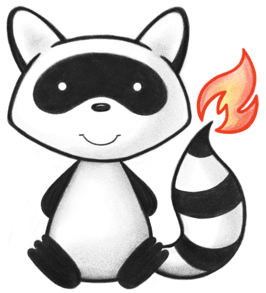
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.NoteType; 042import org.hl7.fhir.r4.model.Enumerations.NoteTypeEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050 051/** 052 * This resource provides the adjudication details from the processing of a 053 * Claim resource. 054 */ 055@ResourceDef(name = "ClaimResponse", profile = "http://hl7.org/fhir/StructureDefinition/ClaimResponse") 056public class ClaimResponse extends DomainResource { 057 058 public enum ClaimResponseStatus { 059 /** 060 * The instance is currently in-force. 061 */ 062 ACTIVE, 063 /** 064 * The instance is withdrawn, rescinded or reversed. 065 */ 066 CANCELLED, 067 /** 068 * A new instance the contents of which is not complete. 069 */ 070 DRAFT, 071 /** 072 * The instance was entered in error. 073 */ 074 ENTEREDINERROR, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static ClaimResponseStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("cancelled".equals(codeString)) 086 return CANCELLED; 087 if ("draft".equals(codeString)) 088 return DRAFT; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown ClaimResponseStatus code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case ACTIVE: 100 return "active"; 101 case CANCELLED: 102 return "cancelled"; 103 case DRAFT: 104 return "draft"; 105 case ENTEREDINERROR: 106 return "entered-in-error"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getSystem() { 115 switch (this) { 116 case ACTIVE: 117 return "http://hl7.org/fhir/fm-status"; 118 case CANCELLED: 119 return "http://hl7.org/fhir/fm-status"; 120 case DRAFT: 121 return "http://hl7.org/fhir/fm-status"; 122 case ENTEREDINERROR: 123 return "http://hl7.org/fhir/fm-status"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDefinition() { 132 switch (this) { 133 case ACTIVE: 134 return "The instance is currently in-force."; 135 case CANCELLED: 136 return "The instance is withdrawn, rescinded or reversed."; 137 case DRAFT: 138 return "A new instance the contents of which is not complete."; 139 case ENTEREDINERROR: 140 return "The instance was entered in error."; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getDisplay() { 149 switch (this) { 150 case ACTIVE: 151 return "Active"; 152 case CANCELLED: 153 return "Cancelled"; 154 case DRAFT: 155 return "Draft"; 156 case ENTEREDINERROR: 157 return "Entered in Error"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 } 165 166 public static class ClaimResponseStatusEnumFactory implements EnumFactory<ClaimResponseStatus> { 167 public ClaimResponseStatus fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("active".equals(codeString)) 172 return ClaimResponseStatus.ACTIVE; 173 if ("cancelled".equals(codeString)) 174 return ClaimResponseStatus.CANCELLED; 175 if ("draft".equals(codeString)) 176 return ClaimResponseStatus.DRAFT; 177 if ("entered-in-error".equals(codeString)) 178 return ClaimResponseStatus.ENTEREDINERROR; 179 throw new IllegalArgumentException("Unknown ClaimResponseStatus code '" + codeString + "'"); 180 } 181 182 public Enumeration<ClaimResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 183 if (code == null) 184 return null; 185 if (code.isEmpty()) 186 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.NULL, code); 187 String codeString = code.asStringValue(); 188 if (codeString == null || "".equals(codeString)) 189 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.NULL, code); 190 if ("active".equals(codeString)) 191 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.ACTIVE, code); 192 if ("cancelled".equals(codeString)) 193 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.CANCELLED, code); 194 if ("draft".equals(codeString)) 195 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.DRAFT, code); 196 if ("entered-in-error".equals(codeString)) 197 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.ENTEREDINERROR, code); 198 throw new FHIRException("Unknown ClaimResponseStatus code '" + codeString + "'"); 199 } 200 201 public String toCode(ClaimResponseStatus code) { 202 if (code == ClaimResponseStatus.NULL) 203 return null; 204 if (code == ClaimResponseStatus.ACTIVE) 205 return "active"; 206 if (code == ClaimResponseStatus.CANCELLED) 207 return "cancelled"; 208 if (code == ClaimResponseStatus.DRAFT) 209 return "draft"; 210 if (code == ClaimResponseStatus.ENTEREDINERROR) 211 return "entered-in-error"; 212 return "?"; 213 } 214 215 public String toSystem(ClaimResponseStatus code) { 216 return code.getSystem(); 217 } 218 } 219 220 public enum Use { 221 /** 222 * The treatment is complete and this represents a Claim for the services. 223 */ 224 CLAIM, 225 /** 226 * The treatment is proposed and this represents a Pre-authorization for the 227 * services. 228 */ 229 PREAUTHORIZATION, 230 /** 231 * The treatment is proposed and this represents a Pre-determination for the 232 * services. 233 */ 234 PREDETERMINATION, 235 /** 236 * added to help the parsers with the generic types 237 */ 238 NULL; 239 240 public static Use fromCode(String codeString) throws FHIRException { 241 if (codeString == null || "".equals(codeString)) 242 return null; 243 if ("claim".equals(codeString)) 244 return CLAIM; 245 if ("preauthorization".equals(codeString)) 246 return PREAUTHORIZATION; 247 if ("predetermination".equals(codeString)) 248 return PREDETERMINATION; 249 if (Configuration.isAcceptInvalidEnums()) 250 return null; 251 else 252 throw new FHIRException("Unknown Use code '" + codeString + "'"); 253 } 254 255 public String toCode() { 256 switch (this) { 257 case CLAIM: 258 return "claim"; 259 case PREAUTHORIZATION: 260 return "preauthorization"; 261 case PREDETERMINATION: 262 return "predetermination"; 263 case NULL: 264 return null; 265 default: 266 return "?"; 267 } 268 } 269 270 public String getSystem() { 271 switch (this) { 272 case CLAIM: 273 return "http://hl7.org/fhir/claim-use"; 274 case PREAUTHORIZATION: 275 return "http://hl7.org/fhir/claim-use"; 276 case PREDETERMINATION: 277 return "http://hl7.org/fhir/claim-use"; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getDefinition() { 286 switch (this) { 287 case CLAIM: 288 return "The treatment is complete and this represents a Claim for the services."; 289 case PREAUTHORIZATION: 290 return "The treatment is proposed and this represents a Pre-authorization for the services."; 291 case PREDETERMINATION: 292 return "The treatment is proposed and this represents a Pre-determination for the services."; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300 public String getDisplay() { 301 switch (this) { 302 case CLAIM: 303 return "Claim"; 304 case PREAUTHORIZATION: 305 return "Preauthorization"; 306 case PREDETERMINATION: 307 return "Predetermination"; 308 case NULL: 309 return null; 310 default: 311 return "?"; 312 } 313 } 314 } 315 316 public static class UseEnumFactory implements EnumFactory<Use> { 317 public Use fromCode(String codeString) throws IllegalArgumentException { 318 if (codeString == null || "".equals(codeString)) 319 if (codeString == null || "".equals(codeString)) 320 return null; 321 if ("claim".equals(codeString)) 322 return Use.CLAIM; 323 if ("preauthorization".equals(codeString)) 324 return Use.PREAUTHORIZATION; 325 if ("predetermination".equals(codeString)) 326 return Use.PREDETERMINATION; 327 throw new IllegalArgumentException("Unknown Use code '" + codeString + "'"); 328 } 329 330 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 331 if (code == null) 332 return null; 333 if (code.isEmpty()) 334 return new Enumeration<Use>(this, Use.NULL, code); 335 String codeString = code.asStringValue(); 336 if (codeString == null || "".equals(codeString)) 337 return new Enumeration<Use>(this, Use.NULL, code); 338 if ("claim".equals(codeString)) 339 return new Enumeration<Use>(this, Use.CLAIM, code); 340 if ("preauthorization".equals(codeString)) 341 return new Enumeration<Use>(this, Use.PREAUTHORIZATION, code); 342 if ("predetermination".equals(codeString)) 343 return new Enumeration<Use>(this, Use.PREDETERMINATION, code); 344 throw new FHIRException("Unknown Use code '" + codeString + "'"); 345 } 346 347 public String toCode(Use code) { 348 if (code == Use.NULL) 349 return null; 350 if (code == Use.CLAIM) 351 return "claim"; 352 if (code == Use.PREAUTHORIZATION) 353 return "preauthorization"; 354 if (code == Use.PREDETERMINATION) 355 return "predetermination"; 356 return "?"; 357 } 358 359 public String toSystem(Use code) { 360 return code.getSystem(); 361 } 362 } 363 364 public enum RemittanceOutcome { 365 /** 366 * The Claim/Pre-authorization/Pre-determination has been received but 367 * processing has not begun. 368 */ 369 QUEUED, 370 /** 371 * The processing has completed without errors 372 */ 373 COMPLETE, 374 /** 375 * One or more errors have been detected in the Claim 376 */ 377 ERROR, 378 /** 379 * No errors have been detected in the Claim and some of the adjudication has 380 * been performed. 381 */ 382 PARTIAL, 383 /** 384 * added to help the parsers with the generic types 385 */ 386 NULL; 387 388 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 389 if (codeString == null || "".equals(codeString)) 390 return null; 391 if ("queued".equals(codeString)) 392 return QUEUED; 393 if ("complete".equals(codeString)) 394 return COMPLETE; 395 if ("error".equals(codeString)) 396 return ERROR; 397 if ("partial".equals(codeString)) 398 return PARTIAL; 399 if (Configuration.isAcceptInvalidEnums()) 400 return null; 401 else 402 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 403 } 404 405 public String toCode() { 406 switch (this) { 407 case QUEUED: 408 return "queued"; 409 case COMPLETE: 410 return "complete"; 411 case ERROR: 412 return "error"; 413 case PARTIAL: 414 return "partial"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getSystem() { 423 switch (this) { 424 case QUEUED: 425 return "http://hl7.org/fhir/remittance-outcome"; 426 case COMPLETE: 427 return "http://hl7.org/fhir/remittance-outcome"; 428 case ERROR: 429 return "http://hl7.org/fhir/remittance-outcome"; 430 case PARTIAL: 431 return "http://hl7.org/fhir/remittance-outcome"; 432 case NULL: 433 return null; 434 default: 435 return "?"; 436 } 437 } 438 439 public String getDefinition() { 440 switch (this) { 441 case QUEUED: 442 return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 443 case COMPLETE: 444 return "The processing has completed without errors"; 445 case ERROR: 446 return "One or more errors have been detected in the Claim"; 447 case PARTIAL: 448 return "No errors have been detected in the Claim and some of the adjudication has been performed."; 449 case NULL: 450 return null; 451 default: 452 return "?"; 453 } 454 } 455 456 public String getDisplay() { 457 switch (this) { 458 case QUEUED: 459 return "Queued"; 460 case COMPLETE: 461 return "Processing Complete"; 462 case ERROR: 463 return "Error"; 464 case PARTIAL: 465 return "Partial Processing"; 466 case NULL: 467 return null; 468 default: 469 return "?"; 470 } 471 } 472 } 473 474 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 475 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 476 if (codeString == null || "".equals(codeString)) 477 if (codeString == null || "".equals(codeString)) 478 return null; 479 if ("queued".equals(codeString)) 480 return RemittanceOutcome.QUEUED; 481 if ("complete".equals(codeString)) 482 return RemittanceOutcome.COMPLETE; 483 if ("error".equals(codeString)) 484 return RemittanceOutcome.ERROR; 485 if ("partial".equals(codeString)) 486 return RemittanceOutcome.PARTIAL; 487 throw new IllegalArgumentException("Unknown RemittanceOutcome code '" + codeString + "'"); 488 } 489 490 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 491 if (code == null) 492 return null; 493 if (code.isEmpty()) 494 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 495 String codeString = code.asStringValue(); 496 if (codeString == null || "".equals(codeString)) 497 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 498 if ("queued".equals(codeString)) 499 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.QUEUED, code); 500 if ("complete".equals(codeString)) 501 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE, code); 502 if ("error".equals(codeString)) 503 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR, code); 504 if ("partial".equals(codeString)) 505 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL, code); 506 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 507 } 508 509 public String toCode(RemittanceOutcome code) { 510 if (code == RemittanceOutcome.NULL) 511 return null; 512 if (code == RemittanceOutcome.QUEUED) 513 return "queued"; 514 if (code == RemittanceOutcome.COMPLETE) 515 return "complete"; 516 if (code == RemittanceOutcome.ERROR) 517 return "error"; 518 if (code == RemittanceOutcome.PARTIAL) 519 return "partial"; 520 return "?"; 521 } 522 523 public String toSystem(RemittanceOutcome code) { 524 return code.getSystem(); 525 } 526 } 527 528 @Block() 529 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 530 /** 531 * A number to uniquely reference the claim item entries. 532 */ 533 @Child(name = "itemSequence", type = { 534 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 535 @Description(shortDefinition = "Claim item instance identifier", formalDefinition = "A number to uniquely reference the claim item entries.") 536 protected PositiveIntType itemSequence; 537 538 /** 539 * The numbers associated with notes below which apply to the adjudication of 540 * this item. 541 */ 542 @Child(name = "noteNumber", type = { 543 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 544 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 545 protected List<PositiveIntType> noteNumber; 546 547 /** 548 * If this item is a group then the values here are a summary of the 549 * adjudication of the detail items. If this item is a simple product or service 550 * then this is the result of the adjudication of this item. 551 */ 552 @Child(name = "adjudication", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 553 @Description(shortDefinition = "Adjudication details", formalDefinition = "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.") 554 protected List<AdjudicationComponent> adjudication; 555 556 /** 557 * A claim detail. Either a simple (a product or service) or a 'group' of 558 * sub-details which are simple items. 559 */ 560 @Child(name = "detail", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 561 @Description(shortDefinition = "Adjudication for claim details", formalDefinition = "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 562 protected List<ItemDetailComponent> detail; 563 564 private static final long serialVersionUID = 701277928L; 565 566 /** 567 * Constructor 568 */ 569 public ItemComponent() { 570 super(); 571 } 572 573 /** 574 * Constructor 575 */ 576 public ItemComponent(PositiveIntType itemSequence) { 577 super(); 578 this.itemSequence = itemSequence; 579 } 580 581 /** 582 * @return {@link #itemSequence} (A number to uniquely reference the claim item 583 * entries.). This is the underlying object with id, value and 584 * extensions. The accessor "getItemSequence" gives direct access to the 585 * value 586 */ 587 public PositiveIntType getItemSequenceElement() { 588 if (this.itemSequence == null) 589 if (Configuration.errorOnAutoCreate()) 590 throw new Error("Attempt to auto-create ItemComponent.itemSequence"); 591 else if (Configuration.doAutoCreate()) 592 this.itemSequence = new PositiveIntType(); // bb 593 return this.itemSequence; 594 } 595 596 public boolean hasItemSequenceElement() { 597 return this.itemSequence != null && !this.itemSequence.isEmpty(); 598 } 599 600 public boolean hasItemSequence() { 601 return this.itemSequence != null && !this.itemSequence.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #itemSequence} (A number to uniquely reference the claim 606 * item entries.). This is the underlying object with id, value and 607 * extensions. The accessor "getItemSequence" gives direct access 608 * to the value 609 */ 610 public ItemComponent setItemSequenceElement(PositiveIntType value) { 611 this.itemSequence = value; 612 return this; 613 } 614 615 /** 616 * @return A number to uniquely reference the claim item entries. 617 */ 618 public int getItemSequence() { 619 return this.itemSequence == null || this.itemSequence.isEmpty() ? 0 : this.itemSequence.getValue(); 620 } 621 622 /** 623 * @param value A number to uniquely reference the claim item entries. 624 */ 625 public ItemComponent setItemSequence(int value) { 626 if (this.itemSequence == null) 627 this.itemSequence = new PositiveIntType(); 628 this.itemSequence.setValue(value); 629 return this; 630 } 631 632 /** 633 * @return {@link #noteNumber} (The numbers associated with notes below which 634 * apply to the adjudication of this item.) 635 */ 636 public List<PositiveIntType> getNoteNumber() { 637 if (this.noteNumber == null) 638 this.noteNumber = new ArrayList<PositiveIntType>(); 639 return this.noteNumber; 640 } 641 642 /** 643 * @return Returns a reference to <code>this</code> for easy method chaining 644 */ 645 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 646 this.noteNumber = theNoteNumber; 647 return this; 648 } 649 650 public boolean hasNoteNumber() { 651 if (this.noteNumber == null) 652 return false; 653 for (PositiveIntType item : this.noteNumber) 654 if (!item.isEmpty()) 655 return true; 656 return false; 657 } 658 659 /** 660 * @return {@link #noteNumber} (The numbers associated with notes below which 661 * apply to the adjudication of this item.) 662 */ 663 public PositiveIntType addNoteNumberElement() {// 2 664 PositiveIntType t = new PositiveIntType(); 665 if (this.noteNumber == null) 666 this.noteNumber = new ArrayList<PositiveIntType>(); 667 this.noteNumber.add(t); 668 return t; 669 } 670 671 /** 672 * @param value {@link #noteNumber} (The numbers associated with notes below 673 * which apply to the adjudication of this item.) 674 */ 675 public ItemComponent addNoteNumber(int value) { // 1 676 PositiveIntType t = new PositiveIntType(); 677 t.setValue(value); 678 if (this.noteNumber == null) 679 this.noteNumber = new ArrayList<PositiveIntType>(); 680 this.noteNumber.add(t); 681 return this; 682 } 683 684 /** 685 * @param value {@link #noteNumber} (The numbers associated with notes below 686 * which apply to the adjudication of this item.) 687 */ 688 public boolean hasNoteNumber(int value) { 689 if (this.noteNumber == null) 690 return false; 691 for (PositiveIntType v : this.noteNumber) 692 if (v.getValue().equals(value)) // positiveInt 693 return true; 694 return false; 695 } 696 697 /** 698 * @return {@link #adjudication} (If this item is a group then the values here 699 * are a summary of the adjudication of the detail items. If this item 700 * is a simple product or service then this is the result of the 701 * adjudication of this item.) 702 */ 703 public List<AdjudicationComponent> getAdjudication() { 704 if (this.adjudication == null) 705 this.adjudication = new ArrayList<AdjudicationComponent>(); 706 return this.adjudication; 707 } 708 709 /** 710 * @return Returns a reference to <code>this</code> for easy method chaining 711 */ 712 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 713 this.adjudication = theAdjudication; 714 return this; 715 } 716 717 public boolean hasAdjudication() { 718 if (this.adjudication == null) 719 return false; 720 for (AdjudicationComponent item : this.adjudication) 721 if (!item.isEmpty()) 722 return true; 723 return false; 724 } 725 726 public AdjudicationComponent addAdjudication() { // 3 727 AdjudicationComponent t = new AdjudicationComponent(); 728 if (this.adjudication == null) 729 this.adjudication = new ArrayList<AdjudicationComponent>(); 730 this.adjudication.add(t); 731 return t; 732 } 733 734 public ItemComponent addAdjudication(AdjudicationComponent t) { // 3 735 if (t == null) 736 return this; 737 if (this.adjudication == null) 738 this.adjudication = new ArrayList<AdjudicationComponent>(); 739 this.adjudication.add(t); 740 return this; 741 } 742 743 /** 744 * @return The first repetition of repeating field {@link #adjudication}, 745 * creating it if it does not already exist 746 */ 747 public AdjudicationComponent getAdjudicationFirstRep() { 748 if (getAdjudication().isEmpty()) { 749 addAdjudication(); 750 } 751 return getAdjudication().get(0); 752 } 753 754 /** 755 * @return {@link #detail} (A claim detail. Either a simple (a product or 756 * service) or a 'group' of sub-details which are simple items.) 757 */ 758 public List<ItemDetailComponent> getDetail() { 759 if (this.detail == null) 760 this.detail = new ArrayList<ItemDetailComponent>(); 761 return this.detail; 762 } 763 764 /** 765 * @return Returns a reference to <code>this</code> for easy method chaining 766 */ 767 public ItemComponent setDetail(List<ItemDetailComponent> theDetail) { 768 this.detail = theDetail; 769 return this; 770 } 771 772 public boolean hasDetail() { 773 if (this.detail == null) 774 return false; 775 for (ItemDetailComponent item : this.detail) 776 if (!item.isEmpty()) 777 return true; 778 return false; 779 } 780 781 public ItemDetailComponent addDetail() { // 3 782 ItemDetailComponent t = new ItemDetailComponent(); 783 if (this.detail == null) 784 this.detail = new ArrayList<ItemDetailComponent>(); 785 this.detail.add(t); 786 return t; 787 } 788 789 public ItemComponent addDetail(ItemDetailComponent t) { // 3 790 if (t == null) 791 return this; 792 if (this.detail == null) 793 this.detail = new ArrayList<ItemDetailComponent>(); 794 this.detail.add(t); 795 return this; 796 } 797 798 /** 799 * @return The first repetition of repeating field {@link #detail}, creating it 800 * if it does not already exist 801 */ 802 public ItemDetailComponent getDetailFirstRep() { 803 if (getDetail().isEmpty()) { 804 addDetail(); 805 } 806 return getDetail().get(0); 807 } 808 809 protected void listChildren(List<Property> children) { 810 super.listChildren(children); 811 children.add(new Property("itemSequence", "positiveInt", "A number to uniquely reference the claim item entries.", 812 0, 1, itemSequence)); 813 children.add(new Property("noteNumber", "positiveInt", 814 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 815 java.lang.Integer.MAX_VALUE, noteNumber)); 816 children.add(new Property("adjudication", "", 817 "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 818 0, java.lang.Integer.MAX_VALUE, adjudication)); 819 children.add(new Property("detail", "", 820 "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 821 0, java.lang.Integer.MAX_VALUE, detail)); 822 } 823 824 @Override 825 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 826 switch (_hash) { 827 case 1977979892: 828 /* itemSequence */ return new Property("itemSequence", "positiveInt", 829 "A number to uniquely reference the claim item entries.", 0, 1, itemSequence); 830 case -1110033957: 831 /* noteNumber */ return new Property("noteNumber", "positiveInt", 832 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 833 java.lang.Integer.MAX_VALUE, noteNumber); 834 case -231349275: 835 /* adjudication */ return new Property("adjudication", "", 836 "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 837 0, java.lang.Integer.MAX_VALUE, adjudication); 838 case -1335224239: 839 /* detail */ return new Property("detail", "", 840 "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 841 0, java.lang.Integer.MAX_VALUE, detail); 842 default: 843 return super.getNamedProperty(_hash, _name, _checkValid); 844 } 845 846 } 847 848 @Override 849 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 850 switch (hash) { 851 case 1977979892: 852 /* itemSequence */ return this.itemSequence == null ? new Base[0] : new Base[] { this.itemSequence }; // PositiveIntType 853 case -1110033957: 854 /* noteNumber */ return this.noteNumber == null ? new Base[0] 855 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 856 case -231349275: 857 /* adjudication */ return this.adjudication == null ? new Base[0] 858 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 859 case -1335224239: 860 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // ItemDetailComponent 861 default: 862 return super.getProperty(hash, name, checkValid); 863 } 864 865 } 866 867 @Override 868 public Base setProperty(int hash, String name, Base value) throws FHIRException { 869 switch (hash) { 870 case 1977979892: // itemSequence 871 this.itemSequence = castToPositiveInt(value); // PositiveIntType 872 return value; 873 case -1110033957: // noteNumber 874 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 875 return value; 876 case -231349275: // adjudication 877 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 878 return value; 879 case -1335224239: // detail 880 this.getDetail().add((ItemDetailComponent) value); // ItemDetailComponent 881 return value; 882 default: 883 return super.setProperty(hash, name, value); 884 } 885 886 } 887 888 @Override 889 public Base setProperty(String name, Base value) throws FHIRException { 890 if (name.equals("itemSequence")) { 891 this.itemSequence = castToPositiveInt(value); // PositiveIntType 892 } else if (name.equals("noteNumber")) { 893 this.getNoteNumber().add(castToPositiveInt(value)); 894 } else if (name.equals("adjudication")) { 895 this.getAdjudication().add((AdjudicationComponent) value); 896 } else if (name.equals("detail")) { 897 this.getDetail().add((ItemDetailComponent) value); 898 } else 899 return super.setProperty(name, value); 900 return value; 901 } 902 903 @Override 904 public void removeChild(String name, Base value) throws FHIRException { 905 if (name.equals("itemSequence")) { 906 this.itemSequence = null; 907 } else if (name.equals("noteNumber")) { 908 this.getNoteNumber().remove(castToPositiveInt(value)); 909 } else if (name.equals("adjudication")) { 910 this.getAdjudication().remove((AdjudicationComponent) value); 911 } else if (name.equals("detail")) { 912 this.getDetail().remove((ItemDetailComponent) value); 913 } else 914 super.removeChild(name, value); 915 916 } 917 918 @Override 919 public Base makeProperty(int hash, String name) throws FHIRException { 920 switch (hash) { 921 case 1977979892: 922 return getItemSequenceElement(); 923 case -1110033957: 924 return addNoteNumberElement(); 925 case -231349275: 926 return addAdjudication(); 927 case -1335224239: 928 return addDetail(); 929 default: 930 return super.makeProperty(hash, name); 931 } 932 933 } 934 935 @Override 936 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 937 switch (hash) { 938 case 1977979892: 939 /* itemSequence */ return new String[] { "positiveInt" }; 940 case -1110033957: 941 /* noteNumber */ return new String[] { "positiveInt" }; 942 case -231349275: 943 /* adjudication */ return new String[] {}; 944 case -1335224239: 945 /* detail */ return new String[] {}; 946 default: 947 return super.getTypesForProperty(hash, name); 948 } 949 950 } 951 952 @Override 953 public Base addChild(String name) throws FHIRException { 954 if (name.equals("itemSequence")) { 955 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.itemSequence"); 956 } else if (name.equals("noteNumber")) { 957 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 958 } else if (name.equals("adjudication")) { 959 return addAdjudication(); 960 } else if (name.equals("detail")) { 961 return addDetail(); 962 } else 963 return super.addChild(name); 964 } 965 966 public ItemComponent copy() { 967 ItemComponent dst = new ItemComponent(); 968 copyValues(dst); 969 return dst; 970 } 971 972 public void copyValues(ItemComponent dst) { 973 super.copyValues(dst); 974 dst.itemSequence = itemSequence == null ? null : itemSequence.copy(); 975 if (noteNumber != null) { 976 dst.noteNumber = new ArrayList<PositiveIntType>(); 977 for (PositiveIntType i : noteNumber) 978 dst.noteNumber.add(i.copy()); 979 } 980 ; 981 if (adjudication != null) { 982 dst.adjudication = new ArrayList<AdjudicationComponent>(); 983 for (AdjudicationComponent i : adjudication) 984 dst.adjudication.add(i.copy()); 985 } 986 ; 987 if (detail != null) { 988 dst.detail = new ArrayList<ItemDetailComponent>(); 989 for (ItemDetailComponent i : detail) 990 dst.detail.add(i.copy()); 991 } 992 ; 993 } 994 995 @Override 996 public boolean equalsDeep(Base other_) { 997 if (!super.equalsDeep(other_)) 998 return false; 999 if (!(other_ instanceof ItemComponent)) 1000 return false; 1001 ItemComponent o = (ItemComponent) other_; 1002 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(noteNumber, o.noteNumber, true) 1003 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 1004 } 1005 1006 @Override 1007 public boolean equalsShallow(Base other_) { 1008 if (!super.equalsShallow(other_)) 1009 return false; 1010 if (!(other_ instanceof ItemComponent)) 1011 return false; 1012 ItemComponent o = (ItemComponent) other_; 1013 return compareValues(itemSequence, o.itemSequence, true) && compareValues(noteNumber, o.noteNumber, true); 1014 } 1015 1016 public boolean isEmpty() { 1017 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, noteNumber, adjudication, detail); 1018 } 1019 1020 public String fhirType() { 1021 return "ClaimResponse.item"; 1022 1023 } 1024 1025 } 1026 1027 @Block() 1028 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 1029 /** 1030 * A code to indicate the information type of this adjudication record. 1031 * Information types may include the value submitted, maximum values or 1032 * percentages allowed or payable under the plan, amounts that: the patient is 1033 * responsible for in aggregate or pertaining to this item; amounts paid by 1034 * other coverages; and, the benefit payable for this item. 1035 */ 1036 @Child(name = "category", type = { 1037 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1038 @Description(shortDefinition = "Type of adjudication information", formalDefinition = "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.") 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication") 1040 protected CodeableConcept category; 1041 1042 /** 1043 * A code supporting the understanding of the adjudication result and explaining 1044 * variance from expected amount. 1045 */ 1046 @Child(name = "reason", type = { 1047 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1048 @Description(shortDefinition = "Explanation of adjudication outcome", formalDefinition = "A code supporting the understanding of the adjudication result and explaining variance from expected amount.") 1049 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication-reason") 1050 protected CodeableConcept reason; 1051 1052 /** 1053 * Monetary amount associated with the category. 1054 */ 1055 @Child(name = "amount", type = { Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1056 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the category.") 1057 protected Money amount; 1058 1059 /** 1060 * A non-monetary value associated with the category. Mutually exclusive to the 1061 * amount element above. 1062 */ 1063 @Child(name = "value", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1064 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value associated with the category. Mutually exclusive to the amount element above.") 1065 protected DecimalType value; 1066 1067 private static final long serialVersionUID = 1559898786L; 1068 1069 /** 1070 * Constructor 1071 */ 1072 public AdjudicationComponent() { 1073 super(); 1074 } 1075 1076 /** 1077 * Constructor 1078 */ 1079 public AdjudicationComponent(CodeableConcept category) { 1080 super(); 1081 this.category = category; 1082 } 1083 1084 /** 1085 * @return {@link #category} (A code to indicate the information type of this 1086 * adjudication record. Information types may include the value 1087 * submitted, maximum values or percentages allowed or payable under the 1088 * plan, amounts that: the patient is responsible for in aggregate or 1089 * pertaining to this item; amounts paid by other coverages; and, the 1090 * benefit payable for this item.) 1091 */ 1092 public CodeableConcept getCategory() { 1093 if (this.category == null) 1094 if (Configuration.errorOnAutoCreate()) 1095 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 1096 else if (Configuration.doAutoCreate()) 1097 this.category = new CodeableConcept(); // cc 1098 return this.category; 1099 } 1100 1101 public boolean hasCategory() { 1102 return this.category != null && !this.category.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #category} (A code to indicate the information type of 1107 * this adjudication record. Information types may include the 1108 * value submitted, maximum values or percentages allowed or 1109 * payable under the plan, amounts that: the patient is responsible 1110 * for in aggregate or pertaining to this item; amounts paid by 1111 * other coverages; and, the benefit payable for this item.) 1112 */ 1113 public AdjudicationComponent setCategory(CodeableConcept value) { 1114 this.category = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return {@link #reason} (A code supporting the understanding of the 1120 * adjudication result and explaining variance from expected amount.) 1121 */ 1122 public CodeableConcept getReason() { 1123 if (this.reason == null) 1124 if (Configuration.errorOnAutoCreate()) 1125 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 1126 else if (Configuration.doAutoCreate()) 1127 this.reason = new CodeableConcept(); // cc 1128 return this.reason; 1129 } 1130 1131 public boolean hasReason() { 1132 return this.reason != null && !this.reason.isEmpty(); 1133 } 1134 1135 /** 1136 * @param value {@link #reason} (A code supporting the understanding of the 1137 * adjudication result and explaining variance from expected 1138 * amount.) 1139 */ 1140 public AdjudicationComponent setReason(CodeableConcept value) { 1141 this.reason = value; 1142 return this; 1143 } 1144 1145 /** 1146 * @return {@link #amount} (Monetary amount associated with the category.) 1147 */ 1148 public Money getAmount() { 1149 if (this.amount == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 1152 else if (Configuration.doAutoCreate()) 1153 this.amount = new Money(); // cc 1154 return this.amount; 1155 } 1156 1157 public boolean hasAmount() { 1158 return this.amount != null && !this.amount.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #amount} (Monetary amount associated with the category.) 1163 */ 1164 public AdjudicationComponent setAmount(Money value) { 1165 this.amount = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return {@link #value} (A non-monetary value associated with the category. 1171 * Mutually exclusive to the amount element above.). This is the 1172 * underlying object with id, value and extensions. The accessor 1173 * "getValue" gives direct access to the value 1174 */ 1175 public DecimalType getValueElement() { 1176 if (this.value == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create AdjudicationComponent.value"); 1179 else if (Configuration.doAutoCreate()) 1180 this.value = new DecimalType(); // bb 1181 return this.value; 1182 } 1183 1184 public boolean hasValueElement() { 1185 return this.value != null && !this.value.isEmpty(); 1186 } 1187 1188 public boolean hasValue() { 1189 return this.value != null && !this.value.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #value} (A non-monetary value associated with the 1194 * category. Mutually exclusive to the amount element above.). This 1195 * is the underlying object with id, value and extensions. The 1196 * accessor "getValue" gives direct access to the value 1197 */ 1198 public AdjudicationComponent setValueElement(DecimalType value) { 1199 this.value = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return A non-monetary value associated with the category. Mutually exclusive 1205 * to the amount element above. 1206 */ 1207 public BigDecimal getValue() { 1208 return this.value == null ? null : this.value.getValue(); 1209 } 1210 1211 /** 1212 * @param value A non-monetary value associated with the category. Mutually 1213 * exclusive to the amount element above. 1214 */ 1215 public AdjudicationComponent setValue(BigDecimal value) { 1216 if (value == null) 1217 this.value = null; 1218 else { 1219 if (this.value == null) 1220 this.value = new DecimalType(); 1221 this.value.setValue(value); 1222 } 1223 return this; 1224 } 1225 1226 /** 1227 * @param value A non-monetary value associated with the category. Mutually 1228 * exclusive to the amount element above. 1229 */ 1230 public AdjudicationComponent setValue(long value) { 1231 this.value = new DecimalType(); 1232 this.value.setValue(value); 1233 return this; 1234 } 1235 1236 /** 1237 * @param value A non-monetary value associated with the category. Mutually 1238 * exclusive to the amount element above. 1239 */ 1240 public AdjudicationComponent setValue(double value) { 1241 this.value = new DecimalType(); 1242 this.value.setValue(value); 1243 return this; 1244 } 1245 1246 protected void listChildren(List<Property> children) { 1247 super.listChildren(children); 1248 children.add(new Property("category", "CodeableConcept", 1249 "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.", 1250 0, 1, category)); 1251 children.add(new Property("reason", "CodeableConcept", 1252 "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 1253 0, 1, reason)); 1254 children.add(new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount)); 1255 children.add(new Property("value", "decimal", 1256 "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, 1257 value)); 1258 } 1259 1260 @Override 1261 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1262 switch (_hash) { 1263 case 50511102: 1264 /* category */ return new Property("category", "CodeableConcept", 1265 "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.", 1266 0, 1, category); 1267 case -934964668: 1268 /* reason */ return new Property("reason", "CodeableConcept", 1269 "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 1270 0, 1, reason); 1271 case -1413853096: 1272 /* amount */ return new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, 1273 amount); 1274 case 111972721: 1275 /* value */ return new Property("value", "decimal", 1276 "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, 1277 value); 1278 default: 1279 return super.getNamedProperty(_hash, _name, _checkValid); 1280 } 1281 1282 } 1283 1284 @Override 1285 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1286 switch (hash) { 1287 case 50511102: 1288 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1289 case -934964668: 1290 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 1291 case -1413853096: 1292 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 1293 case 111972721: 1294 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 1295 default: 1296 return super.getProperty(hash, name, checkValid); 1297 } 1298 1299 } 1300 1301 @Override 1302 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1303 switch (hash) { 1304 case 50511102: // category 1305 this.category = castToCodeableConcept(value); // CodeableConcept 1306 return value; 1307 case -934964668: // reason 1308 this.reason = castToCodeableConcept(value); // CodeableConcept 1309 return value; 1310 case -1413853096: // amount 1311 this.amount = castToMoney(value); // Money 1312 return value; 1313 case 111972721: // value 1314 this.value = castToDecimal(value); // DecimalType 1315 return value; 1316 default: 1317 return super.setProperty(hash, name, value); 1318 } 1319 1320 } 1321 1322 @Override 1323 public Base setProperty(String name, Base value) throws FHIRException { 1324 if (name.equals("category")) { 1325 this.category = castToCodeableConcept(value); // CodeableConcept 1326 } else if (name.equals("reason")) { 1327 this.reason = castToCodeableConcept(value); // CodeableConcept 1328 } else if (name.equals("amount")) { 1329 this.amount = castToMoney(value); // Money 1330 } else if (name.equals("value")) { 1331 this.value = castToDecimal(value); // DecimalType 1332 } else 1333 return super.setProperty(name, value); 1334 return value; 1335 } 1336 1337 @Override 1338 public void removeChild(String name, Base value) throws FHIRException { 1339 if (name.equals("category")) { 1340 this.category = null; 1341 } else if (name.equals("reason")) { 1342 this.reason = null; 1343 } else if (name.equals("amount")) { 1344 this.amount = null; 1345 } else if (name.equals("value")) { 1346 this.value = null; 1347 } else 1348 super.removeChild(name, value); 1349 1350 } 1351 1352 @Override 1353 public Base makeProperty(int hash, String name) throws FHIRException { 1354 switch (hash) { 1355 case 50511102: 1356 return getCategory(); 1357 case -934964668: 1358 return getReason(); 1359 case -1413853096: 1360 return getAmount(); 1361 case 111972721: 1362 return getValueElement(); 1363 default: 1364 return super.makeProperty(hash, name); 1365 } 1366 1367 } 1368 1369 @Override 1370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1371 switch (hash) { 1372 case 50511102: 1373 /* category */ return new String[] { "CodeableConcept" }; 1374 case -934964668: 1375 /* reason */ return new String[] { "CodeableConcept" }; 1376 case -1413853096: 1377 /* amount */ return new String[] { "Money" }; 1378 case 111972721: 1379 /* value */ return new String[] { "decimal" }; 1380 default: 1381 return super.getTypesForProperty(hash, name); 1382 } 1383 1384 } 1385 1386 @Override 1387 public Base addChild(String name) throws FHIRException { 1388 if (name.equals("category")) { 1389 this.category = new CodeableConcept(); 1390 return this.category; 1391 } else if (name.equals("reason")) { 1392 this.reason = new CodeableConcept(); 1393 return this.reason; 1394 } else if (name.equals("amount")) { 1395 this.amount = new Money(); 1396 return this.amount; 1397 } else if (name.equals("value")) { 1398 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 1399 } else 1400 return super.addChild(name); 1401 } 1402 1403 public AdjudicationComponent copy() { 1404 AdjudicationComponent dst = new AdjudicationComponent(); 1405 copyValues(dst); 1406 return dst; 1407 } 1408 1409 public void copyValues(AdjudicationComponent dst) { 1410 super.copyValues(dst); 1411 dst.category = category == null ? null : category.copy(); 1412 dst.reason = reason == null ? null : reason.copy(); 1413 dst.amount = amount == null ? null : amount.copy(); 1414 dst.value = value == null ? null : value.copy(); 1415 } 1416 1417 @Override 1418 public boolean equalsDeep(Base other_) { 1419 if (!super.equalsDeep(other_)) 1420 return false; 1421 if (!(other_ instanceof AdjudicationComponent)) 1422 return false; 1423 AdjudicationComponent o = (AdjudicationComponent) other_; 1424 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) 1425 && compareDeep(amount, o.amount, true) && compareDeep(value, o.value, true); 1426 } 1427 1428 @Override 1429 public boolean equalsShallow(Base other_) { 1430 if (!super.equalsShallow(other_)) 1431 return false; 1432 if (!(other_ instanceof AdjudicationComponent)) 1433 return false; 1434 AdjudicationComponent o = (AdjudicationComponent) other_; 1435 return compareValues(value, o.value, true); 1436 } 1437 1438 public boolean isEmpty() { 1439 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount, value); 1440 } 1441 1442 public String fhirType() { 1443 return "ClaimResponse.item.adjudication"; 1444 1445 } 1446 1447 } 1448 1449 @Block() 1450 public static class ItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 1451 /** 1452 * A number to uniquely reference the claim detail entry. 1453 */ 1454 @Child(name = "detailSequence", type = { 1455 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1456 @Description(shortDefinition = "Claim detail instance identifier", formalDefinition = "A number to uniquely reference the claim detail entry.") 1457 protected PositiveIntType detailSequence; 1458 1459 /** 1460 * The numbers associated with notes below which apply to the adjudication of 1461 * this item. 1462 */ 1463 @Child(name = "noteNumber", type = { 1464 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1465 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 1466 protected List<PositiveIntType> noteNumber; 1467 1468 /** 1469 * The adjudication results. 1470 */ 1471 @Child(name = "adjudication", type = { 1472 AdjudicationComponent.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1473 @Description(shortDefinition = "Detail level adjudication details", formalDefinition = "The adjudication results.") 1474 protected List<AdjudicationComponent> adjudication; 1475 1476 /** 1477 * A sub-detail adjudication of a simple product or service. 1478 */ 1479 @Child(name = "subDetail", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1480 @Description(shortDefinition = "Adjudication for claim sub-details", formalDefinition = "A sub-detail adjudication of a simple product or service.") 1481 protected List<SubDetailComponent> subDetail; 1482 1483 private static final long serialVersionUID = 1066636111L; 1484 1485 /** 1486 * Constructor 1487 */ 1488 public ItemDetailComponent() { 1489 super(); 1490 } 1491 1492 /** 1493 * Constructor 1494 */ 1495 public ItemDetailComponent(PositiveIntType detailSequence) { 1496 super(); 1497 this.detailSequence = detailSequence; 1498 } 1499 1500 /** 1501 * @return {@link #detailSequence} (A number to uniquely reference the claim 1502 * detail entry.). This is the underlying object with id, value and 1503 * extensions. The accessor "getDetailSequence" gives direct access to 1504 * the value 1505 */ 1506 public PositiveIntType getDetailSequenceElement() { 1507 if (this.detailSequence == null) 1508 if (Configuration.errorOnAutoCreate()) 1509 throw new Error("Attempt to auto-create ItemDetailComponent.detailSequence"); 1510 else if (Configuration.doAutoCreate()) 1511 this.detailSequence = new PositiveIntType(); // bb 1512 return this.detailSequence; 1513 } 1514 1515 public boolean hasDetailSequenceElement() { 1516 return this.detailSequence != null && !this.detailSequence.isEmpty(); 1517 } 1518 1519 public boolean hasDetailSequence() { 1520 return this.detailSequence != null && !this.detailSequence.isEmpty(); 1521 } 1522 1523 /** 1524 * @param value {@link #detailSequence} (A number to uniquely reference the 1525 * claim detail entry.). This is the underlying object with id, 1526 * value and extensions. The accessor "getDetailSequence" gives 1527 * direct access to the value 1528 */ 1529 public ItemDetailComponent setDetailSequenceElement(PositiveIntType value) { 1530 this.detailSequence = value; 1531 return this; 1532 } 1533 1534 /** 1535 * @return A number to uniquely reference the claim detail entry. 