 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 7387 } else if (name.equals("net")) { 7388 this.net = new Money(); 7389 return this.net; 7390 } else if (name.equals("udi")) { 7391 return addUdi(); 7392 } else if (name.equals("subDetail")) { 7393 return addSubDetail(); 7394 } else 7395 return super.addChild(name); 7396 } 7397 7398 public DetailComponent copy() { 7399 DetailComponent dst = new DetailComponent(); 7400 copyValues(dst); 7401 return dst; 7402 } 7403 7404 public void copyValues(DetailComponent dst) { 7405 super.copyValues(dst); 7406 dst.sequence = sequence == null ? null : sequence.copy(); 7407 dst.revenue = revenue == null ? null : revenue.copy(); 7408 dst.category = category == null ? null : category.copy(); 7409 dst.productOrService = productOrService == null ? null : productOrService.copy(); 7410 if (modifier != null) { 7411 dst.modifier = new ArrayList<CodeableConcept>(); 7412 for (CodeableConcept i : modifier) 7413 dst.modifier.add(i.copy()); 7414 } 7415 ; 7416 if (programCode != null) { 7417 dst.programCode = new ArrayList<CodeableConcept>(); 7418 for (CodeableConcept i : programCode) 7419 dst.programCode.add(i.copy()); 7420 } 7421 ; 7422 dst.quantity = quantity == null ? null : quantity.copy(); 7423 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7424 dst.factor = factor == null ? null : factor.copy(); 7425 dst.net = net == null ? null : net.copy(); 7426 if (udi != null) { 7427 dst.udi = new ArrayList<Reference>(); 7428 for (Reference i : udi) 7429 dst.udi.add(i.copy()); 7430 } 7431 ; 7432 if (subDetail != null) { 7433 dst.subDetail = new ArrayList<SubDetailComponent>(); 7434 for (SubDetailComponent i : subDetail) 7435 dst.subDetail.add(i.copy()); 7436 } 7437 ; 7438 } 7439 7440 @Override 7441 public boolean equalsDeep(Base other_) { 7442 if (!super.equalsDeep(other_)) 7443 return false; 7444 if (!(other_ instanceof DetailComponent)) 7445 return false; 7446 DetailComponent o = (DetailComponent) other_; 7447 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) 7448 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 7449 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 7450 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 7451 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7452 && compareDeep(subDetail, o.subDetail, true); 7453 } 7454 7455 @Override 7456 public boolean equalsShallow(Base other_) { 7457 if (!super.equalsShallow(other_)) 7458 return false; 7459 if (!(other_ instanceof DetailComponent)) 7460 return false; 7461 DetailComponent o = (DetailComponent) other_; 7462 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 7463 } 7464 7465 public boolean isEmpty() { 7466 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category, productOrService, 7467 modifier, programCode, quantity, unitPrice, factor, net, udi, subDetail); 7468 } 7469 7470 public String fhirType() { 7471 return "Claim.item.detail"; 7472 7473 } 7474 7475 } 7476 7477 @Block() 7478 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 7479 /** 7480 * A number to uniquely identify item entries. 7481 */ 7482 @Child(name = "sequence", type = { 7483 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7484 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 7485 protected PositiveIntType sequence; 7486 7487 /** 7488 * The type of revenue or cost center providing the product and/or service. 7489 */ 7490 @Child(name = "revenue", type = { 7491 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7492 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 7493 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 7494 protected CodeableConcept revenue; 7495 7496 /** 7497 * Code to identify the general type of benefits under which products and 7498 * services are provided. 7499 */ 7500 @Child(name = "category", type = { 7501 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 7502 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 7503 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 7504 protected CodeableConcept category; 7505 7506 /** 7507 * When the value is a group code then this item collects a set of related claim 7508 * details, otherwise this contains the product, service, drug or other billing 7509 * code for the item. 7510 */ 7511 @Child(name = "productOrService", type = { 7512 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 7513 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 7514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 7515 protected CodeableConcept productOrService; 7516 7517 /** 7518 * Item typification or modifiers codes to convey additional context for the 7519 * product or service. 7520 */ 7521 @Child(name = "modifier", type = { 7522 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7523 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 7524 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 7525 protected List<CodeableConcept> modifier; 7526 7527 /** 7528 * Identifies the program under which this may be recovered. 7529 */ 7530 @Child(name = "programCode", type = { 7531 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7532 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 7533 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 7534 protected List<CodeableConcept> programCode; 7535 7536 /** 7537 * The number of repetitions of a service or product. 7538 */ 7539 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 7540 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 7541 protected Quantity quantity; 7542 7543 /** 7544 * If the item is not a group then this is the fee for the product or service, 7545 * otherwise this is the total of the fees for the details of the group. 7546 */ 7547 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 7548 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 7549 protected Money unitPrice; 7550 7551 /** 7552 * A real number that represents a multiplier used in determining the overall 7553 * value of services delivered and/or goods received. The concept of a Factor 7554 * allows for a discount or surcharge multiplier to be applied to a monetary 7555 * amount. 7556 */ 7557 @Child(name = "factor", type = { 7558 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 7559 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 7560 protected DecimalType factor; 7561 7562 /** 7563 * The quantity times the unit price for an additional service or product or 7564 * charge. 7565 */ 7566 @Child(name = "net", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 7567 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 7568 protected Money net; 7569 7570 /** 7571 * Unique Device Identifiers associated with this line item. 7572 */ 7573 @Child(name = "udi", type = { 7574 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7575 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 7576 protected List<Reference> udi; 7577 /** 7578 * The actual objects that are the target of the reference (Unique Device 7579 * Identifiers associated with this line item.) 7580 */ 7581 protected List<Device> udiTarget; 7582 7583 private static final long serialVersionUID = 1133026301L; 7584 7585 /** 7586 * Constructor 7587 */ 7588 public SubDetailComponent() { 7589 super(); 7590 } 7591 7592 /** 7593 * Constructor 7594 */ 7595 public SubDetailComponent(PositiveIntType sequence, CodeableConcept productOrService) { 7596 super(); 7597 this.sequence = sequence; 7598 this.productOrService = productOrService; 7599 } 7600 7601 /** 7602 * @return {@link #sequence} (A number to uniquely identify item entries.). This 7603 * is the underlying object with id, value and extensions. The accessor 7604 * "getSequence" gives direct access to the value 7605 */ 7606 public PositiveIntType getSequenceElement() { 7607 if (this.sequence == null) 7608 if (Configuration.errorOnAutoCreate()) 7609 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 7610 else if (Configuration.doAutoCreate()) 7611 this.sequence = new PositiveIntType(); // bb 7612 return this.sequence; 7613 } 7614 7615 public boolean hasSequenceElement() { 7616 return this.sequence != null && !this.sequence.isEmpty(); 7617 } 7618 7619 public boolean hasSequence() { 7620 return this.sequence != null && !this.sequence.isEmpty(); 7621 } 7622 7623 /** 7624 * @param value {@link #sequence} (A number to uniquely identify item entries.). 7625 * This is the underlying object with id, value and extensions. The 7626 * accessor "getSequence" gives direct access to the value 7627 */ 7628 public SubDetailComponent setSequenceElement(PositiveIntType value) { 7629 this.sequence = value; 7630 return this; 7631 } 7632 7633 /** 7634 * @return A number to uniquely identify item entries. 7635 */ 7636 public int getSequence() { 7637 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7638 } 7639 7640 /** 7641 * @param value A number to uniquely identify item entries. 7642 */ 7643 public SubDetailComponent setSequence(int value) { 7644 if (this.sequence == null) 7645 this.sequence = new PositiveIntType(); 7646 this.sequence.setValue(value); 7647 return this; 7648 } 7649 7650 /** 7651 * @return {@link #revenue} (The type of revenue or cost center providing the 7652 * product and/or service.) 7653 */ 7654 public CodeableConcept getRevenue() { 7655 if (this.revenue == null) 7656 if (Configuration.errorOnAutoCreate()) 7657 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 7658 else if (Configuration.doAutoCreate()) 7659 this.revenue = new CodeableConcept(); // cc 7660 return this.revenue; 7661 } 7662 7663 public boolean hasRevenue() { 7664 return this.revenue != null && !this.revenue.isEmpty(); 7665 } 7666 7667 /** 7668 * @param value {@link #revenue} (The type of revenue or cost center providing 7669 * the product and/or service.) 7670 */ 7671 public SubDetailComponent setRevenue(CodeableConcept value) { 7672 this.revenue = value; 7673 return this; 7674 } 7675 7676 /** 7677 * @return {@link #category} (Code to identify the general type of benefits 7678 * under which products and services are provided.) 7679 */ 7680 public CodeableConcept getCategory() { 7681 if (this.category == null) 7682 if (Configuration.errorOnAutoCreate()) 7683 throw new Error("Attempt to auto-create SubDetailComponent.category"); 7684 else if (Configuration.doAutoCreate()) 7685 this.category = new CodeableConcept(); // cc 7686 return this.category; 7687 } 7688 7689 public boolean hasCategory() { 7690 return this.category != null && !this.category.isEmpty(); 7691 } 7692 7693 /** 7694 * @param value {@link #category} (Code to identify the general type of benefits 7695 * under which products and services are provided.) 7696 */ 7697 public SubDetailComponent setCategory(CodeableConcept value) { 7698 this.category = value; 7699 return this; 7700 } 7701 7702 /** 7703 * @return {@link #productOrService} (When the value is a group code then this 7704 * item collects a set of related claim details, otherwise this contains 7705 * the product, service, drug or other billing code for the item.) 7706 */ 7707 public CodeableConcept getProductOrService() { 7708 if (this.productOrService == null) 7709 if (Configuration.errorOnAutoCreate()) 7710 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 7711 else if (Configuration.doAutoCreate()) 7712 this.productOrService = new CodeableConcept(); // cc 7713 return this.productOrService; 7714 } 7715 7716 public boolean hasProductOrService() { 7717 return this.productOrService != null && !this.productOrService.isEmpty(); 7718 } 7719 7720 /** 7721 * @param value {@link #productOrService} (When the value is a group code then 7722 * this item collects a set of related claim details, otherwise 7723 * this contains the product, service, drug or other billing code 7724 * for the item.) 7725 */ 7726 public SubDetailComponent setProductOrService(CodeableConcept value) { 7727 this.productOrService = value; 7728 return this; 7729 } 7730 7731 /** 7732 * @return {@link #modifier} (Item typification or modifiers codes to convey 7733 * additional context for the product or service.) 7734 */ 7735 public List<CodeableConcept> getModifier() { 7736 if (this.modifier == null) 7737 this.modifier = new ArrayList<CodeableConcept>(); 7738 return this.modifier; 7739 } 7740 7741 /** 7742 * @return Returns a reference to <code>this</code> for easy method chaining 7743 */ 7744 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 7745 this.modifier = theModifier; 7746 return this; 7747 } 7748 7749 public boolean hasModifier() { 7750 if (this.modifier == null) 7751 return false; 7752 for (CodeableConcept item : this.modifier) 7753 if (!item.isEmpty()) 7754 return true; 7755 return false; 7756 } 7757 7758 public CodeableConcept addModifier() { // 3 7759 CodeableConcept t = new CodeableConcept(); 7760 if (this.modifier == null) 7761 this.modifier = new ArrayList<CodeableConcept>(); 7762 this.modifier.add(t); 7763 return t; 7764 } 7765 7766 public SubDetailComponent addModifier(CodeableConcept t) { // 3 7767 if (t == null) 7768 return this; 7769 if (this.modifier == null) 7770 this.modifier = new ArrayList<CodeableConcept>(); 7771 this.modifier.add(t); 7772 return this; 7773 } 7774 7775 /** 7776 * @return The first repetition of repeating field {@link #modifier}, creating 7777 * it if it does not already exist 7778 */ 7779 public CodeableConcept getModifierFirstRep() { 7780 if (getModifier().isEmpty()) { 7781 addModifier(); 7782 } 7783 return getModifier().get(0); 7784 } 7785 7786 /** 7787 * @return {@link #programCode} (Identifies the program under which this may be 7788 * recovered.) 7789 */ 7790 public List<CodeableConcept> getProgramCode() { 7791 if (this.programCode == null) 7792 this.programCode = new ArrayList<CodeableConcept>(); 7793 return this.programCode; 7794 } 7795 7796 /** 7797 * @return Returns a reference to <code>this</code> for easy method chaining 7798 */ 7799 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 7800 this.programCode = theProgramCode; 7801 return this; 7802 } 7803 7804 public boolean hasProgramCode() { 7805 if (this.programCode == null) 7806 return false; 7807 for (CodeableConcept item : this.programCode) 7808 if (!item.isEmpty()) 7809 return true; 7810 return false; 7811 } 7812 7813 public CodeableConcept addProgramCode() { // 3 7814 CodeableConcept t = new CodeableConcept(); 7815 if (this.programCode == null) 7816 this.programCode = new ArrayList<CodeableConcept>(); 7817 this.programCode.add(t); 7818 return t; 7819 } 7820 7821 public SubDetailComponent addProgramCode(CodeableConcept t) { // 3 7822 if (t == null) 7823 return this; 7824 if (this.programCode == null) 7825 this.programCode = new ArrayList<CodeableConcept>(); 7826 this.programCode.add(t); 7827 return this; 7828 } 7829 7830 /** 7831 * @return The first repetition of repeating field {@link #programCode}, 7832 * creating it if it does not already exist 7833 */ 7834 public CodeableConcept getProgramCodeFirstRep() { 7835 if (getProgramCode().isEmpty()) { 7836 addProgramCode(); 7837 } 7838 return getProgramCode().get(0); 7839 } 7840 7841 /** 7842 * @return {@link #quantity} (The number of repetitions of a service or 7843 * product.) 7844 */ 7845 public Quantity getQuantity() { 7846 if (this.quantity == null) 7847 if (Configuration.errorOnAutoCreate()) 7848 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 7849 else if (Configuration.doAutoCreate()) 7850 this.quantity = new Quantity(); // cc 7851 return this.quantity; 7852 } 7853 7854 public boolean hasQuantity() { 7855 return this.quantity != null && !this.quantity.isEmpty(); 7856 } 7857 7858 /** 7859 * @param value {@link #quantity} (The number of repetitions of a service or 7860 * product.) 7861 */ 7862 public SubDetailComponent setQuantity(Quantity value) { 7863 this.quantity = value; 7864 return this; 7865 } 7866 7867 /** 7868 * @return {@link #unitPrice} (If the item is not a group then this is the fee 7869 * for the product or service, otherwise this is the total of the fees 7870 * for the details of the group.) 7871 */ 7872 public Money getUnitPrice() { 7873 if (this.unitPrice == null) 7874 if (Configuration.errorOnAutoCreate()) 7875 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 7876 else if (Configuration.doAutoCreate()) 7877 this.unitPrice = new Money(); // cc 7878 return this.unitPrice; 7879 } 7880 7881 public boolean hasUnitPrice() { 7882 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7883 } 7884 7885 /** 7886 * @param value {@link #unitPrice} (If the item is not a group then this is the 7887 * fee for the product or service, otherwise this is the total of 7888 * the fees for the details of the group.) 7889 */ 7890 public SubDetailComponent setUnitPrice(Money value) { 7891 this.unitPrice = value; 7892 return this; 7893 } 7894 7895 /** 7896 * @return {@link #factor} (A real number that represents a multiplier used in 7897 * determining the overall value of services delivered and/or goods 7898 * received. The concept of a Factor allows for a discount or surcharge 7899 * multiplier to be applied to a monetary amount.). This is the 7900 * underlying object with id, value and extensions. The accessor 7901 * "getFactor" gives direct access to the value 7902 */ 7903 public DecimalType getFactorElement() { 7904 if (this.factor == null) 7905 if (Configuration.errorOnAutoCreate()) 7906 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 7907 else if (Configuration.doAutoCreate()) 7908 this.factor = new DecimalType(); // bb 7909 return this.factor; 7910 } 7911 7912 public boolean hasFactorElement() { 7913 return this.factor != null && !this.factor.isEmpty(); 7914 } 7915 7916 public boolean hasFactor() { 7917 return this.factor != null && !this.factor.isEmpty(); 7918 } 7919 7920 /** 7921 * @param value {@link #factor} (A real number that represents a multiplier used 7922 * in determining the overall value of services delivered and/or 7923 * goods received. The concept of a Factor allows for a discount or 7924 * surcharge multiplier to be applied to a monetary amount.). This 7925 * is the underlying object with id, value and extensions. The 7926 * accessor "getFactor" gives direct access to the value 7927 */ 7928 public SubDetailComponent setFactorElement(DecimalType value) { 7929 this.factor = value; 7930 return this; 7931 } 7932 7933 /** 7934 * @return A real number that represents a multiplier used in determining the 7935 * overall value of services delivered and/or goods received. The 7936 * concept of a Factor allows for a discount or surcharge multiplier to 7937 * be applied to a monetary amount. 