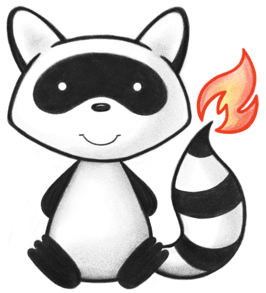
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.NoteType; 042import org.hl7.fhir.r4.model.Enumerations.NoteTypeEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050 051/** 052 * This resource provides the adjudication details from the processing of a 053 * Claim resource. 054 */ 055@ResourceDef(name = "ClaimResponse", profile = "http://hl7.org/fhir/StructureDefinition/ClaimResponse") 056public class ClaimResponse extends DomainResource { 057 058 public enum ClaimResponseStatus { 059 /** 060 * The instance is currently in-force. 061 */ 062 ACTIVE, 063 /** 064 * The instance is withdrawn, rescinded or reversed. 065 */ 066 CANCELLED, 067 /** 068 * A new instance the contents of which is not complete. 069 */ 070 DRAFT, 071 /** 072 * The instance was entered in error. 073 */ 074 ENTEREDINERROR, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static ClaimResponseStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("cancelled".equals(codeString)) 086 return CANCELLED; 087 if ("draft".equals(codeString)) 088 return DRAFT; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown ClaimResponseStatus code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case ACTIVE: 100 return "active"; 101 case CANCELLED: 102 return "cancelled"; 103 case DRAFT: 104 return "draft"; 105 case ENTEREDINERROR: 106 return "entered-in-error"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getSystem() { 115 switch (this) { 116 case ACTIVE: 117 return "http://hl7.org/fhir/fm-status"; 118 case CANCELLED: 119 return "http://hl7.org/fhir/fm-status"; 120 case DRAFT: 121 return "http://hl7.org/fhir/fm-status"; 122 case ENTEREDINERROR: 123 return "http://hl7.org/fhir/fm-status"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDefinition() { 132 switch (this) { 133 case ACTIVE: 134 return "The instance is currently in-force."; 135 case CANCELLED: 136 return "The instance is withdrawn, rescinded or reversed."; 137 case DRAFT: 138 return "A new instance the contents of which is not complete."; 139 case ENTEREDINERROR: 140 return "The instance was entered in error."; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getDisplay() { 149 switch (this) { 150 case ACTIVE: 151 return "Active"; 152 case CANCELLED: 153 return "Cancelled"; 154 case DRAFT: 155 return "Draft"; 156 case ENTEREDINERROR: 157 return "Entered in Error"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 } 165 166 public static class ClaimResponseStatusEnumFactory implements EnumFactory<ClaimResponseStatus> { 167 public ClaimResponseStatus fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("active".equals(codeString)) 172 return ClaimResponseStatus.ACTIVE; 173 if ("cancelled".equals(codeString)) 174 return ClaimResponseStatus.CANCELLED; 175 if ("draft".equals(codeString)) 176 return ClaimResponseStatus.DRAFT; 177 if ("entered-in-error".equals(codeString)) 178 return ClaimResponseStatus.ENTEREDINERROR; 179 throw new IllegalArgumentException("Unknown ClaimResponseStatus code '" + codeString + "'"); 180 } 181 182 public Enumeration<ClaimResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 183 if (code == null) 184 return null; 185 if (code.isEmpty()) 186 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.NULL, code); 187 String codeString = code.asStringValue(); 188 if (codeString == null || "".equals(codeString)) 189 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.NULL, code); 190 if ("active".equals(codeString)) 191 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.ACTIVE, code); 192 if ("cancelled".equals(codeString)) 193 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.CANCELLED, code); 194 if ("draft".equals(codeString)) 195 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.DRAFT, code); 196 if ("entered-in-error".equals(codeString)) 197 return new Enumeration<ClaimResponseStatus>(this, ClaimResponseStatus.ENTEREDINERROR, code); 198 throw new FHIRException("Unknown ClaimResponseStatus code '" + codeString + "'"); 199 } 200 201 public String toCode(ClaimResponseStatus code) { 202 if (code == ClaimResponseStatus.NULL) 203 return null; 204 if (code == ClaimResponseStatus.ACTIVE) 205 return "active"; 206 if (code == ClaimResponseStatus.CANCELLED) 207 return "cancelled"; 208 if (code == ClaimResponseStatus.DRAFT) 209 return "draft"; 210 if (code == ClaimResponseStatus.ENTEREDINERROR) 211 return "entered-in-error"; 212 return "?"; 213 } 214 215 public String toSystem(ClaimResponseStatus code) { 216 return code.getSystem(); 217 } 218 } 219 220 public enum Use { 221 /** 222 * The treatment is complete and this represents a Claim for the services. 223 */ 224 CLAIM, 225 /** 226 * The treatment is proposed and this represents a Pre-authorization for the 227 * services. 228 */ 229 PREAUTHORIZATION, 230 /** 231 * The treatment is proposed and this represents a Pre-determination for the 232 * services. 233 */ 234 PREDETERMINATION, 235 /** 236 * added to help the parsers with the generic types 237 */ 238 NULL; 239 240 public static Use fromCode(String codeString) throws FHIRException { 241 if (codeString == null || "".equals(codeString)) 242 return null; 243 if ("claim".equals(codeString)) 244 return CLAIM; 245 if ("preauthorization".equals(codeString)) 246 return PREAUTHORIZATION; 247 if ("predetermination".equals(codeString)) 248 return PREDETERMINATION; 249 if (Configuration.isAcceptInvalidEnums()) 250 return null; 251 else 252 throw new FHIRException("Unknown Use code '" + codeString + "'"); 253 } 254 255 public String toCode() { 256 switch (this) { 257 case CLAIM: 258 return "claim"; 259 case PREAUTHORIZATION: 260 return "preauthorization"; 261 case PREDETERMINATION: 262 return "predetermination"; 263 case NULL: 264 return null; 265 default: 266 return "?"; 267 } 268 } 269 270 public String getSystem() { 271 switch (this) { 272 case CLAIM: 273 return "http://hl7.org/fhir/claim-use"; 274 case PREAUTHORIZATION: 275 return "http://hl7.org/fhir/claim-use"; 276 case PREDETERMINATION: 277 return "http://hl7.org/fhir/claim-use"; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getDefinition() { 286 switch (this) { 287 case CLAIM: 288 return "The treatment is complete and this represents a Claim for the services."; 289 case PREAUTHORIZATION: 290 return "The treatment is proposed and this represents a Pre-authorization for the services."; 291 case PREDETERMINATION: 292 return "The treatment is proposed and this represents a Pre-determination for the services."; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300 public String getDisplay() { 301 switch (this) { 302 case CLAIM: 303 return "Claim"; 304 case PREAUTHORIZATION: 305 return "Preauthorization"; 306 case PREDETERMINATION: 307 return "Predetermination"; 308 case NULL: 309 return null; 310 default: 311 return "?"; 312 } 313 } 314 } 315 316 public static class UseEnumFactory implements EnumFactory<Use> { 317 public Use fromCode(String codeString) throws IllegalArgumentException { 318 if (codeString == null || "".equals(codeString)) 319 if (codeString == null || "".equals(codeString)) 320 return null; 321 if ("claim".equals(codeString)) 322 return Use.CLAIM; 323 if ("preauthorization".equals(codeString)) 324 return Use.PREAUTHORIZATION; 325 if ("predetermination".equals(codeString)) 326 return Use.PREDETERMINATION; 327 throw new IllegalArgumentException("Unknown Use code '" + codeString + "'"); 328 } 329 330 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 331 if (code == null) 332 return null; 333 if (code.isEmpty()) 334 return new Enumeration<Use>(this, Use.NULL, code); 335 String codeString = code.asStringValue(); 336 if (codeString == null || "".equals(codeString)) 337 return new Enumeration<Use>(this, Use.NULL, code); 338 if ("claim".equals(codeString)) 339 return new Enumeration<Use>(this, Use.CLAIM, code); 340 if ("preauthorization".equals(codeString)) 341 return new Enumeration<Use>(this, Use.PREAUTHORIZATION, code); 342 if ("predetermination".equals(codeString)) 343 return new Enumeration<Use>(this, Use.PREDETERMINATION, code); 344 throw new FHIRException("Unknown Use code '" + codeString + "'"); 345 } 346 347 public String toCode(Use code) { 348 if (code == Use.NULL) 349 return null; 350 if (code == Use.CLAIM) 351 return "claim"; 352 if (code == Use.PREAUTHORIZATION) 353 return "preauthorization"; 354 if (code == Use.PREDETERMINATION) 355 return "predetermination"; 356 return "?"; 357 } 358 359 public String toSystem(Use code) { 360 return code.getSystem(); 361 } 362 } 363 364 public enum RemittanceOutcome { 365 /** 366 * The Claim/Pre-authorization/Pre-determination has been received but 367 * processing has not begun. 368 */ 369 QUEUED, 370 /** 371 * The processing has completed without errors 372 */ 373 COMPLETE, 374 /** 375 * One or more errors have been detected in the Claim 376 */ 377 ERROR, 378 /** 379 * No errors have been detected in the Claim and some of the adjudication has 380 * been performed. 381 */ 382 PARTIAL, 383 /** 384 * added to help the parsers with the generic types 385 */ 386 NULL; 387 388 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 389 if (codeString == null || "".equals(codeString)) 390 return null; 391 if ("queued".equals(codeString)) 392 return QUEUED; 393 if ("complete".equals(codeString)) 394 return COMPLETE; 395 if ("error".equals(codeString)) 396 return ERROR; 397 if ("partial".equals(codeString)) 398 return PARTIAL; 399 if (Configuration.isAcceptInvalidEnums()) 400 return null; 401 else 402 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 403 } 404 405 public String toCode() { 406 switch (this) { 407 case QUEUED: 408 return "queued"; 409 case COMPLETE: 410 return "complete"; 411 case ERROR: 412 return "error"; 413 case PARTIAL: 414 return "partial"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getSystem() { 423 switch (this) { 424 case QUEUED: 425 return "http://hl7.org/fhir/remittance-outcome"; 426 case COMPLETE: 427 return "http://hl7.org/fhir/remittance-outcome"; 428 case ERROR: 429 return "http://hl7.org/fhir/remittance-outcome"; 430 case PARTIAL: 431 return "http://hl7.org/fhir/remittance-outcome"; 432 case NULL: 433 return null; 434 default: 435 return "?"; 436 } 437 } 438 439 public String getDefinition() { 440 switch (this) { 441 case QUEUED: 442 return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 443 case COMPLETE: 444 return "The processing has completed without errors"; 445 case ERROR: 446 return "One or more errors have been detected in the Claim"; 447 case PARTIAL: 448 return "No errors have been detected in the Claim and some of the adjudication has been performed."; 449 case NULL: 450 return null; 451 default: 452 return "?"; 453 } 454 } 455 456 public String getDisplay() { 457 switch (this) { 458 case QUEUED: 459 return "Queued"; 460 case COMPLETE: 461 return "Processing Complete"; 462 case ERROR: 463 return "Error"; 464 case PARTIAL: 465 return "Partial Processing"; 466 case NULL: 467 return null; 468 default: 469 return "?"; 470 } 471 } 472 } 473 474 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 475 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 476 if (codeString == null || "".equals(codeString)) 477 if (codeString == null || "".equals(codeString)) 478 return null; 479 if ("queued".equals(codeString)) 480 return RemittanceOutcome.QUEUED; 481 if ("complete".equals(codeString)) 482 return RemittanceOutcome.COMPLETE; 483 if ("error".equals(codeString)) 484 return RemittanceOutcome.ERROR; 485 if ("partial".equals(codeString)) 486 return RemittanceOutcome.PARTIAL; 487 throw new IllegalArgumentException("Unknown RemittanceOutcome code '" + codeString + "'"); 488 } 489 490 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 491 if (code == null) 492 return null; 493 if (code.isEmpty()) 494 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 495 String codeString = code.asStringValue(); 496 if (codeString == null || "".equals(codeString)) 497 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 498 if ("queued".equals(codeString)) 499 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.QUEUED, code); 500 if ("complete".equals(codeString)) 501 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE, code); 502 if ("error".equals(codeString)) 503 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR, code); 504 if ("partial".equals(codeString)) 505 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL, code); 506 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 507 } 508 509 public String toCode(RemittanceOutcome code) { 510 if (code == RemittanceOutcome.NULL) 511 return null; 512 if (code == RemittanceOutcome.QUEUED) 513 return "queued"; 514 if (code == RemittanceOutcome.COMPLETE) 515 return "complete"; 516 if (code == RemittanceOutcome.ERROR) 517 return "error"; 518 if (code == RemittanceOutcome.PARTIAL) 519 return "partial"; 520 return "?"; 521 } 522 523 public String toSystem(RemittanceOutcome code) { 524 return code.getSystem(); 525 } 526 } 527 528 @Block() 529 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 530 /** 531 * A number to uniquely reference the claim item entries. 532 */ 533 @Child(name = "itemSequence", type = { 534 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 535 @Description(shortDefinition = "Claim item instance identifier", formalDefinition = "A number to uniquely reference the claim item entries.") 536 protected PositiveIntType itemSequence; 537 538 /** 539 * The numbers associated with notes below which apply to the adjudication of 540 * this item. 541 */ 542 @Child(name = "noteNumber", type = { 543 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 544 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 545 protected List<PositiveIntType> noteNumber; 546 547 /** 548 * If this item is a group then the values here are a summary of the 549 * adjudication of the detail items. If this item is a simple product or service 550 * then this is the result of the adjudication of this item. 551 */ 552 @Child(name = "adjudication", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 553 @Description(shortDefinition = "Adjudication details", formalDefinition = "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.") 554 protected List<AdjudicationComponent> adjudication; 555 556 /** 557 * A claim detail. Either a simple (a product or service) or a 'group' of 558 * sub-details which are simple items. 559 */ 560 @Child(name = "detail", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 561 @Description(shortDefinition = "Adjudication for claim details", formalDefinition = "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 562 protected List<ItemDetailComponent> detail; 563 564 private static final long serialVersionUID = 701277928L; 565 566 /** 567 * Constructor 568 */ 569 public ItemComponent() { 570 super(); 571 } 572 573 /** 574 * Constructor 575 */ 576 public ItemComponent(PositiveIntType itemSequence) { 577 super(); 578 this.itemSequence = itemSequence; 579 } 580 581 /** 582 * @return {@link #itemSequence} (A number to uniquely reference the claim item 583 * entries.). This is the underlying object with id, value and 584 * extensions. The accessor "getItemSequence" gives direct access to the 585 * value 586 */ 587 public PositiveIntType getItemSequenceElement() { 588 if (this.itemSequence == null) 589 if (Configuration.errorOnAutoCreate()) 590 throw new Error("Attempt to auto-create ItemComponent.itemSequence"); 591 else if (Configuration.doAutoCreate()) 592 this.itemSequence = new PositiveIntType(); // bb 593 return this.itemSequence; 594 } 595 596 public boolean hasItemSequenceElement() { 597 return this.itemSequence != null && !this.itemSequence.isEmpty(); 598 } 599 600 public boolean hasItemSequence() { 601 return this.itemSequence != null && !this.itemSequence.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #itemSequence} (A number to uniquely reference the claim 606 * item entries.). This is the underlying object with id, value and 607 * extensions. The accessor "getItemSequence" gives direct access 608 * to the value 609 */ 610 public ItemComponent setItemSequenceElement(PositiveIntType value) { 611 this.itemSequence = value; 612 return this; 613 } 614 615 /** 616 * @return A number to uniquely reference the claim item entries. 617 */ 618 public int getItemSequence() { 619 return this.itemSequence == null || this.itemSequence.isEmpty() ? 0 : this.itemSequence.getValue(); 620 } 621 622 /** 623 * @param value A number to uniquely reference the claim item entries. 624 */ 625 public ItemComponent setItemSequence(int value) { 626 if (this.itemSequence == null) 627 this.itemSequence = new PositiveIntType(); 628 this.itemSequence.setValue(value); 629 return this; 630 } 631 632 /** 633 * @return {@link #noteNumber} (The numbers associated with notes below which 634 * apply to the adjudication of this item.) 635 */ 636 public List<PositiveIntType> getNoteNumber() { 637 if (this.noteNumber == null) 638 this.noteNumber = new ArrayList<PositiveIntType>(); 639 return this.noteNumber; 640 } 641 642 /** 643 * @return Returns a reference to <code>this</code> for easy method chaining 644 */ 645 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 646 this.noteNumber = theNoteNumber; 647 return this; 648 } 649 650 public boolean hasNoteNumber() { 651 if (this.noteNumber == null) 652 return false; 653 for (PositiveIntType item : this.noteNumber) 654 if (!item.isEmpty()) 655 return true; 656 return false; 657 } 658 659 /** 660 * @return {@link #noteNumber} (The numbers associated with notes below which 661 * apply to the adjudication of this item.) 662 */ 663 public PositiveIntType addNoteNumberElement() {// 2 664 PositiveIntType t = new PositiveIntType(); 665 if (this.noteNumber == null) 666 this.noteNumber = new ArrayList<PositiveIntType>(); 667 this.noteNumber.add(t); 668 return t; 669 } 670 671 /** 672 * @param value {@link #noteNumber} (The numbers associated with notes below 673 * which apply to the adjudication of this item.) 674 */ 675 public ItemComponent addNoteNumber(int value) { // 1 676 PositiveIntType t = new PositiveIntType(); 677 t.setValue(value); 678 if (this.noteNumber == null) 679 this.noteNumber = new ArrayList<PositiveIntType>(); 680 this.noteNumber.add(t); 681 return this; 682 } 683 684 /** 685 * @param value {@link #noteNumber} (The numbers associated with notes below 686 * which apply to the adjudication of this item.) 687 */ 688 public boolean hasNoteNumber(int value) { 689 if (this.noteNumber == null) 690 return false; 691 for (PositiveIntType v : this.noteNumber) 692 if (v.getValue().equals(value)) // positiveInt 693 return true; 694 return false; 695 } 696 697 /** 698 * @return {@link #adjudication} (If this item is a group then the values here 699 * are a summary of the adjudication of the detail items. If this item 700 * is a simple product or service then this is the result of the 701 * adjudication of this item.) 702 */ 703 public List<AdjudicationComponent> getAdjudication() { 704 if (this.adjudication == null) 705 this.adjudication = new ArrayList<AdjudicationComponent>(); 706 return this.adjudication; 707 } 708 709 /** 710 * @return Returns a reference to <code>this</code> for easy method chaining 711 */ 712 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 713 this.adjudication = theAdjudication; 714 return this; 715 } 716 717 public boolean hasAdjudication() { 718 if (this.adjudication == null) 719 return false; 720 for (AdjudicationComponent item : this.adjudication) 721 if (!item.isEmpty()) 722 return true; 723 return false; 724 } 725 726 public AdjudicationComponent addAdjudication() { // 3 727 AdjudicationComponent t = new AdjudicationComponent(); 728 if (this.adjudication == null) 729 this.adjudication = new ArrayList<AdjudicationComponent>(); 730 this.adjudication.add(t); 731 return t; 732 } 733 734 public ItemComponent addAdjudication(AdjudicationComponent t) { // 3 735 if (t == null) 736 return this; 737 if (this.adjudication == null) 738 this.adjudication = new ArrayList<AdjudicationComponent>(); 739 this.adjudication.add(t); 740 return this; 741 } 742 743 /** 744 * @return The first repetition of repeating field {@link #adjudication}, 745 * creating it if it does not already exist 746 */ 747 public AdjudicationComponent getAdjudicationFirstRep() { 748 if (getAdjudication().isEmpty()) { 749 addAdjudication(); 750 } 751 return getAdjudication().get(0); 752 } 753 754 /** 755 * @return {@link #detail} (A claim detail. Either a simple (a product or 756 * service) or a 'group' of sub-details which are simple items.) 757 */ 758 public List<ItemDetailComponent> getDetail() { 759 if (this.detail == null) 760 this.detail = new ArrayList<ItemDetailComponent>(); 761 return this.detail; 762 } 763 764 /** 765 * @return Returns a reference to <code>this</code> for easy method chaining 766 */ 767 public ItemComponent setDetail(List<ItemDetailComponent> theDetail) { 768 this.detail = theDetail; 769 return this; 770 } 771 772 public boolean hasDetail() { 773 if (this.detail == null) 774 return false; 775 for (ItemDetailComponent item : this.detail) 776 if (!item.isEmpty()) 777 return true; 778 return false; 779 } 780 781 public ItemDetailComponent addDetail() { // 3 782 ItemDetailComponent t = new ItemDetailComponent(); 783 if (this.detail == null) 784 this.detail = new ArrayList<ItemDetailComponent>(); 785 this.detail.add(t); 786 return t; 787 } 788 789 public ItemComponent addDetail(ItemDetailComponent t) { // 3 790 if (t == null) 791 return this; 792 if (this.detail == null) 793 this.detail = new ArrayList<ItemDetailComponent>(); 794 this.detail.add(t); 795 return this; 796 } 797 798 /** 799 * @return The first repetition of repeating field {@link #detail}, creating it 800 * if it does not already exist 801 */ 802 public ItemDetailComponent getDetailFirstRep() { 803 if (getDetail().isEmpty()) { 804 addDetail(); 805 } 806 return getDetail().get(0); 807 } 808 809 protected void listChildren(List<Property> children) { 810 super.listChildren(children); 811 children.add(new Property("itemSequence", "positiveInt", "A number to uniquely reference the claim item entries.", 812 0, 1, itemSequence)); 813 children.add(new Property("noteNumber", "positiveInt", 814 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 815 java.lang.Integer.MAX_VALUE, noteNumber)); 816 children.add(new Property("adjudication", "", 817 "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 818 0, java.lang.Integer.MAX_VALUE, adjudication)); 819 children.add(new Property("detail", "", 820 "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 821 0, java.lang.Integer.MAX_VALUE, detail)); 822 } 823 824 @Override 825 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 826 switch (_hash) { 827 case 1977979892: 828 /* itemSequence */ return new Property("itemSequence", "positiveInt", 829 "A number to uniquely reference the claim item entries.", 0, 1, itemSequence); 830 case -1110033957: 831 /* noteNumber */ return new Property("noteNumber", "positiveInt", 832 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 833 java.lang.Integer.MAX_VALUE, noteNumber); 834 case -231349275: 835 /* adjudication */ return new Property("adjudication", "", 836 "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 837 0, java.lang.Integer.MAX_VALUE, adjudication); 838 case -1335224239: 839 /* detail */ return new Property("detail", "", 840 "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 841 0, java.lang.Integer.MAX_VALUE, detail); 842 default: 843 return super.getNamedProperty(_hash, _name, _checkValid); 844 } 845 846 } 847 848 @Override 849 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 850 switch (hash) { 851 case 1977979892: 852 /* itemSequence */ return this.itemSequence == null ? new Base[0] : new Base[] { this.itemSequence }; // PositiveIntType 853 case -1110033957: 854 /* noteNumber */ return this.noteNumber == null ? new Base[0] 855 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 856 case -231349275: 857 /* adjudication */ return this.adjudication == null ? new Base[0] 858 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 859 case -1335224239: 860 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // ItemDetailComponent 861 default: 862 return super.getProperty(hash, name, checkValid); 863 } 864 865 } 866 867 @Override 868 public Base setProperty(int hash, String name, Base value) throws FHIRException { 869 switch (hash) { 870 case 1977979892: // itemSequence 871 this.itemSequence = castToPositiveInt(value); // PositiveIntType 872 return value; 873 case -1110033957: // noteNumber 874 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 875 return value; 876 case -231349275: // adjudication 877 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 878 return value; 879 case -1335224239: // detail 880 this.getDetail().add((ItemDetailComponent) value); // ItemDetailComponent 881 return value; 882 default: 883 return super.setProperty(hash, name, value); 884 } 885 886 } 887 888 @Override 889 public Base setProperty(String name, Base value) throws FHIRException { 890 if (name.equals("itemSequence")) { 891 this.itemSequence = castToPositiveInt(value); // PositiveIntType 892 } else if (name.equals("noteNumber")) { 893 this.getNoteNumber().add(castToPositiveInt(value)); 894 } else if (name.equals("adjudication")) { 895 this.getAdjudication().add((AdjudicationComponent) value); 896 } else if (name.equals("detail")) { 897 this.getDetail().add((ItemDetailComponent) value); 898 } else 899 return super.setProperty(name, value); 900 return value; 901 } 902 903 @Override 904 public void removeChild(String name, Base value) throws FHIRException { 905 if (name.equals("itemSequence")) { 906 this.itemSequence = null; 907 } else if (name.equals("noteNumber")) { 908 this.getNoteNumber().remove(castToPositiveInt(value)); 909 } else if (name.equals("adjudication")) { 910 this.getAdjudication().remove((AdjudicationComponent) value); 911 } else if (name.equals("detail")) { 912 this.getDetail().remove((ItemDetailComponent) value); 913 } else 914 super.removeChild(name, value); 915 916 } 917 918 @Override 919 public Base makeProperty(int hash, String name) throws FHIRException { 920 switch (hash) { 921 case 1977979892: 922 return getItemSequenceElement(); 923 case -1110033957: 924 return addNoteNumberElement(); 925 case -231349275: 926 return addAdjudication(); 927 case -1335224239: 928 return addDetail(); 929 default: 930 return super.makeProperty(hash, name); 931 } 932 933 } 934 935 @Override 936 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 937 switch (hash) { 938 case 1977979892: 939 /* itemSequence */ return new String[] { "positiveInt" }; 940 case -1110033957: 941 /* noteNumber */ return new String[] { "positiveInt" }; 942 case -231349275: 943 /* adjudication */ return new String[] {}; 944 case -1335224239: 945 /* detail */ return new String[] {}; 946 default: 947 return super.getTypesForProperty(hash, name); 948 } 949 950 } 951 952 @Override 953 public Base addChild(String name) throws FHIRException { 954 if (name.equals("itemSequence")) { 955 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.itemSequence"); 956 } else if (name.equals("noteNumber")) { 957 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 958 } else if (name.equals("adjudication")) { 959 return addAdjudication(); 960 } else if (name.equals("detail")) { 961 return addDetail(); 962 } else 963 return super.addChild(name); 964 } 965 966 public ItemComponent copy() { 967 ItemComponent dst = new ItemComponent(); 968 copyValues(dst); 969 return dst; 970 } 971 972 public void copyValues(ItemComponent dst) { 973 super.copyValues(dst); 974 dst.itemSequence = itemSequence == null ? null : itemSequence.copy(); 975 if (noteNumber != null) { 976 dst.noteNumber = new ArrayList<PositiveIntType>(); 977 for (PositiveIntType i : noteNumber) 978 dst.noteNumber.add(i.copy()); 979 } 980 ; 981 if (adjudication != null) { 982 dst.adjudication = new ArrayList<AdjudicationComponent>(); 983 for (AdjudicationComponent i : adjudication) 984 dst.adjudication.add(i.copy()); 985 } 986 ; 987 if (detail != null) { 988 dst.detail = new ArrayList<ItemDetailComponent>(); 989 for (ItemDetailComponent i : detail) 990 dst.detail.add(i.copy()); 991 } 992 ; 993 } 994 995 @Override 996 public boolean equalsDeep(Base other_) { 997 if (!super.equalsDeep(other_)) 998 return false; 999 if (!(other_ instanceof ItemComponent)) 1000 return false; 1001 ItemComponent o = (ItemComponent) other_; 1002 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(noteNumber, o.noteNumber, true) 1003 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 1004 } 1005 1006 @Override 1007 public boolean equalsShallow(Base other_) { 1008 if (!super.equalsShallow(other_)) 1009 return false; 1010 if (!(other_ instanceof ItemComponent)) 1011 return false; 1012 ItemComponent o = (ItemComponent) other_; 1013 return compareValues(itemSequence, o.itemSequence, true) && compareValues(noteNumber, o.noteNumber, true); 1014 } 1015 1016 public boolean isEmpty() { 1017 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, noteNumber, adjudication, detail); 1018 } 1019 1020 public String fhirType() { 1021 return "ClaimResponse.item"; 1022 1023 } 1024 1025 } 1026 1027 @Block() 1028 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 1029 /** 1030 * A code to indicate the information type of this adjudication record. 1031 * Information types may include the value submitted, maximum values or 1032 * percentages allowed or payable under the plan, amounts that: the patient is 1033 * responsible for in aggregate or pertaining to this item; amounts paid by 1034 * other coverages; and, the benefit payable for this item. 1035 */ 1036 @Child(name = "category", type = { 1037 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1038 @Description(shortDefinition = "Type of adjudication information", formalDefinition = "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.") 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication") 1040 protected CodeableConcept category; 1041 1042 /** 1043 * A code supporting the understanding of the adjudication result and explaining 1044 * variance from expected amount. 1045 */ 1046 @Child(name = "reason", type = { 1047 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1048 @Description(shortDefinition = "Explanation of adjudication outcome", formalDefinition = "A code supporting the understanding of the adjudication result and explaining variance from expected amount.") 1049 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication-reason") 1050 protected CodeableConcept reason; 1051 1052 /** 1053 * Monetary amount associated with the category. 1054 */ 1055 @Child(name = "amount", type = { Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1056 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the category.") 1057 protected Money amount; 1058 1059 /** 1060 * A non-monetary value associated with the category. Mutually exclusive to the 1061 * amount element above. 1062 */ 1063 @Child(name = "value", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1064 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value associated with the category. Mutually exclusive to the amount element above.") 1065 protected DecimalType value; 1066 1067 private static final long serialVersionUID = 1559898786L; 1068 1069 /** 1070 * Constructor 1071 */ 1072 public AdjudicationComponent() { 1073 super(); 1074 } 1075 1076 /** 1077 * Constructor 1078 */ 1079 public AdjudicationComponent(CodeableConcept category) { 1080 super(); 1081 this.category = category; 1082 } 1083 1084 /** 1085 * @return {@link #category} (A code to indicate the information type of this 1086 * adjudication record. Information types may include the value 1087 * submitted, maximum values or percentages allowed or payable under the 1088 * plan, amounts that: the patient is responsible for in aggregate or 1089 * pertaining to this item; amounts paid by other coverages; and, the 1090 * benefit payable for this item.) 1091 */ 1092 public CodeableConcept getCategory() { 1093 if (this.category == null) 1094 if (Configuration.errorOnAutoCreate()) 1095 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 1096 else if (Configuration.doAutoCreate()) 1097 this.category = new CodeableConcept(); // cc 1098 return this.category; 1099 } 1100 1101 public boolean hasCategory() { 1102 return this.category != null && !this.category.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #category} (A code to indicate the information type of 1107 * this adjudication record. Information types may include the 1108 * value submitted, maximum values or percentages allowed or 1109 * payable under the plan, amounts that: the patient is responsible 1110 * for in aggregate or pertaining to this item; amounts paid by 1111 * other coverages; and, the benefit payable for this item.) 1112 */ 1113 public AdjudicationComponent setCategory(CodeableConcept value) { 1114 this.category = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return {@link #reason} (A code supporting the understanding of the 1120 * adjudication result and explaining variance from expected amount.) 1121 */ 1122 public CodeableConcept getReason() { 1123 if (this.reason == null) 1124 if (Configuration.errorOnAutoCreate()) 1125 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 1126 else if (Configuration.doAutoCreate()) 1127 this.reason = new CodeableConcept(); // cc 1128 return this.reason; 1129 } 1130 1131 public boolean hasReason() { 1132 return this.reason != null && !this.reason.isEmpty(); 1133 } 1134 1135 /** 1136 * @param value {@link #reason} (A code supporting the understanding of the 1137 * adjudication result and explaining variance from expected 1138 * amount.) 1139 */ 1140 public AdjudicationComponent setReason(CodeableConcept value) { 1141 this.reason = value; 1142 return this; 1143 } 1144 1145 /** 1146 * @return {@link #amount} (Monetary amount associated with the category.) 1147 */ 1148 public Money getAmount() { 1149 if (this.amount == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 1152 else if (Configuration.doAutoCreate()) 1153 this.amount = new Money(); // cc 1154 return this.amount; 1155 } 1156 1157 public boolean hasAmount() { 1158 return this.amount != null && !this.amount.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #amount} (Monetary amount associated with the category.) 1163 */ 1164 public AdjudicationComponent setAmount(Money value) { 1165 this.amount = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return {@link #value} (A non-monetary value associated with the category. 1171 * Mutually exclusive to the amount element above.). This is the 1172 * underlying object with id, value and extensions. The accessor 1173 * "getValue" gives direct access to the value 1174 */ 1175 public DecimalType getValueElement() { 1176 if (this.value == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create AdjudicationComponent.value"); 1179 else if (Configuration.doAutoCreate()) 1180 this.value = new DecimalType(); // bb 1181 return this.value; 1182 } 1183 1184 public boolean hasValueElement() { 1185 return this.value != null && !this.value.isEmpty(); 1186 } 1187 1188 public boolean hasValue() { 1189 return this.value != null && !this.value.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #value} (A non-monetary value associated with the 1194 * category. Mutually exclusive to the amount element above.). This 1195 * is the underlying object with id, value and extensions. The 1196 * accessor "getValue" gives direct access to the value 1197 */ 1198 public AdjudicationComponent setValueElement(DecimalType value) { 1199 this.value = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return A non-monetary value associated with the category. Mutually exclusive 1205 * to the amount element above. 1206 */ 1207 public BigDecimal getValue() { 1208 return this.value == null ? null : this.value.getValue(); 1209 } 1210 1211 /** 1212 * @param value A non-monetary value associated with the category. Mutually 1213 * exclusive to the amount element above. 1214 */ 1215 public AdjudicationComponent setValue(BigDecimal value) { 1216 if (value == null) 1217 this.value = null; 1218 else { 1219 if (this.value == null) 1220 this.value = new DecimalType(); 1221 this.value.setValue(value); 1222 } 1223 return this; 1224 } 1225 1226 /** 1227 * @param value A non-monetary value associated with the category. Mutually 1228 * exclusive to the amount element above. 1229 */ 1230 public AdjudicationComponent setValue(long value) { 1231 this.value = new DecimalType(); 1232 this.value.setValue(value); 1233 return this; 1234 } 1235 1236 /** 1237 * @param value A non-monetary value associated with the category. Mutually 1238 * exclusive to the amount element above. 1239 */ 1240 public AdjudicationComponent setValue(double value) { 1241 this.value = new DecimalType(); 1242 this.value.setValue(value); 1243 return this; 1244 } 1245 1246 protected void listChildren(List<Property> children) { 1247 super.listChildren(children); 1248 children.add(new Property("category", "CodeableConcept", 1249 "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.", 1250 0, 1, category)); 1251 children.add(new Property("reason", "CodeableConcept", 1252 "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 1253 0, 1, reason)); 1254 children.add(new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount)); 1255 children.add(new Property("value", "decimal", 1256 "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, 1257 value)); 1258 } 1259 1260 @Override 1261 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1262 switch (_hash) { 1263 case 50511102: 1264 /* category */ return new Property("category", "CodeableConcept", 1265 "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.", 1266 0, 1, category); 1267 case -934964668: 1268 /* reason */ return new Property("reason", "CodeableConcept", 1269 "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 1270 0, 1, reason); 1271 case -1413853096: 1272 /* amount */ return new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, 1273 amount); 1274 case 111972721: 1275 /* value */ return new Property("value", "decimal", 1276 "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, 1277 value); 1278 default: 1279 return super.getNamedProperty(_hash, _name, _checkValid); 1280 } 1281 1282 } 1283 1284 @Override 1285 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1286 switch (hash) { 1287 case 50511102: 1288 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1289 case -934964668: 1290 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 1291 case -1413853096: 1292 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 1293 case 111972721: 1294 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 1295 default: 1296 return super.getProperty(hash, name, checkValid); 1297 } 1298 1299 } 1300 1301 @Override 1302 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1303 switch (hash) { 1304 case 50511102: // category 1305 this.category = castToCodeableConcept(value); // CodeableConcept 1306 return value; 1307 case -934964668: // reason 1308 this.reason = castToCodeableConcept(value); // CodeableConcept 1309 return value; 1310 case -1413853096: // amount 1311 this.amount = castToMoney(value); // Money 1312 return value; 1313 case 111972721: // value 1314 this.value = castToDecimal(value); // DecimalType 1315 return value; 1316 default: 1317 return super.setProperty(hash, name, value); 1318 } 1319 1320 } 1321 1322 @Override 1323 public Base setProperty(String name, Base value) throws FHIRException { 1324 if (name.equals("category")) { 1325 this.category = castToCodeableConcept(value); // CodeableConcept 1326 } else if (name.equals("reason")) { 1327 this.reason = castToCodeableConcept(value); // CodeableConcept 1328 } else if (name.equals("amount")) { 1329 this.amount = castToMoney(value); // Money 1330 } else if (name.equals("value")) { 1331 this.value = castToDecimal(value); // DecimalType 1332 } else 1333 return super.setProperty(name, value); 1334 return value; 1335 } 1336 1337 @Override 1338 public void removeChild(String name, Base value) throws FHIRException { 1339 if (name.equals("category")) { 1340 this.category = null; 1341 } else if (name.equals("reason")) { 1342 this.reason = null; 1343 } else if (name.equals("amount")) { 1344 this.amount = null; 1345 } else if (name.equals("value")) { 1346 this.value = null; 1347 } else 1348 super.removeChild(name, value); 1349 1350 } 1351 1352 @Override 1353 public Base makeProperty(int hash, String name) throws FHIRException { 1354 switch (hash) { 1355 case 50511102: 1356 return getCategory(); 1357 case -934964668: 1358 return getReason(); 1359 case -1413853096: 1360 return getAmount(); 1361 case 111972721: 1362 return getValueElement(); 1363 default: 1364 return super.makeProperty(hash, name); 1365 } 1366 1367 } 1368 1369 @Override 1370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1371 switch (hash) { 1372 case 50511102: 1373 /* category */ return new String[] { "CodeableConcept" }; 1374 case -934964668: 1375 /* reason */ return new String[] { "CodeableConcept" }; 1376 case -1413853096: 1377 /* amount */ return new String[] { "Money" }; 1378 case 111972721: 1379 /* value */ return new String[] { "decimal" }; 1380 default: 1381 return super.getTypesForProperty(hash, name); 1382 } 1383 1384 } 1385 1386 @Override 1387 public Base addChild(String name) throws FHIRException { 1388 if (name.equals("category")) { 1389 this.category = new CodeableConcept(); 1390 return this.category; 1391 } else if (name.equals("reason")) { 1392 this.reason = new CodeableConcept(); 1393 return this.reason; 1394 } else if (name.equals("amount")) { 1395 this.amount = new Money(); 1396 return this.amount; 1397 } else if (name.equals("value")) { 1398 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 1399 } else 1400 return super.addChild(name); 1401 } 1402 1403 public AdjudicationComponent copy() { 1404 AdjudicationComponent dst = new AdjudicationComponent(); 1405 copyValues(dst); 1406 return dst; 1407 } 1408 1409 public void copyValues(AdjudicationComponent dst) { 1410 super.copyValues(dst); 1411 dst.category = category == null ? null : category.copy(); 1412 dst.reason = reason == null ? null : reason.copy(); 1413 dst.amount = amount == null ? null : amount.copy(); 1414 dst.value = value == null ? null : value.copy(); 1415 } 1416 1417 @Override 1418 public boolean equalsDeep(Base other_) { 1419 if (!super.equalsDeep(other_)) 1420 return false; 1421 if (!(other_ instanceof AdjudicationComponent)) 1422 return false; 1423 AdjudicationComponent o = (AdjudicationComponent) other_; 1424 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) 1425 && compareDeep(amount, o.amount, true) && compareDeep(value, o.value, true); 1426 } 1427 1428 @Override 1429 public boolean equalsShallow(Base other_) { 1430 if (!super.equalsShallow(other_)) 1431 return false; 1432 if (!(other_ instanceof AdjudicationComponent)) 1433 return false; 1434 AdjudicationComponent o = (AdjudicationComponent) other_; 1435 return compareValues(value, o.value, true); 1436 } 1437 1438 public boolean isEmpty() { 1439 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount, value); 1440 } 1441 1442 public String fhirType() { 1443 return "ClaimResponse.item.adjudication"; 1444 1445 } 1446 1447 } 1448 1449 @Block() 1450 public static class ItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 1451 /** 1452 * A number to uniquely reference the claim detail entry. 1453 */ 1454 @Child(name = "detailSequence", type = { 1455 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1456 @Description(shortDefinition = "Claim detail instance identifier", formalDefinition = "A number to uniquely reference the claim detail entry.") 1457 protected PositiveIntType detailSequence; 1458 1459 /** 1460 * The numbers associated with notes below which apply to the adjudication of 1461 * this item. 1462 */ 1463 @Child(name = "noteNumber", type = { 1464 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1465 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 1466 protected List<PositiveIntType> noteNumber; 1467 1468 /** 1469 * The adjudication results. 1470 */ 1471 @Child(name = "adjudication", type = { 1472 AdjudicationComponent.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1473 @Description(shortDefinition = "Detail level adjudication details", formalDefinition = "The adjudication results.") 1474 protected List<AdjudicationComponent> adjudication; 1475 1476 /** 1477 * A sub-detail adjudication of a simple product or service. 1478 */ 1479 @Child(name = "subDetail", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1480 @Description(shortDefinition = "Adjudication for claim sub-details", formalDefinition = "A sub-detail adjudication of a simple product or service.") 1481 protected List<SubDetailComponent> subDetail; 1482 1483 private static final long serialVersionUID = 1066636111L; 1484 1485 /** 1486 * Constructor 1487 */ 1488 public ItemDetailComponent() { 1489 super(); 1490 } 1491 1492 /** 1493 * Constructor 1494 */ 1495 public ItemDetailComponent(PositiveIntType detailSequence) { 1496 super(); 1497 this.detailSequence = detailSequence; 1498 } 1499 1500 /** 1501 * @return {@link #detailSequence} (A number to uniquely reference the claim 1502 * detail entry.). This is the underlying object with id, value and 1503 * extensions. The accessor "getDetailSequence" gives direct access to 1504 * the value 1505 */ 1506 public PositiveIntType getDetailSequenceElement() { 1507 if (this.detailSequence == null) 1508 if (Configuration.errorOnAutoCreate()) 1509 throw new Error("Attempt to auto-create ItemDetailComponent.detailSequence"); 1510 else if (Configuration.doAutoCreate()) 1511 this.detailSequence = new PositiveIntType(); // bb 1512 return this.detailSequence; 1513 } 1514 1515 public boolean hasDetailSequenceElement() { 1516 return this.detailSequence != null && !this.detailSequence.isEmpty(); 1517 } 1518 1519 public boolean hasDetailSequence() { 1520 return this.detailSequence != null && !this.detailSequence.isEmpty(); 1521 } 1522 1523 /** 1524 * @param value {@link #detailSequence} (A number to uniquely reference the 1525 * claim detail entry.). This is the underlying object with id, 1526 * value and extensions. The accessor "getDetailSequence" gives 1527 * direct access to the value 1528 */ 1529 public ItemDetailComponent setDetailSequenceElement(PositiveIntType value) { 1530 this.detailSequence = value; 1531 return this; 1532 } 1533 1534 /** 1535 * @return A number to uniquely reference the claim detail entry. 