1536 */ 1537 public int getDetailSequence() { 1538 return this.detailSequence == null || this.detailSequence.isEmpty() ? 0 : this.detailSequence.getValue(); 1539 } 1540 1541 /** 1542 * @param value A number to uniquely reference the claim detail entry. 1543 */ 1544 public ItemDetailComponent setDetailSequence(int value) { 1545 if (this.detailSequence == null) 1546 this.detailSequence = new PositiveIntType(); 1547 this.detailSequence.setValue(value); 1548 return this; 1549 } 1550 1551 /** 1552 * @return {@link #noteNumber} (The numbers associated with notes below which 1553 * apply to the adjudication of this item.) 1554 */ 1555 public List<PositiveIntType> getNoteNumber() { 1556 if (this.noteNumber == null) 1557 this.noteNumber = new ArrayList<PositiveIntType>(); 1558 return this.noteNumber; 1559 } 1560 1561 /** 1562 * @return Returns a reference to <code>this</code> for easy method chaining 1563 */ 1564 public ItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 1565 this.noteNumber = theNoteNumber; 1566 return this; 1567 } 1568 1569 public boolean hasNoteNumber() { 1570 if (this.noteNumber == null) 1571 return false; 1572 for (PositiveIntType item : this.noteNumber) 1573 if (!item.isEmpty()) 1574 return true; 1575 return false; 1576 } 1577 1578 /** 1579 * @return {@link #noteNumber} (The numbers associated with notes below which 1580 * apply to the adjudication of this item.) 1581 */ 1582 public PositiveIntType addNoteNumberElement() {// 2 1583 PositiveIntType t = new PositiveIntType(); 1584 if (this.noteNumber == null) 1585 this.noteNumber = new ArrayList<PositiveIntType>(); 1586 this.noteNumber.add(t); 1587 return t; 1588 } 1589 1590 /** 1591 * @param value {@link #noteNumber} (The numbers associated with notes below 1592 * which apply to the adjudication of this item.) 1593 */ 1594 public ItemDetailComponent addNoteNumber(int value) { // 1 1595 PositiveIntType t = new PositiveIntType(); 1596 t.setValue(value); 1597 if (this.noteNumber == null) 1598 this.noteNumber = new ArrayList<PositiveIntType>(); 1599 this.noteNumber.add(t); 1600 return this; 1601 } 1602 1603 /** 1604 * @param value {@link #noteNumber} (The numbers associated with notes below 1605 * which apply to the adjudication of this item.) 1606 */ 1607 public boolean hasNoteNumber(int value) { 1608 if (this.noteNumber == null) 1609 return false; 1610 for (PositiveIntType v : this.noteNumber) 1611 if (v.getValue().equals(value)) // positiveInt 1612 return true; 1613 return false; 1614 } 1615 1616 /** 1617 * @return {@link #adjudication} (The adjudication results.) 1618 */ 1619 public List<AdjudicationComponent> getAdjudication() { 1620 if (this.adjudication == null) 1621 this.adjudication = new ArrayList<AdjudicationComponent>(); 1622 return this.adjudication; 1623 } 1624 1625 /** 1626 * @return Returns a reference to <code>this</code> for easy method chaining 1627 */ 1628 public ItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 1629 this.adjudication = theAdjudication; 1630 return this; 1631 } 1632 1633 public boolean hasAdjudication() { 1634 if (this.adjudication == null) 1635 return false; 1636 for (AdjudicationComponent item : this.adjudication) 1637 if (!item.isEmpty()) 1638 return true; 1639 return false; 1640 } 1641 1642 public AdjudicationComponent addAdjudication() { // 3 1643 AdjudicationComponent t = new AdjudicationComponent(); 1644 if (this.adjudication == null) 1645 this.adjudication = new ArrayList<AdjudicationComponent>(); 1646 this.adjudication.add(t); 1647 return t; 1648 } 1649 1650 public ItemDetailComponent addAdjudication(AdjudicationComponent t) { // 3 1651 if (t == null) 1652 return this; 1653 if (this.adjudication == null) 1654 this.adjudication = new ArrayList<AdjudicationComponent>(); 1655 this.adjudication.add(t); 1656 return this; 1657 } 1658 1659 /** 1660 * @return The first repetition of repeating field {@link #adjudication}, 1661 * creating it if it does not already exist 1662 */ 1663 public AdjudicationComponent getAdjudicationFirstRep() { 1664 if (getAdjudication().isEmpty()) { 1665 addAdjudication(); 1666 } 1667 return getAdjudication().get(0); 1668 } 1669 1670 /** 1671 * @return {@link #subDetail} (A sub-detail adjudication of a simple product or 1672 * service.) 1673 */ 1674 public List<SubDetailComponent> getSubDetail() { 1675 if (this.subDetail == null) 1676 this.subDetail = new ArrayList<SubDetailComponent>(); 1677 return this.subDetail; 1678 } 1679 1680 /** 1681 * @return Returns a reference to <code>this</code> for easy method chaining 1682 */ 1683 public ItemDetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 1684 this.subDetail = theSubDetail; 1685 return this; 1686 } 1687 1688 public boolean hasSubDetail() { 1689 if (this.subDetail == null) 1690 return false; 1691 for (SubDetailComponent item : this.subDetail) 1692 if (!item.isEmpty()) 1693 return true; 1694 return false; 1695 } 1696 1697 public SubDetailComponent addSubDetail() { // 3 1698 SubDetailComponent t = new SubDetailComponent(); 1699 if (this.subDetail == null) 1700 this.subDetail = new ArrayList<SubDetailComponent>(); 1701 this.subDetail.add(t); 1702 return t; 1703 } 1704 1705 public ItemDetailComponent addSubDetail(SubDetailComponent t) { // 3 1706 if (t == null) 1707 return this; 1708 if (this.subDetail == null) 1709 this.subDetail = new ArrayList<SubDetailComponent>(); 1710 this.subDetail.add(t); 1711 return this; 1712 } 1713 1714 /** 1715 * @return The first repetition of repeating field {@link #subDetail}, creating 1716 * it if it does not already exist 1717 */ 1718 public SubDetailComponent getSubDetailFirstRep() { 1719 if (getSubDetail().isEmpty()) { 1720 addSubDetail(); 1721 } 1722 return getSubDetail().get(0); 1723 } 1724 1725 protected void listChildren(List<Property> children) { 1726 super.listChildren(children); 1727 children.add(new Property("detailSequence", "positiveInt", 1728 "A number to uniquely reference the claim detail entry.", 0, 1, detailSequence)); 1729 children.add(new Property("noteNumber", "positiveInt", 1730 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 1731 java.lang.Integer.MAX_VALUE, noteNumber)); 1732 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 1733 java.lang.Integer.MAX_VALUE, adjudication)); 1734 children.add(new Property("subDetail", "", "A sub-detail adjudication of a simple product or service.", 0, 1735 java.lang.Integer.MAX_VALUE, subDetail)); 1736 } 1737 1738 @Override 1739 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1740 switch (_hash) { 1741 case 1321472818: 1742 /* detailSequence */ return new Property("detailSequence", "positiveInt", 1743 "A number to uniquely reference the claim detail entry.", 0, 1, detailSequence); 1744 case -1110033957: 1745 /* noteNumber */ return new Property("noteNumber", "positiveInt", 1746 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 1747 java.lang.Integer.MAX_VALUE, noteNumber); 1748 case -231349275: 1749 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 1750 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 1751 case -828829007: 1752 /* subDetail */ return new Property("subDetail", "", 1753 "A sub-detail adjudication of a simple product or service.", 0, java.lang.Integer.MAX_VALUE, subDetail); 1754 default: 1755 return super.getNamedProperty(_hash, _name, _checkValid); 1756 } 1757 1758 } 1759 1760 @Override 1761 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1762 switch (hash) { 1763 case 1321472818: 1764 /* detailSequence */ return this.detailSequence == null ? new Base[0] : new Base[] { this.detailSequence }; // PositiveIntType 1765 case -1110033957: 1766 /* noteNumber */ return this.noteNumber == null ? new Base[0] 1767 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 1768 case -231349275: 1769 /* adjudication */ return this.adjudication == null ? new Base[0] 1770 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 1771 case -828829007: 1772 /* subDetail */ return this.subDetail == null ? new Base[0] 1773 : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 1774 default: 1775 return super.getProperty(hash, name, checkValid); 1776 } 1777 1778 } 1779 1780 @Override 1781 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1782 switch (hash) { 1783 case 1321472818: // detailSequence 1784 this.detailSequence = castToPositiveInt(value); // PositiveIntType 1785 return value; 1786 case -1110033957: // noteNumber 1787 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 1788 return value; 1789 case -231349275: // adjudication 1790 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 1791 return value; 1792 case -828829007: // subDetail 1793 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 1794 return value; 1795 default: 1796 return super.setProperty(hash, name, value); 1797 } 1798 1799 } 1800 1801 @Override 1802 public Base setProperty(String name, Base value) throws FHIRException { 1803 if (name.equals("detailSequence")) { 1804 this.detailSequence = castToPositiveInt(value); // PositiveIntType 1805 } else if (name.equals("noteNumber")) { 1806 this.getNoteNumber().add(castToPositiveInt(value)); 1807 } else if (name.equals("adjudication")) { 1808 this.getAdjudication().add((AdjudicationComponent) value); 1809 } else if (name.equals("subDetail")) { 1810 this.getSubDetail().add((SubDetailComponent) value); 1811 } else 1812 return super.setProperty(name, value); 1813 return value; 1814 } 1815 1816 @Override 1817 public void removeChild(String name, Base value) throws FHIRException { 1818 if (name.equals("detailSequence")) { 1819 this.detailSequence = null; 1820 } else if (name.equals("noteNumber")) { 1821 this.getNoteNumber().remove(castToPositiveInt(value)); 1822 } else if (name.equals("adjudication")) { 1823 this.getAdjudication().remove((AdjudicationComponent) value); 1824 } else if (name.equals("subDetail")) { 1825 this.getSubDetail().remove((SubDetailComponent) value); 1826 } else 1827 super.removeChild(name, value); 1828 1829 } 1830 1831 @Override 1832 public Base makeProperty(int hash, String name) throws FHIRException { 1833 switch (hash) { 1834 case 1321472818: 1835 return getDetailSequenceElement(); 1836 case -1110033957: 1837 return addNoteNumberElement(); 1838 case -231349275: 1839 return addAdjudication(); 1840 case -828829007: 1841 return addSubDetail(); 1842 default: 1843 return super.makeProperty(hash, name); 1844 } 1845 1846 } 1847 1848 @Override 1849 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1850 switch (hash) { 1851 case 1321472818: 1852 /* detailSequence */ return new String[] { "positiveInt" }; 1853 case -1110033957: 1854 /* noteNumber */ return new String[] { "positiveInt" }; 1855 case -231349275: 1856 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 1857 case -828829007: 1858 /* subDetail */ return new String[] {}; 1859 default: 1860 return super.getTypesForProperty(hash, name); 1861 } 1862 1863 } 1864 1865 @Override 1866 public Base addChild(String name) throws FHIRException { 1867 if (name.equals("detailSequence")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequence"); 1869 } else if (name.equals("noteNumber")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 1871 } else if (name.equals("adjudication")) { 1872 return addAdjudication(); 1873 } else if (name.equals("subDetail")) { 1874 return addSubDetail(); 1875 } else 1876 return super.addChild(name); 1877 } 1878 1879 public ItemDetailComponent copy() { 1880 ItemDetailComponent dst = new ItemDetailComponent(); 1881 copyValues(dst); 1882 return dst; 1883 } 1884 1885 public void copyValues(ItemDetailComponent dst) { 1886 super.copyValues(dst); 1887 dst.detailSequence = detailSequence == null ? null : detailSequence.copy(); 1888 if (noteNumber != null) { 1889 dst.noteNumber = new ArrayList<PositiveIntType>(); 1890 for (PositiveIntType i : noteNumber) 1891 dst.noteNumber.add(i.copy()); 1892 } 1893 ; 1894 if (adjudication != null) { 1895 dst.adjudication = new ArrayList<AdjudicationComponent>(); 1896 for (AdjudicationComponent i : adjudication) 1897 dst.adjudication.add(i.copy()); 1898 } 1899 ; 1900 if (subDetail != null) { 1901 dst.subDetail = new ArrayList<SubDetailComponent>(); 1902 for (SubDetailComponent i : subDetail) 1903 dst.subDetail.add(i.copy()); 1904 } 1905 ; 1906 } 1907 1908 @Override 1909 public boolean equalsDeep(Base other_) { 1910 if (!super.equalsDeep(other_)) 1911 return false; 1912 if (!(other_ instanceof ItemDetailComponent)) 1913 return false; 1914 ItemDetailComponent o = (ItemDetailComponent) other_; 1915 return compareDeep(detailSequence, o.detailSequence, true) && compareDeep(noteNumber, o.noteNumber, true) 1916 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true); 1917 } 1918 1919 @Override 1920 public boolean equalsShallow(Base other_) { 1921 if (!super.equalsShallow(other_)) 1922 return false; 1923 if (!(other_ instanceof ItemDetailComponent)) 1924 return false; 1925 ItemDetailComponent o = (ItemDetailComponent) other_; 1926 return compareValues(detailSequence, o.detailSequence, true) && compareValues(noteNumber, o.noteNumber, true); 1927 } 1928 1929 public boolean isEmpty() { 1930 return super.isEmpty() 1931 && ca.uhn.fhir.util.ElementUtil.isEmpty(detailSequence, noteNumber, adjudication, subDetail); 1932 } 1933 1934 public String fhirType() { 1935 return "ClaimResponse.item.detail"; 1936 1937 } 1938 1939 } 1940 1941 @Block() 1942 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 1943 /** 1944 * A number to uniquely reference the claim sub-detail entry. 1945 */ 1946 @Child(name = "subDetailSequence", type = { 1947 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1948 @Description(shortDefinition = "Claim sub-detail instance identifier", formalDefinition = "A number to uniquely reference the claim sub-detail entry.") 1949 protected PositiveIntType subDetailSequence; 1950 1951 /** 1952 * The numbers associated with notes below which apply to the adjudication of 1953 * this item. 1954 */ 1955 @Child(name = "noteNumber", type = { 1956 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1957 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 1958 protected List<PositiveIntType> noteNumber; 1959 1960 /** 1961 * The adjudication results. 1962 */ 1963 @Child(name = "adjudication", type = { 1964 AdjudicationComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1965 @Description(shortDefinition = "Subdetail level adjudication details", formalDefinition = "The adjudication results.") 1966 protected List<AdjudicationComponent> adjudication; 1967 1968 private static final long serialVersionUID = -1083724362L; 1969 1970 /** 1971 * Constructor 1972 */ 1973 public SubDetailComponent() { 1974 super(); 1975 } 1976 1977 /** 1978 * Constructor 1979 */ 1980 public SubDetailComponent(PositiveIntType subDetailSequence) { 1981 super(); 1982 this.subDetailSequence = subDetailSequence; 1983 } 1984 1985 /** 1986 * @return {@link #subDetailSequence} (A number to uniquely reference the claim 1987 * sub-detail entry.). This is the underlying object with id, value and 1988 * extensions. The accessor "getSubDetailSequence" gives direct access 1989 * to the value 1990 */ 1991 public PositiveIntType getSubDetailSequenceElement() { 1992 if (this.subDetailSequence == null) 1993 if (Configuration.errorOnAutoCreate()) 1994 throw new Error("Attempt to auto-create SubDetailComponent.subDetailSequence"); 1995 else if (Configuration.doAutoCreate()) 1996 this.subDetailSequence = new PositiveIntType(); // bb 1997 return this.subDetailSequence; 1998 } 1999 2000 public boolean hasSubDetailSequenceElement() { 2001 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 2002 } 2003 2004 public boolean hasSubDetailSequence() { 2005 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 2006 } 2007 2008 /** 2009 * @param value {@link #subDetailSequence} (A number to uniquely reference the 2010 * claim sub-detail entry.). This is the underlying object with id, 2011 * value and extensions. The accessor "getSubDetailSequence" gives 2012 * direct access to the value 2013 */ 2014 public SubDetailComponent setSubDetailSequenceElement(PositiveIntType value) { 2015 this.subDetailSequence = value; 2016 return this; 2017 } 2018 2019 /** 2020 * @return A number to uniquely reference the claim sub-detail entry. 2021 */ 2022 public int getSubDetailSequence() { 2023 return this.subDetailSequence == null || this.subDetailSequence.isEmpty() ? 0 : this.subDetailSequence.getValue(); 2024 } 2025 2026 /** 2027 * @param value A number to uniquely reference the claim sub-detail entry. 2028 */ 2029 public SubDetailComponent setSubDetailSequence(int value) { 2030 if (this.subDetailSequence == null) 2031 this.subDetailSequence = new PositiveIntType(); 2032 this.subDetailSequence.setValue(value); 2033 return this; 2034 } 2035 2036 /** 2037 * @return {@link #noteNumber} (The numbers associated with notes below which 2038 * apply to the adjudication of this item.) 2039 */ 2040 public List<PositiveIntType> getNoteNumber() { 2041 if (this.noteNumber == null) 2042 this.noteNumber = new ArrayList<PositiveIntType>(); 2043 return this.noteNumber; 2044 } 2045 2046 /** 2047 * @return Returns a reference to <code>this</code> for easy method chaining 2048 */ 2049 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 2050 this.noteNumber = theNoteNumber; 2051 return this; 2052 } 2053 2054 public boolean hasNoteNumber() { 2055 if (this.noteNumber == null) 2056 return false; 2057 for (PositiveIntType item : this.noteNumber) 2058 if (!item.isEmpty()) 2059 return true; 2060 return false; 2061 } 2062 2063 /** 2064 * @return {@link #noteNumber} (The numbers associated with notes below which 2065 * apply to the adjudication of this item.) 2066 */ 2067 public PositiveIntType addNoteNumberElement() {// 2 2068 PositiveIntType t = new PositiveIntType(); 2069 if (this.noteNumber == null) 2070 this.noteNumber = new ArrayList<PositiveIntType>(); 2071 this.noteNumber.add(t); 2072 return t; 2073 } 2074 2075 /** 2076 * @param value {@link #noteNumber} (The numbers associated with notes below 2077 * which apply to the adjudication of this item.) 2078 */ 2079 public SubDetailComponent addNoteNumber(int value) { // 1 2080 PositiveIntType t = new PositiveIntType(); 2081 t.setValue(value); 2082 if (this.noteNumber == null) 2083 this.noteNumber = new ArrayList<PositiveIntType>(); 2084 this.noteNumber.add(t); 2085 return this; 2086 } 2087 2088 /** 2089 * @param value {@link #noteNumber} (The numbers associated with notes below 2090 * which apply to the adjudication of this item.) 2091 */ 2092 public boolean hasNoteNumber(int value) { 2093 if (this.noteNumber == null) 2094 return false; 2095 for (PositiveIntType v : this.noteNumber) 2096 if (v.getValue().equals(value)) // positiveInt 2097 return true; 2098 return false; 2099 } 2100 2101 /** 2102 * @return {@link #adjudication} (The adjudication results.) 2103 */ 2104 public List<AdjudicationComponent> getAdjudication() { 2105 if (this.adjudication == null) 2106 this.adjudication = new ArrayList<AdjudicationComponent>(); 2107 return this.adjudication; 2108 } 2109 2110 /** 2111 * @return Returns a reference to <code>this</code> for easy method chaining 2112 */ 2113 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 2114 this.adjudication = theAdjudication; 2115 return this; 2116 } 2117 2118 public boolean hasAdjudication() { 2119 if (this.adjudication == null) 2120 return false; 2121 for (AdjudicationComponent item : this.adjudication) 2122 if (!item.isEmpty()) 2123 return true; 2124 return false; 2125 } 2126 2127 public AdjudicationComponent addAdjudication() { // 3 2128 AdjudicationComponent t = new AdjudicationComponent(); 2129 if (this.adjudication == null) 2130 this.adjudication = new ArrayList<AdjudicationComponent>(); 2131 this.adjudication.add(t); 2132 return t; 2133 } 2134 2135 public SubDetailComponent addAdjudication(AdjudicationComponent t) { // 3 2136 if (t == null) 2137 return this; 2138 if (this.adjudication == null) 2139 this.adjudication = new ArrayList<AdjudicationComponent>(); 2140 this.adjudication.add(t); 2141 return this; 2142 } 2143 2144 /** 2145 * @return The first repetition of repeating field {@link #adjudication}, 2146 * creating it if it does not already exist 2147 */ 2148 public AdjudicationComponent getAdjudicationFirstRep() { 2149 if (getAdjudication().isEmpty()) { 2150 addAdjudication(); 2151 } 2152 return getAdjudication().get(0); 2153 } 2154 2155 protected void listChildren(List<Property> children) { 2156 super.listChildren(children); 2157 children.add(new Property("subDetailSequence", "positiveInt", 2158 "A number to uniquely reference the claim sub-detail entry.", 0, 1, subDetailSequence)); 2159 children.add(new Property("noteNumber", "positiveInt", 2160 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 2161 java.lang.Integer.MAX_VALUE, noteNumber)); 2162 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 2163 java.lang.Integer.MAX_VALUE, adjudication)); 2164 } 2165 2166 @Override 2167 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2168 switch (_hash) { 2169 case -855462510: 2170 /* subDetailSequence */ return new Property("subDetailSequence", "positiveInt", 2171 "A number to uniquely reference the claim sub-detail entry.", 0, 1, subDetailSequence); 2172 case -1110033957: 2173 /* noteNumber */ return new Property("noteNumber", "positiveInt", 2174 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 2175 java.lang.Integer.MAX_VALUE, noteNumber); 2176 case -231349275: 2177 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 2178 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 2179 default: 2180 return super.getNamedProperty(_hash, _name, _checkValid); 2181 } 2182 2183 } 2184 2185 @Override 2186 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2187 switch (hash) { 2188 case -855462510: 2189 /* subDetailSequence */ return this.subDetailSequence == null ? new Base[0] 2190 : new Base[] { this.subDetailSequence }; // PositiveIntType 2191 case -1110033957: 2192 /* noteNumber */ return this.noteNumber == null ? new Base[0] 2193 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 2194 case -231349275: 2195 /* adjudication */ return this.adjudication == null ? new Base[0] 2196 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 2197 default: 2198 return super.getProperty(hash, name, checkValid); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2205 switch (hash) { 2206 case -855462510: // subDetailSequence 2207 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 2208 return value; 2209 case -1110033957: // noteNumber 2210 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 2211 return value; 2212 case -231349275: // adjudication 2213 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 2214 return value; 2215 default: 2216 return super.setProperty(hash, name, value); 2217 } 2218 2219 } 2220 2221 @Override 2222 public Base setProperty(String name, Base value) throws FHIRException { 2223 if (name.equals("subDetailSequence")) { 2224 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 2225 } else if (name.equals("noteNumber")) { 2226 this.getNoteNumber().add(castToPositiveInt(value)); 2227 } else if (name.equals("adjudication")) { 2228 this.getAdjudication().add((AdjudicationComponent) value); 2229 } else 2230 return super.setProperty(name, value); 2231 return value; 2232 } 2233 2234 @Override 2235 public void removeChild(String name, Base value) throws FHIRException { 2236 if (name.equals("subDetailSequence")) { 2237 this.subDetailSequence = null; 2238 } else if (name.equals("noteNumber")) { 2239 this.getNoteNumber().remove(castToPositiveInt(value)); 2240 } else if (name.equals("adjudication")) { 2241 this.getAdjudication().remove((AdjudicationComponent) value); 2242 } else 2243 super.removeChild(name, value); 2244 2245 } 2246 2247 @Override 2248 public Base makeProperty(int hash, String name) throws FHIRException { 2249 switch (hash) { 2250 case -855462510: 2251 return getSubDetailSequenceElement(); 2252 case -1110033957: 2253 return addNoteNumberElement(); 2254 case -231349275: 2255 return addAdjudication(); 2256 default: 2257 return super.makeProperty(hash, name); 2258 } 2259 2260 } 2261 2262 @Override 2263 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2264 switch (hash) { 2265 case -855462510: 2266 /* subDetailSequence */ return new String[] { "positiveInt" }; 2267 case -1110033957: 2268 /* noteNumber */ return new String[] { "positiveInt" }; 2269 case -231349275: 2270 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 2271 default: 2272 return super.getTypesForProperty(hash, name); 2273 } 2274 2275 } 2276 2277 @Override 2278 public Base addChild(String name) throws FHIRException { 2279 if (name.equals("subDetailSequence")) { 2280 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subDetailSequence"); 2281 } else if (name.equals("noteNumber")) { 2282 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 2283 } else if (name.equals("adjudication")) { 2284 return addAdjudication(); 2285 } else 2286 return super.addChild(name); 2287 } 2288 2289 public SubDetailComponent copy() { 2290 SubDetailComponent dst = new SubDetailComponent(); 2291 copyValues(dst); 2292 return dst; 2293 } 2294 2295 public void copyValues(SubDetailComponent dst) { 2296 super.copyValues(dst); 2297 dst.subDetailSequence = subDetailSequence == null ? null : subDetailSequence.copy(); 2298 if (noteNumber != null) { 2299 dst.noteNumber = new ArrayList<PositiveIntType>(); 2300 for (PositiveIntType i : noteNumber) 2301 dst.noteNumber.add(i.copy()); 2302 } 2303 ; 2304 if (adjudication != null) { 2305 dst.adjudication = new ArrayList<AdjudicationComponent>(); 2306 for (AdjudicationComponent i : adjudication) 2307 dst.adjudication.add(i.copy()); 2308 } 2309 ; 2310 } 2311 2312 @Override 2313 public boolean equalsDeep(Base other_) { 2314 if (!super.equalsDeep(other_)) 2315 return false; 2316 if (!(other_ instanceof SubDetailComponent)) 2317 return false; 2318 SubDetailComponent o = (SubDetailComponent) other_; 2319 return compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(noteNumber, o.noteNumber, true) 2320 && compareDeep(adjudication, o.adjudication, true); 2321 } 2322 2323 @Override 2324 public boolean equalsShallow(Base other_) { 2325 if (!super.equalsShallow(other_)) 2326 return false; 2327 if (!(other_ instanceof SubDetailComponent)) 2328 return false; 2329 SubDetailComponent o = (SubDetailComponent) other_; 2330 return compareValues(subDetailSequence, o.subDetailSequence, true) 2331 && compareValues(noteNumber, o.noteNumber, true); 2332 } 2333 2334 public boolean isEmpty() { 2335 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subDetailSequence, noteNumber, adjudication); 2336 } 2337 2338 public String fhirType() { 2339 return "ClaimResponse.item.detail.subDetail"; 2340 2341 } 2342 2343 } 2344 2345 @Block() 2346 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 2347 /** 2348 * Claim items which this service line is intended to replace. 2349 */ 2350 @Child(name = "itemSequence", type = { 2351 PositiveIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2352 @Description(shortDefinition = "Item sequence number", formalDefinition = "Claim items which this service line is intended to replace.") 2353 protected List<PositiveIntType> itemSequence; 2354 2355 /** 2356 * The sequence number of the details within the claim item which this line is 2357 * intended to replace. 2358 */ 2359 @Child(name = "detailSequence", type = { 2360 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2361 @Description(shortDefinition = "Detail sequence number", formalDefinition = "The sequence number of the details within the claim item which this line is intended to replace.") 2362 protected List<PositiveIntType> detailSequence; 2363 2364 /** 2365 * The sequence number of the sub-details within the details within the claim 2366 * item which this line is intended to replace. 2367 */ 2368 @Child(name = "subdetailSequence", type = { 2369 PositiveIntType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2370 @Description(shortDefinition = "Subdetail sequence number", formalDefinition = "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.") 2371 protected List<PositiveIntType> subdetailSequence; 2372 2373 /** 2374 * The providers who are authorized for the services rendered to the patient. 2375 */ 2376 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 2377 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2378 @Description(shortDefinition = "Authorized providers", formalDefinition = "The providers who are authorized for the services rendered to the patient.") 2379 protected List<Reference> provider; 2380 /** 2381 * The actual objects that are the target of the reference (The providers who 2382 * are authorized for the services rendered to the patient.) 2383 */ 2384 protected List<Resource> providerTarget; 2385 2386 /** 2387 * When the value is a group code then this item collects a set of related claim 2388 * details, otherwise this contains the product, service, drug or other billing 2389 * code for the item. 2390 */ 2391 @Child(name = "productOrService", type = { 2392 CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 2393 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 2394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 2395 protected CodeableConcept productOrService; 2396 2397 /** 2398 * Item typification or modifiers codes to convey additional context for the 2399 * product or service. 2400 */ 2401 @Child(name = "modifier", type = { 2402 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2403 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 2404 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 2405 protected List<CodeableConcept> modifier; 2406 2407 /** 2408 * Identifies the program under which this may be recovered. 2409 */ 2410 @Child(name = "programCode", type = { 2411 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2412 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 2413 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 2414 protected List<CodeableConcept> programCode; 2415 2416 /** 2417 * The date or dates when the service or product was supplied, performed or 2418 * completed. 2419 */ 2420 @Child(name = "serviced", type = { DateType.class, 2421 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2422 @Description(shortDefinition = "Date or dates of service or product delivery", formalDefinition = "The date or dates when the service or product was supplied, performed or completed.") 2423 protected Type serviced; 2424 2425 /** 2426 * Where the product or service was provided. 2427 */ 2428 @Child(name = "location", type = { CodeableConcept.class, Address.class, 2429 Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2430 @Description(shortDefinition = "Place of service or where product was supplied", formalDefinition = "Where the product or service was provided.") 2431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-place") 2432 protected Type location; 2433 2434 /** 2435 * The number of repetitions of a service or product. 2436 */ 2437 @Child(name = "quantity", type = { 2438 Quantity.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2439 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 2440 protected Quantity quantity; 2441 2442 /** 2443 * If the item is not a group then this is the fee for the product or service, 2444 * otherwise this is the total of the fees for the details of the group. 2445 */ 2446 @Child(name = "unitPrice", type = { Money.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 2447 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 2448 protected Money unitPrice; 2449 2450 /** 2451 * A real number that represents a multiplier used in determining the overall 2452 * value of services delivered and/or goods received. The concept of a Factor 2453 * allows for a discount or surcharge multiplier to be applied to a monetary 2454 * amount. 2455 */ 2456 @Child(name = "factor", type = { 2457 DecimalType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2458 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 2459 protected DecimalType factor; 2460 2461 /** 2462 * The quantity times the unit price for an additional service or product or 2463 * charge. 2464 */ 2465 @Child(name = "net", type = { Money.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2466 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 2467 protected Money net; 2468 2469 /** 2470 * Physical service site on the patient (limb, tooth, etc.). 2471 */ 2472 @Child(name = "bodySite", type = { 2473 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2474 @Description(shortDefinition = "Anatomical location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 2475 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/tooth") 2476 protected CodeableConcept bodySite; 2477 2478 /** 2479 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 2480 */ 2481 @Child(name = "subSite", type = { 2482 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2483 @Description(shortDefinition = "Anatomical sub-location", formalDefinition = "A region or surface of the bodySite, e.g. limb region or tooth surface(s).") 2484 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/surface") 2485 protected List<CodeableConcept> subSite; 2486 2487 /** 2488 * The numbers associated with notes below which apply to the adjudication of 2489 * this item. 2490 */ 2491 @Child(name = "noteNumber", type = { 2492 PositiveIntType.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2493 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 2494 protected List<PositiveIntType> noteNumber; 2495 2496 /** 2497 * The adjudication results. 2498 */ 2499 @Child(name = "adjudication", type = { 2500 AdjudicationComponent.class }, order = 17, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2501 @Description(shortDefinition = "Added items adjudication", formalDefinition = "The adjudication results.") 2502 protected List<AdjudicationComponent> adjudication; 2503 2504 /** 2505 * The second-tier service adjudications for payor added services. 2506 */ 2507 @Child(name = "detail", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2508 @Description(shortDefinition = "Insurer added line details", formalDefinition = "The second-tier service adjudications for payor added services.") 2509 protected List<AddedItemDetailComponent> detail; 2510 2511 private static final long serialVersionUID = -1193747282L; 2512 2513 /** 2514 * Constructor 2515 */ 2516 public AddedItemComponent() { 2517 super(); 2518 } 2519 2520 /** 2521 * Constructor 2522 */ 2523 public AddedItemComponent(CodeableConcept productOrService) { 2524 super(); 2525 this.productOrService = productOrService; 2526 } 2527 2528 /** 2529 * @return {@link #itemSequence} (Claim items which this service line is 2530 * intended to replace.) 2531 */ 2532 public List<PositiveIntType> getItemSequence() { 2533 if (this.itemSequence == null) 2534 this.itemSequence = new ArrayList<PositiveIntType>(); 2535 return this.itemSequence; 2536 } 2537 2538 /** 2539 * @return Returns a reference to <code>this</code> for easy method chaining 2540 */ 2541 public AddedItemComponent setItemSequence(List<PositiveIntType> theItemSequence) { 2542 this.itemSequence = theItemSequence; 2543 return this; 2544 } 2545 2546 public boolean hasItemSequence() { 2547 if (this.itemSequence == null) 2548 return false; 2549 for (PositiveIntType item : this.itemSequence) 2550 if (!item.isEmpty()) 2551 return true; 2552 return false; 2553 } 2554 2555 /** 2556 * @return {@link #itemSequence} (Claim items which this service line is 2557 * intended to replace.) 2558 */ 2559 public PositiveIntType addItemSequenceElement() {// 2 2560 PositiveIntType t = new PositiveIntType(); 2561 if (this.itemSequence == null) 2562 this.itemSequence = new ArrayList<PositiveIntType>(); 2563 this.itemSequence.add(t); 2564 return t; 2565 } 2566 2567 /** 2568 * @param value {@link #itemSequence} (Claim items which this service line is 2569 * intended to replace.) 2570 */ 2571 public AddedItemComponent addItemSequence(int value) { // 1 2572 PositiveIntType t = new PositiveIntType(); 2573 t.setValue(value); 2574 if (this.itemSequence == null) 2575 this.itemSequence = new ArrayList<PositiveIntType>(); 2576 this.itemSequence.add(t); 2577 return this; 2578 } 2579 2580 /** 2581 * @param value {@link #itemSequence} (Claim items which this service line is 2582 * intended to replace.) 2583 */ 2584 public boolean hasItemSequence(int value) { 2585 if (this.itemSequence == null) 2586 return false; 2587 for (PositiveIntType v : this.itemSequence) 2588 if (v.getValue().equals(value)) // positiveInt 2589 return true; 2590 return false; 2591 } 2592 2593 /** 2594 * @return {@link #detailSequence} (The sequence number of the details within 2595 * the claim item which this line is intended to replace.) 2596 */ 2597 public List<PositiveIntType> getDetailSequence() { 2598 if (this.detailSequence == null) 2599 this.detailSequence = new ArrayList<PositiveIntType>(); 2600 return this.detailSequence; 2601 } 2602 2603 /** 2604 * @return Returns a reference to <code>this</code> for easy method chaining 2605 */ 2606 public AddedItemComponent setDetailSequence(List<PositiveIntType> theDetailSequence) { 2607 this.detailSequence = theDetailSequence; 2608 return this; 2609 } 2610 2611 public boolean hasDetailSequence() { 2612 if (this.detailSequence == null) 2613 return false; 2614 for (PositiveIntType item : this.detailSequence) 2615 if (!item.isEmpty()) 2616 return true; 2617 return false; 2618 } 2619 2620 /** 2621 * @return {@link #detailSequence} (The sequence number of the details within 2622 * the claim item which this line is intended to replace.) 2623 */ 2624 public PositiveIntType addDetailSequenceElement() {// 2 2625 PositiveIntType t = new PositiveIntType(); 2626 if (this.detailSequence == null) 2627 this.detailSequence = new ArrayList<PositiveIntType>(); 2628 this.detailSequence.add(t); 2629 return t; 2630 } 2631 2632 /** 2633 * @param value {@link #detailSequence} (The sequence number of the details 2634 * within the claim item which this line is intended to replace.) 2635 */ 2636 public AddedItemComponent addDetailSequence(int value) { // 1 2637 PositiveIntType t = new PositiveIntType(); 2638 t.setValue(value); 2639 if (this.detailSequence == null) 2640 this.detailSequence = new ArrayList<PositiveIntType>(); 2641 this.detailSequence.add(t); 2642 return this; 2643 } 2644 2645 /** 2646 * @param value {@link #detailSequence} (The sequence number of the details 2647 * within the claim item which this line is intended to replace.) 2648 */ 2649 public boolean hasDetailSequence(int value) { 2650 if (this.detailSequence == null) 2651 return false; 2652 for (PositiveIntType v : this.detailSequence) 2653 if (v.getValue().equals(value)) // positiveInt 2654 return true; 2655 return false; 2656 } 2657 2658 /** 2659 * @return {@link #subdetailSequence} (The sequence number of the sub-details 2660 * within the details within the claim item which this line is intended 2661 * to replace.) 2662 */ 2663 public List<PositiveIntType> getSubdetailSequence() { 2664 if (this.subdetailSequence == null) 2665 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2666 return this.subdetailSequence; 2667 } 2668 2669 /** 2670 * @return Returns a reference to <code>this</code> for easy method chaining 2671 */ 2672 public AddedItemComponent setSubdetailSequence(List<PositiveIntType> theSubdetailSequence) { 2673 this.subdetailSequence = theSubdetailSequence; 2674 return this; 2675 } 2676 2677 public boolean hasSubdetailSequence() { 2678 if (this.subdetailSequence == null) 2679 return false; 2680 for (PositiveIntType item : this.subdetailSequence) 2681 if (!item.isEmpty()) 2682 return true; 2683 return false; 2684 } 2685 2686 /** 2687 * @return {@link #subdetailSequence} (The sequence number of the sub-details 2688 * within the details within the claim item which this line is intended 2689 * to replace.) 2690 */ 2691 public PositiveIntType addSubdetailSequenceElement() {// 2 2692 PositiveIntType t = new PositiveIntType(); 2693 if (this.subdetailSequence == null) 2694 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2695 this.subdetailSequence.add(t); 2696 return t; 2697 } 2698 2699 /** 2700 * @param value {@link #subdetailSequence} (The sequence number of the 2701 * sub-details within the details within the claim item which this 2702 * line is intended to replace.) 2703 */ 2704 public AddedItemComponent addSubdetailSequence(int value) { // 1 2705 PositiveIntType t = new PositiveIntType(); 2706 t.setValue(value); 2707 if (this.subdetailSequence == null) 2708 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2709 this.subdetailSequence.add(t); 2710 return this; 2711 } 2712 2713 /** 2714 * @param value {@link #subdetailSequence} (The sequence number of the 2715 * sub-details within the details within the claim item which this 2716 * line is intended to replace.) 2717 */ 2718 public boolean hasSubdetailSequence(int value) { 2719 if (this.subdetailSequence == null) 2720 return false; 2721 for (PositiveIntType v : this.subdetailSequence) 2722 if (v.getValue().equals(value)) // positiveInt 2723 return true; 2724 return false; 2725 } 2726 2727 /** 2728 * @return {@link #provider} (The providers who are authorized for the services 2729 * rendered to the patient.) 2730 */ 2731 public List<Reference> getProvider() { 2732 if (this.provider == null) 2733 this.provider = new ArrayList<Reference>(); 2734 return this.provider; 2735 } 2736 2737 /** 2738 * @return Returns a reference to <code>this</code> for easy method chaining 2739 */ 2740 public AddedItemComponent setProvider(List<Reference> theProvider) { 2741 this.provider = theProvider; 2742 return this; 2743 } 2744 2745 public boolean hasProvider() { 2746 if (this.provider == null) 2747 return false; 2748 for (Reference item : this.provider) 2749 if (!item.isEmpty()) 2750 return true; 2751 return false; 2752 } 2753 2754 public Reference addProvider() { // 3 2755 Reference t = new Reference(); 2756 if (this.provider == null) 2757 this.provider = new ArrayList<Reference>(); 2758 this.provider.add(t); 2759 return t; 2760 } 2761 2762 public AddedItemComponent addProvider(Reference t) { // 3 2763 if (t == null) 2764 return this; 2765 if (this.provider == null) 2766 this.provider = new ArrayList<Reference>(); 2767 this.provider.add(t); 2768 return this; 2769 } 2770 2771 /** 2772 * @return The first repetition of repeating field {@link #provider}, creating 2773 * it if it does not already exist 2774 */ 2775 public Reference getProviderFirstRep() { 2776 if (getProvider().isEmpty()) { 2777 addProvider(); 2778 } 2779 return getProvider().get(0); 2780 } 2781 2782 /** 2783 * @return {@link #productOrService} (When the value is a group code then this 2784 * item collects a set of related claim details, otherwise this contains 2785 * the product, service, drug or other billing code for the item.) 2786 */ 2787 public CodeableConcept getProductOrService() { 2788 if (this.productOrService == null) 2789 if (Configuration.errorOnAutoCreate()) 2790 throw new Error("Attempt to auto-create AddedItemComponent.productOrService"); 2791 else if (Configuration.doAutoCreate()) 2792 this.productOrService = new CodeableConcept(); // cc 2793 return this.productOrService; 2794 } 2795 2796 public boolean hasProductOrService() { 2797 return this.productOrService != null && !this.productOrService.isEmpty(); 2798 } 2799 2800 /** 2801 * @param value {@link #productOrService} (When the value is a group code then 2802 * this item collects a set of related claim details, otherwise 2803 * this contains the product, service, drug or other billing code 2804 * for the item.) 2805 */ 2806 public AddedItemComponent setProductOrService(CodeableConcept value) { 2807 this.productOrService = value; 2808 return this; 2809 } 2810 2811 /** 2812 * @return {@link #modifier} (Item typification or modifiers codes to convey 2813 * additional context for the product or service.) 2814 */ 2815 public List<CodeableConcept> getModifier() { 2816 if (this.modifier == null) 2817 this.modifier = new ArrayList<CodeableConcept>(); 2818 return this.modifier; 2819 } 2820 2821 /** 2822 * @return Returns a reference to <code>this</code> for easy method chaining 2823 */ 2824 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 2825 this.modifier = theModifier; 2826 return this; 2827 } 2828 2829 public boolean hasModifier() { 2830 if (this.modifier == null) 2831 return false; 2832 for (CodeableConcept item : this.modifier) 2833 if (!item.isEmpty()) 2834 return true; 2835 return false; 2836 } 2837 2838 public CodeableConcept addModifier() { // 3 2839 CodeableConcept t = new CodeableConcept(); 2840 if (this.modifier == null) 2841 this.modifier = new ArrayList<CodeableConcept>(); 2842 this.modifier.add(t); 2843 return t; 2844 } 2845 2846 public AddedItemComponent addModifier(CodeableConcept t) { // 3 2847 if (t == null) 2848 return this; 2849 if (this.modifier == null) 2850 this.modifier = new ArrayList<CodeableConcept>(); 2851 this.modifier.add(t); 2852 return this; 2853 } 2854 2855 /** 2856 * @return The first repetition of repeating field {@link #modifier}, creating 2857 * it if it does not already exist 2858 */ 2859 public CodeableConcept getModifierFirstRep() { 2860 if (getModifier().isEmpty()) { 2861 addModifier(); 2862 } 2863 return getModifier().get(0); 2864 } 2865 2866 /** 2867 * @return {@link #programCode} (Identifies the program under which this may be 2868 * recovered.) 