7938 */ 7939 public BigDecimal getFactor() { 7940 return this.factor == null ? null : this.factor.getValue(); 7941 } 7942 7943 /** 7944 * @param value A real number that represents a multiplier used in determining 7945 * the overall value of services delivered and/or goods received. 7946 * The concept of a Factor allows for a discount or surcharge 7947 * multiplier to be applied to a monetary amount. 7948 */ 7949 public SubDetailComponent setFactor(BigDecimal value) { 7950 if (value == null) 7951 this.factor = null; 7952 else { 7953 if (this.factor == null) 7954 this.factor = new DecimalType(); 7955 this.factor.setValue(value); 7956 } 7957 return this; 7958 } 7959 7960 /** 7961 * @param value A real number that represents a multiplier used in determining 7962 * the overall value of services delivered and/or goods received. 7963 * The concept of a Factor allows for a discount or surcharge 7964 * multiplier to be applied to a monetary amount. 7965 */ 7966 public SubDetailComponent setFactor(long value) { 7967 this.factor = new DecimalType(); 7968 this.factor.setValue(value); 7969 return this; 7970 } 7971 7972 /** 7973 * @param value A real number that represents a multiplier used in determining 7974 * the overall value of services delivered and/or goods received. 7975 * The concept of a Factor allows for a discount or surcharge 7976 * multiplier to be applied to a monetary amount. 7977 */ 7978 public SubDetailComponent setFactor(double value) { 7979 this.factor = new DecimalType(); 7980 this.factor.setValue(value); 7981 return this; 7982 } 7983 7984 /** 7985 * @return {@link #net} (The quantity times the unit price for an additional 7986 * service or product or charge.) 7987 */ 7988 public Money getNet() { 7989 if (this.net == null) 7990 if (Configuration.errorOnAutoCreate()) 7991 throw new Error("Attempt to auto-create SubDetailComponent.net"); 7992 else if (Configuration.doAutoCreate()) 7993 this.net = new Money(); // cc 7994 return this.net; 7995 } 7996 7997 public boolean hasNet() { 7998 return this.net != null && !this.net.isEmpty(); 7999 } 8000 8001 /** 8002 * @param value {@link #net} (The quantity times the unit price for an 8003 * additional service or product or charge.) 8004 */ 8005 public SubDetailComponent setNet(Money value) { 8006 this.net = value; 8007 return this; 8008 } 8009 8010 /** 8011 * @return {@link #udi} (Unique Device Identifiers associated with this line 8012 * item.) 8013 */ 8014 public List<Reference> getUdi() { 8015 if (this.udi == null) 8016 this.udi = new ArrayList<Reference>(); 8017 return this.udi; 8018 } 8019 8020 /** 8021 * @return Returns a reference to <code>this</code> for easy method chaining 8022 */ 8023 public SubDetailComponent setUdi(List<Reference> theUdi) { 8024 this.udi = theUdi; 8025 return this; 8026 } 8027 8028 public boolean hasUdi() { 8029 if (this.udi == null) 8030 return false; 8031 for (Reference item : this.udi) 8032 if (!item.isEmpty()) 8033 return true; 8034 return false; 8035 } 8036 8037 public Reference addUdi() { // 3 8038 Reference t = new Reference(); 8039 if (this.udi == null) 8040 this.udi = new ArrayList<Reference>(); 8041 this.udi.add(t); 8042 return t; 8043 } 8044 8045 public SubDetailComponent addUdi(Reference t) { // 3 8046 if (t == null) 8047 return this; 8048 if (this.udi == null) 8049 this.udi = new ArrayList<Reference>(); 8050 this.udi.add(t); 8051 return this; 8052 } 8053 8054 /** 8055 * @return The first repetition of repeating field {@link #udi}, creating it if 8056 * it does not already exist 8057 */ 8058 public Reference getUdiFirstRep() { 8059 if (getUdi().isEmpty()) { 8060 addUdi(); 8061 } 8062 return getUdi().get(0); 8063 } 8064 8065 /** 8066 * @deprecated Use Reference#setResource(IBaseResource) instead 8067 */ 8068 @Deprecated 8069 public List<Device> getUdiTarget() { 8070 if (this.udiTarget == null) 8071 this.udiTarget = new ArrayList<Device>(); 8072 return this.udiTarget; 8073 } 8074 8075 /** 8076 * @deprecated Use Reference#setResource(IBaseResource) instead 8077 */ 8078 @Deprecated 8079 public Device addUdiTarget() { 8080 Device r = new Device(); 8081 if (this.udiTarget == null) 8082 this.udiTarget = new ArrayList<Device>(); 8083 this.udiTarget.add(r); 8084 return r; 8085 } 8086 8087 protected void listChildren(List<Property> children) { 8088 super.listChildren(children); 8089 children 8090 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 8091 children.add(new Property("revenue", "CodeableConcept", 8092 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 8093 children.add(new Property("category", "CodeableConcept", 8094 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 8095 category)); 8096 children.add(new Property("productOrService", "CodeableConcept", 8097 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 8098 0, 1, productOrService)); 8099 children.add(new Property("modifier", "CodeableConcept", 8100 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 8101 java.lang.Integer.MAX_VALUE, modifier)); 8102 children.add(new Property("programCode", "CodeableConcept", 8103 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 8104 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 8105 1, quantity)); 8106 children.add(new Property("unitPrice", "Money", 8107 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 8108 0, 1, unitPrice)); 8109 children.add(new Property("factor", "decimal", 8110 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 8111 0, 1, factor)); 8112 children.add(new Property("net", "Money", 8113 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 8114 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 8115 0, java.lang.Integer.MAX_VALUE, udi)); 8116 } 8117 8118 @Override 8119 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8120 switch (_hash) { 8121 case 1349547969: 8122 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 8123 1, sequence); 8124 case 1099842588: 8125 /* revenue */ return new Property("revenue", "CodeableConcept", 8126 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 8127 case 50511102: 8128 /* category */ return new Property("category", "CodeableConcept", 8129 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 8130 category); 8131 case 1957227299: 8132 /* productOrService */ return new Property("productOrService", "CodeableConcept", 8133 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 8134 0, 1, productOrService); 8135 case -615513385: 8136 /* modifier */ return new Property("modifier", "CodeableConcept", 8137 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 8138 java.lang.Integer.MAX_VALUE, modifier); 8139 case 1010065041: 8140 /* programCode */ return new Property("programCode", "CodeableConcept", 8141 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 8142 case -1285004149: 8143 /* quantity */ return new Property("quantity", "SimpleQuantity", 8144 "The number of repetitions of a service or product.", 0, 1, quantity); 8145 case -486196699: 8146 /* unitPrice */ return new Property("unitPrice", "Money", 8147 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 8148 0, 1, unitPrice); 8149 case -1282148017: 8150 /* factor */ return new Property("factor", "decimal", 8151 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 8152 0, 1, factor); 8153 case 108957: 8154 /* net */ return new Property("net", "Money", 8155 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 8156 case 115642: 8157 /* udi */ return new Property("udi", "Reference(Device)", 8158 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 8159 default: 8160 return super.getNamedProperty(_hash, _name, _checkValid); 8161 } 8162 8163 } 8164 8165 @Override 8166 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8167 switch (hash) { 8168 case 1349547969: 8169 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 8170 case 1099842588: 8171 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 8172 case 50511102: 8173 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 8174 case 1957227299: 8175 /* productOrService */ return this.productOrService == null ? new Base[0] 8176 : new Base[] { this.productOrService }; // CodeableConcept 8177 case -615513385: 8178 /* modifier */ return this.modifier == null ? new Base[0] 8179 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8180 case 1010065041: 8181 /* programCode */ return this.programCode == null ? new Base[0] 8182 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 8183 case -1285004149: 8184 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 8185 case -486196699: 8186 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 8187 case -1282148017: 8188 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 8189 case 108957: 8190 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 8191 case 115642: 8192 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 8193 default: 8194 return super.getProperty(hash, name, checkValid); 8195 } 8196 8197 } 8198 8199 @Override 8200 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8201 switch (hash) { 8202 case 1349547969: // sequence 8203 this.sequence = castToPositiveInt(value); // PositiveIntType 8204 return value; 8205 case 1099842588: // revenue 8206 this.revenue = castToCodeableConcept(value); // CodeableConcept 8207 return value; 8208 case 50511102: // category 8209 this.category = castToCodeableConcept(value); // CodeableConcept 8210 return value; 8211 case 1957227299: // productOrService 8212 this.productOrService = castToCodeableConcept(value); // CodeableConcept 8213 return value; 8214 case -615513385: // modifier 8215 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 8216 return value; 8217 case 1010065041: // programCode 8218 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 8219 return value; 8220 case -1285004149: // quantity 8221 this.quantity = castToQuantity(value); // Quantity 8222 return value; 8223 case -486196699: // unitPrice 8224 this.unitPrice = castToMoney(value); // Money 8225 return value; 8226 case -1282148017: // factor 8227 this.factor = castToDecimal(value); // DecimalType 8228 return value; 8229 case 108957: // net 8230 this.net = castToMoney(value); // Money 8231 return value; 8232 case 115642: // udi 8233 this.getUdi().add(castToReference(value)); // Reference 8234 return value; 8235 default: 8236 return super.setProperty(hash, name, value); 8237 } 8238 8239 } 8240 8241 @Override 8242 public Base setProperty(String name, Base value) throws FHIRException { 8243 if (name.equals("sequence")) { 8244 this.sequence = castToPositiveInt(value); // PositiveIntType 8245 } else if (name.equals("revenue")) { 8246 this.revenue = castToCodeableConcept(value); // CodeableConcept 8247 } else if (name.equals("category")) { 8248 this.category = castToCodeableConcept(value); // CodeableConcept 8249 } else if (name.equals("productOrService")) { 8250 this.productOrService = castToCodeableConcept(value); // CodeableConcept 8251 } else if (name.equals("modifier")) { 8252 this.getModifier().add(castToCodeableConcept(value)); 8253 } else if (name.equals("programCode")) { 8254 this.getProgramCode().add(castToCodeableConcept(value)); 8255 } else if (name.equals("quantity")) { 8256 this.quantity = castToQuantity(value); // Quantity 8257 } else if (name.equals("unitPrice")) { 8258 this.unitPrice = castToMoney(value); // Money 8259 } else if (name.equals("factor")) { 8260 this.factor = castToDecimal(value); // DecimalType 8261 } else if (name.equals("net")) { 8262 this.net = castToMoney(value); // Money 8263 } else if (name.equals("udi")) { 8264 this.getUdi().add(castToReference(value)); 8265 } else 8266 return super.setProperty(name, value); 8267 return value; 8268 } 8269 8270 @Override 8271 public void removeChild(String name, Base value) throws FHIRException { 8272 if (name.equals("sequence")) { 8273 this.sequence = null; 8274 } else if (name.equals("revenue")) { 8275 this.revenue = null; 8276 } else if (name.equals("category")) { 8277 this.category = null; 8278 } else if (name.equals("productOrService")) { 8279 this.productOrService = null; 8280 } else if (name.equals("modifier")) { 8281 this.getModifier().remove(castToCodeableConcept(value)); 8282 } else if (name.equals("programCode")) { 8283 this.getProgramCode().remove(castToCodeableConcept(value)); 8284 } else if (name.equals("quantity")) { 8285 this.quantity = null; 8286 } else if (name.equals("unitPrice")) { 8287 this.unitPrice = null; 8288 } else if (name.equals("factor")) { 8289 this.factor = null; 8290 } else if (name.equals("net")) { 8291 this.net = null; 8292 } else if (name.equals("udi")) { 8293 this.getUdi().remove(castToReference(value)); 8294 } else 8295 super.removeChild(name, value); 8296 8297 } 8298 8299 @Override 8300 public Base makeProperty(int hash, String name) throws FHIRException { 8301 switch (hash) { 8302 case 1349547969: 8303 return getSequenceElement(); 8304 case 1099842588: 8305 return getRevenue(); 8306 case 50511102: 8307 return getCategory(); 8308 case 1957227299: 8309 return getProductOrService(); 8310 case -615513385: 8311 return addModifier(); 8312 case 1010065041: 8313 return addProgramCode(); 8314 case -1285004149: 8315 return getQuantity(); 8316 case -486196699: 8317 return getUnitPrice(); 8318 case -1282148017: 8319 return getFactorElement(); 8320 case 108957: 8321 return getNet(); 8322 case 115642: 8323 return addUdi(); 8324 default: 8325 return super.makeProperty(hash, name); 8326 } 8327 8328 } 8329 8330 @Override 8331 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8332 switch (hash) { 8333 case 1349547969: 8334 /* sequence */ return new String[] { "positiveInt" }; 8335 case 1099842588: 8336 /* revenue */ return new String[] { "CodeableConcept" }; 8337 case 50511102: 8338 /* category */ return new String[] { "CodeableConcept" }; 8339 case 1957227299: 8340 /* productOrService */ return new String[] { "CodeableConcept" }; 8341 case -615513385: 8342 /* modifier */ return new String[] { "CodeableConcept" }; 8343 case 1010065041: 8344 /* programCode */ return new String[] { "CodeableConcept" }; 8345 case -1285004149: 8346 /* quantity */ return new String[] { "SimpleQuantity" }; 8347 case -486196699: 8348 /* unitPrice */ return new String[] { "Money" }; 8349 case -1282148017: 8350 /* factor */ return new String[] { "decimal" }; 8351 case 108957: 8352 /* net */ return new String[] { "Money" }; 8353 case 115642: 8354 /* udi */ return new String[] { "Reference" }; 8355 default: 8356 return super.getTypesForProperty(hash, name); 8357 } 8358 8359 } 8360 8361 @Override 8362 public Base addChild(String name) throws FHIRException { 8363 if (name.equals("sequence")) { 8364 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 8365 } else if (name.equals("revenue")) { 8366 this.revenue = new CodeableConcept(); 8367 return this.revenue; 8368 } else if (name.equals("category")) { 8369 this.category = new CodeableConcept(); 8370 return this.category; 8371 } else if (name.equals("productOrService")) { 8372 this.productOrService = new CodeableConcept(); 8373 return this.productOrService; 8374 } else if (name.equals("modifier")) { 8375 return addModifier(); 8376 } else if (name.equals("programCode")) { 8377 return addProgramCode(); 8378 } else if (name.equals("quantity")) { 8379 this.quantity = new Quantity(); 8380 return this.quantity; 8381 } else if (name.equals("unitPrice")) { 8382 this.unitPrice = new Money(); 8383 return this.unitPrice; 8384 } else if (name.equals("factor")) { 8385 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 8386 } else if (name.equals("net")) { 8387 this.net = new Money(); 8388 return this.net; 8389 } else if (name.equals("udi")) { 8390 return addUdi(); 8391 } else 8392 return super.addChild(name); 8393 } 8394 8395 public SubDetailComponent copy() { 8396 SubDetailComponent dst = new SubDetailComponent(); 8397 copyValues(dst); 8398 return dst; 8399 } 8400 8401 public void copyValues(SubDetailComponent dst) { 8402 super.copyValues(dst); 8403 dst.sequence = sequence == null ? null : sequence.copy(); 8404 dst.revenue = revenue == null ? null : revenue.copy(); 8405 dst.category = category == null ? null : category.copy(); 8406 dst.productOrService = productOrService == null ? null : productOrService.copy(); 8407 if (modifier != null) { 8408 dst.modifier = new ArrayList<CodeableConcept>(); 8409 for (CodeableConcept i : modifier) 8410 dst.modifier.add(i.copy()); 8411 } 8412 ; 8413 if (programCode != null) { 8414 dst.programCode = new ArrayList<CodeableConcept>(); 8415 for (CodeableConcept i : programCode) 8416 dst.programCode.add(i.copy()); 8417 } 8418 ; 8419 dst.quantity = quantity == null ? null : quantity.copy(); 8420 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 8421 dst.factor = factor == null ? null : factor.copy(); 8422 dst.net = net == null ? null : net.copy(); 8423 if (udi != null) { 8424 dst.udi = new ArrayList<Reference>(); 8425 for (Reference i : udi) 8426 dst.udi.add(i.copy()); 8427 } 8428 ; 8429 } 8430 8431 @Override 8432 public boolean equalsDeep(Base other_) { 8433 if (!super.equalsDeep(other_)) 8434 return false; 8435 if (!