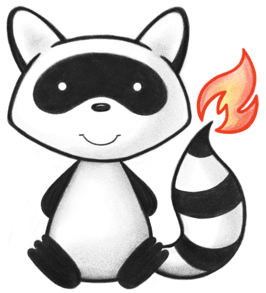
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A record of a clinical assessment performed to determine what problem(s) may 049 * affect the patient and before planning the treatments or management 050 * strategies that are best to manage a patient's condition. Assessments are 051 * often 1:1 with a clinical consultation / encounter, but this varies greatly 052 * depending on the clinical workflow. This resource is called 053 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 054 * the recording of assessment tools such as Apgar score. 055 */ 056@ResourceDef(name = "ClinicalImpression", profile = "http://hl7.org/fhir/StructureDefinition/ClinicalImpression") 057public class ClinicalImpression extends DomainResource { 058 059 public enum ClinicalImpressionStatus { 060 /** 061 * null 062 */ 063 INPROGRESS, 064 /** 065 * null 066 */ 067 COMPLETED, 068 /** 069 * null 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 077 public static ClinicalImpressionStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("in-progress".equals(codeString)) 081 return INPROGRESS; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case INPROGRESS: 095 return "in-progress"; 096 case COMPLETED: 097 return "completed"; 098 case ENTEREDINERROR: 099 return "entered-in-error"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case INPROGRESS: 110 return "http://hl7.org/fhir/event-status"; 111 case COMPLETED: 112 return "http://hl7.org/fhir/event-status"; 113 case ENTEREDINERROR: 114 return "http://hl7.org/fhir/event-status"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case INPROGRESS: 125 return ""; 126 case COMPLETED: 127 return ""; 128 case ENTEREDINERROR: 129 return ""; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDisplay() { 138 switch (this) { 139 case INPROGRESS: 140 return "in-progress"; 141 case COMPLETED: 142 return "completed"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 } 152 153 public static class ClinicalImpressionStatusEnumFactory implements EnumFactory<ClinicalImpressionStatus> { 154 public ClinicalImpressionStatus fromCode(String codeString) throws IllegalArgumentException { 155 if (codeString == null || "".equals(codeString)) 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("in-progress".equals(codeString)) 159 return ClinicalImpressionStatus.INPROGRESS; 160 if ("completed".equals(codeString)) 161 return ClinicalImpressionStatus.COMPLETED; 162 if ("entered-in-error".equals(codeString)) 163 return ClinicalImpressionStatus.ENTEREDINERROR; 164 throw new IllegalArgumentException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 165 } 166 167 public Enumeration<ClinicalImpressionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 168 if (code == null) 169 return null; 170 if (code.isEmpty()) 171 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.NULL, code); 172 String codeString = code.asStringValue(); 173 if (codeString == null || "".equals(codeString)) 174 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.NULL, code); 175 if ("in-progress".equals(codeString)) 176 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.INPROGRESS, code); 177 if ("completed".equals(codeString)) 178 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.COMPLETED, code); 179 if ("entered-in-error".equals(codeString)) 180 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.ENTEREDINERROR, code); 181 throw new FHIRException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 182 } 183 184 public String toCode(ClinicalImpressionStatus code) { 185 if (code == ClinicalImpressionStatus.NULL) 186 return null; 187 if (code == ClinicalImpressionStatus.INPROGRESS) 188 return "in-progress"; 189 if (code == ClinicalImpressionStatus.COMPLETED) 190 return "completed"; 191 if (code == ClinicalImpressionStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 return "?"; 194 } 195 196 public String toSystem(ClinicalImpressionStatus code) { 197 return code.getSystem(); 198 } 199 } 200 201 @Block() 202 public static class ClinicalImpressionInvestigationComponent extends BackboneElement implements IBaseBackboneElement { 203 /** 204 * A name/code for the group ("set") of investigations. Typically, this will be 205 * something like "signs", "symptoms", "clinical", "diagnostic", but the list is 206 * not constrained, and others such groups such as 207 * (exposure|family|travel|nutritional) history may be used. 208 */ 209 @Child(name = "code", type = { 210 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 211 @Description(shortDefinition = "A name/code for the set", formalDefinition = "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutritional) history may be used.") 212 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/investigation-sets") 213 protected CodeableConcept code; 214 215 /** 216 * A record of a specific investigation that was undertaken. 217 */ 218 @Child(name = "item", type = { Observation.class, QuestionnaireResponse.class, FamilyMemberHistory.class, 219 DiagnosticReport.class, RiskAssessment.class, ImagingStudy.class, 220 Media.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 221 @Description(shortDefinition = "Record of a specific investigation", formalDefinition = "A record of a specific investigation that was undertaken.") 222 protected List<Reference> item; 223 /** 224 * The actual objects that are the target of the reference (A record of a 225 * specific investigation that was undertaken.) 226 */ 227 protected List<Resource> itemTarget; 228 229 private static final long serialVersionUID = -301363326L; 230 231 /** 232 * Constructor 233 */ 234 public ClinicalImpressionInvestigationComponent() { 235 super(); 236 } 237 238 /** 239 * Constructor 240 */ 241 public ClinicalImpressionInvestigationComponent(CodeableConcept code) { 242 super(); 243 this.code = code; 244 } 245 246 /** 247 * @return {@link #code} (A name/code for the group ("set") of investigations. 248 * Typically, this will be something like "signs", "symptoms", 249 * "clinical", "diagnostic", but the list is not constrained, and others 250 * such groups such as (exposure|family|travel|nutritional) history may 251 * be used.) 252 */ 253 public CodeableConcept getCode() { 254 if (this.code == null) 255 if (Configuration.errorOnAutoCreate()) 256 throw new Error("Attempt to auto-create ClinicalImpressionInvestigationComponent.code"); 257 else if (Configuration.doAutoCreate()) 258 this.code = new CodeableConcept(); // cc 259 return this.code; 260 } 261 262 public boolean hasCode() { 263 return this.code != null && !this.code.isEmpty(); 264 } 265 266 /** 267 * @param value {@link #code} (A name/code for the group ("set") of 268 * investigations. Typically, this will be something like "signs", 269 * "symptoms", "clinical", "diagnostic", but the list is not 270 * constrained, and others such groups such as 271 * (exposure|family|travel|nutritional) history may be used.) 272 */ 273 public ClinicalImpressionInvestigationComponent setCode(CodeableConcept value) { 274 this.code = value; 275 return this; 276 } 277 278 /** 279 * @return {@link #item} (A record of a specific investigation that was 280 * undertaken.) 281 */ 282 public List<Reference> getItem() { 283 if (this.item == null) 284 this.item = new ArrayList<Reference>(); 285 return this.item; 286 } 287 288 /** 289 * @return Returns a reference to <code>this</code> for easy method chaining 290 */ 291 public ClinicalImpressionInvestigationComponent setItem(List<Reference> theItem) { 292 this.item = theItem; 293 return this; 294 } 295 296 public boolean hasItem() { 297 if (this.item == null) 298 return false; 299 for (Reference item : this.item) 300 if (!item.isEmpty()) 301 return true; 302 return false; 303 } 304 305 public Reference addItem() { // 3 306 Reference t = new Reference(); 307 if (this.item == null) 308 this.item = new ArrayList<Reference>(); 309 this.item.add(t); 310 return t; 311 } 312 313 public ClinicalImpressionInvestigationComponent addItem(Reference t) { // 3 314 if (t == null) 315 return this; 316 if (this.item == null) 317 this.item = new ArrayList<Reference>(); 318 this.item.add(t); 319 return this; 320 } 321 322 /** 323 * @return The first repetition of repeating field {@link #item}, creating it if 324 * it does not already exist 325 */ 326 public Reference getItemFirstRep() { 327 if (getItem().isEmpty()) { 328 addItem(); 329 } 330 return getItem().get(0); 331 } 332 333 protected void listChildren(List<Property> children) { 334 super.listChildren(children); 335 children.add(new Property("code", "CodeableConcept", 336 "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutritional) history may be used.", 337 0, 1, code)); 338 children.add(new Property("item", 339 "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport|RiskAssessment|ImagingStudy|Media)", 340 "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item)); 341 } 342 343 @Override 344 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 345 switch (_hash) { 346 case 3059181: 347 /* code */ return new Property("code", "CodeableConcept", 348 "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutritional) history may be used.", 349 0, 1, code); 350 case 3242771: 351 /* item */ return new Property("item", 352 "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport|RiskAssessment|ImagingStudy|Media)", 353 "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item); 354 default: 355 return super.getNamedProperty(_hash, _name, _checkValid); 356 } 357 358 } 359 360 @Override 361 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 362 switch (hash) { 363 case 3059181: 364 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 365 case 3242771: 366 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // Reference 367 default: 368 return super.getProperty(hash, name, checkValid); 369 } 370 371 } 372 373 @Override 374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 375 switch (hash) { 376 case 3059181: // code 377 this.code = castToCodeableConcept(value); // CodeableConcept 378 return value; 379 case 3242771: // item 380 this.getItem().add(castToReference(value)); // Reference 381 return value; 382 default: 383 return super.setProperty(hash, name, value); 384 } 385 386 } 387 388 @Override 389 public Base setProperty(String name, Base value) throws FHIRException { 390 if (name.equals("code")) { 391 this.code = castToCodeableConcept(value); // CodeableConcept 392 } else if (name.equals("item")) { 393 this.getItem().add(castToReference(value)); 394 } else 395 return super.setProperty(name, value); 396 return value; 397 } 398 399 @Override 400 public void removeChild(String name, Base value) throws FHIRException { 401 if (name.equals("code")) { 402 this.code = null; 403 } else if (name.equals("item")) { 404 this.getItem().remove(castToReference(value)); 405 } else 406 super.removeChild(name, value); 407 408 } 409 410 @Override 411 public Base makeProperty(int hash, String name) throws FHIRException { 412 switch (hash) { 413 case 3059181: 414 return getCode(); 415 case 3242771: 416 return addItem(); 417 default: 418 return super.makeProperty(hash, name); 419 } 420 421 } 422 423 @Override 424 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 425 switch (hash) { 426 case 3059181: 427 /* code */ return new String[] { "CodeableConcept" }; 428 case 3242771: 429 /* item */ return new String[] { "Reference" }; 430 default: 431 return super.getTypesForProperty(hash, name); 432 } 433 434 } 435 436 @Override 437 public Base addChild(String name) throws FHIRException { 438 if (name.equals("code")) { 439 this.code = new CodeableConcept(); 440 return this.code; 441 } else if (name.equals("item")) { 442 return addItem(); 443 } else 444 return super.addChild(name); 445 } 446 447 public ClinicalImpressionInvestigationComponent copy() { 448 ClinicalImpressionInvestigationComponent dst = new ClinicalImpressionInvestigationComponent(); 449 copyValues(dst); 450 return dst; 451 } 452 453 public void copyValues(ClinicalImpressionInvestigationComponent dst) { 454 super.copyValues(dst); 455 dst.code = code == null ? null : code.copy(); 456 if (item != null) { 457 dst.item = new ArrayList<Reference>(); 458 for (Reference i : item) 459 dst.item.add(i.copy()); 460 } 461 ; 462 } 463 464 @Override 465 public boolean equalsDeep(Base other_) { 466 if (!super.equalsDeep(other_)) 467 return false; 468 if (!(other_ instanceof ClinicalImpressionInvestigationComponent)) 469 return false; 470 ClinicalImpressionInvestigationComponent o = (ClinicalImpressionInvestigationComponent) other_; 471 return compareDeep(code, o.code, true) && compareDeep(item, o.item, true); 472 } 473 474 @Override 475 public boolean equalsShallow(Base other_) { 476 if (!super.equalsShallow(other_)) 477 return false; 478 if (!(other_ instanceof ClinicalImpressionInvestigationComponent)) 479 return false; 480 ClinicalImpressionInvestigationComponent o = (ClinicalImpressionInvestigationComponent) other_; 481 return true; 482 } 483 484 public boolean isEmpty() { 485 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, item); 486 } 487 488 public String fhirType() { 489 return "ClinicalImpression.investigation"; 490 491 } 492 493 } 494 495 @Block() 496 public static class ClinicalImpressionFindingComponent extends BackboneElement implements IBaseBackboneElement { 497 /** 498 * Specific text or code for finding or diagnosis, which may include ruled-out 499 * or resolved conditions. 500 */ 501 @Child(name = "itemCodeableConcept", type = { 502 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 503 @Description(shortDefinition = "What was found", formalDefinition = "Specific text or code for finding or diagnosis, which may include ruled-out or resolved conditions.") 504 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 505 protected CodeableConcept itemCodeableConcept; 506 507 /** 508 * Specific reference for finding or diagnosis, which may include ruled-out or 509 * resolved conditions. 