2869 */ 2870 public List<CodeableConcept> getProgramCode() { 2871 if (this.programCode == null) 2872 this.programCode = new ArrayList<CodeableConcept>(); 2873 return this.programCode; 2874 } 2875 2876 /** 2877 * @return Returns a reference to <code>this</code> for easy method chaining 2878 */ 2879 public AddedItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 2880 this.programCode = theProgramCode; 2881 return this; 2882 } 2883 2884 public boolean hasProgramCode() { 2885 if (this.programCode == null) 2886 return false; 2887 for (CodeableConcept item : this.programCode) 2888 if (!item.isEmpty()) 2889 return true; 2890 return false; 2891 } 2892 2893 public CodeableConcept addProgramCode() { // 3 2894 CodeableConcept t = new CodeableConcept(); 2895 if (this.programCode == null) 2896 this.programCode = new ArrayList<CodeableConcept>(); 2897 this.programCode.add(t); 2898 return t; 2899 } 2900 2901 public AddedItemComponent addProgramCode(CodeableConcept t) { // 3 2902 if (t == null) 2903 return this; 2904 if (this.programCode == null) 2905 this.programCode = new ArrayList<CodeableConcept>(); 2906 this.programCode.add(t); 2907 return this; 2908 } 2909 2910 /** 2911 * @return The first repetition of repeating field {@link #programCode}, 2912 * creating it if it does not already exist 2913 */ 2914 public CodeableConcept getProgramCodeFirstRep() { 2915 if (getProgramCode().isEmpty()) { 2916 addProgramCode(); 2917 } 2918 return getProgramCode().get(0); 2919 } 2920 2921 /** 2922 * @return {@link #serviced} (The date or dates when the service or product was 2923 * supplied, performed or completed.) 2924 */ 2925 public Type getServiced() { 2926 return this.serviced; 2927 } 2928 2929 /** 2930 * @return {@link #serviced} (The date or dates when the service or product was 2931 * supplied, performed or completed.) 2932 */ 2933 public DateType getServicedDateType() throws FHIRException { 2934 if (this.serviced == null) 2935 this.serviced = new DateType(); 2936 if (!(this.serviced instanceof DateType)) 2937 throw new FHIRException("Type mismatch: the type DateType was expected, but " 2938 + this.serviced.getClass().getName() + " was encountered"); 2939 return (DateType) this.serviced; 2940 } 2941 2942 public boolean hasServicedDateType() { 2943 return this.serviced instanceof DateType; 2944 } 2945 2946 /** 2947 * @return {@link #serviced} (The date or dates when the service or product was 2948 * supplied, performed or completed.) 2949 */ 2950 public Period getServicedPeriod() throws FHIRException { 2951 if (this.serviced == null) 2952 this.serviced = new Period(); 2953 if (!(this.serviced instanceof Period)) 2954 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 2955 + " was encountered"); 2956 return (Period) this.serviced; 2957 } 2958 2959 public boolean hasServicedPeriod() { 2960 return this.serviced instanceof Period; 2961 } 2962 2963 public boolean hasServiced() { 2964 return this.serviced != null && !this.serviced.isEmpty(); 2965 } 2966 2967 /** 2968 * @param value {@link #serviced} (The date or dates when the service or product 2969 * was supplied, performed or completed.) 2970 */ 2971 public AddedItemComponent setServiced(Type value) { 2972 if (value != null && !(value instanceof DateType || value instanceof Period)) 2973 throw new Error("Not the right type for ClaimResponse.addItem.serviced[x]: " + value.fhirType()); 2974 this.serviced = value; 2975 return this; 2976 } 2977 2978 /** 2979 * @return {@link #location} (Where the product or service was provided.) 2980 */ 2981 public Type getLocation() { 2982 return this.location; 2983 } 2984 2985 /** 2986 * @return {@link #location} (Where the product or service was provided.) 2987 */ 2988 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 2989 if (this.location == null) 2990 this.location = new CodeableConcept(); 2991 if (!(this.location instanceof CodeableConcept)) 2992 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2993 + this.location.getClass().getName() + " was encountered"); 2994 return (CodeableConcept) this.location; 2995 } 2996 2997 public boolean hasLocationCodeableConcept() { 2998 return this.location instanceof CodeableConcept; 2999 } 3000 3001 /** 3002 * @return {@link #location} (Where the product or service was provided.) 3003 */ 3004 public Address getLocationAddress() throws FHIRException { 3005 if (this.location == null) 3006 this.location = new Address(); 3007 if (!(this.location instanceof Address)) 3008 throw new FHIRException("Type mismatch: the type Address was expected, but " 3009 + this.location.getClass().getName() + " was encountered"); 3010 return (Address) this.location; 3011 } 3012 3013 public boolean hasLocationAddress() { 3014 return this.location instanceof Address; 3015 } 3016 3017 /** 3018 * @return {@link #location} (Where the product or service was provided.) 3019 */ 3020 public Reference getLocationReference() throws FHIRException { 3021 if (this.location == null) 3022 this.location = new Reference(); 3023 if (!(this.location instanceof Reference)) 3024 throw new FHIRException("Type mismatch: the type Reference was expected, but " 3025 + this.location.getClass().getName() + " was encountered"); 3026 return (Reference) this.location; 3027 } 3028 3029 public boolean hasLocationReference() { 3030 return this.location instanceof Reference; 3031 } 3032 3033 public boolean hasLocation() { 3034 return this.location != null && !this.location.isEmpty(); 3035 } 3036 3037 /** 3038 * @param value {@link #location} (Where the product or service was provided.) 3039 */ 3040 public AddedItemComponent setLocation(Type value) { 3041 if (value != null 3042 && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 3043 throw new Error("Not the right type for ClaimResponse.addItem.location[x]: " + value.fhirType()); 3044 this.location = value; 3045 return this; 3046 } 3047 3048 /** 3049 * @return {@link #quantity} (The number of repetitions of a service or 3050 * product.) 3051 */ 3052 public Quantity getQuantity() { 3053 if (this.quantity == null) 3054 if (Configuration.errorOnAutoCreate()) 3055 throw new Error("Attempt to auto-create AddedItemComponent.quantity"); 3056 else if (Configuration.doAutoCreate()) 3057 this.quantity = new Quantity(); // cc 3058 return this.quantity; 3059 } 3060 3061 public boolean hasQuantity() { 3062 return this.quantity != null && !this.quantity.isEmpty(); 3063 } 3064 3065 /** 3066 * @param value {@link #quantity} (The number of repetitions of a service or 3067 * product.) 3068 */ 3069 public AddedItemComponent setQuantity(Quantity value) { 3070 this.quantity = value; 3071 return this; 3072 } 3073 3074 /** 3075 * @return {@link #unitPrice} (If the item is not a group then this is the fee 3076 * for the product or service, otherwise this is the total of the fees 3077 * for the details of the group.) 3078 */ 3079 public Money getUnitPrice() { 3080 if (this.unitPrice == null) 3081 if (Configuration.errorOnAutoCreate()) 3082 throw new Error("Attempt to auto-create AddedItemComponent.unitPrice"); 3083 else if (Configuration.doAutoCreate()) 3084 this.unitPrice = new Money(); // cc 3085 return this.unitPrice; 3086 } 3087 3088 public boolean hasUnitPrice() { 3089 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3090 } 3091 3092 /** 3093 * @param value {@link #unitPrice} (If the item is not a group then this is the 3094 * fee for the product or service, otherwise this is the total of 3095 * the fees for the details of the group.) 3096 */ 3097 public AddedItemComponent setUnitPrice(Money value) { 3098 this.unitPrice = value; 3099 return this; 3100 } 3101 3102 /** 3103 * @return {@link #factor} (A real number that represents a multiplier used in 3104 * determining the overall value of services delivered and/or goods 3105 * received. The concept of a Factor allows for a discount or surcharge 3106 * multiplier to be applied to a monetary amount.). This is the 3107 * underlying object with id, value and extensions. The accessor 3108 * "getFactor" gives direct access to the value 3109 */ 3110 public DecimalType getFactorElement() { 3111 if (this.factor == null) 3112 if (Configuration.errorOnAutoCreate()) 3113 throw new Error("Attempt to auto-create AddedItemComponent.factor"); 3114 else if (Configuration.doAutoCreate()) 3115 this.factor = new DecimalType(); // bb 3116 return this.factor; 3117 } 3118 3119 public boolean hasFactorElement() { 3120 return this.factor != null && !this.factor.isEmpty(); 3121 } 3122 3123 public boolean hasFactor() { 3124 return this.factor != null && !this.factor.isEmpty(); 3125 } 3126 3127 /** 3128 * @param value {@link #factor} (A real number that represents a multiplier used 3129 * in determining the overall value of services delivered and/or 3130 * goods received. The concept of a Factor allows for a discount or 3131 * surcharge multiplier to be applied to a monetary amount.). This 3132 * is the underlying object with id, value and extensions. The 3133 * accessor "getFactor" gives direct access to the value 3134 */ 3135 public AddedItemComponent setFactorElement(DecimalType value) { 3136 this.factor = value; 3137 return this; 3138 } 3139 3140 /** 3141 * @return A real number that represents a multiplier used in determining the 3142 * overall value of services delivered and/or goods received. The 3143 * concept of a Factor allows for a discount or surcharge multiplier to 3144 * be applied to a monetary amount. 3145 */ 3146 public BigDecimal getFactor() { 3147 return this.factor == null ? null : this.factor.getValue(); 3148 } 3149 3150 /** 3151 * @param value A real number that represents a multiplier used in determining 3152 * the overall value of services delivered and/or goods received. 3153 * The concept of a Factor allows for a discount or surcharge 3154 * multiplier to be applied to a monetary amount. 3155 */ 3156 public AddedItemComponent setFactor(BigDecimal value) { 3157 if (value == null) 3158 this.factor = null; 3159 else { 3160 if (this.factor == null) 3161 this.factor = new DecimalType(); 3162 this.factor.setValue(value); 3163 } 3164 return this; 3165 } 3166 3167 /** 3168 * @param value A real number that represents a multiplier used in determining 3169 * the overall value of services delivered and/or goods received. 3170 * The concept of a Factor allows for a discount or surcharge 3171 * multiplier to be applied to a monetary amount. 3172 */ 3173 public AddedItemComponent setFactor(long value) { 3174 this.factor = new DecimalType(); 3175 this.factor.setValue(value); 3176 return this; 3177 } 3178 3179 /** 3180 * @param value A real number that represents a multiplier used in determining 3181 * the overall value of services delivered and/or goods received. 3182 * The concept of a Factor allows for a discount or surcharge 3183 * multiplier to be applied to a monetary amount. 3184 */ 3185 public AddedItemComponent setFactor(double value) { 3186 this.factor = new DecimalType(); 3187 this.factor.setValue(value); 3188 return this; 3189 } 3190 3191 /** 3192 * @return {@link #net} (The quantity times the unit price for an additional 3193 * service or product or charge.) 3194 */ 3195 public Money getNet() { 3196 if (this.net == null) 3197 if (Configuration.errorOnAutoCreate()) 3198 throw new Error("Attempt to auto-create AddedItemComponent.net"); 3199 else if (Configuration.doAutoCreate()) 3200 this.net = new Money(); // cc 3201 return this.net; 3202 } 3203 3204 public boolean hasNet() { 3205 return this.net != null && !this.net.isEmpty(); 3206 } 3207 3208 /** 3209 * @param value {@link #net} (The quantity times the unit price for an 3210 * additional service or product or charge.) 3211 */ 3212 public AddedItemComponent setNet(Money value) { 3213 this.net = value; 3214 return this; 3215 } 3216 3217 /** 3218 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 3219 * etc.).) 3220 */ 3221 public CodeableConcept getBodySite() { 3222 if (this.bodySite == null) 3223 if (Configuration.errorOnAutoCreate()) 3224 throw new Error("Attempt to auto-create AddedItemComponent.bodySite"); 3225 else if (Configuration.doAutoCreate()) 3226 this.bodySite = new CodeableConcept(); // cc 3227 return this.bodySite; 3228 } 3229 3230 public boolean hasBodySite() { 3231 return this.bodySite != null && !this.bodySite.isEmpty(); 3232 } 3233 3234 /** 3235 * @param value {@link #bodySite} (Physical service site on the patient (limb, 3236 * tooth, etc.).) 3237 */ 3238 public AddedItemComponent setBodySite(CodeableConcept value) { 3239 this.bodySite = value; 3240 return this; 3241 } 3242 3243 /** 3244 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb 3245 * region or tooth surface(s).) 3246 */ 3247 public List<CodeableConcept> getSubSite() { 3248 if (this.subSite == null) 3249 this.subSite = new ArrayList<CodeableConcept>(); 3250 return this.subSite; 3251 } 3252 3253 /** 3254 * @return Returns a reference to <code>this</code> for easy method chaining 3255 */ 3256 public AddedItemComponent setSubSite(List<CodeableConcept> theSubSite) { 3257 this.subSite = theSubSite; 3258 return this; 3259 } 3260 3261 public boolean hasSubSite() { 3262 if (this.subSite == null) 3263 return false; 3264 for (CodeableConcept item : this.subSite) 3265 if (!item.isEmpty()) 3266 return true; 3267 return false; 3268 } 3269 3270 public CodeableConcept addSubSite() { // 3 3271 CodeableConcept t = new CodeableConcept(); 3272 if (this.subSite == null) 3273 this.subSite = new ArrayList<CodeableConcept>(); 3274 this.subSite.add(t); 3275 return t; 3276 } 3277 3278 public AddedItemComponent addSubSite(CodeableConcept t) { // 3 3279 if (t == null) 3280 return this; 3281 if (this.subSite == null) 3282 this.subSite = new ArrayList<CodeableConcept>(); 3283 this.subSite.add(t); 3284 return this; 3285 } 3286 3287 /** 3288 * @return The first repetition of repeating field {@link #subSite}, creating it 3289 * if it does not already exist 3290 */ 3291 public CodeableConcept getSubSiteFirstRep() { 3292 if (getSubSite().isEmpty()) { 3293 addSubSite(); 3294 } 3295 return getSubSite().get(0); 3296 } 3297 3298 /** 3299 * @return {@link #noteNumber} (The numbers associated with notes below which 3300 * apply to the adjudication of this item.) 3301 */ 3302 public List<PositiveIntType> getNoteNumber() { 3303 if (this.noteNumber == null) 3304 this.noteNumber = new ArrayList<PositiveIntType>(); 3305 return this.noteNumber; 3306 } 3307 3308 /** 3309 * @return Returns a reference to <code>this</code> for easy method chaining 3310 */ 3311 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 3312 this.noteNumber = theNoteNumber; 3313 return this; 3314 } 3315 3316 public boolean hasNoteNumber() { 3317 if (this.noteNumber == null) 3318 return false; 3319 for (PositiveIntType item : this.noteNumber) 3320 if (!item.isEmpty()) 3321 return true; 3322 return false; 3323 } 3324 3325 /** 3326 * @return {@link #noteNumber} (The numbers associated with notes below which 3327 * apply to the adjudication of this item.) 3328 */ 3329 public PositiveIntType addNoteNumberElement() {// 2 3330 PositiveIntType t = new PositiveIntType(); 3331 if (this.noteNumber == null) 3332 this.noteNumber = new ArrayList<PositiveIntType>(); 3333 this.noteNumber.add(t); 3334 return t; 3335 } 3336 3337 /** 3338 * @param value {@link #noteNumber} (The numbers associated with notes below 3339 * which apply to the adjudication of this item.) 3340 */ 3341 public AddedItemComponent addNoteNumber(int value) { // 1 3342 PositiveIntType t = new PositiveIntType(); 3343 t.setValue(value); 3344 if (this.noteNumber == null) 3345 this.noteNumber = new ArrayList<PositiveIntType>(); 3346 this.noteNumber.add(t); 3347 return this; 3348 } 3349 3350 /** 3351 * @param value {@link #noteNumber} (The numbers associated with notes below 3352 * which apply to the adjudication of this item.) 3353 */ 3354 public boolean hasNoteNumber(int value) { 3355 if (this.noteNumber == null) 3356 return false; 3357 for (PositiveIntType v : this.noteNumber) 3358 if (v.getValue().equals(value)) // positiveInt 3359 return true; 3360 return false; 3361 } 3362 3363 /** 3364 * @return {@link #adjudication} (The adjudication results.) 3365 */ 3366 public List<AdjudicationComponent> getAdjudication() { 3367 if (this.adjudication == null) 3368 this.adjudication = new ArrayList<AdjudicationComponent>(); 3369 return this.adjudication; 3370 } 3371 3372 /** 3373 * @return Returns a reference to <code>this</code> for easy method chaining 3374 */ 3375 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 3376 this.adjudication = theAdjudication; 3377 return this; 3378 } 3379 3380 public boolean hasAdjudication() { 3381 if (this.adjudication == null) 3382 return false; 3383 for (AdjudicationComponent item : this.adjudication) 3384 if (!item.isEmpty()) 3385 return true; 3386 return false; 3387 } 3388 3389 public AdjudicationComponent addAdjudication() { // 3 3390 AdjudicationComponent t = new AdjudicationComponent(); 3391 if (this.adjudication == null) 3392 this.adjudication = new ArrayList<AdjudicationComponent>(); 3393 this.adjudication.add(t); 3394 return t; 3395 } 3396 3397 public AddedItemComponent addAdjudication(AdjudicationComponent t) { // 3 3398 if (t == null) 3399 return this; 3400 if (this.adjudication == null) 3401 this.adjudication = new ArrayList<AdjudicationComponent>(); 3402 this.adjudication.add(t); 3403 return this; 3404 } 3405 3406 /** 3407 * @return The first repetition of repeating field {@link #adjudication}, 3408 * creating it if it does not already exist 3409 */ 3410 public AdjudicationComponent getAdjudicationFirstRep() { 3411 if (getAdjudication().isEmpty()) { 3412 addAdjudication(); 3413 } 3414 return getAdjudication().get(0); 3415 } 3416 3417 /** 3418 * @return {@link #detail} (The second-tier service adjudications for payor 3419 * added services.) 3420 */ 3421 public List<AddedItemDetailComponent> getDetail() { 3422 if (this.detail == null) 3423 this.detail = new ArrayList<AddedItemDetailComponent>(); 3424 return this.detail; 3425 } 3426 3427 /** 3428 * @return Returns a reference to <code>this</code> for easy method chaining 3429 */ 3430 public AddedItemComponent setDetail(List<AddedItemDetailComponent> theDetail) { 3431 this.detail = theDetail; 3432 return this; 3433 } 3434 3435 public boolean hasDetail() { 3436 if (this.detail == null) 3437 return false; 3438 for (AddedItemDetailComponent item : this.detail) 3439 if (!item.isEmpty()) 3440 return true; 3441 return false; 3442 } 3443 3444 public AddedItemDetailComponent addDetail() { // 3 3445 AddedItemDetailComponent t = new AddedItemDetailComponent(); 3446 if (this.detail == null) 3447 this.detail = new ArrayList<AddedItemDetailComponent>(); 3448 this.detail.add(t); 3449 return t; 3450 } 3451 3452 public AddedItemComponent addDetail(AddedItemDetailComponent t) { // 3 3453 if (t == null) 3454 return this; 3455 if (this.detail == null) 3456 this.detail = new ArrayList<AddedItemDetailComponent>(); 3457 this.detail.add(t); 3458 return this; 3459 } 3460 3461 /** 3462 * @return The first repetition of repeating field {@link #detail}, creating it 3463 * if it does not already exist 3464 */ 3465 public AddedItemDetailComponent getDetailFirstRep() { 3466 if (getDetail().isEmpty()) { 3467 addDetail(); 3468 } 3469 return getDetail().get(0); 3470 } 3471 3472 protected void listChildren(List<Property> children) { 3473 super.listChildren(children); 3474 children.add(new Property("itemSequence", "positiveInt", 3475 "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence)); 3476 children.add(new Property("detailSequence", "positiveInt", 3477 "The sequence number of the details within the claim item which this line is intended to replace.", 0, 3478 java.lang.Integer.MAX_VALUE, detailSequence)); 3479 children.add(new Property("subdetailSequence", "positiveInt", 3480 "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.", 3481 0, java.lang.Integer.MAX_VALUE, subdetailSequence)); 3482 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 3483 "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 3484 provider)); 3485 children.add(new Property("productOrService", "CodeableConcept", 3486 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 3487 0, 1, productOrService)); 3488 children.add(new Property("modifier", "CodeableConcept", 3489 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 3490 java.lang.Integer.MAX_VALUE, modifier)); 3491 children.add(new Property("programCode", "CodeableConcept", 3492 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 3493 children.add(new Property("serviced[x]", "date|Period", 3494 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 3495 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3496 "Where the product or service was provided.", 0, 1, location)); 3497 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 3498 1, quantity)); 3499 children.add(new Property("unitPrice", "Money", 3500 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 3501 0, 1, unitPrice)); 3502 children.add(new Property("factor", "decimal", 3503 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 3504 0, 1, factor)); 3505 children.add(new Property("net", "Money", 3506 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 3507 children.add(new Property("bodySite", "CodeableConcept", 3508 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite)); 3509 children.add(new Property("subSite", "CodeableConcept", 3510 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, 3511 subSite)); 3512 children.add(new Property("noteNumber", "positiveInt", 3513 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 3514 java.lang.Integer.MAX_VALUE, noteNumber)); 3515 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 3516 java.lang.Integer.MAX_VALUE, adjudication)); 3517 children.add(new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, 3518 java.lang.Integer.MAX_VALUE, detail)); 3519 } 3520 3521 @Override 3522 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3523 switch (_hash) { 3524 case 1977979892: 3525 /* itemSequence */ return new Property("itemSequence", "positiveInt", 3526 "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, 3527 itemSequence); 3528 case 1321472818: 3529 /* detailSequence */ return new Property("detailSequence", "positiveInt", 3530 "The sequence number of the details within the claim item which this line is intended to replace.", 0, 3531 java.lang.Integer.MAX_VALUE, detailSequence); 3532 case 146530674: 3533 /* subdetailSequence */ return new Property("subdetailSequence", "positiveInt", 3534 "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.", 3535 0, java.lang.Integer.MAX_VALUE, subdetailSequence); 3536 case -987494927: 3537 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 3538 "The providers who are authorized for the services rendered to the patient.", 0, 3539 java.lang.Integer.MAX_VALUE, provider); 3540 case 1957227299: 3541 /* productOrService */ return new Property("productOrService", "CodeableConcept", 3542 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 3543 0, 1, productOrService); 3544 case -615513385: 3545 /* modifier */ return new Property("modifier", "CodeableConcept", 3546 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 3547 java.lang.Integer.MAX_VALUE, modifier); 3548 case 1010065041: 3549 /* programCode */ return new Property("programCode", "CodeableConcept", 3550 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 3551 case -1927922223: 3552 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 3553 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3554 case 1379209295: 3555 /* serviced */ return new Property("serviced[x]", "date|Period", 3556 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3557 case 363246749: 3558 /* servicedDate */ return new Property("serviced[x]", "date|Period", 3559 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3560 case 1534966512: 3561 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 3562 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3563 case 552316075: 3564 /* location[x] */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3565 "Where the product or service was provided.", 0, 1, location); 3566 case 1901043637: 3567 /* location */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3568 "Where the product or service was provided.", 0, 1, location); 3569 case -1224800468: 3570 /* locationCodeableConcept */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3571 "Where the product or service was provided.", 0, 1, location); 3572 case -1280020865: 3573 /* locationAddress */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3574 "Where the product or service was provided.", 0, 1, location); 3575 case 755866390: 3576 /* locationReference */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3577 "Where the product or service was provided.", 0, 1, location); 3578 case -1285004149: 3579 /* quantity */ return new Property("quantity", "SimpleQuantity", 3580 "The number of repetitions of a service or product.", 0, 1, quantity); 3581 case -486196699: 3582 /* unitPrice */ return new Property("unitPrice", "Money", 3583 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 3584 0, 1, unitPrice); 3585 case -1282148017: 3586 /* factor */ return new Property("factor", "decimal", 3587 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 3588 0, 1, factor); 3589 case 108957: 3590 /* net */ return new Property("net", "Money", 3591 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 3592 case 1702620169: 3593 /* bodySite */ return new Property("bodySite", "CodeableConcept", 3594 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite); 3595 case -1868566105: 3596 /* subSite */ return new Property("subSite", "CodeableConcept", 3597 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, 3598 java.lang.Integer.MAX_VALUE, subSite); 3599 case -1110033957: 3600 /* noteNumber */ return new Property("noteNumber", "positiveInt", 3601 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 3602 java.lang.Integer.MAX_VALUE, noteNumber); 3603 case -231349275: 3604 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 3605 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 3606 case -1335224239: 3607 /* detail */ return new Property("detail", "", 3608 "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 3609 default: 3610 return super.getNamedProperty(_hash, _name, _checkValid); 3611 } 3612 3613 } 3614 3615 @Override 3616 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3617 switch (hash) { 3618 case 1977979892: 3619 /* itemSequence */ return this.itemSequence == null ? new Base[0] 3620 : this.itemSequence.toArray(new Base[this.itemSequence.size()]); // PositiveIntType 3621 case 1321472818: 3622 /* detailSequence */ return this.detailSequence == null ? new Base[0] 3623 : this.detailSequence.toArray(new Base[this.detailSequence.size()]); // PositiveIntType 3624 case 146530674: 3625 /* subdetailSequence */ return this.subdetailSequence == null ? new Base[0] 3626 : this.subdetailSequence.toArray(new Base[this.subdetailSequence.size()]); // PositiveIntType 3627 case -987494927: 3628 /* provider */ return this.provider == null ? new Base[0] 3629 : this.provider.toArray(new Base[this.provider.size()]); // Reference 3630 case 1957227299: 3631 /* productOrService */ return this.productOrService == null ? new Base[0] 3632 : new Base[] { this.productOrService }; // CodeableConcept 3633 case -615513385: 3634 /* modifier */ return this.modifier == null ? new Base[0] 3635 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 3636 case 1010065041: 3637 /* programCode */ return this.programCode == null ? new Base[0] 3638 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 3639 case 1379209295: 3640 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 3641 case 1901043637: 3642 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 3643 case -1285004149: 3644 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 3645 case -486196699: 3646 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 3647 case -1282148017: 3648 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 3649 case 108957: 3650 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 3651 case 1702620169: 3652 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 3653 case -1868566105: 3654 /* subSite */ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 3655 case -1110033957: 3656 /* noteNumber */ return this.noteNumber == null ? new Base[0] 3657 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 3658 case -231349275: 3659 /* adjudication */ return this.adjudication == null ? new Base[0] 3660 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 3661 case -1335224239: 3662 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemDetailComponent 3663 default: 3664 return super.getProperty(hash, name, checkValid); 3665 } 3666 3667 } 3668 3669 @Override 3670 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3671 switch (hash) { 3672 case 1977979892: // itemSequence 3673 this.getItemSequence().add(castToPositiveInt(value)); // PositiveIntType 3674 return value; 3675 case 1321472818: // detailSequence 3676 this.getDetailSequence().add(castToPositiveInt(value)); // PositiveIntType 3677 return value; 3678 case 146530674: // subdetailSequence 3679 this.getSubdetailSequence().add(castToPositiveInt(value)); // PositiveIntType 3680 return value; 3681 case -987494927: // provider 3682 this.getProvider().add(castToReference(value)); // Reference 3683 return value; 3684 case 1957227299: // productOrService 3685 this.productOrService = castToCodeableConcept(value); // CodeableConcept 3686 return value; 3687 case -615513385: // modifier 3688 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 3689 return value; 3690 case 1010065041: // programCode 3691 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 3692 return value; 3693 case 1379209295: // serviced 3694 this.serviced = castToType(value); // Type 3695 return value; 3696 case 1901043637: // location 3697 this.location = castToType(value); // Type 3698 return value; 3699 case -1285004149: // quantity 3700 this.quantity = castToQuantity(value); // Quantity 3701 return value; 3702 case -486196699: // unitPrice 3703 this.unitPrice = castToMoney(value); // Money 3704 return value; 3705 case -1282148017: // factor 3706 this.factor = castToDecimal(value); // DecimalType 3707 return value; 3708 case 108957: // net 3709 this.net = castToMoney(value); // Money 3710 return value; 3711 case 1702620169: // bodySite 3712 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3713 return value; 3714 case -1868566105: // subSite 3715 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 3716 return value; 3717 case -1110033957: // noteNumber 3718 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 3719 return value; 3720 case -231349275: // adjudication 3721 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 3722 return value; 3723 case -1335224239: // detail 3724 this.getDetail().add((AddedItemDetailComponent) value); // AddedItemDetailComponent 3725 return value; 3726 default: 3727 return super.setProperty(hash, name, value); 3728 } 3729 3730 } 3731 3732 @Override 3733 public Base setProperty(String name, Base value) throws FHIRException { 3734 if (name.equals("itemSequence")) { 3735 this.getItemSequence().add(castToPositiveInt(value)); 3736 } else if (name.equals("detailSequence")) { 3737 this.getDetailSequence().add(castToPositiveInt(value)); 3738 } else if (name.equals("subdetailSequence")) { 3739 this.getSubdetailSequence().add(castToPositiveInt(value)); 3740 } else if (name.equals("provider")) { 3741 this.getProvider().add(castToReference(value)); 3742 } else if (name.equals("productOrService")) { 3743 this.productOrService = castToCodeableConcept(value); // CodeableConcept 3744 } else if (name.equals("modifier")) { 3745 this.getModifier().add(castToCodeableConcept(value)); 3746 } else if (name.equals("programCode")) { 3747 this.getProgramCode().add(castToCodeableConcept(value)); 3748 } else if (name.equals("serviced[x]")) { 3749 this.serviced = castToType(value); // Type 3750 } else if (name.equals("location[x]")) { 3751 this.location = castToType(value); // Type 3752 } else if (name.equals("quantity")) { 3753 this.quantity = castToQuantity(value); // Quantity 3754 } else if (name.equals("unitPrice")) { 3755 this.unitPrice = castToMoney(value); // Money 3756 } else if (name.equals("factor")) { 3757 this.factor = castToDecimal(value); // DecimalType 3758 } else if (name.equals("net")) { 3759 this.net = castToMoney(value); // Money 3760 } else if (name.equals("bodySite")) { 3761 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3762 } else if (name.equals("subSite")) { 3763 this.getSubSite().add(castToCodeableConcept(value)); 3764 } else if (name.equals("noteNumber")) { 3765 this.getNoteNumber().add(castToPositiveInt(value)); 3766 } else if (name.equals("adjudication")) { 3767 this.getAdjudication().add((AdjudicationComponent) value); 3768 } else if (name.equals("detail")) { 3769 this.getDetail().add((AddedItemDetailComponent) value); 3770 } else 3771 return super.setProperty(name, value); 3772 return value; 3773 } 3774 3775 @Override 3776 public void removeChild(String name, Base value) throws FHIRException { 3777 if (name.equals("itemSequence")) { 3778 this.getItemSequence().remove(castToPositiveInt(value)); 3779 } else if (name.equals("detailSequence")) { 3780 this.getDetailSequence().remove(castToPositiveInt(value)); 3781 } else if (name.equals("subdetailSequence")) { 3782 this.getSubdetailSequence().remove(castToPositiveInt(value)); 3783 } else if (name.equals("provider")) { 3784 this.getProvider().remove(castToReference(value)); 3785 } else if (name.equals("productOrService")) { 3786 this.productOrService = null; 3787 } else if (name.equals("modifier")) { 3788 this.getModifier().remove(castToCodeableConcept(value)); 3789 } else if (name.equals("programCode")) { 3790 this.getProgramCode().remove(castToCodeableConcept(value)); 3791 } else if (name.equals("serviced[x]")) { 3792 this.serviced = null; 3793 } else if (name.equals("location[x]")) { 3794 this.location = null; 3795 } else if (name.equals("quantity")) { 3796 this.quantity = null; 3797 } else if (name.equals("unitPrice")) { 3798 this.unitPrice = null; 3799 } else if (name.equals("factor")) { 3800 this.factor = null; 3801 } else if (name.equals("net")) { 3802 this.net = null; 3803 } else if (name.equals("bodySite")) { 3804 this.bodySite = null; 3805 } else if (name.equals("subSite")) { 3806 this.getSubSite().remove(castToCodeableConcept(value)); 3807 } else if (name.equals("noteNumber")) { 3808 this.getNoteNumber().remove(castToPositiveInt(value)); 3809 } else if (name.equals("adjudication")) { 3810 this.getAdjudication().remove((AdjudicationComponent) value); 3811 } else if (name.equals("detail")) { 3812 this.getDetail().remove((AddedItemDetailComponent) value); 3813 } else 3814 super.removeChild(name, value); 3815 3816 } 3817 3818 @Override 3819 public Base makeProperty(int hash, String name) throws FHIRException { 3820 switch (hash) { 3821 case 1977979892: 3822 return addItemSequenceElement(); 3823 case 1321472818: 3824 return addDetailSequenceElement(); 3825 case 146530674: 3826 return addSubdetailSequenceElement(); 3827 case -987494927: 3828 return addProvider(); 3829 case 1957227299: 3830 return getProductOrService(); 3831 case -615513385: 3832 return addModifier(); 3833 case 1010065041: 3834 return addProgramCode(); 3835 case -1927922223: 3836 return getServiced(); 3837 case 1379209295: 3838 return getServiced(); 3839 case 552316075: 3840 return getLocation(); 3841 case 1901043637: 3842 return getLocation(); 3843 case -1285004149: 3844 return getQuantity(); 3845 case -486196699: 3846 return getUnitPrice(); 3847 case -1282148017: 3848 return getFactorElement(); 3849 case 108957: 3850 return getNet(); 3851 case 1702620169: 3852 return getBodySite(); 3853 case -1868566105: 3854 return addSubSite(); 3855 case -1110033957: 3856 return addNoteNumberElement(); 3857 case -231349275: 3858 return addAdjudication(); 3859 case -1335224239: 3860 return addDetail(); 3861 default: 3862 return super.makeProperty(hash, name); 3863 } 3864 3865 } 3866 3867 @Override 3868 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3869 switch (hash) { 3870 case 1977979892: 3871 /* itemSequence */ return new String[] { "positiveInt" }; 3872 case 1321472818: 3873 /* detailSequence */ return new String[] { "positiveInt" }; 3874 case 146530674: 3875 /* subdetailSequence */ return new String[] { "positiveInt" }; 3876 case -987494927: 3877 /* provider */ return new String[] { "Reference" }; 3878 case 1957227299: 3879 /* productOrService */ return new String[] { "CodeableConcept" }; 3880 case -615513385: 3881 /* modifier */ return new String[] { "CodeableConcept" }; 3882 case 1010065041: 3883 /* programCode */ return new String[] { "CodeableConcept" }; 3884 case 1379209295: 3885 /* serviced */ return new String[] { "date", "Period" }; 3886 case 1901043637: 3887 /* location */ return new String[] { "CodeableConcept", "Address", "Reference" }; 3888 case -1285004149: 3889 /* quantity */ return new String[] { "SimpleQuantity" }; 3890 case -486196699: 3891 /* unitPrice */ return new String[] { "Money" }; 3892 case -1282148017: 3893 /* factor */ return new String[] { "decimal" }; 3894 case 108957: 3895 /* net */ return new String[] { "Money" }; 3896 case 1702620169: 3897 /* bodySite */ return new String[] { "CodeableConcept" }; 3898 case -1868566105: 3899 /* subSite */ return new String[] { "CodeableConcept" }; 3900 case -1110033957: 3901 /* noteNumber */ return new String[] { "positiveInt" }; 3902 case -231349275: 3903 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 3904 case -1335224239: 3905 /* detail */ return new String[] {}; 3906 default: 3907 return super.getTypesForProperty(hash, name); 3908 } 3909 3910 } 3911 3912 @Override 3913 public Base addChild(String name) throws FHIRException { 3914 if (name.equals("itemSequence")) { 3915 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.itemSequence"); 3916 } else if (name.equals("detailSequence")) { 3917 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequence"); 3918 } else if (name.equals("subdetailSequence")) { 3919 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subdetailSequence"); 3920 } else if (name.equals("provider")) { 3921 return addProvider(); 3922 } else if (name.equals("productOrService")) { 3923 this.productOrService = new CodeableConcept(); 3924 return this.productOrService; 3925 } else if (name.equals("modifier")) { 3926 return addModifier(); 3927 } else if (name.equals("programCode")) { 3928 return addProgramCode(); 3929 } else if (name.equals("servicedDate")) { 3930 this.serviced = new DateType(); 3931 return this.serviced; 3932 } else if (name.equals("servicedPeriod")) { 3933 this.serviced = new Period(); 3934 return this.serviced; 3935 } else if (name.equals("locationCodeableConcept")) { 3936 this.location = new CodeableConcept(); 3937 return this.location; 3938 } else if (name.equals("locationAddress")) { 3939 this.location = new Address(); 3940 return this.location; 3941 } else if (name.equals("locationReference")) { 3942 this.location = new Reference(); 3943 return this.location; 3944 } else if (name.equals("quantity")) { 3945 this.quantity = new Quantity(); 3946 return this.quantity; 3947 } else if (name.equals("unitPrice")) { 3948 this.unitPrice = new Money(); 3949 return this.unitPrice; 3950 } else if (name.equals("factor")) { 3951 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.factor"); 3952 } else if (name.equals("net")) { 3953 this.net = new Money(); 3954 return this.net; 3955 } else if (name.equals("bodySite")) { 3956 this.bodySite = new CodeableConcept(); 3957 return this.bodySite; 3958 } else if (name.equals("subSite")) { 3959 return addSubSite(); 3960 } else if (name.equals("noteNumber")) { 3961 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 3962 } else if (name.equals("adjudication")) { 3963 return addAdjudication(); 3964 } else if (name.equals("detail")) { 3965 return addDetail(); 3966 } else 3967 return super.addChild(name); 3968 } 3969 3970 public AddedItemComponent copy() { 3971 AddedItemComponent dst = new AddedItemComponent(); 3972 copyValues(dst); 3973 return dst; 3974 } 3975 3976 public void copyValues(AddedItemComponent dst) { 3977 super.copyValues(dst); 3978 if (itemSequence != null) { 3979 dst.itemSequence = new ArrayList<PositiveIntType>(); 3980 for (PositiveIntType i : itemSequence) 3981 dst.itemSequence.add(i.copy()); 3982 } 3983 ; 3984 if (detailSequence != null) { 3985 dst.detailSequence = new ArrayList<PositiveIntType>(); 3986 for (PositiveIntType i : detailSequence) 3987 dst.detailSequence.add(i.copy()); 3988 } 3989 ; 3990 if (subdetailSequence != null) { 3991 dst.subdetailSequence = new ArrayList<PositiveIntType>(); 3992 for (PositiveIntType i : subdetailSequence) 3993 dst.subdetailSequence.add(i.copy()); 3994 } 3995 ; 3996 if (provider != null) { 3997 dst.provider = new ArrayList<Reference>(); 3998 for (Reference i : provider) 3999 dst.provider.add(i.copy()); 4000 } 4001 ; 4002 dst.productOrService = productOrService == null ? null : productOrService.copy(); 4003 if (modifier != null) { 4004 dst.modifier = new ArrayList<CodeableConcept>(); 4005 for (CodeableConcept i : modifier) 4006 dst.modifier.add(i.copy()); 4007 } 4008 ; 4009 if (programCode != null) { 4010 dst.programCode = new ArrayList<CodeableConcept>(); 4011 for (CodeableConcept i : programCode) 4012 dst.programCode.add(i.copy()); 4013 } 4014 ; 4015 dst.serviced = serviced == null ? null : serviced.copy(); 4016 dst.location = location == null ? null : location.copy(); 4017 dst.quantity = quantity == null ? null : quantity.copy(); 4018 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4019 dst.factor = factor == null ? null : factor.copy(); 4020 dst.net = net == null ? null : net.copy(); 4021 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4022 if (subSite != null) { 4023 dst.subSite = new ArrayList<CodeableConcept>(); 4024 for (CodeableConcept i : subSite) 4025 dst.subSite.add(i.copy()); 4026 } 4027 ; 4028 if (noteNumber != null) { 4029 dst.noteNumber = new ArrayList<PositiveIntType>(); 4030 for (PositiveIntType i : noteNumber) 4031 dst.noteNumber.add(i.copy()); 4032 } 4033 ; 4034 if (adjudication != null) { 4035 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4036 for (AdjudicationComponent i : adjudication) 4037 dst.adjudication.add(i.copy()); 4038 } 4039 ; 4040 if (detail != null) { 4041 dst.detail = new ArrayList<AddedItemDetailComponent>(); 4042 for (AddedItemDetailComponent i : detail) 4043 dst.detail.add(i.copy()); 4044 } 4045 ; 4046 } 4047 4048 @Override 4049 public boolean equalsDeep(Base other_) { 4050 if (!super.equalsDeep(other_)) 4051 return false; 4052 if (!(other_ instanceof AddedItemComponent)) 4053 return false; 4054 AddedItemComponent o = (AddedItemComponent) other_; 4055 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 4056 && compareDeep(subdetailSequence, o.subdetailSequence, true) && compareDeep(provider, o.provider, true) 4057 && compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 4058 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 4059 && compareDeep(location, o.location, true) && compareDeep(quantity, o.quantity, true) 4060 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 4061 && compareDeep(net, o.net, true) && compareDeep(bodySite, o.bodySite, true) 4062 && compareDeep(subSite, o.subSite, true) && compareDeep(noteNumber, o.noteNumber, true) 4063 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 4064 } 4065 4066 @Override 4067 public boolean equalsShallow(Base other_) { 4068 if (!super.equalsShallow(other_)) 4069 return false; 4070 if (!(other_ instanceof AddedItemComponent)) 4071 return false; 4072 AddedItemComponent o = (AddedItemComponent) other_; 4073 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 4074 && compareValues(subdetailSequence, o.subdetailSequence, true) && compareValues(factor, o.factor, true) 4075 && compareValues(noteNumber, o.noteNumber, true); 4076 } 4077 4078 public boolean isEmpty() { 4079 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence, subdetailSequence, 4080 provider, productOrService, modifier, programCode, serviced, location, quantity, unitPrice, factor, net, 4081 bodySite, subSite, noteNumber, adjudication, detail); 4082 } 4083 4084 public String fhirType() { 4085 return "ClaimResponse.addItem"; 4086 4087 } 4088 4089 } 4090 4091 @Block() 4092 public static class AddedItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 4093 /** 4094 * When the value is a group code then this item collects a set of related claim 4095 * details, otherwise this contains the product, service, drug or other billing 4096 * code for the item. 4097 */ 4098 @Child(name = "productOrService", type = { 4099 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4100 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4101 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4102 protected CodeableConcept productOrService; 4103 4104 /** 4105 * Item typification or modifiers codes to convey additional context for the 4106 * product or service. 4107 */ 4108 @Child(name = "modifier", type = { 4109 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4110 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4112 protected List<CodeableConcept> modifier; 4113 4114 /** 4115 * The number of repetitions of a service or product. 4116 */ 4117 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4118 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4119 protected Quantity quantity; 4120 4121 /** 4122 * If the item is not a group then this is the fee for the product or service, 4123 * otherwise this is the total of the fees for the details of the group. 4124 */ 4125 @Child(name = "unitPrice", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 4126 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 4127 protected Money unitPrice; 4128 4129 /** 4130 * A real number that represents a multiplier used in determining the overall 4131 * value of services delivered and/or goods received. The concept of a Factor 4132 * allows for a discount or surcharge multiplier to be applied to a monetary 4133 * amount. 