(other_ instanceof SubDetailComponent)) 8436 return false; 8437 SubDetailComponent o = (SubDetailComponent) other_; 8438 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) 8439 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 8440 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 8441 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 8442 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true); 8443 } 8444 8445 @Override 8446 public boolean equalsShallow(Base other_) { 8447 if (!super.equalsShallow(other_)) 8448 return false; 8449 if (!(other_ instanceof SubDetailComponent)) 8450 return false; 8451 SubDetailComponent o = (SubDetailComponent) other_; 8452 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 8453 } 8454 8455 public boolean isEmpty() { 8456 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category, productOrService, 8457 modifier, programCode, quantity, unitPrice, factor, net, udi); 8458 } 8459 8460 public String fhirType() { 8461 return "Claim.item.detail.subDetail"; 8462 8463 } 8464 8465 } 8466 8467 /** 8468 * A unique identifier assigned to this claim. 8469 */ 8470 @Child(name = "identifier", type = { 8471 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8472 @Description(shortDefinition = "Business Identifier for claim", formalDefinition = "A unique identifier assigned to this claim.") 8473 protected List<Identifier> identifier; 8474 8475 /** 8476 * The status of the resource instance. 8477 */ 8478 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 8479 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 8480 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 8481 protected Enumeration<ClaimStatus> status; 8482 8483 /** 8484 * The category of claim, e.g. oral, pharmacy, vision, institutional, 8485 * professional. 8486 */ 8487 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 8488 @Description(shortDefinition = "Category or discipline", formalDefinition = "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.") 8489 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-type") 8490 protected CodeableConcept type; 8491 8492 /** 8493 * A finer grained suite of claim type codes which may convey additional 8494 * information such as Inpatient vs Outpatient and/or a specialty service. 8495 */ 8496 @Child(name = "subType", type = { 8497 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 8498 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 8499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-subtype") 8500 protected CodeableConcept subType; 8501 8502 /** 8503 * A code to indicate whether the nature of the request is: to request 8504 * adjudication of products and services previously rendered; or requesting 8505 * authorization and adjudication for provision in the future; or requesting the 8506 * non-binding adjudication of the listed products and services which could be 8507 * provided in the future. 8508 */ 8509 @Child(name = "use", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 8510 @Description(shortDefinition = "claim | preauthorization | predetermination", formalDefinition = "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.") 8511 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-use") 8512 protected Enumeration<Use> use; 8513 8514 /** 8515 * The party to whom the professional services and/or products have been 8516 * supplied or are being considered and for whom actual or forecast 8517 * reimbursement is sought. 8518 */ 8519 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 8520 @Description(shortDefinition = "The recipient of the products and services", formalDefinition = "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.") 8521 protected Reference patient; 8522 8523 /** 8524 * The actual object that is the target of the reference (The party to whom the 8525 * professional services and/or products have been supplied or are being 8526 * considered and for whom actual or forecast reimbursement is sought.) 8527 */ 8528 protected Patient patientTarget; 8529 8530 /** 8531 * The period for which charges are being submitted. 8532 */ 8533 @Child(name = "billablePeriod", type = { 8534 Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 8535 @Description(shortDefinition = "Relevant time frame for the claim", formalDefinition = "The period for which charges are being submitted.") 8536 protected Period billablePeriod; 8537 8538 /** 8539 * The date this resource was created. 8540 */ 8541 @Child(name = "created", type = { DateTimeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 8542 @Description(shortDefinition = "Resource creation date", formalDefinition = "The date this resource was created.") 8543 protected DateTimeType created; 8544 8545 /** 8546 * Individual who created the claim, predetermination or preauthorization. 8547 */ 8548 @Child(name = "enterer", type = { Practitioner.class, 8549 PractitionerRole.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 8550 @Description(shortDefinition = "Author of the claim", formalDefinition = "Individual who created the claim, predetermination or preauthorization.") 8551 protected Reference enterer; 8552 8553 /** 8554 * The actual object that is the target of the reference (Individual who created 8555 * the claim, predetermination or preauthorization.) 8556 */ 8557 protected Resource entererTarget; 8558 8559 /** 8560 * The Insurer who is target of the request. 8561 */ 8562 @Child(name = "insurer", type = { Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 8563 @Description(shortDefinition = "Target", formalDefinition = "The Insurer who is target of the request.") 8564 protected Reference insurer; 8565 8566 /** 8567 * The actual object that is the target of the reference (The Insurer who is 8568 * target of the request.) 8569 */ 8570 protected Organization insurerTarget; 8571 8572 /** 8573 * The provider which is responsible for the claim, predetermination or 8574 * preauthorization. 8575 */ 8576 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 8577 Organization.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 8578 @Description(shortDefinition = "Party responsible for the claim", formalDefinition = "The provider which is responsible for the claim, predetermination or preauthorization.") 8579 protected Reference provider; 8580 8581 /** 8582 * The actual object that is the target of the reference (The provider which is 8583 * responsible for the claim, predetermination or preauthorization.) 8584 */ 8585 protected Resource providerTarget; 8586 8587 /** 8588 * The provider-required urgency of processing the request. Typical values 8589 * include: stat, routine deferred. 8590 */ 8591 @Child(name = "priority", type = { 8592 CodeableConcept.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 8593 @Description(shortDefinition = "Desired processing ugency", formalDefinition = "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.") 8594 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/process-priority") 8595 protected CodeableConcept priority; 8596 8597 /** 8598 * A code to indicate whether and for whom funds are to be reserved for future 8599 * claims. 8600 */ 8601 @Child(name = "fundsReserve", type = { 8602 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 8603 @Description(shortDefinition = "For whom to reserve funds", formalDefinition = "A code to indicate whether and for whom funds are to be reserved for future claims.") 8604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fundsreserve") 8605 protected CodeableConcept fundsReserve; 8606 8607 /** 8608 * Other claims which are related to this claim such as prior submissions or 8609 * claims for related services or for the same event. 8610 */ 8611 @Child(name = "related", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8612 @Description(shortDefinition = "Prior or corollary claims", formalDefinition = "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.") 8613 protected List<RelatedClaimComponent> related; 8614 8615 /** 8616 * Prescription to support the dispensing of pharmacy, device or vision 8617 * products. 8618 */ 8619 @Child(name = "prescription", type = { DeviceRequest.class, MedicationRequest.class, 8620 VisionPrescription.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 8621 @Description(shortDefinition = "Prescription authorizing services and products", formalDefinition = "Prescription to support the dispensing of pharmacy, device or vision products.") 8622 protected Reference prescription; 8623 8624 /** 8625 * The actual object that is the target of the reference (Prescription to 8626 * support the dispensing of pharmacy, device or vision products.) 8627 */ 8628 protected Resource prescriptionTarget; 8629 8630 /** 8631 * Original prescription which has been superseded by this prescription to 8632 * support the dispensing of pharmacy services, medications or products. 8633 */ 8634 @Child(name = "originalPrescription", type = { DeviceRequest.class, MedicationRequest.class, 8635 VisionPrescription.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 8636 @Description(shortDefinition = "Original prescription if superseded by fulfiller", formalDefinition = "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.") 8637 protected Reference originalPrescription; 8638 8639 /** 8640 * The actual object that is the target of the reference (Original prescription 8641 * which has been superseded by this prescription to support the dispensing of 8642 * pharmacy services, medications or products.) 8643 */ 8644 protected Resource originalPrescriptionTarget; 8645 8646 /** 8647 * The party to be reimbursed for cost of the products and services according to 8648 * the terms of the policy. 8649 */ 8650 @Child(name = "payee", type = {}, order = 16, min = 0, max = 1, modifier = false, summary = false) 8651 @Description(shortDefinition = "Recipient of benefits payable", formalDefinition = "The party to be reimbursed for cost of the products and services according to the terms of the policy.") 8652 protected PayeeComponent payee; 8653 8654 /** 8655 * A reference to a referral resource. 8656 */ 8657 @Child(name = "referral", type = { 8658 ServiceRequest.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 8659 @Description(shortDefinition = "Treatment referral", formalDefinition = "A reference to a referral resource.") 8660 protected Reference referral; 8661 8662 /** 8663 * The actual object that is the target of the reference (A reference to a 8664 * referral resource.) 8665 */ 8666 protected ServiceRequest referralTarget; 8667 8668 /** 8669 * Facility where the services were provided. 8670 */ 8671 @Child(name = "facility", type = { Location.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 8672 @Description(shortDefinition = "Servicing facility", formalDefinition = "Facility where the services were provided.") 8673 protected Reference facility; 8674 8675 /** 8676 * The actual object that is the target of the reference (Facility where the 8677 * services were provided.) 8678 */ 8679 protected Location facilityTarget; 8680 8681 /** 8682 * The members of the team who provided the products and services. 8683 */ 8684 @Child(name = "careTeam", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8685 @Description(shortDefinition = "Members of the care team", formalDefinition = "The members of the team who provided the products and services.") 8686 protected List<CareTeamComponent> careTeam; 8687 8688 /** 8689 * Additional information codes regarding exceptions, special considerations, 8690 * the condition, situation, prior or concurrent issues. 8691 */ 8692 @Child(name = "supportingInfo", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8693 @Description(shortDefinition = "Supporting information", formalDefinition = "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.") 8694 protected List<SupportingInformationComponent> supportingInfo; 8695 8696 /** 8697 * Information about diagnoses relevant to the claim items. 8698 */ 8699 @Child(name = "diagnosis", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8700 @Description(shortDefinition = "Pertinent diagnosis information", formalDefinition = "Information about diagnoses relevant to the claim items.") 8701 protected List<DiagnosisComponent> diagnosis; 8702 8703 /** 8704 * Procedures performed on the patient relevant to the billing items with the 8705 * claim. 8706 */ 8707 @Child(name = "procedure", type = {}, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8708 @Description(shortDefinition = "Clinical procedures performed", formalDefinition = "Procedures performed on the patient relevant to the billing items with the claim.") 8709 protected List<ProcedureComponent> procedure; 8710 8711 /** 8712 * Financial instruments for reimbursement for the health care products and 8713 * services specified on the claim. 8714 */ 8715 @Child(name = "insurance", type = {}, order = 23, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8716 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services specified on the claim.") 8717 protected List<InsuranceComponent> insurance; 8718 8719 /** 8720 * Details of an accident which resulted in injuries which required the products 8721 * and services listed in the claim. 8722 */ 8723 @Child(name = "accident", type = {}, order = 24, min = 0, max = 1, modifier = false, summary = false) 8724 @Description(shortDefinition = "Details of the event", formalDefinition = "Details of an accident which resulted in injuries which required the products and services listed in the claim.") 8725 protected AccidentComponent accident; 8726 8727 /** 8728 * A claim line. Either a simple product or service or a 'group' of details 8729 * which can each be a simple items or groups of sub-details. 8730 */ 8731 @Child(name = "item", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8732 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.") 8733 protected List<ItemComponent> item; 8734 8735 /** 8736 * The total value of the all the items in the claim. 8737 */ 8738 @Child(name = "total", type = { Money.class }, order = 26, min = 0, max = 1, modifier = false, summary = false) 8739 @Description(shortDefinition = "Total claim cost", formalDefinition = "The total value of the all the items in the claim.") 8740 protected Money total; 8741 8742 private static final long serialVersionUID = -80376108L; 8743 8744 /** 8745 * Constructor 8746 */ 8747 public Claim() { 8748 super(); 8749 } 8750 8751 /** 8752 * Constructor 8753 */ 8754 public Claim(Enumeration<ClaimStatus> status, CodeableConcept type, Enumeration<Use> use, Reference patient, 8755 DateTimeType created, Reference provider, CodeableConcept priority) { 8756 super(); 8757 this.status = status; 8758 this.type = type; 8759 this.use = use; 8760 this.patient = patient; 8761 this.created = created; 8762 this.provider = provider; 8763 this.priority = priority; 8764 } 8765 8766 /** 8767 * @return {@link #identifier} (A unique identifier assigned to this claim.) 8768 */ 8769 public List<Identifier> getIdentifier() { 8770 if (this.identifier == null) 8771 this.identifier = new ArrayList<Identifier>(); 8772 return this.identifier; 8773 } 8774 8775 /** 8776 * @return Returns a reference to <code>this</code> for easy method chaining 8777 */ 8778 public Claim setIdentifier(List<Identifier> theIdentifier) { 8779 this.identifier = theIdentifier; 8780 return this; 8781 } 8782 8783 public boolean hasIdentifier() { 8784 if (this.identifier == null) 8785 return false; 8786 for (Identifier item : this.identifier) 8787 if (!item.isEmpty()) 8788 return true; 8789 return false; 8790 } 8791 8792 public Identifier addIdentifier() { // 3 8793 Identifier t = new Identifier(); 8794 if (this.identifier == null) 8795 this.identifier = new ArrayList<Identifier>(); 8796 this.identifier.add(t); 8797 return t; 8798 } 8799 8800 public Claim addIdentifier(Identifier t) { // 3 8801 if (t == null) 8802 return this; 8803 if (this.identifier == null) 8804 this.identifier = new ArrayList<Identifier>(); 8805 this.identifier.add(t); 8806 return this; 8807 } 8808 8809 /** 8810 * @return The first repetition of repeating field {@link #identifier}, creating 8811 * it if it does not already exist 8812 */ 8813 public Identifier getIdentifierFirstRep() { 8814 if (getIdentifier().isEmpty()) { 8815 addIdentifier(); 8816 } 8817 return getIdentifier().get(0); 8818 } 8819 8820 /** 8821 * @return {@link #status} (The status of the resource instance.). This is the 8822 * underlying object with id, value and extensions. The accessor 8823 * "getStatus" gives direct access to the value 8824 */ 8825 public Enumeration<ClaimStatus> getStatusElement() { 8826 if (this.status == null) 8827 if (Configuration.errorOnAutoCreate()) 8828 throw new Error("Attempt to auto-create Claim.status"); 8829 else if (Configuration.doAutoCreate()) 8830 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); // bb 8831 return this.status; 8832 } 8833 8834 public boolean hasStatusElement() { 8835 return this.status != null && !this.status.isEmpty(); 8836 } 8837 8838 public boolean hasStatus() { 8839 return this.status != null && !this.status.isEmpty(); 8840 } 8841 8842 /** 8843 * @param value {@link #status} (The status of the resource instance.). This is 8844 * the underlying object with id, value and extensions. The 8845 * accessor "getStatus" gives direct access to the value 8846 */ 8847 public Claim setStatusElement(Enumeration<ClaimStatus> value) { 8848 this.status = value; 8849 return this; 8850 } 8851 8852 /** 8853 * @return The status of the resource instance. 