510 */ 511 @Child(name = "itemReference", type = { Condition.class, Observation.class, 512 Media.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 513 @Description(shortDefinition = "What was found", formalDefinition = "Specific reference for finding or diagnosis, which may include ruled-out or resolved conditions.") 514 protected Reference itemReference; 515 516 /** 517 * The actual object that is the target of the reference (Specific reference for 518 * finding or diagnosis, which may include ruled-out or resolved conditions.) 519 */ 520 protected Resource itemReferenceTarget; 521 522 /** 523 * Which investigations support finding or diagnosis. 524 */ 525 @Child(name = "basis", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 526 @Description(shortDefinition = "Which investigations support finding", formalDefinition = "Which investigations support finding or diagnosis.") 527 protected StringType basis; 528 529 private static final long serialVersionUID = -1578446448L; 530 531 /** 532 * Constructor 533 */ 534 public ClinicalImpressionFindingComponent() { 535 super(); 536 } 537 538 /** 539 * @return {@link #itemCodeableConcept} (Specific text or code for finding or 540 * diagnosis, which may include ruled-out or resolved conditions.) 541 */ 542 public CodeableConcept getItemCodeableConcept() { 543 if (this.itemCodeableConcept == null) 544 if (Configuration.errorOnAutoCreate()) 545 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.itemCodeableConcept"); 546 else if (Configuration.doAutoCreate()) 547 this.itemCodeableConcept = new CodeableConcept(); // cc 548 return this.itemCodeableConcept; 549 } 550 551 public boolean hasItemCodeableConcept() { 552 return this.itemCodeableConcept != null && !this.itemCodeableConcept.isEmpty(); 553 } 554 555 /** 556 * @param value {@link #itemCodeableConcept} (Specific text or code for finding 557 * or diagnosis, which may include ruled-out or resolved 558 * conditions.) 559 */ 560 public ClinicalImpressionFindingComponent setItemCodeableConcept(CodeableConcept value) { 561 this.itemCodeableConcept = value; 562 return this; 563 } 564 565 /** 566 * @return {@link #itemReference} (Specific reference for finding or diagnosis, 567 * which may include ruled-out or resolved conditions.) 568 */ 569 public Reference getItemReference() { 570 if (this.itemReference == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.itemReference"); 573 else if (Configuration.doAutoCreate()) 574 this.itemReference = new Reference(); // cc 575 return this.itemReference; 576 } 577 578 public boolean hasItemReference() { 579 return this.itemReference != null && !this.itemReference.isEmpty(); 580 } 581 582 /** 583 * @param value {@link #itemReference} (Specific reference for finding or 584 * diagnosis, which may include ruled-out or resolved conditions.) 585 */ 586 public ClinicalImpressionFindingComponent setItemReference(Reference value) { 587 this.itemReference = value; 588 return this; 589 } 590 591 /** 592 * @return {@link #itemReference} The actual object that is the target of the 593 * reference. The reference library doesn't populate this, but you can 594 * use it to hold the resource if you resolve it. (Specific reference 595 * for finding or diagnosis, which may include ruled-out or resolved 596 * conditions.) 597 */ 598 public Resource getItemReferenceTarget() { 599 return this.itemReferenceTarget; 600 } 601 602 /** 603 * @param value {@link #itemReference} The actual object that is the target of 604 * the reference. The reference library doesn't use these, but you 605 * can use it to hold the resource if you resolve it. (Specific 606 * reference for finding or diagnosis, which may include ruled-out 607 * or resolved conditions.) 608 */ 609 public ClinicalImpressionFindingComponent setItemReferenceTarget(Resource value) { 610 this.itemReferenceTarget = value; 611 return this; 612 } 613 614 /** 615 * @return {@link #basis} (Which investigations support finding or diagnosis.). 616 * This is the underlying object with id, value and extensions. The 617 * accessor "getBasis" gives direct access to the value 618 */ 619 public StringType getBasisElement() { 620 if (this.basis == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.basis"); 623 else if (Configuration.doAutoCreate()) 624 this.basis = new StringType(); // bb 625 return this.basis; 626 } 627 628 public boolean hasBasisElement() { 629 return this.basis != null && !this.basis.isEmpty(); 630 } 631 632 public boolean hasBasis() { 633 return this.basis != null && !this.basis.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #basis} (Which investigations support finding or 638 * diagnosis.). This is the underlying object with id, value and 639 * extensions. The accessor "getBasis" gives direct access to the 640 * value 641 */ 642 public ClinicalImpressionFindingComponent setBasisElement(StringType value) { 643 this.basis = value; 644 return this; 645 } 646 647 /** 648 * @return Which investigations support finding or diagnosis. 649 */ 650 public String getBasis() { 651 return this.basis == null ? null : this.basis.getValue(); 652 } 653 654 /** 655 * @param value Which investigations support finding or diagnosis. 656 */ 657 public ClinicalImpressionFindingComponent setBasis(String value) { 658 if (Utilities.noString(value)) 659 this.basis = null; 660 else { 661 if (this.basis == null) 662 this.basis = new StringType(); 663 this.basis.setValue(value); 664 } 665 return this; 666 } 667 668 protected void listChildren(List<Property> children) { 669 super.listChildren(children); 670 children.add(new Property("itemCodeableConcept", "CodeableConcept", 671 "Specific text or code for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 672 itemCodeableConcept)); 673 children.add(new Property("itemReference", "Reference(Condition|Observation|Media)", 674 "Specific reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 675 itemReference)); 676 children.add(new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, basis)); 677 } 678 679 @Override 680 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 681 switch (_hash) { 682 case 106644494: 683 /* itemCodeableConcept */ return new Property("itemCodeableConcept", "CodeableConcept", 684 "Specific text or code for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 685 itemCodeableConcept); 686 case 1376364920: 687 /* itemReference */ return new Property("itemReference", "Reference(Condition|Observation|Media)", 688 "Specific reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 689 itemReference); 690 case 93508670: 691 /* basis */ return new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, 692 basis); 693 default: 694 return super.getNamedProperty(_hash, _name, _checkValid); 695 } 696 697 } 698 699 @Override 700 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 701 switch (hash) { 702 case 106644494: 703 /* itemCodeableConcept */ return this.itemCodeableConcept == null ? new Base[0] 704 : new Base[] { this.itemCodeableConcept }; // CodeableConcept 705 case 1376364920: 706 /* itemReference */ return this.itemReference == null ? new Base[0] : new Base[] { this.itemReference }; // Reference 707 case 93508670: 708 /* basis */ return this.basis == null ? new Base[0] : new Base[] { this.basis }; // StringType 709 default: 710 return super.getProperty(hash, name, checkValid); 711 } 712 713 } 714 715 @Override 716 public Base setProperty(int hash, String name, Base value) throws FHIRException { 717 switch (hash) { 718 case 106644494: // itemCodeableConcept 719 this.itemCodeableConcept = castToCodeableConcept(value); // CodeableConcept 720 return value; 721 case 1376364920: // itemReference 722 this.itemReference = castToReference(value); // Reference 723 return value; 724 case 93508670: // basis 725 this.basis = castToString(value); // StringType 726 return value; 727 default: 728 return super.setProperty(hash, name, value); 729 } 730 731 } 732 733 @Override 734 public Base setProperty(String name, Base value) throws FHIRException { 735 if (name.equals("itemCodeableConcept")) { 736 this.itemCodeableConcept = castToCodeableConcept(value); // CodeableConcept 737 } else if (name.equals("itemReference")) { 738 this.itemReference = castToReference(value); // Reference 739 } else if (name.equals("basis")) { 740 this.basis = castToString(value); // StringType 741 } else 742 return super.setProperty(name, value); 743 return value; 744 } 745 746 @Override 747 public void removeChild(String name, Base value) throws FHIRException { 748 if (name.equals("itemCodeableConcept")) { 749 this.itemCodeableConcept = null; 750 } else if (name.equals("itemReference")) { 751 this.itemReference = null; 752 } else if (name.equals("basis")) { 753 this.basis = null; 754 } else 755 super.removeChild(name, value); 756 757 } 758 759 @Override 760 public Base makeProperty(int hash, String name) throws FHIRException { 761 switch (hash) { 762 case 106644494: 763 return getItemCodeableConcept(); 764 case 1376364920: 765 return getItemReference(); 766 case 93508670: 767 return getBasisElement(); 768 default: 769 return super.makeProperty(hash, name); 770 } 771 772 } 773 774 @Override 775 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 776 switch (hash) { 777 case 106644494: 778 /* itemCodeableConcept */ return new String[] { "CodeableConcept" }; 779 case 1376364920: 780 /* itemReference */ return new String[] { "Reference" }; 781 case 93508670: 782 /* basis */ return new String[] { "string" }; 783 default: 784 return super.getTypesForProperty(hash, name); 785 } 786 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("itemCodeableConcept")) { 792 this.itemCodeableConcept = new CodeableConcept(); 793 return this.itemCodeableConcept; 794 } else if (name.equals("itemReference")) { 795 this.itemReference = new Reference(); 796 return this.itemReference; 797 } else if (name.equals("basis")) { 798 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.basis"); 799 } else 800 return super.addChild(name); 801 } 802 803 public ClinicalImpressionFindingComponent copy() { 804 ClinicalImpressionFindingComponent dst = new ClinicalImpressionFindingComponent(); 805 copyValues(dst); 806 return dst; 807 } 808 809 public void copyValues(ClinicalImpressionFindingComponent dst) { 810 super.copyValues(dst); 811 dst.itemCodeableConcept = itemCodeableConcept == null ? null : itemCodeableConcept.copy(); 812 dst.itemReference = itemReference == null ? null : itemReference.copy(); 813 dst.basis = basis == null ? null : basis.copy(); 814 } 815 816 @Override 817 public boolean equalsDeep(Base other_) { 818 if (!super.equalsDeep(other_)) 819 return false; 820 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 821 return false; 822 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 823 return compareDeep(itemCodeableConcept, o.itemCodeableConcept, true) 824 && compareDeep(itemReference, o.itemReference, true) && compareDeep(basis, o.basis, true); 825 } 826 827 @Override 828 public boolean equalsShallow(Base other_) { 829 if (!super.equalsShallow(other_)) 830 return false; 831 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 832 return false; 833 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 834 return compareValues(basis, o.basis, true); 835 } 836 837 public boolean isEmpty() { 838 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemCodeableConcept, itemReference, basis); 839 } 840 841 public String fhirType() { 842 return "ClinicalImpression.finding"; 843 844 } 845 846 } 847 848 /** 849 * Business identifiers assigned to this clinical impression by the performer or 850 * other systems which remain constant as the resource is updated and propagates 851 * from server to server. 852 */ 853 @Child(name = "identifier", type = { 854 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 855 @Description(shortDefinition = "Business identifier", formalDefinition = "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 856 protected List<Identifier> identifier; 857 858 /** 859 * Identifies the workflow status of the assessment. 860 */ 861 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 862 @Description(shortDefinition = "in-progress | completed | entered-in-error", formalDefinition = "Identifies the workflow status of the assessment.") 863 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinicalimpression-status") 864 protected Enumeration<ClinicalImpressionStatus> status; 865 866 /** 867 * Captures the reason for the current state of the ClinicalImpression. 868 */ 869 @Child(name = "statusReason", type = { 870 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 871 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the ClinicalImpression.") 872 protected CodeableConcept statusReason; 873 874 /** 875 * Categorizes the type of clinical assessment performed. 876 */ 877 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 878 @Description(shortDefinition = "Kind of assessment performed", formalDefinition = "Categorizes the type of clinical assessment performed.") 879 protected CodeableConcept code; 880 881 /** 882 * A summary of the context and/or cause of the assessment - why / where it was 883 * performed, and what patient events/status prompted it. 884 */ 885 @Child(name = "description", type = { 886 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 887 @Description(shortDefinition = "Why/how the assessment was performed", formalDefinition = "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.") 888 protected StringType description; 889 890 /** 891 * The patient or group of individuals assessed as part of this record. 892 */ 893 @Child(name = "subject", type = { Patient.class, 894 Group.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 895 @Description(shortDefinition = "Patient or group assessed", formalDefinition = "The patient or group of individuals assessed as part of this record.") 896 protected Reference subject; 897 898 /** 899 * The actual object that is the target of the reference (The patient or group 900 * of individuals assessed as part of this record.) 