4134 */ 4135 @Child(name = "factor", type = { 4136 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4137 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 4138 protected DecimalType factor; 4139 4140 /** 4141 * The quantity times the unit price for an additional service or product or 4142 * charge. 4143 */ 4144 @Child(name = "net", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4145 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 4146 protected Money net; 4147 4148 /** 4149 * The numbers associated with notes below which apply to the adjudication of 4150 * this item. 4151 */ 4152 @Child(name = "noteNumber", type = { 4153 PositiveIntType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4154 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 4155 protected List<PositiveIntType> noteNumber; 4156 4157 /** 4158 * The adjudication results. 4159 */ 4160 @Child(name = "adjudication", type = { 4161 AdjudicationComponent.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4162 @Description(shortDefinition = "Added items detail adjudication", formalDefinition = "The adjudication results.") 4163 protected List<AdjudicationComponent> adjudication; 4164 4165 /** 4166 * The third-tier service adjudications for payor added services. 4167 */ 4168 @Child(name = "subDetail", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4169 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The third-tier service adjudications for payor added services.") 4170 protected List<AddedItemSubDetailComponent> subDetail; 4171 4172 private static final long serialVersionUID = -1436724060L; 4173 4174 /** 4175 * Constructor 4176 */ 4177 public AddedItemDetailComponent() { 4178 super(); 4179 } 4180 4181 /** 4182 * Constructor 4183 */ 4184 public AddedItemDetailComponent(CodeableConcept productOrService) { 4185 super(); 4186 this.productOrService = productOrService; 4187 } 4188 4189 /** 4190 * @return {@link #productOrService} (When the value is a group code then this 4191 * item collects a set of related claim details, otherwise this contains 4192 * the product, service, drug or other billing code for the item.) 4193 */ 4194 public CodeableConcept getProductOrService() { 4195 if (this.productOrService == null) 4196 if (Configuration.errorOnAutoCreate()) 4197 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrService"); 4198 else if (Configuration.doAutoCreate()) 4199 this.productOrService = new CodeableConcept(); // cc 4200 return this.productOrService; 4201 } 4202 4203 public boolean hasProductOrService() { 4204 return this.productOrService != null && !this.productOrService.isEmpty(); 4205 } 4206 4207 /** 4208 * @param value {@link #productOrService} (When the value is a group code then 4209 * this item collects a set of related claim details, otherwise 4210 * this contains the product, service, drug or other billing code 4211 * for the item.) 4212 */ 4213 public AddedItemDetailComponent setProductOrService(CodeableConcept value) { 4214 this.productOrService = value; 4215 return this; 4216 } 4217 4218 /** 4219 * @return {@link #modifier} (Item typification or modifiers codes to convey 4220 * additional context for the product or service.) 4221 */ 4222 public List<CodeableConcept> getModifier() { 4223 if (this.modifier == null) 4224 this.modifier = new ArrayList<CodeableConcept>(); 4225 return this.modifier; 4226 } 4227 4228 /** 4229 * @return Returns a reference to <code>this</code> for easy method chaining 4230 */ 4231 public AddedItemDetailComponent setModifier(List<CodeableConcept> theModifier) { 4232 this.modifier = theModifier; 4233 return this; 4234 } 4235 4236 public boolean hasModifier() { 4237 if (this.modifier == null) 4238 return false; 4239 for (CodeableConcept item : this.modifier) 4240 if (!item.isEmpty()) 4241 return true; 4242 return false; 4243 } 4244 4245 public CodeableConcept addModifier() { // 3 4246 CodeableConcept t = new CodeableConcept(); 4247 if (this.modifier == null) 4248 this.modifier = new ArrayList<CodeableConcept>(); 4249 this.modifier.add(t); 4250 return t; 4251 } 4252 4253 public AddedItemDetailComponent addModifier(CodeableConcept t) { // 3 4254 if (t == null) 4255 return this; 4256 if (this.modifier == null) 4257 this.modifier = new ArrayList<CodeableConcept>(); 4258 this.modifier.add(t); 4259 return this; 4260 } 4261 4262 /** 4263 * @return The first repetition of repeating field {@link #modifier}, creating 4264 * it if it does not already exist 4265 */ 4266 public CodeableConcept getModifierFirstRep() { 4267 if (getModifier().isEmpty()) { 4268 addModifier(); 4269 } 4270 return getModifier().get(0); 4271 } 4272 4273 /** 4274 * @return {@link #quantity} (The number of repetitions of a service or 4275 * product.) 4276 */ 4277 public Quantity getQuantity() { 4278 if (this.quantity == null) 4279 if (Configuration.errorOnAutoCreate()) 4280 throw new Error("Attempt to auto-create AddedItemDetailComponent.quantity"); 4281 else if (Configuration.doAutoCreate()) 4282 this.quantity = new Quantity(); // cc 4283 return this.quantity; 4284 } 4285 4286 public boolean hasQuantity() { 4287 return this.quantity != null && !this.quantity.isEmpty(); 4288 } 4289 4290 /** 4291 * @param value {@link #quantity} (The number of repetitions of a service or 4292 * product.) 4293 */ 4294 public AddedItemDetailComponent setQuantity(Quantity value) { 4295 this.quantity = value; 4296 return this; 4297 } 4298 4299 /** 4300 * @return {@link #unitPrice} (If the item is not a group then this is the fee 4301 * for the product or service, otherwise this is the total of the fees 4302 * for the details of the group.) 4303 */ 4304 public Money getUnitPrice() { 4305 if (this.unitPrice == null) 4306 if (Configuration.errorOnAutoCreate()) 4307 throw new Error("Attempt to auto-create AddedItemDetailComponent.unitPrice"); 4308 else if (Configuration.doAutoCreate()) 4309 this.unitPrice = new Money(); // cc 4310 return this.unitPrice; 4311 } 4312 4313 public boolean hasUnitPrice() { 4314 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4315 } 4316 4317 /** 4318 * @param value {@link #unitPrice} (If the item is not a group then this is the 4319 * fee for the product or service, otherwise this is the total of 4320 * the fees for the details of the group.) 4321 */ 4322 public AddedItemDetailComponent setUnitPrice(Money value) { 4323 this.unitPrice = value; 4324 return this; 4325 } 4326 4327 /** 4328 * @return {@link #factor} (A real number that represents a multiplier used in 4329 * determining the overall value of services delivered and/or goods 4330 * received. The concept of a Factor allows for a discount or surcharge 4331 * multiplier to be applied to a monetary amount.). This is the 4332 * underlying object with id, value and extensions. The accessor 4333 * "getFactor" gives direct access to the value 4334 */ 4335 public DecimalType getFactorElement() { 4336 if (this.factor == null) 4337 if (Configuration.errorOnAutoCreate()) 4338 throw new Error("Attempt to auto-create AddedItemDetailComponent.factor"); 4339 else if (Configuration.doAutoCreate()) 4340 this.factor = new DecimalType(); // bb 4341 return this.factor; 4342 } 4343 4344 public boolean hasFactorElement() { 4345 return this.factor != null && !this.factor.isEmpty(); 4346 } 4347 4348 public boolean hasFactor() { 4349 return this.factor != null && !this.factor.isEmpty(); 4350 } 4351 4352 /** 4353 * @param value {@link #factor} (A real number that represents a multiplier used 4354 * in determining the overall value of services delivered and/or 4355 * goods received. The concept of a Factor allows for a discount or 4356 * surcharge multiplier to be applied to a monetary amount.). This 4357 * is the underlying object with id, value and extensions. The 4358 * accessor "getFactor" gives direct access to the value 4359 */ 4360 public AddedItemDetailComponent setFactorElement(DecimalType value) { 4361 this.factor = value; 4362 return this; 4363 } 4364 4365 /** 4366 * @return A real number that represents a multiplier used in determining the 4367 * overall value of services delivered and/or goods received. The 4368 * concept of a Factor allows for a discount or surcharge multiplier to 4369 * be applied to a monetary amount. 4370 */ 4371 public BigDecimal getFactor() { 4372 return this.factor == null ? null : this.factor.getValue(); 4373 } 4374 4375 /** 4376 * @param value A real number that represents a multiplier used in determining 4377 * the overall value of services delivered and/or goods received. 4378 * The concept of a Factor allows for a discount or surcharge 4379 * multiplier to be applied to a monetary amount. 4380 */ 4381 public AddedItemDetailComponent setFactor(BigDecimal value) { 4382 if (value == null) 4383 this.factor = null; 4384 else { 4385 if (this.factor == null) 4386 this.factor = new DecimalType(); 4387 this.factor.setValue(value); 4388 } 4389 return this; 4390 } 4391 4392 /** 4393 * @param value A real number that represents a multiplier used in determining 4394 * the overall value of services delivered and/or goods received. 4395 * The concept of a Factor allows for a discount or surcharge 4396 * multiplier to be applied to a monetary amount. 4397 */ 4398 public AddedItemDetailComponent setFactor(long value) { 4399 this.factor = new DecimalType(); 4400 this.factor.setValue(value); 4401 return this; 4402 } 4403 4404 /** 4405 * @param value A real number that represents a multiplier used in determining 4406 * the overall value of services delivered and/or goods received. 4407 * The concept of a Factor allows for a discount or surcharge 4408 * multiplier to be applied to a monetary amount. 4409 */ 4410 public AddedItemDetailComponent setFactor(double value) { 4411 this.factor = new DecimalType(); 4412 this.factor.setValue(value); 4413 return this; 4414 } 4415 4416 /** 4417 * @return {@link #net} (The quantity times the unit price for an additional 4418 * service or product or charge.) 4419 */ 4420 public Money getNet() { 4421 if (this.net == null) 4422 if (Configuration.errorOnAutoCreate()) 4423 throw new Error("Attempt to auto-create AddedItemDetailComponent.net"); 4424 else if (Configuration.doAutoCreate()) 4425 this.net = new Money(); // cc 4426 return this.net; 4427 } 4428 4429 public boolean hasNet() { 4430 return this.net != null && !this.net.isEmpty(); 4431 } 4432 4433 /** 4434 * @param value {@link #net} (The quantity times the unit price for an 4435 * additional service or product or charge.) 4436 */ 4437 public AddedItemDetailComponent setNet(Money value) { 4438 this.net = value; 4439 return this; 4440 } 4441 4442 /** 4443 * @return {@link #noteNumber} (The numbers associated with notes below which 4444 * apply to the adjudication of this item.) 4445 */ 4446 public List<PositiveIntType> getNoteNumber() { 4447 if (this.noteNumber == null) 4448 this.noteNumber = new ArrayList<PositiveIntType>(); 4449 return this.noteNumber; 4450 } 4451 4452 /** 4453 * @return Returns a reference to <code>this</code> for easy method chaining 4454 */ 4455 public AddedItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 4456 this.noteNumber = theNoteNumber; 4457 return this; 4458 } 4459 4460 public boolean hasNoteNumber() { 4461 if (this.noteNumber == null) 4462 return false; 4463 for (PositiveIntType item : this.noteNumber) 4464 if (!item.isEmpty()) 4465 return true; 4466 return false; 4467 } 4468 4469 /** 4470 * @return {@link #noteNumber} (The numbers associated with notes below which 4471 * apply to the adjudication of this item.) 4472 */ 4473 public PositiveIntType addNoteNumberElement() {// 2 4474 PositiveIntType t = new PositiveIntType(); 4475 if (this.noteNumber == null) 4476 this.noteNumber = new ArrayList<PositiveIntType>(); 4477 this.noteNumber.add(t); 4478 return t; 4479 } 4480 4481 /** 4482 * @param value {@link #noteNumber} (The numbers associated with notes below 4483 * which apply to the adjudication of this item.) 4484 */ 4485 public AddedItemDetailComponent addNoteNumber(int value) { // 1 4486 PositiveIntType t = new PositiveIntType(); 4487 t.setValue(value); 4488 if (this.noteNumber == null) 4489 this.noteNumber = new ArrayList<PositiveIntType>(); 4490 this.noteNumber.add(t); 4491 return this; 4492 } 4493 4494 /** 4495 * @param value {@link #noteNumber} (The numbers associated with notes below 4496 * which apply to the adjudication of this item.) 4497 */ 4498 public boolean hasNoteNumber(int value) { 4499 if (this.noteNumber == null) 4500 return false; 4501 for (PositiveIntType v : this.noteNumber) 4502 if (v.getValue().equals(value)) // positiveInt 4503 return true; 4504 return false; 4505 } 4506 4507 /** 4508 * @return {@link #adjudication} (The adjudication results.) 4509 */ 4510 public List<AdjudicationComponent> getAdjudication() { 4511 if (this.adjudication == null) 4512 this.adjudication = new ArrayList<AdjudicationComponent>(); 4513 return this.adjudication; 4514 } 4515 4516 /** 4517 * @return Returns a reference to <code>this</code> for easy method chaining 4518 */ 4519 public AddedItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 4520 this.adjudication = theAdjudication; 4521 return this; 4522 } 4523 4524 public boolean hasAdjudication() { 4525 if (this.adjudication == null) 4526 return false; 4527 for (AdjudicationComponent item : this.adjudication) 4528 if (!item.isEmpty()) 4529 return true; 4530 return false; 4531 } 4532 4533 public AdjudicationComponent addAdjudication() { // 3 4534 AdjudicationComponent t = new AdjudicationComponent(); 4535 if (this.adjudication == null) 4536 this.adjudication = new ArrayList<AdjudicationComponent>(); 4537 this.adjudication.add(t); 4538 return t; 4539 } 4540 4541 public AddedItemDetailComponent addAdjudication(AdjudicationComponent t) { // 3 4542 if (t == null) 4543 return this; 4544 if (this.adjudication == null) 4545 this.adjudication = new ArrayList<AdjudicationComponent>(); 4546 this.adjudication.add(t); 4547 return this; 4548 } 4549 4550 /** 4551 * @return The first repetition of repeating field {@link #adjudication}, 4552 * creating it if it does not already exist 4553 */ 4554 public AdjudicationComponent getAdjudicationFirstRep() { 4555 if (getAdjudication().isEmpty()) { 4556 addAdjudication(); 4557 } 4558 return getAdjudication().get(0); 4559 } 4560 4561 /** 4562 * @return {@link #subDetail} (The third-tier service adjudications for payor 4563 * added services.) 4564 */ 4565 public List<AddedItemSubDetailComponent> getSubDetail() { 4566 if (this.subDetail == null) 4567 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4568 return this.subDetail; 4569 } 4570 4571 /** 4572 * @return Returns a reference to <code>this</code> for easy method chaining 4573 */ 4574 public AddedItemDetailComponent setSubDetail(List<AddedItemSubDetailComponent> theSubDetail) { 4575 this.subDetail = theSubDetail; 4576 return this; 4577 } 4578 4579 public boolean hasSubDetail() { 4580 if (this.subDetail == null) 4581 return false; 4582 for (AddedItemSubDetailComponent item : this.subDetail) 4583 if (!item.isEmpty()) 4584 return true; 4585 return false; 4586 } 4587 4588 public AddedItemSubDetailComponent addSubDetail() { // 3 4589 AddedItemSubDetailComponent t = new AddedItemSubDetailComponent(); 4590 if (this.subDetail == null) 4591 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4592 this.subDetail.add(t); 4593 return t; 4594 } 4595 4596 public AddedItemDetailComponent addSubDetail(AddedItemSubDetailComponent t) { // 3 4597 if (t == null) 4598 return this; 4599 if (this.subDetail == null) 4600 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4601 this.subDetail.add(t); 4602 return this; 4603 } 4604 4605 /** 4606 * @return The first repetition of repeating field {@link #subDetail}, creating 4607 * it if it does not already exist 4608 */ 4609 public AddedItemSubDetailComponent getSubDetailFirstRep() { 4610 if (getSubDetail().isEmpty()) { 4611 addSubDetail(); 4612 } 4613 return getSubDetail().get(0); 4614 } 4615 4616 protected void listChildren(List<Property> children) { 4617 super.listChildren(children); 4618 children.add(new Property("productOrService", "CodeableConcept", 4619 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 4620 0, 1, productOrService)); 4621 children.add(new Property("modifier", "CodeableConcept", 4622 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 4623 java.lang.Integer.MAX_VALUE, modifier)); 4624 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 4625 1, quantity)); 4626 children.add(new Property("unitPrice", "Money", 4627 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 4628 0, 1, unitPrice)); 4629 children.add(new Property("factor", "decimal", 4630 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 4631 0, 1, factor)); 4632 children.add(new Property("net", "Money", 4633 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 4634 children.add(new Property("noteNumber", "positiveInt", 4635 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 4636 java.lang.Integer.MAX_VALUE, noteNumber)); 4637 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 4638 java.lang.Integer.MAX_VALUE, adjudication)); 4639 children.add(new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, 4640 java.lang.Integer.MAX_VALUE, subDetail)); 4641 } 4642 4643 @Override 4644 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4645 switch (_hash) { 4646 case 1957227299: 4647 /* productOrService */ return new Property("productOrService", "CodeableConcept", 4648 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 4649 0, 1, productOrService); 4650 case -615513385: 4651 /* modifier */ return new Property("modifier", "CodeableConcept", 4652 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 4653 java.lang.Integer.MAX_VALUE, modifier); 4654 case -1285004149: 4655 /* quantity */ return new Property("quantity", "SimpleQuantity", 4656 "The number of repetitions of a service or product.", 0, 1, quantity); 4657 case -486196699: 4658 /* unitPrice */ return new Property("unitPrice", "Money", 4659 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 4660 0, 1, unitPrice); 4661 case -1282148017: 4662 /* factor */ return new Property("factor", "decimal", 4663 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 4664 0, 1, factor); 4665 case 108957: 4666 /* net */ return new Property("net", "Money", 4667 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 4668 case -1110033957: 4669 /* noteNumber */ return new Property("noteNumber", "positiveInt", 4670 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 4671 java.lang.Integer.MAX_VALUE, noteNumber); 4672 case -231349275: 4673 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 4674 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 4675 case -828829007: 4676 /* subDetail */ return new Property("subDetail", "", 4677 "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, 4678 subDetail); 4679 default: 4680 return super.getNamedProperty(_hash, _name, _checkValid); 4681 } 4682 4683 } 4684 4685 @Override 4686 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4687 switch (hash) { 4688 case 1957227299: 4689 /* productOrService */ return this.productOrService == null ? new Base[0] 4690 : new Base[] { this.productOrService }; // CodeableConcept 4691 case -615513385: 4692 /* modifier */ return this.modifier == null ? new Base[0] 4693 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4694 case -1285004149: 4695 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 4696 case -486196699: 4697 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 4698 case -1282148017: 4699 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 4700 case 108957: 4701 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 4702 case -1110033957: 4703 /* noteNumber */ return this.noteNumber == null ? new Base[0] 4704 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 4705 case -231349275: 4706 /* adjudication */ return this.adjudication == null ? new Base[0] 4707 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 4708 case -828829007: 4709 /* subDetail */ return this.subDetail == null ? new Base[0] 4710 : this.subDetail.toArray(new Base[this.subDetail.size()]); // AddedItemSubDetailComponent 4711 default: 4712 return super.getProperty(hash, name, checkValid); 4713 } 4714 4715 } 4716 4717 @Override 4718 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4719 switch (hash) { 4720 case 1957227299: // productOrService 4721 this.productOrService = castToCodeableConcept(value); // CodeableConcept 4722 return value; 4723 case -615513385: // modifier 4724 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 4725 return value; 4726 case -1285004149: // quantity 4727 this.quantity = castToQuantity(value); // Quantity 4728 return value; 4729 case -486196699: // unitPrice 4730 this.unitPrice = castToMoney(value); // Money 4731 return value; 4732 case -1282148017: // factor 4733 this.factor = castToDecimal(value); // DecimalType 4734 return value; 4735 case 108957: // net 4736 this.net = castToMoney(value); // Money 4737 return value; 4738 case -1110033957: // noteNumber 4739 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 4740 return value; 4741 case -231349275: // adjudication 4742 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 4743 return value; 4744 case -828829007: // subDetail 4745 this.getSubDetail().add((AddedItemSubDetailComponent) value); // AddedItemSubDetailComponent 4746 return value; 4747 default: 4748 return super.setProperty(hash, name, value); 4749 } 4750 4751 } 4752 4753 @Override 4754 public Base setProperty(String name, Base value) throws FHIRException { 4755 if (name.equals("productOrService")) { 4756 this.productOrService = castToCodeableConcept(value); // CodeableConcept 4757 } else if (name.equals("modifier")) { 4758 this.getModifier().add(castToCodeableConcept(value)); 4759 } else if (name.equals("quantity")) { 4760 this.quantity = castToQuantity(value); // Quantity 4761 } else if (name.equals("unitPrice")) { 4762 this.unitPrice = castToMoney(value); // Money 4763 } else if (name.equals("factor")) { 4764 this.factor = castToDecimal(value); // DecimalType 4765 } else if (name.equals("net")) { 4766 this.net = castToMoney(value); // Money 4767 } else if (name.equals("noteNumber")) { 4768 this.getNoteNumber().add(castToPositiveInt(value)); 4769 } else if (name.equals("adjudication")) { 4770 this.getAdjudication().add((AdjudicationComponent) value); 4771 } else if (name.equals("subDetail")) { 4772 this.getSubDetail().add((AddedItemSubDetailComponent) value); 4773 } else 4774 return super.setProperty(name, value); 4775 return value; 4776 } 4777 4778 @Override 4779 public void removeChild(String name, Base value) throws FHIRException { 4780 if (name.equals("productOrService")) { 4781 this.productOrService = null; 4782 } else if (name.equals("modifier")) { 4783 this.getModifier().remove(castToCodeableConcept(value)); 4784 } else if (name.equals("quantity")) { 4785 this.quantity = null; 4786 } else if (name.equals("unitPrice")) { 4787 this.unitPrice = null; 4788 } else if (name.equals("factor")) { 4789 this.factor = null; 4790 } else if (name.equals("net")) { 4791 this.net = null; 4792 } else if (name.equals("noteNumber")) { 4793 this.getNoteNumber().remove(castToPositiveInt(value)); 4794 } else if (name.equals("adjudication")) { 4795 this.getAdjudication().remove((AdjudicationComponent) value); 4796 } else if (name.equals("subDetail")) { 4797 this.getSubDetail().remove((AddedItemSubDetailComponent) value); 4798 } else 4799 super.removeChild(name, value); 4800 4801 } 4802 4803 @Override 4804 public Base makeProperty(int hash, String name) throws FHIRException { 4805 switch (hash) { 4806 case 1957227299: 4807 return getProductOrService(); 4808 case -615513385: 4809 return addModifier(); 4810 case -1285004149: 4811 return getQuantity(); 4812 case -486196699: 4813 return getUnitPrice(); 4814 case -1282148017: 4815 return getFactorElement(); 4816 case 108957: 4817 return getNet(); 4818 case -1110033957: 4819 return addNoteNumberElement(); 4820 case -231349275: 4821 return addAdjudication(); 4822 case -828829007: 4823 return addSubDetail(); 4824 default: 4825 return super.makeProperty(hash, name); 4826 } 4827 4828 } 4829 4830 @Override 4831 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4832 switch (hash) { 4833 case 1957227299: 4834 /* productOrService */ return new String[] { "CodeableConcept" }; 4835 case -615513385: 4836 /* modifier */ return new String[] { "CodeableConcept" }; 4837 case -1285004149: 4838 /* quantity */ return new String[] { "SimpleQuantity" }; 4839 case -486196699: 4840 /* unitPrice */ return new String[] { "Money" }; 4841 case -1282148017: 4842 /* factor */ return new String[] { "decimal" }; 4843 case 108957: 4844 /* net */ return new String[] { "Money" }; 4845 case -1110033957: 4846 /* noteNumber */ return new String[] { "positiveInt" }; 4847 case -231349275: 4848 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 4849 case -828829007: 4850 /* subDetail */ return new String[] {}; 4851 default: 4852 return super.getTypesForProperty(hash, name); 4853 } 4854 4855 } 4856 4857 @Override 4858 public Base addChild(String name) throws FHIRException { 4859 if (name.equals("productOrService")) { 4860 this.productOrService = new CodeableConcept(); 4861 return this.productOrService; 4862 } else if (name.equals("modifier")) { 4863 return addModifier(); 4864 } else if (name.equals("quantity")) { 4865 this.quantity = new Quantity(); 4866 return this.quantity; 4867 } else if (name.equals("unitPrice")) { 4868 this.unitPrice = new Money(); 4869 return this.unitPrice; 4870 } else if (name.equals("factor")) { 4871 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.factor"); 4872 } else if (name.equals("net")) { 4873 this.net = new Money(); 4874 return this.net; 4875 } else if (name.equals("noteNumber")) { 4876 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 4877 } else if (name.equals("adjudication")) { 4878 return addAdjudication(); 4879 } else if (name.equals("subDetail")) { 4880 return addSubDetail(); 4881 } else 4882 return super.addChild(name); 4883 } 4884 4885 public AddedItemDetailComponent copy() { 4886 AddedItemDetailComponent dst = new AddedItemDetailComponent(); 4887 copyValues(dst); 4888 return dst; 4889 } 4890 4891 public void copyValues(AddedItemDetailComponent dst) { 4892 super.copyValues(dst); 4893 dst.productOrService = productOrService == null ? null : productOrService.copy(); 4894 if (modifier != null) { 4895 dst.modifier = new ArrayList<CodeableConcept>(); 4896 for (CodeableConcept i : modifier) 4897 dst.modifier.add(i.copy()); 4898 } 4899 ; 4900 dst.quantity = quantity == null ? null : quantity.copy(); 4901 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4902 dst.factor = factor == null ? null : factor.copy(); 4903 dst.net = net == null ? null : net.copy(); 4904 if (noteNumber != null) { 4905 dst.noteNumber = new ArrayList<PositiveIntType>(); 4906 for (PositiveIntType i : noteNumber) 4907 dst.noteNumber.add(i.copy()); 4908 } 4909 ; 4910 if (adjudication != null) { 4911 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4912 for (AdjudicationComponent i : adjudication) 4913 dst.adjudication.add(i.copy()); 4914 } 4915 ; 4916 if (subDetail != null) { 4917 dst.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4918 for (AddedItemSubDetailComponent i : subDetail) 4919 dst.subDetail.add(i.copy()); 4920 } 4921 ; 4922 } 4923 4924 @Override 4925 public boolean equalsDeep(Base other_) { 4926 if (!super.equalsDeep(other_)) 4927 return false; 4928 if (!(other_ instanceof AddedItemDetailComponent)) 4929 return false; 4930 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 4931 return compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 4932 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 4933 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) 4934 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 4935 && compareDeep(subDetail, o.subDetail, true); 4936 } 4937 4938 @Override 4939 public boolean equalsShallow(Base other_) { 4940 if (!super.equalsShallow(other_)) 4941 return false; 4942 if (!(other_ instanceof AddedItemDetailComponent)) 4943 return false; 4944 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 4945 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 4946 } 4947 4948 public boolean isEmpty() { 4949 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productOrService, modifier, quantity, unitPrice, 4950 factor, net, noteNumber, adjudication, subDetail); 4951 } 4952 4953 public String fhirType() { 4954 return "ClaimResponse.addItem.detail"; 4955 4956 } 4957 4958 } 4959 4960 @Block() 4961 public static class AddedItemSubDetailComponent extends BackboneElement implements IBaseBackboneElement { 4962 /** 4963 * When the value is a group code then this item collects a set of related claim 4964 * details, otherwise this contains the product, service, drug or other billing 4965 * code for the item. 4966 */ 4967 @Child(name = "productOrService", type = { 4968 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4969 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4970 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4971 protected CodeableConcept productOrService; 4972 4973 /** 4974 * Item typification or modifiers codes to convey additional context for the 4975 * product or service. 4976 */ 4977 @Child(name = "modifier", type = { 4978 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4979 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4981 protected List<CodeableConcept> modifier; 4982 4983 /** 4984 * The number of repetitions of a service or product. 4985 */ 4986 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4987 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4988 protected Quantity quantity; 4989 4990 /** 4991 * If the item is not a group then this is the fee for the product or service, 4992 * otherwise this is the total of the fees for the details of the group. 4993 */ 4994 @Child(name = "unitPrice", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 4995 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 4996 protected Money unitPrice; 4997 4998 /** 4999 * A real number that represents a multiplier used in determining the overall 5000 * value of services delivered and/or goods received. The concept of a Factor 5001 * allows for a discount or surcharge multiplier to be applied to a monetary 5002 * amount. 5003 */ 5004 @Child(name = "factor", type = { 5005 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 5006 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 5007 protected DecimalType factor; 5008 5009 /** 5010 * The quantity times the unit price for an additional service or product or 5011 * charge. 5012 */ 5013 @Child(name = "net", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 5014 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 5015 protected Money net; 5016 5017 /** 5018 * The numbers associated with notes below which apply to the adjudication of 5019 * this item. 5020 */ 5021 @Child(name = "noteNumber", type = { 5022 PositiveIntType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5023 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 5024 protected List<PositiveIntType> noteNumber; 5025 5026 /** 5027 * The adjudication results. 5028 */ 5029 @Child(name = "adjudication", type = { 5030 AdjudicationComponent.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5031 @Description(shortDefinition = "Added items detail adjudication", formalDefinition = "The adjudication results.") 5032 protected List<AdjudicationComponent> adjudication; 5033 5034 private static final long serialVersionUID = 1301363592L; 5035 5036 /** 5037 * Constructor 5038 */ 5039 public AddedItemSubDetailComponent() { 5040 super(); 5041 } 5042 5043 /** 5044 * Constructor 5045 */ 5046 public AddedItemSubDetailComponent(CodeableConcept productOrService) { 5047 super(); 5048 this.productOrService = productOrService; 5049 } 5050 5051 /** 5052 * @return {@link #productOrService} (When the value is a group code then this 5053 * item collects a set of related claim details, otherwise this contains 5054 * the product, service, drug or other billing code for the item.) 5055 */ 5056 public CodeableConcept getProductOrService() { 5057 if (this.productOrService == null) 5058 if (Configuration.errorOnAutoCreate()) 5059 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.productOrService"); 5060 else if (Configuration.doAutoCreate()) 5061 this.productOrService = new CodeableConcept(); // cc 5062 return this.productOrService; 5063 } 5064 5065 public boolean hasProductOrService() { 5066 return this.productOrService != null && !this.productOrService.isEmpty(); 5067 } 5068 5069 /** 5070 * @param value {@link #productOrService} (When the value is a group code then 5071 * this item collects a set of related claim details, otherwise 5072 * this contains the product, service, drug or other billing code 5073 * for the item.) 5074 */ 5075 public AddedItemSubDetailComponent setProductOrService(CodeableConcept value) { 5076 this.productOrService = value; 5077 return this; 5078 } 5079 5080 /** 5081 * @return {@link #modifier} (Item typification or modifiers codes to convey 5082 * additional context for the product or service.) 5083 */ 5084 public List<CodeableConcept> getModifier() { 5085 if (this.modifier == null) 5086 this.modifier = new ArrayList<CodeableConcept>(); 5087 return this.modifier; 5088 } 5089 5090 /** 5091 * @return Returns a reference to <code>this</code> for easy method chaining 5092 */ 5093 public AddedItemSubDetailComponent setModifier(List<CodeableConcept> theModifier) { 5094 this.modifier = theModifier; 5095 return this; 5096 } 5097 5098 public boolean hasModifier() { 5099 if (this.modifier == null) 5100 return false; 5101 for (CodeableConcept item : this.modifier) 5102 if (!item.isEmpty()) 5103 return true; 5104 return false; 5105 } 5106 5107 public CodeableConcept addModifier() { // 3 5108 CodeableConcept t = new CodeableConcept(); 5109 if (this.modifier == null) 5110 this.modifier = new ArrayList<CodeableConcept>(); 5111 this.modifier.add(t); 5112 return t; 5113 } 5114 5115 public AddedItemSubDetailComponent addModifier(CodeableConcept t) { // 3 5116 if (t == null) 5117 return this; 5118 if (this.modifier == null) 5119 this.modifier = new ArrayList<CodeableConcept>(); 5120 this.modifier.add(t); 5121 return this; 5122 } 5123 5124 /** 5125 * @return The first repetition of repeating field {@link #modifier}, creating 5126 * it if it does not already exist 5127 */ 5128 public CodeableConcept getModifierFirstRep() { 5129 if (getModifier().isEmpty()) { 5130 addModifier(); 5131 } 5132 return getModifier().get(0); 5133 } 5134 5135 /** 5136 * @return {@link #quantity} (The number of repetitions of a service or 5137 * product.) 5138 */ 5139 public Quantity getQuantity() { 5140 if (this.quantity == null) 5141 if (Configuration.errorOnAutoCreate()) 5142 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.quantity"); 5143 else if (Configuration.doAutoCreate()) 5144 this.quantity = new Quantity(); // cc 5145 return this.quantity; 5146 } 5147 5148 public boolean hasQuantity() { 5149 return this.quantity != null && !this.quantity.isEmpty(); 5150 } 5151 5152 /** 5153 * @param value {@link #quantity} (The number of repetitions of a service or 5154 * product.) 5155 */ 5156 public AddedItemSubDetailComponent setQuantity(Quantity value) { 5157 this.quantity = value; 5158 return this; 5159 } 5160 5161 /** 5162 * @return {@link #unitPrice} (If the item is not a group then this is the fee 5163 * for the product or service, otherwise this is the total of the fees 5164 * for the details of the group.) 5165 */ 5166 public Money getUnitPrice() { 5167 if (this.unitPrice == null) 5168 if (Configuration.errorOnAutoCreate()) 5169 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.unitPrice"); 5170 else if (Configuration.doAutoCreate()) 5171 this.unitPrice = new Money(); // cc 5172 return this.unitPrice; 5173 } 5174 5175 public boolean hasUnitPrice() { 5176 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5177 } 5178 5179 /** 5180 * @param value {@link #unitPrice} (If the item is not a group then this is the 5181 * fee for the product or service, otherwise this is the total of 5182 * the fees for the details of the group.) 5183 */ 5184 public AddedItemSubDetailComponent setUnitPrice(Money value) { 5185 this.unitPrice = value; 5186 return this; 5187 } 5188 5189 /** 5190 * @return {@link #factor} (A real number that represents a multiplier used in 5191 * determining the overall value of services delivered and/or goods 5192 * received. The concept of a Factor allows for a discount or surcharge 5193 * multiplier to be applied to a monetary amount.). This is the 5194 * underlying object with id, value and extensions. The accessor 5195 * "getFactor" gives direct access to the value 5196 */ 5197 public DecimalType getFactorElement() { 5198 if (this.factor == null) 5199 if (Configuration.errorOnAutoCreate()) 5200 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.factor"); 5201 else if (Configuration.doAutoCreate()) 5202 this.factor = new DecimalType(); // bb 5203 return this.factor; 5204 } 5205 5206 public boolean hasFactorElement() { 5207 return this.factor != null && !this.factor.isEmpty(); 5208 } 5209 5210 public boolean hasFactor() { 5211 return this.factor != null && !this.factor.isEmpty(); 5212 } 5213 5214 /** 5215 * @param value {@link #factor} (A real number that represents a multiplier used 5216 * in determining the overall value of services delivered and/or 5217 * goods received. The concept of a Factor allows for a discount or 5218 * surcharge multiplier to be applied to a monetary amount.). This 5219 * is the underlying object with id, value and extensions. The 5220 * accessor "getFactor" gives direct access to the value 5221 */ 5222 public AddedItemSubDetailComponent setFactorElement(DecimalType value) { 5223 this.factor = value; 5224 return this; 5225 } 5226 5227 /** 5228 * @return A real number that represents a multiplier used in determining the 5229 * overall value of services delivered and/or goods received. The 5230 * concept of a Factor allows for a discount or surcharge multiplier to 5231 * be applied to a monetary amount. 5232 */ 5233 public BigDecimal getFactor() { 5234 return this.factor == null ? null : this.factor.getValue(); 5235 } 5236 5237 /** 5238 * @param value A real number that represents a multiplier used in determining 5239 * the overall value of services delivered and/or goods received. 5240 * The concept of a Factor allows for a discount or surcharge 5241 * multiplier to be applied to a monetary amount. 5242 */ 5243 public AddedItemSubDetailComponent setFactor(BigDecimal value) { 5244 if (value == null) 5245 this.factor = null; 5246 else { 5247 if (this.factor == null) 5248 this.factor = new DecimalType(); 5249 this.factor.setValue(value); 5250 } 5251 return this; 5252 } 5253 5254 /** 5255 * @param value A real number that represents a multiplier used in determining 5256 * the overall value of services delivered and/or goods received. 5257 * The concept of a Factor allows for a discount or surcharge 5258 * multiplier to be applied to a monetary amount. 5259 */ 5260 public AddedItemSubDetailComponent setFactor(long value) { 5261 this.factor = new DecimalType(); 5262 this.factor.setValue(value); 5263 return this; 5264 } 5265 5266 /** 5267 * @param value A real number that represents a multiplier used in determining 5268 * the overall value of services delivered and/or goods received. 5269 * The concept of a Factor allows for a discount or surcharge 5270 * multiplier to be applied to a monetary amount. 5271 */ 5272 public AddedItemSubDetailComponent setFactor(double value) { 5273 this.factor = new DecimalType(); 5274 this.factor.setValue(value); 5275 return this; 5276 } 5277 5278 /** 5279 * @return {@link #net} (The quantity times the unit price for an additional 5280 * service or product or charge.) 5281 */ 5282 public Money getNet() { 5283 if (this.net == null) 5284 if (Configuration.errorOnAutoCreate()) 5285 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.net"); 5286 else if (Configuration.doAutoCreate()) 5287 this.net = new Money(); // cc 5288 return this.net; 5289 } 5290 5291 public boolean hasNet() { 5292 return this.net != null && !this.net.isEmpty(); 5293 } 5294 5295 /** 5296 * @param value {@link #net} (The quantity times the unit price for an 5297 * additional service or product or charge.) 5298 */ 5299 public AddedItemSubDetailComponent setNet(Money value) { 5300 this.net = value; 5301 return this; 5302 } 5303 5304 /** 5305 * @return {@link #noteNumber} (The numbers associated with notes below which 5306 * apply to the adjudication of this item.) 5307 */ 5308 public List<PositiveIntType> getNoteNumber() { 5309 if (this.noteNumber == null) 5310 this.noteNumber = new ArrayList<PositiveIntType>(); 5311 return this.noteNumber; 5312 } 5313 5314 /** 5315 * @return Returns a reference to <code>this</code> for easy method chaining 5316 */ 5317 public AddedItemSubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 5318 this.noteNumber = theNoteNumber; 5319 return this; 5320 } 5321 5322 public boolean hasNoteNumber() { 5323 if (this.noteNumber == null) 5324 return false; 5325 for (PositiveIntType item : this.noteNumber) 5326 if (!item.isEmpty()) 5327 return true; 5328 return false; 5329 } 5330 5331 /** 5332 * @return {@link #noteNumber} (The numbers associated with notes below which 5333 * apply to the adjudication of this item.) 5334 */ 5335 public PositiveIntType addNoteNumberElement() {// 2 5336 PositiveIntType t = new PositiveIntType(); 5337 if (this.noteNumber == null) 5338 this.noteNumber = new ArrayList<PositiveIntType>(); 5339 this.noteNumber.add(t); 5340 return t; 5341 } 5342 5343 /** 5344 * @param value {@link #noteNumber} (The numbers associated with notes below 5345 * which apply to the adjudication of this item.) 5346 */ 5347 public AddedItemSubDetailComponent addNoteNumber(int value) { // 1 5348 PositiveIntType t = new PositiveIntType(); 5349 t.setValue(value); 5350 if (this.noteNumber == null) 5351 this.noteNumber = new ArrayList<PositiveIntType>(); 5352 this.noteNumber.add(t); 5353 return this; 5354 } 5355 5356 /** 5357 * @param value {@link #noteNumber} (The numbers associated with notes below 5358 * which apply to the adjudication of this item.) 5359 */ 5360 public boolean hasNoteNumber(int value) { 5361 if (this.noteNumber == null) 5362 return false; 5363 for (PositiveIntType v : this.noteNumber) 5364 if (v.getValue().equals(value)) // positiveInt 5365 return true; 5366 return false; 5367 } 5368 5369 /** 5370 * @return {@link #adjudication} (The adjudication results.) 