1536 */ 1537 public int getDetailSequence() { 1538 return this.detailSequence == null || this.detailSequence.isEmpty() ? 0 : this.detailSequence.getValue(); 1539 } 1540 1541 /** 1542 * @param value A number to uniquely reference the claim detail entry. 1543 */ 1544 public ItemDetailComponent setDetailSequence(int value) { 1545 if (this.detailSequence == null) 1546 this.detailSequence = new PositiveIntType(); 1547 this.detailSequence.setValue(value); 1548 return this; 1549 } 1550 1551 /** 1552 * @return {@link #noteNumber} (The numbers associated with notes below which 1553 * apply to the adjudication of this item.) 1554 */ 1555 public List<PositiveIntType> getNoteNumber() { 1556 if (this.noteNumber == null) 1557 this.noteNumber = new ArrayList<PositiveIntType>(); 1558 return this.noteNumber; 1559 } 1560 1561 /** 1562 * @return Returns a reference to <code>this</code> for easy method chaining 1563 */ 1564 public ItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 1565 this.noteNumber = theNoteNumber; 1566 return this; 1567 } 1568 1569 public boolean hasNoteNumber() { 1570 if (this.noteNumber == null) 1571 return false; 1572 for (PositiveIntType item : this.noteNumber) 1573 if (!item.isEmpty()) 1574 return true; 1575 return false; 1576 } 1577 1578 /** 1579 * @return {@link #noteNumber} (The numbers associated with notes below which 1580 * apply to the adjudication of this item.) 1581 */ 1582 public PositiveIntType addNoteNumberElement() {// 2 1583 PositiveIntType t = new PositiveIntType(); 1584 if (this.noteNumber == null) 1585 this.noteNumber = new ArrayList<PositiveIntType>(); 1586 this.noteNumber.add(t); 1587 return t; 1588 } 1589 1590 /** 1591 * @param value {@link #noteNumber} (The numbers associated with notes below 1592 * which apply to the adjudication of this item.) 1593 */ 1594 public ItemDetailComponent addNoteNumber(int value) { // 1 1595 PositiveIntType t = new PositiveIntType(); 1596 t.setValue(value); 1597 if (this.noteNumber == null) 1598 this.noteNumber = new ArrayList<PositiveIntType>(); 1599 this.noteNumber.add(t); 1600 return this; 1601 } 1602 1603 /** 1604 * @param value {@link #noteNumber} (The numbers associated with notes below 1605 * which apply to the adjudication of this item.) 1606 */ 1607 public boolean hasNoteNumber(int value) { 1608 if (this.noteNumber == null) 1609 return false; 1610 for (PositiveIntType v : this.noteNumber) 1611 if (v.getValue().equals(value)) // positiveInt 1612 return true; 1613 return false; 1614 } 1615 1616 /** 1617 * @return {@link #adjudication} (The adjudication results.) 1618 */ 1619 public List<AdjudicationComponent> getAdjudication() { 1620 if (this.adjudication == null) 1621 this.adjudication = new ArrayList<AdjudicationComponent>(); 1622 return this.adjudication; 1623 } 1624 1625 /** 1626 * @return Returns a reference to <code>this</code> for easy method chaining 1627 */ 1628 public ItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 1629 this.adjudication = theAdjudication; 1630 return this; 1631 } 1632 1633 public boolean hasAdjudication() { 1634 if (this.adjudication == null) 1635 return false; 1636 for (AdjudicationComponent item : this.adjudication) 1637 if (!item.isEmpty()) 1638 return true; 1639 return false; 1640 } 1641 1642 public AdjudicationComponent addAdjudication() { // 3 1643 AdjudicationComponent t = new AdjudicationComponent(); 1644 if (this.adjudication == null) 1645 this.adjudication = new ArrayList<AdjudicationComponent>(); 1646 this.adjudication.add(t); 1647 return t; 1648 } 1649 1650 public ItemDetailComponent addAdjudication(AdjudicationComponent t) { // 3 1651 if (t == null) 1652 return this; 1653 if (this.adjudication == null) 1654 this.adjudication = new ArrayList<AdjudicationComponent>(); 1655 this.adjudication.add(t); 1656 return this; 1657 } 1658 1659 /** 1660 * @return The first repetition of repeating field {@link #adjudication}, 1661 * creating it if it does not already exist 1662 */ 1663 public AdjudicationComponent getAdjudicationFirstRep() { 1664 if (getAdjudication().isEmpty()) { 1665 addAdjudication(); 1666 } 1667 return getAdjudication().get(0); 1668 } 1669 1670 /** 1671 * @return {@link #subDetail} (A sub-detail adjudication of a simple product or 1672 * service.) 1673 */ 1674 public List<SubDetailComponent> getSubDetail() { 1675 if (this.subDetail == null) 1676 this.subDetail = new ArrayList<SubDetailComponent>(); 1677 return this.subDetail; 1678 } 1679 1680 /** 1681 * @return Returns a reference to <code>this</code> for easy method chaining 1682 */ 1683 public ItemDetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 1684 this.subDetail = theSubDetail; 1685 return this; 1686 } 1687 1688 public boolean hasSubDetail() { 1689 if (this.subDetail == null) 1690 return false; 1691 for (SubDetailComponent item : this.subDetail) 1692 if (!item.isEmpty()) 1693 return true; 1694 return false; 1695 } 1696 1697 public SubDetailComponent addSubDetail() { // 3 1698 SubDetailComponent t = new SubDetailComponent(); 1699 if (this.subDetail == null) 1700 this.subDetail = new ArrayList<SubDetailComponent>(); 1701 this.subDetail.add(t); 1702 return t; 1703 } 1704 1705 public ItemDetailComponent addSubDetail(SubDetailComponent t) { // 3 1706 if (t == null) 1707 return this; 1708 if (this.subDetail == null) 1709 this.subDetail = new ArrayList<SubDetailComponent>(); 1710 this.subDetail.add(t); 1711 return this; 1712 } 1713 1714 /** 1715 * @return The first repetition of repeating field {@link #subDetail}, creating 1716 * it if it does not already exist 1717 */ 1718 public SubDetailComponent getSubDetailFirstRep() { 1719 if (getSubDetail().isEmpty()) { 1720 addSubDetail(); 1721 } 1722 return getSubDetail().get(0); 1723 } 1724 1725 protected void listChildren(List<Property> children) { 1726 super.listChildren(children); 1727 children.add(new Property("detailSequence", "positiveInt", 1728 "A number to uniquely reference the claim detail entry.", 0, 1, detailSequence)); 1729 children.add(new Property("noteNumber", "positiveInt", 1730 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 1731 java.lang.Integer.MAX_VALUE, noteNumber)); 1732 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 1733 java.lang.Integer.MAX_VALUE, adjudication)); 1734 children.add(new Property("subDetail", "", "A sub-detail adjudication of a simple product or service.", 0, 1735 java.lang.Integer.MAX_VALUE, subDetail)); 1736 } 1737 1738 @Override 1739 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1740 switch (_hash) { 1741 case 1321472818: 1742 /* detailSequence */ return new Property("detailSequence", "positiveInt", 1743 "A number to uniquely reference the claim detail entry.", 0, 1, detailSequence); 1744 case -1110033957: 1745 /* noteNumber */ return new Property("noteNumber", "positiveInt", 1746 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 1747 java.lang.Integer.MAX_VALUE, noteNumber); 1748 case -231349275: 1749 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 1750 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 1751 case -828829007: 1752 /* subDetail */ return new Property("subDetail", "", 1753 "A sub-detail adjudication of a simple product or service.", 0, java.lang.Integer.MAX_VALUE, subDetail); 1754 default: 1755 return super.getNamedProperty(_hash, _name, _checkValid); 1756 } 1757 1758 } 1759 1760 @Override 1761 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1762 switch (hash) { 1763 case 1321472818: 1764 /* detailSequence */ return this.detailSequence == null ? new Base[0] : new Base[] { this.detailSequence }; // PositiveIntType 1765 case -1110033957: 1766 /* noteNumber */ return this.noteNumber == null ? new Base[0] 1767 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 1768 case -231349275: 1769 /* adjudication */ return this.adjudication == null ? new Base[0] 1770 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 1771 case -828829007: 1772 /* subDetail */ return this.subDetail == null ? new Base[0] 1773 : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 1774 default: 1775 return super.getProperty(hash, name, checkValid); 1776 } 1777 1778 } 1779 1780 @Override 1781 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1782 switch (hash) { 1783 case 1321472818: // detailSequence 1784 this.detailSequence = castToPositiveInt(value); // PositiveIntType 1785 return value; 1786 case -1110033957: // noteNumber 1787 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 1788 return value; 1789 case -231349275: // adjudication 1790 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 1791 return value; 1792 case -828829007: // subDetail 1793 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 1794 return value; 1795 default: 1796 return super.setProperty(hash, name, value); 1797 } 1798 1799 } 1800 1801 @Override 1802 public Base setProperty(String name, Base value) throws FHIRException { 1803 if (name.equals("detailSequence")) { 1804 this.detailSequence = castToPositiveInt(value); // PositiveIntType 1805 } else if (name.equals("noteNumber")) { 1806 this.getNoteNumber().add(castToPositiveInt(value)); 1807 } else if (name.equals("adjudication")) { 1808 this.getAdjudication().add((AdjudicationComponent) value); 1809 } else if (name.equals("subDetail")) { 1810 this.getSubDetail().add((SubDetailComponent) value); 1811 } else 1812 return super.setProperty(name, value); 1813 return value; 1814 } 1815 1816 @Override 1817 public void removeChild(String name, Base value) throws FHIRException { 1818 if (name.equals("detailSequence")) { 1819 this.detailSequence = null; 1820 } else if (name.equals("noteNumber")) { 1821 this.getNoteNumber().remove(castToPositiveInt(value)); 1822 } else if (name.equals("adjudication")) { 1823 this.getAdjudication().remove((AdjudicationComponent) value); 1824 } else if (name.equals("subDetail")) { 1825 this.getSubDetail().remove((SubDetailComponent) value); 1826 } else 1827 super.removeChild(name, value); 1828 1829 } 1830 1831 @Override 1832 public Base makeProperty(int hash, String name) throws FHIRException { 1833 switch (hash) { 1834 case 1321472818: 1835 return getDetailSequenceElement(); 1836 case -1110033957: 1837 return addNoteNumberElement(); 1838 case -231349275: 1839 return addAdjudication(); 1840 case -828829007: 1841 return addSubDetail(); 1842 default: 1843 return super.makeProperty(hash, name); 1844 } 1845 1846 } 1847 1848 @Override 1849 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1850 switch (hash) { 1851 case 1321472818: 1852 /* detailSequence */ return new String[] { "positiveInt" }; 1853 case -1110033957: 1854 /* noteNumber */ return new String[] { "positiveInt" }; 1855 case -231349275: 1856 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 1857 case -828829007: 1858 /* subDetail */ return new String[] {}; 1859 default: 1860 return super.getTypesForProperty(hash, name); 1861 } 1862 1863 } 1864 1865 @Override 1866 public Base addChild(String name) throws FHIRException { 1867 if (name.equals("detailSequence")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequence"); 1869 } else if (name.equals("noteNumber")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 1871 } else if (name.equals("adjudication")) { 1872 return addAdjudication(); 1873 } else if (name.equals("subDetail")) { 1874 return addSubDetail(); 1875 } else 1876 return super.addChild(name); 1877 } 1878 1879 public ItemDetailComponent copy() { 1880 ItemDetailComponent dst = new ItemDetailComponent(); 1881 copyValues(dst); 1882 return dst; 1883 } 1884 1885 public void copyValues(ItemDetailComponent dst) { 1886 super.copyValues(dst); 1887 dst.detailSequence = detailSequence == null ? null : detailSequence.copy(); 1888 if (noteNumber != null) { 1889 dst.noteNumber = new ArrayList<PositiveIntType>(); 1890 for (PositiveIntType i : noteNumber) 1891 dst.noteNumber.add(i.copy()); 1892 } 1893 ; 1894 if (adjudication != null) { 1895 dst.adjudication = new ArrayList<AdjudicationComponent>(); 1896 for (AdjudicationComponent i : adjudication) 1897 dst.adjudication.add(i.copy()); 1898 } 1899 ; 1900 if (subDetail != null) { 1901 dst.subDetail = new ArrayList<SubDetailComponent>(); 1902 for (SubDetailComponent i : subDetail) 1903 dst.subDetail.add(i.copy()); 1904 } 1905 ; 1906 } 1907 1908 @Override 1909 public boolean equalsDeep(Base other_) { 1910 if (!super.equalsDeep(other_)) 1911 return false; 1912 if (!(other_ instanceof ItemDetailComponent)) 1913 return false; 1914 ItemDetailComponent o = (ItemDetailComponent) other_; 1915 return compareDeep(detailSequence, o.detailSequence, true) && compareDeep(noteNumber, o.noteNumber, true) 1916 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true); 1917 } 1918 1919 @Override 1920 public boolean equalsShallow(Base other_) { 1921 if (!super.equalsShallow(other_)) 1922 return false; 1923 if (!(other_ instanceof ItemDetailComponent)) 1924 return false; 1925 ItemDetailComponent o = (ItemDetailComponent) other_; 1926 return compareValues(detailSequence, o.detailSequence, true) && compareValues(noteNumber, o.noteNumber, true); 1927 } 1928 1929 public boolean isEmpty() { 1930 return super.isEmpty() 1931 && ca.uhn.fhir.util.ElementUtil.isEmpty(detailSequence, noteNumber, adjudication, subDetail); 1932 } 1933 1934 public String fhirType() { 1935 return "ClaimResponse.item.detail"; 1936 1937 } 1938 1939 } 1940 1941 @Block() 1942 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 1943 /** 1944 * A number to uniquely reference the claim sub-detail entry. 1945 */ 1946 @Child(name = "subDetailSequence", type = { 1947 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1948 @Description(shortDefinition = "Claim sub-detail instance identifier", formalDefinition = "A number to uniquely reference the claim sub-detail entry.") 1949 protected PositiveIntType subDetailSequence; 1950 1951 /** 1952 * The numbers associated with notes below which apply to the adjudication of 1953 * this item. 1954 */ 1955 @Child(name = "noteNumber", type = { 1956 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1957 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 1958 protected List<PositiveIntType> noteNumber; 1959 1960 /** 1961 * The adjudication results. 1962 */ 1963 @Child(name = "adjudication", type = { 1964 AdjudicationComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1965 @Description(shortDefinition = "Subdetail level adjudication details", formalDefinition = "The adjudication results.") 1966 protected List<AdjudicationComponent> adjudication; 1967 1968 private static final long serialVersionUID = -1083724362L; 1969 1970 /** 1971 * Constructor 1972 */ 1973 public SubDetailComponent() { 1974 super(); 1975 } 1976 1977 /** 1978 * Constructor 1979 */ 1980 public SubDetailComponent(PositiveIntType subDetailSequence) { 1981 super(); 1982 this.subDetailSequence = subDetailSequence; 1983 } 1984 1985 /** 1986 * @return {@link #subDetailSequence} (A number to uniquely reference the claim 1987 * sub-detail entry.). This is the underlying object with id, value and 1988 * extensions. The accessor "getSubDetailSequence" gives direct access 1989 * to the value 1990 */ 1991 public PositiveIntType getSubDetailSequenceElement() { 1992 if (this.subDetailSequence == null) 1993 if (Configuration.errorOnAutoCreate()) 1994 throw new Error("Attempt to auto-create SubDetailComponent.subDetailSequence"); 1995 else if (Configuration.doAutoCreate()) 1996 this.subDetailSequence = new PositiveIntType(); // bb 1997 return this.subDetailSequence; 1998 } 1999 2000 public boolean hasSubDetailSequenceElement() { 2001 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 2002 } 2003 2004 public boolean hasSubDetailSequence() { 2005 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 2006 } 2007 2008 /** 2009 * @param value {@link #subDetailSequence} (A number to uniquely reference the 2010 * claim sub-detail entry.). This is the underlying object with id, 2011 * value and extensions. The accessor "getSubDetailSequence" gives 2012 * direct access to the value 2013 */ 2014 public SubDetailComponent setSubDetailSequenceElement(PositiveIntType value) { 2015 this.subDetailSequence = value; 2016 return this; 2017 } 2018 2019 /** 2020 * @return A number to uniquely reference the claim sub-detail entry. 2021 */ 2022 public int getSubDetailSequence() { 2023 return this.subDetailSequence == null || this.subDetailSequence.isEmpty() ? 0 : this.subDetailSequence.getValue(); 2024 } 2025 2026 /** 2027 * @param value A number to uniquely reference the claim sub-detail entry. 2028 */ 2029 public SubDetailComponent setSubDetailSequence(int value) { 2030 if (this.subDetailSequence == null) 2031 this.subDetailSequence = new PositiveIntType(); 2032 this.subDetailSequence.setValue(value); 2033 return this; 2034 } 2035 2036 /** 2037 * @return {@link #noteNumber} (The numbers associated with notes below which 2038 * apply to the adjudication of this item.) 2039 */ 2040 public List<PositiveIntType> getNoteNumber() { 2041 if (this.noteNumber == null) 2042 this.noteNumber = new ArrayList<PositiveIntType>(); 2043 return this.noteNumber; 2044 } 2045 2046 /** 2047 * @return Returns a reference to <code>this</code> for easy method chaining 2048 */ 2049 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 2050 this.noteNumber = theNoteNumber; 2051 return this; 2052 } 2053 2054 public boolean hasNoteNumber() { 2055 if (this.noteNumber == null) 2056 return false; 2057 for (PositiveIntType item : this.noteNumber) 2058 if (!item.isEmpty()) 2059 return true; 2060 return false; 2061 } 2062 2063 /** 2064 * @return {@link #noteNumber} (The numbers associated with notes below which 2065 * apply to the adjudication of this item.) 2066 */ 2067 public PositiveIntType addNoteNumberElement() {// 2 2068 PositiveIntType t = new PositiveIntType(); 2069 if (this.noteNumber == null) 2070 this.noteNumber = new ArrayList<PositiveIntType>(); 2071 this.noteNumber.add(t); 2072 return t; 2073 } 2074 2075 /** 2076 * @param value {@link #noteNumber} (The numbers associated with notes below 2077 * which apply to the adjudication of this item.) 2078 */ 2079 public SubDetailComponent addNoteNumber(int value) { // 1 2080 PositiveIntType t = new PositiveIntType(); 2081 t.setValue(value); 2082 if (this.noteNumber == null) 2083 this.noteNumber = new ArrayList<PositiveIntType>(); 2084 this.noteNumber.add(t); 2085 return this; 2086 } 2087 2088 /** 2089 * @param value {@link #noteNumber} (The numbers associated with notes below 2090 * which apply to the adjudication of this item.) 2091 */ 2092 public boolean hasNoteNumber(int value) { 2093 if (this.noteNumber == null) 2094 return false; 2095 for (PositiveIntType v : this.noteNumber) 2096 if (v.getValue().equals(value)) // positiveInt 2097 return true; 2098 return false; 2099 } 2100 2101 /** 2102 * @return {@link #adjudication} (The adjudication results.) 2103 */ 2104 public List<AdjudicationComponent> getAdjudication() { 2105 if (this.adjudication == null) 2106 this.adjudication = new ArrayList<AdjudicationComponent>(); 2107 return this.adjudication; 2108 } 2109 2110 /** 2111 * @return Returns a reference to <code>this</code> for easy method chaining 2112 */ 2113 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 2114 this.adjudication = theAdjudication; 2115 return this; 2116 } 2117 2118 public boolean hasAdjudication() { 2119 if (this.adjudication == null) 2120 return false; 2121 for (AdjudicationComponent item : this.adjudication) 2122 if (!item.isEmpty()) 2123 return true; 2124 return false; 2125 } 2126 2127 public AdjudicationComponent addAdjudication() { // 3 2128 AdjudicationComponent t = new AdjudicationComponent(); 2129 if (this.adjudication == null) 2130 this.adjudication = new ArrayList<AdjudicationComponent>(); 2131 this.adjudication.add(t); 2132 return t; 2133 } 2134 2135 public SubDetailComponent addAdjudication(AdjudicationComponent t) { // 3 2136 if (t == null) 2137 return this; 2138 if (this.adjudication == null) 2139 this.adjudication = new ArrayList<AdjudicationComponent>(); 2140 this.adjudication.add(t); 2141 return this; 2142 } 2143 2144 /** 2145 * @return The first repetition of repeating field {@link #adjudication}, 2146 * creating it if it does not already exist 2147 */ 2148 public AdjudicationComponent getAdjudicationFirstRep() { 2149 if (getAdjudication().isEmpty()) { 2150 addAdjudication(); 2151 } 2152 return getAdjudication().get(0); 2153 } 2154 2155 protected void listChildren(List<Property> children) { 2156 super.listChildren(children); 2157 children.add(new Property("subDetailSequence", "positiveInt", 2158 "A number to uniquely reference the claim sub-detail entry.", 0, 1, subDetailSequence)); 2159 children.add(new Property("noteNumber", "positiveInt", 2160 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 2161 java.lang.Integer.MAX_VALUE, noteNumber)); 2162 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 2163 java.lang.Integer.MAX_VALUE, adjudication)); 2164 } 2165 2166 @Override 2167 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2168 switch (_hash) { 2169 case -855462510: 2170 /* subDetailSequence */ return new Property("subDetailSequence", "positiveInt", 2171 "A number to uniquely reference the claim sub-detail entry.", 0, 1, subDetailSequence); 2172 case -1110033957: 2173 /* noteNumber */ return new Property("noteNumber", "positiveInt", 2174 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 2175 java.lang.Integer.MAX_VALUE, noteNumber); 2176 case -231349275: 2177 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 2178 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 2179 default: 2180 return super.getNamedProperty(_hash, _name, _checkValid); 2181 } 2182 2183 } 2184 2185 @Override 2186 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2187 switch (hash) { 2188 case -855462510: 2189 /* subDetailSequence */ return this.subDetailSequence == null ? new Base[0] 2190 : new Base[] { this.subDetailSequence }; // PositiveIntType 2191 case -1110033957: 2192 /* noteNumber */ return this.noteNumber == null ? new Base[0] 2193 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 2194 case -231349275: 2195 /* adjudication */ return this.adjudication == null ? new Base[0] 2196 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 2197 default: 2198 return super.getProperty(hash, name, checkValid); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2205 switch (hash) { 2206 case -855462510: // subDetailSequence 2207 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 2208 return value; 2209 case -1110033957: // noteNumber 2210 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 2211 return value; 2212 case -231349275: // adjudication 2213 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 2214 return value; 2215 default: 2216 return super.setProperty(hash, name, value); 2217 } 2218 2219 } 2220 2221 @Override 2222 public Base setProperty(String name, Base value) throws FHIRException { 2223 if (name.equals("subDetailSequence")) { 2224 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 2225 } else if (name.equals("noteNumber")) { 2226 this.getNoteNumber().add(castToPositiveInt(value)); 2227 } else if (name.equals("adjudication")) { 2228 this.getAdjudication().add((AdjudicationComponent) value); 2229 } else 2230 return super.setProperty(name, value); 2231 return value; 2232 } 2233 2234 @Override 2235 public void removeChild(String name, Base value) throws FHIRException { 2236 if (name.equals("subDetailSequence")) { 2237 this.subDetailSequence = null; 2238 } else if (name.equals("noteNumber")) { 2239 this.getNoteNumber().remove(castToPositiveInt(value)); 2240 } else if (name.equals("adjudication")) { 2241 this.getAdjudication().remove((AdjudicationComponent) value); 2242 } else 2243 super.removeChild(name, value); 2244 2245 } 2246 2247 @Override 2248 public Base makeProperty(int hash, String name) throws FHIRException { 2249 switch (hash) { 2250 case -855462510: 2251 return getSubDetailSequenceElement(); 2252 case -1110033957: 2253 return addNoteNumberElement(); 2254 case -231349275: 2255 return addAdjudication(); 2256 default: 2257 return super.makeProperty(hash, name); 2258 } 2259 2260 } 2261 2262 @Override 2263 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2264 switch (hash) { 2265 case -855462510: 2266 /* subDetailSequence */ return new String[] { "positiveInt" }; 2267 case -1110033957: 2268 /* noteNumber */ return new String[] { "positiveInt" }; 2269 case -231349275: 2270 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 2271 default: 2272 return super.getTypesForProperty(hash, name); 2273 } 2274 2275 } 2276 2277 @Override 2278 public Base addChild(String name) throws FHIRException { 2279 if (name.equals("subDetailSequence")) { 2280 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subDetailSequence"); 2281 } else if (name.equals("noteNumber")) { 2282 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 2283 } else if (name.equals("adjudication")) { 2284 return addAdjudication(); 2285 } else 2286 return super.addChild(name); 2287 } 2288 2289 public SubDetailComponent copy() { 2290 SubDetailComponent dst = new SubDetailComponent(); 2291 copyValues(dst); 2292 return dst; 2293 } 2294 2295 public void copyValues(SubDetailComponent dst) { 2296 super.copyValues(dst); 2297 dst.subDetailSequence = subDetailSequence == null ? null : subDetailSequence.copy(); 2298 if (noteNumber != null) { 2299 dst.noteNumber = new ArrayList<PositiveIntType>(); 2300 for (PositiveIntType i : noteNumber) 2301 dst.noteNumber.add(i.copy()); 2302 } 2303 ; 2304 if (adjudication != null) { 2305 dst.adjudication = new ArrayList<AdjudicationComponent>(); 2306 for (AdjudicationComponent i : adjudication) 2307 dst.adjudication.add(i.copy()); 2308 } 2309 ; 2310 } 2311 2312 @Override 2313 public boolean equalsDeep(Base other_) { 2314 if (!super.equalsDeep(other_)) 2315 return false; 2316 if (!(other_ instanceof SubDetailComponent)) 2317 return false; 2318 SubDetailComponent o = (SubDetailComponent) other_; 2319 return compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(noteNumber, o.noteNumber, true) 2320 && compareDeep(adjudication, o.adjudication, true); 2321 } 2322 2323 @Override 2324 public boolean equalsShallow(Base other_) { 2325 if (!super.equalsShallow(other_)) 2326 return false; 2327 if (!(other_ instanceof SubDetailComponent)) 2328 return false; 2329 SubDetailComponent o = (SubDetailComponent) other_; 2330 return compareValues(subDetailSequence, o.subDetailSequence, true) 2331 && compareValues(noteNumber, o.noteNumber, true); 2332 } 2333 2334 public boolean isEmpty() { 2335 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subDetailSequence, noteNumber, adjudication); 2336 } 2337 2338 public String fhirType() { 2339 return "ClaimResponse.item.detail.subDetail"; 2340 2341 } 2342 2343 } 2344 2345 @Block() 2346 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 2347 /** 2348 * Claim items which this service line is intended to replace. 2349 */ 2350 @Child(name = "itemSequence", type = { 2351 PositiveIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2352 @Description(shortDefinition = "Item sequence number", formalDefinition = "Claim items which this service line is intended to replace.") 2353 protected List<PositiveIntType> itemSequence; 2354 2355 /** 2356 * The sequence number of the details within the claim item which this line is 2357 * intended to replace. 2358 */ 2359 @Child(name = "detailSequence", type = { 2360 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2361 @Description(shortDefinition = "Detail sequence number", formalDefinition = "The sequence number of the details within the claim item which this line is intended to replace.") 2362 protected List<PositiveIntType> detailSequence; 2363 2364 /** 2365 * The sequence number of the sub-details within the details within the claim 2366 * item which this line is intended to replace. 2367 */ 2368 @Child(name = "subdetailSequence", type = { 2369 PositiveIntType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2370 @Description(shortDefinition = "Subdetail sequence number", formalDefinition = "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.") 2371 protected List<PositiveIntType> subdetailSequence; 2372 2373 /** 2374 * The providers who are authorized for the services rendered to the patient. 2375 */ 2376 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 2377 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2378 @Description(shortDefinition = "Authorized providers", formalDefinition = "The providers who are authorized for the services rendered to the patient.") 2379 protected List<Reference> provider; 2380 /** 2381 * The actual objects that are the target of the reference (The providers who 2382 * are authorized for the services rendered to the patient.) 2383 */ 2384 protected List<Resource> providerTarget; 2385 2386 /** 2387 * When the value is a group code then this item collects a set of related claim 2388 * details, otherwise this contains the product, service, drug or other billing 2389 * code for the item. 2390 */ 2391 @Child(name = "productOrService", type = { 2392 CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 2393 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 2394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 2395 protected CodeableConcept productOrService; 2396 2397 /** 2398 * Item typification or modifiers codes to convey additional context for the 2399 * product or service. 2400 */ 2401 @Child(name = "modifier", type = { 2402 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2403 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 2404 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 2405 protected List<CodeableConcept> modifier; 2406 2407 /** 2408 * Identifies the program under which this may be recovered. 2409 */ 2410 @Child(name = "programCode", type = { 2411 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2412 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 2413 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 2414 protected List<CodeableConcept> programCode; 2415 2416 /** 2417 * The date or dates when the service or product was supplied, performed or 2418 * completed. 2419 */ 2420 @Child(name = "serviced", type = { DateType.class, 2421 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2422 @Description(shortDefinition = "Date or dates of service or product delivery", formalDefinition = "The date or dates when the service or product was supplied, performed or completed.") 2423 protected Type serviced; 2424 2425 /** 2426 * Where the product or service was provided. 2427 */ 2428 @Child(name = "location", type = { CodeableConcept.class, Address.class, 2429 Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2430 @Description(shortDefinition = "Place of service or where product was supplied", formalDefinition = "Where the product or service was provided.") 2431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-place") 2432 protected Type location; 2433 2434 /** 2435 * The number of repetitions of a service or product. 2436 */ 2437 @Child(name = "quantity", type = { 2438 Quantity.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2439 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 2440 protected Quantity quantity; 2441 2442 /** 2443 * If the item is not a group then this is the fee for the product or service, 2444 * otherwise this is the total of the fees for the details of the group. 2445 */ 2446 @Child(name = "unitPrice", type = { Money.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 2447 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 2448 protected Money unitPrice; 2449 2450 /** 2451 * A real number that represents a multiplier used in determining the overall 2452 * value of services delivered and/or goods received. The concept of a Factor 2453 * allows for a discount or surcharge multiplier to be applied to a monetary 2454 * amount. 2455 */ 2456 @Child(name = "factor", type = { 2457 DecimalType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2458 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 2459 protected DecimalType factor; 2460 2461 /** 2462 * The quantity times the unit price for an additional service or product or 2463 * charge. 2464 */ 2465 @Child(name = "net", type = { Money.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2466 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 2467 protected Money net; 2468 2469 /** 2470 * Physical service site on the patient (limb, tooth, etc.). 2471 */ 2472 @Child(name = "bodySite", type = { 2473 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2474 @Description(shortDefinition = "Anatomical location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 2475 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/tooth") 2476 protected CodeableConcept bodySite; 2477 2478 /** 2479 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 2480 */ 2481 @Child(name = "subSite", type = { 2482 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2483 @Description(shortDefinition = "Anatomical sub-location", formalDefinition = "A region or surface of the bodySite, e.g. limb region or tooth surface(s).") 2484 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/surface") 2485 protected List<CodeableConcept> subSite; 2486 2487 /** 2488 * The numbers associated with notes below which apply to the adjudication of 2489 * this item. 2490 */ 2491 @Child(name = "noteNumber", type = { 2492 PositiveIntType.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2493 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 2494 protected List<PositiveIntType> noteNumber; 2495 2496 /** 2497 * The adjudication results. 2498 */ 2499 @Child(name = "adjudication", type = { 2500 AdjudicationComponent.class }, order = 17, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2501 @Description(shortDefinition = "Added items adjudication", formalDefinition = "The adjudication results.") 2502 protected List<AdjudicationComponent> adjudication; 2503 2504 /** 2505 * The second-tier service adjudications for payor added services. 2506 */ 2507 @Child(name = "detail", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2508 @Description(shortDefinition = "Insurer added line details", formalDefinition = "The second-tier service adjudications for payor added services.") 2509 protected List<AddedItemDetailComponent> detail; 2510 2511 private static final long serialVersionUID = -1193747282L; 2512 2513 /** 2514 * Constructor 2515 */ 2516 public AddedItemComponent() { 2517 super(); 2518 } 2519 2520 /** 2521 * Constructor 2522 */ 2523 public AddedItemComponent(CodeableConcept productOrService) { 2524 super(); 2525 this.productOrService = productOrService; 2526 } 2527 2528 /** 2529 * @return {@link #itemSequence} (Claim items which this service line is 2530 * intended to replace.) 2531 */ 2532 public List<PositiveIntType> getItemSequence() { 2533 if (this.itemSequence == null) 2534 this.itemSequence = new ArrayList<PositiveIntType>(); 2535 return this.itemSequence; 2536 } 2537 2538 /** 2539 * @return Returns a reference to <code>this</code> for easy method chaining 2540 */ 2541 public AddedItemComponent setItemSequence(List<PositiveIntType> theItemSequence) { 2542 this.itemSequence = theItemSequence; 2543 return this; 2544 } 2545 2546 public boolean hasItemSequence() { 2547 if (this.itemSequence == null) 2548 return false; 2549 for (PositiveIntType item : this.itemSequence) 2550 if (!item.isEmpty()) 2551 return true; 2552 return false; 2553 } 2554 2555 /** 2556 * @return {@link #itemSequence} (Claim items which this service line is 2557 * intended to replace.) 2558 */ 2559 public PositiveIntType addItemSequenceElement() {// 2 2560 PositiveIntType t = new PositiveIntType(); 2561 if (this.itemSequence == null) 2562 this.itemSequence = new ArrayList<PositiveIntType>(); 2563 this.itemSequence.add(t); 2564 return t; 2565 } 2566 2567 /** 2568 * @param value {@link #itemSequence} (Claim items which this service line is 2569 * intended to replace.) 2570 */ 2571 public AddedItemComponent addItemSequence(int value) { // 1 2572 PositiveIntType t = new PositiveIntType(); 2573 t.setValue(value); 2574 if (this.itemSequence == null) 2575 this.itemSequence = new ArrayList<PositiveIntType>(); 2576 this.itemSequence.add(t); 2577 return this; 2578 } 2579 2580 /** 2581 * @param value {@link #itemSequence} (Claim items which this service line is 2582 * intended to replace.) 2583 */ 2584 public boolean hasItemSequence(int value) { 2585 if (this.itemSequence == null) 2586 return false; 2587 for (PositiveIntType v : this.itemSequence) 2588 if (v.getValue().equals(value)) // positiveInt 2589 return true; 2590 return false; 2591 } 2592 2593 /** 2594 * @return {@link #detailSequence} (The sequence number of the details within 2595 * the claim item which this line is intended to replace.) 2596 */ 2597 public List<PositiveIntType> getDetailSequence() { 2598 if (this.detailSequence == null) 2599 this.detailSequence = new ArrayList<PositiveIntType>(); 2600 return this.detailSequence; 2601 } 2602 2603 /** 2604 * @return Returns a reference to <code>this</code> for easy method chaining 2605 */ 2606 public AddedItemComponent setDetailSequence(List<PositiveIntType> theDetailSequence) { 2607 this.detailSequence = theDetailSequence; 2608 return this; 2609 } 2610 2611 public boolean hasDetailSequence() { 2612 if (this.detailSequence == null) 2613 return false; 2614 for (PositiveIntType item : this.detailSequence) 2615 if (!item.isEmpty()) 2616 return true; 2617 return false; 2618 } 2619 2620 /** 2621 * @return {@link #detailSequence} (The sequence number of the details within 2622 * the claim item which this line is intended to replace.) 2623 */ 2624 public PositiveIntType addDetailSequenceElement() {// 2 2625 PositiveIntType t = new PositiveIntType(); 2626 if (this.detailSequence == null) 2627 this.detailSequence = new ArrayList<PositiveIntType>(); 2628 this.detailSequence.add(t); 2629 return t; 2630 } 2631 2632 /** 2633 * @param value {@link #detailSequence} (The sequence number of the details 2634 * within the claim item which this line is intended to replace.) 2635 */ 2636 public AddedItemComponent addDetailSequence(int value) { // 1 2637 PositiveIntType t = new PositiveIntType(); 2638 t.setValue(value); 2639 if (this.detailSequence == null) 2640 this.detailSequence = new ArrayList<PositiveIntType>(); 2641 this.detailSequence.add(t); 2642 return this; 2643 } 2644 2645 /** 2646 * @param value {@link #detailSequence} (The sequence number of the details 2647 * within the claim item which this line is intended to replace.) 2648 */ 2649 public boolean hasDetailSequence(int value) { 2650 if (this.detailSequence == null) 2651 return false; 2652 for (PositiveIntType v : this.detailSequence) 2653 if (v.getValue().equals(value)) // positiveInt 2654 return true; 2655 return false; 2656 } 2657 2658 /** 2659 * @return {@link #subdetailSequence} (The sequence number of the sub-details 2660 * within the details within the claim item which this line is intended 2661 * to replace.) 2662 */ 2663 public List<PositiveIntType> getSubdetailSequence() { 2664 if (this.subdetailSequence == null) 2665 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2666 return this.subdetailSequence; 2667 } 2668 2669 /** 2670 * @return Returns a reference to <code>this</code> for easy method chaining 2671 */ 2672 public AddedItemComponent setSubdetailSequence(List<PositiveIntType> theSubdetailSequence) { 2673 this.subdetailSequence = theSubdetailSequence; 2674 return this; 2675 } 2676 2677 public boolean hasSubdetailSequence() { 2678 if (this.subdetailSequence == null) 2679 return false; 2680 for (PositiveIntType item : this.subdetailSequence) 2681 if (!item.isEmpty()) 2682 return true; 2683 return false; 2684 } 2685 2686 /** 2687 * @return {@link #subdetailSequence} (The sequence number of the sub-details 2688 * within the details within the claim item which this line is intended 2689 * to replace.) 2690 */ 2691 public PositiveIntType addSubdetailSequenceElement() {// 2 2692 PositiveIntType t = new PositiveIntType(); 2693 if (this.subdetailSequence == null) 2694 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2695 this.subdetailSequence.add(t); 2696 return t; 2697 } 2698 2699 /** 2700 * @param value {@link #subdetailSequence} (The sequence number of the 2701 * sub-details within the details within the claim item which this 2702 * line is intended to replace.) 2703 */ 2704 public AddedItemComponent addSubdetailSequence(int value) { // 1 2705 PositiveIntType t = new PositiveIntType(); 2706 t.setValue(value); 2707 if (this.subdetailSequence == null) 2708 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2709 this.subdetailSequence.add(t); 2710 return this; 2711 } 2712 2713 /** 2714 * @param value {@link #subdetailSequence} (The sequence number of the 2715 * sub-details within the details within the claim item which this 2716 * line is intended to replace.) 2717 */ 2718 public boolean hasSubdetailSequence(int value) { 2719 if (this.subdetailSequence == null) 2720 return false; 2721 for (PositiveIntType v : this.subdetailSequence) 2722 if (v.getValue().equals(value)) // positiveInt 2723 return true; 2724 return false; 2725 } 2726 2727 /** 2728 * @return {@link #provider} (The providers who are authorized for the services 2729 * rendered to the patient.) 2730 */ 2731 public List<Reference> getProvider() { 2732 if (this.provider == null) 2733 this.provider = new ArrayList<Reference>(); 2734 return this.provider; 2735 } 2736 2737 /** 2738 * @return Returns a reference to <code>this</code> for easy method chaining 2739 */ 2740 public AddedItemComponent setProvider(List<Reference> theProvider) { 2741 this.provider = theProvider; 2742 return this; 2743 } 2744 2745 public boolean hasProvider() { 2746 if (this.provider == null) 2747 return false; 2748 for (Reference item : this.provider) 2749 if (!item.isEmpty()) 2750 return true; 2751 return false; 2752 } 2753 2754 public Reference addProvider() { // 3 2755 Reference t = new Reference(); 2756 if (this.provider == null) 2757 this.provider = new ArrayList<Reference>(); 2758 this.provider.add(t); 2759 return t; 2760 } 2761 2762 public AddedItemComponent addProvider(Reference t) { // 3 2763 if (t == null) 2764 return this; 2765 if (this.provider == null) 2766 this.provider = new ArrayList<Reference>(); 2767 this.provider.add(t); 2768 return this; 2769 } 2770 2771 /** 2772 * @return The first repetition of repeating field {@link #provider}, creating 2773 * it if it does not already exist 2774 */ 2775 public Reference getProviderFirstRep() { 2776 if (getProvider().isEmpty()) { 2777 addProvider(); 2778 } 2779 return getProvider().get(0); 2780 } 2781 2782 /** 2783 * @deprecated Use Reference#setResource(IBaseResource) instead 2784 */ 2785 @Deprecated 2786 public List<Resource> getProviderTarget() { 2787 if (this.providerTarget == null) 2788 this.providerTarget = new ArrayList<Resource>(); 2789 return this.providerTarget; 2790 } 2791 2792 /** 2793 * @return {@link #productOrService} (When the value is a group code then this 2794 * item collects a set of related claim details, otherwise this contains 2795 * the product, service, drug or other billing code for the item.) 2796 */ 2797 public CodeableConcept getProductOrService() { 2798 if (this.productOrService == null) 2799 if (Configuration.errorOnAutoCreate()) 2800 throw new Error("Attempt to auto-create AddedItemComponent.productOrService"); 2801 else if (Configuration.doAutoCreate()) 2802 this.productOrService = new CodeableConcept(); // cc 2803 return this.productOrService; 2804 } 2805 2806 public boolean hasProductOrService() { 2807 return this.productOrService != null && !this.productOrService.isEmpty(); 2808 } 2809 2810 /** 2811 * @param value {@link #productOrService} (When the value is a group code then 2812 * this item collects a set of related claim details, otherwise 2813 * this contains the product, service, drug or other billing code 2814 * for the item.) 2815 */ 2816 public AddedItemComponent setProductOrService(CodeableConcept value) { 2817 this.productOrService = value; 2818 return this; 2819 } 2820 2821 /** 2822 * @return {@link #modifier} (Item typification or modifiers codes to convey 2823 * additional context for the product or service.) 2824 */ 2825 public List<CodeableConcept> getModifier() { 2826 if (this.modifier == null) 2827 this.modifier = new ArrayList<CodeableConcept>(); 2828 return this.modifier; 2829 } 2830 2831 /** 2832 * @return Returns a reference to <code>this</code> for easy method chaining 2833 */ 2834 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 2835 this.modifier = theModifier; 2836 return this; 2837 } 2838 2839 public boolean hasModifier() { 2840 if (this.modifier == null) 2841 return false; 2842 for (CodeableConcept item : this.modifier) 2843 if (!item.isEmpty()) 2844 return true; 2845 return false; 2846 } 2847 2848 public CodeableConcept addModifier() { // 3 2849 CodeableConcept t = new CodeableConcept(); 2850 if (this.modifier == null) 2851 this.modifier = new ArrayList<CodeableConcept>(); 2852 this.modifier.add(t); 2853 return t; 2854 } 2855 2856 public AddedItemComponent addModifier(CodeableConcept t) { // 3 2857 if (t == null) 2858 return this; 2859 if (this.modifier == null) 2860 this.modifier = new ArrayList<CodeableConcept>(); 2861 this.modifier.add(t); 2862 return this; 2863 } 2864 2865 /** 2866 * @return The first repetition of repeating field {@link #modifier}, creating 2867 * it if it does not already exist 2868 */ 2869 public CodeableConcept getModifierFirstRep() { 2870 if (getModifier().isEmpty()) { 2871 addModifier(); 2872 } 2873 return getModifier().get(0); 2874 } 2875 2876 /** 2877 * @return {@link #programCode} (Identifies the program under which this may be 2878 * recovered.) 