901 */ 902 protected Resource subjectTarget; 903 904 /** 905 * The Encounter during which this ClinicalImpression was created or to which 906 * the creation of this record is tightly associated. 907 */ 908 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 909 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.") 910 protected Reference encounter; 911 912 /** 913 * The actual object that is the target of the reference (The Encounter during 914 * which this ClinicalImpression was created or to which the creation of this 915 * record is tightly associated.) 916 */ 917 protected Encounter encounterTarget; 918 919 /** 920 * The point in time or period over which the subject was assessed. 921 */ 922 @Child(name = "effective", type = { DateTimeType.class, 923 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 924 @Description(shortDefinition = "Time of assessment", formalDefinition = "The point in time or period over which the subject was assessed.") 925 protected Type effective; 926 927 /** 928 * Indicates when the documentation of the assessment was complete. 929 */ 930 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 931 @Description(shortDefinition = "When the assessment was documented", formalDefinition = "Indicates when the documentation of the assessment was complete.") 932 protected DateTimeType date; 933 934 /** 935 * The clinician performing the assessment. 936 */ 937 @Child(name = "assessor", type = { Practitioner.class, 938 PractitionerRole.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 939 @Description(shortDefinition = "The clinician performing the assessment", formalDefinition = "The clinician performing the assessment.") 940 protected Reference assessor; 941 942 /** 943 * The actual object that is the target of the reference (The clinician 944 * performing the assessment.) 945 */ 946 protected Resource assessorTarget; 947 948 /** 949 * A reference to the last assessment that was conducted on this patient. 950 * Assessments are often/usually ongoing in nature; a care provider 951 * (practitioner or team) will make new assessments on an ongoing basis as new 952 * data arises or the patient's conditions changes. 953 */ 954 @Child(name = "previous", type = { 955 ClinicalImpression.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 956 @Description(shortDefinition = "Reference to last assessment", formalDefinition = "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.") 957 protected Reference previous; 958 959 /** 960 * The actual object that is the target of the reference (A reference to the 961 * last assessment that was conducted on this patient. Assessments are 962 * often/usually ongoing in nature; a care provider (practitioner or team) will 963 * make new assessments on an ongoing basis as new data arises or the patient's 964 * conditions changes.) 965 */ 966 protected ClinicalImpression previousTarget; 967 968 /** 969 * A list of the relevant problems/conditions for a patient. 970 */ 971 @Child(name = "problem", type = { Condition.class, 972 AllergyIntolerance.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 973 @Description(shortDefinition = "Relevant impressions of patient state", formalDefinition = "A list of the relevant problems/conditions for a patient.") 974 protected List<Reference> problem; 975 /** 976 * The actual objects that are the target of the reference (A list of the 977 * relevant problems/conditions for a patient.) 978 */ 979 protected List<Resource> problemTarget; 980 981 /** 982 * One or more sets of investigations (signs, symptoms, etc.). The actual 983 * grouping of investigations varies greatly depending on the type and context 984 * of the assessment. These investigations may include data generated during the 985 * assessment process, or data previously generated and recorded that is 986 * pertinent to the outcomes. 987 */ 988 @Child(name = "investigation", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 989 @Description(shortDefinition = "One or more sets of investigations (signs, symptoms, etc.)", formalDefinition = "One or more sets of investigations (signs, symptoms, etc.). The actual grouping of investigations varies greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.") 990 protected List<ClinicalImpressionInvestigationComponent> investigation; 991 992 /** 993 * Reference to a specific published clinical protocol that was followed during 994 * this assessment, and/or that provides evidence in support of the diagnosis. 995 */ 996 @Child(name = "protocol", type = { 997 UriType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 998 @Description(shortDefinition = "Clinical Protocol followed", formalDefinition = "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.") 999 protected List<UriType> protocol; 1000 1001 /** 1002 * A text summary of the investigations and the diagnosis. 1003 */ 1004 @Child(name = "summary", type = { StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1005 @Description(shortDefinition = "Summary of the assessment", formalDefinition = "A text summary of the investigations and the diagnosis.") 1006 protected StringType summary; 1007 1008 /** 1009 * Specific findings or diagnoses that were considered likely or relevant to 1010 * ongoing treatment. 1011 */ 1012 @Child(name = "finding", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1013 @Description(shortDefinition = "Possible or likely findings and diagnoses", formalDefinition = "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.") 1014 protected List<ClinicalImpressionFindingComponent> finding; 1015 1016 /** 1017 * Estimate of likely outcome. 1018 */ 1019 @Child(name = "prognosisCodeableConcept", type = { 1020 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1021 @Description(shortDefinition = "Estimate of likely outcome", formalDefinition = "Estimate of likely outcome.") 1022 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinicalimpression-prognosis") 1023 protected List<CodeableConcept> prognosisCodeableConcept; 1024 1025 /** 1026 * RiskAssessment expressing likely outcome. 1027 */ 1028 @Child(name = "prognosisReference", type = { 1029 RiskAssessment.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1030 @Description(shortDefinition = "RiskAssessment expressing likely outcome", formalDefinition = "RiskAssessment expressing likely outcome.") 1031 protected List<Reference> prognosisReference; 1032 /** 1033 * The actual objects that are the target of the reference (RiskAssessment 1034 * expressing likely outcome.) 1035 */ 1036 protected List<RiskAssessment> prognosisReferenceTarget; 1037 1038 /** 1039 * Information supporting the clinical impression. 1040 */ 1041 @Child(name = "supportingInfo", type = { 1042 Reference.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1043 @Description(shortDefinition = "Information supporting the clinical impression", formalDefinition = "Information supporting the clinical impression.") 1044 protected List<Reference> supportingInfo; 1045 /** 1046 * The actual objects that are the target of the reference (Information 1047 * supporting the clinical impression.) 1048 */ 1049 protected List<Resource> supportingInfoTarget; 1050 1051 /** 1052 * Commentary about the impression, typically recorded after the impression 1053 * itself was made, though supplemental notes by the original author could also 1054 * appear. 1055 */ 1056 @Child(name = "note", type = { 1057 Annotation.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1058 @Description(shortDefinition = "Comments made about the ClinicalImpression", formalDefinition = "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.") 1059 protected List<Annotation> note; 1060 1061 private static final long serialVersionUID = 1158874575L; 1062 1063 /** 1064 * Constructor 1065 */ 1066 public ClinicalImpression() { 1067 super(); 1068 } 1069 1070 /** 1071 * Constructor 1072 */ 1073 public ClinicalImpression(Enumeration<ClinicalImpressionStatus> status, Reference subject) { 1074 super(); 1075 this.status = status; 1076 this.subject = subject; 1077 } 1078 1079 /** 1080 * @return {@link #identifier} (Business identifiers assigned to this clinical 1081 * impression by the performer or other systems which remain constant as 1082 * the resource is updated and propagates from server to server.) 1083 */ 1084 public List<Identifier> getIdentifier() { 1085 if (this.identifier == null) 1086 this.identifier = new ArrayList<Identifier>(); 1087 return this.identifier; 1088 } 1089 1090 /** 1091 * @return Returns a reference to <code>this</code> for easy method chaining 1092 */ 1093 public ClinicalImpression setIdentifier(List<Identifier> theIdentifier) { 1094 this.identifier = theIdentifier; 1095 return this; 1096 } 1097 1098 public boolean hasIdentifier() { 1099 if (this.identifier == null) 1100 return false; 1101 for (Identifier item : this.identifier) 1102 if (!item.isEmpty()) 1103 return true; 1104 return false; 1105 } 1106 1107 public Identifier addIdentifier() { // 3 1108 Identifier t = new Identifier(); 1109 if (this.identifier == null) 1110 this.identifier = new ArrayList<Identifier>(); 1111 this.identifier.add(t); 1112 return t; 1113 } 1114 1115 public ClinicalImpression addIdentifier(Identifier t) { // 3 1116 if (t == null) 1117 return this; 1118 if (this.identifier == null) 1119 this.identifier = new ArrayList<Identifier>(); 1120 this.identifier.add(t); 1121 return this; 1122 } 1123 1124 /** 1125 * @return The first repetition of repeating field {@link #identifier}, creating 1126 * it if it does not already exist 1127 */ 1128 public Identifier getIdentifierFirstRep() { 1129 if (getIdentifier().isEmpty()) { 1130 addIdentifier(); 1131 } 1132 return getIdentifier().get(0); 1133 } 1134 1135 /** 1136 * @return {@link #status} (Identifies the workflow status of the assessment.). 1137 * This is the underlying object with id, value and extensions. The 1138 * accessor "getStatus" gives direct access to the value 1139 */ 1140 public Enumeration<ClinicalImpressionStatus> getStatusElement() { 1141 if (this.status == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create ClinicalImpression.status"); 1144 else if (Configuration.doAutoCreate()) 1145 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); // bb 1146 return this.status; 1147 } 1148 1149 public boolean hasStatusElement() { 1150 return this.status != null && !this.status.isEmpty(); 1151 } 1152 1153 public boolean hasStatus() { 1154 return this.status != null && !this.status.isEmpty(); 1155 } 1156 1157 /** 1158 * @param value {@link #status} (Identifies the workflow status of the 1159 * assessment.). This is the underlying object with id, value and 1160 * extensions. The accessor "getStatus" gives direct access to the 1161 * value 1162 */ 1163 public ClinicalImpression setStatusElement(Enumeration<ClinicalImpressionStatus> value) { 1164 this.status = value; 1165 return this; 1166 } 1167 1168 /** 1169 * @return Identifies the workflow status of the assessment. 1170 */ 1171 public ClinicalImpressionStatus getStatus() { 1172 return this.status == null ? null : this.status.getValue(); 1173 } 1174 1175 /** 1176 * @param value Identifies the workflow status of the assessment. 1177 */ 1178 public ClinicalImpression setStatus(ClinicalImpressionStatus value) { 1179 if (this.status == null) 1180 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); 1181 this.status.setValue(value); 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #statusReason} (Captures the reason for the current state of 1187 * the ClinicalImpression.) 1188 */ 1189 public CodeableConcept getStatusReason() { 1190 if (this.statusReason == null) 1191 if (Configuration.errorOnAutoCreate()) 1192 throw new Error("Attempt to auto-create ClinicalImpression.statusReason"); 1193 else if (Configuration.doAutoCreate()) 1194 this.statusReason = new CodeableConcept(); // cc 1195 return this.statusReason; 1196 } 1197 1198 public boolean hasStatusReason() { 1199 return this.statusReason != null && !this.statusReason.isEmpty(); 1200 } 1201 1202 /** 1203 * @param value {@link #statusReason} (Captures the reason for the current state 1204 * of the ClinicalImpression.) 1205 */ 1206 public ClinicalImpression setStatusReason(CodeableConcept value) { 1207 this.statusReason = value; 1208 return this; 1209 } 1210 1211 /** 1212 * @return {@link #code} (Categorizes the type of clinical assessment 1213 * performed.) 1214 */ 1215 public CodeableConcept getCode() { 1216 if (this.code == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create ClinicalImpression.code"); 1219 else if (Configuration.doAutoCreate()) 1220 this.code = new CodeableConcept(); // cc 1221 return this.code; 1222 } 1223 1224 public boolean hasCode() { 1225 return this.code != null && !this.code.isEmpty(); 1226 } 1227 1228 /** 1229 * @param value {@link #code} (Categorizes the type of clinical assessment 1230 * performed.) 1231 */ 1232 public ClinicalImpression setCode(CodeableConcept value) { 1233 this.code = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #description} (A summary of the context and/or cause of the 1239 * assessment - why / where it was performed, and what patient 1240 * events/status prompted it.). This is the underlying object with id, 1241 * value and extensions. The accessor "getDescription" gives direct 1242 * access to the value 1243 */ 1244 public StringType getDescriptionElement() { 1245 if (this.description == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create ClinicalImpression.description"); 1248 else if (Configuration.doAutoCreate()) 1249 this.description = new StringType(); // bb 1250 return this.description; 1251 } 1252 1253 public boolean hasDescriptionElement() { 1254 return this.description != null && !this.description.isEmpty(); 1255 } 1256 1257 public boolean hasDescription() { 1258 return this.description != null && !this.description.