5371 */ 5372 public List<AdjudicationComponent> getAdjudication() { 5373 if (this.adjudication == null) 5374 this.adjudication = new ArrayList<AdjudicationComponent>(); 5375 return this.adjudication; 5376 } 5377 5378 /** 5379 * @return Returns a reference to <code>this</code> for easy method chaining 5380 */ 5381 public AddedItemSubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 5382 this.adjudication = theAdjudication; 5383 return this; 5384 } 5385 5386 public boolean hasAdjudication() { 5387 if (this.adjudication == null) 5388 return false; 5389 for (AdjudicationComponent item : this.adjudication) 5390 if (!item.isEmpty()) 5391 return true; 5392 return false; 5393 } 5394 5395 public AdjudicationComponent addAdjudication() { // 3 5396 AdjudicationComponent t = new AdjudicationComponent(); 5397 if (this.adjudication == null) 5398 this.adjudication = new ArrayList<AdjudicationComponent>(); 5399 this.adjudication.add(t); 5400 return t; 5401 } 5402 5403 public AddedItemSubDetailComponent addAdjudication(AdjudicationComponent t) { // 3 5404 if (t == null) 5405 return this; 5406 if (this.adjudication == null) 5407 this.adjudication = new ArrayList<AdjudicationComponent>(); 5408 this.adjudication.add(t); 5409 return this; 5410 } 5411 5412 /** 5413 * @return The first repetition of repeating field {@link #adjudication}, 5414 * creating it if it does not already exist 5415 */ 5416 public AdjudicationComponent getAdjudicationFirstRep() { 5417 if (getAdjudication().isEmpty()) { 5418 addAdjudication(); 5419 } 5420 return getAdjudication().get(0); 5421 } 5422 5423 protected void listChildren(List<Property> children) { 5424 super.listChildren(children); 5425 children.add(new Property("productOrService", "CodeableConcept", 5426 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5427 0, 1, productOrService)); 5428 children.add(new Property("modifier", "CodeableConcept", 5429 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5430 java.lang.Integer.MAX_VALUE, modifier)); 5431 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 5432 1, quantity)); 5433 children.add(new Property("unitPrice", "Money", 5434 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5435 0, 1, unitPrice)); 5436 children.add(new Property("factor", "decimal", 5437 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5438 0, 1, factor)); 5439 children.add(new Property("net", "Money", 5440 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 5441 children.add(new Property("noteNumber", "positiveInt", 5442 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 5443 java.lang.Integer.MAX_VALUE, noteNumber)); 5444 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 5445 java.lang.Integer.MAX_VALUE, adjudication)); 5446 } 5447 5448 @Override 5449 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5450 switch (_hash) { 5451 case 1957227299: 5452 /* productOrService */ return new Property("productOrService", "CodeableConcept", 5453 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5454 0, 1, productOrService); 5455 case -615513385: 5456 /* modifier */ return new Property("modifier", "CodeableConcept", 5457 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5458 java.lang.Integer.MAX_VALUE, modifier); 5459 case -1285004149: 5460 /* quantity */ return new Property("quantity", "SimpleQuantity", 5461 "The number of repetitions of a service or product.", 0, 1, quantity); 5462 case -486196699: 5463 /* unitPrice */ return new Property("unitPrice", "Money", 5464 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5465 0, 1, unitPrice); 5466 case -1282148017: 5467 /* factor */ return new Property("factor", "decimal", 5468 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5469 0, 1, factor); 5470 case 108957: 5471 /* net */ return new Property("net", "Money", 5472 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 5473 case -1110033957: 5474 /* noteNumber */ return new Property("noteNumber", "positiveInt", 5475 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 5476 java.lang.Integer.MAX_VALUE, noteNumber); 5477 case -231349275: 5478 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 5479 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 5480 default: 5481 return super.getNamedProperty(_hash, _name, _checkValid); 5482 } 5483 5484 } 5485 5486 @Override 5487 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5488 switch (hash) { 5489 case 1957227299: 5490 /* productOrService */ return this.productOrService == null ? new Base[0] 5491 : new Base[] { this.productOrService }; // CodeableConcept 5492 case -615513385: 5493 /* modifier */ return this.modifier == null ? new Base[0] 5494 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5495 case -1285004149: 5496 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5497 case -486196699: 5498 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 5499 case -1282148017: 5500 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 5501 case 108957: 5502 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 5503 case -1110033957: 5504 /* noteNumber */ return this.noteNumber == null ? new Base[0] 5505 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5506 case -231349275: 5507 /* adjudication */ return this.adjudication == null ? new Base[0] 5508 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5509 default: 5510 return super.getProperty(hash, name, checkValid); 5511 } 5512 5513 } 5514 5515 @Override 5516 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5517 switch (hash) { 5518 case 1957227299: // productOrService 5519 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5520 return value; 5521 case -615513385: // modifier 5522 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5523 return value; 5524 case -1285004149: // quantity 5525 this.quantity = castToQuantity(value); // Quantity 5526 return value; 5527 case -486196699: // unitPrice 5528 this.unitPrice = castToMoney(value); // Money 5529 return value; 5530 case -1282148017: // factor 5531 this.factor = castToDecimal(value); // DecimalType 5532 return value; 5533 case 108957: // net 5534 this.net = castToMoney(value); // Money 5535 return value; 5536 case -1110033957: // noteNumber 5537 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 5538 return value; 5539 case -231349275: // adjudication 5540 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5541 return value; 5542 default: 5543 return super.setProperty(hash, name, value); 5544 } 5545 5546 } 5547 5548 @Override 5549 public Base setProperty(String name, Base value) throws FHIRException { 5550 if (name.equals("productOrService")) { 5551 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5552 } else if (name.equals("modifier")) { 5553 this.getModifier().add(castToCodeableConcept(value)); 5554 } else if (name.equals("quantity")) { 5555 this.quantity = castToQuantity(value); // Quantity 5556 } else if (name.equals("unitPrice")) { 5557 this.unitPrice = castToMoney(value); // Money 5558 } else if (name.equals("factor")) { 5559 this.factor = castToDecimal(value); // DecimalType 5560 } else if (name.equals("net")) { 5561 this.net = castToMoney(value); // Money 5562 } else if (name.equals("noteNumber")) { 5563 this.getNoteNumber().add(castToPositiveInt(value)); 5564 } else if (name.equals("adjudication")) { 5565 this.getAdjudication().add((AdjudicationComponent) value); 5566 } else 5567 return super.setProperty(name, value); 5568 return value; 5569 } 5570 5571 @Override 5572 public void removeChild(String name, Base value) throws FHIRException { 5573 if (name.equals("productOrService")) { 5574 this.productOrService = null; 5575 } else if (name.equals("modifier")) { 5576 this.getModifier().remove(castToCodeableConcept(value)); 5577 } else if (name.equals("quantity")) { 5578 this.quantity = null; 5579 } else if (name.equals("unitPrice")) { 5580 this.unitPrice = null; 5581 } else if (name.equals("factor")) { 5582 this.factor = null; 5583 } else if (name.equals("net")) { 5584 this.net = null; 5585 } else if (name.equals("noteNumber")) { 5586 this.getNoteNumber().remove(castToPositiveInt(value)); 5587 } else if (name.equals("adjudication")) { 5588 this.getAdjudication().remove((AdjudicationComponent) value); 5589 } else 5590 super.removeChild(name, value); 5591 5592 } 5593 5594 @Override 5595 public Base makeProperty(int hash, String name) throws FHIRException { 5596 switch (hash) { 5597 case 1957227299: 5598 return getProductOrService(); 5599 case -615513385: 5600 return addModifier(); 5601 case -1285004149: 5602 return getQuantity(); 5603 case -486196699: 5604 return getUnitPrice(); 5605 case -1282148017: 5606 return getFactorElement(); 5607 case 108957: 5608 return getNet(); 5609 case -1110033957: 5610 return addNoteNumberElement(); 5611 case -231349275: 5612 return addAdjudication(); 5613 default: 5614 return super.makeProperty(hash, name); 5615 } 5616 5617 } 5618 5619 @Override 5620 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5621 switch (hash) { 5622 case 1957227299: 5623 /* productOrService */ return new String[] { "CodeableConcept" }; 5624 case -615513385: 5625 /* modifier */ return new String[] { "CodeableConcept" }; 5626 case -1285004149: 5627 /* quantity */ return new String[] { "SimpleQuantity" }; 5628 case -486196699: 5629 /* unitPrice */ return new String[] { "Money" }; 5630 case -1282148017: 5631 /* factor */ return new String[] { "decimal" }; 5632 case 108957: 5633 /* net */ return new String[] { "Money" }; 5634 case -1110033957: 5635 /* noteNumber */ return new String[] { "positiveInt" }; 5636 case -231349275: 5637 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 5638 default: 5639 return super.getTypesForProperty(hash, name); 5640 } 5641 5642 } 5643 5644 @Override 5645 public Base addChild(String name) throws FHIRException { 5646 if (name.equals("productOrService")) { 5647 this.productOrService = new CodeableConcept(); 5648 return this.productOrService; 5649 } else if (name.equals("modifier")) { 5650 return addModifier(); 5651 } else if (name.equals("quantity")) { 5652 this.quantity = new Quantity(); 5653 return this.quantity; 5654 } else if (name.equals("unitPrice")) { 5655 this.unitPrice = new Money(); 5656 return this.unitPrice; 5657 } else if (name.equals("factor")) { 5658 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.factor"); 5659 } else if (name.equals("net")) { 5660 this.net = new Money(); 5661 return this.net; 5662 } else if (name.equals("noteNumber")) { 5663 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 5664 } else if (name.equals("adjudication")) { 5665 return addAdjudication(); 5666 } else 5667 return super.addChild(name); 5668 } 5669 5670 public AddedItemSubDetailComponent copy() { 5671 AddedItemSubDetailComponent dst = new AddedItemSubDetailComponent(); 5672 copyValues(dst); 5673 return dst; 5674 } 5675 5676 public void copyValues(AddedItemSubDetailComponent dst) { 5677 super.copyValues(dst); 5678 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5679 if (modifier != null) { 5680 dst.modifier = new ArrayList<CodeableConcept>(); 5681 for (CodeableConcept i : modifier) 5682 dst.modifier.add(i.copy()); 5683 } 5684 ; 5685 dst.quantity = quantity == null ? null : quantity.copy(); 5686 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5687 dst.factor = factor == null ? null : factor.copy(); 5688 dst.net = net == null ? null : net.copy(); 5689 if (noteNumber != null) { 5690 dst.noteNumber = new ArrayList<PositiveIntType>(); 5691 for (PositiveIntType i : noteNumber) 5692 dst.noteNumber.add(i.copy()); 5693 } 5694 ; 5695 if (adjudication != null) { 5696 dst.adjudication = new ArrayList<AdjudicationComponent>(); 5697 for (AdjudicationComponent i : adjudication) 5698 dst.adjudication.add(i.copy()); 5699 } 5700 ; 5701 } 5702 5703 @Override 5704 public boolean equalsDeep(Base other_) { 5705 if (!super.equalsDeep(other_)) 5706 return false; 5707 if (!(other_ instanceof AddedItemSubDetailComponent)) 5708 return false; 5709 AddedItemSubDetailComponent o = (AddedItemSubDetailComponent) other_; 5710 return compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 5711 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 5712 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) 5713 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true); 5714 } 5715 5716 @Override 5717 public boolean equalsShallow(Base other_) { 5718 if (!super.equalsShallow(other_)) 5719 return false; 5720 if (!(other_ instanceof AddedItemSubDetailComponent)) 5721 return false; 5722 AddedItemSubDetailComponent o = (AddedItemSubDetailComponent) other_; 5723 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 5724 } 5725 5726 public boolean isEmpty() { 5727 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productOrService, modifier, quantity, unitPrice, 5728 factor, net, noteNumber, adjudication); 5729 } 5730 5731 public String fhirType() { 5732 return "ClaimResponse.addItem.detail.subDetail"; 5733 5734 } 5735 5736 } 5737 5738 @Block() 5739 public static class TotalComponent extends BackboneElement implements IBaseBackboneElement { 5740 /** 5741 * A code to indicate the information type of this adjudication record. 5742 * Information types may include: the value submitted, maximum values or 5743 * percentages allowed or payable under the plan, amounts that the patient is 5744 * responsible for in aggregate or pertaining to this item, amounts paid by 5745 * other coverages, and the benefit payable for this item. 5746 */ 5747 @Child(name = "category", type = { 5748 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 5749 @Description(shortDefinition = "Type of adjudication information", formalDefinition = "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.") 5750 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication") 5751 protected CodeableConcept category; 5752 5753 /** 5754 * Monetary total amount associated with the category. 5755 */ 5756 @Child(name = "amount", type = { Money.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 5757 @Description(shortDefinition = "Financial total for the category", formalDefinition = "Monetary total amount associated with the category.") 5758 protected Money amount; 5759 5760 private static final long serialVersionUID = 2012310309L; 5761 5762 /** 5763 * Constructor 5764 */ 5765 public TotalComponent() { 5766 super(); 5767 } 5768 5769 /** 5770 * Constructor 5771 */ 5772 public TotalComponent(CodeableConcept category, Money amount) { 5773 super(); 5774 this.category = category; 5775 this.amount = amount; 5776 } 5777 5778 /** 5779 * @return {@link #category} (A code to indicate the information type of this 5780 * adjudication record. Information types may include: the value 5781 * submitted, maximum values or percentages allowed or payable under the 5782 * plan, amounts that the patient is responsible for in aggregate or 5783 * pertaining to this item, amounts paid by other coverages, and the 5784 * benefit payable for this item.) 5785 */ 5786 public CodeableConcept getCategory() { 5787 if (this.category == null) 5788 if (Configuration.errorOnAutoCreate()) 5789 throw new Error("Attempt to auto-create TotalComponent.category"); 5790 else if (Configuration.doAutoCreate()) 5791 this.category = new CodeableConcept(); // cc 5792 return this.category; 5793 } 5794 5795 public boolean hasCategory() { 5796 return this.category != null && !this.category.isEmpty(); 5797 } 5798 5799 /** 5800 * @param value {@link #category} (A code to indicate the information type of 5801 * this adjudication record. Information types may include: the 5802 * value submitted, maximum values or percentages allowed or 5803 * payable under the plan, amounts that the patient is responsible 5804 * for in aggregate or pertaining to this item, amounts paid by 5805 * other coverages, and the benefit payable for this item.) 5806 */ 5807 public TotalComponent setCategory(CodeableConcept value) { 5808 this.category = value; 5809 return this; 5810 } 5811 5812 /** 5813 * @return {@link #amount} (Monetary total amount associated with the category.) 5814 */ 5815 public Money getAmount() { 5816 if (this.amount == null) 5817 if (Configuration.errorOnAutoCreate()) 5818 throw new Error("Attempt to auto-create TotalComponent.amount"); 5819 else if (Configuration.doAutoCreate()) 5820 this.amount = new Money(); // cc 5821 return this.amount; 5822 } 5823 5824 public boolean hasAmount() { 5825 return this.amount != null && !this.amount.isEmpty(); 5826 } 5827 5828 /** 5829 * @param value {@link #amount} (Monetary total amount associated with the 5830 * category.) 5831 */ 5832 public TotalComponent setAmount(Money value) { 5833 this.amount = value; 5834 return this; 5835 } 5836 5837 protected void listChildren(List<Property> children) { 5838 super.listChildren(children); 5839 children.add(new Property("category", "CodeableConcept", 5840 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 5841 0, 1, category)); 5842 children 5843 .add(new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount)); 5844 } 5845 5846 @Override 5847 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5848 switch (_hash) { 5849 case 50511102: 5850 /* category */ return new Property("category", "CodeableConcept", 5851 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 5852 0, 1, category); 5853 case -1413853096: 5854 /* amount */ return new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, 5855 amount); 5856 default: 5857 return super.getNamedProperty(_hash, _name, _checkValid); 5858 } 5859 5860 } 5861 5862 @Override 5863 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5864 switch (hash) { 5865 case 50511102: 5866 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 5867 case -1413853096: 5868 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 5869 default: 5870 return super.getProperty(hash, name, checkValid); 5871 } 5872 5873 } 5874 5875 @Override 5876 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5877 switch (hash) { 5878 case 50511102: // category 5879 this.category = castToCodeableConcept(value); // CodeableConcept 5880 return value; 5881 case -1413853096: // amount 5882 this.amount = castToMoney(value); // Money 5883 return value; 5884 default: 5885 return super.setProperty(hash, name, value); 5886 } 5887 5888 } 5889 5890 @Override 5891 public Base setProperty(String name, Base value) throws FHIRException { 5892 if (name.equals("category")) { 5893 this.category = castToCodeableConcept(value); // CodeableConcept 5894 } else if (name.equals("amount")) { 5895 this.amount = castToMoney(value); // Money 5896 } else 5897 return super.setProperty(name, value); 5898 return value; 5899 } 5900 5901 @Override 5902 public void removeChild(String name, Base value) throws FHIRException { 5903 if (name.equals("category")) { 5904 this.category = null; 5905 } else if (name.equals("amount")) { 5906 this.amount = null; 5907 } else 5908 super.removeChild(name, value); 5909 5910 } 5911 5912 @Override 5913 public Base makeProperty(int hash, String name) throws FHIRException { 5914 switch (hash) { 5915 case 50511102: 5916 return getCategory(); 5917 case -1413853096: 5918 return getAmount(); 5919 default: 5920 return super.makeProperty(hash, name); 5921 } 5922 5923 } 5924 5925 @Override 5926 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5927 switch (hash) { 5928 case 50511102: 5929 /* category */ return new String[] { "CodeableConcept" }; 5930 case -1413853096: 5931 /* amount */ return new String[] { "Money" }; 5932 default: 5933 return super.getTypesForProperty(hash, name); 5934 } 5935 5936 } 5937 5938 @Override 5939 public Base addChild(String name) throws FHIRException { 5940 if (name.equals("category")) { 5941 this.category = new CodeableConcept(); 5942 return this.category; 5943 } else if (name.equals("amount")) { 5944 this.amount = new Money(); 5945 return this.amount; 5946 } else 5947 return super.addChild(name); 5948 } 5949 5950 public TotalComponent copy() { 5951 TotalComponent dst = new TotalComponent(); 5952 copyValues(dst); 5953 return dst; 5954 } 5955 5956 public void copyValues(TotalComponent dst) { 5957 super.copyValues(dst); 5958 dst.category = category == null ? null : category.copy(); 5959 dst.amount = amount == null ? null : amount.copy(); 5960 } 5961 5962 @Override 5963 public boolean equalsDeep(Base other_) { 5964 if (!super.equalsDeep(other_)) 5965 return false; 5966 if (!(other_ instanceof TotalComponent)) 5967 return false; 5968 TotalComponent o = (TotalComponent) other_; 5969 return compareDeep(category, o.category, true) && compareDeep(amount, o.amount, true); 5970 } 5971 5972 @Override 5973 public boolean equalsShallow(Base other_) { 5974 if (!super.equalsShallow(other_)) 5975 return false; 5976 if (!(other_ instanceof TotalComponent)) 5977 return false; 5978 TotalComponent o = (TotalComponent) other_; 5979 return true; 5980 } 5981 5982 public boolean isEmpty() { 5983 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, amount); 5984 } 5985 5986 public String fhirType() { 5987 return "ClaimResponse.total"; 5988 5989 } 5990 5991 } 5992 5993 @Block() 5994 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 5995 /** 5996 * Whether this represents partial or complete payment of the benefits payable. 5997 */ 5998 @Child(name = "type", type = { 5999 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6000 @Description(shortDefinition = "Partial or complete payment", formalDefinition = "Whether this represents partial or complete payment of the benefits payable.") 6001 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-paymenttype") 6002 protected CodeableConcept type; 6003 6004 /** 6005 * Total amount of all adjustments to this payment included in this transaction 6006 * which are not related to this claim's adjudication. 6007 */ 6008 @Child(name = "adjustment", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6009 @Description(shortDefinition = "Payment adjustment for non-claim issues", formalDefinition = "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.") 6010 protected Money adjustment; 6011 6012 /** 6013 * Reason for the payment adjustment. 6014 */ 6015 @Child(name = "adjustmentReason", type = { 6016 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6017 @Description(shortDefinition = "Explanation for the adjustment", formalDefinition = "Reason for the payment adjustment.") 6018 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 6019 protected CodeableConcept adjustmentReason; 6020 6021 /** 6022 * Estimated date the payment will be issued or the actual issue date of 6023 * payment. 6024 */ 6025 @Child(name = "date", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6026 @Description(shortDefinition = "Expected date of payment", formalDefinition = "Estimated date the payment will be issued or the actual issue date of payment.") 6027 protected DateType date; 6028 6029 /** 6030 * Benefits payable less any payment adjustment. 6031 */ 6032 @Child(name = "amount", type = { Money.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 6033 @Description(shortDefinition = "Payable amount after adjustment", formalDefinition = "Benefits payable less any payment adjustment.") 6034 protected Money amount; 6035 6036 /** 6037 * Issuer's unique identifier for the payment instrument. 6038 */ 6039 @Child(name = "identifier", type = { 6040 Identifier.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6041 @Description(shortDefinition = "Business identifier for the payment", formalDefinition = "Issuer's unique identifier for the payment instrument.") 6042 protected Identifier identifier; 6043 6044 private static final long serialVersionUID = 1539906026L; 6045 6046 /** 6047 * Constructor 6048 */ 6049 public PaymentComponent() { 6050 super(); 6051 } 6052 6053 /** 6054 * Constructor 6055 */ 6056 public PaymentComponent(CodeableConcept type, Money amount) { 6057 super(); 6058 this.type = type; 6059 this.amount = amount; 6060 } 6061 6062 /** 6063 * @return {@link #type} (Whether this represents partial or complete payment of 6064 * the benefits payable.) 6065 */ 6066 public CodeableConcept getType() { 6067 if (this.type == null) 6068 if (Configuration.errorOnAutoCreate()) 6069 throw new Error("Attempt to auto-create PaymentComponent.type"); 6070 else if (Configuration.doAutoCreate()) 6071 this.type = new CodeableConcept(); // cc 6072 return this.type; 6073 } 6074 6075 public boolean hasType() { 6076 return this.type != null && !this.type.isEmpty(); 6077 } 6078 6079 /** 6080 * @param value {@link #type} (Whether this represents partial or complete 6081 * payment of the benefits payable.) 6082 */ 6083 public PaymentComponent setType(CodeableConcept value) { 6084 this.type = value; 6085 return this; 6086 } 6087 6088 /** 6089 * @return {@link #adjustment} (Total amount of all adjustments to this payment 6090 * included in this transaction which are not related to this claim's 6091 * adjudication.) 6092 */ 6093 public Money getAdjustment() { 6094 if (this.adjustment == null) 6095 if (Configuration.errorOnAutoCreate()) 6096 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 6097 else if (Configuration.doAutoCreate()) 6098 this.adjustment = new Money(); // cc 6099 return this.adjustment; 6100 } 6101 6102 public boolean hasAdjustment() { 6103 return this.adjustment != null && !this.adjustment.isEmpty(); 6104 } 6105 6106 /** 6107 * @param value {@link #adjustment} (Total amount of all adjustments to this 6108 * payment included in this transaction which are not related to 6109 * this claim's adjudication.) 6110 */ 6111 public PaymentComponent setAdjustment(Money value) { 6112 this.adjustment = value; 6113 return this; 6114 } 6115 6116 /** 6117 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 6118 */ 6119 public CodeableConcept getAdjustmentReason() { 6120 if (this.adjustmentReason == null) 6121 if (Configuration.errorOnAutoCreate()) 6122 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 6123 else if (Configuration.doAutoCreate()) 6124 this.adjustmentReason = new CodeableConcept(); // cc 6125 return this.adjustmentReason; 6126 } 6127 6128 public boolean hasAdjustmentReason() { 6129 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 6130 } 6131 6132 /** 6133 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 6134 */ 6135 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 6136 this.adjustmentReason = value; 6137 return this; 6138 } 6139 6140 /** 6141 * @return {@link #date} (Estimated date the payment will be issued or the 6142 * actual issue date of payment.). This is the underlying object with 6143 * id, value and extensions. The accessor "getDate" gives direct access 6144 * to the value 6145 */ 6146 public DateType getDateElement() { 6147 if (this.date == null) 6148 if (Configuration.errorOnAutoCreate()) 6149 throw new Error("Attempt to auto-create PaymentComponent.date"); 6150 else if (Configuration.doAutoCreate()) 6151 this.date = new DateType(); // bb 6152 return this.date; 6153 } 6154 6155 public boolean hasDateElement() { 6156 return this.date != null && !this.date.isEmpty(); 6157 } 6158 6159 public boolean hasDate() { 6160 return this.date != null && !this.date.isEmpty(); 6161 } 6162 6163 /** 6164 * @param value {@link #date} (Estimated date the payment will be issued or the 6165 * actual issue date of payment.). This is the underlying object 6166 * with id, value and extensions. The accessor "getDate" gives 6167 * direct access to the value 6168 */ 6169 public PaymentComponent setDateElement(DateType value) { 6170 this.date = value; 6171 return this; 6172 } 6173 6174 /** 6175 * @return Estimated date the payment will be issued or the actual issue date of 6176 * payment. 6177 */ 6178 public Date getDate() { 6179 return this.date == null ? null : this.date.getValue(); 6180 } 6181 6182 /** 6183 * @param value Estimated date the payment will be issued or the actual issue 6184 * date of payment. 6185 */ 6186 public PaymentComponent setDate(Date value) { 6187 if (value == null) 6188 this.date = null; 6189 else { 6190 if (this.date == null) 6191 this.date = new DateType(); 6192 this.date.setValue(value); 6193 } 6194 return this; 6195 } 6196 6197 /** 6198 * @return {@link #amount} (Benefits payable less any payment adjustment.) 6199 */ 6200 public Money getAmount() { 6201 if (this.amount == null) 6202 if (Configuration.errorOnAutoCreate()) 6203 throw new Error("Attempt to auto-create PaymentComponent.amount"); 6204 else if (Configuration.doAutoCreate()) 6205 this.amount = new Money(); // cc 6206 return this.amount; 6207 } 6208 6209 public boolean hasAmount() { 6210 return this.amount != null && !this.amount.isEmpty(); 6211 } 6212 6213 /** 6214 * @param value {@link #amount} (Benefits payable less any payment adjustment.) 6215 */ 6216 public PaymentComponent setAmount(Money value) { 6217 this.amount = value; 6218 return this; 6219 } 6220 6221 /** 6222 * @return {@link #identifier} (Issuer's unique identifier for the payment 6223 * instrument.) 6224 */ 6225 public Identifier getIdentifier() { 6226 if (this.identifier == null) 6227 if (Configuration.errorOnAutoCreate()) 6228 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 6229 else if (Configuration.doAutoCreate()) 6230 this.identifier = new Identifier(); // cc 6231 return this.identifier; 6232 } 6233 6234 public boolean hasIdentifier() { 6235 return this.identifier != null && !this.identifier.isEmpty(); 6236 } 6237 6238 /** 6239 * @param value {@link #identifier} (Issuer's unique identifier for the payment 6240 * instrument.) 6241 */ 6242 public PaymentComponent setIdentifier(Identifier value) { 6243 this.identifier = value; 6244 return this; 6245 } 6246 6247 protected void listChildren(List<Property> children) { 6248 super.listChildren(children); 6249 children.add(new Property("type", "CodeableConcept", 6250 "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type)); 6251 children.add(new Property("adjustment", "Money", 6252 "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 6253 0, 1, adjustment)); 6254 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, 6255 adjustmentReason)); 6256 children.add(new Property("date", "date", 6257 "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date)); 6258 children.add(new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount)); 6259 children.add(new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 6260 1, identifier)); 6261 } 6262 6263 @Override 6264 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6265 switch (_hash) { 6266 case 3575610: 6267 /* type */ return new Property("type", "CodeableConcept", 6268 "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type); 6269 case 1977085293: 6270 /* adjustment */ return new Property("adjustment", "Money", 6271 "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 6272 0, 1, adjustment); 6273 case -1255938543: 6274 /* adjustmentReason */ return new Property("adjustmentReason", "CodeableConcept", 6275 "Reason for the payment adjustment.", 0, 1, adjustmentReason); 6276 case 3076014: 6277 /* date */ return new Property("date", "date", 6278 "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date); 6279 case -1413853096: 6280 /* amount */ return new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, 6281 amount); 6282 case -1618432855: 6283 /* identifier */ return new Property("identifier", "Identifier", 6284 "Issuer's unique identifier for the payment instrument.", 0, 1, identifier); 6285 default: 6286 return super.getNamedProperty(_hash, _name, _checkValid); 6287 } 6288 6289 } 6290 6291 @Override 6292 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6293 switch (hash) { 6294 case 3575610: 6295 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 6296 case 1977085293: 6297 /* adjustment */ return this.adjustment == null ? new Base[0] : new Base[] { this.adjustment }; // Money 6298 case -1255938543: 6299 /* adjustmentReason */ return this.adjustmentReason == null ? new Base[0] 6300 : new Base[] { this.adjustmentReason }; // CodeableConcept 6301 case 3076014: 6302 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 6303 case -1413853096: 6304 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 6305 case -1618432855: 6306 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 6307 default: 6308 return super.getProperty(hash, name, checkValid); 6309 } 6310 6311 } 6312 6313 @Override 6314 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6315 switch (hash) { 6316 case 3575610: // type 6317 this.type = castToCodeableConcept(value); // CodeableConcept 6318 return value; 6319 case 1977085293: // adjustment 6320 this.adjustment = castToMoney(value); // Money 6321 return value; 6322 case -1255938543: // adjustmentReason 6323 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 6324 return value; 6325 case 3076014: // date 6326 this.date = castToDate(value); // DateType 6327 return value; 6328 case -1413853096: // amount 6329 this.amount = castToMoney(value); // Money 6330 return value; 6331 case -1618432855: // identifier 6332 this.identifier = castToIdentifier(value); // Identifier 6333 return value; 6334 default: 6335 return super.setProperty(hash, name, value); 6336 } 6337 6338 } 6339 6340 @Override 6341 public Base setProperty(String name, Base value) throws FHIRException { 6342 if (name.equals("type")) { 6343 this.type = castToCodeableConcept(value); // CodeableConcept 6344 } else if (name.equals("adjustment")) { 6345 this.adjustment = castToMoney(value); // Money 6346 } else if (name.equals("adjustmentReason")) { 6347 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 6348 } else if (name.equals("date")) { 6349 this.date = castToDate(value); // DateType 6350 } else if (name.equals("amount")) { 6351 this.amount = castToMoney(value); // Money 6352 } else if (name.equals("identifier")) { 6353 this.identifier = castToIdentifier(value); // Identifier 6354 } else 6355 return super.setProperty(name, value); 6356 return value; 6357 } 6358 6359 @Override 6360 public void removeChild(String name, Base value) throws FHIRException { 6361 if (name.equals("type")) { 6362 this.type = null; 6363 } else if (name.equals("adjustment")) { 6364 this.adjustment = null; 6365 } else if (name.equals("adjustmentReason")) { 6366 this.adjustmentReason = null; 6367 } else if (name.equals("date")) { 6368 this.date = null; 6369 } else if (name.equals("amount")) { 6370 this.amount = null; 6371 } else if (name.equals("identifier")) { 6372 this.identifier = null; 6373 } else 6374 super.removeChild(name, value); 6375 6376 } 6377 6378 @Override 6379 public Base makeProperty(int hash, String name) throws FHIRException { 6380 switch (hash) { 6381 case 3575610: 6382 return getType(); 6383 case 1977085293: 6384 return getAdjustment(); 6385 case -1255938543: 6386 return getAdjustmentReason(); 6387 case 3076014: 6388 return getDateElement(); 6389 case -1413853096: 6390 return getAmount(); 6391 case -1618432855: 6392 return getIdentifier(); 6393 default: 6394 return super.makeProperty(hash, name); 6395 } 6396 6397 } 6398 6399 @Override 6400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6401 switch (hash) { 6402 case 3575610: 6403 /* type */ return new String[] { "CodeableConcept" }; 6404 case 1977085293: 6405 /* adjustment */ return new String[] { "Money" }; 6406 case -1255938543: 6407 /* adjustmentReason */ return new String[] { "CodeableConcept" }; 6408 case 3076014: 6409 /* date */ return new String[] { "date" }; 6410 case -1413853096: 6411 /* amount */ return new String[] { "Money" }; 6412 case -1618432855: 6413 /* identifier */ return new String[] { "Identifier" }; 6414 default: 6415 return super.getTypesForProperty(hash, name); 6416 } 6417 6418 } 6419 6420 @Override 6421 public Base addChild(String name) throws FHIRException { 6422 if (name.equals("type")) { 6423 this.type = new CodeableConcept(); 6424 return this.type; 6425 } else if (name.equals("adjustment")) { 6426 this.adjustment = new Money(); 6427 return this.adjustment; 6428 } else if (name.equals("adjustmentReason")) { 6429 this.adjustmentReason = new CodeableConcept(); 6430 return this.adjustmentReason; 6431 } else if (name.equals("date")) { 6432 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.date"); 6433 } else if (name.equals("amount")) { 6434 this.amount = new Money(); 6435 return this.amount; 6436 } else if (name.equals("identifier")) { 6437 this.identifier = new Identifier(); 6438 return this.identifier; 6439 } else 6440 return super.addChild(name); 6441 } 6442 6443 public PaymentComponent copy() { 6444 PaymentComponent dst = new PaymentComponent(); 6445 copyValues(dst); 6446 return dst; 6447 } 6448 6449 public void copyValues(PaymentComponent dst) { 6450 super.copyValues(dst); 6451 dst.type = type == null ? null : type.copy(); 6452 dst.adjustment = adjustment == null ? null : adjustment.copy(); 6453 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 6454 dst.date = date == null ? null : date.copy(); 6455 dst.amount = amount == null ? null : amount.copy(); 6456 dst.identifier = identifier == null ? null : identifier.copy(); 6457 } 6458 6459 @Override 6460 public boolean equalsDeep(Base other_) { 6461 if (!super.equalsDeep(other_)) 6462 return false; 6463 if (!(other_ instanceof PaymentComponent)) 6464 return false; 6465 PaymentComponent o = (PaymentComponent) other_; 6466 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) 6467 && compareDeep(adjustmentReason, o.adjustmentReason, true) && compareDeep(date, o.date, true) 6468 && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true); 6469 } 6470 6471 @Override 6472 public boolean equalsShallow(Base other_) { 6473 if (!super.equalsShallow(other_)) 6474 return false; 6475 if (!(other_ instanceof PaymentComponent)) 6476 return false; 6477 PaymentComponent o = (PaymentComponent) other_; 6478 return compareValues(date, o.date, true); 6479 } 6480 6481 public boolean isEmpty() { 6482 return super.isEmpty() 6483 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason, date, amount, identifier); 6484 } 6485 6486 public String fhirType() { 6487 return "ClaimResponse.payment"; 6488 6489 } 6490 6491 } 6492 6493 @Block() 6494 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 6495 /** 6496 * A number to uniquely identify a note entry. 6497 */ 6498 @Child(name = "number", type = { 6499 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6500 @Description(shortDefinition = "Note instance identifier", formalDefinition = "A number to uniquely identify a note entry.") 6501 protected PositiveIntType number; 6502 6503 /** 6504 * The business purpose of the note text. 6505 */ 6506 @Child(name = "type", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6507 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The business purpose of the note text.") 6508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/note-type") 6509 protected Enumeration<NoteType> type; 6510 6511 /** 6512 * The explanation or description associated with the processing. 6513 */ 6514 @Child(name = "text", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 6515 @Description(shortDefinition = "Note explanatory text", formalDefinition = "The explanation or description associated with the processing.") 6516 protected StringType text; 6517 6518 /** 6519 * A code to define the language used in the text of the note. 6520 */ 6521 @Child(name = "language", type = { 6522 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6523 @Description(shortDefinition = "Language of the text", formalDefinition = "A code to define the language used in the text of the note.") 6524 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 6525 protected CodeableConcept language; 6526 6527 private static final long serialVersionUID = -385184277L; 6528 6529 /** 6530 * Constructor 6531 */ 6532 public NoteComponent() { 6533 super(); 6534 } 6535 6536 /** 6537 * Constructor 6538 */ 6539 public NoteComponent(StringType text) { 6540 super(); 6541 this.text = text; 6542 } 6543 6544 /** 6545 * @return {@link #number} (A number to uniquely identify a note entry.). This 6546 * is the underlying object with id, value and extensions. The accessor 6547 * "getNumber" gives direct access to the value 6548 */ 6549 public PositiveIntType getNumberElement() { 6550 if (this.number == null) 6551 if (Configuration.errorOnAutoCreate()) 6552 throw new Error("Attempt to auto-create NoteComponent.number"); 6553 else if (Configuration.doAutoCreate()) 6554 this.number = new PositiveIntType(); // bb 6555 return this.number; 6556 } 6557 6558 public boolean hasNumberElement() { 6559 return this.number != null && !this.number.isEmpty(); 6560 } 6561 6562 public boolean hasNumber() { 6563 return this.number != null && !this.number.isEmpty(); 6564 } 6565 6566 /** 6567 * @param value {@link #number} (A number to uniquely identify a note entry.). 6568 * This is the underlying object with id, value and extensions. The 6569 * accessor "getNumber" gives direct access to the value 6570 */ 6571 public NoteComponent setNumberElement(PositiveIntType value) { 6572 this.number = value; 6573 return this; 6574 } 6575 6576 /** 6577 * @return A number to uniquely identify a note entry. 6578 */ 6579 public int getNumber() { 6580 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 6581 } 6582 6583 /** 6584 * @param value A number to uniquely identify a note entry. 6585 */ 6586 public NoteComponent setNumber(int value) { 6587 if (this.number == null) 6588 this.number = new PositiveIntType(); 6589 this.number.setValue(value); 6590 return this; 6591 } 6592 6593 /** 6594 * @return {@link #type} (The business purpose of the note text.). This is the 6595 * underlying object with id, value and extensions. The accessor 6596 * "getType" gives direct access to the value 6597 */ 6598 public Enumeration<NoteType> getTypeElement() { 6599 if (this.type == null) 6600 if (Configuration.errorOnAutoCreate()) 6601 throw new Error("Attempt to auto-create NoteComponent.type"); 6602 else if (Configuration.doAutoCreate()) 6603 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); // bb 6604 return this.type; 6605 } 6606 6607 public boolean hasTypeElement() { 6608 return this.type != null && !this.type.isEmpty(); 6609 } 6610 6611 public boolean hasType() { 6612 return this.type != null && !this.type.isEmpty(); 6613 } 6614 6615 /** 6616 * @param value {@link #type} (The business purpose of the note text.). This is 6617 * the underlying object with id, value and extensions. The 6618 * accessor "getType" gives direct access to the value 6619 */ 6620 public NoteComponent setTypeElement(Enumeration<NoteType> value) { 6621 this.type = value; 6622 return this; 6623 } 6624 6625 /** 6626 * @return The business purpose of the note text. 6627 */ 6628 public NoteType getType() { 6629 return this.type == null ? null : this.type.getValue(); 6630 } 6631 6632 /** 6633 * @param value The business purpose of the note text. 6634 */ 6635 public NoteComponent setType(NoteType value) { 6636 if (value == null) 6637 this.type = null; 6638 else { 6639 if (this.type == null) 6640 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); 6641 this.type.setValue(value); 6642 } 6643 return this; 6644 } 6645 6646 /** 6647 * @return {@link #text} (The explanation or description associated with the 6648 * processing.). This is the underlying object with id, value and 6649 * extensions. The accessor "getText" gives direct access to the value 6650 */ 6651 public StringType getTextElement() { 6652 if (this.text == null) 6653 if (Configuration.errorOnAutoCreate()) 6654 throw new Error("Attempt to auto-create NoteComponent.text"); 6655 else if (Configuration.doAutoCreate()) 6656 this.text = new StringType(); // bb 6657 return this.text; 6658 } 6659 6660 public boolean hasTextElement() { 6661 return this.text != null && !this.text.isEmpty(); 6662 } 6663 6664 public boolean hasText() { 6665 return this.text != null && !this.text.isEmpty(); 6666 } 6667 6668 /** 6669 * @param value {@link #text} (The explanation or description associated with 6670 * the processing.). This is the underlying object with id, value 6671 * and extensions. The accessor "getText" gives direct access to 6672 * the value 6673 */ 6674 public NoteComponent setTextElement(StringType value) { 6675 this.text = value; 6676 return this; 6677 } 6678 6679 /** 6680 * @return The explanation or description associated with the processing. 6681 */ 6682 public String getText() { 6683 return this.text == null ? null : this.text.getValue(); 6684 } 6685 6686 /** 6687 * @param value The explanation or description associated with the processing. 6688 */ 6689 public NoteComponent setText(String value) { 6690 if (this.text == null) 6691 this.text = new StringType(); 6692 this.text.setValue(value); 6693 return this; 6694 } 6695 6696 /** 6697 * @return {@link #language} (A code to define the language used in the text of 6698 * the note.) 6699 */ 6700 public CodeableConcept getLanguage() { 6701 if (this.language == null) 6702 if (Configuration.errorOnAutoCreate()) 6703 throw new Error("Attempt to auto-create NoteComponent.language"); 6704 else if (Configuration.doAutoCreate()) 6705 this.language = new CodeableConcept(); // cc 6706 return this.language; 6707 } 6708 6709 public boolean hasLanguage() { 6710 return this.language != null && !this.language.isEmpty(); 6711 } 6712 6713 /** 6714 * @param value {@link #language} (A code to define the language used in the 6715 * text of the note.) 6716 */ 6717 public NoteComponent setLanguage(CodeableConcept value) { 6718 this.language = value; 6719 return this; 6720 } 6721 6722 protected void listChildren(List<Property> children) { 6723 super.listChildren(children); 6724 children.add(new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number)); 6725 children.add(new Property("type", "code", "The business purpose of the note text.", 0, 1, type)); 6726 children.add( 6727 new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 6728 children.add(new Property("language", "CodeableConcept", 6729 "A code to define the language used in the text of the note.", 0, 1, language)); 6730 } 6731 6732 @Override 6733 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6734 switch (_hash) { 6735 case -1034364087: 6736 /* number */ return new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, 6737 number); 6738 case 3575610: 6739 /* type */ return new Property("type", "code", "The business purpose of the note text.", 0, 1, type); 6740 case 3556653: 6741 /* text */ return new Property("text", "string", 6742 "The explanation or description associated with the processing.", 0, 1, text); 6743 case -1613589672: 6744 /* language */ return new Property("language", "CodeableConcept", 6745 "A code to define the language used in the text of the note.", 0, 1, language); 6746 default: 6747 return super.getNamedProperty(_hash, _name, _checkValid); 6748 } 6749 6750 } 6751 6752 @Override 6753 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6754 switch (hash) { 6755 case -1034364087: 6756 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // PositiveIntType 6757 case 3575610: 6758 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<NoteType> 6759 case 3556653: 6760 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 6761 case -1613589672: 6762 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 6763 default: 6764 return super.getProperty(hash, name, checkValid); 6765 } 6766 6767 } 6768 6769 @Override 6770 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6771 switch (hash) { 6772 case -1034364087: // number 6773 this.number = castToPositiveInt(value); // PositiveIntType 6774 return value; 6775 case 3575610: // type 6776 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 6777 this.type = (Enumeration) value; // Enumeration<NoteType> 6778 return value; 6779 case 3556653: // text 6780 this.text = castToString(value); // StringType 6781 return value; 6782 case -1613589672: // language 6783 this.language = castToCodeableConcept(value); // CodeableConcept 6784 return value; 6785 default: 6786 return super.setProperty(hash, name, value); 6787 } 6788 6789 } 6790 6791 @Override 6792 public Base setProperty(String name, Base value) throws FHIRException { 6793 if (name.equals("number")) { 6794 this.number = castToPositiveInt(value); // PositiveIntType 6795 } else if (name.equals("type")) { 6796 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 6797 this.type = (Enumeration) value; // Enumeration<NoteType> 6798 } else if (name.equals("text")) { 6799 this.text = castToString(value); // StringType 6800 } else if (name.equals("language")) { 6801 this.language = castToCodeableConcept(value); // CodeableConcept 6802 } else 6803 return super.setProperty(name, value); 6804 return value; 6805 } 6806 6807 @Override 6808 public void removeChild(String name, Base value) throws FHIRException { 6809 if (name.equals("number")) { 6810 this.number = null; 6811 } else if (name.equals("type")) { 6812 this.type = null; 6813 } else if (name.equals("text")) { 6814 this.text = null; 6815 } else if (name.equals("language")) { 6816 this.language = null; 6817 } else 6818 super.removeChild(name, value); 6819 6820 } 6821 6822 @Override 6823 public Base makeProperty(int hash, String name) throws FHIRException { 6824 switch (hash) { 6825 case -1034364087: 6826 return getNumberElement(); 6827 case 3575610: 6828 return getTypeElement(); 6829 case 3556653: 6830 return getTextElement(); 6831 case -1613589672: 6832 return getLanguage(); 6833 default: 6834 return super.makeProperty(hash, name); 6835 } 6836 6837 } 6838 6839 @Override 6840 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6841 switch (hash) { 6842 case -1034364087: 6843 /* number */ return new String[] { "positiveInt" }; 6844 case 3575610: 6845 /* type */ return new String[] { "code" }; 6846 case 3556653: 6847 /* text */ return new String[] { "string" }; 6848 case -1613589672: 6849 /* language */ return new String[] { "CodeableConcept" }; 6850 default: 6851 return super.getTypesForProperty(hash, name); 6852 } 6853 6854 } 6855 6856 @Override 6857 public Base addChild(String name) throws FHIRException { 6858 if (name.equals("number")) { 6859 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.number"); 6860 } else if (name.equals("type")) { 6861 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.type"); 6862 } else if (name.equals("text")) { 6863 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.text"); 6864 } else if (name.equals("language")) { 6865 this.language = new CodeableConcept(); 6866 return this.language; 6867 } else 6868 return super.addChild(name); 6869 } 6870 6871 public NoteComponent copy() { 6872 NoteComponent dst = new NoteComponent(); 6873 copyValues(dst); 6874 return dst; 6875 } 6876 6877 public void copyValues(NoteComponent dst) { 6878 super.copyValues(dst); 6879 dst.number = number == null ? null : number.copy(); 6880 dst.type = type == null ? null : type.copy(); 6881 dst.text = text == null ? null : text.copy(); 6882 dst.language = language == null ? null : language.copy(); 6883 } 6884 6885 @Override 6886 public boolean equalsDeep(Base other_) { 6887 if (!super.equalsDeep(other_)) 6888 return false; 6889 if (!