8854 */ 8855 public ClaimStatus getStatus() { 8856 return this.status == null ? null : this.status.getValue(); 8857 } 8858 8859 /** 8860 * @param value The status of the resource instance. 8861 */ 8862 public Claim setStatus(ClaimStatus value) { 8863 if (this.status == null) 8864 this.status = new Enumeration<ClaimStatus>(new ClaimStatusEnumFactory()); 8865 this.status.setValue(value); 8866 return this; 8867 } 8868 8869 /** 8870 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, 8871 * institutional, professional.) 8872 */ 8873 public CodeableConcept getType() { 8874 if (this.type == null) 8875 if (Configuration.errorOnAutoCreate()) 8876 throw new Error("Attempt to auto-create Claim.type"); 8877 else if (Configuration.doAutoCreate()) 8878 this.type = new CodeableConcept(); // cc 8879 return this.type; 8880 } 8881 8882 public boolean hasType() { 8883 return this.type != null && !this.type.isEmpty(); 8884 } 8885 8886 /** 8887 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, 8888 * vision, institutional, professional.) 8889 */ 8890 public Claim setType(CodeableConcept value) { 8891 this.type = value; 8892 return this; 8893 } 8894 8895 /** 8896 * @return {@link #subType} (A finer grained suite of claim type codes which may 8897 * convey additional information such as Inpatient vs Outpatient and/or 8898 * a specialty service.) 8899 */ 8900 public CodeableConcept getSubType() { 8901 if (this.subType == null) 8902 if (Configuration.errorOnAutoCreate()) 8903 throw new Error("Attempt to auto-create Claim.subType"); 8904 else if (Configuration.doAutoCreate()) 8905 this.subType = new CodeableConcept(); // cc 8906 return this.subType; 8907 } 8908 8909 public boolean hasSubType() { 8910 return this.subType != null && !this.subType.isEmpty(); 8911 } 8912 8913 /** 8914 * @param value {@link #subType} (A finer grained suite of claim type codes 8915 * which may convey additional information such as Inpatient vs 8916 * Outpatient and/or a specialty service.) 8917 */ 8918 public Claim setSubType(CodeableConcept value) { 8919 this.subType = value; 8920 return this; 8921 } 8922 8923 /** 8924 * @return {@link #use} (A code to indicate whether the nature of the request 8925 * is: to request adjudication of products and services previously 8926 * rendered; or requesting authorization and adjudication for provision 8927 * in the future; or requesting the non-binding adjudication of the 8928 * listed products and services which could be provided in the future.). 8929 * This is the underlying object with id, value and extensions. The 8930 * accessor "getUse" gives direct access to the value 8931 */ 8932 public Enumeration<Use> getUseElement() { 8933 if (this.use == null) 8934 if (Configuration.errorOnAutoCreate()) 8935 throw new Error("Attempt to auto-create Claim.use"); 8936 else if (Configuration.doAutoCreate()) 8937 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 8938 return this.use; 8939 } 8940 8941 public boolean hasUseElement() { 8942 return this.use != null && !this.use.isEmpty(); 8943 } 8944 8945 public boolean hasUse() { 8946 return this.use != null && !this.use.isEmpty(); 8947 } 8948 8949 /** 8950 * @param value {@link #use} (A code to indicate whether the nature of the 8951 * request is: to request adjudication of products and services 8952 * previously rendered; or requesting authorization and 8953 * adjudication for provision in the future; or requesting the 8954 * non-binding adjudication of the listed products and services 8955 * which could be provided in the future.). This is the underlying 8956 * object with id, value and extensions. The accessor "getUse" 8957 * gives direct access to the value 8958 */ 8959 public Claim setUseElement(Enumeration<Use> value) { 8960 this.use = value; 8961 return this; 8962 } 8963 8964 /** 8965 * @return A code to indicate whether the nature of the request is: to request 8966 * adjudication of products and services previously rendered; or 8967 * requesting authorization and adjudication for provision in the 8968 * future; or requesting the non-binding adjudication of the listed 8969 * products and services which could be provided in the future. 8970 */ 8971 public Use getUse() { 8972 return this.use == null ? null : this.use.getValue(); 8973 } 8974 8975 /** 8976 * @param value A code to indicate whether the nature of the request is: to 8977 * request adjudication of products and services previously 8978 * rendered; or requesting authorization and adjudication for 8979 * provision in the future; or requesting the non-binding 8980 * adjudication of the listed products and services which could be 8981 * provided in the future. 8982 */ 8983 public Claim setUse(Use value) { 8984 if (this.use == null) 8985 this.use = new Enumeration<Use>(new UseEnumFactory()); 8986 this.use.setValue(value); 8987 return this; 8988 } 8989 8990 /** 8991 * @return {@link #patient} (The party to whom the professional services and/or 8992 * products have been supplied or are being considered and for whom 8993 * actual or forecast reimbursement is sought.) 8994 */ 8995 public Reference getPatient() { 8996 if (this.patient == null) 8997 if (Configuration.errorOnAutoCreate()) 8998 throw new Error("Attempt to auto-create Claim.patient"); 8999 else if (Configuration.doAutoCreate()) 9000 this.patient = new Reference(); // cc 9001 return this.patient; 9002 } 9003 9004 public boolean hasPatient() { 9005 return this.patient != null && !this.patient.isEmpty(); 9006 } 9007 9008 /** 9009 * @param value {@link #patient} (The party to whom the professional services 9010 * and/or products have been supplied or are being considered and 9011 * for whom actual or forecast reimbursement is sought.) 9012 */ 9013 public Claim setPatient(Reference value) { 9014 this.patient = value; 9015 return this; 9016 } 9017 9018 /** 9019 * @return {@link #patient} The actual object that is the target of the 9020 * reference. The reference library doesn't populate this, but you can 9021 * use it to hold the resource if you resolve it. (The party to whom the 9022 * professional services and/or products have been supplied or are being 9023 * considered and for whom actual or forecast reimbursement is sought.) 9024 */ 9025 public Patient getPatientTarget() { 9026 if (this.patientTarget == null) 9027 if (Configuration.errorOnAutoCreate()) 9028 throw new Error("Attempt to auto-create Claim.patient"); 9029 else if (Configuration.doAutoCreate()) 9030 this.patientTarget = new Patient(); // aa 9031 return this.patientTarget; 9032 } 9033 9034 /** 9035 * @param value {@link #patient} The actual object that is the target of the 9036 * reference. The reference library doesn't use these, but you can 9037 * use it to hold the resource if you resolve it. (The party to 9038 * whom the professional services and/or products have been 9039 * supplied or are being considered and for whom actual or forecast 9040 * reimbursement is sought.) 9041 */ 9042 public Claim setPatientTarget(Patient value) { 9043 this.patientTarget = value; 9044 return this; 9045 } 9046 9047 /** 9048 * @return {@link #billablePeriod} (The period for which charges are being 9049 * submitted.) 9050 */ 9051 public Period getBillablePeriod() { 9052 if (this.billablePeriod == null) 9053 if (Configuration.errorOnAutoCreate()) 9054 throw new Error("Attempt to auto-create Claim.billablePeriod"); 9055 else if (Configuration.doAutoCreate()) 9056 this.billablePeriod = new Period(); // cc 9057 return this.billablePeriod; 9058 } 9059 9060 public boolean hasBillablePeriod() { 9061 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 9062 } 9063 9064 /** 9065 * @param value {@link #billablePeriod} (The period for which charges are being 9066 * submitted.) 9067 */ 9068 public Claim setBillablePeriod(Period value) { 9069 this.billablePeriod = value; 9070 return this; 9071 } 9072 9073 /** 9074 * @return {@link #created} (The date this resource was created.). This is the 9075 * underlying object with id, value and extensions. The accessor 9076 * "getCreated" gives direct access to the value 9077 */ 9078 public DateTimeType getCreatedElement() { 9079 if (this.created == null) 9080 if (Configuration.errorOnAutoCreate()) 9081 throw new Error("Attempt to auto-create Claim.created"); 9082 else if (Configuration.doAutoCreate()) 9083 this.created = new DateTimeType(); // bb 9084 return this.created; 9085 } 9086 9087 public boolean hasCreatedElement() { 9088 return this.created != null && !this.created.isEmpty(); 9089 } 9090 9091 public boolean hasCreated() { 9092 return this.created != null && !this.created.isEmpty(); 9093 } 9094 9095 /** 9096 * @param value {@link #created} (The date this resource was created.). This is 9097 * the underlying object with id, value and extensions. The 9098 * accessor "getCreated" gives direct access to the value 9099 */ 9100 public Claim setCreatedElement(DateTimeType value) { 9101 this.created = value; 9102 return this; 9103 } 9104 9105 /** 9106 * @return The date this resource was created. 9107 */ 9108 public Date getCreated() { 9109 return this.created == null ? null : this.created.getValue(); 9110 } 9111 9112 /** 9113 * @param value The date this resource was created. 9114 */ 9115 public Claim setCreated(Date value) { 9116 if (this.created == null) 9117 this.created = new DateTimeType(); 9118 this.created.setValue(value); 9119 return this; 9120 } 9121 9122 /** 9123 * @return {@link #enterer} (Individual who created the claim, predetermination 9124 * or preauthorization.) 9125 */ 9126 public Reference getEnterer() { 9127 if (this.enterer == null) 9128 if (Configuration.errorOnAutoCreate()) 9129 throw new Error("Attempt to auto-create Claim.enterer"); 9130 else if (Configuration.doAutoCreate()) 9131 this.enterer = new Reference(); // cc 9132 return this.enterer; 9133 } 9134 9135 public boolean hasEnterer() { 9136 return this.enterer != null && !this.enterer.isEmpty(); 9137 } 9138 9139 /** 9140 * @param value {@link #enterer} (Individual who created the claim, 9141 * predetermination or preauthorization.) 9142 */ 9143 public Claim setEnterer(Reference value) { 9144 this.enterer = value; 9145 return this; 9146 } 9147 9148 /** 9149 * @return {@link #enterer} The actual object that is the target of the 9150 * reference. The reference library doesn't populate this, but you can 9151 * use it to hold the resource if you resolve it. (Individual who 9152 * created the claim, predetermination or preauthorization.) 9153 */ 9154 public Resource getEntererTarget() { 9155 return this.entererTarget; 9156 } 9157 9158 /** 9159 * @param value {@link #enterer} The actual object that is the target of the 9160 * reference. The reference library doesn't use these, but you can 9161 * use it to hold the resource if you resolve it. (Individual who 9162 * created the claim, predetermination or preauthorization.) 9163 */ 9164 public Claim setEntererTarget(Resource value) { 9165 this.entererTarget = value; 9166 return this; 9167 } 9168 9169 /** 9170 * @return {@link #insurer} (The Insurer who is target of the request.) 9171 */ 9172 public Reference getInsurer() { 9173 if (this.insurer == null) 9174 if (Configuration.errorOnAutoCreate()) 9175 throw new Error("Attempt to auto-create Claim.insurer"); 9176 else if (Configuration.doAutoCreate()) 9177 this.insurer = new Reference(); // cc 9178 return this.insurer; 9179 } 9180 9181 public boolean hasInsurer() { 9182 return this.insurer != null && !this.insurer.isEmpty(); 9183 } 9184 9185 /** 9186 * @param value {@link #insurer} (The Insurer who is target of the request.) 9187 */ 9188 public Claim setInsurer(Reference value) { 9189 this.insurer = value; 9190 return this; 9191 } 9192 9193 /** 9194 * @return {@link #insurer} The actual object that is the target of the 9195 * reference. The reference library doesn't populate this, but you can 9196 * use it to hold the resource if you resolve it. (The Insurer who is 9197 * target of the request.) 9198 */ 9199 public Organization getInsurerTarget() { 9200 if (this.insurerTarget == null) 9201 if (Configuration.errorOnAutoCreate()) 9202 throw new Error("Attempt to auto-create Claim.insurer"); 9203 else if (Configuration.doAutoCreate()) 9204 this.insurerTarget = new Organization(); // aa 9205 return this.insurerTarget; 9206 } 9207 9208 /** 9209 * @param value {@link #insurer} The actual object that is the target of the 9210 * reference. The reference library doesn't use these, but you can 9211 * use it to hold the resource if you resolve it. (The Insurer who 9212 * is target of the request.) 9213 */ 9214 public Claim setInsurerTarget(Organization value) { 9215 this.insurerTarget = value; 9216 return this; 9217 } 9218 9219 /** 9220 * @return {@link #provider} (The provider which is responsible for the claim, 9221 * predetermination or preauthorization.) 9222 */ 9223 public Reference getProvider() { 9224 if (this.provider == null) 9225 if (Configuration.errorOnAutoCreate()) 9226 throw new Error("Attempt to auto-create Claim.provider"); 9227 else if (Configuration.doAutoCreate()) 9228 this.provider = new Reference(); // cc 9229 return this.provider; 9230 } 9231 9232 public boolean hasProvider() { 9233 return this.provider != null && !this.provider.isEmpty(); 9234 } 9235 9236 /** 9237 * @param value {@link #provider} (The provider which is responsible for the 9238 * claim, predetermination or preauthorization.) 9239 */ 9240 public Claim setProvider(Reference value) { 9241 this.provider = value; 9242 return this; 9243 } 9244 9245 /** 9246 * @return {@link #provider} The actual object that is the target of the 9247 * reference. The reference library doesn't populate this, but you can 9248 * use it to hold the resource if you resolve it. (The provider which is 9249 * responsible for the claim, predetermination or preauthorization.) 9250 */ 9251 public Resource getProviderTarget() { 9252 return this.providerTarget; 9253 } 9254 9255 /** 9256 * @param value {@link #provider} The actual object that is the target of the 9257 * reference. The reference library doesn't use these, but you can 9258 * use it to hold the resource if you resolve it. (The provider 9259 * which is responsible for the claim, predetermination or 9260 * preauthorization.) 9261 */ 9262 public Claim setProviderTarget(Resource value) { 9263 this.providerTarget = value; 9264 return this; 9265 } 9266 9267 /** 9268 * @return {@link #priority} (The provider-required urgency of processing the 9269 * request. Typical values include: stat, routine deferred.) 9270 */ 9271 public CodeableConcept getPriority() { 9272 if (this.priority == null) 9273 if (Configuration.errorOnAutoCreate()) 9274 throw new Error("Attempt to auto-create Claim.priority"); 9275 else if (Configuration.doAutoCreate()) 9276 this.priority = new CodeableConcept(); // cc 9277 return this.priority; 9278 } 9279 9280 public boolean hasPriority() { 9281 return this.priority != null && !this.priority.isEmpty(); 9282 } 9283 9284 /** 9285 * @param value {@link #priority} (The provider-required urgency of processing 9286 * the request. Typical values include: stat, routine deferred.) 9287 */ 9288 public Claim setPriority(CodeableConcept value) { 9289 this.priority = value; 9290 return this; 9291 } 9292 9293 /** 9294 * @return {@link #fundsReserve} (A code to indicate whether and for whom funds 9295 * are to be reserved for future claims.) 9296 */ 9297 public CodeableConcept getFundsReserve() { 9298 if (this.fundsReserve == null) 9299 if (Configuration.errorOnAutoCreate()) 9300 throw new Error("Attempt to auto-create Claim.fundsReserve"); 9301 else if (Configuration.doAutoCreate()) 9302 this.fundsReserve = new CodeableConcept(); // cc 9303 return this.fundsReserve; 9304 } 9305 9306 public boolean hasFundsReserve() { 9307 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 9308 } 9309 9310 /** 9311 * @param value {@link #fundsReserve} (A code to indicate whether and for whom 9312 * funds are to be reserved for future claims.) 9313 */ 9314 public Claim setFundsReserve(CodeableConcept value) { 9315 this.fundsReserve = value; 9316 return this; 9317 } 9318 9319 /** 9320 * @return {@link #related} (Other claims which are related to this claim such 9321 * as prior submissions or claims for related services or for the same 9322 * event.) 9323 */ 9324 public List<RelatedClaimComponent> getRelated() { 9325 if (this.related == null) 9326 this.related = new ArrayList<RelatedClaimComponent>(); 9327 return this.related; 9328 } 9329 9330 /** 9331 * @return Returns a reference to <code>this</code> for easy method chaining 9332 */ 9333 public Claim setRelated(List<RelatedClaimComponent> theRelated) { 9334 this.related = theRelated; 9335 return this; 9336 } 9337 9338 public boolean hasRelated() { 9339 if (this.related == null) 9340 return false; 9341 for (RelatedClaimComponent item : this.related) 9342 if (!item.isEmpty()) 9343 return true; 9344 return false; 9345 } 9346 9347 public RelatedClaimComponent addRelated() { // 3 9348 RelatedClaimComponent t = new RelatedClaimComponent(); 9349 if (this.related == null) 9350 this.related = new ArrayList<RelatedClaimComponent>(); 9351 this.related.add(t); 9352 return t; 9353 } 9354 9355 public Claim addRelated(RelatedClaimComponent t) { // 3 9356 if (t == null) 9357 return this; 9358 if (this.related == null) 9359 this.related = new ArrayList<RelatedClaimComponent>(); 9360 this.related.add(t); 9361 return this; 9362 } 9363 9364 /** 9365 * @return The first repetition of repeating field {@link #related}, creating it 9366 * if it does not already exist 9367 */ 9368 public RelatedClaimComponent getRelatedFirstRep() { 9369 if (getRelated().isEmpty()) { 9370 addRelated(); 9371 } 9372 return getRelated().get(0); 9373 } 9374 9375 /** 9376 * @return {@link #prescription} (Prescription to support the dispensing of 9377 * pharmacy, device or vision products.) 