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #description} (A summary of the context and/or cause of 1263 * the assessment - why / where it was performed, and what patient 1264 * events/status prompted it.). This is the underlying object with 1265 * id, value and extensions. The accessor "getDescription" gives 1266 * direct access to the value 1267 */ 1268 public ClinicalImpression setDescriptionElement(StringType value) { 1269 this.description = value; 1270 return this; 1271 } 1272 1273 /** 1274 * @return A summary of the context and/or cause of the assessment - why / where 1275 * it was performed, and what patient events/status prompted it. 1276 */ 1277 public String getDescription() { 1278 return this.description == null ? null : this.description.getValue(); 1279 } 1280 1281 /** 1282 * @param value A summary of the context and/or cause of the assessment - why / 1283 * where it was performed, and what patient events/status prompted 1284 * it. 1285 */ 1286 public ClinicalImpression setDescription(String value) { 1287 if (Utilities.noString(value)) 1288 this.description = null; 1289 else { 1290 if (this.description == null) 1291 this.description = new StringType(); 1292 this.description.setValue(value); 1293 } 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #subject} (The patient or group of individuals assessed as 1299 * part of this record.) 1300 */ 1301 public Reference getSubject() { 1302 if (this.subject == null) 1303 if (Configuration.errorOnAutoCreate()) 1304 throw new Error("Attempt to auto-create ClinicalImpression.subject"); 1305 else if (Configuration.doAutoCreate()) 1306 this.subject = new Reference(); // cc 1307 return this.subject; 1308 } 1309 1310 public boolean hasSubject() { 1311 return this.subject != null && !this.subject.isEmpty(); 1312 } 1313 1314 /** 1315 * @param value {@link #subject} (The patient or group of individuals assessed 1316 * as part of this record.) 1317 */ 1318 public ClinicalImpression setSubject(Reference value) { 1319 this.subject = value; 1320 return this; 1321 } 1322 1323 /** 1324 * @return {@link #subject} The actual object that is the target of the 1325 * reference. The reference library doesn't populate this, but you can 1326 * use it to hold the resource if you resolve it. (The patient or group 1327 * of individuals assessed as part of this record.) 1328 */ 1329 public Resource getSubjectTarget() { 1330 return this.subjectTarget; 1331 } 1332 1333 /** 1334 * @param value {@link #subject} The actual object that is the target of the 1335 * reference. The reference library doesn't use these, but you can 1336 * use it to hold the resource if you resolve it. (The patient or 1337 * group of individuals assessed as part of this record.) 1338 */ 1339 public ClinicalImpression setSubjectTarget(Resource value) { 1340 this.subjectTarget = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return {@link #encounter} (The Encounter during which this 1346 * ClinicalImpression was created or to which the creation of this 1347 * record is tightly associated.) 1348 */ 1349 public Reference getEncounter() { 1350 if (this.encounter == null) 1351 if (Configuration.errorOnAutoCreate()) 1352 throw new Error("Attempt to auto-create ClinicalImpression.encounter"); 1353 else if (Configuration.doAutoCreate()) 1354 this.encounter = new Reference(); // cc 1355 return this.encounter; 1356 } 1357 1358 public boolean hasEncounter() { 1359 return this.encounter != null && !this.encounter.isEmpty(); 1360 } 1361 1362 /** 1363 * @param value {@link #encounter} (The Encounter during which this 1364 * ClinicalImpression was created or to which the creation of this 1365 * record is tightly associated.) 1366 */ 1367 public ClinicalImpression setEncounter(Reference value) { 1368 this.encounter = value; 1369 return this; 1370 } 1371 1372 /** 1373 * @return {@link #encounter} The actual object that is the target of the 1374 * reference. The reference library doesn't populate this, but you can 1375 * use it to hold the resource if you resolve it. (The Encounter during 1376 * which this ClinicalImpression was created or to which the creation of 1377 * this record is tightly associated.) 1378 */ 1379 public Encounter getEncounterTarget() { 1380 if (this.encounterTarget == null) 1381 if (Configuration.errorOnAutoCreate()) 1382 throw new Error("Attempt to auto-create ClinicalImpression.encounter"); 1383 else if (Configuration.doAutoCreate()) 1384 this.encounterTarget = new Encounter(); // aa 1385 return this.encounterTarget; 1386 } 1387 1388 /** 1389 * @param value {@link #encounter} The actual object that is the target of the 1390 * reference. The reference library doesn't use these, but you can 1391 * use it to hold the resource if you resolve it. (The Encounter 1392 * during which this ClinicalImpression was created or to which the 1393 * creation of this record is tightly associated.) 1394 */ 1395 public ClinicalImpression setEncounterTarget(Encounter value) { 1396 this.encounterTarget = value; 1397 return this; 1398 } 1399 1400 /** 1401 * @return {@link #effective} (The point in time or period over which the 1402 * subject was assessed.) 1403 */ 1404 public Type getEffective() { 1405 return this.effective; 1406 } 1407 1408 /** 1409 * @return {@link #effective} (The point in time or period over which the 1410 * subject was assessed.) 1411 */ 1412 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1413 if (this.effective == null) 1414 this.effective = new DateTimeType(); 1415 if (!(this.effective instanceof DateTimeType)) 1416 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1417 + this.effective.getClass().getName() + " was encountered"); 1418 return (DateTimeType) this.effective; 1419 } 1420 1421 public boolean hasEffectiveDateTimeType() { 1422 return this.effective instanceof DateTimeType; 1423 } 1424 1425 /** 1426 * @return {@link #effective} (The point in time or period over which the 1427 * subject was assessed.) 1428 */ 1429 public Period getEffectivePeriod() throws FHIRException { 1430 if (this.effective == null) 1431 this.effective = new Period(); 1432 if (!(this.effective instanceof Period)) 1433 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1434 + " was encountered"); 1435 return (Period) this.effective; 1436 } 1437 1438 public boolean hasEffectivePeriod() { 1439 return this.effective instanceof Period; 1440 } 1441 1442 public boolean hasEffective() { 1443 return this.effective != null && !this.effective.isEmpty(); 1444 } 1445 1446 /** 1447 * @param value {@link #effective} (The point in time or period over which the 1448 * subject was assessed.) 1449 */ 1450 public ClinicalImpression setEffective(Type value) { 1451 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1452 throw new Error("Not the right type for ClinicalImpression.effective[x]: " + value.fhirType()); 1453 this.effective = value; 1454 return this; 1455 } 1456 1457 /** 1458 * @return {@link #date} (Indicates when the documentation of the assessment was 1459 * complete.). This is the underlying object with id, value and 1460 * extensions. The accessor "getDate" gives direct access to the value 1461 */ 1462 public DateTimeType getDateElement() { 1463 if (this.date == null) 1464 if (Configuration.errorOnAutoCreate()) 1465 throw new Error("Attempt to auto-create ClinicalImpression.date"); 1466 else if (Configuration.doAutoCreate()) 1467 this.date = new DateTimeType(); // bb 1468 return this.date; 1469 } 1470 1471 public boolean hasDateElement() { 1472 return this.date != null && !this.date.isEmpty(); 1473 } 1474 1475 public boolean hasDate() { 1476 return this.date != null && !this.date.isEmpty(); 1477 } 1478 1479 /** 1480 * @param value {@link #date} (Indicates when the documentation of the 1481 * assessment was complete.). This is the underlying object with 1482 * id, value and extensions. The accessor "getDate" gives direct 1483 * access to the value 1484 */ 1485 public ClinicalImpression setDateElement(DateTimeType value) { 1486 this.date = value; 1487 return this; 1488 } 1489 1490 /** 1491 * @return Indicates when the documentation of the assessment was complete. 1492 */ 1493 public Date getDate() { 1494 return this.date == null ? null : this.date.getValue(); 1495 } 1496 1497 /** 1498 * @param value Indicates when the documentation of the assessment was complete. 1499 */ 1500 public ClinicalImpression setDate(Date value) { 1501 if (value == null) 1502 this.date = null; 1503 else { 1504 if (this.date == null) 1505 this.date = new DateTimeType(); 1506 this.date.setValue(value); 1507 } 1508 return this; 1509 } 1510 1511 /** 1512 * @return {@link #assessor} (The clinician performing the assessment.) 1513 */ 1514 public Reference getAssessor() { 1515 if (this.assessor == null) 1516 if (Configuration.errorOnAutoCreate()) 1517 throw new Error("Attempt to auto-create ClinicalImpression.assessor"); 1518 else if (Configuration.doAutoCreate()) 1519 this.assessor = new Reference(); // cc 1520 return this.assessor; 1521 } 1522 1523 public boolean hasAssessor() { 1524 return this.assessor != null && !this.assessor.isEmpty(); 1525 } 1526 1527 /** 1528 * @param value {@link #assessor} (The clinician performing the assessment.) 1529 */ 1530 public ClinicalImpression setAssessor(Reference value) { 1531 this.assessor = value; 1532 return this; 1533 } 1534 1535 /** 1536 * @return {@link #assessor} The actual object that is the target of the 1537 * reference. The reference library doesn't populate this, but you can 1538 * use it to hold the resource if you resolve it. (The clinician 1539 * performing the assessment.) 1540 */ 1541 public Resource getAssessorTarget() { 1542 return this.assessorTarget; 1543 } 1544 1545 /** 1546 * @param value {@link #assessor} The actual object that is the target of the 1547 * reference. The reference library doesn't use these, but you can 1548 * use it to hold the resource if you resolve it. (The clinician 1549 * performing the assessment.) 1550 */ 1551 public ClinicalImpression setAssessorTarget(Resource value) { 1552 this.assessorTarget = value; 1553 return this; 1554 } 1555 1556 /** 1557 * @return {@link #previous} (A reference to the last assessment that was 1558 * conducted on this patient. Assessments are often/usually ongoing in 1559 * nature; a care provider (practitioner or team) will make new 1560 * assessments on an ongoing basis as new data arises or the patient's 1561 * conditions changes.) 1562 */ 1563 public Reference getPrevious() { 1564 if (this.previous == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1567 else if (Configuration.doAutoCreate()) 1568 this.previous = new Reference(); // cc 1569 return this.previous; 1570 } 1571 1572 public boolean hasPrevious() { 1573 return this.previous != null && !this.previous.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #previous} (A reference to the last assessment that was 1578 * conducted on this patient. Assessments are often/usually ongoing 1579 * in nature; a care provider (practitioner or team) will make new 1580 * assessments on an ongoing basis as new data arises or the 1581 * patient's conditions changes.) 1582 */ 1583 public ClinicalImpression setPrevious(Reference value) { 1584 this.previous = value; 1585 return this; 1586 } 1587 1588 /** 1589 * @return {@link #previous} The actual object that is the target of the 1590 * reference. The reference library doesn't populate this, but you can 1591 * use it to hold the resource if you resolve it. (A reference to the 1592 * last assessment that was conducted on this patient. Assessments are 1593 * often/usually ongoing in nature; a care provider (practitioner or 1594 * team) will make new assessments on an ongoing basis as new data 1595 * arises or the patient's conditions changes.) 1596 */ 1597 public ClinicalImpression getPreviousTarget() { 1598 if (this.previousTarget == null) 1599 if (Configuration.errorOnAutoCreate()) 1600 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1601 else if (Configuration.doAutoCreate()) 1602 this.previousTarget = new ClinicalImpression(); // aa 1603 return this.previousTarget; 1604 } 1605 1606 /** 1607 * @param value {@link #previous} The actual object that is the target of the 1608 * reference. The reference library doesn't use these, but you can 1609 * use it to hold the resource if you resolve it. (A reference to 1610 * the last assessment that was conducted on this patient. 1611 * Assessments are often/usually ongoing in nature; a care provider 1612 * (practitioner or team) will make new assessments on an ongoing 1613 * basis as new data arises or the patient's conditions changes.) 1614 */ 1615 public ClinicalImpression setPreviousTarget(ClinicalImpression value) { 1616 this.previousTarget = value; 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #problem} (A list of the relevant problems/conditions for a 1622 * patient.) 1623 */ 1624 public List<Reference> getProblem() { 1625 if (this.problem == null) 1626 this.problem = new ArrayList<Reference>(); 1627 return this.problem; 1628 } 1629 1630 /** 1631 * @return Returns a reference to <code>this</code> for easy method chaining 1632 */ 1633 public ClinicalImpression setProblem(List<Reference> theProblem) { 1634 this.problem = theProblem; 1635 return this; 1636 } 1637 1638 public boolean hasProblem() { 1639 if (this.problem == null) 1640 return false; 1641 for (Reference item : this.problem) 1642 if (!item.isEmpty()) 1643 return true; 1644 return false; 1645 } 1646 1647 public Reference addProblem() { // 3 1648 Reference t = new Reference(); 1649 if (this.problem == null) 1650 this.problem = new ArrayList<Reference>(); 1651 this.problem.add(t); 1652 return t; 1653 } 1654 1655 public ClinicalImpression addProblem(Reference t) { // 3 1656 if (t == null) 1657 return this; 1658 if (this.problem == null) 1659 this.problem = new ArrayList<Reference>(); 1660 this.problem.add(t); 1661 return this; 1662 } 1663 1664 /** 1665 * @return The first repetition of repeating field {@link #problem}, creating it 1666 * if it does not already exist 1667 */ 1668 public Reference getProblemFirstRep() { 1669 if (getProblem().isEmpty()) { 1670 addProblem(); 1671 } 1672 return getProblem().get(0); 1673 } 1674 1675 /** 1676 * @return {@link #investigation} (One or more sets of investigations (signs, 1677 * symptoms, etc.). The actual grouping of investigations varies greatly 1678 * depending on the type and context of the assessment. These 1679 * investigations may include data generated during the assessment 1680 * process, or data previously generated and recorded that is pertinent 1681 * to the outcomes.) 