2879 */ 2880 public List<CodeableConcept> getProgramCode() { 2881 if (this.programCode == null) 2882 this.programCode = new ArrayList<CodeableConcept>(); 2883 return this.programCode; 2884 } 2885 2886 /** 2887 * @return Returns a reference to <code>this</code> for easy method chaining 2888 */ 2889 public AddedItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 2890 this.programCode = theProgramCode; 2891 return this; 2892 } 2893 2894 public boolean hasProgramCode() { 2895 if (this.programCode == null) 2896 return false; 2897 for (CodeableConcept item : this.programCode) 2898 if (!item.isEmpty()) 2899 return true; 2900 return false; 2901 } 2902 2903 public CodeableConcept addProgramCode() { // 3 2904 CodeableConcept t = new CodeableConcept(); 2905 if (this.programCode == null) 2906 this.programCode = new ArrayList<CodeableConcept>(); 2907 this.programCode.add(t); 2908 return t; 2909 } 2910 2911 public AddedItemComponent addProgramCode(CodeableConcept t) { // 3 2912 if (t == null) 2913 return this; 2914 if (this.programCode == null) 2915 this.programCode = new ArrayList<CodeableConcept>(); 2916 this.programCode.add(t); 2917 return this; 2918 } 2919 2920 /** 2921 * @return The first repetition of repeating field {@link #programCode}, 2922 * creating it if it does not already exist 2923 */ 2924 public CodeableConcept getProgramCodeFirstRep() { 2925 if (getProgramCode().isEmpty()) { 2926 addProgramCode(); 2927 } 2928 return getProgramCode().get(0); 2929 } 2930 2931 /** 2932 * @return {@link #serviced} (The date or dates when the service or product was 2933 * supplied, performed or completed.) 2934 */ 2935 public Type getServiced() { 2936 return this.serviced; 2937 } 2938 2939 /** 2940 * @return {@link #serviced} (The date or dates when the service or product was 2941 * supplied, performed or completed.) 2942 */ 2943 public DateType getServicedDateType() throws FHIRException { 2944 if (this.serviced == null) 2945 this.serviced = new DateType(); 2946 if (!(this.serviced instanceof DateType)) 2947 throw new FHIRException("Type mismatch: the type DateType was expected, but " 2948 + this.serviced.getClass().getName() + " was encountered"); 2949 return (DateType) this.serviced; 2950 } 2951 2952 public boolean hasServicedDateType() { 2953 return this != null && this.serviced instanceof DateType; 2954 } 2955 2956 /** 2957 * @return {@link #serviced} (The date or dates when the service or product was 2958 * supplied, performed or completed.) 2959 */ 2960 public Period getServicedPeriod() throws FHIRException { 2961 if (this.serviced == null) 2962 this.serviced = new Period(); 2963 if (!(this.serviced instanceof Period)) 2964 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 2965 + " was encountered"); 2966 return (Period) this.serviced; 2967 } 2968 2969 public boolean hasServicedPeriod() { 2970 return this != null && this.serviced instanceof Period; 2971 } 2972 2973 public boolean hasServiced() { 2974 return this.serviced != null && !this.serviced.isEmpty(); 2975 } 2976 2977 /** 2978 * @param value {@link #serviced} (The date or dates when the service or product 2979 * was supplied, performed or completed.) 2980 */ 2981 public AddedItemComponent setServiced(Type value) { 2982 if (value != null && !(value instanceof DateType || value instanceof Period)) 2983 throw new Error("Not the right type for ClaimResponse.addItem.serviced[x]: " + value.fhirType()); 2984 this.serviced = value; 2985 return this; 2986 } 2987 2988 /** 2989 * @return {@link #location} (Where the product or service was provided.) 2990 */ 2991 public Type getLocation() { 2992 return this.location; 2993 } 2994 2995 /** 2996 * @return {@link #location} (Where the product or service was provided.) 2997 */ 2998 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 2999 if (this.location == null) 3000 this.location = new CodeableConcept(); 3001 if (!(this.location instanceof CodeableConcept)) 3002 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 3003 + this.location.getClass().getName() + " was encountered"); 3004 return (CodeableConcept) this.location; 3005 } 3006 3007 public boolean hasLocationCodeableConcept() { 3008 return this != null && this.location instanceof CodeableConcept; 3009 } 3010 3011 /** 3012 * @return {@link #location} (Where the product or service was provided.) 3013 */ 3014 public Address getLocationAddress() throws FHIRException { 3015 if (this.location == null) 3016 this.location = new Address(); 3017 if (!(this.location instanceof Address)) 3018 throw new FHIRException("Type mismatch: the type Address was expected, but " 3019 + this.location.getClass().getName() + " was encountered"); 3020 return (Address) this.location; 3021 } 3022 3023 public boolean hasLocationAddress() { 3024 return this != null && this.location instanceof Address; 3025 } 3026 3027 /** 3028 * @return {@link #location} (Where the product or service was provided.) 3029 */ 3030 public Reference getLocationReference() throws FHIRException { 3031 if (this.location == null) 3032 this.location = new Reference(); 3033 if (!(this.location instanceof Reference)) 3034 throw new FHIRException("Type mismatch: the type Reference was expected, but " 3035 + this.location.getClass().getName() + " was encountered"); 3036 return (Reference) this.location; 3037 } 3038 3039 public boolean hasLocationReference() { 3040 return this != null && this.location instanceof Reference; 3041 } 3042 3043 public boolean hasLocation() { 3044 return this.location != null && !this.location.isEmpty(); 3045 } 3046 3047 /** 3048 * @param value {@link #location} (Where the product or service was provided.) 3049 */ 3050 public AddedItemComponent setLocation(Type value) { 3051 if (value != null 3052 && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 3053 throw new Error("Not the right type for ClaimResponse.addItem.location[x]: " + value.fhirType()); 3054 this.location = value; 3055 return this; 3056 } 3057 3058 /** 3059 * @return {@link #quantity} (The number of repetitions of a service or 3060 * product.) 3061 */ 3062 public Quantity getQuantity() { 3063 if (this.quantity == null) 3064 if (Configuration.errorOnAutoCreate()) 3065 throw new Error("Attempt to auto-create AddedItemComponent.quantity"); 3066 else if (Configuration.doAutoCreate()) 3067 this.quantity = new Quantity(); // cc 3068 return this.quantity; 3069 } 3070 3071 public boolean hasQuantity() { 3072 return this.quantity != null && !this.quantity.isEmpty(); 3073 } 3074 3075 /** 3076 * @param value {@link #quantity} (The number of repetitions of a service or 3077 * product.) 3078 */ 3079 public AddedItemComponent setQuantity(Quantity value) { 3080 this.quantity = value; 3081 return this; 3082 } 3083 3084 /** 3085 * @return {@link #unitPrice} (If the item is not a group then this is the fee 3086 * for the product or service, otherwise this is the total of the fees 3087 * for the details of the group.) 3088 */ 3089 public Money getUnitPrice() { 3090 if (this.unitPrice == null) 3091 if (Configuration.errorOnAutoCreate()) 3092 throw new Error("Attempt to auto-create AddedItemComponent.unitPrice"); 3093 else if (Configuration.doAutoCreate()) 3094 this.unitPrice = new Money(); // cc 3095 return this.unitPrice; 3096 } 3097 3098 public boolean hasUnitPrice() { 3099 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3100 } 3101 3102 /** 3103 * @param value {@link #unitPrice} (If the item is not a group then this is the 3104 * fee for the product or service, otherwise this is the total of 3105 * the fees for the details of the group.) 3106 */ 3107 public AddedItemComponent setUnitPrice(Money value) { 3108 this.unitPrice = value; 3109 return this; 3110 } 3111 3112 /** 3113 * @return {@link #factor} (A real number that represents a multiplier used in 3114 * determining the overall value of services delivered and/or goods 3115 * received. The concept of a Factor allows for a discount or surcharge 3116 * multiplier to be applied to a monetary amount.). This is the 3117 * underlying object with id, value and extensions. The accessor 3118 * "getFactor" gives direct access to the value 3119 */ 3120 public DecimalType getFactorElement() { 3121 if (this.factor == null) 3122 if (Configuration.errorOnAutoCreate()) 3123 throw new Error("Attempt to auto-create AddedItemComponent.factor"); 3124 else if (Configuration.doAutoCreate()) 3125 this.factor = new DecimalType(); // bb 3126 return this.factor; 3127 } 3128 3129 public boolean hasFactorElement() { 3130 return this.factor != null && !this.factor.isEmpty(); 3131 } 3132 3133 public boolean hasFactor() { 3134 return this.factor != null && !this.factor.isEmpty(); 3135 } 3136 3137 /** 3138 * @param value {@link #factor} (A real number that represents a multiplier used 3139 * in determining the overall value of services delivered and/or 3140 * goods received. The concept of a Factor allows for a discount or 3141 * surcharge multiplier to be applied to a monetary amount.). This 3142 * is the underlying object with id, value and extensions. The 3143 * accessor "getFactor" gives direct access to the value 3144 */ 3145 public AddedItemComponent setFactorElement(DecimalType value) { 3146 this.factor = value; 3147 return this; 3148 } 3149 3150 /** 3151 * @return A real number that represents a multiplier used in determining the 3152 * overall value of services delivered and/or goods received. The 3153 * concept of a Factor allows for a discount or surcharge multiplier to 3154 * be applied to a monetary amount. 3155 */ 3156 public BigDecimal getFactor() { 3157 return this.factor == null ? null : this.factor.getValue(); 3158 } 3159 3160 /** 3161 * @param value A real number that represents a multiplier used in determining 3162 * the overall value of services delivered and/or goods received. 3163 * The concept of a Factor allows for a discount or surcharge 3164 * multiplier to be applied to a monetary amount. 3165 */ 3166 public AddedItemComponent setFactor(BigDecimal value) { 3167 if (value == null) 3168 this.factor = null; 3169 else { 3170 if (this.factor == null) 3171 this.factor = new DecimalType(); 3172 this.factor.setValue(value); 3173 } 3174 return this; 3175 } 3176 3177 /** 3178 * @param value A real number that represents a multiplier used in determining 3179 * the overall value of services delivered and/or goods received. 3180 * The concept of a Factor allows for a discount or surcharge 3181 * multiplier to be applied to a monetary amount. 3182 */ 3183 public AddedItemComponent setFactor(long value) { 3184 this.factor = new DecimalType(); 3185 this.factor.setValue(value); 3186 return this; 3187 } 3188 3189 /** 3190 * @param value A real number that represents a multiplier used in determining 3191 * the overall value of services delivered and/or goods received. 3192 * The concept of a Factor allows for a discount or surcharge 3193 * multiplier to be applied to a monetary amount. 3194 */ 3195 public AddedItemComponent setFactor(double value) { 3196 this.factor = new DecimalType(); 3197 this.factor.setValue(value); 3198 return this; 3199 } 3200 3201 /** 3202 * @return {@link #net} (The quantity times the unit price for an additional 3203 * service or product or charge.) 3204 */ 3205 public Money getNet() { 3206 if (this.net == null) 3207 if (Configuration.errorOnAutoCreate()) 3208 throw new Error("Attempt to auto-create AddedItemComponent.net"); 3209 else if (Configuration.doAutoCreate()) 3210 this.net = new Money(); // cc 3211 return this.net; 3212 } 3213 3214 public boolean hasNet() { 3215 return this.net != null && !this.net.isEmpty(); 3216 } 3217 3218 /** 3219 * @param value {@link #net} (The quantity times the unit price for an 3220 * additional service or product or charge.) 3221 */ 3222 public AddedItemComponent setNet(Money value) { 3223 this.net = value; 3224 return this; 3225 } 3226 3227 /** 3228 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 3229 * etc.).) 3230 */ 3231 public CodeableConcept getBodySite() { 3232 if (this.bodySite == null) 3233 if (Configuration.errorOnAutoCreate()) 3234 throw new Error("Attempt to auto-create AddedItemComponent.bodySite"); 3235 else if (Configuration.doAutoCreate()) 3236 this.bodySite = new CodeableConcept(); // cc 3237 return this.bodySite; 3238 } 3239 3240 public boolean hasBodySite() { 3241 return this.bodySite != null && !this.bodySite.isEmpty(); 3242 } 3243 3244 /** 3245 * @param value {@link #bodySite} (Physical service site on the patient (limb, 3246 * tooth, etc.).) 3247 */ 3248 public AddedItemComponent setBodySite(CodeableConcept value) { 3249 this.bodySite = value; 3250 return this; 3251 } 3252 3253 /** 3254 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb 3255 * region or tooth surface(s).) 3256 */ 3257 public List<CodeableConcept> getSubSite() { 3258 if (this.subSite == null) 3259 this.subSite = new ArrayList<CodeableConcept>(); 3260 return this.subSite; 3261 } 3262 3263 /** 3264 * @return Returns a reference to <code>this</code> for easy method chaining 3265 */ 3266 public AddedItemComponent setSubSite(List<CodeableConcept> theSubSite) { 3267 this.subSite = theSubSite; 3268 return this; 3269 } 3270 3271 public boolean hasSubSite() { 3272 if (this.subSite == null) 3273 return false; 3274 for (CodeableConcept item : this.subSite) 3275 if (!item.isEmpty()) 3276 return true; 3277 return false; 3278 } 3279 3280 public CodeableConcept addSubSite() { // 3 3281 CodeableConcept t = new CodeableConcept(); 3282 if (this.subSite == null) 3283 this.subSite = new ArrayList<CodeableConcept>(); 3284 this.subSite.add(t); 3285 return t; 3286 } 3287 3288 public AddedItemComponent addSubSite(CodeableConcept t) { // 3 3289 if (t == null) 3290 return this; 3291 if (this.subSite == null) 3292 this.subSite = new ArrayList<CodeableConcept>(); 3293 this.subSite.add(t); 3294 return this; 3295 } 3296 3297 /** 3298 * @return The first repetition of repeating field {@link #subSite}, creating it 3299 * if it does not already exist 3300 */ 3301 public CodeableConcept getSubSiteFirstRep() { 3302 if (getSubSite().isEmpty()) { 3303 addSubSite(); 3304 } 3305 return getSubSite().get(0); 3306 } 3307 3308 /** 3309 * @return {@link #noteNumber} (The numbers associated with notes below which 3310 * apply to the adjudication of this item.) 3311 */ 3312 public List<PositiveIntType> getNoteNumber() { 3313 if (this.noteNumber == null) 3314 this.noteNumber = new ArrayList<PositiveIntType>(); 3315 return this.noteNumber; 3316 } 3317 3318 /** 3319 * @return Returns a reference to <code>this</code> for easy method chaining 3320 */ 3321 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 3322 this.noteNumber = theNoteNumber; 3323 return this; 3324 } 3325 3326 public boolean hasNoteNumber() { 3327 if (this.noteNumber == null) 3328 return false; 3329 for (PositiveIntType item : this.noteNumber) 3330 if (!item.isEmpty()) 3331 return true; 3332 return false; 3333 } 3334 3335 /** 3336 * @return {@link #noteNumber} (The numbers associated with notes below which 3337 * apply to the adjudication of this item.) 3338 */ 3339 public PositiveIntType addNoteNumberElement() {// 2 3340 PositiveIntType t = new PositiveIntType(); 3341 if (this.noteNumber == null) 3342 this.noteNumber = new ArrayList<PositiveIntType>(); 3343 this.noteNumber.add(t); 3344 return t; 3345 } 3346 3347 /** 3348 * @param value {@link #noteNumber} (The numbers associated with notes below 3349 * which apply to the adjudication of this item.) 3350 */ 3351 public AddedItemComponent addNoteNumber(int value) { // 1 3352 PositiveIntType t = new PositiveIntType(); 3353 t.setValue(value); 3354 if (this.noteNumber == null) 3355 this.noteNumber = new ArrayList<PositiveIntType>(); 3356 this.noteNumber.add(t); 3357 return this; 3358 } 3359 3360 /** 3361 * @param value {@link #noteNumber} (The numbers associated with notes below 3362 * which apply to the adjudication of this item.) 3363 */ 3364 public boolean hasNoteNumber(int value) { 3365 if (this.noteNumber == null) 3366 return false; 3367 for (PositiveIntType v : this.noteNumber) 3368 if (v.getValue().equals(value)) // positiveInt 3369 return true; 3370 return false; 3371 } 3372 3373 /** 3374 * @return {@link #adjudication} (The adjudication results.) 3375 */ 3376 public List<AdjudicationComponent> getAdjudication() { 3377 if (this.adjudication == null) 3378 this.adjudication = new ArrayList<AdjudicationComponent>(); 3379 return this.adjudication; 3380 } 3381 3382 /** 3383 * @return Returns a reference to <code>this</code> for easy method chaining 3384 */ 3385 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 3386 this.adjudication = theAdjudication; 3387 return this; 3388 } 3389 3390 public boolean hasAdjudication() { 3391 if (this.adjudication == null) 3392 return false; 3393 for (AdjudicationComponent item : this.adjudication) 3394 if (!item.isEmpty()) 3395 return true; 3396 return false; 3397 } 3398 3399 public AdjudicationComponent addAdjudication() { // 3 3400 AdjudicationComponent t = new AdjudicationComponent(); 3401 if (this.adjudication == null) 3402 this.adjudication = new ArrayList<AdjudicationComponent>(); 3403 this.adjudication.add(t); 3404 return t; 3405 } 3406 3407 public AddedItemComponent addAdjudication(AdjudicationComponent t) { // 3 3408 if (t == null) 3409 return this; 3410 if (this.adjudication == null) 3411 this.adjudication = new ArrayList<AdjudicationComponent>(); 3412 this.adjudication.add(t); 3413 return this; 3414 } 3415 3416 /** 3417 * @return The first repetition of repeating field {@link #adjudication}, 3418 * creating it if it does not already exist 3419 */ 3420 public AdjudicationComponent getAdjudicationFirstRep() { 3421 if (getAdjudication().isEmpty()) { 3422 addAdjudication(); 3423 } 3424 return getAdjudication().get(0); 3425 } 3426 3427 /** 3428 * @return {@link #detail} (The second-tier service adjudications for payor 3429 * added services.) 3430 */ 3431 public List<AddedItemDetailComponent> getDetail() { 3432 if (this.detail == null) 3433 this.detail = new ArrayList<AddedItemDetailComponent>(); 3434 return this.detail; 3435 } 3436 3437 /** 3438 * @return Returns a reference to <code>this</code> for easy method chaining 3439 */ 3440 public AddedItemComponent setDetail(List<AddedItemDetailComponent> theDetail) { 3441 this.detail = theDetail; 3442 return this; 3443 } 3444 3445 public boolean hasDetail() { 3446 if (this.detail == null) 3447 return false; 3448 for (AddedItemDetailComponent item : this.detail) 3449 if (!item.isEmpty()) 3450 return true; 3451 return false; 3452 } 3453 3454 public AddedItemDetailComponent addDetail() { // 3 3455 AddedItemDetailComponent t = new AddedItemDetailComponent(); 3456 if (this.detail == null) 3457 this.detail = new ArrayList<AddedItemDetailComponent>(); 3458 this.detail.add(t); 3459 return t; 3460 } 3461 3462 public AddedItemComponent addDetail(AddedItemDetailComponent t) { // 3 3463 if (t == null) 3464 return this; 3465 if (this.detail == null) 3466 this.detail = new ArrayList<AddedItemDetailComponent>(); 3467 this.detail.add(t); 3468 return this; 3469 } 3470 3471 /** 3472 * @return The first repetition of repeating field {@link #detail}, creating it 3473 * if it does not already exist 3474 */ 3475 public AddedItemDetailComponent getDetailFirstRep() { 3476 if (getDetail().isEmpty()) { 3477 addDetail(); 3478 } 3479 return getDetail().get(0); 3480 } 3481 3482 protected void listChildren(List<Property> children) { 3483 super.listChildren(children); 3484 children.add(new Property("itemSequence", "positiveInt", 3485 "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence)); 3486 children.add(new Property("detailSequence", "positiveInt", 3487 "The sequence number of the details within the claim item which this line is intended to replace.", 0, 3488 java.lang.Integer.MAX_VALUE, detailSequence)); 3489 children.add(new Property("subdetailSequence", "positiveInt", 3490 "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.", 3491 0, java.lang.Integer.MAX_VALUE, subdetailSequence)); 3492 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 3493 "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 3494 provider)); 3495 children.add(new Property("productOrService", "CodeableConcept", 3496 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 3497 0, 1, productOrService)); 3498 children.add(new Property("modifier", "CodeableConcept", 3499 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 3500 java.lang.Integer.MAX_VALUE, modifier)); 3501 children.add(new Property("programCode", "CodeableConcept", 3502 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 3503 children.add(new Property("serviced[x]", "date|Period", 3504 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 3505 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3506 "Where the product or service was provided.", 0, 1, location)); 3507 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 3508 1, quantity)); 3509 children.add(new Property("unitPrice", "Money", 3510 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 3511 0, 1, unitPrice)); 3512 children.add(new Property("factor", "decimal", 3513 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 3514 0, 1, factor)); 3515 children.add(new Property("net", "Money", 3516 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 3517 children.add(new Property("bodySite", "CodeableConcept", 3518 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite)); 3519 children.add(new Property("subSite", "CodeableConcept", 3520 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, 3521 subSite)); 3522 children.add(new Property("noteNumber", "positiveInt", 3523 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 3524 java.lang.Integer.MAX_VALUE, noteNumber)); 3525 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 3526 java.lang.Integer.MAX_VALUE, adjudication)); 3527 children.add(new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, 3528 java.lang.Integer.MAX_VALUE, detail)); 3529 } 3530 3531 @Override 3532 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3533 switch (_hash) { 3534 case 1977979892: 3535 /* itemSequence */ return new Property("itemSequence", "positiveInt", 3536 "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, 3537 itemSequence); 3538 case 1321472818: 3539 /* detailSequence */ return new Property("detailSequence", "positiveInt", 3540 "The sequence number of the details within the claim item which this line is intended to replace.", 0, 3541 java.lang.Integer.MAX_VALUE, detailSequence); 3542 case 146530674: 3543 /* subdetailSequence */ return new Property("subdetailSequence", "positiveInt", 3544 "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.", 3545 0, java.lang.Integer.MAX_VALUE, subdetailSequence); 3546 case -987494927: 3547 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 3548 "The providers who are authorized for the services rendered to the patient.", 0, 3549 java.lang.Integer.MAX_VALUE, provider); 3550 case 1957227299: 3551 /* productOrService */ return new Property("productOrService", "CodeableConcept", 3552 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 3553 0, 1, productOrService); 3554 case -615513385: 3555 /* modifier */ return new Property("modifier", "CodeableConcept", 3556 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 3557 java.lang.Integer.MAX_VALUE, modifier); 3558 case 1010065041: 3559 /* programCode */ return new Property("programCode", "CodeableConcept", 3560 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 3561 case -1927922223: 3562 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 3563 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3564 case 1379209295: 3565 /* serviced */ return new Property("serviced[x]", "date|Period", 3566 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3567 case 363246749: 3568 /* servicedDate */ return new Property("serviced[x]", "date|Period", 3569 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3570 case 1534966512: 3571 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 3572 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3573 case 552316075: 3574 /* location[x] */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3575 "Where the product or service was provided.", 0, 1, location); 3576 case 1901043637: 3577 /* location */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3578 "Where the product or service was provided.", 0, 1, location); 3579 case -1224800468: 3580 /* locationCodeableConcept */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3581 "Where the product or service was provided.", 0, 1, location); 3582 case -1280020865: 3583 /* locationAddress */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3584 "Where the product or service was provided.", 0, 1, location); 3585 case 755866390: 3586 /* locationReference */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 3587 "Where the product or service was provided.", 0, 1, location); 3588 case -1285004149: 3589 /* quantity */ return new Property("quantity", "SimpleQuantity", 3590 "The number of repetitions of a service or product.", 0, 1, quantity); 3591 case -486196699: 3592 /* unitPrice */ return new Property("unitPrice", "Money", 3593 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 3594 0, 1, unitPrice); 3595 case -1282148017: 3596 /* factor */ return new Property("factor", "decimal", 3597 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 3598 0, 1, factor); 3599 case 108957: 3600 /* net */ return new Property("net", "Money", 3601 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 3602 case 1702620169: 3603 /* bodySite */ return new Property("bodySite", "CodeableConcept", 3604 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite); 3605 case -1868566105: 3606 /* subSite */ return new Property("subSite", "CodeableConcept", 3607 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, 3608 java.lang.Integer.MAX_VALUE, subSite); 3609 case -1110033957: 3610 /* noteNumber */ return new Property("noteNumber", "positiveInt", 3611 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 3612 java.lang.Integer.MAX_VALUE, noteNumber); 3613 case -231349275: 3614 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 3615 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 3616 case -1335224239: 3617 /* detail */ return new Property("detail", "", 3618 "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 3619 default: 3620 return super.getNamedProperty(_hash, _name, _checkValid); 3621 } 3622 3623 } 3624 3625 @Override 3626 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3627 switch (hash) { 3628 case 1977979892: 3629 /* itemSequence */ return this.itemSequence == null ? new Base[0] 3630 : this.itemSequence.toArray(new Base[this.itemSequence.size()]); // PositiveIntType 3631 case 1321472818: 3632 /* detailSequence */ return this.detailSequence == null ? new Base[0] 3633 : this.detailSequence.toArray(new Base[this.detailSequence.size()]); // PositiveIntType 3634 case 146530674: 3635 /* subdetailSequence */ return this.subdetailSequence == null ? new Base[0] 3636 : this.subdetailSequence.toArray(new Base[this.subdetailSequence.size()]); // PositiveIntType 3637 case -987494927: 3638 /* provider */ return this.provider == null ? new Base[0] 3639 : this.provider.toArray(new Base[this.provider.size()]); // Reference 3640 case 1957227299: 3641 /* productOrService */ return this.productOrService == null ? new Base[0] 3642 : new Base[] { this.productOrService }; // CodeableConcept 3643 case -615513385: 3644 /* modifier */ return this.modifier == null ? new Base[0] 3645 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 3646 case 1010065041: 3647 /* programCode */ return this.programCode == null ? new Base[0] 3648 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 3649 case 1379209295: 3650 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 3651 case 1901043637: 3652 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 3653 case -1285004149: 3654 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 3655 case -486196699: 3656 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 3657 case -1282148017: 3658 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 3659 case 108957: 3660 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 3661 case 1702620169: 3662 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 3663 case -1868566105: 3664 /* subSite */ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 3665 case -1110033957: 3666 /* noteNumber */ return this.noteNumber == null ? new Base[0] 3667 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 3668 case -231349275: 3669 /* adjudication */ return this.adjudication == null ? new Base[0] 3670 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 3671 case -1335224239: 3672 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemDetailComponent 3673 default: 3674 return super.getProperty(hash, name, checkValid); 3675 } 3676 3677 } 3678 3679 @Override 3680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3681 switch (hash) { 3682 case 1977979892: // itemSequence 3683 this.getItemSequence().add(castToPositiveInt(value)); // PositiveIntType 3684 return value; 3685 case 1321472818: // detailSequence 3686 this.getDetailSequence().add(castToPositiveInt(value)); // PositiveIntType 3687 return value; 3688 case 146530674: // subdetailSequence 3689 this.getSubdetailSequence().add(castToPositiveInt(value)); // PositiveIntType 3690 return value; 3691 case -987494927: // provider 3692 this.getProvider().add(castToReference(value)); // Reference 3693 return value; 3694 case 1957227299: // productOrService 3695 this.productOrService = castToCodeableConcept(value); // CodeableConcept 3696 return value; 3697 case -615513385: // modifier 3698 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 3699 return value; 3700 case 1010065041: // programCode 3701 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 3702 return value; 3703 case 1379209295: // serviced 3704 this.serviced = castToType(value); // Type 3705 return value; 3706 case 1901043637: // location 3707 this.location = castToType(value); // Type 3708 return value; 3709 case -1285004149: // quantity 3710 this.quantity = castToQuantity(value); // Quantity 3711 return value; 3712 case -486196699: // unitPrice 3713 this.unitPrice = castToMoney(value); // Money 3714 return value; 3715 case -1282148017: // factor 3716 this.factor = castToDecimal(value); // DecimalType 3717 return value; 3718 case 108957: // net 3719 this.net = castToMoney(value); // Money 3720 return value; 3721 case 1702620169: // bodySite 3722 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3723 return value; 3724 case -1868566105: // subSite 3725 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 3726 return value; 3727 case -1110033957: // noteNumber 3728 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 3729 return value; 3730 case -231349275: // adjudication 3731 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 3732 return value; 3733 case -1335224239: // detail 3734 this.getDetail().add((AddedItemDetailComponent) value); // AddedItemDetailComponent 3735 return value; 3736 default: 3737 return super.setProperty(hash, name, value); 3738 } 3739 3740 } 3741 3742 @Override 3743 public Base setProperty(String name, Base value) throws FHIRException { 3744 if (name.equals("itemSequence")) { 3745 this.getItemSequence().add(castToPositiveInt(value)); 3746 } else if (name.equals("detailSequence")) { 3747 this.getDetailSequence().add(castToPositiveInt(value)); 3748 } else if (name.equals("subdetailSequence")) { 3749 this.getSubdetailSequence().add(castToPositiveInt(value)); 3750 } else if (name.equals("provider")) { 3751 this.getProvider().add(castToReference(value)); 3752 } else if (name.equals("productOrService")) { 3753 this.productOrService = castToCodeableConcept(value); // CodeableConcept 3754 } else if (name.equals("modifier")) { 3755 this.getModifier().add(castToCodeableConcept(value)); 3756 } else if (name.equals("programCode")) { 3757 this.getProgramCode().add(castToCodeableConcept(value)); 3758 } else if (name.equals("serviced[x]")) { 3759 this.serviced = castToType(value); // Type 3760 } else if (name.equals("location[x]")) { 3761 this.location = castToType(value); // Type 3762 } else if (name.equals("quantity")) { 3763 this.quantity = castToQuantity(value); // Quantity 3764 } else if (name.equals("unitPrice")) { 3765 this.unitPrice = castToMoney(value); // Money 3766 } else if (name.equals("factor")) { 3767 this.factor = castToDecimal(value); // DecimalType 3768 } else if (name.equals("net")) { 3769 this.net = castToMoney(value); // Money 3770 } else if (name.equals("bodySite")) { 3771 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3772 } else if (name.equals("subSite")) { 3773 this.getSubSite().add(castToCodeableConcept(value)); 3774 } else if (name.equals("noteNumber")) { 3775 this.getNoteNumber().add(castToPositiveInt(value)); 3776 } else if (name.equals("adjudication")) { 3777 this.getAdjudication().add((AdjudicationComponent) value); 3778 } else if (name.equals("detail")) { 3779 this.getDetail().add((AddedItemDetailComponent) value); 3780 } else 3781 return super.setProperty(name, value); 3782 return value; 3783 } 3784 3785 @Override 3786 public void removeChild(String name, Base value) throws FHIRException { 3787 if (name.equals("itemSequence")) { 3788 this.getItemSequence().remove(castToPositiveInt(value)); 3789 } else if (name.equals("detailSequence")) { 3790 this.getDetailSequence().remove(castToPositiveInt(value)); 3791 } else if (name.equals("subdetailSequence")) { 3792 this.getSubdetailSequence().remove(castToPositiveInt(value)); 3793 } else if (name.equals("provider")) { 3794 this.getProvider().remove(castToReference(value)); 3795 } else if (name.equals("productOrService")) { 3796 this.productOrService = null; 3797 } else if (name.equals("modifier")) { 3798 this.getModifier().remove(castToCodeableConcept(value)); 3799 } else if (name.equals("programCode")) { 3800 this.getProgramCode().remove(castToCodeableConcept(value)); 3801 } else if (name.equals("serviced[x]")) { 3802 this.serviced = null; 3803 } else if (name.equals("location[x]")) { 3804 this.location = null; 3805 } else if (name.equals("quantity")) { 3806 this.quantity = null; 3807 } else if (name.equals("unitPrice")) { 3808 this.unitPrice = null; 3809 } else if (name.equals("factor")) { 3810 this.factor = null; 3811 } else if (name.equals("net")) { 3812 this.net = null; 3813 } else if (name.equals("bodySite")) { 3814 this.bodySite = null; 3815 } else if (name.equals("subSite")) { 3816 this.getSubSite().remove(castToCodeableConcept(value)); 3817 } else if (name.equals("noteNumber")) { 3818 this.getNoteNumber().remove(castToPositiveInt(value)); 3819 } else if (name.equals("adjudication")) { 3820 this.getAdjudication().remove((AdjudicationComponent) value); 3821 } else if (name.equals("detail")) { 3822 this.getDetail().remove((AddedItemDetailComponent) value); 3823 } else 3824 super.removeChild(name, value); 3825 3826 } 3827 3828 @Override 3829 public Base makeProperty(int hash, String name) throws FHIRException { 3830 switch (hash) { 3831 case 1977979892: 3832 return addItemSequenceElement(); 3833 case 1321472818: 3834 return addDetailSequenceElement(); 3835 case 146530674: 3836 return addSubdetailSequenceElement(); 3837 case -987494927: 3838 return addProvider(); 3839 case 1957227299: 3840 return getProductOrService(); 3841 case -615513385: 3842 return addModifier(); 3843 case 1010065041: 3844 return addProgramCode(); 3845 case -1927922223: 3846 return getServiced(); 3847 case 1379209295: 3848 return getServiced(); 3849 case 552316075: 3850 return getLocation(); 3851 case 1901043637: 3852 return getLocation(); 3853 case -1285004149: 3854 return getQuantity(); 3855 case -486196699: 3856 return getUnitPrice(); 3857 case -1282148017: 3858 return getFactorElement(); 3859 case 108957: 3860 return getNet(); 3861 case 1702620169: 3862 return getBodySite(); 3863 case -1868566105: 3864 return addSubSite(); 3865 case -1110033957: 3866 return addNoteNumberElement(); 3867 case -231349275: 3868 return addAdjudication(); 3869 case -1335224239: 3870 return addDetail(); 3871 default: 3872 return super.makeProperty(hash, name); 3873 } 3874 3875 } 3876 3877 @Override 3878 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3879 switch (hash) { 3880 case 1977979892: 3881 /* itemSequence */ return new String[] { "positiveInt" }; 3882 case 1321472818: 3883 /* detailSequence */ return new String[] { "positiveInt" }; 3884 case 146530674: 3885 /* subdetailSequence */ return new String[] { "positiveInt" }; 3886 case -987494927: 3887 /* provider */ return new String[] { "Reference" }; 3888 case 1957227299: 3889 /* productOrService */ return new String[] { "CodeableConcept" }; 3890 case -615513385: 3891 /* modifier */ return new String[] { "CodeableConcept" }; 3892 case 1010065041: 3893 /* programCode */ return new String[] { "CodeableConcept" }; 3894 case 1379209295: 3895 /* serviced */ return new String[] { "date", "Period" }; 3896 case 1901043637: 3897 /* location */ return new String[] { "CodeableConcept", "Address", "Reference" }; 3898 case -1285004149: 3899 /* quantity */ return new String[] { "SimpleQuantity" }; 3900 case -486196699: 3901 /* unitPrice */ return new String[] { "Money" }; 3902 case -1282148017: 3903 /* factor */ return new String[] { "decimal" }; 3904 case 108957: 3905 /* net */ return new String[] { "Money" }; 3906 case 1702620169: 3907 /* bodySite */ return new String[] { "CodeableConcept" }; 3908 case -1868566105: 3909 /* subSite */ return new String[] { "CodeableConcept" }; 3910 case -1110033957: 3911 /* noteNumber */ return new String[] { "positiveInt" }; 3912 case -231349275: 3913 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 3914 case -1335224239: 3915 /* detail */ return new String[] {}; 3916 default: 3917 return super.getTypesForProperty(hash, name); 3918 } 3919 3920 } 3921 3922 @Override 3923 public Base addChild(String name) throws FHIRException { 3924 if (name.equals("itemSequence")) { 3925 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.itemSequence"); 3926 } else if (name.equals("detailSequence")) { 3927 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequence"); 3928 } else if (name.equals("subdetailSequence")) { 3929 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subdetailSequence"); 3930 } else if (name.equals("provider")) { 3931 return addProvider(); 3932 } else if (name.equals("productOrService")) { 3933 this.productOrService = new CodeableConcept(); 3934 return this.productOrService; 3935 } else if (name.equals("modifier")) { 3936 return addModifier(); 3937 } else if (name.equals("programCode")) { 3938 return addProgramCode(); 3939 } else if (name.equals("servicedDate")) { 3940 this.serviced = new DateType(); 3941 return this.serviced; 3942 } else if (name.equals("servicedPeriod")) { 3943 this.serviced = new Period(); 3944 return this.serviced; 3945 } else if (name.equals("locationCodeableConcept")) { 3946 this.location = new CodeableConcept(); 3947 return this.location; 3948 } else if (name.equals("locationAddress")) { 3949 this.location = new Address(); 3950 return this.location; 3951 } else if (name.equals("locationReference")) { 3952 this.location = new Reference(); 3953 return this.location; 3954 } else if (name.equals("quantity")) { 3955 this.quantity = new Quantity(); 3956 return this.quantity; 3957 } else if (name.equals("unitPrice")) { 3958 this.unitPrice = new Money(); 3959 return this.unitPrice; 3960 } else if (name.equals("factor")) { 3961 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.factor"); 3962 } else if (name.equals("net")) { 3963 this.net = new Money(); 3964 return this.net; 3965 } else if (name.equals("bodySite")) { 3966 this.bodySite = new CodeableConcept(); 3967 return this.bodySite; 3968 } else if (name.equals("subSite")) { 3969 return addSubSite(); 3970 } else if (name.equals("noteNumber")) { 3971 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 3972 } else if (name.equals("adjudication")) { 3973 return addAdjudication(); 3974 } else if (name.equals("detail")) { 3975 return addDetail(); 3976 } else 3977 return super.addChild(name); 3978 } 3979 3980 public AddedItemComponent copy() { 3981 AddedItemComponent dst = new AddedItemComponent(); 3982 copyValues(dst); 3983 return dst; 3984 } 3985 3986 public void copyValues(AddedItemComponent dst) { 3987 super.copyValues(dst); 3988 if (itemSequence != null) { 3989 dst.itemSequence = new ArrayList<PositiveIntType>(); 3990 for (PositiveIntType i : itemSequence) 3991 dst.itemSequence.add(i.copy()); 3992 } 3993 ; 3994 if (detailSequence != null) { 3995 dst.detailSequence = new ArrayList<PositiveIntType>(); 3996 for (PositiveIntType i : detailSequence) 3997 dst.detailSequence.add(i.copy()); 3998 } 3999 ; 4000 if (subdetailSequence != null) { 4001 dst.subdetailSequence = new ArrayList<PositiveIntType>(); 4002 for (PositiveIntType i : subdetailSequence) 4003 dst.subdetailSequence.add(i.copy()); 4004 } 4005 ; 4006 if (provider != null) { 4007 dst.provider = new ArrayList<Reference>(); 4008 for (Reference i : provider) 4009 dst.provider.add(i.copy()); 4010 } 4011 ; 4012 dst.productOrService = productOrService == null ? null : productOrService.copy(); 4013 if (modifier != null) { 4014 dst.modifier = new ArrayList<CodeableConcept>(); 4015 for (CodeableConcept i : modifier) 4016 dst.modifier.add(i.copy()); 4017 } 4018 ; 4019 if (programCode != null) { 4020 dst.programCode = new ArrayList<CodeableConcept>(); 4021 for (CodeableConcept i : programCode) 4022 dst.programCode.add(i.copy()); 4023 } 4024 ; 4025 dst.serviced = serviced == null ? null : serviced.copy(); 4026 dst.location = location == null ? null : location.copy(); 4027 dst.quantity = quantity == null ? null : quantity.copy(); 4028 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4029 dst.factor = factor == null ? null : factor.copy(); 4030 dst.net = net == null ? null : net.copy(); 4031 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4032 if (subSite != null) { 4033 dst.subSite = new ArrayList<CodeableConcept>(); 4034 for (CodeableConcept i : subSite) 4035 dst.subSite.add(i.copy()); 4036 } 4037 ; 4038 if (noteNumber != null) { 4039 dst.noteNumber = new ArrayList<PositiveIntType>(); 4040 for (PositiveIntType i : noteNumber) 4041 dst.noteNumber.add(i.copy()); 4042 } 4043 ; 4044 if (adjudication != null) { 4045 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4046 for (AdjudicationComponent i : adjudication) 4047 dst.adjudication.add(i.copy()); 4048 } 4049 ; 4050 if (detail != null) { 4051 dst.detail = new ArrayList<AddedItemDetailComponent>(); 4052 for (AddedItemDetailComponent i : detail) 4053 dst.detail.add(i.copy()); 4054 } 4055 ; 4056 } 4057 4058 @Override 4059 public boolean equalsDeep(Base other_) { 4060 if (!super.equalsDeep(other_)) 4061 return false; 4062 if (!(other_ instanceof AddedItemComponent)) 4063 return false; 4064 AddedItemComponent o = (AddedItemComponent) other_; 4065 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 4066 && compareDeep(subdetailSequence, o.subdetailSequence, true) && compareDeep(provider, o.provider, true) 4067 && compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 4068 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 4069 && compareDeep(location, o.location, true) && compareDeep(quantity, o.quantity, true) 4070 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 4071 && compareDeep(net, o.net, true) && compareDeep(bodySite, o.bodySite, true) 4072 && compareDeep(subSite, o.subSite, true) && compareDeep(noteNumber, o.noteNumber, true) 4073 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 4074 } 4075 4076 @Override 4077 public boolean equalsShallow(Base other_) { 4078 if (!super.equalsShallow(other_)) 4079 return false; 4080 if (!(other_ instanceof AddedItemComponent)) 4081 return false; 4082 AddedItemComponent o = (AddedItemComponent) other_; 4083 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 4084 && compareValues(subdetailSequence, o.subdetailSequence, true) && compareValues(factor, o.factor, true) 4085 && compareValues(noteNumber, o.noteNumber, true); 4086 } 4087 4088 public boolean isEmpty() { 4089 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence, subdetailSequence, 4090 provider, productOrService, modifier, programCode, serviced, location, quantity, unitPrice, factor, net, 4091 bodySite, subSite, noteNumber, adjudication, detail); 4092 } 4093 4094 public String fhirType() { 4095 return "ClaimResponse.addItem"; 4096 4097 } 4098 4099 } 4100 4101 @Block() 4102 public static class AddedItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 4103 /** 4104 * When the value is a group code then this item collects a set of related claim 4105 * details, otherwise this contains the product, service, drug or other billing 4106 * code for the item. 4107 */ 4108 @Child(name = "productOrService", type = { 4109 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4110 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4112 protected CodeableConcept productOrService; 4113 4114 /** 4115 * Item typification or modifiers codes to convey additional context for the 4116 * product or service. 4117 */ 4118 @Child(name = "modifier", type = { 4119 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4120 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4121 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4122 protected List<CodeableConcept> modifier; 4123 4124 /** 4125 * The number of repetitions of a service or product. 4126 */ 4127 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4128 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4129 protected Quantity quantity; 4130 4131 /** 4132 * If the item is not a group then this is the fee for the product or service, 4133 * otherwise this is the total of the fees for the details of the group. 4134 */ 4135 @Child(name = "unitPrice", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 4136 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 4137 protected Money unitPrice; 4138 4139 /** 4140 * A real number that represents a multiplier used in determining the overall 4141 * value of services delivered and/or goods received. The concept of a Factor 4142 * allows for a discount or surcharge multiplier to be applied to a monetary 4143 * amount. 