9378 */ 9379 public Reference getPrescription() { 9380 if (this.prescription == null) 9381 if (Configuration.errorOnAutoCreate()) 9382 throw new Error("Attempt to auto-create Claim.prescription"); 9383 else if (Configuration.doAutoCreate()) 9384 this.prescription = new Reference(); // cc 9385 return this.prescription; 9386 } 9387 9388 public boolean hasPrescription() { 9389 return this.prescription != null && !this.prescription.isEmpty(); 9390 } 9391 9392 /** 9393 * @param value {@link #prescription} (Prescription to support the dispensing of 9394 * pharmacy, device or vision products.) 9395 */ 9396 public Claim setPrescription(Reference value) { 9397 this.prescription = value; 9398 return this; 9399 } 9400 9401 /** 9402 * @return {@link #prescription} The actual object that is the target of the 9403 * reference. The reference library doesn't populate this, but you can 9404 * use it to hold the resource if you resolve it. (Prescription to 9405 * support the dispensing of pharmacy, device or vision products.) 9406 */ 9407 public Resource getPrescriptionTarget() { 9408 return this.prescriptionTarget; 9409 } 9410 9411 /** 9412 * @param value {@link #prescription} The actual object that is the target of 9413 * the reference. The reference library doesn't use these, but you 9414 * can use it to hold the resource if you resolve it. (Prescription 9415 * to support the dispensing of pharmacy, device or vision 9416 * products.) 9417 */ 9418 public Claim setPrescriptionTarget(Resource value) { 9419 this.prescriptionTarget = value; 9420 return this; 9421 } 9422 9423 /** 9424 * @return {@link #originalPrescription} (Original prescription which has been 9425 * superseded by this prescription to support the dispensing of pharmacy 9426 * services, medications or products.) 9427 */ 9428 public Reference getOriginalPrescription() { 9429 if (this.originalPrescription == null) 9430 if (Configuration.errorOnAutoCreate()) 9431 throw new Error("Attempt to auto-create Claim.originalPrescription"); 9432 else if (Configuration.doAutoCreate()) 9433 this.originalPrescription = new Reference(); // cc 9434 return this.originalPrescription; 9435 } 9436 9437 public boolean hasOriginalPrescription() { 9438 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 9439 } 9440 9441 /** 9442 * @param value {@link #originalPrescription} (Original prescription which has 9443 * been superseded by this prescription to support the dispensing 9444 * of pharmacy services, medications or products.) 9445 */ 9446 public Claim setOriginalPrescription(Reference value) { 9447 this.originalPrescription = value; 9448 return this; 9449 } 9450 9451 /** 9452 * @return {@link #originalPrescription} The actual object that is the target of 9453 * the reference. The reference library doesn't populate this, but you 9454 * can use it to hold the resource if you resolve it. (Original 9455 * prescription which has been superseded by this prescription to 9456 * support the dispensing of pharmacy services, medications or 9457 * products.) 9458 */ 9459 public Resource getOriginalPrescriptionTarget() { 9460 return this.originalPrescriptionTarget; 9461 } 9462 9463 /** 9464 * @param value {@link #originalPrescription} The actual object that is the 9465 * target of the reference. The reference library doesn't use 9466 * these, but you can use it to hold the resource if you resolve 9467 * it. (Original prescription which has been superseded by this 9468 * prescription to support the dispensing of pharmacy services, 9469 * medications or products.) 9470 */ 9471 public Claim setOriginalPrescriptionTarget(Resource value) { 9472 this.originalPrescriptionTarget = value; 9473 return this; 9474 } 9475 9476 /** 9477 * @return {@link #payee} (The party to be reimbursed for cost of the products 9478 * and services according to the terms of the policy.) 9479 */ 9480 public PayeeComponent getPayee() { 9481 if (this.payee == null) 9482 if (Configuration.errorOnAutoCreate()) 9483 throw new Error("Attempt to auto-create Claim.payee"); 9484 else if (Configuration.doAutoCreate()) 9485 this.payee = new PayeeComponent(); // cc 9486 return this.payee; 9487 } 9488 9489 public boolean hasPayee() { 9490 return this.payee != null && !this.payee.isEmpty(); 9491 } 9492 9493 /** 9494 * @param value {@link #payee} (The party to be reimbursed for cost of the 9495 * products and services according to the terms of the policy.) 9496 */ 9497 public Claim setPayee(PayeeComponent value) { 9498 this.payee = value; 9499 return this; 9500 } 9501 9502 /** 9503 * @return {@link #referral} (A reference to a referral resource.) 9504 */ 9505 public Reference getReferral() { 9506 if (this.referral == null) 9507 if (Configuration.errorOnAutoCreate()) 9508 throw new Error("Attempt to auto-create Claim.referral"); 9509 else if (Configuration.doAutoCreate()) 9510 this.referral = new Reference(); // cc 9511 return this.referral; 9512 } 9513 9514 public boolean hasReferral() { 9515 return this.referral != null && !this.referral.isEmpty(); 9516 } 9517 9518 /** 9519 * @param value {@link #referral} (A reference to a referral resource.) 9520 */ 9521 public Claim setReferral(Reference value) { 9522 this.referral = value; 9523 return this; 9524 } 9525 9526 /** 9527 * @return {@link #referral} The actual object that is the target of the 9528 * reference. The reference library doesn't populate this, but you can 9529 * use it to hold the resource if you resolve it. (A reference to a 9530 * referral resource.) 9531 */ 9532 public ServiceRequest getReferralTarget() { 9533 if (this.referralTarget == null) 9534 if (Configuration.errorOnAutoCreate()) 9535 throw new Error("Attempt to auto-create Claim.referral"); 9536 else if (Configuration.doAutoCreate()) 9537 this.referralTarget = new ServiceRequest(); // aa 9538 return this.referralTarget; 9539 } 9540 9541 /** 9542 * @param value {@link #referral} The actual object that is the target of the 9543 * reference. The reference library doesn't use these, but you can 9544 * use it to hold the resource if you resolve it. (A reference to a 9545 * referral resource.) 9546 */ 9547 public Claim setReferralTarget(ServiceRequest value) { 9548 this.referralTarget = value; 9549 return this; 9550 } 9551 9552 /** 9553 * @return {@link #facility} (Facility where the services were provided.) 9554 */ 9555 public Reference getFacility() { 9556 if (this.facility == null) 9557 if (Configuration.errorOnAutoCreate()) 9558 throw new Error("Attempt to auto-create Claim.facility"); 9559 else if (Configuration.doAutoCreate()) 9560 this.facility = new Reference(); // cc 9561 return this.facility; 9562 } 9563 9564 public boolean hasFacility() { 9565 return this.facility != null && !this.facility.isEmpty(); 9566 } 9567 9568 /** 9569 * @param value {@link #facility} (Facility where the services were provided.) 9570 */ 9571 public Claim setFacility(Reference value) { 9572 this.facility = value; 9573 return this; 9574 } 9575 9576 /** 9577 * @return {@link #facility} The actual object that is the target of the 9578 * reference. The reference library doesn't populate this, but you can 9579 * use it to hold the resource if you resolve it. (Facility where the 9580 * services were provided.) 9581 */ 9582 public Location getFacilityTarget() { 9583 if (this.facilityTarget == null) 9584 if (Configuration.errorOnAutoCreate()) 9585 throw new Error("Attempt to auto-create Claim.facility"); 9586 else if (Configuration.doAutoCreate()) 9587 this.facilityTarget = new Location(); // aa 9588 return this.facilityTarget; 9589 } 9590 9591 /** 9592 * @param value {@link #facility} The actual object that is the target of the 9593 * reference. The reference library doesn't use these, but you can 9594 * use it to hold the resource if you resolve it. (Facility where 9595 * the services were provided.) 9596 */ 9597 public Claim setFacilityTarget(Location value) { 9598 this.facilityTarget = value; 9599 return this; 9600 } 9601 9602 /** 9603 * @return {@link #careTeam} (The members of the team who provided the products 9604 * and services.) 9605 */ 9606 public List<CareTeamComponent> getCareTeam() { 9607 if (this.careTeam == null) 9608 this.careTeam = new ArrayList<CareTeamComponent>(); 9609 return this.careTeam; 9610 } 9611 9612 /** 9613 * @return Returns a reference to <code>this</code> for easy method chaining 9614 */ 9615 public Claim setCareTeam(List<CareTeamComponent> theCareTeam) { 9616 this.careTeam = theCareTeam; 9617 return this; 9618 } 9619 9620 public boolean hasCareTeam() { 9621 if (this.careTeam == null) 9622 return false; 9623 for (CareTeamComponent item : this.careTeam) 9624 if (!item.isEmpty()) 9625 return true; 9626 return false; 9627 } 9628 9629 public CareTeamComponent addCareTeam() { // 3 9630 CareTeamComponent t = new CareTeamComponent(); 9631 if (this.careTeam == null) 9632 this.careTeam = new ArrayList<CareTeamComponent>(); 9633 this.careTeam.add(t); 9634 return t; 9635 } 9636 9637 public Claim addCareTeam(CareTeamComponent t) { // 3 9638 if (t == null) 9639 return this; 9640 if (this.careTeam == null) 9641 this.careTeam = new ArrayList<CareTeamComponent>(); 9642 this.careTeam.add(t); 9643 return this; 9644 } 9645 9646 /** 9647 * @return The first repetition of repeating field {@link #careTeam}, creating 9648 * it if it does not already exist 9649 */ 9650 public CareTeamComponent getCareTeamFirstRep() { 9651 if (getCareTeam().isEmpty()) { 9652 addCareTeam(); 9653 } 9654 return getCareTeam().get(0); 9655 } 9656 9657 /** 9658 * @return {@link #supportingInfo} (Additional information codes regarding 9659 * exceptions, special considerations, the condition, situation, prior 9660 * or concurrent issues.) 9661 */ 9662 public List<SupportingInformationComponent> getSupportingInfo() { 9663 if (this.supportingInfo == null) 9664 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9665 return this.supportingInfo; 9666 } 9667 9668 /** 9669 * @return Returns a reference to <code>this</code> for easy method chaining 9670 */ 9671 public Claim setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 9672 this.supportingInfo = theSupportingInfo; 9673 return this; 9674 } 9675 9676 public boolean hasSupportingInfo() { 9677 if (this.supportingInfo == null) 9678 return false; 9679 for (SupportingInformationComponent item : this.supportingInfo) 9680 if (!item.isEmpty()) 9681 return true; 9682 return false; 9683 } 9684 9685 public SupportingInformationComponent addSupportingInfo() { // 3 9686 SupportingInformationComponent t = new SupportingInformationComponent(); 9687 if (this.supportingInfo == null) 9688 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9689 this.supportingInfo.add(t); 9690 return t; 9691 } 9692 9693 public Claim addSupportingInfo(SupportingInformationComponent t) { // 3 9694 if (t == null) 9695 return this; 9696 if (this.supportingInfo == null) 9697 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9698 this.supportingInfo.add(t); 9699 return this; 9700 } 9701 9702 /** 9703 * @return The first repetition of repeating field {@link #supportingInfo}, 9704 * creating it if it does not already exist 9705 */ 9706 public SupportingInformationComponent getSupportingInfoFirstRep() { 9707 if (getSupportingInfo().isEmpty()) { 9708 addSupportingInfo(); 9709 } 9710 return getSupportingInfo().get(0); 9711 } 9712 9713 /** 9714 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim 9715 * items.) 9716 */ 9717 public List<DiagnosisComponent> getDiagnosis() { 9718 if (this.diagnosis == null) 9719 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9720 return this.diagnosis; 9721 } 9722 9723 /** 9724 * @return Returns a reference to <code>this</code> for easy method chaining 9725 */ 9726 public Claim setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 9727 this.diagnosis = theDiagnosis; 9728 return this; 9729 } 9730 9731 public boolean hasDiagnosis() { 9732 if (this.diagnosis == null) 9733 return false; 9734 for (DiagnosisComponent item : this.diagnosis) 9735 if (!item.isEmpty()) 9736 return true; 9737 return false; 9738 } 9739 9740 public DiagnosisComponent addDiagnosis() { // 3 9741 DiagnosisComponent t = new DiagnosisComponent(); 9742 if (this.diagnosis == null) 9743 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9744 this.diagnosis.add(t); 9745 return t; 9746 } 9747 9748 public Claim addDiagnosis(DiagnosisComponent t) { // 3 9749 if (t == null) 9750 return this; 9751 if (this.diagnosis == null) 9752 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9753 this.diagnosis.add(t); 9754 return this; 9755 } 9756 9757 /** 9758 * @return The first repetition of repeating field {@link #diagnosis}, creating 9759 * it if it does not already exist 9760 */ 9761 public DiagnosisComponent getDiagnosisFirstRep() { 9762 if (getDiagnosis().isEmpty()) { 9763 addDiagnosis(); 9764 } 9765 return getDiagnosis().get(0); 9766 } 9767 9768 /** 9769 * @return {@link #procedure} (Procedures performed on the patient relevant to 9770 * the billing items with the claim.) 9771 */ 9772 public List<ProcedureComponent> getProcedure() { 9773 if (this.procedure == null) 9774 this.procedure = new ArrayList<ProcedureComponent>(); 9775 return this.procedure; 9776 } 9777 9778 /** 9779 * @return Returns a reference to <code>this</code> for easy method chaining 9780 */ 9781 public Claim setProcedure(List<ProcedureComponent> theProcedure) { 9782 this.procedure = theProcedure; 9783 return this; 9784 } 9785 9786 public boolean hasProcedure() { 9787 if (this.procedure == null) 9788 return false; 9789 for (ProcedureComponent item : this.procedure) 9790 if (!item.isEmpty()) 9791 return true; 9792 return false; 9793 } 9794 9795 public ProcedureComponent addProcedure() { // 3 9796 ProcedureComponent t = new ProcedureComponent(); 9797 if (this.procedure == null) 9798 this.procedure = new ArrayList<ProcedureComponent>(); 9799 this.procedure.add(t); 9800 return t; 9801 } 9802 9803 public Claim addProcedure(ProcedureComponent t) { // 3 9804 if (t == null) 9805 return this; 9806 if (this.procedure == null) 9807 this.procedure = new ArrayList<ProcedureComponent>(); 9808 this.procedure.add(t); 9809 return this; 9810 } 9811 9812 /** 9813 * @return The first repetition of repeating field {@link #procedure}, creating 9814 * it if it does not already exist 9815 */ 9816 public ProcedureComponent getProcedureFirstRep() { 9817 if (getProcedure().isEmpty()) { 9818 addProcedure(); 9819 } 9820 return getProcedure().get(0); 9821 } 9822 9823 /** 9824 * @return {@link #insurance} (Financial instruments for reimbursement for the 9825 * health care products and services specified on the claim.) 9826 */ 9827 public List<InsuranceComponent> getInsurance() { 9828 if (this.insurance == null) 9829 this.insurance = new ArrayList<InsuranceComponent>(); 9830 return this.insurance; 9831 } 9832 9833 /** 9834 * @return Returns a reference to <code>this</code> for easy method chaining 9835 */ 9836 public Claim setInsurance(List<InsuranceComponent> theInsurance) { 9837 this.insurance = theInsurance; 9838 return this; 9839 } 9840 9841 public boolean hasInsurance() { 9842 if (this.insurance == null) 9843 return false; 9844 for (InsuranceComponent item : this.insurance) 9845 if (!item.isEmpty()) 9846 return true; 9847 return false; 9848 } 9849 9850 public InsuranceComponent addInsurance() { // 3 9851 InsuranceComponent t = new InsuranceComponent(); 9852 if (this.insurance == null) 9853 this.insurance = new ArrayList<InsuranceComponent>(); 9854 this.insurance.add(t); 9855 return t; 9856 } 9857 9858 public Claim addInsurance(InsuranceComponent t) { // 3 9859 if (t == null) 9860 return this; 9861 if (this.insurance == null) 9862 this.insurance = new ArrayList<InsuranceComponent>(); 9863 this.insurance.add(t); 9864 return this; 9865 } 9866 9867 /** 9868 * @return The first repetition of repeating field {@link #insurance}, creating 9869 * it if it does not already exist 9870 */ 9871 public InsuranceComponent getInsuranceFirstRep() { 9872 if (getInsurance().isEmpty()) { 9873 addInsurance(); 9874 } 9875 return getInsurance().get(0); 9876 } 9877 9878 /** 9879 * @return {@link #accident} (Details of an accident which resulted in injuries 9880 * which required the products and services listed in the claim.) 9881 */ 9882 public AccidentComponent getAccident() { 9883 if (this.accident == null) 9884 if (Configuration.errorOnAutoCreate()) 9885 throw new Error("Attempt to auto-create Claim.accident"); 9886 else if (Configuration.doAutoCreate()) 9887 this.accident = new AccidentComponent(); // cc 9888 return this.accident; 9889 } 9890 9891 public boolean hasAccident() { 9892 return this.accident != null && !this.accident.isEmpty(); 9893 } 9894 9895 /** 9896 * @param value {@link #accident} (Details of an accident which resulted in 9897 * injuries which required the products and services listed in the 9898 * claim.) 