1682 */ 1683 public List<ClinicalImpressionInvestigationComponent> getInvestigation() { 1684 if (this.investigation == null) 1685 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1686 return this.investigation; 1687 } 1688 1689 /** 1690 * @return Returns a reference to <code>this</code> for easy method chaining 1691 */ 1692 public ClinicalImpression setInvestigation(List<ClinicalImpressionInvestigationComponent> theInvestigation) { 1693 this.investigation = theInvestigation; 1694 return this; 1695 } 1696 1697 public boolean hasInvestigation() { 1698 if (this.investigation == null) 1699 return false; 1700 for (ClinicalImpressionInvestigationComponent item : this.investigation) 1701 if (!item.isEmpty()) 1702 return true; 1703 return false; 1704 } 1705 1706 public ClinicalImpressionInvestigationComponent addInvestigation() { // 3 1707 ClinicalImpressionInvestigationComponent t = new ClinicalImpressionInvestigationComponent(); 1708 if (this.investigation == null) 1709 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1710 this.investigation.add(t); 1711 return t; 1712 } 1713 1714 public ClinicalImpression addInvestigation(ClinicalImpressionInvestigationComponent t) { // 3 1715 if (t == null) 1716 return this; 1717 if (this.investigation == null) 1718 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1719 this.investigation.add(t); 1720 return this; 1721 } 1722 1723 /** 1724 * @return The first repetition of repeating field {@link #investigation}, 1725 * creating it if it does not already exist 1726 */ 1727 public ClinicalImpressionInvestigationComponent getInvestigationFirstRep() { 1728 if (getInvestigation().isEmpty()) { 1729 addInvestigation(); 1730 } 1731 return getInvestigation().get(0); 1732 } 1733 1734 /** 1735 * @return {@link #protocol} (Reference to a specific published clinical 1736 * protocol that was followed during this assessment, and/or that 1737 * provides evidence in support of the diagnosis.) 1738 */ 1739 public List<UriType> getProtocol() { 1740 if (this.protocol == null) 1741 this.protocol = new ArrayList<UriType>(); 1742 return this.protocol; 1743 } 1744 1745 /** 1746 * @return Returns a reference to <code>this</code> for easy method chaining 1747 */ 1748 public ClinicalImpression setProtocol(List<UriType> theProtocol) { 1749 this.protocol = theProtocol; 1750 return this; 1751 } 1752 1753 public boolean hasProtocol() { 1754 if (this.protocol == null) 1755 return false; 1756 for (UriType item : this.protocol) 1757 if (!item.isEmpty()) 1758 return true; 1759 return false; 1760 } 1761 1762 /** 1763 * @return {@link #protocol} (Reference to a specific published clinical 1764 * protocol that was followed during this assessment, and/or that 1765 * provides evidence in support of the diagnosis.) 1766 */ 1767 public UriType addProtocolElement() {// 2 1768 UriType t = new UriType(); 1769 if (this.protocol == null) 1770 this.protocol = new ArrayList<UriType>(); 1771 this.protocol.add(t); 1772 return t; 1773 } 1774 1775 /** 1776 * @param value {@link #protocol} (Reference to a specific published clinical 1777 * protocol that was followed during this assessment, and/or that 1778 * provides evidence in support of the diagnosis.) 1779 */ 1780 public ClinicalImpression addProtocol(String value) { // 1 1781 UriType t = new UriType(); 1782 t.setValue(value); 1783 if (this.protocol == null) 1784 this.protocol = new ArrayList<UriType>(); 1785 this.protocol.add(t); 1786 return this; 1787 } 1788 1789 /** 1790 * @param value {@link #protocol} (Reference to a specific published clinical 1791 * protocol that was followed during this assessment, and/or that 1792 * provides evidence in support of the diagnosis.) 1793 */ 1794 public boolean hasProtocol(String value) { 1795 if (this.protocol == null) 1796 return false; 1797 for (UriType v : this.protocol) 1798 if (v.getValue().equals(value)) // uri 1799 return true; 1800 return false; 1801 } 1802 1803 /** 1804 * @return {@link #summary} (A text summary of the investigations and the 1805 * diagnosis.). This is the underlying object with id, value and 1806 * extensions. The accessor "getSummary" gives direct access to the 1807 * value 1808 */ 1809 public StringType getSummaryElement() { 1810 if (this.summary == null) 1811 if (Configuration.errorOnAutoCreate()) 1812 throw new Error("Attempt to auto-create ClinicalImpression.summary"); 1813 else if (Configuration.doAutoCreate()) 1814 this.summary = new StringType(); // bb 1815 return this.summary; 1816 } 1817 1818 public boolean hasSummaryElement() { 1819 return this.summary != null && !this.summary.isEmpty(); 1820 } 1821 1822 public boolean hasSummary() { 1823 return this.summary != null && !this.summary.isEmpty(); 1824 } 1825 1826 /** 1827 * @param value {@link #summary} (A text summary of the investigations and the 1828 * diagnosis.). This is the underlying object with id, value and 1829 * extensions. The accessor "getSummary" gives direct access to the 1830 * value 1831 */ 1832 public ClinicalImpression setSummaryElement(StringType value) { 1833 this.summary = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return A text summary of the investigations and the diagnosis. 1839 */ 1840 public String getSummary() { 1841 return this.summary == null ? null : this.summary.getValue(); 1842 } 1843 1844 /** 1845 * @param value A text summary of the investigations and the diagnosis. 1846 */ 1847 public ClinicalImpression setSummary(String value) { 1848 if (Utilities.noString(value)) 1849 this.summary = null; 1850 else { 1851 if (this.summary == null) 1852 this.summary = new StringType(); 1853 this.summary.setValue(value); 1854 } 1855 return this; 1856 } 1857 1858 /** 1859 * @return {@link #finding} (Specific findings or diagnoses that were considered 1860 * likely or relevant to ongoing treatment.) 1861 */ 1862 public List<ClinicalImpressionFindingComponent> getFinding() { 1863 if (this.finding == null) 1864 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1865 return this.finding; 1866 } 1867 1868 /** 1869 * @return Returns a reference to <code>this</code> for easy method chaining 1870 */ 1871 public ClinicalImpression setFinding(List<ClinicalImpressionFindingComponent> theFinding) { 1872 this.finding = theFinding; 1873 return this; 1874 } 1875 1876 public boolean hasFinding() { 1877 if (this.finding == null) 1878 return false; 1879 for (ClinicalImpressionFindingComponent item : this.finding) 1880 if (!item.isEmpty()) 1881 return true; 1882 return false; 1883 } 1884 1885 public ClinicalImpressionFindingComponent addFinding() { // 3 1886 ClinicalImpressionFindingComponent t = new ClinicalImpressionFindingComponent(); 1887 if (this.finding == null) 1888 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1889 this.finding.add(t); 1890 return t; 1891 } 1892 1893 public ClinicalImpression addFinding(ClinicalImpressionFindingComponent t) { // 3 1894 if (t == null) 1895 return this; 1896 if (this.finding == null) 1897 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1898 this.finding.add(t); 1899 return this; 1900 } 1901 1902 /** 1903 * @return The first repetition of repeating field {@link #finding}, creating it 1904 * if it does not already exist 1905 */ 1906 public ClinicalImpressionFindingComponent getFindingFirstRep() { 1907 if (getFinding().isEmpty()) { 1908 addFinding(); 1909 } 1910 return getFinding().get(0); 1911 } 1912 1913 /** 1914 * @return {@link #prognosisCodeableConcept} (Estimate of likely outcome.) 1915 */ 1916 public List<CodeableConcept> getPrognosisCodeableConcept() { 1917 if (this.prognosisCodeableConcept == null) 1918 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1919 return this.prognosisCodeableConcept; 1920 } 1921 1922 /** 1923 * @return Returns a reference to <code>this</code> for easy method chaining 1924 */ 1925 public ClinicalImpression setPrognosisCodeableConcept(List<CodeableConcept> thePrognosisCodeableConcept) { 1926 this.prognosisCodeableConcept = thePrognosisCodeableConcept; 1927 return this; 1928 } 1929 1930 public boolean hasPrognosisCodeableConcept() { 1931 if (this.prognosisCodeableConcept == null) 1932 return false; 1933 for (CodeableConcept item : this.prognosisCodeableConcept) 1934 if (!item.isEmpty()) 1935 return true; 1936 return false; 1937 } 1938 1939 public CodeableConcept addPrognosisCodeableConcept() { // 3 1940 CodeableConcept t = new CodeableConcept(); 1941 if (this.prognosisCodeableConcept == null) 1942 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1943 this.prognosisCodeableConcept.add(t); 1944 return t; 1945 } 1946 1947 public ClinicalImpression addPrognosisCodeableConcept(CodeableConcept t) { // 3 1948 if (t == null) 1949 return this; 1950 if (this.prognosisCodeableConcept == null) 1951 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1952 this.prognosisCodeableConcept.add(t); 1953 return this; 1954 } 1955 1956 /** 1957 * @return The first repetition of repeating field 1958 * {@link #prognosisCodeableConcept}, creating it if it does not already 1959 * exist 1960 */ 1961 public CodeableConcept getPrognosisCodeableConceptFirstRep() { 1962 if (getPrognosisCodeableConcept().isEmpty()) { 1963 addPrognosisCodeableConcept(); 1964 } 1965 return getPrognosisCodeableConcept().get(0); 1966 } 1967 1968 /** 1969 * @return {@link #prognosisReference} (RiskAssessment expressing likely 1970 * outcome.) 1971 */ 1972 public List<Reference> getPrognosisReference() { 1973 if (this.prognosisReference == null) 1974 this.prognosisReference = new ArrayList<Reference>(); 1975 return this.prognosisReference; 1976 } 1977 1978 /** 1979 * @return Returns a reference to <code>this</code> for easy method chaining 1980 */ 1981 public ClinicalImpression setPrognosisReference(List<Reference> thePrognosisReference) { 1982 this.prognosisReference = thePrognosisReference; 1983 return this; 1984 } 1985 1986 public boolean hasPrognosisReference() { 1987 if (this.prognosisReference == null) 1988 return false; 1989 for (Reference item : this.prognosisReference) 1990 if (!item.isEmpty()) 1991 return true; 1992 return false; 1993 } 1994 1995 public Reference addPrognosisReference() { // 3 1996 Reference t = new Reference(); 1997 if (this.prognosisReference == null) 1998 this.prognosisReference = new ArrayList<Reference>(); 1999 this.prognosisReference.add(t); 2000 return t; 2001 } 2002 2003 public ClinicalImpression addPrognosisReference(Reference t) { // 3 2004 if (t == null) 2005 return this; 2006 if (this.prognosisReference == null) 2007 this.prognosisReference = new ArrayList<Reference>(); 2008 this.prognosisReference.add(t); 2009 return this; 2010 } 2011 2012 /** 2013 * @return The first repetition of repeating field {@link #prognosisReference}, 2014 * creating it if it does not already exist 2015 */ 2016 public Reference getPrognosisReferenceFirstRep() { 2017 if (getPrognosisReference().isEmpty()) { 2018 addPrognosisReference(); 2019 } 2020 return getPrognosisReference().get(0); 2021 } 2022 2023 /** 2024 * @return {@link #supportingInfo} (Information supporting the clinical 2025 * impression.) 2026 */ 2027 public List<Reference> getSupportingInfo() { 2028 if (this.supportingInfo == null) 2029 this.supportingInfo = new ArrayList<Reference>(); 2030 return this.supportingInfo; 2031 } 2032 2033 /** 2034 * @return Returns a reference to <code>this</code> for easy method chaining 2035 */ 2036 public ClinicalImpression setSupportingInfo(List<Reference> theSupportingInfo) { 2037 this.supportingInfo = theSupportingInfo; 2038 return this; 2039 } 2040 2041 public boolean hasSupportingInfo() { 2042 if (this.supportingInfo == null) 2043 return false; 2044 for (Reference item : this.supportingInfo) 2045 if (!item.isEmpty()) 2046 return true; 2047 return false; 2048 } 2049 2050 public Reference addSupportingInfo() { // 3 2051 Reference t = new Reference(); 2052 if (this.supportingInfo == null) 2053 this.supportingInfo = new ArrayList<Reference>(); 2054 this.supportingInfo.add(t); 2055 return t; 2056 } 2057 2058 public ClinicalImpression addSupportingInfo(Reference t) { // 3 2059 if (t == null) 2060 return this; 2061 if (this.supportingInfo == null) 2062 this.supportingInfo = new ArrayList<Reference>(); 2063 this.supportingInfo.add(t); 2064 return this; 2065 } 2066 2067 /** 2068 * @return The first repetition of repeating field {@link #supportingInfo}, 2069 * creating it if it does not already exist 2070 */ 2071 public Reference getSupportingInfoFirstRep() { 2072 if (getSupportingInfo().isEmpty()) { 2073 addSupportingInfo(); 2074 } 2075 return getSupportingInfo().get(0); 2076 } 2077 2078 /** 2079 * @return {@link #note} (Commentary about the impression, typically recorded 2080 * after the impression itself was made, though supplemental notes by 2081 * the original author could also appear.) 