4144 */ 4145 @Child(name = "factor", type = { 4146 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4147 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 4148 protected DecimalType factor; 4149 4150 /** 4151 * The quantity times the unit price for an additional service or product or 4152 * charge. 4153 */ 4154 @Child(name = "net", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4155 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 4156 protected Money net; 4157 4158 /** 4159 * The numbers associated with notes below which apply to the adjudication of 4160 * this item. 4161 */ 4162 @Child(name = "noteNumber", type = { 4163 PositiveIntType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4164 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 4165 protected List<PositiveIntType> noteNumber; 4166 4167 /** 4168 * The adjudication results. 4169 */ 4170 @Child(name = "adjudication", type = { 4171 AdjudicationComponent.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4172 @Description(shortDefinition = "Added items detail adjudication", formalDefinition = "The adjudication results.") 4173 protected List<AdjudicationComponent> adjudication; 4174 4175 /** 4176 * The third-tier service adjudications for payor added services. 4177 */ 4178 @Child(name = "subDetail", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4179 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The third-tier service adjudications for payor added services.") 4180 protected List<AddedItemSubDetailComponent> subDetail; 4181 4182 private static final long serialVersionUID = -1436724060L; 4183 4184 /** 4185 * Constructor 4186 */ 4187 public AddedItemDetailComponent() { 4188 super(); 4189 } 4190 4191 /** 4192 * Constructor 4193 */ 4194 public AddedItemDetailComponent(CodeableConcept productOrService) { 4195 super(); 4196 this.productOrService = productOrService; 4197 } 4198 4199 /** 4200 * @return {@link #productOrService} (When the value is a group code then this 4201 * item collects a set of related claim details, otherwise this contains 4202 * the product, service, drug or other billing code for the item.) 4203 */ 4204 public CodeableConcept getProductOrService() { 4205 if (this.productOrService == null) 4206 if (Configuration.errorOnAutoCreate()) 4207 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrService"); 4208 else if (Configuration.doAutoCreate()) 4209 this.productOrService = new CodeableConcept(); // cc 4210 return this.productOrService; 4211 } 4212 4213 public boolean hasProductOrService() { 4214 return this.productOrService != null && !this.productOrService.isEmpty(); 4215 } 4216 4217 /** 4218 * @param value {@link #productOrService} (When the value is a group code then 4219 * this item collects a set of related claim details, otherwise 4220 * this contains the product, service, drug or other billing code 4221 * for the item.) 4222 */ 4223 public AddedItemDetailComponent setProductOrService(CodeableConcept value) { 4224 this.productOrService = value; 4225 return this; 4226 } 4227 4228 /** 4229 * @return {@link #modifier} (Item typification or modifiers codes to convey 4230 * additional context for the product or service.) 4231 */ 4232 public List<CodeableConcept> getModifier() { 4233 if (this.modifier == null) 4234 this.modifier = new ArrayList<CodeableConcept>(); 4235 return this.modifier; 4236 } 4237 4238 /** 4239 * @return Returns a reference to <code>this</code> for easy method chaining 4240 */ 4241 public AddedItemDetailComponent setModifier(List<CodeableConcept> theModifier) { 4242 this.modifier = theModifier; 4243 return this; 4244 } 4245 4246 public boolean hasModifier() { 4247 if (this.modifier == null) 4248 return false; 4249 for (CodeableConcept item : this.modifier) 4250 if (!item.isEmpty()) 4251 return true; 4252 return false; 4253 } 4254 4255 public CodeableConcept addModifier() { // 3 4256 CodeableConcept t = new CodeableConcept(); 4257 if (this.modifier == null) 4258 this.modifier = new ArrayList<CodeableConcept>(); 4259 this.modifier.add(t); 4260 return t; 4261 } 4262 4263 public AddedItemDetailComponent addModifier(CodeableConcept t) { // 3 4264 if (t == null) 4265 return this; 4266 if (this.modifier == null) 4267 this.modifier = new ArrayList<CodeableConcept>(); 4268 this.modifier.add(t); 4269 return this; 4270 } 4271 4272 /** 4273 * @return The first repetition of repeating field {@link #modifier}, creating 4274 * it if it does not already exist 4275 */ 4276 public CodeableConcept getModifierFirstRep() { 4277 if (getModifier().isEmpty()) { 4278 addModifier(); 4279 } 4280 return getModifier().get(0); 4281 } 4282 4283 /** 4284 * @return {@link #quantity} (The number of repetitions of a service or 4285 * product.) 4286 */ 4287 public Quantity getQuantity() { 4288 if (this.quantity == null) 4289 if (Configuration.errorOnAutoCreate()) 4290 throw new Error("Attempt to auto-create AddedItemDetailComponent.quantity"); 4291 else if (Configuration.doAutoCreate()) 4292 this.quantity = new Quantity(); // cc 4293 return this.quantity; 4294 } 4295 4296 public boolean hasQuantity() { 4297 return this.quantity != null && !this.quantity.isEmpty(); 4298 } 4299 4300 /** 4301 * @param value {@link #quantity} (The number of repetitions of a service or 4302 * product.) 4303 */ 4304 public AddedItemDetailComponent setQuantity(Quantity value) { 4305 this.quantity = value; 4306 return this; 4307 } 4308 4309 /** 4310 * @return {@link #unitPrice} (If the item is not a group then this is the fee 4311 * for the product or service, otherwise this is the total of the fees 4312 * for the details of the group.) 4313 */ 4314 public Money getUnitPrice() { 4315 if (this.unitPrice == null) 4316 if (Configuration.errorOnAutoCreate()) 4317 throw new Error("Attempt to auto-create AddedItemDetailComponent.unitPrice"); 4318 else if (Configuration.doAutoCreate()) 4319 this.unitPrice = new Money(); // cc 4320 return this.unitPrice; 4321 } 4322 4323 public boolean hasUnitPrice() { 4324 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4325 } 4326 4327 /** 4328 * @param value {@link #unitPrice} (If the item is not a group then this is the 4329 * fee for the product or service, otherwise this is the total of 4330 * the fees for the details of the group.) 4331 */ 4332 public AddedItemDetailComponent setUnitPrice(Money value) { 4333 this.unitPrice = value; 4334 return this; 4335 } 4336 4337 /** 4338 * @return {@link #factor} (A real number that represents a multiplier used in 4339 * determining the overall value of services delivered and/or goods 4340 * received. The concept of a Factor allows for a discount or surcharge 4341 * multiplier to be applied to a monetary amount.). This is the 4342 * underlying object with id, value and extensions. The accessor 4343 * "getFactor" gives direct access to the value 4344 */ 4345 public DecimalType getFactorElement() { 4346 if (this.factor == null) 4347 if (Configuration.errorOnAutoCreate()) 4348 throw new Error("Attempt to auto-create AddedItemDetailComponent.factor"); 4349 else if (Configuration.doAutoCreate()) 4350 this.factor = new DecimalType(); // bb 4351 return this.factor; 4352 } 4353 4354 public boolean hasFactorElement() { 4355 return this.factor != null && !this.factor.isEmpty(); 4356 } 4357 4358 public boolean hasFactor() { 4359 return this.factor != null && !this.factor.isEmpty(); 4360 } 4361 4362 /** 4363 * @param value {@link #factor} (A real number that represents a multiplier used 4364 * in determining the overall value of services delivered and/or 4365 * goods received. The concept of a Factor allows for a discount or 4366 * surcharge multiplier to be applied to a monetary amount.). This 4367 * is the underlying object with id, value and extensions. The 4368 * accessor "getFactor" gives direct access to the value 4369 */ 4370 public AddedItemDetailComponent setFactorElement(DecimalType value) { 4371 this.factor = value; 4372 return this; 4373 } 4374 4375 /** 4376 * @return A real number that represents a multiplier used in determining the 4377 * overall value of services delivered and/or goods received. The 4378 * concept of a Factor allows for a discount or surcharge multiplier to 4379 * be applied to a monetary amount. 4380 */ 4381 public BigDecimal getFactor() { 4382 return this.factor == null ? null : this.factor.getValue(); 4383 } 4384 4385 /** 4386 * @param value A real number that represents a multiplier used in determining 4387 * the overall value of services delivered and/or goods received. 4388 * The concept of a Factor allows for a discount or surcharge 4389 * multiplier to be applied to a monetary amount. 4390 */ 4391 public AddedItemDetailComponent setFactor(BigDecimal value) { 4392 if (value == null) 4393 this.factor = null; 4394 else { 4395 if (this.factor == null) 4396 this.factor = new DecimalType(); 4397 this.factor.setValue(value); 4398 } 4399 return this; 4400 } 4401 4402 /** 4403 * @param value A real number that represents a multiplier used in determining 4404 * the overall value of services delivered and/or goods received. 4405 * The concept of a Factor allows for a discount or surcharge 4406 * multiplier to be applied to a monetary amount. 4407 */ 4408 public AddedItemDetailComponent setFactor(long value) { 4409 this.factor = new DecimalType(); 4410 this.factor.setValue(value); 4411 return this; 4412 } 4413 4414 /** 4415 * @param value A real number that represents a multiplier used in determining 4416 * the overall value of services delivered and/or goods received. 4417 * The concept of a Factor allows for a discount or surcharge 4418 * multiplier to be applied to a monetary amount. 4419 */ 4420 public AddedItemDetailComponent setFactor(double value) { 4421 this.factor = new DecimalType(); 4422 this.factor.setValue(value); 4423 return this; 4424 } 4425 4426 /** 4427 * @return {@link #net} (The quantity times the unit price for an additional 4428 * service or product or charge.) 4429 */ 4430 public Money getNet() { 4431 if (this.net == null) 4432 if (Configuration.errorOnAutoCreate()) 4433 throw new Error("Attempt to auto-create AddedItemDetailComponent.net"); 4434 else if (Configuration.doAutoCreate()) 4435 this.net = new Money(); // cc 4436 return this.net; 4437 } 4438 4439 public boolean hasNet() { 4440 return this.net != null && !this.net.isEmpty(); 4441 } 4442 4443 /** 4444 * @param value {@link #net} (The quantity times the unit price for an 4445 * additional service or product or charge.) 4446 */ 4447 public AddedItemDetailComponent setNet(Money value) { 4448 this.net = value; 4449 return this; 4450 } 4451 4452 /** 4453 * @return {@link #noteNumber} (The numbers associated with notes below which 4454 * apply to the adjudication of this item.) 4455 */ 4456 public List<PositiveIntType> getNoteNumber() { 4457 if (this.noteNumber == null) 4458 this.noteNumber = new ArrayList<PositiveIntType>(); 4459 return this.noteNumber; 4460 } 4461 4462 /** 4463 * @return Returns a reference to <code>this</code> for easy method chaining 4464 */ 4465 public AddedItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 4466 this.noteNumber = theNoteNumber; 4467 return this; 4468 } 4469 4470 public boolean hasNoteNumber() { 4471 if (this.noteNumber == null) 4472 return false; 4473 for (PositiveIntType item : this.noteNumber) 4474 if (!item.isEmpty()) 4475 return true; 4476 return false; 4477 } 4478 4479 /** 4480 * @return {@link #noteNumber} (The numbers associated with notes below which 4481 * apply to the adjudication of this item.) 4482 */ 4483 public PositiveIntType addNoteNumberElement() {// 2 4484 PositiveIntType t = new PositiveIntType(); 4485 if (this.noteNumber == null) 4486 this.noteNumber = new ArrayList<PositiveIntType>(); 4487 this.noteNumber.add(t); 4488 return t; 4489 } 4490 4491 /** 4492 * @param value {@link #noteNumber} (The numbers associated with notes below 4493 * which apply to the adjudication of this item.) 4494 */ 4495 public AddedItemDetailComponent addNoteNumber(int value) { // 1 4496 PositiveIntType t = new PositiveIntType(); 4497 t.setValue(value); 4498 if (this.noteNumber == null) 4499 this.noteNumber = new ArrayList<PositiveIntType>(); 4500 this.noteNumber.add(t); 4501 return this; 4502 } 4503 4504 /** 4505 * @param value {@link #noteNumber} (The numbers associated with notes below 4506 * which apply to the adjudication of this item.) 4507 */ 4508 public boolean hasNoteNumber(int value) { 4509 if (this.noteNumber == null) 4510 return false; 4511 for (PositiveIntType v : this.noteNumber) 4512 if (v.getValue().equals(value)) // positiveInt 4513 return true; 4514 return false; 4515 } 4516 4517 /** 4518 * @return {@link #adjudication} (The adjudication results.) 4519 */ 4520 public List<AdjudicationComponent> getAdjudication() { 4521 if (this.adjudication == null) 4522 this.adjudication = new ArrayList<AdjudicationComponent>(); 4523 return this.adjudication; 4524 } 4525 4526 /** 4527 * @return Returns a reference to <code>this</code> for easy method chaining 4528 */ 4529 public AddedItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 4530 this.adjudication = theAdjudication; 4531 return this; 4532 } 4533 4534 public boolean hasAdjudication() { 4535 if (this.adjudication == null) 4536 return false; 4537 for (AdjudicationComponent item : this.adjudication) 4538 if (!item.isEmpty()) 4539 return true; 4540 return false; 4541 } 4542 4543 public AdjudicationComponent addAdjudication() { // 3 4544 AdjudicationComponent t = new AdjudicationComponent(); 4545 if (this.adjudication == null) 4546 this.adjudication = new ArrayList<AdjudicationComponent>(); 4547 this.adjudication.add(t); 4548 return t; 4549 } 4550 4551 public AddedItemDetailComponent addAdjudication(AdjudicationComponent t) { // 3 4552 if (t == null) 4553 return this; 4554 if (this.adjudication == null) 4555 this.adjudication = new ArrayList<AdjudicationComponent>(); 4556 this.adjudication.add(t); 4557 return this; 4558 } 4559 4560 /** 4561 * @return The first repetition of repeating field {@link #adjudication}, 4562 * creating it if it does not already exist 4563 */ 4564 public AdjudicationComponent getAdjudicationFirstRep() { 4565 if (getAdjudication().isEmpty()) { 4566 addAdjudication(); 4567 } 4568 return getAdjudication().get(0); 4569 } 4570 4571 /** 4572 * @return {@link #subDetail} (The third-tier service adjudications for payor 4573 * added services.) 4574 */ 4575 public List<AddedItemSubDetailComponent> getSubDetail() { 4576 if (this.subDetail == null) 4577 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4578 return this.subDetail; 4579 } 4580 4581 /** 4582 * @return Returns a reference to <code>this</code> for easy method chaining 4583 */ 4584 public AddedItemDetailComponent setSubDetail(List<AddedItemSubDetailComponent> theSubDetail) { 4585 this.subDetail = theSubDetail; 4586 return this; 4587 } 4588 4589 public boolean hasSubDetail() { 4590 if (this.subDetail == null) 4591 return false; 4592 for (AddedItemSubDetailComponent item : this.subDetail) 4593 if (!item.isEmpty()) 4594 return true; 4595 return false; 4596 } 4597 4598 public AddedItemSubDetailComponent addSubDetail() { // 3 4599 AddedItemSubDetailComponent t = new AddedItemSubDetailComponent(); 4600 if (this.subDetail == null) 4601 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4602 this.subDetail.add(t); 4603 return t; 4604 } 4605 4606 public AddedItemDetailComponent addSubDetail(AddedItemSubDetailComponent t) { // 3 4607 if (t == null) 4608 return this; 4609 if (this.subDetail == null) 4610 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4611 this.subDetail.add(t); 4612 return this; 4613 } 4614 4615 /** 4616 * @return The first repetition of repeating field {@link #subDetail}, creating 4617 * it if it does not already exist 4618 */ 4619 public AddedItemSubDetailComponent getSubDetailFirstRep() { 4620 if (getSubDetail().isEmpty()) { 4621 addSubDetail(); 4622 } 4623 return getSubDetail().get(0); 4624 } 4625 4626 protected void listChildren(List<Property> children) { 4627 super.listChildren(children); 4628 children.add(new Property("productOrService", "CodeableConcept", 4629 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 4630 0, 1, productOrService)); 4631 children.add(new Property("modifier", "CodeableConcept", 4632 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 4633 java.lang.Integer.MAX_VALUE, modifier)); 4634 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 4635 1, quantity)); 4636 children.add(new Property("unitPrice", "Money", 4637 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 4638 0, 1, unitPrice)); 4639 children.add(new Property("factor", "decimal", 4640 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 4641 0, 1, factor)); 4642 children.add(new Property("net", "Money", 4643 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 4644 children.add(new Property("noteNumber", "positiveInt", 4645 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 4646 java.lang.Integer.MAX_VALUE, noteNumber)); 4647 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 4648 java.lang.Integer.MAX_VALUE, adjudication)); 4649 children.add(new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, 4650 java.lang.Integer.MAX_VALUE, subDetail)); 4651 } 4652 4653 @Override 4654 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4655 switch (_hash) { 4656 case 1957227299: 4657 /* productOrService */ return new Property("productOrService", "CodeableConcept", 4658 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 4659 0, 1, productOrService); 4660 case -615513385: 4661 /* modifier */ return new Property("modifier", "CodeableConcept", 4662 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 4663 java.lang.Integer.MAX_VALUE, modifier); 4664 case -1285004149: 4665 /* quantity */ return new Property("quantity", "SimpleQuantity", 4666 "The number of repetitions of a service or product.", 0, 1, quantity); 4667 case -486196699: 4668 /* unitPrice */ return new Property("unitPrice", "Money", 4669 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 4670 0, 1, unitPrice); 4671 case -1282148017: 4672 /* factor */ return new Property("factor", "decimal", 4673 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 4674 0, 1, factor); 4675 case 108957: 4676 /* net */ return new Property("net", "Money", 4677 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 4678 case -1110033957: 4679 /* noteNumber */ return new Property("noteNumber", "positiveInt", 4680 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 4681 java.lang.Integer.MAX_VALUE, noteNumber); 4682 case -231349275: 4683 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 4684 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 4685 case -828829007: 4686 /* subDetail */ return new Property("subDetail", "", 4687 "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, 4688 subDetail); 4689 default: 4690 return super.getNamedProperty(_hash, _name, _checkValid); 4691 } 4692 4693 } 4694 4695 @Override 4696 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4697 switch (hash) { 4698 case 1957227299: 4699 /* productOrService */ return this.productOrService == null ? new Base[0] 4700 : new Base[] { this.productOrService }; // CodeableConcept 4701 case -615513385: 4702 /* modifier */ return this.modifier == null ? new Base[0] 4703 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4704 case -1285004149: 4705 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 4706 case -486196699: 4707 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 4708 case -1282148017: 4709 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 4710 case 108957: 4711 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 4712 case -1110033957: 4713 /* noteNumber */ return this.noteNumber == null ? new Base[0] 4714 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 4715 case -231349275: 4716 /* adjudication */ return this.adjudication == null ? new Base[0] 4717 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 4718 case -828829007: 4719 /* subDetail */ return this.subDetail == null ? new Base[0] 4720 : this.subDetail.toArray(new Base[this.subDetail.size()]); // AddedItemSubDetailComponent 4721 default: 4722 return super.getProperty(hash, name, checkValid); 4723 } 4724 4725 } 4726 4727 @Override 4728 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4729 switch (hash) { 4730 case 1957227299: // productOrService 4731 this.productOrService = castToCodeableConcept(value); // CodeableConcept 4732 return value; 4733 case -615513385: // modifier 4734 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 4735 return value; 4736 case -1285004149: // quantity 4737 this.quantity = castToQuantity(value); // Quantity 4738 return value; 4739 case -486196699: // unitPrice 4740 this.unitPrice = castToMoney(value); // Money 4741 return value; 4742 case -1282148017: // factor 4743 this.factor = castToDecimal(value); // DecimalType 4744 return value; 4745 case 108957: // net 4746 this.net = castToMoney(value); // Money 4747 return value; 4748 case -1110033957: // noteNumber 4749 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 4750 return value; 4751 case -231349275: // adjudication 4752 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 4753 return value; 4754 case -828829007: // subDetail 4755 this.getSubDetail().add((AddedItemSubDetailComponent) value); // AddedItemSubDetailComponent 4756 return value; 4757 default: 4758 return super.setProperty(hash, name, value); 4759 } 4760 4761 } 4762 4763 @Override 4764 public Base setProperty(String name, Base value) throws FHIRException { 4765 if (name.equals("productOrService")) { 4766 this.productOrService = castToCodeableConcept(value); // CodeableConcept 4767 } else if (name.equals("modifier")) { 4768 this.getModifier().add(castToCodeableConcept(value)); 4769 } else if (name.equals("quantity")) { 4770 this.quantity = castToQuantity(value); // Quantity 4771 } else if (name.equals("unitPrice")) { 4772 this.unitPrice = castToMoney(value); // Money 4773 } else if (name.equals("factor")) { 4774 this.factor = castToDecimal(value); // DecimalType 4775 } else if (name.equals("net")) { 4776 this.net = castToMoney(value); // Money 4777 } else if (name.equals("noteNumber")) { 4778 this.getNoteNumber().add(castToPositiveInt(value)); 4779 } else if (name.equals("adjudication")) { 4780 this.getAdjudication().add((AdjudicationComponent) value); 4781 } else if (name.equals("subDetail")) { 4782 this.getSubDetail().add((AddedItemSubDetailComponent) value); 4783 } else 4784 return super.setProperty(name, value); 4785 return value; 4786 } 4787 4788 @Override 4789 public void removeChild(String name, Base value) throws FHIRException { 4790 if (name.equals("productOrService")) { 4791 this.productOrService = null; 4792 } else if (name.equals("modifier")) { 4793 this.getModifier().remove(castToCodeableConcept(value)); 4794 } else if (name.equals("quantity")) { 4795 this.quantity = null; 4796 } else if (name.equals("unitPrice")) { 4797 this.unitPrice = null; 4798 } else if (name.equals("factor")) { 4799 this.factor = null; 4800 } else if (name.equals("net")) { 4801 this.net = null; 4802 } else if (name.equals("noteNumber")) { 4803 this.getNoteNumber().remove(castToPositiveInt(value)); 4804 } else if (name.equals("adjudication")) { 4805 this.getAdjudication().remove((AdjudicationComponent) value); 4806 } else if (name.equals("subDetail")) { 4807 this.getSubDetail().remove((AddedItemSubDetailComponent) value); 4808 } else 4809 super.removeChild(name, value); 4810 4811 } 4812 4813 @Override 4814 public Base makeProperty(int hash, String name) throws FHIRException { 4815 switch (hash) { 4816 case 1957227299: 4817 return getProductOrService(); 4818 case -615513385: 4819 return addModifier(); 4820 case -1285004149: 4821 return getQuantity(); 4822 case -486196699: 4823 return getUnitPrice(); 4824 case -1282148017: 4825 return getFactorElement(); 4826 case 108957: 4827 return getNet(); 4828 case -1110033957: 4829 return addNoteNumberElement(); 4830 case -231349275: 4831 return addAdjudication(); 4832 case -828829007: 4833 return addSubDetail(); 4834 default: 4835 return super.makeProperty(hash, name); 4836 } 4837 4838 } 4839 4840 @Override 4841 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4842 switch (hash) { 4843 case 1957227299: 4844 /* productOrService */ return new String[] { "CodeableConcept" }; 4845 case -615513385: 4846 /* modifier */ return new String[] { "CodeableConcept" }; 4847 case -1285004149: 4848 /* quantity */ return new String[] { "SimpleQuantity" }; 4849 case -486196699: 4850 /* unitPrice */ return new String[] { "Money" }; 4851 case -1282148017: 4852 /* factor */ return new String[] { "decimal" }; 4853 case 108957: 4854 /* net */ return new String[] { "Money" }; 4855 case -1110033957: 4856 /* noteNumber */ return new String[] { "positiveInt" }; 4857 case -231349275: 4858 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 4859 case -828829007: 4860 /* subDetail */ return new String[] {}; 4861 default: 4862 return super.getTypesForProperty(hash, name); 4863 } 4864 4865 } 4866 4867 @Override 4868 public Base addChild(String name) throws FHIRException { 4869 if (name.equals("productOrService")) { 4870 this.productOrService = new CodeableConcept(); 4871 return this.productOrService; 4872 } else if (name.equals("modifier")) { 4873 return addModifier(); 4874 } else if (name.equals("quantity")) { 4875 this.quantity = new Quantity(); 4876 return this.quantity; 4877 } else if (name.equals("unitPrice")) { 4878 this.unitPrice = new Money(); 4879 return this.unitPrice; 4880 } else if (name.equals("factor")) { 4881 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.factor"); 4882 } else if (name.equals("net")) { 4883 this.net = new Money(); 4884 return this.net; 4885 } else if (name.equals("noteNumber")) { 4886 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 4887 } else if (name.equals("adjudication")) { 4888 return addAdjudication(); 4889 } else if (name.equals("subDetail")) { 4890 return addSubDetail(); 4891 } else 4892 return super.addChild(name); 4893 } 4894 4895 public AddedItemDetailComponent copy() { 4896 AddedItemDetailComponent dst = new AddedItemDetailComponent(); 4897 copyValues(dst); 4898 return dst; 4899 } 4900 4901 public void copyValues(AddedItemDetailComponent dst) { 4902 super.copyValues(dst); 4903 dst.productOrService = productOrService == null ? null : productOrService.copy(); 4904 if (modifier != null) { 4905 dst.modifier = new ArrayList<CodeableConcept>(); 4906 for (CodeableConcept i : modifier) 4907 dst.modifier.add(i.copy()); 4908 } 4909 ; 4910 dst.quantity = quantity == null ? null : quantity.copy(); 4911 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4912 dst.factor = factor == null ? null : factor.copy(); 4913 dst.net = net == null ? null : net.copy(); 4914 if (noteNumber != null) { 4915 dst.noteNumber = new ArrayList<PositiveIntType>(); 4916 for (PositiveIntType i : noteNumber) 4917 dst.noteNumber.add(i.copy()); 4918 } 4919 ; 4920 if (adjudication != null) { 4921 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4922 for (AdjudicationComponent i : adjudication) 4923 dst.adjudication.add(i.copy()); 4924 } 4925 ; 4926 if (subDetail != null) { 4927 dst.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 4928 for (AddedItemSubDetailComponent i : subDetail) 4929 dst.subDetail.add(i.copy()); 4930 } 4931 ; 4932 } 4933 4934 @Override 4935 public boolean equalsDeep(Base other_) { 4936 if (!super.equalsDeep(other_)) 4937 return false; 4938 if (!(other_ instanceof AddedItemDetailComponent)) 4939 return false; 4940 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 4941 return compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 4942 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 4943 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) 4944 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 4945 && compareDeep(subDetail, o.subDetail, true); 4946 } 4947 4948 @Override 4949 public boolean equalsShallow(Base other_) { 4950 if (!super.equalsShallow(other_)) 4951 return false; 4952 if (!(other_ instanceof AddedItemDetailComponent)) 4953 return false; 4954 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 4955 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 4956 } 4957 4958 public boolean isEmpty() { 4959 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productOrService, modifier, quantity, unitPrice, 4960 factor, net, noteNumber, adjudication, subDetail); 4961 } 4962 4963 public String fhirType() { 4964 return "ClaimResponse.addItem.detail"; 4965 4966 } 4967 4968 } 4969 4970 @Block() 4971 public static class AddedItemSubDetailComponent extends BackboneElement implements IBaseBackboneElement { 4972 /** 4973 * When the value is a group code then this item collects a set of related claim 4974 * details, otherwise this contains the product, service, drug or other billing 4975 * code for the item. 4976 */ 4977 @Child(name = "productOrService", type = { 4978 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4979 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4981 protected CodeableConcept productOrService; 4982 4983 /** 4984 * Item typification or modifiers codes to convey additional context for the 4985 * product or service. 4986 */ 4987 @Child(name = "modifier", type = { 4988 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4989 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4991 protected List<CodeableConcept> modifier; 4992 4993 /** 4994 * The number of repetitions of a service or product. 4995 */ 4996 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4997 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4998 protected Quantity quantity; 4999 5000 /** 5001 * If the item is not a group then this is the fee for the product or service, 5002 * otherwise this is the total of the fees for the details of the group. 5003 */ 5004 @Child(name = "unitPrice", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5005 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 5006 protected Money unitPrice; 5007 5008 /** 5009 * A real number that represents a multiplier used in determining the overall 5010 * value of services delivered and/or goods received. The concept of a Factor 5011 * allows for a discount or surcharge multiplier to be applied to a monetary 5012 * amount. 5013 */ 5014 @Child(name = "factor", type = { 5015 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 5016 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 5017 protected DecimalType factor; 5018 5019 /** 5020 * The quantity times the unit price for an additional service or product or 5021 * charge. 5022 */ 5023 @Child(name = "net", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 5024 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 5025 protected Money net; 5026 5027 /** 5028 * The numbers associated with notes below which apply to the adjudication of 5029 * this item. 5030 */ 5031 @Child(name = "noteNumber", type = { 5032 PositiveIntType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5033 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 5034 protected List<PositiveIntType> noteNumber; 5035 5036 /** 5037 * The adjudication results. 5038 */ 5039 @Child(name = "adjudication", type = { 5040 AdjudicationComponent.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5041 @Description(shortDefinition = "Added items detail adjudication", formalDefinition = "The adjudication results.") 5042 protected List<AdjudicationComponent> adjudication; 5043 5044 private static final long serialVersionUID = 1301363592L; 5045 5046 /** 5047 * Constructor 5048 */ 5049 public AddedItemSubDetailComponent() { 5050 super(); 5051 } 5052 5053 /** 5054 * Constructor 5055 */ 5056 public AddedItemSubDetailComponent(CodeableConcept productOrService) { 5057 super(); 5058 this.productOrService = productOrService; 5059 } 5060 5061 /** 5062 * @return {@link #productOrService} (When the value is a group code then this 5063 * item collects a set of related claim details, otherwise this contains 5064 * the product, service, drug or other billing code for the item.) 5065 */ 5066 public CodeableConcept getProductOrService() { 5067 if (this.productOrService == null) 5068 if (Configuration.errorOnAutoCreate()) 5069 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.productOrService"); 5070 else if (Configuration.doAutoCreate()) 5071 this.productOrService = new CodeableConcept(); // cc 5072 return this.productOrService; 5073 } 5074 5075 public boolean hasProductOrService() { 5076 return this.productOrService != null && !this.productOrService.isEmpty(); 5077 } 5078 5079 /** 5080 * @param value {@link #productOrService} (When the value is a group code then 5081 * this item collects a set of related claim details, otherwise 5082 * this contains the product, service, drug or other billing code 5083 * for the item.) 5084 */ 5085 public AddedItemSubDetailComponent setProductOrService(CodeableConcept value) { 5086 this.productOrService = value; 5087 return this; 5088 } 5089 5090 /** 5091 * @return {@link #modifier} (Item typification or modifiers codes to convey 5092 * additional context for the product or service.) 5093 */ 5094 public List<CodeableConcept> getModifier() { 5095 if (this.modifier == null) 5096 this.modifier = new ArrayList<CodeableConcept>(); 5097 return this.modifier; 5098 } 5099 5100 /** 5101 * @return Returns a reference to <code>this</code> for easy method chaining 5102 */ 5103 public AddedItemSubDetailComponent setModifier(List<CodeableConcept> theModifier) { 5104 this.modifier = theModifier; 5105 return this; 5106 } 5107 5108 public boolean hasModifier() { 5109 if (this.modifier == null) 5110 return false; 5111 for (CodeableConcept item : this.modifier) 5112 if (!item.isEmpty()) 5113 return true; 5114 return false; 5115 } 5116 5117 public CodeableConcept addModifier() { // 3 5118 CodeableConcept t = new CodeableConcept(); 5119 if (this.modifier == null) 5120 this.modifier = new ArrayList<CodeableConcept>(); 5121 this.modifier.add(t); 5122 return t; 5123 } 5124 5125 public AddedItemSubDetailComponent addModifier(CodeableConcept t) { // 3 5126 if (t == null) 5127 return this; 5128 if (this.modifier == null) 5129 this.modifier = new ArrayList<CodeableConcept>(); 5130 this.modifier.add(t); 5131 return this; 5132 } 5133 5134 /** 5135 * @return The first repetition of repeating field {@link #modifier}, creating 5136 * it if it does not already exist 5137 */ 5138 public CodeableConcept getModifierFirstRep() { 5139 if (getModifier().isEmpty()) { 5140 addModifier(); 5141 } 5142 return getModifier().get(0); 5143 } 5144 5145 /** 5146 * @return {@link #quantity} (The number of repetitions of a service or 5147 * product.) 5148 */ 5149 public Quantity getQuantity() { 5150 if (this.quantity == null) 5151 if (Configuration.errorOnAutoCreate()) 5152 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.quantity"); 5153 else if (Configuration.doAutoCreate()) 5154 this.quantity = new Quantity(); // cc 5155 return this.quantity; 5156 } 5157 5158 public boolean hasQuantity() { 5159 return this.quantity != null && !this.quantity.isEmpty(); 5160 } 5161 5162 /** 5163 * @param value {@link #quantity} (The number of repetitions of a service or 5164 * product.) 5165 */ 5166 public AddedItemSubDetailComponent setQuantity(Quantity value) { 5167 this.quantity = value; 5168 return this; 5169 } 5170 5171 /** 5172 * @return {@link #unitPrice} (If the item is not a group then this is the fee 5173 * for the product or service, otherwise this is the total of the fees 5174 * for the details of the group.) 5175 */ 5176 public Money getUnitPrice() { 5177 if (this.unitPrice == null) 5178 if (Configuration.errorOnAutoCreate()) 5179 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.unitPrice"); 5180 else if (Configuration.doAutoCreate()) 5181 this.unitPrice = new Money(); // cc 5182 return this.unitPrice; 5183 } 5184 5185 public boolean hasUnitPrice() { 5186 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5187 } 5188 5189 /** 5190 * @param value {@link #unitPrice} (If the item is not a group then this is the 5191 * fee for the product or service, otherwise this is the total of 5192 * the fees for the details of the group.) 5193 */ 5194 public AddedItemSubDetailComponent setUnitPrice(Money value) { 5195 this.unitPrice = value; 5196 return this; 5197 } 5198 5199 /** 5200 * @return {@link #factor} (A real number that represents a multiplier used in 5201 * determining the overall value of services delivered and/or goods 5202 * received. The concept of a Factor allows for a discount or surcharge 5203 * multiplier to be applied to a monetary amount.). This is the 5204 * underlying object with id, value and extensions. The accessor 5205 * "getFactor" gives direct access to the value 5206 */ 5207 public DecimalType getFactorElement() { 5208 if (this.factor == null) 5209 if (Configuration.errorOnAutoCreate()) 5210 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.factor"); 5211 else if (Configuration.doAutoCreate()) 5212 this.factor = new DecimalType(); // bb 5213 return this.factor; 5214 } 5215 5216 public boolean hasFactorElement() { 5217 return this.factor != null && !this.factor.isEmpty(); 5218 } 5219 5220 public boolean hasFactor() { 5221 return this.factor != null && !this.factor.isEmpty(); 5222 } 5223 5224 /** 5225 * @param value {@link #factor} (A real number that represents a multiplier used 5226 * in determining the overall value of services delivered and/or 5227 * goods received. The concept of a Factor allows for a discount or 5228 * surcharge multiplier to be applied to a monetary amount.). This 5229 * is the underlying object with id, value and extensions. The 5230 * accessor "getFactor" gives direct access to the value 5231 */ 5232 public AddedItemSubDetailComponent setFactorElement(DecimalType value) { 5233 this.factor = value; 5234 return this; 5235 } 5236 5237 /** 5238 * @return A real number that represents a multiplier used in determining the 5239 * overall value of services delivered and/or goods received. The 5240 * concept of a Factor allows for a discount or surcharge multiplier to 5241 * be applied to a monetary amount. 5242 */ 5243 public BigDecimal getFactor() { 5244 return this.factor == null ? null : this.factor.getValue(); 5245 } 5246 5247 /** 5248 * @param value A real number that represents a multiplier used in determining 5249 * the overall value of services delivered and/or goods received. 5250 * The concept of a Factor allows for a discount or surcharge 5251 * multiplier to be applied to a monetary amount. 5252 */ 5253 public AddedItemSubDetailComponent setFactor(BigDecimal value) { 5254 if (value == null) 5255 this.factor = null; 5256 else { 5257 if (this.factor == null) 5258 this.factor = new DecimalType(); 5259 this.factor.setValue(value); 5260 } 5261 return this; 5262 } 5263 5264 /** 5265 * @param value A real number that represents a multiplier used in determining 5266 * the overall value of services delivered and/or goods received. 5267 * The concept of a Factor allows for a discount or surcharge 5268 * multiplier to be applied to a monetary amount. 5269 */ 5270 public AddedItemSubDetailComponent setFactor(long value) { 5271 this.factor = new DecimalType(); 5272 this.factor.setValue(value); 5273 return this; 5274 } 5275 5276 /** 5277 * @param value A real number that represents a multiplier used in determining 5278 * the overall value of services delivered and/or goods received. 5279 * The concept of a Factor allows for a discount or surcharge 5280 * multiplier to be applied to a monetary amount. 5281 */ 5282 public AddedItemSubDetailComponent setFactor(double value) { 5283 this.factor = new DecimalType(); 5284 this.factor.setValue(value); 5285 return this; 5286 } 5287 5288 /** 5289 * @return {@link #net} (The quantity times the unit price for an additional 5290 * service or product or charge.) 5291 */ 5292 public Money getNet() { 5293 if (this.net == null) 5294 if (Configuration.errorOnAutoCreate()) 5295 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.net"); 5296 else if (Configuration.doAutoCreate()) 5297 this.net = new Money(); // cc 5298 return this.net; 5299 } 5300 5301 public boolean hasNet() { 5302 return this.net != null && !this.net.isEmpty(); 5303 } 5304 5305 /** 5306 * @param value {@link #net} (The quantity times the unit price for an 5307 * additional service or product or charge.) 5308 */ 5309 public AddedItemSubDetailComponent setNet(Money value) { 5310 this.net = value; 5311 return this; 5312 } 5313 5314 /** 5315 * @return {@link #noteNumber} (The numbers associated with notes below which 5316 * apply to the adjudication of this item.) 5317 */ 5318 public List<PositiveIntType> getNoteNumber() { 5319 if (this.noteNumber == null) 5320 this.noteNumber = new ArrayList<PositiveIntType>(); 5321 return this.noteNumber; 5322 } 5323 5324 /** 5325 * @return Returns a reference to <code>this</code> for easy method chaining 5326 */ 5327 public AddedItemSubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 5328 this.noteNumber = theNoteNumber; 5329 return this; 5330 } 5331 5332 public boolean hasNoteNumber() { 5333 if (this.noteNumber == null) 5334 return false; 5335 for (PositiveIntType item : this.noteNumber) 5336 if (!item.isEmpty()) 5337 return true; 5338 return false; 5339 } 5340 5341 /** 5342 * @return {@link #noteNumber} (The numbers associated with notes below which 5343 * apply to the adjudication of this item.) 5344 */ 5345 public PositiveIntType addNoteNumberElement() {// 2 5346 PositiveIntType t = new PositiveIntType(); 5347 if (this.noteNumber == null) 5348 this.noteNumber = new ArrayList<PositiveIntType>(); 5349 this.noteNumber.add(t); 5350 return t; 5351 } 5352 5353 /** 5354 * @param value {@link #noteNumber} (The numbers associated with notes below 5355 * which apply to the adjudication of this item.) 5356 */ 5357 public AddedItemSubDetailComponent addNoteNumber(int value) { // 1 5358 PositiveIntType t = new PositiveIntType(); 5359 t.setValue(value); 5360 if (this.noteNumber == null) 5361 this.noteNumber = new ArrayList<PositiveIntType>(); 5362 this.noteNumber.add(t); 5363 return this; 5364 } 5365 5366 /** 5367 * @param value {@link #noteNumber} (The numbers associated with notes below 5368 * which apply to the adjudication of this item.) 5369 */ 5370 public boolean hasNoteNumber(int value) { 5371 if (this.noteNumber == null) 5372 return false; 5373 for (PositiveIntType v : this.noteNumber) 5374 if (v.getValue().equals(value)) // positiveInt 5375 return true; 5376 return false; 5377 } 5378 5379 /** 5380 * @return {@link #adjudication} (The adjudication results.) 