9899 */ 9900 public Claim setAccident(AccidentComponent value) { 9901 this.accident = value; 9902 return this; 9903 } 9904 9905 /** 9906 * @return {@link #item} (A claim line. Either a simple product or service or a 9907 * 'group' of details which can each be a simple items or groups of 9908 * sub-details.) 9909 */ 9910 public List<ItemComponent> getItem() { 9911 if (this.item == null) 9912 this.item = new ArrayList<ItemComponent>(); 9913 return this.item; 9914 } 9915 9916 /** 9917 * @return Returns a reference to <code>this</code> for easy method chaining 9918 */ 9919 public Claim setItem(List<ItemComponent> theItem) { 9920 this.item = theItem; 9921 return this; 9922 } 9923 9924 public boolean hasItem() { 9925 if (this.item == null) 9926 return false; 9927 for (ItemComponent item : this.item) 9928 if (!item.isEmpty()) 9929 return true; 9930 return false; 9931 } 9932 9933 public ItemComponent addItem() { // 3 9934 ItemComponent t = new ItemComponent(); 9935 if (this.item == null) 9936 this.item = new ArrayList<ItemComponent>(); 9937 this.item.add(t); 9938 return t; 9939 } 9940 9941 public Claim addItem(ItemComponent t) { // 3 9942 if (t == null) 9943 return this; 9944 if (this.item == null) 9945 this.item = new ArrayList<ItemComponent>(); 9946 this.item.add(t); 9947 return this; 9948 } 9949 9950 /** 9951 * @return The first repetition of repeating field {@link #item}, creating it if 9952 * it does not already exist 9953 */ 9954 public ItemComponent getItemFirstRep() { 9955 if (getItem().isEmpty()) { 9956 addItem(); 9957 } 9958 return getItem().get(0); 9959 } 9960 9961 /** 9962 * @return {@link #total} (The total value of the all the items in the claim.) 9963 */ 9964 public Money getTotal() { 9965 if (this.total == null) 9966 if (Configuration.errorOnAutoCreate()) 9967 throw new Error("Attempt to auto-create Claim.total"); 9968 else if (Configuration.doAutoCreate()) 9969 this.total = new Money(); // cc 9970 return this.total; 9971 } 9972 9973 public boolean hasTotal() { 9974 return this.total != null && !this.total.isEmpty(); 9975 } 9976 9977 /** 9978 * @param value {@link #total} (The total value of the all the items in the 9979 * claim.) 9980 */ 9981 public Claim setTotal(Money value) { 9982 this.total = value; 9983 return this; 9984 } 9985 9986 protected void listChildren(List<Property> children) { 9987 super.listChildren(children); 9988 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, 9989 java.lang.Integer.MAX_VALUE, identifier)); 9990 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 9991 children.add(new Property("type", "CodeableConcept", 9992 "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 9993 children.add(new Property("subType", "CodeableConcept", 9994 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9995 0, 1, subType)); 9996 children.add(new Property("use", "code", 9997 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 9998 0, 1, use)); 9999 children.add(new Property("patient", "Reference(Patient)", 10000 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 10001 0, 1, patient)); 10002 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, 10003 billablePeriod)); 10004 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 10005 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole)", 10006 "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 10007 children.add( 10008 new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 10009 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 10010 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 10011 children.add(new Property("priority", "CodeableConcept", 10012 "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.", 0, 10013 1, priority)); 10014 children.add(new Property("fundsReserve", "CodeableConcept", 10015 "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve)); 10016 children.add(new Property("related", "", 10017 "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 10018 0, java.lang.Integer.MAX_VALUE, related)); 10019 children.add(new Property("prescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10020 "Prescription to support the dispensing of pharmacy, device or vision products.", 0, 1, prescription)); 10021 children.add(new Property("originalPrescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10022 "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 10023 0, 1, originalPrescription)); 10024 children.add(new Property("payee", "", 10025 "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, 10026 payee)); 10027 children.add( 10028 new Property("referral", "Reference(ServiceRequest)", "A reference to a referral resource.", 0, 1, referral)); 10029 children.add( 10030 new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 10031 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, 10032 java.lang.Integer.MAX_VALUE, careTeam)); 10033 children.add(new Property("supportingInfo", "", 10034 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 10035 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 10036 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, 10037 java.lang.Integer.MAX_VALUE, diagnosis)); 10038 children.add(new Property("procedure", "", 10039 "Procedures performed on the patient relevant to the billing items with the claim.", 0, 10040 java.lang.Integer.MAX_VALUE, procedure)); 10041 children.add(new Property("insurance", "", 10042 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, 10043 java.lang.Integer.MAX_VALUE, insurance)); 10044 children.add(new Property("accident", "", 10045 "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 10046 0, 1, accident)); 10047 children.add(new Property("item", "", 10048 "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 10049 0, java.lang.Integer.MAX_VALUE, item)); 10050 children.add(new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, total)); 10051 } 10052 10053 @Override 10054 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10055 switch (_hash) { 10056 case -1618432855: 10057 /* identifier */ return new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, 10058 java.lang.Integer.MAX_VALUE, identifier); 10059 case -892481550: 10060 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 10061 case 3575610: 10062 /* type */ return new Property("type", "CodeableConcept", 10063 "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 10064 case -1868521062: 10065 /* subType */ return new Property("subType", "CodeableConcept", 10066 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 10067 0, 1, subType); 10068 case 116103: 10069 /* use */ return new Property("use", "code", 10070 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 10071 0, 1, use); 10072 case -791418107: 10073 /* patient */ return new Property("patient", "Reference(Patient)", 10074 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 10075 0, 1, patient); 10076 case -332066046: 10077 /* billablePeriod */ return new Property("billablePeriod", "Period", 10078 "The period for which charges are being submitted.", 0, 1, billablePeriod); 10079 case 1028554472: 10080 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 10081 case -1591951995: 10082 /* enterer */ return new Property("enterer", "Reference(Practitioner|PractitionerRole)", 10083 "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 10084 case 1957615864: 10085 /* insurer */ return new Property("insurer", "Reference(Organization)", 10086 "The Insurer who is target of the request.", 0, 1, insurer); 10087 case -987494927: 10088 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 10089 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 10090 case -1165461084: 10091 /* priority */ return new Property("priority", "CodeableConcept", 10092 "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.", 0, 10093 1, priority); 10094 case 1314609806: 10095 /* fundsReserve */ return new Property("fundsReserve", "CodeableConcept", 10096 "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve); 10097 case 1090493483: 10098 /* related */ return new Property("related", "", 10099 "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 10100 0, java.lang.Integer.MAX_VALUE, related); 10101 case 460301338: 10102 /* prescription */ return new Property("prescription", 10103 "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10104 "Prescription to support the dispensing of pharmacy, device or vision products.", 0, 1, prescription); 10105 case -1814015861: 10106 /* originalPrescription */ return new Property("originalPrescription", 10107 "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", 10108 "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 10109 0, 1, originalPrescription); 10110 case 106443592: 10111 /* payee */ return new Property("payee", "", 10112 "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 10113 1, payee); 10114 case -722568291: 10115 /* referral */ return new Property("referral", "Reference(ServiceRequest)", "A reference to a referral resource.", 10116 0, 1, referral); 10117 case 501116579: 10118 /* facility */ return new Property("facility", "Reference(Location)", 10119 "Facility where the services were provided.", 0, 1, facility); 10120 case -7323378: 10121 /* careTeam */ return new Property("careTeam", "", 10122 "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 10123 case 1922406657: 10124 /* supportingInfo */ return new Property("supportingInfo", "", 10125 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 10126 0, java.lang.Integer.MAX_VALUE, supportingInfo); 10127 case 1196993265: 10128 /* diagnosis */ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 10129 0, java.lang.Integer.MAX_VALUE, diagnosis); 10130 case -1095204141: 10131 /* procedure */ return new Property("procedure", "", 10132 "Procedures performed on the patient relevant to the billing items with the claim.", 0, 10133 java.lang.Integer.MAX_VALUE, procedure); 10134 case 73049818: 10135 /* insurance */ return new Property("insurance", "", 10136 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 10137 0, java.lang.Integer.MAX_VALUE, insurance); 10138 case -2143202801: 10139 /* accident */ return new Property("accident", "", 10140 "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 10141 0, 1, accident); 10142 case 3242771: 10143 /* item */ return new Property("item", "", 10144 "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 10145 0, java.lang.Integer.MAX_VALUE, item); 10146 case 110549828: 10147 /* total */ return new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, 10148 total); 10149 default: 10150 return super.getNamedProperty(_hash, _name, _checkValid); 10151 } 10152 10153 } 10154 10155 @Override 10156 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10157 switch (hash) { 10158 case -1618432855: 10159 /* identifier */ return this.identifier == null ? new Base[0] 10160 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 10161 case -892481550: 10162 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ClaimStatus> 10163 case 3575610: 10164 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 10165 case -1868521062: 10166 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 10167 case 116103: 10168 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<Use> 10169 case -791418107: 10170 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 10171 case -332066046: 10172 /* billablePeriod */ return this.billablePeriod == null ? new Base[0] : new Base[] { this.billablePeriod }; // Period 10173 case 1028554472: 10174 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 10175 case -1591951995: 10176 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 10177 case 1957615864: 10178 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 10179 case -987494927: 10180 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 10181 case -1165461084: 10182 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 10183 case 1314609806: 10184 /* fundsReserve */ return this.fundsReserve == null ? new Base[0] : new Base[] { this.fundsReserve }; // CodeableConcept 10185 case 1090493483: 10186 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 10187 case 460301338: 10188 /* prescription */ return this.prescription == null ? new Base[0] : new Base[] { this.prescription }; // Reference 10189 case -1814015861: 10190 /* originalPrescription */ return this.originalPrescription == null ? new Base[0] 10191 : new Base[] { this.originalPrescription }; // Reference 10192 case 106443592: 10193 /* payee */ return this.payee == null ? new Base[0] : new Base[] { this.payee }; // PayeeComponent 10194 case -722568291: 10195 /* referral */ return this.referral == null ? new Base[0] : new Base[] { this.referral }; // Reference 10196 case 501116579: 10197 /* facility */ return this.facility == null ? new Base[0] : new Base[] { this.facility }; // Reference 10198 case -7323378: 10199 /* careTeam */ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 10200 case 1922406657: 10201 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 10202 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 10203 case 1196993265: 10204 /* diagnosis */ return this.diagnosis == null ? new Base[0] 10205 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 10206 case -1095204141: 10207 /* procedure */ return this.procedure == null ? new Base[0] 10208 : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 10209 case 73049818: 10210 /* insurance */ return this.insurance == null ? new Base[0] 10211 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 10212 case -2143202801: 10213 /* accident */ return this.accident == null ? new Base[0] : new Base[] { this.accident }; // AccidentComponent 10214 case 3242771: 10215 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 10216 case 110549828: 10217 /* total */ return this.total == null ? new Base[0] : new Base[] { this.total }; // Money 10218 default: 10219 return super.getProperty(hash, name, checkValid); 10220 } 10221 10222 } 10223 10224 @Override 10225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10226 switch (hash) { 10227 case -1618432855: // identifier 10228 this.getIdentifier().add(castToIdentifier(value)); // Identifier 10229 return value; 10230 case -892481550: // status 10231 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 10232 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 10233 return value; 10234 case 3575610: // type 10235 this.type = castToCodeableConcept(value); // CodeableConcept 10236 return value; 10237 case -1868521062: // subType 10238 this.subType = castToCodeableConcept(value); // CodeableConcept 10239 return value; 10240 case 116103: // use 10241 value = new UseEnumFactory().fromType(castToCode(value)); 10242 this.use = (Enumeration) value; // Enumeration<Use> 10243 return value; 10244 case -791418107: // patient 10245 this.patient = castToReference(value); // Reference 10246 return value; 10247 case -332066046: // billablePeriod 10248 this.billablePeriod = castToPeriod(value); // Period 10249 return value; 10250 case 1028554472: // created 10251 this.created = castToDateTime(value); // DateTimeType 10252 return value; 10253 case -1591951995: // enterer 10254 this.enterer = castToReference(value); // Reference 10255 return value; 10256 case 1957615864: // insurer 10257 this.insurer = castToReference(value); // Reference 10258 return value; 10259 case -987494927: // provider 10260 this.provider = castToReference(value); // Reference 10261 return value; 10262 case -1165461084: // priority 10263 this.priority = castToCodeableConcept(value); // CodeableConcept 10264 return value; 10265 case 1314609806: // fundsReserve 10266 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 10267 return value; 10268 case 1090493483: // related 10269 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 10270 return value; 10271 case 460301338: // prescription 10272 this.prescription = castToReference(value); // Reference 10273 return value; 10274 case -1814015861: // originalPrescription 10275 this.originalPrescription = castToReference(value); // Reference 10276 return value; 10277 case 106443592: // payee 10278 this.payee = (PayeeComponent) value; // PayeeComponent 10279 return value; 10280 case -722568291: // referral 10281 this.referral = castToReference(value); // Reference 10282 return value; 10283 case 501116579: // facility 10284 this.facility = castToReference(value); // Reference 10285 return value; 10286 case -7323378: // careTeam 10287 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 10288 return value; 10289 case 1922406657: // supportingInfo 10290 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 10291 return value; 10292 case 1196993265: // diagnosis 10293 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 10294 return value; 10295 case -1095204141: // procedure 10296 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 10297 return value; 10298 case 73049818: // insurance 10299 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 10300 return value; 10301 case -2143202801: // accident 10302 this.accident = (AccidentComponent) value; // AccidentComponent 10303 return value; 10304 case 3242771: // item 10305 this.getItem().add((ItemComponent) value); // ItemComponent 10306 return value; 10307 case 110549828: // total 10308 this.total = castToMoney(value); // Money 10309 return value; 10310 default: 10311 return super.setProperty(hash, name, value); 10312 } 10313 10314 } 10315 10316 @Override 10317 public Base setProperty(String name, Base value) throws FHIRException { 10318 if (name.equals("identifier")) { 10319 this.getIdentifier().add(castToIdentifier(value)); 10320 } else if (name.equals("status")) { 10321 value = new