2082 */ 2083 public List<Annotation> getNote() { 2084 if (this.note == null) 2085 this.note = new ArrayList<Annotation>(); 2086 return this.note; 2087 } 2088 2089 /** 2090 * @return Returns a reference to <code>this</code> for easy method chaining 2091 */ 2092 public ClinicalImpression setNote(List<Annotation> theNote) { 2093 this.note = theNote; 2094 return this; 2095 } 2096 2097 public boolean hasNote() { 2098 if (this.note == null) 2099 return false; 2100 for (Annotation item : this.note) 2101 if (!item.isEmpty()) 2102 return true; 2103 return false; 2104 } 2105 2106 public Annotation addNote() { // 3 2107 Annotation t = new Annotation(); 2108 if (this.note == null) 2109 this.note = new ArrayList<Annotation>(); 2110 this.note.add(t); 2111 return t; 2112 } 2113 2114 public ClinicalImpression addNote(Annotation t) { // 3 2115 if (t == null) 2116 return this; 2117 if (this.note == null) 2118 this.note = new ArrayList<Annotation>(); 2119 this.note.add(t); 2120 return this; 2121 } 2122 2123 /** 2124 * @return The first repetition of repeating field {@link #note}, creating it if 2125 * it does not already exist 2126 */ 2127 public Annotation getNoteFirstRep() { 2128 if (getNote().isEmpty()) { 2129 addNote(); 2130 } 2131 return getNote().get(0); 2132 } 2133 2134 protected void listChildren(List<Property> children) { 2135 super.listChildren(children); 2136 children.add(new Property("identifier", "Identifier", 2137 "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2138 0, java.lang.Integer.MAX_VALUE, identifier)); 2139 children.add(new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, status)); 2140 children.add(new Property("statusReason", "CodeableConcept", 2141 "Captures the reason for the current state of the ClinicalImpression.", 0, 1, statusReason)); 2142 children.add( 2143 new Property("code", "CodeableConcept", "Categorizes the type of clinical assessment performed.", 0, 1, code)); 2144 children.add(new Property("description", "string", 2145 "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.", 2146 0, 1, description)); 2147 children.add(new Property("subject", "Reference(Patient|Group)", 2148 "The patient or group of individuals assessed as part of this record.", 0, 1, subject)); 2149 children.add(new Property("encounter", "Reference(Encounter)", 2150 "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.", 2151 0, 1, encounter)); 2152 children.add(new Property("effective[x]", "dateTime|Period", 2153 "The point in time or period over which the subject was assessed.", 0, 1, effective)); 2154 children.add(new Property("date", "dateTime", "Indicates when the documentation of the assessment was complete.", 0, 2155 1, date)); 2156 children.add(new Property("assessor", "Reference(Practitioner|PractitionerRole)", 2157 "The clinician performing the assessment.", 0, 1, assessor)); 2158 children.add(new Property("previous", "Reference(ClinicalImpression)", 2159 "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 2160 0, 1, previous)); 2161 children.add(new Property("problem", "Reference(Condition|AllergyIntolerance)", 2162 "A list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem)); 2163 children.add(new Property("investigation", "", 2164 "One or more sets of investigations (signs, symptoms, etc.). The actual grouping of investigations varies greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 2165 0, java.lang.Integer.MAX_VALUE, investigation)); 2166 children.add(new Property("protocol", "uri", 2167 "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 2168 0, java.lang.Integer.MAX_VALUE, protocol)); 2169 children.add( 2170 new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1, summary)); 2171 children.add(new Property("finding", "", 2172 "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.", 0, 2173 java.lang.Integer.MAX_VALUE, finding)); 2174 children.add(new Property("prognosisCodeableConcept", "CodeableConcept", "Estimate of likely outcome.", 0, 2175 java.lang.Integer.MAX_VALUE, prognosisCodeableConcept)); 2176 children.add(new Property("prognosisReference", "Reference(RiskAssessment)", 2177 "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference)); 2178 children.add(new Property("supportingInfo", "Reference(Any)", "Information supporting the clinical impression.", 0, 2179 java.lang.Integer.MAX_VALUE, supportingInfo)); 2180 children.add(new Property("note", "Annotation", 2181 "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 2182 0, java.lang.Integer.MAX_VALUE, note)); 2183 } 2184 2185 @Override 2186 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2187 switch (_hash) { 2188 case -1618432855: 2189 /* identifier */ return new Property("identifier", "Identifier", 2190 "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2191 0, java.lang.Integer.MAX_VALUE, identifier); 2192 case -892481550: 2193 /* status */ return new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, 2194 status); 2195 case 2051346646: 2196 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2197 "Captures the reason for the current state of the ClinicalImpression.", 0, 1, statusReason); 2198 case 3059181: 2199 /* code */ return new Property("code", "CodeableConcept", 2200 "Categorizes the type of clinical assessment performed.", 0, 1, code); 2201 case -1724546052: 2202 /* description */ return new Property("description", "string", 2203 "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.", 2204 0, 1, description); 2205 case -1867885268: 2206 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2207 "The patient or group of individuals assessed as part of this record.", 0, 1, subject); 2208 case 1524132147: 2209 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2210 "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.", 2211 0, 1, encounter); 2212 case 247104889: 2213 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 2214 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2215 case -1468651097: 2216 /* effective */ return new Property("effective[x]", "dateTime|Period", 2217 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2218 case -275306910: 2219 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 2220 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2221 case -403934648: 2222 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 2223 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2224 case 3076014: 2225 /* date */ return new Property("date", "dateTime", 2226 "Indicates when the documentation of the assessment was complete.", 0, 1, date); 2227 case -373213113: 2228 /* assessor */ return new Property("assessor", "Reference(Practitioner|PractitionerRole)", 2229 "The clinician performing the assessment.", 0, 1, assessor); 2230 case -1273775369: 2231 /* previous */ return new Property("previous", "Reference(ClinicalImpression)", 2232 "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 2233 0, 1, previous); 2234 case -309542241: 2235 /* problem */ return new Property("problem", "Reference(Condition|AllergyIntolerance)", 2236 "A list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem); 2237 case 956015362: 2238 /* investigation */ return new Property("investigation", "", 2239 "One or more sets of investigations (signs, symptoms, etc.). The actual grouping of investigations varies greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 2240 0, java.lang.Integer.MAX_VALUE, investigation); 2241 case -989163880: 2242 /* protocol */ return new Property("protocol", "uri", 2243 "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 2244 0, java.lang.Integer.MAX_VALUE, protocol); 2245 case -1857640538: 2246 /* summary */ return new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 2247 0, 1, summary); 2248 case -853173367: 2249 /* finding */ return new Property("finding", "", 2250 "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.", 0, 2251 java.lang.Integer.MAX_VALUE, finding); 2252 case -676337953: 2253 /* prognosisCodeableConcept */ return new Property("prognosisCodeableConcept", "CodeableConcept", 2254 "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisCodeableConcept); 2255 case -587137783: 2256 /* prognosisReference */ return new Property("prognosisReference", "Reference(RiskAssessment)", 2257 "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference); 2258 case 1922406657: 2259 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 2260 "Information supporting the clinical impression.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2261 case 3387378: 2262 /* note */ return new Property("note", "Annotation", 2263 "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 2264 0, java.lang.Integer.MAX_VALUE, note); 2265 default: 2266 return super.getNamedProperty(_hash, _name, _checkValid); 2267 } 2268 2269 } 2270 2271 @Override 2272 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2273 switch (hash) { 2274 case -1618432855: 2275 /* identifier */ return this.identifier == null ? new Base[0] 2276 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2277 case -892481550: 2278 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ClinicalImpressionStatus> 2279 case 2051346646: 2280 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2281 case 3059181: 2282 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2283 case -1724546052: 2284 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2285 case -1867885268: 2286 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2287 case 1524132147: 2288 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2289 case -1468651097: 2290 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 2291 case 3076014: 2292 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2293 case -373213113: 2294 /* assessor */ return this.assessor == null ? new Base[0] : new Base[] { this.assessor }; // Reference 2295 case -1273775369: 2296 /* previous */ return this.previous == null ? new Base[0] : new Base[] { this.previous }; // Reference 2297 case -309542241: 2298 /* problem */ return this.problem == null ? new Base[0] : this.problem.toArray(new Base[this.problem.size()]); // Reference 2299 case 956015362: 2300 /* investigation */ return this.investigation == null ? new Base[0] 2301 : this.investigation.toArray(new Base[this.investigation.size()]); // ClinicalImpressionInvestigationComponent 2302 case -989163880: 2303 /* protocol */ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // UriType 2304 case -1857640538: 2305 /* summary */ return this.summary == null ? new Base[0] : new Base[] { this.summary }; // StringType 2306 case -853173367: 2307 /* finding */ return this.finding == null ? new Base[0] : this.finding.toArray(new Base[this.finding.size()]); // ClinicalImpressionFindingComponent 2308 case -676337953: 2309 /* prognosisCodeableConcept */ return this.prognosisCodeableConcept == null ? new Base[0] 2310 : this.prognosisCodeableConcept.toArray(new Base[this.prognosisCodeableConcept.size()]); // CodeableConcept 2311 case -587137783: 2312 /* prognosisReference */ return this.prognosisReference == null ? new Base[0] 2313 : this.prognosisReference.toArray(new Base[this.prognosisReference.size()]); // Reference 2314 case 1922406657: 2315 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 2316 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2317 case 3387378: 2318 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2319 default: 2320 return super.getProperty(hash, name, checkValid); 2321 } 2322 2323 } 2324 2325 @Override 2326 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2327 switch (hash) { 2328 case -1618432855: // identifier 2329 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2330 return value; 2331 case -892481550: // status 2332 value = new ClinicalImpressionStatusEnumFactory().fromType(castToCode(value)); 2333 this.status = (Enumeration) value; // Enumeration<ClinicalImpressionStatus> 2334 return value; 2335 case 2051346646: // statusReason 2336 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2337 return value; 2338 case 3059181: // code 2339 this.code = castToCodeableConcept(value); // CodeableConcept 2340 return value; 2341 case -1724546052: // description 2342 this.description = castToString(value); // StringType 2343 return value; 2344 case -1867885268: // subject 2345 this.subject = castToReference(value); // Reference 2346 return value; 2347 case 1524132147: // encounter 2348 this.encounter = castToReference(value); // Reference 2349 return value; 2350 case -1468651097: // effective 2351 this.effective = castToType(value); // Type 2352 return value; 2353 case 3076014: // date 2354 this.date = castToDateTime(value); // DateTimeType 2355 return value; 2356 case -373213113: // assessor 2357 this.assessor = castToReference(value); // Reference 2358 return value; 2359 case -1273775369: // previous 2360 this.previous = castToReference(value); // Reference 2361 return value; 2362 case -309542241: // problem 2363 this.getProblem().add(castToReference(value)); // Reference 2364 return value; 2365 case 956015362: // investigation 2366 this.getInvestigation().add((ClinicalImpressionInvestigationComponent) value); // ClinicalImpressionInvestigationComponent 2367 return value; 2368 case -989163880: // protocol 2369 this.getProtocol().add(castToUri(value)); // UriType 2370 return value; 2371 case -1857640538: // summary 2372 this.summary = castToString(value); // StringType 2373 return value; 2374 case -853173367: // finding 2375 this.getFinding().add((ClinicalImpressionFindingComponent) value); // ClinicalImpressionFindingComponent 2376 return value; 2377 case -676337953: // prognosisCodeableConcept 2378 this.getPrognosisCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 2379 return value; 2380 case -587137783: // prognosisReference 2381 this.getPrognosisReference().add(castToReference(value)); // Reference 2382 return value; 2383 case 1922406657: // supportingInfo 2384 this.getSupportingInfo().add(castToReference(value)); // Reference 2385 return value; 2386 case 3387378: // note 2387 this.getNote().add(castToAnnotation(value)); // Annotation 2388 return value; 2389 default: 2390 return super.setProperty(hash, name, value); 2391 } 2392 2393 } 2394 2395 @Override 2396 public Base setProperty(String name, Base value) throws FHIRException { 2397 if (name.equals("identifier")) { 2398 this.getIdentifier().add(castToIdentifier(value)); 2399 } else if (name.equals("status")) { 2400 value = new ClinicalImpressionStatusEnumFactory().fromType(castToCode(value)); 2401 this.status = (Enumeration) value; // Enumeration<ClinicalImpressionStatus> 2402 } else if (name.equals("statusReason")) { 2403 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2404 } else if (name.equals("code")) { 2405 this.code = castToCodeableConcept(value); // CodeableConcept 2406 } else if (name.equals("description")) { 2407 this.description = castToString(value); // StringType 2408 } else if (name.equals("subject")) { 2409 this.subject = castToReference(value); // Reference 2410 } else if (name.equals("encounter")) { 2411 this.encounter = castToReference(value); // Reference 2412 } else if (name.equals("effective[x]")) { 2413 this.effective = castToType(value); // Type 2414 } else if (name.equals("date")) { 2415 this.date = castToDateTime(value); // DateTimeType 2416 } else if (name.equals("assessor")) { 2417 this.assessor = castToReference(value); // Reference 2418 } else if (name.equals("previous")) { 2419 this.previous = castToReference(value); // Reference 2420 } else if (name.equals("problem")) { 2421 this.getProblem().add(castToReference(value)); 2422 } else if (name.equals("investigation")) { 2423 this.getInvestigation().add((ClinicalImpressionInvestigationComponent) value); 2424 } else if (name.equals("protocol")) { 2425 this.getProtocol().add(castToUri(value)); 2426 } else if (name.equals("summary")) { 2427 this.summary = castToString(value); // StringType 2428 } else if (name.equals("finding")) { 2429 this.getFinding().add((ClinicalImpressionFindingComponent) value); 2430 } else if (name.equals("prognosisCodeableConcept")) { 2431 this.getPrognosisCodeableConcept().add(castToCodeableConcept(value)); 2432 } else if (name.equals("prognosisReference")) { 2433 this.getPrognosisReference().add(castToReference(value)); 2434 } else if (name.equals("supportingInfo")) { 2435 this.getSupportingInfo().add(castToReference(value)); 2436 } else if (name.equals("note")) { 2437 this.getNote().add(castToAnnotation(value)); 2438 } else 2439 return super.setProperty(name, value); 2440 return value; 2441 } 2442 2443 @Override 2444 public void removeChild(String name, Base value) throws FHIRException { 2445 if (name.equals("identifier")) { 2446 this.getIdentifier().remove(castToIdentifier(value)); 2447 } else if (name.equals("status")) { 2448 this.status = null; 2449 } else if (name.equals("statusReason")) { 2450 this.statusReason = null; 2451 } else if (name.equals("code")) { 2452 this.code = null; 2453 } else if (name.equals("description")) { 2454 this.description = null; 2455 } else if (name.equals("subject")) { 2456 this.subject = null; 2457 } else if (name.equals("encounter")) { 2458 this.encounter = null; 2459 } else if (name.equals("effective[x]")) { 2460 this.effective = null; 2461 } else if (name.equals("date")) { 2462 this.date = null; 2463 } else if (name.equals("assessor")) { 2464 this.assessor = null; 2465 } else if (name.equals("previous")) { 2466 this.previous = null; 2467 } else if (name.equals("problem")) { 2468 this.getProblem().remove(castToReference(value)); 2469 } else if (name.equals("investigation")) { 2470 this.getInvestigation().remove((ClinicalImpressionInvestigationComponent) value); 2471 } else if (name.equals("protocol")) { 2472 this.getProtocol().remove(castToUri(value)); 2473 } else if (name.equals("summary")) { 2474 this.summary = null; 2475 } else if (name.equals("finding")) { 2476 this.getFinding().remove((ClinicalImpressionFindingComponent) value); 2477 } else if (name.equals("prognosisCodeableConcept")) { 2478 this.getPrognosisCodeableConcept().remove(castToCodeableConcept(value)); 2479 } else if (name.equals("prognosisReference")) { 2480 this.getPrognosisReference().remove(castToReference(value)); 2481 } else if (name.equals("supportingInfo")) { 2482 this.getSupportingInfo().remove(castToReference(value)); 2483 } else if (name.equals("note")) { 2484 this.getNote().remove(castToAnnotation(value)); 2485 } else 2486 super.removeChild(name, value); 2487 2488 } 2489 2490 @Override 2491 public Base makeProperty(int hash, String name) throws FHIRException { 2492 switch (hash) { 2493 case -1618432855: 2494 return addIdentifier(); 2495 case -892481550: 2496 return getStatusElement(); 2497 case 2051346646: 2498 return getStatusReason(); 2499 case 3059181: 2500 return getCode(); 2501 case -1724546052: 2502 return getDescriptionElement(); 2503 case -1867885268: 2504 return getSubject(); 2505 case 1524132147: 2506 return getEncounter(); 2507 case 247104889: 2508 return getEffective(); 2509 case -1468651097: 2510 return getEffective(); 2511 case 3076014: 2512 return getDateElement(); 2513 case -373213113: 2514 return getAssessor(); 2515 case -1273775369: 2516 return getPrevious(); 2517 case -309542241: 2518 return addProblem(); 2519 case 956015362: 2520 return addInvestigation(); 2521 case -989163880: 2522 return addProtocolElement(); 2523 case -1857640538: 2524 return getSummaryElement(); 2525 case -853173367: 2526 return addFinding(); 2527 case -676337953: 2528 return addPrognosisCodeableConcept(); 2529 case -587137783: 2530 return addPrognosisReference(); 2531 case 1922406657: 2532 return addSupportingInfo(); 2533 case 3387378: 2534 return addNote(); 2535 default: 2536 return super.makeProperty(hash, name); 2537 } 2538 2539 } 2540 2541 @Override 2542 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2543 switch (hash) { 2544 case -1618432855: 2545 /* identifier */ return new String[] { "Identifier" }; 2546 case -892481550: 2547 /* status */ return new String[] { "code" }; 2548 case 2051346646: 2549 /* statusReason */ return new String[] { "CodeableConcept" }; 2550 case 3059181: 2551 /* code */ return new String[] { "CodeableConcept" }; 2552 case -1724546052: 2553 /* description */ return new String[] { "string" }; 2554 case -1867885268: 2555 /* subject */ return new String[] { "Reference" }; 2556 case 1524132147: 2557 /* encounter */ return new String[] { "Reference" }; 2558 case -1468651097: 2559 /* effective */ return new String[] { "dateTime", "Period" }; 2560 case 3076014: 2561 /* date */ return new String[] { "dateTime" }; 2562 case -373213113: 2563 /* assessor */ return new String[] { "Reference" }; 2564 case -1273775369: 2565 /* previous */ return new String[] { "Reference" }; 2566 case -309542241: 2567 /* problem */ return new String[] { "Reference" }; 2568 case 956015362: 2569 /* investigation */ return new String[] {}; 2570 case -989163880: 2571 /* protocol */ return new String[] { "uri" }; 2572 case -1857640538: 2573 /* summary */ return new String[] { "string" }; 2574 case -853173367: 2575 /* finding */ return new String[] {}; 2576 case -676337953: 2577 /* prognosisCodeableConcept */ return new String[] { "CodeableConcept" }; 2578 case -587137783: 2579 /* prognosisReference */ return new String[] { "Reference" }; 2580 case 1922406657: 2581 /* supportingInfo */ return new String[] { "Reference" }; 2582 case 3387378: 2583 /* note */ return new String[] { "Annotation" }; 2584 default: 2585 return super.getTypesForProperty(hash, name); 2586 } 2587 2588 } 2589 2590 @Override 2591 public Base addChild(String name) throws FHIRException { 2592 if (name.equals("identifier")) { 2593 return addIdentifier(); 2594 } else if (name.equals("status")) { 2595 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.status"); 2596 } else if (name.equals("statusReason")) { 2597 this.statusReason = new CodeableConcept(); 2598 return this.statusReason; 2599 } else if (name.equals("code")) { 2600 this.code = new CodeableConcept(); 2601 return this.code; 2602 } else if (name.equals("description")) { 2603 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.description"); 2604 } else if (name.equals("subject")) { 2605 this.subject = new Reference(); 2606 return this.subject; 2607 } else if (name.equals("encounter")) { 2608 this.encounter = new Reference(); 2609 return this.encounter; 2610 } else if (name.equals("effectiveDateTime")) { 2611 this.effective = new DateTimeType(); 2612 return this.effective; 2613 } else if (name.equals("effectivePeriod")) { 2614 this.effective = new Period(); 2615 return this.effective; 2616 } else if (name.equals("date")) { 2617 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.date"); 2618 } else if (name.equals("assessor")) { 2619 this.assessor = new Reference(); 2620 return this.assessor; 2621 } else if (name.equals("previous")) { 2622 this.previous = new Reference(); 2623 return this.previous; 2624 } else if (name.equals("problem")) { 2625 return addProblem(); 2626 } else if (name.equals("investigation")) { 2627 return addInvestigation(); 2628 } else if (name.equals("protocol")) { 2629 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.protocol"); 2630 } else if (name.equals("summary")) { 2631 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.summary"); 2632 } else if (name.equals("finding")) { 2633 return addFinding(); 2634 } else if (name.equals("prognosisCodeableConcept")) { 2635 return addPrognosisCodeableConcept(); 2636 } else if (name.equals("prognosisReference")) { 2637 return addPrognosisReference(); 2638 } else if (name.equals("supportingInfo")) { 2639 return addSupportingInfo(); 2640 } else if (name.equals("note")) { 2641 return addNote(); 2642 } else 2643 return super.addChild(name); 2644 } 2645 2646 public String fhirType() { 2647 return "ClinicalImpression"; 2648 2649 } 2650 2651 public ClinicalImpression copy() { 2652 ClinicalImpression dst = new ClinicalImpression(); 2653 copyValues(dst); 2654 return dst; 2655 } 2656 2657 public void copyValues(ClinicalImpression dst) { 2658 super.copyValues(dst); 2659 if (identifier != null) { 2660 dst.identifier = new ArrayList<Identifier>(); 2661 for (Identifier i : identifier) 2662 dst.identifier.add(i.copy()); 2663 } 2664 ; 2665 dst.status = status == null ? null : status.copy(); 2666 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2667 dst.code = code == null ? null : code.copy(); 2668 dst.description = description == null ? null : description.copy(); 2669 dst.subject = subject == null ? null : subject.copy(); 2670 dst.encounter = encounter == null ? null : encounter.copy(); 2671 dst.effective = effective == null ? null : effective.copy(); 2672 dst.date = date == null ? null : date.copy(); 2673 dst.assessor = assessor == null ? null : assessor.copy(); 2674 dst.previous = previous == null ? null : previous.copy(); 2675 if (problem != null) { 2676 dst.problem = new ArrayList<Reference>(); 2677 for (Reference i : problem) 2678 dst.problem.add(i.copy()); 2679 } 2680 ; 2681 if (investigation != null) { 2682 dst.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 2683 for (ClinicalImpressionInvestigationComponent i : investigation) 2684 dst.investigation.add(i.copy()); 2685 } 2686 ; 2687 if (protocol != null) { 2688 dst.protocol = new ArrayList<UriType>(); 2689 for (UriType i : protocol) 2690 dst.protocol.add(i.copy()); 2691 } 2692 ; 2693 dst.summary = summary == null ? null : summary.copy(); 2694 if (finding != null) { 2695 dst.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 2696 for (ClinicalImpressionFindingComponent i : finding) 2697 dst.finding.add(i.copy()); 2698 } 2699 ; 2700 if (prognosisCodeableConcept != null) { 2701 dst.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 2702 for (CodeableConcept i : prognosisCodeableConcept) 2703 dst.prognosisCodeableConcept.add(i.copy()); 2704 } 2705 ; 2706 if (prognosisReference != null) { 2707 dst.prognosisReference = new ArrayList<Reference>(); 2708 for (Reference i : prognosisReference) 2709 dst.prognosisReference.add(i.copy()); 2710 } 2711 ; 2712 if (supportingInfo != null) { 2713 dst.supportingInfo = new ArrayList<Reference>(); 2714 for (Reference i : supportingInfo) 2715 dst.supportingInfo.add(i.copy()); 2716 } 2717 ; 2718 if (note != null) { 2719 dst.note = new ArrayList<Annotation>(); 2720 for (Annotation i : note) 2721 dst.note.add(i.copy()); 2722 } 2723 ; 2724 } 2725 2726 protected ClinicalImpression typedCopy() { 2727 return copy(); 2728 } 2729 2730 @Override 2731 public boolean equalsDeep(Base other_) { 2732 if (!super.equalsDeep(other_)) 2733 return false; 2734 if (!(other_ instanceof ClinicalImpression)) 2735 return false; 2736 ClinicalImpression o = (ClinicalImpression) other_; 2737 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2738 && compareDeep(statusReason, o.statusReason, true) && compareDeep(code, o.code, true) 2739 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) 2740 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) 2741 && compareDeep(date, o.date, true) && compareDeep(assessor, o.assessor, true) 2742 && compareDeep(previous, o.previous, true) && compareDeep(problem, o.problem, true) 2743 && compareDeep(investigation, o.investigation, true) && compareDeep(protocol, o.protocol, true) 2744 && compareDeep(summary, o.summary, true) && compareDeep(finding, o.finding, true) 2745 && compareDeep(prognosisCodeableConcept, o.prognosisCodeableConcept, true) 2746 && compareDeep(prognosisReference, o.prognosisReference, true) 2747 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(note, o.note, true); 2748 } 2749 2750 @Override 2751 public boolean equalsShallow(Base other_) { 2752 if (!super.equalsShallow(other_)) 2753 return false; 2754 if (!