5381 */ 5382 public List<AdjudicationComponent> getAdjudication() { 5383 if (this.adjudication == null) 5384 this.adjudication = new ArrayList<AdjudicationComponent>(); 5385 return this.adjudication; 5386 } 5387 5388 /** 5389 * @return Returns a reference to <code>this</code> for easy method chaining 5390 */ 5391 public AddedItemSubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 5392 this.adjudication = theAdjudication; 5393 return this; 5394 } 5395 5396 public boolean hasAdjudication() { 5397 if (this.adjudication == null) 5398 return false; 5399 for (AdjudicationComponent item : this.adjudication) 5400 if (!item.isEmpty()) 5401 return true; 5402 return false; 5403 } 5404 5405 public AdjudicationComponent addAdjudication() { // 3 5406 AdjudicationComponent t = new AdjudicationComponent(); 5407 if (this.adjudication == null) 5408 this.adjudication = new ArrayList<AdjudicationComponent>(); 5409 this.adjudication.add(t); 5410 return t; 5411 } 5412 5413 public AddedItemSubDetailComponent addAdjudication(AdjudicationComponent t) { // 3 5414 if (t == null) 5415 return this; 5416 if (this.adjudication == null) 5417 this.adjudication = new ArrayList<AdjudicationComponent>(); 5418 this.adjudication.add(t); 5419 return this; 5420 } 5421 5422 /** 5423 * @return The first repetition of repeating field {@link #adjudication}, 5424 * creating it if it does not already exist 5425 */ 5426 public AdjudicationComponent getAdjudicationFirstRep() { 5427 if (getAdjudication().isEmpty()) { 5428 addAdjudication(); 5429 } 5430 return getAdjudication().get(0); 5431 } 5432 5433 protected void listChildren(List<Property> children) { 5434 super.listChildren(children); 5435 children.add(new Property("productOrService", "CodeableConcept", 5436 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5437 0, 1, productOrService)); 5438 children.add(new Property("modifier", "CodeableConcept", 5439 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5440 java.lang.Integer.MAX_VALUE, modifier)); 5441 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 5442 1, quantity)); 5443 children.add(new Property("unitPrice", "Money", 5444 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5445 0, 1, unitPrice)); 5446 children.add(new Property("factor", "decimal", 5447 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5448 0, 1, factor)); 5449 children.add(new Property("net", "Money", 5450 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 5451 children.add(new Property("noteNumber", "positiveInt", 5452 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 5453 java.lang.Integer.MAX_VALUE, noteNumber)); 5454 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, 5455 java.lang.Integer.MAX_VALUE, adjudication)); 5456 } 5457 5458 @Override 5459 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5460 switch (_hash) { 5461 case 1957227299: 5462 /* productOrService */ return new Property("productOrService", "CodeableConcept", 5463 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5464 0, 1, productOrService); 5465 case -615513385: 5466 /* modifier */ return new Property("modifier", "CodeableConcept", 5467 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5468 java.lang.Integer.MAX_VALUE, modifier); 5469 case -1285004149: 5470 /* quantity */ return new Property("quantity", "SimpleQuantity", 5471 "The number of repetitions of a service or product.", 0, 1, quantity); 5472 case -486196699: 5473 /* unitPrice */ return new Property("unitPrice", "Money", 5474 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5475 0, 1, unitPrice); 5476 case -1282148017: 5477 /* factor */ return new Property("factor", "decimal", 5478 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5479 0, 1, factor); 5480 case 108957: 5481 /* net */ return new Property("net", "Money", 5482 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 5483 case -1110033957: 5484 /* noteNumber */ return new Property("noteNumber", "positiveInt", 5485 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 5486 java.lang.Integer.MAX_VALUE, noteNumber); 5487 case -231349275: 5488 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 5489 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 5490 default: 5491 return super.getNamedProperty(_hash, _name, _checkValid); 5492 } 5493 5494 } 5495 5496 @Override 5497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5498 switch (hash) { 5499 case 1957227299: 5500 /* productOrService */ return this.productOrService == null ? new Base[0] 5501 : new Base[] { this.productOrService }; // CodeableConcept 5502 case -615513385: 5503 /* modifier */ return this.modifier == null ? new Base[0] 5504 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5505 case -1285004149: 5506 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5507 case -486196699: 5508 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 5509 case -1282148017: 5510 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 5511 case 108957: 5512 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 5513 case -1110033957: 5514 /* noteNumber */ return this.noteNumber == null ? new Base[0] 5515 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5516 case -231349275: 5517 /* adjudication */ return this.adjudication == null ? new Base[0] 5518 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5519 default: 5520 return super.getProperty(hash, name, checkValid); 5521 } 5522 5523 } 5524 5525 @Override 5526 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5527 switch (hash) { 5528 case 1957227299: // productOrService 5529 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5530 return value; 5531 case -615513385: // modifier 5532 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5533 return value; 5534 case -1285004149: // quantity 5535 this.quantity = castToQuantity(value); // Quantity 5536 return value; 5537 case -486196699: // unitPrice 5538 this.unitPrice = castToMoney(value); // Money 5539 return value; 5540 case -1282148017: // factor 5541 this.factor = castToDecimal(value); // DecimalType 5542 return value; 5543 case 108957: // net 5544 this.net = castToMoney(value); // Money 5545 return value; 5546 case -1110033957: // noteNumber 5547 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 5548 return value; 5549 case -231349275: // adjudication 5550 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5551 return value; 5552 default: 5553 return super.setProperty(hash, name, value); 5554 } 5555 5556 } 5557 5558 @Override 5559 public Base setProperty(String name, Base value) throws FHIRException { 5560 if (name.equals("productOrService")) { 5561 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5562 } else if (name.equals("modifier")) { 5563 this.getModifier().add(castToCodeableConcept(value)); 5564 } else if (name.equals("quantity")) { 5565 this.quantity = castToQuantity(value); // Quantity 5566 } else if (name.equals("unitPrice")) { 5567 this.unitPrice = castToMoney(value); // Money 5568 } else if (name.equals("factor")) { 5569 this.factor = castToDecimal(value); // DecimalType 5570 } else if (name.equals("net")) { 5571 this.net = castToMoney(value); // Money 5572 } else if (name.equals("noteNumber")) { 5573 this.getNoteNumber().add(castToPositiveInt(value)); 5574 } else if (name.equals("adjudication")) { 5575 this.getAdjudication().add((AdjudicationComponent) value); 5576 } else 5577 return super.setProperty(name, value); 5578 return value; 5579 } 5580 5581 @Override 5582 public void removeChild(String name, Base value) throws FHIRException { 5583 if (name.equals("productOrService")) { 5584 this.productOrService = null; 5585 } else if (name.equals("modifier")) { 5586 this.getModifier().remove(castToCodeableConcept(value)); 5587 } else if (name.equals("quantity")) { 5588 this.quantity = null; 5589 } else if (name.equals("unitPrice")) { 5590 this.unitPrice = null; 5591 } else if (name.equals("factor")) { 5592 this.factor = null; 5593 } else if (name.equals("net")) { 5594 this.net = null; 5595 } else if (name.equals("noteNumber")) { 5596 this.getNoteNumber().remove(castToPositiveInt(value)); 5597 } else if (name.equals("adjudication")) { 5598 this.getAdjudication().remove((AdjudicationComponent) value); 5599 } else 5600 super.removeChild(name, value); 5601 5602 } 5603 5604 @Override 5605 public Base makeProperty(int hash, String name) throws FHIRException { 5606 switch (hash) { 5607 case 1957227299: 5608 return getProductOrService(); 5609 case -615513385: 5610 return addModifier(); 5611 case -1285004149: 5612 return getQuantity(); 5613 case -486196699: 5614 return getUnitPrice(); 5615 case -1282148017: 5616 return getFactorElement(); 5617 case 108957: 5618 return getNet(); 5619 case -1110033957: 5620 return addNoteNumberElement(); 5621 case -231349275: 5622 return addAdjudication(); 5623 default: 5624 return super.makeProperty(hash, name); 5625 } 5626 5627 } 5628 5629 @Override 5630 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5631 switch (hash) { 5632 case 1957227299: 5633 /* productOrService */ return new String[] { "CodeableConcept" }; 5634 case -615513385: 5635 /* modifier */ return new String[] { "CodeableConcept" }; 5636 case -1285004149: 5637 /* quantity */ return new String[] { "SimpleQuantity" }; 5638 case -486196699: 5639 /* unitPrice */ return new String[] { "Money" }; 5640 case -1282148017: 5641 /* factor */ return new String[] { "decimal" }; 5642 case 108957: 5643 /* net */ return new String[] { "Money" }; 5644 case -1110033957: 5645 /* noteNumber */ return new String[] { "positiveInt" }; 5646 case -231349275: 5647 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 5648 default: 5649 return super.getTypesForProperty(hash, name); 5650 } 5651 5652 } 5653 5654 @Override 5655 public Base addChild(String name) throws FHIRException { 5656 if (name.equals("productOrService")) { 5657 this.productOrService = new CodeableConcept(); 5658 return this.productOrService; 5659 } else if (name.equals("modifier")) { 5660 return addModifier(); 5661 } else if (name.equals("quantity")) { 5662 this.quantity = new Quantity(); 5663 return this.quantity; 5664 } else if (name.equals("unitPrice")) { 5665 this.unitPrice = new Money(); 5666 return this.unitPrice; 5667 } else if (name.equals("factor")) { 5668 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.factor"); 5669 } else if (name.equals("net")) { 5670 this.net = new Money(); 5671 return this.net; 5672 } else if (name.equals("noteNumber")) { 5673 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 5674 } else if (name.equals("adjudication")) { 5675 return addAdjudication(); 5676 } else 5677 return super.addChild(name); 5678 } 5679 5680 public AddedItemSubDetailComponent copy() { 5681 AddedItemSubDetailComponent dst = new AddedItemSubDetailComponent(); 5682 copyValues(dst); 5683 return dst; 5684 } 5685 5686 public void copyValues(AddedItemSubDetailComponent dst) { 5687 super.copyValues(dst); 5688 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5689 if (modifier != null) { 5690 dst.modifier = new ArrayList<CodeableConcept>(); 5691 for (CodeableConcept i : modifier) 5692 dst.modifier.add(i.copy()); 5693 } 5694 ; 5695 dst.quantity = quantity == null ? null : quantity.copy(); 5696 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5697 dst.factor = factor == null ? null : factor.copy(); 5698 dst.net = net == null ? null : net.copy(); 5699 if (noteNumber != null) { 5700 dst.noteNumber = new ArrayList<PositiveIntType>(); 5701 for (PositiveIntType i : noteNumber) 5702 dst.noteNumber.add(i.copy()); 5703 } 5704 ; 5705 if (adjudication != null) { 5706 dst.adjudication = new ArrayList<AdjudicationComponent>(); 5707 for (AdjudicationComponent i : adjudication) 5708 dst.adjudication.add(i.copy()); 5709 } 5710 ; 5711 } 5712 5713 @Override 5714 public boolean equalsDeep(Base other_) { 5715 if (!super.equalsDeep(other_)) 5716 return false; 5717 if (!(other_ instanceof AddedItemSubDetailComponent)) 5718 return false; 5719 AddedItemSubDetailComponent o = (AddedItemSubDetailComponent) other_; 5720 return compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 5721 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 5722 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) 5723 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true); 5724 } 5725 5726 @Override 5727 public boolean equalsShallow(Base other_) { 5728 if (!super.equalsShallow(other_)) 5729 return false; 5730 if (!(other_ instanceof AddedItemSubDetailComponent)) 5731 return false; 5732 AddedItemSubDetailComponent o = (AddedItemSubDetailComponent) other_; 5733 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 5734 } 5735 5736 public boolean isEmpty() { 5737 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productOrService, modifier, quantity, unitPrice, 5738 factor, net, noteNumber, adjudication); 5739 } 5740 5741 public String fhirType() { 5742 return "ClaimResponse.addItem.detail.subDetail"; 5743 5744 } 5745 5746 } 5747 5748 @Block() 5749 public static class TotalComponent extends BackboneElement implements IBaseBackboneElement { 5750 /** 5751 * A code to indicate the information type of this adjudication record. 5752 * Information types may include: the value submitted, maximum values or 5753 * percentages allowed or payable under the plan, amounts that the patient is 5754 * responsible for in aggregate or pertaining to this item, amounts paid by 5755 * other coverages, and the benefit payable for this item. 5756 */ 5757 @Child(name = "category", type = { 5758 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 5759 @Description(shortDefinition = "Type of adjudication information", formalDefinition = "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.") 5760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication") 5761 protected CodeableConcept category; 5762 5763 /** 5764 * Monetary total amount associated with the category. 5765 */ 5766 @Child(name = "amount", type = { Money.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 5767 @Description(shortDefinition = "Financial total for the category", formalDefinition = "Monetary total amount associated with the category.") 5768 protected Money amount; 5769 5770 private static final long serialVersionUID = 2012310309L; 5771 5772 /** 5773 * Constructor 5774 */ 5775 public TotalComponent() { 5776 super(); 5777 } 5778 5779 /** 5780 * Constructor 5781 */ 5782 public TotalComponent(CodeableConcept category, Money amount) { 5783 super(); 5784 this.category = category; 5785 this.amount = amount; 5786 } 5787 5788 /** 5789 * @return {@link #category} (A code to indicate the information type of this 5790 * adjudication record. Information types may include: the value 5791 * submitted, maximum values or percentages allowed or payable under the 5792 * plan, amounts that the patient is responsible for in aggregate or 5793 * pertaining to this item, amounts paid by other coverages, and the 5794 * benefit payable for this item.) 5795 */ 5796 public CodeableConcept getCategory() { 5797 if (this.category == null) 5798 if (Configuration.errorOnAutoCreate()) 5799 throw new Error("Attempt to auto-create TotalComponent.category"); 5800 else if (Configuration.doAutoCreate()) 5801 this.category = new CodeableConcept(); // cc 5802 return this.category; 5803 } 5804 5805 public boolean hasCategory() { 5806 return this.category != null && !this.category.isEmpty(); 5807 } 5808 5809 /** 5810 * @param value {@link #category} (A code to indicate the information type of 5811 * this adjudication record. Information types may include: the 5812 * value submitted, maximum values or percentages allowed or 5813 * payable under the plan, amounts that the patient is responsible 5814 * for in aggregate or pertaining to this item, amounts paid by 5815 * other coverages, and the benefit payable for this item.) 5816 */ 5817 public TotalComponent setCategory(CodeableConcept value) { 5818 this.category = value; 5819 return this; 5820 } 5821 5822 /** 5823 * @return {@link #amount} (Monetary total amount associated with the category.) 5824 */ 5825 public Money getAmount() { 5826 if (this.amount == null) 5827 if (Configuration.errorOnAutoCreate()) 5828 throw new Error("Attempt to auto-create TotalComponent.amount"); 5829 else if (Configuration.doAutoCreate()) 5830 this.amount = new Money(); // cc 5831 return this.amount; 5832 } 5833 5834 public boolean hasAmount() { 5835 return this.amount != null && !this.amount.isEmpty(); 5836 } 5837 5838 /** 5839 * @param value {@link #amount} (Monetary total amount associated with the 5840 * category.) 5841 */ 5842 public TotalComponent setAmount(Money value) { 5843 this.amount = value; 5844 return this; 5845 } 5846 5847 protected void listChildren(List<Property> children) { 5848 super.listChildren(children); 5849 children.add(new Property("category", "CodeableConcept", 5850 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 5851 0, 1, category)); 5852 children 5853 .add(new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount)); 5854 } 5855 5856 @Override 5857 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5858 switch (_hash) { 5859 case 50511102: 5860 /* category */ return new Property("category", "CodeableConcept", 5861 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 5862 0, 1, category); 5863 case -1413853096: 5864 /* amount */ return new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, 5865 amount); 5866 default: 5867 return super.getNamedProperty(_hash, _name, _checkValid); 5868 } 5869 5870 } 5871 5872 @Override 5873 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5874 switch (hash) { 5875 case 50511102: 5876 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 5877 case -1413853096: 5878 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 5879 default: 5880 return super.getProperty(hash, name, checkValid); 5881 } 5882 5883 } 5884 5885 @Override 5886 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5887 switch (hash) { 5888 case 50511102: // category 5889 this.category = castToCodeableConcept(value); // CodeableConcept 5890 return value; 5891 case -1413853096: // amount 5892 this.amount = castToMoney(value); // Money 5893 return value; 5894 default: 5895 return super.setProperty(hash, name, value); 5896 } 5897 5898 } 5899 5900 @Override 5901 public Base setProperty(String name, Base value) throws FHIRException { 5902 if (name.equals("category")) { 5903 this.category = castToCodeableConcept(value); // CodeableConcept 5904 } else if (name.equals("amount")) { 5905 this.amount = castToMoney(value); // Money 5906 } else 5907 return super.setProperty(name, value); 5908 return value; 5909 } 5910 5911 @Override 5912 public void removeChild(String name, Base value) throws FHIRException { 5913 if (name.equals("category")) { 5914 this.category = null; 5915 } else if (name.equals("amount")) { 5916 this.amount = null; 5917 } else 5918 super.removeChild(name, value); 5919 5920 } 5921 5922 @Override 5923 public Base makeProperty(int hash, String name) throws FHIRException { 5924 switch (hash) { 5925 case 50511102: 5926 return getCategory(); 5927 case -1413853096: 5928 return getAmount(); 5929 default: 5930 return super.makeProperty(hash, name); 5931 } 5932 5933 } 5934 5935 @Override 5936 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5937 switch (hash) { 5938 case 50511102: 5939 /* category */ return new String[] { "CodeableConcept" }; 5940 case -1413853096: 5941 /* amount */ return new String[] { "Money" }; 5942 default: 5943 return super.getTypesForProperty(hash, name); 5944 } 5945 5946 } 5947 5948 @Override 5949 public Base addChild(String name) throws FHIRException { 5950 if (name.equals("category")) { 5951 this.category = new CodeableConcept(); 5952 return this.category; 5953 } else if (name.equals("amount")) { 5954 this.amount = new Money(); 5955 return this.amount; 5956 } else 5957 return super.addChild(name); 5958 } 5959 5960 public TotalComponent copy() { 5961 TotalComponent dst = new TotalComponent(); 5962 copyValues(dst); 5963 return dst; 5964 } 5965 5966 public void copyValues(TotalComponent dst) { 5967 super.copyValues(dst); 5968 dst.category = category == null ? null : category.copy(); 5969 dst.amount = amount == null ? null : amount.copy(); 5970 } 5971 5972 @Override 5973 public boolean equalsDeep(Base other_) { 5974 if (!super.equalsDeep(other_)) 5975 return false; 5976 if (!(other_ instanceof TotalComponent)) 5977 return false; 5978 TotalComponent o = (TotalComponent) other_; 5979 return compareDeep(category, o.category, true) && compareDeep(amount, o.amount, true); 5980 } 5981 5982 @Override 5983 public boolean equalsShallow(Base other_) { 5984 if (!super.equalsShallow(other_)) 5985 return false; 5986 if (!(other_ instanceof TotalComponent)) 5987 return false; 5988 TotalComponent o = (TotalComponent) other_; 5989 return true; 5990 } 5991 5992 public boolean isEmpty() { 5993 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, amount); 5994 } 5995 5996 public String fhirType() { 5997 return "ClaimResponse.total"; 5998 5999 } 6000 6001 } 6002 6003 @Block() 6004 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 6005 /** 6006 * Whether this represents partial or complete payment of the benefits payable. 6007 */ 6008 @Child(name = "type", type = { 6009 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6010 @Description(shortDefinition = "Partial or complete payment", formalDefinition = "Whether this represents partial or complete payment of the benefits payable.") 6011 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-paymenttype") 6012 protected CodeableConcept type; 6013 6014 /** 6015 * Total amount of all adjustments to this payment included in this transaction 6016 * which are not related to this claim's adjudication. 6017 */ 6018 @Child(name = "adjustment", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6019 @Description(shortDefinition = "Payment adjustment for non-claim issues", formalDefinition = "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.") 6020 protected Money adjustment; 6021 6022 /** 6023 * Reason for the payment adjustment. 6024 */ 6025 @Child(name = "adjustmentReason", type = { 6026 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6027 @Description(shortDefinition = "Explanation for the adjustment", formalDefinition = "Reason for the payment adjustment.") 6028 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 6029 protected CodeableConcept adjustmentReason; 6030 6031 /** 6032 * Estimated date the payment will be issued or the actual issue date of 6033 * payment. 6034 */ 6035 @Child(name = "date", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6036 @Description(shortDefinition = "Expected date of payment", formalDefinition = "Estimated date the payment will be issued or the actual issue date of payment.") 6037 protected DateType date; 6038 6039 /** 6040 * Benefits payable less any payment adjustment. 6041 */ 6042 @Child(name = "amount", type = { Money.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 6043 @Description(shortDefinition = "Payable amount after adjustment", formalDefinition = "Benefits payable less any payment adjustment.") 6044 protected Money amount; 6045 6046 /** 6047 * Issuer's unique identifier for the payment instrument. 6048 */ 6049 @Child(name = "identifier", type = { 6050 Identifier.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6051 @Description(shortDefinition = "Business identifier for the payment", formalDefinition = "Issuer's unique identifier for the payment instrument.") 6052 protected Identifier identifier; 6053 6054 private static final long serialVersionUID = 1539906026L; 6055 6056 /** 6057 * Constructor 6058 */ 6059 public PaymentComponent() { 6060 super(); 6061 } 6062 6063 /** 6064 * Constructor 6065 */ 6066 public PaymentComponent(CodeableConcept type, Money amount) { 6067 super(); 6068 this.type = type; 6069 this.amount = amount; 6070 } 6071 6072 /** 6073 * @return {@link #type} (Whether this represents partial or complete payment of 6074 * the benefits payable.) 6075 */ 6076 public CodeableConcept getType() { 6077 if (this.type == null) 6078 if (Configuration.errorOnAutoCreate()) 6079 throw new Error("Attempt to auto-create PaymentComponent.type"); 6080 else if (Configuration.doAutoCreate()) 6081 this.type = new CodeableConcept(); // cc 6082 return this.type; 6083 } 6084 6085 public boolean hasType() { 6086 return this.type != null && !this.type.isEmpty(); 6087 } 6088 6089 /** 6090 * @param value {@link #type} (Whether this represents partial or complete 6091 * payment of the benefits payable.) 6092 */ 6093 public PaymentComponent setType(CodeableConcept value) { 6094 this.type = value; 6095 return this; 6096 } 6097 6098 /** 6099 * @return {@link #adjustment} (Total amount of all adjustments to this payment 6100 * included in this transaction which are not related to this claim's 6101 * adjudication.) 6102 */ 6103 public Money getAdjustment() { 6104 if (this.adjustment == null) 6105 if (Configuration.errorOnAutoCreate()) 6106 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 6107 else if (Configuration.doAutoCreate()) 6108 this.adjustment = new Money(); // cc 6109 return this.adjustment; 6110 } 6111 6112 public boolean hasAdjustment() { 6113 return this.adjustment != null && !this.adjustment.isEmpty(); 6114 } 6115 6116 /** 6117 * @param value {@link #adjustment} (Total amount of all adjustments to this 6118 * payment included in this transaction which are not related to 6119 * this claim's adjudication.) 6120 */ 6121 public PaymentComponent setAdjustment(Money value) { 6122 this.adjustment = value; 6123 return this; 6124 } 6125 6126 /** 6127 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 6128 */ 6129 public CodeableConcept getAdjustmentReason() { 6130 if (this.adjustmentReason == null) 6131 if (Configuration.errorOnAutoCreate()) 6132 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 6133 else if (Configuration.doAutoCreate()) 6134 this.adjustmentReason = new CodeableConcept(); // cc 6135 return this.adjustmentReason; 6136 } 6137 6138 public boolean hasAdjustmentReason() { 6139 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 6140 } 6141 6142 /** 6143 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 6144 */ 6145 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 6146 this.adjustmentReason = value; 6147 return this; 6148 } 6149 6150 /** 6151 * @return {@link #date} (Estimated date the payment will be issued or the 6152 * actual issue date of payment.). This is the underlying object with 6153 * id, value and extensions. The accessor "getDate" gives direct access 6154 * to the value 6155 */ 6156 public DateType getDateElement() { 6157 if (this.date == null) 6158 if (Configuration.errorOnAutoCreate()) 6159 throw new Error("Attempt to auto-create PaymentComponent.date"); 6160 else if (Configuration.doAutoCreate()) 6161 this.date = new DateType(); // bb 6162 return this.date; 6163 } 6164 6165 public boolean hasDateElement() { 6166 return this.date != null && !this.date.isEmpty(); 6167 } 6168 6169 public boolean hasDate() { 6170 return this.date != null && !this.date.isEmpty(); 6171 } 6172 6173 /** 6174 * @param value {@link #date} (Estimated date the payment will be issued or the 6175 * actual issue date of payment.). This is the underlying object 6176 * with id, value and extensions. The accessor "getDate" gives 6177 * direct access to the value 6178 */ 6179 public PaymentComponent setDateElement(DateType value) { 6180 this.date = value; 6181 return this; 6182 } 6183 6184 /** 6185 * @return Estimated date the payment will be issued or the actual issue date of 6186 * payment. 6187 */ 6188 public Date getDate() { 6189 return this.date == null ? null : this.date.getValue(); 6190 } 6191 6192 /** 6193 * @param value Estimated date the payment will be issued or the actual issue 6194 * date of payment. 6195 */ 6196 public PaymentComponent setDate(Date value) { 6197 if (value == null) 6198 this.date = null; 6199 else { 6200 if (this.date == null) 6201 this.date = new DateType(); 6202 this.date.setValue(value); 6203 } 6204 return this; 6205 } 6206 6207 /** 6208 * @return {@link #amount} (Benefits payable less any payment adjustment.) 6209 */ 6210 public Money getAmount() { 6211 if (this.amount == null) 6212 if (Configuration.errorOnAutoCreate()) 6213 throw new Error("Attempt to auto-create PaymentComponent.amount"); 6214 else if (Configuration.doAutoCreate()) 6215 this.amount = new Money(); // cc 6216 return this.amount; 6217 } 6218 6219 public boolean hasAmount() { 6220 return this.amount != null && !this.amount.isEmpty(); 6221 } 6222 6223 /** 6224 * @param value {@link #amount} (Benefits payable less any payment adjustment.) 6225 */ 6226 public PaymentComponent setAmount(Money value) { 6227 this.amount = value; 6228 return this; 6229 } 6230 6231 /** 6232 * @return {@link #identifier} (Issuer's unique identifier for the payment 6233 * instrument.) 6234 */ 6235 public Identifier getIdentifier() { 6236 if (this.identifier == null) 6237 if (Configuration.errorOnAutoCreate()) 6238 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 6239 else if (Configuration.doAutoCreate()) 6240 this.identifier = new Identifier(); // cc 6241 return this.identifier; 6242 } 6243 6244 public boolean hasIdentifier() { 6245 return this.identifier != null && !this.identifier.isEmpty(); 6246 } 6247 6248 /** 6249 * @param value {@link #identifier} (Issuer's unique identifier for the payment 6250 * instrument.) 6251 */ 6252 public PaymentComponent setIdentifier(Identifier value) { 6253 this.identifier = value; 6254 return this; 6255 } 6256 6257 protected void listChildren(List<Property> children) { 6258 super.listChildren(children); 6259 children.add(new Property("type", "CodeableConcept", 6260 "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type)); 6261 children.add(new Property("adjustment", "Money", 6262 "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 6263 0, 1, adjustment)); 6264 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, 6265 adjustmentReason)); 6266 children.add(new Property("date", "date", 6267 "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date)); 6268 children.add(new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount)); 6269 children.add(new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 6270 1, identifier)); 6271 } 6272 6273 @Override 6274 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6275 switch (_hash) { 6276 case 3575610: 6277 /* type */ return new Property("type", "CodeableConcept", 6278 "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type); 6279 case 1977085293: 6280 /* adjustment */ return new Property("adjustment", "Money", 6281 "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 6282 0, 1, adjustment); 6283 case -1255938543: 6284 /* adjustmentReason */ return new Property("adjustmentReason", "CodeableConcept", 6285 "Reason for the payment adjustment.", 0, 1, adjustmentReason); 6286 case 3076014: 6287 /* date */ return new Property("date", "date", 6288 "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date); 6289 case -1413853096: 6290 /* amount */ return new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, 6291 amount); 6292 case -1618432855: 6293 /* identifier */ return new Property("identifier", "Identifier", 6294 "Issuer's unique identifier for the payment instrument.", 0, 1, identifier); 6295 default: 6296 return super.getNamedProperty(_hash, _name, _checkValid); 6297 } 6298 6299 } 6300 6301 @Override 6302 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6303 switch (hash) { 6304 case 3575610: 6305 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 6306 case 1977085293: 6307 /* adjustment */ return this.adjustment == null ? new Base[0] : new Base[] { this.adjustment }; // Money 6308 case -1255938543: 6309 /* adjustmentReason */ return this.adjustmentReason == null ? new Base[0] 6310 : new Base[] { this.adjustmentReason }; // CodeableConcept 6311 case 3076014: 6312 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 6313 case -1413853096: 6314 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 6315 case -1618432855: 6316 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 6317 default: 6318 return super.getProperty(hash, name, checkValid); 6319 } 6320 6321 } 6322 6323 @Override 6324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6325 switch (hash) { 6326 case 3575610: // type 6327 this.type = castToCodeableConcept(value); // CodeableConcept 6328 return value; 6329 case 1977085293: // adjustment 6330 this.adjustment = castToMoney(value); // Money 6331 return value; 6332 case -1255938543: // adjustmentReason 6333 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 6334 return value; 6335 case 3076014: // date 6336 this.date = castToDate(value); // DateType 6337 return value; 6338 case -1413853096: // amount 6339 this.amount = castToMoney(value); // Money 6340 return value; 6341 case -1618432855: // identifier 6342 this.identifier = castToIdentifier(value); // Identifier 6343 return value; 6344 default: 6345 return super.setProperty(hash, name, value); 6346 } 6347 6348 } 6349 6350 @Override 6351 public Base setProperty(String name, Base value) throws FHIRException { 6352 if (name.equals("type")) { 6353 this.type = castToCodeableConcept(value); // CodeableConcept 6354 } else if (name.equals("adjustment")) { 6355 this.adjustment = castToMoney(value); // Money 6356 } else if (name.equals("adjustmentReason")) { 6357 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 6358 } else if (name.equals("date")) { 6359 this.date = castToDate(value); // DateType 6360 } else if (name.equals("amount")) { 6361 this.amount = castToMoney(value); // Money 6362 } else if (name.equals("identifier")) { 6363 this.identifier = castToIdentifier(value); // Identifier 6364 } else 6365 return super.setProperty(name, value); 6366 return value; 6367 } 6368 6369 @Override 6370 public void removeChild(String name, Base value) throws FHIRException { 6371 if (name.equals("type")) { 6372 this.type = null; 6373 } else if (name.equals("adjustment")) { 6374 this.adjustment = null; 6375 } else if (name.equals("adjustmentReason")) { 6376 this.adjustmentReason = null; 6377 } else if (name.equals("date")) { 6378 this.date = null; 6379 } else if (name.equals("amount")) { 6380 this.amount = null; 6381 } else if (name.equals("identifier")) { 6382 this.identifier = null; 6383 } else 6384 super.removeChild(name, value); 6385 6386 } 6387 6388 @Override 6389 public Base makeProperty(int hash, String name) throws FHIRException { 6390 switch (hash) { 6391 case 3575610: 6392 return getType(); 6393 case 1977085293: 6394 return getAdjustment(); 6395 case -1255938543: 6396 return getAdjustmentReason(); 6397 case 3076014: 6398 return getDateElement(); 6399 case -1413853096: 6400 return getAmount(); 6401 case -1618432855: 6402 return getIdentifier(); 6403 default: 6404 return super.makeProperty(hash, name); 6405 } 6406 6407 } 6408 6409 @Override 6410 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6411 switch (hash) { 6412 case 3575610: 6413 /* type */ return new String[] { "CodeableConcept" }; 6414 case 1977085293: 6415 /* adjustment */ return new String[] { "Money" }; 6416 case -1255938543: 6417 /* adjustmentReason */ return new String[] { "CodeableConcept" }; 6418 case 3076014: 6419 /* date */ return new String[] { "date" }; 6420 case -1413853096: 6421 /* amount */ return new String[] { "Money" }; 6422 case -1618432855: 6423 /* identifier */ return new String[] { "Identifier" }; 6424 default: 6425 return super.getTypesForProperty(hash, name); 6426 } 6427 6428 } 6429 6430 @Override 6431 public Base addChild(String name) throws FHIRException { 6432 if (name.equals("type")) { 6433 this.type = new CodeableConcept(); 6434 return this.type; 6435 } else if (name.equals("adjustment")) { 6436 this.adjustment = new Money(); 6437 return this.adjustment; 6438 } else if (name.equals("adjustmentReason")) { 6439 this.adjustmentReason = new CodeableConcept(); 6440 return this.adjustmentReason; 6441 } else if (name.equals("date")) { 6442 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.date"); 6443 } else if (name.equals("amount")) { 6444 this.amount = new Money(); 6445 return this.amount; 6446 } else if (name.equals("identifier")) { 6447 this.identifier = new Identifier(); 6448 return this.identifier; 6449 } else 6450 return super.addChild(name); 6451 } 6452 6453 public PaymentComponent copy() { 6454 PaymentComponent dst = new PaymentComponent(); 6455 copyValues(dst); 6456 return dst; 6457 } 6458 6459 public void copyValues(PaymentComponent dst) { 6460 super.copyValues(dst); 6461 dst.type = type == null ? null : type.copy(); 6462 dst.adjustment = adjustment == null ? null : adjustment.copy(); 6463 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 6464 dst.date = date == null ? null : date.copy(); 6465 dst.amount = amount == null ? null : amount.copy(); 6466 dst.identifier = identifier == null ? null : identifier.copy(); 6467 } 6468 6469 @Override 6470 public boolean equalsDeep(Base other_) { 6471 if (!super.equalsDeep(other_)) 6472 return false; 6473 if (!(other_ instanceof PaymentComponent)) 6474 return false; 6475 PaymentComponent o = (PaymentComponent) other_; 6476 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) 6477 && compareDeep(adjustmentReason, o.adjustmentReason, true) && compareDeep(date, o.date, true) 6478 && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true); 6479 } 6480 6481 @Override 6482 public boolean equalsShallow(Base other_) { 6483 if (!super.equalsShallow(other_)) 6484 return false; 6485 if (!(other_ instanceof PaymentComponent)) 6486 return false; 6487 PaymentComponent o = (PaymentComponent) other_; 6488 return compareValues(date, o.date, true); 6489 } 6490 6491 public boolean isEmpty() { 6492 return super.isEmpty() 6493 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason, date, amount, identifier); 6494 } 6495 6496 public String fhirType() { 6497 return "ClaimResponse.payment"; 6498 6499 } 6500 6501 } 6502 6503 @Block() 6504 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 6505 /** 6506 * A number to uniquely identify a note entry. 6507 */ 6508 @Child(name = "number", type = { 6509 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6510 @Description(shortDefinition = "Note instance identifier", formalDefinition = "A number to uniquely identify a note entry.") 6511 protected PositiveIntType number; 6512 6513 /** 6514 * The business purpose of the note text. 6515 */ 6516 @Child(name = "type", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6517 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The business purpose of the note text.") 6518 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/note-type") 6519 protected Enumeration<NoteType> type; 6520 6521 /** 6522 * The explanation or description associated with the processing. 6523 */ 6524 @Child(name = "text", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 6525 @Description(shortDefinition = "Note explanatory text", formalDefinition = "The explanation or description associated with the processing.") 6526 protected StringType text; 6527 6528 /** 6529 * A code to define the language used in the text of the note. 6530 */ 6531 @Child(name = "language", type = { 6532 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6533 @Description(shortDefinition = "Language of the text", formalDefinition = "A code to define the language used in the text of the note.") 6534 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 6535 protected CodeableConcept language; 6536 6537 private static final long serialVersionUID = -385184277L; 6538 6539 /** 6540 * Constructor 6541 */ 6542 public NoteComponent() { 6543 super(); 6544 } 6545 6546 /** 6547 * Constructor 6548 */ 6549 public NoteComponent(StringType text) { 6550 super(); 6551 this.text = text; 6552 } 6553 6554 /** 6555 * @return {@link #number} (A number to uniquely identify a note entry.). This 6556 * is the underlying object with id, value and extensions. The accessor 6557 * "getNumber" gives direct access to the value 6558 */ 6559 public PositiveIntType getNumberElement() { 6560 if (this.number == null) 6561 if (Configuration.errorOnAutoCreate()) 6562 throw new Error("Attempt to auto-create NoteComponent.number"); 6563 else if (Configuration.doAutoCreate()) 6564 this.number = new PositiveIntType(); // bb 6565 return this.number; 6566 } 6567 6568 public boolean hasNumberElement() { 6569 return this.number != null && !this.number.isEmpty(); 6570 } 6571 6572 public boolean hasNumber() { 6573 return this.number != null && !this.number.isEmpty(); 6574 } 6575 6576 /** 6577 * @param value {@link #number} (A number to uniquely identify a note entry.). 6578 * This is the underlying object with id, value and extensions. The 6579 * accessor "getNumber" gives direct access to the value 6580 */ 6581 public NoteComponent setNumberElement(PositiveIntType value) { 6582 this.number = value; 6583 return this; 6584 } 6585 6586 /** 6587 * @return A number to uniquely identify a note entry. 6588 */ 6589 public int getNumber() { 6590 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 6591 } 6592 6593 /** 6594 * @param value A number to uniquely identify a note entry. 6595 */ 6596 public NoteComponent setNumber(int value) { 6597 if (this.number == null) 6598 this.number = new PositiveIntType(); 6599 this.number.setValue(value); 6600 return this; 6601 } 6602 6603 /** 6604 * @return {@link #type} (The business purpose of the note text.). This is the 6605 * underlying object with id, value and extensions. The accessor 6606 * "getType" gives direct access to the value 6607 */ 6608 public Enumeration<NoteType> getTypeElement() { 6609 if (this.type == null) 6610 if (Configuration.errorOnAutoCreate()) 6611 throw new Error("Attempt to auto-create NoteComponent.type"); 6612 else if (Configuration.doAutoCreate()) 6613 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); // bb 6614 return this.type; 6615 } 6616 6617 public boolean hasTypeElement() { 6618 return this.type != null && !this.type.isEmpty(); 6619 } 6620 6621 public boolean hasType() { 6622 return this.type != null && !this.type.isEmpty(); 6623 } 6624 6625 /** 6626 * @param value {@link #type} (The business purpose of the note text.). This is 6627 * the underlying object with id, value and extensions. The 6628 * accessor "getType" gives direct access to the value 6629 */ 6630 public NoteComponent setTypeElement(Enumeration<NoteType> value) { 6631 this.type = value; 6632 return this; 6633 } 6634 6635 /** 6636 * @return The business purpose of the note text. 6637 */ 6638 public NoteType getType() { 6639 return this.type == null ? null : this.type.getValue(); 6640 } 6641 6642 /** 6643 * @param value The business purpose of the note text. 6644 */ 6645 public NoteComponent setType(NoteType value) { 6646 if (value == null) 6647 this.type = null; 6648 else { 6649 if (this.type == null) 6650 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); 6651 this.type.setValue(value); 6652 } 6653 return this; 6654 } 6655 6656 /** 6657 * @return {@link #text} (The explanation or description associated with the 6658 * processing.). This is the underlying object with id, value and 6659 * extensions. The accessor "getText" gives direct access to the value 6660 */ 6661 public StringType getTextElement() { 6662 if (this.text == null) 6663 if (Configuration.errorOnAutoCreate()) 6664 throw new Error("Attempt to auto-create NoteComponent.text"); 6665 else if (Configuration.doAutoCreate()) 6666 this.text = new StringType(); // bb 6667 return this.text; 6668 } 6669 6670 public boolean hasTextElement() { 6671 return this.text != null && !this.text.isEmpty(); 6672 } 6673 6674 public boolean hasText() { 6675 return this.text != null && !this.text.isEmpty(); 6676 } 6677 6678 /** 6679 * @param value {@link #text} (The explanation or description associated with 6680 * the processing.). This is the underlying object with id, value 6681 * and extensions. The accessor "getText" gives direct access to 6682 * the value 6683 */ 6684 public NoteComponent setTextElement(StringType value) { 6685 this.text = value; 6686 return this; 6687 } 6688 6689 /** 6690 * @return The explanation or description associated with the processing. 6691 */ 6692 public String getText() { 6693 return this.text == null ? null : this.text.getValue(); 6694 } 6695 6696 /** 6697 * @param value The explanation or description associated with the processing. 6698 */ 6699 public NoteComponent setText(String value) { 6700 if (this.text == null) 6701 this.text = new StringType(); 6702 this.text.setValue(value); 6703 return this; 6704 } 6705 6706 /** 6707 * @return {@link #language} (A code to define the language used in the text of 6708 * the note.) 6709 */ 6710 public CodeableConcept getLanguage() { 6711 if (this.language == null) 6712 if (Configuration.errorOnAutoCreate()) 6713 throw new Error("Attempt to auto-create NoteComponent.language"); 6714 else if (Configuration.doAutoCreate()) 6715 this.language = new CodeableConcept(); // cc 6716 return this.language; 6717 } 6718 6719 public boolean hasLanguage() { 6720 return this.language != null && !this.language.isEmpty(); 6721 } 6722 6723 /** 6724 * @param value {@link #language} (A code to define the language used in the 6725 * text of the note.) 6726 */ 6727 public NoteComponent setLanguage(CodeableConcept value) { 6728 this.language = value; 6729 return this; 6730 } 6731 6732 protected void listChildren(List<Property> children) { 6733 super.listChildren(children); 6734 children.add(new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number)); 6735 children.add(new Property("type", "code", "The business purpose of the note text.", 0, 1, type)); 6736 children.add( 6737 new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 6738 children.add(new Property("language", "CodeableConcept", 6739 "A code to define the language used in the text of the note.", 0, 1, language)); 6740 } 6741 6742 @Override 6743 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6744 switch (_hash) { 6745 case -1034364087: 6746 /* number */ return new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, 6747 number); 6748 case 3575610: 6749 /* type */ return new Property("type", "code", "The business purpose of the note text.", 0, 1, type); 6750 case 3556653: 6751 /* text */ return new Property("text", "string", 6752 "The explanation or description associated with the processing.", 0, 1, text); 6753 case -1613589672: 6754 /* language */ return new Property("language", "CodeableConcept", 6755 "A code to define the language used in the text of the note.", 0, 1, language); 6756 default: 6757 return super.getNamedProperty(_hash, _name, _checkValid); 6758 } 6759 6760 } 6761 6762 @Override 6763 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6764 switch (hash) { 6765 case -1034364087: 6766 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // PositiveIntType 6767 case 3575610: 6768 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<NoteType> 6769 case 3556653: 6770 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 6771 case -1613589672: 6772 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 6773 default: 6774 return super.getProperty(hash, name, checkValid); 6775 } 6776 6777 } 6778 6779 @Override 6780 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6781 switch (hash) { 6782 case -1034364087: // number 6783 this.number = castToPositiveInt(value); // PositiveIntType 6784 return value; 6785 case 3575610: // type 6786 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 6787 this.type = (Enumeration) value; // Enumeration<NoteType> 6788 return value; 6789 case 3556653: // text 6790 this.text = castToString(value); // StringType 6791 return value; 6792 case -1613589672: // language 6793 this.language = castToCodeableConcept(value); // CodeableConcept 6794 return value; 6795 default: 6796 return super.setProperty(hash, name, value); 6797 } 6798 6799 } 6800 6801 @Override 6802 public Base setProperty(String name, Base value) throws FHIRException { 6803 if (name.equals("number")) { 6804 this.number = castToPositiveInt(value); // PositiveIntType 6805 } else if (name.equals("type")) { 6806 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 6807 this.type = (Enumeration) value; // Enumeration<NoteType> 6808 } else if (name.equals("text")) { 6809 this.text = castToString(value); // StringType 6810 } else if (name.equals("language")) { 6811 this.language = castToCodeableConcept(value); // CodeableConcept 6812 } else 6813 return super.setProperty(name, value); 6814 return value; 6815 } 6816 6817 @Override 6818 public void removeChild(String name, Base value) throws FHIRException { 6819 if (name.equals("number")) { 6820 this.number = null; 6821 } else if (name.equals("type")) { 6822 this.type = null; 6823 } else if (name.equals("text")) { 6824 this.text = null; 6825 } else if (name.equals("language")) { 6826 this.language = null; 6827 } else 6828 super.removeChild(name, value); 6829 6830 } 6831 6832 @Override 6833 public Base makeProperty(int hash, String name) throws FHIRException { 6834 switch (hash) { 6835 case -1034364087: 6836 return getNumberElement(); 6837 case 3575610: 6838 return getTypeElement(); 6839 case 3556653: 6840 return getTextElement(); 6841 case -1613589672: 6842 return getLanguage(); 6843 default: 6844 return super.makeProperty(hash, name); 6845 } 6846 6847 } 6848 6849 @Override 6850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6851 switch (hash) { 6852 case -1034364087: 6853 /* number */ return new String[] { "positiveInt" }; 6854 case 3575610: 6855 /* type */ return new String[] { "code" }; 6856 case 3556653: 6857 /* text */ return new String[] { "string" }; 6858 case -1613589672: 6859 /* language */ return new String[] { "CodeableConcept" }; 6860 default: 6861 return super.getTypesForProperty(hash, name); 6862 } 6863 6864 } 6865 6866 @Override 6867 public Base addChild(String name) throws FHIRException { 6868 if (name.equals("number")) { 6869 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.number"); 6870 } else if (name.equals("type")) { 6871 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.type"); 6872 } else if (name.equals("text")) { 6873 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.text"); 6874 } else if (name.equals("language")) { 6875 this.language = new CodeableConcept(); 6876 return this.language; 6877 } else 6878 return super.addChild(name); 6879 } 6880 6881 public NoteComponent copy() { 6882 NoteComponent dst = new NoteComponent(); 6883 copyValues(dst); 6884 return dst; 6885 } 6886 6887 public void copyValues(NoteComponent dst) { 6888 super.copyValues(dst); 6889 dst.number = number == null ? null : number.copy(); 6890 dst.type = type == null ? null : type.copy(); 6891 dst.text = text == null ? null : text.copy(); 6892 dst.language = language == null ? null : language.copy(); 6893 } 6894 6895 @Override 6896 public boolean equalsDeep(Base other_) { 6897 if (!super.equalsDeep(other_)) 6898 return false; 6899 if (!