ClaimStatusEnumFactory().fromType(castToCode(value)); 10322 this.status = (Enumeration) value; // Enumeration<ClaimStatus> 10323 } else if (name.equals("type")) { 10324 this.type = castToCodeableConcept(value); // CodeableConcept 10325 } else if (name.equals("subType")) { 10326 this.subType = castToCodeableConcept(value); // CodeableConcept 10327 } else if (name.equals("use")) { 10328 value = new UseEnumFactory().fromType(castToCode(value)); 10329 this.use = (Enumeration) value; // Enumeration<Use> 10330 } else if (name.equals("patient")) { 10331 this.patient = castToReference(value); // Reference 10332 } else if (name.equals("billablePeriod")) { 10333 this.billablePeriod = castToPeriod(value); // Period 10334 } else if (name.equals("created")) { 10335 this.created = castToDateTime(value); // DateTimeType 10336 } else if (name.equals("enterer")) { 10337 this.enterer = castToReference(value); // Reference 10338 } else if (name.equals("insurer")) { 10339 this.insurer = castToReference(value); // Reference 10340 } else if (name.equals("provider")) { 10341 this.provider = castToReference(value); // Reference 10342 } else if (name.equals("priority")) { 10343 this.priority = castToCodeableConcept(value); // CodeableConcept 10344 } else if (name.equals("fundsReserve")) { 10345 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 10346 } else if (name.equals("related")) { 10347 this.getRelated().add((RelatedClaimComponent) value); 10348 } else if (name.equals("prescription")) { 10349 this.prescription = castToReference(value); // Reference 10350 } else if (name.equals("originalPrescription")) { 10351 this.originalPrescription = castToReference(value); // Reference 10352 } else if (name.equals("payee")) { 10353 this.payee = (PayeeComponent) value; // PayeeComponent 10354 } else if (name.equals("referral")) { 10355 this.referral = castToReference(value); // Reference 10356 } else if (name.equals("facility")) { 10357 this.facility = castToReference(value); // Reference 10358 } else if (name.equals("careTeam")) { 10359 this.getCareTeam().add((CareTeamComponent) value); 10360 } else if (name.equals("supportingInfo")) { 10361 this.getSupportingInfo().add((SupportingInformationComponent) value); 10362 } else if (name.equals("diagnosis")) { 10363 this.getDiagnosis().add((DiagnosisComponent) value); 10364 } else if (name.equals("procedure")) { 10365 this.getProcedure().add((ProcedureComponent) value); 10366 } else if (name.equals("insurance")) { 10367 this.getInsurance().add((InsuranceComponent) value); 10368 } else if (name.equals("accident")) { 10369 this.accident = (AccidentComponent) value; // AccidentComponent 10370 } else if (name.equals("item")) { 10371 this.getItem().add((ItemComponent) value); 10372 } else if (name.equals("total")) { 10373 this.total = castToMoney(value); // Money 10374 } else 10375 return super.setProperty(name, value); 10376 return value; 10377 } 10378 10379 @Override 10380 public void removeChild(String name, Base value) throws FHIRException { 10381 if (name.equals("identifier")) { 10382 this.getIdentifier().remove(castToIdentifier(value)); 10383 } else if (name.equals("status")) { 10384 this.status = null; 10385 } else if (name.equals("type")) { 10386 this.type = null; 10387 } else if (name.equals("subType")) { 10388 this.subType = null; 10389 } else if (name.equals("use")) { 10390 this.use = null; 10391 } else if (name.equals("patient")) { 10392 this.patient = null; 10393 } else if (name.equals("billablePeriod")) { 10394 this.billablePeriod = null; 10395 } else if (name.equals("created")) { 10396 this.created = null; 10397 } else if (name.equals("enterer")) { 10398 this.enterer = null; 10399 } else if (name.equals("insurer")) { 10400 this.insurer = null; 10401 } else if (name.equals("provider")) { 10402 this.provider = null; 10403 } else if (name.equals("priority")) { 10404 this.priority = null; 10405 } else if (name.equals("fundsReserve")) { 10406 this.fundsReserve = null; 10407 } else if (name.equals("related")) { 10408 this.getRelated().remove((RelatedClaimComponent) value); 10409 } else if (name.equals("prescription")) { 10410 this.prescription = null; 10411 } else if (name.equals("originalPrescription")) { 10412 this.originalPrescription = null; 10413 } else if (name.equals("payee")) { 10414 this.payee = (PayeeComponent) value; // PayeeComponent 10415 } else if (name.equals("referral")) { 10416 this.referral = null; 10417 } else if (name.equals("facility")) { 10418 this.facility = null; 10419 } else if (name.equals("careTeam")) { 10420 this.getCareTeam().remove((CareTeamComponent) value); 10421 } else if (name.equals("supportingInfo")) { 10422 this.getSupportingInfo().remove((SupportingInformationComponent) value); 10423 } else if (name.equals("diagnosis")) { 10424 this.getDiagnosis().remove((DiagnosisComponent) value); 10425 } else if (name.equals("procedure")) { 10426 this.getProcedure().remove((ProcedureComponent) value); 10427 } else if (name.equals("insurance")) { 10428 this.getInsurance().remove((InsuranceComponent) value); 10429 } else if (name.equals("accident")) { 10430 this.accident = (AccidentComponent) value; // AccidentComponent 10431 } else if (name.equals("item")) { 10432 this.getItem().remove((ItemComponent) value); 10433 } else if (name.equals("total")) { 10434 this.total = null; 10435 } else 10436 super.removeChild(name, value); 10437 10438 } 10439 10440 @Override 10441 public Base makeProperty(int hash, String name) throws FHIRException { 10442 switch (hash) { 10443 case -1618432855: 10444 return addIdentifier(); 10445 case -892481550: 10446 return getStatusElement(); 10447 case 3575610: 10448 return getType(); 10449 case -1868521062: 10450 return getSubType(); 10451 case 116103: 10452 return getUseElement(); 10453 case -791418107: 10454 return getPatient(); 10455 case -332066046: 10456 return getBillablePeriod(); 10457 case 1028554472: 10458 return getCreatedElement(); 10459 case -1591951995: 10460 return getEnterer(); 10461 case 1957615864: 10462 return getInsurer(); 10463 case -987494927: 10464 return getProvider(); 10465 case -1165461084: 10466 return getPriority(); 10467 case 1314609806: 10468 return getFundsReserve(); 10469 case 1090493483: 10470 return addRelated(); 10471 case 460301338: 10472 return getPrescription(); 10473 case -1814015861: 10474 return getOriginalPrescription(); 10475 case 106443592: 10476 return getPayee(); 10477 case -722568291: 10478 return getReferral(); 10479 case 501116579: 10480 return getFacility(); 10481 case -7323378: 10482 return addCareTeam(); 10483 case 1922406657: 10484 return addSupportingInfo(); 10485 case 1196993265: 10486 return addDiagnosis(); 10487 case -1095204141: 10488 return addProcedure(); 10489 case 73049818: 10490 return addInsurance(); 10491 case -2143202801: 10492 return getAccident(); 10493 case 3242771: 10494 return addItem(); 10495 case 110549828: 10496 return getTotal(); 10497 default: 10498 return super.makeProperty(hash, name); 10499 } 10500 10501 } 10502 10503 @Override 10504 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10505 switch (hash) { 10506 case -1618432855: 10507 /* identifier */ return new String[] { "Identifier" }; 10508 case -892481550: 10509 /* status */ return new String[] { "code" }; 10510 case 3575610: 10511 /* type */ return new String[] { "CodeableConcept" }; 10512 case -1868521062: 10513 /* subType */ return new String[] { "CodeableConcept" }; 10514 case 116103: 10515 /* use */ return new String[] { "code" }; 10516 case -791418107: 10517 /* patient */ return new String[] { "Reference" }; 10518 case -332066046: 10519 /* billablePeriod */ return new String[] { "Period" }; 10520 case 1028554472: 10521 /* created */ return new String[] { "dateTime" }; 10522 case -1591951995: 10523 /* enterer */ return new String[] { "Reference" }; 10524 case 1957615864: 10525 /* insurer */ return new String[] { "Reference" }; 10526 case -987494927: 10527 /* provider */ return new String[] { "Reference" }; 10528 case -1165461084: 10529 /* priority */ return new String[] { "CodeableConcept" }; 10530 case 1314609806: 10531 /* fundsReserve */ return new String[] { "CodeableConcept" }; 10532 case 1090493483: 10533 /* related */ return new String[] {}; 10534 case 460301338: 10535 /* prescription */ return new String[] { "Reference" }; 10536 case -1814015861: 10537 /* originalPrescription */ return new String[] { "Reference" }; 10538 case 106443592: 10539 /* payee */ return new String[] {}; 10540 case -722568291: 10541 /* referral */ return new String[] { "Reference" }; 10542 case 501116579: 10543 /* facility */ return new String[] { "Reference" }; 10544 case -7323378: 10545 /* careTeam */ return new String[] {}; 10546 case 1922406657: 10547 /* supportingInfo */ return new String[] {}; 10548 case 1196993265: 10549 /* diagnosis */ return new String[] {}; 10550 case -1095204141: 10551 /* procedure */ return new String[] {}; 10552 case 73049818: 10553 /* insurance */ return new String[] {}; 10554 case -2143202801: 10555 /* accident */ return new String[] {}; 10556 case 3242771: 10557 /* item */ return new String[] {}; 10558 case 110549828: 10559 /* total */ return new String[] { "Money" }; 10560 default: 10561 return super.getTypesForProperty(hash, name); 10562 } 10563 10564 } 10565 10566 @Override 10567 public Base addChild(String name) throws FHIRException { 10568 if (name.equals("identifier")) { 10569 return addIdentifier(); 10570 } else if (name.equals("status")) { 10571 throw new FHIRException("Cannot call addChild on a singleton property Claim.status"); 10572 } else if (name.equals("type")) { 10573 this.type = new CodeableConcept(); 10574 return this.type; 10575 } else if (name.equals("subType")) { 10576 this.subType = new CodeableConcept(); 10577 return this.subType; 10578 } else if (name.equals("use")) { 10579 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 10580 } else if (name.equals("patient")) { 10581 this.patient = new Reference(); 10582 return this.patient; 10583 } else if (name.equals("billablePeriod")) { 10584 this.billablePeriod = new Period(); 10585 return this.billablePeriod; 10586 } else if (name.equals("created")) { 10587 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 10588 } else if (name.equals("enterer")) { 10589 this.enterer = new Reference(); 10590 return this.enterer; 10591 } else if (name.equals("insurer")) { 10592 this.insurer = new Reference(); 10593 return this.insurer; 10594 } else if (name.equals("provider")) { 10595 this.provider = new Reference(); 10596 return this.provider; 10597 } else if (name.equals("priority")) { 10598 this.priority = new CodeableConcept(); 10599 return this.priority; 10600 } else if (name.equals("fundsReserve")) { 10601 this.fundsReserve = new CodeableConcept(); 10602 return this.fundsReserve; 10603 } else if (name.equals("related")) { 10604 return addRelated(); 10605 } else if (name.equals("prescription")) { 10606 this.prescription = new Reference(); 10607 return this.prescription; 10608 } else if (name.equals("originalPrescription")) { 10609 this.originalPrescription = new Reference(); 10610 return this.originalPrescription; 10611 } else if (name.equals("payee")) { 10612 this.payee = new PayeeComponent(); 10613 return this.payee; 10614 } else if (name.equals("referral")) { 10615 this.referral = new Reference(); 10616 return this.referral; 10617 } else if (name.equals("facility")) { 10618 this.facility = new Reference(); 10619 return this.facility; 10620 } else if (name.equals("careTeam")) { 10621 return addCareTeam(); 10622 } else if (name.equals("supportingInfo")) { 10623 return addSupportingInfo(); 10624 } else if (name.equals("diagnosis")) { 10625 return addDiagnosis(); 10626 } else if (name.equals("procedure")) { 10627 return addProcedure(); 10628 } else if (name.equals("insurance")) { 10629 return addInsurance(); 10630 } else if (name.equals("accident")) { 10631 this.accident = new AccidentComponent(); 10632 return this.accident; 10633 } else if (name.equals("item")) { 10634 return addItem(); 10635 } else if (name.equals("total")) { 10636 this.total = new Money(); 10637 return this.total; 10638 } else 10639 return super.addChild(name); 10640 } 10641 10642 public String fhirType() { 10643 return "Claim"; 10644 10645 } 10646 10647 public Claim copy() { 10648 Claim dst = new Claim(); 10649 copyValues(dst); 10650 return dst; 10651 } 10652 10653 public void copyValues(Claim dst) { 10654 super.copyValues(dst); 10655 if (identifier != null) { 10656 dst.identifier = new ArrayList<Identifier>(); 10657 for (Identifier i : identifier) 10658 dst.identifier.add(i.copy()); 10659 } 10660 ; 10661 dst.status = status == null ? null : status.copy(); 10662 dst.type = type == null ? null : type.copy(); 10663 dst.subType = subType == null ? null : subType.copy(); 10664 dst.use = use == null ? null : use.copy(); 10665 dst.patient = patient == null ? null : patient.copy(); 10666 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 10667 dst.created = created == null ? null : created.copy(); 10668 dst.enterer = enterer == null ? null : enterer.copy(); 10669 dst.insurer = insurer == null ? null : insurer.copy(); 10670 dst.provider = provider == null ? null : provider.copy(); 10671 dst.priority = priority == null ? null : priority.copy(); 10672 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 10673 if (related != null) { 10674 dst.related = new ArrayList<RelatedClaimComponent>(); 10675 for (RelatedClaimComponent i : related) 10676 dst.related.add(i.copy()); 10677 } 10678 ; 10679 dst.prescription = prescription == null ? null : prescription.copy(); 10680 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 10681 dst.payee = payee == null ? null : payee.copy(); 10682 dst.referral = referral == null ? null : referral.copy(); 10683 dst.facility = facility == null ? null : facility.copy(); 10684 if (careTeam != null) { 10685 dst.careTeam = new ArrayList<CareTeamComponent>(); 10686 for (CareTeamComponent i : careTeam) 10687 dst.careTeam.add(i.copy()); 10688 } 10689 ; 10690 if (supportingInfo != null) { 10691 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 10692 for (SupportingInformationComponent i : supportingInfo) 10693 dst.supportingInfo.add(i.copy()); 10694 } 10695 ; 10696 if (diagnosis != null) { 10697 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 10698 for (DiagnosisComponent i : diagnosis) 10699 dst.diagnosis.add(i.copy()); 10700 } 10701 ; 10702 if (procedure != null) { 10703 dst.procedure = new ArrayList<ProcedureComponent>(); 10704 for (ProcedureComponent i : procedure) 10705 dst.procedure.add(i.copy()); 10706 } 10707 ; 10708 if (insurance != null) { 10709 dst.insurance = new ArrayList<InsuranceComponent>(); 10710 for (InsuranceComponent i : insurance) 10711 dst.insurance.add(i.copy()); 10712 } 10713 ; 10714 dst.accident = accident == null ? null : accident.copy(); 10715 if (item != null) { 10716 dst.item = new ArrayList<ItemComponent>(); 10717 for (ItemComponent i : item) 10718 dst.item.add(i.copy()); 10719 } 10720 ; 10721 dst.total = total == null ? null : total.copy(); 10722 } 10723 10724 protected Claim typedCopy() { 10725 return copy(); 10726 } 10727 10728 @Override 10729 public boolean equalsDeep(Base other_) { 10730 if (!super.equalsDeep(other_)) 10731 return false; 10732 if (!(other_ instanceof Claim)) 10733 return false; 10734 Claim o = (Claim) other_; 10735 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 10736 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) 10737 && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 10738 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) 10739 && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) 10740 && compareDeep(priority, o.priority, true) && compareDeep(fundsReserve, o.fundsReserve, true) 10741 && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) 10742 && compareDeep(originalPrescription, o.originalPrescription, true) && compareDeep(payee, o.payee, true) 10743 && compareDeep(referral, o.referral, true) && compareDeep(facility, o.facility, true) 10744 && compareDeep(careTeam, o.careTeam, true) && compareDeep(supportingInfo, o.supportingInfo, true) 10745 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) 10746 && compareDeep(insurance, o.insurance, true) && compareDeep(accident, o.accident, true) 10747 && compareDeep(item, o.item, true) && compareDeep(total, o.total, true); 10748 } 10749 10750 @Override 10751 public boolean equalsShallow(Base other_) { 10752 if (!super.equalsShallow(other_)) 10753 return false; 10754 if (!(other_ instanceof Claim)) 10755 return false; 10756 Claim o = (Claim) other_; 10757 return compareValues(status, o.status, true) && compareValues(use, o.use, true) 10758 && compareValues(created, o.created, true); 10759 } 10760 10761 public boolean isEmpty() { 10762 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, subType, use, patient, 10763 billablePeriod, created, enterer, insurer, provider, priority, fundsReserve, related, prescription, 10764 originalPrescription, payee, referral, facility, careTeam, supportingInfo, diagnosis, procedure, insurance, 10765 accident, item, total); 10766 } 10767 10768 @Override 10769 public ResourceType getResourceType() { 10770 return ResourceType.Claim; 10771 } 10772 10773 /** 10774 * Search parameter: <b>care-team</b> 10775 * <p> 10776 * Description: <b>Member of the CareTeam</b><br> 10777 * Type: <b>reference</b><br> 10778 * Path: <b>Claim.careTeam.provider</b><br> 10779 * </p> 10780 */ 10781 @SearchParamDefinition(name = "care-team", path = "Claim.careTeam.provider", description = "Member of the CareTeam", type = "reference", providesMembershipIn = { 10782 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 10783 Practitioner.class, PractitionerRole.class }) 10784 public static final String SP_CARE_TEAM = "care-team"; 10785 /** 10786 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 10787 * <p> 10788 * Description: <b>Member of the CareTeam</b><br> 10789 * Type: <b>reference</b><br> 10790 * Path: <b>Claim.careTeam.provider</b><br> 10791 * </p> 10792 */ 10793 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10794 SP_CARE_TEAM); 10795 10796 /** 10797 * Constant for fluent queries to be used to add include statements. Specifies 10798 * the path value of "<b>Claim:care-team</b>". 