(other_ instanceof NoteComponent)) 6890 return false; 6891 NoteComponent o = (NoteComponent) other_; 6892 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 6893 && compareDeep(language, o.language, true); 6894 } 6895 6896 @Override 6897 public boolean equalsShallow(Base other_) { 6898 if (!super.equalsShallow(other_)) 6899 return false; 6900 if (!(other_ instanceof NoteComponent)) 6901 return false; 6902 NoteComponent o = (NoteComponent) other_; 6903 return compareValues(number, o.number, true) && compareValues(type, o.type, true) 6904 && compareValues(text, o.text, true); 6905 } 6906 6907 public boolean isEmpty() { 6908 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language); 6909 } 6910 6911 public String fhirType() { 6912 return "ClaimResponse.processNote"; 6913 6914 } 6915 6916 } 6917 6918 @Block() 6919 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 6920 /** 6921 * A number to uniquely identify insurance entries and provide a sequence of 6922 * coverages to convey coordination of benefit order. 6923 */ 6924 @Child(name = "sequence", type = { 6925 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6926 @Description(shortDefinition = "Insurance instance identifier", formalDefinition = "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.") 6927 protected PositiveIntType sequence; 6928 6929 /** 6930 * A flag to indicate that this Coverage is to be used for adjudication of this 6931 * claim when set to true. 6932 */ 6933 @Child(name = "focal", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 6934 @Description(shortDefinition = "Coverage to be used for adjudication", formalDefinition = "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.") 6935 protected BooleanType focal; 6936 6937 /** 6938 * Reference to the insurance card level information contained in the Coverage 6939 * resource. The coverage issuing insurer will use these details to locate the 6940 * patient's actual coverage within the insurer's information system. 6941 */ 6942 @Child(name = "coverage", type = { Coverage.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 6943 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 6944 protected Reference coverage; 6945 6946 /** 6947 * The actual object that is the target of the reference (Reference to the 6948 * insurance card level information contained in the Coverage resource. The 6949 * coverage issuing insurer will use these details to locate the patient's 6950 * actual coverage within the insurer's information system.) 6951 */ 6952 protected Coverage coverageTarget; 6953 6954 /** 6955 * A business agreement number established between the provider and the insurer 6956 * for special business processing purposes. 6957 */ 6958 @Child(name = "businessArrangement", type = { 6959 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6960 @Description(shortDefinition = "Additional provider contract number", formalDefinition = "A business agreement number established between the provider and the insurer for special business processing purposes.") 6961 protected StringType businessArrangement; 6962 6963 /** 6964 * The result of the adjudication of the line items for the Coverage specified 6965 * in this insurance. 6966 */ 6967 @Child(name = "claimResponse", type = { 6968 ClaimResponse.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6969 @Description(shortDefinition = "Adjudication results", formalDefinition = "The result of the adjudication of the line items for the Coverage specified in this insurance.") 6970 protected Reference claimResponse; 6971 6972 /** 6973 * The actual object that is the target of the reference (The result of the 6974 * adjudication of the line items for the Coverage specified in this insurance.) 6975 */ 6976 protected ClaimResponse claimResponseTarget; 6977 6978 private static final long serialVersionUID = 282380584L; 6979 6980 /** 6981 * Constructor 6982 */ 6983 public InsuranceComponent() { 6984 super(); 6985 } 6986 6987 /** 6988 * Constructor 6989 */ 6990 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 6991 super(); 6992 this.sequence = sequence; 6993 this.focal = focal; 6994 this.coverage = coverage; 6995 } 6996 6997 /** 6998 * @return {@link #sequence} (A number to uniquely identify insurance entries 6999 * and provide a sequence of coverages to convey coordination of benefit 7000 * order.). This is the underlying object with id, value and extensions. 7001 * The accessor "getSequence" gives direct access to the value 7002 */ 7003 public PositiveIntType getSequenceElement() { 7004 if (this.sequence == null) 7005 if (Configuration.errorOnAutoCreate()) 7006 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 7007 else if (Configuration.doAutoCreate()) 7008 this.sequence = new PositiveIntType(); // bb 7009 return this.sequence; 7010 } 7011 7012 public boolean hasSequenceElement() { 7013 return this.sequence != null && !this.sequence.isEmpty(); 7014 } 7015 7016 public boolean hasSequence() { 7017 return this.sequence != null && !this.sequence.isEmpty(); 7018 } 7019 7020 /** 7021 * @param value {@link #sequence} (A number to uniquely identify insurance 7022 * entries and provide a sequence of coverages to convey 7023 * coordination of benefit order.). This is the underlying object 7024 * with id, value and extensions. The accessor "getSequence" gives 7025 * direct access to the value 7026 */ 7027 public InsuranceComponent setSequenceElement(PositiveIntType value) { 7028 this.sequence = value; 7029 return this; 7030 } 7031 7032 /** 7033 * @return A number to uniquely identify insurance entries and provide a 7034 * sequence of coverages to convey coordination of benefit order. 7035 */ 7036 public int getSequence() { 7037 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7038 } 7039 7040 /** 7041 * @param value A number to uniquely identify insurance entries and provide a 7042 * sequence of coverages to convey coordination of benefit order. 7043 */ 7044 public InsuranceComponent setSequence(int value) { 7045 if (this.sequence == null) 7046 this.sequence = new PositiveIntType(); 7047 this.sequence.setValue(value); 7048 return this; 7049 } 7050 7051 /** 7052 * @return {@link #focal} (A flag to indicate that this Coverage is to be used 7053 * for adjudication of this claim when set to true.). This is the 7054 * underlying object with id, value and extensions. The accessor 7055 * "getFocal" gives direct access to the value 7056 */ 7057 public BooleanType getFocalElement() { 7058 if (this.focal == null) 7059 if (Configuration.errorOnAutoCreate()) 7060 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 7061 else if (Configuration.doAutoCreate()) 7062 this.focal = new BooleanType(); // bb 7063 return this.focal; 7064 } 7065 7066 public boolean hasFocalElement() { 7067 return this.focal != null && !this.focal.isEmpty(); 7068 } 7069 7070 public boolean hasFocal() { 7071 return this.focal != null && !this.focal.isEmpty(); 7072 } 7073 7074 /** 7075 * @param value {@link #focal} (A flag to indicate that this Coverage is to be 7076 * used for adjudication of this claim when set to true.). This is 7077 * the underlying object with id, value and extensions. The 7078 * accessor "getFocal" gives direct access to the value 7079 */ 7080 public InsuranceComponent setFocalElement(BooleanType value) { 7081 this.focal = value; 7082 return this; 7083 } 7084 7085 /** 7086 * @return A flag to indicate that this Coverage is to be used for adjudication 7087 * of this claim when set to true. 7088 */ 7089 public boolean getFocal() { 7090 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 7091 } 7092 7093 /** 7094 * @param value A flag to indicate that this Coverage is to be used for 7095 * adjudication of this claim when set to true. 7096 */ 7097 public InsuranceComponent setFocal(boolean value) { 7098 if (this.focal == null) 7099 this.focal = new BooleanType(); 7100 this.focal.setValue(value); 7101 return this; 7102 } 7103 7104 /** 7105 * @return {@link #coverage} (Reference to the insurance card level information 7106 * contained in the Coverage resource. The coverage issuing insurer will 7107 * use these details to locate the patient's actual coverage within the 7108 * insurer's information system.) 7109 */ 7110 public Reference getCoverage() { 7111 if (this.coverage == null) 7112 if (Configuration.errorOnAutoCreate()) 7113 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 7114 else if (Configuration.doAutoCreate()) 7115 this.coverage = new Reference(); // cc 7116 return this.coverage; 7117 } 7118 7119 public boolean hasCoverage() { 7120 return this.coverage != null && !this.coverage.isEmpty(); 7121 } 7122 7123 /** 7124 * @param value {@link #coverage} (Reference to the insurance card level 7125 * information contained in the Coverage resource. The coverage 7126 * issuing insurer will use these details to locate the patient's 7127 * actual coverage within the insurer's information system.) 7128 */ 7129 public InsuranceComponent setCoverage(Reference value) { 7130 this.coverage = value; 7131 return this; 7132 } 7133 7134 /** 7135 * @return {@link #coverage} The actual object that is the target of the 7136 * reference. The reference library doesn't populate this, but you can 7137 * use it to hold the resource if you resolve it. (Reference to the 7138 * insurance card level information contained in the Coverage resource. 7139 * The coverage issuing insurer will use these details to locate the 7140 * patient's actual coverage within the insurer's information system.) 7141 */ 7142 public Coverage getCoverageTarget() { 7143 if (this.coverageTarget == null) 7144 if (Configuration.errorOnAutoCreate()) 7145 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 7146 else if (Configuration.doAutoCreate()) 7147 this.coverageTarget = new Coverage(); // aa 7148 return this.coverageTarget; 7149 } 7150 7151 /** 7152 * @param value {@link #coverage} The actual object that is the target of the 7153 * reference. The reference library doesn't use these, but you can 7154 * use it to hold the resource if you resolve it. (Reference to the 7155 * insurance card level information contained in the Coverage 7156 * resource. The coverage issuing insurer will use these details to 7157 * locate the patient's actual coverage within the insurer's 7158 * information system.) 7159 */ 7160 public InsuranceComponent setCoverageTarget(Coverage value) { 7161 this.coverageTarget = value; 7162 return this; 7163 } 7164 7165 /** 7166 * @return {@link #businessArrangement} (A business agreement number established 7167 * between the provider and the insurer for special business processing 7168 * purposes.). This is the underlying object with id, value and 7169 * extensions. The accessor "getBusinessArrangement" gives direct access 7170 * to the value 7171 */ 7172 public StringType getBusinessArrangementElement() { 7173 if (this.businessArrangement == null) 7174 if (Configuration.errorOnAutoCreate()) 7175 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 7176 else if (Configuration.doAutoCreate()) 7177 this.businessArrangement = new StringType(); // bb 7178 return this.businessArrangement; 7179 } 7180 7181 public boolean hasBusinessArrangementElement() { 7182 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 7183 } 7184 7185 public boolean hasBusinessArrangement() { 7186 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 7187 } 7188 7189 /** 7190 * @param value {@link #businessArrangement} (A business agreement number 7191 * established between the provider and the insurer for special 7192 * business processing purposes.). This is the underlying object 7193 * with id, value and extensions. The accessor 7194 * "getBusinessArrangement" gives direct access to the value 7195 */ 7196 public InsuranceComponent setBusinessArrangementElement(StringType value) { 7197 this.businessArrangement = value; 7198 return this; 7199 } 7200 7201 /** 7202 * @return A business agreement number established between the provider and the 7203 * insurer for special business processing purposes. 7204 */ 7205 public String getBusinessArrangement() { 7206 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 7207 } 7208 7209 /** 7210 * @param value A business agreement number established between the provider and 7211 * the insurer for special business processing purposes. 7212 */ 7213 public InsuranceComponent setBusinessArrangement(String value) { 7214 if (Utilities.noString(value)) 7215 this.businessArrangement = null; 7216 else { 7217 if (this.businessArrangement == null) 7218 this.businessArrangement = new StringType(); 7219 this.businessArrangement.setValue(value); 7220 } 7221 return this; 7222 } 7223 7224 /** 7225 * @return {@link #claimResponse} (The result of the adjudication of the line 7226 * items for the Coverage specified in this insurance.) 7227 */ 7228 public Reference getClaimResponse() { 7229 if (this.claimResponse == null) 7230 if (Configuration.errorOnAutoCreate()) 7231 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 7232 else if (Configuration.doAutoCreate()) 7233 this.claimResponse = new Reference(); // cc 7234 return this.claimResponse; 7235 } 7236 7237 public boolean hasClaimResponse() { 7238 return this.claimResponse != null && !this.claimResponse.isEmpty(); 7239 } 7240 7241 /** 7242 * @param value {@link #claimResponse} (The result of the adjudication of the 7243 * line items for the Coverage specified in this insurance.) 7244 */ 7245 public InsuranceComponent setClaimResponse(Reference value) { 7246 this.claimResponse = value; 7247 return this; 7248 } 7249 7250 /** 7251 * @return {@link #claimResponse} The actual object that is the target of the 7252 * reference. The reference library doesn't populate this, but you can 7253 * use it to hold the resource if you resolve it. (The result of the 7254 * adjudication of the line items for the Coverage specified in this 7255 * insurance.) 7256 */ 7257 public ClaimResponse getClaimResponseTarget() { 7258 if (this.claimResponseTarget == null) 7259 if (Configuration.errorOnAutoCreate()) 7260 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 7261 else if (Configuration.doAutoCreate()) 7262 this.claimResponseTarget = new ClaimResponse(); // aa 7263 return this.claimResponseTarget; 7264 } 7265 7266 /** 7267 * @param value {@link #claimResponse} The actual object that is the target of 7268 * the reference. The reference library doesn't use these, but you 7269 * can use it to hold the resource if you resolve it. (The result 7270 * of the adjudication of the line items for the Coverage specified 7271 * in this insurance.) 7272 */ 7273 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 7274 this.claimResponseTarget = value; 7275 return this; 7276 } 7277 7278 protected void listChildren(List<Property> children) { 7279 super.listChildren(children); 7280 children.add(new Property("sequence", "positiveInt", 7281 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 7282 0, 1, sequence)); 7283 children.add(new Property("focal", "boolean", 7284 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, 7285 focal)); 7286 children.add(new Property("coverage", "Reference(Coverage)", 7287 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 7288 0, 1, coverage)); 7289 children.add(new Property("businessArrangement", "string", 7290 "A business agreement number established between the provider and the insurer for special business processing purposes.", 7291 0, 1, businessArrangement)); 7292 children.add(new Property("claimResponse", "Reference(ClaimResponse)", 7293 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 7294 claimResponse)); 7295 } 7296 7297 @Override 7298 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7299 switch (_hash) { 7300 case 1349547969: 7301 /* sequence */ return new Property("sequence", "positiveInt", 7302 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 7303 0, 1, sequence); 7304 case 97604197: 7305 /* focal */ return new Property("focal", "boolean", 7306 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 7307 1, focal); 7308 case -351767064: 7309 /* coverage */ return new Property("coverage", "Reference(Coverage)", 7310 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 7311 0, 1, coverage); 7312 case 259920682: 7313 /* businessArrangement */ return new Property("businessArrangement", "string", 7314 "A business agreement number established between the provider and the insurer for special business processing purposes.", 7315 0, 1, businessArrangement); 7316 case 689513629: 7317 /* claimResponse */ return new Property("claimResponse", "Reference(ClaimResponse)", 7318 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 7319 claimResponse); 7320 default: 7321 return super.getNamedProperty(_hash, _name, _checkValid); 7322 } 7323 7324 } 7325 7326 @Override 7327 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7328 switch (hash) { 7329 case 1349547969: 7330 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 7331 case 97604197: 7332 /* focal */ return this.focal == null ? new Base[0] : new Base[] { this.focal }; // BooleanType 7333 case -351767064: 7334 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 7335 case 259920682: 7336 /* businessArrangement */ return this.businessArrangement == null ? new Base[0] 7337 : new Base[] { this.businessArrangement }; // StringType 7338 case 689513629: 7339 /* claimResponse */ return this.claimResponse == null ? new Base[0] : new Base[] { this.claimResponse }; // Reference 7340 default: 7341 return super.getProperty(hash, name, checkValid); 7342 } 7343 7344 } 7345 7346 @Override 7347 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7348 switch (hash) { 7349 case 1349547969: // sequence 7350 this.sequence = castToPositiveInt(value); // PositiveIntType 7351 return value; 7352 case 97604197: // focal 7353 this.focal = castToBoolean(value); // BooleanType 7354 return value; 7355 case -351767064: // coverage 7356 this.coverage = castToReference(value); // Reference 7357 return value; 7358 case 259920682: // businessArrangement 7359 this.businessArrangement = castToString(value); // StringType 7360 return value; 7361 case 689513629: // claimResponse 7362 this.claimResponse = castToReference(value); // Reference 7363 return value; 7364 default: 7365 return super.setProperty(hash, name, value); 7366 } 7367 7368 } 7369 7370 @Override 7371 public Base setProperty(String name, Base value) throws FHIRException { 7372 if (name.equals("sequence")) { 7373 this.sequence = castToPositiveInt(value); // PositiveIntType 7374 } else if (name.equals("focal")) { 7375 this.focal = castToBoolean(value); // BooleanType 7376 } else if (name.equals("coverage")) { 7377 this.coverage = castToReference(value); // Reference 7378 } else if (name.equals("businessArrangement")) { 7379 this.businessArrangement = castToString(value); // StringType 7380 } else if (name.equals("claimResponse")) { 7381 this.claimResponse = castToReference(value); // Reference 7382 } else 7383 return super.setProperty(name, value); 7384 return value; 7385 } 7386 7387 @Override 7388 public void removeChild(String name, Base value) throws FHIRException { 7389 if (name.equals("sequence")) { 7390 this.sequence = null; 7391 } else if (name.equals("focal")) { 7392 this.focal = null; 7393 } else if (name.equals("coverage")) { 7394 this.coverage = null; 7395 } else if (name.equals("businessArrangement")) { 7396 this.businessArrangement = null; 7397 } else if (name.equals("claimResponse")) { 7398 this.claimResponse = null; 7399 } else 7400 super.removeChild(name, value); 7401 7402 } 7403 7404 @Override 7405 public Base makeProperty(int hash, String name) throws FHIRException { 7406 switch (hash) { 7407 case 1349547969: 7408 return getSequenceElement(); 7409 case 97604197: 7410 return getFocalElement(); 7411 case -351767064: 7412 return getCoverage(); 7413 case 259920682: 7414 return getBusinessArrangementElement(); 7415 case 689513629: 7416 return getClaimResponse(); 7417 default: 7418 return super.makeProperty(hash, name); 7419 } 7420 7421 } 7422 7423 @Override 7424 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7425 switch (hash) { 7426 case 1349547969: 7427 /* sequence */ return new String[] { "positiveInt" }; 7428 case 97604197: 7429 /* focal */ return new String[] { "boolean" }; 7430 case -351767064: 7431 /* coverage */ return new String[] { "Reference" }; 7432 case 259920682: 7433 /* businessArrangement */ return new String[] { "string" }; 7434 case 689513629: 7435 /* claimResponse */ return new String[] { "Reference" }; 7436 default: 7437 return super.getTypesForProperty(hash, name); 7438 } 7439 7440 } 7441 7442 @Override 7443 public Base addChild(String name) throws FHIRException { 7444 if (name.equals("sequence")) { 7445 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequence"); 7446 } else if (name.equals("focal")) { 7447 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.focal"); 7448 } else if (name.equals("coverage")) { 7449 this.coverage = new Reference(); 7450 return this.coverage; 7451 } else if (name.equals("businessArrangement")) { 7452 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.businessArrangement"); 7453 } else if (name.equals("claimResponse")) { 7454 this.claimResponse = new Reference(); 7455 return this.claimResponse; 7456 } else 7457 return super.addChild(name); 7458 } 7459 7460 public InsuranceComponent copy() { 7461 InsuranceComponent dst = new InsuranceComponent(); 7462 copyValues(dst); 7463 return dst; 7464 } 7465 7466 public void copyValues(InsuranceComponent dst) { 7467 super.copyValues(dst); 7468 dst.sequence = sequence == null ? null : sequence.copy(); 7469 dst.focal = focal == null ? null : focal.copy(); 7470 dst.coverage = coverage == null ? null : coverage.copy(); 7471 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 7472 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 7473 } 7474 7475 @Override 7476 public boolean equalsDeep(Base other_) { 7477 if (!super.equalsDeep(other_)) 7478 return false; 7479 if (!(other_ instanceof InsuranceComponent)) 7480 return false; 7481 InsuranceComponent o = (InsuranceComponent) other_; 7482 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) 7483 && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 7484 && compareDeep(claimResponse, o.claimResponse, true); 7485 } 7486 7487 @Override 7488 public boolean equalsShallow(Base other_) { 7489 if (!super.equalsShallow(other_)) 7490 return false; 7491 if (!(other_ instanceof InsuranceComponent)) 7492 return false; 7493 InsuranceComponent o = (InsuranceComponent) other_; 7494 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) 7495 && compareValues(businessArrangement, o.businessArrangement, true); 7496 } 7497 7498 public boolean isEmpty() { 7499 return super.isEmpty() 7500 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, coverage, businessArrangement, claimResponse); 7501 } 7502 7503 public String fhirType() { 7504 return "ClaimResponse.insurance"; 7505 7506 } 7507 7508 } 7509 7510 @Block() 7511 public static class ErrorComponent extends BackboneElement implements IBaseBackboneElement { 7512 /** 7513 * The sequence number of the line item submitted which contains the error. This 7514 * value is omitted when the error occurs outside of the item structure. 7515 */ 7516 @Child(name = "itemSequence", type = { 7517 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 7518 @Description(shortDefinition = "Item sequence number", formalDefinition = "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.") 7519 protected PositiveIntType itemSequence; 7520 7521 /** 7522 * The sequence number of the detail within the line item submitted which 7523 * contains the error. This value is omitted when the error occurs outside of 7524 * the item structure. 7525 */ 7526 @Child(name = "detailSequence", type = { 7527 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7528 @Description(shortDefinition = "Detail sequence number", formalDefinition = "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.") 7529 protected PositiveIntType detailSequence; 7530 7531 /** 7532 * The sequence number of the sub-detail within the detail within the line item 7533 * submitted which contains the error. This value is omitted when the error 7534 * occurs outside of the item structure. 7535 */ 7536 @Child(name = "subDetailSequence", type = { 7537 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 7538 @Description(shortDefinition = "Subdetail sequence number", formalDefinition = "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.") 7539 protected PositiveIntType subDetailSequence; 7540 7541 /** 7542 * An error code, from a specified code system, which details why the claim 7543 * could not be adjudicated. 7544 */ 7545 @Child(name = "code", type = { 7546 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 7547 @Description(shortDefinition = "Error code detailing processing issues", formalDefinition = "An error code, from a specified code system, which details why the claim could not be adjudicated.") 7548 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication-error") 7549 protected CodeableConcept code; 7550 7551 private static final long serialVersionUID = 843818320L; 7552 7553 /** 7554 * Constructor 7555 */ 7556 public ErrorComponent() { 7557 super(); 7558 } 7559 7560 /** 7561 * Constructor 7562 */ 7563 public ErrorComponent(CodeableConcept code) { 7564 super(); 7565 this.code = code; 7566 } 7567 7568 /** 7569 * @return {@link #itemSequence} (The sequence number of the line item submitted 7570 * which contains the error. This value is omitted when the error occurs 7571 * outside of the item structure.). This is the underlying object with 7572 * id, value and extensions. The accessor "getItemSequence" gives direct 7573 * access to the value 7574 */ 7575 public PositiveIntType getItemSequenceElement() { 7576 if (this.itemSequence == null) 7577 if (Configuration.errorOnAutoCreate()) 7578 throw new Error("Attempt to auto-create ErrorComponent.itemSequence"); 7579 else if (Configuration.doAutoCreate()) 7580 this.itemSequence = new PositiveIntType(); // bb 7581 return this.itemSequence; 7582 } 7583 7584 public boolean hasItemSequenceElement() { 7585 return this.itemSequence != null && !this.itemSequence.isEmpty(); 7586 } 7587 7588 public boolean hasItemSequence() { 7589 return this.itemSequence != null && !this.itemSequence.isEmpty(); 7590 } 7591 7592 /** 7593 * @param value {@link #itemSequence} (The sequence number of the line item 7594 * submitted which contains the error. This value is omitted when 7595 * the error occurs outside of the item structure.). This is the 7596 * underlying object with id, value and extensions. The accessor 7597 * "getItemSequence" gives direct access to the value 7598 */ 7599 public ErrorComponent setItemSequenceElement(PositiveIntType value) { 7600 this.itemSequence = value; 7601 return this; 7602 } 7603 7604 /** 7605 * @return The sequence number of the line item submitted which contains the 7606 * error. This value is omitted when the error occurs outside of the 7607 * item structure. 7608 */ 7609 public int getItemSequence() { 7610 return this.itemSequence == null || this.itemSequence.isEmpty() ? 0 : this.itemSequence.getValue(); 7611 } 7612 7613 /** 7614 * @param value The sequence number of the line item submitted which contains 7615 * the error. This value is omitted when the error occurs outside 7616 * of the item structure. 7617 */ 7618 public ErrorComponent setItemSequence(int value) { 7619 if (this.itemSequence == null) 7620 this.itemSequence = new PositiveIntType(); 7621 this.itemSequence.setValue(value); 7622 return this; 7623 } 7624 7625 /** 7626 * @return {@link #detailSequence} (The sequence number of the detail within the 7627 * line item submitted which contains the error. This value is omitted 7628 * when the error occurs outside of the item structure.). This is the 7629 * underlying object with id, value and extensions. The accessor 7630 * "getDetailSequence" gives direct access to the value 7631 */ 7632 public PositiveIntType getDetailSequenceElement() { 7633 if (this.detailSequence == null) 7634 if (Configuration.errorOnAutoCreate()) 7635 throw new Error("Attempt to auto-create ErrorComponent.detailSequence"); 7636 else if (Configuration.doAutoCreate()) 7637 this.detailSequence = new PositiveIntType(); // bb 7638 return this.detailSequence; 7639 } 7640 7641 public boolean hasDetailSequenceElement() { 7642 return this.detailSequence != null && !this.detailSequence.isEmpty(); 7643 } 7644 7645 public boolean hasDetailSequence() { 7646 return this.detailSequence != null && !this.detailSequence.isEmpty(); 7647 } 7648 7649 /** 7650 * @param value {@link #detailSequence} (The sequence number of the detail 7651 * within the line item submitted which contains the error. This 7652 * value is omitted when the error occurs outside of the item 7653 * structure.). This is the underlying object with id, value and 7654 * extensions. The accessor "getDetailSequence" gives direct access 7655 * to the value 7656 */ 7657 public ErrorComponent setDetailSequenceElement(PositiveIntType value) { 7658 this.detailSequence = value; 7659 return this; 7660 } 7661 7662 /** 7663 * @return The sequence number of the detail within the line item submitted 7664 * which contains the error. This value is omitted when the error occurs 7665 * outside of the item structure. 7666 */ 7667 public int getDetailSequence() { 7668 return this.detailSequence == null || this.detailSequence.isEmpty() ? 0 : this.detailSequence.getValue(); 7669 } 7670 7671 /** 7672 * @param value The sequence number of the detail within the line item submitted 7673 * which contains the error. This value is omitted when the error 7674 * occurs outside of the item structure. 7675 */ 7676 public ErrorComponent setDetailSequence(int value) { 7677 if (this.detailSequence == null) 7678 this.detailSequence = new PositiveIntType(); 7679 this.detailSequence.setValue(value); 7680 return this; 7681 } 7682 7683 /** 7684 * @return {@link #subDetailSequence} (The sequence number of the sub-detail 7685 * within the detail within the line item submitted which contains the 7686 * error. This value is omitted when the error occurs outside of the 7687 * item structure.). This is the underlying object with id, value and 7688 * extensions. The accessor "getSubDetailSequence" gives direct access 7689 * to the value 7690 */ 7691 public PositiveIntType getSubDetailSequenceElement() { 7692 if (this.subDetailSequence == null) 7693 if (Configuration.errorOnAutoCreate()) 7694 throw new Error("Attempt to auto-create ErrorComponent.subDetailSequence"); 7695 else if (Configuration.doAutoCreate()) 7696 this.subDetailSequence = new PositiveIntType(); // bb 7697 return this.subDetailSequence; 7698 } 7699 7700 public boolean hasSubDetailSequenceElement() { 7701 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 7702 } 7703 7704 public boolean hasSubDetailSequence() { 7705 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 7706 } 7707 7708 /** 7709 * @param value {@link #subDetailSequence} (The sequence number of the 7710 * sub-detail within the detail within the line item submitted 7711 * which contains the error. This value is omitted when the error 7712 * occurs outside of the item structure.). This is the underlying 7713 * object with id, value and extensions. The accessor 7714 * "getSubDetailSequence" gives direct access to the value 7715 */ 7716 public ErrorComponent setSubDetailSequenceElement(PositiveIntType value) { 7717 this.subDetailSequence = value; 7718 return this; 7719 } 7720 7721 /** 7722 * @return The sequence number of the sub-detail within the detail within the 7723 * line item submitted which contains the error. This value is omitted 7724 * when the error occurs outside of the item structure. 7725 */ 7726 public int getSubDetailSequence() { 7727 return this.subDetailSequence == null || this.subDetailSequence.isEmpty() ? 0 : this.subDetailSequence.getValue(); 7728 } 7729 7730 /** 7731 * @param value The sequence number of the sub-detail within the detail within 7732 * the line item submitted which contains the error. This value is 7733 * omitted when the error occurs outside of the item structure. 7734 */ 7735 public ErrorComponent setSubDetailSequence(int value) { 7736 if (this.subDetailSequence == null) 7737 this.subDetailSequence = new PositiveIntType(); 7738 this.subDetailSequence.setValue(value); 7739 return this; 7740 } 7741 7742 /** 7743 * @return {@link #code} (An error code, from a specified code system, which 7744 * details why the claim could not be adjudicated.) 7745 */ 7746 public CodeableConcept getCode() { 7747 if (this.code == null) 7748 if (Configuration.errorOnAutoCreate()) 7749 throw new Error("Attempt to auto-create ErrorComponent.code"); 7750 else if (Configuration.doAutoCreate()) 7751 this.code = new CodeableConcept(); // cc 7752 return this.code; 7753 } 7754 7755 public boolean hasCode() { 7756 return this.code != null && !this.code.isEmpty(); 7757 } 7758 7759 /** 7760 * @param value {@link #code} (An error code, from a specified code system, 7761 * which details why the claim could not be adjudicated.) 7762 */ 7763 public ErrorComponent setCode(CodeableConcept value) { 7764 this.code = value; 7765 return this; 7766 } 7767 7768 protected void listChildren(List<Property> children) { 7769 super.listChildren(children); 7770 children.add(new Property("itemSequence", "positiveInt", 7771 "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7772 0, 1, itemSequence)); 7773 children.add(new Property("detailSequence", "positiveInt", 7774 "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7775 0, 1, detailSequence)); 7776 children.add(new Property("subDetailSequence", "positiveInt", 7777 "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7778 0, 1, subDetailSequence)); 7779 children.add(new Property("code", "CodeableConcept", 7780 "An error code, from a specified code system, which details why the claim could not be adjudicated.", 0, 1, 7781 code)); 7782 } 7783 7784 @Override 7785 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7786 switch (_hash) { 7787 case 1977979892: 7788 /* itemSequence */ return new Property("itemSequence", "positiveInt", 7789 "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7790 0, 1, itemSequence); 7791 case 1321472818: 7792 /* detailSequence */ return new Property("detailSequence", "positiveInt", 7793 "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7794 0, 1, detailSequence); 7795 case -855462510: 7796 /* subDetailSequence */ return new Property("subDetailSequence", "positiveInt", 7797 "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7798 0, 1, subDetailSequence); 7799 case 3059181: 7800 /* code */ return new Property("code", "CodeableConcept", 7801 "An error code, from a specified code system, which details why the claim could not be adjudicated.", 0, 1, 7802 code); 7803 default: 7804 return super.getNamedProperty(_hash, _name, _checkValid); 7805 } 7806 7807 } 7808 7809 @Override 7810 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7811 switch (hash) { 7812 case 1977979892: 7813 /* itemSequence */ return this.itemSequence == null ? new Base[0] : new Base[] { this.itemSequence }; // PositiveIntType 7814 case 1321472818: 7815 /* detailSequence */ return this.detailSequence == null ? new Base[0] : new Base[] { this.detailSequence }; // PositiveIntType 7816 case -855462510: 7817 /* subDetailSequence */ return this.subDetailSequence == null ? new Base[0] 7818 : new Base[] { this.subDetailSequence }; // PositiveIntType 7819 case 3059181: 7820 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 7821 default: 7822 return super.getProperty(hash, name, checkValid); 7823 } 7824 7825 } 7826 7827 @Override 7828 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7829 switch (hash) { 7830 case 1977979892: // itemSequence 7831 this.itemSequence = castToPositiveInt(value); // PositiveIntType 7832 return value; 7833 case 1321472818: // detailSequence 7834 this.detailSequence = castToPositiveInt(value); // PositiveIntType 7835 return value; 7836 case -855462510: // subDetailSequence 7837 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 7838 return value; 7839 case 3059181: // code 7840 this.code = castToCodeableConcept(value); // CodeableConcept 7841 return value; 7842 default: 7843 return super.setProperty(hash, name, value); 7844 } 7845 7846 } 7847 7848 @Override 7849 public Base setProperty(String name, Base value) throws FHIRException { 7850 if (name.equals("itemSequence")) { 7851 this.itemSequence = castToPositiveInt(value); // PositiveIntType 7852 } else if (name.equals("detailSequence")) { 7853 this.detailSequence = castToPositiveInt(value); // PositiveIntType 7854 } else if (name.equals("subDetailSequence")) { 7855 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 7856 } else if (name.equals("code")) { 7857 this.code = castToCodeableConcept(value); // CodeableConcept 7858 } else 7859 return super.setProperty(name, value); 7860 return value; 7861 } 7862 7863 @Override 7864 public void removeChild(String name, Base value) throws FHIRException { 7865 if (name.equals("itemSequence")) { 7866 this.itemSequence = null; 7867 } else if (name.equals("detailSequence")) { 7868 this.detailSequence = null; 7869 } else if (name.equals("subDetailSequence")) { 7870 this.subDetailSequence = null; 7871 } else if (name.equals("code")) { 7872 this.code = null; 7873 } else 7874 super.removeChild(name, value); 7875 7876 } 7877 7878 @Override 7879 public Base makeProperty(int hash, String name) throws FHIRException { 7880 switch (hash) { 7881 case 1977979892: 7882 return getItemSequenceElement(); 7883 case 1321472818: 7884 return getDetailSequenceElement(); 7885 case -855462510: 7886 return getSubDetailSequenceElement(); 7887 case 3059181: 7888 return getCode(); 7889 default: 7890 return super.makeProperty(hash, name); 7891 } 7892 7893 } 7894 7895 @Override 7896 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7897 switch (hash) { 7898 case 1977979892: 7899 /* itemSequence */ return new String[] { "positiveInt" }; 7900 case 1321472818: 7901 /* detailSequence */ return new String[] { "positiveInt" }; 7902 case -855462510: 7903 /* subDetailSequence */ return new String[] { "positiveInt" }; 7904 case 3059181: 7905 /* code */ return new String[] { "CodeableConcept" }; 7906 default: 7907 return super.getTypesForProperty(hash, name); 7908 } 7909 7910 } 7911 7912 @Override 7913 public Base addChild(String name) throws FHIRException { 7914 if (name.equals("itemSequence")) { 7915 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.itemSequence"); 7916 } else if (name.equals("detailSequence")) { 7917 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequence"); 7918 } else if (name.equals("subDetailSequence")) { 7919 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subDetailSequence"); 7920 } else if (name.equals("code")) { 7921 this.code = new CodeableConcept(); 7922 return this.code; 7923 } else 7924 return super.addChild(name); 7925 } 7926 7927 public ErrorComponent copy() { 7928 ErrorComponent dst = new ErrorComponent(); 7929 copyValues(dst); 7930 return dst; 7931 } 7932 7933 public void copyValues(ErrorComponent dst) { 7934 super.copyValues(dst); 7935 dst.itemSequence = itemSequence == null ? null : itemSequence.copy(); 7936 dst.detailSequence = detailSequence == null ? null : detailSequence.copy(); 7937 dst.subDetailSequence = subDetailSequence == null ? null : subDetailSequence.copy(); 7938 dst.code = code == null ? null : code.copy(); 7939 } 7940 7941 @Override 7942 public boolean equalsDeep(Base other_) { 7943 if (!super.equalsDeep(other_)) 7944 return false; 7945 if (!(other_ instanceof ErrorComponent)) 7946 return false; 7947 ErrorComponent o = (ErrorComponent) other_; 7948 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 7949 && compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(code, o.code, true); 7950 } 7951 7952 @Override 7953 public boolean equalsShallow(Base other_) { 7954 if (!super.equalsShallow(other_)) 7955 return false; 7956 if (!(other_ instanceof ErrorComponent)) 7957 return false; 7958 ErrorComponent o = (ErrorComponent) other_; 7959 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 7960 && compareValues(subDetailSequence, o.subDetailSequence, true); 7961 } 7962 7963 public boolean isEmpty() { 7964 return super.isEmpty() 7965 && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence, subDetailSequence, code); 7966 } 7967 7968 public String fhirType() { 7969 return "ClaimResponse.error"; 7970 7971 } 7972 7973 } 7974 7975 /** 7976 * A unique identifier assigned to this claim response. 7977 */ 7978 @Child(name = "identifier", type = { 7979 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7980 @Description(shortDefinition = "Business Identifier for a claim response", formalDefinition = "A unique identifier assigned to this claim response.") 7981 protected List<Identifier> identifier; 7982 7983 /** 7984 * The status of the resource instance. 7985 */ 7986 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 7987 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 7988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 7989 protected Enumeration<ClaimResponseStatus> status; 7990 7991 /** 7992 * A finer grained suite of claim type codes which may convey additional 7993 * information such as Inpatient vs Outpatient and/or a specialty service. 7994 */ 7995 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 7996 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 7997 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-type") 7998 protected CodeableConcept type; 7999 8000 /** 8001 * A finer grained suite of claim type codes which may convey additional 8002 * information such as Inpatient vs Outpatient and/or a specialty service. 8003 */ 8004 @Child(name = "subType", type = { 8005 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 8006 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 8007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-subtype") 8008 protected CodeableConcept subType; 8009 8010 /** 8011 * A code to indicate whether the nature of the request is: to request 8012 * adjudication of products and services previously rendered; or requesting 8013 * authorization and adjudication for provision in the future; or requesting the 8014 * non-binding adjudication of the listed products and services which could be 8015 * provided in the future. 8016 */ 8017 @Child(name = "use", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 8018 @Description(shortDefinition = "claim | preauthorization | predetermination", formalDefinition = "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.") 8019 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-use") 8020 protected Enumeration<Use> use; 8021 8022 /** 8023 * The party to whom the professional services and/or products have been 8024 * supplied or are being considered and for whom actual for facast reimbursement 8025 * is sought. 8026 */ 8027 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 8028 @Description(shortDefinition = "The recipient of the products and services", formalDefinition = "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.") 8029 protected Reference patient; 8030 8031 /** 8032 * The actual object that is the target of the reference (The party to whom the 8033 * professional services and/or products have been supplied or are being 8034 * considered and for whom actual for facast reimbursement is sought.) 8035 */ 8036 protected Patient patientTarget; 8037 8038 /** 8039 * The date this resource was created. 8040 */ 8041 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 8042 @Description(shortDefinition = "Response creation date", formalDefinition = "The date this resource was created.") 8043 protected DateTimeType created; 8044 8045 /** 8046 * The party responsible for authorization, adjudication and reimbursement. 8047 */ 8048 @Child(name = "insurer", type = { Organization.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 8049 @Description(shortDefinition = "Party responsible for reimbursement", formalDefinition = "The party responsible for authorization, adjudication and reimbursement.") 8050 protected Reference insurer; 8051 8052 /** 8053 * The actual object that is the target of the reference (The party responsible 8054 * for authorization, adjudication and reimbursement.) 8055 */ 8056 protected Organization insurerTarget; 8057 8058 /** 8059 * The provider which is responsible for the claim, predetermination or 8060 * preauthorization. 