(other_ instanceof NoteComponent)) 6900 return false; 6901 NoteComponent o = (NoteComponent) other_; 6902 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 6903 && compareDeep(language, o.language, true); 6904 } 6905 6906 @Override 6907 public boolean equalsShallow(Base other_) { 6908 if (!super.equalsShallow(other_)) 6909 return false; 6910 if (!(other_ instanceof NoteComponent)) 6911 return false; 6912 NoteComponent o = (NoteComponent) other_; 6913 return compareValues(number, o.number, true) && compareValues(type, o.type, true) 6914 && compareValues(text, o.text, true); 6915 } 6916 6917 public boolean isEmpty() { 6918 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language); 6919 } 6920 6921 public String fhirType() { 6922 return "ClaimResponse.processNote"; 6923 6924 } 6925 6926 } 6927 6928 @Block() 6929 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 6930 /** 6931 * A number to uniquely identify insurance entries and provide a sequence of 6932 * coverages to convey coordination of benefit order. 6933 */ 6934 @Child(name = "sequence", type = { 6935 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6936 @Description(shortDefinition = "Insurance instance identifier", formalDefinition = "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.") 6937 protected PositiveIntType sequence; 6938 6939 /** 6940 * A flag to indicate that this Coverage is to be used for adjudication of this 6941 * claim when set to true. 6942 */ 6943 @Child(name = "focal", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 6944 @Description(shortDefinition = "Coverage to be used for adjudication", formalDefinition = "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.") 6945 protected BooleanType focal; 6946 6947 /** 6948 * Reference to the insurance card level information contained in the Coverage 6949 * resource. The coverage issuing insurer will use these details to locate the 6950 * patient's actual coverage within the insurer's information system. 6951 */ 6952 @Child(name = "coverage", type = { Coverage.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 6953 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 6954 protected Reference coverage; 6955 6956 /** 6957 * The actual object that is the target of the reference (Reference to the 6958 * insurance card level information contained in the Coverage resource. The 6959 * coverage issuing insurer will use these details to locate the patient's 6960 * actual coverage within the insurer's information system.) 6961 */ 6962 protected Coverage coverageTarget; 6963 6964 /** 6965 * A business agreement number established between the provider and the insurer 6966 * for special business processing purposes. 6967 */ 6968 @Child(name = "businessArrangement", type = { 6969 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6970 @Description(shortDefinition = "Additional provider contract number", formalDefinition = "A business agreement number established between the provider and the insurer for special business processing purposes.") 6971 protected StringType businessArrangement; 6972 6973 /** 6974 * The result of the adjudication of the line items for the Coverage specified 6975 * in this insurance. 6976 */ 6977 @Child(name = "claimResponse", type = { 6978 ClaimResponse.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6979 @Description(shortDefinition = "Adjudication results", formalDefinition = "The result of the adjudication of the line items for the Coverage specified in this insurance.") 6980 protected Reference claimResponse; 6981 6982 /** 6983 * The actual object that is the target of the reference (The result of the 6984 * adjudication of the line items for the Coverage specified in this insurance.) 6985 */ 6986 protected ClaimResponse claimResponseTarget; 6987 6988 private static final long serialVersionUID = 282380584L; 6989 6990 /** 6991 * Constructor 6992 */ 6993 public InsuranceComponent() { 6994 super(); 6995 } 6996 6997 /** 6998 * Constructor 6999 */ 7000 public InsuranceComponent(PositiveIntType sequence, BooleanType focal, Reference coverage) { 7001 super(); 7002 this.sequence = sequence; 7003 this.focal = focal; 7004 this.coverage = coverage; 7005 } 7006 7007 /** 7008 * @return {@link #sequence} (A number to uniquely identify insurance entries 7009 * and provide a sequence of coverages to convey coordination of benefit 7010 * order.). This is the underlying object with id, value and extensions. 7011 * The accessor "getSequence" gives direct access to the value 7012 */ 7013 public PositiveIntType getSequenceElement() { 7014 if (this.sequence == null) 7015 if (Configuration.errorOnAutoCreate()) 7016 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 7017 else if (Configuration.doAutoCreate()) 7018 this.sequence = new PositiveIntType(); // bb 7019 return this.sequence; 7020 } 7021 7022 public boolean hasSequenceElement() { 7023 return this.sequence != null && !this.sequence.isEmpty(); 7024 } 7025 7026 public boolean hasSequence() { 7027 return this.sequence != null && !this.sequence.isEmpty(); 7028 } 7029 7030 /** 7031 * @param value {@link #sequence} (A number to uniquely identify insurance 7032 * entries and provide a sequence of coverages to convey 7033 * coordination of benefit order.). This is the underlying object 7034 * with id, value and extensions. The accessor "getSequence" gives 7035 * direct access to the value 7036 */ 7037 public InsuranceComponent setSequenceElement(PositiveIntType value) { 7038 this.sequence = value; 7039 return this; 7040 } 7041 7042 /** 7043 * @return A number to uniquely identify insurance entries and provide a 7044 * sequence of coverages to convey coordination of benefit order. 7045 */ 7046 public int getSequence() { 7047 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7048 } 7049 7050 /** 7051 * @param value A number to uniquely identify insurance entries and provide a 7052 * sequence of coverages to convey coordination of benefit order. 7053 */ 7054 public InsuranceComponent setSequence(int value) { 7055 if (this.sequence == null) 7056 this.sequence = new PositiveIntType(); 7057 this.sequence.setValue(value); 7058 return this; 7059 } 7060 7061 /** 7062 * @return {@link #focal} (A flag to indicate that this Coverage is to be used 7063 * for adjudication of this claim when set to true.). This is the 7064 * underlying object with id, value and extensions. The accessor 7065 * "getFocal" gives direct access to the value 7066 */ 7067 public BooleanType getFocalElement() { 7068 if (this.focal == null) 7069 if (Configuration.errorOnAutoCreate()) 7070 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 7071 else if (Configuration.doAutoCreate()) 7072 this.focal = new BooleanType(); // bb 7073 return this.focal; 7074 } 7075 7076 public boolean hasFocalElement() { 7077 return this.focal != null && !this.focal.isEmpty(); 7078 } 7079 7080 public boolean hasFocal() { 7081 return this.focal != null && !this.focal.isEmpty(); 7082 } 7083 7084 /** 7085 * @param value {@link #focal} (A flag to indicate that this Coverage is to be 7086 * used for adjudication of this claim when set to true.). This is 7087 * the underlying object with id, value and extensions. The 7088 * accessor "getFocal" gives direct access to the value 7089 */ 7090 public InsuranceComponent setFocalElement(BooleanType value) { 7091 this.focal = value; 7092 return this; 7093 } 7094 7095 /** 7096 * @return A flag to indicate that this Coverage is to be used for adjudication 7097 * of this claim when set to true. 7098 */ 7099 public boolean getFocal() { 7100 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 7101 } 7102 7103 /** 7104 * @param value A flag to indicate that this Coverage is to be used for 7105 * adjudication of this claim when set to true. 7106 */ 7107 public InsuranceComponent setFocal(boolean value) { 7108 if (this.focal == null) 7109 this.focal = new BooleanType(); 7110 this.focal.setValue(value); 7111 return this; 7112 } 7113 7114 /** 7115 * @return {@link #coverage} (Reference to the insurance card level information 7116 * contained in the Coverage resource. The coverage issuing insurer will 7117 * use these details to locate the patient's actual coverage within the 7118 * insurer's information system.) 7119 */ 7120 public Reference getCoverage() { 7121 if (this.coverage == null) 7122 if (Configuration.errorOnAutoCreate()) 7123 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 7124 else if (Configuration.doAutoCreate()) 7125 this.coverage = new Reference(); // cc 7126 return this.coverage; 7127 } 7128 7129 public boolean hasCoverage() { 7130 return this.coverage != null && !this.coverage.isEmpty(); 7131 } 7132 7133 /** 7134 * @param value {@link #coverage} (Reference to the insurance card level 7135 * information contained in the Coverage resource. The coverage 7136 * issuing insurer will use these details to locate the patient's 7137 * actual coverage within the insurer's information system.) 7138 */ 7139 public InsuranceComponent setCoverage(Reference value) { 7140 this.coverage = value; 7141 return this; 7142 } 7143 7144 /** 7145 * @return {@link #coverage} The actual object that is the target of the 7146 * reference. The reference library doesn't populate this, but you can 7147 * use it to hold the resource if you resolve it. (Reference to the 7148 * insurance card level information contained in the Coverage resource. 7149 * The coverage issuing insurer will use these details to locate the 7150 * patient's actual coverage within the insurer's information system.) 7151 */ 7152 public Coverage getCoverageTarget() { 7153 if (this.coverageTarget == null) 7154 if (Configuration.errorOnAutoCreate()) 7155 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 7156 else if (Configuration.doAutoCreate()) 7157 this.coverageTarget = new Coverage(); // aa 7158 return this.coverageTarget; 7159 } 7160 7161 /** 7162 * @param value {@link #coverage} The actual object that is the target of the 7163 * reference. The reference library doesn't use these, but you can 7164 * use it to hold the resource if you resolve it. (Reference to the 7165 * insurance card level information contained in the Coverage 7166 * resource. The coverage issuing insurer will use these details to 7167 * locate the patient's actual coverage within the insurer's 7168 * information system.) 7169 */ 7170 public InsuranceComponent setCoverageTarget(Coverage value) { 7171 this.coverageTarget = value; 7172 return this; 7173 } 7174 7175 /** 7176 * @return {@link #businessArrangement} (A business agreement number established 7177 * between the provider and the insurer for special business processing 7178 * purposes.). This is the underlying object with id, value and 7179 * extensions. The accessor "getBusinessArrangement" gives direct access 7180 * to the value 7181 */ 7182 public StringType getBusinessArrangementElement() { 7183 if (this.businessArrangement == null) 7184 if (Configuration.errorOnAutoCreate()) 7185 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 7186 else if (Configuration.doAutoCreate()) 7187 this.businessArrangement = new StringType(); // bb 7188 return this.businessArrangement; 7189 } 7190 7191 public boolean hasBusinessArrangementElement() { 7192 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 7193 } 7194 7195 public boolean hasBusinessArrangement() { 7196 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 7197 } 7198 7199 /** 7200 * @param value {@link #businessArrangement} (A business agreement number 7201 * established between the provider and the insurer for special 7202 * business processing purposes.). This is the underlying object 7203 * with id, value and extensions. The accessor 7204 * "getBusinessArrangement" gives direct access to the value 7205 */ 7206 public InsuranceComponent setBusinessArrangementElement(StringType value) { 7207 this.businessArrangement = value; 7208 return this; 7209 } 7210 7211 /** 7212 * @return A business agreement number established between the provider and the 7213 * insurer for special business processing purposes. 7214 */ 7215 public String getBusinessArrangement() { 7216 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 7217 } 7218 7219 /** 7220 * @param value A business agreement number established between the provider and 7221 * the insurer for special business processing purposes. 7222 */ 7223 public InsuranceComponent setBusinessArrangement(String value) { 7224 if (Utilities.noString(value)) 7225 this.businessArrangement = null; 7226 else { 7227 if (this.businessArrangement == null) 7228 this.businessArrangement = new StringType(); 7229 this.businessArrangement.setValue(value); 7230 } 7231 return this; 7232 } 7233 7234 /** 7235 * @return {@link #claimResponse} (The result of the adjudication of the line 7236 * items for the Coverage specified in this insurance.) 7237 */ 7238 public Reference getClaimResponse() { 7239 if (this.claimResponse == null) 7240 if (Configuration.errorOnAutoCreate()) 7241 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 7242 else if (Configuration.doAutoCreate()) 7243 this.claimResponse = new Reference(); // cc 7244 return this.claimResponse; 7245 } 7246 7247 public boolean hasClaimResponse() { 7248 return this.claimResponse != null && !this.claimResponse.isEmpty(); 7249 } 7250 7251 /** 7252 * @param value {@link #claimResponse} (The result of the adjudication of the 7253 * line items for the Coverage specified in this insurance.) 7254 */ 7255 public InsuranceComponent setClaimResponse(Reference value) { 7256 this.claimResponse = value; 7257 return this; 7258 } 7259 7260 /** 7261 * @return {@link #claimResponse} The actual object that is the target of the 7262 * reference. The reference library doesn't populate this, but you can 7263 * use it to hold the resource if you resolve it. (The result of the 7264 * adjudication of the line items for the Coverage specified in this 7265 * insurance.) 7266 */ 7267 public ClaimResponse getClaimResponseTarget() { 7268 if (this.claimResponseTarget == null) 7269 if (Configuration.errorOnAutoCreate()) 7270 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 7271 else if (Configuration.doAutoCreate()) 7272 this.claimResponseTarget = new ClaimResponse(); // aa 7273 return this.claimResponseTarget; 7274 } 7275 7276 /** 7277 * @param value {@link #claimResponse} The actual object that is the target of 7278 * the reference. The reference library doesn't use these, but you 7279 * can use it to hold the resource if you resolve it. (The result 7280 * of the adjudication of the line items for the Coverage specified 7281 * in this insurance.) 7282 */ 7283 public InsuranceComponent setClaimResponseTarget(ClaimResponse value) { 7284 this.claimResponseTarget = value; 7285 return this; 7286 } 7287 7288 protected void listChildren(List<Property> children) { 7289 super.listChildren(children); 7290 children.add(new Property("sequence", "positiveInt", 7291 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 7292 0, 1, sequence)); 7293 children.add(new Property("focal", "boolean", 7294 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, 7295 focal)); 7296 children.add(new Property("coverage", "Reference(Coverage)", 7297 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 7298 0, 1, coverage)); 7299 children.add(new Property("businessArrangement", "string", 7300 "A business agreement number established between the provider and the insurer for special business processing purposes.", 7301 0, 1, businessArrangement)); 7302 children.add(new Property("claimResponse", "Reference(ClaimResponse)", 7303 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 7304 claimResponse)); 7305 } 7306 7307 @Override 7308 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7309 switch (_hash) { 7310 case 1349547969: 7311 /* sequence */ return new Property("sequence", "positiveInt", 7312 "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 7313 0, 1, sequence); 7314 case 97604197: 7315 /* focal */ return new Property("focal", "boolean", 7316 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 7317 1, focal); 7318 case -351767064: 7319 /* coverage */ return new Property("coverage", "Reference(Coverage)", 7320 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 7321 0, 1, coverage); 7322 case 259920682: 7323 /* businessArrangement */ return new Property("businessArrangement", "string", 7324 "A business agreement number established between the provider and the insurer for special business processing purposes.", 7325 0, 1, businessArrangement); 7326 case 689513629: 7327 /* claimResponse */ return new Property("claimResponse", "Reference(ClaimResponse)", 7328 "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, 7329 claimResponse); 7330 default: 7331 return super.getNamedProperty(_hash, _name, _checkValid); 7332 } 7333 7334 } 7335 7336 @Override 7337 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7338 switch (hash) { 7339 case 1349547969: 7340 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 7341 case 97604197: 7342 /* focal */ return this.focal == null ? new Base[0] : new Base[] { this.focal }; // BooleanType 7343 case -351767064: 7344 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 7345 case 259920682: 7346 /* businessArrangement */ return this.businessArrangement == null ? new Base[0] 7347 : new Base[] { this.businessArrangement }; // StringType 7348 case 689513629: 7349 /* claimResponse */ return this.claimResponse == null ? new Base[0] : new Base[] { this.claimResponse }; // Reference 7350 default: 7351 return super.getProperty(hash, name, checkValid); 7352 } 7353 7354 } 7355 7356 @Override 7357 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7358 switch (hash) { 7359 case 1349547969: // sequence 7360 this.sequence = castToPositiveInt(value); // PositiveIntType 7361 return value; 7362 case 97604197: // focal 7363 this.focal = castToBoolean(value); // BooleanType 7364 return value; 7365 case -351767064: // coverage 7366 this.coverage = castToReference(value); // Reference 7367 return value; 7368 case 259920682: // businessArrangement 7369 this.businessArrangement = castToString(value); // StringType 7370 return value; 7371 case 689513629: // claimResponse 7372 this.claimResponse = castToReference(value); // Reference 7373 return value; 7374 default: 7375 return super.setProperty(hash, name, value); 7376 } 7377 7378 } 7379 7380 @Override 7381 public Base setProperty(String name, Base value) throws FHIRException { 7382 if (name.equals("sequence")) { 7383 this.sequence = castToPositiveInt(value); // PositiveIntType 7384 } else if (name.equals("focal")) { 7385 this.focal = castToBoolean(value); // BooleanType 7386 } else if (name.equals("coverage")) { 7387 this.coverage = castToReference(value); // Reference 7388 } else if (name.equals("businessArrangement")) { 7389 this.businessArrangement = castToString(value); // StringType 7390 } else if (name.equals("claimResponse")) { 7391 this.claimResponse = castToReference(value); // Reference 7392 } else 7393 return super.setProperty(name, value); 7394 return value; 7395 } 7396 7397 @Override 7398 public void removeChild(String name, Base value) throws FHIRException { 7399 if (name.equals("sequence")) { 7400 this.sequence = null; 7401 } else if (name.equals("focal")) { 7402 this.focal = null; 7403 } else if (name.equals("coverage")) { 7404 this.coverage = null; 7405 } else if (name.equals("businessArrangement")) { 7406 this.businessArrangement = null; 7407 } else if (name.equals("claimResponse")) { 7408 this.claimResponse = null; 7409 } else 7410 super.removeChild(name, value); 7411 7412 } 7413 7414 @Override 7415 public Base makeProperty(int hash, String name) throws FHIRException { 7416 switch (hash) { 7417 case 1349547969: 7418 return getSequenceElement(); 7419 case 97604197: 7420 return getFocalElement(); 7421 case -351767064: 7422 return getCoverage(); 7423 case 259920682: 7424 return getBusinessArrangementElement(); 7425 case 689513629: 7426 return getClaimResponse(); 7427 default: 7428 return super.makeProperty(hash, name); 7429 } 7430 7431 } 7432 7433 @Override 7434 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7435 switch (hash) { 7436 case 1349547969: 7437 /* sequence */ return new String[] { "positiveInt" }; 7438 case 97604197: 7439 /* focal */ return new String[] { "boolean" }; 7440 case -351767064: 7441 /* coverage */ return new String[] { "Reference" }; 7442 case 259920682: 7443 /* businessArrangement */ return new String[] { "string" }; 7444 case 689513629: 7445 /* claimResponse */ return new String[] { "Reference" }; 7446 default: 7447 return super.getTypesForProperty(hash, name); 7448 } 7449 7450 } 7451 7452 @Override 7453 public Base addChild(String name) throws FHIRException { 7454 if (name.equals("sequence")) { 7455 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequence"); 7456 } else if (name.equals("focal")) { 7457 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.focal"); 7458 } else if (name.equals("coverage")) { 7459 this.coverage = new Reference(); 7460 return this.coverage; 7461 } else if (name.equals("businessArrangement")) { 7462 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.businessArrangement"); 7463 } else if (name.equals("claimResponse")) { 7464 this.claimResponse = new Reference(); 7465 return this.claimResponse; 7466 } else 7467 return super.addChild(name); 7468 } 7469 7470 public InsuranceComponent copy() { 7471 InsuranceComponent dst = new InsuranceComponent(); 7472 copyValues(dst); 7473 return dst; 7474 } 7475 7476 public void copyValues(InsuranceComponent dst) { 7477 super.copyValues(dst); 7478 dst.sequence = sequence == null ? null : sequence.copy(); 7479 dst.focal = focal == null ? null : focal.copy(); 7480 dst.coverage = coverage == null ? null : coverage.copy(); 7481 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 7482 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 7483 } 7484 7485 @Override 7486 public boolean equalsDeep(Base other_) { 7487 if (!super.equalsDeep(other_)) 7488 return false; 7489 if (!(other_ instanceof InsuranceComponent)) 7490 return false; 7491 InsuranceComponent o = (InsuranceComponent) other_; 7492 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) 7493 && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 7494 && compareDeep(claimResponse, o.claimResponse, true); 7495 } 7496 7497 @Override 7498 public boolean equalsShallow(Base other_) { 7499 if (!super.equalsShallow(other_)) 7500 return false; 7501 if (!(other_ instanceof InsuranceComponent)) 7502 return false; 7503 InsuranceComponent o = (InsuranceComponent) other_; 7504 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) 7505 && compareValues(businessArrangement, o.businessArrangement, true); 7506 } 7507 7508 public boolean isEmpty() { 7509 return super.isEmpty() 7510 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, coverage, businessArrangement, claimResponse); 7511 } 7512 7513 public String fhirType() { 7514 return "ClaimResponse.insurance"; 7515 7516 } 7517 7518 } 7519 7520 @Block() 7521 public static class ErrorComponent extends BackboneElement implements IBaseBackboneElement { 7522 /** 7523 * The sequence number of the line item submitted which contains the error. This 7524 * value is omitted when the error occurs outside of the item structure. 7525 */ 7526 @Child(name = "itemSequence", type = { 7527 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 7528 @Description(shortDefinition = "Item sequence number", formalDefinition = "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.") 7529 protected PositiveIntType itemSequence; 7530 7531 /** 7532 * The sequence number of the detail within the line item submitted which 7533 * contains the error. This value is omitted when the error occurs outside of 7534 * the item structure. 7535 */ 7536 @Child(name = "detailSequence", type = { 7537 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7538 @Description(shortDefinition = "Detail sequence number", formalDefinition = "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.") 7539 protected PositiveIntType detailSequence; 7540 7541 /** 7542 * The sequence number of the sub-detail within the detail within the line item 7543 * submitted which contains the error. This value is omitted when the error 7544 * occurs outside of the item structure. 7545 */ 7546 @Child(name = "subDetailSequence", type = { 7547 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 7548 @Description(shortDefinition = "Subdetail sequence number", formalDefinition = "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.") 7549 protected PositiveIntType subDetailSequence; 7550 7551 /** 7552 * An error code, from a specified code system, which details why the claim 7553 * could not be adjudicated. 7554 */ 7555 @Child(name = "code", type = { 7556 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 7557 @Description(shortDefinition = "Error code detailing processing issues", formalDefinition = "An error code, from a specified code system, which details why the claim could not be adjudicated.") 7558 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication-error") 7559 protected CodeableConcept code; 7560 7561 private static final long serialVersionUID = 843818320L; 7562 7563 /** 7564 * Constructor 7565 */ 7566 public ErrorComponent() { 7567 super(); 7568 } 7569 7570 /** 7571 * Constructor 7572 */ 7573 public ErrorComponent(CodeableConcept code) { 7574 super(); 7575 this.code = code; 7576 } 7577 7578 /** 7579 * @return {@link #itemSequence} (The sequence number of the line item submitted 7580 * which contains the error. This value is omitted when the error occurs 7581 * outside of the item structure.). This is the underlying object with 7582 * id, value and extensions. The accessor "getItemSequence" gives direct 7583 * access to the value 7584 */ 7585 public PositiveIntType getItemSequenceElement() { 7586 if (this.itemSequence == null) 7587 if (Configuration.errorOnAutoCreate()) 7588 throw new Error("Attempt to auto-create ErrorComponent.itemSequence"); 7589 else if (Configuration.doAutoCreate()) 7590 this.itemSequence = new PositiveIntType(); // bb 7591 return this.itemSequence; 7592 } 7593 7594 public boolean hasItemSequenceElement() { 7595 return this.itemSequence != null && !this.itemSequence.isEmpty(); 7596 } 7597 7598 public boolean hasItemSequence() { 7599 return this.itemSequence != null && !this.itemSequence.isEmpty(); 7600 } 7601 7602 /** 7603 * @param value {@link #itemSequence} (The sequence number of the line item 7604 * submitted which contains the error. This value is omitted when 7605 * the error occurs outside of the item structure.). This is the 7606 * underlying object with id, value and extensions. The accessor 7607 * "getItemSequence" gives direct access to the value 7608 */ 7609 public ErrorComponent setItemSequenceElement(PositiveIntType value) { 7610 this.itemSequence = value; 7611 return this; 7612 } 7613 7614 /** 7615 * @return The sequence number of the line item submitted which contains the 7616 * error. This value is omitted when the error occurs outside of the 7617 * item structure. 7618 */ 7619 public int getItemSequence() { 7620 return this.itemSequence == null || this.itemSequence.isEmpty() ? 0 : this.itemSequence.getValue(); 7621 } 7622 7623 /** 7624 * @param value The sequence number of the line item submitted which contains 7625 * the error. This value is omitted when the error occurs outside 7626 * of the item structure. 7627 */ 7628 public ErrorComponent setItemSequence(int value) { 7629 if (this.itemSequence == null) 7630 this.itemSequence = new PositiveIntType(); 7631 this.itemSequence.setValue(value); 7632 return this; 7633 } 7634 7635 /** 7636 * @return {@link #detailSequence} (The sequence number of the detail within the 7637 * line item submitted which contains the error. This value is omitted 7638 * when the error occurs outside of the item structure.). This is the 7639 * underlying object with id, value and extensions. The accessor 7640 * "getDetailSequence" gives direct access to the value 7641 */ 7642 public PositiveIntType getDetailSequenceElement() { 7643 if (this.detailSequence == null) 7644 if (Configuration.errorOnAutoCreate()) 7645 throw new Error("Attempt to auto-create ErrorComponent.detailSequence"); 7646 else if (Configuration.doAutoCreate()) 7647 this.detailSequence = new PositiveIntType(); // bb 7648 return this.detailSequence; 7649 } 7650 7651 public boolean hasDetailSequenceElement() { 7652 return this.detailSequence != null && !this.detailSequence.isEmpty(); 7653 } 7654 7655 public boolean hasDetailSequence() { 7656 return this.detailSequence != null && !this.detailSequence.isEmpty(); 7657 } 7658 7659 /** 7660 * @param value {@link #detailSequence} (The sequence number of the detail 7661 * within the line item submitted which contains the error. This 7662 * value is omitted when the error occurs outside of the item 7663 * structure.). This is the underlying object with id, value and 7664 * extensions. The accessor "getDetailSequence" gives direct access 7665 * to the value 7666 */ 7667 public ErrorComponent setDetailSequenceElement(PositiveIntType value) { 7668 this.detailSequence = value; 7669 return this; 7670 } 7671 7672 /** 7673 * @return The sequence number of the detail within the line item submitted 7674 * which contains the error. This value is omitted when the error occurs 7675 * outside of the item structure. 7676 */ 7677 public int getDetailSequence() { 7678 return this.detailSequence == null || this.detailSequence.isEmpty() ? 0 : this.detailSequence.getValue(); 7679 } 7680 7681 /** 7682 * @param value The sequence number of the detail within the line item submitted 7683 * which contains the error. This value is omitted when the error 7684 * occurs outside of the item structure. 7685 */ 7686 public ErrorComponent setDetailSequence(int value) { 7687 if (this.detailSequence == null) 7688 this.detailSequence = new PositiveIntType(); 7689 this.detailSequence.setValue(value); 7690 return this; 7691 } 7692 7693 /** 7694 * @return {@link #subDetailSequence} (The sequence number of the sub-detail 7695 * within the detail within the line item submitted which contains the 7696 * error. This value is omitted when the error occurs outside of the 7697 * item structure.). This is the underlying object with id, value and 7698 * extensions. The accessor "getSubDetailSequence" gives direct access 7699 * to the value 7700 */ 7701 public PositiveIntType getSubDetailSequenceElement() { 7702 if (this.subDetailSequence == null) 7703 if (Configuration.errorOnAutoCreate()) 7704 throw new Error("Attempt to auto-create ErrorComponent.subDetailSequence"); 7705 else if (Configuration.doAutoCreate()) 7706 this.subDetailSequence = new PositiveIntType(); // bb 7707 return this.subDetailSequence; 7708 } 7709 7710 public boolean hasSubDetailSequenceElement() { 7711 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 7712 } 7713 7714 public boolean hasSubDetailSequence() { 7715 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 7716 } 7717 7718 /** 7719 * @param value {@link #subDetailSequence} (The sequence number of the 7720 * sub-detail within the detail within the line item submitted 7721 * which contains the error. This value is omitted when the error 7722 * occurs outside of the item structure.). This is the underlying 7723 * object with id, value and extensions. The accessor 7724 * "getSubDetailSequence" gives direct access to the value 7725 */ 7726 public ErrorComponent setSubDetailSequenceElement(PositiveIntType value) { 7727 this.subDetailSequence = value; 7728 return this; 7729 } 7730 7731 /** 7732 * @return The sequence number of the sub-detail within the detail within the 7733 * line item submitted which contains the error. This value is omitted 7734 * when the error occurs outside of the item structure. 7735 */ 7736 public int getSubDetailSequence() { 7737 return this.subDetailSequence == null || this.subDetailSequence.isEmpty() ? 0 : this.subDetailSequence.getValue(); 7738 } 7739 7740 /** 7741 * @param value The sequence number of the sub-detail within the detail within 7742 * the line item submitted which contains the error. This value is 7743 * omitted when the error occurs outside of the item structure. 7744 */ 7745 public ErrorComponent setSubDetailSequence(int value) { 7746 if (this.subDetailSequence == null) 7747 this.subDetailSequence = new PositiveIntType(); 7748 this.subDetailSequence.setValue(value); 7749 return this; 7750 } 7751 7752 /** 7753 * @return {@link #code} (An error code, from a specified code system, which 7754 * details why the claim could not be adjudicated.) 7755 */ 7756 public CodeableConcept getCode() { 7757 if (this.code == null) 7758 if (Configuration.errorOnAutoCreate()) 7759 throw new Error("Attempt to auto-create ErrorComponent.code"); 7760 else if (Configuration.doAutoCreate()) 7761 this.code = new CodeableConcept(); // cc 7762 return this.code; 7763 } 7764 7765 public boolean hasCode() { 7766 return this.code != null && !this.code.isEmpty(); 7767 } 7768 7769 /** 7770 * @param value {@link #code} (An error code, from a specified code system, 7771 * which details why the claim could not be adjudicated.) 7772 */ 7773 public ErrorComponent setCode(CodeableConcept value) { 7774 this.code = value; 7775 return this; 7776 } 7777 7778 protected void listChildren(List<Property> children) { 7779 super.listChildren(children); 7780 children.add(new Property("itemSequence", "positiveInt", 7781 "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7782 0, 1, itemSequence)); 7783 children.add(new Property("detailSequence", "positiveInt", 7784 "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7785 0, 1, detailSequence)); 7786 children.add(new Property("subDetailSequence", "positiveInt", 7787 "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7788 0, 1, subDetailSequence)); 7789 children.add(new Property("code", "CodeableConcept", 7790 "An error code, from a specified code system, which details why the claim could not be adjudicated.", 0, 1, 7791 code)); 7792 } 7793 7794 @Override 7795 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7796 switch (_hash) { 7797 case 1977979892: 7798 /* itemSequence */ return new Property("itemSequence", "positiveInt", 7799 "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7800 0, 1, itemSequence); 7801 case 1321472818: 7802 /* detailSequence */ return new Property("detailSequence", "positiveInt", 7803 "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7804 0, 1, detailSequence); 7805 case -855462510: 7806 /* subDetailSequence */ return new Property("subDetailSequence", "positiveInt", 7807 "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 7808 0, 1, subDetailSequence); 7809 case 3059181: 7810 /* code */ return new Property("code", "CodeableConcept", 7811 "An error code, from a specified code system, which details why the claim could not be adjudicated.", 0, 1, 7812 code); 7813 default: 7814 return super.getNamedProperty(_hash, _name, _checkValid); 7815 } 7816 7817 } 7818 7819 @Override 7820 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7821 switch (hash) { 7822 case 1977979892: 7823 /* itemSequence */ return this.itemSequence == null ? new Base[0] : new Base[] { this.itemSequence }; // PositiveIntType 7824 case 1321472818: 7825 /* detailSequence */ return this.detailSequence == null ? new Base[0] : new Base[] { this.detailSequence }; // PositiveIntType 7826 case -855462510: 7827 /* subDetailSequence */ return this.subDetailSequence == null ? new Base[0] 7828 : new Base[] { this.subDetailSequence }; // PositiveIntType 7829 case 3059181: 7830 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 7831 default: 7832 return super.getProperty(hash, name, checkValid); 7833 } 7834 7835 } 7836 7837 @Override 7838 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7839 switch (hash) { 7840 case 1977979892: // itemSequence 7841 this.itemSequence = castToPositiveInt(value); // PositiveIntType 7842 return value; 7843 case 1321472818: // detailSequence 7844 this.detailSequence = castToPositiveInt(value); // PositiveIntType 7845 return value; 7846 case -855462510: // subDetailSequence 7847 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 7848 return value; 7849 case 3059181: // code 7850 this.code = castToCodeableConcept(value); // CodeableConcept 7851 return value; 7852 default: 7853 return super.setProperty(hash, name, value); 7854 } 7855 7856 } 7857 7858 @Override 7859 public Base setProperty(String name, Base value) throws FHIRException { 7860 if (name.equals("itemSequence")) { 7861 this.itemSequence = castToPositiveInt(value); // PositiveIntType 7862 } else if (name.equals("detailSequence")) { 7863 this.detailSequence = castToPositiveInt(value); // PositiveIntType 7864 } else if (name.equals("subDetailSequence")) { 7865 this.subDetailSequence = castToPositiveInt(value); // PositiveIntType 7866 } else if (name.equals("code")) { 7867 this.code = castToCodeableConcept(value); // CodeableConcept 7868 } else 7869 return super.setProperty(name, value); 7870 return value; 7871 } 7872 7873 @Override 7874 public void removeChild(String name, Base value) throws FHIRException { 7875 if (name.equals("itemSequence")) { 7876 this.itemSequence = null; 7877 } else if (name.equals("detailSequence")) { 7878 this.detailSequence = null; 7879 } else if (name.equals("subDetailSequence")) { 7880 this.subDetailSequence = null; 7881 } else if (name.equals("code")) { 7882 this.code = null; 7883 } else 7884 super.removeChild(name, value); 7885 7886 } 7887 7888 @Override 7889 public Base makeProperty(int hash, String name) throws FHIRException { 7890 switch (hash) { 7891 case 1977979892: 7892 return getItemSequenceElement(); 7893 case 1321472818: 7894 return getDetailSequenceElement(); 7895 case -855462510: 7896 return getSubDetailSequenceElement(); 7897 case 3059181: 7898 return getCode(); 7899 default: 7900 return super.makeProperty(hash, name); 7901 } 7902 7903 } 7904 7905 @Override 7906 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7907 switch (hash) { 7908 case 1977979892: 7909 /* itemSequence */ return new String[] { "positiveInt" }; 7910 case 1321472818: 7911 /* detailSequence */ return new String[] { "positiveInt" }; 7912 case -855462510: 7913 /* subDetailSequence */ return new String[] { "positiveInt" }; 7914 case 3059181: 7915 /* code */ return new String[] { "CodeableConcept" }; 7916 default: 7917 return super.getTypesForProperty(hash, name); 7918 } 7919 7920 } 7921 7922 @Override 7923 public Base addChild(String name) throws FHIRException { 7924 if (name.equals("itemSequence")) { 7925 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.itemSequence"); 7926 } else if (name.equals("detailSequence")) { 7927 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequence"); 7928 } else if (name.equals("subDetailSequence")) { 7929 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subDetailSequence"); 7930 } else if (name.equals("code")) { 7931 this.code = new CodeableConcept(); 7932 return this.code; 7933 } else 7934 return super.addChild(name); 7935 } 7936 7937 public ErrorComponent copy() { 7938 ErrorComponent dst = new ErrorComponent(); 7939 copyValues(dst); 7940 return dst; 7941 } 7942 7943 public void copyValues(ErrorComponent dst) { 7944 super.copyValues(dst); 7945 dst.itemSequence = itemSequence == null ? null : itemSequence.copy(); 7946 dst.detailSequence = detailSequence == null ? null : detailSequence.copy(); 7947 dst.subDetailSequence = subDetailSequence == null ? null : subDetailSequence.copy(); 7948 dst.code = code == null ? null : code.copy(); 7949 } 7950 7951 @Override 7952 public boolean equalsDeep(Base other_) { 7953 if (!super.equalsDeep(other_)) 7954 return false; 7955 if (!(other_ instanceof ErrorComponent)) 7956 return false; 7957 ErrorComponent o = (ErrorComponent) other_; 7958 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 7959 && compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(code, o.code, true); 7960 } 7961 7962 @Override 7963 public boolean equalsShallow(Base other_) { 7964 if (!super.equalsShallow(other_)) 7965 return false; 7966 if (!(other_ instanceof ErrorComponent)) 7967 return false; 7968 ErrorComponent o = (ErrorComponent) other_; 7969 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 7970 && compareValues(subDetailSequence, o.subDetailSequence, true); 7971 } 7972 7973 public boolean isEmpty() { 7974 return super.isEmpty() 7975 && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence, subDetailSequence, code); 7976 } 7977 7978 public String fhirType() { 7979 return "ClaimResponse.error"; 7980 7981 } 7982 7983 } 7984 7985 /** 7986 * A unique identifier assigned to this claim response. 7987 */ 7988 @Child(name = "identifier", type = { 7989 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7990 @Description(shortDefinition = "Business Identifier for a claim response", formalDefinition = "A unique identifier assigned to this claim response.") 7991 protected List<Identifier> identifier; 7992 7993 /** 7994 * The status of the resource instance. 7995 */ 7996 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 7997 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 7998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 7999 protected Enumeration<ClaimResponseStatus> status; 8000 8001 /** 8002 * A finer grained suite of claim type codes which may convey additional 8003 * information such as Inpatient vs Outpatient and/or a specialty service. 8004 */ 8005 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 8006 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 8007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-type") 8008 protected CodeableConcept type; 8009 8010 /** 8011 * A finer grained suite of claim type codes which may convey additional 8012 * information such as Inpatient vs Outpatient and/or a specialty service. 8013 */ 8014 @Child(name = "subType", type = { 8015 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 8016 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 8017 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-subtype") 8018 protected CodeableConcept subType; 8019 8020 /** 8021 * A code to indicate whether the nature of the request is: to request 8022 * adjudication of products and services previously rendered; or requesting 8023 * authorization and adjudication for provision in the future; or requesting the 8024 * non-binding adjudication of the listed products and services which could be 8025 * provided in the future. 8026 */ 8027 @Child(name = "use", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 8028 @Description(shortDefinition = "claim | preauthorization | predetermination", formalDefinition = "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.") 8029 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-use") 8030 protected Enumeration<Use> use; 8031 8032 /** 8033 * The party to whom the professional services and/or products have been 8034 * supplied or are being considered and for whom actual for facast reimbursement 8035 * is sought. 8036 */ 8037 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 8038 @Description(shortDefinition = "The recipient of the products and services", formalDefinition = "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.") 8039 protected Reference patient; 8040 8041 /** 8042 * The actual object that is the target of the reference (The party to whom the 8043 * professional services and/or products have been supplied or are being 8044 * considered and for whom actual for facast reimbursement is sought.) 8045 */ 8046 protected Patient patientTarget; 8047 8048 /** 8049 * The date this resource was created. 8050 */ 8051 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 8052 @Description(shortDefinition = "Response creation date", formalDefinition = "The date this resource was created.") 8053 protected DateTimeType created; 8054 8055 /** 8056 * The party responsible for authorization, adjudication and reimbursement. 8057 */ 8058 @Child(name = "insurer", type = { Organization.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 8059 @Description(shortDefinition = "Party responsible for reimbursement", formalDefinition = "The party responsible for authorization, adjudication and reimbursement.") 8060 protected Reference insurer; 8061 8062 /** 8063 * The actual object that is the target of the reference (The party responsible 8064 * for authorization, adjudication and reimbursement.) 8065 */ 8066 protected Organization insurerTarget; 8067 8068 /** 8069 * The provider which is responsible for the claim, predetermination or 8070 * preauthorization. 8071 */ 8072 @Child(name = "requestor", type = { Practitioner.class, PractitionerRole.class, 8073 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 8074 @Description(shortDefinition = "Party responsible for the claim", formalDefinition = "The provider which is responsible for the claim, predetermination or preauthorization.") 8075 protected Reference requestor; 8076 8077 /** 8078 * The actual object that is the target of the reference (The provider which is 8079 * responsible for the claim, predetermination or preauthorization.) 