10799 */ 10800 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include( 10801 "Claim:care-team").toLocked(); 10802 10803 /** 10804 * Search parameter: <b>identifier</b> 10805 * <p> 10806 * Description: <b>The primary identifier of the financial resource</b><br> 10807 * Type: <b>token</b><br> 10808 * Path: <b>Claim.identifier</b><br> 10809 * </p> 10810 */ 10811 @SearchParamDefinition(name = "identifier", path = "Claim.identifier", description = "The primary identifier of the financial resource", type = "token") 10812 public static final String SP_IDENTIFIER = "identifier"; 10813 /** 10814 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10815 * <p> 10816 * Description: <b>The primary identifier of the financial resource</b><br> 10817 * Type: <b>token</b><br> 10818 * Path: <b>Claim.identifier</b><br> 10819 * </p> 10820 */ 10821 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10822 SP_IDENTIFIER); 10823 10824 /** 10825 * Search parameter: <b>use</b> 10826 * <p> 10827 * Description: <b>The kind of financial resource</b><br> 10828 * Type: <b>token</b><br> 10829 * Path: <b>Claim.use</b><br> 10830 * </p> 10831 */ 10832 @SearchParamDefinition(name = "use", path = "Claim.use", description = "The kind of financial resource", type = "token") 10833 public static final String SP_USE = "use"; 10834 /** 10835 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10836 * <p> 10837 * Description: <b>The kind of financial resource</b><br> 10838 * Type: <b>token</b><br> 10839 * Path: <b>Claim.use</b><br> 10840 * </p> 10841 */ 10842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10843 SP_USE); 10844 10845 /** 10846 * Search parameter: <b>created</b> 10847 * <p> 10848 * Description: <b>The creation date for the Claim</b><br> 10849 * Type: <b>date</b><br> 10850 * Path: <b>Claim.created</b><br> 10851 * </p> 10852 */ 10853 @SearchParamDefinition(name = "created", path = "Claim.created", description = "The creation date for the Claim", type = "date") 10854 public static final String SP_CREATED = "created"; 10855 /** 10856 * <b>Fluent Client</b> search parameter constant for <b>created</b> 10857 * <p> 10858 * Description: <b>The creation date for the Claim</b><br> 10859 * Type: <b>date</b><br> 10860 * Path: <b>Claim.created</b><br> 10861 * </p> 10862 */ 10863 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 10864 SP_CREATED); 10865 10866 /** 10867 * Search parameter: <b>encounter</b> 10868 * <p> 10869 * Description: <b>Encounters associated with a billed line item</b><br> 10870 * Type: <b>reference</b><br> 10871 * Path: <b>Claim.item.encounter</b><br> 10872 * </p> 10873 */ 10874 @SearchParamDefinition(name = "encounter", path = "Claim.item.encounter", description = "Encounters associated with a billed line item", type = "reference", providesMembershipIn = { 10875 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 10876 public static final String SP_ENCOUNTER = "encounter"; 10877 /** 10878 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 10879 * <p> 10880 * Description: <b>Encounters associated with a billed line item</b><br> 10881 * Type: <b>reference</b><br> 10882 * Path: <b>Claim.item.encounter</b><br> 10883 * </p> 10884 */ 10885 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10886 SP_ENCOUNTER); 10887 10888 /** 10889 * Constant for fluent queries to be used to add include statements. Specifies 10890 * the path value of "<b>Claim:encounter</b>". 10891 */ 10892 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 10893 "Claim:encounter").toLocked(); 10894 10895 /** 10896 * Search parameter: <b>priority</b> 10897 * <p> 10898 * Description: <b>Processing priority requested</b><br> 10899 * Type: <b>token</b><br> 10900 * Path: <b>Claim.priority</b><br> 10901 * </p> 10902 */ 10903 @SearchParamDefinition(name = "priority", path = "Claim.priority", description = "Processing priority requested", type = "token") 10904 public static final String SP_PRIORITY = "priority"; 10905 /** 10906 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 10907 * <p> 10908 * Description: <b>Processing priority requested</b><br> 10909 * Type: <b>token</b><br> 10910 * Path: <b>Claim.priority</b><br> 10911 * </p> 10912 */ 10913 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10914 SP_PRIORITY); 10915 10916 /** 10917 * Search parameter: <b>payee</b> 10918 * <p> 10919 * Description: <b>The party receiving any payment for the Claim</b><br> 10920 * Type: <b>reference</b><br> 10921 * Path: <b>Claim.payee.party</b><br> 10922 * </p> 10923 */ 10924 @SearchParamDefinition(name = "payee", path = "Claim.payee.party", description = "The party receiving any payment for the Claim", type = "reference", providesMembershipIn = { 10925 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 10926 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 10927 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 10928 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 10929 public static final String SP_PAYEE = "payee"; 10930 /** 10931 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 10932 * <p> 10933 * Description: <b>The party receiving any payment for the Claim</b><br> 10934 * Type: <b>reference</b><br> 10935 * Path: <b>Claim.payee.party</b><br> 10936 * </p> 10937 */ 10938 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10939 SP_PAYEE); 10940 10941 /** 10942 * Constant for fluent queries to be used to add include statements. Specifies 10943 * the path value of "<b>Claim:payee</b>". 10944 */ 10945 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("Claim:payee") 10946 .toLocked(); 10947 10948 /** 10949 * Search parameter: <b>provider</b> 10950 * <p> 10951 * Description: <b>Provider responsible for the Claim</b><br> 10952 * Type: <b>reference</b><br> 10953 * Path: <b>Claim.provider</b><br> 10954 * </p> 10955 */ 10956 @SearchParamDefinition(name = "provider", path = "Claim.provider", description = "Provider responsible for the Claim", type = "reference", providesMembershipIn = { 10957 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 10958 Practitioner.class, PractitionerRole.class }) 10959 public static final String SP_PROVIDER = "provider"; 10960 /** 10961 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 10962 * <p> 10963 * Description: <b>Provider responsible for the Claim</b><br> 10964 * Type: <b>reference</b><br> 10965 * Path: <b>Claim.provider</b><br> 10966 * </p> 10967 */ 10968 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10969 SP_PROVIDER); 10970 10971 /** 10972 * Constant for fluent queries to be used to add include statements. Specifies 10973 * the path value of "<b>Claim:provider</b>". 10974 */ 10975 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include( 10976 "Claim:provider").toLocked(); 10977 10978 /** 10979 * Search parameter: <b>patient</b> 10980 * <p> 10981 * Description: <b>Patient receiving the products or services</b><br> 10982 * Type: <b>reference</b><br> 10983 * Path: <b>Claim.patient</b><br> 10984 * </p> 10985 */ 10986 @SearchParamDefinition(name = "patient", path = "Claim.patient", description = "Patient receiving the products or services", type = "reference", providesMembershipIn = { 10987 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 10988 public static final String SP_PATIENT = "patient"; 10989 /** 10990 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 10991 * <p> 10992 * Description: <b>Patient receiving the products or services</b><br> 10993 * Type: <b>reference</b><br> 10994 * Path: <b>Claim.patient</b><br> 10995 * </p> 10996 */ 10997 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10998 SP_PATIENT); 10999 11000 /** 11001 * Constant for fluent queries to be used to add include statements. Specifies 11002 * the path value of "<b>Claim:patient</b>". 11003 */ 11004 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Claim:patient") 11005 .toLocked(); 11006 11007 /** 11008 * Search parameter: <b>insurer</b> 11009 * <p> 11010 * Description: <b>The target payor/insurer for the Claim</b><br> 11011 * Type: <b>reference</b><br> 11012 * Path: <b>Claim.insurer</b><br> 11013 * </p> 11014 */ 11015 @SearchParamDefinition(name = "insurer", path = "Claim.insurer", description = "The target payor/insurer for the Claim", type = "reference", target = { 11016 Organization.class }) 11017 public static final String SP_INSURER = "insurer"; 11018 /** 11019 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 11020 * <p> 11021 * Description: <b>The target payor/insurer for the Claim</b><br> 11022 * Type: <b>reference</b><br> 11023 * Path: <b>Claim.insurer</b><br> 11024 * </p> 11025 */ 11026 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11027 SP_INSURER); 11028 11029 /** 11030 * Constant for fluent queries to be used to add include statements. Specifies 11031 * the path value of "<b>Claim:insurer</b>". 11032 */ 11033 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Claim:insurer") 11034 .toLocked(); 11035 11036 /** 11037 * Search parameter: <b>detail-udi</b> 11038 * <p> 11039 * Description: <b>UDI associated with a line item, detail product or 11040 * service</b><br> 11041 * Type: <b>reference</b><br> 11042 * Path: <b>Claim.item.detail.udi</b><br> 11043 * </p> 11044 */ 11045 @SearchParamDefinition(name = "detail-udi", path = "Claim.item.detail.udi", description = "UDI associated with a line item, detail product or service", type = "reference", providesMembershipIn = { 11046 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11047 public static final String SP_DETAIL_UDI = "detail-udi"; 11048 /** 11049 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 11050 * <p> 11051 * Description: <b>UDI associated with a line item, detail product or 11052 * service</b><br> 11053 * Type: <b>reference</b><br> 11054 * Path: <b>Claim.item.detail.udi</b><br> 11055 * </p> 11056 */ 11057 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11058 SP_DETAIL_UDI); 11059 11060 /** 11061 * Constant for fluent queries to be used to add include statements. Specifies 11062 * the path value of "<b>Claim:detail-udi</b>". 11063 */ 11064 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include( 11065 "Claim:detail-udi").toLocked(); 11066 11067 /** 11068 * Search parameter: <b>enterer</b> 11069 * <p> 11070 * Description: <b>The party responsible for the entry of the Claim</b><br> 11071 * Type: <b>reference</b><br> 11072 * Path: <b>Claim.enterer</b><br> 11073 * </p> 11074 */ 11075 @SearchParamDefinition(name = "enterer", path = "Claim.enterer", description = "The party responsible for the entry of the Claim", type = "reference", providesMembershipIn = { 11076 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 11077 PractitionerRole.class }) 11078 public static final String SP_ENTERER = "enterer"; 11079 /** 11080 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 11081 * <p> 11082 * Description: <b>The party responsible for the entry of the Claim</b><br> 11083 * Type: <b>reference</b><br> 11084 * Path: <b>Claim.enterer</b><br> 11085 * </p> 11086 */ 11087 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11088 SP_ENTERER); 11089 11090 /** 11091 * Constant for fluent queries to be used to add include statements. Specifies 11092 * the path value of "<b>Claim:enterer</b>". 11093 */ 11094 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("Claim:enterer") 11095 .toLocked(); 11096 11097 /** 11098 * Search parameter: <b>procedure-udi</b> 11099 * <p> 11100 * Description: <b>UDI associated with a procedure</b><br> 11101 * Type: <b>reference</b><br> 11102 * Path: <b>Claim.procedure.udi</b><br> 11103 * </p> 11104 */ 11105 @SearchParamDefinition(name = "procedure-udi", path = "Claim.procedure.udi", description = "UDI associated with a procedure", type = "reference", providesMembershipIn = { 11106 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11107 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 11108 /** 11109 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 11110 * <p> 11111 * Description: <b>UDI associated with a procedure</b><br> 11112 * Type: <b>reference</b><br> 11113 * Path: <b>Claim.procedure.udi</b><br> 11114 * </p> 11115 */ 11116 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11117 SP_PROCEDURE_UDI); 11118 11119 /** 11120 * Constant for fluent queries to be used to add include statements. Specifies 11121 * the path value of "<b>Claim:procedure-udi</b>". 11122 */ 11123 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include( 11124 "Claim:procedure-udi").toLocked(); 11125 11126 /** 11127 * Search parameter: <b>subdetail-udi</b> 11128 * <p> 11129 * Description: <b>UDI associated with a line item, detail, subdetail product or 11130 * service</b><br> 11131 * Type: <b>reference</b><br> 11132 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 11133 * </p> 11134 */ 11135 @SearchParamDefinition(name = "subdetail-udi", path = "Claim.item.detail.subDetail.udi", description = "UDI associated with a line item, detail, subdetail product or service", type = "reference", providesMembershipIn = { 11136 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11137 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 11138 /** 11139 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 11140 * <p> 11141 * Description: <b>UDI associated with a line item, detail, subdetail product or 11142 * service</b><br> 11143 * Type: <b>reference</b><br> 11144 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 11145 * </p> 11146 */ 11147 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11148 SP_SUBDETAIL_UDI); 11149 11150 /** 11151 * Constant for fluent queries to be used to add include statements. Specifies 11152 * the path value of "<b>Claim:subdetail-udi</b>". 11153 */ 11154 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include( 11155 "Claim:subdetail-udi").toLocked(); 11156 11157 /** 11158 * Search parameter: <b>facility</b> 11159 * <p> 11160 * Description: <b>Facility where the products or services have been or will be 11161 * provided</b><br> 11162 * Type: <b>reference</b><br> 11163 * Path: <b>Claim.facility</b><br> 11164 * </p> 11165 */ 11166 @SearchParamDefinition(name = "facility", path = "Claim.facility", description = "Facility where the products or services have been or will be provided", type = "reference", target = { 11167 Location.class }) 11168 public static final String SP_FACILITY = "facility"; 11169 /** 11170 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 11171 * <p> 11172 * Description: <b>Facility where the products or services have been or will be 11173 * provided</b><br> 11174 * Type: <b>reference</b><br> 11175 * Path: <b>Claim.facility</b><br> 11176 * </p> 11177 */ 11178 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11179 SP_FACILITY); 11180 11181 /** 11182 * Constant for fluent queries to be used to add include statements. Specifies 11183 * the path value of "<b>Claim:facility</b>". 11184 */ 11185 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include( 11186 "Claim:facility").toLocked(); 11187 11188 /** 11189 * Search parameter: <b>item-udi</b> 11190 * <p> 11191 * Description: <b>UDI associated with a line item product or service</b><br> 11192 * Type: <b>reference</b><br> 11193 * Path: <b>Claim.item.udi</b><br> 11194 * </p> 11195 */ 11196 @SearchParamDefinition(name = "item-udi", path = "Claim.item.udi", description = "UDI associated with a line item product or service", type = "reference", providesMembershipIn = { 11197 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 11198 public static final String SP_ITEM_UDI = "item-udi"; 11199 /** 11200 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 11201 * <p> 11202 * Description: <b>UDI associated with a line item product or service</b><br> 11203 * Type: <b>reference</b><br> 11204 * Path: <b>Claim.item.udi</b><br> 11205 * </p> 11206 */ 11207 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 11208 SP_ITEM_UDI); 11209 11210 /** 11211 * Constant for fluent queries to be used to add include statements. Specifies 11212 * the path value of "<b>Claim:item-udi</b>". 11213 */ 11214 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include( 11215 "Claim:item-udi").toLocked(); 11216 11217 /** 11218 * Search parameter: <b>status</b> 11219 * <p> 11220 * Description: <b>The status of the Claim instance.</b><br> 11221 * Type: <b>token</b><br> 11222 * Path: <b>Claim.status</b><br> 11223 * </p> 11224 */ 11225 @SearchParamDefinition(name = "status", path = "Claim.status", description = "The status of the Claim instance.", type = "token") 11226 public static final String SP_STATUS = "status"; 11227 /** 11228 * <b>Fluent Client</b> search parameter constant for <b>status</b> 11229 * <p> 11230 * Description: <b>The status of the Claim instance.</b><br> 11231 * Type: <b>token</b><br> 11232 * Path: <b>Claim.status</b><br> 11233 * </p> 11234 */ 11235 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11236 SP_STATUS); 11237 11238}