8061 */ 8062 @Child(name = "requestor", type = { Practitioner.class, PractitionerRole.class, 8063 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 8064 @Description(shortDefinition = "Party responsible for the claim", formalDefinition = "The provider which is responsible for the claim, predetermination or preauthorization.") 8065 protected Reference requestor; 8066 8067 /** 8068 * The actual object that is the target of the reference (The provider which is 8069 * responsible for the claim, predetermination or preauthorization.) 8070 */ 8071 protected Resource requestorTarget; 8072 8073 /** 8074 * Original request resource reference. 8075 */ 8076 @Child(name = "request", type = { Claim.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 8077 @Description(shortDefinition = "Id of resource triggering adjudication", formalDefinition = "Original request resource reference.") 8078 protected Reference request; 8079 8080 /** 8081 * The actual object that is the target of the reference (Original request 8082 * resource reference.) 8083 */ 8084 protected Claim requestTarget; 8085 8086 /** 8087 * The outcome of the claim, predetermination, or preauthorization processing. 8088 */ 8089 @Child(name = "outcome", type = { CodeType.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 8090 @Description(shortDefinition = "queued | complete | error | partial", formalDefinition = "The outcome of the claim, predetermination, or preauthorization processing.") 8091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/remittance-outcome") 8092 protected Enumeration<RemittanceOutcome> outcome; 8093 8094 /** 8095 * A human readable description of the status of the adjudication. 8096 */ 8097 @Child(name = "disposition", type = { 8098 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 8099 @Description(shortDefinition = "Disposition Message", formalDefinition = "A human readable description of the status of the adjudication.") 8100 protected StringType disposition; 8101 8102 /** 8103 * Reference from the Insurer which is used in later communications which refers 8104 * to this adjudication. 8105 */ 8106 @Child(name = "preAuthRef", type = { 8107 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 8108 @Description(shortDefinition = "Preauthorization reference", formalDefinition = "Reference from the Insurer which is used in later communications which refers to this adjudication.") 8109 protected StringType preAuthRef; 8110 8111 /** 8112 * The time frame during which this authorization is effective. 8113 */ 8114 @Child(name = "preAuthPeriod", type = { 8115 Period.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 8116 @Description(shortDefinition = "Preauthorization reference effective period", formalDefinition = "The time frame during which this authorization is effective.") 8117 protected Period preAuthPeriod; 8118 8119 /** 8120 * Type of Party to be reimbursed: subscriber, provider, other. 8121 */ 8122 @Child(name = "payeeType", type = { 8123 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 8124 @Description(shortDefinition = "Party to be paid any benefits payable", formalDefinition = "Type of Party to be reimbursed: subscriber, provider, other.") 8125 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payeetype") 8126 protected CodeableConcept payeeType; 8127 8128 /** 8129 * A claim line. Either a simple (a product or service) or a 'group' of details 8130 * which can also be a simple items or groups of sub-details. 8131 */ 8132 @Child(name = "item", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8133 @Description(shortDefinition = "Adjudication for claim line items", formalDefinition = "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.") 8134 protected List<ItemComponent> item; 8135 8136 /** 8137 * The first-tier service adjudications for payor added product or service 8138 * lines. 8139 */ 8140 @Child(name = "addItem", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8141 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The first-tier service adjudications for payor added product or service lines.") 8142 protected List<AddedItemComponent> addItem; 8143 8144 /** 8145 * The adjudication results which are presented at the header level rather than 8146 * at the line-item or add-item levels. 8147 */ 8148 @Child(name = "adjudication", type = { 8149 AdjudicationComponent.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8150 @Description(shortDefinition = "Header-level adjudication", formalDefinition = "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.") 8151 protected List<AdjudicationComponent> adjudication; 8152 8153 /** 8154 * Categorized monetary totals for the adjudication. 8155 */ 8156 @Child(name = "total", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8157 @Description(shortDefinition = "Adjudication totals", formalDefinition = "Categorized monetary totals for the adjudication.") 8158 protected List<TotalComponent> total; 8159 8160 /** 8161 * Payment details for the adjudication of the claim. 8162 */ 8163 @Child(name = "payment", type = {}, order = 19, min = 0, max = 1, modifier = false, summary = false) 8164 @Description(shortDefinition = "Payment Details", formalDefinition = "Payment details for the adjudication of the claim.") 8165 protected PaymentComponent payment; 8166 8167 /** 8168 * A code, used only on a response to a preauthorization, to indicate whether 8169 * the benefits payable have been reserved and for whom. 8170 */ 8171 @Child(name = "fundsReserve", type = { 8172 CodeableConcept.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 8173 @Description(shortDefinition = "Funds reserved status", formalDefinition = "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.") 8174 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fundsreserve") 8175 protected CodeableConcept fundsReserve; 8176 8177 /** 8178 * A code for the form to be used for printing the content. 8179 */ 8180 @Child(name = "formCode", type = { 8181 CodeableConcept.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 8182 @Description(shortDefinition = "Printed form identifier", formalDefinition = "A code for the form to be used for printing the content.") 8183 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/forms") 8184 protected CodeableConcept formCode; 8185 8186 /** 8187 * The actual form, by reference or inclusion, for printing the content or an 8188 * EOB. 8189 */ 8190 @Child(name = "form", type = { Attachment.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 8191 @Description(shortDefinition = "Printed reference or actual form", formalDefinition = "The actual form, by reference or inclusion, for printing the content or an EOB.") 8192 protected Attachment form; 8193 8194 /** 8195 * A note that describes or explains adjudication results in a human readable 8196 * form. 8197 */ 8198 @Child(name = "processNote", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8199 @Description(shortDefinition = "Note concerning adjudication", formalDefinition = "A note that describes or explains adjudication results in a human readable form.") 8200 protected List<NoteComponent> processNote; 8201 8202 /** 8203 * Request for additional supporting or authorizing information. 8204 */ 8205 @Child(name = "communicationRequest", type = { 8206 CommunicationRequest.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8207 @Description(shortDefinition = "Request for additional information", formalDefinition = "Request for additional supporting or authorizing information.") 8208 protected List<Reference> communicationRequest; 8209 /** 8210 * The actual objects that are the target of the reference (Request for 8211 * additional supporting or authorizing information.) 8212 */ 8213 protected List<CommunicationRequest> communicationRequestTarget; 8214 8215 /** 8216 * Financial instruments for reimbursement for the health care products and 8217 * services specified on the claim. 8218 */ 8219 @Child(name = "insurance", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8220 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services specified on the claim.") 8221 protected List<InsuranceComponent> insurance; 8222 8223 /** 8224 * Errors encountered during the processing of the adjudication. 8225 */ 8226 @Child(name = "error", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8227 @Description(shortDefinition = "Processing errors", formalDefinition = "Errors encountered during the processing of the adjudication.") 8228 protected List<ErrorComponent> error; 8229 8230 private static final long serialVersionUID = 731586651L; 8231 8232 /** 8233 * Constructor 8234 */ 8235 public ClaimResponse() { 8236 super(); 8237 } 8238 8239 /** 8240 * Constructor 8241 */ 8242 public ClaimResponse(Enumeration<ClaimResponseStatus> status, CodeableConcept type, Enumeration<Use> use, 8243 Reference patient, DateTimeType created, Reference insurer, Enumeration<RemittanceOutcome> outcome) { 8244 super(); 8245 this.status = status; 8246 this.type = type; 8247 this.use = use; 8248 this.patient = patient; 8249 this.created = created; 8250 this.insurer = insurer; 8251 this.outcome = outcome; 8252 } 8253 8254 /** 8255 * @return {@link #identifier} (A unique identifier assigned to this claim 8256 * response.) 8257 */ 8258 public List<Identifier> getIdentifier() { 8259 if (this.identifier == null) 8260 this.identifier = new ArrayList<Identifier>(); 8261 return this.identifier; 8262 } 8263 8264 /** 8265 * @return Returns a reference to <code>this</code> for easy method chaining 8266 */ 8267 public ClaimResponse setIdentifier(List<Identifier> theIdentifier) { 8268 this.identifier = theIdentifier; 8269 return this; 8270 } 8271 8272 public boolean hasIdentifier() { 8273 if (this.identifier == null) 8274 return false; 8275 for (Identifier item : this.identifier) 8276 if (!item.isEmpty()) 8277 return true; 8278 return false; 8279 } 8280 8281 public Identifier addIdentifier() { // 3 8282 Identifier t = new Identifier(); 8283 if (this.identifier == null) 8284 this.identifier = new ArrayList<Identifier>(); 8285 this.identifier.add(t); 8286 return t; 8287 } 8288 8289 public ClaimResponse addIdentifier(Identifier t) { // 3 8290 if (t == null) 8291 return this; 8292 if (this.identifier == null) 8293 this.identifier = new ArrayList<Identifier>(); 8294 this.identifier.add(t); 8295 return this; 8296 } 8297 8298 /** 8299 * @return The first repetition of repeating field {@link #identifier}, creating 8300 * it if it does not already exist 8301 */ 8302 public Identifier getIdentifierFirstRep() { 8303 if (getIdentifier().isEmpty()) { 8304 addIdentifier(); 8305 } 8306 return getIdentifier().get(0); 8307 } 8308 8309 /** 8310 * @return {@link #status} (The status of the resource instance.). This is the 8311 * underlying object with id, value and extensions. The accessor 8312 * "getStatus" gives direct access to the value 8313 */ 8314 public Enumeration<ClaimResponseStatus> getStatusElement() { 8315 if (this.status == null) 8316 if (Configuration.errorOnAutoCreate()) 8317 throw new Error("Attempt to auto-create ClaimResponse.status"); 8318 else if (Configuration.doAutoCreate()) 8319 this.status = new Enumeration<ClaimResponseStatus>(new ClaimResponseStatusEnumFactory()); // bb 8320 return this.status; 8321 } 8322 8323 public boolean hasStatusElement() { 8324 return this.status != null && !this.status.isEmpty(); 8325 } 8326 8327 public boolean hasStatus() { 8328 return this.status != null && !this.status.isEmpty(); 8329 } 8330 8331 /** 8332 * @param value {@link #status} (The status of the resource instance.). This is 8333 * the underlying object with id, value and extensions. The 8334 * accessor "getStatus" gives direct access to the value 8335 */ 8336 public ClaimResponse setStatusElement(Enumeration<ClaimResponseStatus> value) { 8337 this.status = value; 8338 return this; 8339 } 8340 8341 /** 8342 * @return The status of the resource instance. 8343 */ 8344 public ClaimResponseStatus getStatus() { 8345 return this.status == null ? null : this.status.getValue(); 8346 } 8347 8348 /** 8349 * @param value The status of the resource instance. 8350 */ 8351 public ClaimResponse setStatus(ClaimResponseStatus value) { 8352 if (this.status == null) 8353 this.status = new Enumeration<ClaimResponseStatus>(new ClaimResponseStatusEnumFactory()); 8354 this.status.setValue(value); 8355 return this; 8356 } 8357 8358 /** 8359 * @return {@link #type} (A finer grained suite of claim type codes which may 8360 * convey additional information such as Inpatient vs Outpatient and/or 8361 * a specialty service.) 8362 */ 8363 public CodeableConcept getType() { 8364 if (this.type == null) 8365 if (Configuration.errorOnAutoCreate()) 8366 throw new Error("Attempt to auto-create ClaimResponse.type"); 8367 else if (Configuration.doAutoCreate()) 8368 this.type = new CodeableConcept(); // cc 8369 return this.type; 8370 } 8371 8372 public boolean hasType() { 8373 return this.type != null && !this.type.isEmpty(); 8374 } 8375 8376 /** 8377 * @param value {@link #type} (A finer grained suite of claim type codes which 8378 * may convey additional information such as Inpatient vs 8379 * Outpatient and/or a specialty service.) 8380 */ 8381 public ClaimResponse setType(CodeableConcept value) { 8382 this.type = value; 8383 return this; 8384 } 8385 8386 /** 8387 * @return {@link #subType} (A finer grained suite of claim type codes which may 8388 * convey additional information such as Inpatient vs Outpatient and/or 8389 * a specialty service.) 8390 */ 8391 public CodeableConcept getSubType() { 8392 if (this.subType == null) 8393 if (Configuration.errorOnAutoCreate()) 8394 throw new Error("Attempt to auto-create ClaimResponse.subType"); 8395 else if (Configuration.doAutoCreate()) 8396 this.subType = new CodeableConcept(); // cc 8397 return this.subType; 8398 } 8399 8400 public boolean hasSubType() { 8401 return this.subType != null && !this.subType.isEmpty(); 8402 } 8403 8404 /** 8405 * @param value {@link #subType} (A finer grained suite of claim type codes 8406 * which may convey additional information such as Inpatient vs 8407 * Outpatient and/or a specialty service.) 8408 */ 8409 public ClaimResponse setSubType(CodeableConcept value) { 8410 this.subType = value; 8411 return this; 8412 } 8413 8414 /** 8415 * @return {@link #use} (A code to indicate whether the nature of the request 8416 * is: to request adjudication of products and services previously 8417 * rendered; or requesting authorization and adjudication for provision 8418 * in the future; or requesting the non-binding adjudication of the 8419 * listed products and services which could be provided in the future.). 8420 * This is the underlying object with id, value and extensions. The 8421 * accessor "getUse" gives direct access to the value 8422 */ 8423 public Enumeration<Use> getUseElement() { 8424 if (this.use == null) 8425 if (Configuration.errorOnAutoCreate()) 8426 throw new Error("Attempt to auto-create ClaimResponse.use"); 8427 else if (Configuration.doAutoCreate()) 8428 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 8429 return this.use; 8430 } 8431 8432 public boolean hasUseElement() { 8433 return this.use != null && !this.use.isEmpty(); 8434 } 8435 8436 public boolean hasUse() { 8437 return this.use != null && !this.use.isEmpty(); 8438 } 8439 8440 /** 8441 * @param value {@link #use} (A code to indicate whether the nature of the 8442 * request is: to request adjudication of products and services 8443 * previously rendered; or requesting authorization and 8444 * adjudication for provision in the future; or requesting the 8445 * non-binding adjudication of the listed products and services 8446 * which could be provided in the future.). This is the underlying 8447 * object with id, value and extensions. The accessor "getUse" 8448 * gives direct access to the value 8449 */ 8450 public ClaimResponse setUseElement(Enumeration<Use> value) { 8451 this.use = value; 8452 return this; 8453 } 8454 8455 /** 8456 * @return A code to indicate whether the nature of the request is: to request 8457 * adjudication of products and services previously rendered; or 8458 * requesting authorization and adjudication for provision in the 8459 * future; or requesting the non-binding adjudication of the listed 8460 * products and services which could be provided in the future. 8461 */ 8462 public Use getUse() { 8463 return this.use == null ? null : this.use.getValue(); 8464 } 8465 8466 /** 8467 * @param value A code to indicate whether the nature of the request is: to 8468 * request adjudication of products and services previously 8469 * rendered; or requesting authorization and adjudication for 8470 * provision in the future; or requesting the non-binding 8471 * adjudication of the listed products and services which could be 8472 * provided in the future. 8473 */ 8474 public ClaimResponse setUse(Use value) { 8475 if (this.use == null) 8476 this.use = new Enumeration<Use>(new UseEnumFactory()); 8477 this.use.setValue(value); 8478 return this; 8479 } 8480 8481 /** 8482 * @return {@link #patient} (The party to whom the professional services and/or 8483 * products have been supplied or are being considered and for whom 8484 * actual for facast reimbursement is sought.) 8485 */ 8486 public Reference getPatient() { 8487 if (this.patient == null) 8488 if (Configuration.errorOnAutoCreate()) 8489 throw new Error("Attempt to auto-create ClaimResponse.patient"); 8490 else if (Configuration.doAutoCreate()) 8491 this.patient = new Reference(); // cc 8492 return this.patient; 8493 } 8494 8495 public boolean hasPatient() { 8496 return this.patient != null && !this.patient.isEmpty(); 8497 } 8498 8499 /** 8500 * @param value {@link #patient} (The party to whom the professional services 8501 * and/or products have been supplied or are being considered and 8502 * for whom actual for facast reimbursement is sought.) 8503 */ 8504 public ClaimResponse setPatient(Reference value) { 8505 this.patient = value; 8506 return this; 8507 } 8508 8509 /** 8510 * @return {@link #patient} The actual object that is the target of the 8511 * reference. The reference library doesn't populate this, but you can 8512 * use it to hold the resource if you resolve it. (The party to whom the 8513 * professional services and/or products have been supplied or are being 8514 * considered and for whom actual for facast reimbursement is sought.) 8515 */ 8516 public Patient getPatientTarget() { 8517 if (this.patientTarget == null) 8518 if (Configuration.errorOnAutoCreate()) 8519 throw new Error("Attempt to auto-create ClaimResponse.patient"); 8520 else if (Configuration.doAutoCreate()) 8521 this.patientTarget = new Patient(); // aa 8522 return this.patientTarget; 8523 } 8524 8525 /** 8526 * @param value {@link #patient} The actual object that is the target of the 8527 * reference. The reference library doesn't use these, but you can 8528 * use it to hold the resource if you resolve it. (The party to 8529 * whom the professional services and/or products have been 8530 * supplied or are being considered and for whom actual for facast 8531 * reimbursement is sought.) 8532 */ 8533 public ClaimResponse setPatientTarget(Patient value) { 8534 this.patientTarget = value; 8535 return this; 8536 } 8537 8538 /** 8539 * @return {@link #created} (The date this resource was created.). This is the 8540 * underlying object with id, value and extensions. The accessor 8541 * "getCreated" gives direct access to the value 8542 */ 8543 public DateTimeType getCreatedElement() { 8544 if (this.created == null) 8545 if (Configuration.errorOnAutoCreate()) 8546 throw new Error("Attempt to auto-create ClaimResponse.created"); 8547 else if (Configuration.doAutoCreate()) 8548 this.created = new DateTimeType(); // bb 8549 return this.created; 8550 } 8551 8552 public boolean hasCreatedElement() { 8553 return this.created != null && !this.created.isEmpty(); 8554 } 8555 8556 public boolean hasCreated() { 8557 return this.created != null && !this.created.isEmpty(); 8558 } 8559 8560 /** 8561 * @param value {@link #created} (The date this resource was created.). This is 8562 * the underlying object with id, value and extensions. The 8563 * accessor "getCreated" gives direct access to the value 8564 */ 8565 public ClaimResponse setCreatedElement(DateTimeType value) { 8566 this.created = value; 8567 return this; 8568 } 8569 8570 /** 8571 * @return The date this resource was created. 8572 */ 8573 public Date getCreated() { 8574 return this.created == null ? null : this.created.getValue(); 8575 } 8576 8577 /** 8578 * @param value The date this resource was created. 8579 */ 8580 public ClaimResponse setCreated(Date value) { 8581 if (this.created == null) 8582 this.created = new DateTimeType(); 8583 this.created.setValue(value); 8584 return this; 8585 } 8586 8587 /** 8588 * @return {@link #insurer} (The party responsible for authorization, 8589 * adjudication and reimbursement.) 8590 */ 8591 public Reference getInsurer() { 8592 if (this.insurer == null) 8593 if (Configuration.errorOnAutoCreate()) 8594 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 8595 else if (Configuration.doAutoCreate()) 8596 this.insurer = new Reference(); // cc 8597 return this.insurer; 8598 } 8599 8600 public boolean hasInsurer() { 8601 return this.insurer != null && !this.insurer.isEmpty(); 8602 } 8603 8604 /** 8605 * @param value {@link #insurer} (The party responsible for authorization, 8606 * adjudication and reimbursement.) 8607 */ 8608 public ClaimResponse setInsurer(Reference value) { 8609 this.insurer = value; 8610 return this; 8611 } 8612 8613 /** 8614 * @return {@link #insurer} The actual object that is the target of the 8615 * reference. The reference library doesn't populate this, but you can 8616 * use it to hold the resource if you resolve it. (The party responsible 8617 * for authorization, adjudication and reimbursement.) 8618 */ 8619 public Organization getInsurerTarget() { 8620 if (this.insurerTarget == null) 8621 if (Configuration.errorOnAutoCreate()) 8622 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 8623 else if (Configuration.doAutoCreate()) 8624 this.insurerTarget = new Organization(); // aa 8625 return this.insurerTarget; 8626 } 8627 8628 /** 8629 * @param value {@link #insurer} The actual object that is the target of the 8630 * reference. The reference library doesn't use these, but you can 8631 * use it to hold the resource if you resolve it. (The party 8632 * responsible for authorization, adjudication and reimbursement.) 8633 */ 8634 public ClaimResponse setInsurerTarget(Organization value) { 8635 this.insurerTarget = value; 8636 return this; 8637 } 8638 8639 /** 8640 * @return {@link #requestor} (The provider which is responsible for the claim, 8641 * predetermination or preauthorization.) 8642 */ 8643 public Reference getRequestor() { 8644 if (this.requestor == null) 8645 if (Configuration.errorOnAutoCreate()) 8646 throw new Error("Attempt to auto-create ClaimResponse.requestor"); 8647 else if (Configuration.doAutoCreate()) 8648 this.requestor = new Reference(); // cc 8649 return this.requestor; 8650 } 8651 8652 public boolean hasRequestor() { 8653 return this.requestor != null && !this.requestor.isEmpty(); 8654 } 8655 8656 /** 8657 * @param value {@link #requestor} (The provider which is responsible for the 8658 * claim, predetermination or preauthorization.) 8659 */ 8660 public ClaimResponse setRequestor(Reference value) { 8661 this.requestor = value; 8662 return this; 8663 } 8664 8665 /** 8666 * @return {@link #requestor} The actual object that is the target of the 8667 * reference. The reference library doesn't populate this, but you can 8668 * use it to hold the resource if you resolve it. (The provider which is 8669 * responsible for the claim, predetermination or preauthorization.) 8670 */ 8671 public Resource getRequestorTarget() { 8672 return this.requestorTarget; 8673 } 8674 8675 /** 8676 * @param value {@link #requestor} The actual object that is the target of the 8677 * reference. The reference library doesn't use these, but you can 8678 * use it to hold the resource if you resolve it. (The provider 8679 * which is responsible for the claim, predetermination or 8680 * preauthorization.) 8681 */ 8682 public ClaimResponse setRequestorTarget(Resource value) { 8683 this.requestorTarget = value; 8684 return this; 8685 } 8686 8687 /** 8688 * @return {@link #request} (Original request resource reference.) 8689 */ 8690 public Reference getRequest() { 8691 if (this.request == null) 8692 if (Configuration.errorOnAutoCreate()) 8693 throw new Error("Attempt to auto-create ClaimResponse.request"); 8694 else if (Configuration.doAutoCreate()) 8695 this.request = new Reference(); // cc 8696 return this.request; 8697 } 8698 8699 public boolean hasRequest() { 8700 return this.request != null && !this.request.isEmpty(); 8701 } 8702 8703 /** 8704 * @param value {@link #request} (Original request resource reference.) 8705 */ 8706 public ClaimResponse setRequest(Reference value) { 8707 this.request = value; 8708 return this; 8709 } 8710 8711 /** 8712 * @return {@link #request} The actual object that is the target of the 8713 * reference. The reference library doesn't populate this, but you can 8714 * use it to hold the resource if you resolve it. (Original request 8715 * resource reference.) 8716 */ 8717 public Claim getRequestTarget() { 8718 if (this.requestTarget == null) 8719 if (Configuration.errorOnAutoCreate()) 8720 throw new Error("Attempt to auto-create ClaimResponse.request"); 8721 else if (Configuration.doAutoCreate()) 8722 this.requestTarget = new Claim(); // aa 8723 return this.requestTarget; 8724 } 8725 8726 /** 8727 * @param value {@link #request} The actual object that is the target of the 8728 * reference. The reference library doesn't use these, but you can 8729 * use it to hold the resource if you resolve it. (Original request 8730 * resource reference.) 8731 */ 8732 public ClaimResponse setRequestTarget(Claim value) { 8733 this.requestTarget = value; 8734 return this; 8735 } 8736 8737 /** 8738 * @return {@link #outcome} (The outcome of the claim, predetermination, or 8739 * preauthorization processing.). This is the underlying object with id, 8740 * value and extensions. The accessor "getOutcome" gives direct access 8741 * to the value 8742 */ 8743 public Enumeration<RemittanceOutcome> getOutcomeElement() { 8744 if (this.outcome == null) 8745 if (Configuration.errorOnAutoCreate()) 8746 throw new Error("Attempt to auto-create ClaimResponse.outcome"); 8747 else if (Configuration.doAutoCreate()) 8748 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 8749 return this.outcome; 8750 } 8751 8752 public boolean hasOutcomeElement() { 8753 return this.outcome != null && !this.outcome.isEmpty(); 8754 } 8755 8756 public boolean hasOutcome() { 8757 return this.outcome != null && !this.outcome.isEmpty(); 8758 } 8759 8760 /** 8761 * @param value {@link #outcome} (The outcome of the claim, predetermination, or 8762 * preauthorization processing.). This is the underlying object 8763 * with id, value and extensions. The accessor "getOutcome" gives 8764 * direct access to the value 8765 */ 8766 public ClaimResponse setOutcomeElement(Enumeration<RemittanceOutcome> value) { 8767 this.outcome = value; 8768 return this; 8769 } 8770 8771 /** 8772 * @return The outcome of the claim, predetermination, or preauthorization 8773 * processing. 8774 */ 8775 public RemittanceOutcome getOutcome() { 8776 return this.outcome == null ? null : this.outcome.getValue(); 8777 } 8778 8779 /** 8780 * @param value The outcome of the claim, predetermination, or preauthorization 8781 * processing. 8782 */ 8783 public ClaimResponse setOutcome(RemittanceOutcome value) { 8784 if (this.outcome == null) 8785 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 8786 this.outcome.setValue(value); 8787 return this; 8788 } 8789 8790 /** 8791 * @return {@link #disposition} (A human readable description of the status of 8792 * the adjudication.). This is the underlying object with id, value and 8793 * extensions. The accessor "getDisposition" gives direct access to the 8794 * value 8795 */ 8796 public StringType getDispositionElement() { 8797 if (this.disposition == null) 8798 if (Configuration.errorOnAutoCreate()) 8799 throw new Error("Attempt to auto-create ClaimResponse.disposition"); 8800 else if (Configuration.doAutoCreate()) 8801 this.disposition = new StringType(); // bb 8802 return this.disposition; 8803 } 8804 8805 public boolean hasDispositionElement() { 8806 return this.disposition != null && !this.disposition.isEmpty(); 8807 } 8808 8809 public boolean hasDisposition() { 8810 return this.disposition != null && !this.disposition.isEmpty(); 8811 } 8812 8813 /** 8814 * @param value {@link #disposition} (A human readable description of the status 8815 * of the adjudication.). This is the underlying object with id, 8816 * value and extensions. The accessor "getDisposition" gives direct 8817 * access to the value 8818 */ 8819 public ClaimResponse setDispositionElement(StringType value) { 8820 this.disposition = value; 8821 return this; 8822 } 8823 8824 /** 8825 * @return A human readable description of the status of the adjudication. 8826 */ 8827 public String getDisposition() { 8828 return this.disposition == null ? null : this.disposition.getValue(); 8829 } 8830 8831 /** 8832 * @param value A human readable description of the status of the adjudication. 8833 */ 8834 public ClaimResponse setDisposition(String value) { 8835 if (Utilities.noString(value)) 8836 this.disposition = null; 8837 else { 8838 if (this.disposition == null) 8839 this.disposition = new StringType(); 8840 this.disposition.setValue(value); 8841 } 8842 return this; 8843 } 8844 8845 /** 8846 * @return {@link #preAuthRef} (Reference from the Insurer which is used in 8847 * later communications which refers to this adjudication.). This is the 8848 * underlying object with id, value and extensions. The accessor 8849 * "getPreAuthRef" gives direct access to the value 8850 */ 8851 public StringType getPreAuthRefElement() { 8852 if (this.preAuthRef == null) 8853 if (Configuration.errorOnAutoCreate()) 8854 throw new Error("Attempt to auto-create ClaimResponse.preAuthRef"); 8855 else if (Configuration.doAutoCreate()) 8856 this.preAuthRef = new StringType(); // bb 8857 return this.preAuthRef; 8858 } 8859 8860 public boolean hasPreAuthRefElement() { 8861 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 8862 } 8863 8864 public boolean hasPreAuthRef() { 8865 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 8866 } 8867 8868 /** 8869 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in 8870 * later communications which refers to this adjudication.). This 8871 * is the underlying object with id, value and extensions. The 8872 * accessor "getPreAuthRef" gives direct access to the value 8873 */ 8874 public ClaimResponse setPreAuthRefElement(StringType value) { 8875 this.preAuthRef = value; 8876 return this; 8877 } 8878 8879 /** 8880 * @return Reference from the Insurer which is used in later communications 8881 * which refers to this adjudication. 8882 */ 8883 public String getPreAuthRef() { 8884 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 8885 } 8886 8887 /** 8888 * @param value Reference from the Insurer which is used in later communications 8889 * which refers to this adjudication. 8890 */ 8891 public ClaimResponse setPreAuthRef(String value) { 8892 if (Utilities.noString(value)) 8893 this.preAuthRef = null; 8894 else { 8895 if (this.preAuthRef == null) 8896 this.preAuthRef = new StringType(); 8897 this.preAuthRef.setValue(value); 8898 } 8899 return this; 8900 } 8901 8902 /** 8903 * @return {@link #preAuthPeriod} (The time frame during which this 8904 * authorization is effective.) 8905 */ 8906 public Period getPreAuthPeriod() { 8907 if (this.preAuthPeriod == null) 8908 if (Configuration.errorOnAutoCreate()) 8909 throw new Error("Attempt to auto-create ClaimResponse.preAuthPeriod"); 8910 else if (Configuration.doAutoCreate()) 8911 this.preAuthPeriod = new Period(); // cc 8912 return this.preAuthPeriod; 8913 } 8914 8915 public boolean hasPreAuthPeriod() { 8916 return this.preAuthPeriod != null && !this.preAuthPeriod.isEmpty(); 8917 } 8918 8919 /** 8920 * @param value {@link #preAuthPeriod} (The time frame during which this 8921 * authorization is effective.) 8922 */ 8923 public ClaimResponse setPreAuthPeriod(Period value) { 8924 this.preAuthPeriod = value; 8925 return this; 8926 } 8927 8928 /** 8929 * @return {@link #payeeType} (Type of Party to be reimbursed: subscriber, 8930 * provider, other.) 8931 */ 8932 public CodeableConcept getPayeeType() { 8933 if (this.payeeType == null) 8934 if (Configuration.errorOnAutoCreate()) 8935 throw new Error("Attempt to auto-create ClaimResponse.payeeType"); 8936 else if (Configuration.doAutoCreate()) 8937 this.payeeType = new CodeableConcept(); // cc 8938 return this.payeeType; 8939 } 8940 8941 public boolean hasPayeeType() { 8942 return this.payeeType != null && !this.payeeType.isEmpty(); 8943 } 8944 8945 /** 8946 * @param value {@link #payeeType} (Type of Party to be reimbursed: subscriber, 8947 * provider, other.) 8948 */ 8949 public ClaimResponse setPayeeType(CodeableConcept value) { 8950 this.payeeType = value; 8951 return this; 8952 } 8953 8954 /** 8955 * @return {@link #item} (A claim line. Either a simple (a product or service) 8956 * or a 'group' of details which can also be a simple items or groups of 8957 * sub-details.) 8958 */ 8959 public List<ItemComponent> getItem() { 8960 if (this.item == null) 8961 this.item = new ArrayList<ItemComponent>(); 8962 return this.item; 8963 } 8964 8965 /** 8966 * @return Returns a reference to <code>this</code> for easy method chaining 8967 */ 8968 public ClaimResponse setItem(List<ItemComponent> theItem) { 8969 this.item = theItem; 8970 return this; 8971 } 8972 8973 public boolean hasItem() { 8974 if (this.item == null) 8975 return false; 8976 for (ItemComponent item : this.item) 8977 if (!item.isEmpty()) 8978 return true; 8979 return false; 8980 } 8981 8982 public ItemComponent addItem() { // 3 8983 ItemComponent t = new ItemComponent(); 8984 if (this.item == null) 8985 this.item = new ArrayList<ItemComponent>(); 8986 this.item.add(t); 8987 return t; 8988 } 8989 8990 public ClaimResponse addItem(ItemComponent t) { // 3 8991 if (t == null) 8992 return this; 8993 if (this.item == null) 8994 this.item = new ArrayList<ItemComponent>(); 8995 this.item.add(t); 8996 return this; 8997 } 8998 8999 /** 9000 * @return The first repetition of repeating field {@link #item}, creating it if 9001 * it does not already exist 9002 */ 9003 public ItemComponent getItemFirstRep() { 9004 if (getItem().isEmpty()) { 9005 addItem(); 9006 } 9007 return getItem().get(0); 9008 } 9009 9010 /** 9011 * @return {@link #addItem} (The first-tier service adjudications for payor 9012 * added product or service lines.) 9013 */ 9014 public List<AddedItemComponent> getAddItem() { 9015 if (this.addItem == null) 9016 this.addItem = new ArrayList<AddedItemComponent>(); 9017 return this.addItem; 9018 } 9019 9020 /** 9021 * @return Returns a reference to <code>this</code> for easy method chaining 9022 */ 9023 public ClaimResponse setAddItem(List<AddedItemComponent> theAddItem) { 9024 this.addItem = theAddItem; 9025 return this; 9026 } 9027 9028 public boolean hasAddItem() { 9029 if (this.addItem == null) 9030 return false; 9031 for (AddedItemComponent item : this.addItem) 9032 if (!item.isEmpty()) 9033 return true; 9034 return false; 9035 } 9036 9037 public AddedItemComponent addAddItem() { // 3 9038 AddedItemComponent t = new AddedItemComponent(); 9039 if (this.addItem == null) 9040 this.addItem = new ArrayList<AddedItemComponent>(); 9041 this.addItem.add(t); 9042 return t; 9043 } 9044 9045 public ClaimResponse addAddItem(AddedItemComponent t) { // 3 9046 if (t == null) 9047 return this; 9048 if (this.addItem == null) 9049 this.addItem = new ArrayList<AddedItemComponent>(); 9050 this.addItem.add(t); 9051 return this; 9052 } 9053 9054 /** 9055 * @return The first repetition of repeating field {@link #addItem}, creating it 9056 * if it does not already exist 9057 */ 9058 public AddedItemComponent getAddItemFirstRep() { 9059 if (getAddItem().isEmpty()) { 9060 addAddItem(); 9061 } 9062 return getAddItem().get(0); 9063 } 9064 9065 /** 9066 * @return {@link #adjudication} (The adjudication results which are presented 9067 * at the header level rather than at the line-item or add-item levels.) 9068 */ 9069 public List<AdjudicationComponent> getAdjudication() { 9070 if (this.adjudication == null) 9071 this.adjudication = new ArrayList<AdjudicationComponent>(); 9072 return this.adjudication; 9073 } 9074 9075 /** 9076 * @return Returns a reference to <code>this</code> for easy method chaining 9077 */ 9078 public ClaimResponse setAdjudication(List<AdjudicationComponent> theAdjudication) { 9079 this.adjudication = theAdjudication; 9080 return this; 9081 } 9082 9083 public boolean hasAdjudication() { 9084 if (this.adjudication == null) 9085 return false; 9086 for (AdjudicationComponent item : this.adjudication) 9087 if (!item.isEmpty()) 9088 return true; 9089 return false; 9090 } 9091 9092 public AdjudicationComponent addAdjudication() { // 3 9093 AdjudicationComponent t = new AdjudicationComponent(); 9094 if (this.adjudication == null) 9095 this.adjudication = new ArrayList<AdjudicationComponent>(); 9096 this.adjudication.add(t); 9097 return t; 9098 } 9099 9100 public ClaimResponse addAdjudication(AdjudicationComponent t) { // 3 9101 if (t == null) 9102 return this; 9103 if (this.adjudication == null) 9104 this.adjudication = new ArrayList<AdjudicationComponent>(); 9105 this.adjudication.add(t); 9106 return this; 9107 } 9108 9109 /** 9110 * @return The first repetition of repeating field {@link #adjudication}, 9111 * creating it if it does not already exist 9112 */ 9113 public AdjudicationComponent getAdjudicationFirstRep() { 9114 if (getAdjudication().isEmpty()) { 9115 addAdjudication(); 9116 } 9117 return getAdjudication().get(0); 9118 } 9119 9120 /** 9121 * @return {@link #total} (Categorized monetary totals for the adjudication.) 9122 */ 9123 public List<TotalComponent> getTotal() { 9124 if (this.total == null) 9125 this.total = new ArrayList<TotalComponent>(); 9126 return this.total; 9127 } 9128 9129 /** 9130 * @return Returns a reference to <code>this</code> for easy method chaining 9131 */ 9132 public ClaimResponse setTotal(List<TotalComponent> theTotal) { 9133 this.total = theTotal; 9134 return this; 9135 } 9136 9137 public boolean hasTotal() { 9138 if (this.total == null) 9139 return false; 9140 for (TotalComponent item : this.total) 9141 if (!item.isEmpty()) 9142 return true; 9143 return false; 9144 } 9145 9146 public TotalComponent addTotal() { // 3 9147 TotalComponent t = new TotalComponent(); 9148 if (this.total == null) 9149 this.total = new ArrayList<TotalComponent>(); 9150 this.total.add(t); 9151 return t; 9152 } 9153 9154 public ClaimResponse addTotal(TotalComponent t) { // 3 9155 if (t == null) 9156 return this; 9157 if (this.total == null) 9158 this.total = new ArrayList<TotalComponent>(); 9159 this.total.add(t); 9160 return this; 9161 } 9162 9163 /** 9164 * @return The first repetition of repeating field {@link #total}, creating it 9165 * if it does not already exist 9166 */ 9167 public TotalComponent getTotalFirstRep() { 9168 if (getTotal().isEmpty()) { 9169 addTotal(); 9170 } 9171 return getTotal().get(0); 9172 } 9173 9174 /** 9175 * @return {@link #payment} (Payment details for the adjudication of the claim.) 9176 */ 9177 public PaymentComponent getPayment() { 9178 if (this.payment == null) 9179 if (Configuration.errorOnAutoCreate()) 9180 throw new Error("Attempt to auto-create ClaimResponse.payment"); 9181 else if (Configuration.doAutoCreate()) 9182 this.payment = new PaymentComponent(); // cc 9183 return this.payment; 9184 } 9185 9186 public boolean hasPayment() { 9187 return this.payment != null && !this.payment.isEmpty(); 9188 } 9189 9190 /** 9191 * @param value {@link #payment} (Payment details for the adjudication of the 9192 * claim.) 9193 */ 9194 public ClaimResponse setPayment(PaymentComponent value) { 9195 this.payment = value; 9196 return this; 9197 } 9198 9199 /** 9200 * @return {@link #fundsReserve} (A code, used only on a response to a 9201 * preauthorization, to indicate whether the benefits payable have been 9202 * reserved and for whom.) 9203 */ 9204 public CodeableConcept getFundsReserve() { 9205 if (this.fundsReserve == null) 9206 if (Configuration.errorOnAutoCreate()) 9207 throw new Error("Attempt to auto-create ClaimResponse.fundsReserve"); 9208 else if (Configuration.doAutoCreate()) 9209 this.fundsReserve = new CodeableConcept(); // cc 9210 return this.fundsReserve; 9211 } 9212 9213 public boolean hasFundsReserve() { 9214 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 9215 } 9216 9217 /** 9218 * @param value {@link #fundsReserve} (A code, used only on a response to a 9219 * preauthorization, to indicate whether the benefits payable have 9220 * been reserved and for whom.) 9221 */ 9222 public ClaimResponse setFundsReserve(CodeableConcept value) { 9223 this.fundsReserve = value; 9224 return this; 9225 } 9226 9227 /** 9228 * @return {@link #formCode} (A code for the form to be used for printing the 9229 * content.) 9230 */ 9231 public CodeableConcept getFormCode() { 9232 if (this.formCode == null) 9233 if (Configuration.errorOnAutoCreate()) 9234 throw new Error("Attempt to auto-create ClaimResponse.formCode"); 9235 else if (Configuration.doAutoCreate()) 9236 this.formCode = new CodeableConcept(); // cc 9237 return this.formCode; 9238 } 9239 9240 public boolean hasFormCode() { 9241 return this.formCode != null && !this.formCode.isEmpty(); 9242 } 9243 9244 /** 9245 * @param value {@link #formCode} (A code for the form to be used for printing 9246 * the content.) 9247 */ 9248 public ClaimResponse setFormCode(CodeableConcept value) { 9249 this.formCode = value; 9250 return this; 9251 } 9252 9253 /** 9254 * @return {@link #form} (The actual form, by reference or inclusion, for 9255 * printing the content or an EOB.) 9256 */ 9257 public Attachment getForm() { 9258 if (this.form == null) 9259 if (Configuration.errorOnAutoCreate()) 9260 throw new Error("Attempt to auto-create ClaimResponse.form"); 9261 else if (Configuration.doAutoCreate()) 9262 this.form = new Attachment(); // cc 9263 return this.form; 9264 } 9265 9266 public boolean hasForm() { 9267 return this.form != null && !this.form.isEmpty(); 9268 } 9269 9270 /** 9271 * @param value {@link #form} (The actual form, by reference or inclusion, for 9272 * printing the content or an EOB.) 9273 */ 9274 public ClaimResponse setForm(Attachment value) { 9275 this.form = value; 9276 return this; 9277 } 9278 9279 /** 9280 * @return {@link #processNote} (A note that describes or explains adjudication 9281 * results in a human readable form.) 9282 */ 9283 public List<NoteComponent> getProcessNote() { 9284 if (this.processNote == null) 9285 this.processNote = new ArrayList<NoteComponent>(); 9286 return this.processNote; 9287 } 9288 9289 /** 9290 * @return Returns a reference to <code>this</code> for easy method chaining 9291 */ 9292 public ClaimResponse setProcessNote(List<NoteComponent> theProcessNote) { 9293 this.processNote = theProcessNote; 9294 return this; 9295 } 9296 9297 public boolean hasProcessNote() { 9298 if (this.processNote == null) 9299 return false; 9300 for (NoteComponent item : this.processNote) 9301 if (!item.isEmpty()) 9302 return true; 9303 return false; 9304 } 9305 9306 public NoteComponent addProcessNote() { // 3 9307 NoteComponent t = new NoteComponent(); 9308 if (this.processNote == null) 9309 this.processNote = new ArrayList<NoteComponent>(); 9310 this.processNote.add(t); 9311 return t; 9312 } 9313 9314 public ClaimResponse addProcessNote(NoteComponent t) { // 3 9315 if (t == null) 9316 return this; 9317 if (this.processNote == null) 9318 this.processNote = new ArrayList<NoteComponent>(); 9319 this.processNote.add(t); 9320 return this; 9321 } 9322 9323 /** 9324 * @return The first repetition of repeating field {@link #processNote}, 9325 * creating it if it does not already exist 9326 */ 9327 public NoteComponent getProcessNoteFirstRep() { 9328 if (getProcessNote().isEmpty()) { 9329 addProcessNote(); 9330 } 9331 return getProcessNote().get(0); 9332 } 9333 9334 /** 9335 * @return {@link #communicationRequest} (Request for additional supporting or 9336 * authorizing information.) 9337 */ 9338 public List<Reference> getCommunicationRequest() { 9339 if (this.communicationRequest == null) 9340 this.communicationRequest = new ArrayList<Reference>(); 9341 return this.communicationRequest; 9342 } 9343 9344 /** 9345 * @return Returns a reference to <code>this</code> for easy method chaining 9346 */ 9347 public ClaimResponse setCommunicationRequest(List<Reference> theCommunicationRequest) { 9348 this.communicationRequest = theCommunicationRequest; 9349 return this; 9350 } 9351 9352 public boolean hasCommunicationRequest() { 9353 if (this.communicationRequest == null) 9354 return false; 9355 for (Reference item : this.communicationRequest) 9356 if (!item.isEmpty()) 9357 return true; 9358 return false; 9359 } 9360 9361 public Reference addCommunicationRequest() { // 3 9362 Reference t = new Reference(); 9363 if (this.communicationRequest == null) 9364 this.communicationRequest = new ArrayList<Reference>(); 9365 this.communicationRequest.add(t); 9366 return t; 9367 } 9368 9369 public ClaimResponse addCommunicationRequest(Reference t) { // 3 9370 if (t == null) 9371 return this; 9372 if (this.communicationRequest == null) 9373 this.communicationRequest = new ArrayList<Reference>(); 9374 this.communicationRequest.add(t); 9375 return this; 9376 } 9377 9378 /** 9379 * @return The first repetition of repeating field 9380 * {@link #communicationRequest}, creating it if it does not already 9381 * exist 9382 */ 9383 public Reference getCommunicationRequestFirstRep() { 9384 if (getCommunicationRequest().isEmpty()) { 9385 addCommunicationRequest(); 9386 } 9387 return getCommunicationRequest().get(0); 9388 } 9389 9390 /** 9391 * @return {@link #insurance} (Financial instruments for reimbursement for the 9392 * health care products and services specified on the claim.) 