8080 */ 8081 protected Resource requestorTarget; 8082 8083 /** 8084 * Original request resource reference. 8085 */ 8086 @Child(name = "request", type = { Claim.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 8087 @Description(shortDefinition = "Id of resource triggering adjudication", formalDefinition = "Original request resource reference.") 8088 protected Reference request; 8089 8090 /** 8091 * The actual object that is the target of the reference (Original request 8092 * resource reference.) 8093 */ 8094 protected Claim requestTarget; 8095 8096 /** 8097 * The outcome of the claim, predetermination, or preauthorization processing. 8098 */ 8099 @Child(name = "outcome", type = { CodeType.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 8100 @Description(shortDefinition = "queued | complete | error | partial", formalDefinition = "The outcome of the claim, predetermination, or preauthorization processing.") 8101 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/remittance-outcome") 8102 protected Enumeration<RemittanceOutcome> outcome; 8103 8104 /** 8105 * A human readable description of the status of the adjudication. 8106 */ 8107 @Child(name = "disposition", type = { 8108 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 8109 @Description(shortDefinition = "Disposition Message", formalDefinition = "A human readable description of the status of the adjudication.") 8110 protected StringType disposition; 8111 8112 /** 8113 * Reference from the Insurer which is used in later communications which refers 8114 * to this adjudication. 8115 */ 8116 @Child(name = "preAuthRef", type = { 8117 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 8118 @Description(shortDefinition = "Preauthorization reference", formalDefinition = "Reference from the Insurer which is used in later communications which refers to this adjudication.") 8119 protected StringType preAuthRef; 8120 8121 /** 8122 * The time frame during which this authorization is effective. 8123 */ 8124 @Child(name = "preAuthPeriod", type = { 8125 Period.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 8126 @Description(shortDefinition = "Preauthorization reference effective period", formalDefinition = "The time frame during which this authorization is effective.") 8127 protected Period preAuthPeriod; 8128 8129 /** 8130 * Type of Party to be reimbursed: subscriber, provider, other. 8131 */ 8132 @Child(name = "payeeType", type = { 8133 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 8134 @Description(shortDefinition = "Party to be paid any benefits payable", formalDefinition = "Type of Party to be reimbursed: subscriber, provider, other.") 8135 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payeetype") 8136 protected CodeableConcept payeeType; 8137 8138 /** 8139 * A claim line. Either a simple (a product or service) or a 'group' of details 8140 * which can also be a simple items or groups of sub-details. 8141 */ 8142 @Child(name = "item", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8143 @Description(shortDefinition = "Adjudication for claim line items", formalDefinition = "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.") 8144 protected List<ItemComponent> item; 8145 8146 /** 8147 * The first-tier service adjudications for payor added product or service 8148 * lines. 8149 */ 8150 @Child(name = "addItem", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8151 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The first-tier service adjudications for payor added product or service lines.") 8152 protected List<AddedItemComponent> addItem; 8153 8154 /** 8155 * The adjudication results which are presented at the header level rather than 8156 * at the line-item or add-item levels. 8157 */ 8158 @Child(name = "adjudication", type = { 8159 AdjudicationComponent.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8160 @Description(shortDefinition = "Header-level adjudication", formalDefinition = "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.") 8161 protected List<AdjudicationComponent> adjudication; 8162 8163 /** 8164 * Categorized monetary totals for the adjudication. 8165 */ 8166 @Child(name = "total", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 8167 @Description(shortDefinition = "Adjudication totals", formalDefinition = "Categorized monetary totals for the adjudication.") 8168 protected List<TotalComponent> total; 8169 8170 /** 8171 * Payment details for the adjudication of the claim. 8172 */ 8173 @Child(name = "payment", type = {}, order = 19, min = 0, max = 1, modifier = false, summary = false) 8174 @Description(shortDefinition = "Payment Details", formalDefinition = "Payment details for the adjudication of the claim.") 8175 protected PaymentComponent payment; 8176 8177 /** 8178 * A code, used only on a response to a preauthorization, to indicate whether 8179 * the benefits payable have been reserved and for whom. 8180 */ 8181 @Child(name = "fundsReserve", type = { 8182 CodeableConcept.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 8183 @Description(shortDefinition = "Funds reserved status", formalDefinition = "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.") 8184 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fundsreserve") 8185 protected CodeableConcept fundsReserve; 8186 8187 /** 8188 * A code for the form to be used for printing the content. 8189 */ 8190 @Child(name = "formCode", type = { 8191 CodeableConcept.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 8192 @Description(shortDefinition = "Printed form identifier", formalDefinition = "A code for the form to be used for printing the content.") 8193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/forms") 8194 protected CodeableConcept formCode; 8195 8196 /** 8197 * The actual form, by reference or inclusion, for printing the content or an 8198 * EOB. 8199 */ 8200 @Child(name = "form", type = { Attachment.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 8201 @Description(shortDefinition = "Printed reference or actual form", formalDefinition = "The actual form, by reference or inclusion, for printing the content or an EOB.") 8202 protected Attachment form; 8203 8204 /** 8205 * A note that describes or explains adjudication results in a human readable 8206 * form. 8207 */ 8208 @Child(name = "processNote", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8209 @Description(shortDefinition = "Note concerning adjudication", formalDefinition = "A note that describes or explains adjudication results in a human readable form.") 8210 protected List<NoteComponent> processNote; 8211 8212 /** 8213 * Request for additional supporting or authorizing information. 8214 */ 8215 @Child(name = "communicationRequest", type = { 8216 CommunicationRequest.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8217 @Description(shortDefinition = "Request for additional information", formalDefinition = "Request for additional supporting or authorizing information.") 8218 protected List<Reference> communicationRequest; 8219 /** 8220 * The actual objects that are the target of the reference (Request for 8221 * additional supporting or authorizing information.) 8222 */ 8223 protected List<CommunicationRequest> communicationRequestTarget; 8224 8225 /** 8226 * Financial instruments for reimbursement for the health care products and 8227 * services specified on the claim. 8228 */ 8229 @Child(name = "insurance", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8230 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services specified on the claim.") 8231 protected List<InsuranceComponent> insurance; 8232 8233 /** 8234 * Errors encountered during the processing of the adjudication. 8235 */ 8236 @Child(name = "error", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8237 @Description(shortDefinition = "Processing errors", formalDefinition = "Errors encountered during the processing of the adjudication.") 8238 protected List<ErrorComponent> error; 8239 8240 private static final long serialVersionUID = 731586651L; 8241 8242 /** 8243 * Constructor 8244 */ 8245 public ClaimResponse() { 8246 super(); 8247 } 8248 8249 /** 8250 * Constructor 8251 */ 8252 public ClaimResponse(Enumeration<ClaimResponseStatus> status, CodeableConcept type, Enumeration<Use> use, 8253 Reference patient, DateTimeType created, Reference insurer, Enumeration<RemittanceOutcome> outcome) { 8254 super(); 8255 this.status = status; 8256 this.type = type; 8257 this.use = use; 8258 this.patient = patient; 8259 this.created = created; 8260 this.insurer = insurer; 8261 this.outcome = outcome; 8262 } 8263 8264 /** 8265 * @return {@link #identifier} (A unique identifier assigned to this claim 8266 * response.) 8267 */ 8268 public List<Identifier> getIdentifier() { 8269 if (this.identifier == null) 8270 this.identifier = new ArrayList<Identifier>(); 8271 return this.identifier; 8272 } 8273 8274 /** 8275 * @return Returns a reference to <code>this</code> for easy method chaining 8276 */ 8277 public ClaimResponse setIdentifier(List<Identifier> theIdentifier) { 8278 this.identifier = theIdentifier; 8279 return this; 8280 } 8281 8282 public boolean hasIdentifier() { 8283 if (this.identifier == null) 8284 return false; 8285 for (Identifier item : this.identifier) 8286 if (!item.isEmpty()) 8287 return true; 8288 return false; 8289 } 8290 8291 public Identifier addIdentifier() { // 3 8292 Identifier t = new Identifier(); 8293 if (this.identifier == null) 8294 this.identifier = new ArrayList<Identifier>(); 8295 this.identifier.add(t); 8296 return t; 8297 } 8298 8299 public ClaimResponse addIdentifier(Identifier t) { // 3 8300 if (t == null) 8301 return this; 8302 if (this.identifier == null) 8303 this.identifier = new ArrayList<Identifier>(); 8304 this.identifier.add(t); 8305 return this; 8306 } 8307 8308 /** 8309 * @return The first repetition of repeating field {@link #identifier}, creating 8310 * it if it does not already exist 8311 */ 8312 public Identifier getIdentifierFirstRep() { 8313 if (getIdentifier().isEmpty()) { 8314 addIdentifier(); 8315 } 8316 return getIdentifier().get(0); 8317 } 8318 8319 /** 8320 * @return {@link #status} (The status of the resource instance.). This is the 8321 * underlying object with id, value and extensions. The accessor 8322 * "getStatus" gives direct access to the value 8323 */ 8324 public Enumeration<ClaimResponseStatus> getStatusElement() { 8325 if (this.status == null) 8326 if (Configuration.errorOnAutoCreate()) 8327 throw new Error("Attempt to auto-create ClaimResponse.status"); 8328 else if (Configuration.doAutoCreate()) 8329 this.status = new Enumeration<ClaimResponseStatus>(new ClaimResponseStatusEnumFactory()); // bb 8330 return this.status; 8331 } 8332 8333 public boolean hasStatusElement() { 8334 return this.status != null && !this.status.isEmpty(); 8335 } 8336 8337 public boolean hasStatus() { 8338 return this.status != null && !this.status.isEmpty(); 8339 } 8340 8341 /** 8342 * @param value {@link #status} (The status of the resource instance.). This is 8343 * the underlying object with id, value and extensions. The 8344 * accessor "getStatus" gives direct access to the value 8345 */ 8346 public ClaimResponse setStatusElement(Enumeration<ClaimResponseStatus> value) { 8347 this.status = value; 8348 return this; 8349 } 8350 8351 /** 8352 * @return The status of the resource instance. 8353 */ 8354 public ClaimResponseStatus getStatus() { 8355 return this.status == null ? null : this.status.getValue(); 8356 } 8357 8358 /** 8359 * @param value The status of the resource instance. 8360 */ 8361 public ClaimResponse setStatus(ClaimResponseStatus value) { 8362 if (this.status == null) 8363 this.status = new Enumeration<ClaimResponseStatus>(new ClaimResponseStatusEnumFactory()); 8364 this.status.setValue(value); 8365 return this; 8366 } 8367 8368 /** 8369 * @return {@link #type} (A finer grained suite of claim type codes which may 8370 * convey additional information such as Inpatient vs Outpatient and/or 8371 * a specialty service.) 8372 */ 8373 public CodeableConcept getType() { 8374 if (this.type == null) 8375 if (Configuration.errorOnAutoCreate()) 8376 throw new Error("Attempt to auto-create ClaimResponse.type"); 8377 else if (Configuration.doAutoCreate()) 8378 this.type = new CodeableConcept(); // cc 8379 return this.type; 8380 } 8381 8382 public boolean hasType() { 8383 return this.type != null && !this.type.isEmpty(); 8384 } 8385 8386 /** 8387 * @param value {@link #type} (A finer grained suite of claim type codes which 8388 * may convey additional information such as Inpatient vs 8389 * Outpatient and/or a specialty service.) 8390 */ 8391 public ClaimResponse setType(CodeableConcept value) { 8392 this.type = value; 8393 return this; 8394 } 8395 8396 /** 8397 * @return {@link #subType} (A finer grained suite of claim type codes which may 8398 * convey additional information such as Inpatient vs Outpatient and/or 8399 * a specialty service.) 8400 */ 8401 public CodeableConcept getSubType() { 8402 if (this.subType == null) 8403 if (Configuration.errorOnAutoCreate()) 8404 throw new Error("Attempt to auto-create ClaimResponse.subType"); 8405 else if (Configuration.doAutoCreate()) 8406 this.subType = new CodeableConcept(); // cc 8407 return this.subType; 8408 } 8409 8410 public boolean hasSubType() { 8411 return this.subType != null && !this.subType.isEmpty(); 8412 } 8413 8414 /** 8415 * @param value {@link #subType} (A finer grained suite of claim type codes 8416 * which may convey additional information such as Inpatient vs 8417 * Outpatient and/or a specialty service.) 8418 */ 8419 public ClaimResponse setSubType(CodeableConcept value) { 8420 this.subType = value; 8421 return this; 8422 } 8423 8424 /** 8425 * @return {@link #use} (A code to indicate whether the nature of the request 8426 * is: to request adjudication of products and services previously 8427 * rendered; or requesting authorization and adjudication for provision 8428 * in the future; or requesting the non-binding adjudication of the 8429 * listed products and services which could be provided in the future.). 8430 * This is the underlying object with id, value and extensions. The 8431 * accessor "getUse" gives direct access to the value 8432 */ 8433 public Enumeration<Use> getUseElement() { 8434 if (this.use == null) 8435 if (Configuration.errorOnAutoCreate()) 8436 throw new Error("Attempt to auto-create ClaimResponse.use"); 8437 else if (Configuration.doAutoCreate()) 8438 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 8439 return this.use; 8440 } 8441 8442 public boolean hasUseElement() { 8443 return this.use != null && !this.use.isEmpty(); 8444 } 8445 8446 public boolean hasUse() { 8447 return this.use != null && !this.use.isEmpty(); 8448 } 8449 8450 /** 8451 * @param value {@link #use} (A code to indicate whether the nature of the 8452 * request is: to request adjudication of products and services 8453 * previously rendered; or requesting authorization and 8454 * adjudication for provision in the future; or requesting the 8455 * non-binding adjudication of the listed products and services 8456 * which could be provided in the future.). This is the underlying 8457 * object with id, value and extensions. The accessor "getUse" 8458 * gives direct access to the value 8459 */ 8460 public ClaimResponse setUseElement(Enumeration<Use> value) { 8461 this.use = value; 8462 return this; 8463 } 8464 8465 /** 8466 * @return A code to indicate whether the nature of the request is: to request 8467 * adjudication of products and services previously rendered; or 8468 * requesting authorization and adjudication for provision in the 8469 * future; or requesting the non-binding adjudication of the listed 8470 * products and services which could be provided in the future. 8471 */ 8472 public Use getUse() { 8473 return this.use == null ? null : this.use.getValue(); 8474 } 8475 8476 /** 8477 * @param value A code to indicate whether the nature of the request is: to 8478 * request adjudication of products and services previously 8479 * rendered; or requesting authorization and adjudication for 8480 * provision in the future; or requesting the non-binding 8481 * adjudication of the listed products and services which could be 8482 * provided in the future. 8483 */ 8484 public ClaimResponse setUse(Use value) { 8485 if (this.use == null) 8486 this.use = new Enumeration<Use>(new UseEnumFactory()); 8487 this.use.setValue(value); 8488 return this; 8489 } 8490 8491 /** 8492 * @return {@link #patient} (The party to whom the professional services and/or 8493 * products have been supplied or are being considered and for whom 8494 * actual for facast reimbursement is sought.) 8495 */ 8496 public Reference getPatient() { 8497 if (this.patient == null) 8498 if (Configuration.errorOnAutoCreate()) 8499 throw new Error("Attempt to auto-create ClaimResponse.patient"); 8500 else if (Configuration.doAutoCreate()) 8501 this.patient = new Reference(); // cc 8502 return this.patient; 8503 } 8504 8505 public boolean hasPatient() { 8506 return this.patient != null && !this.patient.isEmpty(); 8507 } 8508 8509 /** 8510 * @param value {@link #patient} (The party to whom the professional services 8511 * and/or products have been supplied or are being considered and 8512 * for whom actual for facast reimbursement is sought.) 8513 */ 8514 public ClaimResponse setPatient(Reference value) { 8515 this.patient = value; 8516 return this; 8517 } 8518 8519 /** 8520 * @return {@link #patient} The actual object that is the target of the 8521 * reference. The reference library doesn't populate this, but you can 8522 * use it to hold the resource if you resolve it. (The party to whom the 8523 * professional services and/or products have been supplied or are being 8524 * considered and for whom actual for facast reimbursement is sought.) 8525 */ 8526 public Patient getPatientTarget() { 8527 if (this.patientTarget == null) 8528 if (Configuration.errorOnAutoCreate()) 8529 throw new Error("Attempt to auto-create ClaimResponse.patient"); 8530 else if (Configuration.doAutoCreate()) 8531 this.patientTarget = new Patient(); // aa 8532 return this.patientTarget; 8533 } 8534 8535 /** 8536 * @param value {@link #patient} The actual object that is the target of the 8537 * reference. The reference library doesn't use these, but you can 8538 * use it to hold the resource if you resolve it. (The party to 8539 * whom the professional services and/or products have been 8540 * supplied or are being considered and for whom actual for facast 8541 * reimbursement is sought.) 8542 */ 8543 public ClaimResponse setPatientTarget(Patient value) { 8544 this.patientTarget = value; 8545 return this; 8546 } 8547 8548 /** 8549 * @return {@link #created} (The date this resource was created.). This is the 8550 * underlying object with id, value and extensions. The accessor 8551 * "getCreated" gives direct access to the value 8552 */ 8553 public DateTimeType getCreatedElement() { 8554 if (this.created == null) 8555 if (Configuration.errorOnAutoCreate()) 8556 throw new Error("Attempt to auto-create ClaimResponse.created"); 8557 else if (Configuration.doAutoCreate()) 8558 this.created = new DateTimeType(); // bb 8559 return this.created; 8560 } 8561 8562 public boolean hasCreatedElement() { 8563 return this.created != null && !this.created.isEmpty(); 8564 } 8565 8566 public boolean hasCreated() { 8567 return this.created != null && !this.created.isEmpty(); 8568 } 8569 8570 /** 8571 * @param value {@link #created} (The date this resource was created.). This is 8572 * the underlying object with id, value and extensions. The 8573 * accessor "getCreated" gives direct access to the value 8574 */ 8575 public ClaimResponse setCreatedElement(DateTimeType value) { 8576 this.created = value; 8577 return this; 8578 } 8579 8580 /** 8581 * @return The date this resource was created. 8582 */ 8583 public Date getCreated() { 8584 return this.created == null ? null : this.created.getValue(); 8585 } 8586 8587 /** 8588 * @param value The date this resource was created. 8589 */ 8590 public ClaimResponse setCreated(Date value) { 8591 if (this.created == null) 8592 this.created = new DateTimeType(); 8593 this.created.setValue(value); 8594 return this; 8595 } 8596 8597 /** 8598 * @return {@link #insurer} (The party responsible for authorization, 8599 * adjudication and reimbursement.) 8600 */ 8601 public Reference getInsurer() { 8602 if (this.insurer == null) 8603 if (Configuration.errorOnAutoCreate()) 8604 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 8605 else if (Configuration.doAutoCreate()) 8606 this.insurer = new Reference(); // cc 8607 return this.insurer; 8608 } 8609 8610 public boolean hasInsurer() { 8611 return this.insurer != null && !this.insurer.isEmpty(); 8612 } 8613 8614 /** 8615 * @param value {@link #insurer} (The party responsible for authorization, 8616 * adjudication and reimbursement.) 8617 */ 8618 public ClaimResponse setInsurer(Reference value) { 8619 this.insurer = value; 8620 return this; 8621 } 8622 8623 /** 8624 * @return {@link #insurer} The actual object that is the target of the 8625 * reference. The reference library doesn't populate this, but you can 8626 * use it to hold the resource if you resolve it. (The party responsible 8627 * for authorization, adjudication and reimbursement.) 8628 */ 8629 public Organization getInsurerTarget() { 8630 if (this.insurerTarget == null) 8631 if (Configuration.errorOnAutoCreate()) 8632 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 8633 else if (Configuration.doAutoCreate()) 8634 this.insurerTarget = new Organization(); // aa 8635 return this.insurerTarget; 8636 } 8637 8638 /** 8639 * @param value {@link #insurer} The actual object that is the target of the 8640 * reference. The reference library doesn't use these, but you can 8641 * use it to hold the resource if you resolve it. (The party 8642 * responsible for authorization, adjudication and reimbursement.) 8643 */ 8644 public ClaimResponse setInsurerTarget(Organization value) { 8645 this.insurerTarget = value; 8646 return this; 8647 } 8648 8649 /** 8650 * @return {@link #requestor} (The provider which is responsible for the claim, 8651 * predetermination or preauthorization.) 8652 */ 8653 public Reference getRequestor() { 8654 if (this.requestor == null) 8655 if (Configuration.errorOnAutoCreate()) 8656 throw new Error("Attempt to auto-create ClaimResponse.requestor"); 8657 else if (Configuration.doAutoCreate()) 8658 this.requestor = new Reference(); // cc 8659 return this.requestor; 8660 } 8661 8662 public boolean hasRequestor() { 8663 return this.requestor != null && !this.requestor.isEmpty(); 8664 } 8665 8666 /** 8667 * @param value {@link #requestor} (The provider which is responsible for the 8668 * claim, predetermination or preauthorization.) 8669 */ 8670 public ClaimResponse setRequestor(Reference value) { 8671 this.requestor = value; 8672 return this; 8673 } 8674 8675 /** 8676 * @return {@link #requestor} The actual object that is the target of the 8677 * reference. The reference library doesn't populate this, but you can 8678 * use it to hold the resource if you resolve it. (The provider which is 8679 * responsible for the claim, predetermination or preauthorization.) 8680 */ 8681 public Resource getRequestorTarget() { 8682 return this.requestorTarget; 8683 } 8684 8685 /** 8686 * @param value {@link #requestor} The actual object that is the target of the 8687 * reference. The reference library doesn't use these, but you can 8688 * use it to hold the resource if you resolve it. (The provider 8689 * which is responsible for the claim, predetermination or 8690 * preauthorization.) 8691 */ 8692 public ClaimResponse setRequestorTarget(Resource value) { 8693 this.requestorTarget = value; 8694 return this; 8695 } 8696 8697 /** 8698 * @return {@link #request} (Original request resource reference.) 8699 */ 8700 public Reference getRequest() { 8701 if (this.request == null) 8702 if (Configuration.errorOnAutoCreate()) 8703 throw new Error("Attempt to auto-create ClaimResponse.request"); 8704 else if (Configuration.doAutoCreate()) 8705 this.request = new Reference(); // cc 8706 return this.request; 8707 } 8708 8709 public boolean hasRequest() { 8710 return this.request != null && !this.request.isEmpty(); 8711 } 8712 8713 /** 8714 * @param value {@link #request} (Original request resource reference.) 8715 */ 8716 public ClaimResponse setRequest(Reference value) { 8717 this.request = value; 8718 return this; 8719 } 8720 8721 /** 8722 * @return {@link #request} The actual object that is the target of the 8723 * reference. The reference library doesn't populate this, but you can 8724 * use it to hold the resource if you resolve it. (Original request 8725 * resource reference.) 8726 */ 8727 public Claim getRequestTarget() { 8728 if (this.requestTarget == null) 8729 if (Configuration.errorOnAutoCreate()) 8730 throw new Error("Attempt to auto-create ClaimResponse.request"); 8731 else if (Configuration.doAutoCreate()) 8732 this.requestTarget = new Claim(); // aa 8733 return this.requestTarget; 8734 } 8735 8736 /** 8737 * @param value {@link #request} The actual object that is the target of the 8738 * reference. The reference library doesn't use these, but you can 8739 * use it to hold the resource if you resolve it. (Original request 8740 * resource reference.) 8741 */ 8742 public ClaimResponse setRequestTarget(Claim value) { 8743 this.requestTarget = value; 8744 return this; 8745 } 8746 8747 /** 8748 * @return {@link #outcome} (The outcome of the claim, predetermination, or 8749 * preauthorization processing.). This is the underlying object with id, 8750 * value and extensions. The accessor "getOutcome" gives direct access 8751 * to the value 8752 */ 8753 public Enumeration<RemittanceOutcome> getOutcomeElement() { 8754 if (this.outcome == null) 8755 if (Configuration.errorOnAutoCreate()) 8756 throw new Error("Attempt to auto-create ClaimResponse.outcome"); 8757 else if (Configuration.doAutoCreate()) 8758 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 8759 return this.outcome; 8760 } 8761 8762 public boolean hasOutcomeElement() { 8763 return this.outcome != null && !this.outcome.isEmpty(); 8764 } 8765 8766 public boolean hasOutcome() { 8767 return this.outcome != null && !this.outcome.isEmpty(); 8768 } 8769 8770 /** 8771 * @param value {@link #outcome} (The outcome of the claim, predetermination, or 8772 * preauthorization processing.). This is the underlying object 8773 * with id, value and extensions. The accessor "getOutcome" gives 8774 * direct access to the value 8775 */ 8776 public ClaimResponse setOutcomeElement(Enumeration<RemittanceOutcome> value) { 8777 this.outcome = value; 8778 return this; 8779 } 8780 8781 /** 8782 * @return The outcome of the claim, predetermination, or preauthorization 8783 * processing. 8784 */ 8785 public RemittanceOutcome getOutcome() { 8786 return this.outcome == null ? null : this.outcome.getValue(); 8787 } 8788 8789 /** 8790 * @param value The outcome of the claim, predetermination, or preauthorization 8791 * processing. 8792 */ 8793 public ClaimResponse setOutcome(RemittanceOutcome value) { 8794 if (this.outcome == null) 8795 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 8796 this.outcome.setValue(value); 8797 return this; 8798 } 8799 8800 /** 8801 * @return {@link #disposition} (A human readable description of the status of 8802 * the adjudication.). This is the underlying object with id, value and 8803 * extensions. The accessor "getDisposition" gives direct access to the 8804 * value 8805 */ 8806 public StringType getDispositionElement() { 8807 if (this.disposition == null) 8808 if (Configuration.errorOnAutoCreate()) 8809 throw new Error("Attempt to auto-create ClaimResponse.disposition"); 8810 else if (Configuration.doAutoCreate()) 8811 this.disposition = new StringType(); // bb 8812 return this.disposition; 8813 } 8814 8815 public boolean hasDispositionElement() { 8816 return this.disposition != null && !this.disposition.isEmpty(); 8817 } 8818 8819 public boolean hasDisposition() { 8820 return this.disposition != null && !this.disposition.isEmpty(); 8821 } 8822 8823 /** 8824 * @param value {@link #disposition} (A human readable description of the status 8825 * of the adjudication.). This is the underlying object with id, 8826 * value and extensions. The accessor "getDisposition" gives direct 8827 * access to the value 8828 */ 8829 public ClaimResponse setDispositionElement(StringType value) { 8830 this.disposition = value; 8831 return this; 8832 } 8833 8834 /** 8835 * @return A human readable description of the status of the adjudication. 8836 */ 8837 public String getDisposition() { 8838 return this.disposition == null ? null : this.disposition.getValue(); 8839 } 8840 8841 /** 8842 * @param value A human readable description of the status of the adjudication. 8843 */ 8844 public ClaimResponse setDisposition(String value) { 8845 if (Utilities.noString(value)) 8846 this.disposition = null; 8847 else { 8848 if (this.disposition == null) 8849 this.disposition = new StringType(); 8850 this.disposition.setValue(value); 8851 } 8852 return this; 8853 } 8854 8855 /** 8856 * @return {@link #preAuthRef} (Reference from the Insurer which is used in 8857 * later communications which refers to this adjudication.). This is the 8858 * underlying object with id, value and extensions. The accessor 8859 * "getPreAuthRef" gives direct access to the value 8860 */ 8861 public StringType getPreAuthRefElement() { 8862 if (this.preAuthRef == null) 8863 if (Configuration.errorOnAutoCreate()) 8864 throw new Error("Attempt to auto-create ClaimResponse.preAuthRef"); 8865 else if (Configuration.doAutoCreate()) 8866 this.preAuthRef = new StringType(); // bb 8867 return this.preAuthRef; 8868 } 8869 8870 public boolean hasPreAuthRefElement() { 8871 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 8872 } 8873 8874 public boolean hasPreAuthRef() { 8875 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 8876 } 8877 8878 /** 8879 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in 8880 * later communications which refers to this adjudication.). This 8881 * is the underlying object with id, value and extensions. The 8882 * accessor "getPreAuthRef" gives direct access to the value 8883 */ 8884 public ClaimResponse setPreAuthRefElement(StringType value) { 8885 this.preAuthRef = value; 8886 return this; 8887 } 8888 8889 /** 8890 * @return Reference from the Insurer which is used in later communications 8891 * which refers to this adjudication. 8892 */ 8893 public String getPreAuthRef() { 8894 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 8895 } 8896 8897 /** 8898 * @param value Reference from the Insurer which is used in later communications 8899 * which refers to this adjudication. 8900 */ 8901 public ClaimResponse setPreAuthRef(String value) { 8902 if (Utilities.noString(value)) 8903 this.preAuthRef = null; 8904 else { 8905 if (this.preAuthRef == null) 8906 this.preAuthRef = new StringType(); 8907 this.preAuthRef.setValue(value); 8908 } 8909 return this; 8910 } 8911 8912 /** 8913 * @return {@link #preAuthPeriod} (The time frame during which this 8914 * authorization is effective.) 8915 */ 8916 public Period getPreAuthPeriod() { 8917 if (this.preAuthPeriod == null) 8918 if (Configuration.errorOnAutoCreate()) 8919 throw new Error("Attempt to auto-create ClaimResponse.preAuthPeriod"); 8920 else if (Configuration.doAutoCreate()) 8921 this.preAuthPeriod = new Period(); // cc 8922 return this.preAuthPeriod; 8923 } 8924 8925 public boolean hasPreAuthPeriod() { 8926 return this.preAuthPeriod != null && !this.preAuthPeriod.isEmpty(); 8927 } 8928 8929 /** 8930 * @param value {@link #preAuthPeriod} (The time frame during which this 8931 * authorization is effective.) 8932 */ 8933 public ClaimResponse setPreAuthPeriod(Period value) { 8934 this.preAuthPeriod = value; 8935 return this; 8936 } 8937 8938 /** 8939 * @return {@link #payeeType} (Type of Party to be reimbursed: subscriber, 8940 * provider, other.) 8941 */ 8942 public CodeableConcept getPayeeType() { 8943 if (this.payeeType == null) 8944 if (Configuration.errorOnAutoCreate()) 8945 throw new Error("Attempt to auto-create ClaimResponse.payeeType"); 8946 else if (Configuration.doAutoCreate()) 8947 this.payeeType = new CodeableConcept(); // cc 8948 return this.payeeType; 8949 } 8950 8951 public boolean hasPayeeType() { 8952 return this.payeeType != null && !this.payeeType.isEmpty(); 8953 } 8954 8955 /** 8956 * @param value {@link #payeeType} (Type of Party to be reimbursed: subscriber, 8957 * provider, other.) 8958 */ 8959 public ClaimResponse setPayeeType(CodeableConcept value) { 8960 this.payeeType = value; 8961 return this; 8962 } 8963 8964 /** 8965 * @return {@link #item} (A claim line. Either a simple (a product or service) 8966 * or a 'group' of details which can also be a simple items or groups of 8967 * sub-details.) 8968 */ 8969 public List<ItemComponent> getItem() { 8970 if (this.item == null) 8971 this.item = new ArrayList<ItemComponent>(); 8972 return this.item; 8973 } 8974 8975 /** 8976 * @return Returns a reference to <code>this</code> for easy method chaining 8977 */ 8978 public ClaimResponse setItem(List<ItemComponent> theItem) { 8979 this.item = theItem; 8980 return this; 8981 } 8982 8983 public boolean hasItem() { 8984 if (this.item == null) 8985 return false; 8986 for (ItemComponent item : this.item) 8987 if (!item.isEmpty()) 8988 return true; 8989 return false; 8990 } 8991 8992 public ItemComponent addItem() { // 3 8993 ItemComponent t = new ItemComponent(); 8994 if (this.item == null) 8995 this.item = new ArrayList<ItemComponent>(); 8996 this.item.add(t); 8997 return t; 8998 } 8999 9000 public ClaimResponse addItem(ItemComponent t) { // 3 9001 if (t == null) 9002 return this; 9003 if (this.item == null) 9004 this.item = new ArrayList<ItemComponent>(); 9005 this.item.add(t); 9006 return this; 9007 } 9008 9009 /** 9010 * @return The first repetition of repeating field {@link #item}, creating it if 9011 * it does not already exist 9012 */ 9013 public ItemComponent getItemFirstRep() { 9014 if (getItem().isEmpty()) { 9015 addItem(); 9016 } 9017 return getItem().get(0); 9018 } 9019 9020 /** 9021 * @return {@link #addItem} (The first-tier service adjudications for payor 9022 * added product or service lines.) 9023 */ 9024 public List<AddedItemComponent> getAddItem() { 9025 if (this.addItem == null) 9026 this.addItem = new ArrayList<AddedItemComponent>(); 9027 return this.addItem; 9028 } 9029 9030 /** 9031 * @return Returns a reference to <code>this</code> for easy method chaining 9032 */ 9033 public ClaimResponse setAddItem(List<AddedItemComponent> theAddItem) { 9034 this.addItem = theAddItem; 9035 return this; 9036 } 9037 9038 public boolean hasAddItem() { 9039 if (this.addItem == null) 9040 return false; 9041 for (AddedItemComponent item : this.addItem) 9042 if (!item.isEmpty()) 9043 return true; 9044 return false; 9045 } 9046 9047 public AddedItemComponent addAddItem() { // 3 9048 AddedItemComponent t = new AddedItemComponent(); 9049 if (this.addItem == null) 9050 this.addItem = new ArrayList<AddedItemComponent>(); 9051 this.addItem.add(t); 9052 return t; 9053 } 9054 9055 public ClaimResponse addAddItem(AddedItemComponent t) { // 3 9056 if (t == null) 9057 return this; 9058 if (this.addItem == null) 9059 this.addItem = new ArrayList<AddedItemComponent>(); 9060 this.addItem.add(t); 9061 return this; 9062 } 9063 9064 /** 9065 * @return The first repetition of repeating field {@link #addItem}, creating it 9066 * if it does not already exist 9067 */ 9068 public AddedItemComponent getAddItemFirstRep() { 9069 if (getAddItem().isEmpty()) { 9070 addAddItem(); 9071 } 9072 return getAddItem().get(0); 9073 } 9074 9075 /** 9076 * @return {@link #adjudication} (The adjudication results which are presented 9077 * at the header level rather than at the line-item or add-item levels.) 9078 */ 9079 public List<AdjudicationComponent> getAdjudication() { 9080 if (this.adjudication == null) 9081 this.adjudication = new ArrayList<AdjudicationComponent>(); 9082 return this.adjudication; 9083 } 9084 9085 /** 9086 * @return Returns a reference to <code>this</code> for easy method chaining 9087 */ 9088 public ClaimResponse setAdjudication(List<AdjudicationComponent> theAdjudication) { 9089 this.adjudication = theAdjudication; 9090 return this; 9091 } 9092 9093 public boolean hasAdjudication() { 9094 if (this.adjudication == null) 9095 return false; 9096 for (AdjudicationComponent item : this.adjudication) 9097 if (!item.isEmpty()) 9098 return true; 9099 return false; 9100 } 9101 9102 public AdjudicationComponent addAdjudication() { // 3 9103 AdjudicationComponent t = new AdjudicationComponent(); 9104 if (this.adjudication == null) 9105 this.adjudication = new ArrayList<AdjudicationComponent>(); 9106 this.adjudication.add(t); 9107 return t; 9108 } 9109 9110 public ClaimResponse addAdjudication(AdjudicationComponent t) { // 3 9111 if (t == null) 9112 return this; 9113 if (this.adjudication == null) 9114 this.adjudication = new ArrayList<AdjudicationComponent>(); 9115 this.adjudication.add(t); 9116 return this; 9117 } 9118 9119 /** 9120 * @return The first repetition of repeating field {@link #adjudication}, 9121 * creating it if it does not already exist 9122 */ 9123 public AdjudicationComponent getAdjudicationFirstRep() { 9124 if (getAdjudication().isEmpty()) { 9125 addAdjudication(); 9126 } 9127 return getAdjudication().get(0); 9128 } 9129 9130 /** 9131 * @return {@link #total} (Categorized monetary totals for the adjudication.) 9132 */ 9133 public List<TotalComponent> getTotal() { 9134 if (this.total == null) 9135 this.total = new ArrayList<TotalComponent>(); 9136 return this.total; 9137 } 9138 9139 /** 9140 * @return Returns a reference to <code>this</code> for easy method chaining 9141 */ 9142 public ClaimResponse setTotal(List<TotalComponent> theTotal) { 9143 this.total = theTotal; 9144 return this; 9145 } 9146 9147 public boolean hasTotal() { 9148 if (this.total == null) 9149 return false; 9150 for (TotalComponent item : this.total) 9151 if (!item.isEmpty()) 9152 return true; 9153 return false; 9154 } 9155 9156 public TotalComponent addTotal() { // 3 9157 TotalComponent t = new TotalComponent(); 9158 if (this.total == null) 9159 this.total = new ArrayList<TotalComponent>(); 9160 this.total.add(t); 9161 return t; 9162 } 9163 9164 public ClaimResponse addTotal(TotalComponent t) { // 3 9165 if (t == null) 9166 return this; 9167 if (this.total == null) 9168 this.total = new ArrayList<TotalComponent>(); 9169 this.total.add(t); 9170 return this; 9171 } 9172 9173 /** 9174 * @return The first repetition of repeating field {@link #total}, creating it 9175 * if it does not already exist 9176 */ 9177 public TotalComponent getTotalFirstRep() { 9178 if (getTotal().isEmpty()) { 9179 addTotal(); 9180 } 9181 return getTotal().get(0); 9182 } 9183 9184 /** 9185 * @return {@link #payment} (Payment details for the adjudication of the claim.) 9186 */ 9187 public PaymentComponent getPayment() { 9188 if (this.payment == null) 9189 if (Configuration.errorOnAutoCreate()) 9190 throw new Error("Attempt to auto-create ClaimResponse.payment"); 9191 else if (Configuration.doAutoCreate()) 9192 this.payment = new PaymentComponent(); // cc 9193 return this.payment; 9194 } 9195 9196 public boolean hasPayment() { 9197 return this.payment != null && !this.payment.isEmpty(); 9198 } 9199 9200 /** 9201 * @param value {@link #payment} (Payment details for the adjudication of the 9202 * claim.) 9203 */ 9204 public ClaimResponse setPayment(PaymentComponent value) { 9205 this.payment = value; 9206 return this; 9207 } 9208 9209 /** 9210 * @return {@link #fundsReserve} (A code, used only on a response to a 9211 * preauthorization, to indicate whether the benefits payable have been 9212 * reserved and for whom.) 9213 */ 9214 public CodeableConcept getFundsReserve() { 9215 if (this.fundsReserve == null) 9216 if (Configuration.errorOnAutoCreate()) 9217 throw new Error("Attempt to auto-create ClaimResponse.fundsReserve"); 9218 else if (Configuration.doAutoCreate()) 9219 this.fundsReserve = new CodeableConcept(); // cc 9220 return this.fundsReserve; 9221 } 9222 9223 public boolean hasFundsReserve() { 9224 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 9225 } 9226 9227 /** 9228 * @param value {@link #fundsReserve} (A code, used only on a response to a 9229 * preauthorization, to indicate whether the benefits payable have 9230 * been reserved and for whom.) 9231 */ 9232 public ClaimResponse setFundsReserve(CodeableConcept value) { 9233 this.fundsReserve = value; 9234 return this; 9235 } 9236 9237 /** 9238 * @return {@link #formCode} (A code for the form to be used for printing the 9239 * content.) 9240 */ 9241 public CodeableConcept getFormCode() { 9242 if (this.formCode == null) 9243 if (Configuration.errorOnAutoCreate()) 9244 throw new Error("Attempt to auto-create ClaimResponse.formCode"); 9245 else if (Configuration.doAutoCreate()) 9246 this.formCode = new CodeableConcept(); // cc 9247 return this.formCode; 9248 } 9249 9250 public boolean hasFormCode() { 9251 return this.formCode != null && !this.formCode.isEmpty(); 9252 } 9253 9254 /** 9255 * @param value {@link #formCode} (A code for the form to be used for printing 9256 * the content.) 9257 */ 9258 public ClaimResponse setFormCode(CodeableConcept value) { 9259 this.formCode = value; 9260 return this; 9261 } 9262 9263 /** 9264 * @return {@link #form} (The actual form, by reference or inclusion, for 9265 * printing the content or an EOB.) 9266 */ 9267 public Attachment getForm() { 9268 if (this.form == null) 9269 if (Configuration.errorOnAutoCreate()) 9270 throw new Error("Attempt to auto-create ClaimResponse.form"); 9271 else if (Configuration.doAutoCreate()) 9272 this.form = new Attachment(); // cc 9273 return this.form; 9274 } 9275 9276 public boolean hasForm() { 9277 return this.form != null && !this.form.isEmpty(); 9278 } 9279 9280 /** 9281 * @param value {@link #form} (The actual form, by reference or inclusion, for 9282 * printing the content or an EOB.) 9283 */ 9284 public ClaimResponse setForm(Attachment value) { 9285 this.form = value; 9286 return this; 9287 } 9288 9289 /** 9290 * @return {@link #processNote} (A note that describes or explains adjudication 9291 * results in a human readable form.) 9292 */ 9293 public List<NoteComponent> getProcessNote() { 9294 if (this.processNote == null) 9295 this.processNote = new ArrayList<NoteComponent>(); 9296 return this.processNote; 9297 } 9298 9299 /** 9300 * @return Returns a reference to <code>this</code> for easy method chaining 9301 */ 9302 public ClaimResponse setProcessNote(List<NoteComponent> theProcessNote) { 9303 this.processNote = theProcessNote; 9304 return this; 9305 } 9306 9307 public boolean hasProcessNote() { 9308 if (this.processNote == null) 9309 return false; 9310 for (NoteComponent item : this.processNote) 9311 if (!item.isEmpty()) 9312 return true; 9313 return false; 9314 } 9315 9316 public NoteComponent addProcessNote() { // 3 9317 NoteComponent t = new NoteComponent(); 9318 if (this.processNote == null) 9319 this.processNote = new ArrayList<NoteComponent>(); 9320 this.processNote.add(t); 9321 return t; 9322 } 9323 9324 public ClaimResponse addProcessNote(NoteComponent t) { // 3 9325 if (t == null) 9326 return this; 9327 if (this.processNote == null) 9328 this.processNote = new ArrayList<NoteComponent>(); 9329 this.processNote.add(t); 9330 return this; 9331 } 9332 9333 /** 9334 * @return The first repetition of repeating field {@link #processNote}, 9335 * creating it if it does not already exist 9336 */ 9337 public NoteComponent getProcessNoteFirstRep() { 9338 if (getProcessNote().isEmpty()) { 9339 addProcessNote(); 9340 } 9341 return getProcessNote().get(0); 9342 } 9343 9344 /** 9345 * @return {@link #communicationRequest} (Request for additional supporting or 9346 * authorizing information.) 9347 */ 9348 public List<Reference> getCommunicationRequest() { 9349 if (this.communicationRequest == null) 9350 this.communicationRequest = new ArrayList<Reference>(); 9351 return this.communicationRequest; 9352 } 9353 9354 /** 9355 * @return Returns a reference to <code>this</code> for easy method chaining 9356 */ 9357 public ClaimResponse setCommunicationRequest(List<Reference> theCommunicationRequest) { 9358 this.communicationRequest = theCommunicationRequest; 9359 return this; 9360 } 9361 9362 public boolean hasCommunicationRequest() { 9363 if (this.communicationRequest == null) 9364 return false; 9365 for (Reference item : this.communicationRequest) 9366 if (!item.isEmpty()) 9367 return true; 9368 return false; 9369 } 9370 9371 public Reference addCommunicationRequest() { // 3 9372 Reference t = new Reference(); 9373 if (this.communicationRequest == null) 9374 this.communicationRequest = new ArrayList<Reference>(); 9375 this.communicationRequest.add(t); 9376 return t; 9377 } 9378 9379 public ClaimResponse addCommunicationRequest(Reference t) { // 3 9380 if (t == null) 9381 return this; 9382 if (this.communicationRequest == null) 9383 this.communicationRequest = new ArrayList<Reference>(); 9384 this.communicationRequest.add(t); 9385 return this; 9386 } 9387 9388 /** 9389 * @return The first repetition of repeating field 9390 * {@link #communicationRequest}, creating it if it does not already 9391 * exist 9392 */ 9393 public Reference getCommunicationRequestFirstRep() { 9394 if (getCommunicationRequest().isEmpty()) { 9395 addCommunicationRequest(); 9396 } 9397 return getCommunicationRequest().get(0); 9398 } 9399 9400 /** 9401 * @deprecated Use Reference#setResource(IBaseResource) instead 9402 */ 9403 @Deprecated 9404 public List<CommunicationRequest> getCommunicationRequestTarget() { 9405 if (this.communicationRequestTarget == null) 9406 this.communicationRequestTarget = new ArrayList<CommunicationRequest>(); 9407 return this.communicationRequestTarget; 9408 } 9409 9410 /** 9411 * @deprecated Use Reference#setResource(IBaseResource) instead 9412 */ 9413 @Deprecated 9414 public CommunicationRequest addCommunicationRequestTarget() { 9415 CommunicationRequest r = new CommunicationRequest(); 9416 if (this.communicationRequestTarget == null) 9417 this.communicationRequestTarget = new ArrayList<CommunicationRequest>(); 9418 this.communicationRequestTarget.add(r); 9419 return r; 9420 } 9421 9422 /** 9423 * @return {@link #insurance} (Financial instruments for reimbursement for the 9424 * health care products and services specified on the claim.) 