(other_ instanceof ClinicalImpression)) 2755 return false; 2756 ClinicalImpression o = (ClinicalImpression) other_; 2757 return compareValues(status, o.status, true) && compareValues(description, o.description, true) 2758 && compareValues(date, o.date, true) && compareValues(protocol, o.protocol, true) 2759 && compareValues(summary, o.summary, true); 2760 } 2761 2762 public boolean isEmpty() { 2763 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusReason, code, description, 2764 subject, encounter, effective, date, assessor, previous, problem, investigation, protocol, summary, finding, 2765 prognosisCodeableConcept, prognosisReference, supportingInfo, note); 2766 } 2767 2768 @Override 2769 public ResourceType getResourceType() { 2770 return ResourceType.ClinicalImpression; 2771 } 2772 2773 /** 2774 * Search parameter: <b>date</b> 2775 * <p> 2776 * Description: <b>When the assessment was documented</b><br> 2777 * Type: <b>date</b><br> 2778 * Path: <b>ClinicalImpression.date</b><br> 2779 * </p> 2780 */ 2781 @SearchParamDefinition(name = "date", path = "ClinicalImpression.date", description = "When the assessment was documented", type = "date") 2782 public static final String SP_DATE = "date"; 2783 /** 2784 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2785 * <p> 2786 * Description: <b>When the assessment was documented</b><br> 2787 * Type: <b>date</b><br> 2788 * Path: <b>ClinicalImpression.date</b><br> 2789 * </p> 2790 */ 2791 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2792 SP_DATE); 2793 2794 /** 2795 * Search parameter: <b>identifier</b> 2796 * <p> 2797 * Description: <b>Business identifier</b><br> 2798 * Type: <b>token</b><br> 2799 * Path: <b>ClinicalImpression.identifier</b><br> 2800 * </p> 2801 */ 2802 @SearchParamDefinition(name = "identifier", path = "ClinicalImpression.identifier", description = "Business identifier", type = "token") 2803 public static final String SP_IDENTIFIER = "identifier"; 2804 /** 2805 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2806 * <p> 2807 * Description: <b>Business identifier</b><br> 2808 * Type: <b>token</b><br> 2809 * Path: <b>ClinicalImpression.identifier</b><br> 2810 * </p> 2811 */ 2812 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2813 SP_IDENTIFIER); 2814 2815 /** 2816 * Search parameter: <b>previous</b> 2817 * <p> 2818 * Description: <b>Reference to last assessment</b><br> 2819 * Type: <b>reference</b><br> 2820 * Path: <b>ClinicalImpression.previous</b><br> 2821 * </p> 2822 */ 2823 @SearchParamDefinition(name = "previous", path = "ClinicalImpression.previous", description = "Reference to last assessment", type = "reference", target = { 2824 ClinicalImpression.class }) 2825 public static final String SP_PREVIOUS = "previous"; 2826 /** 2827 * <b>Fluent Client</b> search parameter constant for <b>previous</b> 2828 * <p> 2829 * Description: <b>Reference to last assessment</b><br> 2830 * Type: <b>reference</b><br> 2831 * Path: <b>ClinicalImpression.previous</b><br> 2832 * </p> 2833 */ 2834 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREVIOUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2835 SP_PREVIOUS); 2836 2837 /** 2838 * Constant for fluent queries to be used to add include statements. Specifies 2839 * the path value of "<b>ClinicalImpression:previous</b>". 2840 */ 2841 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREVIOUS = new ca.uhn.fhir.model.api.Include( 2842 "ClinicalImpression:previous").toLocked(); 2843 2844 /** 2845 * Search parameter: <b>finding-code</b> 2846 * <p> 2847 * Description: <b>What was found</b><br> 2848 * Type: <b>token</b><br> 2849 * Path: <b>ClinicalImpression.finding.itemCodeableConcept</b><br> 2850 * </p> 2851 */ 2852 @SearchParamDefinition(name = "finding-code", path = "ClinicalImpression.finding.itemCodeableConcept", description = "What was found", type = "token") 2853 public static final String SP_FINDING_CODE = "finding-code"; 2854 /** 2855 * <b>Fluent Client</b> search parameter constant for <b>finding-code</b> 2856 * <p> 2857 * Description: <b>What was found</b><br> 2858 * Type: <b>token</b><br> 2859 * Path: <b>ClinicalImpression.finding.itemCodeableConcept</b><br> 2860 * </p> 2861 */ 2862 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FINDING_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2863 SP_FINDING_CODE); 2864 2865 /** 2866 * Search parameter: <b>assessor</b> 2867 * <p> 2868 * Description: <b>The clinician performing the assessment</b><br> 2869 * Type: <b>reference</b><br> 2870 * Path: <b>ClinicalImpression.assessor</b><br> 2871 * </p> 2872 */ 2873 @SearchParamDefinition(name = "assessor", path = "ClinicalImpression.assessor", description = "The clinician performing the assessment", type = "reference", providesMembershipIn = { 2874 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 2875 PractitionerRole.class }) 2876 public static final String SP_ASSESSOR = "assessor"; 2877 /** 2878 * <b>Fluent Client</b> search parameter constant for <b>assessor</b> 2879 * <p> 2880 * Description: <b>The clinician performing the assessment</b><br> 2881 * Type: <b>reference</b><br> 2882 * Path: <b>ClinicalImpression.assessor</b><br> 2883 * </p> 2884 */ 2885 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2886 SP_ASSESSOR); 2887 2888 /** 2889 * Constant for fluent queries to be used to add include statements. Specifies 2890 * the path value of "<b>ClinicalImpression:assessor</b>". 2891 */ 2892 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSESSOR = new ca.uhn.fhir.model.api.Include( 2893 "ClinicalImpression:assessor").toLocked(); 2894 2895 /** 2896 * Search parameter: <b>subject</b> 2897 * <p> 2898 * Description: <b>Patient or group assessed</b><br> 2899 * Type: <b>reference</b><br> 2900 * Path: <b>ClinicalImpression.subject</b><br> 2901 * </p> 2902 */ 2903 @SearchParamDefinition(name = "subject", path = "ClinicalImpression.subject", description = "Patient or group assessed", type = "reference", providesMembershipIn = { 2904 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2905 public static final String SP_SUBJECT = "subject"; 2906 /** 2907 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2908 * <p> 2909 * Description: <b>Patient or group assessed</b><br> 2910 * Type: <b>reference</b><br> 2911 * Path: <b>ClinicalImpression.subject</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2915 SP_SUBJECT); 2916 2917 /** 2918 * Constant for fluent queries to be used to add include statements. Specifies 2919 * the path value of "<b>ClinicalImpression:subject</b>". 2920 */ 2921 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2922 "ClinicalImpression:subject").toLocked(); 2923 2924 /** 2925 * Search parameter: <b>encounter</b> 2926 * <p> 2927 * Description: <b>Encounter created as part of</b><br> 2928 * Type: <b>reference</b><br> 2929 * Path: <b>ClinicalImpression.encounter</b><br> 2930 * </p> 2931 */ 2932 @SearchParamDefinition(name = "encounter", path = "ClinicalImpression.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2933 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2934 public static final String SP_ENCOUNTER = "encounter"; 2935 /** 2936 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2937 * <p> 2938 * Description: <b>Encounter created as part of</b><br> 2939 * Type: <b>reference</b><br> 2940 * Path: <b>ClinicalImpression.encounter</b><br> 2941 * </p> 2942 */ 2943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2944 SP_ENCOUNTER); 2945 2946 /** 2947 * Constant for fluent queries to be used to add include statements. Specifies 2948 * the path value of "<b>ClinicalImpression:encounter</b>". 2949 */ 2950 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2951 "ClinicalImpression:encounter").toLocked(); 2952 2953 /** 2954 * Search parameter: <b>finding-ref</b> 2955 * <p> 2956 * Description: <b>What was found</b><br> 2957 * Type: <b>reference</b><br> 2958 * Path: <b>ClinicalImpression.finding.itemReference</b><br> 2959 * </p> 2960 */ 2961 @SearchParamDefinition(name = "finding-ref", path = "ClinicalImpression.finding.itemReference", description = "What was found", type = "reference", target = { 2962 Condition.class, Media.class, Observation.class }) 2963 public static final String SP_FINDING_REF = "finding-ref"; 2964 /** 2965 * <b>Fluent Client</b> search parameter constant for <b>finding-ref</b> 2966 * <p> 2967 * Description: <b>What was found</b><br> 2968 * Type: <b>reference</b><br> 2969 * Path: <b>ClinicalImpression.finding.itemReference</b><br> 2970 * </p> 2971 */ 2972 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FINDING_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2973 SP_FINDING_REF); 2974 2975 /** 2976 * Constant for fluent queries to be used to add include statements. Specifies 2977 * the path value of "<b>ClinicalImpression:finding-ref</b>". 2978 */ 2979 public static final ca.uhn.fhir.model.api.Include INCLUDE_FINDING_REF = new ca.uhn.fhir.model.api.Include( 2980 "ClinicalImpression:finding-ref").toLocked(); 2981 2982 /** 2983 * Search parameter: <b>problem</b> 2984 * <p> 2985 * Description: <b>Relevant impressions of patient state</b><br> 2986 * Type: <b>reference</b><br> 2987 * Path: <b>ClinicalImpression.problem</b><br> 2988 * </p> 2989 */ 2990 @SearchParamDefinition(name = "problem", path = "ClinicalImpression.problem", description = "Relevant impressions of patient state", type = "reference", target = { 2991 AllergyIntolerance.class, Condition.class }) 2992 public static final String SP_PROBLEM = "problem"; 2993 /** 2994 * <b>Fluent Client</b> search parameter constant for <b>problem</b> 2995 * <p> 2996 * Description: <b>Relevant impressions of patient state</b><br> 2997 * Type: <b>reference</b><br> 2998 * Path: <b>ClinicalImpression.problem</b><br> 2999 * </p> 3000 */ 3001 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROBLEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3002 SP_PROBLEM); 3003 3004 /** 3005 * Constant for fluent queries to be used to add include statements. Specifies 3006 * the path value of "<b>ClinicalImpression:problem</b>". 3007 */ 3008 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROBLEM = new ca.uhn.fhir.model.api.Include( 3009 "ClinicalImpression:problem").toLocked(); 3010 3011 /** 3012 * Search parameter: <b>patient</b> 3013 * <p> 3014 * Description: <b>Patient or group assessed</b><br> 3015 * Type: <b>reference</b><br> 3016 * Path: <b>ClinicalImpression.subject</b><br> 3017 * </p> 3018 */ 3019 @SearchParamDefinition(name = "patient", path = "ClinicalImpression.subject.where(resolve() is Patient)", description = "Patient or group assessed", type = "reference", target = { 3020 Patient.class }) 3021 public static final String SP_PATIENT = "patient"; 3022 /** 3023 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3024 * <p> 3025 * Description: <b>Patient or group assessed</b><br> 3026 * Type: <b>reference</b><br> 3027 * Path: <b>ClinicalImpression.subject</b><br> 3028 * </p> 3029 */ 3030 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3031 SP_PATIENT); 3032 3033 /** 3034 * Constant for fluent queries to be used to add include statements. Specifies 3035 * the path value of "<b>ClinicalImpression:patient</b>". 3036 */ 3037 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3038 "ClinicalImpression:patient").toLocked(); 3039 3040 /** 3041 * Search parameter: <b>supporting-info</b> 3042 * <p> 3043 * Description: <b>Information supporting the clinical impression</b><br> 3044 * Type: <b>reference</b><br> 3045 * Path: <b>ClinicalImpression.supportingInfo</b><br> 3046 * </p> 3047 */ 3048 @SearchParamDefinition(name = "supporting-info", path = "ClinicalImpression.supportingInfo", description = "Information supporting the clinical impression", type = "reference") 3049 public static final String SP_SUPPORTING_INFO = "supporting-info"; 3050 /** 3051 * <b>Fluent Client</b> search parameter constant for <b>supporting-info</b> 3052 * <p> 3053 * Description: <b>Information supporting the clinical impression</b><br> 3054 * Type: <b>reference</b><br> 3055 * Path: <b>ClinicalImpression.supportingInfo</b><br> 3056 * </p> 3057 */ 3058 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTING_INFO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3059 SP_SUPPORTING_INFO); 3060 3061 /** 3062 * Constant for fluent queries to be used to add include statements. Specifies 3063 * the path value of "<b>ClinicalImpression:supporting-info</b>". 3064 */ 3065 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTING_INFO = new ca.uhn.fhir.model.api.Include( 3066 "ClinicalImpression:supporting-info").toLocked(); 3067 3068 /** 3069 * Search parameter: <b>investigation</b> 3070 * <p> 3071 * Description: <b>Record of a specific investigation</b><br> 3072 * Type: <b>reference</b><br> 3073 * Path: <b>ClinicalImpression.investigation.item</b><br> 3074 * </p> 3075 */ 3076 @SearchParamDefinition(name = "investigation", path = "ClinicalImpression.investigation.item", description = "Record of a specific investigation", type = "reference", target = { 3077 DiagnosticReport.class, FamilyMemberHistory.class, ImagingStudy.class, Media.class, Observation.class, 3078 QuestionnaireResponse.class, RiskAssessment.class }) 3079 public static final String SP_INVESTIGATION = "investigation"; 3080 /** 3081 * <b>Fluent Client</b> search parameter constant for <b>investigation</b> 3082 * <p> 3083 * Description: <b>Record of a specific investigation</b><br> 3084 * Type: <b>reference</b><br> 3085 * Path: <b>ClinicalImpression.investigation.item</b><br> 3086 * </p> 3087 */ 3088 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INVESTIGATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3089 SP_INVESTIGATION); 3090 3091 /** 3092 * Constant for fluent queries to be used to add include statements. Specifies 3093 * the path value of "<b>ClinicalImpression:investigation</b>". 3094 */ 3095 public static final ca.uhn.fhir.model.api.Include INCLUDE_INVESTIGATION = new ca.uhn.fhir.model.api.Include( 3096 "ClinicalImpression:investigation").toLocked(); 3097 3098 /** 3099 * Search parameter: <b>status</b> 3100 * <p> 3101 * Description: <b>in-progress | completed | entered-in-error</b><br> 3102 * Type: <b>token</b><br> 3103 * Path: <b>ClinicalImpression.status</b><br> 3104 * </p> 3105 */ 3106 @SearchParamDefinition(name = "status", path = "ClinicalImpression.status", description = "in-progress | completed | entered-in-error", type = "token") 3107 public static final String SP_STATUS = "status"; 3108 /** 3109 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3110 * <p> 3111 * Description: <b>in-progress | completed | entered-in-error</b><br> 3112 * Type: <b>token</b><br> 3113 * Path: <b>ClinicalImpression.status</b><br> 3114 * </p> 3115 */ 3116 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3117 SP_STATUS); 3118 3119}