9393 */ 9394 public List<InsuranceComponent> getInsurance() { 9395 if (this.insurance == null) 9396 this.insurance = new ArrayList<InsuranceComponent>(); 9397 return this.insurance; 9398 } 9399 9400 /** 9401 * @return Returns a reference to <code>this</code> for easy method chaining 9402 */ 9403 public ClaimResponse setInsurance(List<InsuranceComponent> theInsurance) { 9404 this.insurance = theInsurance; 9405 return this; 9406 } 9407 9408 public boolean hasInsurance() { 9409 if (this.insurance == null) 9410 return false; 9411 for (InsuranceComponent item : this.insurance) 9412 if (!item.isEmpty()) 9413 return true; 9414 return false; 9415 } 9416 9417 public InsuranceComponent addInsurance() { // 3 9418 InsuranceComponent t = new InsuranceComponent(); 9419 if (this.insurance == null) 9420 this.insurance = new ArrayList<InsuranceComponent>(); 9421 this.insurance.add(t); 9422 return t; 9423 } 9424 9425 public ClaimResponse addInsurance(InsuranceComponent t) { // 3 9426 if (t == null) 9427 return this; 9428 if (this.insurance == null) 9429 this.insurance = new ArrayList<InsuranceComponent>(); 9430 this.insurance.add(t); 9431 return this; 9432 } 9433 9434 /** 9435 * @return The first repetition of repeating field {@link #insurance}, creating 9436 * it if it does not already exist 9437 */ 9438 public InsuranceComponent getInsuranceFirstRep() { 9439 if (getInsurance().isEmpty()) { 9440 addInsurance(); 9441 } 9442 return getInsurance().get(0); 9443 } 9444 9445 /** 9446 * @return {@link #error} (Errors encountered during the processing of the 9447 * adjudication.) 9448 */ 9449 public List<ErrorComponent> getError() { 9450 if (this.error == null) 9451 this.error = new ArrayList<ErrorComponent>(); 9452 return this.error; 9453 } 9454 9455 /** 9456 * @return Returns a reference to <code>this</code> for easy method chaining 9457 */ 9458 public ClaimResponse setError(List<ErrorComponent> theError) { 9459 this.error = theError; 9460 return this; 9461 } 9462 9463 public boolean hasError() { 9464 if (this.error == null) 9465 return false; 9466 for (ErrorComponent item : this.error) 9467 if (!item.isEmpty()) 9468 return true; 9469 return false; 9470 } 9471 9472 public ErrorComponent addError() { // 3 9473 ErrorComponent t = new ErrorComponent(); 9474 if (this.error == null) 9475 this.error = new ArrayList<ErrorComponent>(); 9476 this.error.add(t); 9477 return t; 9478 } 9479 9480 public ClaimResponse addError(ErrorComponent t) { // 3 9481 if (t == null) 9482 return this; 9483 if (this.error == null) 9484 this.error = new ArrayList<ErrorComponent>(); 9485 this.error.add(t); 9486 return this; 9487 } 9488 9489 /** 9490 * @return The first repetition of repeating field {@link #error}, creating it 9491 * if it does not already exist 9492 */ 9493 public ErrorComponent getErrorFirstRep() { 9494 if (getError().isEmpty()) { 9495 addError(); 9496 } 9497 return getError().get(0); 9498 } 9499 9500 protected void listChildren(List<Property> children) { 9501 super.listChildren(children); 9502 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim response.", 0, 9503 java.lang.Integer.MAX_VALUE, identifier)); 9504 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 9505 children.add(new Property("type", "CodeableConcept", 9506 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9507 0, 1, type)); 9508 children.add(new Property("subType", "CodeableConcept", 9509 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9510 0, 1, subType)); 9511 children.add(new Property("use", "code", 9512 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 9513 0, 1, use)); 9514 children.add(new Property("patient", "Reference(Patient)", 9515 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.", 9516 0, 1, patient)); 9517 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 9518 children.add(new Property("insurer", "Reference(Organization)", 9519 "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer)); 9520 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 9521 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, requestor)); 9522 children.add(new Property("request", "Reference(Claim)", "Original request resource reference.", 0, 1, request)); 9523 children.add(new Property("outcome", "code", 9524 "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome)); 9525 children.add(new Property("disposition", "string", 9526 "A human readable description of the status of the adjudication.", 0, 1, disposition)); 9527 children.add(new Property("preAuthRef", "string", 9528 "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, 9529 preAuthRef)); 9530 children.add(new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 9531 0, 1, preAuthPeriod)); 9532 children.add(new Property("payeeType", "CodeableConcept", 9533 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, payeeType)); 9534 children.add(new Property("item", "", 9535 "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 9536 0, java.lang.Integer.MAX_VALUE, item)); 9537 children.add( 9538 new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, 9539 java.lang.Integer.MAX_VALUE, addItem)); 9540 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", 9541 "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 9542 0, java.lang.Integer.MAX_VALUE, adjudication)); 9543 children.add(new Property("total", "", "Categorized monetary totals for the adjudication.", 0, 9544 java.lang.Integer.MAX_VALUE, total)); 9545 children.add(new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment)); 9546 children.add(new Property("fundsReserve", "CodeableConcept", 9547 "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 9548 0, 1, fundsReserve)); 9549 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 9550 0, 1, formCode)); 9551 children.add(new Property("form", "Attachment", 9552 "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form)); 9553 children.add(new Property("processNote", "", 9554 "A note that describes or explains adjudication results in a human readable form.", 0, 9555 java.lang.Integer.MAX_VALUE, processNote)); 9556 children.add(new Property("communicationRequest", "Reference(CommunicationRequest)", 9557 "Request for additional supporting or authorizing information.", 0, java.lang.Integer.MAX_VALUE, 9558 communicationRequest)); 9559 children.add(new Property("insurance", "", 9560 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, 9561 java.lang.Integer.MAX_VALUE, insurance)); 9562 children.add(new Property("error", "", "Errors encountered during the processing of the adjudication.", 0, 9563 java.lang.Integer.MAX_VALUE, error)); 9564 } 9565 9566 @Override 9567 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9568 switch (_hash) { 9569 case -1618432855: 9570 /* identifier */ return new Property("identifier", "Identifier", 9571 "A unique identifier assigned to this claim response.", 0, java.lang.Integer.MAX_VALUE, identifier); 9572 case -892481550: 9573 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 9574 case 3575610: 9575 /* type */ return new Property("type", "CodeableConcept", 9576 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9577 0, 1, type); 9578 case -1868521062: 9579 /* subType */ return new Property("subType", "CodeableConcept", 9580 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9581 0, 1, subType); 9582 case 116103: 9583 /* use */ return new Property("use", "code", 9584 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 9585 0, 1, use); 9586 case -791418107: 9587 /* patient */ return new Property("patient", "Reference(Patient)", 9588 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.", 9589 0, 1, patient); 9590 case 1028554472: 9591 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 9592 case 1957615864: 9593 /* insurer */ return new Property("insurer", "Reference(Organization)", 9594 "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer); 9595 case 693934258: 9596 /* requestor */ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 9597 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, requestor); 9598 case 1095692943: 9599 /* request */ return new Property("request", "Reference(Claim)", "Original request resource reference.", 0, 1, 9600 request); 9601 case -1106507950: 9602 /* outcome */ return new Property("outcome", "code", 9603 "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome); 9604 case 583380919: 9605 /* disposition */ return new Property("disposition", "string", 9606 "A human readable description of the status of the adjudication.", 0, 1, disposition); 9607 case 522246568: 9608 /* preAuthRef */ return new Property("preAuthRef", "string", 9609 "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, 9610 preAuthRef); 9611 case 1819164812: 9612 /* preAuthPeriod */ return new Property("preAuthPeriod", "Period", 9613 "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod); 9614 case -316321118: 9615 /* payeeType */ return new Property("payeeType", "CodeableConcept", 9616 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, payeeType); 9617 case 3242771: 9618 /* item */ return new Property("item", "", 9619 "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 9620 0, java.lang.Integer.MAX_VALUE, item); 9621 case -1148899500: 9622 /* addItem */ return new Property("addItem", "", 9623 "The first-tier service adjudications for payor added product or service lines.", 0, 9624 java.lang.Integer.MAX_VALUE, addItem); 9625 case -231349275: 9626 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 9627 "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 9628 0, java.lang.Integer.MAX_VALUE, adjudication); 9629 case 110549828: 9630 /* total */ return new Property("total", "", "Categorized monetary totals for the adjudication.", 0, 9631 java.lang.Integer.MAX_VALUE, total); 9632 case -786681338: 9633 /* payment */ return new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, 9634 payment); 9635 case 1314609806: 9636 /* fundsReserve */ return new Property("fundsReserve", "CodeableConcept", 9637 "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 9638 0, 1, fundsReserve); 9639 case 473181393: 9640 /* formCode */ return new Property("formCode", "CodeableConcept", 9641 "A code for the form to be used for printing the content.", 0, 1, formCode); 9642 case 3148996: 9643 /* form */ return new Property("form", "Attachment", 9644 "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form); 9645 case 202339073: 9646 /* processNote */ return new Property("processNote", "", 9647 "A note that describes or explains adjudication results in a human readable form.", 0, 9648 java.lang.Integer.MAX_VALUE, processNote); 9649 case -2071896615: 9650 /* communicationRequest */ return new Property("communicationRequest", "Reference(CommunicationRequest)", 9651 "Request for additional supporting or authorizing information.", 0, java.lang.Integer.MAX_VALUE, 9652 communicationRequest); 9653 case 73049818: 9654 /* insurance */ return new Property("insurance", "", 9655 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 9656 0, java.lang.Integer.MAX_VALUE, insurance); 9657 case 96784904: 9658 /* error */ return new Property("error", "", "Errors encountered during the processing of the adjudication.", 0, 9659 java.lang.Integer.MAX_VALUE, error); 9660 default: 9661 return super.getNamedProperty(_hash, _name, _checkValid); 9662 } 9663 9664 } 9665 9666 @Override 9667 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9668 switch (hash) { 9669 case -1618432855: 9670 /* identifier */ return this.identifier == null ? new Base[0] 9671 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 9672 case -892481550: 9673 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ClaimResponseStatus> 9674 case 3575610: 9675 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 9676 case -1868521062: 9677 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 9678 case 116103: 9679 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<Use> 9680 case -791418107: 9681 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 9682 case 1028554472: 9683 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 9684 case 1957615864: 9685 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 9686 case 693934258: 9687 /* requestor */ return this.requestor == null ? new Base[0] : new Base[] { this.requestor }; // Reference 9688 case 1095692943: 9689 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 9690 case -1106507950: 9691 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<RemittanceOutcome> 9692 case 583380919: 9693 /* disposition */ return this.disposition == null ? new Base[0] : new Base[] { this.disposition }; // StringType 9694 case 522246568: 9695 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] : new Base[] { this.preAuthRef }; // StringType 9696 case 1819164812: 9697 /* preAuthPeriod */ return this.preAuthPeriod == null ? new Base[0] : new Base[] { this.preAuthPeriod }; // Period 9698 case -316321118: 9699 /* payeeType */ return this.payeeType == null ? new Base[0] : new Base[] { this.payeeType }; // CodeableConcept 9700 case 3242771: 9701 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 9702 case -1148899500: 9703 /* addItem */ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 9704 case -231349275: 9705 /* adjudication */ return this.adjudication == null ? new Base[0] 9706 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 9707 case 110549828: 9708 /* total */ return this.total == null ? new Base[0] : this.total.toArray(new Base[this.total.size()]); // TotalComponent 9709 case -786681338: 9710 /* payment */ return this.payment == null ? new Base[0] : new Base[] { this.payment }; // PaymentComponent 9711 case 1314609806: 9712 /* fundsReserve */ return this.fundsReserve == null ? new Base[0] : new Base[] { this.fundsReserve }; // CodeableConcept 9713 case 473181393: 9714 /* formCode */ return this.formCode == null ? new Base[0] : new Base[] { this.formCode }; // CodeableConcept 9715 case 3148996: 9716 /* form */ return this.form == null ? new Base[0] : new Base[] { this.form }; // Attachment 9717 case 202339073: 9718 /* processNote */ return this.processNote == null ? new Base[0] 9719 : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 9720 case -2071896615: 9721 /* communicationRequest */ return this.communicationRequest == null ? new Base[0] 9722 : this.communicationRequest.toArray(new Base[this.communicationRequest.size()]); // Reference 9723 case 73049818: 9724 /* insurance */ return this.insurance == null ? new Base[0] 9725 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 9726 case 96784904: 9727 /* error */ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorComponent 9728 default: 9729 return super.getProperty(hash, name, checkValid); 9730 } 9731 9732 } 9733 9734 @Override 9735 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9736 switch (hash) { 9737 case -1618432855: // identifier 9738 this.getIdentifier().add(castToIdentifier(value)); // Identifier 9739 return value; 9740 case -892481550: // status 9741 value = new ClaimResponseStatusEnumFactory().fromType(castToCode(value)); 9742 this.status = (Enumeration) value; // Enumeration<ClaimResponseStatus> 9743 return value; 9744 case 3575610: // type 9745 this.type = castToCodeableConcept(value); // CodeableConcept 9746 return value; 9747 case -1868521062: // subType 9748 this.subType = castToCodeableConcept(value); // CodeableConcept 9749 return value; 9750 case 116103: // use 9751 value = new UseEnumFactory().fromType(castToCode(value)); 9752 this.use = (Enumeration) value; // Enumeration<Use> 9753 return value; 9754 case -791418107: // patient 9755 this.patient = castToReference(value); // Reference 9756 return value; 9757 case 1028554472: // created 9758 this.created = castToDateTime(value); // DateTimeType 9759 return value; 9760 case 1957615864: // insurer 9761 this.insurer = castToReference(value); // Reference 9762 return value; 9763 case 693934258: // requestor 9764 this.requestor = castToReference(value); // Reference 9765 return value; 9766 case 1095692943: // request 9767 this.request = castToReference(value); // Reference 9768 return value; 9769 case -1106507950: // outcome 9770 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 9771 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 9772 return value; 9773 case 583380919: // disposition 9774 this.disposition = castToString(value); // StringType 9775 return value; 9776 case 522246568: // preAuthRef 9777 this.preAuthRef = castToString(value); // StringType 9778 return value; 9779 case 1819164812: // preAuthPeriod 9780 this.preAuthPeriod = castToPeriod(value); // Period 9781 return value; 9782 case -316321118: // payeeType 9783 this.payeeType = castToCodeableConcept(value); // CodeableConcept 9784 return value; 9785 case 3242771: // item 9786 this.getItem().add((ItemComponent) value); // ItemComponent 9787 return value; 9788 case -1148899500: // addItem 9789 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 9790 return value; 9791 case -231349275: // adjudication 9792 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 9793 return value; 9794 case 110549828: // total 9795 this.getTotal().add((TotalComponent) value); // TotalComponent 9796 return value; 9797 case -786681338: // payment 9798 this.payment = (PaymentComponent) value; // PaymentComponent 9799 return value; 9800 case 1314609806: // fundsReserve 9801 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 9802 return value; 9803 case 473181393: // formCode 9804 this.formCode = castToCodeableConcept(value); // CodeableConcept 9805 return value; 9806 case 3148996: // form 9807 this.form = castToAttachment(value); // Attachment 9808 return value; 9809 case 202339073: // processNote 9810 this.getProcessNote().add((NoteComponent) value); // NoteComponent 9811 return value; 9812 case -2071896615: // communicationRequest 9813 this.getCommunicationRequest().add(castToReference(value)); // Reference 9814 return value; 9815 case 73049818: // insurance 9816 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 9817 return value; 9818 case 96784904: // error 9819 this.getError().add((ErrorComponent) value); // ErrorComponent 9820 return value; 9821 default: 9822 return super.setProperty(hash, name, value); 9823 } 9824 9825 } 9826 9827 @Override 9828 public Base setProperty(String name, Base value) throws FHIRException { 9829 if (name.equals("identifier")) { 9830 this.getIdentifier().add(castToIdentifier(value)); 9831 } else if (name.equals("status")) { 9832 value = new ClaimResponseStatusEnumFactory().fromType(castToCode(value)); 9833 this.status = (Enumeration) value; // Enumeration<ClaimResponseStatus> 9834 } else if (name.equals("type")) { 9835 this.type = castToCodeableConcept(value); // CodeableConcept 9836 } else if (name.equals("subType")) { 9837 this.subType = castToCodeableConcept(value); // CodeableConcept 9838 } else if (name.equals("use")) { 9839 value = new UseEnumFactory().fromType(castToCode(value)); 9840 this.use = (Enumeration) value; // Enumeration<Use> 9841 } else if (name.equals("patient")) { 9842 this.patient = castToReference(value); // Reference 9843 } else if (name.equals("created")) { 9844 this.created = castToDateTime(value); // DateTimeType 9845 } else if (name.equals("insurer")) { 9846 this.insurer = castToReference(value); // Reference 9847 } else if (name.equals("requestor")) { 9848 this.requestor = castToReference(value); // Reference 9849 } else if (name.equals("request")) { 9850 this.request = castToReference(value); // Reference 9851 } else if (name.equals("outcome")) { 9852 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 9853 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 9854 } else if (name.equals("disposition")) { 9855 this.disposition = castToString(value); // StringType 9856 } else if (name.equals("preAuthRef")) { 9857 this.preAuthRef = castToString(value); // StringType 9858 } else if (name.equals("preAuthPeriod")) { 9859 this.preAuthPeriod = castToPeriod(value); // Period 9860 } else if (name.equals("payeeType")) { 9861 this.payeeType = castToCodeableConcept(value); // CodeableConcept 9862 } else if (name.equals("item")) { 9863 this.getItem().add((ItemComponent) value); 9864 } else if (name.equals("addItem")) { 9865 this.getAddItem().add((AddedItemComponent) value); 9866 } else if (name.equals("adjudication")) { 9867 this.getAdjudication().add((AdjudicationComponent) value); 9868 } else if (name.equals("total")) { 9869 this.getTotal().add((TotalComponent) value); 9870 } else if (name.equals("payment")) { 9871 this.payment = (PaymentComponent) value; // PaymentComponent 9872 } else if (name.equals("fundsReserve")) { 9873 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 9874 } else if (name.equals("formCode")) { 9875 this.formCode = castToCodeableConcept(value); // CodeableConcept 9876 } else if (name.equals("form")) { 9877 this.form = castToAttachment(value); // Attachment 9878 } else if (name.equals("processNote")) { 9879 this.getProcessNote().add((NoteComponent) value); 9880 } else if (name.equals("communicationRequest")) { 9881 this.getCommunicationRequest().add(castToReference(value)); 9882 } else if (name.equals("insurance")) { 9883 this.getInsurance().add((InsuranceComponent) value); 9884 } else if (name.equals("error")) { 9885 this.getError().add((ErrorComponent) value); 9886 } else 9887 return super.setProperty(name, value); 9888 return value; 9889 } 9890 9891 @Override 9892 public void removeChild(String name, Base value) throws FHIRException { 9893 if (name.equals("identifier")) { 9894 this.getIdentifier().remove(castToIdentifier(value)); 9895 } else if (name.equals("status")) { 9896 this.status = null; 9897 } else if (name.equals("type")) { 9898 this.type = null; 9899 } else if (name.equals("subType")) { 9900 this.subType = null; 9901 } else if (name.equals("use")) { 9902 this.use = null; 9903 } else if (name.equals("patient")) { 9904 this.patient = null; 9905 } else if (name.equals("created")) { 9906 this.created = null; 9907 } else if (name.equals("insurer")) { 9908 this.insurer = null; 9909 } else if (name.equals("requestor")) { 9910 this.requestor = null; 9911 } else if (name.equals("request")) { 9912 this.request = null; 9913 } else if (name.equals("outcome")) { 9914 this.outcome = null; 9915 } else if (name.equals("disposition")) { 9916 this.disposition = null; 9917 } else if (name.equals("preAuthRef")) { 9918 this.preAuthRef = null; 9919 } else if (name.equals("preAuthPeriod")) { 9920 this.preAuthPeriod = null; 9921 } else if (name.equals("payeeType")) { 9922 this.payeeType = null; 9923 } else if (name.equals("item")) { 9924 this.getItem().remove((ItemComponent) value); 9925 } else if (name.equals("addItem")) { 9926 this.getAddItem().remove((AddedItemComponent) value); 9927 } else if (name.equals("adjudication")) { 9928 this.getAdjudication().remove((AdjudicationComponent) value); 9929 } else if (name.equals("total")) { 9930 this.getTotal().remove((TotalComponent) value); 9931 } else if (name.equals("payment")) { 9932 this.payment = (PaymentComponent) value; // PaymentComponent 9933 } else if (name.equals("fundsReserve")) { 9934 this.fundsReserve = null; 9935 } else if (name.equals("formCode")) { 9936 this.formCode = null; 9937 } else if (name.equals("form")) { 9938 this.form = null; 9939 } else if (name.equals("processNote")) { 9940 this.getProcessNote().remove((NoteComponent) value); 9941 } else if (name.equals("communicationRequest")) { 9942 this.getCommunicationRequest().remove(castToReference(value)); 9943 } else if (name.equals("insurance")) { 9944 this.getInsurance().remove((InsuranceComponent) value); 9945 } else if (name.equals("error")) { 9946 this.getError().remove((ErrorComponent) value); 9947 } else 9948 super.removeChild(name, value); 9949 9950 } 9951 9952 @Override 9953 public Base makeProperty(int hash, String name) throws FHIRException { 9954 switch (hash) { 9955 case -1618432855: 9956 return addIdentifier(); 9957 case -892481550: 9958 return getStatusElement(); 9959 case 3575610: 9960 return getType(); 9961 case -1868521062: 9962 return getSubType(); 9963 case 116103: 9964 return getUseElement(); 9965 case -791418107: 9966 return getPatient(); 9967 case 1028554472: 9968 return getCreatedElement(); 9969 case 1957615864: 9970 return getInsurer(); 9971 case 693934258: 9972 return getRequestor(); 9973 case 1095692943: 9974 return getRequest(); 9975 case -1106507950: 9976 return getOutcomeElement(); 9977 case 583380919: 9978 return getDispositionElement(); 9979 case 522246568: 9980 return getPreAuthRefElement(); 9981 case 1819164812: 9982 return getPreAuthPeriod(); 9983 case -316321118: 9984 return getPayeeType(); 9985 case 3242771: 9986 return addItem(); 9987 case -1148899500: 9988 return addAddItem(); 9989 case -231349275: 9990 return addAdjudication(); 9991 case 110549828: 9992 return addTotal(); 9993 case -786681338: 9994 return getPayment(); 9995 case 1314609806: 9996 return getFundsReserve(); 9997 case 473181393: 9998 return getFormCode(); 9999 case 3148996: 10000 return getForm(); 10001 case 202339073: 10002 return addProcessNote(); 10003 case -2071896615: 10004 return addCommunicationRequest(); 10005 case 73049818: 10006 return addInsurance(); 10007 case 96784904: 10008 return addError(); 10009 default: 10010 return super.makeProperty(hash, name); 10011 } 10012 10013 } 10014 10015 @Override 10016 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10017 switch (hash) { 10018 case -1618432855: 10019 /* identifier */ return new String[] { "Identifier" }; 10020 case -892481550: 10021 /* status */ return new String[] { "code" }; 10022 case 3575610: 10023 /* type */ return new String[] { "CodeableConcept" }; 10024 case -1868521062: 10025 /* subType */ return new String[] { "CodeableConcept" }; 10026 case 116103: 10027 /* use */ return new String[] { "code" }; 10028 case -791418107: 10029 /* patient */ return new String[] { "Reference" }; 10030 case 1028554472: 10031 /* created */ return new String[] { "dateTime" }; 10032 case 1957615864: 10033 /* insurer */ return new String[] { "Reference" }; 10034 case 693934258: 10035 /* requestor */ return new String[] { "Reference" }; 10036 case 1095692943: 10037 /* request */ return new String[] { "Reference" }; 10038 case -1106507950: 10039 /* outcome */ return new String[] { "code" }; 10040 case 583380919: 10041 /* disposition */ return new String[] { "string" }; 10042 case 522246568: 10043 /* preAuthRef */ return new String[] { "string" }; 10044 case 1819164812: 10045 /* preAuthPeriod */ return new String[] { "Period" }; 10046 case -316321118: 10047 /* payeeType */ return new String[] { "CodeableConcept" }; 10048 case 3242771: 10049 /* item */ return new String[] {}; 10050 case -1148899500: 10051 /* addItem */ return new String[] {}; 10052 case -231349275: 10053 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 10054 case 110549828: 10055 /* total */ return new String[] {}; 10056 case -786681338: 10057 /* payment */ return new String[] {}; 10058 case 1314609806: 10059 /* fundsReserve */ return new String[] { "CodeableConcept" }; 10060 case 473181393: 10061 /* formCode */ return new String[] { "CodeableConcept" }; 10062 case 3148996: 10063 /* form */ return new String[] { "Attachment" }; 10064 case 202339073: 10065 /* processNote */ return new String[] {}; 10066 case -2071896615: 10067 /* communicationRequest */ return new String[] { "Reference" }; 10068 case 73049818: 10069 /* insurance */ return new String[] {}; 10070 case 96784904: 10071 /* error */ return new String[] {}; 10072 default: 10073 return super.getTypesForProperty(hash, name); 10074 } 10075 10076 } 10077 10078 @Override 10079 public Base addChild(String name) throws FHIRException { 10080 if (name.equals("identifier")) { 10081 return addIdentifier(); 10082 } else if (name.equals("status")) { 10083 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.status"); 10084 } else if (name.equals("type")) { 10085 this.type = new CodeableConcept(); 10086 return this.type; 10087 } else if (name.equals("subType")) { 10088 this.subType = new CodeableConcept(); 10089 return this.subType; 10090 } else if (name.equals("use")) { 10091 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.use"); 10092 } else if (name.equals("patient")) { 10093 this.patient = new Reference(); 10094 return this.patient; 10095 } else if (name.equals("created")) { 10096 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.created"); 10097 } else if (name.equals("insurer")) { 10098 this.insurer = new Reference(); 10099 return this.insurer; 10100 } else if (name.equals("requestor")) { 10101 this.requestor = new Reference(); 10102 return this.requestor; 10103 } else if (name.equals("request")) { 10104 this.request = new Reference(); 10105 return this.request; 10106 } else if (name.equals("outcome")) { 10107 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.outcome"); 10108 } else if (name.equals("disposition")) { 10109 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.disposition"); 10110 } else if (name.equals("preAuthRef")) { 10111 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.preAuthRef"); 10112 } else if (name.equals("preAuthPeriod")) { 10113 this.preAuthPeriod = new Period(); 10114 return this.preAuthPeriod; 10115 } else if (name.equals("payeeType")) { 10116 this.payeeType = new CodeableConcept(); 10117 return this.payeeType; 10118 } else if (name.equals("item")) { 10119 return addItem(); 10120 } else if (name.equals("addItem")) { 10121 return addAddItem(); 10122 } else if (name.equals("adjudication")) { 10123 return addAdjudication(); 10124 } else if (name.equals("total")) { 10125 return addTotal(); 10126 } else if (name.equals("payment")) { 10127 this.payment = new PaymentComponent(); 10128 return this.payment; 10129 } else if (name.equals("fundsReserve")) { 10130 this.fundsReserve = new CodeableConcept(); 10131 return this.fundsReserve; 10132 } else if (name.equals("formCode")) { 10133 this.formCode = new CodeableConcept(); 10134 return this.formCode; 10135 } else if (name.equals("form")) { 10136 this.form = new Attachment(); 10137 return this.form; 10138 } else if (name.equals("processNote")) { 10139 return addProcessNote(); 10140 } else if (name.equals("communicationRequest")) { 10141 return addCommunicationRequest(); 10142 } else if (name.equals("insurance")) { 10143 return addInsurance(); 10144 } else if (name.equals("error")) { 10145 return addError(); 10146 } else 10147 return super.addChild(name); 10148 } 10149 10150 public String fhirType() { 10151 return "ClaimResponse"; 10152 10153 } 10154 10155 public ClaimResponse copy() { 10156 ClaimResponse dst = new ClaimResponse(); 10157 copyValues(dst); 10158 return dst; 10159 } 10160 10161 public void copyValues(ClaimResponse dst) { 10162 super.copyValues(dst); 10163 if (identifier != null) { 10164 dst.identifier = new ArrayList<Identifier>(); 10165 for (Identifier i : identifier) 10166 dst.identifier.add(i.copy()); 10167 } 10168 ; 10169 dst.status = status == null ? null : status.copy(); 10170 dst.type = type == null ? null : type.copy(); 10171 dst.subType = subType == null ? null : subType.copy(); 10172 dst.use = use == null ? null : use.copy(); 10173 dst.patient = patient == null ? null : patient.copy(); 10174 dst.created = created == null ? null : created.copy(); 10175 dst.insurer = insurer == null ? null : insurer.copy(); 10176 dst.requestor = requestor == null ? null : requestor.copy(); 10177 dst.request = request == null ? null : request.copy(); 10178 dst.outcome = outcome == null ? null : outcome.copy(); 10179 dst.disposition = disposition == null ? null : disposition.copy(); 10180 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 10181 dst.preAuthPeriod = preAuthPeriod == null ? null : preAuthPeriod.copy(); 10182 dst.payeeType = payeeType == null ? null : payeeType.copy(); 10183 if (item != null) { 10184 dst.item = new ArrayList<ItemComponent>(); 10185 for (ItemComponent i : item) 10186 dst.item.add(i.copy()); 10187 } 10188 ; 10189 if (addItem != null) { 10190 dst.addItem = new ArrayList<AddedItemComponent>(); 10191 for (AddedItemComponent i : addItem) 10192 dst.addItem.add(i.copy()); 10193 } 10194 ; 10195 if (adjudication != null) { 10196 dst.adjudication = new ArrayList<AdjudicationComponent>(); 10197 for (AdjudicationComponent i : adjudication) 10198 dst.adjudication.add(i.copy()); 10199 } 10200 ; 10201 if (total != null) { 10202 dst.total = new ArrayList<TotalComponent>(); 10203 for (TotalComponent i : total) 10204 dst.total.add(i.copy()); 10205 } 10206 ; 10207 dst.payment = payment == null ? null : payment.copy(); 10208 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 10209 dst.formCode = formCode == null ? null : formCode.copy(); 10210 dst.form = form == null ? null : form.copy(); 10211 if (processNote != null) { 10212 dst.processNote = new ArrayList<NoteComponent>(); 10213 for (NoteComponent i : processNote) 10214 dst.processNote.add(i.copy()); 10215 } 10216 ; 10217 if (communicationRequest != null) { 10218 dst.communicationRequest = new ArrayList<Reference>(); 10219 for (Reference i : communicationRequest) 10220 dst.communicationRequest.add(i.copy()); 10221 } 10222 ; 10223 if (insurance != null) { 10224 dst.insurance = new ArrayList<InsuranceComponent>(); 10225 for (InsuranceComponent i : insurance) 10226 dst.insurance.add(i.copy()); 10227 } 10228 ; 10229 if (error != null) { 10230 dst.error = new ArrayList<ErrorComponent>(); 10231 for (ErrorComponent i : error) 10232 dst.error.add(i.copy()); 10233 } 10234 ; 10235 } 10236 10237 protected ClaimResponse typedCopy() { 10238 return copy(); 10239 } 10240 10241 @Override 10242 public boolean equalsDeep(Base other_) { 10243 if (!super.equalsDeep(other_)) 10244 return false; 10245 if (!(other_ instanceof ClaimResponse)) 10246 return false; 10247 ClaimResponse o = (ClaimResponse) other_; 10248 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 10249 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) 10250 && compareDeep(patient, o.patient, true) && compareDeep(created, o.created, true) 10251 && compareDeep(insurer, o.insurer, true) && compareDeep(requestor, o.requestor, true) 10252 && compareDeep(request, o.request, true) && compareDeep(outcome, o.outcome, true) 10253 && compareDeep(disposition, o.disposition, true) && compareDeep(preAuthRef, o.preAuthRef, true) 10254 && compareDeep(preAuthPeriod, o.preAuthPeriod, true) && compareDeep(payeeType, o.payeeType, true) 10255 && compareDeep(item, o.item, true) && compareDeep(addItem, o.addItem, true) 10256 && compareDeep(adjudication, o.adjudication, true) && compareDeep(total, o.total, true) 10257 && compareDeep(payment, o.payment, true) && compareDeep(fundsReserve, o.fundsReserve, true) 10258 && compareDeep(formCode, o.formCode, true) && compareDeep(form, o.form, true) 10259 && compareDeep(processNote, o.processNote, true) 10260 && compareDeep(communicationRequest, o.communicationRequest, true) && compareDeep(insurance, o.insurance, true) 10261 && compareDeep(error, o.error, true); 10262 } 10263 10264 @Override 10265 public boolean equalsShallow(Base other_) { 10266 if (!super.equalsShallow(other_)) 10267 return false; 10268 if (!(other_ instanceof ClaimResponse)) 10269 return false; 10270 ClaimResponse o = (ClaimResponse) other_; 10271 return compareValues(status, o.status, true) && compareValues(use, o.use, true) 10272 && compareValues(created, o.created, true) && compareValues(outcome, o.outcome, true) 10273 && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true); 10274 } 10275 10276 public boolean isEmpty() { 10277 return super.isEmpty() 10278 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, subType, use, patient, created, insurer, 10279 requestor, request, outcome, disposition, preAuthRef, preAuthPeriod, payeeType, item, addItem, adjudication, 10280 total, payment, fundsReserve, formCode, form, processNote, communicationRequest, insurance, error); 10281 } 10282 10283 @Override 10284 public ResourceType getResourceType() { 10285 return ResourceType.ClaimResponse; 10286 } 10287 10288 /** 10289 * Search parameter: <b>identifier</b> 10290 * <p> 10291 * Description: <b>The identity of the ClaimResponse</b><br> 10292 * Type: <b>token</b><br> 10293 * Path: <b>ClaimResponse.identifier</b><br> 10294 * </p> 10295 */ 10296 @SearchParamDefinition(name = "identifier", path = "ClaimResponse.identifier", description = "The identity of the ClaimResponse", type = "token") 10297 public static final String SP_IDENTIFIER = "identifier"; 10298 /** 10299 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10300 * <p> 10301 * Description: <b>The identity of the ClaimResponse</b><br> 10302 * Type: <b>token</b><br> 10303 * Path: <b>ClaimResponse.identifier</b><br> 10304 * </p> 10305 */ 10306 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10307 SP_IDENTIFIER); 10308 10309 /** 10310 * Search parameter: <b>request</b> 10311 * <p> 10312 * Description: <b>The claim reference</b><br> 10313 * Type: <b>reference</b><br> 10314 * Path: <b>ClaimResponse.request</b><br> 10315 * </p> 10316 */ 10317 @SearchParamDefinition(name = "request", path = "ClaimResponse.request", description = "The claim reference", type = "reference", target = { 10318 Claim.class }) 10319 public static final String SP_REQUEST = "request"; 10320 /** 10321 * <b>Fluent Client</b> search parameter constant for <b>request</b> 10322 * <p> 10323 * Description: <b>The claim reference</b><br> 10324 * Type: <b>reference</b><br> 10325 * Path: <b>ClaimResponse.request</b><br> 10326 * </p> 10327 */ 10328 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10329 SP_REQUEST); 10330 10331 /** 10332 * Constant for fluent queries to be used to add include statements. Specifies 10333 * the path value of "<b>ClaimResponse:request</b>". 10334 */ 10335 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 10336 "ClaimResponse:request").toLocked(); 10337 10338 /** 10339 * Search parameter: <b>disposition</b> 10340 * <p> 10341 * Description: <b>The contents of the disposition message</b><br> 10342 * Type: <b>string</b><br> 10343 * Path: <b>ClaimResponse.disposition</b><br> 10344 * </p> 10345 */ 10346 @SearchParamDefinition(name = "disposition", path = "ClaimResponse.disposition", description = "The contents of the disposition message", type = "string") 10347 public static final String SP_DISPOSITION = "disposition"; 10348 /** 10349 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 10350 * <p> 10351 * Description: <b>The contents of the disposition message</b><br> 10352 * Type: <b>string</b><br> 10353 * Path: <b>ClaimResponse.disposition</b><br> 10354 * </p> 10355 */ 10356 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam( 10357 SP_DISPOSITION); 10358 10359 /** 10360 * Search parameter: <b>insurer</b> 10361 * <p> 10362 * Description: <b>The organization which generated this resource</b><br> 10363 * Type: <b>reference</b><br> 10364 * Path: <b>ClaimResponse.insurer</b><br> 10365 * </p> 10366 */ 10367 @SearchParamDefinition(name = "insurer", path = "ClaimResponse.insurer", description = "The organization which generated this resource", type = "reference", target = { 10368 Organization.class }) 10369 public static final String SP_INSURER = "insurer"; 10370 /** 10371 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 10372 * <p> 10373 * Description: <b>The organization which generated this resource</b><br> 10374 * Type: <b>reference</b><br> 10375 * Path: <b>ClaimResponse.insurer</b><br> 10376 * </p> 10377 */ 10378 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10379 SP_INSURER); 10380 10381 /** 10382 * Constant for fluent queries to be used to add include statements. Specifies 10383 * the path value of "<b>ClaimResponse:insurer</b>". 10384 */ 10385 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include( 10386 "ClaimResponse:insurer").toLocked(); 10387 10388 /** 10389 * Search parameter: <b>created</b> 10390 * <p> 10391 * Description: <b>The creation date</b><br> 10392 * Type: <b>date</b><br> 10393 * Path: <b>ClaimResponse.created</b><br> 10394 * </p> 10395 */ 10396 @SearchParamDefinition(name = "created", path = "ClaimResponse.created", description = "The creation date", type = "date") 10397 public static final String SP_CREATED = "created"; 10398 /** 10399 * <b>Fluent Client</b> search parameter constant for <b>created</b> 10400 * <p> 10401 * Description: <b>The creation date</b><br> 10402 * Type: <b>date</b><br> 10403 * Path: <b>ClaimResponse.created</b><br> 10404 * </p> 10405 */ 10406 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 10407 SP_CREATED); 10408 10409 /** 10410 * Search parameter: <b>patient</b> 10411 * <p> 10412 * Description: <b>The subject of care</b><br> 10413 * Type: <b>reference</b><br> 10414 * Path: <b>ClaimResponse.patient</b><br> 10415 * </p> 10416 */ 10417 @SearchParamDefinition(name = "patient", path = "ClaimResponse.patient", description = "The subject of care", type = "reference", providesMembershipIn = { 10418 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 10419 public static final String SP_PATIENT = "patient"; 10420 /** 10421 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 10422 * <p> 10423 * Description: <b>The subject of care</b><br> 10424 * Type: <b>reference</b><br> 10425 * Path: <b>ClaimResponse.patient</b><br> 10426 * </p> 10427 */ 10428 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10429 SP_PATIENT); 10430 10431 /** 10432 * Constant for fluent queries to be used to add include statements. Specifies 10433 * the path value of "<b>ClaimResponse:patient</b>". 10434 */ 10435 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 10436 "ClaimResponse:patient").toLocked(); 10437 10438 /** 10439 * Search parameter: <b>use</b> 10440 * <p> 10441 * Description: <b>The type of claim</b><br> 10442 * Type: <b>token</b><br> 10443 * Path: <b>ClaimResponse.use</b><br> 10444 * </p> 10445 */ 10446 @SearchParamDefinition(name = "use", path = "ClaimResponse.use", description = "The type of claim", type = "token") 10447 public static final String SP_USE = "use"; 10448 /** 10449 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10450 * <p> 10451 * Description: <b>The type of claim</b><br> 10452 * Type: <b>token</b><br> 10453 * Path: <b>ClaimResponse.use</b><br> 10454 * </p> 10455 */ 10456 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10457 SP_USE); 10458 10459 /** 10460 * Search parameter: <b>payment-date</b> 10461 * <p> 10462 * Description: <b>The expected payment date</b><br> 10463 * Type: <b>date</b><br> 10464 * Path: <b>ClaimResponse.payment.date</b><br> 10465 * </p> 10466 */ 10467 @SearchParamDefinition(name = "payment-date", path = "ClaimResponse.payment.date", description = "The expected payment date", type = "date") 10468 public static final String SP_PAYMENT_DATE = "payment-date"; 10469 /** 10470 * <b>Fluent Client</b> search parameter constant for <b>payment-date</b> 10471 * <p> 10472 * Description: <b>The expected payment date</b><br> 10473 * Type: <b>date</b><br> 10474 * Path: <b>ClaimResponse.payment.date</b><br> 10475 * </p> 10476 */ 10477 public static final ca.uhn.fhir.rest.gclient.DateClientParam PAYMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 10478 SP_PAYMENT_DATE); 10479 10480 /** 10481 * Search parameter: <b>outcome</b> 10482 * <p> 10483 * Description: <b>The processing outcome</b><br> 10484 * Type: <b>token</b><br> 10485 * Path: <b>ClaimResponse.outcome</b><br> 10486 * </p> 10487 */ 10488 @SearchParamDefinition(name = "outcome", path = "ClaimResponse.outcome", description = "The processing outcome", type = "token") 10489 public static final String SP_OUTCOME = "outcome"; 10490 /** 10491 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 10492 * <p> 10493 * Description: <b>The processing outcome</b><br> 10494 * Type: <b>token</b><br> 10495 * Path: <b>ClaimResponse.outcome</b><br> 10496 * </p> 10497 */ 10498 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10499 SP_OUTCOME); 10500 10501 /** 10502 * Search parameter: <b>requestor</b> 10503 * <p> 10504 * Description: <b>The Provider of the claim</b><br> 10505 * Type: <b>reference</b><br> 10506 * Path: <b>ClaimResponse.requestor</b><br> 10507 * </p> 10508 */ 10509 @SearchParamDefinition(name = "requestor", path = "ClaimResponse.requestor", description = "The Provider of the claim", type = "reference", providesMembershipIn = { 10510 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 10511 Practitioner.class, PractitionerRole.class }) 10512 public static final String SP_REQUESTOR = "requestor"; 10513 /** 10514 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 10515 * <p> 10516 * Description: <b>The Provider of the claim</b><br> 10517 * Type: <b>reference</b><br> 10518 * Path: <b>ClaimResponse.requestor</b><br> 10519 * </p> 10520 */ 10521 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10522 SP_REQUESTOR); 10523 10524 /** 10525 * Constant for fluent queries to be used to add include statements. Specifies 10526 * the path value of "<b>ClaimResponse:requestor</b>". 10527 */ 10528 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include( 10529 "ClaimResponse:requestor").toLocked(); 10530 10531 /** 10532 * Search parameter: <b>status</b> 10533 * <p> 10534 * Description: <b>The status of the ClaimResponse</b><br> 10535 * Type: <b>token</b><br> 10536 * Path: <b>ClaimResponse.status</b><br> 10537 * </p> 10538 */ 10539 @SearchParamDefinition(name = "status", path = "ClaimResponse.status", description = "The status of the ClaimResponse", type = "token") 10540 public static final String SP_STATUS = "status"; 10541 /** 10542 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10543 * <p> 10544 * Description: <b>The status of the ClaimResponse</b><br> 10545 * Type: <b>token</b><br> 10546 * Path: <b>ClaimResponse.status</b><br> 10547 * </p> 10548 */ 10549 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10550 SP_STATUS); 10551 10552}