9425 */ 9426 public List<InsuranceComponent> getInsurance() { 9427 if (this.insurance == null) 9428 this.insurance = new ArrayList<InsuranceComponent>(); 9429 return this.insurance; 9430 } 9431 9432 /** 9433 * @return Returns a reference to <code>this</code> for easy method chaining 9434 */ 9435 public ClaimResponse setInsurance(List<InsuranceComponent> theInsurance) { 9436 this.insurance = theInsurance; 9437 return this; 9438 } 9439 9440 public boolean hasInsurance() { 9441 if (this.insurance == null) 9442 return false; 9443 for (InsuranceComponent item : this.insurance) 9444 if (!item.isEmpty()) 9445 return true; 9446 return false; 9447 } 9448 9449 public InsuranceComponent addInsurance() { // 3 9450 InsuranceComponent t = new InsuranceComponent(); 9451 if (this.insurance == null) 9452 this.insurance = new ArrayList<InsuranceComponent>(); 9453 this.insurance.add(t); 9454 return t; 9455 } 9456 9457 public ClaimResponse addInsurance(InsuranceComponent t) { // 3 9458 if (t == null) 9459 return this; 9460 if (this.insurance == null) 9461 this.insurance = new ArrayList<InsuranceComponent>(); 9462 this.insurance.add(t); 9463 return this; 9464 } 9465 9466 /** 9467 * @return The first repetition of repeating field {@link #insurance}, creating 9468 * it if it does not already exist 9469 */ 9470 public InsuranceComponent getInsuranceFirstRep() { 9471 if (getInsurance().isEmpty()) { 9472 addInsurance(); 9473 } 9474 return getInsurance().get(0); 9475 } 9476 9477 /** 9478 * @return {@link #error} (Errors encountered during the processing of the 9479 * adjudication.) 9480 */ 9481 public List<ErrorComponent> getError() { 9482 if (this.error == null) 9483 this.error = new ArrayList<ErrorComponent>(); 9484 return this.error; 9485 } 9486 9487 /** 9488 * @return Returns a reference to <code>this</code> for easy method chaining 9489 */ 9490 public ClaimResponse setError(List<ErrorComponent> theError) { 9491 this.error = theError; 9492 return this; 9493 } 9494 9495 public boolean hasError() { 9496 if (this.error == null) 9497 return false; 9498 for (ErrorComponent item : this.error) 9499 if (!item.isEmpty()) 9500 return true; 9501 return false; 9502 } 9503 9504 public ErrorComponent addError() { // 3 9505 ErrorComponent t = new ErrorComponent(); 9506 if (this.error == null) 9507 this.error = new ArrayList<ErrorComponent>(); 9508 this.error.add(t); 9509 return t; 9510 } 9511 9512 public ClaimResponse addError(ErrorComponent t) { // 3 9513 if (t == null) 9514 return this; 9515 if (this.error == null) 9516 this.error = new ArrayList<ErrorComponent>(); 9517 this.error.add(t); 9518 return this; 9519 } 9520 9521 /** 9522 * @return The first repetition of repeating field {@link #error}, creating it 9523 * if it does not already exist 9524 */ 9525 public ErrorComponent getErrorFirstRep() { 9526 if (getError().isEmpty()) { 9527 addError(); 9528 } 9529 return getError().get(0); 9530 } 9531 9532 protected void listChildren(List<Property> children) { 9533 super.listChildren(children); 9534 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim response.", 0, 9535 java.lang.Integer.MAX_VALUE, identifier)); 9536 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 9537 children.add(new Property("type", "CodeableConcept", 9538 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9539 0, 1, type)); 9540 children.add(new Property("subType", "CodeableConcept", 9541 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9542 0, 1, subType)); 9543 children.add(new Property("use", "code", 9544 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 9545 0, 1, use)); 9546 children.add(new Property("patient", "Reference(Patient)", 9547 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.", 9548 0, 1, patient)); 9549 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 9550 children.add(new Property("insurer", "Reference(Organization)", 9551 "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer)); 9552 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 9553 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, requestor)); 9554 children.add(new Property("request", "Reference(Claim)", "Original request resource reference.", 0, 1, request)); 9555 children.add(new Property("outcome", "code", 9556 "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome)); 9557 children.add(new Property("disposition", "string", 9558 "A human readable description of the status of the adjudication.", 0, 1, disposition)); 9559 children.add(new Property("preAuthRef", "string", 9560 "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, 9561 preAuthRef)); 9562 children.add(new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 9563 0, 1, preAuthPeriod)); 9564 children.add(new Property("payeeType", "CodeableConcept", 9565 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, payeeType)); 9566 children.add(new Property("item", "", 9567 "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 9568 0, java.lang.Integer.MAX_VALUE, item)); 9569 children.add( 9570 new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, 9571 java.lang.Integer.MAX_VALUE, addItem)); 9572 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", 9573 "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 9574 0, java.lang.Integer.MAX_VALUE, adjudication)); 9575 children.add(new Property("total", "", "Categorized monetary totals for the adjudication.", 0, 9576 java.lang.Integer.MAX_VALUE, total)); 9577 children.add(new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment)); 9578 children.add(new Property("fundsReserve", "CodeableConcept", 9579 "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 9580 0, 1, fundsReserve)); 9581 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 9582 0, 1, formCode)); 9583 children.add(new Property("form", "Attachment", 9584 "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form)); 9585 children.add(new Property("processNote", "", 9586 "A note that describes or explains adjudication results in a human readable form.", 0, 9587 java.lang.Integer.MAX_VALUE, processNote)); 9588 children.add(new Property("communicationRequest", "Reference(CommunicationRequest)", 9589 "Request for additional supporting or authorizing information.", 0, java.lang.Integer.MAX_VALUE, 9590 communicationRequest)); 9591 children.add(new Property("insurance", "", 9592 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, 9593 java.lang.Integer.MAX_VALUE, insurance)); 9594 children.add(new Property("error", "", "Errors encountered during the processing of the adjudication.", 0, 9595 java.lang.Integer.MAX_VALUE, error)); 9596 } 9597 9598 @Override 9599 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9600 switch (_hash) { 9601 case -1618432855: 9602 /* identifier */ return new Property("identifier", "Identifier", 9603 "A unique identifier assigned to this claim response.", 0, java.lang.Integer.MAX_VALUE, identifier); 9604 case -892481550: 9605 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 9606 case 3575610: 9607 /* type */ return new Property("type", "CodeableConcept", 9608 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9609 0, 1, type); 9610 case -1868521062: 9611 /* subType */ return new Property("subType", "CodeableConcept", 9612 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 9613 0, 1, subType); 9614 case 116103: 9615 /* use */ return new Property("use", "code", 9616 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 9617 0, 1, use); 9618 case -791418107: 9619 /* patient */ return new Property("patient", "Reference(Patient)", 9620 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.", 9621 0, 1, patient); 9622 case 1028554472: 9623 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 9624 case 1957615864: 9625 /* insurer */ return new Property("insurer", "Reference(Organization)", 9626 "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer); 9627 case 693934258: 9628 /* requestor */ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 9629 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, requestor); 9630 case 1095692943: 9631 /* request */ return new Property("request", "Reference(Claim)", "Original request resource reference.", 0, 1, 9632 request); 9633 case -1106507950: 9634 /* outcome */ return new Property("outcome", "code", 9635 "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome); 9636 case 583380919: 9637 /* disposition */ return new Property("disposition", "string", 9638 "A human readable description of the status of the adjudication.", 0, 1, disposition); 9639 case 522246568: 9640 /* preAuthRef */ return new Property("preAuthRef", "string", 9641 "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, 9642 preAuthRef); 9643 case 1819164812: 9644 /* preAuthPeriod */ return new Property("preAuthPeriod", "Period", 9645 "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod); 9646 case -316321118: 9647 /* payeeType */ return new Property("payeeType", "CodeableConcept", 9648 "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, payeeType); 9649 case 3242771: 9650 /* item */ return new Property("item", "", 9651 "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 9652 0, java.lang.Integer.MAX_VALUE, item); 9653 case -1148899500: 9654 /* addItem */ return new Property("addItem", "", 9655 "The first-tier service adjudications for payor added product or service lines.", 0, 9656 java.lang.Integer.MAX_VALUE, addItem); 9657 case -231349275: 9658 /* adjudication */ return new Property("adjudication", "@ClaimResponse.item.adjudication", 9659 "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 9660 0, java.lang.Integer.MAX_VALUE, adjudication); 9661 case 110549828: 9662 /* total */ return new Property("total", "", "Categorized monetary totals for the adjudication.", 0, 9663 java.lang.Integer.MAX_VALUE, total); 9664 case -786681338: 9665 /* payment */ return new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, 9666 payment); 9667 case 1314609806: 9668 /* fundsReserve */ return new Property("fundsReserve", "CodeableConcept", 9669 "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 9670 0, 1, fundsReserve); 9671 case 473181393: 9672 /* formCode */ return new Property("formCode", "CodeableConcept", 9673 "A code for the form to be used for printing the content.", 0, 1, formCode); 9674 case 3148996: 9675 /* form */ return new Property("form", "Attachment", 9676 "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form); 9677 case 202339073: 9678 /* processNote */ return new Property("processNote", "", 9679 "A note that describes or explains adjudication results in a human readable form.", 0, 9680 java.lang.Integer.MAX_VALUE, processNote); 9681 case -2071896615: 9682 /* communicationRequest */ return new Property("communicationRequest", "Reference(CommunicationRequest)", 9683 "Request for additional supporting or authorizing information.", 0, java.lang.Integer.MAX_VALUE, 9684 communicationRequest); 9685 case 73049818: 9686 /* insurance */ return new Property("insurance", "", 9687 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 9688 0, java.lang.Integer.MAX_VALUE, insurance); 9689 case 96784904: 9690 /* error */ return new Property("error", "", "Errors encountered during the processing of the adjudication.", 0, 9691 java.lang.Integer.MAX_VALUE, error); 9692 default: 9693 return super.getNamedProperty(_hash, _name, _checkValid); 9694 } 9695 9696 } 9697 9698 @Override 9699 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9700 switch (hash) { 9701 case -1618432855: 9702 /* identifier */ return this.identifier == null ? new Base[0] 9703 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 9704 case -892481550: 9705 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ClaimResponseStatus> 9706 case 3575610: 9707 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 9708 case -1868521062: 9709 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 9710 case 116103: 9711 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<Use> 9712 case -791418107: 9713 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 9714 case 1028554472: 9715 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 9716 case 1957615864: 9717 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 9718 case 693934258: 9719 /* requestor */ return this.requestor == null ? new Base[0] : new Base[] { this.requestor }; // Reference 9720 case 1095692943: 9721 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 9722 case -1106507950: 9723 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<RemittanceOutcome> 9724 case 583380919: 9725 /* disposition */ return this.disposition == null ? new Base[0] : new Base[] { this.disposition }; // StringType 9726 case 522246568: 9727 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] : new Base[] { this.preAuthRef }; // StringType 9728 case 1819164812: 9729 /* preAuthPeriod */ return this.preAuthPeriod == null ? new Base[0] : new Base[] { this.preAuthPeriod }; // Period 9730 case -316321118: 9731 /* payeeType */ return this.payeeType == null ? new Base[0] : new Base[] { this.payeeType }; // CodeableConcept 9732 case 3242771: 9733 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 9734 case -1148899500: 9735 /* addItem */ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 9736 case -231349275: 9737 /* adjudication */ return this.adjudication == null ? new Base[0] 9738 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 9739 case 110549828: 9740 /* total */ return this.total == null ? new Base[0] : this.total.toArray(new Base[this.total.size()]); // TotalComponent 9741 case -786681338: 9742 /* payment */ return this.payment == null ? new Base[0] : new Base[] { this.payment }; // PaymentComponent 9743 case 1314609806: 9744 /* fundsReserve */ return this.fundsReserve == null ? new Base[0] : new Base[] { this.fundsReserve }; // CodeableConcept 9745 case 473181393: 9746 /* formCode */ return this.formCode == null ? new Base[0] : new Base[] { this.formCode }; // CodeableConcept 9747 case 3148996: 9748 /* form */ return this.form == null ? new Base[0] : new Base[] { this.form }; // Attachment 9749 case 202339073: 9750 /* processNote */ return this.processNote == null ? new Base[0] 9751 : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 9752 case -2071896615: 9753 /* communicationRequest */ return this.communicationRequest == null ? new Base[0] 9754 : this.communicationRequest.toArray(new Base[this.communicationRequest.size()]); // Reference 9755 case 73049818: 9756 /* insurance */ return this.insurance == null ? new Base[0] 9757 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 9758 case 96784904: 9759 /* error */ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorComponent 9760 default: 9761 return super.getProperty(hash, name, checkValid); 9762 } 9763 9764 } 9765 9766 @Override 9767 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9768 switch (hash) { 9769 case -1618432855: // identifier 9770 this.getIdentifier().add(castToIdentifier(value)); // Identifier 9771 return value; 9772 case -892481550: // status 9773 value = new ClaimResponseStatusEnumFactory().fromType(castToCode(value)); 9774 this.status = (Enumeration) value; // Enumeration<ClaimResponseStatus> 9775 return value; 9776 case 3575610: // type 9777 this.type = castToCodeableConcept(value); // CodeableConcept 9778 return value; 9779 case -1868521062: // subType 9780 this.subType = castToCodeableConcept(value); // CodeableConcept 9781 return value; 9782 case 116103: // use 9783 value = new UseEnumFactory().fromType(castToCode(value)); 9784 this.use = (Enumeration) value; // Enumeration<Use> 9785 return value; 9786 case -791418107: // patient 9787 this.patient = castToReference(value); // Reference 9788 return value; 9789 case 1028554472: // created 9790 this.created = castToDateTime(value); // DateTimeType 9791 return value; 9792 case 1957615864: // insurer 9793 this.insurer = castToReference(value); // Reference 9794 return value; 9795 case 693934258: // requestor 9796 this.requestor = castToReference(value); // Reference 9797 return value; 9798 case 1095692943: // request 9799 this.request = castToReference(value); // Reference 9800 return value; 9801 case -1106507950: // outcome 9802 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 9803 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 9804 return value; 9805 case 583380919: // disposition 9806 this.disposition = castToString(value); // StringType 9807 return value; 9808 case 522246568: // preAuthRef 9809 this.preAuthRef = castToString(value); // StringType 9810 return value; 9811 case 1819164812: // preAuthPeriod 9812 this.preAuthPeriod = castToPeriod(value); // Period 9813 return value; 9814 case -316321118: // payeeType 9815 this.payeeType = castToCodeableConcept(value); // CodeableConcept 9816 return value; 9817 case 3242771: // item 9818 this.getItem().add((ItemComponent) value); // ItemComponent 9819 return value; 9820 case -1148899500: // addItem 9821 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 9822 return value; 9823 case -231349275: // adjudication 9824 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 9825 return value; 9826 case 110549828: // total 9827 this.getTotal().add((TotalComponent) value); // TotalComponent 9828 return value; 9829 case -786681338: // payment 9830 this.payment = (PaymentComponent) value; // PaymentComponent 9831 return value; 9832 case 1314609806: // fundsReserve 9833 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 9834 return value; 9835 case 473181393: // formCode 9836 this.formCode = castToCodeableConcept(value); // CodeableConcept 9837 return value; 9838 case 3148996: // form 9839 this.form = castToAttachment(value); // Attachment 9840 return value; 9841 case 202339073: // processNote 9842 this.getProcessNote().add((NoteComponent) value); // NoteComponent 9843 return value; 9844 case -2071896615: // communicationRequest 9845 this.getCommunicationRequest().add(castToReference(value)); // Reference 9846 return value; 9847 case 73049818: // insurance 9848 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 9849 return value; 9850 case 96784904: // error 9851 this.getError().add((ErrorComponent) value); // ErrorComponent 9852 return value; 9853 default: 9854 return super.setProperty(hash, name, value); 9855 } 9856 9857 } 9858 9859 @Override 9860 public Base setProperty(String name, Base value) throws FHIRException { 9861 if (name.equals("identifier")) { 9862 this.getIdentifier().add(castToIdentifier(value)); 9863 } else if (name.equals("status")) { 9864 value = new ClaimResponseStatusEnumFactory().fromType(castToCode(value)); 9865 this.status = (Enumeration) value; // Enumeration<ClaimResponseStatus> 9866 } else if (name.equals("type")) { 9867 this.type = castToCodeableConcept(value); // CodeableConcept 9868 } else if (name.equals("subType")) { 9869 this.subType = castToCodeableConcept(value); // CodeableConcept 9870 } else if (name.equals("use")) { 9871 value = new UseEnumFactory().fromType(castToCode(value)); 9872 this.use = (Enumeration) value; // Enumeration<Use> 9873 } else if (name.equals("patient")) { 9874 this.patient = castToReference(value); // Reference 9875 } else if (name.equals("created")) { 9876 this.created = castToDateTime(value); // DateTimeType 9877 } else if (name.equals("insurer")) { 9878 this.insurer = castToReference(value); // Reference 9879 } else if (name.equals("requestor")) { 9880 this.requestor = castToReference(value); // Reference 9881 } else if (name.equals("request")) { 9882 this.request = castToReference(value); // Reference 9883 } else if (name.equals("outcome")) { 9884 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 9885 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 9886 } else if (name.equals("disposition")) { 9887 this.disposition = castToString(value); // StringType 9888 } else if (name.equals("preAuthRef")) { 9889 this.preAuthRef = castToString(value); // StringType 9890 } else if (name.equals("preAuthPeriod")) { 9891 this.preAuthPeriod = castToPeriod(value); // Period 9892 } else if (name.equals("payeeType")) { 9893 this.payeeType = castToCodeableConcept(value); // CodeableConcept 9894 } else if (name.equals("item")) { 9895 this.getItem().add((ItemComponent) value); 9896 } else if (name.equals("addItem")) { 9897 this.getAddItem().add((AddedItemComponent) value); 9898 } else if (name.equals("adjudication")) { 9899 this.getAdjudication().add((AdjudicationComponent) value); 9900 } else if (name.equals("total")) { 9901 this.getTotal().add((TotalComponent) value); 9902 } else if (name.equals("payment")) { 9903 this.payment = (PaymentComponent) value; // PaymentComponent 9904 } else if (name.equals("fundsReserve")) { 9905 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 9906 } else if (name.equals("formCode")) { 9907 this.formCode = castToCodeableConcept(value); // CodeableConcept 9908 } else if (name.equals("form")) { 9909 this.form = castToAttachment(value); // Attachment 9910 } else if (name.equals("processNote")) { 9911 this.getProcessNote().add((NoteComponent) value); 9912 } else if (name.equals("communicationRequest")) { 9913 this.getCommunicationRequest().add(castToReference(value)); 9914 } else if (name.equals("insurance")) { 9915 this.getInsurance().add((InsuranceComponent) value); 9916 } else if (name.equals("error")) { 9917 this.getError().add((ErrorComponent) value); 9918 } else 9919 return super.setProperty(name, value); 9920 return value; 9921 } 9922 9923 @Override 9924 public void removeChild(String name, Base value) throws FHIRException { 9925 if (name.equals("identifier")) { 9926 this.getIdentifier().remove(castToIdentifier(value)); 9927 } else if (name.equals("status")) { 9928 this.status = null; 9929 } else if (name.equals("type")) { 9930 this.type = null; 9931 } else if (name.equals("subType")) { 9932 this.subType = null; 9933 } else if (name.equals("use")) { 9934 this.use = null; 9935 } else if (name.equals("patient")) { 9936 this.patient = null; 9937 } else if (name.equals("created")) { 9938 this.created = null; 9939 } else if (name.equals("insurer")) { 9940 this.insurer = null; 9941 } else if (name.equals("requestor")) { 9942 this.requestor = null; 9943 } else if (name.equals("request")) { 9944 this.request = null; 9945 } else if (name.equals("outcome")) { 9946 this.outcome = null; 9947 } else if (name.equals("disposition")) { 9948 this.disposition = null; 9949 } else if (name.equals("preAuthRef")) { 9950 this.preAuthRef = null; 9951 } else if (name.equals("preAuthPeriod")) { 9952 this.preAuthPeriod = null; 9953 } else if (name.equals("payeeType")) { 9954 this.payeeType = null; 9955 } else if (name.equals("item")) { 9956 this.getItem().remove((ItemComponent) value); 9957 } else if (name.equals("addItem")) { 9958 this.getAddItem().remove((AddedItemComponent) value); 9959 } else if (name.equals("adjudication")) { 9960 this.getAdjudication().remove((AdjudicationComponent) value); 9961 } else if (name.equals("total")) { 9962 this.getTotal().remove((TotalComponent) value); 9963 } else if (name.equals("payment")) { 9964 this.payment = (PaymentComponent) value; // PaymentComponent 9965 } else if (name.equals("fundsReserve")) { 9966 this.fundsReserve = null; 9967 } else if (name.equals("formCode")) { 9968 this.formCode = null; 9969 } else if (name.equals("form")) { 9970 this.form = null; 9971 } else if (name.equals("processNote")) { 9972 this.getProcessNote().remove((NoteComponent) value); 9973 } else if (name.equals("communicationRequest")) { 9974 this.getCommunicationRequest().remove(castToReference(value)); 9975 } else if (name.equals("insurance")) { 9976 this.getInsurance().remove((InsuranceComponent) value); 9977 } else if (name.equals("error")) { 9978 this.getError().remove((ErrorComponent) value); 9979 } else 9980 super.removeChild(name, value); 9981 9982 } 9983 9984 @Override 9985 public Base makeProperty(int hash, String name) throws FHIRException { 9986 switch (hash) { 9987 case -1618432855: 9988 return addIdentifier(); 9989 case -892481550: 9990 return getStatusElement(); 9991 case 3575610: 9992 return getType(); 9993 case -1868521062: 9994 return getSubType(); 9995 case 116103: 9996 return getUseElement(); 9997 case -791418107: 9998 return getPatient(); 9999 case 1028554472: 10000 return getCreatedElement(); 10001 case 1957615864: 10002 return getInsurer(); 10003 case 693934258: 10004 return getRequestor(); 10005 case 1095692943: 10006 return getRequest(); 10007 case -1106507950: 10008 return getOutcomeElement(); 10009 case 583380919: 10010 return getDispositionElement(); 10011 case 522246568: 10012 return getPreAuthRefElement(); 10013 case 1819164812: 10014 return getPreAuthPeriod(); 10015 case -316321118: 10016 return getPayeeType(); 10017 case 3242771: 10018 return addItem(); 10019 case -1148899500: 10020 return addAddItem(); 10021 case -231349275: 10022 return addAdjudication(); 10023 case 110549828: 10024 return addTotal(); 10025 case -786681338: 10026 return getPayment(); 10027 case 1314609806: 10028 return getFundsReserve(); 10029 case 473181393: 10030 return getFormCode(); 10031 case 3148996: 10032 return getForm(); 10033 case 202339073: 10034 return addProcessNote(); 10035 case -2071896615: 10036 return addCommunicationRequest(); 10037 case 73049818: 10038 return addInsurance(); 10039 case 96784904: 10040 return addError(); 10041 default: 10042 return super.makeProperty(hash, name); 10043 } 10044 10045 } 10046 10047 @Override 10048 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10049 switch (hash) { 10050 case -1618432855: 10051 /* identifier */ return new String[] { "Identifier" }; 10052 case -892481550: 10053 /* status */ return new String[] { "code" }; 10054 case 3575610: 10055 /* type */ return new String[] { "CodeableConcept" }; 10056 case -1868521062: 10057 /* subType */ return new String[] { "CodeableConcept" }; 10058 case 116103: 10059 /* use */ return new String[] { "code" }; 10060 case -791418107: 10061 /* patient */ return new String[] { "Reference" }; 10062 case 1028554472: 10063 /* created */ return new String[] { "dateTime" }; 10064 case 1957615864: 10065 /* insurer */ return new String[] { "Reference" }; 10066 case 693934258: 10067 /* requestor */ return new String[] { "Reference" }; 10068 case 1095692943: 10069 /* request */ return new String[] { "Reference" }; 10070 case -1106507950: 10071 /* outcome */ return new String[] { "code" }; 10072 case 583380919: 10073 /* disposition */ return new String[] { "string" }; 10074 case 522246568: 10075 /* preAuthRef */ return new String[] { "string" }; 10076 case 1819164812: 10077 /* preAuthPeriod */ return new String[] { "Period" }; 10078 case -316321118: 10079 /* payeeType */ return new String[] { "CodeableConcept" }; 10080 case 3242771: 10081 /* item */ return new String[] {}; 10082 case -1148899500: 10083 /* addItem */ return new String[] {}; 10084 case -231349275: 10085 /* adjudication */ return new String[] { "@ClaimResponse.item.adjudication" }; 10086 case 110549828: 10087 /* total */ return new String[] {}; 10088 case -786681338: 10089 /* payment */ return new String[] {}; 10090 case 1314609806: 10091 /* fundsReserve */ return new String[] { "CodeableConcept" }; 10092 case 473181393: 10093 /* formCode */ return new String[] { "CodeableConcept" }; 10094 case 3148996: 10095 /* form */ return new String[] { "Attachment" }; 10096 case 202339073: 10097 /* processNote */ return new String[] {}; 10098 case -2071896615: 10099 /* communicationRequest */ return new String[] { "Reference" }; 10100 case 73049818: 10101 /* insurance */ return new String[] {}; 10102 case 96784904: 10103 /* error */ return new String[] {}; 10104 default: 10105 return super.getTypesForProperty(hash, name); 10106 } 10107 10108 } 10109 10110 @Override 10111 public Base addChild(String name) throws FHIRException { 10112 if (name.equals("identifier")) { 10113 return addIdentifier(); 10114 } else if (name.equals("status")) { 10115 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.status"); 10116 } else if (name.equals("type")) { 10117 this.type = new CodeableConcept(); 10118 return this.type; 10119 } else if (name.equals("subType")) { 10120 this.subType = new CodeableConcept(); 10121 return this.subType; 10122 } else if (name.equals("use")) { 10123 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.use"); 10124 } else if (name.equals("patient")) { 10125 this.patient = new Reference(); 10126 return this.patient; 10127 } else if (name.equals("created")) { 10128 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.created"); 10129 } else if (name.equals("insurer")) { 10130 this.insurer = new Reference(); 10131 return this.insurer; 10132 } else if (name.equals("requestor")) { 10133 this.requestor = new Reference(); 10134 return this.requestor; 10135 } else if (name.equals("request")) { 10136 this.request = new Reference(); 10137 return this.request; 10138 } else if (name.equals("outcome")) { 10139 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.outcome"); 10140 } else if (name.equals("disposition")) { 10141 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.disposition"); 10142 } else if (name.equals("preAuthRef")) { 10143 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.preAuthRef"); 10144 } else if (name.equals("preAuthPeriod")) { 10145 this.preAuthPeriod = new Period(); 10146 return this.preAuthPeriod; 10147 } else if (name.equals("payeeType")) { 10148 this.payeeType = new CodeableConcept(); 10149 return this.payeeType; 10150 } else if (name.equals("item")) { 10151 return addItem(); 10152 } else if (name.equals("addItem")) { 10153 return addAddItem(); 10154 } else if (name.equals("adjudication")) { 10155 return addAdjudication(); 10156 } else if (name.equals("total")) { 10157 return addTotal(); 10158 } else if (name.equals("payment")) { 10159 this.payment = new PaymentComponent(); 10160 return this.payment; 10161 } else if (name.equals("fundsReserve")) { 10162 this.fundsReserve = new CodeableConcept(); 10163 return this.fundsReserve; 10164 } else if (name.equals("formCode")) { 10165 this.formCode = new CodeableConcept(); 10166 return this.formCode; 10167 } else if (name.equals("form")) { 10168 this.form = new Attachment(); 10169 return this.form; 10170 } else if (name.equals("processNote")) { 10171 return addProcessNote(); 10172 } else if (name.equals("communicationRequest")) { 10173 return addCommunicationRequest(); 10174 } else if (name.equals("insurance")) { 10175 return addInsurance(); 10176 } else if (name.equals("error")) { 10177 return addError(); 10178 } else 10179 return super.addChild(name); 10180 } 10181 10182 public String fhirType() { 10183 return "ClaimResponse"; 10184 10185 } 10186 10187 public ClaimResponse copy() { 10188 ClaimResponse dst = new ClaimResponse(); 10189 copyValues(dst); 10190 return dst; 10191 } 10192 10193 public void copyValues(ClaimResponse dst) { 10194 super.copyValues(dst); 10195 if (identifier != null) { 10196 dst.identifier = new ArrayList<Identifier>(); 10197 for (Identifier i : identifier) 10198 dst.identifier.add(i.copy()); 10199 } 10200 ; 10201 dst.status = status == null ? null : status.copy(); 10202 dst.type = type == null ? null : type.copy(); 10203 dst.subType = subType == null ? null : subType.copy(); 10204 dst.use = use == null ? null : use.copy(); 10205 dst.patient = patient == null ? null : patient.copy(); 10206 dst.created = created == null ? null : created.copy(); 10207 dst.insurer = insurer == null ? null : insurer.copy(); 10208 dst.requestor = requestor == null ? null : requestor.copy(); 10209 dst.request = request == null ? null : request.copy(); 10210 dst.outcome = outcome == null ? null : outcome.copy(); 10211 dst.disposition = disposition == null ? null : disposition.copy(); 10212 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 10213 dst.preAuthPeriod = preAuthPeriod == null ? null : preAuthPeriod.copy(); 10214 dst.payeeType = payeeType == null ? null : payeeType.copy(); 10215 if (item != null) { 10216 dst.item = new ArrayList<ItemComponent>(); 10217 for (ItemComponent i : item) 10218 dst.item.add(i.copy()); 10219 } 10220 ; 10221 if (addItem != null) { 10222 dst.addItem = new ArrayList<AddedItemComponent>(); 10223 for (AddedItemComponent i : addItem) 10224 dst.addItem.add(i.copy()); 10225 } 10226 ; 10227 if (adjudication != null) { 10228 dst.adjudication = new ArrayList<AdjudicationComponent>(); 10229 for (AdjudicationComponent i : adjudication) 10230 dst.adjudication.add(i.copy()); 10231 } 10232 ; 10233 if (total != null) { 10234 dst.total = new ArrayList<TotalComponent>(); 10235 for (TotalComponent i : total) 10236 dst.total.add(i.copy()); 10237 } 10238 ; 10239 dst.payment = payment == null ? null : payment.copy(); 10240 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 10241 dst.formCode = formCode == null ? null : formCode.copy(); 10242 dst.form = form == null ? null : form.copy(); 10243 if (processNote != null) { 10244 dst.processNote = new ArrayList<NoteComponent>(); 10245 for (NoteComponent i : processNote) 10246 dst.processNote.add(i.copy()); 10247 } 10248 ; 10249 if (communicationRequest != null) { 10250 dst.communicationRequest = new ArrayList<Reference>(); 10251 for (Reference i : communicationRequest) 10252 dst.communicationRequest.add(i.copy()); 10253 } 10254 ; 10255 if (insurance != null) { 10256 dst.insurance = new ArrayList<InsuranceComponent>(); 10257 for (InsuranceComponent i : insurance) 10258 dst.insurance.add(i.copy()); 10259 } 10260 ; 10261 if (error != null) { 10262 dst.error = new ArrayList<ErrorComponent>(); 10263 for (ErrorComponent i : error) 10264 dst.error.add(i.copy()); 10265 } 10266 ; 10267 } 10268 10269 protected ClaimResponse typedCopy() { 10270 return copy(); 10271 } 10272 10273 @Override 10274 public boolean equalsDeep(Base other_) { 10275 if (!super.equalsDeep(other_)) 10276 return false; 10277 if (!(other_ instanceof ClaimResponse)) 10278 return false; 10279 ClaimResponse o = (ClaimResponse) other_; 10280 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 10281 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) 10282 && compareDeep(patient, o.patient, true) && compareDeep(created, o.created, true) 10283 && compareDeep(insurer, o.insurer, true) && compareDeep(requestor, o.requestor, true) 10284 && compareDeep(request, o.request, true) && compareDeep(outcome, o.outcome, true) 10285 && compareDeep(disposition, o.disposition, true) && compareDeep(preAuthRef, o.preAuthRef, true) 10286 && compareDeep(preAuthPeriod, o.preAuthPeriod, true) && compareDeep(payeeType, o.payeeType, true) 10287 && compareDeep(item, o.item, true) && compareDeep(addItem, o.addItem, true) 10288 && compareDeep(adjudication, o.adjudication, true) && compareDeep(total, o.total, true) 10289 && compareDeep(payment, o.payment, true) && compareDeep(fundsReserve, o.fundsReserve, true) 10290 && compareDeep(formCode, o.formCode, true) && compareDeep(form, o.form, true) 10291 && compareDeep(processNote, o.processNote, true) 10292 && compareDeep(communicationRequest, o.communicationRequest, true) && compareDeep(insurance, o.insurance, true) 10293 && compareDeep(error, o.error, true); 10294 } 10295 10296 @Override 10297 public boolean equalsShallow(Base other_) { 10298 if (!super.equalsShallow(other_)) 10299 return false; 10300 if (!(other_ instanceof ClaimResponse)) 10301 return false; 10302 ClaimResponse o = (ClaimResponse) other_; 10303 return compareValues(status, o.status, true) && compareValues(use, o.use, true) 10304 && compareValues(created, o.created, true) && compareValues(outcome, o.outcome, true) 10305 && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true); 10306 } 10307 10308 public boolean isEmpty() { 10309 return super.isEmpty() 10310 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, subType, use, patient, created, insurer, 10311 requestor, request, outcome, disposition, preAuthRef, preAuthPeriod, payeeType, item, addItem, adjudication, 10312 total, payment, fundsReserve, formCode, form, processNote, communicationRequest, insurance, error); 10313 } 10314 10315 @Override 10316 public ResourceType getResourceType() { 10317 return ResourceType.ClaimResponse; 10318 } 10319 10320 /** 10321 * Search parameter: <b>identifier</b> 10322 * <p> 10323 * Description: <b>The identity of the ClaimResponse</b><br> 10324 * Type: <b>token</b><br> 10325 * Path: <b>ClaimResponse.identifier</b><br> 10326 * </p> 10327 */ 10328 @SearchParamDefinition(name = "identifier", path = "ClaimResponse.identifier", description = "The identity of the ClaimResponse", type = "token") 10329 public static final String SP_IDENTIFIER = "identifier"; 10330 /** 10331 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10332 * <p> 10333 * Description: <b>The identity of the ClaimResponse</b><br> 10334 * Type: <b>token</b><br> 10335 * Path: <b>ClaimResponse.identifier</b><br> 10336 * </p> 10337 */ 10338 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10339 SP_IDENTIFIER); 10340 10341 /** 10342 * Search parameter: <b>request</b> 10343 * <p> 10344 * Description: <b>The claim reference</b><br> 10345 * Type: <b>reference</b><br> 10346 * Path: <b>ClaimResponse.request</b><br> 10347 * </p> 10348 */ 10349 @SearchParamDefinition(name = "request", path = "ClaimResponse.request", description = "The claim reference", type = "reference", target = { 10350 Claim.class }) 10351 public static final String SP_REQUEST = "request"; 10352 /** 10353 * <b>Fluent Client</b> search parameter constant for <b>request</b> 10354 * <p> 10355 * Description: <b>The claim reference</b><br> 10356 * Type: <b>reference</b><br> 10357 * Path: <b>ClaimResponse.request</b><br> 10358 * </p> 10359 */ 10360 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10361 SP_REQUEST); 10362 10363 /** 10364 * Constant for fluent queries to be used to add include statements. Specifies 10365 * the path value of "<b>ClaimResponse:request</b>". 10366 */ 10367 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 10368 "ClaimResponse:request").toLocked(); 10369 10370 /** 10371 * Search parameter: <b>disposition</b> 10372 * <p> 10373 * Description: <b>The contents of the disposition message</b><br> 10374 * Type: <b>string</b><br> 10375 * Path: <b>ClaimResponse.disposition</b><br> 10376 * </p> 10377 */ 10378 @SearchParamDefinition(name = "disposition", path = "ClaimResponse.disposition", description = "The contents of the disposition message", type = "string") 10379 public static final String SP_DISPOSITION = "disposition"; 10380 /** 10381 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 10382 * <p> 10383 * Description: <b>The contents of the disposition message</b><br> 10384 * Type: <b>string</b><br> 10385 * Path: <b>ClaimResponse.disposition</b><br> 10386 * </p> 10387 */ 10388 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam( 10389 SP_DISPOSITION); 10390 10391 /** 10392 * Search parameter: <b>insurer</b> 10393 * <p> 10394 * Description: <b>The organization which generated this resource</b><br> 10395 * Type: <b>reference</b><br> 10396 * Path: <b>ClaimResponse.insurer</b><br> 10397 * </p> 10398 */ 10399 @SearchParamDefinition(name = "insurer", path = "ClaimResponse.insurer", description = "The organization which generated this resource", type = "reference", target = { 10400 Organization.class }) 10401 public static final String SP_INSURER = "insurer"; 10402 /** 10403 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 10404 * <p> 10405 * Description: <b>The organization which generated this resource</b><br> 10406 * Type: <b>reference</b><br> 10407 * Path: <b>ClaimResponse.insurer</b><br> 10408 * </p> 10409 */ 10410 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10411 SP_INSURER); 10412 10413 /** 10414 * Constant for fluent queries to be used to add include statements. Specifies 10415 * the path value of "<b>ClaimResponse:insurer</b>". 10416 */ 10417 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include( 10418 "ClaimResponse:insurer").toLocked(); 10419 10420 /** 10421 * Search parameter: <b>created</b> 10422 * <p> 10423 * Description: <b>The creation date</b><br> 10424 * Type: <b>date</b><br> 10425 * Path: <b>ClaimResponse.created</b><br> 10426 * </p> 10427 */ 10428 @SearchParamDefinition(name = "created", path = "ClaimResponse.created", description = "The creation date", type = "date") 10429 public static final String SP_CREATED = "created"; 10430 /** 10431 * <b>Fluent Client</b> search parameter constant for <b>created</b> 10432 * <p> 10433 * Description: <b>The creation date</b><br> 10434 * Type: <b>date</b><br> 10435 * Path: <b>ClaimResponse.created</b><br> 10436 * </p> 10437 */ 10438 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 10439 SP_CREATED); 10440 10441 /** 10442 * Search parameter: <b>patient</b> 10443 * <p> 10444 * Description: <b>The subject of care</b><br> 10445 * Type: <b>reference</b><br> 10446 * Path: <b>ClaimResponse.patient</b><br> 10447 * </p> 10448 */ 10449 @SearchParamDefinition(name = "patient", path = "ClaimResponse.patient", description = "The subject of care", type = "reference", providesMembershipIn = { 10450 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 10451 public static final String SP_PATIENT = "patient"; 10452 /** 10453 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 10454 * <p> 10455 * Description: <b>The subject of care</b><br> 10456 * Type: <b>reference</b><br> 10457 * Path: <b>ClaimResponse.patient</b><br> 10458 * </p> 10459 */ 10460 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10461 SP_PATIENT); 10462 10463 /** 10464 * Constant for fluent queries to be used to add include statements. Specifies 10465 * the path value of "<b>ClaimResponse:patient</b>". 10466 */ 10467 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 10468 "ClaimResponse:patient").toLocked(); 10469 10470 /** 10471 * Search parameter: <b>use</b> 10472 * <p> 10473 * Description: <b>The type of claim</b><br> 10474 * Type: <b>token</b><br> 10475 * Path: <b>ClaimResponse.use</b><br> 10476 * </p> 10477 */ 10478 @SearchParamDefinition(name = "use", path = "ClaimResponse.use", description = "The type of claim", type = "token") 10479 public static final String SP_USE = "use"; 10480 /** 10481 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10482 * <p> 10483 * Description: <b>The type of claim</b><br> 10484 * Type: <b>token</b><br> 10485 * Path: <b>ClaimResponse.use</b><br> 10486 * </p> 10487 */ 10488 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10489 SP_USE); 10490 10491 /** 10492 * Search parameter: <b>payment-date</b> 10493 * <p> 10494 * Description: <b>The expected payment date</b><br> 10495 * Type: <b>date</b><br> 10496 * Path: <b>ClaimResponse.payment.date</b><br> 10497 * </p> 10498 */ 10499 @SearchParamDefinition(name = "payment-date", path = "ClaimResponse.payment.date", description = "The expected payment date", type = "date") 10500 public static final String SP_PAYMENT_DATE = "payment-date"; 10501 /** 10502 * <b>Fluent Client</b> search parameter constant for <b>payment-date</b> 10503 * <p> 10504 * Description: <b>The expected payment date</b><br> 10505 * Type: <b>date</b><br> 10506 * Path: <b>ClaimResponse.payment.date</b><br> 10507 * </p> 10508 */ 10509 public static final ca.uhn.fhir.rest.gclient.DateClientParam PAYMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 10510 SP_PAYMENT_DATE); 10511 10512 /** 10513 * Search parameter: <b>outcome</b> 10514 * <p> 10515 * Description: <b>The processing outcome</b><br> 10516 * Type: <b>token</b><br> 10517 * Path: <b>ClaimResponse.outcome</b><br> 10518 * </p> 10519 */ 10520 @SearchParamDefinition(name = "outcome", path = "ClaimResponse.outcome", description = "The processing outcome", type = "token") 10521 public static final String SP_OUTCOME = "outcome"; 10522 /** 10523 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 10524 * <p> 10525 * Description: <b>The processing outcome</b><br> 10526 * Type: <b>token</b><br> 10527 * Path: <b>ClaimResponse.outcome</b><br> 10528 * </p> 10529 */ 10530 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10531 SP_OUTCOME); 10532 10533 /** 10534 * Search parameter: <b>requestor</b> 10535 * <p> 10536 * Description: <b>The Provider of the claim</b><br> 10537 * Type: <b>reference</b><br> 10538 * Path: <b>ClaimResponse.requestor</b><br> 10539 * </p> 10540 */ 10541 @SearchParamDefinition(name = "requestor", path = "ClaimResponse.requestor", description = "The Provider of the claim", type = "reference", providesMembershipIn = { 10542 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 10543 Practitioner.class, PractitionerRole.class }) 10544 public static final String SP_REQUESTOR = "requestor"; 10545 /** 10546 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 10547 * <p> 10548 * Description: <b>The Provider of the claim</b><br> 10549 * Type: <b>reference</b><br> 10550 * Path: <b>ClaimResponse.requestor</b><br> 10551 * </p> 10552 */ 10553 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 10554 SP_REQUESTOR); 10555 10556 /** 10557 * Constant for fluent queries to be used to add include statements. Specifies 10558 * the path value of "<b>ClaimResponse:requestor</b>". 10559 */ 10560 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include( 10561 "ClaimResponse:requestor").toLocked(); 10562 10563 /** 10564 * Search parameter: <b>status</b> 10565 * <p> 10566 * Description: <b>The status of the ClaimResponse</b><br> 10567 * Type: <b>token</b><br> 10568 * Path: <b>ClaimResponse.status</b><br> 10569 * </p> 10570 */ 10571 @SearchParamDefinition(name = "status", path = "ClaimResponse.status", description = "The status of the ClaimResponse", type = "token") 10572 public static final String SP_STATUS = "status"; 10573 /** 10574 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10575 * <p> 10576 * Description: <b>The status of the ClaimResponse</b><br> 10577 * Type: <b>token</b><br> 10578 * Path: <b>ClaimResponse.status</b><br> 10579 * </p> 10580 */ 10581 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 10582 SP_STATUS); 10583 10584}